Undoubled String
VerifiedAdded on 2019/09/20
|7
|1486
|129
Report
AI Summary
The assignment is about creating a program in Java that undoubles a string input by the user and checks if the input is doubled or not. The program uses two methods: `getUndoubledString()` to remove the doubling of characters and `checkForDoubledInput()` to verify if the input is doubled. The main method repeatedly asks for input, undoubles it, and prints it until the user decides to stop.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
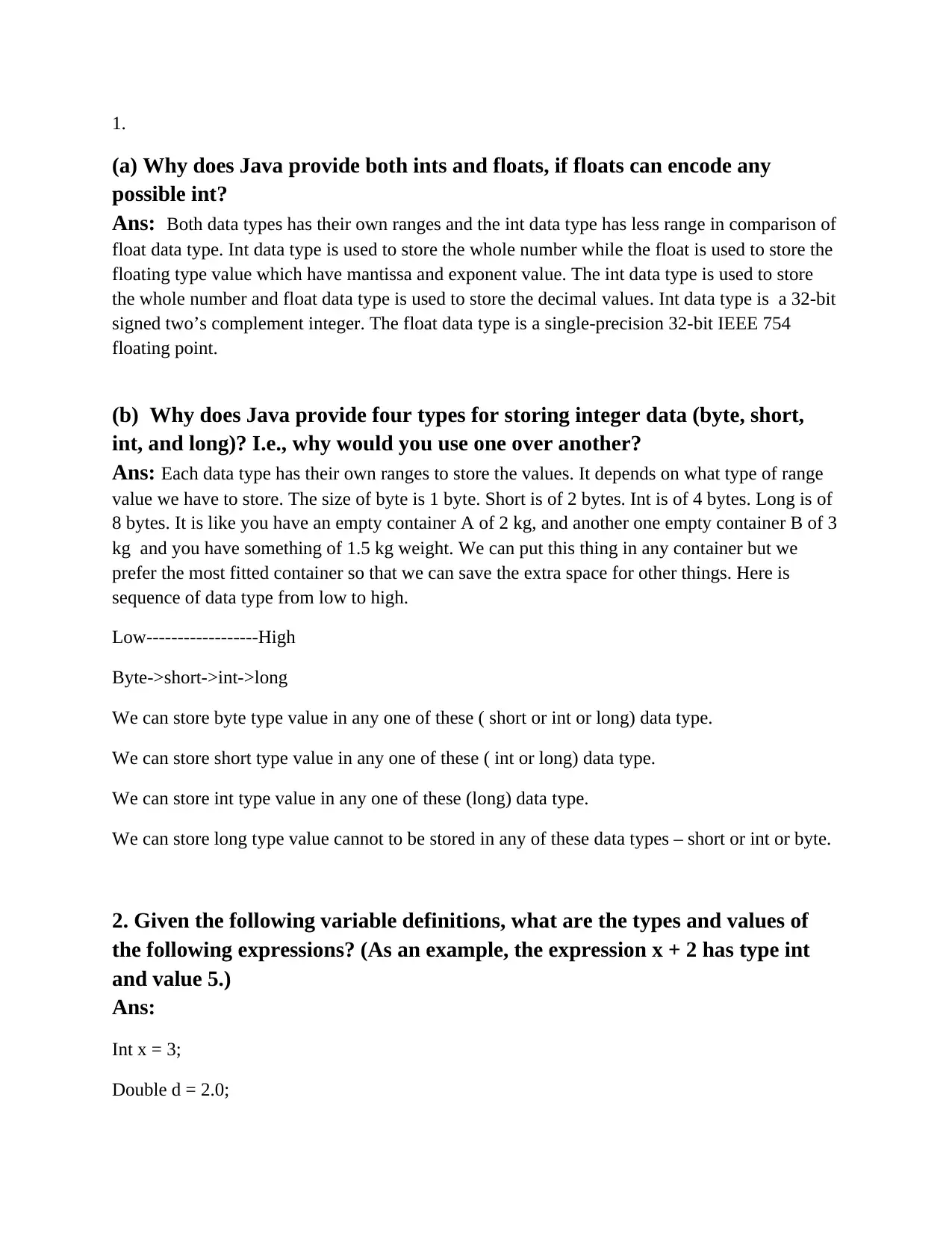
1.
(a) Why does Java provide both ints and floats, if floats can encode any
possible int?
Ans: Both data types has their own ranges and the int data type has less range in comparison of
float data type. Int data type is used to store the whole number while the float is used to store the
floating type value which have mantissa and exponent value. The int data type is used to store
the whole number and float data type is used to store the decimal values. Int data type is a 32-bit
signed two’s complement integer. The float data type is a single-precision 32-bit IEEE 754
floating point.
(b) Why does Java provide four types for storing integer data (byte, short,
int, and long)? I.e., why would you use one over another?
Ans: Each data type has their own ranges to store the values. It depends on what type of range
value we have to store. The size of byte is 1 byte. Short is of 2 bytes. Int is of 4 bytes. Long is of
8 bytes. It is like you have an empty container A of 2 kg, and another one empty container B of 3
kg and you have something of 1.5 kg weight. We can put this thing in any container but we
prefer the most fitted container so that we can save the extra space for other things. Here is
sequence of data type from low to high.
Low------------------High
Byte->short->int->long
We can store byte type value in any one of these ( short or int or long) data type.
We can store short type value in any one of these ( int or long) data type.
We can store int type value in any one of these (long) data type.
We can store long type value cannot to be stored in any of these data types – short or int or byte.
2. Given the following variable definitions, what are the types and values of
the following expressions? (As an example, the expression x + 2 has type int
and value 5.)
Ans:
Int x = 3;
Double d = 2.0;
(a) Why does Java provide both ints and floats, if floats can encode any
possible int?
Ans: Both data types has their own ranges and the int data type has less range in comparison of
float data type. Int data type is used to store the whole number while the float is used to store the
floating type value which have mantissa and exponent value. The int data type is used to store
the whole number and float data type is used to store the decimal values. Int data type is a 32-bit
signed two’s complement integer. The float data type is a single-precision 32-bit IEEE 754
floating point.
(b) Why does Java provide four types for storing integer data (byte, short,
int, and long)? I.e., why would you use one over another?
Ans: Each data type has their own ranges to store the values. It depends on what type of range
value we have to store. The size of byte is 1 byte. Short is of 2 bytes. Int is of 4 bytes. Long is of
8 bytes. It is like you have an empty container A of 2 kg, and another one empty container B of 3
kg and you have something of 1.5 kg weight. We can put this thing in any container but we
prefer the most fitted container so that we can save the extra space for other things. Here is
sequence of data type from low to high.
Low------------------High
Byte->short->int->long
We can store byte type value in any one of these ( short or int or long) data type.
We can store short type value in any one of these ( int or long) data type.
We can store int type value in any one of these (long) data type.
We can store long type value cannot to be stored in any of these data types – short or int or byte.
2. Given the following variable definitions, what are the types and values of
the following expressions? (As an example, the expression x + 2 has type int
and value 5.)
Ans:
Int x = 3;
Double d = 2.0;
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
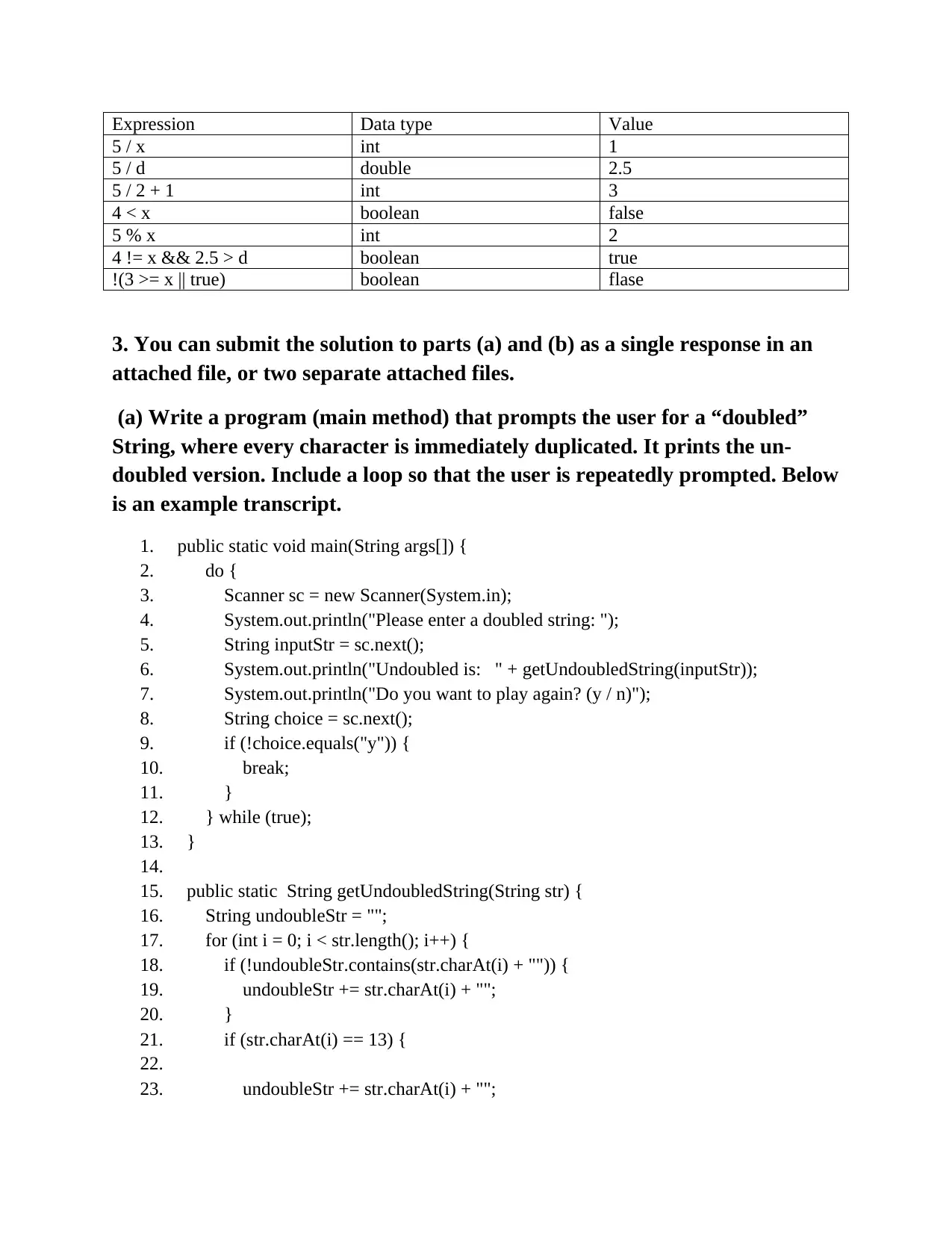
Expression Data type Value
5 / x int 1
5 / d double 2.5
5 / 2 + 1 int 3
4 < x boolean false
5 % x int 2
4 != x && 2.5 > d boolean true
!(3 >= x || true) boolean flase
3. You can submit the solution to parts (a) and (b) as a single response in an
attached file, or two separate attached files.
(a) Write a program (main method) that prompts the user for a “doubled”
String, where every character is immediately duplicated. It prints the un-
doubled version. Include a loop so that the user is repeatedly prompted. Below
is an example transcript.
1. public static void main(String args[]) {
2. do {
3. Scanner sc = new Scanner(System.in);
4. System.out.println("Please enter a doubled string: ");
5. String inputStr = sc.next();
6. System.out.println("Undoubled is: " + getUndoubledString(inputStr));
7. System.out.println("Do you want to play again? (y / n)");
8. String choice = sc.next();
9. if (!choice.equals("y")) {
10. break;
11. }
12. } while (true);
13. }
14.
15. public static String getUndoubledString(String str) {
16. String undoubleStr = "";
17. for (int i = 0; i < str.length(); i++) {
18. if (!undoubleStr.contains(str.charAt(i) + "")) {
19. undoubleStr += str.charAt(i) + "";
20. }
21. if (str.charAt(i) == 13) {
22.
23. undoubleStr += str.charAt(i) + "";
5 / x int 1
5 / d double 2.5
5 / 2 + 1 int 3
4 < x boolean false
5 % x int 2
4 != x && 2.5 > d boolean true
!(3 >= x || true) boolean flase
3. You can submit the solution to parts (a) and (b) as a single response in an
attached file, or two separate attached files.
(a) Write a program (main method) that prompts the user for a “doubled”
String, where every character is immediately duplicated. It prints the un-
doubled version. Include a loop so that the user is repeatedly prompted. Below
is an example transcript.
1. public static void main(String args[]) {
2. do {
3. Scanner sc = new Scanner(System.in);
4. System.out.println("Please enter a doubled string: ");
5. String inputStr = sc.next();
6. System.out.println("Undoubled is: " + getUndoubledString(inputStr));
7. System.out.println("Do you want to play again? (y / n)");
8. String choice = sc.next();
9. if (!choice.equals("y")) {
10. break;
11. }
12. } while (true);
13. }
14.
15. public static String getUndoubledString(String str) {
16. String undoubleStr = "";
17. for (int i = 0; i < str.length(); i++) {
18. if (!undoubleStr.contains(str.charAt(i) + "")) {
19. undoubleStr += str.charAt(i) + "";
20. }
21. if (str.charAt(i) == 13) {
22.
23. undoubleStr += str.charAt(i) + "";
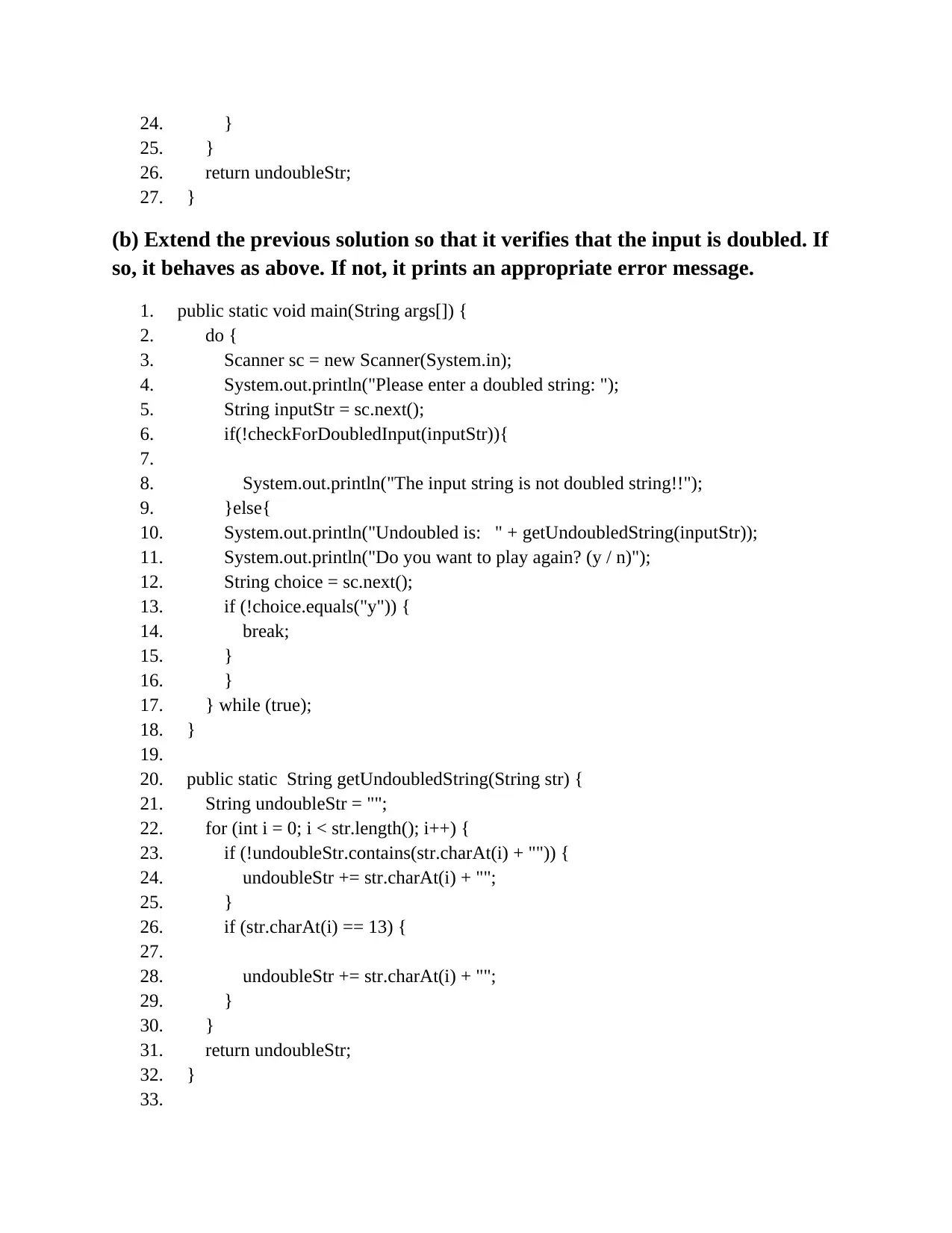
24. }
25. }
26. return undoubleStr;
27. }
(b) Extend the previous solution so that it verifies that the input is doubled. If
so, it behaves as above. If not, it prints an appropriate error message.
1. public static void main(String args[]) {
2. do {
3. Scanner sc = new Scanner(System.in);
4. System.out.println("Please enter a doubled string: ");
5. String inputStr = sc.next();
6. if(!checkForDoubledInput(inputStr)){
7.
8. System.out.println("The input string is not doubled string!!");
9. }else{
10. System.out.println("Undoubled is: " + getUndoubledString(inputStr));
11. System.out.println("Do you want to play again? (y / n)");
12. String choice = sc.next();
13. if (!choice.equals("y")) {
14. break;
15. }
16. }
17. } while (true);
18. }
19.
20. public static String getUndoubledString(String str) {
21. String undoubleStr = "";
22. for (int i = 0; i < str.length(); i++) {
23. if (!undoubleStr.contains(str.charAt(i) + "")) {
24. undoubleStr += str.charAt(i) + "";
25. }
26. if (str.charAt(i) == 13) {
27.
28. undoubleStr += str.charAt(i) + "";
29. }
30. }
31. return undoubleStr;
32. }
33.
25. }
26. return undoubleStr;
27. }
(b) Extend the previous solution so that it verifies that the input is doubled. If
so, it behaves as above. If not, it prints an appropriate error message.
1. public static void main(String args[]) {
2. do {
3. Scanner sc = new Scanner(System.in);
4. System.out.println("Please enter a doubled string: ");
5. String inputStr = sc.next();
6. if(!checkForDoubledInput(inputStr)){
7.
8. System.out.println("The input string is not doubled string!!");
9. }else{
10. System.out.println("Undoubled is: " + getUndoubledString(inputStr));
11. System.out.println("Do you want to play again? (y / n)");
12. String choice = sc.next();
13. if (!choice.equals("y")) {
14. break;
15. }
16. }
17. } while (true);
18. }
19.
20. public static String getUndoubledString(String str) {
21. String undoubleStr = "";
22. for (int i = 0; i < str.length(); i++) {
23. if (!undoubleStr.contains(str.charAt(i) + "")) {
24. undoubleStr += str.charAt(i) + "";
25. }
26. if (str.charAt(i) == 13) {
27.
28. undoubleStr += str.charAt(i) + "";
29. }
30. }
31. return undoubleStr;
32. }
33.
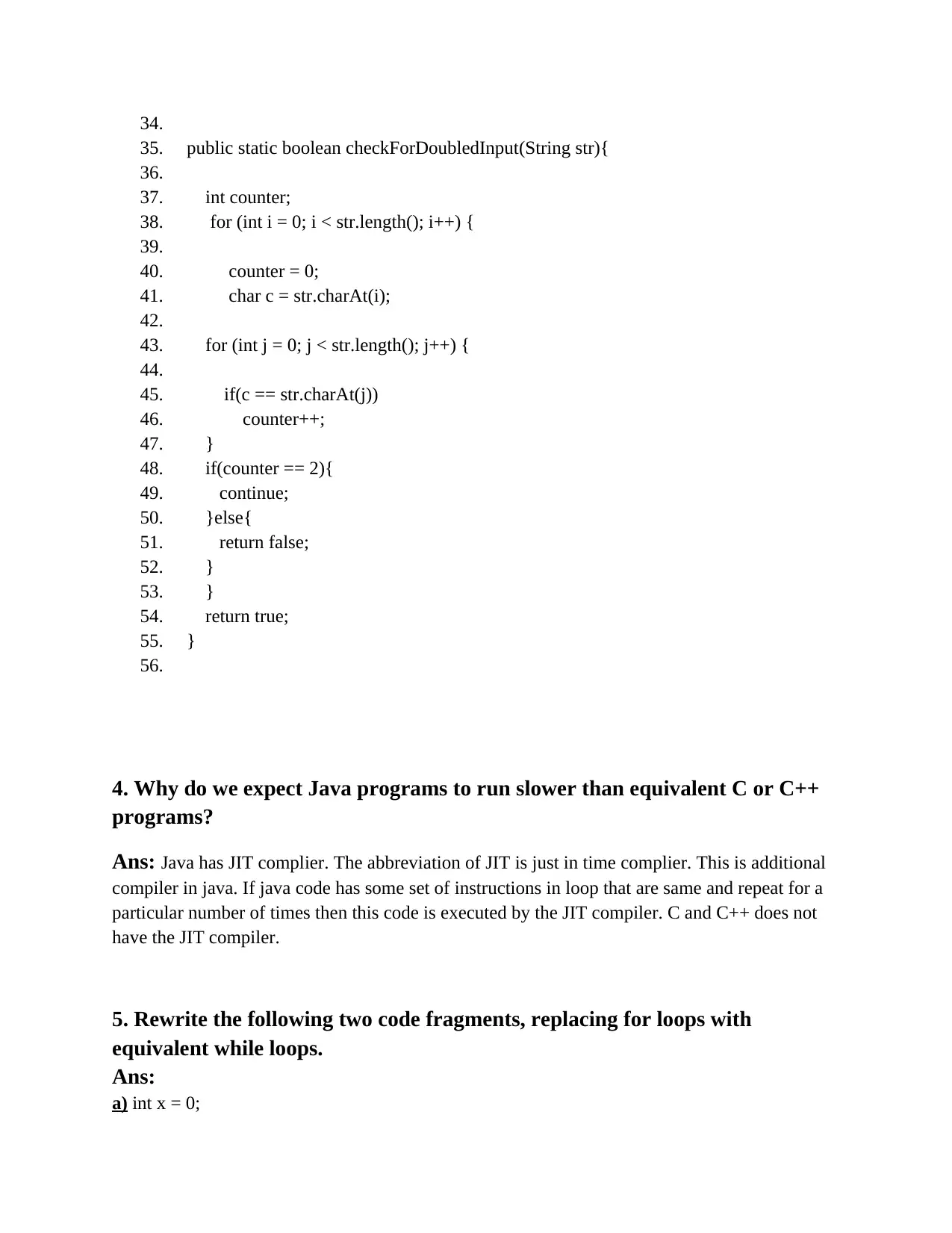
34.
35. public static boolean checkForDoubledInput(String str){
36.
37. int counter;
38. for (int i = 0; i < str.length(); i++) {
39.
40. counter = 0;
41. char c = str.charAt(i);
42.
43. for (int j = 0; j < str.length(); j++) {
44.
45. if(c == str.charAt(j))
46. counter++;
47. }
48. if(counter == 2){
49. continue;
50. }else{
51. return false;
52. }
53. }
54. return true;
55. }
56.
4. Why do we expect Java programs to run slower than equivalent C or C++
programs?
Ans: Java has JIT complier. The abbreviation of JIT is just in time complier. This is additional
compiler in java. If java code has some set of instructions in loop that are same and repeat for a
particular number of times then this code is executed by the JIT compiler. C and C++ does not
have the JIT compiler.
5. Rewrite the following two code fragments, replacing for loops with
equivalent while loops.
Ans:
a) int x = 0;
35. public static boolean checkForDoubledInput(String str){
36.
37. int counter;
38. for (int i = 0; i < str.length(); i++) {
39.
40. counter = 0;
41. char c = str.charAt(i);
42.
43. for (int j = 0; j < str.length(); j++) {
44.
45. if(c == str.charAt(j))
46. counter++;
47. }
48. if(counter == 2){
49. continue;
50. }else{
51. return false;
52. }
53. }
54. return true;
55. }
56.
4. Why do we expect Java programs to run slower than equivalent C or C++
programs?
Ans: Java has JIT complier. The abbreviation of JIT is just in time complier. This is additional
compiler in java. If java code has some set of instructions in loop that are same and repeat for a
particular number of times then this code is executed by the JIT compiler. C and C++ does not
have the JIT compiler.
5. Rewrite the following two code fragments, replacing for loops with
equivalent while loops.
Ans:
a) int x = 0;
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
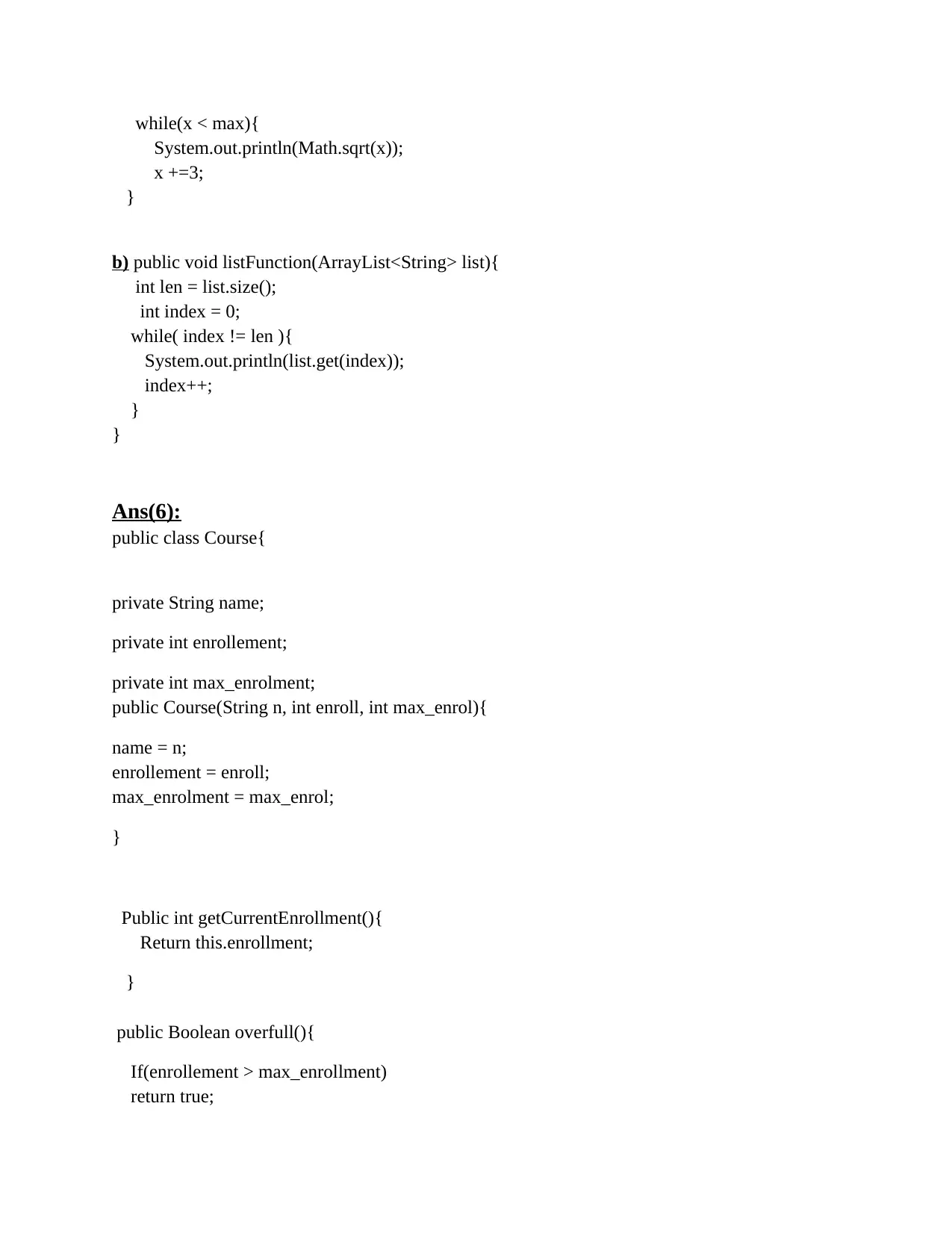
while(x < max){
System.out.println(Math.sqrt(x));
x +=3;
}
b) public void listFunction(ArrayList<String> list){
int len = list.size();
int index = 0;
while( index != len ){
System.out.println(list.get(index));
index++;
}
}
Ans(6):
public class Course{
private String name;
private int enrollement;
private int max_enrolment;
public Course(String n, int enroll, int max_enrol){
name = n;
enrollement = enroll;
max_enrolment = max_enrol;
}
Public int getCurrentEnrollment(){
Return this.enrollment;
}
public Boolean overfull(){
If(enrollement > max_enrollment)
return true;
System.out.println(Math.sqrt(x));
x +=3;
}
b) public void listFunction(ArrayList<String> list){
int len = list.size();
int index = 0;
while( index != len ){
System.out.println(list.get(index));
index++;
}
}
Ans(6):
public class Course{
private String name;
private int enrollement;
private int max_enrolment;
public Course(String n, int enroll, int max_enrol){
name = n;
enrollement = enroll;
max_enrolment = max_enrol;
}
Public int getCurrentEnrollment(){
Return this.enrollment;
}
public Boolean overfull(){
If(enrollement > max_enrollment)
return true;
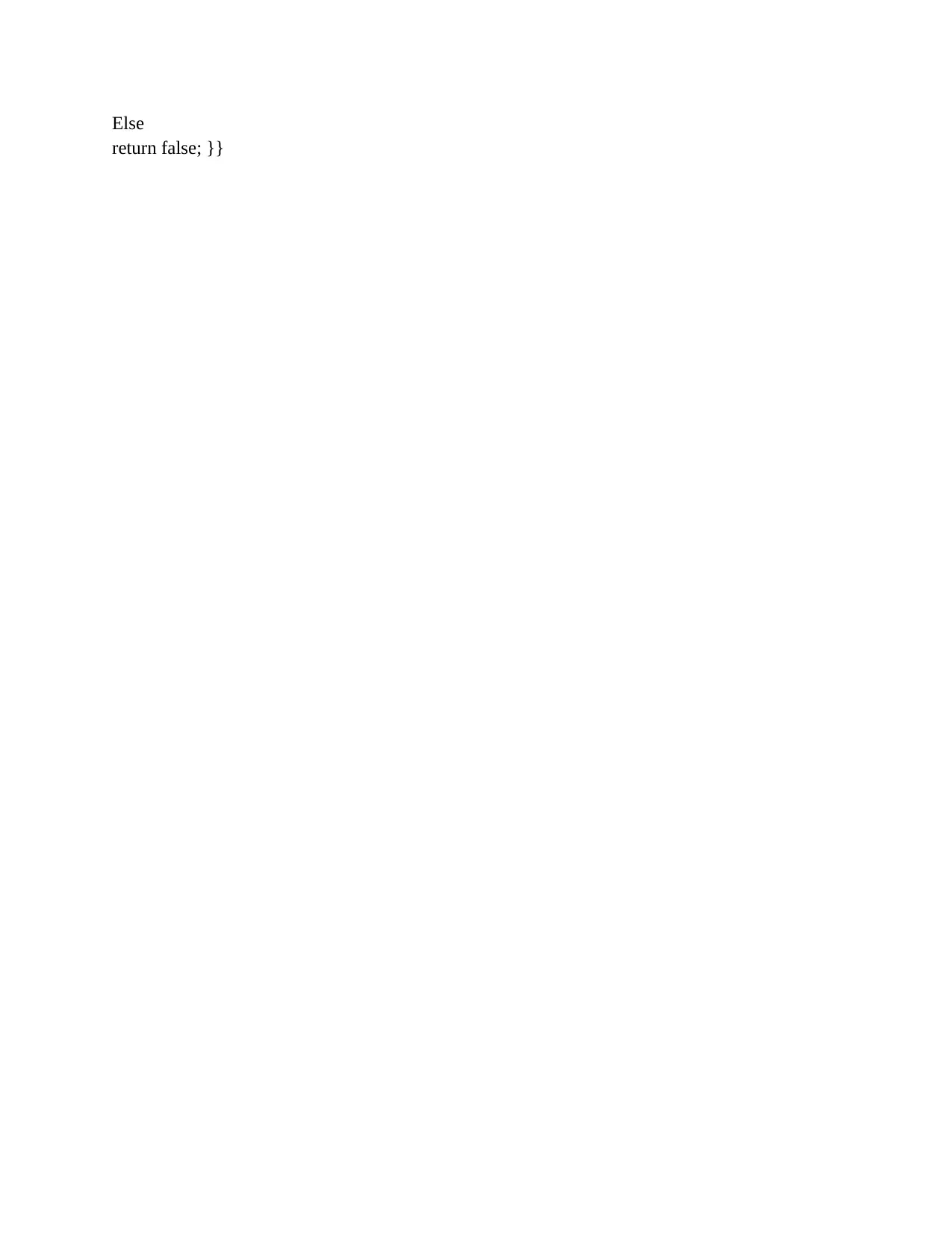
Else
return false; }}
return false; }}
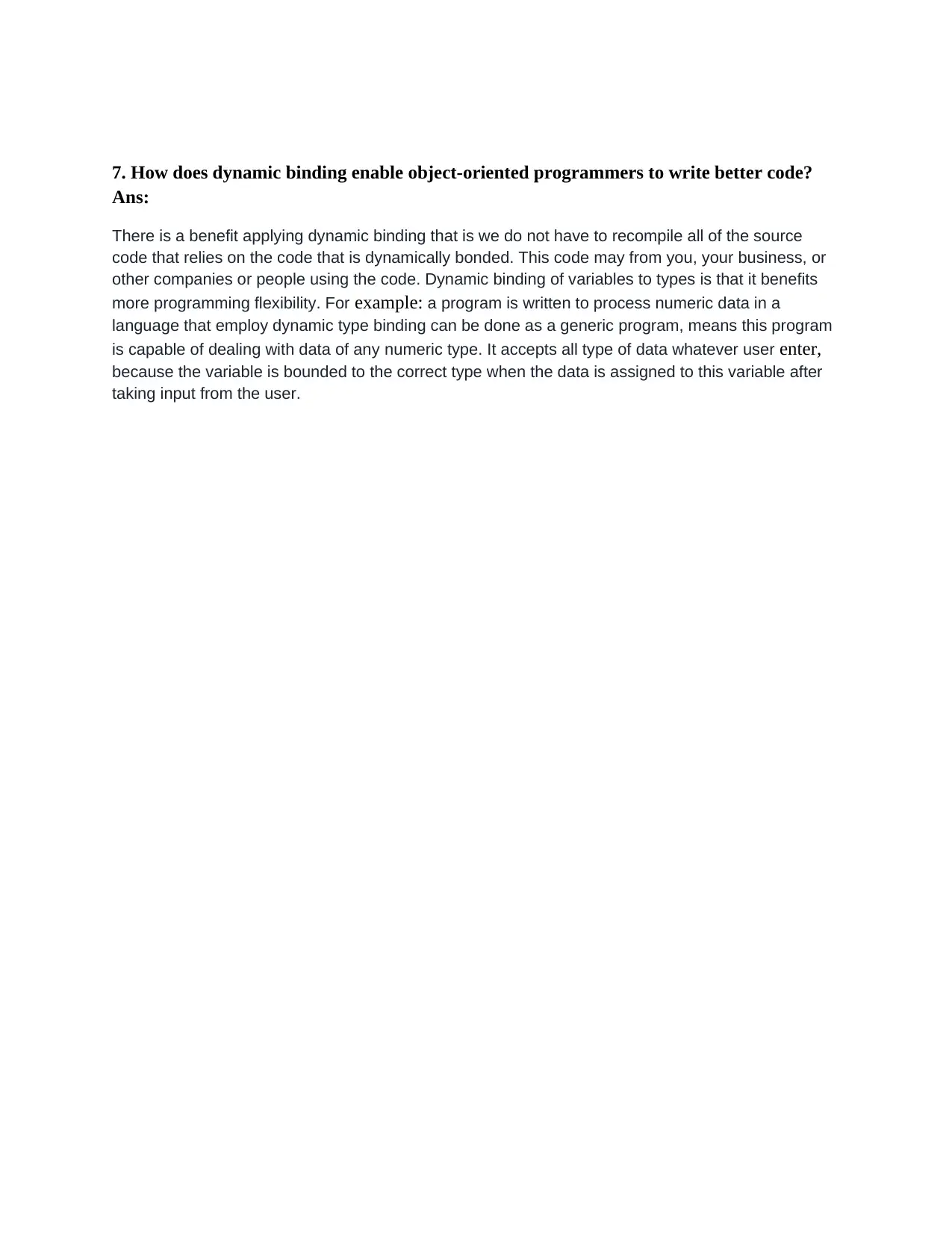
7. How does dynamic binding enable object-oriented programmers to write better code?
Ans:
There is a benefit applying dynamic binding that is we do not have to recompile all of the source
code that relies on the code that is dynamically bonded. This code may from you, your business, or
other companies or people using the code. Dynamic binding of variables to types is that it benefits
more programming flexibility. For example: a program is written to process numeric data in a
language that employ dynamic type binding can be done as a generic program, means this program
is capable of dealing with data of any numeric type. It accepts all type of data whatever user enter,
because the variable is bounded to the correct type when the data is assigned to this variable after
taking input from the user.
Ans:
There is a benefit applying dynamic binding that is we do not have to recompile all of the source
code that relies on the code that is dynamically bonded. This code may from you, your business, or
other companies or people using the code. Dynamic binding of variables to types is that it benefits
more programming flexibility. For example: a program is written to process numeric data in a
language that employ dynamic type binding can be done as a generic program, means this program
is capable of dealing with data of any numeric type. It accepts all type of data whatever user enter,
because the variable is bounded to the correct type when the data is assigned to this variable after
taking input from the user.
1 out of 7
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.