Program Evaluation Framework
VerifiedAdded on  2020/06/04
|29
|7403
|83
AI Summary
This assignment delves into a comprehensive program evaluation framework. It outlines the steps involved in planning, implementing, and assessing the outcomes of a program. The framework includes tools and strategies for identifying objectives, determining program components, measuring process effectiveness, and evaluating overall program success. Key elements covered include capacity assessment, program planning, process evaluation, and outcome measurement using various design models.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
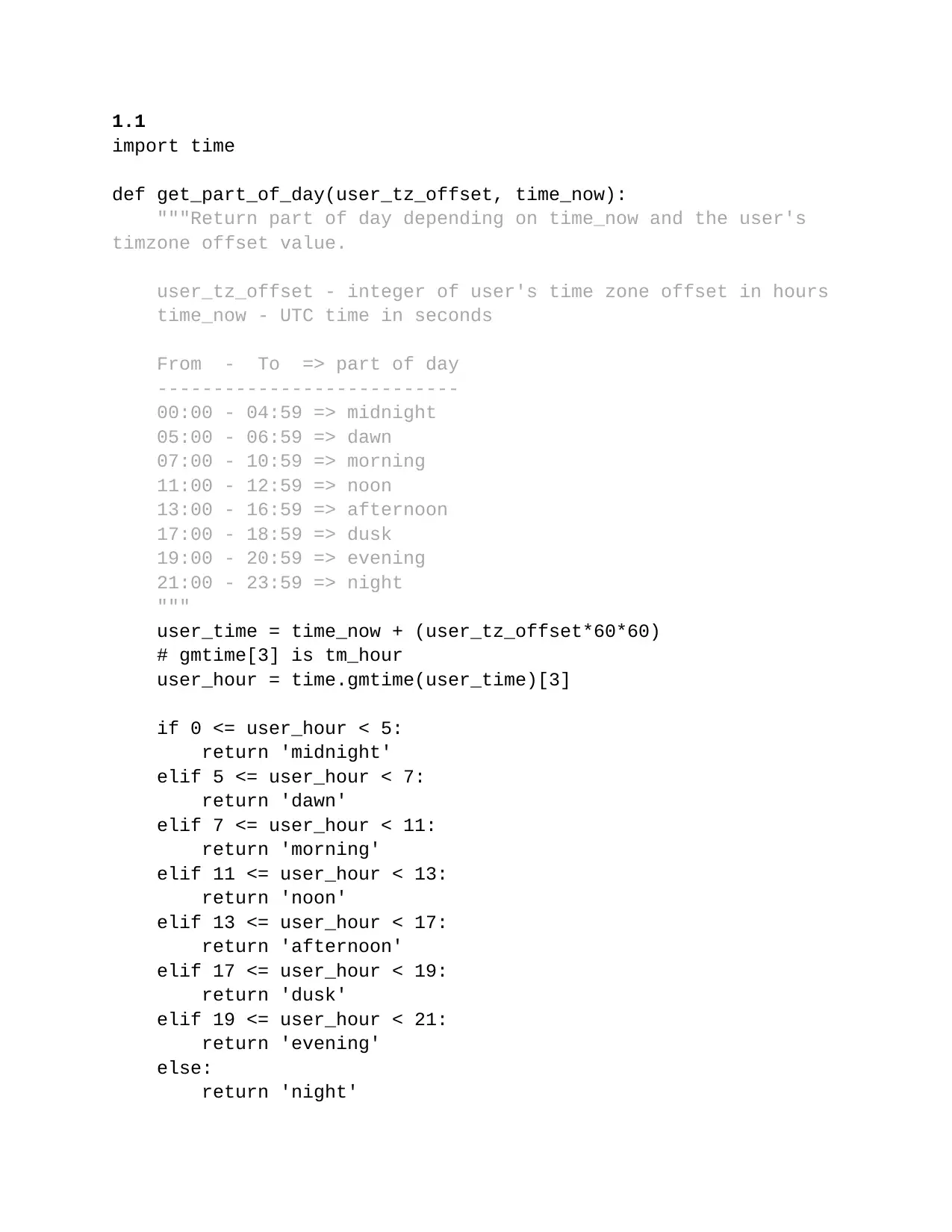
1.1
import time
def get_part_of_day(user_tz_offset, time_now):
"""Return part of day depending on time_now and the user's
timzone offset value.
user_tz_offset - integer of user's time zone offset in hours
time_now - UTC time in seconds
From - To => part of day
---------------------------
00:00 - 04:59 => midnight
05:00 - 06:59 => dawn
07:00 - 10:59 => morning
11:00 - 12:59 => noon
13:00 - 16:59 => afternoon
17:00 - 18:59 => dusk
19:00 - 20:59 => evening
21:00 - 23:59 => night
"""
user_time = time_now + (user_tz_offset*60*60)
# gmtime[3] is tm_hour
user_hour = time.gmtime(user_time)[3]
if 0 <= user_hour < 5:
return 'midnight'
elif 5 <= user_hour < 7:
return 'dawn'
elif 7 <= user_hour < 11:
return 'morning'
elif 11 <= user_hour < 13:
return 'noon'
elif 13 <= user_hour < 17:
return 'afternoon'
elif 17 <= user_hour < 19:
return 'dusk'
elif 19 <= user_hour < 21:
return 'evening'
else:
return 'night'
import time
def get_part_of_day(user_tz_offset, time_now):
"""Return part of day depending on time_now and the user's
timzone offset value.
user_tz_offset - integer of user's time zone offset in hours
time_now - UTC time in seconds
From - To => part of day
---------------------------
00:00 - 04:59 => midnight
05:00 - 06:59 => dawn
07:00 - 10:59 => morning
11:00 - 12:59 => noon
13:00 - 16:59 => afternoon
17:00 - 18:59 => dusk
19:00 - 20:59 => evening
21:00 - 23:59 => night
"""
user_time = time_now + (user_tz_offset*60*60)
# gmtime[3] is tm_hour
user_hour = time.gmtime(user_time)[3]
if 0 <= user_hour < 5:
return 'midnight'
elif 5 <= user_hour < 7:
return 'dawn'
elif 7 <= user_hour < 11:
return 'morning'
elif 11 <= user_hour < 13:
return 'noon'
elif 13 <= user_hour < 17:
return 'afternoon'
elif 17 <= user_hour < 19:
return 'dusk'
elif 19 <= user_hour < 21:
return 'evening'
else:
return 'night'
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
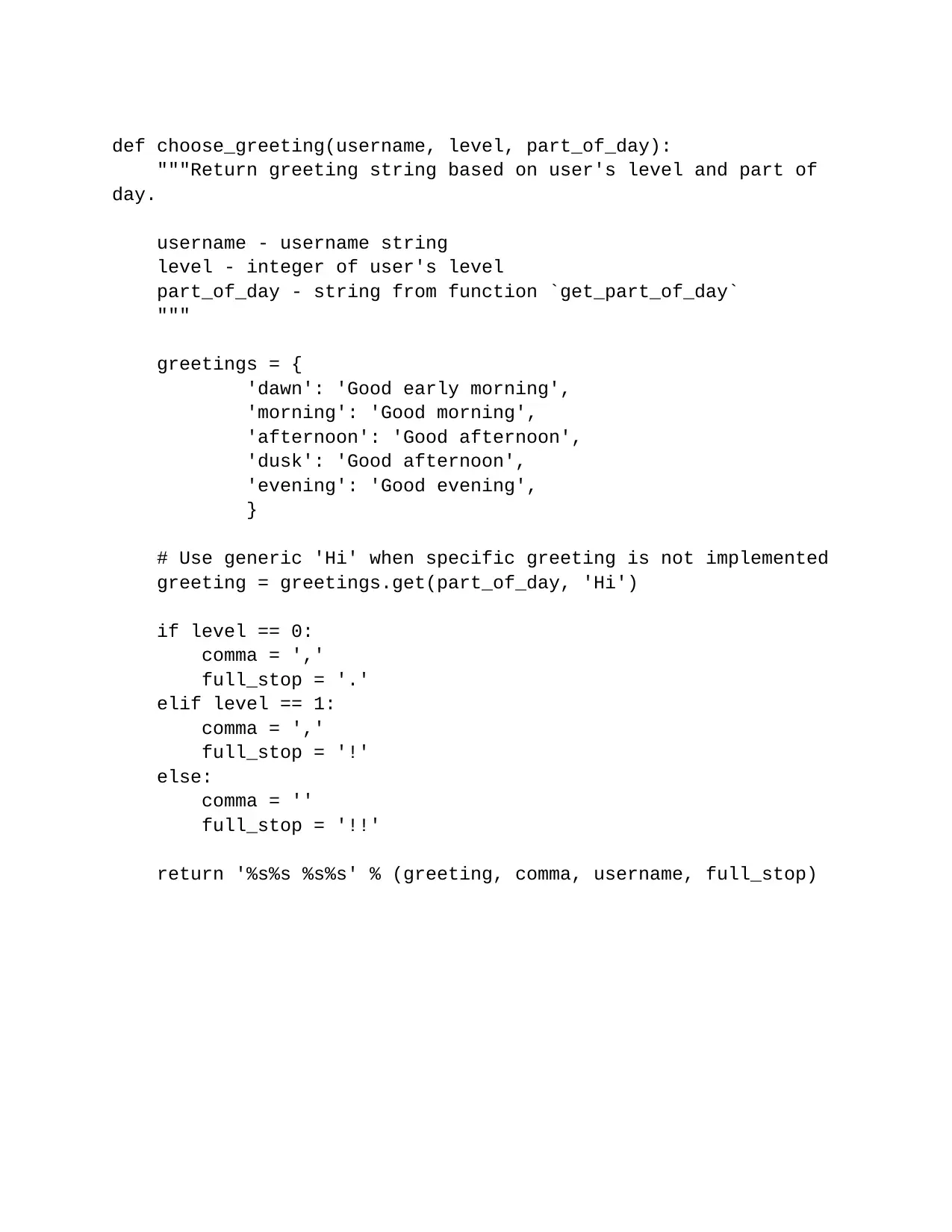
def choose_greeting(username, level, part_of_day):
"""Return greeting string based on user's level and part of
day.
username - username string
level - integer of user's level
part_of_day - string from function `get_part_of_day`
"""
greetings = {
'dawn': 'Good early morning',
'morning': 'Good morning',
'afternoon': 'Good afternoon',
'dusk': 'Good afternoon',
'evening': 'Good evening',
}
# Use generic 'Hi' when specific greeting is not implemented
greeting = greetings.get(part_of_day, 'Hi')
if level == 0:
comma = ','
full_stop = '.'
elif level == 1:
comma = ','
full_stop = '!'
else:
comma = ''
full_stop = '!!'
return '%s%s %s%s' % (greeting, comma, username, full_stop)
"""Return greeting string based on user's level and part of
day.
username - username string
level - integer of user's level
part_of_day - string from function `get_part_of_day`
"""
greetings = {
'dawn': 'Good early morning',
'morning': 'Good morning',
'afternoon': 'Good afternoon',
'dusk': 'Good afternoon',
'evening': 'Good evening',
}
# Use generic 'Hi' when specific greeting is not implemented
greeting = greetings.get(part_of_day, 'Hi')
if level == 0:
comma = ','
full_stop = '.'
elif level == 1:
comma = ','
full_stop = '!'
else:
comma = ''
full_stop = '!!'
return '%s%s %s%s' % (greeting, comma, username, full_stop)
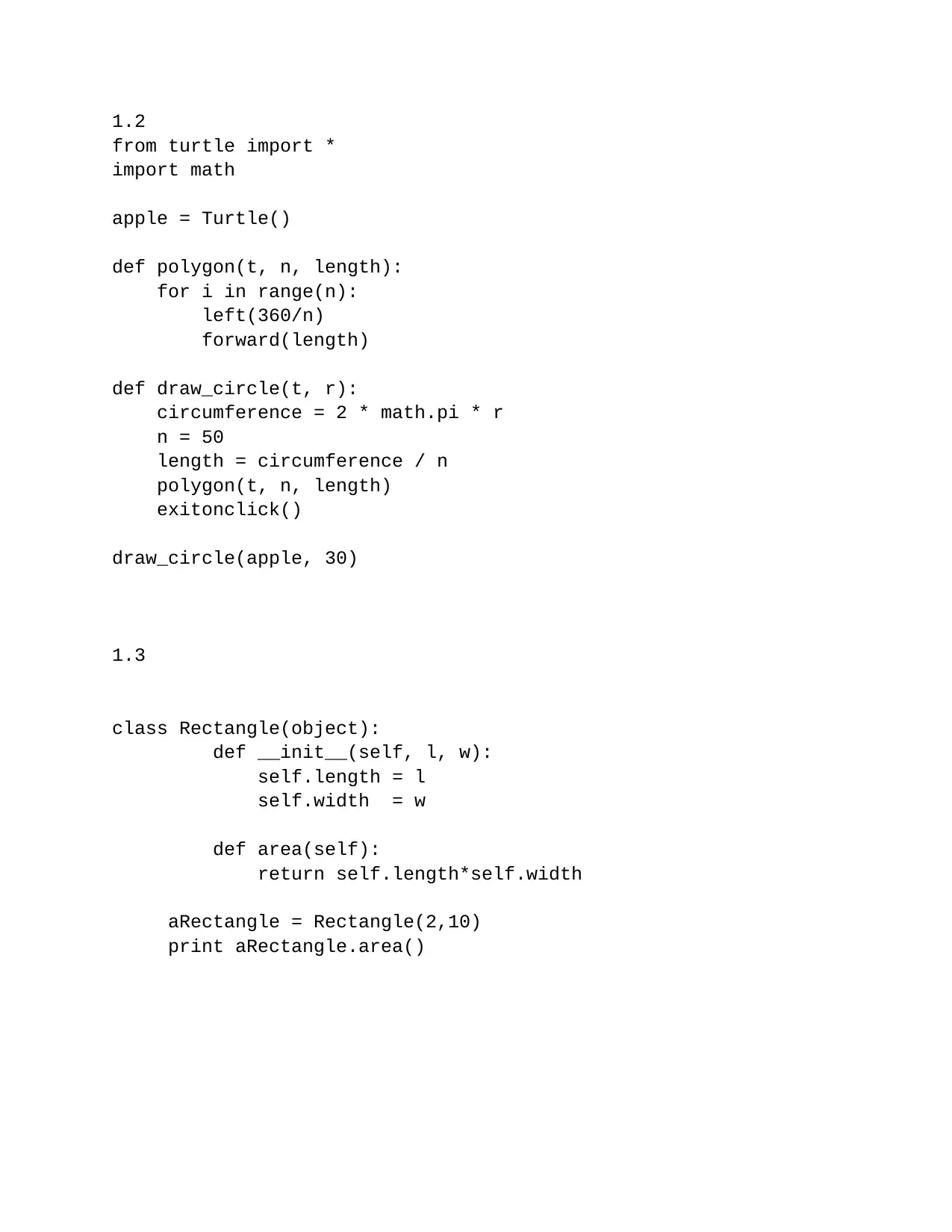
1.2
from turtle import *
import math
apple = Turtle()
def polygon(t, n, length):
for i in range(n):
left(360/n)
forward(length)
def draw_circle(t, r):
circumference = 2 * math.pi * r
n = 50
length = circumference / n
polygon(t, n, length)
exitonclick()
draw_circle(apple, 30)
1.3
class Rectangle(object):
def __init__(self, l, w):
self.length = l
self.width = w
def area(self):
return self.length*self.width
aRectangle = Rectangle(2,10)
print aRectangle.area()
from turtle import *
import math
apple = Turtle()
def polygon(t, n, length):
for i in range(n):
left(360/n)
forward(length)
def draw_circle(t, r):
circumference = 2 * math.pi * r
n = 50
length = circumference / n
polygon(t, n, length)
exitonclick()
draw_circle(apple, 30)
1.3
class Rectangle(object):
def __init__(self, l, w):
self.length = l
self.width = w
def area(self):
return self.length*self.width
aRectangle = Rectangle(2,10)
print aRectangle.area()
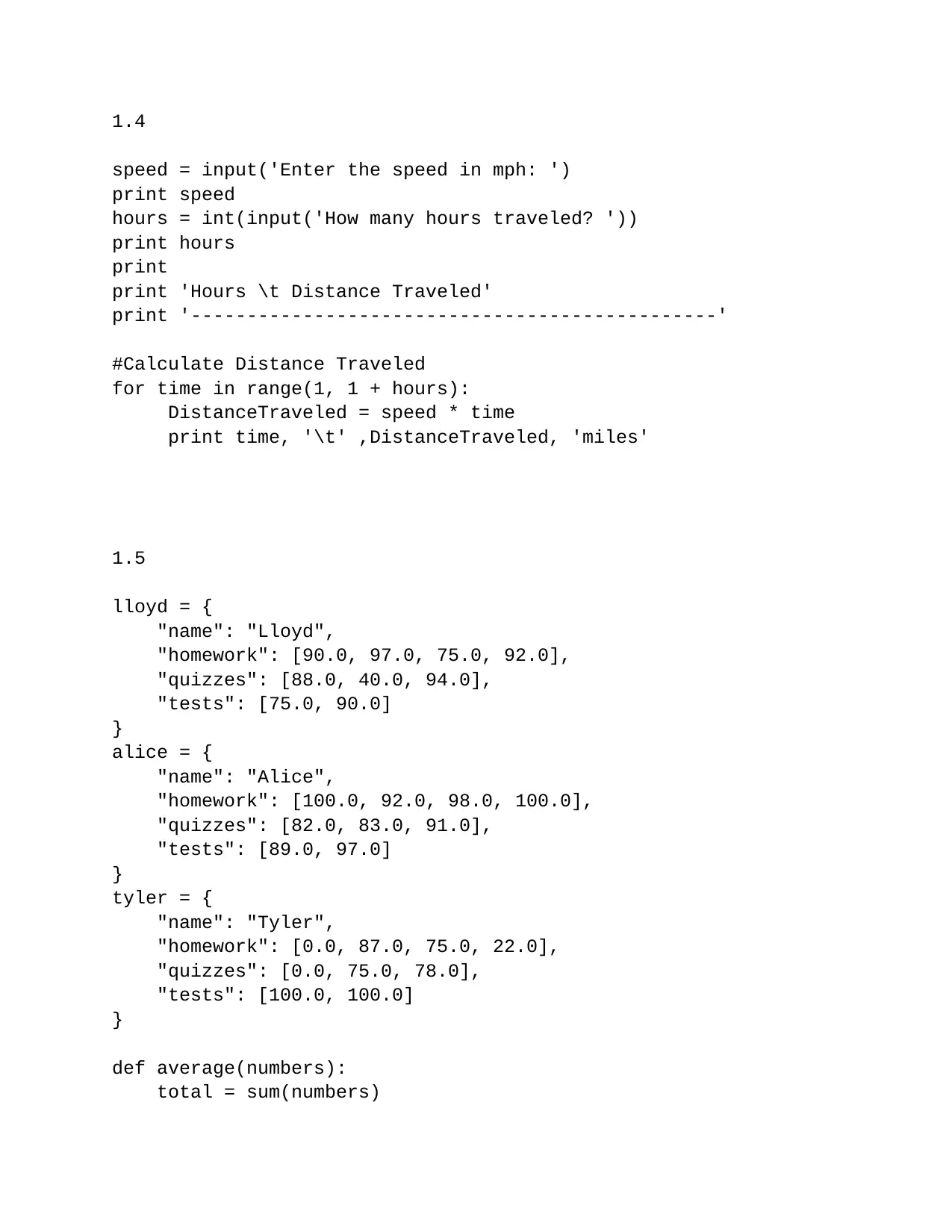
1.4
speed = input('Enter the speed in mph: ')
print speed
hours = int(input('How many hours traveled? '))
print hours
print
print 'Hours \t Distance Traveled'
print '-----------------------------------------------'
#Calculate Distance Traveled
for time in range(1, 1 + hours):
DistanceTraveled = speed * time
print time, '\t' ,DistanceTraveled, 'miles'
1.5
lloyd = {
"name": "Lloyd",
"homework": [90.0, 97.0, 75.0, 92.0],
"quizzes": [88.0, 40.0, 94.0],
"tests": [75.0, 90.0]
}
alice = {
"name": "Alice",
"homework": [100.0, 92.0, 98.0, 100.0],
"quizzes": [82.0, 83.0, 91.0],
"tests": [89.0, 97.0]
}
tyler = {
"name": "Tyler",
"homework": [0.0, 87.0, 75.0, 22.0],
"quizzes": [0.0, 75.0, 78.0],
"tests": [100.0, 100.0]
}
def average(numbers):
total = sum(numbers)
speed = input('Enter the speed in mph: ')
print speed
hours = int(input('How many hours traveled? '))
print hours
print 'Hours \t Distance Traveled'
print '-----------------------------------------------'
#Calculate Distance Traveled
for time in range(1, 1 + hours):
DistanceTraveled = speed * time
print time, '\t' ,DistanceTraveled, 'miles'
1.5
lloyd = {
"name": "Lloyd",
"homework": [90.0, 97.0, 75.0, 92.0],
"quizzes": [88.0, 40.0, 94.0],
"tests": [75.0, 90.0]
}
alice = {
"name": "Alice",
"homework": [100.0, 92.0, 98.0, 100.0],
"quizzes": [82.0, 83.0, 91.0],
"tests": [89.0, 97.0]
}
tyler = {
"name": "Tyler",
"homework": [0.0, 87.0, 75.0, 22.0],
"quizzes": [0.0, 75.0, 78.0],
"tests": [100.0, 100.0]
}
def average(numbers):
total = sum(numbers)
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
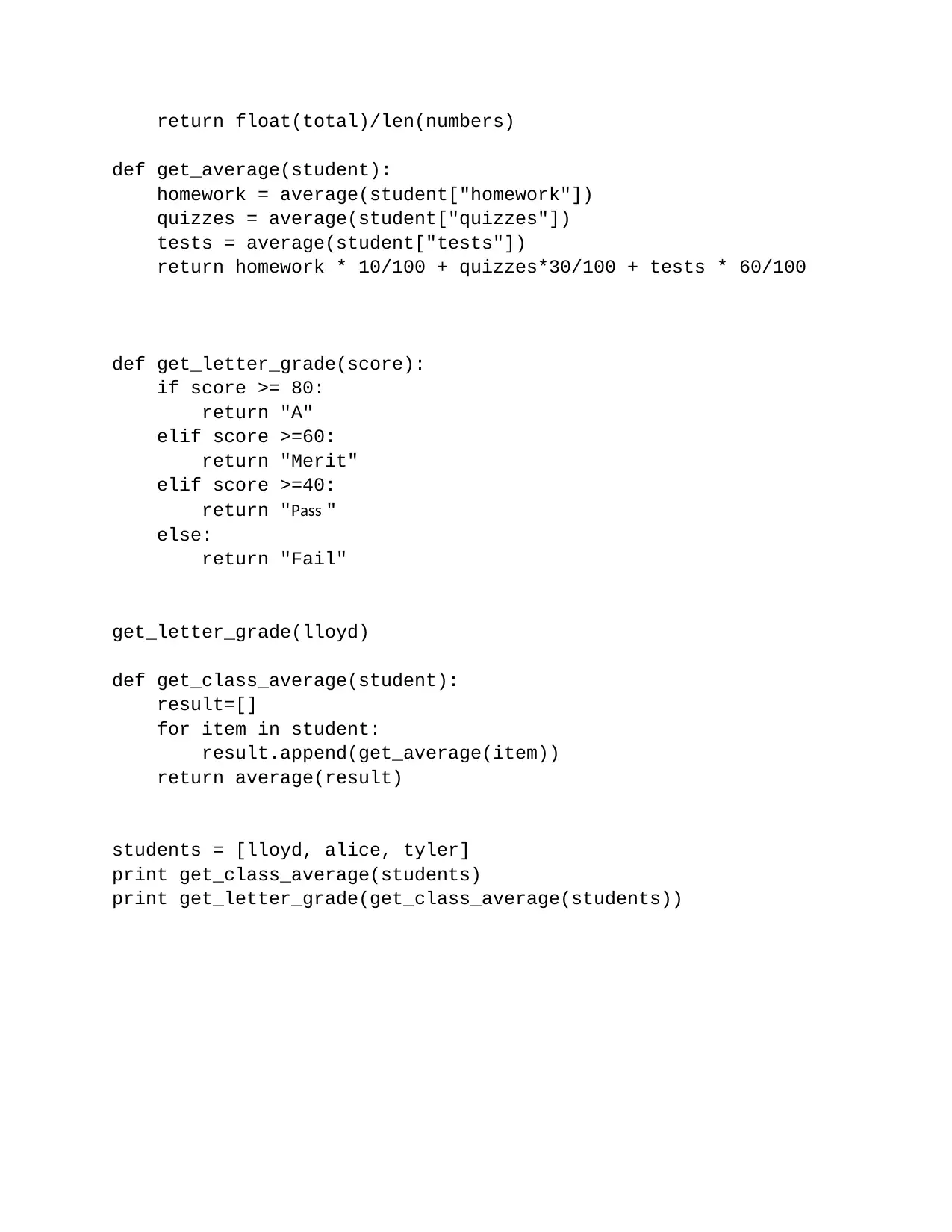
return float(total)/len(numbers)
def get_average(student):
homework = average(student["homework"])
quizzes = average(student["quizzes"])
tests = average(student["tests"])
return homework * 10/100 + quizzes*30/100 + tests * 60/100
def get_letter_grade(score):
if score >= 80:
return "A"
elif score >=60:
return "Merit"
elif score >=40:
return "Pass "
else:
return "Fail"
get_letter_grade(lloyd)
def get_class_average(student):
result=[]
for item in student:
result.append(get_average(item))
return average(result)
students = [lloyd, alice, tyler]
print get_class_average(students)
print get_letter_grade(get_class_average(students))
def get_average(student):
homework = average(student["homework"])
quizzes = average(student["quizzes"])
tests = average(student["tests"])
return homework * 10/100 + quizzes*30/100 + tests * 60/100
def get_letter_grade(score):
if score >= 80:
return "A"
elif score >=60:
return "Merit"
elif score >=40:
return "Pass "
else:
return "Fail"
get_letter_grade(lloyd)
def get_class_average(student):
result=[]
for item in student:
result.append(get_average(item))
return average(result)
students = [lloyd, alice, tyler]
print get_class_average(students)
print get_letter_grade(get_class_average(students))
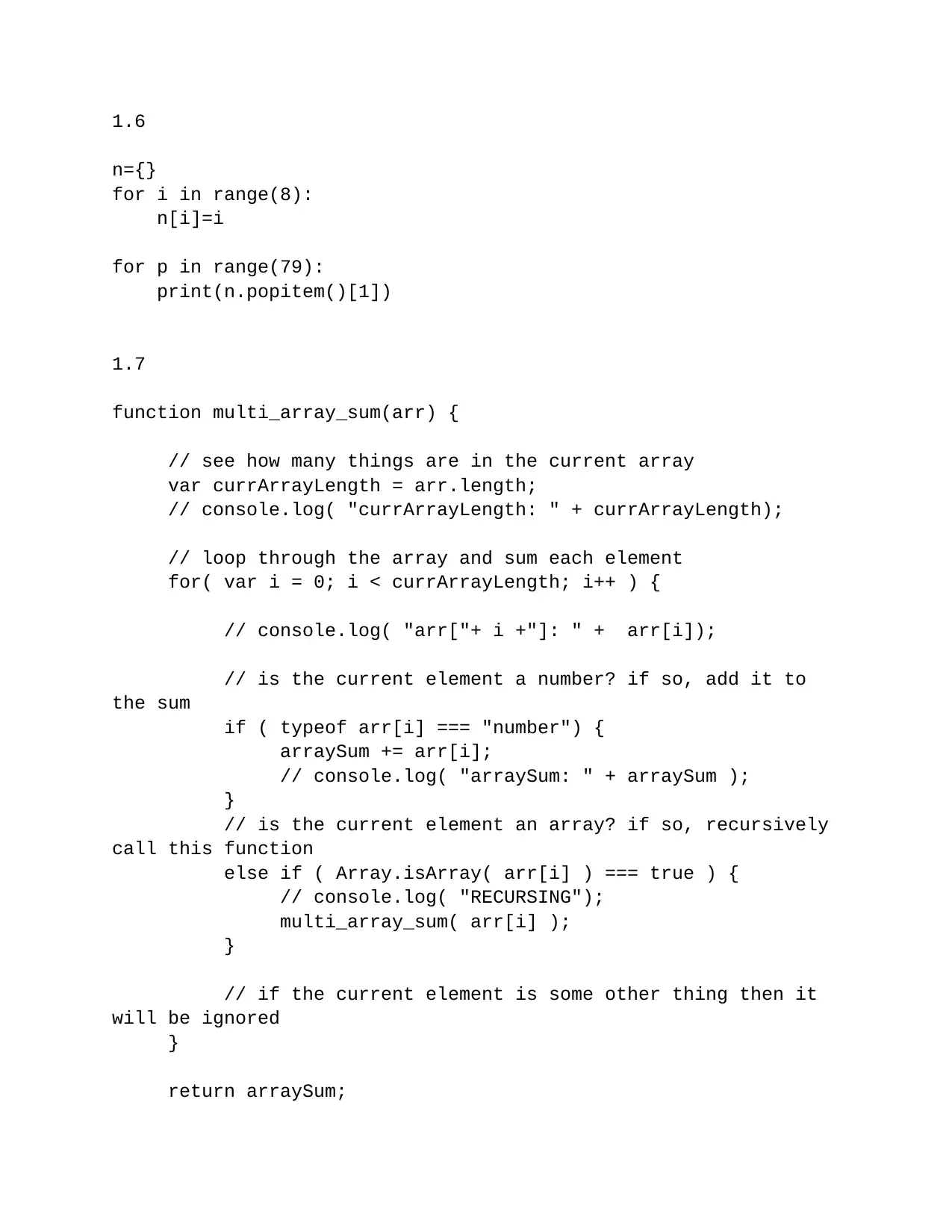
1.6
n={}
for i in range(8):
n[i]=i
for p in range(79):
print(n.popitem()[1])
1.7
function multi_array_sum(arr) {
// see how many things are in the current array
var currArrayLength = arr.length;
// console.log( "currArrayLength: " + currArrayLength);
// loop through the array and sum each element
for( var i = 0; i < currArrayLength; i++ ) {
// console.log( "arr["+ i +"]: " + arr[i]);
// is the current element a number? if so, add it to
the sum
if ( typeof arr[i] === "number") {
arraySum += arr[i];
// console.log( "arraySum: " + arraySum );
}
// is the current element an array? if so, recursively
call this function
else if ( Array.isArray( arr[i] ) === true ) {
// console.log( "RECURSING");
multi_array_sum( arr[i] );
}
// if the current element is some other thing then it
will be ignored
}
return arraySum;
n={}
for i in range(8):
n[i]=i
for p in range(79):
print(n.popitem()[1])
1.7
function multi_array_sum(arr) {
// see how many things are in the current array
var currArrayLength = arr.length;
// console.log( "currArrayLength: " + currArrayLength);
// loop through the array and sum each element
for( var i = 0; i < currArrayLength; i++ ) {
// console.log( "arr["+ i +"]: " + arr[i]);
// is the current element a number? if so, add it to
the sum
if ( typeof arr[i] === "number") {
arraySum += arr[i];
// console.log( "arraySum: " + arraySum );
}
// is the current element an array? if so, recursively
call this function
else if ( Array.isArray( arr[i] ) === true ) {
// console.log( "RECURSING");
multi_array_sum( arr[i] );
}
// if the current element is some other thing then it
will be ignored
}
return arraySum;
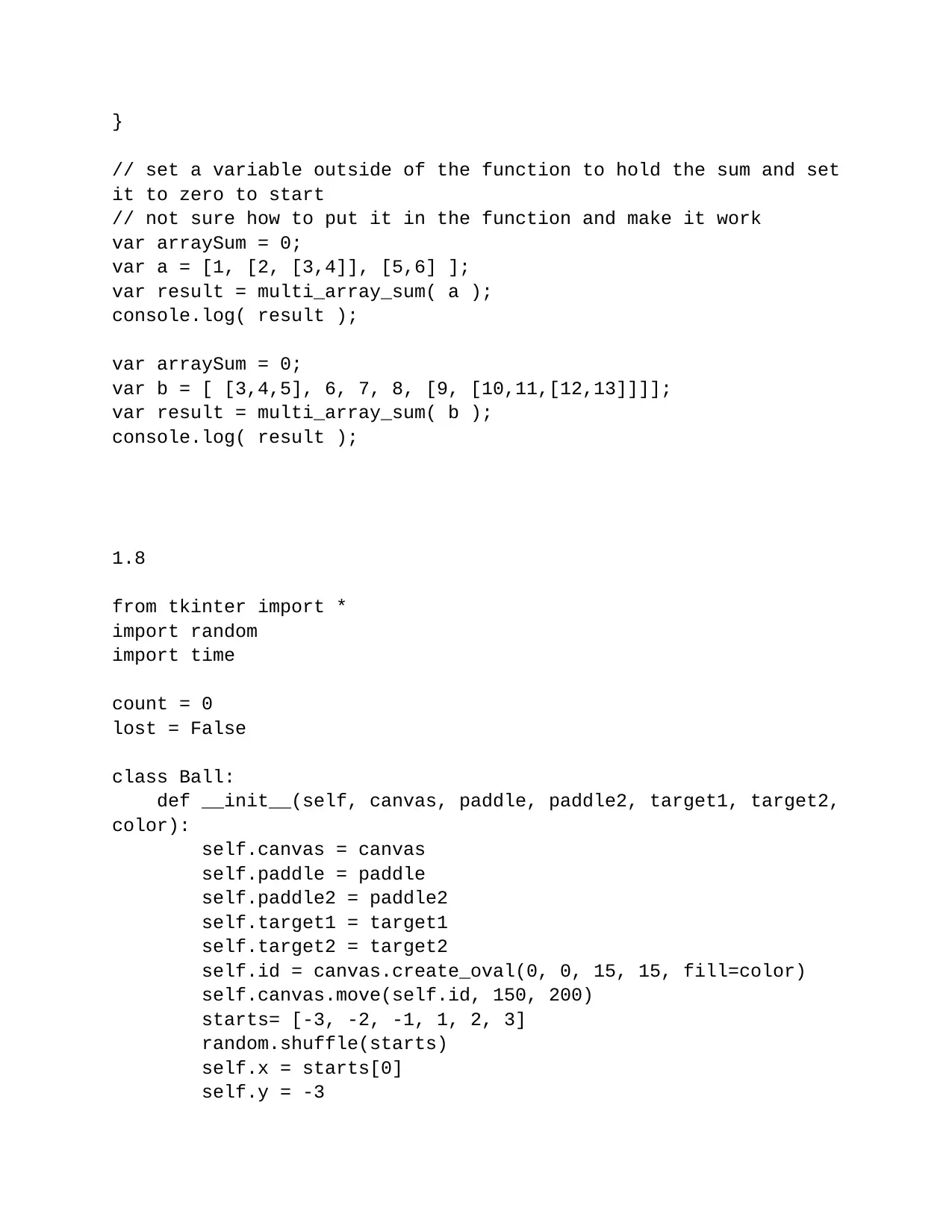
}
// set a variable outside of the function to hold the sum and set
it to zero to start
// not sure how to put it in the function and make it work
var arraySum = 0;
var a = [1, [2, [3,4]], [5,6] ];
var result = multi_array_sum( a );
console.log( result );
var arraySum = 0;
var b = [ [3,4,5], 6, 7, 8, [9, [10,11,[12,13]]]];
var result = multi_array_sum( b );
console.log( result );
1.8
from tkinter import *
import random
import time
count = 0
lost = False
class Ball:
def __init__(self, canvas, paddle, paddle2, target1, target2,
color):
self.canvas = canvas
self.paddle = paddle
self.paddle2 = paddle2
self.target1 = target1
self.target2 = target2
self.id = canvas.create_oval(0, 0, 15, 15, fill=color)
self.canvas.move(self.id, 150, 200)
starts= [-3, -2, -1, 1, 2, 3]
random.shuffle(starts)
self.x = starts[0]
self.y = -3
// set a variable outside of the function to hold the sum and set
it to zero to start
// not sure how to put it in the function and make it work
var arraySum = 0;
var a = [1, [2, [3,4]], [5,6] ];
var result = multi_array_sum( a );
console.log( result );
var arraySum = 0;
var b = [ [3,4,5], 6, 7, 8, [9, [10,11,[12,13]]]];
var result = multi_array_sum( b );
console.log( result );
1.8
from tkinter import *
import random
import time
count = 0
lost = False
class Ball:
def __init__(self, canvas, paddle, paddle2, target1, target2,
color):
self.canvas = canvas
self.paddle = paddle
self.paddle2 = paddle2
self.target1 = target1
self.target2 = target2
self.id = canvas.create_oval(0, 0, 15, 15, fill=color)
self.canvas.move(self.id, 150, 200)
starts= [-3, -2, -1, 1, 2, 3]
random.shuffle(starts)
self.x = starts[0]
self.y = -3
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
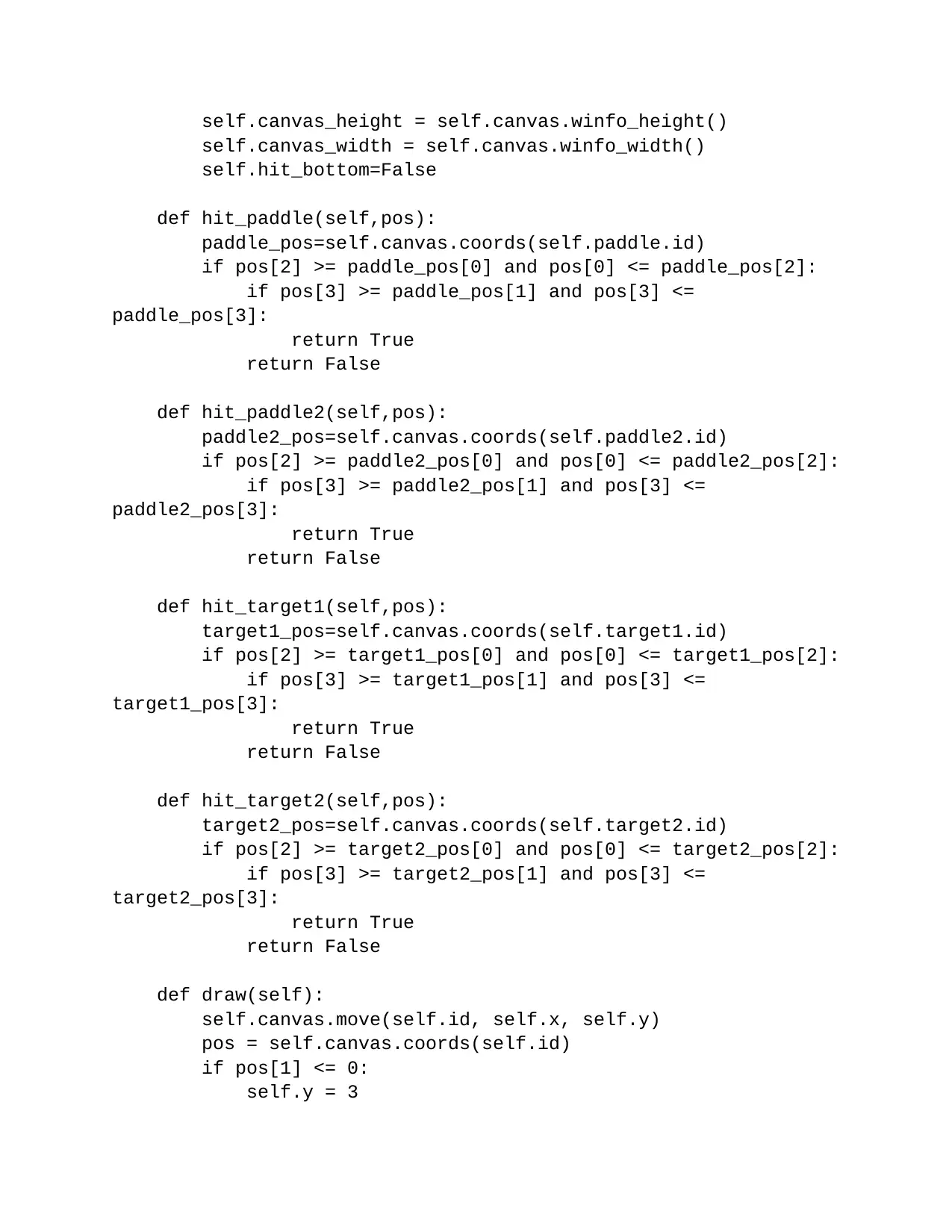
self.canvas_height = self.canvas.winfo_height()
self.canvas_width = self.canvas.winfo_width()
self.hit_bottom=False
def hit_paddle(self,pos):
paddle_pos=self.canvas.coords(self.paddle.id)
if pos[2] >= paddle_pos[0] and pos[0] <= paddle_pos[2]:
if pos[3] >= paddle_pos[1] and pos[3] <=
paddle_pos[3]:
return True
return False
def hit_paddle2(self,pos):
paddle2_pos=self.canvas.coords(self.paddle2.id)
if pos[2] >= paddle2_pos[0] and pos[0] <= paddle2_pos[2]:
if pos[3] >= paddle2_pos[1] and pos[3] <=
paddle2_pos[3]:
return True
return False
def hit_target1(self,pos):
target1_pos=self.canvas.coords(self.target1.id)
if pos[2] >= target1_pos[0] and pos[0] <= target1_pos[2]:
if pos[3] >= target1_pos[1] and pos[3] <=
target1_pos[3]:
return True
return False
def hit_target2(self,pos):
target2_pos=self.canvas.coords(self.target2.id)
if pos[2] >= target2_pos[0] and pos[0] <= target2_pos[2]:
if pos[3] >= target2_pos[1] and pos[3] <=
target2_pos[3]:
return True
return False
def draw(self):
self.canvas.move(self.id, self.x, self.y)
pos = self.canvas.coords(self.id)
if pos[1] <= 0:
self.y = 3
self.canvas_width = self.canvas.winfo_width()
self.hit_bottom=False
def hit_paddle(self,pos):
paddle_pos=self.canvas.coords(self.paddle.id)
if pos[2] >= paddle_pos[0] and pos[0] <= paddle_pos[2]:
if pos[3] >= paddle_pos[1] and pos[3] <=
paddle_pos[3]:
return True
return False
def hit_paddle2(self,pos):
paddle2_pos=self.canvas.coords(self.paddle2.id)
if pos[2] >= paddle2_pos[0] and pos[0] <= paddle2_pos[2]:
if pos[3] >= paddle2_pos[1] and pos[3] <=
paddle2_pos[3]:
return True
return False
def hit_target1(self,pos):
target1_pos=self.canvas.coords(self.target1.id)
if pos[2] >= target1_pos[0] and pos[0] <= target1_pos[2]:
if pos[3] >= target1_pos[1] and pos[3] <=
target1_pos[3]:
return True
return False
def hit_target2(self,pos):
target2_pos=self.canvas.coords(self.target2.id)
if pos[2] >= target2_pos[0] and pos[0] <= target2_pos[2]:
if pos[3] >= target2_pos[1] and pos[3] <=
target2_pos[3]:
return True
return False
def draw(self):
self.canvas.move(self.id, self.x, self.y)
pos = self.canvas.coords(self.id)
if pos[1] <= 0:
self.y = 3
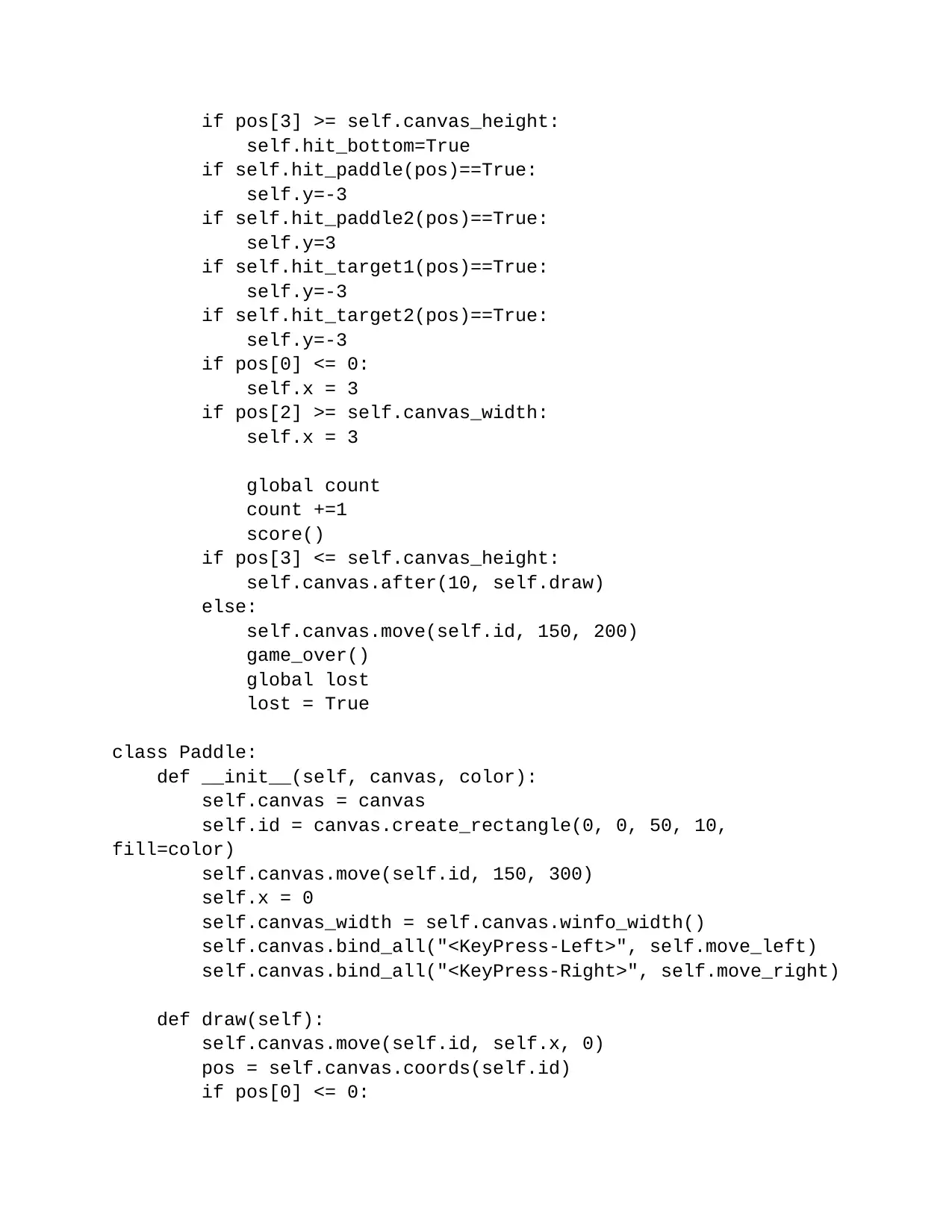
if pos[3] >= self.canvas_height:
self.hit_bottom=True
if self.hit_paddle(pos)==True:
self.y=-3
if self.hit_paddle2(pos)==True:
self.y=3
if self.hit_target1(pos)==True:
self.y=-3
if self.hit_target2(pos)==True:
self.y=-3
if pos[0] <= 0:
self.x = 3
if pos[2] >= self.canvas_width:
self.x = 3
global count
count +=1
score()
if pos[3] <= self.canvas_height:
self.canvas.after(10, self.draw)
else:
self.canvas.move(self.id, 150, 200)
game_over()
global lost
lost = True
class Paddle:
def __init__(self, canvas, color):
self.canvas = canvas
self.id = canvas.create_rectangle(0, 0, 50, 10,
fill=color)
self.canvas.move(self.id, 150, 300)
self.x = 0
self.canvas_width = self.canvas.winfo_width()
self.canvas.bind_all("<KeyPress-Left>", self.move_left)
self.canvas.bind_all("<KeyPress-Right>", self.move_right)
def draw(self):
self.canvas.move(self.id, self.x, 0)
pos = self.canvas.coords(self.id)
if pos[0] <= 0:
self.hit_bottom=True
if self.hit_paddle(pos)==True:
self.y=-3
if self.hit_paddle2(pos)==True:
self.y=3
if self.hit_target1(pos)==True:
self.y=-3
if self.hit_target2(pos)==True:
self.y=-3
if pos[0] <= 0:
self.x = 3
if pos[2] >= self.canvas_width:
self.x = 3
global count
count +=1
score()
if pos[3] <= self.canvas_height:
self.canvas.after(10, self.draw)
else:
self.canvas.move(self.id, 150, 200)
game_over()
global lost
lost = True
class Paddle:
def __init__(self, canvas, color):
self.canvas = canvas
self.id = canvas.create_rectangle(0, 0, 50, 10,
fill=color)
self.canvas.move(self.id, 150, 300)
self.x = 0
self.canvas_width = self.canvas.winfo_width()
self.canvas.bind_all("<KeyPress-Left>", self.move_left)
self.canvas.bind_all("<KeyPress-Right>", self.move_right)
def draw(self):
self.canvas.move(self.id, self.x, 0)
pos = self.canvas.coords(self.id)
if pos[0] <= 0:
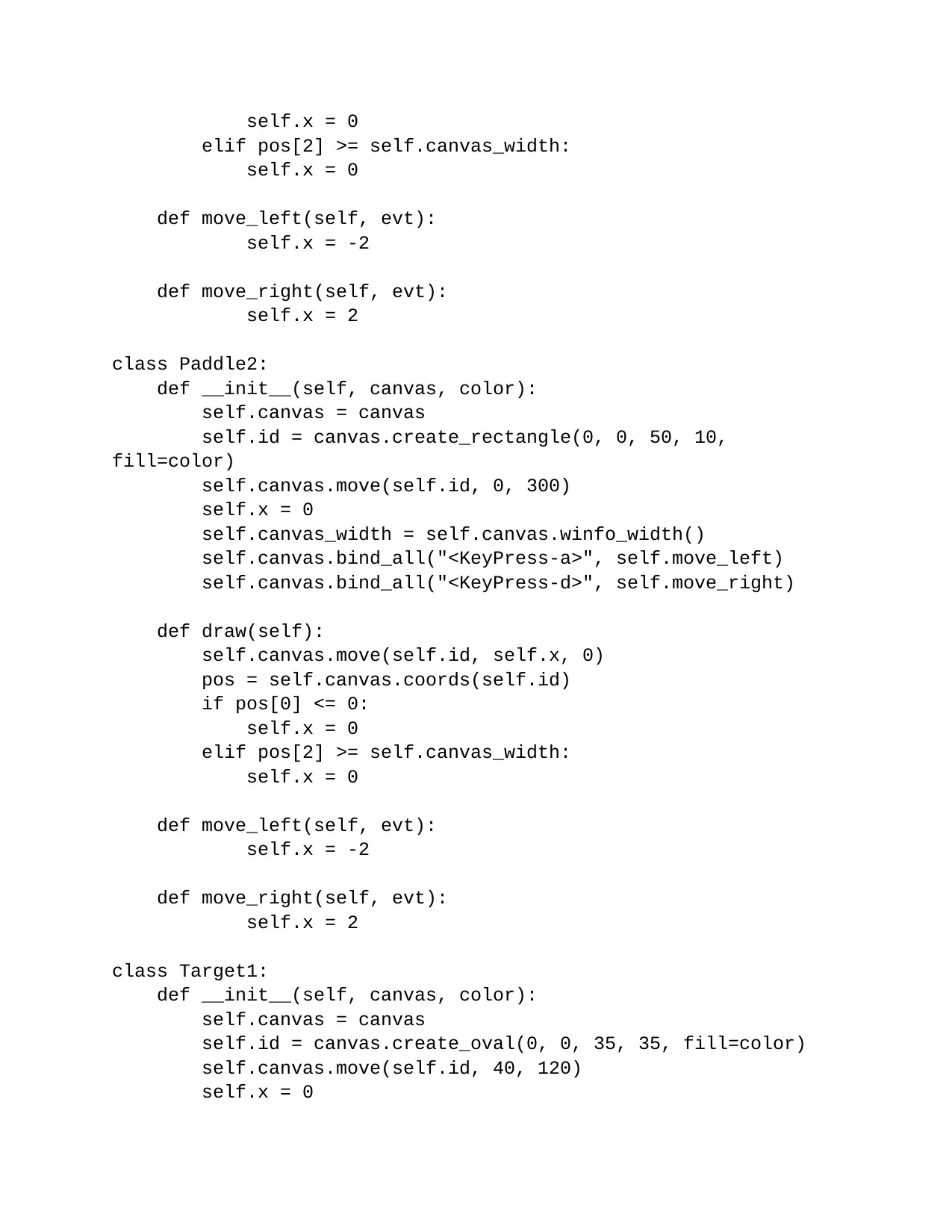
self.x = 0
elif pos[2] >= self.canvas_width:
self.x = 0
def move_left(self, evt):
self.x = -2
def move_right(self, evt):
self.x = 2
class Paddle2:
def __init__(self, canvas, color):
self.canvas = canvas
self.id = canvas.create_rectangle(0, 0, 50, 10,
fill=color)
self.canvas.move(self.id, 0, 300)
self.x = 0
self.canvas_width = self.canvas.winfo_width()
self.canvas.bind_all("<KeyPress-a>", self.move_left)
self.canvas.bind_all("<KeyPress-d>", self.move_right)
def draw(self):
self.canvas.move(self.id, self.x, 0)
pos = self.canvas.coords(self.id)
if pos[0] <= 0:
self.x = 0
elif pos[2] >= self.canvas_width:
self.x = 0
def move_left(self, evt):
self.x = -2
def move_right(self, evt):
self.x = 2
class Target1:
def __init__(self, canvas, color):
self.canvas = canvas
self.id = canvas.create_oval(0, 0, 35, 35, fill=color)
self.canvas.move(self.id, 40, 120)
self.x = 0
elif pos[2] >= self.canvas_width:
self.x = 0
def move_left(self, evt):
self.x = -2
def move_right(self, evt):
self.x = 2
class Paddle2:
def __init__(self, canvas, color):
self.canvas = canvas
self.id = canvas.create_rectangle(0, 0, 50, 10,
fill=color)
self.canvas.move(self.id, 0, 300)
self.x = 0
self.canvas_width = self.canvas.winfo_width()
self.canvas.bind_all("<KeyPress-a>", self.move_left)
self.canvas.bind_all("<KeyPress-d>", self.move_right)
def draw(self):
self.canvas.move(self.id, self.x, 0)
pos = self.canvas.coords(self.id)
if pos[0] <= 0:
self.x = 0
elif pos[2] >= self.canvas_width:
self.x = 0
def move_left(self, evt):
self.x = -2
def move_right(self, evt):
self.x = 2
class Target1:
def __init__(self, canvas, color):
self.canvas = canvas
self.id = canvas.create_oval(0, 0, 35, 35, fill=color)
self.canvas.move(self.id, 40, 120)
self.x = 0
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
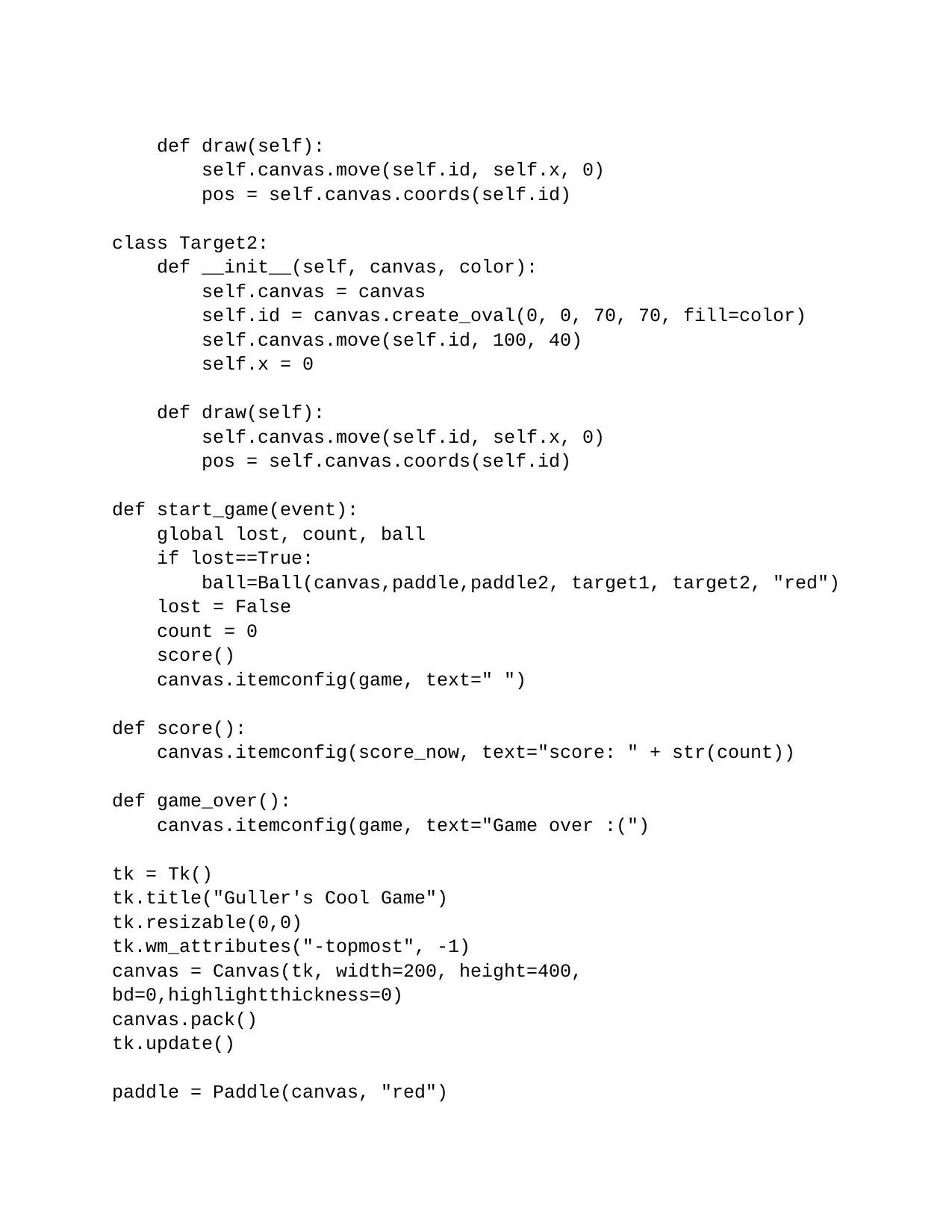
def draw(self):
self.canvas.move(self.id, self.x, 0)
pos = self.canvas.coords(self.id)
class Target2:
def __init__(self, canvas, color):
self.canvas = canvas
self.id = canvas.create_oval(0, 0, 70, 70, fill=color)
self.canvas.move(self.id, 100, 40)
self.x = 0
def draw(self):
self.canvas.move(self.id, self.x, 0)
pos = self.canvas.coords(self.id)
def start_game(event):
global lost, count, ball
if lost==True:
ball=Ball(canvas,paddle,paddle2, target1, target2, "red")
lost = False
count = 0
score()
canvas.itemconfig(game, text=" ")
def score():
canvas.itemconfig(score_now, text="score: " + str(count))
def game_over():
canvas.itemconfig(game, text="Game over :(")
tk = Tk()
tk.title("Guller's Cool Game")
tk.resizable(0,0)
tk.wm_attributes("-topmost", -1)
canvas = Canvas(tk, width=200, height=400,
bd=0,highlightthickness=0)
canvas.pack()
tk.update()
paddle = Paddle(canvas, "red")
self.canvas.move(self.id, self.x, 0)
pos = self.canvas.coords(self.id)
class Target2:
def __init__(self, canvas, color):
self.canvas = canvas
self.id = canvas.create_oval(0, 0, 70, 70, fill=color)
self.canvas.move(self.id, 100, 40)
self.x = 0
def draw(self):
self.canvas.move(self.id, self.x, 0)
pos = self.canvas.coords(self.id)
def start_game(event):
global lost, count, ball
if lost==True:
ball=Ball(canvas,paddle,paddle2, target1, target2, "red")
lost = False
count = 0
score()
canvas.itemconfig(game, text=" ")
def score():
canvas.itemconfig(score_now, text="score: " + str(count))
def game_over():
canvas.itemconfig(game, text="Game over :(")
tk = Tk()
tk.title("Guller's Cool Game")
tk.resizable(0,0)
tk.wm_attributes("-topmost", -1)
canvas = Canvas(tk, width=200, height=400,
bd=0,highlightthickness=0)
canvas.pack()
tk.update()
paddle = Paddle(canvas, "red")
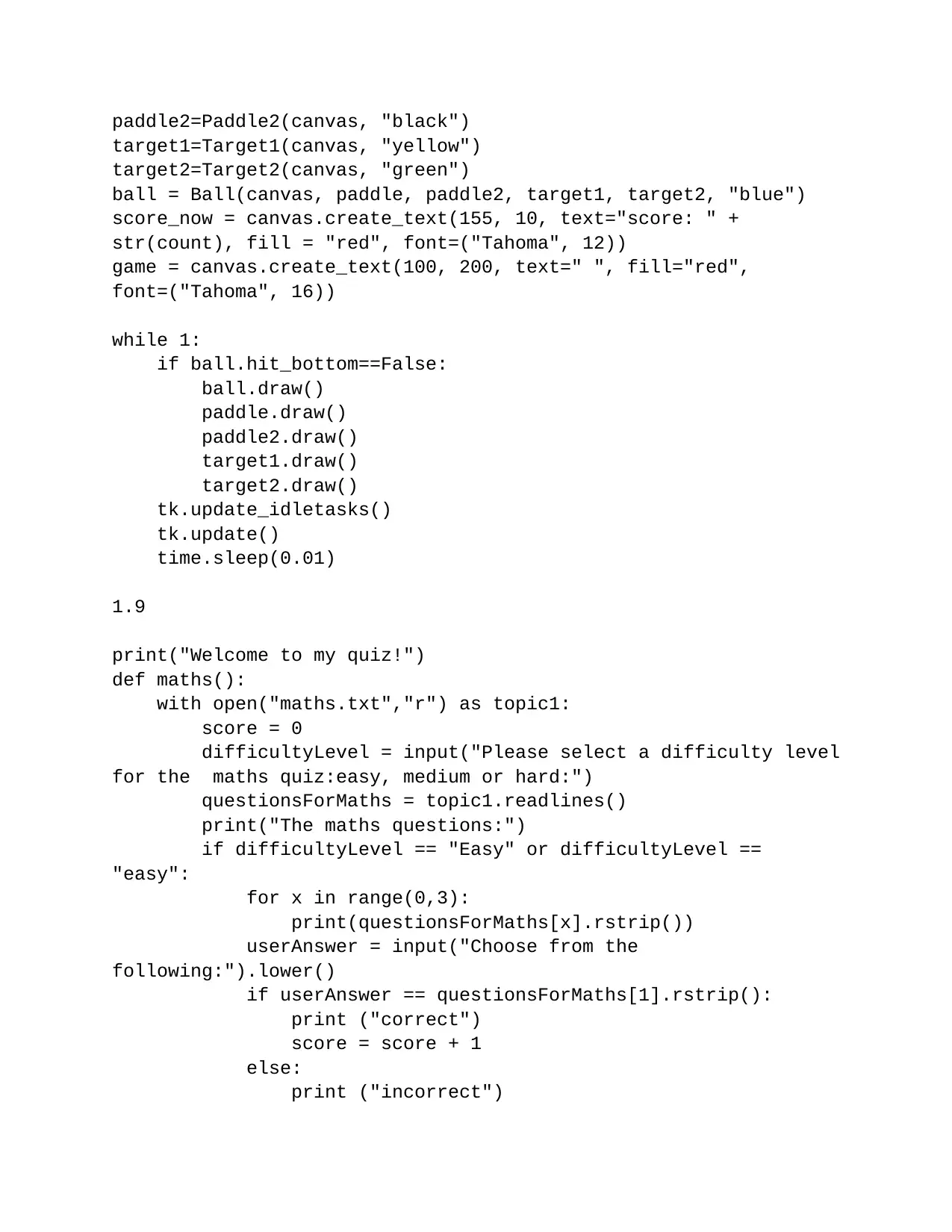
paddle2=Paddle2(canvas, "black")
target1=Target1(canvas, "yellow")
target2=Target2(canvas, "green")
ball = Ball(canvas, paddle, paddle2, target1, target2, "blue")
score_now = canvas.create_text(155, 10, text="score: " +
str(count), fill = "red", font=("Tahoma", 12))
game = canvas.create_text(100, 200, text=" ", fill="red",
font=("Tahoma", 16))
while 1:
if ball.hit_bottom==False:
ball.draw()
paddle.draw()
paddle2.draw()
target1.draw()
target2.draw()
tk.update_idletasks()
tk.update()
time.sleep(0.01)
1.9
print("Welcome to my quiz!")
def maths():
with open("maths.txt","r") as topic1:
score = 0
difficultyLevel = input("Please select a difficulty level
for the maths quiz:easy, medium or hard:")
questionsForMaths = topic1.readlines()
print("The maths questions:")
if difficultyLevel == "Easy" or difficultyLevel ==
"easy":
for x in range(0,3):
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the
following:").lower()
if userAnswer == questionsForMaths[1].rstrip():
print ("correct")
score = score + 1
else:
print ("incorrect")
target1=Target1(canvas, "yellow")
target2=Target2(canvas, "green")
ball = Ball(canvas, paddle, paddle2, target1, target2, "blue")
score_now = canvas.create_text(155, 10, text="score: " +
str(count), fill = "red", font=("Tahoma", 12))
game = canvas.create_text(100, 200, text=" ", fill="red",
font=("Tahoma", 16))
while 1:
if ball.hit_bottom==False:
ball.draw()
paddle.draw()
paddle2.draw()
target1.draw()
target2.draw()
tk.update_idletasks()
tk.update()
time.sleep(0.01)
1.9
print("Welcome to my quiz!")
def maths():
with open("maths.txt","r") as topic1:
score = 0
difficultyLevel = input("Please select a difficulty level
for the maths quiz:easy, medium or hard:")
questionsForMaths = topic1.readlines()
print("The maths questions:")
if difficultyLevel == "Easy" or difficultyLevel ==
"easy":
for x in range(0,3):
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the
following:").lower()
if userAnswer == questionsForMaths[1].rstrip():
print ("correct")
score = score + 1
else:
print ("incorrect")
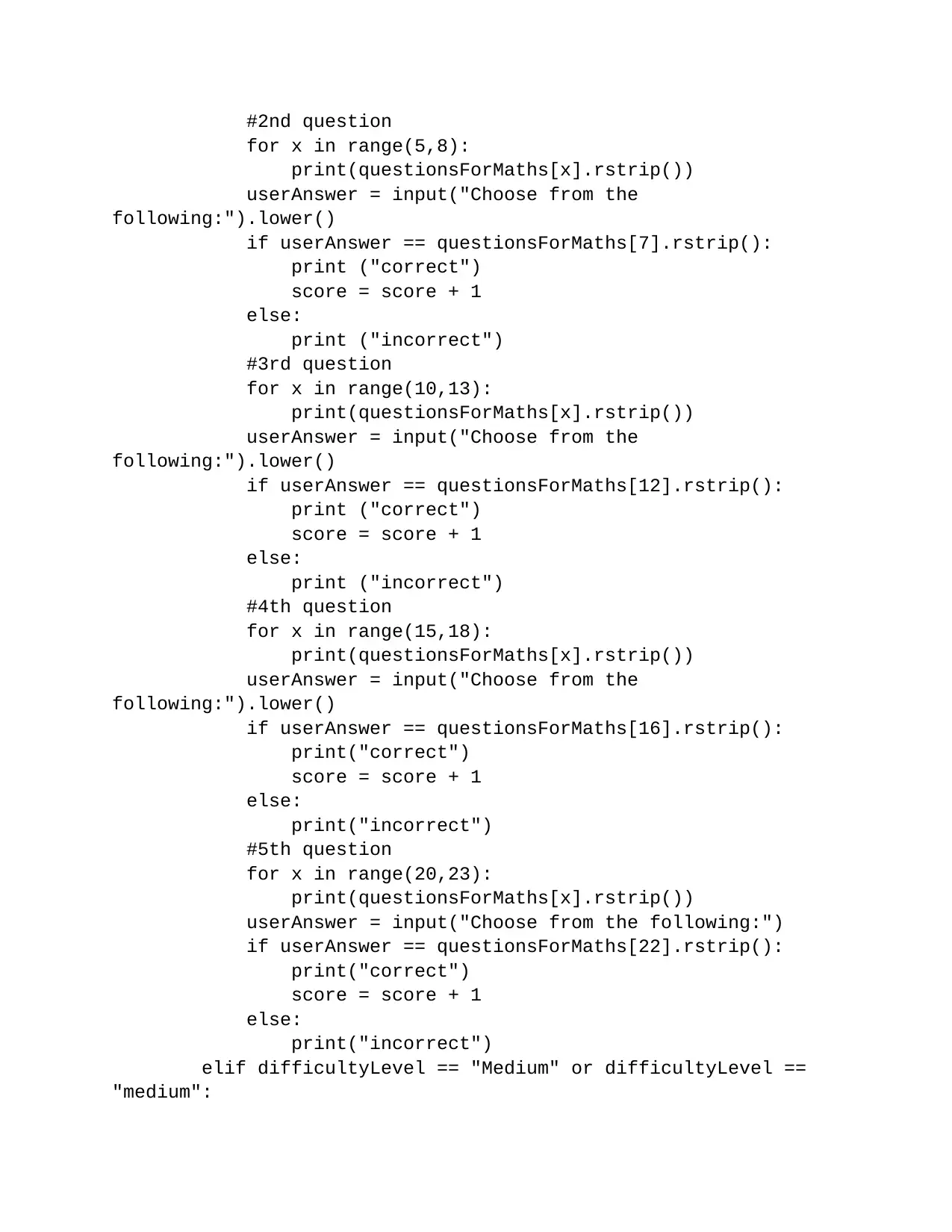
#2nd question
for x in range(5,8):
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the
following:").lower()
if userAnswer == questionsForMaths[7].rstrip():
print ("correct")
score = score + 1
else:
print ("incorrect")
#3rd question
for x in range(10,13):
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the
following:").lower()
if userAnswer == questionsForMaths[12].rstrip():
print ("correct")
score = score + 1
else:
print ("incorrect")
#4th question
for x in range(15,18):
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the
following:").lower()
if userAnswer == questionsForMaths[16].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
#5th question
for x in range(20,23):
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the following:")
if userAnswer == questionsForMaths[22].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
elif difficultyLevel == "Medium" or difficultyLevel ==
"medium":
for x in range(5,8):
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the
following:").lower()
if userAnswer == questionsForMaths[7].rstrip():
print ("correct")
score = score + 1
else:
print ("incorrect")
#3rd question
for x in range(10,13):
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the
following:").lower()
if userAnswer == questionsForMaths[12].rstrip():
print ("correct")
score = score + 1
else:
print ("incorrect")
#4th question
for x in range(15,18):
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the
following:").lower()
if userAnswer == questionsForMaths[16].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
#5th question
for x in range(20,23):
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the following:")
if userAnswer == questionsForMaths[22].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
elif difficultyLevel == "Medium" or difficultyLevel ==
"medium":
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
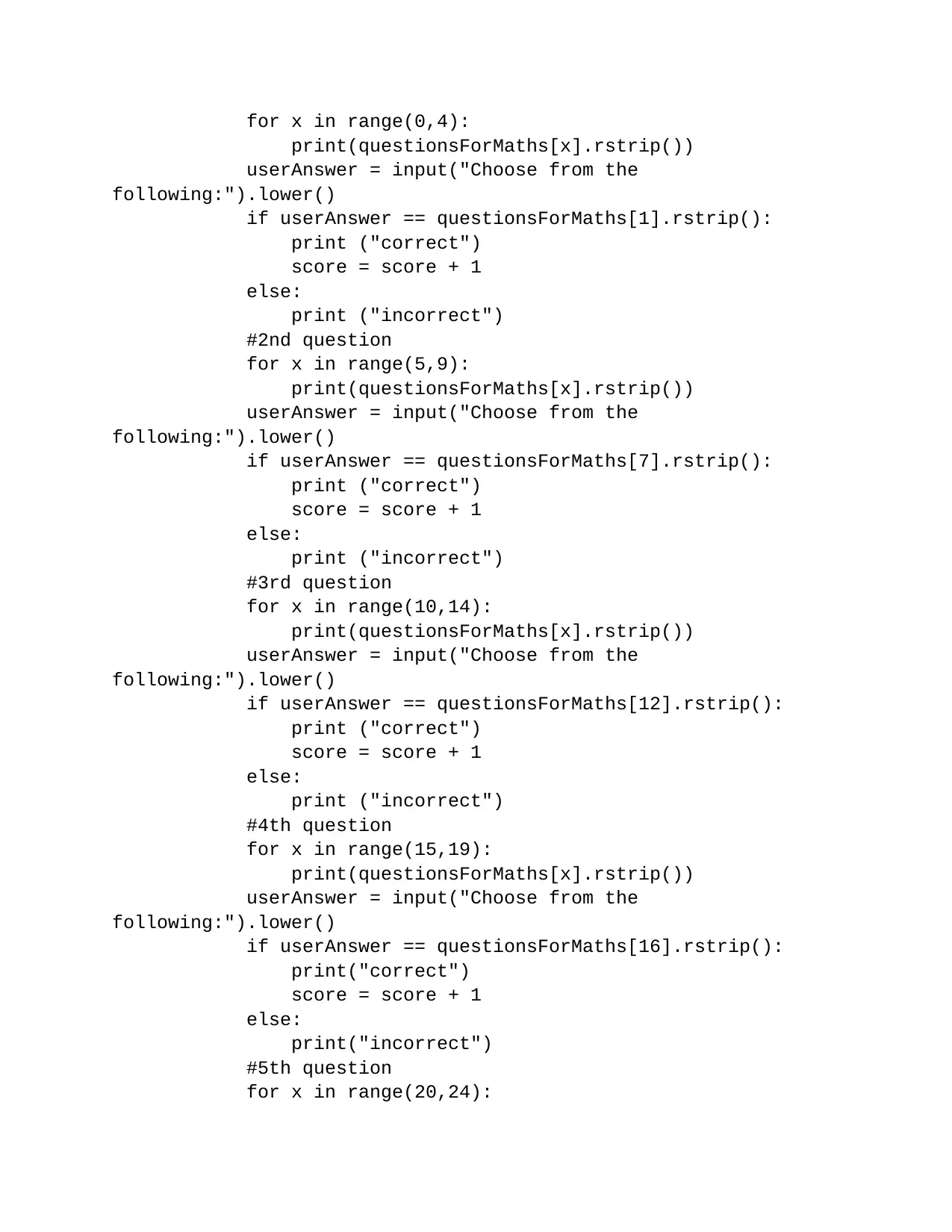
for x in range(0,4):
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the
following:").lower()
if userAnswer == questionsForMaths[1].rstrip():
print ("correct")
score = score + 1
else:
print ("incorrect")
#2nd question
for x in range(5,9):
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the
following:").lower()
if userAnswer == questionsForMaths[7].rstrip():
print ("correct")
score = score + 1
else:
print ("incorrect")
#3rd question
for x in range(10,14):
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the
following:").lower()
if userAnswer == questionsForMaths[12].rstrip():
print ("correct")
score = score + 1
else:
print ("incorrect")
#4th question
for x in range(15,19):
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the
following:").lower()
if userAnswer == questionsForMaths[16].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
#5th question
for x in range(20,24):
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the
following:").lower()
if userAnswer == questionsForMaths[1].rstrip():
print ("correct")
score = score + 1
else:
print ("incorrect")
#2nd question
for x in range(5,9):
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the
following:").lower()
if userAnswer == questionsForMaths[7].rstrip():
print ("correct")
score = score + 1
else:
print ("incorrect")
#3rd question
for x in range(10,14):
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the
following:").lower()
if userAnswer == questionsForMaths[12].rstrip():
print ("correct")
score = score + 1
else:
print ("incorrect")
#4th question
for x in range(15,19):
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the
following:").lower()
if userAnswer == questionsForMaths[16].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
#5th question
for x in range(20,24):
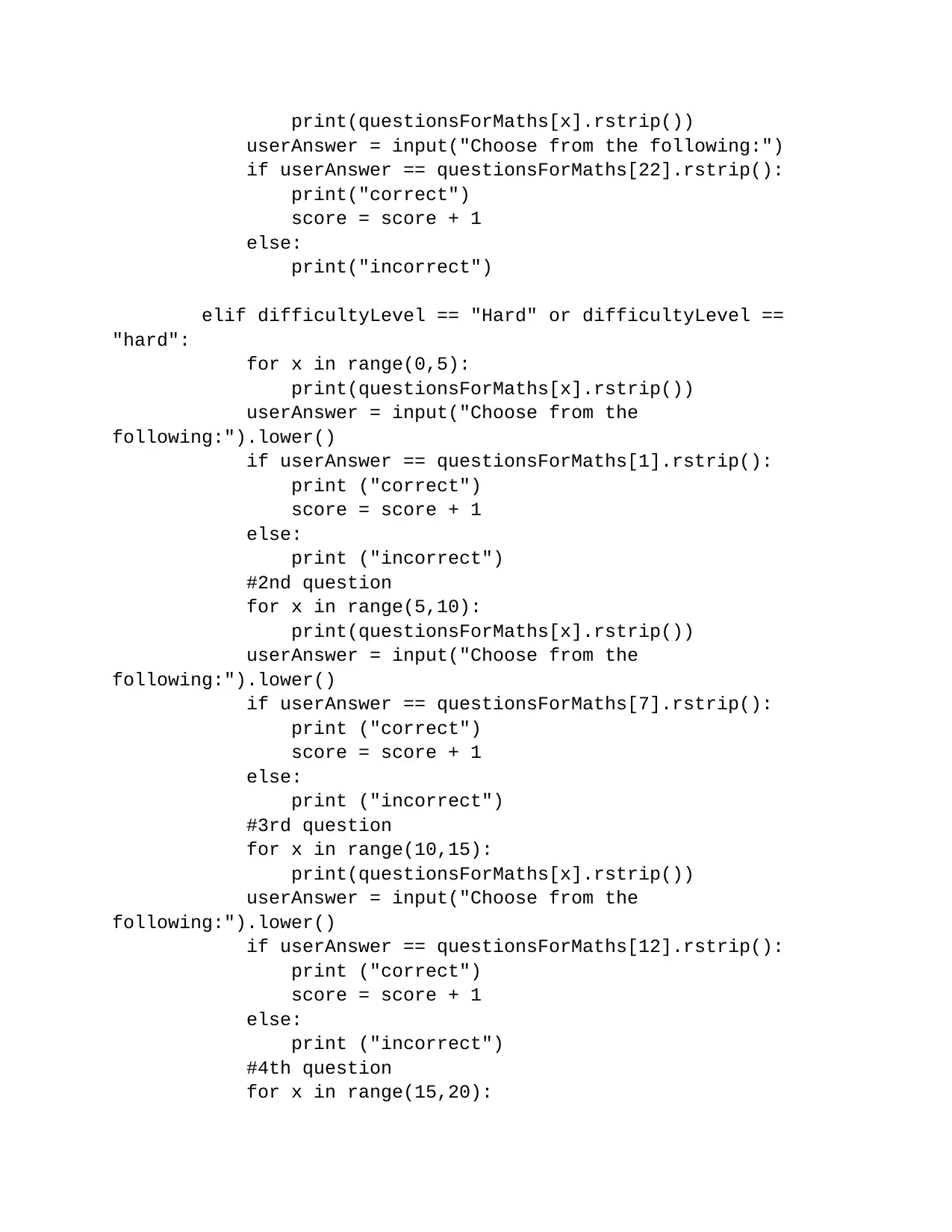
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the following:")
if userAnswer == questionsForMaths[22].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
elif difficultyLevel == "Hard" or difficultyLevel ==
"hard":
for x in range(0,5):
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the
following:").lower()
if userAnswer == questionsForMaths[1].rstrip():
print ("correct")
score = score + 1
else:
print ("incorrect")
#2nd question
for x in range(5,10):
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the
following:").lower()
if userAnswer == questionsForMaths[7].rstrip():
print ("correct")
score = score + 1
else:
print ("incorrect")
#3rd question
for x in range(10,15):
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the
following:").lower()
if userAnswer == questionsForMaths[12].rstrip():
print ("correct")
score = score + 1
else:
print ("incorrect")
#4th question
for x in range(15,20):
userAnswer = input("Choose from the following:")
if userAnswer == questionsForMaths[22].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
elif difficultyLevel == "Hard" or difficultyLevel ==
"hard":
for x in range(0,5):
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the
following:").lower()
if userAnswer == questionsForMaths[1].rstrip():
print ("correct")
score = score + 1
else:
print ("incorrect")
#2nd question
for x in range(5,10):
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the
following:").lower()
if userAnswer == questionsForMaths[7].rstrip():
print ("correct")
score = score + 1
else:
print ("incorrect")
#3rd question
for x in range(10,15):
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the
following:").lower()
if userAnswer == questionsForMaths[12].rstrip():
print ("correct")
score = score + 1
else:
print ("incorrect")
#4th question
for x in range(15,20):
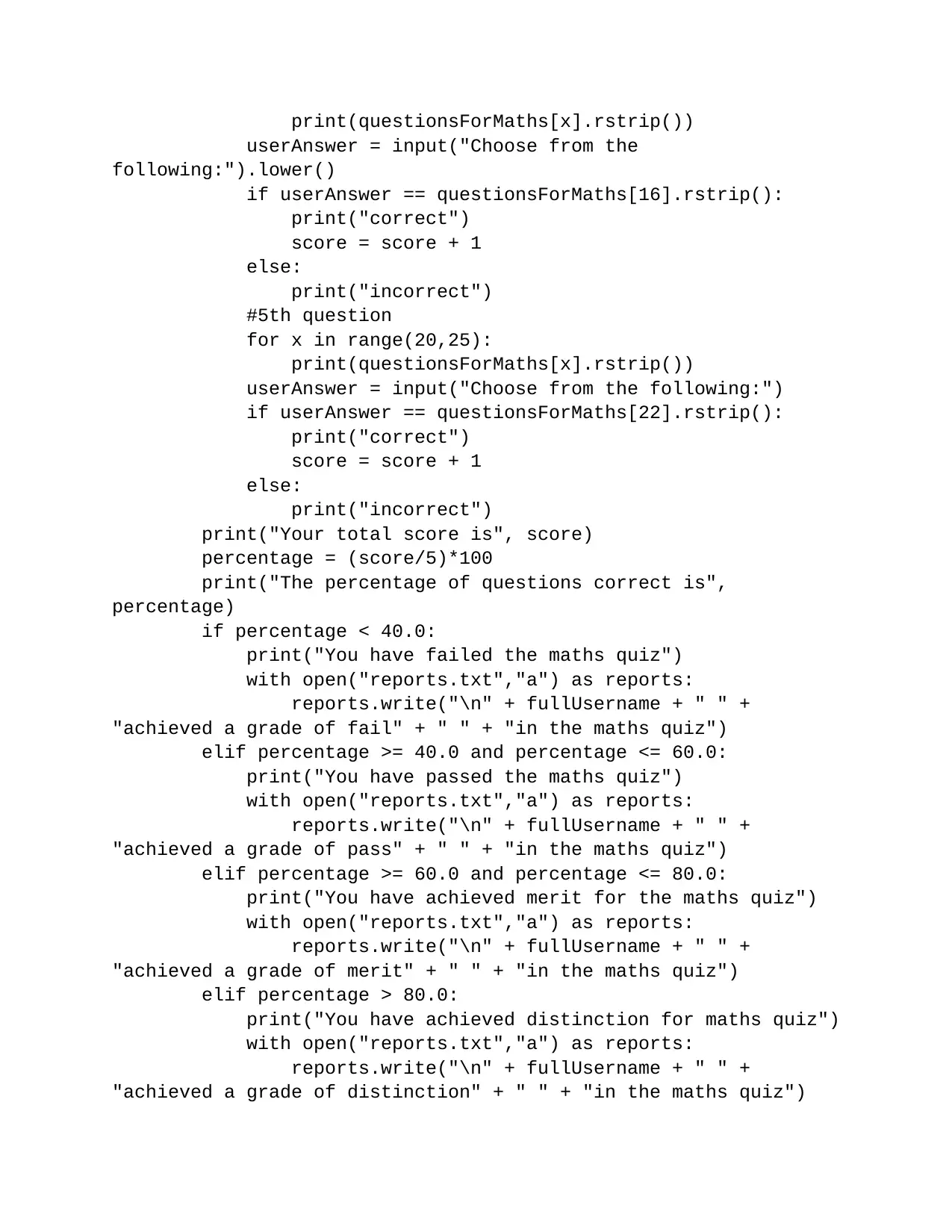
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the
following:").lower()
if userAnswer == questionsForMaths[16].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
#5th question
for x in range(20,25):
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the following:")
if userAnswer == questionsForMaths[22].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
print("Your total score is", score)
percentage = (score/5)*100
print("The percentage of questions correct is",
percentage)
if percentage < 40.0:
print("You have failed the maths quiz")
with open("reports.txt","a") as reports:
reports.write("\n" + fullUsername + " " +
"achieved a grade of fail" + " " + "in the maths quiz")
elif percentage >= 40.0 and percentage <= 60.0:
print("You have passed the maths quiz")
with open("reports.txt","a") as reports:
reports.write("\n" + fullUsername + " " +
"achieved a grade of pass" + " " + "in the maths quiz")
elif percentage >= 60.0 and percentage <= 80.0:
print("You have achieved merit for the maths quiz")
with open("reports.txt","a") as reports:
reports.write("\n" + fullUsername + " " +
"achieved a grade of merit" + " " + "in the maths quiz")
elif percentage > 80.0:
print("You have achieved distinction for maths quiz")
with open("reports.txt","a") as reports:
reports.write("\n" + fullUsername + " " +
"achieved a grade of distinction" + " " + "in the maths quiz")
userAnswer = input("Choose from the
following:").lower()
if userAnswer == questionsForMaths[16].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
#5th question
for x in range(20,25):
print(questionsForMaths[x].rstrip())
userAnswer = input("Choose from the following:")
if userAnswer == questionsForMaths[22].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
print("Your total score is", score)
percentage = (score/5)*100
print("The percentage of questions correct is",
percentage)
if percentage < 40.0:
print("You have failed the maths quiz")
with open("reports.txt","a") as reports:
reports.write("\n" + fullUsername + " " +
"achieved a grade of fail" + " " + "in the maths quiz")
elif percentage >= 40.0 and percentage <= 60.0:
print("You have passed the maths quiz")
with open("reports.txt","a") as reports:
reports.write("\n" + fullUsername + " " +
"achieved a grade of pass" + " " + "in the maths quiz")
elif percentage >= 60.0 and percentage <= 80.0:
print("You have achieved merit for the maths quiz")
with open("reports.txt","a") as reports:
reports.write("\n" + fullUsername + " " +
"achieved a grade of merit" + " " + "in the maths quiz")
elif percentage > 80.0:
print("You have achieved distinction for maths quiz")
with open("reports.txt","a") as reports:
reports.write("\n" + fullUsername + " " +
"achieved a grade of distinction" + " " + "in the maths quiz")
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
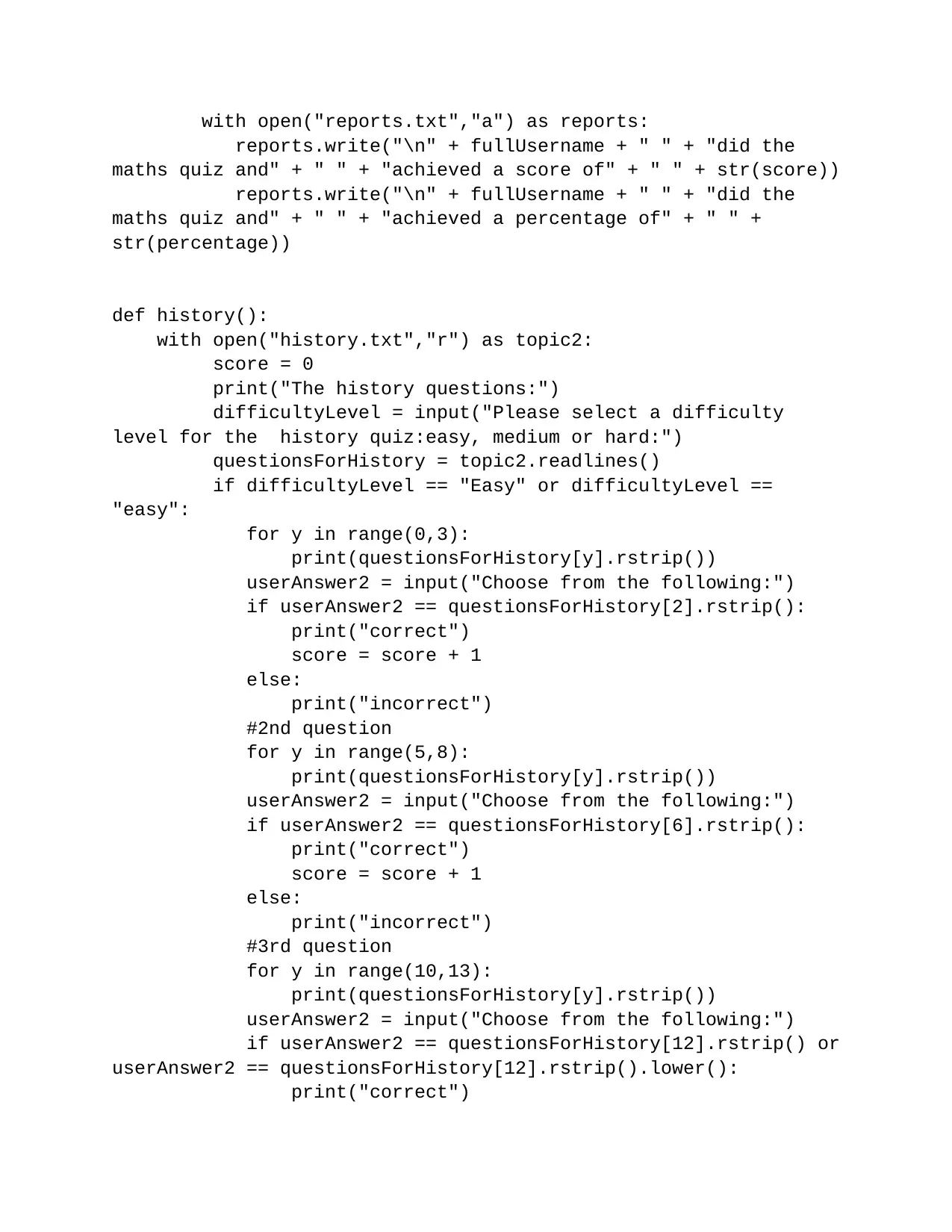
with open("reports.txt","a") as reports:
reports.write("\n" + fullUsername + " " + "did the
maths quiz and" + " " + "achieved a score of" + " " + str(score))
reports.write("\n" + fullUsername + " " + "did the
maths quiz and" + " " + "achieved a percentage of" + " " +
str(percentage))
def history():
with open("history.txt","r") as topic2:
score = 0
print("The history questions:")
difficultyLevel = input("Please select a difficulty
level for the history quiz:easy, medium or hard:")
questionsForHistory = topic2.readlines()
if difficultyLevel == "Easy" or difficultyLevel ==
"easy":
for y in range(0,3):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[2].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
#2nd question
for y in range(5,8):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[6].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
#3rd question
for y in range(10,13):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[12].rstrip() or
userAnswer2 == questionsForHistory[12].rstrip().lower():
print("correct")
reports.write("\n" + fullUsername + " " + "did the
maths quiz and" + " " + "achieved a score of" + " " + str(score))
reports.write("\n" + fullUsername + " " + "did the
maths quiz and" + " " + "achieved a percentage of" + " " +
str(percentage))
def history():
with open("history.txt","r") as topic2:
score = 0
print("The history questions:")
difficultyLevel = input("Please select a difficulty
level for the history quiz:easy, medium or hard:")
questionsForHistory = topic2.readlines()
if difficultyLevel == "Easy" or difficultyLevel ==
"easy":
for y in range(0,3):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[2].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
#2nd question
for y in range(5,8):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[6].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
#3rd question
for y in range(10,13):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[12].rstrip() or
userAnswer2 == questionsForHistory[12].rstrip().lower():
print("correct")
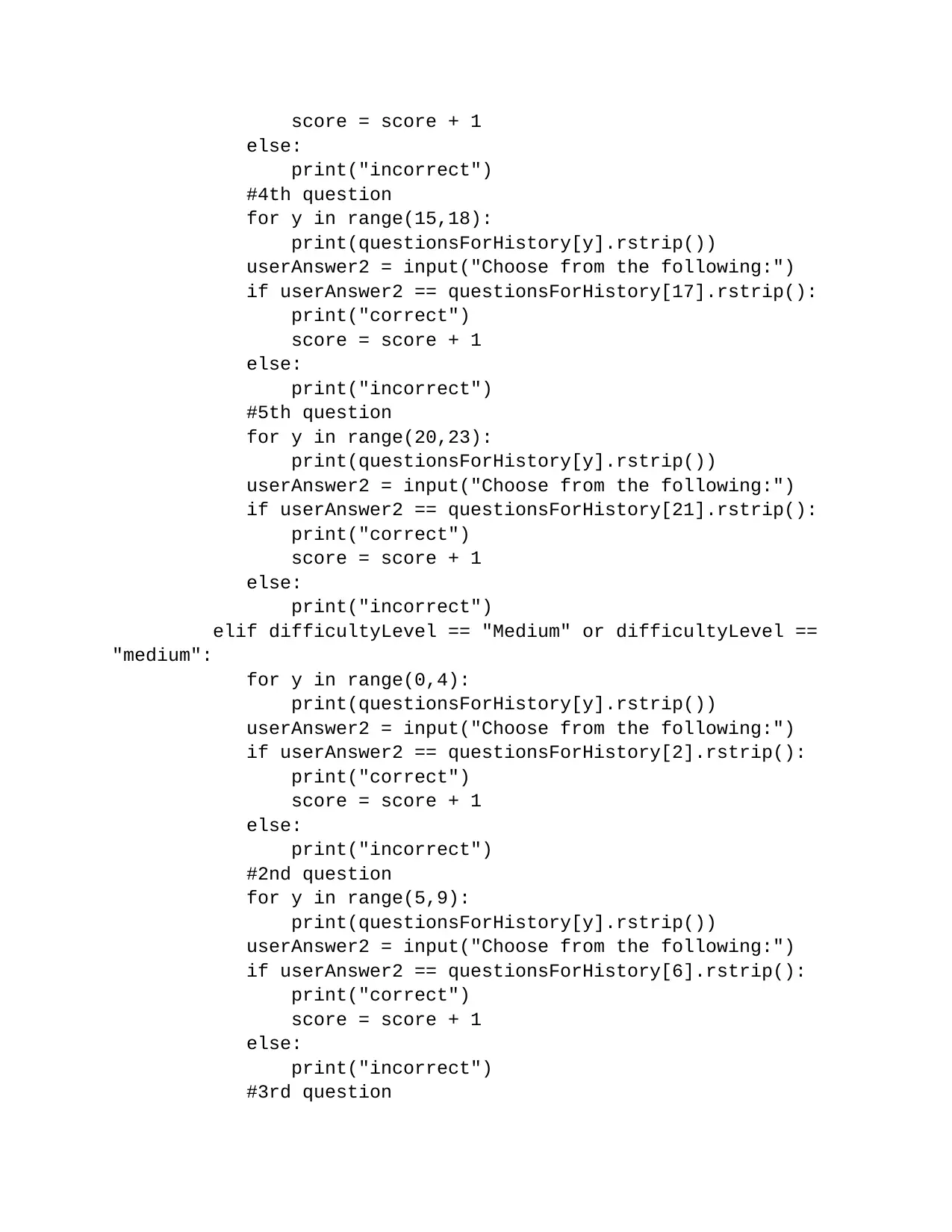
score = score + 1
else:
print("incorrect")
#4th question
for y in range(15,18):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[17].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
#5th question
for y in range(20,23):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[21].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
elif difficultyLevel == "Medium" or difficultyLevel ==
"medium":
for y in range(0,4):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[2].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
#2nd question
for y in range(5,9):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[6].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
#3rd question
else:
print("incorrect")
#4th question
for y in range(15,18):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[17].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
#5th question
for y in range(20,23):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[21].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
elif difficultyLevel == "Medium" or difficultyLevel ==
"medium":
for y in range(0,4):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[2].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
#2nd question
for y in range(5,9):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[6].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
#3rd question
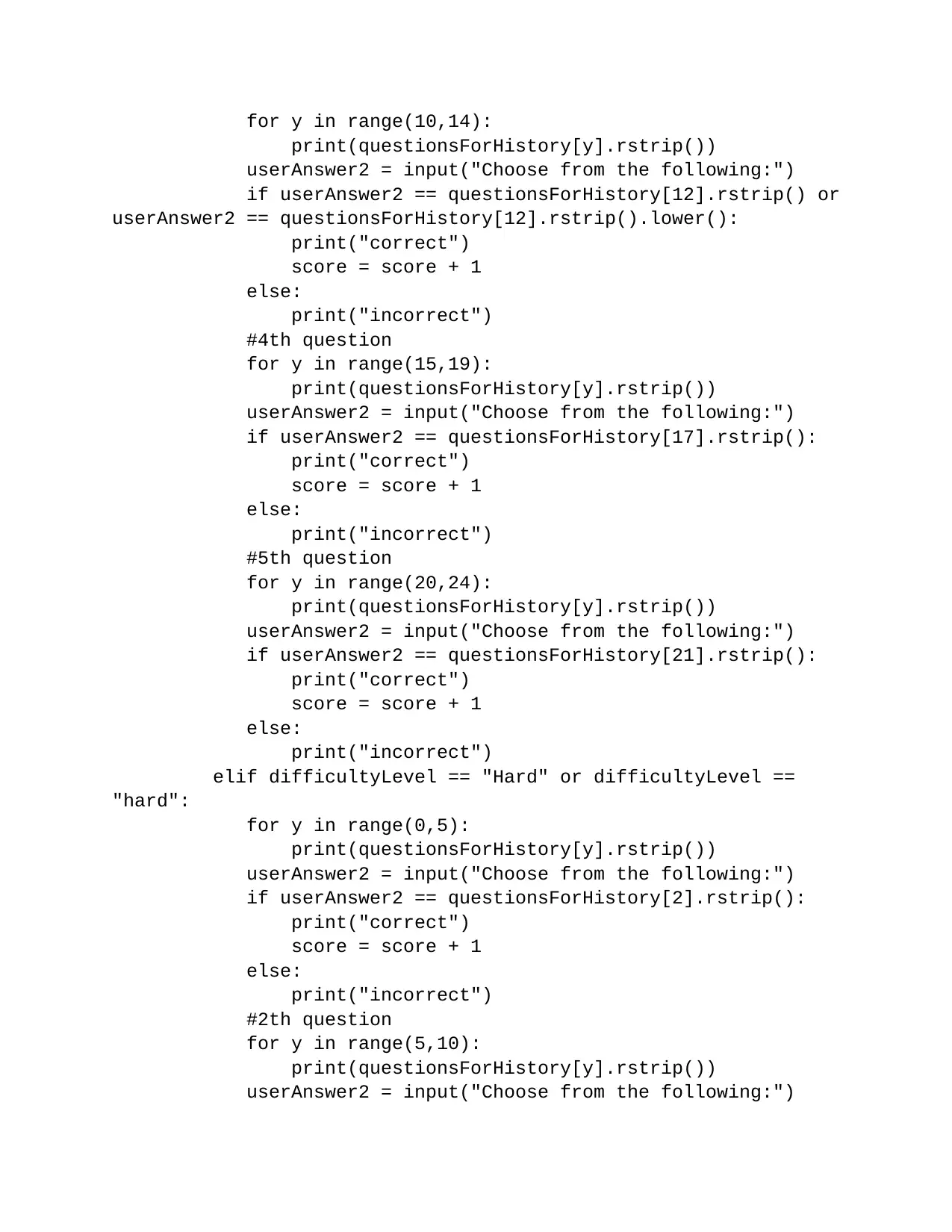
for y in range(10,14):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[12].rstrip() or
userAnswer2 == questionsForHistory[12].rstrip().lower():
print("correct")
score = score + 1
else:
print("incorrect")
#4th question
for y in range(15,19):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[17].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
#5th question
for y in range(20,24):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[21].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
elif difficultyLevel == "Hard" or difficultyLevel ==
"hard":
for y in range(0,5):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[2].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
#2th question
for y in range(5,10):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[12].rstrip() or
userAnswer2 == questionsForHistory[12].rstrip().lower():
print("correct")
score = score + 1
else:
print("incorrect")
#4th question
for y in range(15,19):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[17].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
#5th question
for y in range(20,24):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[21].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
elif difficultyLevel == "Hard" or difficultyLevel ==
"hard":
for y in range(0,5):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[2].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
#2th question
for y in range(5,10):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
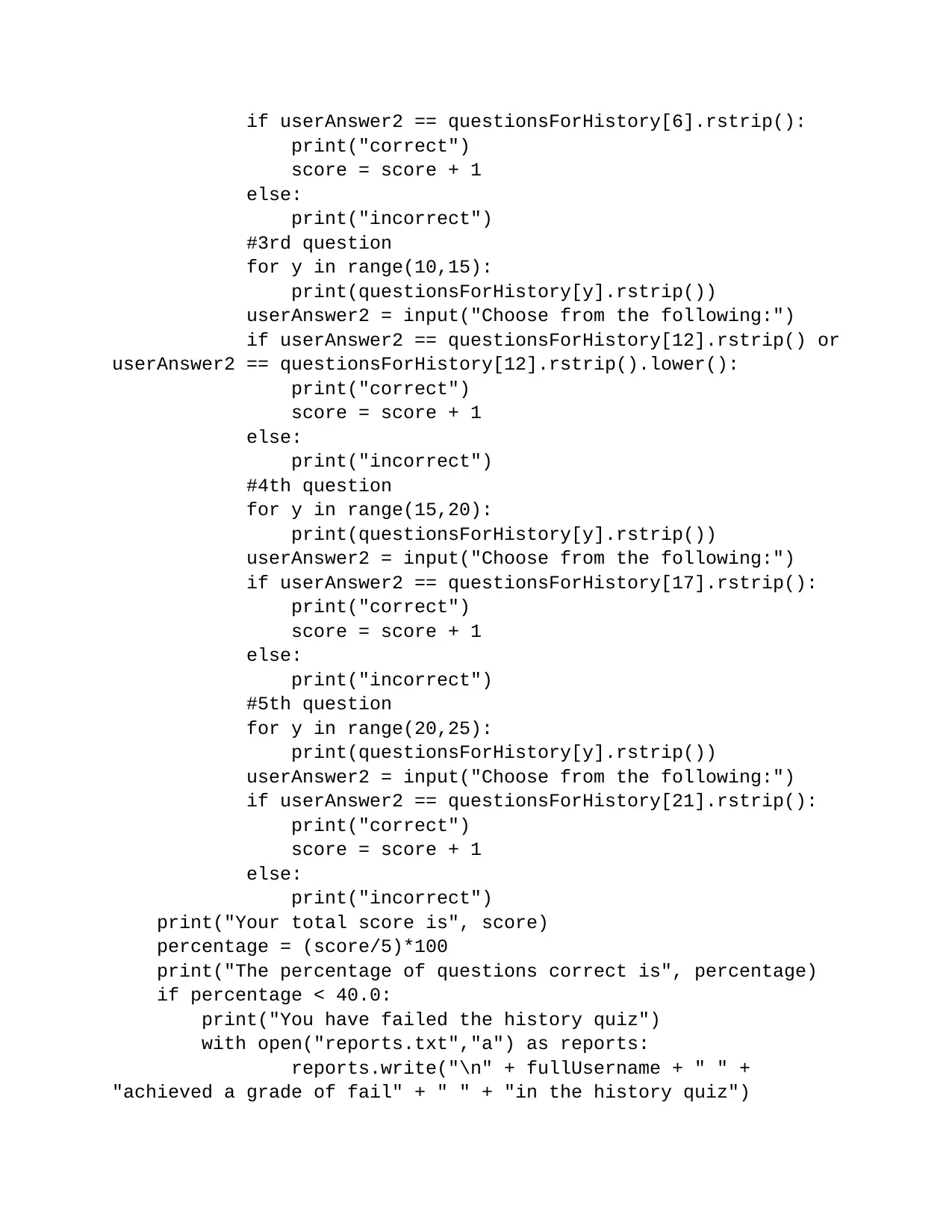
if userAnswer2 == questionsForHistory[6].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
#3rd question
for y in range(10,15):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[12].rstrip() or
userAnswer2 == questionsForHistory[12].rstrip().lower():
print("correct")
score = score + 1
else:
print("incorrect")
#4th question
for y in range(15,20):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[17].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
#5th question
for y in range(20,25):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[21].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
print("Your total score is", score)
percentage = (score/5)*100
print("The percentage of questions correct is", percentage)
if percentage < 40.0:
print("You have failed the history quiz")
with open("reports.txt","a") as reports:
reports.write("\n" + fullUsername + " " +
"achieved a grade of fail" + " " + "in the history quiz")
print("correct")
score = score + 1
else:
print("incorrect")
#3rd question
for y in range(10,15):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[12].rstrip() or
userAnswer2 == questionsForHistory[12].rstrip().lower():
print("correct")
score = score + 1
else:
print("incorrect")
#4th question
for y in range(15,20):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[17].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
#5th question
for y in range(20,25):
print(questionsForHistory[y].rstrip())
userAnswer2 = input("Choose from the following:")
if userAnswer2 == questionsForHistory[21].rstrip():
print("correct")
score = score + 1
else:
print("incorrect")
print("Your total score is", score)
percentage = (score/5)*100
print("The percentage of questions correct is", percentage)
if percentage < 40.0:
print("You have failed the history quiz")
with open("reports.txt","a") as reports:
reports.write("\n" + fullUsername + " " +
"achieved a grade of fail" + " " + "in the history quiz")
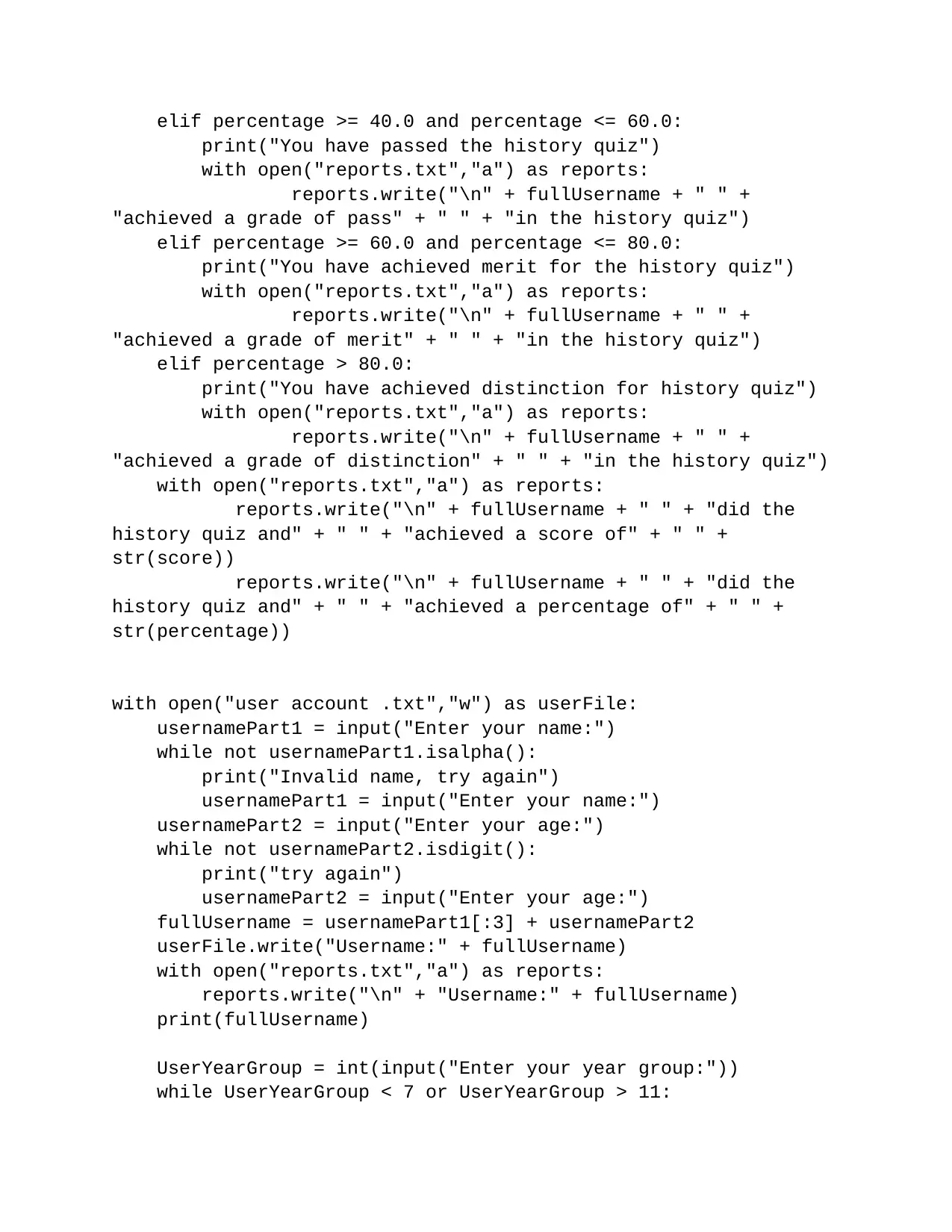
elif percentage >= 40.0 and percentage <= 60.0:
print("You have passed the history quiz")
with open("reports.txt","a") as reports:
reports.write("\n" + fullUsername + " " +
"achieved a grade of pass" + " " + "in the history quiz")
elif percentage >= 60.0 and percentage <= 80.0:
print("You have achieved merit for the history quiz")
with open("reports.txt","a") as reports:
reports.write("\n" + fullUsername + " " +
"achieved a grade of merit" + " " + "in the history quiz")
elif percentage > 80.0:
print("You have achieved distinction for history quiz")
with open("reports.txt","a") as reports:
reports.write("\n" + fullUsername + " " +
"achieved a grade of distinction" + " " + "in the history quiz")
with open("reports.txt","a") as reports:
reports.write("\n" + fullUsername + " " + "did the
history quiz and" + " " + "achieved a score of" + " " +
str(score))
reports.write("\n" + fullUsername + " " + "did the
history quiz and" + " " + "achieved a percentage of" + " " +
str(percentage))
with open("user account .txt","w") as userFile:
usernamePart1 = input("Enter your name:")
while not usernamePart1.isalpha():
print("Invalid name, try again")
usernamePart1 = input("Enter your name:")
usernamePart2 = input("Enter your age:")
while not usernamePart2.isdigit():
print("try again")
usernamePart2 = input("Enter your age:")
fullUsername = usernamePart1[:3] + usernamePart2
userFile.write("Username:" + fullUsername)
with open("reports.txt","a") as reports:
reports.write("\n" + "Username:" + fullUsername)
print(fullUsername)
UserYearGroup = int(input("Enter your year group:"))
while UserYearGroup < 7 or UserYearGroup > 11:
print("You have passed the history quiz")
with open("reports.txt","a") as reports:
reports.write("\n" + fullUsername + " " +
"achieved a grade of pass" + " " + "in the history quiz")
elif percentage >= 60.0 and percentage <= 80.0:
print("You have achieved merit for the history quiz")
with open("reports.txt","a") as reports:
reports.write("\n" + fullUsername + " " +
"achieved a grade of merit" + " " + "in the history quiz")
elif percentage > 80.0:
print("You have achieved distinction for history quiz")
with open("reports.txt","a") as reports:
reports.write("\n" + fullUsername + " " +
"achieved a grade of distinction" + " " + "in the history quiz")
with open("reports.txt","a") as reports:
reports.write("\n" + fullUsername + " " + "did the
history quiz and" + " " + "achieved a score of" + " " +
str(score))
reports.write("\n" + fullUsername + " " + "did the
history quiz and" + " " + "achieved a percentage of" + " " +
str(percentage))
with open("user account .txt","w") as userFile:
usernamePart1 = input("Enter your name:")
while not usernamePart1.isalpha():
print("Invalid name, try again")
usernamePart1 = input("Enter your name:")
usernamePart2 = input("Enter your age:")
while not usernamePart2.isdigit():
print("try again")
usernamePart2 = input("Enter your age:")
fullUsername = usernamePart1[:3] + usernamePart2
userFile.write("Username:" + fullUsername)
with open("reports.txt","a") as reports:
reports.write("\n" + "Username:" + fullUsername)
print(fullUsername)
UserYearGroup = int(input("Enter your year group:"))
while UserYearGroup < 7 or UserYearGroup > 11:
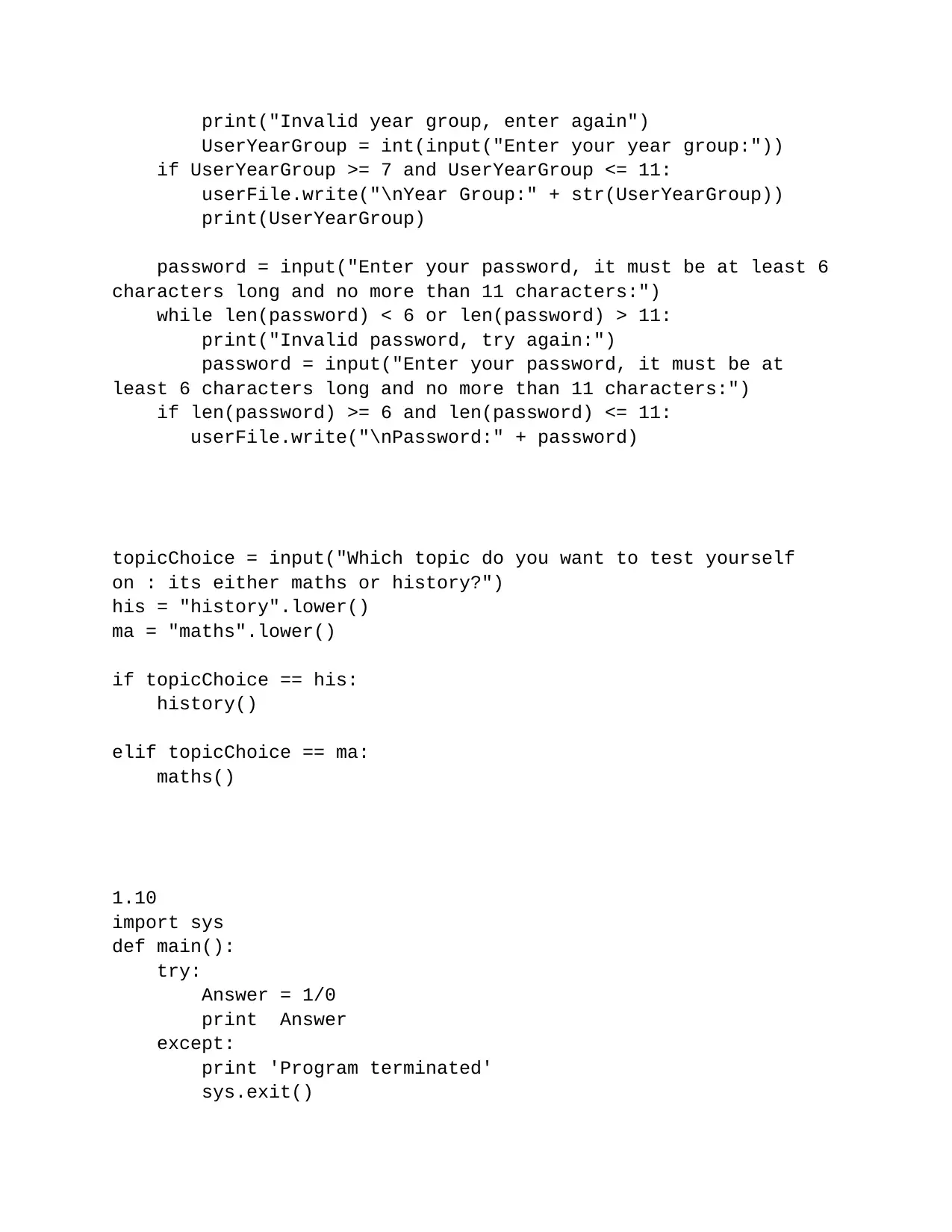
print("Invalid year group, enter again")
UserYearGroup = int(input("Enter your year group:"))
if UserYearGroup >= 7 and UserYearGroup <= 11:
userFile.write("\nYear Group:" + str(UserYearGroup))
print(UserYearGroup)
password = input("Enter your password, it must be at least 6
characters long and no more than 11 characters:")
while len(password) < 6 or len(password) > 11:
print("Invalid password, try again:")
password = input("Enter your password, it must be at
least 6 characters long and no more than 11 characters:")
if len(password) >= 6 and len(password) <= 11:
userFile.write("\nPassword:" + password)
topicChoice = input("Which topic do you want to test yourself
on : its either maths or history?")
his = "history".lower()
ma = "maths".lower()
if topicChoice == his:
history()
elif topicChoice == ma:
maths()
1.10
import sys
def main():
try:
Answer = 1/0
print Answer
except:
print 'Program terminated'
sys.exit()
UserYearGroup = int(input("Enter your year group:"))
if UserYearGroup >= 7 and UserYearGroup <= 11:
userFile.write("\nYear Group:" + str(UserYearGroup))
print(UserYearGroup)
password = input("Enter your password, it must be at least 6
characters long and no more than 11 characters:")
while len(password) < 6 or len(password) > 11:
print("Invalid password, try again:")
password = input("Enter your password, it must be at
least 6 characters long and no more than 11 characters:")
if len(password) >= 6 and len(password) <= 11:
userFile.write("\nPassword:" + password)
topicChoice = input("Which topic do you want to test yourself
on : its either maths or history?")
his = "history".lower()
ma = "maths".lower()
if topicChoice == his:
history()
elif topicChoice == ma:
maths()
1.10
import sys
def main():
try:
Answer = 1/0
print Answer
except:
print 'Program terminated'
sys.exit()
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
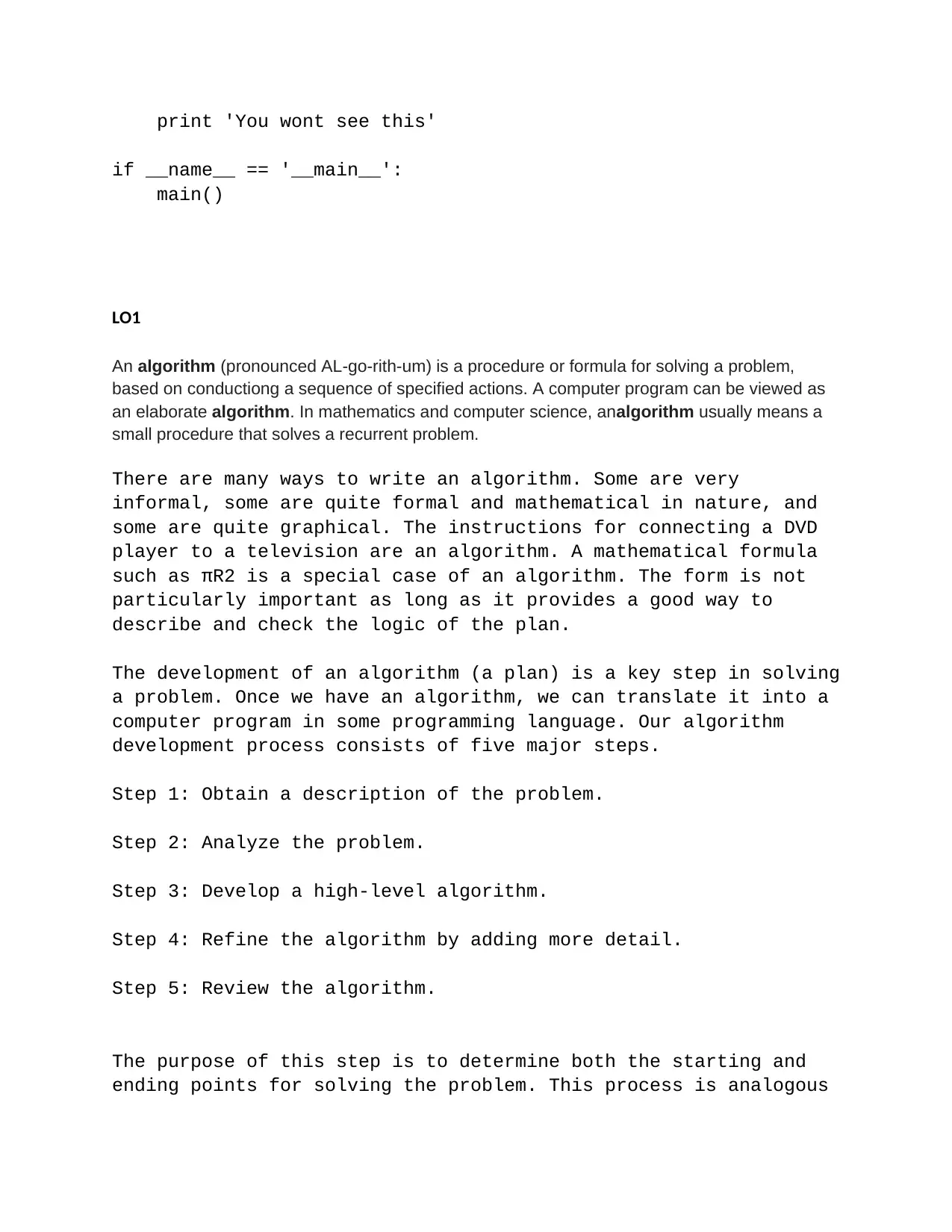
print 'You wont see this'
if __name__ == '__main__':
main()
LO1
An algorithm (pronounced AL-go-rith-um) is a procedure or formula for solving a problem,
based on conductiong a sequence of specified actions. A computer program can be viewed as
an elaborate algorithm. In mathematics and computer science, analgorithm usually means a
small procedure that solves a recurrent problem.
There are many ways to write an algorithm. Some are very
informal, some are quite formal and mathematical in nature, and
some are quite graphical. The instructions for connecting a DVD
player to a television are an algorithm. A mathematical formula
such as πR2 is a special case of an algorithm. The form is not
particularly important as long as it provides a good way to
describe and check the logic of the plan.
The development of an algorithm (a plan) is a key step in solving
a problem. Once we have an algorithm, we can translate it into a
computer program in some programming language. Our algorithm
development process consists of five major steps.
Step 1: Obtain a description of the problem.
Step 2: Analyze the problem.
Step 3: Develop a high-level algorithm.
Step 4: Refine the algorithm by adding more detail.
Step 5: Review the algorithm.
The purpose of this step is to determine both the starting and
ending points for solving the problem. This process is analogous
if __name__ == '__main__':
main()
LO1
An algorithm (pronounced AL-go-rith-um) is a procedure or formula for solving a problem,
based on conductiong a sequence of specified actions. A computer program can be viewed as
an elaborate algorithm. In mathematics and computer science, analgorithm usually means a
small procedure that solves a recurrent problem.
There are many ways to write an algorithm. Some are very
informal, some are quite formal and mathematical in nature, and
some are quite graphical. The instructions for connecting a DVD
player to a television are an algorithm. A mathematical formula
such as πR2 is a special case of an algorithm. The form is not
particularly important as long as it provides a good way to
describe and check the logic of the plan.
The development of an algorithm (a plan) is a key step in solving
a problem. Once we have an algorithm, we can translate it into a
computer program in some programming language. Our algorithm
development process consists of five major steps.
Step 1: Obtain a description of the problem.
Step 2: Analyze the problem.
Step 3: Develop a high-level algorithm.
Step 4: Refine the algorithm by adding more detail.
Step 5: Review the algorithm.
The purpose of this step is to determine both the starting and
ending points for solving the problem. This process is analogous
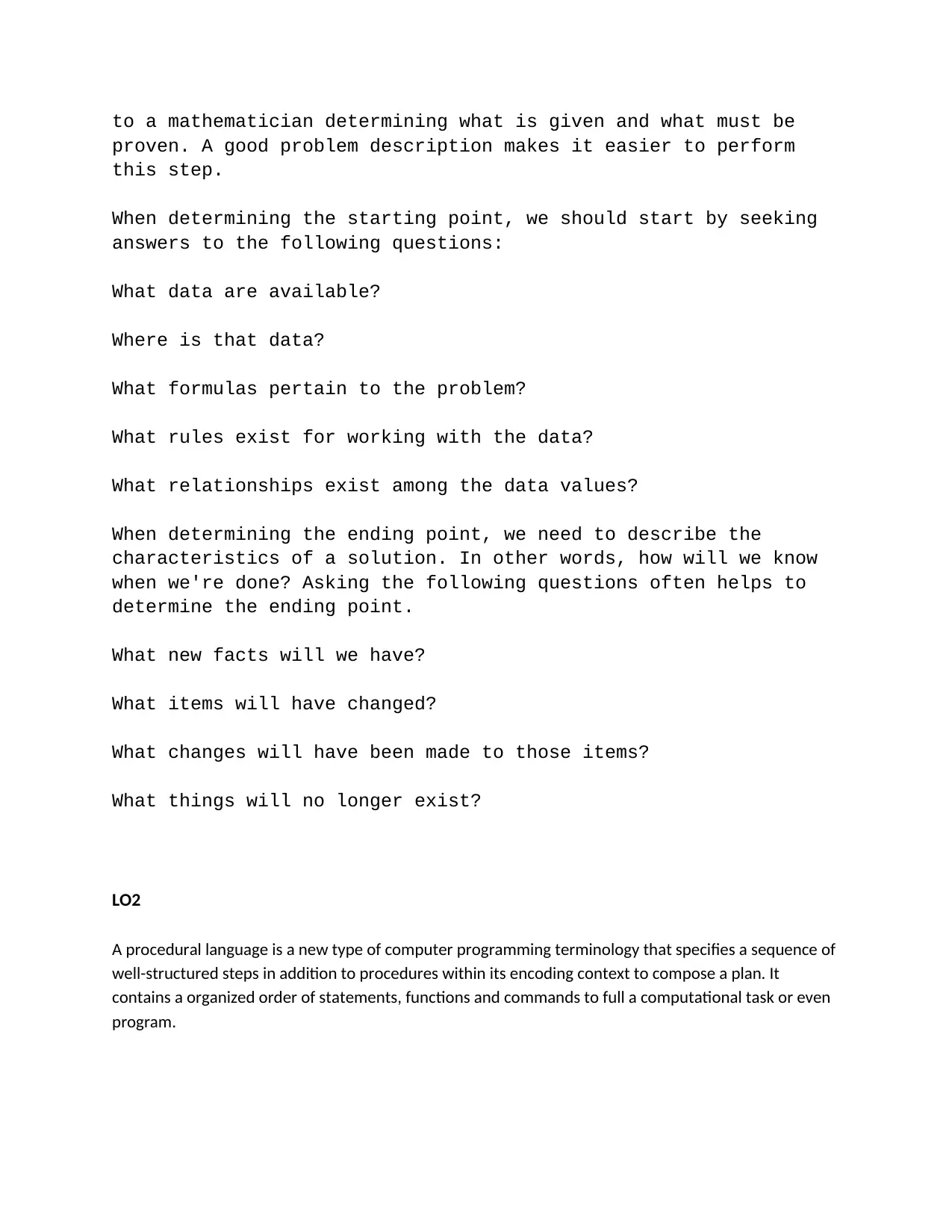
to a mathematician determining what is given and what must be
proven. A good problem description makes it easier to perform
this step.
When determining the starting point, we should start by seeking
answers to the following questions:
What data are available?
Where is that data?
What formulas pertain to the problem?
What rules exist for working with the data?
What relationships exist among the data values?
When determining the ending point, we need to describe the
characteristics of a solution. In other words, how will we know
when we're done? Asking the following questions often helps to
determine the ending point.
What new facts will we have?
What items will have changed?
What changes will have been made to those items?
What things will no longer exist?
LO2
A procedural language is a new type of computer programming terminology that specifies a sequence of
well-structured steps in addition to procedures within its encoding context to compose a plan. It
contains a organized order of statements, functions and commands to full a computational task or even
program.
proven. A good problem description makes it easier to perform
this step.
When determining the starting point, we should start by seeking
answers to the following questions:
What data are available?
Where is that data?
What formulas pertain to the problem?
What rules exist for working with the data?
What relationships exist among the data values?
When determining the ending point, we need to describe the
characteristics of a solution. In other words, how will we know
when we're done? Asking the following questions often helps to
determine the ending point.
What new facts will we have?
What items will have changed?
What changes will have been made to those items?
What things will no longer exist?
LO2
A procedural language is a new type of computer programming terminology that specifies a sequence of
well-structured steps in addition to procedures within its encoding context to compose a plan. It
contains a organized order of statements, functions and commands to full a computational task or even
program.
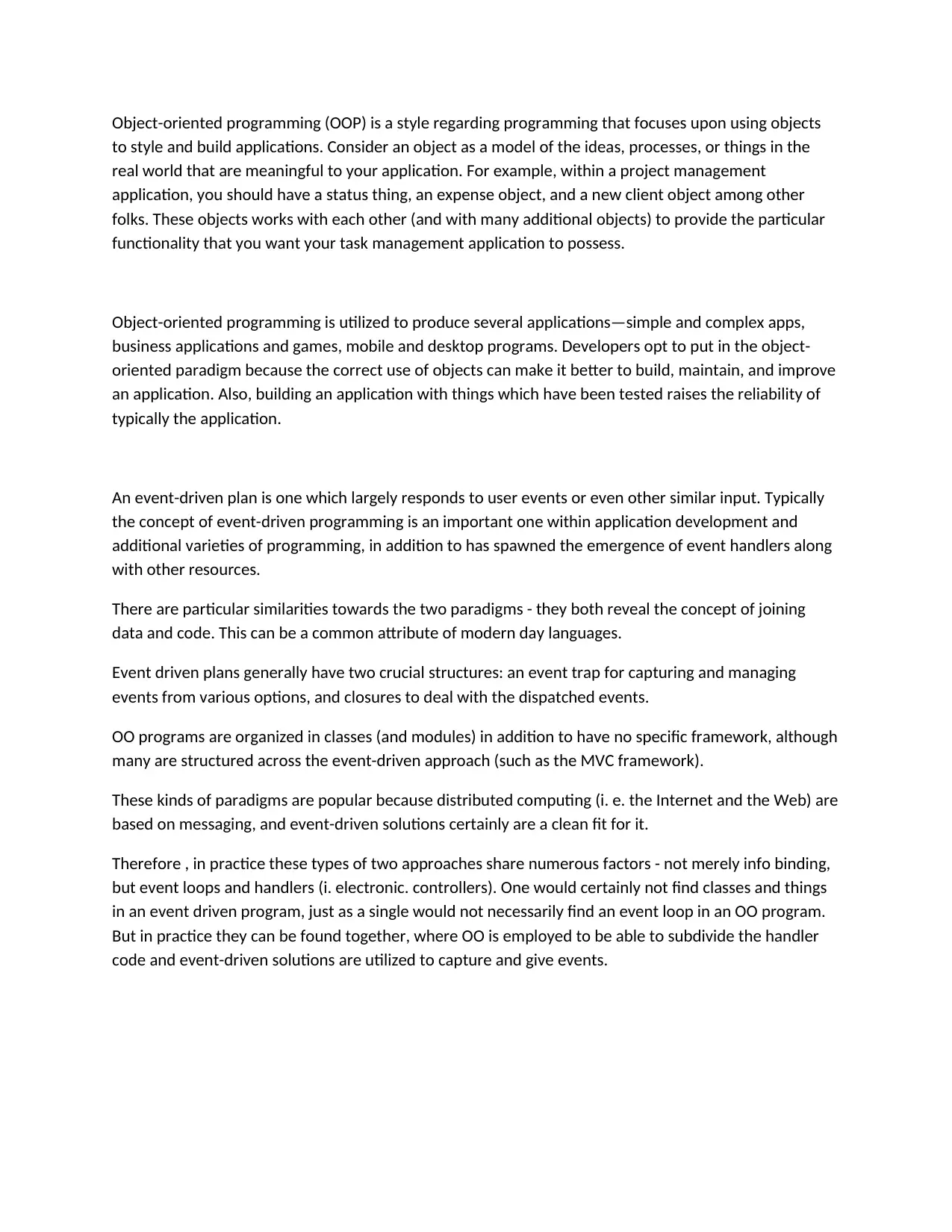
Object-oriented programming (OOP) is a style regarding programming that focuses upon using objects
to style and build applications. Consider an object as a model of the ideas, processes, or things in the
real world that are meaningful to your application. For example, within a project management
application, you should have a status thing, an expense object, and a new client object among other
folks. These objects works with each other (and with many additional objects) to provide the particular
functionality that you want your task management application to possess.
Object-oriented programming is utilized to produce several applications—simple and complex apps,
business applications and games, mobile and desktop programs. Developers opt to put in the object-
oriented paradigm because the correct use of objects can make it better to build, maintain, and improve
an application. Also, building an application with things which have been tested raises the reliability of
typically the application.
An event-driven plan is one which largely responds to user events or even other similar input. Typically
the concept of event-driven programming is an important one within application development and
additional varieties of programming, in addition to has spawned the emergence of event handlers along
with other resources.
There are particular similarities towards the two paradigms - they both reveal the concept of joining
data and code. This can be a common attribute of modern day languages.
Event driven plans generally have two crucial structures: an event trap for capturing and managing
events from various options, and closures to deal with the dispatched events.
OO programs are organized in classes (and modules) in addition to have no specific framework, although
many are structured across the event-driven approach (such as the MVC framework).
These kinds of paradigms are popular because distributed computing (i. e. the Internet and the Web) are
based on messaging, and event-driven solutions certainly are a clean fit for it.
Therefore , in practice these types of two approaches share numerous factors - not merely info binding,
but event loops and handlers (i. electronic. controllers). One would certainly not find classes and things
in an event driven program, just as a single would not necessarily find an event loop in an OO program.
But in practice they can be found together, where OO is employed to be able to subdivide the handler
code and event-driven solutions are utilized to capture and give events.
to style and build applications. Consider an object as a model of the ideas, processes, or things in the
real world that are meaningful to your application. For example, within a project management
application, you should have a status thing, an expense object, and a new client object among other
folks. These objects works with each other (and with many additional objects) to provide the particular
functionality that you want your task management application to possess.
Object-oriented programming is utilized to produce several applications—simple and complex apps,
business applications and games, mobile and desktop programs. Developers opt to put in the object-
oriented paradigm because the correct use of objects can make it better to build, maintain, and improve
an application. Also, building an application with things which have been tested raises the reliability of
typically the application.
An event-driven plan is one which largely responds to user events or even other similar input. Typically
the concept of event-driven programming is an important one within application development and
additional varieties of programming, in addition to has spawned the emergence of event handlers along
with other resources.
There are particular similarities towards the two paradigms - they both reveal the concept of joining
data and code. This can be a common attribute of modern day languages.
Event driven plans generally have two crucial structures: an event trap for capturing and managing
events from various options, and closures to deal with the dispatched events.
OO programs are organized in classes (and modules) in addition to have no specific framework, although
many are structured across the event-driven approach (such as the MVC framework).
These kinds of paradigms are popular because distributed computing (i. e. the Internet and the Web) are
based on messaging, and event-driven solutions certainly are a clean fit for it.
Therefore , in practice these types of two approaches share numerous factors - not merely info binding,
but event loops and handlers (i. electronic. controllers). One would certainly not find classes and things
in an event driven program, just as a single would not necessarily find an event loop in an OO program.
But in practice they can be found together, where OO is employed to be able to subdivide the handler
code and event-driven solutions are utilized to capture and give events.
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
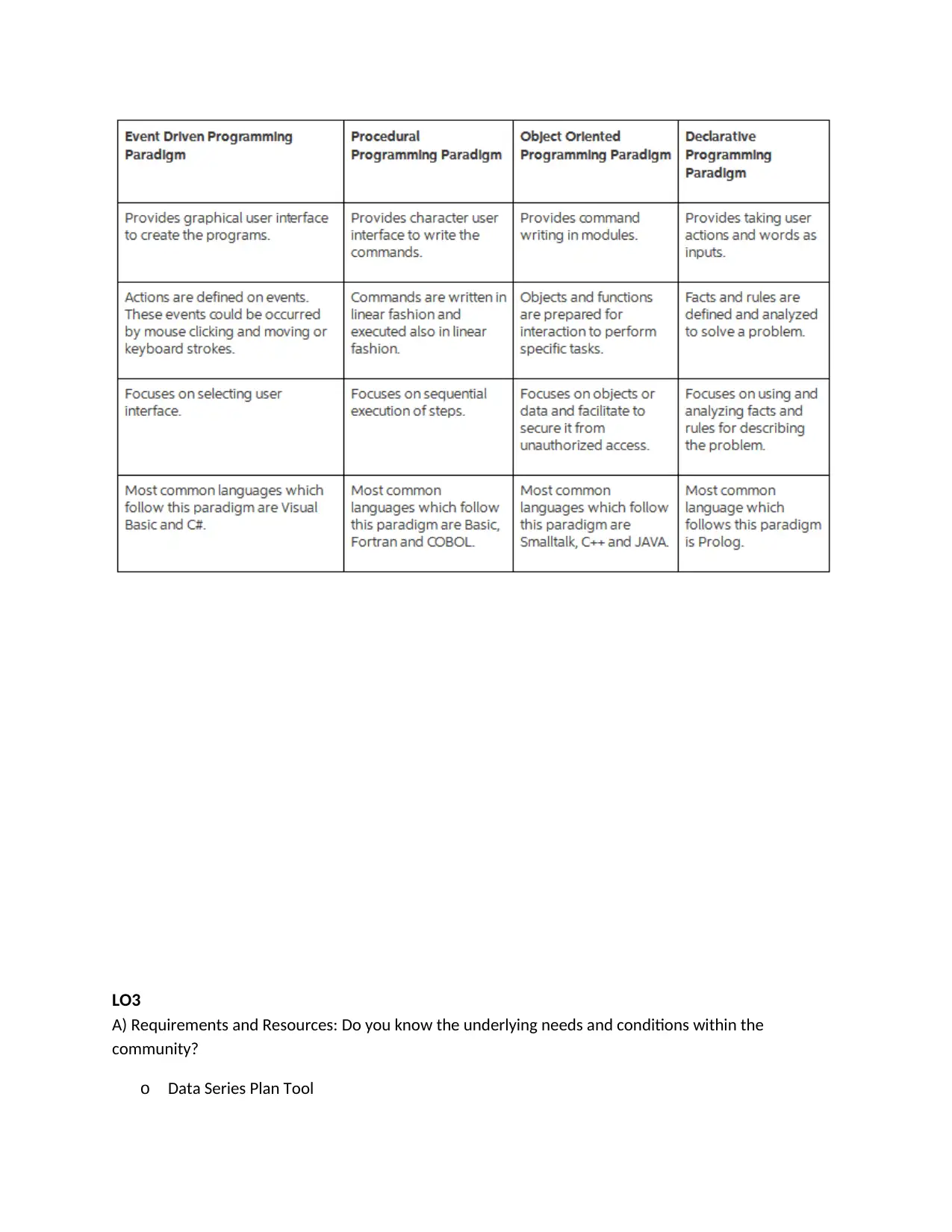
LO3
A) Requirements and Resources: Do you know the underlying needs and conditions within the
community?
o Data Series Plan Tool
A) Requirements and Resources: Do you know the underlying needs and conditions within the
community?
o Data Series Plan Tool
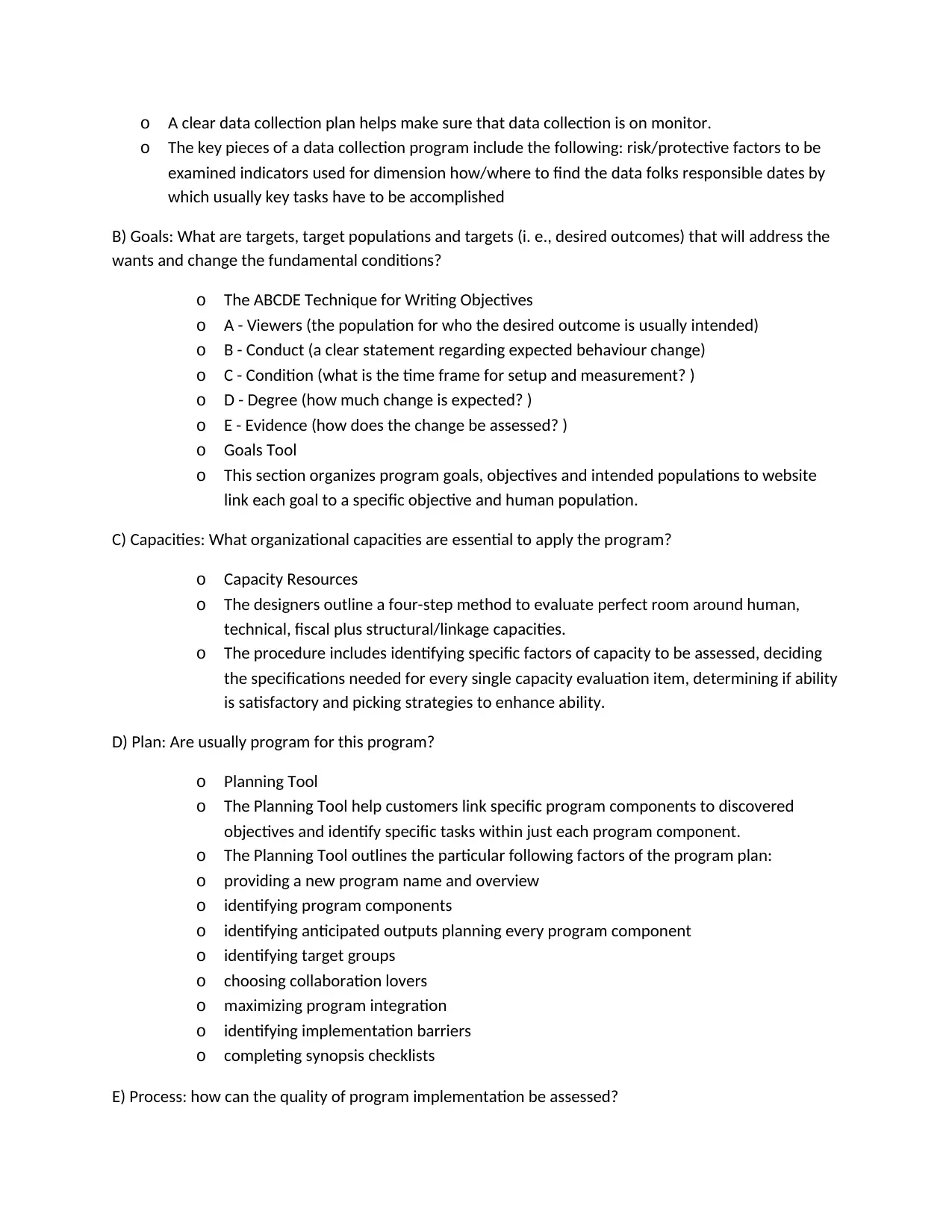
o A clear data collection plan helps make sure that data collection is on monitor.
o The key pieces of a data collection program include the following: risk/protective factors to be
examined indicators used for dimension how/where to find the data folks responsible dates by
which usually key tasks have to be accomplished
B) Goals: What are targets, target populations and targets (i. e., desired outcomes) that will address the
wants and change the fundamental conditions?
o The ABCDE Technique for Writing Objectives
o A - Viewers (the population for who the desired outcome is usually intended)
o B - Conduct (a clear statement regarding expected behaviour change)
o C - Condition (what is the time frame for setup and measurement? )
o D - Degree (how much change is expected? )
o E - Evidence (how does the change be assessed? )
o Goals Tool
o This section organizes program goals, objectives and intended populations to website
link each goal to a specific objective and human population.
C) Capacities: What organizational capacities are essential to apply the program?
o Capacity Resources
o The designers outline a four-step method to evaluate perfect room around human,
technical, fiscal plus structural/linkage capacities.
o The procedure includes identifying specific factors of capacity to be assessed, deciding
the specifications needed for every single capacity evaluation item, determining if ability
is satisfactory and picking strategies to enhance ability.
D) Plan: Are usually program for this program?
o Planning Tool
o The Planning Tool help customers link specific program components to discovered
objectives and identify specific tasks within just each program component.
o The Planning Tool outlines the particular following factors of the program plan:
o providing a new program name and overview
o identifying program components
o identifying anticipated outputs planning every program component
o identifying target groups
o choosing collaboration lovers
o maximizing program integration
o identifying implementation barriers
o completing synopsis checklists
E) Process: how can the quality of program implementation be assessed?
o The key pieces of a data collection program include the following: risk/protective factors to be
examined indicators used for dimension how/where to find the data folks responsible dates by
which usually key tasks have to be accomplished
B) Goals: What are targets, target populations and targets (i. e., desired outcomes) that will address the
wants and change the fundamental conditions?
o The ABCDE Technique for Writing Objectives
o A - Viewers (the population for who the desired outcome is usually intended)
o B - Conduct (a clear statement regarding expected behaviour change)
o C - Condition (what is the time frame for setup and measurement? )
o D - Degree (how much change is expected? )
o E - Evidence (how does the change be assessed? )
o Goals Tool
o This section organizes program goals, objectives and intended populations to website
link each goal to a specific objective and human population.
C) Capacities: What organizational capacities are essential to apply the program?
o Capacity Resources
o The designers outline a four-step method to evaluate perfect room around human,
technical, fiscal plus structural/linkage capacities.
o The procedure includes identifying specific factors of capacity to be assessed, deciding
the specifications needed for every single capacity evaluation item, determining if ability
is satisfactory and picking strategies to enhance ability.
D) Plan: Are usually program for this program?
o Planning Tool
o The Planning Tool help customers link specific program components to discovered
objectives and identify specific tasks within just each program component.
o The Planning Tool outlines the particular following factors of the program plan:
o providing a new program name and overview
o identifying program components
o identifying anticipated outputs planning every program component
o identifying target groups
o choosing collaboration lovers
o maximizing program integration
o identifying implementation barriers
o completing synopsis checklists
E) Process: how can the quality of program implementation be assessed?
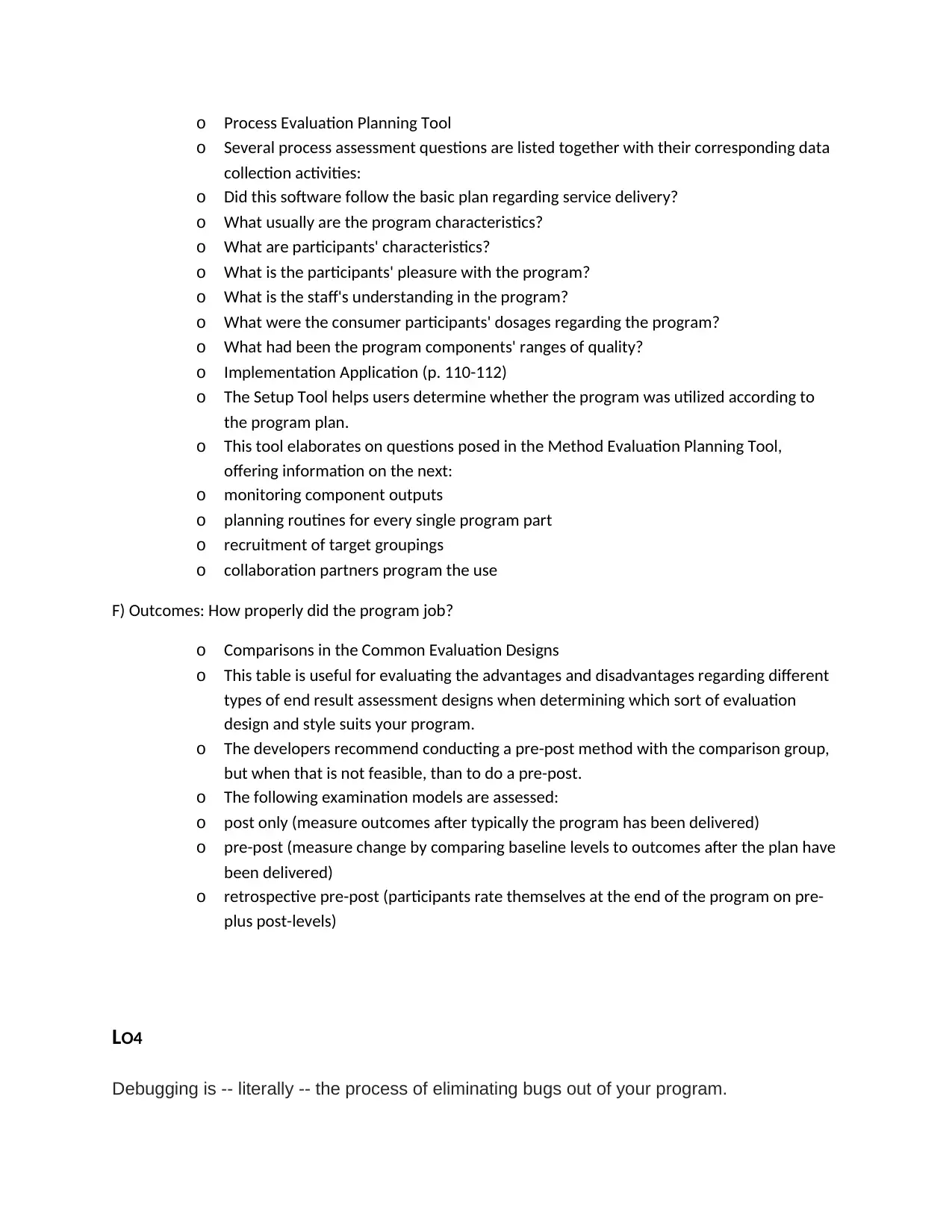
o Process Evaluation Planning Tool
o Several process assessment questions are listed together with their corresponding data
collection activities:
o Did this software follow the basic plan regarding service delivery?
o What usually are the program characteristics?
o What are participants' characteristics?
o What is the participants' pleasure with the program?
o What is the staff's understanding in the program?
o What were the consumer participants' dosages regarding the program?
o What had been the program components' ranges of quality?
o Implementation Application (p. 110-112)
o The Setup Tool helps users determine whether the program was utilized according to
the program plan.
o This tool elaborates on questions posed in the Method Evaluation Planning Tool,
offering information on the next:
o monitoring component outputs
o planning routines for every single program part
o recruitment of target groupings
o collaboration partners program the use
F) Outcomes: How properly did the program job?
o Comparisons in the Common Evaluation Designs
o This table is useful for evaluating the advantages and disadvantages regarding different
types of end result assessment designs when determining which sort of evaluation
design and style suits your program.
o The developers recommend conducting a pre-post method with the comparison group,
but when that is not feasible, than to do a pre-post.
o The following examination models are assessed:
o post only (measure outcomes after typically the program has been delivered)
o pre-post (measure change by comparing baseline levels to outcomes after the plan have
been delivered)
o retrospective pre-post (participants rate themselves at the end of the program on pre-
plus post-levels)
LO4
Debugging is -- literally -- the process of eliminating bugs out of your program.
o Several process assessment questions are listed together with their corresponding data
collection activities:
o Did this software follow the basic plan regarding service delivery?
o What usually are the program characteristics?
o What are participants' characteristics?
o What is the participants' pleasure with the program?
o What is the staff's understanding in the program?
o What were the consumer participants' dosages regarding the program?
o What had been the program components' ranges of quality?
o Implementation Application (p. 110-112)
o The Setup Tool helps users determine whether the program was utilized according to
the program plan.
o This tool elaborates on questions posed in the Method Evaluation Planning Tool,
offering information on the next:
o monitoring component outputs
o planning routines for every single program part
o recruitment of target groupings
o collaboration partners program the use
F) Outcomes: How properly did the program job?
o Comparisons in the Common Evaluation Designs
o This table is useful for evaluating the advantages and disadvantages regarding different
types of end result assessment designs when determining which sort of evaluation
design and style suits your program.
o The developers recommend conducting a pre-post method with the comparison group,
but when that is not feasible, than to do a pre-post.
o The following examination models are assessed:
o post only (measure outcomes after typically the program has been delivered)
o pre-post (measure change by comparing baseline levels to outcomes after the plan have
been delivered)
o retrospective pre-post (participants rate themselves at the end of the program on pre-
plus post-levels)
LO4
Debugging is -- literally -- the process of eliminating bugs out of your program.
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
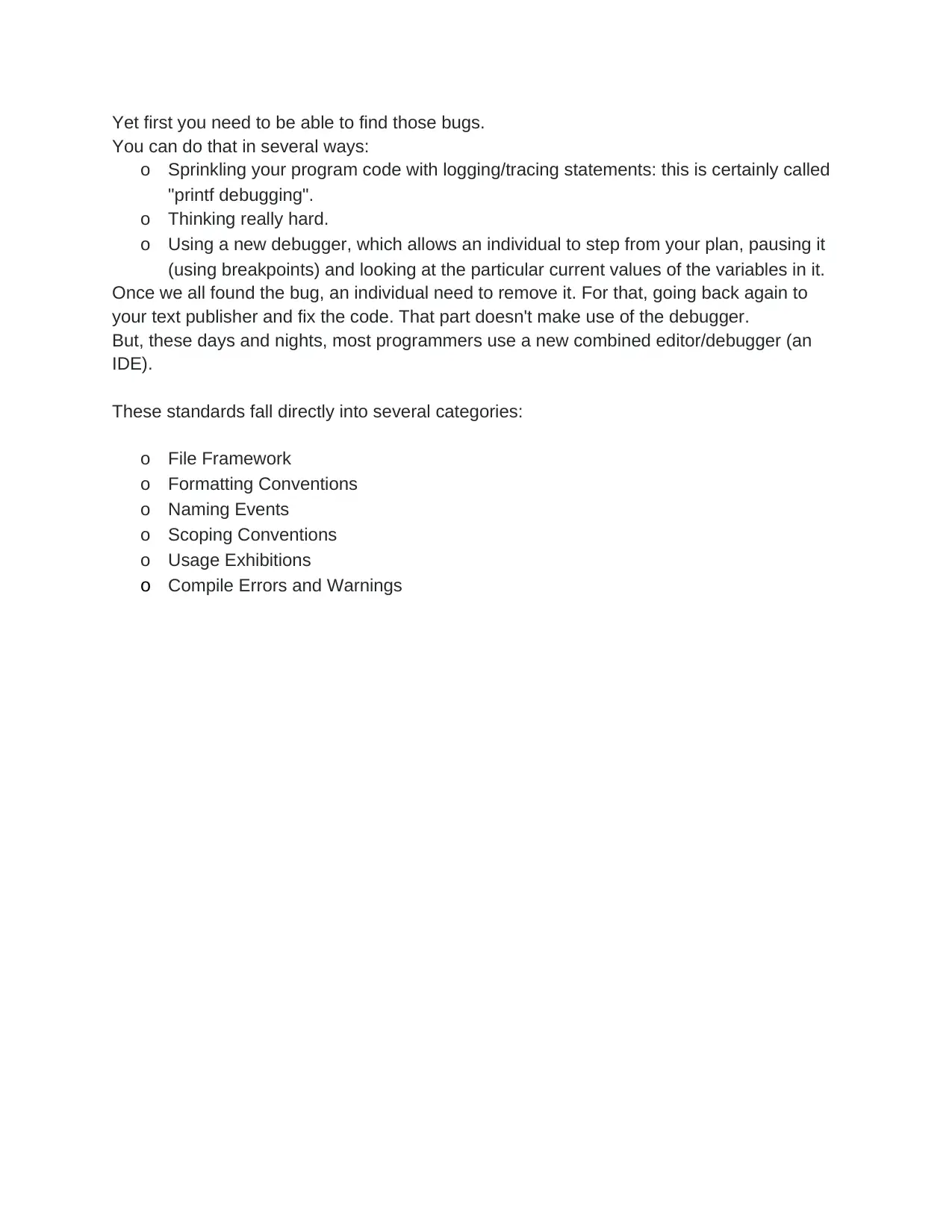
Yet first you need to be able to find those bugs.
You can do that in several ways:
o Sprinkling your program code with logging/tracing statements: this is certainly called
"printf debugging".
o Thinking really hard.
o Using a new debugger, which allows an individual to step from your plan, pausing it
(using breakpoints) and looking at the particular current values of the variables in it.
Once we all found the bug, an individual need to remove it. For that, going back again to
your text publisher and fix the code. That part doesn't make use of the debugger.
But, these days and nights, most programmers use a new combined editor/debugger (an
IDE).
These standards fall directly into several categories:
o File Framework
o Formatting Conventions
o Naming Events
o Scoping Conventions
o Usage Exhibitions
o Compile Errors and Warnings
You can do that in several ways:
o Sprinkling your program code with logging/tracing statements: this is certainly called
"printf debugging".
o Thinking really hard.
o Using a new debugger, which allows an individual to step from your plan, pausing it
(using breakpoints) and looking at the particular current values of the variables in it.
Once we all found the bug, an individual need to remove it. For that, going back again to
your text publisher and fix the code. That part doesn't make use of the debugger.
But, these days and nights, most programmers use a new combined editor/debugger (an
IDE).
These standards fall directly into several categories:
o File Framework
o Formatting Conventions
o Naming Events
o Scoping Conventions
o Usage Exhibitions
o Compile Errors and Warnings
1 out of 29
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
 +13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024  |  Zucol Services PVT LTD  |  All rights reserved.