Code Convention Standards and Programming Ethics
VerifiedAdded on 2020/05/04
|27
|4937
|106
AI Summary
This assignment delves into the significance of adhering to coding standards within software development. It emphasizes the benefits of using descriptive names for variables, functions, and classes, as well as the importance of clear comments for enhancing code readability and collaboration. The document also discusses the ethical implications of following coding conventions, promoting a culture of professionalism and maintainability in software projects.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
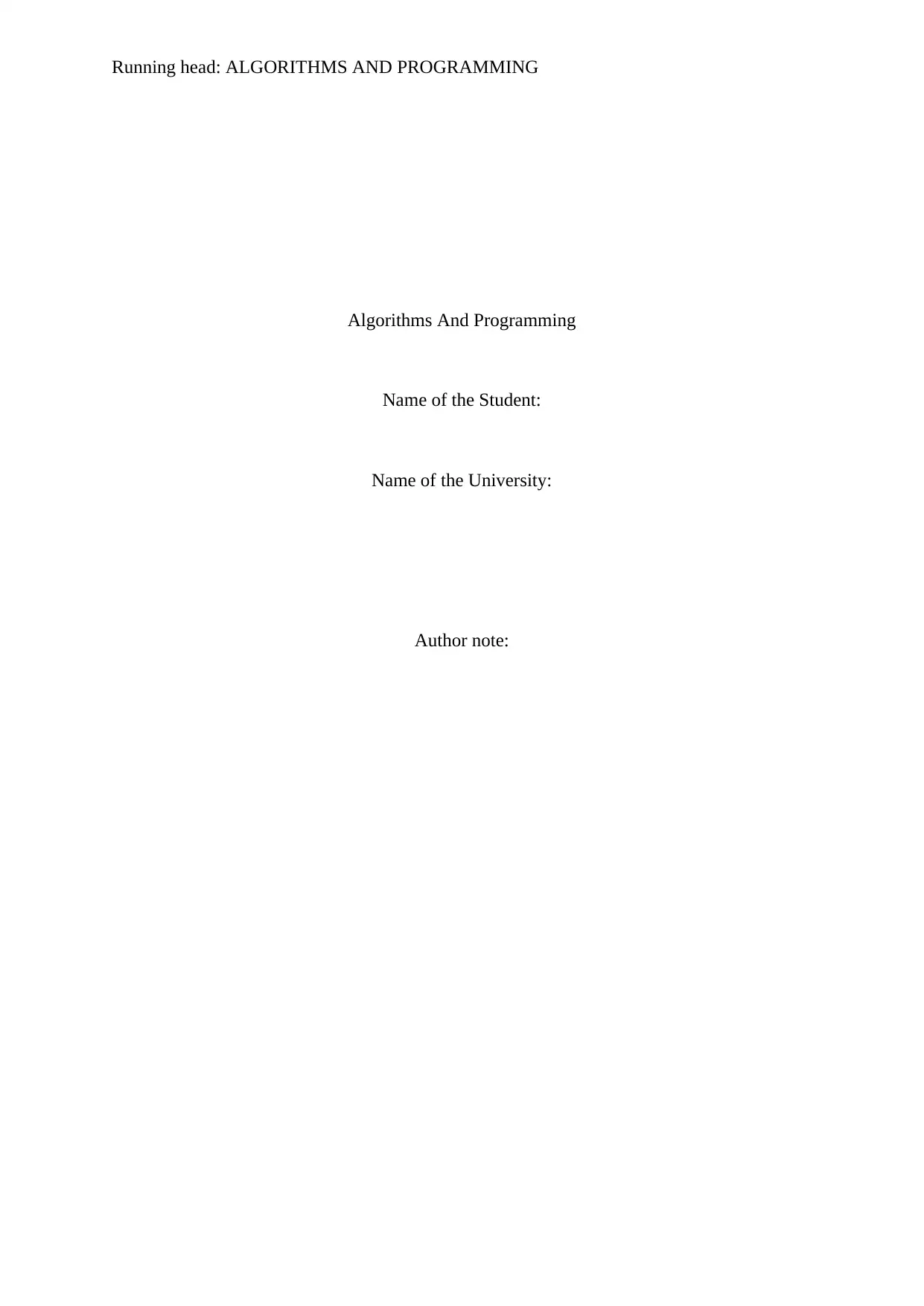
Running head: ALGORITHMS AND PROGRAMMING
Algorithms And Programming
Name of the Student:
Name of the University:
Author note:
Algorithms And Programming
Name of the Student:
Name of the University:
Author note:
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
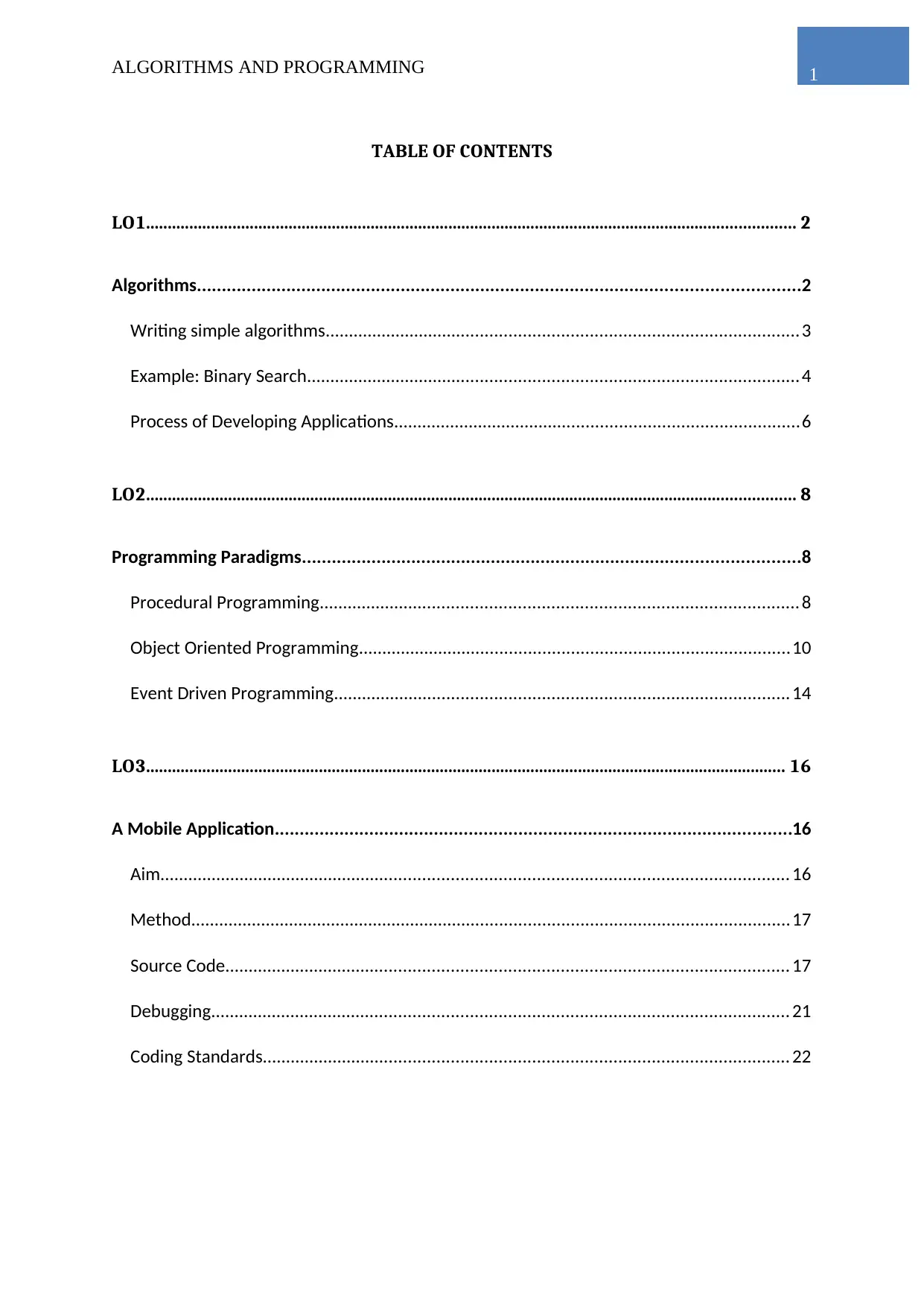
1ALGORITHMS AND PROGRAMMING
TABLE OF CONTENTS
LO1...................................................................................................................................................... 2
Algorithms.........................................................................................................................2
Writing simple algorithms....................................................................................................3
Example: Binary Search........................................................................................................4
Process of Developing Applications......................................................................................6
LO2...................................................................................................................................................... 8
Programming Paradigms....................................................................................................8
Procedural Programming..................................................................................................... 8
Object Oriented Programming...........................................................................................10
Event Driven Programming................................................................................................ 14
LO3.................................................................................................................................................... 16
A Mobile Application........................................................................................................16
Aim..................................................................................................................................... 16
Method...............................................................................................................................17
Source Code....................................................................................................................... 17
Debugging.......................................................................................................................... 21
Coding Standards............................................................................................................... 22
TABLE OF CONTENTS
LO1...................................................................................................................................................... 2
Algorithms.........................................................................................................................2
Writing simple algorithms....................................................................................................3
Example: Binary Search........................................................................................................4
Process of Developing Applications......................................................................................6
LO2...................................................................................................................................................... 8
Programming Paradigms....................................................................................................8
Procedural Programming..................................................................................................... 8
Object Oriented Programming...........................................................................................10
Event Driven Programming................................................................................................ 14
LO3.................................................................................................................................................... 16
A Mobile Application........................................................................................................16
Aim..................................................................................................................................... 16
Method...............................................................................................................................17
Source Code....................................................................................................................... 17
Debugging.......................................................................................................................... 21
Coding Standards............................................................................................................... 22
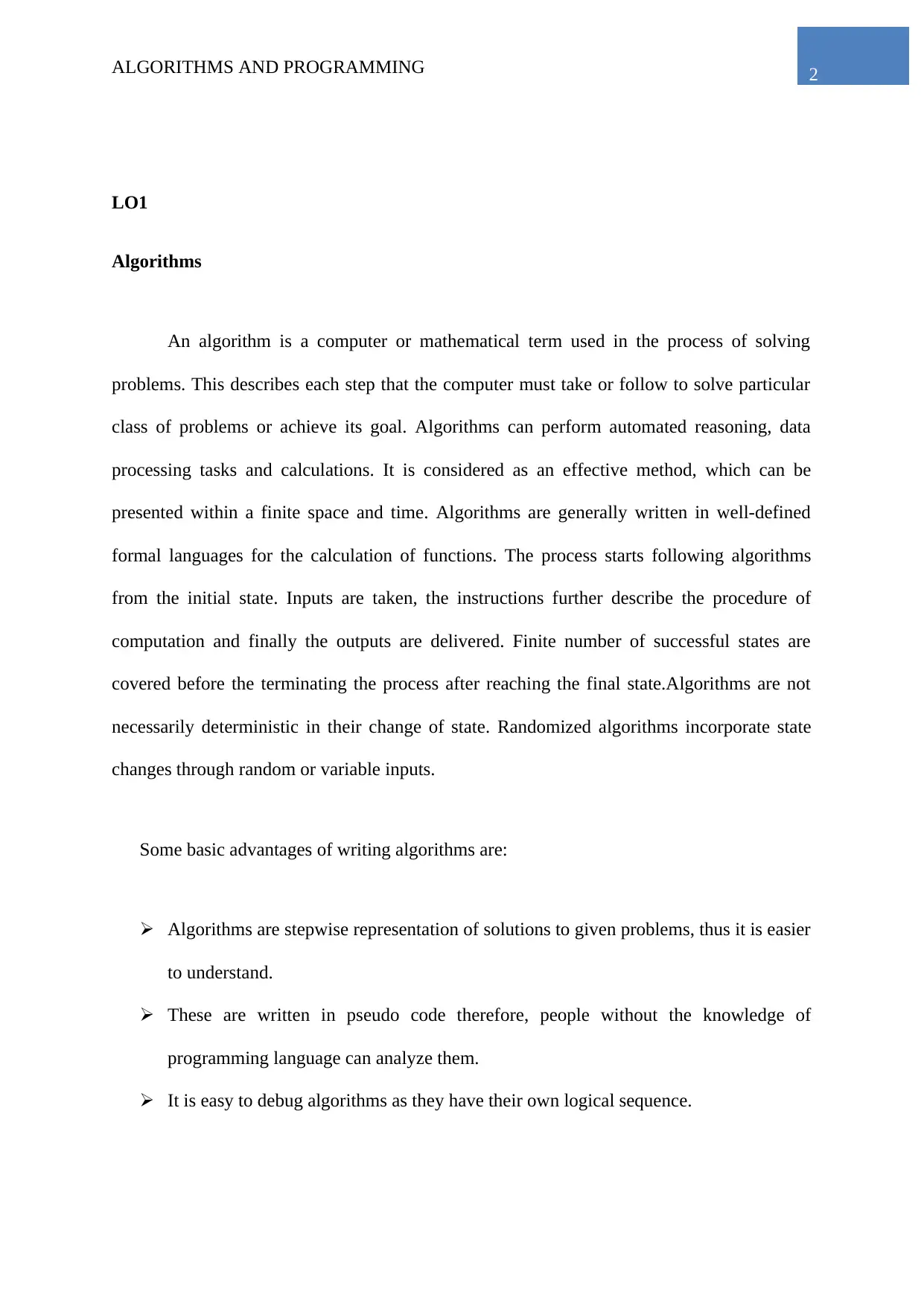
2ALGORITHMS AND PROGRAMMING
LO1
Algorithms
An algorithm is a computer or mathematical term used in the process of solving
problems. This describes each step that the computer must take or follow to solve particular
class of problems or achieve its goal. Algorithms can perform automated reasoning, data
processing tasks and calculations. It is considered as an effective method, which can be
presented within a finite space and time. Algorithms are generally written in well-defined
formal languages for the calculation of functions. The process starts following algorithms
from the initial state. Inputs are taken, the instructions further describe the procedure of
computation and finally the outputs are delivered. Finite number of successful states are
covered before the terminating the process after reaching the final state.Algorithms are not
necessarily deterministic in their change of state. Randomized algorithms incorporate state
changes through random or variable inputs.
Some basic advantages of writing algorithms are:
Algorithms are stepwise representation of solutions to given problems, thus it is easier
to understand.
These are written in pseudo code therefore, people without the knowledge of
programming language can analyze them.
It is easy to debug algorithms as they have their own logical sequence.
LO1
Algorithms
An algorithm is a computer or mathematical term used in the process of solving
problems. This describes each step that the computer must take or follow to solve particular
class of problems or achieve its goal. Algorithms can perform automated reasoning, data
processing tasks and calculations. It is considered as an effective method, which can be
presented within a finite space and time. Algorithms are generally written in well-defined
formal languages for the calculation of functions. The process starts following algorithms
from the initial state. Inputs are taken, the instructions further describe the procedure of
computation and finally the outputs are delivered. Finite number of successful states are
covered before the terminating the process after reaching the final state.Algorithms are not
necessarily deterministic in their change of state. Randomized algorithms incorporate state
changes through random or variable inputs.
Some basic advantages of writing algorithms are:
Algorithms are stepwise representation of solutions to given problems, thus it is easier
to understand.
These are written in pseudo code therefore, people without the knowledge of
programming language can analyze them.
It is easy to debug algorithms as they have their own logical sequence.
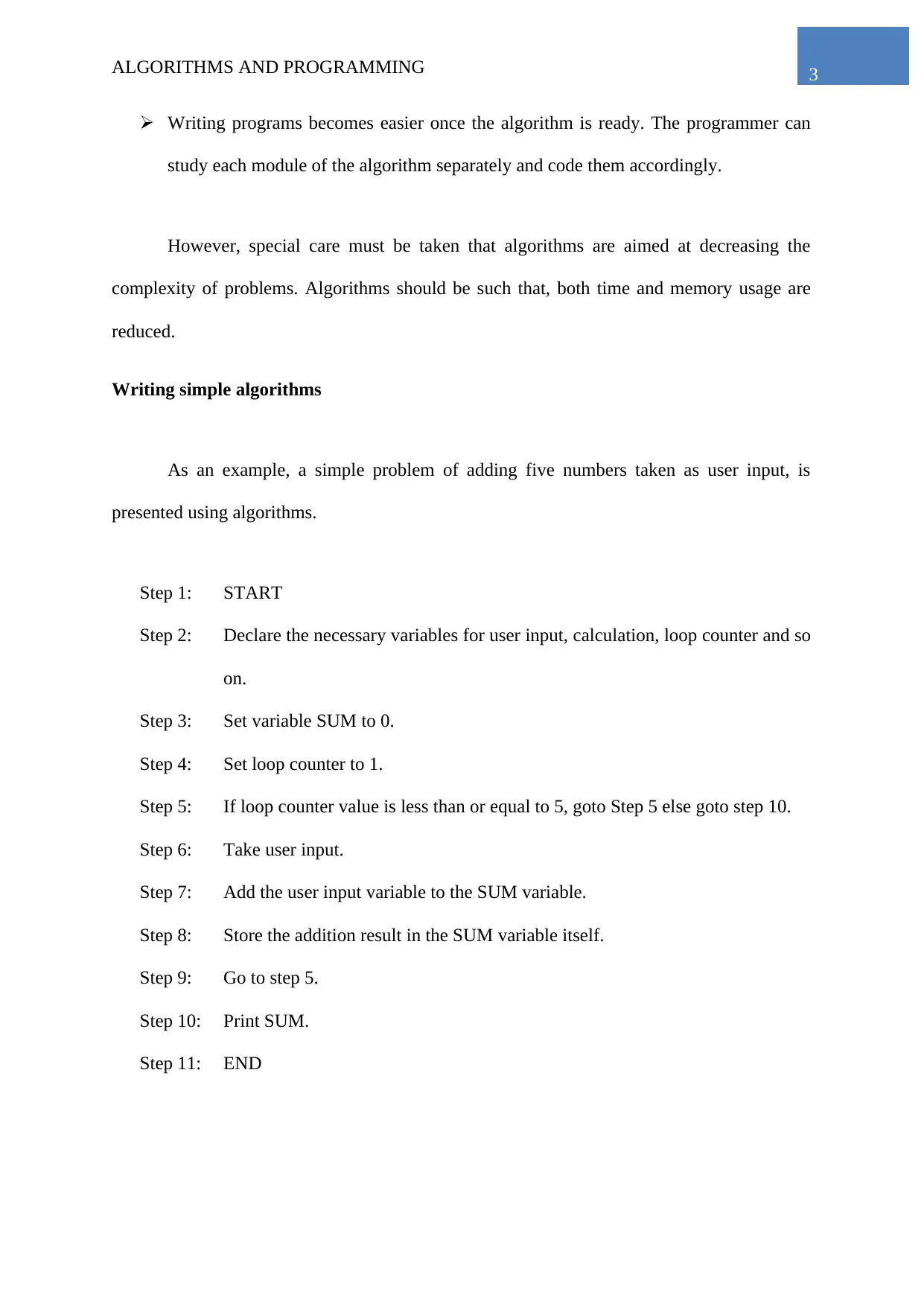
3ALGORITHMS AND PROGRAMMING
Writing programs becomes easier once the algorithm is ready. The programmer can
study each module of the algorithm separately and code them accordingly.
However, special care must be taken that algorithms are aimed at decreasing the
complexity of problems. Algorithms should be such that, both time and memory usage are
reduced.
Writing simple algorithms
As an example, a simple problem of adding five numbers taken as user input, is
presented using algorithms.
Step 1: START
Step 2: Declare the necessary variables for user input, calculation, loop counter and so
on.
Step 3: Set variable SUM to 0.
Step 4: Set loop counter to 1.
Step 5: If loop counter value is less than or equal to 5, goto Step 5 else goto step 10.
Step 6: Take user input.
Step 7: Add the user input variable to the SUM variable.
Step 8: Store the addition result in the SUM variable itself.
Step 9: Go to step 5.
Step 10: Print SUM.
Step 11: END
Writing programs becomes easier once the algorithm is ready. The programmer can
study each module of the algorithm separately and code them accordingly.
However, special care must be taken that algorithms are aimed at decreasing the
complexity of problems. Algorithms should be such that, both time and memory usage are
reduced.
Writing simple algorithms
As an example, a simple problem of adding five numbers taken as user input, is
presented using algorithms.
Step 1: START
Step 2: Declare the necessary variables for user input, calculation, loop counter and so
on.
Step 3: Set variable SUM to 0.
Step 4: Set loop counter to 1.
Step 5: If loop counter value is less than or equal to 5, goto Step 5 else goto step 10.
Step 6: Take user input.
Step 7: Add the user input variable to the SUM variable.
Step 8: Store the addition result in the SUM variable itself.
Step 9: Go to step 5.
Step 10: Print SUM.
Step 11: END
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
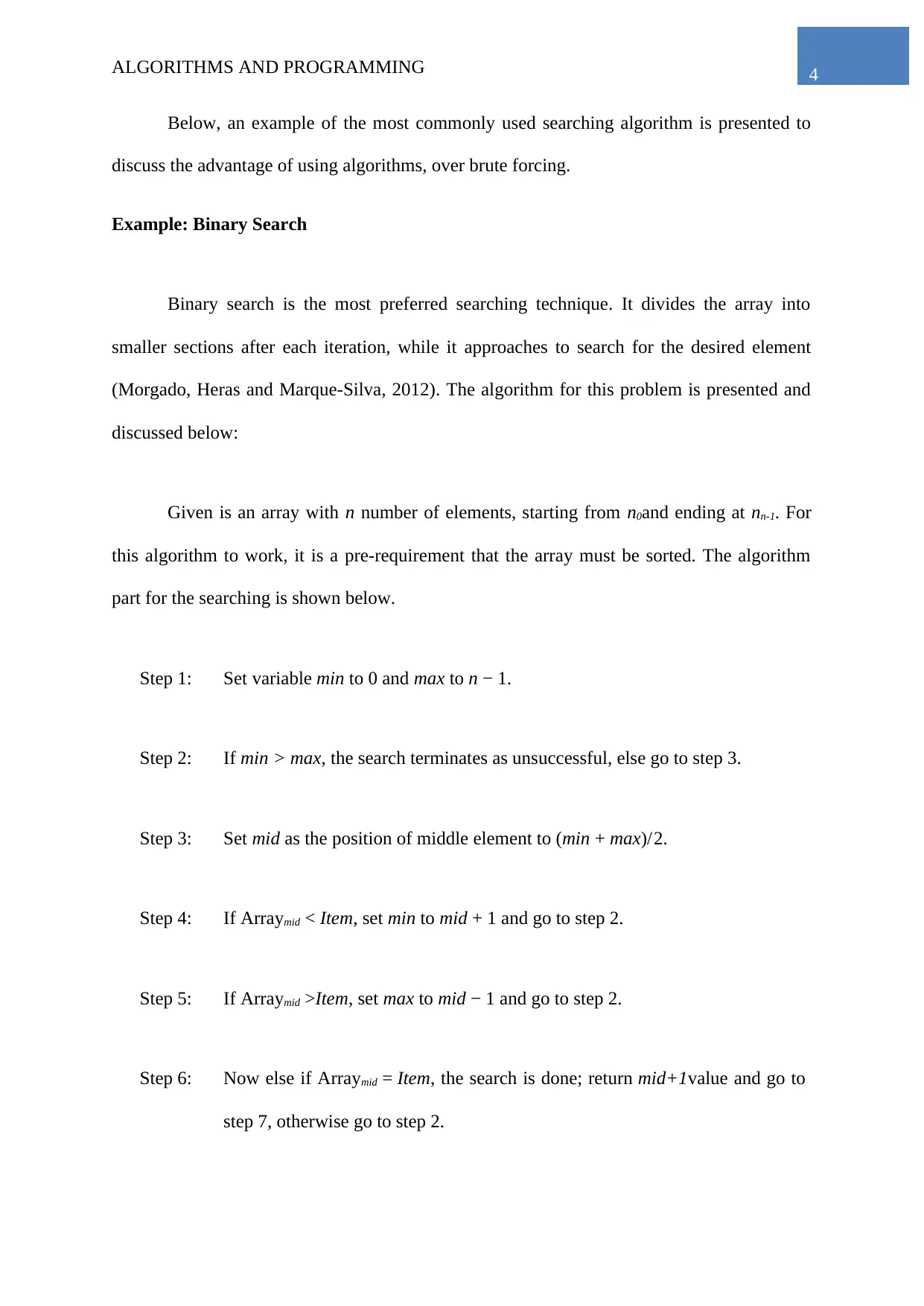
4ALGORITHMS AND PROGRAMMING
Below, an example of the most commonly used searching algorithm is presented to
discuss the advantage of using algorithms, over brute forcing.
Example: Binary Search
Binary search is the most preferred searching technique. It divides the array into
smaller sections after each iteration, while it approaches to search for the desired element
(Morgado, Heras and Marque-Silva, 2012). The algorithm for this problem is presented and
discussed below:
Given is an array with n number of elements, starting from n0and ending at nn-1. For
this algorithm to work, it is a pre-requirement that the array must be sorted. The algorithm
part for the searching is shown below.
Step 1: Set variable min to 0 and max to n − 1.
Step 2: If min > max, the search terminates as unsuccessful, else go to step 3.
Step 3: Set mid as the position of middle element to (min + max)/ 2.
Step 4: If Arraymid < Item, set min to mid + 1 and go to step 2.
Step 5: If Arraymid >Item, set max to mid − 1 and go to step 2.
Step 6: Now else if Arraymid = Item, the search is done; return mid+1value and go to
step 7, otherwise go to step 2.
Below, an example of the most commonly used searching algorithm is presented to
discuss the advantage of using algorithms, over brute forcing.
Example: Binary Search
Binary search is the most preferred searching technique. It divides the array into
smaller sections after each iteration, while it approaches to search for the desired element
(Morgado, Heras and Marque-Silva, 2012). The algorithm for this problem is presented and
discussed below:
Given is an array with n number of elements, starting from n0and ending at nn-1. For
this algorithm to work, it is a pre-requirement that the array must be sorted. The algorithm
part for the searching is shown below.
Step 1: Set variable min to 0 and max to n − 1.
Step 2: If min > max, the search terminates as unsuccessful, else go to step 3.
Step 3: Set mid as the position of middle element to (min + max)/ 2.
Step 4: If Arraymid < Item, set min to mid + 1 and go to step 2.
Step 5: If Arraymid >Item, set max to mid − 1 and go to step 2.
Step 6: Now else if Arraymid = Item, the search is done; return mid+1value and go to
step 7, otherwise go to step 2.
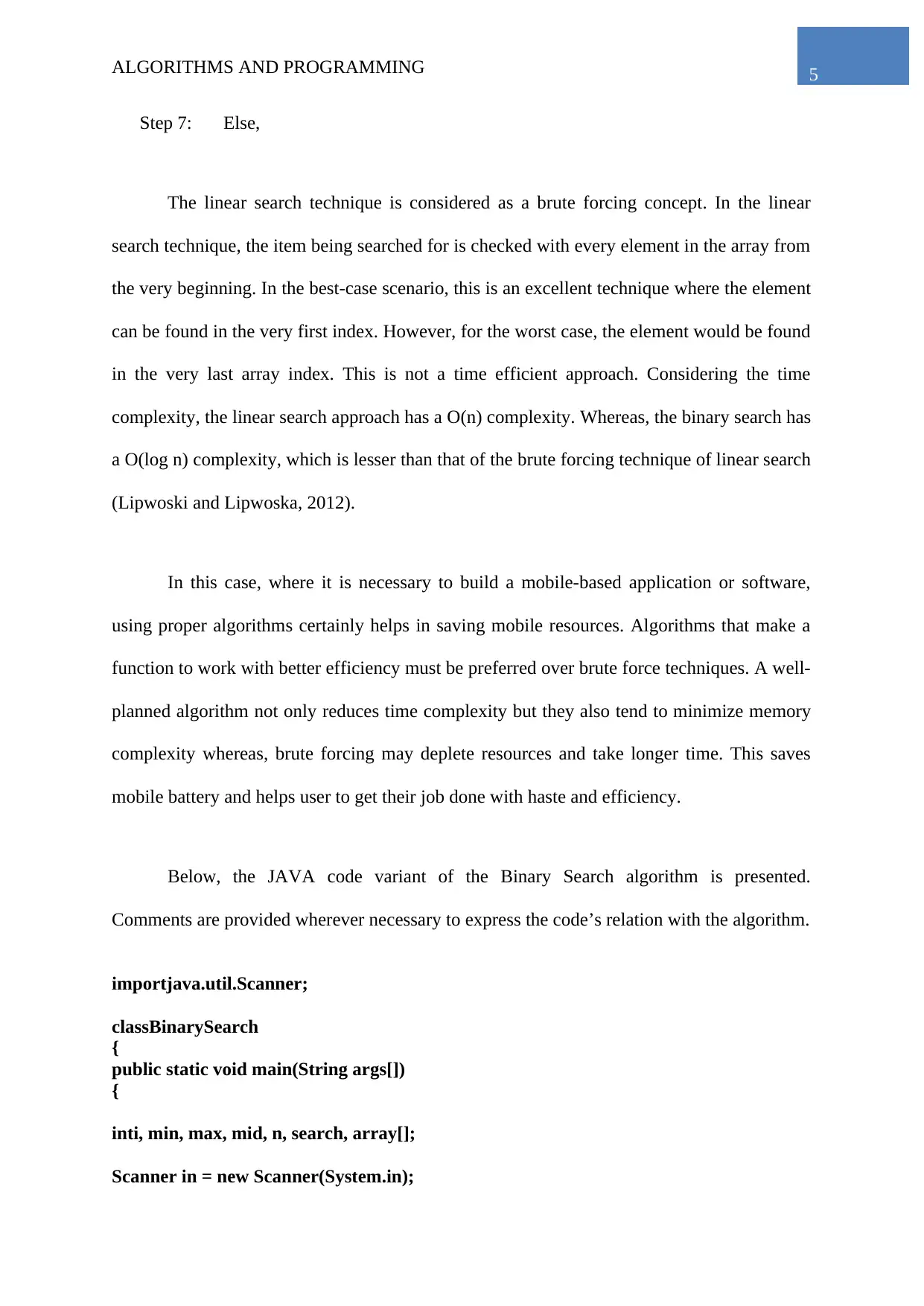
5ALGORITHMS AND PROGRAMMING
Step 7: Else,
The linear search technique is considered as a brute forcing concept. In the linear
search technique, the item being searched for is checked with every element in the array from
the very beginning. In the best-case scenario, this is an excellent technique where the element
can be found in the very first index. However, for the worst case, the element would be found
in the very last array index. This is not a time efficient approach. Considering the time
complexity, the linear search approach has a O(n) complexity. Whereas, the binary search has
a O(log n) complexity, which is lesser than that of the brute forcing technique of linear search
(Lipwoski and Lipwoska, 2012).
In this case, where it is necessary to build a mobile-based application or software,
using proper algorithms certainly helps in saving mobile resources. Algorithms that make a
function to work with better efficiency must be preferred over brute force techniques. A well-
planned algorithm not only reduces time complexity but they also tend to minimize memory
complexity whereas, brute forcing may deplete resources and take longer time. This saves
mobile battery and helps user to get their job done with haste and efficiency.
Below, the JAVA code variant of the Binary Search algorithm is presented.
Comments are provided wherever necessary to express the code’s relation with the algorithm.
importjava.util.Scanner;
classBinarySearch
{
public static void main(String args[])
{
inti, min, max, mid, n, search, array[];
Scanner in = new Scanner(System.in);
Step 7: Else,
The linear search technique is considered as a brute forcing concept. In the linear
search technique, the item being searched for is checked with every element in the array from
the very beginning. In the best-case scenario, this is an excellent technique where the element
can be found in the very first index. However, for the worst case, the element would be found
in the very last array index. This is not a time efficient approach. Considering the time
complexity, the linear search approach has a O(n) complexity. Whereas, the binary search has
a O(log n) complexity, which is lesser than that of the brute forcing technique of linear search
(Lipwoski and Lipwoska, 2012).
In this case, where it is necessary to build a mobile-based application or software,
using proper algorithms certainly helps in saving mobile resources. Algorithms that make a
function to work with better efficiency must be preferred over brute force techniques. A well-
planned algorithm not only reduces time complexity but they also tend to minimize memory
complexity whereas, brute forcing may deplete resources and take longer time. This saves
mobile battery and helps user to get their job done with haste and efficiency.
Below, the JAVA code variant of the Binary Search algorithm is presented.
Comments are provided wherever necessary to express the code’s relation with the algorithm.
importjava.util.Scanner;
classBinarySearch
{
public static void main(String args[])
{
inti, min, max, mid, n, search, array[];
Scanner in = new Scanner(System.in);
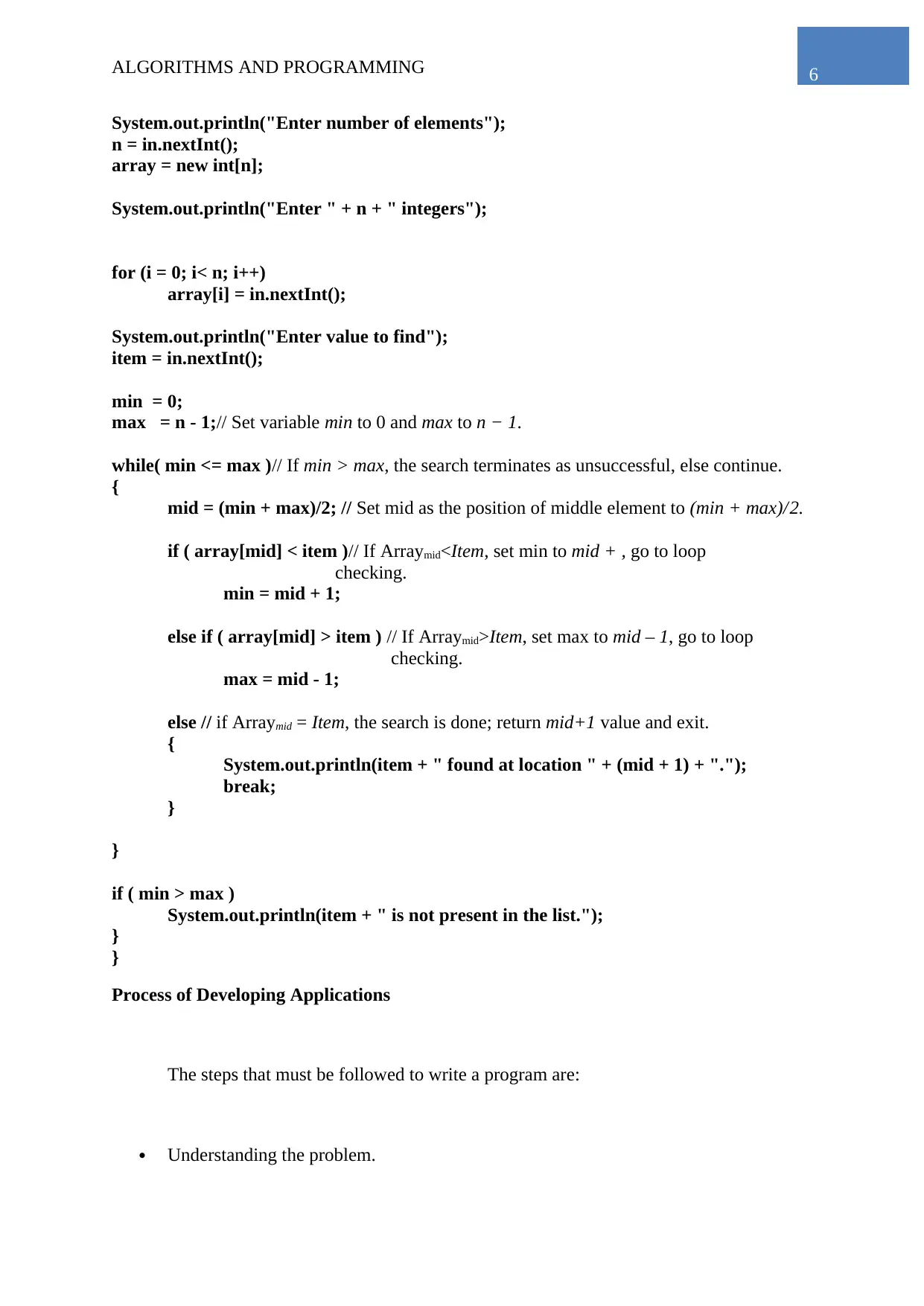
6ALGORITHMS AND PROGRAMMING
System.out.println("Enter number of elements");
n = in.nextInt();
array = new int[n];
System.out.println("Enter " + n + " integers");
for (i = 0; i< n; i++)
array[i] = in.nextInt();
System.out.println("Enter value to find");
item = in.nextInt();
min = 0;
max = n - 1;// Set variable min to 0 and max to n − 1.
while( min <= max )// If min > max, the search terminates as unsuccessful, else continue.
{
mid = (min + max)/2; // Set mid as the position of middle element to (min + max)/ 2.
if ( array[mid] < item )// If Arraymid<Item, set min to mid + , go to loop
checking.
min = mid + 1;
else if ( array[mid] > item ) // If Arraymid>Item, set max to mid – 1, go to loop
checking.
max = mid - 1;
else // if Arraymid = Item, the search is done; return mid+1 value and exit.
{
System.out.println(item + " found at location " + (mid + 1) + ".");
break;
}
}
if ( min > max )
System.out.println(item + " is not present in the list.");
}
}
Process of Developing Applications
The steps that must be followed to write a program are:
Understanding the problem.
System.out.println("Enter number of elements");
n = in.nextInt();
array = new int[n];
System.out.println("Enter " + n + " integers");
for (i = 0; i< n; i++)
array[i] = in.nextInt();
System.out.println("Enter value to find");
item = in.nextInt();
min = 0;
max = n - 1;// Set variable min to 0 and max to n − 1.
while( min <= max )// If min > max, the search terminates as unsuccessful, else continue.
{
mid = (min + max)/2; // Set mid as the position of middle element to (min + max)/ 2.
if ( array[mid] < item )// If Arraymid<Item, set min to mid + , go to loop
checking.
min = mid + 1;
else if ( array[mid] > item ) // If Arraymid>Item, set max to mid – 1, go to loop
checking.
max = mid - 1;
else // if Arraymid = Item, the search is done; return mid+1 value and exit.
{
System.out.println(item + " found at location " + (mid + 1) + ".");
break;
}
}
if ( min > max )
System.out.println(item + " is not present in the list.");
}
}
Process of Developing Applications
The steps that must be followed to write a program are:
Understanding the problem.
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
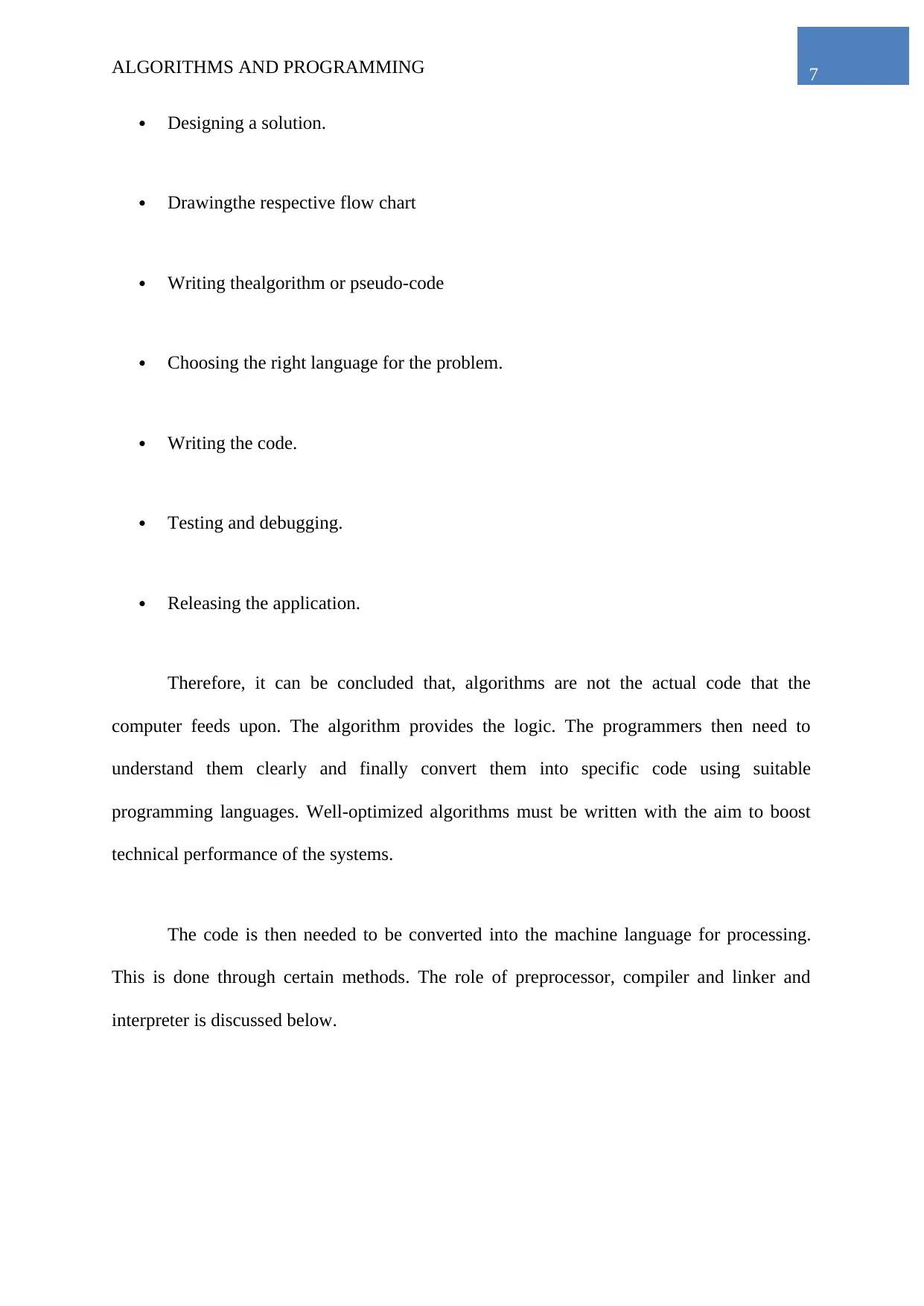
7ALGORITHMS AND PROGRAMMING
Designing a solution.
Drawingthe respective flow chart
Writing thealgorithm or pseudo-code
Choosing the right language for the problem.
Writing the code.
Testing and debugging.
Releasing the application.
Therefore, it can be concluded that, algorithms are not the actual code that the
computer feeds upon. The algorithm provides the logic. The programmers then need to
understand them clearly and finally convert them into specific code using suitable
programming languages. Well-optimized algorithms must be written with the aim to boost
technical performance of the systems.
The code is then needed to be converted into the machine language for processing.
This is done through certain methods. The role of preprocessor, compiler and linker and
interpreter is discussed below.
Designing a solution.
Drawingthe respective flow chart
Writing thealgorithm or pseudo-code
Choosing the right language for the problem.
Writing the code.
Testing and debugging.
Releasing the application.
Therefore, it can be concluded that, algorithms are not the actual code that the
computer feeds upon. The algorithm provides the logic. The programmers then need to
understand them clearly and finally convert them into specific code using suitable
programming languages. Well-optimized algorithms must be written with the aim to boost
technical performance of the systems.
The code is then needed to be converted into the machine language for processing.
This is done through certain methods. The role of preprocessor, compiler and linker and
interpreter is discussed below.
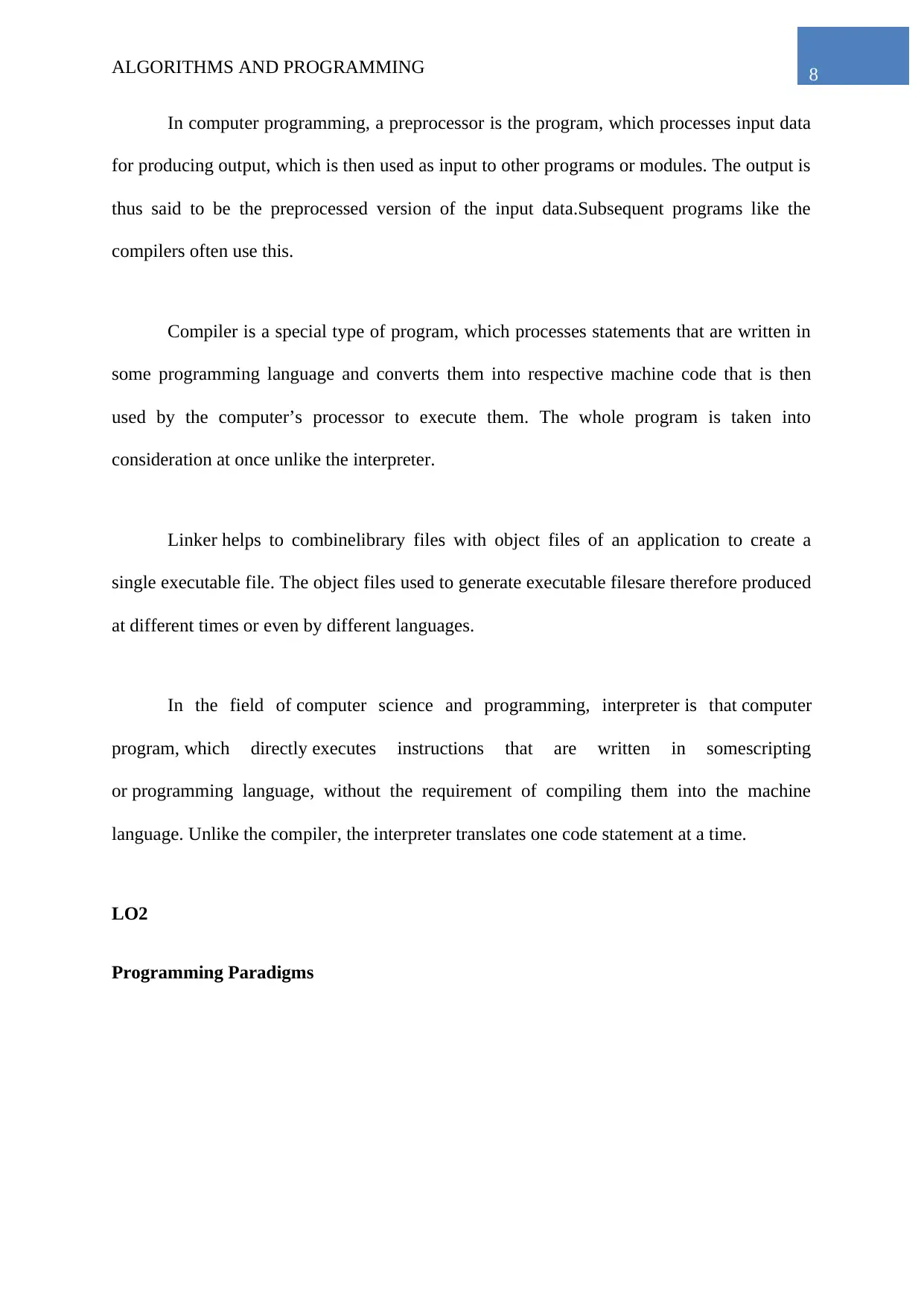
8ALGORITHMS AND PROGRAMMING
In computer programming, a preprocessor is the program, which processes input data
for producing output, which is then used as input to other programs or modules. The output is
thus said to be the preprocessed version of the input data.Subsequent programs like the
compilers often use this.
Compiler is a special type of program, which processes statements that are written in
some programming language and converts them into respective machine code that is then
used by the computer’s processor to execute them. The whole program is taken into
consideration at once unlike the interpreter.
Linker helps to combinelibrary files with object files of an application to create a
single executable file. The object files used to generate executable filesare therefore produced
at different times or even by different languages.
In the field of computer science and programming, interpreter is that computer
program, which directly executes instructions that are written in somescripting
or programming language, without the requirement of compiling them into the machine
language. Unlike the compiler, the interpreter translates one code statement at a time.
LO2
Programming Paradigms
In computer programming, a preprocessor is the program, which processes input data
for producing output, which is then used as input to other programs or modules. The output is
thus said to be the preprocessed version of the input data.Subsequent programs like the
compilers often use this.
Compiler is a special type of program, which processes statements that are written in
some programming language and converts them into respective machine code that is then
used by the computer’s processor to execute them. The whole program is taken into
consideration at once unlike the interpreter.
Linker helps to combinelibrary files with object files of an application to create a
single executable file. The object files used to generate executable filesare therefore produced
at different times or even by different languages.
In the field of computer science and programming, interpreter is that computer
program, which directly executes instructions that are written in somescripting
or programming language, without the requirement of compiling them into the machine
language. Unlike the compiler, the interpreter translates one code statement at a time.
LO2
Programming Paradigms
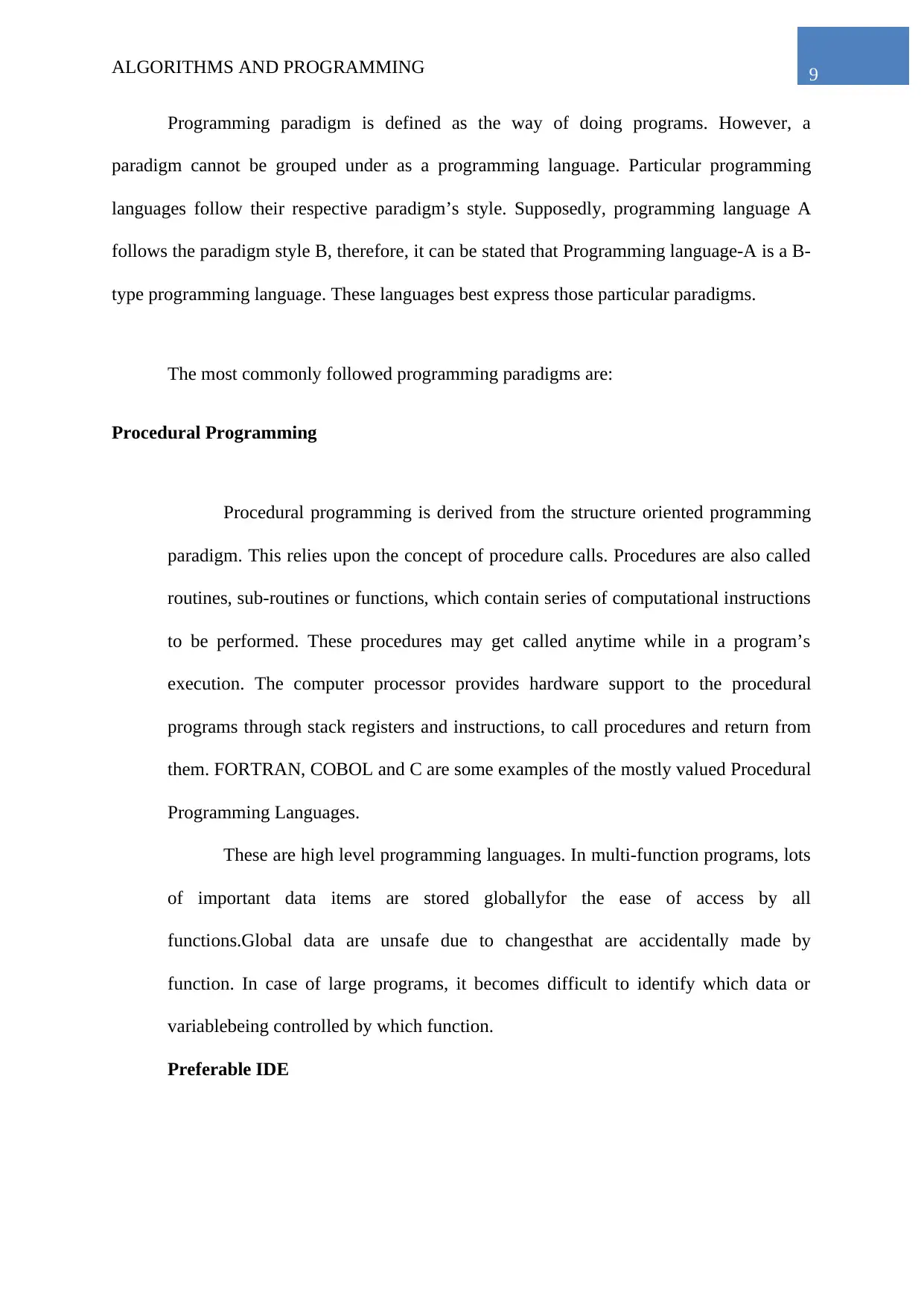
9ALGORITHMS AND PROGRAMMING
Programming paradigm is defined as the way of doing programs. However, a
paradigm cannot be grouped under as a programming language. Particular programming
languages follow their respective paradigm’s style. Supposedly, programming language A
follows the paradigm style B, therefore, it can be stated that Programming language-A is a B-
type programming language. These languages best express those particular paradigms.
The most commonly followed programming paradigms are:
Procedural Programming
Procedural programming is derived from the structure oriented programming
paradigm. This relies upon the concept of procedure calls. Procedures are also called
routines, sub-routines or functions, which contain series of computational instructions
to be performed. These procedures may get called anytime while in a program’s
execution. The computer processor provides hardware support to the procedural
programs through stack registers and instructions, to call procedures and return from
them. FORTRAN, COBOL and C are some examples of the mostly valued Procedural
Programming Languages.
These are high level programming languages. In multi-function programs, lots
of important data items are stored globallyfor the ease of access by all
functions.Global data are unsafe due to changesthat are accidentally made by
function. In case of large programs, it becomes difficult to identify which data or
variablebeing controlled by which function.
Preferable IDE
Programming paradigm is defined as the way of doing programs. However, a
paradigm cannot be grouped under as a programming language. Particular programming
languages follow their respective paradigm’s style. Supposedly, programming language A
follows the paradigm style B, therefore, it can be stated that Programming language-A is a B-
type programming language. These languages best express those particular paradigms.
The most commonly followed programming paradigms are:
Procedural Programming
Procedural programming is derived from the structure oriented programming
paradigm. This relies upon the concept of procedure calls. Procedures are also called
routines, sub-routines or functions, which contain series of computational instructions
to be performed. These procedures may get called anytime while in a program’s
execution. The computer processor provides hardware support to the procedural
programs through stack registers and instructions, to call procedures and return from
them. FORTRAN, COBOL and C are some examples of the mostly valued Procedural
Programming Languages.
These are high level programming languages. In multi-function programs, lots
of important data items are stored globallyfor the ease of access by all
functions.Global data are unsafe due to changesthat are accidentally made by
function. In case of large programs, it becomes difficult to identify which data or
variablebeing controlled by which function.
Preferable IDE
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
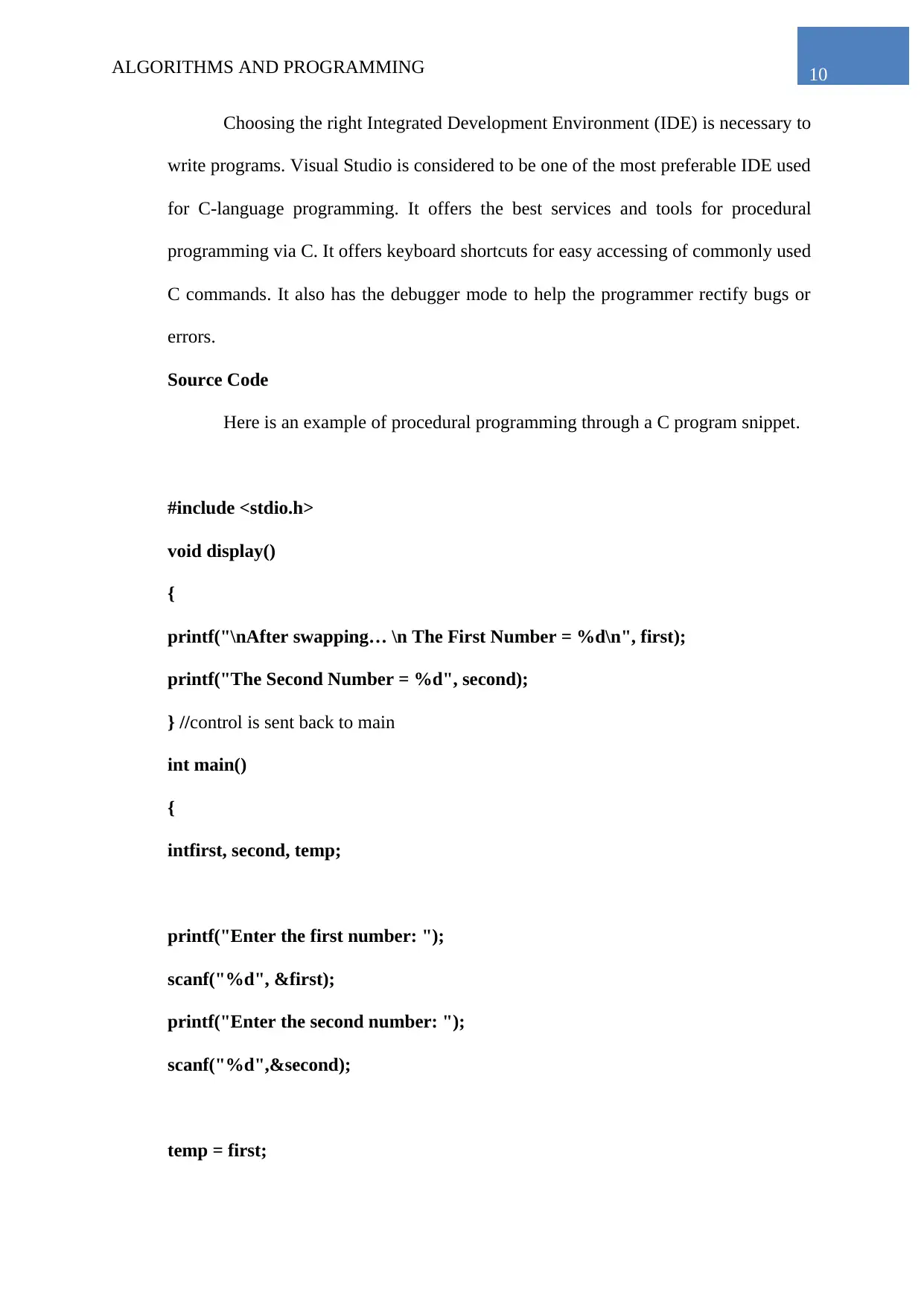
10ALGORITHMS AND PROGRAMMING
Choosing the right Integrated Development Environment (IDE) is necessary to
write programs. Visual Studio is considered to be one of the most preferable IDE used
for C-language programming. It offers the best services and tools for procedural
programming via C. It offers keyboard shortcuts for easy accessing of commonly used
C commands. It also has the debugger mode to help the programmer rectify bugs or
errors.
Source Code
Here is an example of procedural programming through a C program snippet.
#include <stdio.h>
void display()
{
printf("\nAfter swapping… \n The First Number = %d\n", first);
printf("The Second Number = %d", second);
} //control is sent back to main
int main()
{
intfirst, second, temp;
printf("Enter the first number: ");
scanf("%d", &first);
printf("Enter the second number: ");
scanf("%d",&second);
temp = first;
Choosing the right Integrated Development Environment (IDE) is necessary to
write programs. Visual Studio is considered to be one of the most preferable IDE used
for C-language programming. It offers the best services and tools for procedural
programming via C. It offers keyboard shortcuts for easy accessing of commonly used
C commands. It also has the debugger mode to help the programmer rectify bugs or
errors.
Source Code
Here is an example of procedural programming through a C program snippet.
#include <stdio.h>
void display()
{
printf("\nAfter swapping… \n The First Number = %d\n", first);
printf("The Second Number = %d", second);
} //control is sent back to main
int main()
{
intfirst, second, temp;
printf("Enter the first number: ");
scanf("%d", &first);
printf("Enter the second number: ");
scanf("%d",&second);
temp = first;
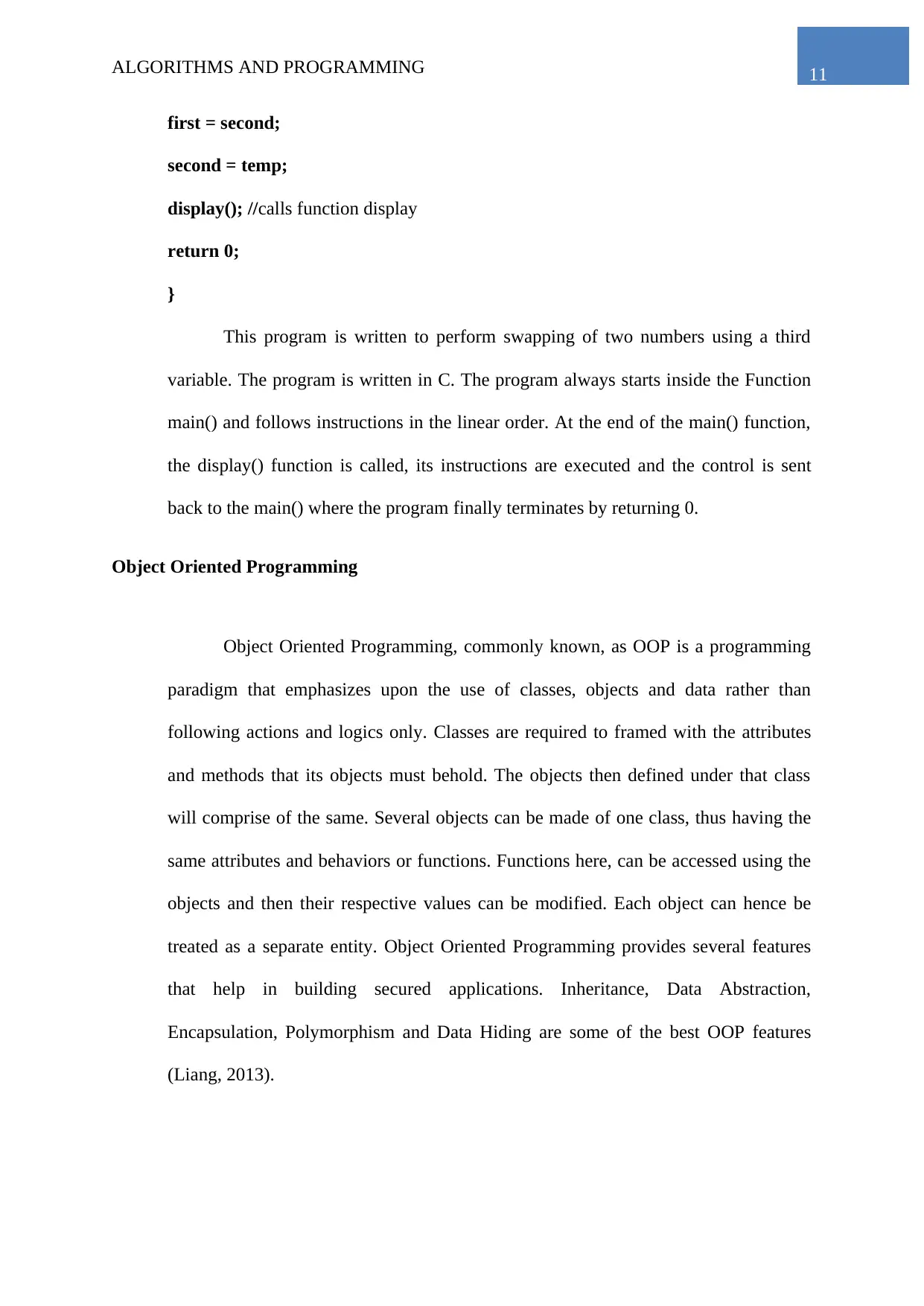
11ALGORITHMS AND PROGRAMMING
first = second;
second = temp;
display(); //calls function display
return 0;
}
This program is written to perform swapping of two numbers using a third
variable. The program is written in C. The program always starts inside the Function
main() and follows instructions in the linear order. At the end of the main() function,
the display() function is called, its instructions are executed and the control is sent
back to the main() where the program finally terminates by returning 0.
Object Oriented Programming
Object Oriented Programming, commonly known, as OOP is a programming
paradigm that emphasizes upon the use of classes, objects and data rather than
following actions and logics only. Classes are required to framed with the attributes
and methods that its objects must behold. The objects then defined under that class
will comprise of the same. Several objects can be made of one class, thus having the
same attributes and behaviors or functions. Functions here, can be accessed using the
objects and then their respective values can be modified. Each object can hence be
treated as a separate entity. Object Oriented Programming provides several features
that help in building secured applications. Inheritance, Data Abstraction,
Encapsulation, Polymorphism and Data Hiding are some of the best OOP features
(Liang, 2013).
first = second;
second = temp;
display(); //calls function display
return 0;
}
This program is written to perform swapping of two numbers using a third
variable. The program is written in C. The program always starts inside the Function
main() and follows instructions in the linear order. At the end of the main() function,
the display() function is called, its instructions are executed and the control is sent
back to the main() where the program finally terminates by returning 0.
Object Oriented Programming
Object Oriented Programming, commonly known, as OOP is a programming
paradigm that emphasizes upon the use of classes, objects and data rather than
following actions and logics only. Classes are required to framed with the attributes
and methods that its objects must behold. The objects then defined under that class
will comprise of the same. Several objects can be made of one class, thus having the
same attributes and behaviors or functions. Functions here, can be accessed using the
objects and then their respective values can be modified. Each object can hence be
treated as a separate entity. Object Oriented Programming provides several features
that help in building secured applications. Inheritance, Data Abstraction,
Encapsulation, Polymorphism and Data Hiding are some of the best OOP features
(Liang, 2013).
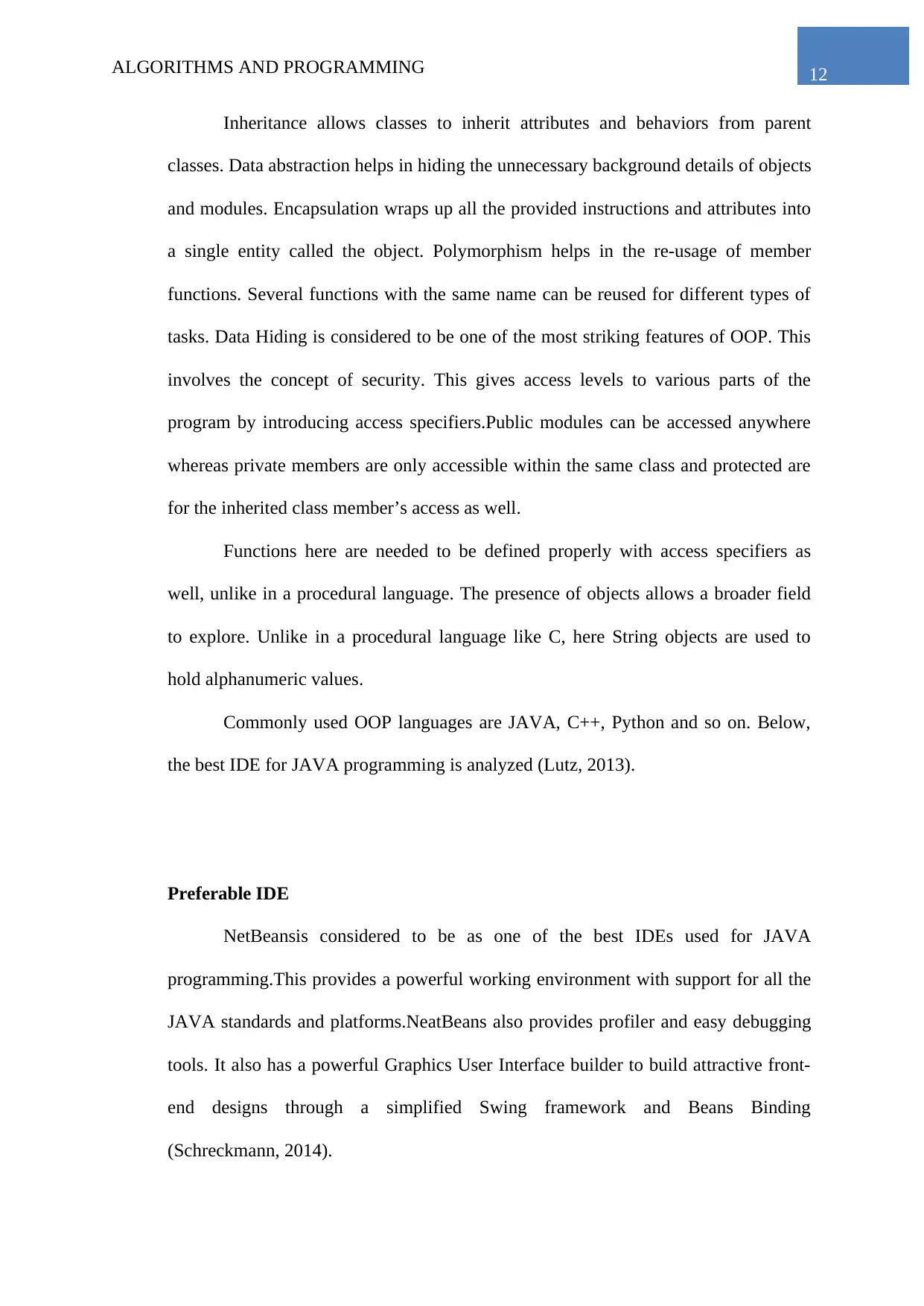
12ALGORITHMS AND PROGRAMMING
Inheritance allows classes to inherit attributes and behaviors from parent
classes. Data abstraction helps in hiding the unnecessary background details of objects
and modules. Encapsulation wraps up all the provided instructions and attributes into
a single entity called the object. Polymorphism helps in the re-usage of member
functions. Several functions with the same name can be reused for different types of
tasks. Data Hiding is considered to be one of the most striking features of OOP. This
involves the concept of security. This gives access levels to various parts of the
program by introducing access specifiers.Public modules can be accessed anywhere
whereas private members are only accessible within the same class and protected are
for the inherited class member’s access as well.
Functions here are needed to be defined properly with access specifiers as
well, unlike in a procedural language. The presence of objects allows a broader field
to explore. Unlike in a procedural language like C, here String objects are used to
hold alphanumeric values.
Commonly used OOP languages are JAVA, C++, Python and so on. Below,
the best IDE for JAVA programming is analyzed (Lutz, 2013).
Preferable IDE
NetBeansis considered to be as one of the best IDEs used for JAVA
programming.This provides a powerful working environment with support for all the
JAVA standards and platforms.NeatBeans also provides profiler and easy debugging
tools. It also has a powerful Graphics User Interface builder to build attractive front-
end designs through a simplified Swing framework and Beans Binding
(Schreckmann, 2014).
Inheritance allows classes to inherit attributes and behaviors from parent
classes. Data abstraction helps in hiding the unnecessary background details of objects
and modules. Encapsulation wraps up all the provided instructions and attributes into
a single entity called the object. Polymorphism helps in the re-usage of member
functions. Several functions with the same name can be reused for different types of
tasks. Data Hiding is considered to be one of the most striking features of OOP. This
involves the concept of security. This gives access levels to various parts of the
program by introducing access specifiers.Public modules can be accessed anywhere
whereas private members are only accessible within the same class and protected are
for the inherited class member’s access as well.
Functions here are needed to be defined properly with access specifiers as
well, unlike in a procedural language. The presence of objects allows a broader field
to explore. Unlike in a procedural language like C, here String objects are used to
hold alphanumeric values.
Commonly used OOP languages are JAVA, C++, Python and so on. Below,
the best IDE for JAVA programming is analyzed (Lutz, 2013).
Preferable IDE
NetBeansis considered to be as one of the best IDEs used for JAVA
programming.This provides a powerful working environment with support for all the
JAVA standards and platforms.NeatBeans also provides profiler and easy debugging
tools. It also has a powerful Graphics User Interface builder to build attractive front-
end designs through a simplified Swing framework and Beans Binding
(Schreckmann, 2014).
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
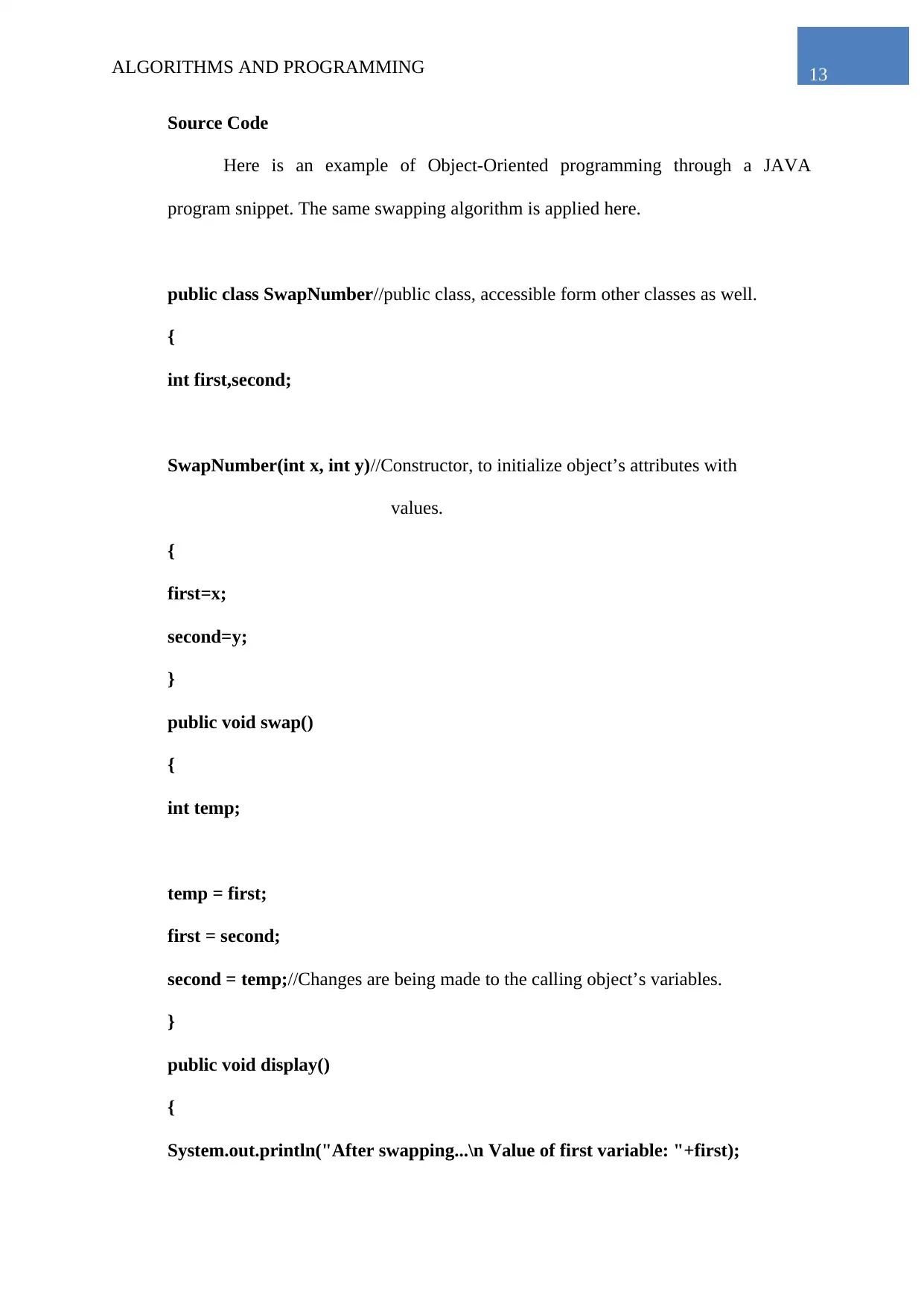
13ALGORITHMS AND PROGRAMMING
Source Code
Here is an example of Object-Oriented programming through a JAVA
program snippet. The same swapping algorithm is applied here.
public class SwapNumber//public class, accessible form other classes as well.
{
int first,second;
SwapNumber(int x, int y)//Constructor, to initialize object’s attributes with
values.
{
first=x;
second=y;
}
public void swap()
{
int temp;
temp = first;
first = second;
second = temp;//Changes are being made to the calling object’s variables.
}
public void display()
{
System.out.println("After swapping...\n Value of first variable: "+first);
Source Code
Here is an example of Object-Oriented programming through a JAVA
program snippet. The same swapping algorithm is applied here.
public class SwapNumber//public class, accessible form other classes as well.
{
int first,second;
SwapNumber(int x, int y)//Constructor, to initialize object’s attributes with
values.
{
first=x;
second=y;
}
public void swap()
{
int temp;
temp = first;
first = second;
second = temp;//Changes are being made to the calling object’s variables.
}
public void display()
{
System.out.println("After swapping...\n Value of first variable: "+first);
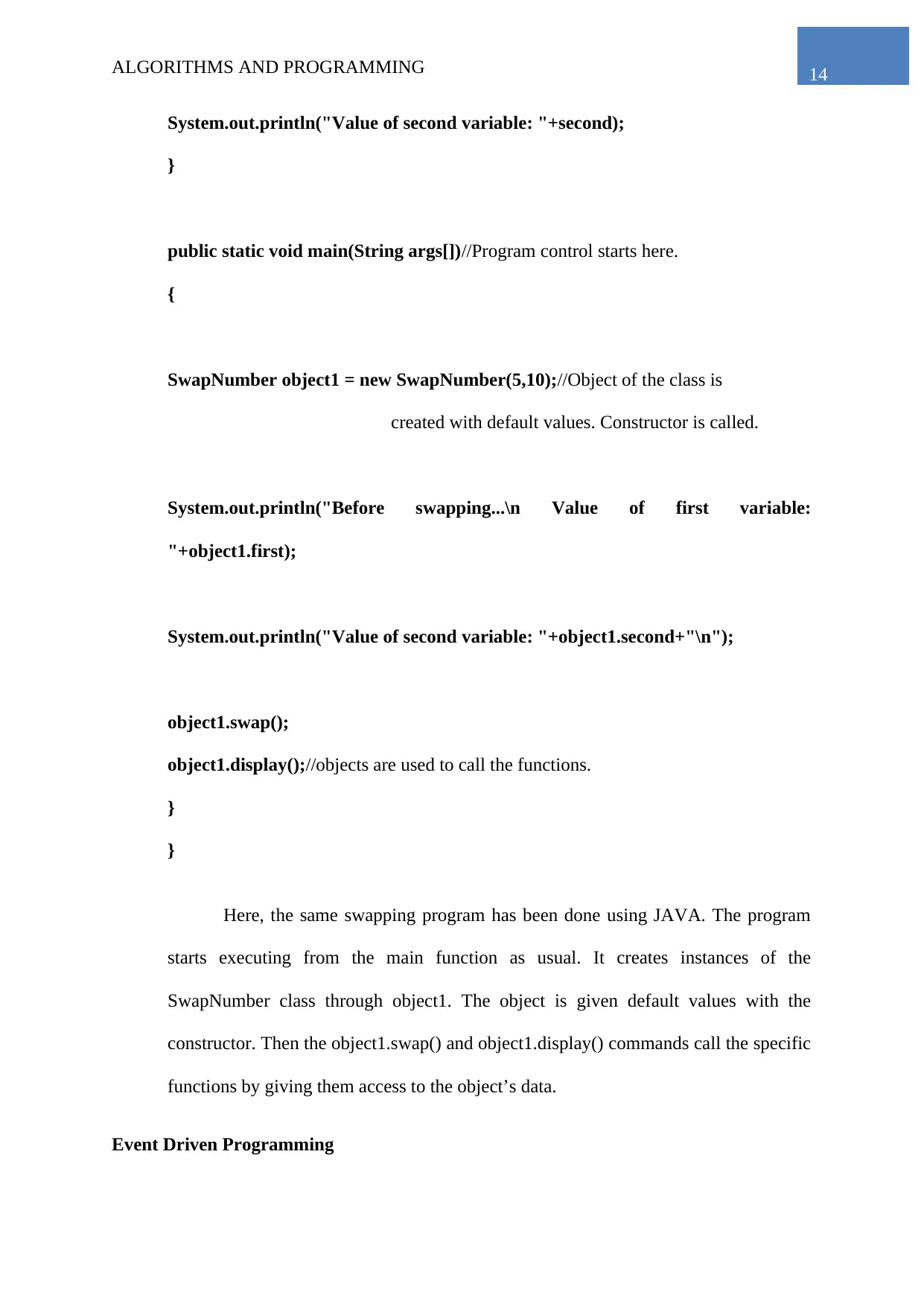
14ALGORITHMS AND PROGRAMMING
System.out.println("Value of second variable: "+second);
}
public static void main(String args[])//Program control starts here.
{
SwapNumber object1 = new SwapNumber(5,10);//Object of the class is
created with default values. Constructor is called.
System.out.println("Before swapping...\n Value of first variable:
"+object1.first);
System.out.println("Value of second variable: "+object1.second+"\n");
object1.swap();
object1.display();//objects are used to call the functions.
}
}
Here, the same swapping program has been done using JAVA. The program
starts executing from the main function as usual. It creates instances of the
SwapNumber class through object1. The object is given default values with the
constructor. Then the object1.swap() and object1.display() commands call the specific
functions by giving them access to the object’s data.
Event Driven Programming
System.out.println("Value of second variable: "+second);
}
public static void main(String args[])//Program control starts here.
{
SwapNumber object1 = new SwapNumber(5,10);//Object of the class is
created with default values. Constructor is called.
System.out.println("Before swapping...\n Value of first variable:
"+object1.first);
System.out.println("Value of second variable: "+object1.second+"\n");
object1.swap();
object1.display();//objects are used to call the functions.
}
}
Here, the same swapping program has been done using JAVA. The program
starts executing from the main function as usual. It creates instances of the
SwapNumber class through object1. The object is given default values with the
constructor. Then the object1.swap() and object1.display() commands call the specific
functions by giving them access to the object’s data.
Event Driven Programming
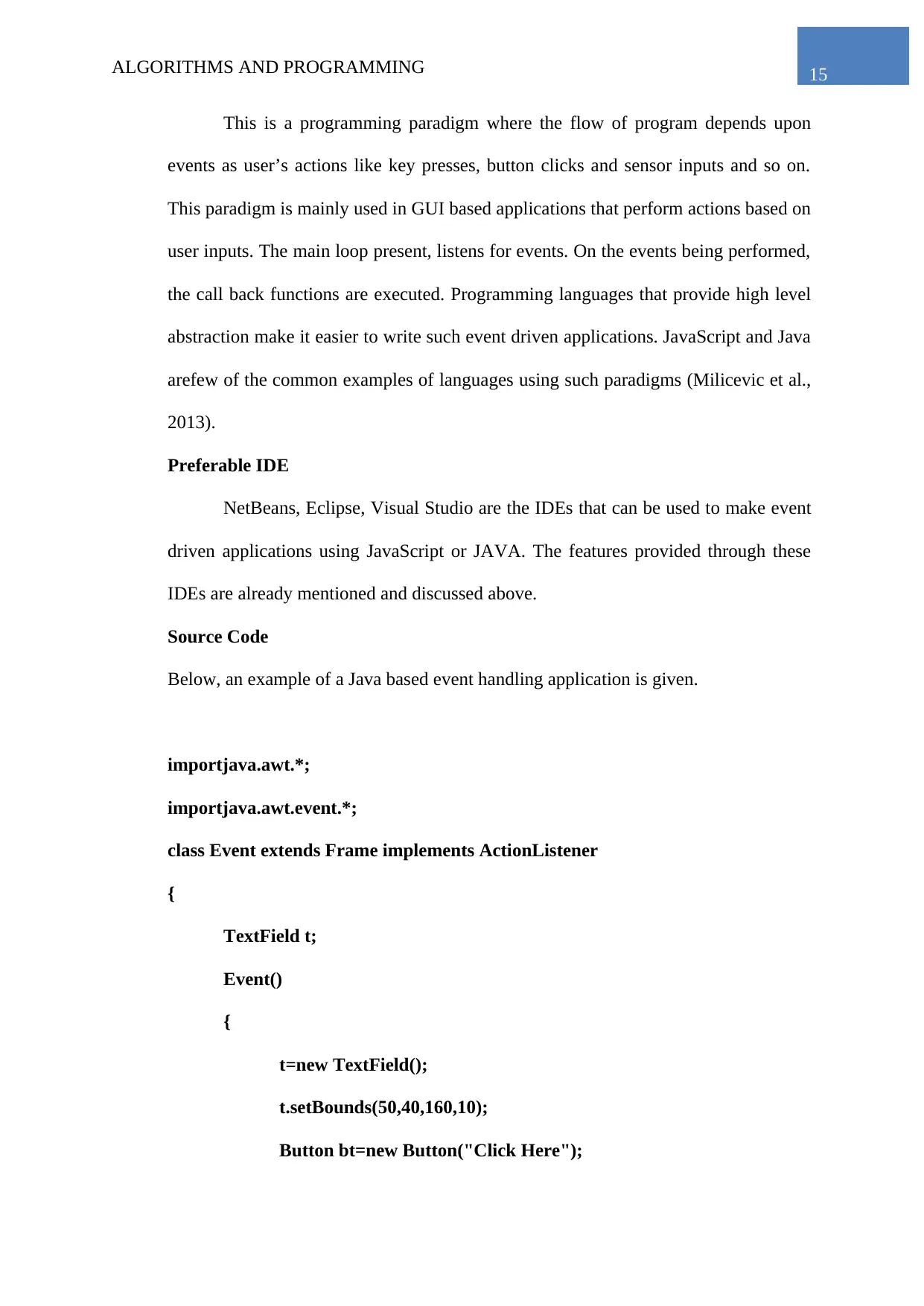
15ALGORITHMS AND PROGRAMMING
This is a programming paradigm where the flow of program depends upon
events as user’s actions like key presses, button clicks and sensor inputs and so on.
This paradigm is mainly used in GUI based applications that perform actions based on
user inputs. The main loop present, listens for events. On the events being performed,
the call back functions are executed. Programming languages that provide high level
abstraction make it easier to write such event driven applications. JavaScript and Java
arefew of the common examples of languages using such paradigms (Milicevic et al.,
2013).
Preferable IDE
NetBeans, Eclipse, Visual Studio are the IDEs that can be used to make event
driven applications using JavaScript or JAVA. The features provided through these
IDEs are already mentioned and discussed above.
Source Code
Below, an example of a Java based event handling application is given.
importjava.awt.*;
importjava.awt.event.*;
class Event extends Frame implements ActionListener
{
TextField t;
Event()
{
t=new TextField();
t.setBounds(50,40,160,10);
Button bt=new Button("Click Here");
This is a programming paradigm where the flow of program depends upon
events as user’s actions like key presses, button clicks and sensor inputs and so on.
This paradigm is mainly used in GUI based applications that perform actions based on
user inputs. The main loop present, listens for events. On the events being performed,
the call back functions are executed. Programming languages that provide high level
abstraction make it easier to write such event driven applications. JavaScript and Java
arefew of the common examples of languages using such paradigms (Milicevic et al.,
2013).
Preferable IDE
NetBeans, Eclipse, Visual Studio are the IDEs that can be used to make event
driven applications using JavaScript or JAVA. The features provided through these
IDEs are already mentioned and discussed above.
Source Code
Below, an example of a Java based event handling application is given.
importjava.awt.*;
importjava.awt.event.*;
class Event extends Frame implements ActionListener
{
TextField t;
Event()
{
t=new TextField();
t.setBounds(50,40,160,10);
Button bt=new Button("Click Here");
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
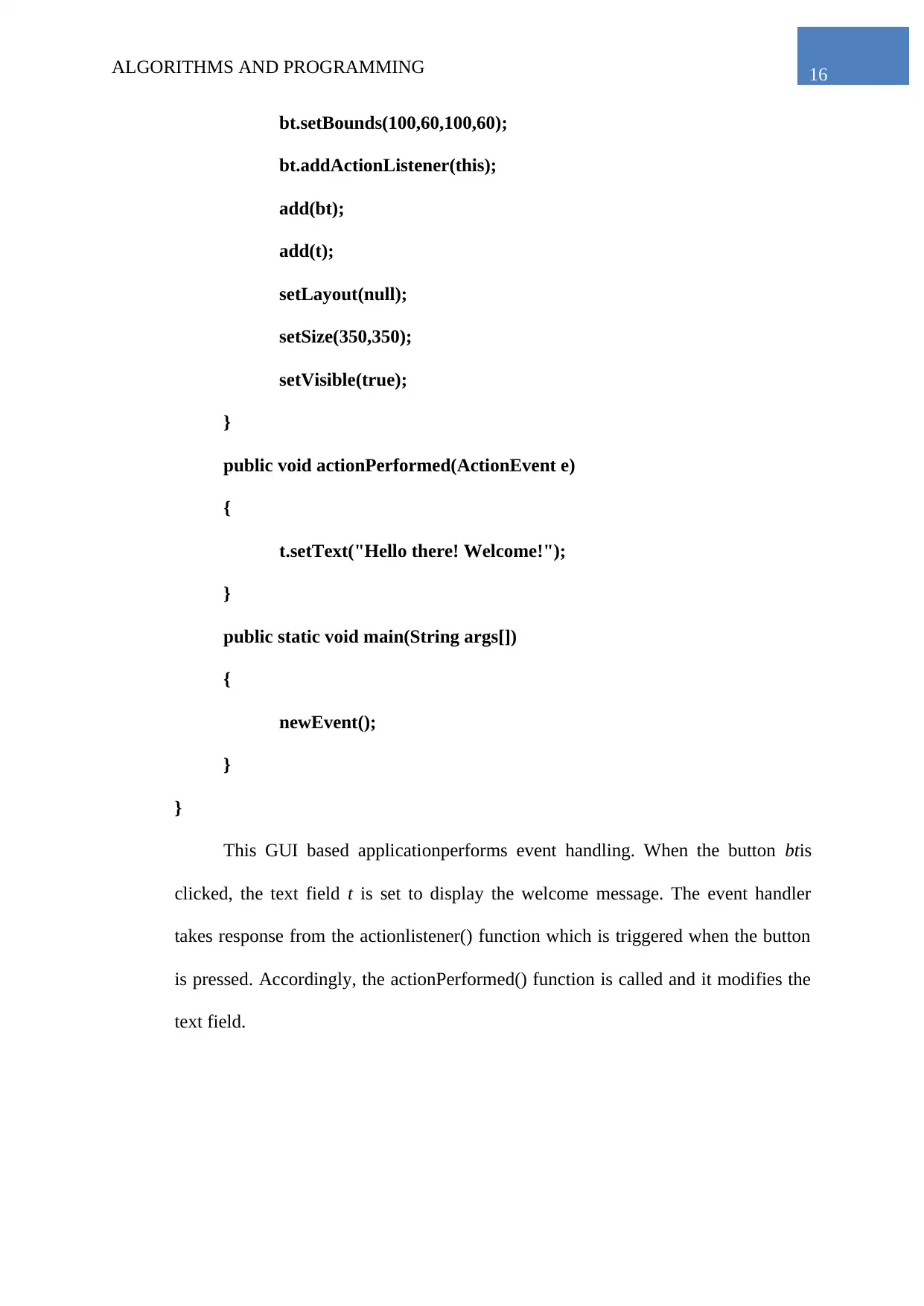
16ALGORITHMS AND PROGRAMMING
bt.setBounds(100,60,100,60);
bt.addActionListener(this);
add(bt);
add(t);
setLayout(null);
setSize(350,350);
setVisible(true);
}
public void actionPerformed(ActionEvent e)
{
t.setText("Hello there! Welcome!");
}
public static void main(String args[])
{
newEvent();
}
}
This GUI based applicationperforms event handling. When the button btis
clicked, the text field t is set to display the welcome message. The event handler
takes response from the actionlistener() function which is triggered when the button
is pressed. Accordingly, the actionPerformed() function is called and it modifies the
text field.
bt.setBounds(100,60,100,60);
bt.addActionListener(this);
add(bt);
add(t);
setLayout(null);
setSize(350,350);
setVisible(true);
}
public void actionPerformed(ActionEvent e)
{
t.setText("Hello there! Welcome!");
}
public static void main(String args[])
{
newEvent();
}
}
This GUI based applicationperforms event handling. When the button btis
clicked, the text field t is set to display the welcome message. The event handler
takes response from the actionlistener() function which is triggered when the button
is pressed. Accordingly, the actionPerformed() function is called and it modifies the
text field.
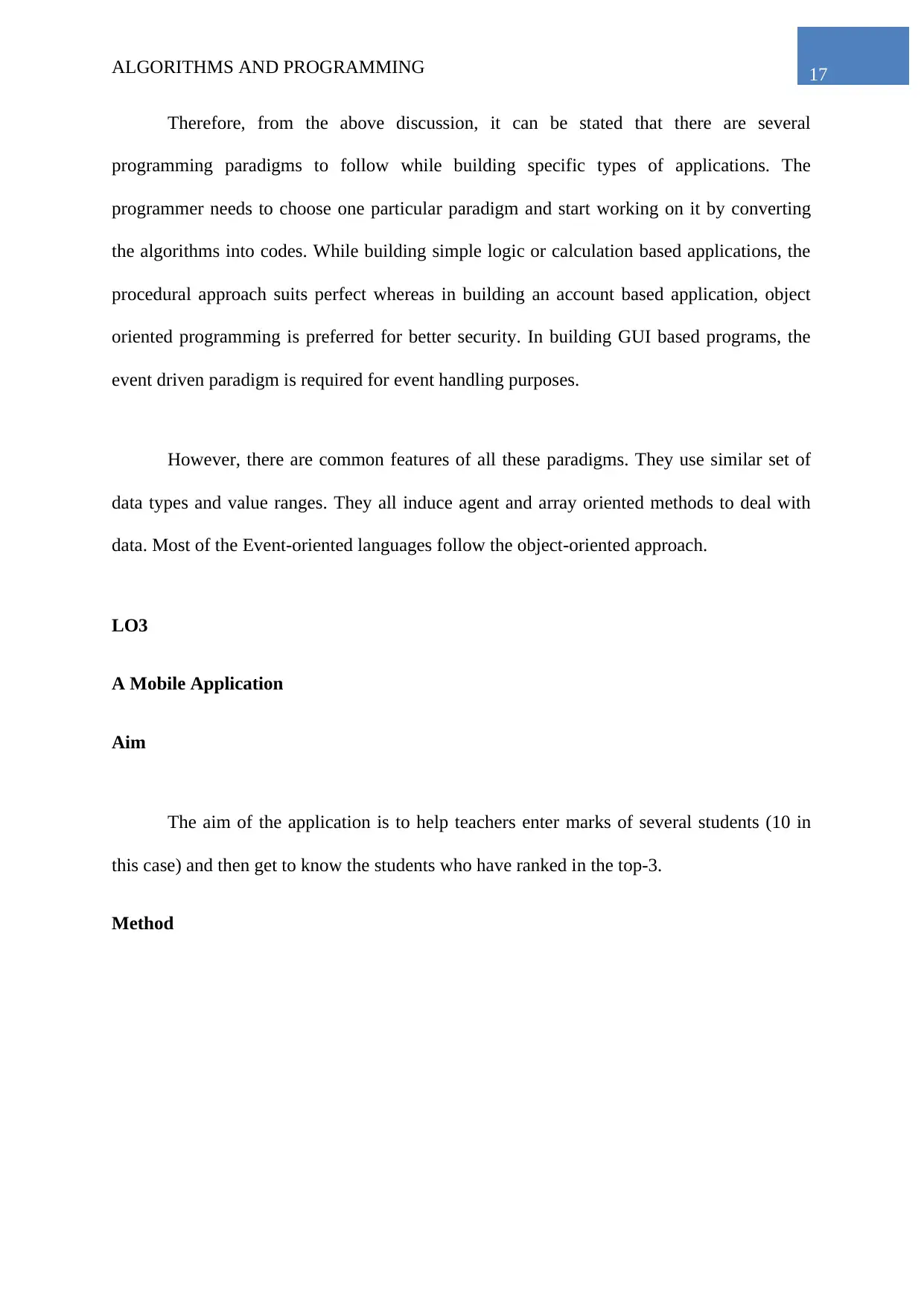
17ALGORITHMS AND PROGRAMMING
Therefore, from the above discussion, it can be stated that there are several
programming paradigms to follow while building specific types of applications. The
programmer needs to choose one particular paradigm and start working on it by converting
the algorithms into codes. While building simple logic or calculation based applications, the
procedural approach suits perfect whereas in building an account based application, object
oriented programming is preferred for better security. In building GUI based programs, the
event driven paradigm is required for event handling purposes.
However, there are common features of all these paradigms. They use similar set of
data types and value ranges. They all induce agent and array oriented methods to deal with
data. Most of the Event-oriented languages follow the object-oriented approach.
LO3
A Mobile Application
Aim
The aim of the application is to help teachers enter marks of several students (10 in
this case) and then get to know the students who have ranked in the top-3.
Method
Therefore, from the above discussion, it can be stated that there are several
programming paradigms to follow while building specific types of applications. The
programmer needs to choose one particular paradigm and start working on it by converting
the algorithms into codes. While building simple logic or calculation based applications, the
procedural approach suits perfect whereas in building an account based application, object
oriented programming is preferred for better security. In building GUI based programs, the
event driven paradigm is required for event handling purposes.
However, there are common features of all these paradigms. They use similar set of
data types and value ranges. They all induce agent and array oriented methods to deal with
data. Most of the Event-oriented languages follow the object-oriented approach.
LO3
A Mobile Application
Aim
The aim of the application is to help teachers enter marks of several students (10 in
this case) and then get to know the students who have ranked in the top-3.
Method
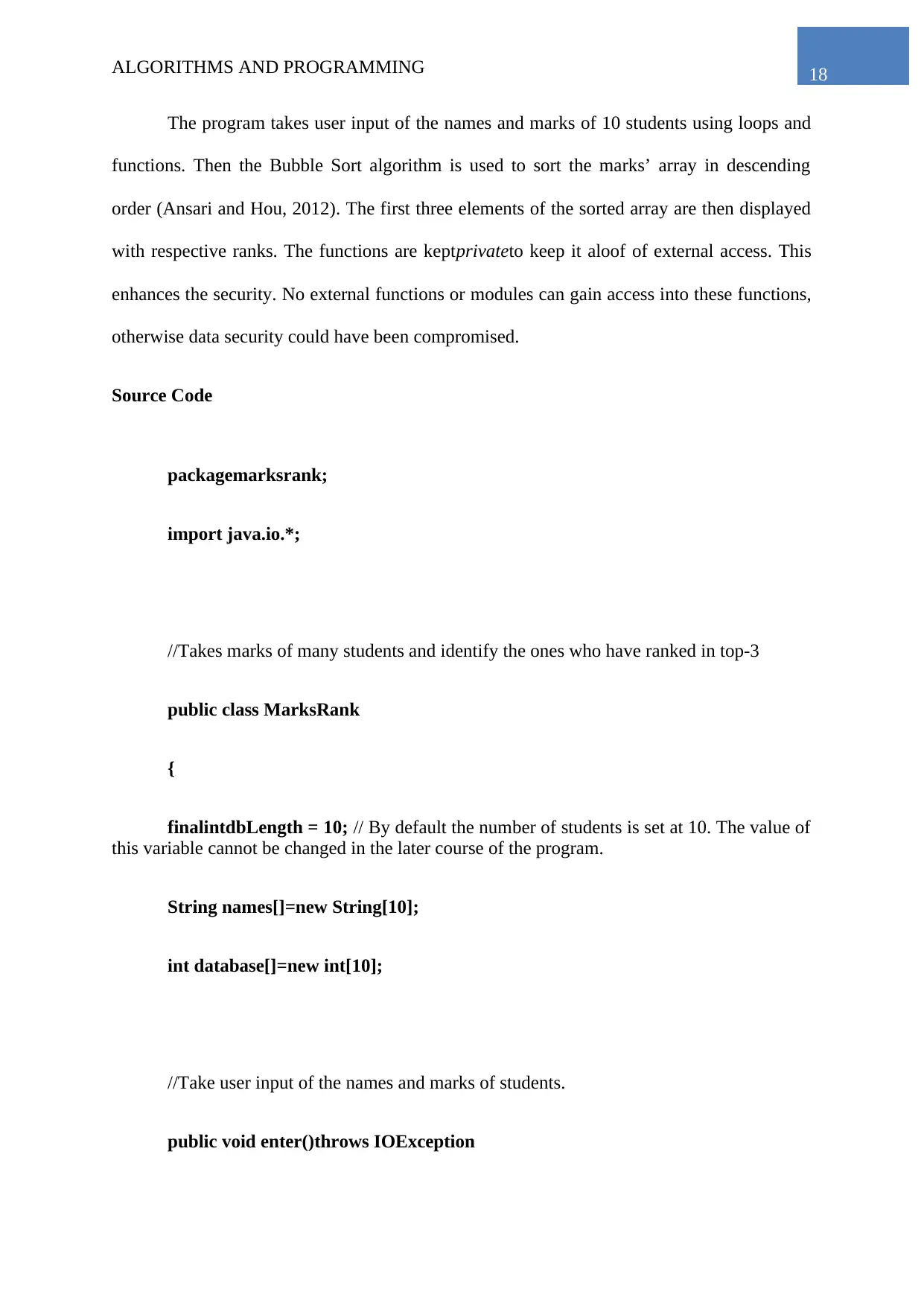
18ALGORITHMS AND PROGRAMMING
The program takes user input of the names and marks of 10 students using loops and
functions. Then the Bubble Sort algorithm is used to sort the marks’ array in descending
order (Ansari and Hou, 2012). The first three elements of the sorted array are then displayed
with respective ranks. The functions are keptprivateto keep it aloof of external access. This
enhances the security. No external functions or modules can gain access into these functions,
otherwise data security could have been compromised.
Source Code
packagemarksrank;
import java.io.*;
//Takes marks of many students and identify the ones who have ranked in top-3
public class MarksRank
{
finalintdbLength = 10; // By default the number of students is set at 10. The value of
this variable cannot be changed in the later course of the program.
String names[]=new String[10];
int database[]=new int[10];
//Take user input of the names and marks of students.
public void enter()throws IOException
The program takes user input of the names and marks of 10 students using loops and
functions. Then the Bubble Sort algorithm is used to sort the marks’ array in descending
order (Ansari and Hou, 2012). The first three elements of the sorted array are then displayed
with respective ranks. The functions are keptprivateto keep it aloof of external access. This
enhances the security. No external functions or modules can gain access into these functions,
otherwise data security could have been compromised.
Source Code
packagemarksrank;
import java.io.*;
//Takes marks of many students and identify the ones who have ranked in top-3
public class MarksRank
{
finalintdbLength = 10; // By default the number of students is set at 10. The value of
this variable cannot be changed in the later course of the program.
String names[]=new String[10];
int database[]=new int[10];
//Take user input of the names and marks of students.
public void enter()throws IOException
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
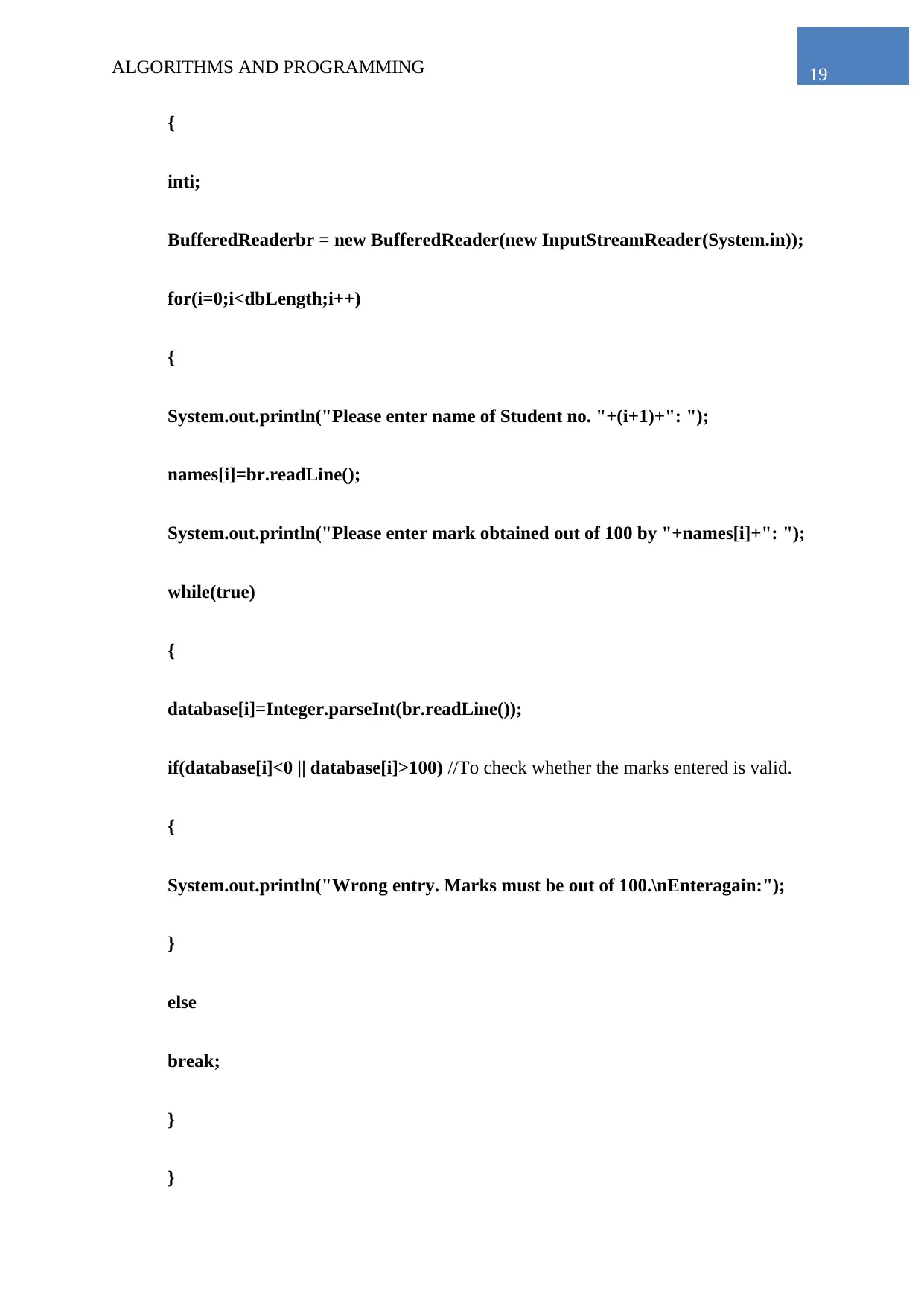
19ALGORITHMS AND PROGRAMMING
{
inti;
BufferedReaderbr = new BufferedReader(new InputStreamReader(System.in));
for(i=0;i<dbLength;i++)
{
System.out.println("Please enter name of Student no. "+(i+1)+": ");
names[i]=br.readLine();
System.out.println("Please enter mark obtained out of 100 by "+names[i]+": ");
while(true)
{
database[i]=Integer.parseInt(br.readLine());
if(database[i]<0 || database[i]>100) //To check whether the marks entered is valid.
{
System.out.println("Wrong entry. Marks must be out of 100.\nEnteragain:");
}
else
break;
}
}
{
inti;
BufferedReaderbr = new BufferedReader(new InputStreamReader(System.in));
for(i=0;i<dbLength;i++)
{
System.out.println("Please enter name of Student no. "+(i+1)+": ");
names[i]=br.readLine();
System.out.println("Please enter mark obtained out of 100 by "+names[i]+": ");
while(true)
{
database[i]=Integer.parseInt(br.readLine());
if(database[i]<0 || database[i]>100) //To check whether the marks entered is valid.
{
System.out.println("Wrong entry. Marks must be out of 100.\nEnteragain:");
}
else
break;
}
}
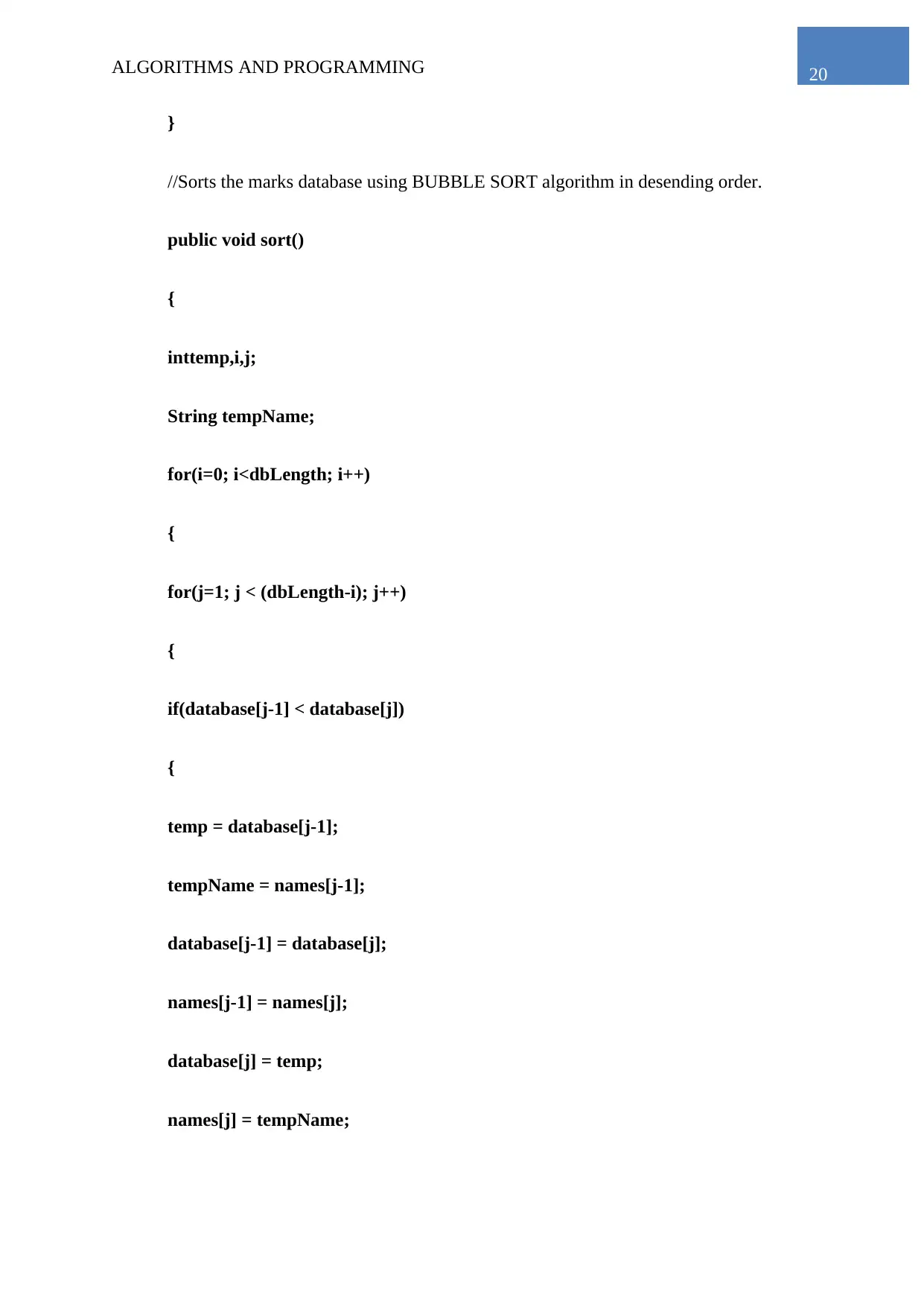
20ALGORITHMS AND PROGRAMMING
}
//Sorts the marks database using BUBBLE SORT algorithm in desending order.
public void sort()
{
inttemp,i,j;
String tempName;
for(i=0; i<dbLength; i++)
{
for(j=1; j < (dbLength-i); j++)
{
if(database[j-1] < database[j])
{
temp = database[j-1];
tempName = names[j-1];
database[j-1] = database[j];
names[j-1] = names[j];
database[j] = temp;
names[j] = tempName;
}
//Sorts the marks database using BUBBLE SORT algorithm in desending order.
public void sort()
{
inttemp,i,j;
String tempName;
for(i=0; i<dbLength; i++)
{
for(j=1; j < (dbLength-i); j++)
{
if(database[j-1] < database[j])
{
temp = database[j-1];
tempName = names[j-1];
database[j-1] = database[j];
names[j-1] = names[j];
database[j] = temp;
names[j] = tempName;
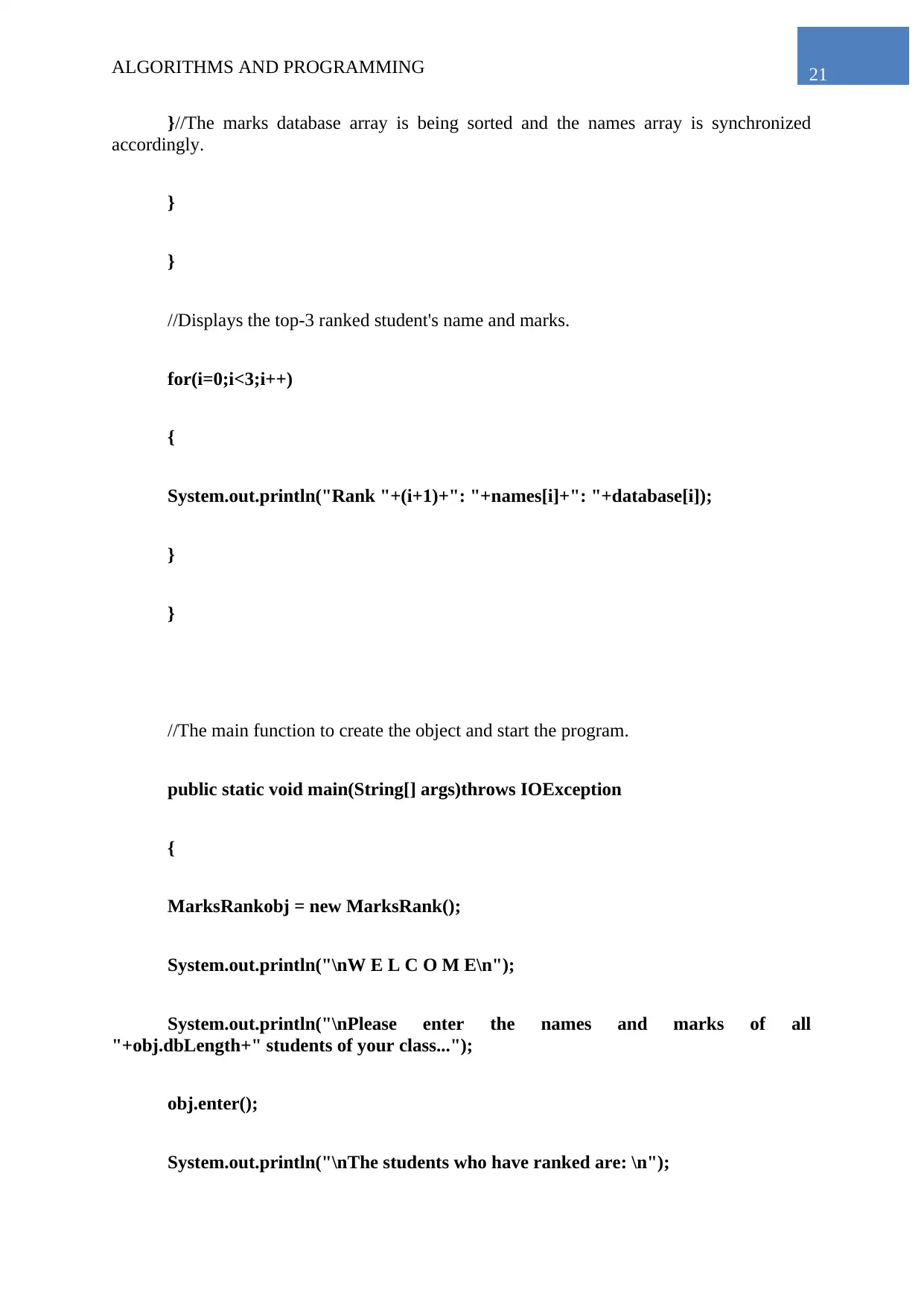
21ALGORITHMS AND PROGRAMMING
}//The marks database array is being sorted and the names array is synchronized
accordingly.
}
}
//Displays the top-3 ranked student's name and marks.
for(i=0;i<3;i++)
{
System.out.println("Rank "+(i+1)+": "+names[i]+": "+database[i]);
}
}
//The main function to create the object and start the program.
public static void main(String[] args)throws IOException
{
MarksRankobj = new MarksRank();
System.out.println("\nW E L C O M E\n");
System.out.println("\nPlease enter the names and marks of all
"+obj.dbLength+" students of your class...");
obj.enter();
System.out.println("\nThe students who have ranked are: \n");
}//The marks database array is being sorted and the names array is synchronized
accordingly.
}
}
//Displays the top-3 ranked student's name and marks.
for(i=0;i<3;i++)
{
System.out.println("Rank "+(i+1)+": "+names[i]+": "+database[i]);
}
}
//The main function to create the object and start the program.
public static void main(String[] args)throws IOException
{
MarksRankobj = new MarksRank();
System.out.println("\nW E L C O M E\n");
System.out.println("\nPlease enter the names and marks of all
"+obj.dbLength+" students of your class...");
obj.enter();
System.out.println("\nThe students who have ranked are: \n");
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
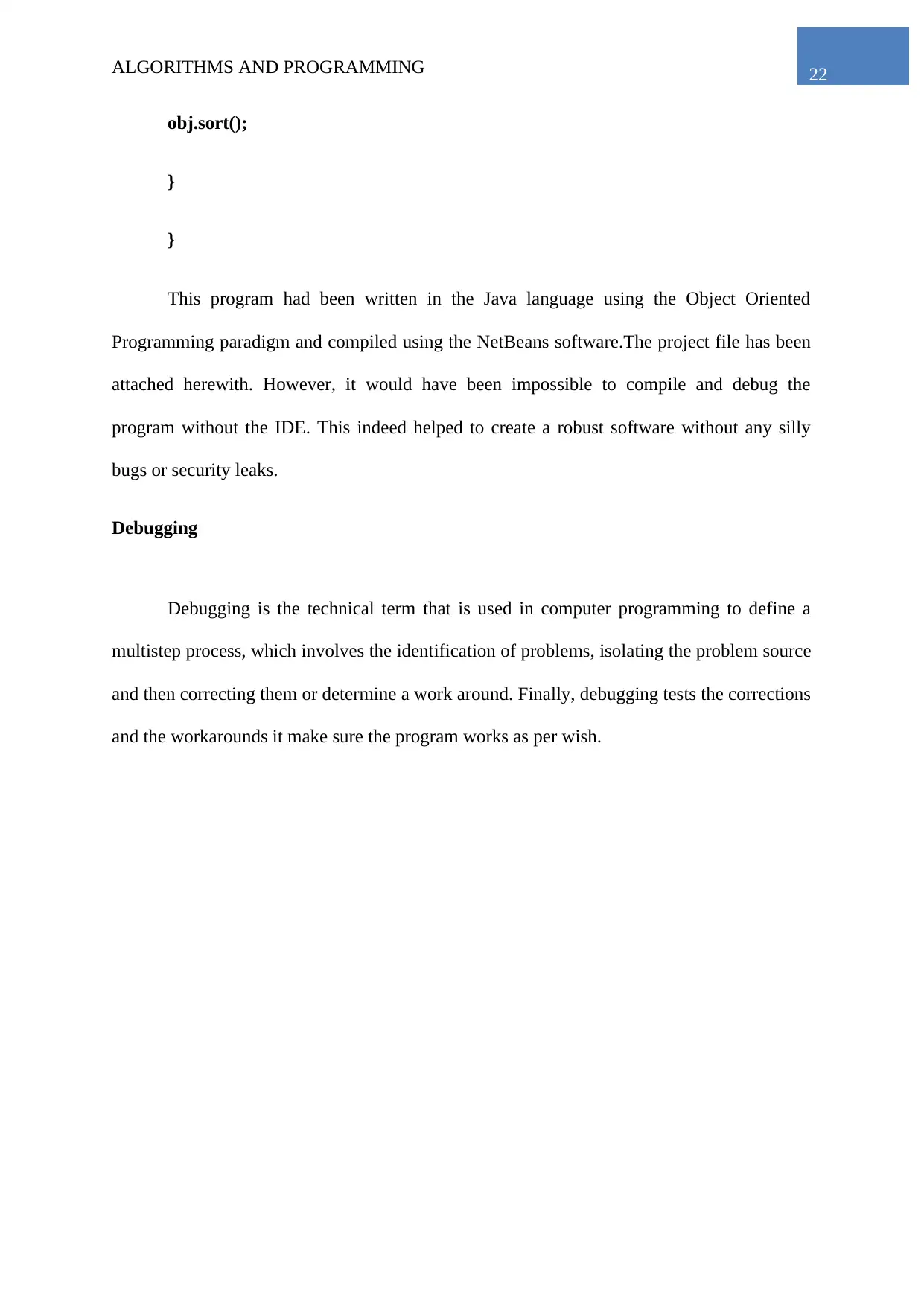
22ALGORITHMS AND PROGRAMMING
obj.sort();
}
}
This program had been written in the Java language using the Object Oriented
Programming paradigm and compiled using the NetBeans software.The project file has been
attached herewith. However, it would have been impossible to compile and debug the
program without the IDE. This indeed helped to create a robust software without any silly
bugs or security leaks.
Debugging
Debugging is the technical term that is used in computer programming to define a
multistep process, which involves the identification of problems, isolating the problem source
and then correcting them or determine a work around. Finally, debugging tests the corrections
and the workarounds it make sure the program works as per wish.
obj.sort();
}
}
This program had been written in the Java language using the Object Oriented
Programming paradigm and compiled using the NetBeans software.The project file has been
attached herewith. However, it would have been impossible to compile and debug the
program without the IDE. This indeed helped to create a robust software without any silly
bugs or security leaks.
Debugging
Debugging is the technical term that is used in computer programming to define a
multistep process, which involves the identification of problems, isolating the problem source
and then correcting them or determine a work around. Finally, debugging tests the corrections
and the workarounds it make sure the program works as per wish.
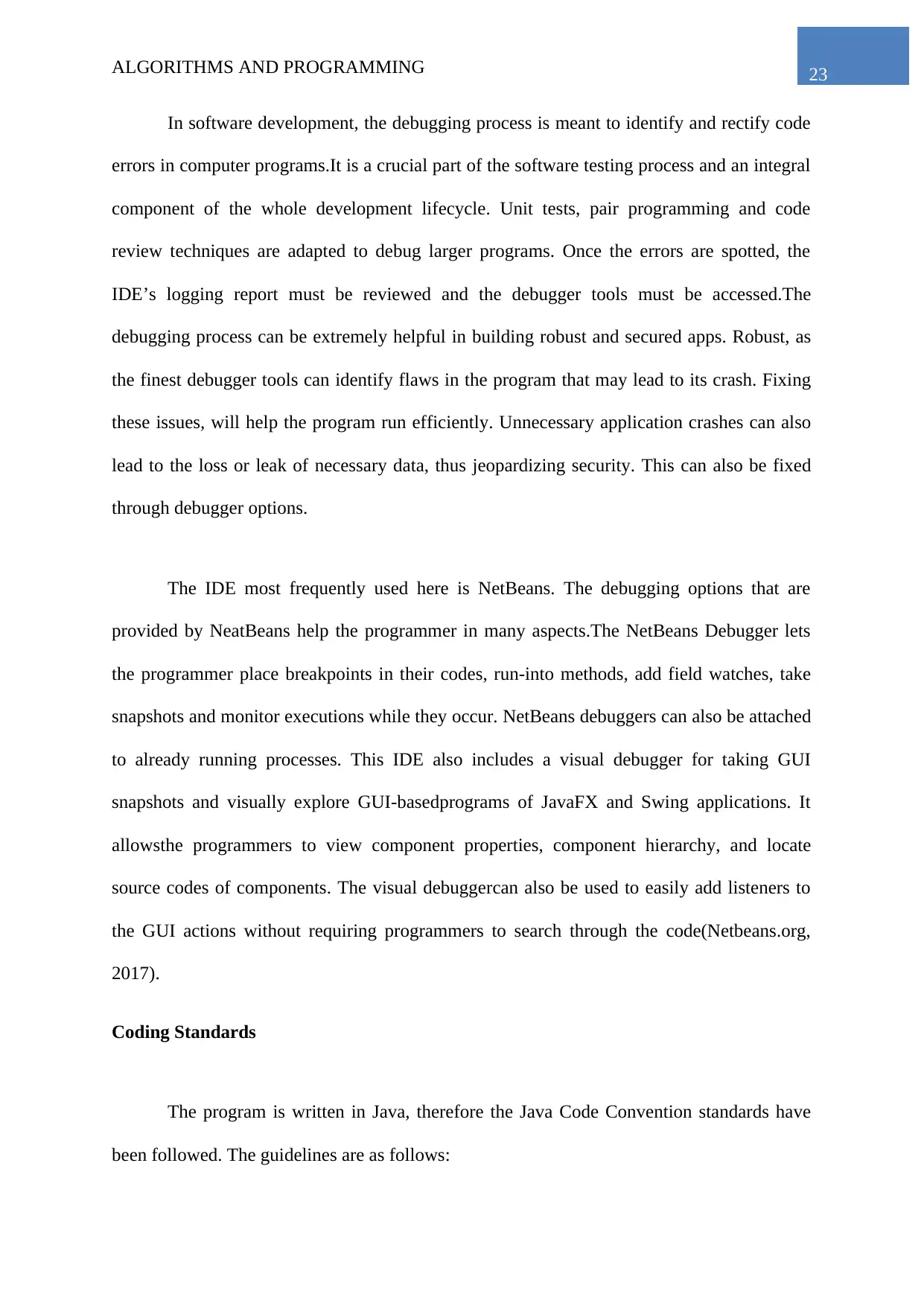
23ALGORITHMS AND PROGRAMMING
In software development, the debugging process is meant to identify and rectify code
errors in computer programs.It is a crucial part of the software testing process and an integral
component of the whole development lifecycle. Unit tests, pair programming and code
review techniques are adapted to debug larger programs. Once the errors are spotted, the
IDE’s logging report must be reviewed and the debugger tools must be accessed.The
debugging process can be extremely helpful in building robust and secured apps. Robust, as
the finest debugger tools can identify flaws in the program that may lead to its crash. Fixing
these issues, will help the program run efficiently. Unnecessary application crashes can also
lead to the loss or leak of necessary data, thus jeopardizing security. This can also be fixed
through debugger options.
The IDE most frequently used here is NetBeans. The debugging options that are
provided by NeatBeans help the programmer in many aspects.The NetBeans Debugger lets
the programmer place breakpoints in their codes, run-into methods, add field watches, take
snapshots and monitor executions while they occur. NetBeans debuggers can also be attached
to already running processes. This IDE also includes a visual debugger for taking GUI
snapshots and visually explore GUI-basedprograms of JavaFX and Swing applications. It
allowsthe programmers to view component properties, component hierarchy, and locate
source codes of components. The visual debuggercan also be used to easily add listeners to
the GUI actions without requiring programmers to search through the code(Netbeans.org,
2017).
Coding Standards
The program is written in Java, therefore the Java Code Convention standards have
been followed. The guidelines are as follows:
In software development, the debugging process is meant to identify and rectify code
errors in computer programs.It is a crucial part of the software testing process and an integral
component of the whole development lifecycle. Unit tests, pair programming and code
review techniques are adapted to debug larger programs. Once the errors are spotted, the
IDE’s logging report must be reviewed and the debugger tools must be accessed.The
debugging process can be extremely helpful in building robust and secured apps. Robust, as
the finest debugger tools can identify flaws in the program that may lead to its crash. Fixing
these issues, will help the program run efficiently. Unnecessary application crashes can also
lead to the loss or leak of necessary data, thus jeopardizing security. This can also be fixed
through debugger options.
The IDE most frequently used here is NetBeans. The debugging options that are
provided by NeatBeans help the programmer in many aspects.The NetBeans Debugger lets
the programmer place breakpoints in their codes, run-into methods, add field watches, take
snapshots and monitor executions while they occur. NetBeans debuggers can also be attached
to already running processes. This IDE also includes a visual debugger for taking GUI
snapshots and visually explore GUI-basedprograms of JavaFX and Swing applications. It
allowsthe programmers to view component properties, component hierarchy, and locate
source codes of components. The visual debuggercan also be used to easily add listeners to
the GUI actions without requiring programmers to search through the code(Netbeans.org,
2017).
Coding Standards
The program is written in Java, therefore the Java Code Convention standards have
been followed. The guidelines are as follows:
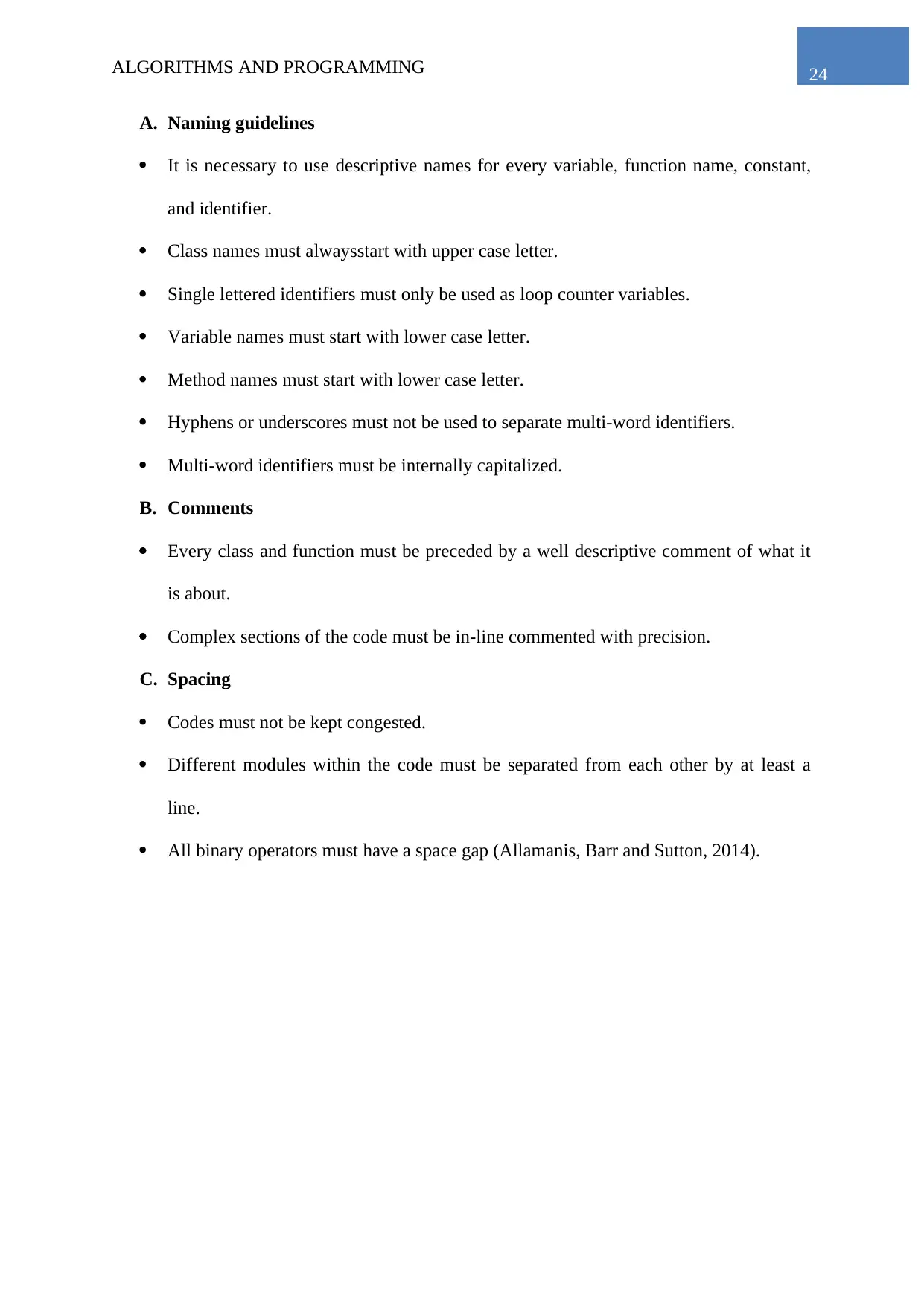
24ALGORITHMS AND PROGRAMMING
A. Naming guidelines
It is necessary to use descriptive names for every variable, function name, constant,
and identifier.
Class names must alwaysstart with upper case letter.
Single lettered identifiers must only be used as loop counter variables.
Variable names must start with lower case letter.
Method names must start with lower case letter.
Hyphens or underscores must not be used to separate multi-word identifiers.
Multi-word identifiers must be internally capitalized.
B. Comments
Every class and function must be preceded by a well descriptive comment of what it
is about.
Complex sections of the code must be in-line commented with precision.
C. Spacing
Codes must not be kept congested.
Different modules within the code must be separated from each other by at least a
line.
All binary operators must have a space gap (Allamanis, Barr and Sutton, 2014).
A. Naming guidelines
It is necessary to use descriptive names for every variable, function name, constant,
and identifier.
Class names must alwaysstart with upper case letter.
Single lettered identifiers must only be used as loop counter variables.
Variable names must start with lower case letter.
Method names must start with lower case letter.
Hyphens or underscores must not be used to separate multi-word identifiers.
Multi-word identifiers must be internally capitalized.
B. Comments
Every class and function must be preceded by a well descriptive comment of what it
is about.
Complex sections of the code must be in-line commented with precision.
C. Spacing
Codes must not be kept congested.
Different modules within the code must be separated from each other by at least a
line.
All binary operators must have a space gap (Allamanis, Barr and Sutton, 2014).
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
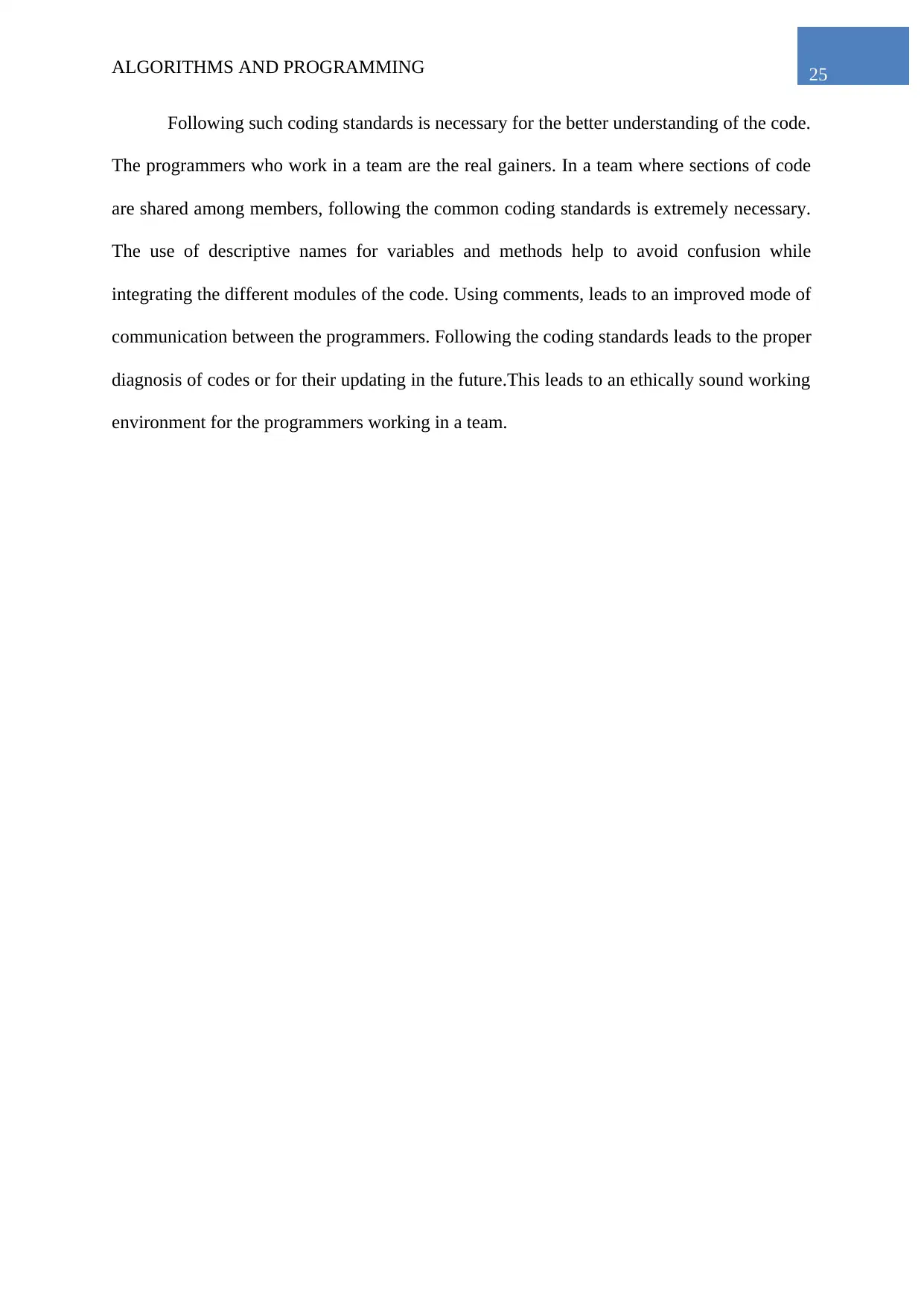
25ALGORITHMS AND PROGRAMMING
Following such coding standards is necessary for the better understanding of the code.
The programmers who work in a team are the real gainers. In a team where sections of code
are shared among members, following the common coding standards is extremely necessary.
The use of descriptive names for variables and methods help to avoid confusion while
integrating the different modules of the code. Using comments, leads to an improved mode of
communication between the programmers. Following the coding standards leads to the proper
diagnosis of codes or for their updating in the future.This leads to an ethically sound working
environment for the programmers working in a team.
Following such coding standards is necessary for the better understanding of the code.
The programmers who work in a team are the real gainers. In a team where sections of code
are shared among members, following the common coding standards is extremely necessary.
The use of descriptive names for variables and methods help to avoid confusion while
integrating the different modules of the code. Using comments, leads to an improved mode of
communication between the programmers. Following the coding standards leads to the proper
diagnosis of codes or for their updating in the future.This leads to an ethically sound working
environment for the programmers working in a team.
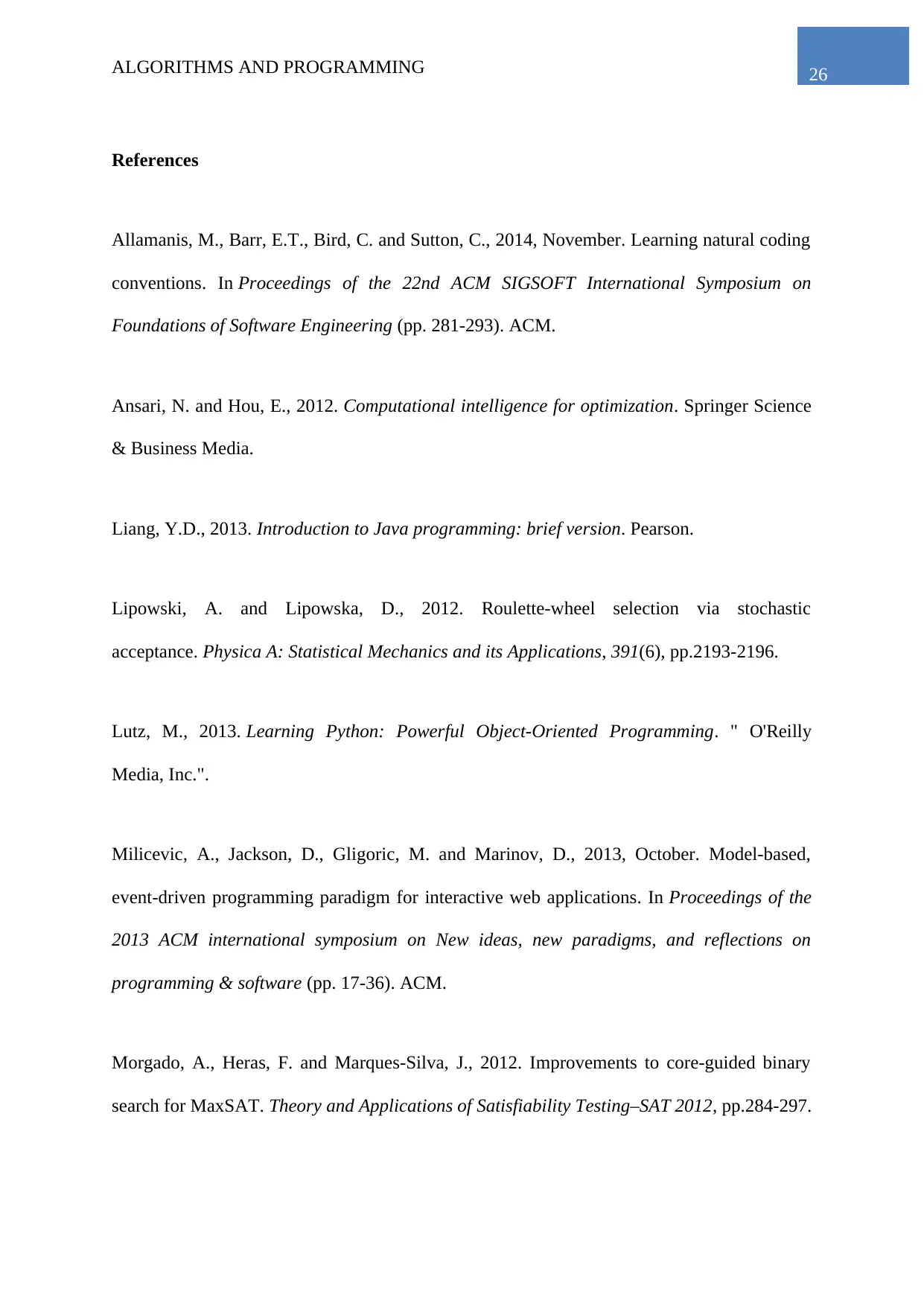
26ALGORITHMS AND PROGRAMMING
References
Allamanis, M., Barr, E.T., Bird, C. and Sutton, C., 2014, November. Learning natural coding
conventions. In Proceedings of the 22nd ACM SIGSOFT International Symposium on
Foundations of Software Engineering (pp. 281-293). ACM.
Ansari, N. and Hou, E., 2012. Computational intelligence for optimization. Springer Science
& Business Media.
Liang, Y.D., 2013. Introduction to Java programming: brief version. Pearson.
Lipowski, A. and Lipowska, D., 2012. Roulette-wheel selection via stochastic
acceptance. Physica A: Statistical Mechanics and its Applications, 391(6), pp.2193-2196.
Lutz, M., 2013. Learning Python: Powerful Object-Oriented Programming. " O'Reilly
Media, Inc.".
Milicevic, A., Jackson, D., Gligoric, M. and Marinov, D., 2013, October. Model-based,
event-driven programming paradigm for interactive web applications. In Proceedings of the
2013 ACM international symposium on New ideas, new paradigms, and reflections on
programming & software (pp. 17-36). ACM.
Morgado, A., Heras, F. and Marques-Silva, J., 2012. Improvements to core-guided binary
search for MaxSAT. Theory and Applications of Satisfiability Testing–SAT 2012, pp.284-297.
References
Allamanis, M., Barr, E.T., Bird, C. and Sutton, C., 2014, November. Learning natural coding
conventions. In Proceedings of the 22nd ACM SIGSOFT International Symposium on
Foundations of Software Engineering (pp. 281-293). ACM.
Ansari, N. and Hou, E., 2012. Computational intelligence for optimization. Springer Science
& Business Media.
Liang, Y.D., 2013. Introduction to Java programming: brief version. Pearson.
Lipowski, A. and Lipowska, D., 2012. Roulette-wheel selection via stochastic
acceptance. Physica A: Statistical Mechanics and its Applications, 391(6), pp.2193-2196.
Lutz, M., 2013. Learning Python: Powerful Object-Oriented Programming. " O'Reilly
Media, Inc.".
Milicevic, A., Jackson, D., Gligoric, M. and Marinov, D., 2013, October. Model-based,
event-driven programming paradigm for interactive web applications. In Proceedings of the
2013 ACM international symposium on New ideas, new paradigms, and reflections on
programming & software (pp. 17-36). ACM.
Morgado, A., Heras, F. and Marques-Silva, J., 2012. Improvements to core-guided binary
search for MaxSAT. Theory and Applications of Satisfiability Testing–SAT 2012, pp.284-297.
1 out of 27
Related Documents
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.