Assignment on Algorithms And Programming
Added on 2020-05-04
27 Pages4937 Words106 Views
Running head: ALGORITHMS AND PROGRAMMING
Algorithms And Programming
Name of the Student:
Name of the University:
Author note:
Algorithms And Programming
Name of the Student:
Name of the University:
Author note:
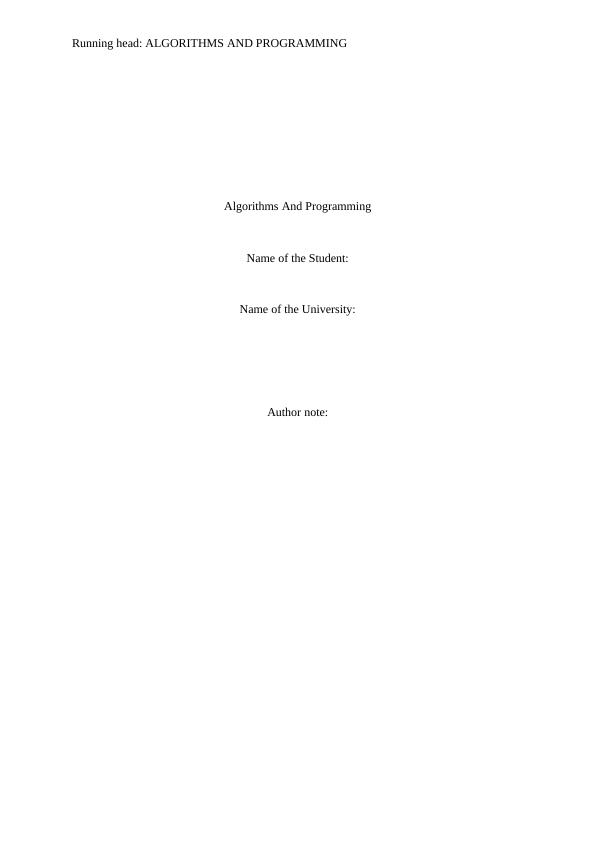
1
ALGORITHMS AND PROGRAMMING
TABLE OF CONTENTS
LO1.................................................................................................. 2
Algorithms................................................................................ 2
Writing simple algorithms........................................................................3
Example: Binary Search...........................................................................4
Process of Developing Applications..........................................................6
LO2.................................................................................................. 8
Programming Paradigms...........................................................8
Procedural Programming..........................................................................8
Object Oriented Programming...............................................................10
Event Driven Programming....................................................................14
LO3................................................................................................ 16
A Mobile Application................................................................16
Aim.........................................................................................................16
Method...................................................................................................17
Source Code...........................................................................................17
Debugging..............................................................................................21
Coding Standards...................................................................................22
ALGORITHMS AND PROGRAMMING
TABLE OF CONTENTS
LO1.................................................................................................. 2
Algorithms................................................................................ 2
Writing simple algorithms........................................................................3
Example: Binary Search...........................................................................4
Process of Developing Applications..........................................................6
LO2.................................................................................................. 8
Programming Paradigms...........................................................8
Procedural Programming..........................................................................8
Object Oriented Programming...............................................................10
Event Driven Programming....................................................................14
LO3................................................................................................ 16
A Mobile Application................................................................16
Aim.........................................................................................................16
Method...................................................................................................17
Source Code...........................................................................................17
Debugging..............................................................................................21
Coding Standards...................................................................................22
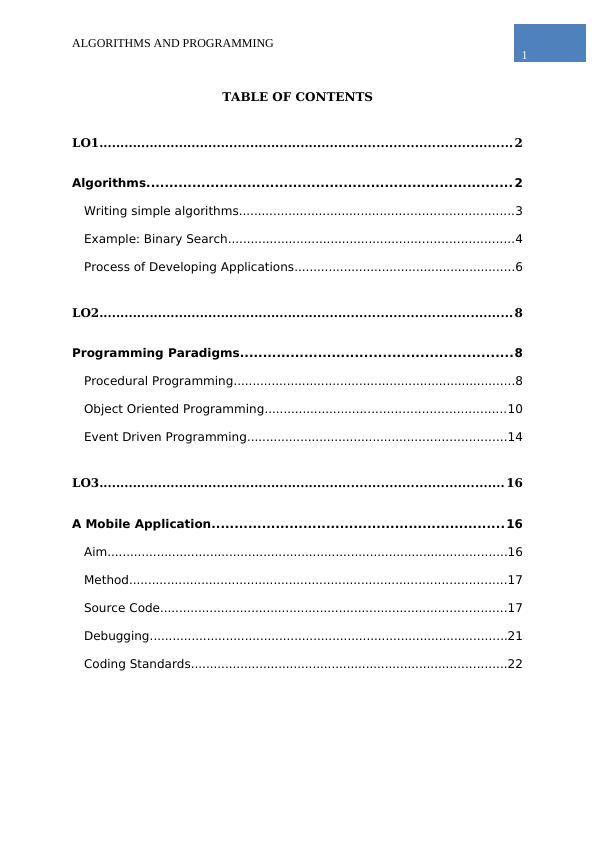
2
ALGORITHMS AND PROGRAMMING
LO1
Algorithms
An algorithm is a computer or mathematical term used in the process of solving
problems. This describes each step that the computer must take or follow to solve particular
class of problems or achieve its goal. Algorithms can perform automated reasoning, data
processing tasks and calculations. It is considered as an effective method, which can be
presented within a finite space and time. Algorithms are generally written in well-defined
formal languages for the calculation of functions. The process starts following algorithms
from the initial state. Inputs are taken, the instructions further describe the procedure of
computation and finally the outputs are delivered. Finite number of successful states are
covered before the terminating the process after reaching the final state.Algorithms are not
necessarily deterministic in their change of state. Randomized algorithms incorporate state
changes through random or variable inputs.
Some basic advantages of writing algorithms are:
Algorithms are stepwise representation of solutions to given problems, thus it is easier
to understand.
These are written in pseudo code therefore, people without the knowledge of
programming language can analyze them.
It is easy to debug algorithms as they have their own logical sequence.
ALGORITHMS AND PROGRAMMING
LO1
Algorithms
An algorithm is a computer or mathematical term used in the process of solving
problems. This describes each step that the computer must take or follow to solve particular
class of problems or achieve its goal. Algorithms can perform automated reasoning, data
processing tasks and calculations. It is considered as an effective method, which can be
presented within a finite space and time. Algorithms are generally written in well-defined
formal languages for the calculation of functions. The process starts following algorithms
from the initial state. Inputs are taken, the instructions further describe the procedure of
computation and finally the outputs are delivered. Finite number of successful states are
covered before the terminating the process after reaching the final state.Algorithms are not
necessarily deterministic in their change of state. Randomized algorithms incorporate state
changes through random or variable inputs.
Some basic advantages of writing algorithms are:
Algorithms are stepwise representation of solutions to given problems, thus it is easier
to understand.
These are written in pseudo code therefore, people without the knowledge of
programming language can analyze them.
It is easy to debug algorithms as they have their own logical sequence.
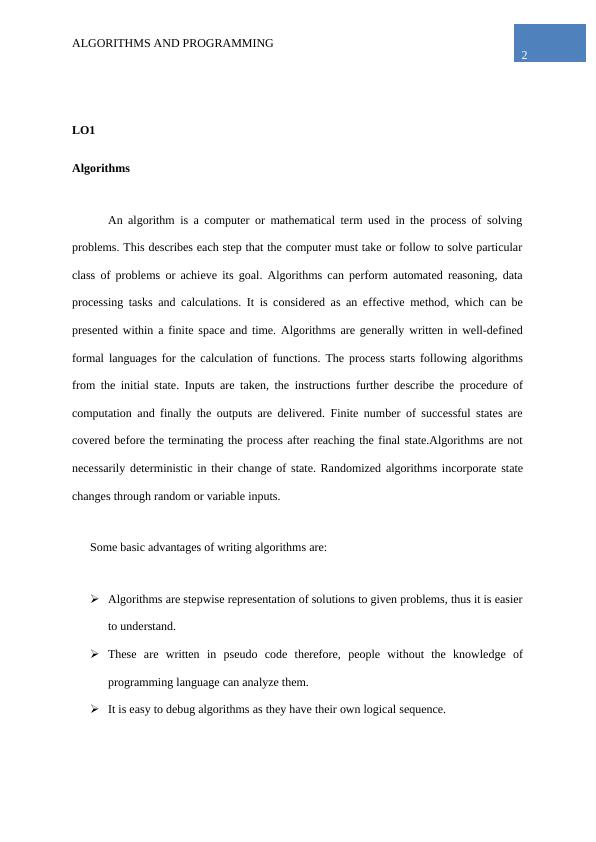
3
ALGORITHMS AND PROGRAMMING
Writing programs becomes easier once the algorithm is ready. The programmer can
study each module of the algorithm separately and code them accordingly.
However, special care must be taken that algorithms are aimed at decreasing the
complexity of problems. Algorithms should be such that, both time and memory usage are
reduced.
Writing simple algorithms
As an example, a simple problem of adding five numbers taken as user input, is
presented using algorithms.
Step 1: START
Step 2: Declare the necessary variables for user input, calculation, loop counter and so
on.
Step 3: Set variable SUM to 0.
Step 4: Set loop counter to 1.
Step 5: If loop counter value is less than or equal to 5, goto Step 5 else goto step 10.
Step 6: Take user input.
Step 7: Add the user input variable to the SUM variable.
Step 8: Store the addition result in the SUM variable itself.
Step 9: Go to step 5.
Step 10: Print SUM.
Step 11: END
ALGORITHMS AND PROGRAMMING
Writing programs becomes easier once the algorithm is ready. The programmer can
study each module of the algorithm separately and code them accordingly.
However, special care must be taken that algorithms are aimed at decreasing the
complexity of problems. Algorithms should be such that, both time and memory usage are
reduced.
Writing simple algorithms
As an example, a simple problem of adding five numbers taken as user input, is
presented using algorithms.
Step 1: START
Step 2: Declare the necessary variables for user input, calculation, loop counter and so
on.
Step 3: Set variable SUM to 0.
Step 4: Set loop counter to 1.
Step 5: If loop counter value is less than or equal to 5, goto Step 5 else goto step 10.
Step 6: Take user input.
Step 7: Add the user input variable to the SUM variable.
Step 8: Store the addition result in the SUM variable itself.
Step 9: Go to step 5.
Step 10: Print SUM.
Step 11: END
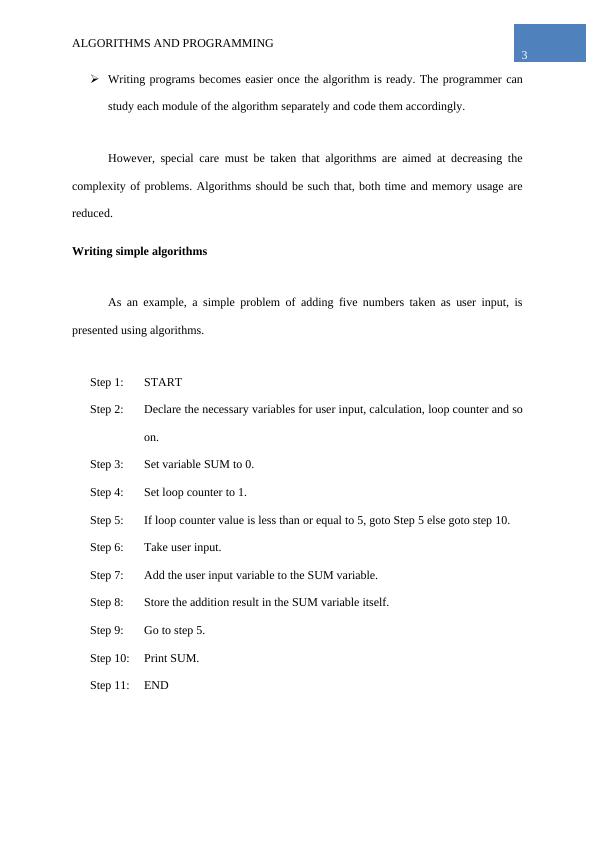
4
ALGORITHMS AND PROGRAMMING
Below, an example of the most commonly used searching algorithm is presented to
discuss the advantage of using algorithms, over brute forcing.
Example: Binary Search
Binary search is the most preferred searching technique. It divides the array into
smaller sections after each iteration, while it approaches to search for the desired element
(Morgado, Heras and Marque-Silva, 2012). The algorithm for this problem is presented and
discussed below:
Given is an array with n number of elements, starting from n0and ending at nn-1. For
this algorithm to work, it is a pre-requirement that the array must be sorted. The algorithm
part for the searching is shown below.
Step 1: Set variable min to 0 and max to n − 1.
Step 2: If min > max, the search terminates as unsuccessful, else go to step 3.
Step 3: Set mid as the position of middle element to (min + max)/2.
Step 4: If Arraymid < Item, set min to mid + 1 and go to step 2.
Step 5: If Arraymid >Item, set max to mid − 1 and go to step 2.
Step 6: Now else if Arraymid = Item, the search is done; return mid+1value and go to
step 7, otherwise go to step 2.
ALGORITHMS AND PROGRAMMING
Below, an example of the most commonly used searching algorithm is presented to
discuss the advantage of using algorithms, over brute forcing.
Example: Binary Search
Binary search is the most preferred searching technique. It divides the array into
smaller sections after each iteration, while it approaches to search for the desired element
(Morgado, Heras and Marque-Silva, 2012). The algorithm for this problem is presented and
discussed below:
Given is an array with n number of elements, starting from n0and ending at nn-1. For
this algorithm to work, it is a pre-requirement that the array must be sorted. The algorithm
part for the searching is shown below.
Step 1: Set variable min to 0 and max to n − 1.
Step 2: If min > max, the search terminates as unsuccessful, else go to step 3.
Step 3: Set mid as the position of middle element to (min + max)/2.
Step 4: If Arraymid < Item, set min to mid + 1 and go to step 2.
Step 5: If Arraymid >Item, set max to mid − 1 and go to step 2.
Step 6: Now else if Arraymid = Item, the search is done; return mid+1value and go to
step 7, otherwise go to step 2.

5
ALGORITHMS AND PROGRAMMING
Step 7: Else,
The linear search technique is considered as a brute forcing concept. In the linear
search technique, the item being searched for is checked with every element in the array from
the very beginning. In the best-case scenario, this is an excellent technique where the element
can be found in the very first index. However, for the worst case, the element would be found
in the very last array index. This is not a time efficient approach. Considering the time
complexity, the linear search approach has a O(n) complexity. Whereas, the binary search has
a O(log n) complexity, which is lesser than that of the brute forcing technique of linear search
(Lipwoski and Lipwoska, 2012).
In this case, where it is necessary to build a mobile-based application or software,
using proper algorithms certainly helps in saving mobile resources. Algorithms that make a
function to work with better efficiency must be preferred over brute force techniques. A well-
planned algorithm not only reduces time complexity but they also tend to minimize memory
complexity whereas, brute forcing may deplete resources and take longer time. This saves
mobile battery and helps user to get their job done with haste and efficiency.
Below, the JAVA code variant of the Binary Search algorithm is presented.
Comments are provided wherever necessary to express the code’s relation with the algorithm.
importjava.util.Scanner;
classBinarySearch
{
public static void main(String args[])
{
inti, min, max, mid, n, search, array[];
Scanner in = new Scanner(System.in);
ALGORITHMS AND PROGRAMMING
Step 7: Else,
The linear search technique is considered as a brute forcing concept. In the linear
search technique, the item being searched for is checked with every element in the array from
the very beginning. In the best-case scenario, this is an excellent technique where the element
can be found in the very first index. However, for the worst case, the element would be found
in the very last array index. This is not a time efficient approach. Considering the time
complexity, the linear search approach has a O(n) complexity. Whereas, the binary search has
a O(log n) complexity, which is lesser than that of the brute forcing technique of linear search
(Lipwoski and Lipwoska, 2012).
In this case, where it is necessary to build a mobile-based application or software,
using proper algorithms certainly helps in saving mobile resources. Algorithms that make a
function to work with better efficiency must be preferred over brute force techniques. A well-
planned algorithm not only reduces time complexity but they also tend to minimize memory
complexity whereas, brute forcing may deplete resources and take longer time. This saves
mobile battery and helps user to get their job done with haste and efficiency.
Below, the JAVA code variant of the Binary Search algorithm is presented.
Comments are provided wherever necessary to express the code’s relation with the algorithm.
importjava.util.Scanner;
classBinarySearch
{
public static void main(String args[])
{
inti, min, max, mid, n, search, array[];
Scanner in = new Scanner(System.in);

End of preview
Want to access all the pages? Upload your documents or become a member.
Related Documents
Temperature Recording Program: Java Implementation for Daily and Weekly Temperature Trackinglg...
|7
|1100
|494
Algorithm vs Code: Understanding the Relationship and Processlg...
|36
|7367
|30
Case Study: Wage Commission Application Developmentlg...
|28
|5209
|493
Benefits of Using Binary Arithmetic Systemlg...
|10
|2213
|290
Assignment on Algorithm PDFlg...
|11
|1949
|86
The Internet runs on algorithmslg...
|9
|1989
|18