C++ Linked List Math Formula Editor
VerifiedAdded on  2019/09/19
|3
|1237
|618
Project
AI Summary
The assignment requires students to design and implement a C++ program using Microsoft Visual Studio 2015 (MSVS) that stores and displays mathematical symbol objects using a linked list. The program will allow users to create, edit, delete, and display a set of mathematical formulae objects, including constants, variables, operators, left and right brackets. The user interface will include options such as quit, insert an object, delete an object, edit, contents, save to file, load from file, replace a constant/variable/operator object, and verify formula. The program will also include error checking and testing for correct operation.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
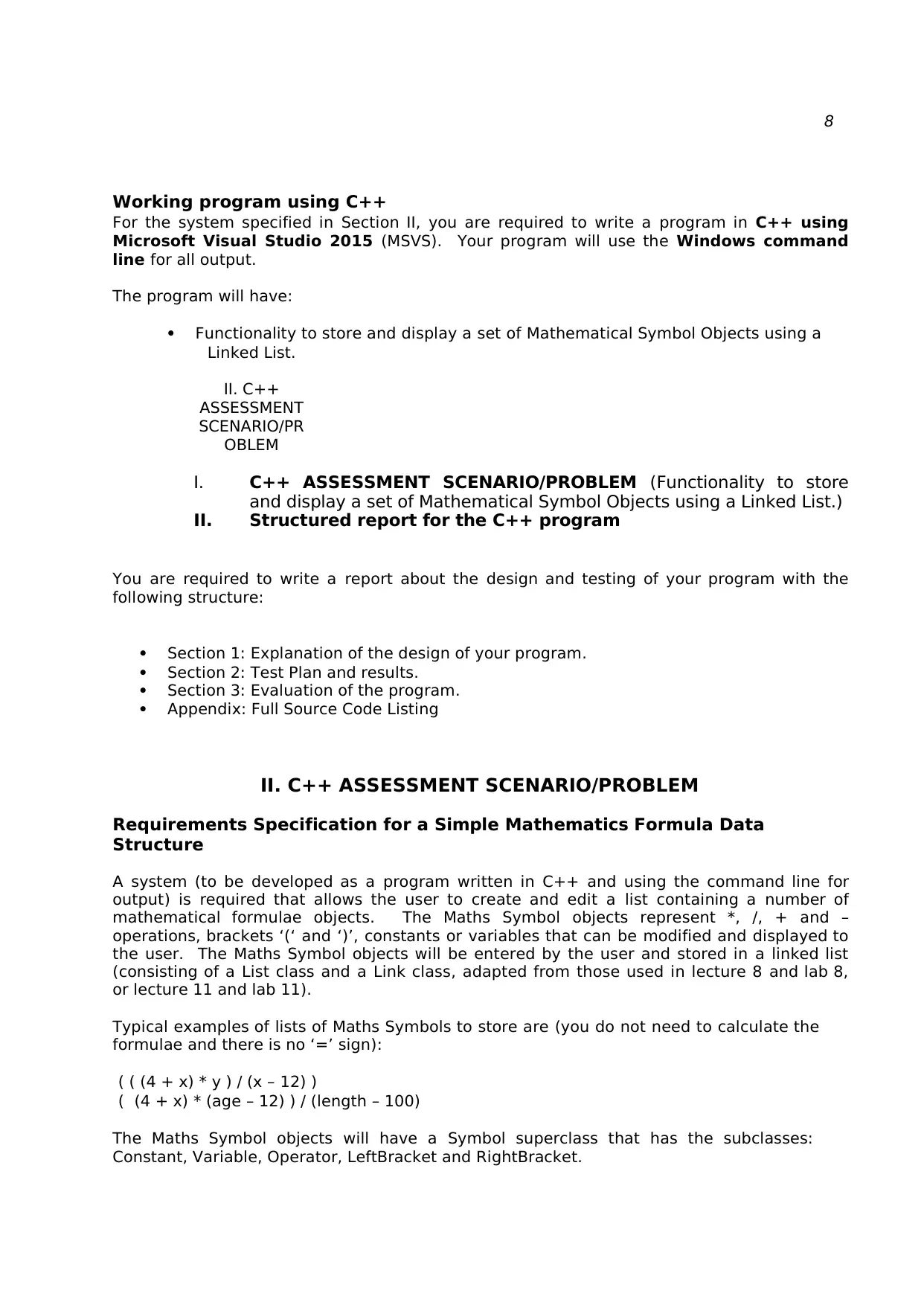
8
Working program using C++
For the system specified in Section II, you are required to write a program in C++ using
Microsoft Visual Studio 2015 (MSVS). Your program will use the Windows command
line for all output.
The program will have:
ï‚· Functionality to store and display a set of Mathematical Symbol Objects using a
Linked List.
II. C++
ASSESSMENT
SCENARIO/PR
OBLEM
I. C++ ASSESSMENT SCENARIO/PROBLEM (Functionality to store
and display a set of Mathematical Symbol Objects using a Linked List.)
II. Structured report for the C++ program
You are required to write a report about the design and testing of your program with the
following structure:
ï‚· Section 1: Explanation of the design of your program.
ï‚· Section 2: Test Plan and results.
ï‚· Section 3: Evaluation of the program.
ï‚· Appendix: Full Source Code Listing
II. C++ ASSESSMENT S CE NARIO/ PROBLE M
Requirements Specification for a Simple Mathematics Formula Data
Structure
A system (to be developed as a program written in C++ and using the command line for
output) is required that allows the user to create and edit a list containing a number of
mathematical formulae objects. The Maths Symbol objects represent *, /, + and –
operations, brackets ‘(‘ and ‘)’, constants or variables that can be modified and displayed to
the user. The Maths Symbol objects will be entered by the user and stored in a linked list
(consisting of a List class and a Link class, adapted from those used in lecture 8 and lab 8,
or lecture 11 and lab 11).
Typical examples of lists of Maths Symbols to store are (you do not need to calculate the
formulae and there is no ‘=’ sign):
( ( (4 + x) * y ) / (x – 12) )
( (4 + x) * (age – 12) ) / (length – 100)
The Maths Symbol objects will have a Symbol superclass that has the subclasses:
Constant, Variable, Operator, LeftBracket and RightBracket.
Working program using C++
For the system specified in Section II, you are required to write a program in C++ using
Microsoft Visual Studio 2015 (MSVS). Your program will use the Windows command
line for all output.
The program will have:
ï‚· Functionality to store and display a set of Mathematical Symbol Objects using a
Linked List.
II. C++
ASSESSMENT
SCENARIO/PR
OBLEM
I. C++ ASSESSMENT SCENARIO/PROBLEM (Functionality to store
and display a set of Mathematical Symbol Objects using a Linked List.)
II. Structured report for the C++ program
You are required to write a report about the design and testing of your program with the
following structure:
ï‚· Section 1: Explanation of the design of your program.
ï‚· Section 2: Test Plan and results.
ï‚· Section 3: Evaluation of the program.
ï‚· Appendix: Full Source Code Listing
II. C++ ASSESSMENT S CE NARIO/ PROBLE M
Requirements Specification for a Simple Mathematics Formula Data
Structure
A system (to be developed as a program written in C++ and using the command line for
output) is required that allows the user to create and edit a list containing a number of
mathematical formulae objects. The Maths Symbol objects represent *, /, + and –
operations, brackets ‘(‘ and ‘)’, constants or variables that can be modified and displayed to
the user. The Maths Symbol objects will be entered by the user and stored in a linked list
(consisting of a List class and a Link class, adapted from those used in lecture 8 and lab 8,
or lecture 11 and lab 11).
Typical examples of lists of Maths Symbols to store are (you do not need to calculate the
formulae and there is no ‘=’ sign):
( ( (4 + x) * y ) / (x – 12) )
( (4 + x) * (age – 12) ) / (length – 100)
The Maths Symbol objects will have a Symbol superclass that has the subclasses:
Constant, Variable, Operator, LeftBracket and RightBracket.
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
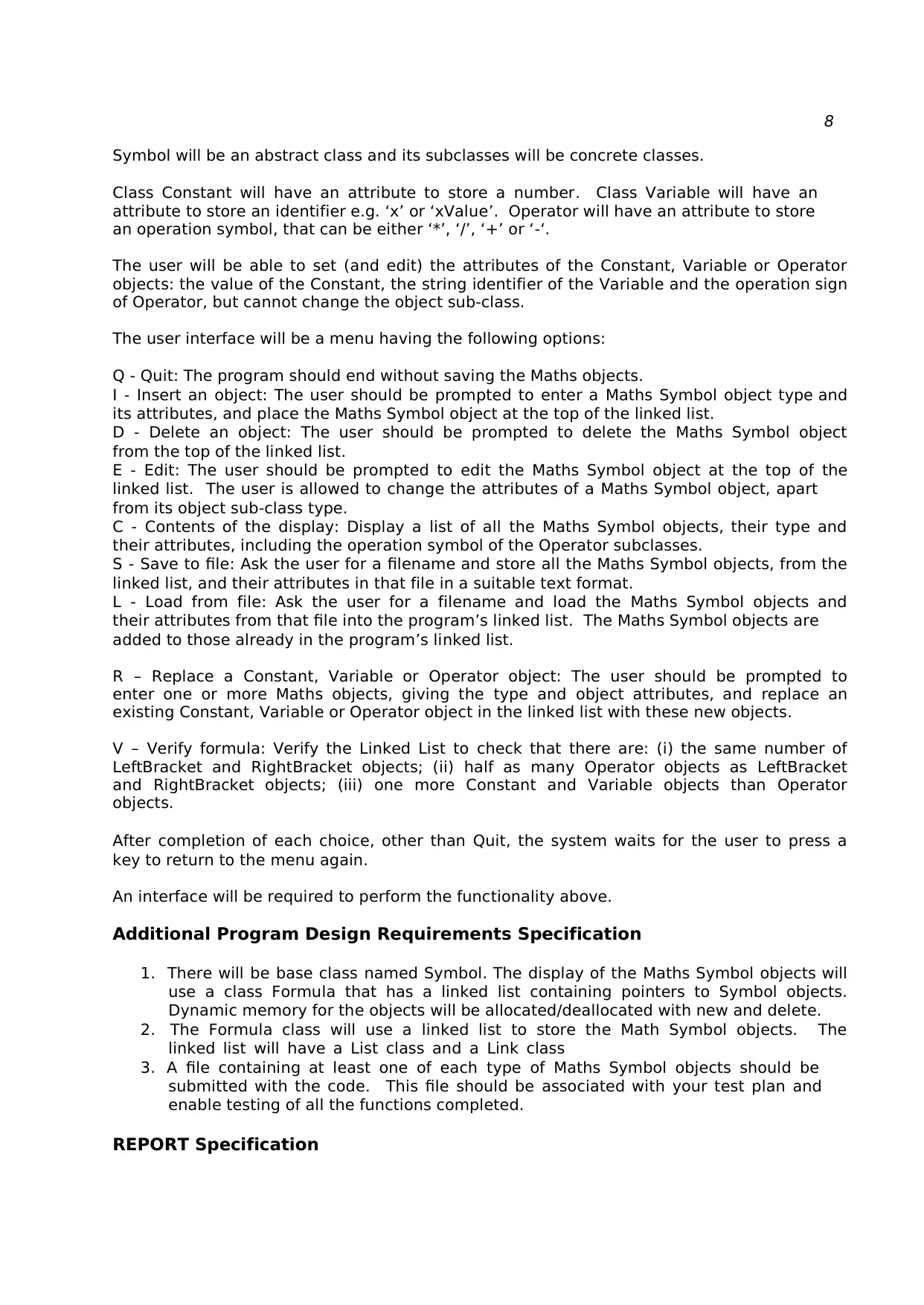
8
Symbol will be an abstract class and its subclasses will be concrete classes.
Class Constant will have an attribute to store a number. Class Variable will have an
attribute to store an identifier e.g. ‘x’ or ‘xValue’. Operator will have an attribute to store
an operation symbol, that can be either ‘*’, ‘/’, ‘+’ or ‘-‘.
The user will be able to set (and edit) the attributes of the Constant, Variable or Operator
objects: the value of the Constant, the string identifier of the Variable and the operation sign
of Operator, but cannot change the object sub-class.
The user interface will be a menu having the following options:
Q - Quit: The program should end without saving the Maths objects.
I - Insert an object: The user should be prompted to enter a Maths Symbol object type and
its attributes, and place the Maths Symbol object at the top of the linked list.
D - Delete an object: The user should be prompted to delete the Maths Symbol object
from the top of the linked list.
E - Edit: The user should be prompted to edit the Maths Symbol object at the top of the
linked list. The user is allowed to change the attributes of a Maths Symbol object, apart
from its object sub-class type.
C - Contents of the display: Display a list of all the Maths Symbol objects, their type and
their attributes, including the operation symbol of the Operator subclasses.
S - Save to file: Ask the user for a filename and store all the Maths Symbol objects, from the
linked list, and their attributes in that file in a suitable text format.
L - Load from file: Ask the user for a filename and load the Maths Symbol objects and
their attributes from that file into the program’s linked list. The Maths Symbol objects are
added to those already in the program’s linked list.
R – Replace a Constant, Variable or Operator object: The user should be prompted to
enter one or more Maths objects, giving the type and object attributes, and replace an
existing Constant, Variable or Operator object in the linked list with these new objects.
V – Verify formula: Verify the Linked List to check that there are: (i) the same number of
LeftBracket and RightBracket objects; (ii) half as many Operator objects as LeftBracket
and RightBracket objects; (iii) one more Constant and Variable objects than Operator
objects.
After completion of each choice, other than Quit, the system waits for the user to press a
key to return to the menu again.
An interface will be required to perform the functionality above.
Additional Program Design Requirements Specification
1. There will be base class named Symbol. The display of the Maths Symbol objects will
use a class Formula that has a linked list containing pointers to Symbol objects.
Dynamic memory for the objects will be allocated/deallocated with new and delete.
2. The Formula class will use a linked list to store the Math Symbol objects. The
linked list will have a List class and a Link class
3. A file containing at least one of each type of Maths Symbol objects should be
submitted with the code. This file should be associated with your test plan and
enable testing of all the functions completed.
REPORT Specification
Symbol will be an abstract class and its subclasses will be concrete classes.
Class Constant will have an attribute to store a number. Class Variable will have an
attribute to store an identifier e.g. ‘x’ or ‘xValue’. Operator will have an attribute to store
an operation symbol, that can be either ‘*’, ‘/’, ‘+’ or ‘-‘.
The user will be able to set (and edit) the attributes of the Constant, Variable or Operator
objects: the value of the Constant, the string identifier of the Variable and the operation sign
of Operator, but cannot change the object sub-class.
The user interface will be a menu having the following options:
Q - Quit: The program should end without saving the Maths objects.
I - Insert an object: The user should be prompted to enter a Maths Symbol object type and
its attributes, and place the Maths Symbol object at the top of the linked list.
D - Delete an object: The user should be prompted to delete the Maths Symbol object
from the top of the linked list.
E - Edit: The user should be prompted to edit the Maths Symbol object at the top of the
linked list. The user is allowed to change the attributes of a Maths Symbol object, apart
from its object sub-class type.
C - Contents of the display: Display a list of all the Maths Symbol objects, their type and
their attributes, including the operation symbol of the Operator subclasses.
S - Save to file: Ask the user for a filename and store all the Maths Symbol objects, from the
linked list, and their attributes in that file in a suitable text format.
L - Load from file: Ask the user for a filename and load the Maths Symbol objects and
their attributes from that file into the program’s linked list. The Maths Symbol objects are
added to those already in the program’s linked list.
R – Replace a Constant, Variable or Operator object: The user should be prompted to
enter one or more Maths objects, giving the type and object attributes, and replace an
existing Constant, Variable or Operator object in the linked list with these new objects.
V – Verify formula: Verify the Linked List to check that there are: (i) the same number of
LeftBracket and RightBracket objects; (ii) half as many Operator objects as LeftBracket
and RightBracket objects; (iii) one more Constant and Variable objects than Operator
objects.
After completion of each choice, other than Quit, the system waits for the user to press a
key to return to the menu again.
An interface will be required to perform the functionality above.
Additional Program Design Requirements Specification
1. There will be base class named Symbol. The display of the Maths Symbol objects will
use a class Formula that has a linked list containing pointers to Symbol objects.
Dynamic memory for the objects will be allocated/deallocated with new and delete.
2. The Formula class will use a linked list to store the Math Symbol objects. The
linked list will have a List class and a Link class
3. A file containing at least one of each type of Maths Symbol objects should be
submitted with the code. This file should be associated with your test plan and
enable testing of all the functions completed.
REPORT Specification
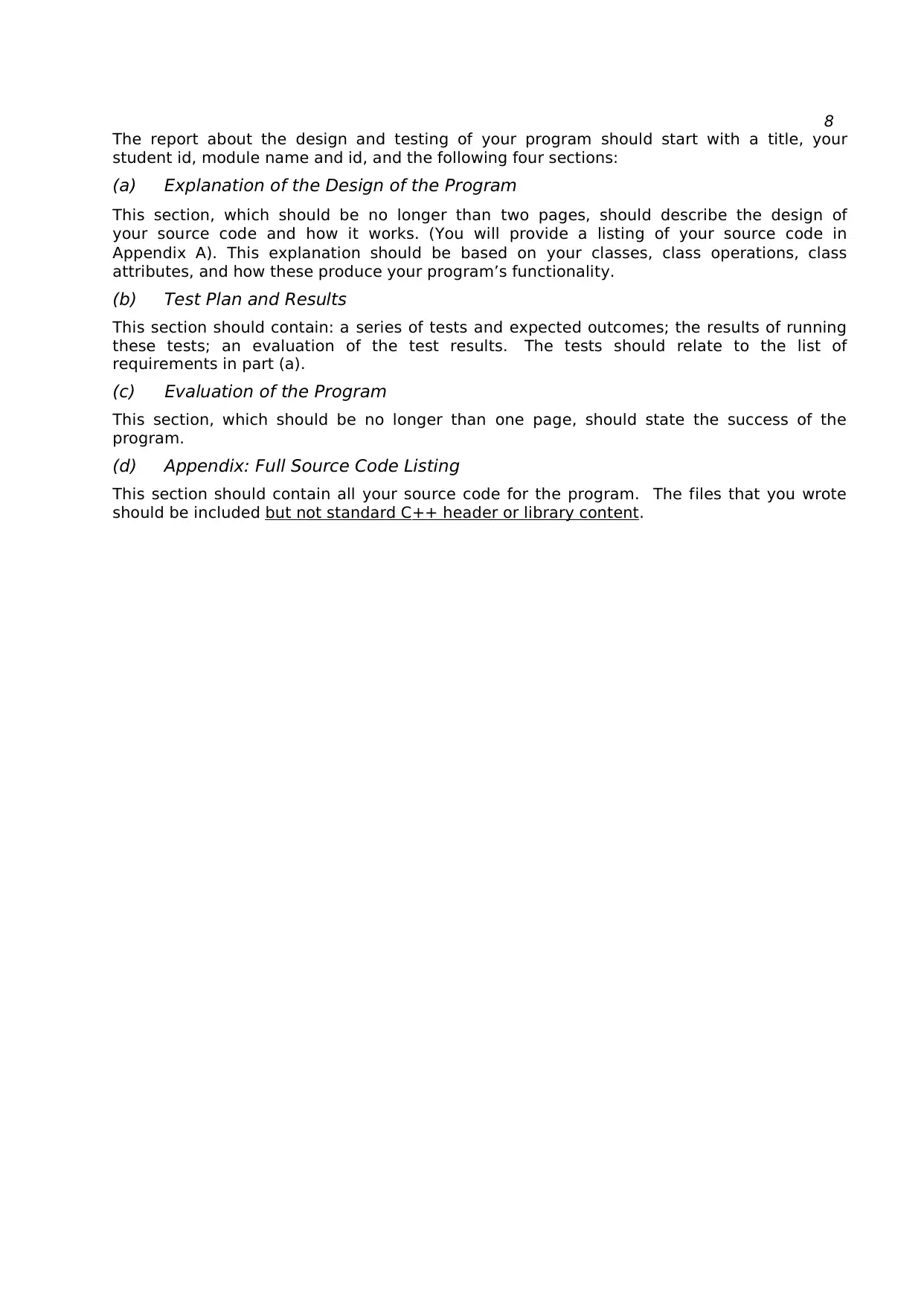
8
The report about the design and testing of your program should start with a title, your
student id, module name and id, and the following four sections:
(a) Explanation of the Design of the Program
This section, which should be no longer than two pages, should describe the design of
your source code and how it works. (You will provide a listing of your source code in
Appendix A). This explanation should be based on your classes, class operations, class
attributes, and how these produce your program’s functionality.
(b) Test Plan and Results
This section should contain: a series of tests and expected outcomes; the results of running
these tests; an evaluation of the test results. The tests should relate to the list of
requirements in part (a).
(c) Evaluation of the Program
This section, which should be no longer than one page, should state the success of the
program.
(d) Appendix: Full Source Code Listing
This section should contain all your source code for the program. The files that you wrote
should be included but not standard C++ header or library content.
The report about the design and testing of your program should start with a title, your
student id, module name and id, and the following four sections:
(a) Explanation of the Design of the Program
This section, which should be no longer than two pages, should describe the design of
your source code and how it works. (You will provide a listing of your source code in
Appendix A). This explanation should be based on your classes, class operations, class
attributes, and how these produce your program’s functionality.
(b) Test Plan and Results
This section should contain: a series of tests and expected outcomes; the results of running
these tests; an evaluation of the test results. The tests should relate to the list of
requirements in part (a).
(c) Evaluation of the Program
This section, which should be no longer than one page, should state the success of the
program.
(d) Appendix: Full Source Code Listing
This section should contain all your source code for the program. The files that you wrote
should be included but not standard C++ header or library content.
1 out of 3
Related Documents
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
 +13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024  |  Zucol Services PVT LTD  |  All rights reserved.