Assignment 1: Computers, Data and Programming
VerifiedAdded on 2023/02/01
|6
|1387
|85
AI Summary
This document provides solutions to questions on converting numbers from different bases, coding in a programming language, handling interrupts, and the benefits of multiple-bus architecture.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
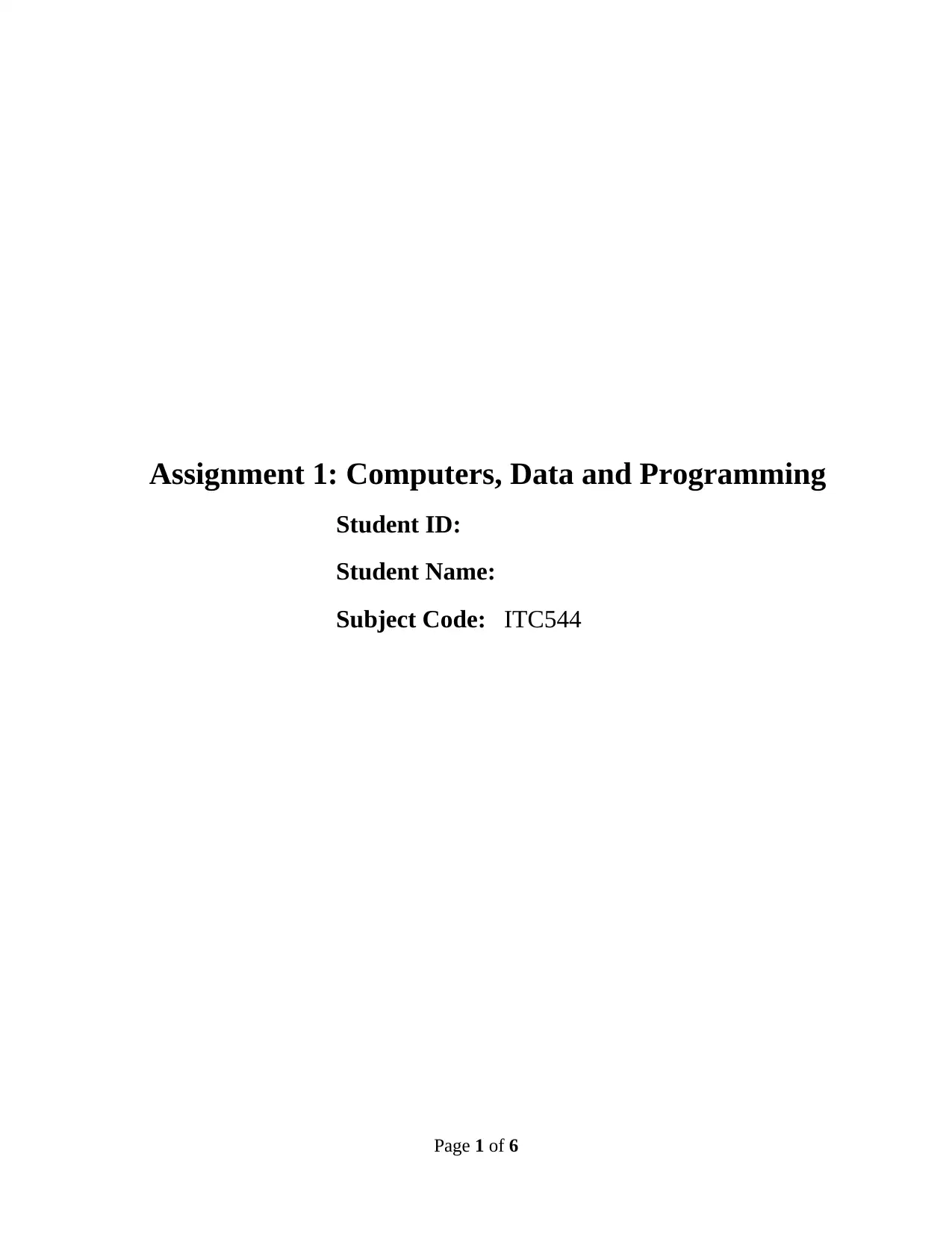
Assignment 1: Computers, Data and Programming
Student ID:
Student Name:
Subject Code: ITC544
Page 1 of 6
Student ID:
Student Name:
Subject Code: ITC544
Page 1 of 6
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
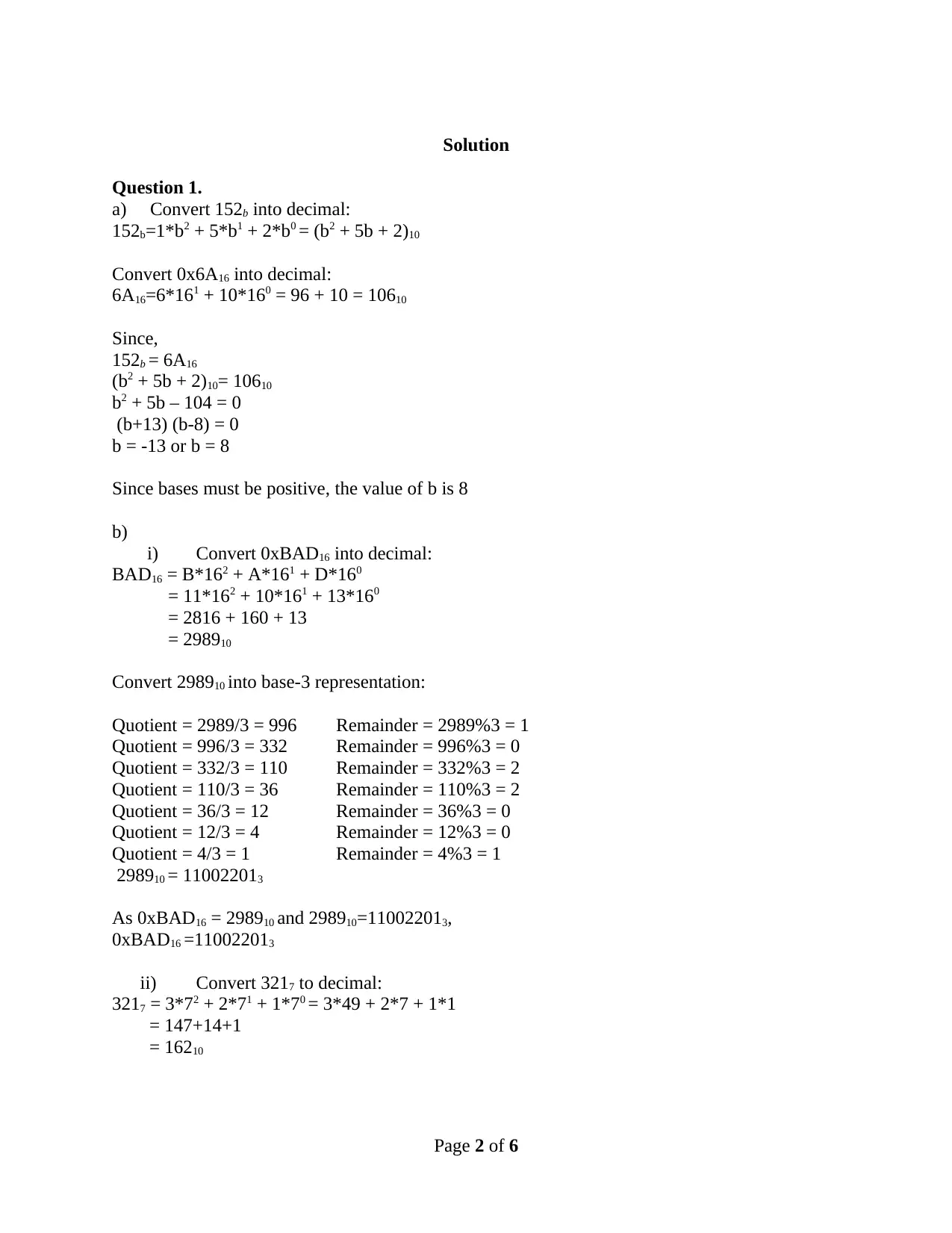
Solution
Question 1.
a) Convert 152b into decimal:
152b=1*b2 + 5*b1 + 2*b0 = (b2 + 5b + 2)10
Convert 0x6A16 into decimal:
6A16=6*161 + 10*160 = 96 + 10 = 10610
Since,
152b = 6A16
(b2 + 5b + 2)10= 10610
b2 + 5b – 104 = 0
(b+13) (b-8) = 0
b = -13 or b = 8
Since bases must be positive, the value of b is 8
b)
i) Convert 0xBAD16 into decimal:
BAD16 = B*162 + A*161 + D*160
= 11*162 + 10*161 + 13*160
= 2816 + 160 + 13
= 298910
Convert 298910 into base-3 representation:
Quotient = 2989/3 = 996 Remainder = 2989%3 = 1
Quotient = 996/3 = 332 Remainder = 996%3 = 0
Quotient = 332/3 = 110 Remainder = 332%3 = 2
Quotient = 110/3 = 36 Remainder = 110%3 = 2
Quotient = 36/3 = 12 Remainder = 36%3 = 0
Quotient = 12/3 = 4 Remainder = 12%3 = 0
Quotient = 4/3 = 1 Remainder = 4%3 = 1
298910 = 110022013
As 0xBAD16 = 298910 and 298910=110022013,
0xBAD16 =110022013
ii) Convert 3217 to decimal:
3217 = 3*72 + 2*71 + 1*70 = 3*49 + 2*7 + 1*1
= 147+14+1
= 16210
Page 2 of 6
Question 1.
a) Convert 152b into decimal:
152b=1*b2 + 5*b1 + 2*b0 = (b2 + 5b + 2)10
Convert 0x6A16 into decimal:
6A16=6*161 + 10*160 = 96 + 10 = 10610
Since,
152b = 6A16
(b2 + 5b + 2)10= 10610
b2 + 5b – 104 = 0
(b+13) (b-8) = 0
b = -13 or b = 8
Since bases must be positive, the value of b is 8
b)
i) Convert 0xBAD16 into decimal:
BAD16 = B*162 + A*161 + D*160
= 11*162 + 10*161 + 13*160
= 2816 + 160 + 13
= 298910
Convert 298910 into base-3 representation:
Quotient = 2989/3 = 996 Remainder = 2989%3 = 1
Quotient = 996/3 = 332 Remainder = 996%3 = 0
Quotient = 332/3 = 110 Remainder = 332%3 = 2
Quotient = 110/3 = 36 Remainder = 110%3 = 2
Quotient = 36/3 = 12 Remainder = 36%3 = 0
Quotient = 12/3 = 4 Remainder = 12%3 = 0
Quotient = 4/3 = 1 Remainder = 4%3 = 1
298910 = 110022013
As 0xBAD16 = 298910 and 298910=110022013,
0xBAD16 =110022013
ii) Convert 3217 to decimal:
3217 = 3*72 + 2*71 + 1*70 = 3*49 + 2*7 + 1*1
= 147+14+1
= 16210
Page 2 of 6
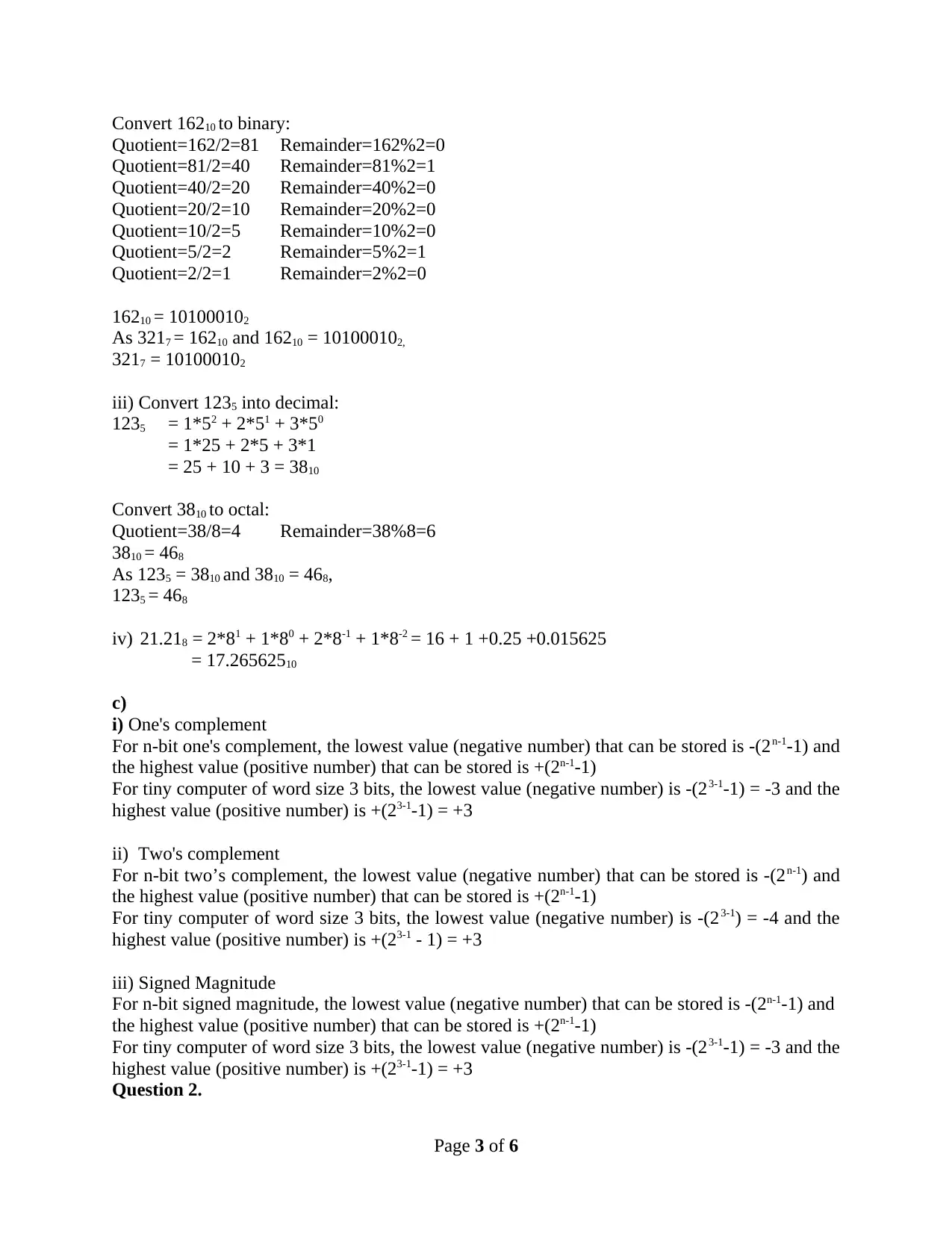
Convert 16210 to binary:
Quotient=162/2=81 Remainder=162%2=0
Quotient=81/2=40 Remainder=81%2=1
Quotient=40/2=20 Remainder=40%2=0
Quotient=20/2=10 Remainder=20%2=0
Quotient=10/2=5 Remainder=10%2=0
Quotient=5/2=2 Remainder=5%2=1
Quotient=2/2=1 Remainder=2%2=0
16210 = 101000102
As 3217 = 16210 and 16210 = 101000102,
3217 = 101000102
iii) Convert 1235 into decimal:
1235 = 1*52 + 2*51 + 3*50
= 1*25 + 2*5 + 3*1
= 25 + 10 + 3 = 3810
Convert 3810 to octal:
Quotient=38/8=4 Remainder=38%8=6
3810 = 468
As 1235 = 3810 and 3810 = 468,
1235 = 468
iv) 21.218 = 2*81 + 1*80 + 2*8-1 + 1*8-2 = 16 + 1 +0.25 +0.015625
= 17.26562510
c)
i) One's complement
For n-bit one's complement, the lowest value (negative number) that can be stored is -(2n-1-1) and
the highest value (positive number) that can be stored is +(2n-1-1)
For tiny computer of word size 3 bits, the lowest value (negative number) is -(23-1-1) = -3 and the
highest value (positive number) is +(23-1-1) = +3
ii) Two's complement
For n-bit two’s complement, the lowest value (negative number) that can be stored is -(2n-1) and
the highest value (positive number) that can be stored is +(2n-1-1)
For tiny computer of word size 3 bits, the lowest value (negative number) is -(23-1) = -4 and the
highest value (positive number) is +(23-1 - 1) = +3
iii) Signed Magnitude
For n-bit signed magnitude, the lowest value (negative number) that can be stored is -(2n-1-1) and
the highest value (positive number) that can be stored is +(2n-1-1)
For tiny computer of word size 3 bits, the lowest value (negative number) is -(23-1-1) = -3 and the
highest value (positive number) is +(23-1-1) = +3
Question 2.
Page 3 of 6
Quotient=162/2=81 Remainder=162%2=0
Quotient=81/2=40 Remainder=81%2=1
Quotient=40/2=20 Remainder=40%2=0
Quotient=20/2=10 Remainder=20%2=0
Quotient=10/2=5 Remainder=10%2=0
Quotient=5/2=2 Remainder=5%2=1
Quotient=2/2=1 Remainder=2%2=0
16210 = 101000102
As 3217 = 16210 and 16210 = 101000102,
3217 = 101000102
iii) Convert 1235 into decimal:
1235 = 1*52 + 2*51 + 3*50
= 1*25 + 2*5 + 3*1
= 25 + 10 + 3 = 3810
Convert 3810 to octal:
Quotient=38/8=4 Remainder=38%8=6
3810 = 468
As 1235 = 3810 and 3810 = 468,
1235 = 468
iv) 21.218 = 2*81 + 1*80 + 2*8-1 + 1*8-2 = 16 + 1 +0.25 +0.015625
= 17.26562510
c)
i) One's complement
For n-bit one's complement, the lowest value (negative number) that can be stored is -(2n-1-1) and
the highest value (positive number) that can be stored is +(2n-1-1)
For tiny computer of word size 3 bits, the lowest value (negative number) is -(23-1-1) = -3 and the
highest value (positive number) is +(23-1-1) = +3
ii) Two's complement
For n-bit two’s complement, the lowest value (negative number) that can be stored is -(2n-1) and
the highest value (positive number) that can be stored is +(2n-1-1)
For tiny computer of word size 3 bits, the lowest value (negative number) is -(23-1) = -4 and the
highest value (positive number) is +(23-1 - 1) = +3
iii) Signed Magnitude
For n-bit signed magnitude, the lowest value (negative number) that can be stored is -(2n-1-1) and
the highest value (positive number) that can be stored is +(2n-1-1)
For tiny computer of word size 3 bits, the lowest value (negative number) is -(23-1-1) = -3 and the
highest value (positive number) is +(23-1-1) = +3
Question 2.
Page 3 of 6
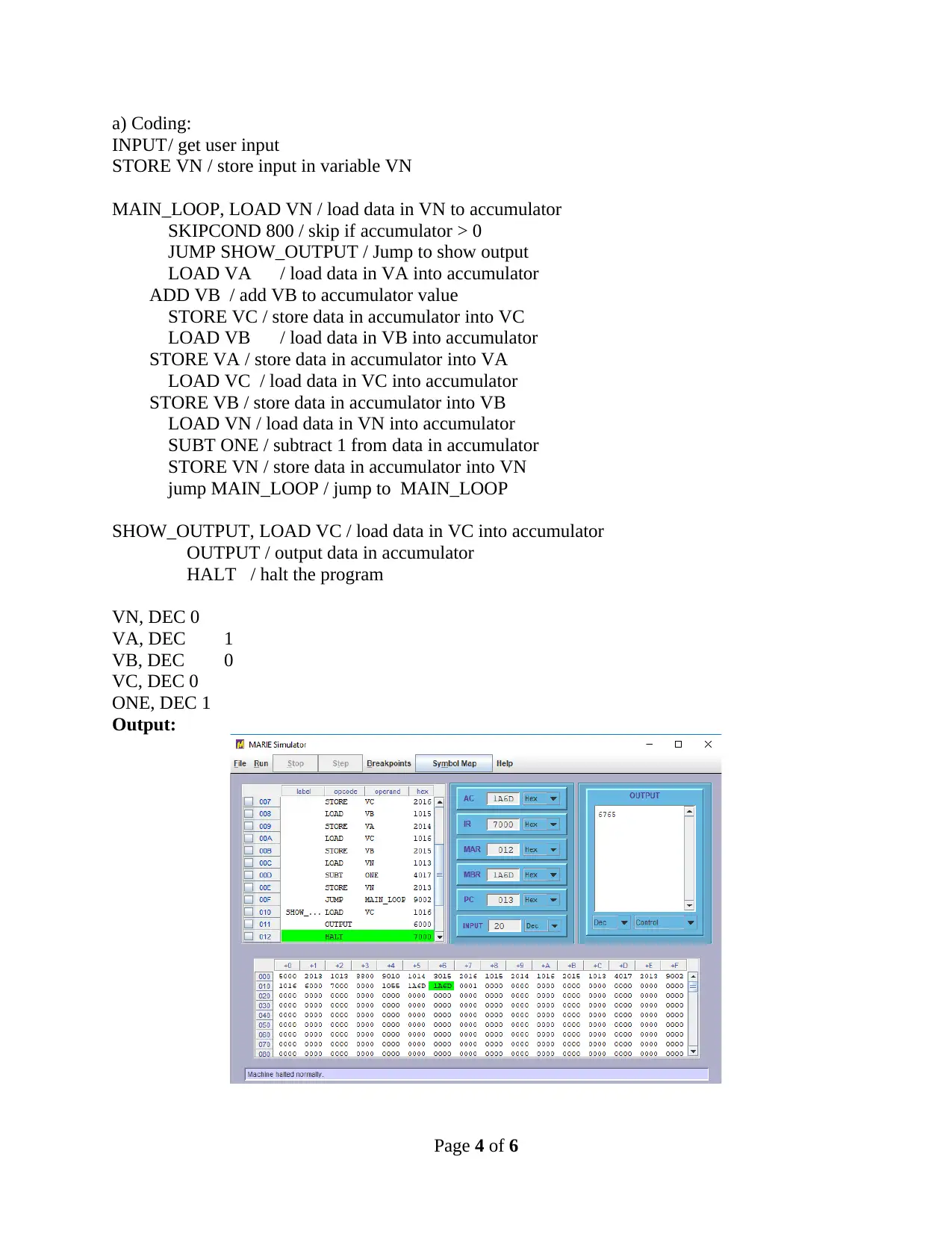
a) Coding:
INPUT/ get user input
STORE VN / store input in variable VN
MAIN_LOOP, LOAD VN / load data in VN to accumulator
SKIPCOND 800 / skip if accumulator > 0
JUMP SHOW_OUTPUT / Jump to show output
LOAD VA / load data in VA into accumulator
ADD VB / add VB to accumulator value
STORE VC / store data in accumulator into VC
LOAD VB / load data in VB into accumulator
STORE VA / store data in accumulator into VA
LOAD VC / load data in VC into accumulator
STORE VB / store data in accumulator into VB
LOAD VN / load data in VN into accumulator
SUBT ONE / subtract 1 from data in accumulator
STORE VN / store data in accumulator into VN
jump MAIN_LOOP / jump to MAIN_LOOP
SHOW_OUTPUT, LOAD VC / load data in VC into accumulator
OUTPUT / output data in accumulator
HALT / halt the program
VN, DEC 0
VA, DEC 1
VB, DEC 0
VC, DEC 0
ONE, DEC 1
Output:
Page 4 of 6
INPUT/ get user input
STORE VN / store input in variable VN
MAIN_LOOP, LOAD VN / load data in VN to accumulator
SKIPCOND 800 / skip if accumulator > 0
JUMP SHOW_OUTPUT / Jump to show output
LOAD VA / load data in VA into accumulator
ADD VB / add VB to accumulator value
STORE VC / store data in accumulator into VC
LOAD VB / load data in VB into accumulator
STORE VA / store data in accumulator into VA
LOAD VC / load data in VC into accumulator
STORE VB / store data in accumulator into VB
LOAD VN / load data in VN into accumulator
SUBT ONE / subtract 1 from data in accumulator
STORE VN / store data in accumulator into VN
jump MAIN_LOOP / jump to MAIN_LOOP
SHOW_OUTPUT, LOAD VC / load data in VC into accumulator
OUTPUT / output data in accumulator
HALT / halt the program
VN, DEC 0
VA, DEC 1
VB, DEC 0
VC, DEC 0
ONE, DEC 1
Output:
Page 4 of 6
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
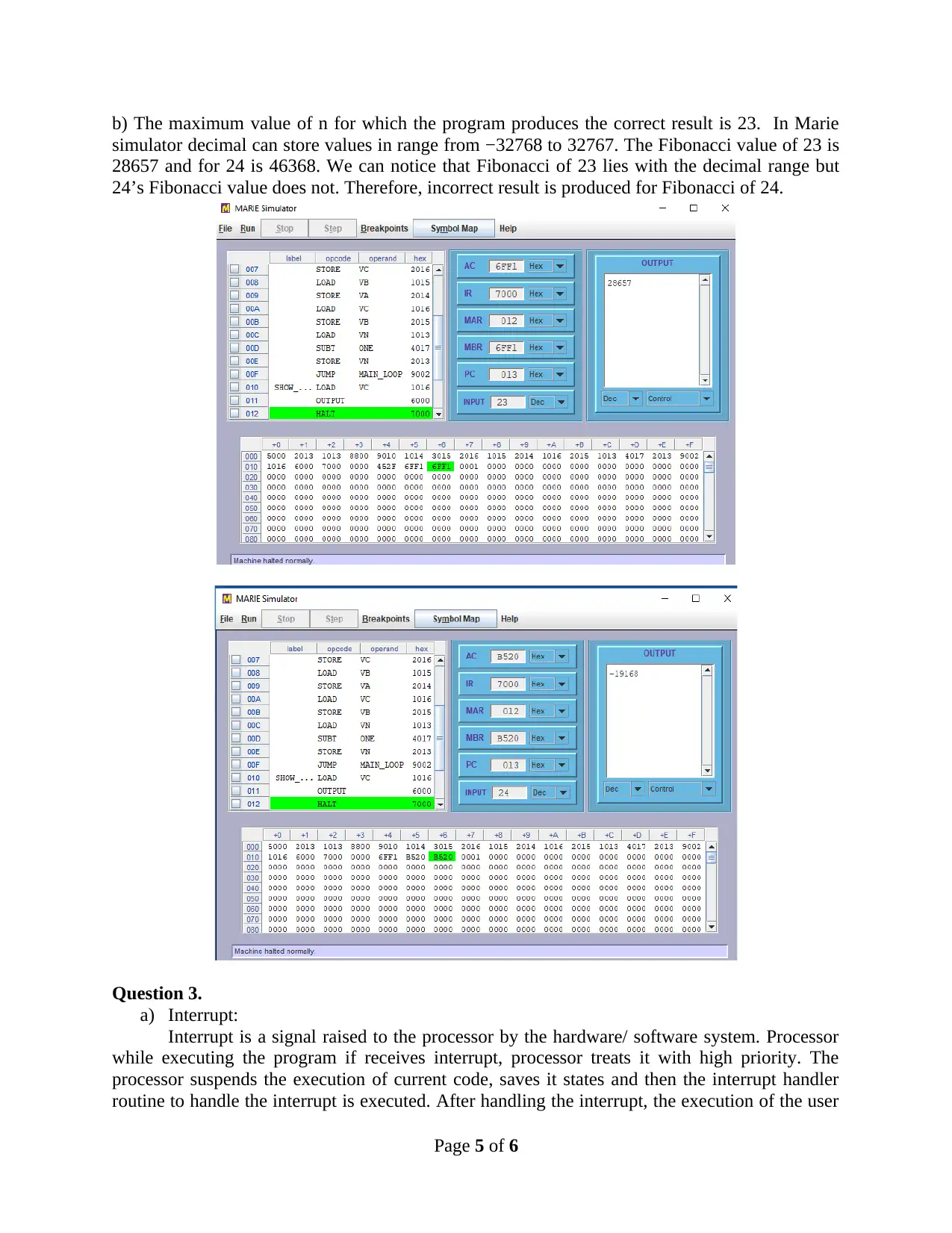
b) The maximum value of n for which the program produces the correct result is 23. In Marie
simulator decimal can store values in range from −32768 to 32767. The Fibonacci value of 23 is
28657 and for 24 is 46368. We can notice that Fibonacci of 23 lies with the decimal range but
24’s Fibonacci value does not. Therefore, incorrect result is produced for Fibonacci of 24.
Question 3.
a) Interrupt:
Interrupt is a signal raised to the processor by the hardware/ software system. Processor
while executing the program if receives interrupt, processor treats it with high priority. The
processor suspends the execution of current code, saves it states and then the interrupt handler
routine to handle the interrupt is executed. After handling the interrupt, the execution of the user
Page 5 of 6
simulator decimal can store values in range from −32768 to 32767. The Fibonacci value of 23 is
28657 and for 24 is 46368. We can notice that Fibonacci of 23 lies with the decimal range but
24’s Fibonacci value does not. Therefore, incorrect result is produced for Fibonacci of 24.
Question 3.
a) Interrupt:
Interrupt is a signal raised to the processor by the hardware/ software system. Processor
while executing the program if receives interrupt, processor treats it with high priority. The
processor suspends the execution of current code, saves it states and then the interrupt handler
routine to handle the interrupt is executed. After handling the interrupt, the execution of the user
Page 5 of 6
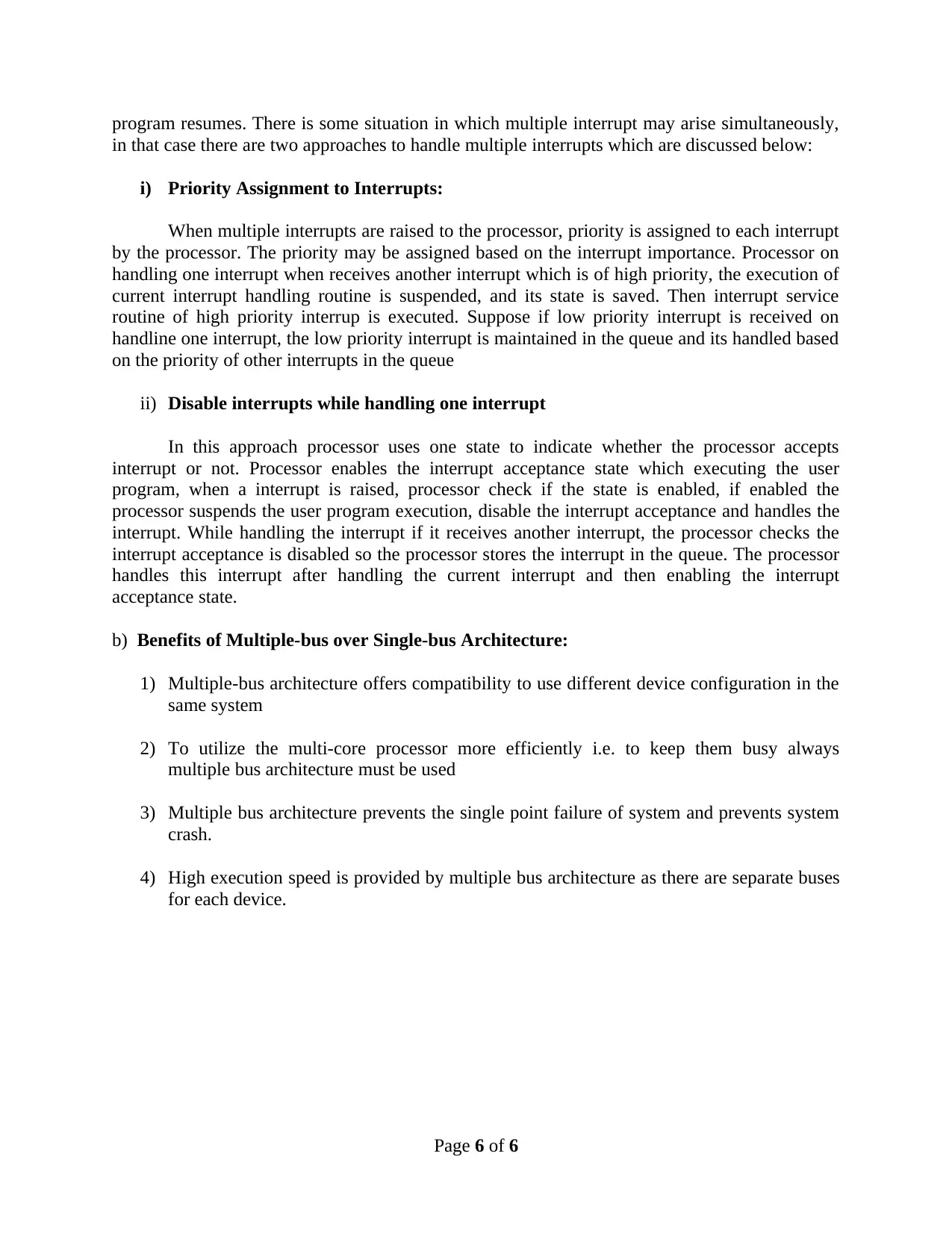
program resumes. There is some situation in which multiple interrupt may arise simultaneously,
in that case there are two approaches to handle multiple interrupts which are discussed below:
i) Priority Assignment to Interrupts:
When multiple interrupts are raised to the processor, priority is assigned to each interrupt
by the processor. The priority may be assigned based on the interrupt importance. Processor on
handling one interrupt when receives another interrupt which is of high priority, the execution of
current interrupt handling routine is suspended, and its state is saved. Then interrupt service
routine of high priority interrup is executed. Suppose if low priority interrupt is received on
handline one interrupt, the low priority interrupt is maintained in the queue and its handled based
on the priority of other interrupts in the queue
ii) Disable interrupts while handling one interrupt
In this approach processor uses one state to indicate whether the processor accepts
interrupt or not. Processor enables the interrupt acceptance state which executing the user
program, when a interrupt is raised, processor check if the state is enabled, if enabled the
processor suspends the user program execution, disable the interrupt acceptance and handles the
interrupt. While handling the interrupt if it receives another interrupt, the processor checks the
interrupt acceptance is disabled so the processor stores the interrupt in the queue. The processor
handles this interrupt after handling the current interrupt and then enabling the interrupt
acceptance state.
b) Benefits of Multiple-bus over Single-bus Architecture:
1) Multiple-bus architecture offers compatibility to use different device configuration in the
same system
2) To utilize the multi-core processor more efficiently i.e. to keep them busy always
multiple bus architecture must be used
3) Multiple bus architecture prevents the single point failure of system and prevents system
crash.
4) High execution speed is provided by multiple bus architecture as there are separate buses
for each device.
Page 6 of 6
in that case there are two approaches to handle multiple interrupts which are discussed below:
i) Priority Assignment to Interrupts:
When multiple interrupts are raised to the processor, priority is assigned to each interrupt
by the processor. The priority may be assigned based on the interrupt importance. Processor on
handling one interrupt when receives another interrupt which is of high priority, the execution of
current interrupt handling routine is suspended, and its state is saved. Then interrupt service
routine of high priority interrup is executed. Suppose if low priority interrupt is received on
handline one interrupt, the low priority interrupt is maintained in the queue and its handled based
on the priority of other interrupts in the queue
ii) Disable interrupts while handling one interrupt
In this approach processor uses one state to indicate whether the processor accepts
interrupt or not. Processor enables the interrupt acceptance state which executing the user
program, when a interrupt is raised, processor check if the state is enabled, if enabled the
processor suspends the user program execution, disable the interrupt acceptance and handles the
interrupt. While handling the interrupt if it receives another interrupt, the processor checks the
interrupt acceptance is disabled so the processor stores the interrupt in the queue. The processor
handles this interrupt after handling the current interrupt and then enabling the interrupt
acceptance state.
b) Benefits of Multiple-bus over Single-bus Architecture:
1) Multiple-bus architecture offers compatibility to use different device configuration in the
same system
2) To utilize the multi-core processor more efficiently i.e. to keep them busy always
multiple bus architecture must be used
3) Multiple bus architecture prevents the single point failure of system and prevents system
crash.
4) High execution speed is provided by multiple bus architecture as there are separate buses
for each device.
Page 6 of 6
1 out of 6
Related Documents
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.