Assignment On Sound Objects & Its Arrays
9 Pages3588 Words296 Views
Added on 2019-09-25
Assignment On Sound Objects & Its Arrays
Added on 2019-09-25
ShareRelated Documents
Assignment 1 – Fridge Magnet Poetry & Song!The main idea behind this assignment is to work with Sound objects and arrays of Sound objects, as well as to use the MIDIPlayer class to program a song. Prep Work1.Download and unzip the file linked at Assignment 1 Starting Code in Canvas. This file, A1Files.zip, when unzipped, will produce three files you’ll need:Assignment1_ITIS1213.javaAudioPoetry.javaCupSong.javaMake sure you remember where you stored the unzipped files.2.For the first three parts of this assignment, you will be working in the Assignment1_ITIS1213.java file (which contains the main method) and the AudioPoetry.java file. (In Part 4, you will need CupSong.java.)3.Create a new Java project in Netbeans, with your name in the project. The project name must follow this format: LastnameFirstname_Assignment1_ITIS1213. So, if Professor Long was doing the assignment, the project would be named: LongBruce_Assignment1_ITIS1213. Make sure to uncheck the “Create Main Class” checkbox before creating the project. 4.You’ll get a new project, and when you open the Source Packages folder in the Netbeans Navigator window, it will show an empty <default package>. Right-click on Source Packages and select New Java Packages. In the dialog that comes up, create a package that has the same name as your project. 5.Copy the assignment files (Assignment1_ITIS1213.java, AudioPoem.java, and CupSong.java)into the package/source file directory on your computer. (If you’re not sure where your NetBeans projects files are, click the File tab in the upper left window of NetBeans (it should be next to the Projects tab), right-click on src, then click Properties. The All Filesline shows you the folder on your hard drive where your source files are stored.) Once you’ve copied those files to your src folder they should automatically appear in your new package under the Projects tab. Open each of these files and change the package name at the top of each file to match the package name in your project.6.Finally, add the MediaCompClasses.jar file to the library for your project. You’re ready to go!Part 1 – Create audio poetry methods (25%)1.Read through the two java files provided to try to understand what they do and how they work together. Assignment1_ITIS1213.java is the test harness that runs the program because it has the main method. The beginning of the main method reads in a number of tmplhe60ogoUNCCMediaComp2Assignment1v0420160924docx-3624.docxPage 1 of 9
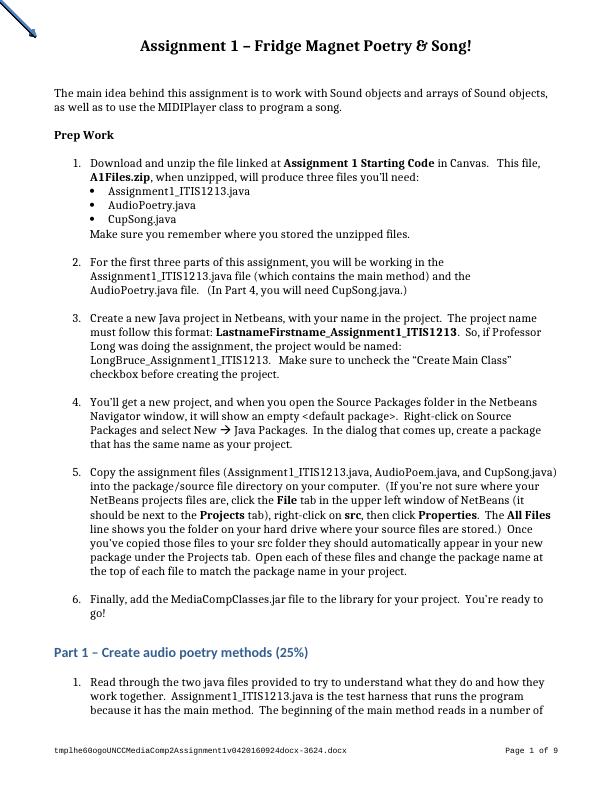
Assignment 1 – Fridge Magnet Poetry & Song!splice points. These are just the sample numbers within the .wav file that indicate where individual words start and stop. The two important lines are at the end of the main method. One creates a new AudioPoem object called myPoem then calls the play()method on the myPoem object. The real processing happens in the AudioPoem class.2.To see how this works, copy the thisisatest_splice.rtf file into your mediasources directory and make sure that you have the thisisatest.wav file in that directory as well.3.In the main method, look for the line near the beginning that sets the value of the String path variable. Change it to the path to yourmediasources directory.4.Run the project to see what it does.a.First you should see a popup window asking you to specify where your main method is. Select Assignment1_ITIS1213.java and click OK. (That should be the last time you see that window.)b.Next, you should see a popup window that asks you to “Please select a sound file ...”. (Look in the upper left of the popup window for the message.) Select and open thisisatest.wav.c.Next, you should see a popup window that asks “Now select the file that contains the splice points...”. Select and open thisisatest_splice.rtf.d.Once you have selected the proper input files you should hear four words slightly separated: this ... is ... a ... test. (That’s one of the authors of the MC textbook speakingby the way.)5.You will notice that running the test harness just plays the sound, using the AudioPoem’s play() method, which is completed for you. The AudioPoem class takes the sound object and the splice points and creates an array of sound objects each element of which is one of the separate words from the sound. The rest of the methods in AudioPoem (look at lines 36 thru 118) are stub methods, which means they exist, but they are empty – there is no code inside of them; they’re just placeholders. These methods are meant to play the individual words in different ways. Your job in Part 1 of the Assignment is to complete these stub methods and test each of them by adding appropriate code to your main method. Look at the Javadoc comments above each stub to understand what each method should do. Look at the code in the play() method to see how to play the four individual sounds one after the other. In your main method pause one second between invoking each of the AudioPoem methods. While you’re testing your methods, you can comment out all the methods that work so you’re only listening to one at a time. When you submit this Assignment remove those comments so you make sure you hear all the methods tested when you run the test harness program.Part 2 – Overload audio poetry methods (25%)Do this part of the assignment once Part 1 is complete.tmplhe60ogoUNCCMediaComp2Assignment1v0420160924docx-3624.docxPage 2 of 9
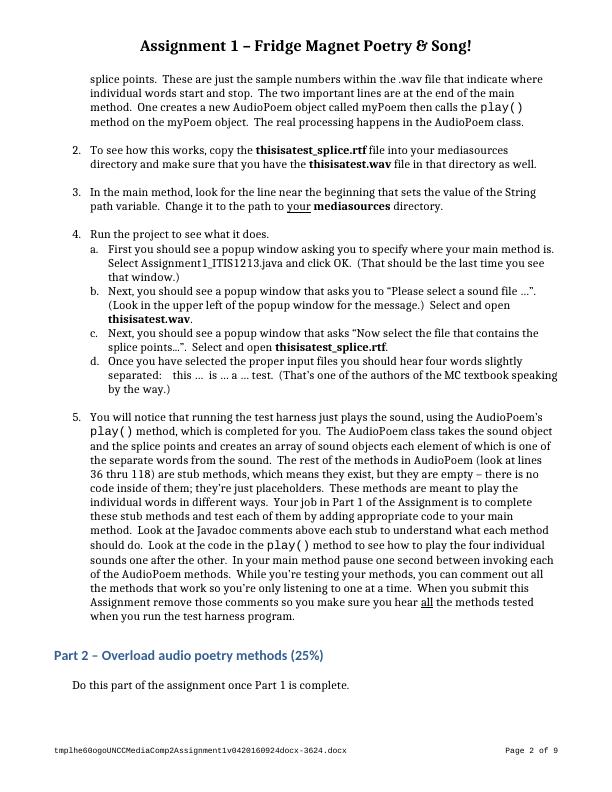
Assignment 1 – Fridge Magnet Poetry & Song!1.Your job is to create overloaded methods in the AudioPoem class. These new methods savethe set of sounds created by the method to a file in a directory you specify. Each of the overloaded methods takes two additional String parameters. These parameters represent the filename and the path to where you are storing files. So, for example, the overloaded method playReverseOrder(int pause, String filename, String path)should play the words in the audio poem in reverse order, but it should also save the new sound that plays the same thing. If you do this correctly, anyone should be able to play the sound file you create and hear the same sounds you did when your method ran. To do this, you will need to create a new sound object to hold the sounds as well as the gaps between the words, copy the sounds (and the gfaps) into it as they are played, and then write that new Sound object out to a file before the method returns.2.When this part of the assignment is done, you should have the following methods working properly (the ones in bold are the new ones that save the sound):play()play(int pause)play(int pause, String filename, String path)playDoublets(int numDoublets)playDoublets(int numDoublets, String filename, String path)playRandomOrder(int totalWords, int pause)playRandomOrder(int totalWords, int pause, String filename, String path)playRandomUnique(int pause)playRandomUnique(int pause, String filename, String path)playReverseOrder(int pause)playReverseOrder(int pause, String filename, String path)playTriplets(int numTriplets)playTriplets(int numTriplets, String filename, String path)3.Note that some of these new methods will be a bit tricky, because you won’t know how big your new sound should be. How many samples do you need for example, for the playRandomOrder sound? It’s going to play a bunch of words, but you don’t know ahead of time, which words, and each word sound contains a different number of samples. Hint: play it safe, and find out which word in the array is longest, and how many samples are in that word. Also, how many samples do you need for a 100 millisecond pause? You’ll need to query the sampling rate to handle this.Hint: You may want to create some private helper methods so that you don’t have to retypea lot of code. For example you may want a calculateNumberOfSilentSamplesmethod and a writeSoundToFile method. Create all the helper methods you like.4.Add appropriate JavaDoc documentation for these methods.5.Test these new overloaded methods by calling them from your test harness file and specifying filenames. You should also listen to the.wav files your code created to make surethey sound exactly the same as when the method is called from your test harness program. (Just play them with whatever your default audio player is on your computer.)tmplhe60ogoUNCCMediaComp2Assignment1v0420160924docx-3624.docxPage 3 of 9
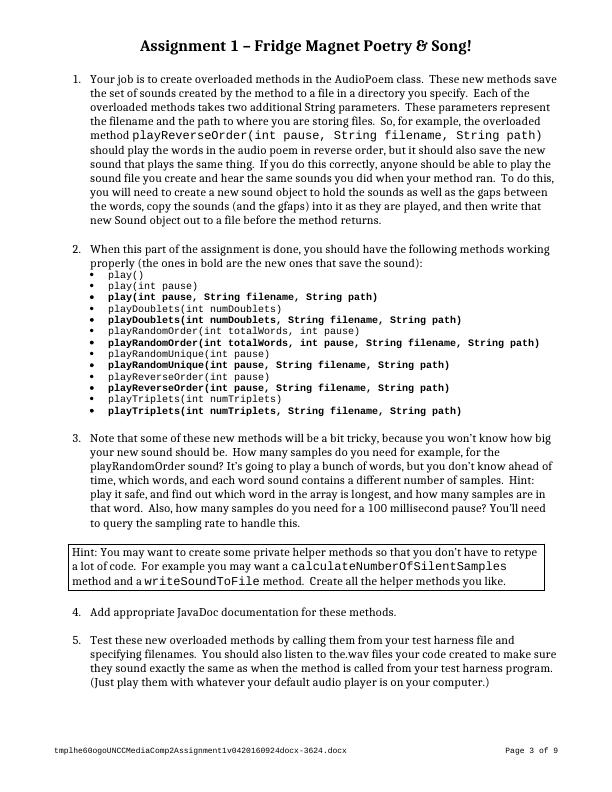
End of preview
Want to access all the pages? Upload your documents or become a member.
Related Documents
Java Programming with Spring Boot Frameworklg...
|5
|580
|442
Hints for completing Assignment 1 for an Android application projectlg...
|9
|4524
|331
MiniJava-Compiler =================.lg...
|4
|450
|88
Visual Studio Project | Assignment On C# Console Applicationlg...
|3
|1002
|81