C++ and Java Programming: Concurrency, Data Sensitivity, Scope Minimization, and Exception Handling
VerifiedAdded on 2022/11/13
|15
|2335
|378
AI Summary
This document discusses the object-oriented programming languages C++ and Java, focusing on concurrency, data sensitivity, scope minimization, and exception handling. It provides insights into the best practices and sample codes for each topic. The document also highlights the benefits of object-oriented programming and how it leads to faster development and easier maintenance of software. The document is a valuable resource for students and professionals alike.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
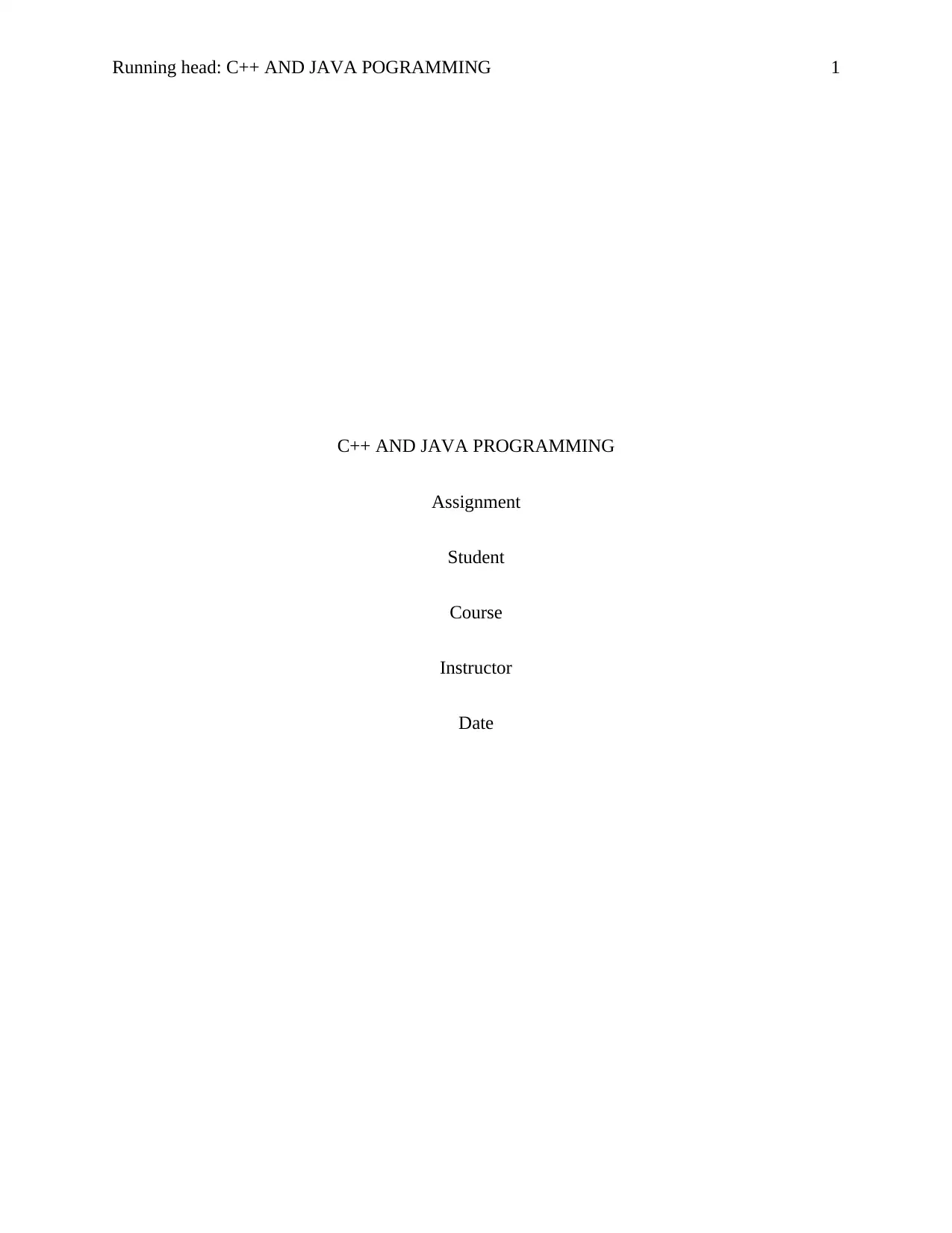
Running head: C++ AND JAVA POGRAMMING 1
C++ AND JAVA PROGRAMMING
Assignment
Student
Course
Instructor
Date
C++ AND JAVA PROGRAMMING
Assignment
Student
Course
Instructor
Date
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
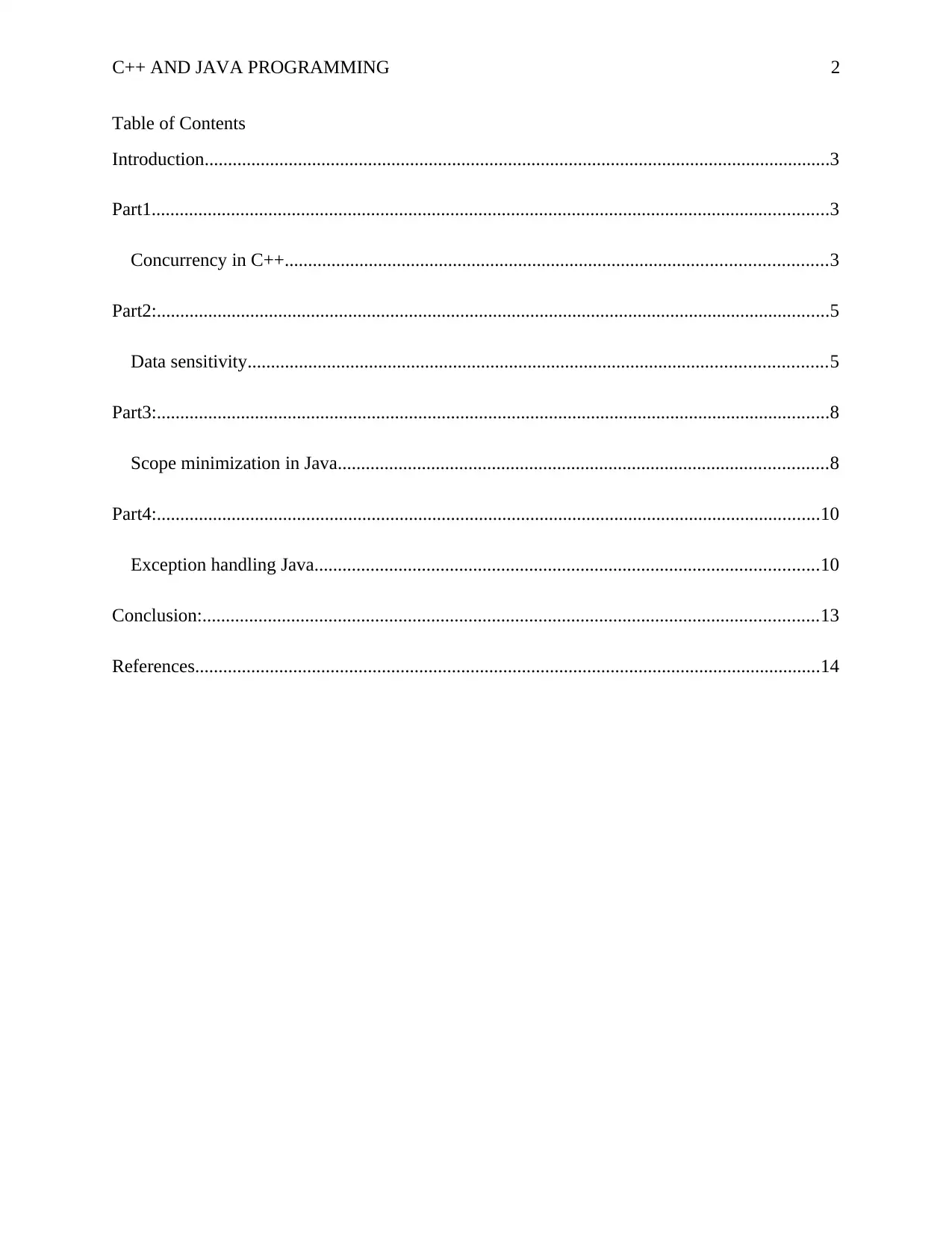
C++ AND JAVA PROGRAMMING 2
Table of Contents
Introduction......................................................................................................................................3
Part1.................................................................................................................................................3
Concurrency in C++....................................................................................................................3
Part2:................................................................................................................................................5
Data sensitivity............................................................................................................................5
Part3:................................................................................................................................................8
Scope minimization in Java.........................................................................................................8
Part4:..............................................................................................................................................10
Exception handling Java............................................................................................................10
Conclusion:....................................................................................................................................13
References......................................................................................................................................14
Table of Contents
Introduction......................................................................................................................................3
Part1.................................................................................................................................................3
Concurrency in C++....................................................................................................................3
Part2:................................................................................................................................................5
Data sensitivity............................................................................................................................5
Part3:................................................................................................................................................8
Scope minimization in Java.........................................................................................................8
Part4:..............................................................................................................................................10
Exception handling Java............................................................................................................10
Conclusion:....................................................................................................................................13
References......................................................................................................................................14
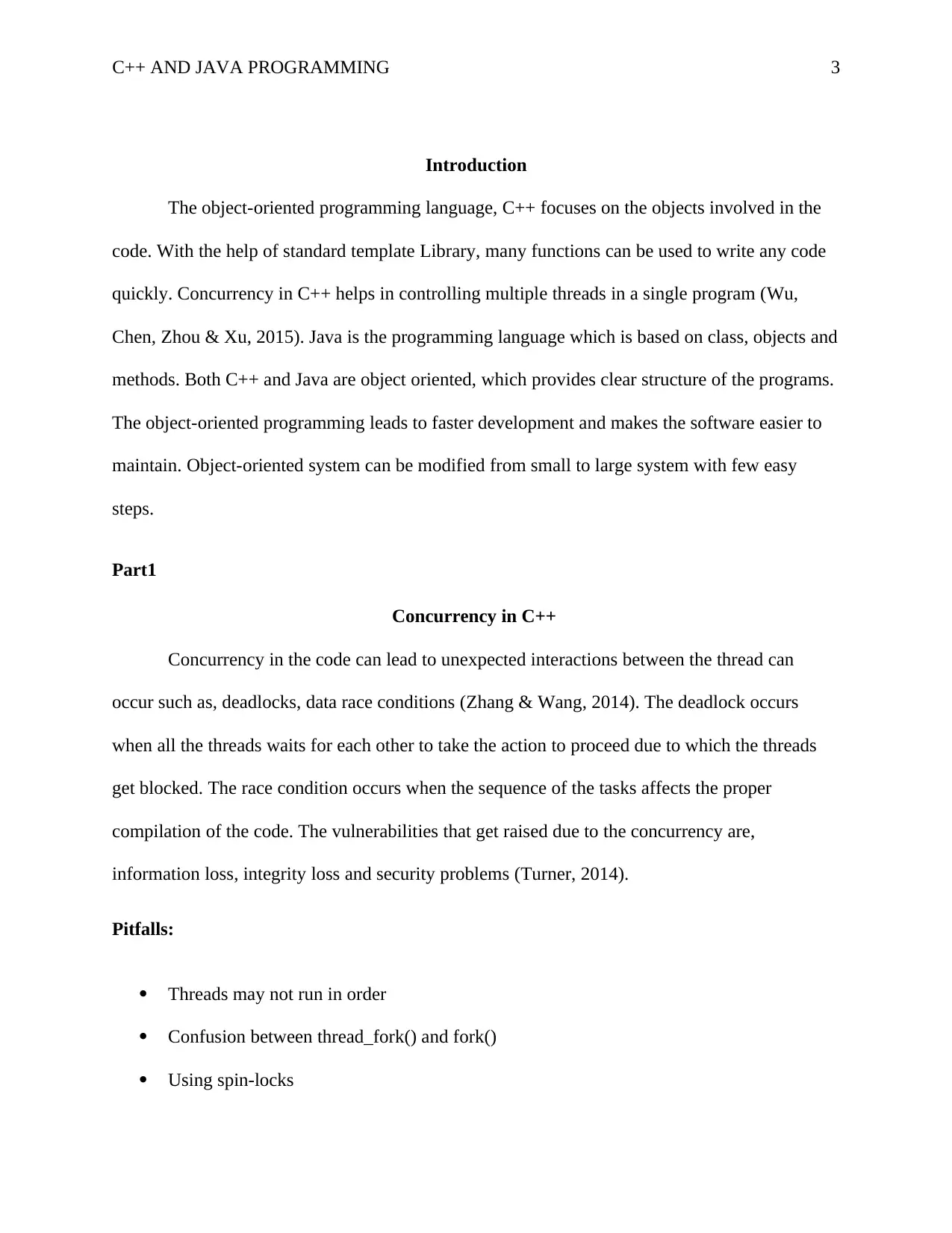
C++ AND JAVA PROGRAMMING 3
Introduction
The object-oriented programming language, C++ focuses on the objects involved in the
code. With the help of standard template Library, many functions can be used to write any code
quickly. Concurrency in C++ helps in controlling multiple threads in a single program (Wu,
Chen, Zhou & Xu, 2015). Java is the programming language which is based on class, objects and
methods. Both C++ and Java are object oriented, which provides clear structure of the programs.
The object-oriented programming leads to faster development and makes the software easier to
maintain. Object-oriented system can be modified from small to large system with few easy
steps.
Part1
Concurrency in C++
Concurrency in the code can lead to unexpected interactions between the thread can
occur such as, deadlocks, data race conditions (Zhang & Wang, 2014). The deadlock occurs
when all the threads waits for each other to take the action to proceed due to which the threads
get blocked. The race condition occurs when the sequence of the tasks affects the proper
compilation of the code. The vulnerabilities that get raised due to the concurrency are,
information loss, integrity loss and security problems (Turner, 2014).
Pitfalls:
Threads may not run in order
Confusion between thread_fork() and fork()
Using spin-locks
Introduction
The object-oriented programming language, C++ focuses on the objects involved in the
code. With the help of standard template Library, many functions can be used to write any code
quickly. Concurrency in C++ helps in controlling multiple threads in a single program (Wu,
Chen, Zhou & Xu, 2015). Java is the programming language which is based on class, objects and
methods. Both C++ and Java are object oriented, which provides clear structure of the programs.
The object-oriented programming leads to faster development and makes the software easier to
maintain. Object-oriented system can be modified from small to large system with few easy
steps.
Part1
Concurrency in C++
Concurrency in the code can lead to unexpected interactions between the thread can
occur such as, deadlocks, data race conditions (Zhang & Wang, 2014). The deadlock occurs
when all the threads waits for each other to take the action to proceed due to which the threads
get blocked. The race condition occurs when the sequence of the tasks affects the proper
compilation of the code. The vulnerabilities that get raised due to the concurrency are,
information loss, integrity loss and security problems (Turner, 2014).
Pitfalls:
Threads may not run in order
Confusion between thread_fork() and fork()
Using spin-locks
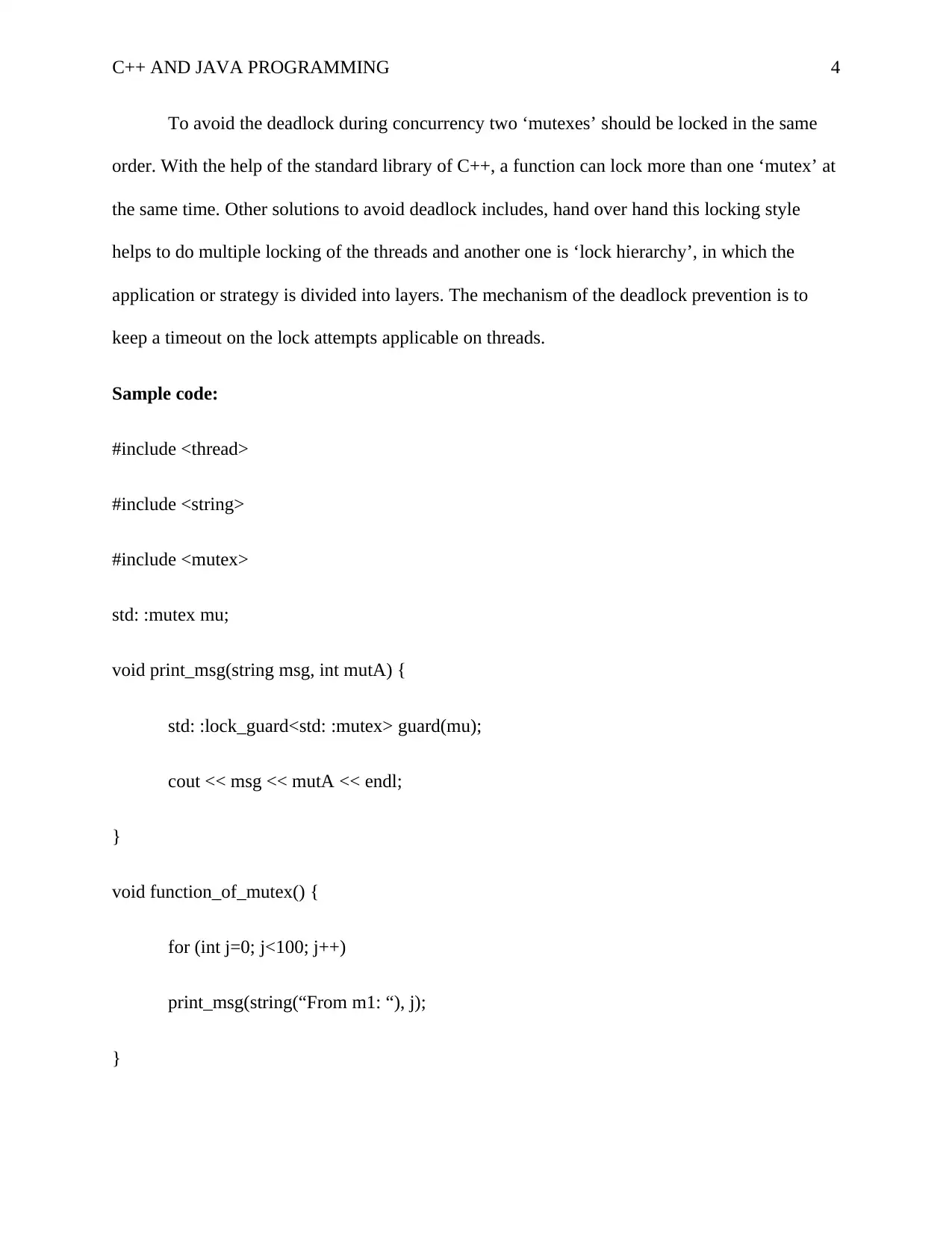
C++ AND JAVA PROGRAMMING 4
To avoid the deadlock during concurrency two ‘mutexes’ should be locked in the same
order. With the help of the standard library of C++, a function can lock more than one ‘mutex’ at
the same time. Other solutions to avoid deadlock includes, hand over hand this locking style
helps to do multiple locking of the threads and another one is ‘lock hierarchy’, in which the
application or strategy is divided into layers. The mechanism of the deadlock prevention is to
keep a timeout on the lock attempts applicable on threads.
Sample code:
#include <thread>
#include <string>
#include <mutex>
std: :mutex mu;
void print_msg(string msg, int mutA) {
std: :lock_guard<std: :mutex> guard(mu);
cout << msg << mutA << endl;
}
void function_of_mutex() {
for (int j=0; j<100; j++)
print_msg(string(“From m1: “), j);
}
To avoid the deadlock during concurrency two ‘mutexes’ should be locked in the same
order. With the help of the standard library of C++, a function can lock more than one ‘mutex’ at
the same time. Other solutions to avoid deadlock includes, hand over hand this locking style
helps to do multiple locking of the threads and another one is ‘lock hierarchy’, in which the
application or strategy is divided into layers. The mechanism of the deadlock prevention is to
keep a timeout on the lock attempts applicable on threads.
Sample code:
#include <thread>
#include <string>
#include <mutex>
std: :mutex mu;
void print_msg(string msg, int mutA) {
std: :lock_guard<std: :mutex> guard(mu);
cout << msg << mutA << endl;
}
void function_of_mutex() {
for (int j=0; j<100; j++)
print_msg(string(“From m1: “), j);
}
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
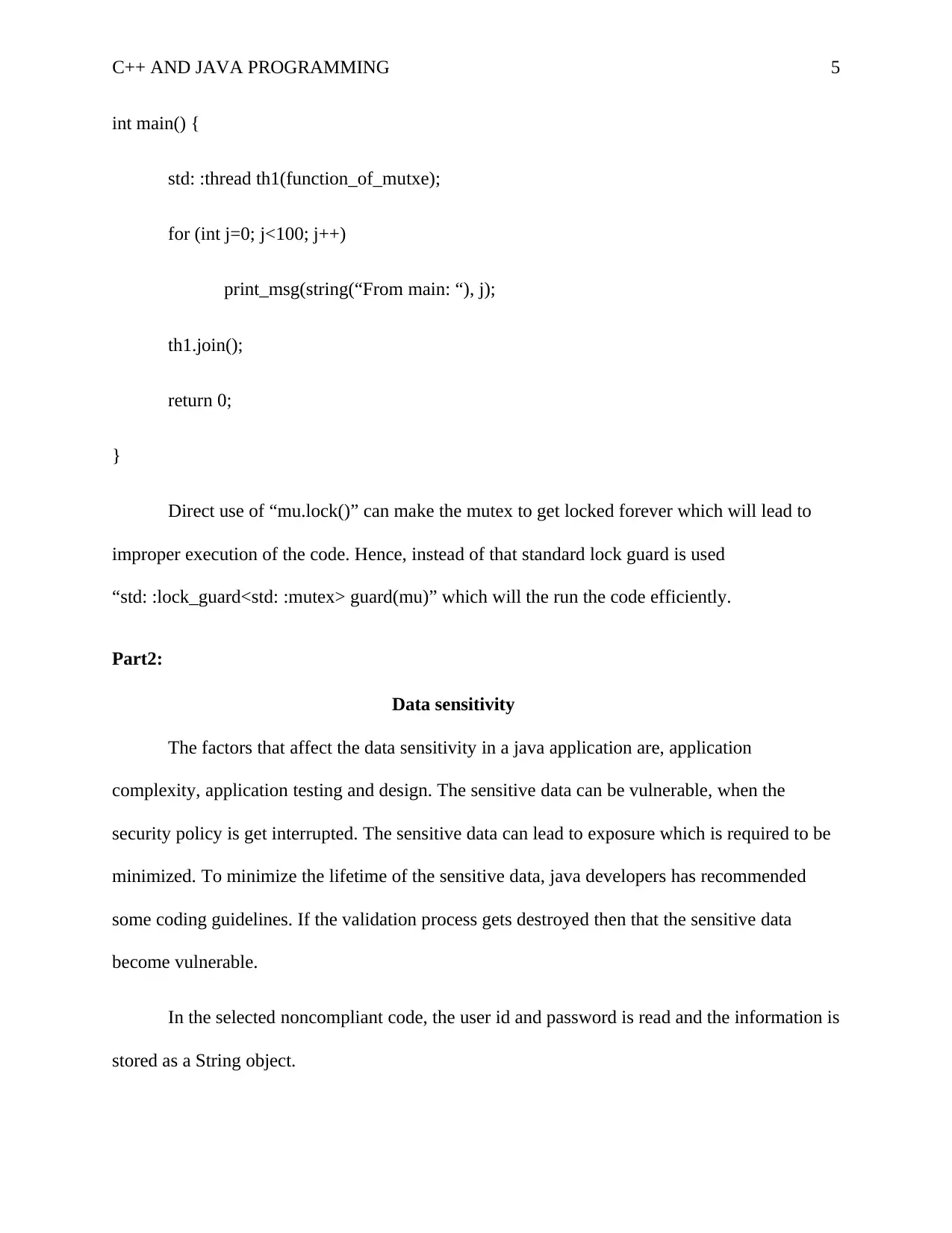
C++ AND JAVA PROGRAMMING 5
int main() {
std: :thread th1(function_of_mutxe);
for (int j=0; j<100; j++)
print_msg(string(“From main: “), j);
th1.join();
return 0;
}
Direct use of “mu.lock()” can make the mutex to get locked forever which will lead to
improper execution of the code. Hence, instead of that standard lock guard is used
“std: :lock_guard<std: :mutex> guard(mu)” which will the run the code efficiently.
Part2:
Data sensitivity
The factors that affect the data sensitivity in a java application are, application
complexity, application testing and design. The sensitive data can be vulnerable, when the
security policy is get interrupted. The sensitive data can lead to exposure which is required to be
minimized. To minimize the lifetime of the sensitive data, java developers has recommended
some coding guidelines. If the validation process gets destroyed then that the sensitive data
become vulnerable.
In the selected noncompliant code, the user id and password is read and the information is
stored as a String object.
int main() {
std: :thread th1(function_of_mutxe);
for (int j=0; j<100; j++)
print_msg(string(“From main: “), j);
th1.join();
return 0;
}
Direct use of “mu.lock()” can make the mutex to get locked forever which will lead to
improper execution of the code. Hence, instead of that standard lock guard is used
“std: :lock_guard<std: :mutex> guard(mu)” which will the run the code efficiently.
Part2:
Data sensitivity
The factors that affect the data sensitivity in a java application are, application
complexity, application testing and design. The sensitive data can be vulnerable, when the
security policy is get interrupted. The sensitive data can lead to exposure which is required to be
minimized. To minimize the lifetime of the sensitive data, java developers has recommended
some coding guidelines. If the validation process gets destroyed then that the sensitive data
become vulnerable.
In the selected noncompliant code, the user id and password is read and the information is
stored as a String object.
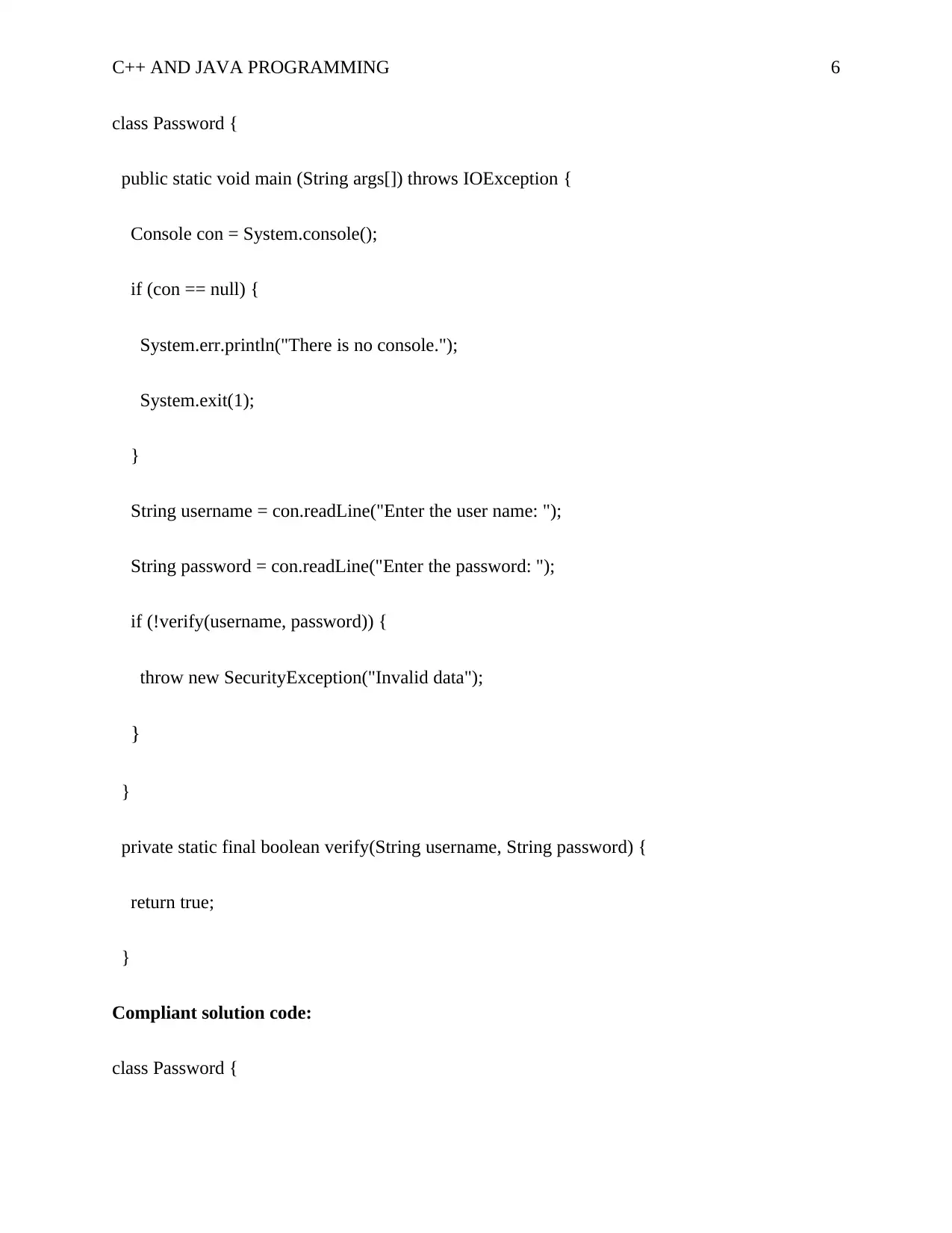
C++ AND JAVA PROGRAMMING 6
class Password {
public static void main (String args[]) throws IOException {
Console con = System.console();
if (con == null) {
System.err.println("There is no console.");
System.exit(1);
}
String username = con.readLine("Enter the user name: ");
String password = con.readLine("Enter the password: ");
if (!verify(username, password)) {
throw new SecurityException("Invalid data");
}
}
private static final boolean verify(String username, String password) {
return true;
}
Compliant solution code:
class Password {
class Password {
public static void main (String args[]) throws IOException {
Console con = System.console();
if (con == null) {
System.err.println("There is no console.");
System.exit(1);
}
String username = con.readLine("Enter the user name: ");
String password = con.readLine("Enter the password: ");
if (!verify(username, password)) {
throw new SecurityException("Invalid data");
}
}
private static final boolean verify(String username, String password) {
return true;
}
Compliant solution code:
class Password {
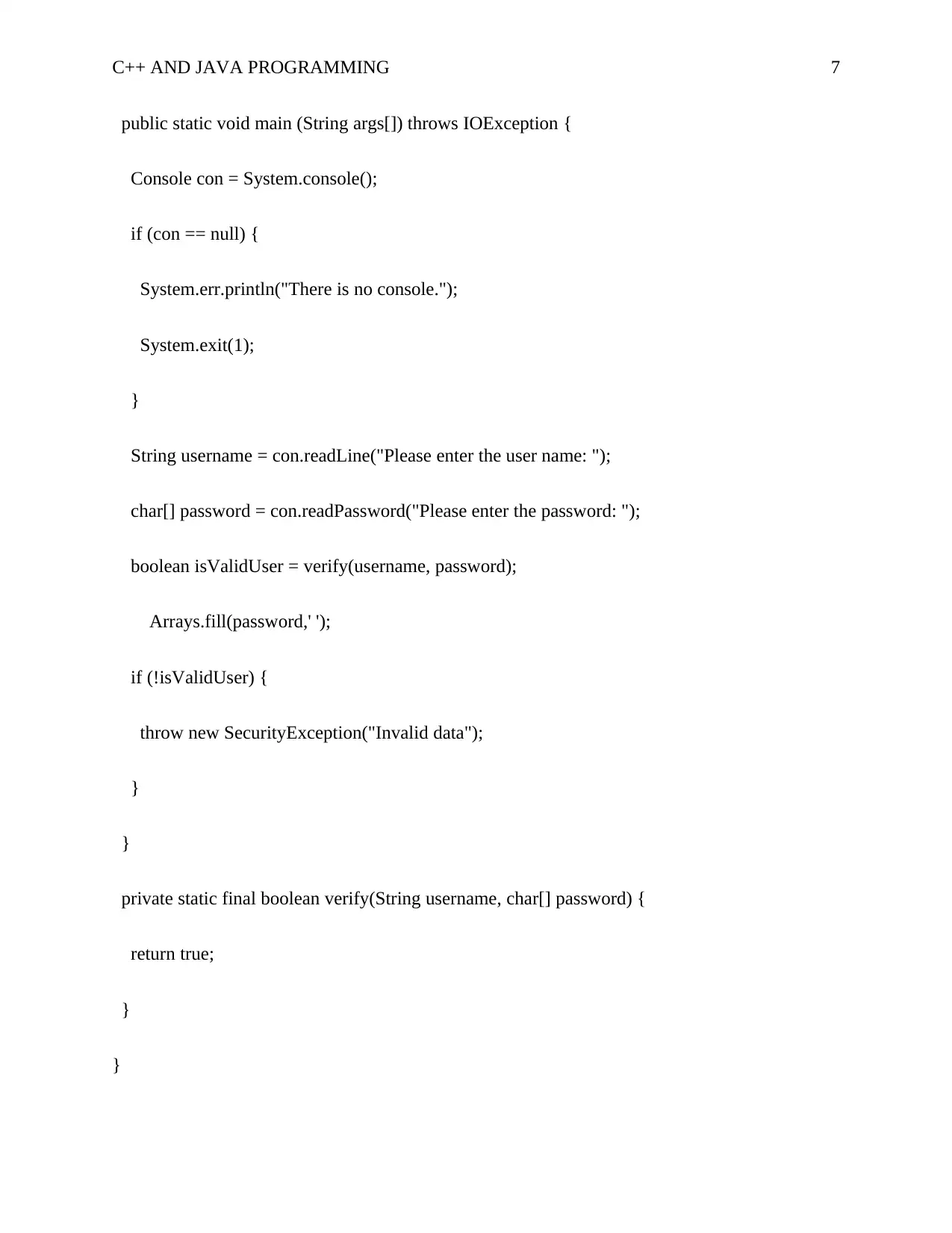
C++ AND JAVA PROGRAMMING 7
public static void main (String args[]) throws IOException {
Console con = System.console();
if (con == null) {
System.err.println("There is no console.");
System.exit(1);
}
String username = con.readLine("Please enter the user name: ");
char[] password = con.readPassword("Please enter the password: ");
boolean isValidUser = verify(username, password);
Arrays.fill(password,' ');
if (!isValidUser) {
throw new SecurityException("Invalid data");
}
}
private static final boolean verify(String username, char[] password) {
return true;
}
}
public static void main (String args[]) throws IOException {
Console con = System.console();
if (con == null) {
System.err.println("There is no console.");
System.exit(1);
}
String username = con.readLine("Please enter the user name: ");
char[] password = con.readPassword("Please enter the password: ");
boolean isValidUser = verify(username, password);
Arrays.fill(password,' ');
if (!isValidUser) {
throw new SecurityException("Invalid data");
}
}
private static final boolean verify(String username, char[] password) {
return true;
}
}
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
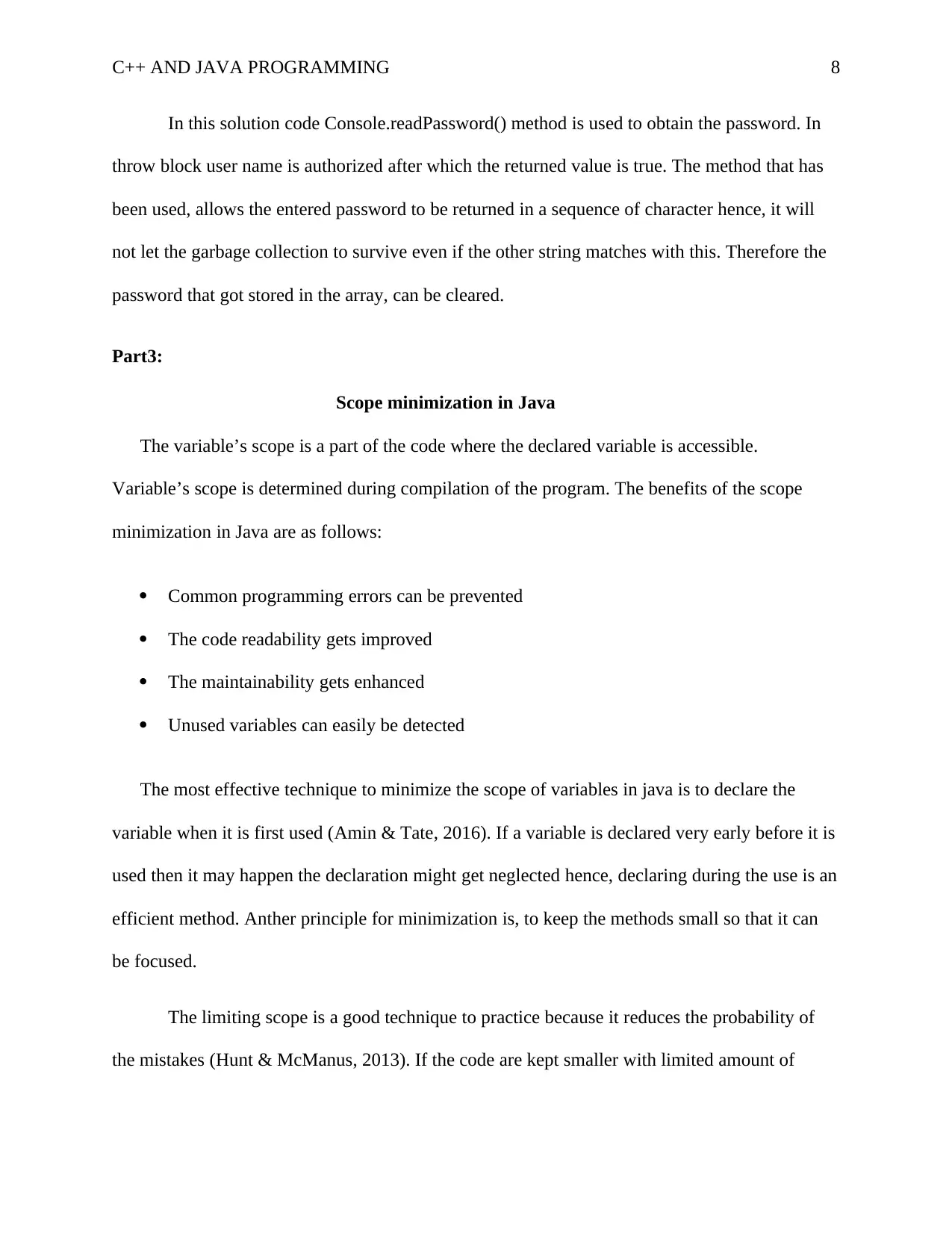
C++ AND JAVA PROGRAMMING 8
In this solution code Console.readPassword() method is used to obtain the password. In
throw block user name is authorized after which the returned value is true. The method that has
been used, allows the entered password to be returned in a sequence of character hence, it will
not let the garbage collection to survive even if the other string matches with this. Therefore the
password that got stored in the array, can be cleared.
Part3:
Scope minimization in Java
The variable’s scope is a part of the code where the declared variable is accessible.
Variable’s scope is determined during compilation of the program. The benefits of the scope
minimization in Java are as follows:
Common programming errors can be prevented
The code readability gets improved
The maintainability gets enhanced
Unused variables can easily be detected
The most effective technique to minimize the scope of variables in java is to declare the
variable when it is first used (Amin & Tate, 2016). If a variable is declared very early before it is
used then it may happen the declaration might get neglected hence, declaring during the use is an
efficient method. Anther principle for minimization is, to keep the methods small so that it can
be focused.
The limiting scope is a good technique to practice because it reduces the probability of
the mistakes (Hunt & McManus, 2013). If the code are kept smaller with limited amount of
In this solution code Console.readPassword() method is used to obtain the password. In
throw block user name is authorized after which the returned value is true. The method that has
been used, allows the entered password to be returned in a sequence of character hence, it will
not let the garbage collection to survive even if the other string matches with this. Therefore the
password that got stored in the array, can be cleared.
Part3:
Scope minimization in Java
The variable’s scope is a part of the code where the declared variable is accessible.
Variable’s scope is determined during compilation of the program. The benefits of the scope
minimization in Java are as follows:
Common programming errors can be prevented
The code readability gets improved
The maintainability gets enhanced
Unused variables can easily be detected
The most effective technique to minimize the scope of variables in java is to declare the
variable when it is first used (Amin & Tate, 2016). If a variable is declared very early before it is
used then it may happen the declaration might get neglected hence, declaring during the use is an
efficient method. Anther principle for minimization is, to keep the methods small so that it can
be focused.
The limiting scope is a good technique to practice because it reduces the probability of
the mistakes (Hunt & McManus, 2013). If the code are kept smaller with limited amount of
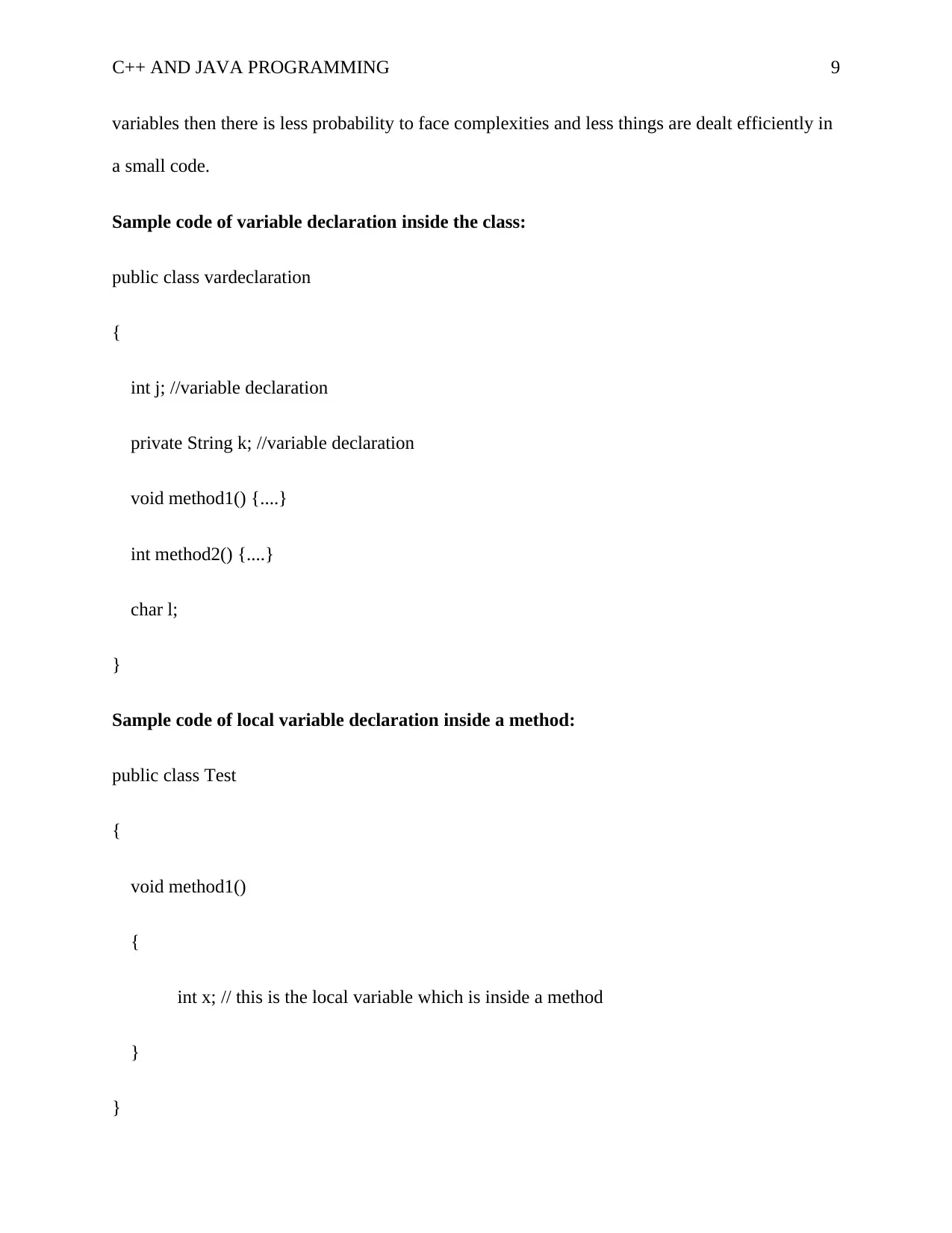
C++ AND JAVA PROGRAMMING 9
variables then there is less probability to face complexities and less things are dealt efficiently in
a small code.
Sample code of variable declaration inside the class:
public class vardeclaration
{
int j; //variable declaration
private String k; //variable declaration
void method1() {....}
int method2() {....}
char l;
}
Sample code of local variable declaration inside a method:
public class Test
{
void method1()
{
int x; // this is the local variable which is inside a method
}
}
variables then there is less probability to face complexities and less things are dealt efficiently in
a small code.
Sample code of variable declaration inside the class:
public class vardeclaration
{
int j; //variable declaration
private String k; //variable declaration
void method1() {....}
int method2() {....}
char l;
}
Sample code of local variable declaration inside a method:
public class Test
{
void method1()
{
int x; // this is the local variable which is inside a method
}
}
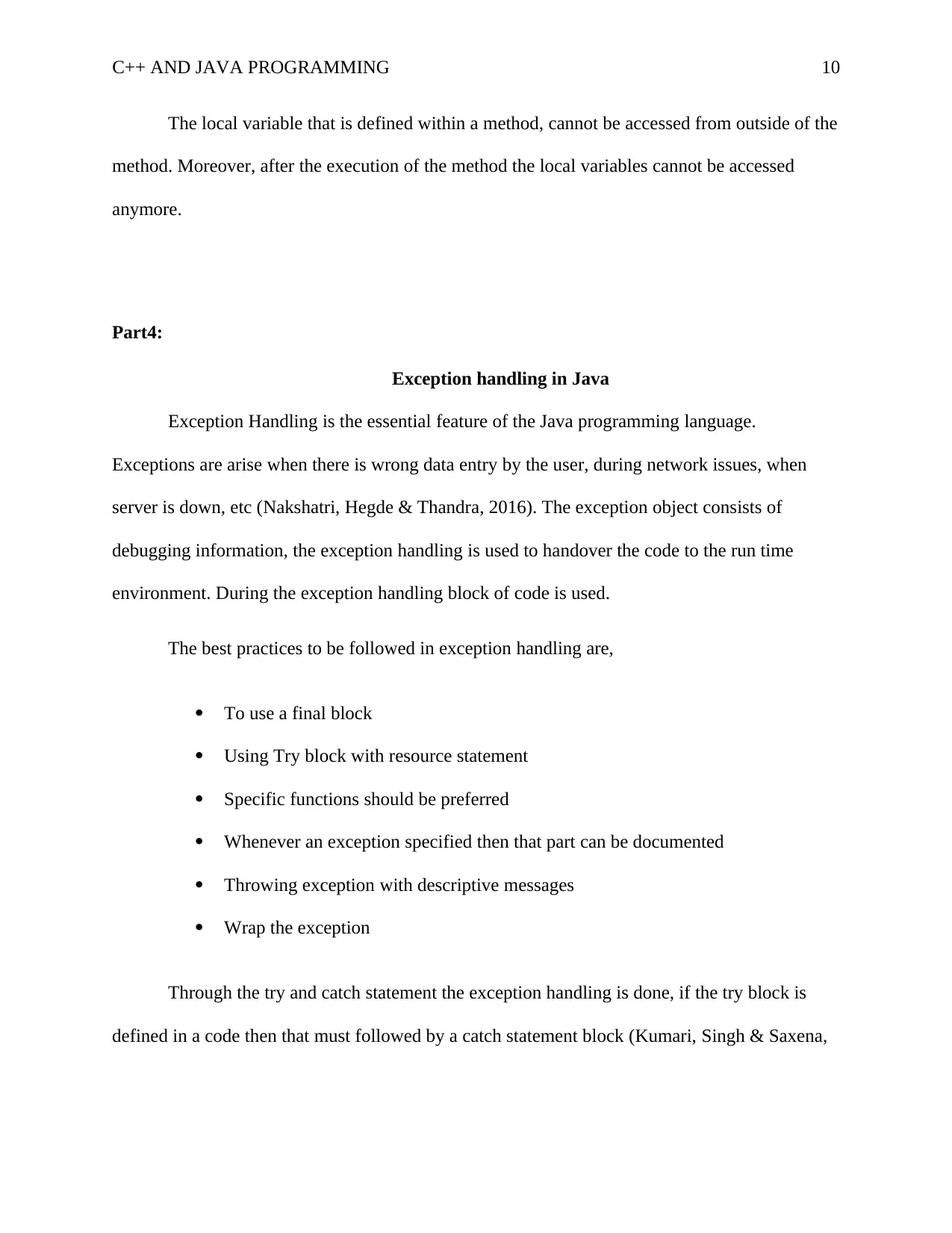
C++ AND JAVA PROGRAMMING 10
The local variable that is defined within a method, cannot be accessed from outside of the
method. Moreover, after the execution of the method the local variables cannot be accessed
anymore.
Part4:
Exception handling in Java
Exception Handling is the essential feature of the Java programming language.
Exceptions are arise when there is wrong data entry by the user, during network issues, when
server is down, etc (Nakshatri, Hegde & Thandra, 2016). The exception object consists of
debugging information, the exception handling is used to handover the code to the run time
environment. During the exception handling block of code is used.
The best practices to be followed in exception handling are,
To use a final block
Using Try block with resource statement
Specific functions should be preferred
Whenever an exception specified then that part can be documented
Throwing exception with descriptive messages
Wrap the exception
Through the try and catch statement the exception handling is done, if the try block is
defined in a code then that must followed by a catch statement block (Kumari, Singh & Saxena,
The local variable that is defined within a method, cannot be accessed from outside of the
method. Moreover, after the execution of the method the local variables cannot be accessed
anymore.
Part4:
Exception handling in Java
Exception Handling is the essential feature of the Java programming language.
Exceptions are arise when there is wrong data entry by the user, during network issues, when
server is down, etc (Nakshatri, Hegde & Thandra, 2016). The exception object consists of
debugging information, the exception handling is used to handover the code to the run time
environment. During the exception handling block of code is used.
The best practices to be followed in exception handling are,
To use a final block
Using Try block with resource statement
Specific functions should be preferred
Whenever an exception specified then that part can be documented
Throwing exception with descriptive messages
Wrap the exception
Through the try and catch statement the exception handling is done, if the try block is
defined in a code then that must followed by a catch statement block (Kumari, Singh & Saxena,
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
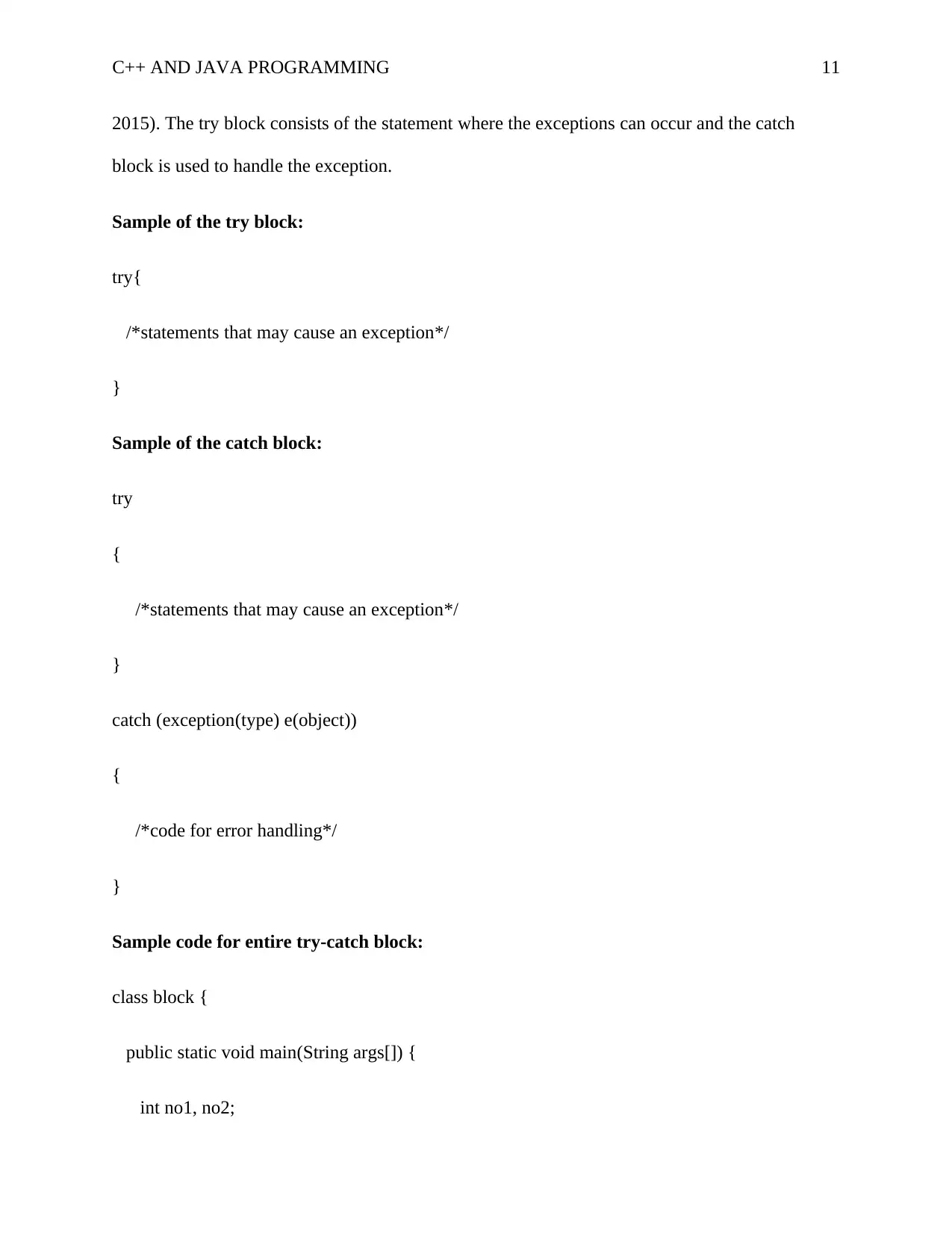
C++ AND JAVA PROGRAMMING 11
2015). The try block consists of the statement where the exceptions can occur and the catch
block is used to handle the exception.
Sample of the try block:
try{
/*statements that may cause an exception*/
}
Sample of the catch block:
try
{
/*statements that may cause an exception*/
}
catch (exception(type) e(object))
{
/*code for error handling*/
}
Sample code for entire try-catch block:
class block {
public static void main(String args[]) {
int no1, no2;
2015). The try block consists of the statement where the exceptions can occur and the catch
block is used to handle the exception.
Sample of the try block:
try{
/*statements that may cause an exception*/
}
Sample of the catch block:
try
{
/*statements that may cause an exception*/
}
catch (exception(type) e(object))
{
/*code for error handling*/
}
Sample code for entire try-catch block:
class block {
public static void main(String args[]) {
int no1, no2;
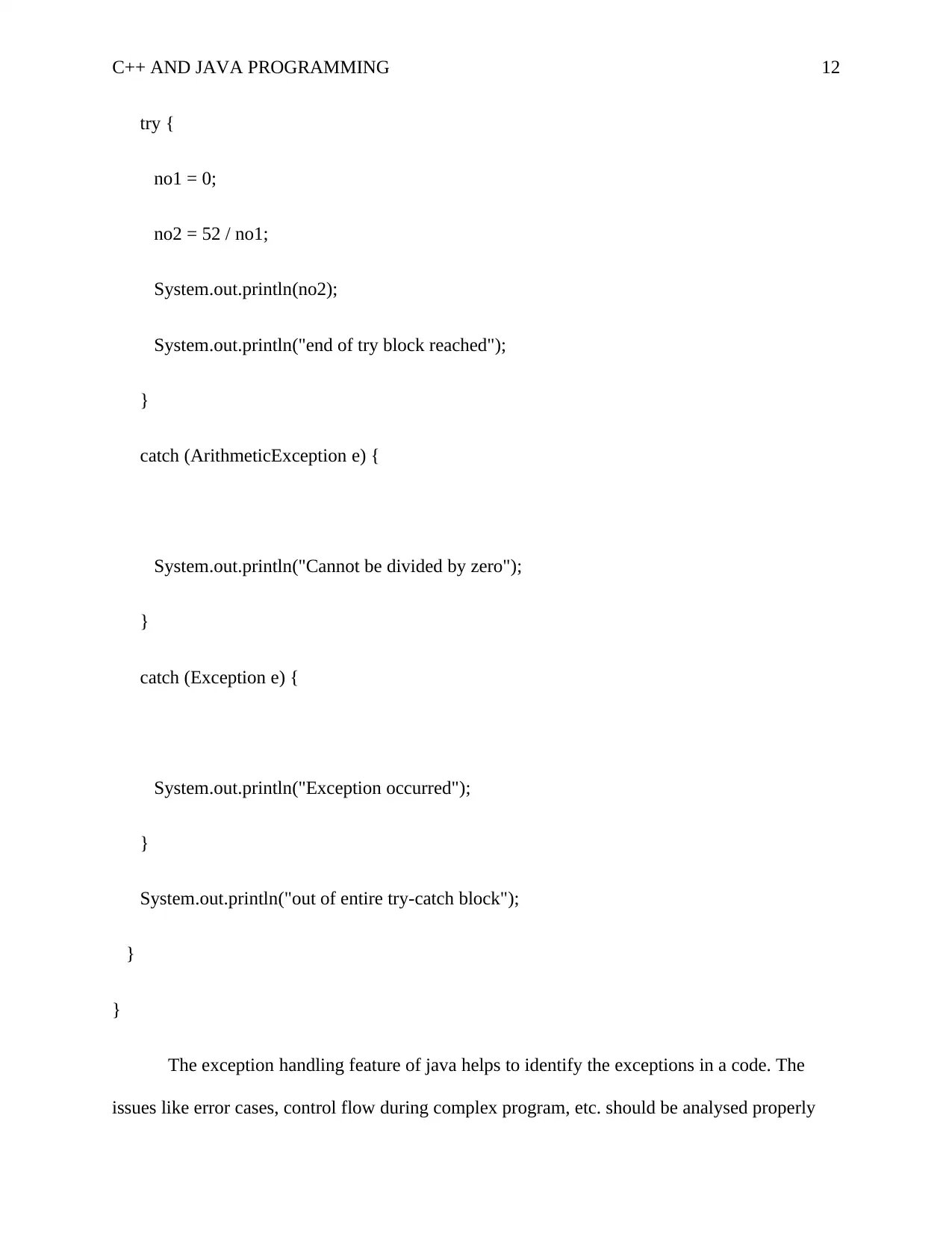
C++ AND JAVA PROGRAMMING 12
try {
no1 = 0;
no2 = 52 / no1;
System.out.println(no2);
System.out.println("end of try block reached");
}
catch (ArithmeticException e) {
System.out.println("Cannot be divided by zero");
}
catch (Exception e) {
System.out.println("Exception occurred");
}
System.out.println("out of entire try-catch block");
}
}
The exception handling feature of java helps to identify the exceptions in a code. The
issues like error cases, control flow during complex program, etc. should be analysed properly
try {
no1 = 0;
no2 = 52 / no1;
System.out.println(no2);
System.out.println("end of try block reached");
}
catch (ArithmeticException e) {
System.out.println("Cannot be divided by zero");
}
catch (Exception e) {
System.out.println("Exception occurred");
}
System.out.println("out of entire try-catch block");
}
}
The exception handling feature of java helps to identify the exceptions in a code. The
issues like error cases, control flow during complex program, etc. should be analysed properly
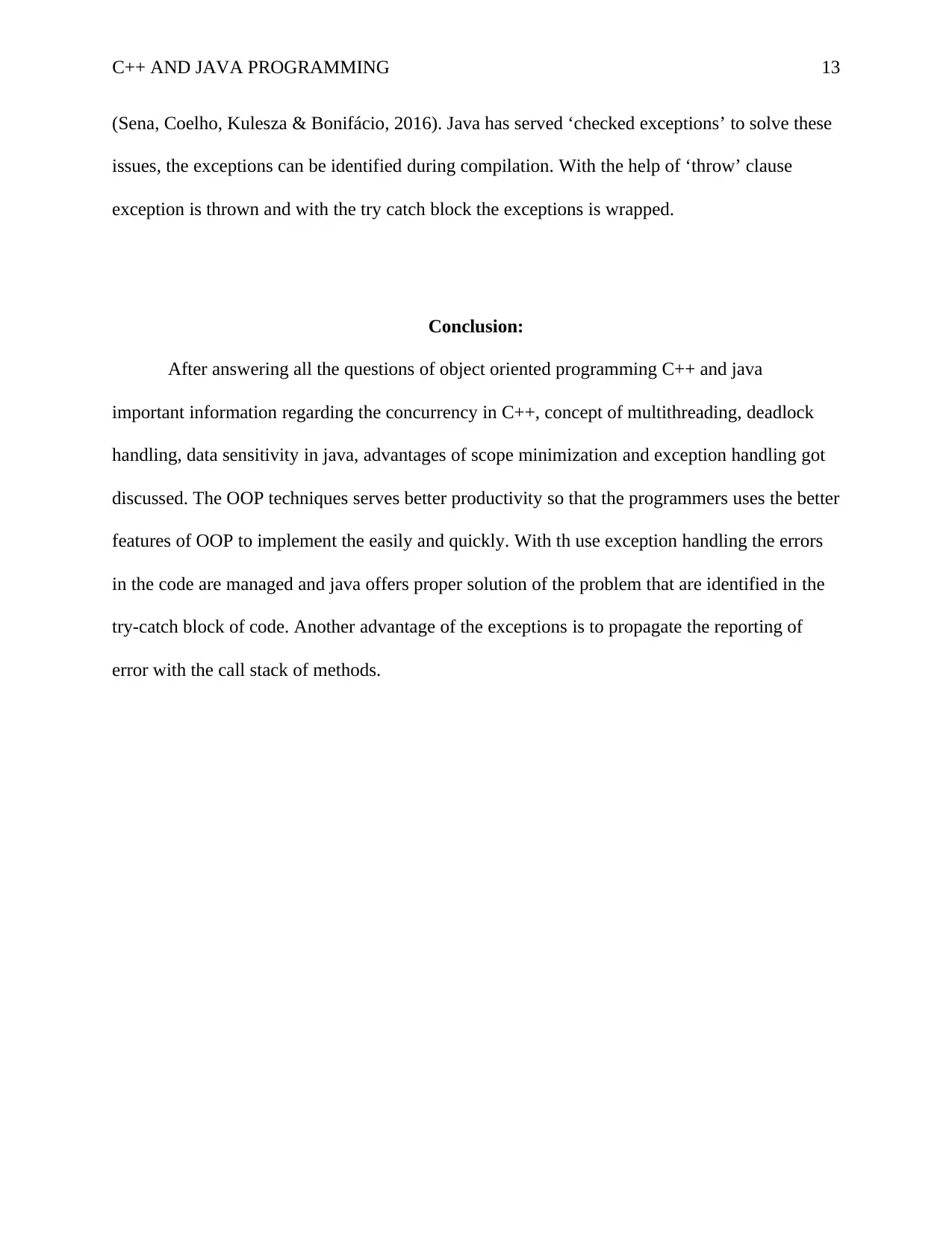
C++ AND JAVA PROGRAMMING 13
(Sena, Coelho, Kulesza & Bonifácio, 2016). Java has served ‘checked exceptions’ to solve these
issues, the exceptions can be identified during compilation. With the help of ‘throw’ clause
exception is thrown and with the try catch block the exceptions is wrapped.
Conclusion:
After answering all the questions of object oriented programming C++ and java
important information regarding the concurrency in C++, concept of multithreading, deadlock
handling, data sensitivity in java, advantages of scope minimization and exception handling got
discussed. The OOP techniques serves better productivity so that the programmers uses the better
features of OOP to implement the easily and quickly. With th use exception handling the errors
in the code are managed and java offers proper solution of the problem that are identified in the
try-catch block of code. Another advantage of the exceptions is to propagate the reporting of
error with the call stack of methods.
(Sena, Coelho, Kulesza & Bonifácio, 2016). Java has served ‘checked exceptions’ to solve these
issues, the exceptions can be identified during compilation. With the help of ‘throw’ clause
exception is thrown and with the try catch block the exceptions is wrapped.
Conclusion:
After answering all the questions of object oriented programming C++ and java
important information regarding the concurrency in C++, concept of multithreading, deadlock
handling, data sensitivity in java, advantages of scope minimization and exception handling got
discussed. The OOP techniques serves better productivity so that the programmers uses the better
features of OOP to implement the easily and quickly. With th use exception handling the errors
in the code are managed and java offers proper solution of the problem that are identified in the
try-catch block of code. Another advantage of the exceptions is to propagate the reporting of
error with the call stack of methods.
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
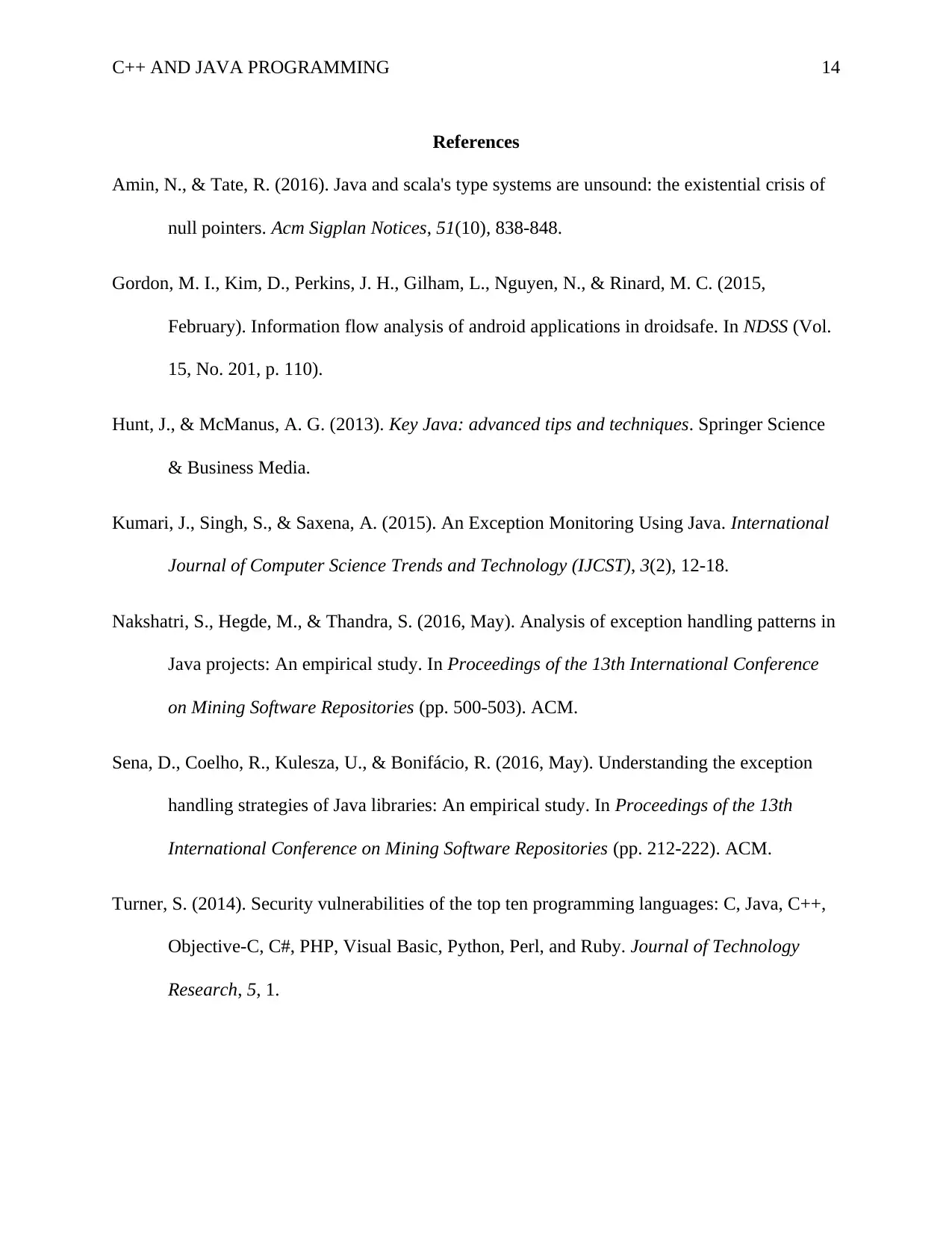
C++ AND JAVA PROGRAMMING 14
References
Amin, N., & Tate, R. (2016). Java and scala's type systems are unsound: the existential crisis of
null pointers. Acm Sigplan Notices, 51(10), 838-848.
Gordon, M. I., Kim, D., Perkins, J. H., Gilham, L., Nguyen, N., & Rinard, M. C. (2015,
February). Information flow analysis of android applications in droidsafe. In NDSS (Vol.
15, No. 201, p. 110).
Hunt, J., & McManus, A. G. (2013). Key Java: advanced tips and techniques. Springer Science
& Business Media.
Kumari, J., Singh, S., & Saxena, A. (2015). An Exception Monitoring Using Java. International
Journal of Computer Science Trends and Technology (IJCST), 3(2), 12-18.
Nakshatri, S., Hegde, M., & Thandra, S. (2016, May). Analysis of exception handling patterns in
Java projects: An empirical study. In Proceedings of the 13th International Conference
on Mining Software Repositories (pp. 500-503). ACM.
Sena, D., Coelho, R., Kulesza, U., & Bonifácio, R. (2016, May). Understanding the exception
handling strategies of Java libraries: An empirical study. In Proceedings of the 13th
International Conference on Mining Software Repositories (pp. 212-222). ACM.
Turner, S. (2014). Security vulnerabilities of the top ten programming languages: C, Java, C++,
Objective-C, C#, PHP, Visual Basic, Python, Perl, and Ruby. Journal of Technology
Research, 5, 1.
References
Amin, N., & Tate, R. (2016). Java and scala's type systems are unsound: the existential crisis of
null pointers. Acm Sigplan Notices, 51(10), 838-848.
Gordon, M. I., Kim, D., Perkins, J. H., Gilham, L., Nguyen, N., & Rinard, M. C. (2015,
February). Information flow analysis of android applications in droidsafe. In NDSS (Vol.
15, No. 201, p. 110).
Hunt, J., & McManus, A. G. (2013). Key Java: advanced tips and techniques. Springer Science
& Business Media.
Kumari, J., Singh, S., & Saxena, A. (2015). An Exception Monitoring Using Java. International
Journal of Computer Science Trends and Technology (IJCST), 3(2), 12-18.
Nakshatri, S., Hegde, M., & Thandra, S. (2016, May). Analysis of exception handling patterns in
Java projects: An empirical study. In Proceedings of the 13th International Conference
on Mining Software Repositories (pp. 500-503). ACM.
Sena, D., Coelho, R., Kulesza, U., & Bonifácio, R. (2016, May). Understanding the exception
handling strategies of Java libraries: An empirical study. In Proceedings of the 13th
International Conference on Mining Software Repositories (pp. 212-222). ACM.
Turner, S. (2014). Security vulnerabilities of the top ten programming languages: C, Java, C++,
Objective-C, C#, PHP, Visual Basic, Python, Perl, and Ruby. Journal of Technology
Research, 5, 1.
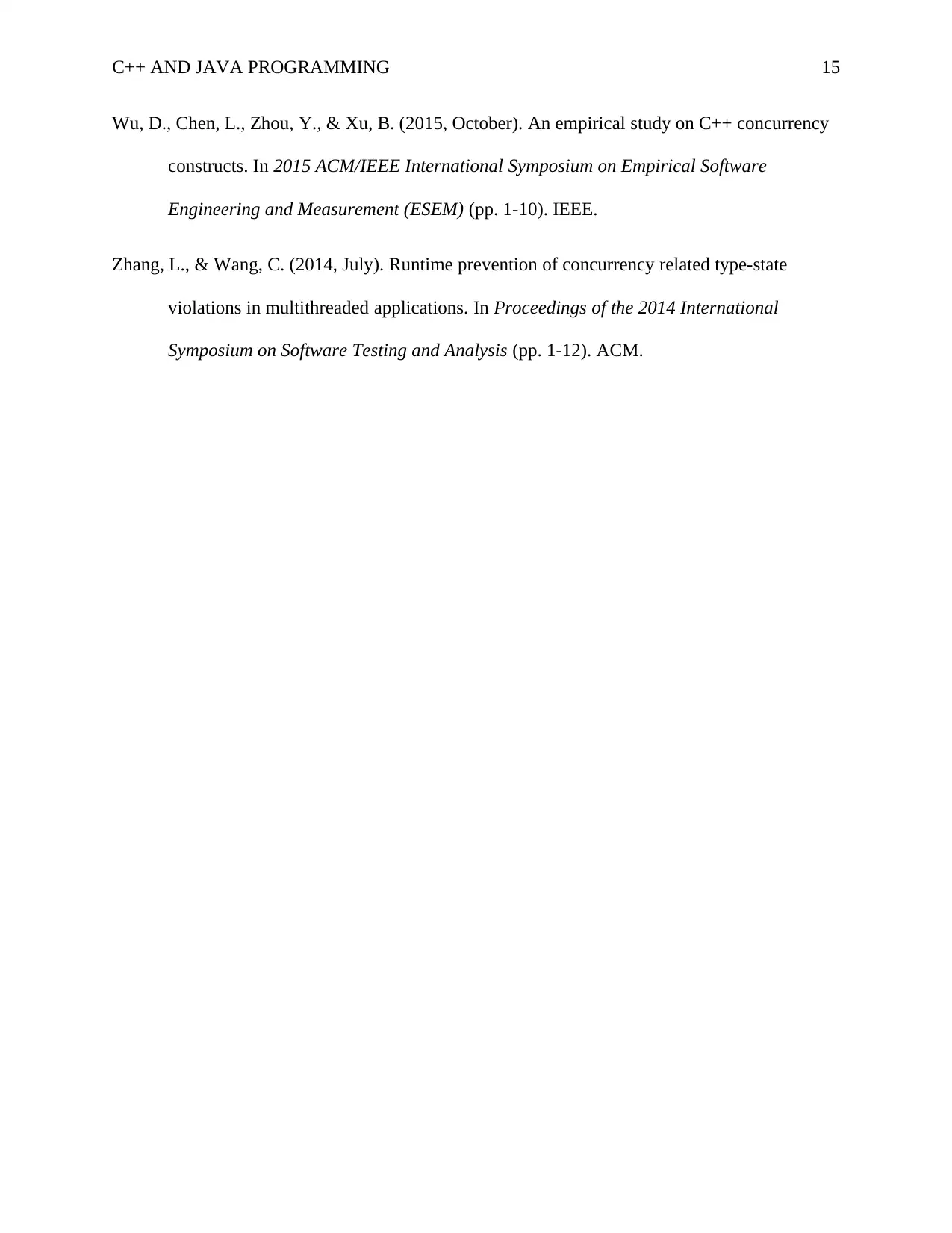
C++ AND JAVA PROGRAMMING 15
Wu, D., Chen, L., Zhou, Y., & Xu, B. (2015, October). An empirical study on C++ concurrency
constructs. In 2015 ACM/IEEE International Symposium on Empirical Software
Engineering and Measurement (ESEM) (pp. 1-10). IEEE.
Zhang, L., & Wang, C. (2014, July). Runtime prevention of concurrency related type-state
violations in multithreaded applications. In Proceedings of the 2014 International
Symposium on Software Testing and Analysis (pp. 1-12). ACM.
Wu, D., Chen, L., Zhou, Y., & Xu, B. (2015, October). An empirical study on C++ concurrency
constructs. In 2015 ACM/IEEE International Symposium on Empirical Software
Engineering and Measurement (ESEM) (pp. 1-10). IEEE.
Zhang, L., & Wang, C. (2014, July). Runtime prevention of concurrency related type-state
violations in multithreaded applications. In Proceedings of the 2014 International
Symposium on Software Testing and Analysis (pp. 1-12). ACM.
1 out of 15
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.