CLDA Loan Management Application: View, Request, Sort and Accept Loans
Build a peer to peer lending application that loads loan requests and loan offers from files.
14 Pages3975 Words327 Views
Added on 2023-05-28
About This Document
This article provides step-by-step instructions and code snippets for viewing loan requests, requesting a loan, viewing loan offers, sorting loan offers, accepting loan offers, viewing account wallet, and saving and exiting the CLDA Loan Management Application.
CLDA Loan Management Application: View, Request, Sort and Accept Loans
Build a peer to peer lending application that loads loan requests and loan offers from files.
Added on 2023-05-28
ShareRelated Documents
Following are MENU items in application:
1. View Loan Requests
2. Request a Loan
3. View Loan Offers
4. Sort Loan Offers
5. Accept Loan Offer
6. View Account Wallet
7. Save and Exit CLDA
View Loan Request:
This option in application will help user to a detail of loans requested in proper format.
Steps to execute this operation are:
1. Open file in read mode where loan offer details are stored.
2. Check file exist or not. If not exist display proper message and return to main menu.
3. If file exist then read loan details from file using loop and store it in to a structure.
4. Display the details on screen using structure.
Request Loan:
This operation will create new request for user about loan.
Steps to execute this operation are:
1. Ask loan details from user.
2. Store it into a structure.
3. Open loan request file in append mode and add new detail in file.
View Loan Offer:
This operation will display all loan offer details to user along with offer time interest rate.
Steps to execute this operation are:
1. Open file in read mode where loan offer details are stored.
2. Check file exist or not. If not exist display proper message and return to main menu.
3. If file exist then read loan details from file using loop and store it in to a structure.
4. Display the details on screen using structure.
Sort Loan Offers.
This operation will help to sort all offered loans based on interest rate, so that it can help
user to accept the offered loan.
1. Read structure data.
1. View Loan Requests
2. Request a Loan
3. View Loan Offers
4. Sort Loan Offers
5. Accept Loan Offer
6. View Account Wallet
7. Save and Exit CLDA
View Loan Request:
This option in application will help user to a detail of loans requested in proper format.
Steps to execute this operation are:
1. Open file in read mode where loan offer details are stored.
2. Check file exist or not. If not exist display proper message and return to main menu.
3. If file exist then read loan details from file using loop and store it in to a structure.
4. Display the details on screen using structure.
Request Loan:
This operation will create new request for user about loan.
Steps to execute this operation are:
1. Ask loan details from user.
2. Store it into a structure.
3. Open loan request file in append mode and add new detail in file.
View Loan Offer:
This operation will display all loan offer details to user along with offer time interest rate.
Steps to execute this operation are:
1. Open file in read mode where loan offer details are stored.
2. Check file exist or not. If not exist display proper message and return to main menu.
3. If file exist then read loan details from file using loop and store it in to a structure.
4. Display the details on screen using structure.
Sort Loan Offers.
This operation will help to sort all offered loans based on interest rate, so that it can help
user to accept the offered loan.
1. Read structure data.
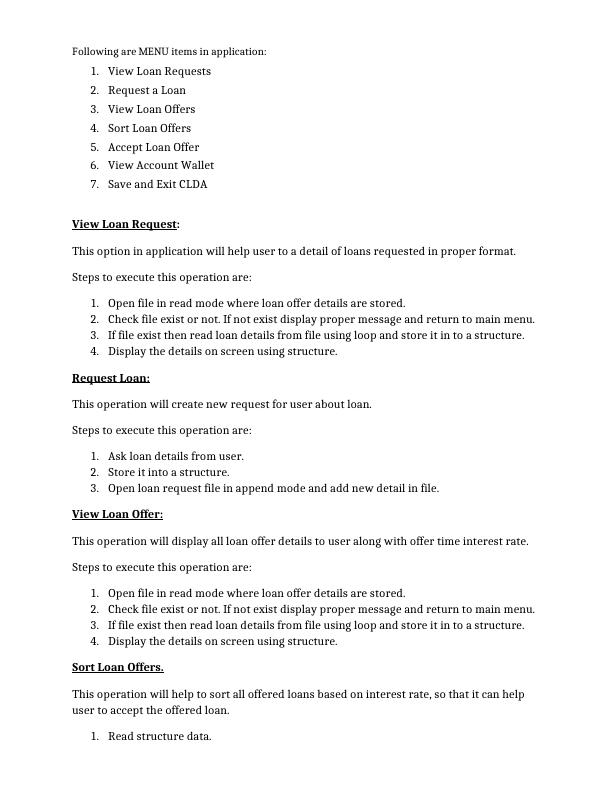
2. Apply bubble sort algorithm to sort an array of structure.
Following is the code for the same:
void sort_loan_offers()
{
int i=0, j=0;
struct LoanOfferData lod;
for(i = 0 ; i < (TotalOffer-1) ; i++)
{
for(j = (i+1) ; j < TotalOffer ; j++)
{
if(LoanData[i].OfferRate < LoanData[j].OfferRate)
{
lod = LoanData[i];
LoanData[i] = LoanData[j];
LoanData[j] = lod;
}
}
}
printf("\n\nTotal Loan Offers = %d",TotalOffer);
PrintLoanOfferData();
}
Accept loan offer:
This operation will allow user to accept a loan offered by bank.
Steps to execute this operation are:
1. Display offered loan details along with serial number.
2. Ask user to enter serial number of loan which he/she want to accept.
3. Change status of that loan from Not Accepted to Accepted.
View Account wallet.
This operation will allow user to view his/her account information.
Save and exit.
This operation will save all data from structure to the text file for permanent storage.
Complete code of this application
#include <stdio.h>
#include <conio.h>
#include <time.h>
#include <string.h>
#include <ctype.h>
#include <stdlib.h>
#include <dirent.h>
//global variable declaration:
Following is the code for the same:
void sort_loan_offers()
{
int i=0, j=0;
struct LoanOfferData lod;
for(i = 0 ; i < (TotalOffer-1) ; i++)
{
for(j = (i+1) ; j < TotalOffer ; j++)
{
if(LoanData[i].OfferRate < LoanData[j].OfferRate)
{
lod = LoanData[i];
LoanData[i] = LoanData[j];
LoanData[j] = lod;
}
}
}
printf("\n\nTotal Loan Offers = %d",TotalOffer);
PrintLoanOfferData();
}
Accept loan offer:
This operation will allow user to accept a loan offered by bank.
Steps to execute this operation are:
1. Display offered loan details along with serial number.
2. Ask user to enter serial number of loan which he/she want to accept.
3. Change status of that loan from Not Accepted to Accepted.
View Account wallet.
This operation will allow user to view his/her account information.
Save and exit.
This operation will save all data from structure to the text file for permanent storage.
Complete code of this application
#include <stdio.h>
#include <conio.h>
#include <time.h>
#include <string.h>
#include <ctype.h>
#include <stdlib.h>
#include <dirent.h>
//global variable declaration:
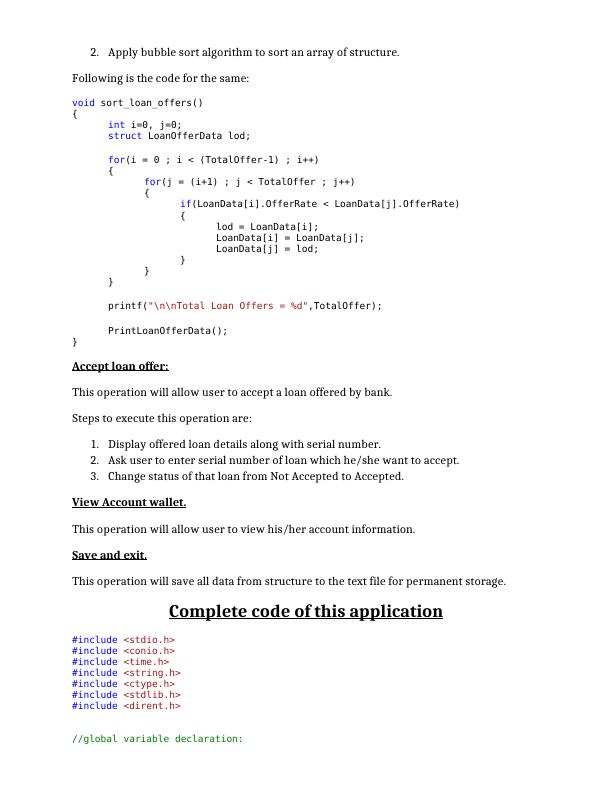
int TotalOffer = 0;
int TotalRequest = 0;
/**
* Function Name :
* Description :
* @param (Data Ttpe : | Data Value : )
* @return (Data Ttpe : | Data Value : )
*/
/**
* Structure Name : "LoanRequestData"
* Description : This will contain data members which can hold the data of
requested loans.
*/
struct LoanRequestData
{
time_t RequestTime;
float AmountRequested;
float AmountPledged;
int LoanPeriod;
float InterestRate;
int Status;
}*LoanReqData;
/**
* Structure Name : "LoanOfferData"
* Description : This will contain data members which can hold the data of Offered
loans.
*/
struct LoanOfferData
{
char FileName[100];
time_t RequestTimeStamp;
time_t OfferTimeStamp;
char OfferAddress[60];
float OfferRate;
int AcceptedFlag;
}*LoanData;
/**
* Function Name : "DeleteOldLoan"
* Description : Function will delete loan offered before 72 hours
* @param (Data Ttpe : VOID | Data Value : Nothing)
* @return (Data Ttpe : int | Data Value : user's choice)
*/
void DeleteOldLoan()
{
long current_time;
int i;
time_t epoch = time(NULL);
current_time = (long)epoch;
for(i=0;i<TotalOffer;i++)
{
if(current_time - (long)(LoanData[i].OfferTimeStamp) > (72*60*60))
{
printf("\n\n%s File Removed...!!!",LoanData[i].FileName);
remove(LoanData[i].FileName);
}
}
getch();
}
int TotalRequest = 0;
/**
* Function Name :
* Description :
* @param (Data Ttpe : | Data Value : )
* @return (Data Ttpe : | Data Value : )
*/
/**
* Structure Name : "LoanRequestData"
* Description : This will contain data members which can hold the data of
requested loans.
*/
struct LoanRequestData
{
time_t RequestTime;
float AmountRequested;
float AmountPledged;
int LoanPeriod;
float InterestRate;
int Status;
}*LoanReqData;
/**
* Structure Name : "LoanOfferData"
* Description : This will contain data members which can hold the data of Offered
loans.
*/
struct LoanOfferData
{
char FileName[100];
time_t RequestTimeStamp;
time_t OfferTimeStamp;
char OfferAddress[60];
float OfferRate;
int AcceptedFlag;
}*LoanData;
/**
* Function Name : "DeleteOldLoan"
* Description : Function will delete loan offered before 72 hours
* @param (Data Ttpe : VOID | Data Value : Nothing)
* @return (Data Ttpe : int | Data Value : user's choice)
*/
void DeleteOldLoan()
{
long current_time;
int i;
time_t epoch = time(NULL);
current_time = (long)epoch;
for(i=0;i<TotalOffer;i++)
{
if(current_time - (long)(LoanData[i].OfferTimeStamp) > (72*60*60))
{
printf("\n\n%s File Removed...!!!",LoanData[i].FileName);
remove(LoanData[i].FileName);
}
}
getch();
}
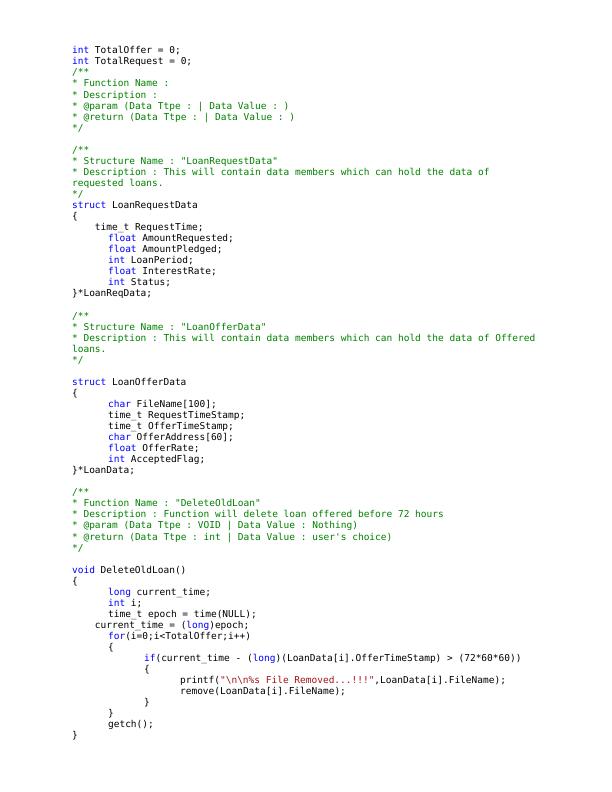
/**
* Function Name : "display_menu"
* Description : Function will display main menu optrions require to perform
transactions.
* @param (Data Ttpe : VOID | Data Value : Nothing)
* @return (Data Ttpe : int | Data Value : user's choice)
*/
int display_menu()
{
int choice = 0;
system("CLS");
printf("\n 1. View Loan Requests.");
printf("\n 2. Request a Loan.");
printf("\n 3. View Loan Offers.");
printf("\n 4. Sort Loan Offers.");
printf("\n 5. Accept Loan Offer.");
printf("\n 6. View Account Wallet.");
printf("\n 7. Save and Exit CLDA.");
printf("\n\nEnter your choice : ");
scanf("%d",&choice);
return choice;
}
/**
* Function Name : PrintLoanRequestData
* Description : Print details of requested loans
* @param (Data Ttpe : LoanRequestData| Data Value : Object of structure
LoanRequestData)
* @return (Data Ttpe : VOID| Data Value : )
*/
void PrintLoanRequestData (struct LoanRequestData lrd)
{
printf("%16.2f%16.2f%13d%15.2f%8d %s",lrd.AmountRequested,
lrd.AmountPledged, lrd.LoanPeriod, lrd.InterestRate, lrd.Status,
asctime(gmtime(&(lrd.RequestTime))));
}
/**
* Function Name : "load_request_data"
* Description : Function will read text file in which data for requested loan is
stored, and load those
* data into an array of structure object
* @param (Data Ttpe : VOID | Data Value : Nothing)
* @return (Data Ttpe : VOID | Data Value : Nothing)
*/
void load_request_data()
{
time_t epoch;
int i = 0;
char string[200];
int count = 0; //count and store total number of records in loanrequest
file.
struct LoanRequestData *LRD;
FILE *infile;
//reading and counting total number of records from file.
infile = fopen("D:\\886866\\LoanRequest.txt","r");
if(infile == NULL)
printf("\nFile Can't Open");
* Function Name : "display_menu"
* Description : Function will display main menu optrions require to perform
transactions.
* @param (Data Ttpe : VOID | Data Value : Nothing)
* @return (Data Ttpe : int | Data Value : user's choice)
*/
int display_menu()
{
int choice = 0;
system("CLS");
printf("\n 1. View Loan Requests.");
printf("\n 2. Request a Loan.");
printf("\n 3. View Loan Offers.");
printf("\n 4. Sort Loan Offers.");
printf("\n 5. Accept Loan Offer.");
printf("\n 6. View Account Wallet.");
printf("\n 7. Save and Exit CLDA.");
printf("\n\nEnter your choice : ");
scanf("%d",&choice);
return choice;
}
/**
* Function Name : PrintLoanRequestData
* Description : Print details of requested loans
* @param (Data Ttpe : LoanRequestData| Data Value : Object of structure
LoanRequestData)
* @return (Data Ttpe : VOID| Data Value : )
*/
void PrintLoanRequestData (struct LoanRequestData lrd)
{
printf("%16.2f%16.2f%13d%15.2f%8d %s",lrd.AmountRequested,
lrd.AmountPledged, lrd.LoanPeriod, lrd.InterestRate, lrd.Status,
asctime(gmtime(&(lrd.RequestTime))));
}
/**
* Function Name : "load_request_data"
* Description : Function will read text file in which data for requested loan is
stored, and load those
* data into an array of structure object
* @param (Data Ttpe : VOID | Data Value : Nothing)
* @return (Data Ttpe : VOID | Data Value : Nothing)
*/
void load_request_data()
{
time_t epoch;
int i = 0;
char string[200];
int count = 0; //count and store total number of records in loanrequest
file.
struct LoanRequestData *LRD;
FILE *infile;
//reading and counting total number of records from file.
infile = fopen("D:\\886866\\LoanRequest.txt","r");
if(infile == NULL)
printf("\nFile Can't Open");
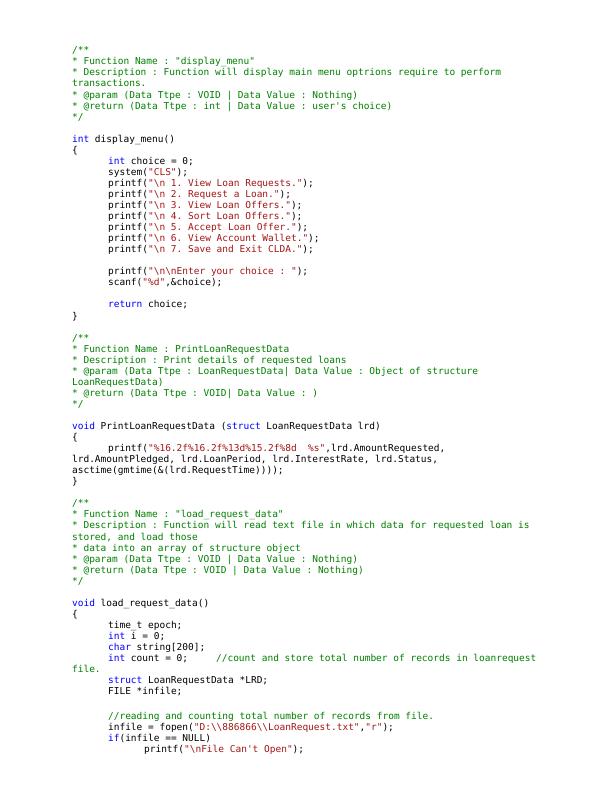
End of preview
Want to access all the pages? Upload your documents or become a member.