COMP 1900 Introduction to Computer Science Assignment
Added on 2019-09-16
5 Pages3958 Words329 Views
COMP 1900 – Fall 2016 – Project 2Number of People: Individual.Due: Please try to finish by Sunday, Nov. 20th, 2016. The due date is Sunday, Nov. 27th, 2016.Submission: Zip your BlueJ project folder into a single file and upload it to the proper folder in the eCoursewaredropbox at https://elearn.memphis.edu.Coding Style: Use descriptive variable names. Use consistent indentation. Use standard Java naming conventions for variableAndMethodNames, ClassNames, CONSTANT_NAMES. Include a reasonable amount of comments.In a standard deck of 52 playing cards, each card is one of the following kinds: Ace (A), 2, 3, 4, 5, 6, 7, 8, 9, 10, Jack (J), Queen (Q), andKing (K). In the card game Poker, you can think of the Jack, Queen, and King as being like 11, 12, and 13, respectively. An Ace is special because it can be treated either as a 1 (called low) or as a 14 (called high), but not both.Each card is also one of the following suits: Spades (S), Hearts (H), Clubs (C), or Diamonds (D). A deck contains exactly one card for each combination of kind and suit. For example, there are four Aces which can be written AS, AH, AC, and AD representing the Ace of Spades, Ace of Hearts, Ace of Clubs, and Ace of Diamonds respectively. There are also four twos (which can be written 2S, 2H, 2C, 2D), etc.The card game Poker uses a standard deck of 52 playing cards. A player is dealt a hand consisting of five cards at random. The player’s hand is then scored into one of a number of categories. Please read the description of Poker hand categories at:https://en.wikipedia.org/wiki/List_of_poker_hand_categoriesNote that the category “Five of a kind” does not apply to a standard deck of 52 playing cards. Also, note that a hand in the category “High Card” can be more precisely described using one of the following sub-categories determined by the highest ranking card in thehand. In a “High Card” hand, any Ace is always treated as high, i.e., as a 14, and thus has the highest rank. 1. Seven high2. Eight high3. Nine high4. Ten high5. Jack high6. Queen high7. King high8. Ace highIf more than one category applies to a hand, then only the highest ranking applicable category is used to describe the hand. For example, any “straight flush” is also a “straight” and a “flush,” but would simply be described as a straight flush since that category outranks the categories straight and flush. And it is not possible to have a “five high” or “six high” hand because it would also be a straight and the category straight outranks “high card.”Note that when considering whether a hand is a straight or straight flush, an Ace can be treated as either as a 1 (called low, for example the hand AH, 2D, 3C, 4H, 5S is a straight) or as a 14 (called high, for example the hand 10H, JD, QC, KH, AS is a straight). An Ace cannot be treated as both low and high in the same hand. Note that the order in which the cards are listed in the hand does notmatter, so that the hand 5S, 2D, 4H, AH, 3C (these are the same cards as in the first example of a straight but in a different order) is also a straight.You program should represent a card by storing the card’s kind and suit as follows. A card’s kind should be the integer 1 if it is an Ace, the integer 2 if it is a two, the integer 3 if it is a three, etc., the integer 10 if is a ten, the integer 11 if it is a Jack, the integer 12 if it is Queen, and the integer 13 if it is a King. The card’s suit should be the integer 0 if it is a Diamond, 1 if it is a Heart, 2 if it is a Club, and 3 if it is a Spade. So a card consists of two pieces of data, but we would like to be able to treat a card a single “thing” (for example, we would like to be able to return a “card” from a method). Thus, your program should use an array (one-dimensional) of integers with two elements to represent a card. The first element represents the card’s kind and the second element represents the card’s suit.Write a method with the following header which generates a random card by randomly generating integers in the appropriate ranges for the card’s kind and suit. (Note that zero is valid for the suit but not for the kind.) The method should return a reference
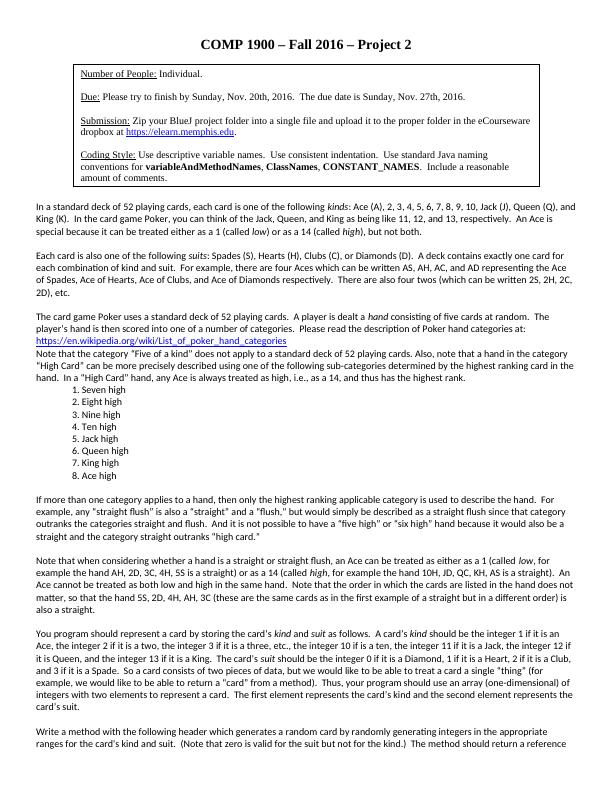
to an array of integers containing two elements – the first element represents the card’s kind and the second the card’s suit.public static int[] drawCard()To represent a 5-card hand, use a two-dimensional array with 5 rows and 2 columns. The 5 rows represent the 5 cards. You can think of each row as a one-dimensional array representing one card. That is, the first column represents each card’s kind. The second column represents each card’s suit.Write a method with the following header which generates a random 5-card hand. It should make one or more calls to drawCard()to generate each card in the hand. Before adding a card to the hand, it must check whether a card with the same kindand suit already appears in the hand. If so, it must not add the card to the hand but instead continue calling drawCard()until a card is generated whose combination of kind and suit does not yet appear in the hand. Once a hand consisting of 5 distinct cards has been generated, the method should return a reference to the two dimensional array representing the hand.public static int[][] drawHand()To repeat, you must ensure that no card appears twice in the same hand. For example, the hand 2D, AC, 9S, 2D, 9H is not a legal hand because the two of diamonds appears twice. Of course it is fine that there are two 9’s in this hand because they are of different suits.Next, write a method with the following header that classifies the hand into one of the following categories, which are listed in increasing order of rank. The method should return an integer from 1 to 17 indicating the highest of the 17 ranks below which the hand satisfies. You must remember that when considering whether a hand is a straight or straight flush, an Ace can count as either a1 or a 14, but not both. A Royal Flush is a Straight Flush in which a 10 is the lowest ranking card in the hand, such as 10S, JS, QS, KS, AS. Again, order does not matter, so QH, 10H, AH, KH, JH is also a Royal Flush.public static int classifyHand(int[][] hand)1. Seven high2. Eight high3. Nine high4. Ten high5. Jack high6. Queen high7. King high8. Ace high9. One Pair10. Two pair11. 3 of a kind12. Straight13. Flush14. Full house15. 4 of a kind16. Straight flush17. Royal FlushYour main method should generate 10,000,000 random hands by making calls to drawhand and classify each hand by calling classifyHand. It should keep counts of the number of hands that fall into each category above. For each of the first 50 hands (out of the 10,000,000 hands) generated, your program should display the hand number (1-50), the five cards in the hand including the kind and suit of each card using the same notation used here, and the name of the (highest) appropriate rank of the hand from the 17 categories above. After the first 50 hands, display the hand count, hand, and hand rank only if the count of the hands so far for that category is ten or less.After 10,000,000 random hands have been generated, display the name of each hand category followed by the percentage of hands that fell into that category. After printing the percentages for categories 1-8 (which are sub-categories of the “High Card” category), print a blank line followed by a “High Card” category with the total percentage from the sub-categories 1-8. Then continue printing the percentages for categories 9-17. Please see the example output that appears at the end of this document, including the category percentages at the very end.Write your own code for all computations. Do not call any methods that come with Java which operate on entire arrays. If your program is written correctly, the percentages for each hand category should be very close to those in the “Probability” column at:https://en.wikipedia.org/wiki/Poker_probability
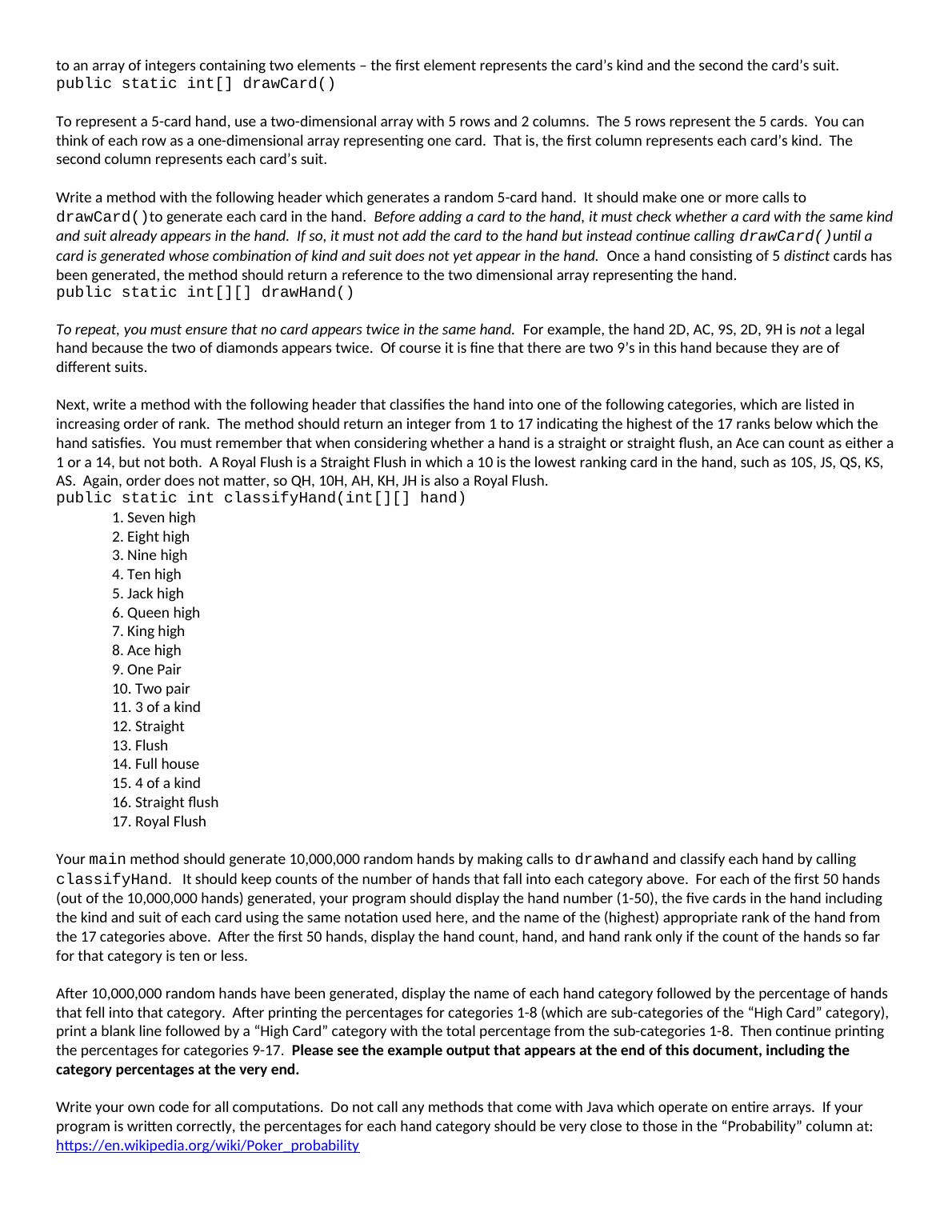
End of preview
Want to access all the pages? Upload your documents or become a member.
Related Documents
Using enum and LinkedList structures for Deckliblg...
|8
|2037
|334