Designing a Queue Management System
VerifiedAdded on 2019/09/13
|10
|2062
|432
Report
AI Summary
The assignment is to create a program that simulates a call center. The program should allow users to add calls to the queue, put calls through to operators, remove calls from the queue (customer disconnect), and update the queue. The program should also calculate the average number of calls waiting and the average wait time for a call to be sent to an operator. Additional functionality can be added as necessary.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.

Data structure & algorithms
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.

Task 1
This assignment requires you to produce fully working program/programs, which will
compare operation times of various sorting algorithms for given sets of data.
You should carefully consider the criteria below and then develop a fully documented
program that will calculate the time taken to sort lists of 10, 100, 1000, and 10,000 integer
numbers which will be supplied by the tutor in class.
Note: The program has been done if you need I would forward the code as well
This code done by visual basic studio 2010
Task 1:
#include<iostream>
#include <conio.h>
#include <time.h>
#include <windows.h>
using namespace std;
int menuChoice = 0;
int main()
{
/*int a[50],n,i,j,temp;
cout<<"Enter the size of array: ";
cin>>n;
cout<<"Enter the array elements: ";
*/
DWORD starttime,endtime;//variables to store start and end times
float totaltime;//a float variable for the total time
cout << "How many numbers?\n";
cin >> menuChoice;
starttime = GetTickCount();//get the start time
srand(time(NULL));
int a[10000];
for(int i = 0; i < menuChoice; i++)
{
a[i] = rand() % 10000 + 1;
}
int temp;
for(int i=1;i<menuChoice;++i)
{
for(int j=0;j<(menuChoice-i);++j)
if(a[j]>a[j+1])
{
temp=a[j];
a[j]=a[j+1];
a[j+1]=temp;
}
This assignment requires you to produce fully working program/programs, which will
compare operation times of various sorting algorithms for given sets of data.
You should carefully consider the criteria below and then develop a fully documented
program that will calculate the time taken to sort lists of 10, 100, 1000, and 10,000 integer
numbers which will be supplied by the tutor in class.
Note: The program has been done if you need I would forward the code as well
This code done by visual basic studio 2010
Task 1:
#include<iostream>
#include <conio.h>
#include <time.h>
#include <windows.h>
using namespace std;
int menuChoice = 0;
int main()
{
/*int a[50],n,i,j,temp;
cout<<"Enter the size of array: ";
cin>>n;
cout<<"Enter the array elements: ";
*/
DWORD starttime,endtime;//variables to store start and end times
float totaltime;//a float variable for the total time
cout << "How many numbers?\n";
cin >> menuChoice;
starttime = GetTickCount();//get the start time
srand(time(NULL));
int a[10000];
for(int i = 0; i < menuChoice; i++)
{
a[i] = rand() % 10000 + 1;
}
int temp;
for(int i=1;i<menuChoice;++i)
{
for(int j=0;j<(menuChoice-i);++j)
if(a[j]>a[j+1])
{
temp=a[j];
a[j]=a[j+1];
a[j+1]=temp;
}
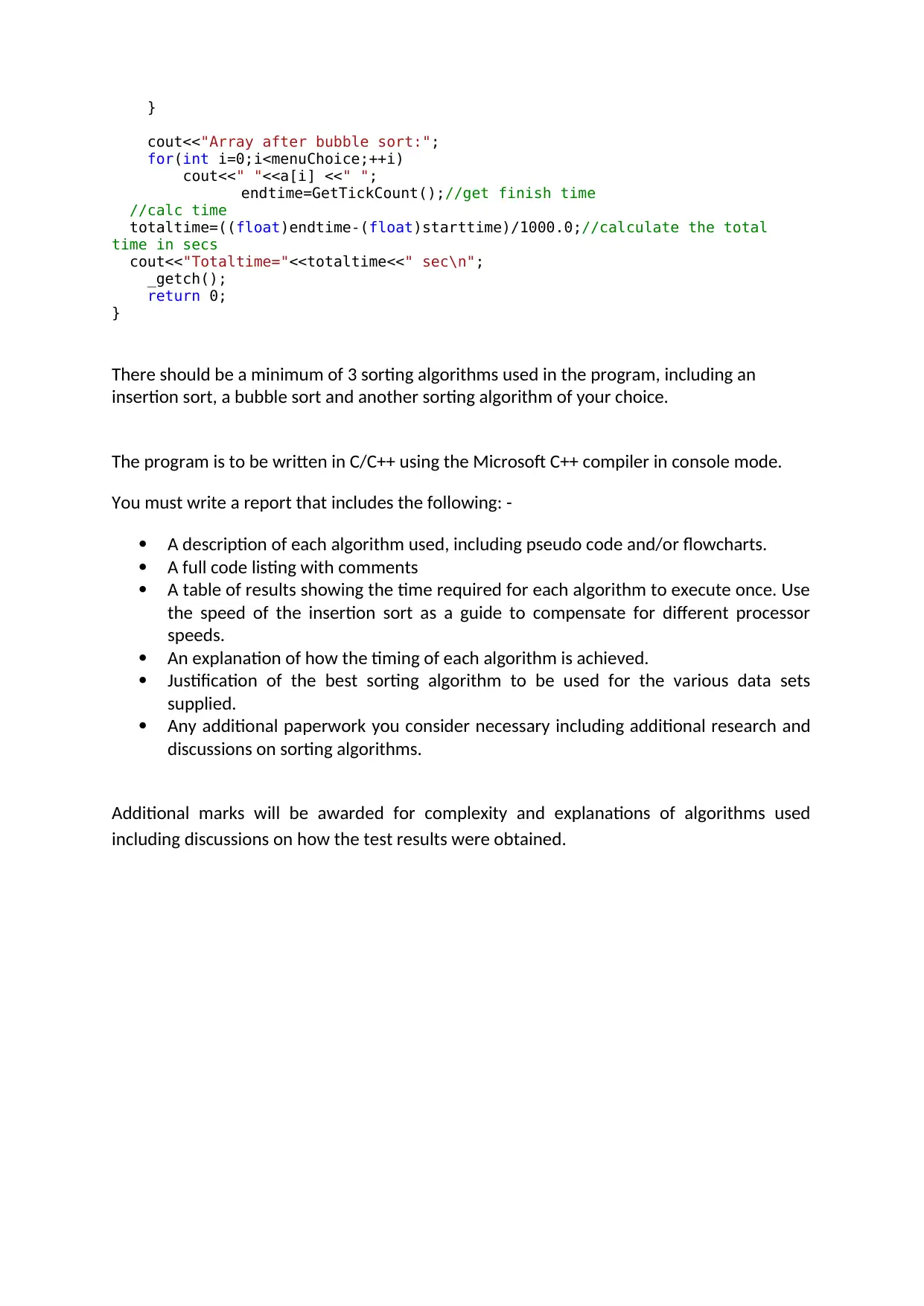
}
cout<<"Array after bubble sort:";
for(int i=0;i<menuChoice;++i)
cout<<" "<<a[i] <<" ";
endtime=GetTickCount();//get finish time
//calc time
totaltime=((float)endtime-(float)starttime)/1000.0;//calculate the total
time in secs
cout<<"Totaltime="<<totaltime<<" sec\n";
_getch();
return 0;
}
There should be a minimum of 3 sorting algorithms used in the program, including an
insertion sort, a bubble sort and another sorting algorithm of your choice.
The program is to be written in C/C++ using the Microsoft C++ compiler in console mode.
You must write a report that includes the following: -
A description of each algorithm used, including pseudo code and/or flowcharts.
A full code listing with comments
A table of results showing the time required for each algorithm to execute once. Use
the speed of the insertion sort as a guide to compensate for different processor
speeds.
An explanation of how the timing of each algorithm is achieved.
Justification of the best sorting algorithm to be used for the various data sets
supplied.
Any additional paperwork you consider necessary including additional research and
discussions on sorting algorithms.
Additional marks will be awarded for complexity and explanations of algorithms used
including discussions on how the test results were obtained.
cout<<"Array after bubble sort:";
for(int i=0;i<menuChoice;++i)
cout<<" "<<a[i] <<" ";
endtime=GetTickCount();//get finish time
//calc time
totaltime=((float)endtime-(float)starttime)/1000.0;//calculate the total
time in secs
cout<<"Totaltime="<<totaltime<<" sec\n";
_getch();
return 0;
}
There should be a minimum of 3 sorting algorithms used in the program, including an
insertion sort, a bubble sort and another sorting algorithm of your choice.
The program is to be written in C/C++ using the Microsoft C++ compiler in console mode.
You must write a report that includes the following: -
A description of each algorithm used, including pseudo code and/or flowcharts.
A full code listing with comments
A table of results showing the time required for each algorithm to execute once. Use
the speed of the insertion sort as a guide to compensate for different processor
speeds.
An explanation of how the timing of each algorithm is achieved.
Justification of the best sorting algorithm to be used for the various data sets
supplied.
Any additional paperwork you consider necessary including additional research and
discussions on sorting algorithms.
Additional marks will be awarded for complexity and explanations of algorithms used
including discussions on how the test results were obtained.
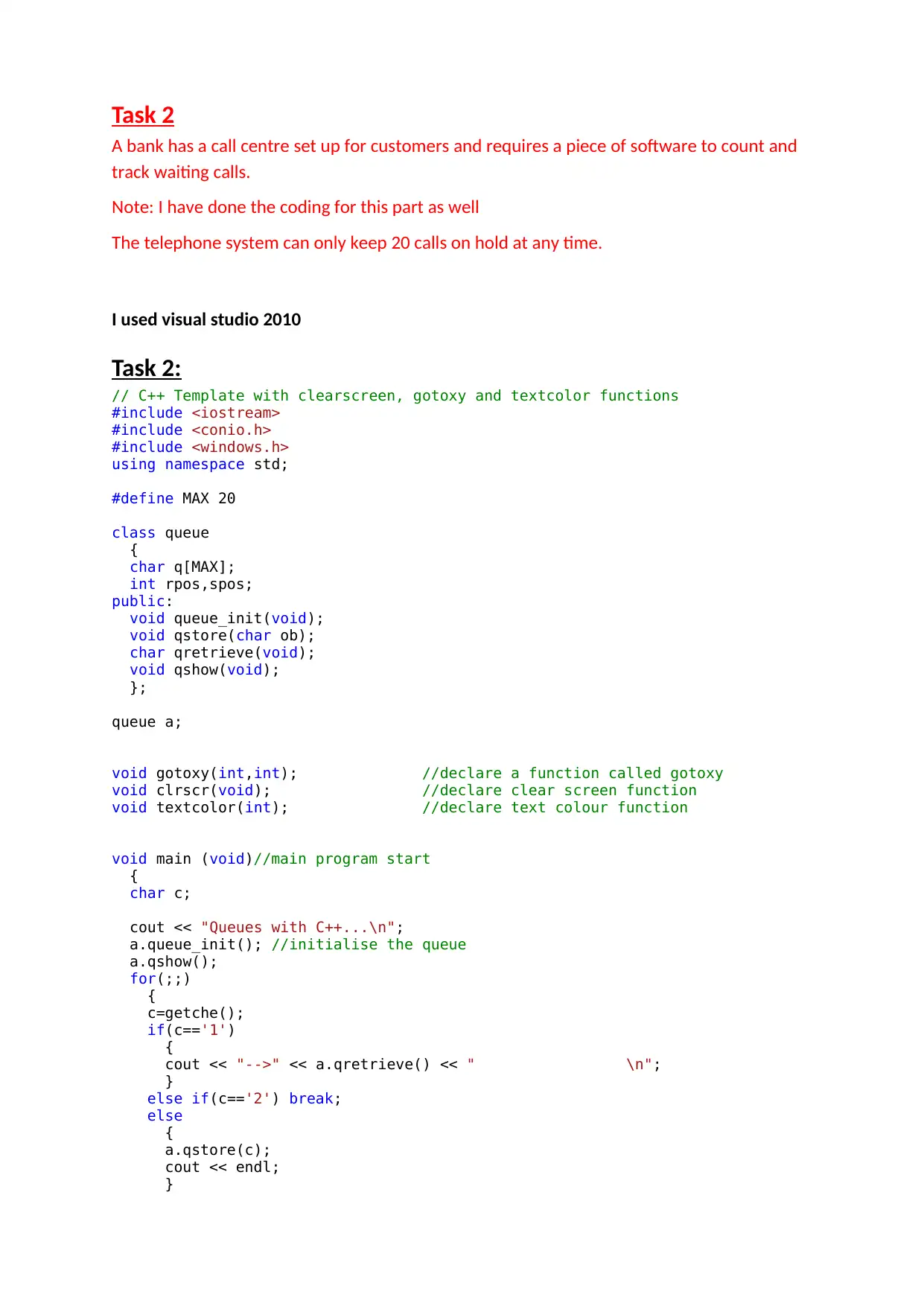
Task 2
A bank has a call centre set up for customers and requires a piece of software to count and
track waiting calls.
Note: I have done the coding for this part as well
The telephone system can only keep 20 calls on hold at any time.
I used visual studio 2010
Task 2:
// C++ Template with clearscreen, gotoxy and textcolor functions
#include <iostream>
#include <conio.h>
#include <windows.h>
using namespace std;
#define MAX 20
class queue
{
char q[MAX];
int rpos,spos;
public:
void queue_init(void);
void qstore(char ob);
char qretrieve(void);
void qshow(void);
};
queue a;
void gotoxy(int,int); //declare a function called gotoxy
void clrscr(void); //declare clear screen function
void textcolor(int); //declare text colour function
void main (void)//main program start
{
char c;
cout << "Queues with C++...\n";
a.queue_init(); //initialise the queue
a.qshow();
for(;;)
{
c=getche();
if(c=='1')
{
cout << "-->" << a.qretrieve() << " \n";
}
else if(c=='2') break;
else
{
a.qstore(c);
cout << endl;
}
A bank has a call centre set up for customers and requires a piece of software to count and
track waiting calls.
Note: I have done the coding for this part as well
The telephone system can only keep 20 calls on hold at any time.
I used visual studio 2010
Task 2:
// C++ Template with clearscreen, gotoxy and textcolor functions
#include <iostream>
#include <conio.h>
#include <windows.h>
using namespace std;
#define MAX 20
class queue
{
char q[MAX];
int rpos,spos;
public:
void queue_init(void);
void qstore(char ob);
char qretrieve(void);
void qshow(void);
};
queue a;
void gotoxy(int,int); //declare a function called gotoxy
void clrscr(void); //declare clear screen function
void textcolor(int); //declare text colour function
void main (void)//main program start
{
char c;
cout << "Queues with C++...\n";
a.queue_init(); //initialise the queue
a.qshow();
for(;;)
{
c=getche();
if(c=='1')
{
cout << "-->" << a.qretrieve() << " \n";
}
else if(c=='2') break;
else
{
a.qstore(c);
cout << endl;
}
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.

a.qshow();
}
}//end of the main code
//put functions here
//store a character
void queue::qstore(char ob) // push
{
if((rpos==MAX-1 && spos==0) || (rpos==spos-1))//check if full
{
cout << "Queue full \n";
return;
}
if(spos==-1 && rpos==-1)//special case if no data in queue
{
spos++;
rpos++;
q[spos]=ob;
}
else
{
rpos++;
if(rpos==MAX) rpos=0;
q[rpos]=ob;
}
}
//retrieve a character
char queue::qretrieve(void) // pop
{
char temp;
if (rpos==-1 && spos==-1)//no data to retrieve
{
cout << "Queue empty \n";
return (char)0;
}
if(rpos==spos && rpos!=-1)//if there is only one piece of data in the queue
{
temp=q[spos];
q[spos]=' ';
spos=-1;
rpos=-1;
}
else
{
temp=q[spos];
q[spos]=' ';
spos++;
if (spos==MAX) spos=0;
}
return temp;
}
//initialise the queue
void queue::queue_init(void)
{
spos=-1;
rpos=-1;
}
}
}//end of the main code
//put functions here
//store a character
void queue::qstore(char ob) // push
{
if((rpos==MAX-1 && spos==0) || (rpos==spos-1))//check if full
{
cout << "Queue full \n";
return;
}
if(spos==-1 && rpos==-1)//special case if no data in queue
{
spos++;
rpos++;
q[spos]=ob;
}
else
{
rpos++;
if(rpos==MAX) rpos=0;
q[rpos]=ob;
}
}
//retrieve a character
char queue::qretrieve(void) // pop
{
char temp;
if (rpos==-1 && spos==-1)//no data to retrieve
{
cout << "Queue empty \n";
return (char)0;
}
if(rpos==spos && rpos!=-1)//if there is only one piece of data in the queue
{
temp=q[spos];
q[spos]=' ';
spos=-1;
rpos=-1;
}
else
{
temp=q[spos];
q[spos]=' ';
spos++;
if (spos==MAX) spos=0;
}
return temp;
}
//initialise the queue
void queue::queue_init(void)
{
spos=-1;
rpos=-1;
}

void queue::qshow(void)
{
int i;
for(i=0;i<MAX;i++)
{
gotoxy(40,3+i);
cout << i << " = " << a.q[i];
}
cout << endl;
gotoxy(20,3);
cout << "spos = " << spos << " "<<endl;
gotoxy(20,4);
cout << "rpos = " << rpos << " "<<endl;
gotoxy(1,2);
}
//Alter the code below at your peril!!!!
//It defines the textcolor,gotoxy and clrscr functions
void textcolor(int c)
{
HANDLE hConsole;
hConsole = GetStdHandle(STD_OUTPUT_HANDLE);
SetConsoleTextAttribute(hConsole, c);
}
void gotoxy(int x, int y) //define gotoxy function
{
static HANDLE hStdout = NULL;
COORD coord;
coord.X = x;
coord.Y = y;
if(!hStdout) {hStdout = GetStdHandle(STD_OUTPUT_HANDLE);}
SetConsoleCursorPosition(hStdout,coord);
}
void clrscr(void)
{
static HANDLE hStdout = NULL;
static CONSOLE_SCREEN_BUFFER_INFO csbi;
const COORD startCoords = {0,0};
DWORD dummy;
if(!hStdout)
{
hStdout = GetStdHandle(STD_OUTPUT_HANDLE);
GetConsoleScreenBufferInfo(hStdout,&csbi);
}
FillConsoleOutputCharacter(hStdout,' ',csbi.dwSize.X *
csbi.dwSize.Y,startCoords,&dummy);
gotoxy(0,0);
}
Once a call is made to the bank the following information is logged on a computer and put
into a queue.
Call number
Time called
Queue ID number
{
int i;
for(i=0;i<MAX;i++)
{
gotoxy(40,3+i);
cout << i << " = " << a.q[i];
}
cout << endl;
gotoxy(20,3);
cout << "spos = " << spos << " "<<endl;
gotoxy(20,4);
cout << "rpos = " << rpos << " "<<endl;
gotoxy(1,2);
}
//Alter the code below at your peril!!!!
//It defines the textcolor,gotoxy and clrscr functions
void textcolor(int c)
{
HANDLE hConsole;
hConsole = GetStdHandle(STD_OUTPUT_HANDLE);
SetConsoleTextAttribute(hConsole, c);
}
void gotoxy(int x, int y) //define gotoxy function
{
static HANDLE hStdout = NULL;
COORD coord;
coord.X = x;
coord.Y = y;
if(!hStdout) {hStdout = GetStdHandle(STD_OUTPUT_HANDLE);}
SetConsoleCursorPosition(hStdout,coord);
}
void clrscr(void)
{
static HANDLE hStdout = NULL;
static CONSOLE_SCREEN_BUFFER_INFO csbi;
const COORD startCoords = {0,0};
DWORD dummy;
if(!hStdout)
{
hStdout = GetStdHandle(STD_OUTPUT_HANDLE);
GetConsoleScreenBufferInfo(hStdout,&csbi);
}
FillConsoleOutputCharacter(hStdout,' ',csbi.dwSize.X *
csbi.dwSize.Y,startCoords,&dummy);
gotoxy(0,0);
}
Once a call is made to the bank the following information is logged on a computer and put
into a queue.
Call number
Time called
Queue ID number

The program will initially have no calls in memory. A basic menu screen will allow the user to
perform the following operations: -
1. Add data to the queue.
2. Put call through to operator.
3. Remove call from the queue (customer disconnect).
4. Update queue.
When a call comes in the data is added to the queue.
If a call is at the top of the queue then it will be the next one to get a free operator.
Some calls will be disconnected by the customer before they reach the top of the
queue, your program should allow for this.
On the menu screen you should also display the calls waiting and the time each call has
been waiting.
This information is then to be used to calculate an average number of calls waiting and also
the average wait time for a call to be sent to an operator. This should also be shown on the
screen.
You may add additional functionality to the program if you consider it necessary.
Task 2.1
Discuss the use and implementation of various methods to store data and also discuss the
method you will use to create a working program which is best suited for this problem.
Task 2.2
You must produce a specification for the data structure used. Fully explain how you will
implement the data structure.
Task 2.3
Produce a working program in c++ which creates and uses your chosen algorithm for this
scenario. Each call is to be created as a new memory object using object orientated
techniques
perform the following operations: -
1. Add data to the queue.
2. Put call through to operator.
3. Remove call from the queue (customer disconnect).
4. Update queue.
When a call comes in the data is added to the queue.
If a call is at the top of the queue then it will be the next one to get a free operator.
Some calls will be disconnected by the customer before they reach the top of the
queue, your program should allow for this.
On the menu screen you should also display the calls waiting and the time each call has
been waiting.
This information is then to be used to calculate an average number of calls waiting and also
the average wait time for a call to be sent to an operator. This should also be shown on the
screen.
You may add additional functionality to the program if you consider it necessary.
Task 2.1
Discuss the use and implementation of various methods to store data and also discuss the
method you will use to create a working program which is best suited for this problem.
Task 2.2
You must produce a specification for the data structure used. Fully explain how you will
implement the data structure.
Task 2.3
Produce a working program in c++ which creates and uses your chosen algorithm for this
scenario. Each call is to be created as a new memory object using object orientated
techniques
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser

Document and test the program and provide screenshots and a full code listing of your
program.
Include any additional information necessary.
Task 2.4
Evaluate your program and suggest areas for improvement.
Marking Criteria:
Marks will be awarded on the basis of:
Requirements Marks
available
Marks
awarded
Feedback
Task 1
Inclusion of 3 sorting algorithms
(5 marks per algorithm)
Explanation of algorithms, test data and use
of C++ language
Evaluation of sorting algorithms used for
different sizes of data sets
(tables of results, graphs, evaluate data)
Additional features
(Additional algorithms, additional testing,
15
15
10
10
program.
Include any additional information necessary.
Task 2.4
Evaluate your program and suggest areas for improvement.
Marking Criteria:
Marks will be awarded on the basis of:
Requirements Marks
available
Marks
awarded
Feedback
Task 1
Inclusion of 3 sorting algorithms
(5 marks per algorithm)
Explanation of algorithms, test data and use
of C++ language
Evaluation of sorting algorithms used for
different sizes of data sets
(tables of results, graphs, evaluate data)
Additional features
(Additional algorithms, additional testing,
15
15
10
10
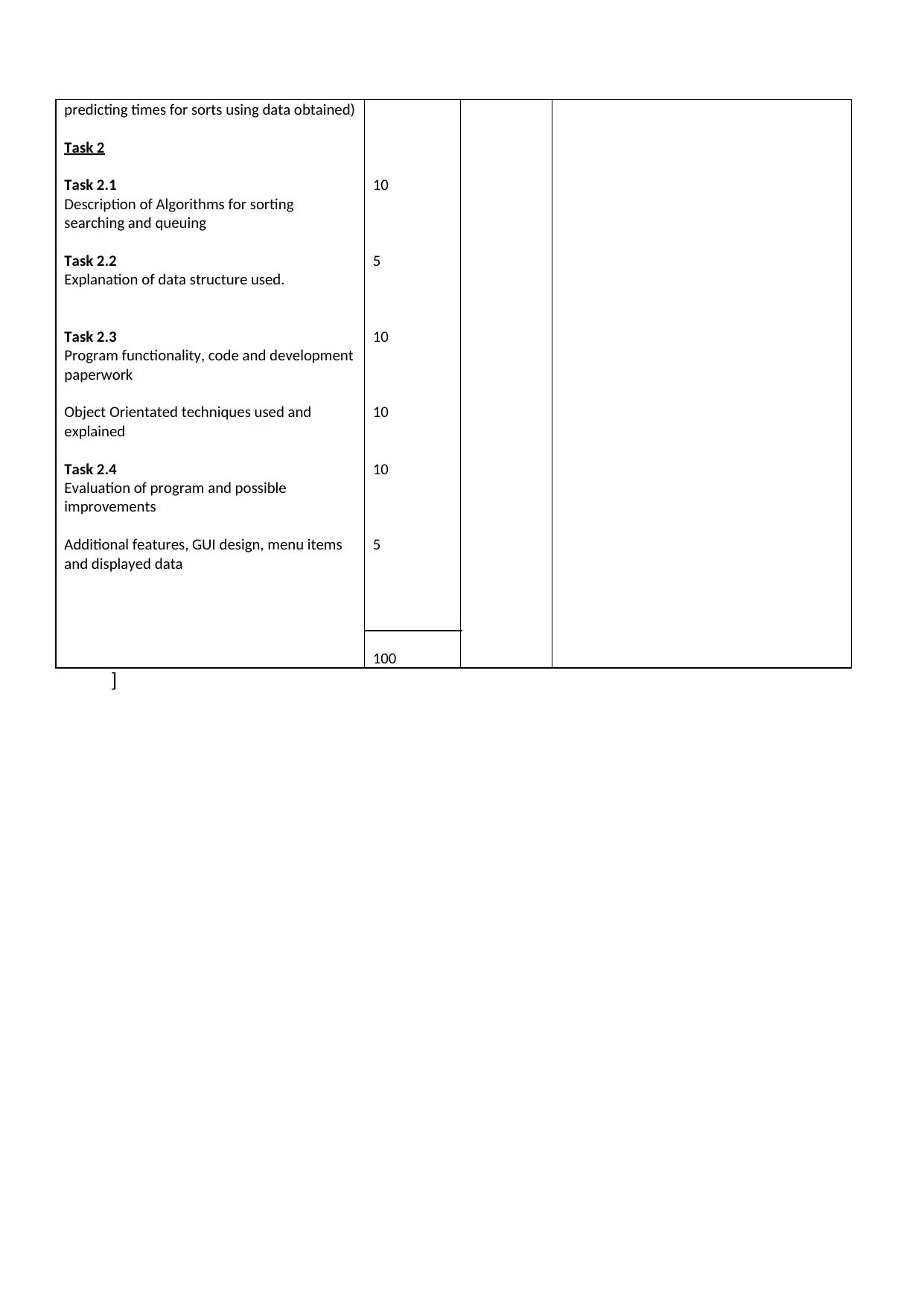
predicting times for sorts using data obtained)
Task 2
Task 2.1
Description of Algorithms for sorting
searching and queuing
Task 2.2
Explanation of data structure used.
Task 2.3
Program functionality, code and development
paperwork
Object Orientated techniques used and
explained
Task 2.4
Evaluation of program and possible
improvements
Additional features, GUI design, menu items
and displayed data
10
5
10
10
10
5
100
]
Task 2
Task 2.1
Description of Algorithms for sorting
searching and queuing
Task 2.2
Explanation of data structure used.
Task 2.3
Program functionality, code and development
paperwork
Object Orientated techniques used and
explained
Task 2.4
Evaluation of program and possible
improvements
Additional features, GUI design, menu items
and displayed data
10
5
10
10
10
5
100
]
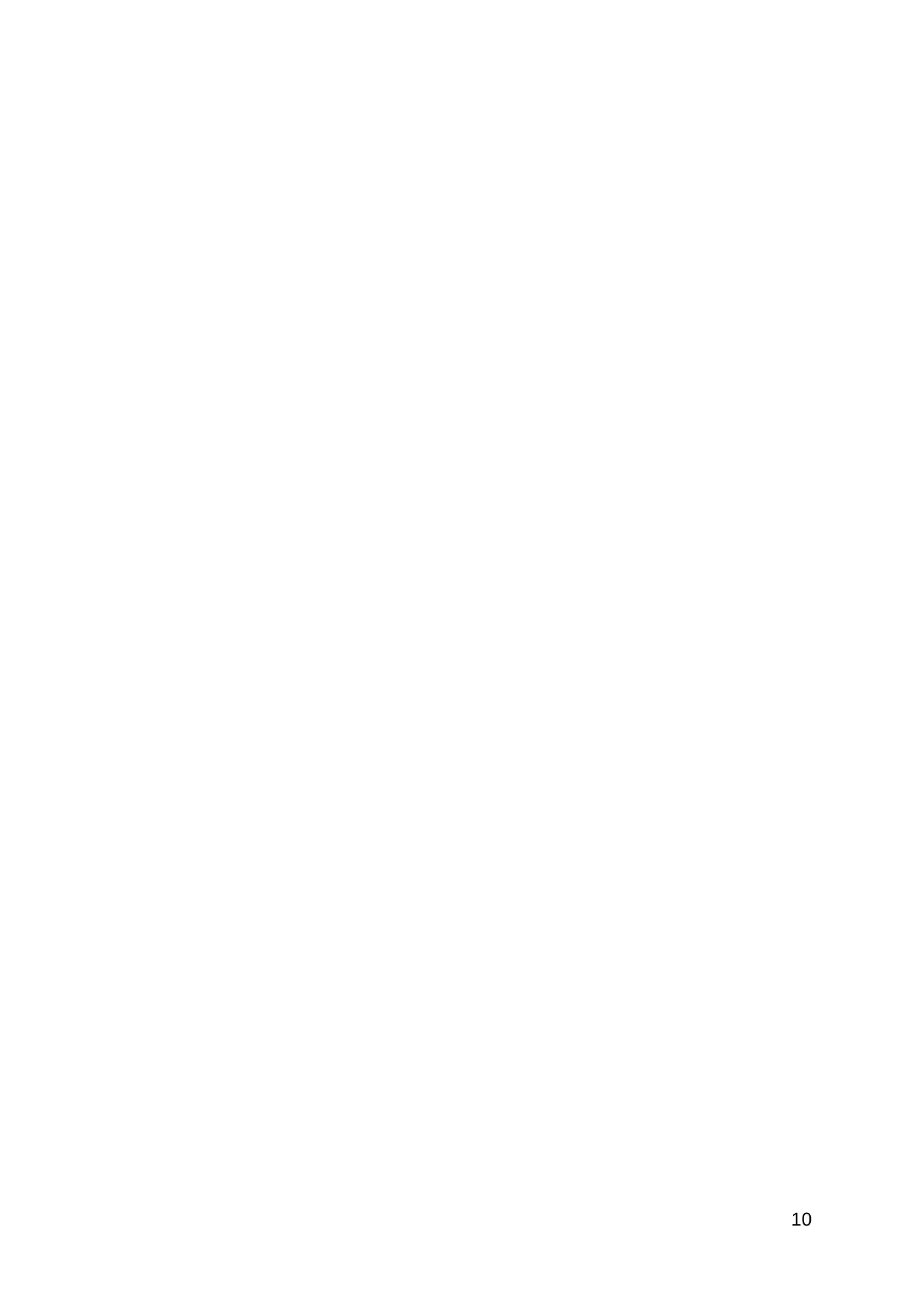
10
1 out of 10
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.