Data Structure and Algorithms Australia Report 2022
VerifiedAdded on 2022/10/13
|17
|2852
|12
AI Summary
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
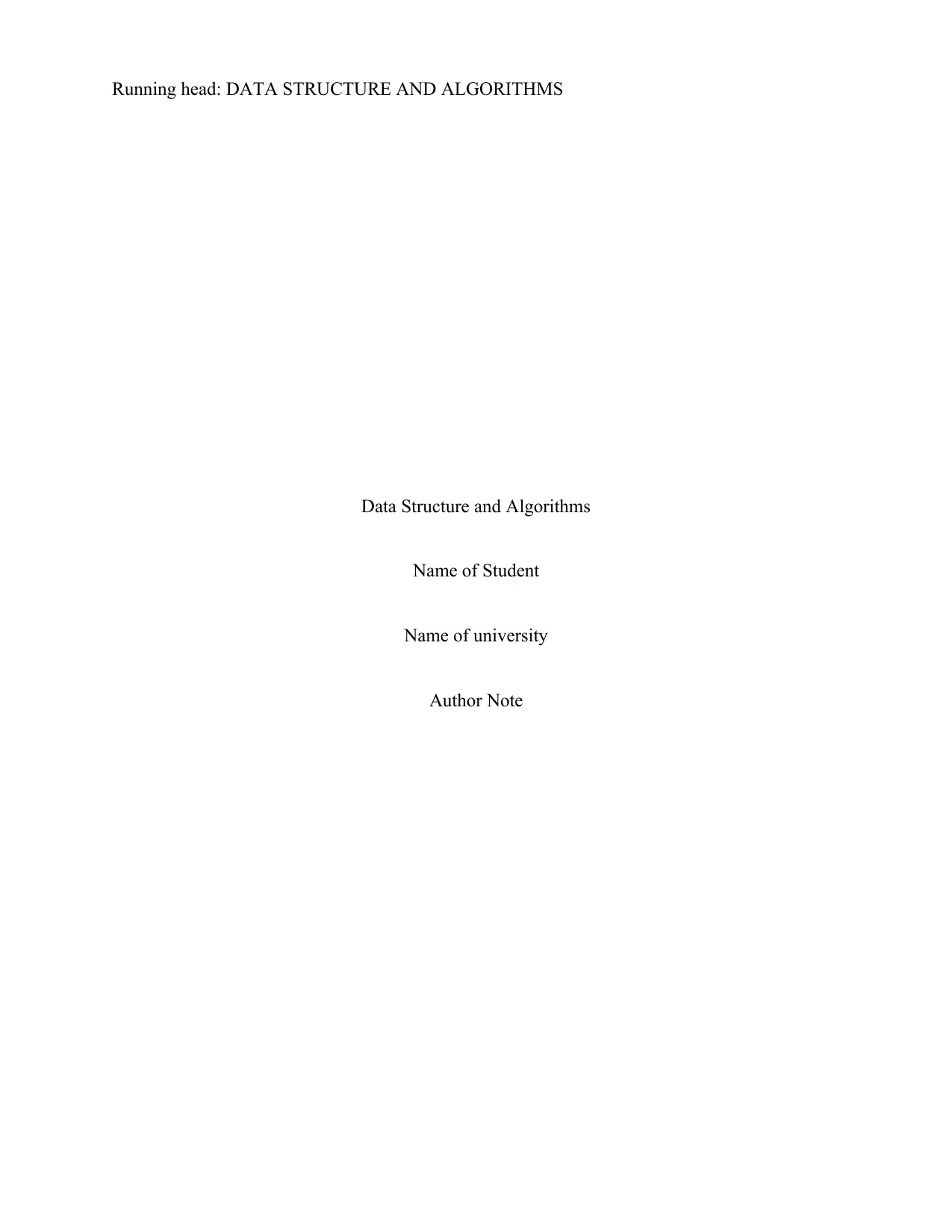
Running head: DATA STRUCTURE AND ALGORITHMS
Data Structure and Algorithms
Name of Student
Name of university
Author Note
Data Structure and Algorithms
Name of Student
Name of university
Author Note
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
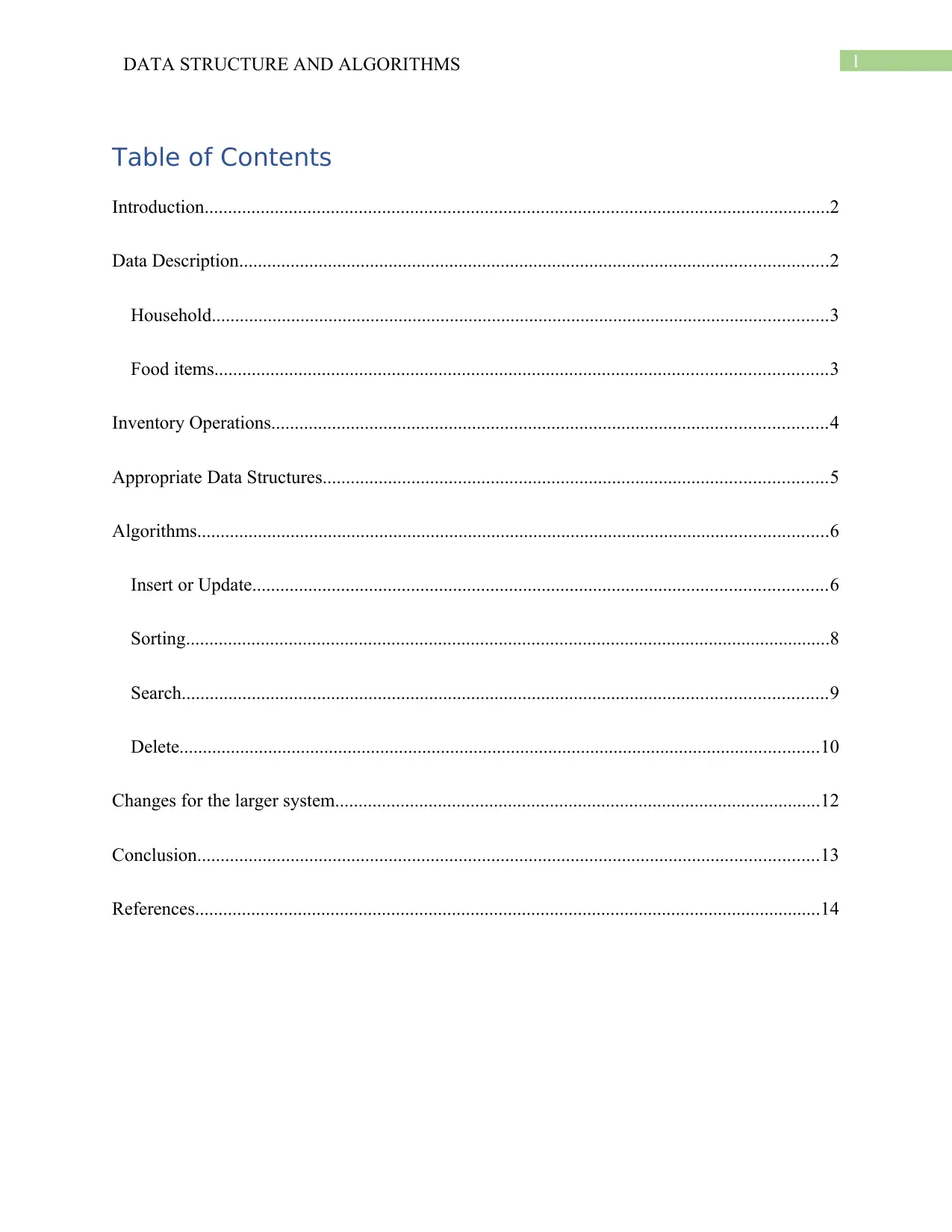
1DATA STRUCTURE AND ALGORITHMS
Table of Contents
Introduction......................................................................................................................................2
Data Description..............................................................................................................................2
Household....................................................................................................................................3
Food items...................................................................................................................................3
Inventory Operations.......................................................................................................................4
Appropriate Data Structures............................................................................................................5
Algorithms.......................................................................................................................................6
Insert or Update...........................................................................................................................6
Sorting..........................................................................................................................................8
Search..........................................................................................................................................9
Delete.........................................................................................................................................10
Changes for the larger system........................................................................................................12
Conclusion.....................................................................................................................................13
References......................................................................................................................................14
Table of Contents
Introduction......................................................................................................................................2
Data Description..............................................................................................................................2
Household....................................................................................................................................3
Food items...................................................................................................................................3
Inventory Operations.......................................................................................................................4
Appropriate Data Structures............................................................................................................5
Algorithms.......................................................................................................................................6
Insert or Update...........................................................................................................................6
Sorting..........................................................................................................................................8
Search..........................................................................................................................................9
Delete.........................................................................................................................................10
Changes for the larger system........................................................................................................12
Conclusion.....................................................................................................................................13
References......................................................................................................................................14
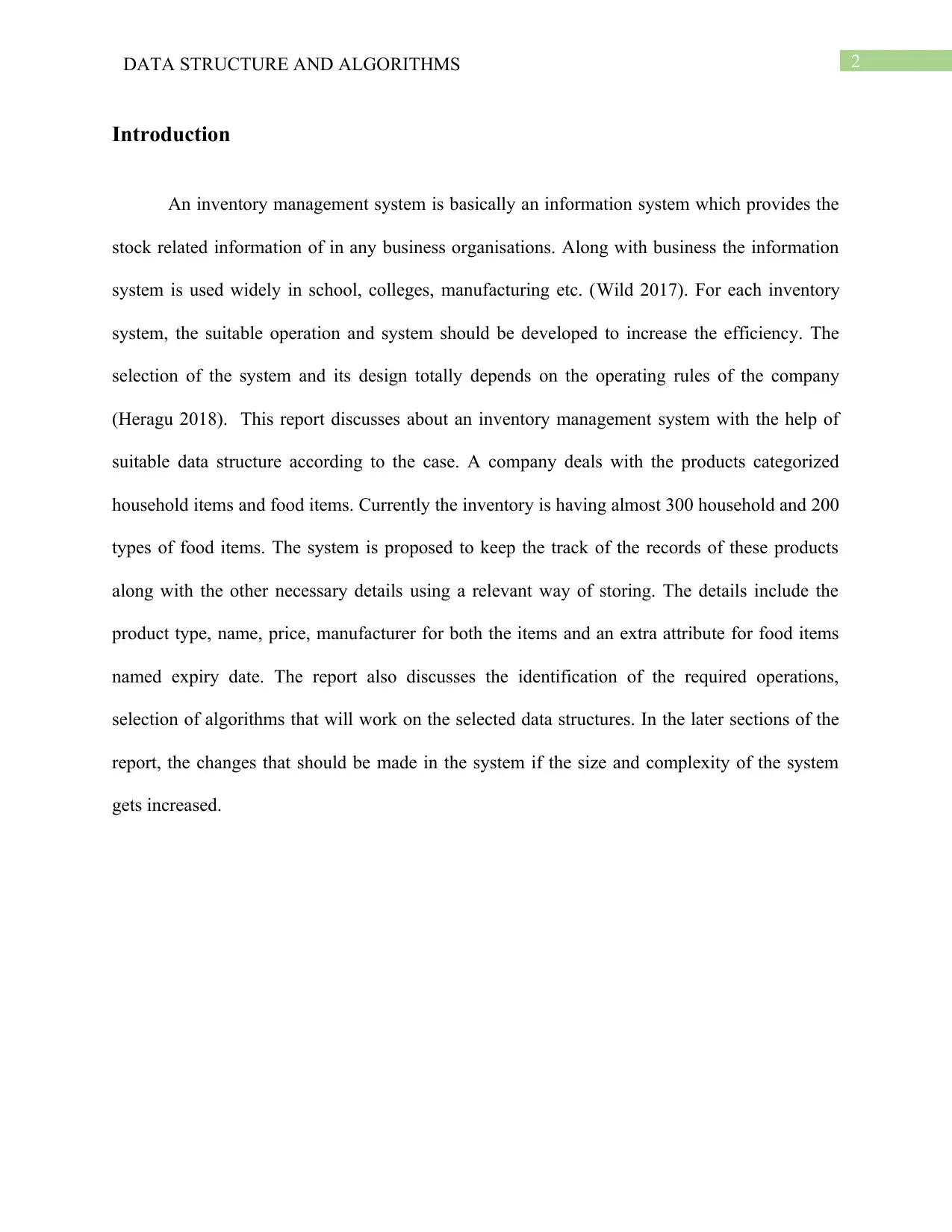
2DATA STRUCTURE AND ALGORITHMS
Introduction
An inventory management system is basically an information system which provides the
stock related information of in any business organisations. Along with business the information
system is used widely in school, colleges, manufacturing etc. (Wild 2017). For each inventory
system, the suitable operation and system should be developed to increase the efficiency. The
selection of the system and its design totally depends on the operating rules of the company
(Heragu 2018). This report discusses about an inventory management system with the help of
suitable data structure according to the case. A company deals with the products categorized
household items and food items. Currently the inventory is having almost 300 household and 200
types of food items. The system is proposed to keep the track of the records of these products
along with the other necessary details using a relevant way of storing. The details include the
product type, name, price, manufacturer for both the items and an extra attribute for food items
named expiry date. The report also discusses the identification of the required operations,
selection of algorithms that will work on the selected data structures. In the later sections of the
report, the changes that should be made in the system if the size and complexity of the system
gets increased.
Introduction
An inventory management system is basically an information system which provides the
stock related information of in any business organisations. Along with business the information
system is used widely in school, colleges, manufacturing etc. (Wild 2017). For each inventory
system, the suitable operation and system should be developed to increase the efficiency. The
selection of the system and its design totally depends on the operating rules of the company
(Heragu 2018). This report discusses about an inventory management system with the help of
suitable data structure according to the case. A company deals with the products categorized
household items and food items. Currently the inventory is having almost 300 household and 200
types of food items. The system is proposed to keep the track of the records of these products
along with the other necessary details using a relevant way of storing. The details include the
product type, name, price, manufacturer for both the items and an extra attribute for food items
named expiry date. The report also discusses the identification of the required operations,
selection of algorithms that will work on the selected data structures. In the later sections of the
report, the changes that should be made in the system if the size and complexity of the system
gets increased.
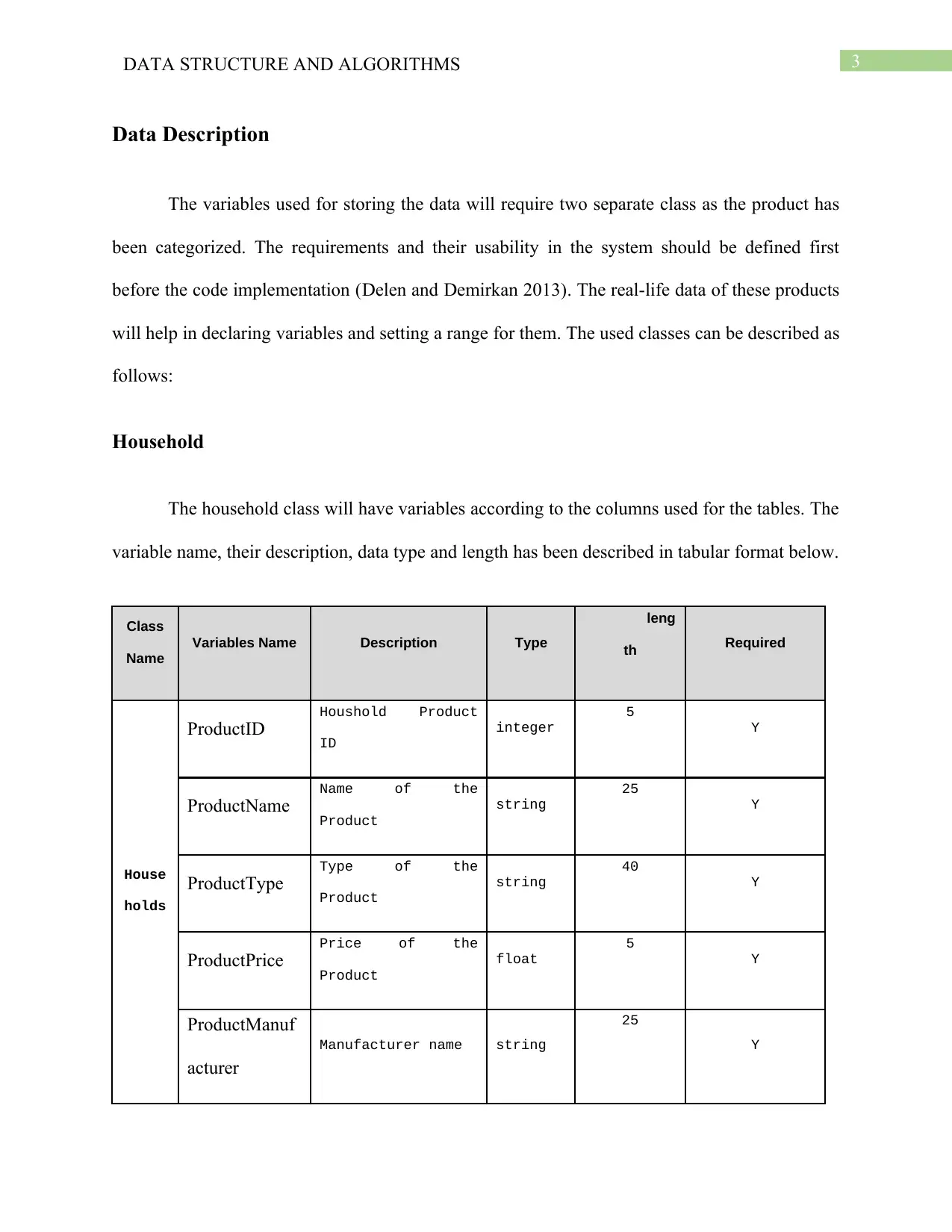
3DATA STRUCTURE AND ALGORITHMS
Data Description
The variables used for storing the data will require two separate class as the product has
been categorized. The requirements and their usability in the system should be defined first
before the code implementation (Delen and Demirkan 2013). The real-life data of these products
will help in declaring variables and setting a range for them. The used classes can be described as
follows:
Household
The household class will have variables according to the columns used for the tables. The
variable name, their description, data type and length has been described in tabular format below.
Class
Name
Variables Name Description Type
leng
th Required
House
holds
ProductID
Houshold Product
ID
integer
5
Y
ProductName
Name of the
Product
string
25
Y
ProductType
Type of the
Product
string
40
Y
ProductPrice
Price of the
Product
float
5
Y
ProductManuf
acturer
Manufacturer name string
25
Y
Data Description
The variables used for storing the data will require two separate class as the product has
been categorized. The requirements and their usability in the system should be defined first
before the code implementation (Delen and Demirkan 2013). The real-life data of these products
will help in declaring variables and setting a range for them. The used classes can be described as
follows:
Household
The household class will have variables according to the columns used for the tables. The
variable name, their description, data type and length has been described in tabular format below.
Class
Name
Variables Name Description Type
leng
th Required
House
holds
ProductID
Houshold Product
ID
integer
5
Y
ProductName
Name of the
Product
string
25
Y
ProductType
Type of the
Product
string
40
Y
ProductPrice
Price of the
Product
float
5
Y
ProductManuf
acturer
Manufacturer name string
25
Y
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
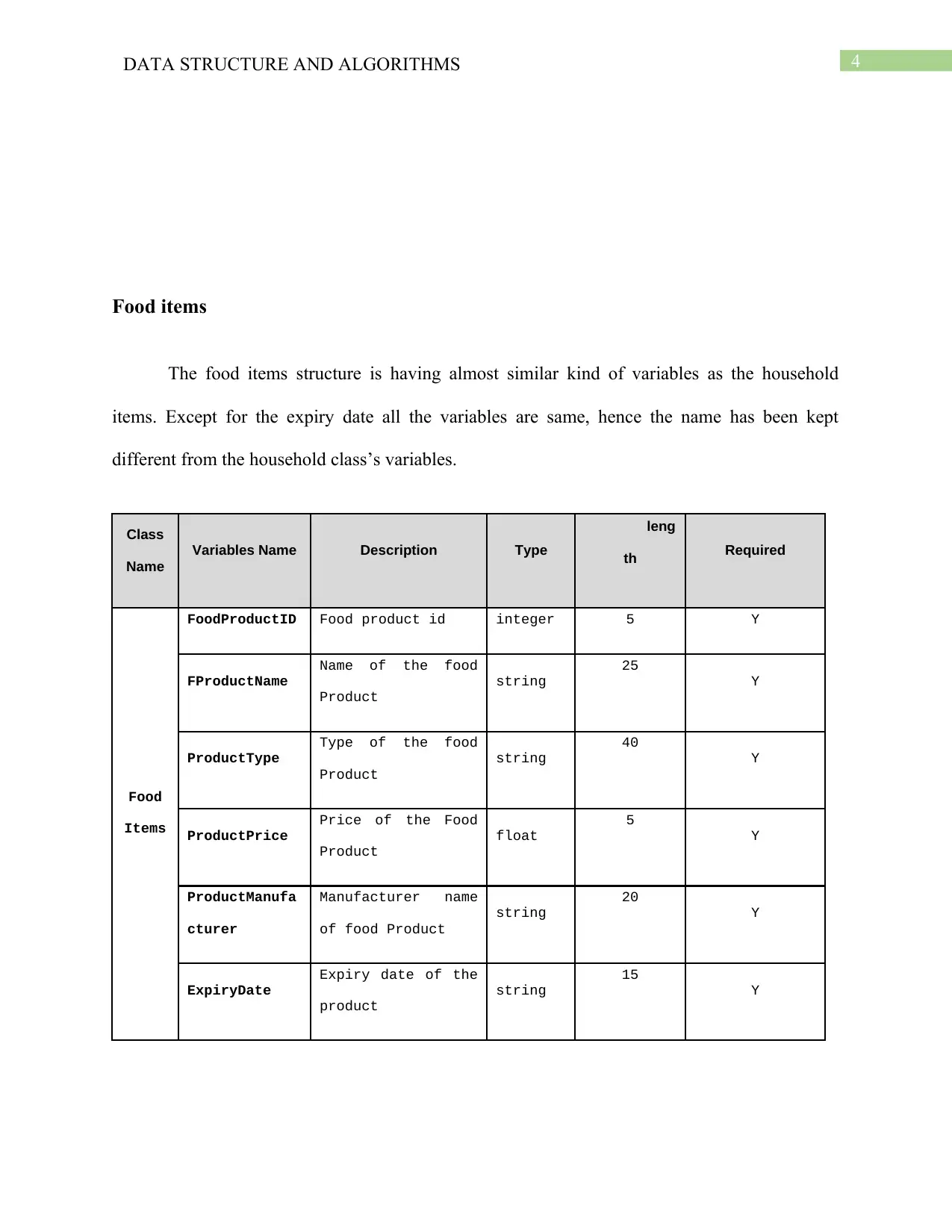
4DATA STRUCTURE AND ALGORITHMS
Food items
The food items structure is having almost similar kind of variables as the household
items. Except for the expiry date all the variables are same, hence the name has been kept
different from the household class’s variables.
Class
Name
Variables Name Description Type
leng
th Required
Food
Items
FoodProductID Food product id integer 5 Y
FProductName
Name of the food
Product
string
25
Y
ProductType
Type of the food
Product
string
40
Y
ProductPrice
Price of the Food
Product
float
5
Y
ProductManufa
cturer
Manufacturer name
of food Product
string
20
Y
ExpiryDate
Expiry date of the
product
string
15
Y
Food items
The food items structure is having almost similar kind of variables as the household
items. Except for the expiry date all the variables are same, hence the name has been kept
different from the household class’s variables.
Class
Name
Variables Name Description Type
leng
th Required
Food
Items
FoodProductID Food product id integer 5 Y
FProductName
Name of the food
Product
string
25
Y
ProductType
Type of the food
Product
string
40
Y
ProductPrice
Price of the Food
Product
float
5
Y
ProductManufa
cturer
Manufacturer name
of food Product
string
20
Y
ExpiryDate
Expiry date of the
product
string
15
Y
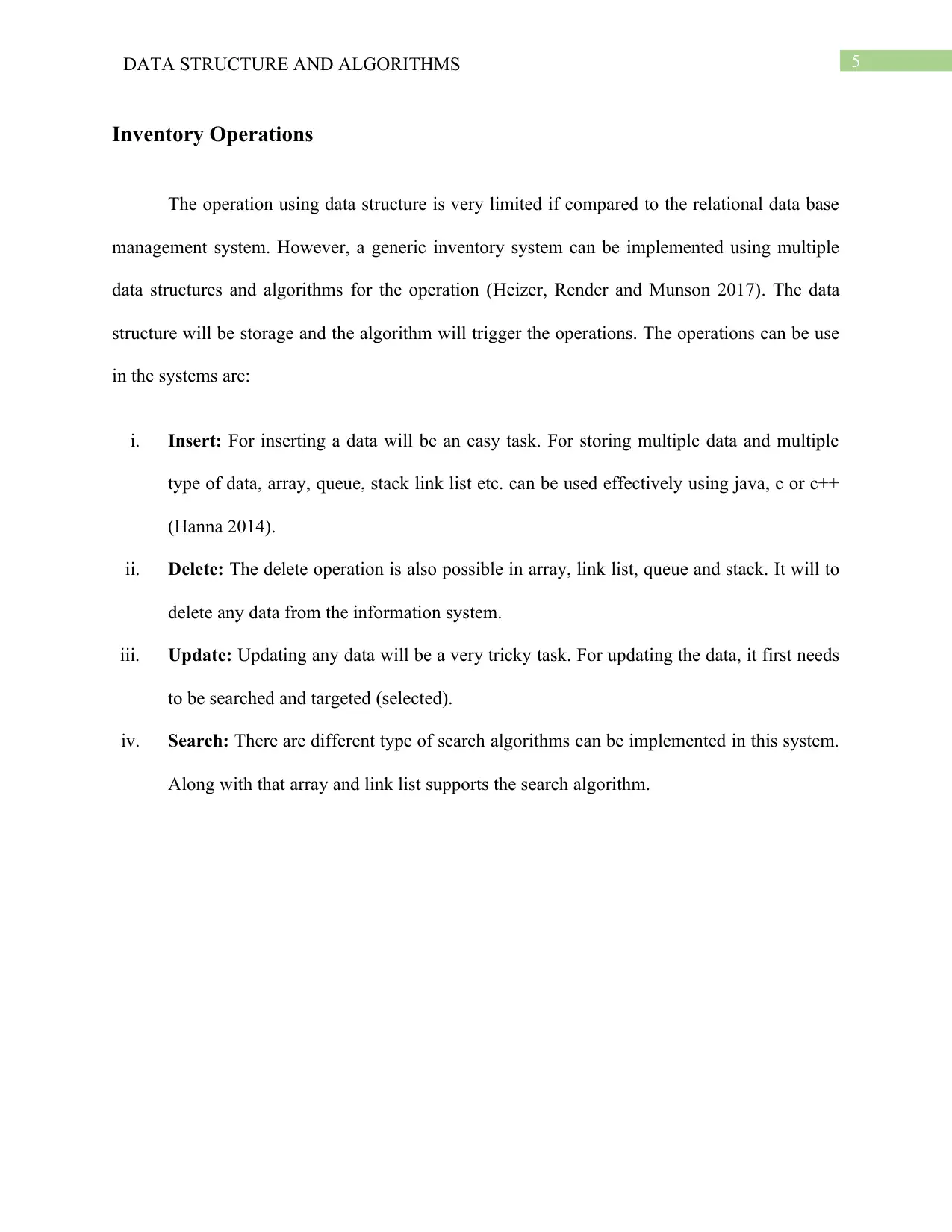
5DATA STRUCTURE AND ALGORITHMS
Inventory Operations
The operation using data structure is very limited if compared to the relational data base
management system. However, a generic inventory system can be implemented using multiple
data structures and algorithms for the operation (Heizer, Render and Munson 2017). The data
structure will be storage and the algorithm will trigger the operations. The operations can be use
in the systems are:
i. Insert: For inserting a data will be an easy task. For storing multiple data and multiple
type of data, array, queue, stack link list etc. can be used effectively using java, c or c++
(Hanna 2014).
ii. Delete: The delete operation is also possible in array, link list, queue and stack. It will to
delete any data from the information system.
iii. Update: Updating any data will be a very tricky task. For updating the data, it first needs
to be searched and targeted (selected).
iv. Search: There are different type of search algorithms can be implemented in this system.
Along with that array and link list supports the search algorithm.
Inventory Operations
The operation using data structure is very limited if compared to the relational data base
management system. However, a generic inventory system can be implemented using multiple
data structures and algorithms for the operation (Heizer, Render and Munson 2017). The data
structure will be storage and the algorithm will trigger the operations. The operations can be use
in the systems are:
i. Insert: For inserting a data will be an easy task. For storing multiple data and multiple
type of data, array, queue, stack link list etc. can be used effectively using java, c or c++
(Hanna 2014).
ii. Delete: The delete operation is also possible in array, link list, queue and stack. It will to
delete any data from the information system.
iii. Update: Updating any data will be a very tricky task. For updating the data, it first needs
to be searched and targeted (selected).
iv. Search: There are different type of search algorithms can be implemented in this system.
Along with that array and link list supports the search algorithm.
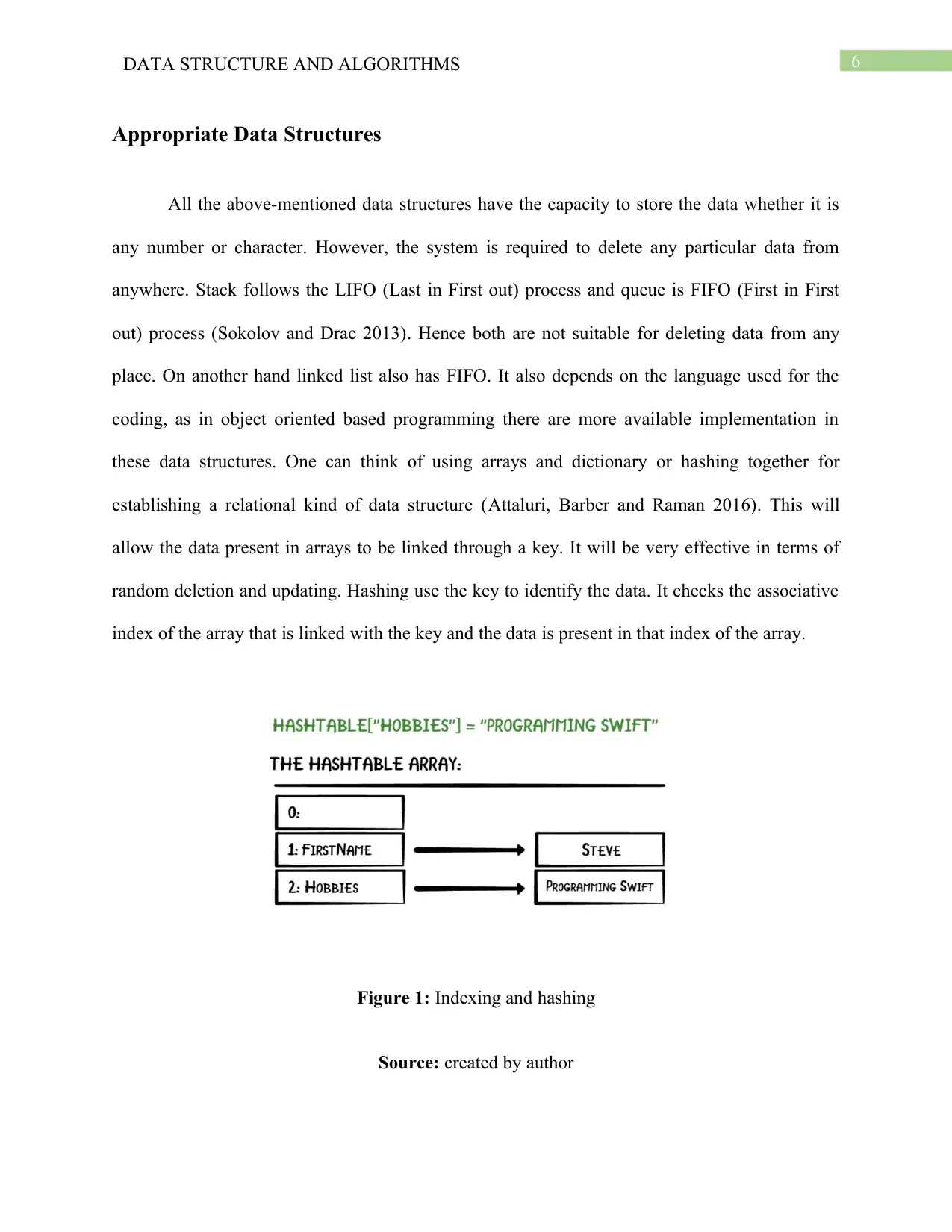
6DATA STRUCTURE AND ALGORITHMS
Appropriate Data Structures
All the above-mentioned data structures have the capacity to store the data whether it is
any number or character. However, the system is required to delete any particular data from
anywhere. Stack follows the LIFO (Last in First out) process and queue is FIFO (First in First
out) process (Sokolov and Drac 2013). Hence both are not suitable for deleting data from any
place. On another hand linked list also has FIFO. It also depends on the language used for the
coding, as in object oriented based programming there are more available implementation in
these data structures. One can think of using arrays and dictionary or hashing together for
establishing a relational kind of data structure (Attaluri, Barber and Raman 2016). This will
allow the data present in arrays to be linked through a key. It will be very effective in terms of
random deletion and updating. Hashing use the key to identify the data. It checks the associative
index of the array that is linked with the key and the data is present in that index of the array.
Figure 1: Indexing and hashing
Source: created by author
Appropriate Data Structures
All the above-mentioned data structures have the capacity to store the data whether it is
any number or character. However, the system is required to delete any particular data from
anywhere. Stack follows the LIFO (Last in First out) process and queue is FIFO (First in First
out) process (Sokolov and Drac 2013). Hence both are not suitable for deleting data from any
place. On another hand linked list also has FIFO. It also depends on the language used for the
coding, as in object oriented based programming there are more available implementation in
these data structures. One can think of using arrays and dictionary or hashing together for
establishing a relational kind of data structure (Attaluri, Barber and Raman 2016). This will
allow the data present in arrays to be linked through a key. It will be very effective in terms of
random deletion and updating. Hashing use the key to identify the data. It checks the associative
index of the array that is linked with the key and the data is present in that index of the array.
Figure 1: Indexing and hashing
Source: created by author
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
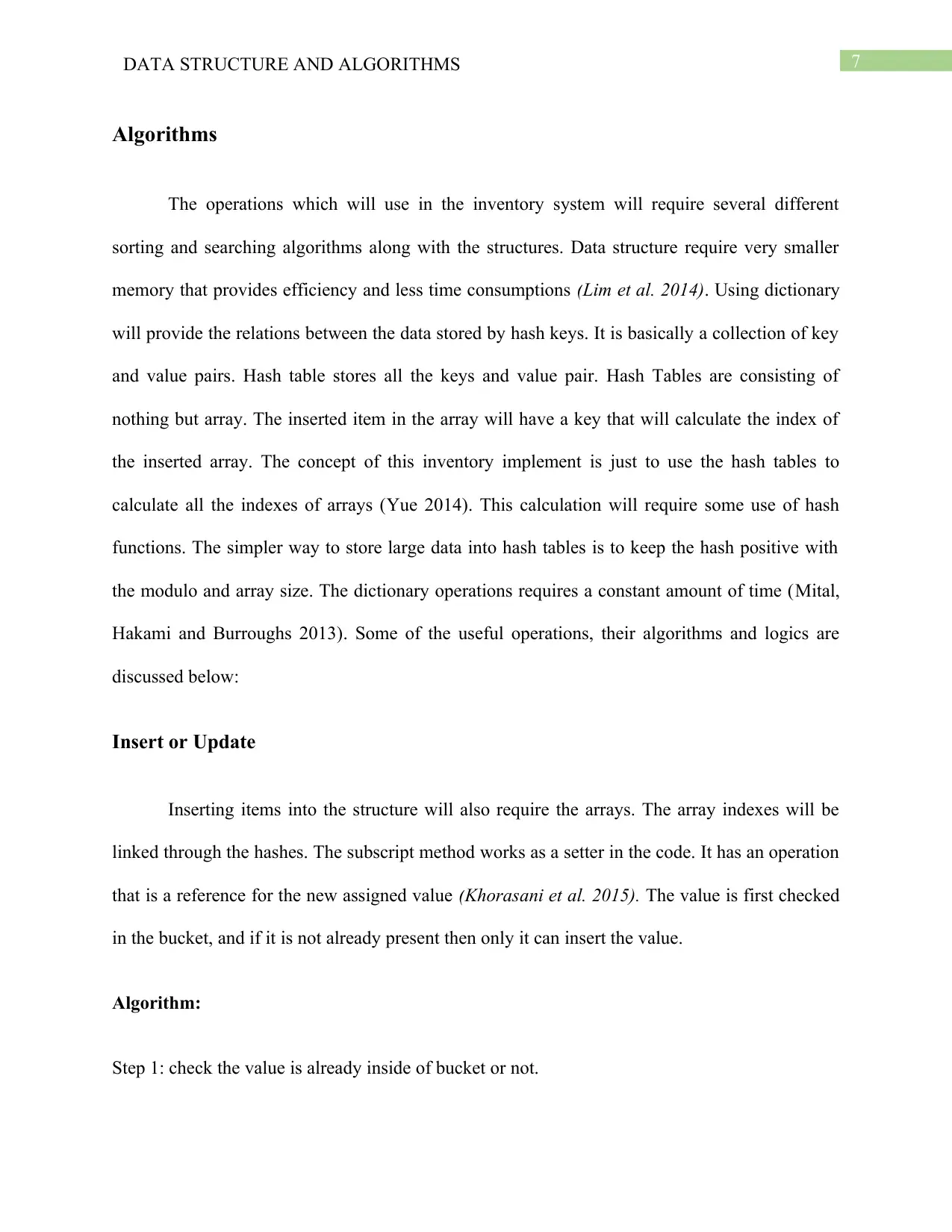
7DATA STRUCTURE AND ALGORITHMS
Algorithms
The operations which will use in the inventory system will require several different
sorting and searching algorithms along with the structures. Data structure require very smaller
memory that provides efficiency and less time consumptions (Lim et al. 2014). Using dictionary
will provide the relations between the data stored by hash keys. It is basically a collection of key
and value pairs. Hash table stores all the keys and value pair. Hash Tables are consisting of
nothing but array. The inserted item in the array will have a key that will calculate the index of
the inserted array. The concept of this inventory implement is just to use the hash tables to
calculate all the indexes of arrays (Yue 2014). This calculation will require some use of hash
functions. The simpler way to store large data into hash tables is to keep the hash positive with
the modulo and array size. The dictionary operations requires a constant amount of time (Mital,
Hakami and Burroughs 2013). Some of the useful operations, their algorithms and logics are
discussed below:
Insert or Update
Inserting items into the structure will also require the arrays. The array indexes will be
linked through the hashes. The subscript method works as a setter in the code. It has an operation
that is a reference for the new assigned value (Khorasani et al. 2015). The value is first checked
in the bucket, and if it is not already present then only it can insert the value.
Algorithm:
Step 1: check the value is already inside of bucket or not.
Algorithms
The operations which will use in the inventory system will require several different
sorting and searching algorithms along with the structures. Data structure require very smaller
memory that provides efficiency and less time consumptions (Lim et al. 2014). Using dictionary
will provide the relations between the data stored by hash keys. It is basically a collection of key
and value pairs. Hash table stores all the keys and value pair. Hash Tables are consisting of
nothing but array. The inserted item in the array will have a key that will calculate the index of
the inserted array. The concept of this inventory implement is just to use the hash tables to
calculate all the indexes of arrays (Yue 2014). This calculation will require some use of hash
functions. The simpler way to store large data into hash tables is to keep the hash positive with
the modulo and array size. The dictionary operations requires a constant amount of time (Mital,
Hakami and Burroughs 2013). Some of the useful operations, their algorithms and logics are
discussed below:
Insert or Update
Inserting items into the structure will also require the arrays. The array indexes will be
linked through the hashes. The subscript method works as a setter in the code. It has an operation
that is a reference for the new assigned value (Khorasani et al. 2015). The value is first checked
in the bucket, and if it is not already present then only it can insert the value.
Algorithm:
Step 1: check the value is already inside of bucket or not.
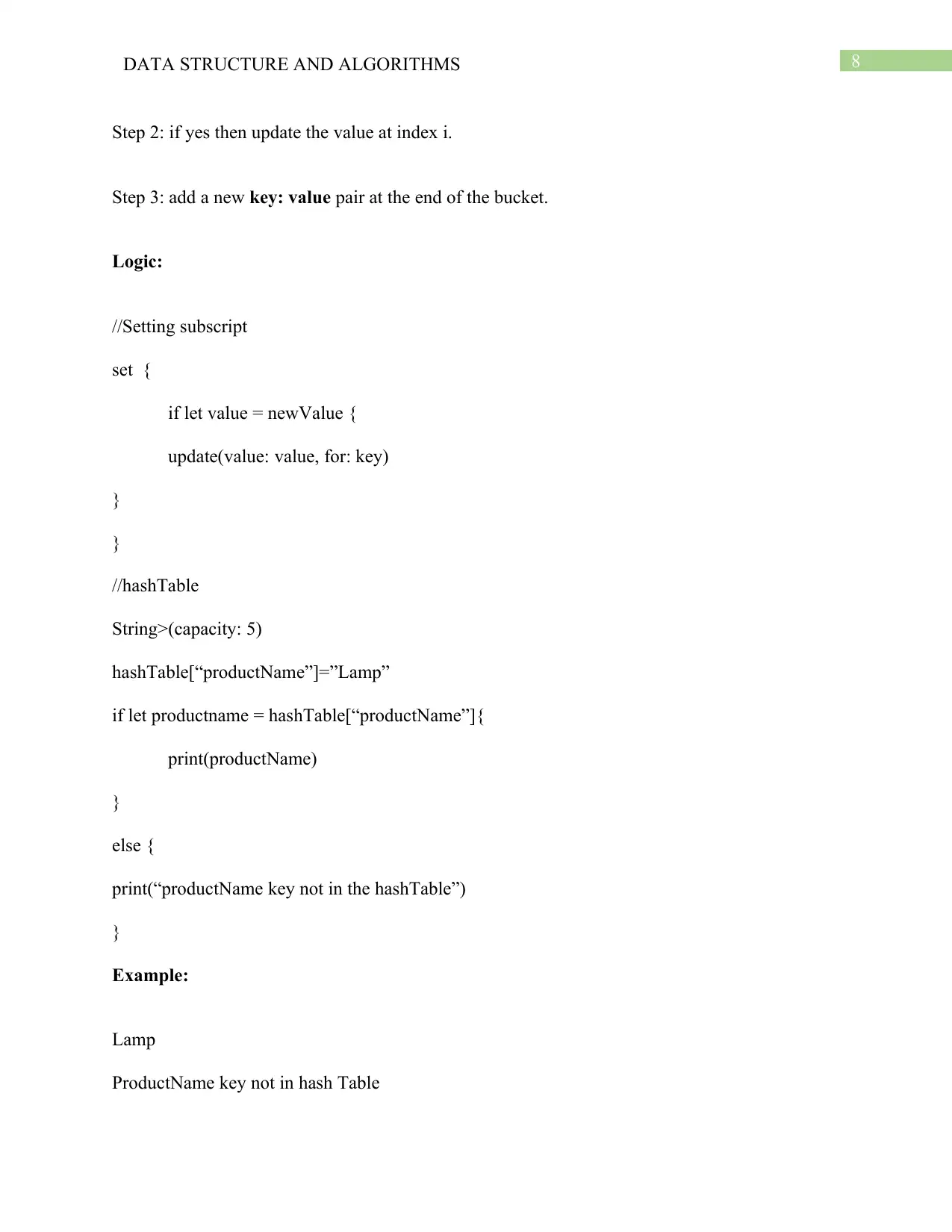
8DATA STRUCTURE AND ALGORITHMS
Step 2: if yes then update the value at index i.
Step 3: add a new key: value pair at the end of the bucket.
Logic:
//Setting subscript
set {
if let value = newValue {
update(value: value, for: key)
}
}
//hashTable
String>(capacity: 5)
hashTable[“productName”]=”Lamp”
if let productname = hashTable[“productName”]{
print(productName)
}
else {
print(“productName key not in the hashTable”)
}
Example:
Lamp
ProductName key not in hash Table
Step 2: if yes then update the value at index i.
Step 3: add a new key: value pair at the end of the bucket.
Logic:
//Setting subscript
set {
if let value = newValue {
update(value: value, for: key)
}
}
//hashTable
String>(capacity: 5)
hashTable[“productName”]=”Lamp”
if let productname = hashTable[“productName”]{
print(productName)
}
else {
print(“productName key not in the hashTable”)
}
Example:
Lamp
ProductName key not in hash Table
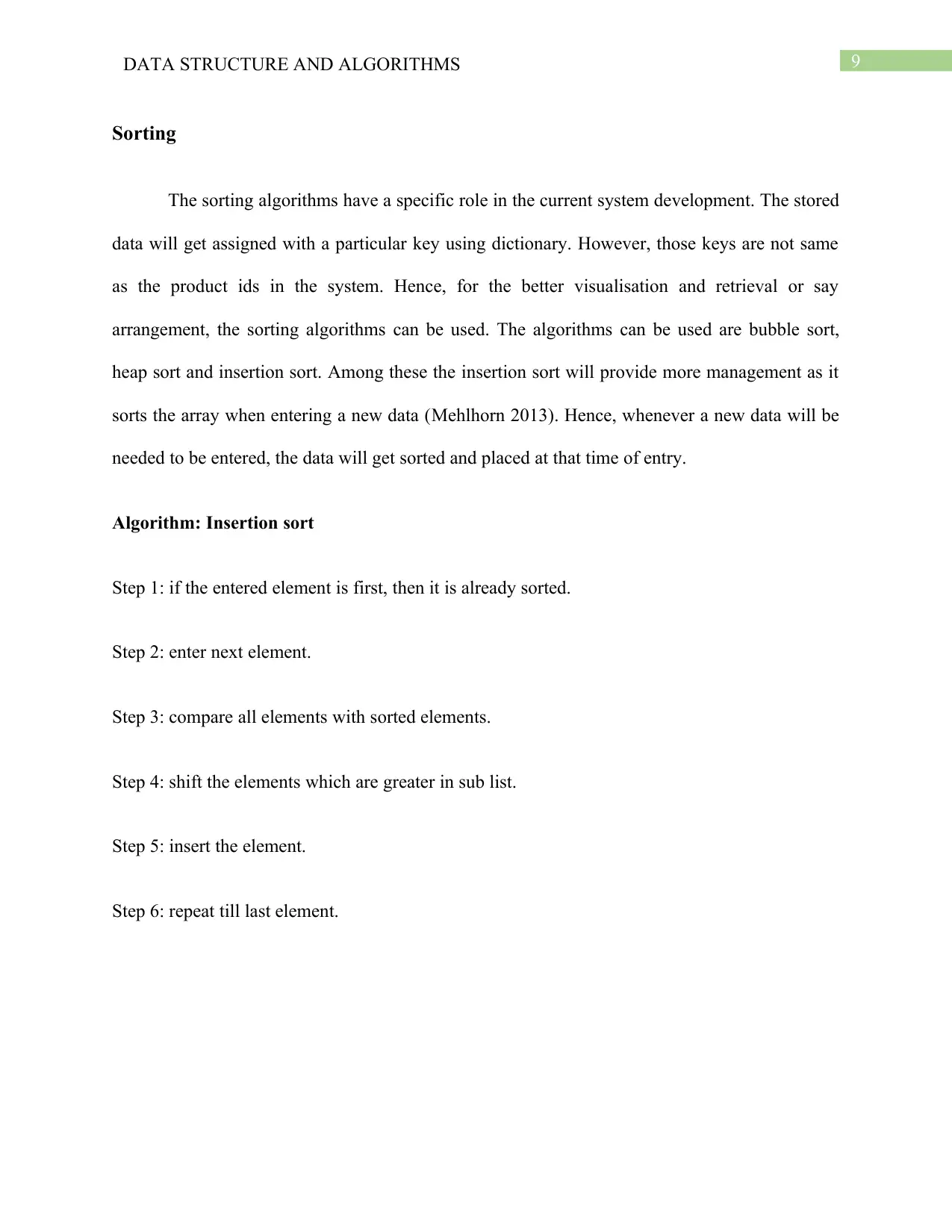
9DATA STRUCTURE AND ALGORITHMS
Sorting
The sorting algorithms have a specific role in the current system development. The stored
data will get assigned with a particular key using dictionary. However, those keys are not same
as the product ids in the system. Hence, for the better visualisation and retrieval or say
arrangement, the sorting algorithms can be used. The algorithms can be used are bubble sort,
heap sort and insertion sort. Among these the insertion sort will provide more management as it
sorts the array when entering a new data (Mehlhorn 2013). Hence, whenever a new data will be
needed to be entered, the data will get sorted and placed at that time of entry.
Algorithm: Insertion sort
Step 1: if the entered element is first, then it is already sorted.
Step 2: enter next element.
Step 3: compare all elements with sorted elements.
Step 4: shift the elements which are greater in sub list.
Step 5: insert the element.
Step 6: repeat till last element.
Sorting
The sorting algorithms have a specific role in the current system development. The stored
data will get assigned with a particular key using dictionary. However, those keys are not same
as the product ids in the system. Hence, for the better visualisation and retrieval or say
arrangement, the sorting algorithms can be used. The algorithms can be used are bubble sort,
heap sort and insertion sort. Among these the insertion sort will provide more management as it
sorts the array when entering a new data (Mehlhorn 2013). Hence, whenever a new data will be
needed to be entered, the data will get sorted and placed at that time of entry.
Algorithm: Insertion sort
Step 1: if the entered element is first, then it is already sorted.
Step 2: enter next element.
Step 3: compare all elements with sorted elements.
Step 4: shift the elements which are greater in sub list.
Step 5: insert the element.
Step 6: repeat till last element.
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
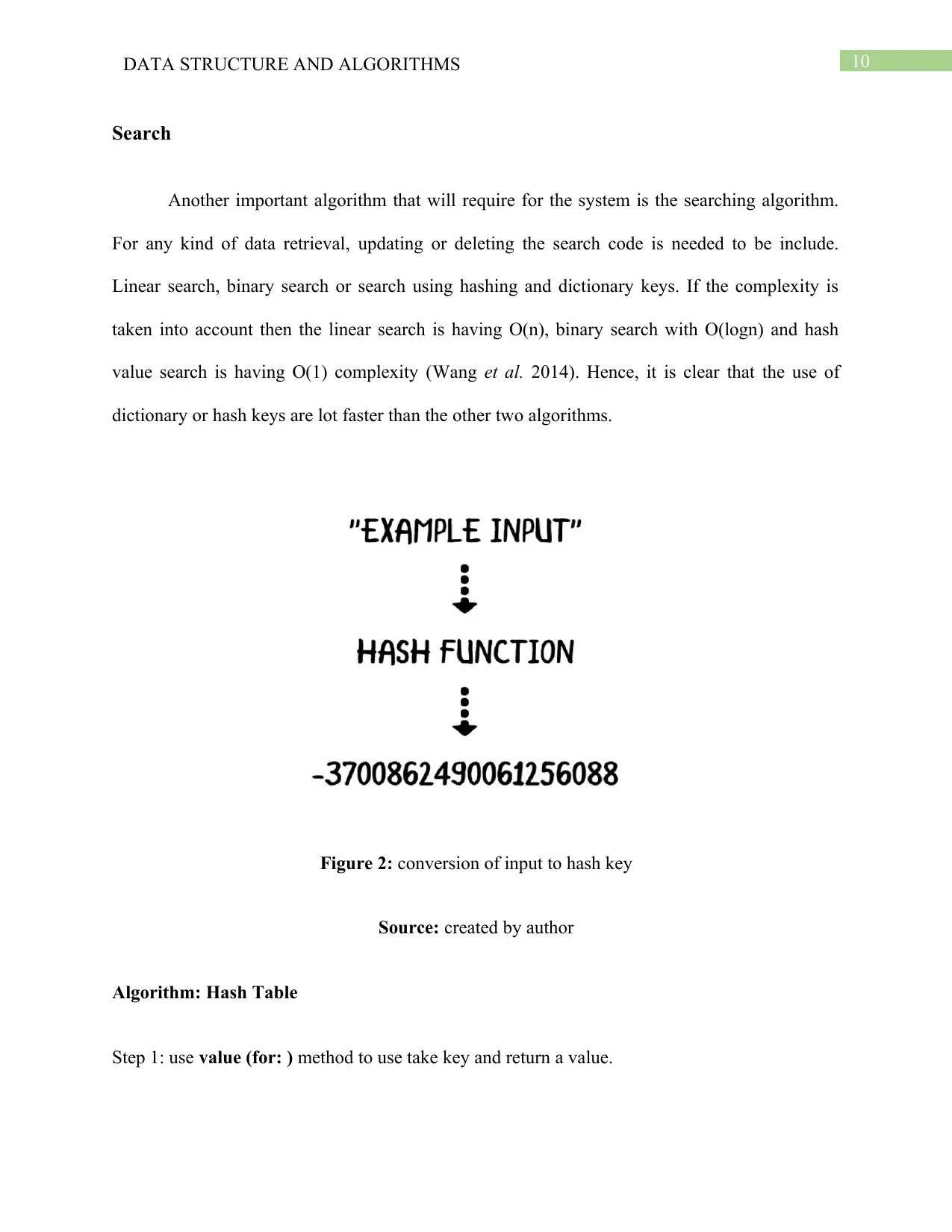
10DATA STRUCTURE AND ALGORITHMS
Search
Another important algorithm that will require for the system is the searching algorithm.
For any kind of data retrieval, updating or deleting the search code is needed to be include.
Linear search, binary search or search using hashing and dictionary keys. If the complexity is
taken into account then the linear search is having O(n), binary search with O(logn) and hash
value search is having O(1) complexity (Wang et al. 2014). Hence, it is clear that the use of
dictionary or hash keys are lot faster than the other two algorithms.
Figure 2: conversion of input to hash key
Source: created by author
Algorithm: Hash Table
Step 1: use value (for: ) method to use take key and return a value.
Search
Another important algorithm that will require for the system is the searching algorithm.
For any kind of data retrieval, updating or deleting the search code is needed to be include.
Linear search, binary search or search using hashing and dictionary keys. If the complexity is
taken into account then the linear search is having O(n), binary search with O(logn) and hash
value search is having O(1) complexity (Wang et al. 2014). Hence, it is clear that the use of
dictionary or hash keys are lot faster than the other two algorithms.
Figure 2: conversion of input to hash key
Source: created by author
Algorithm: Hash Table
Step 1: use value (for: ) method to use take key and return a value.
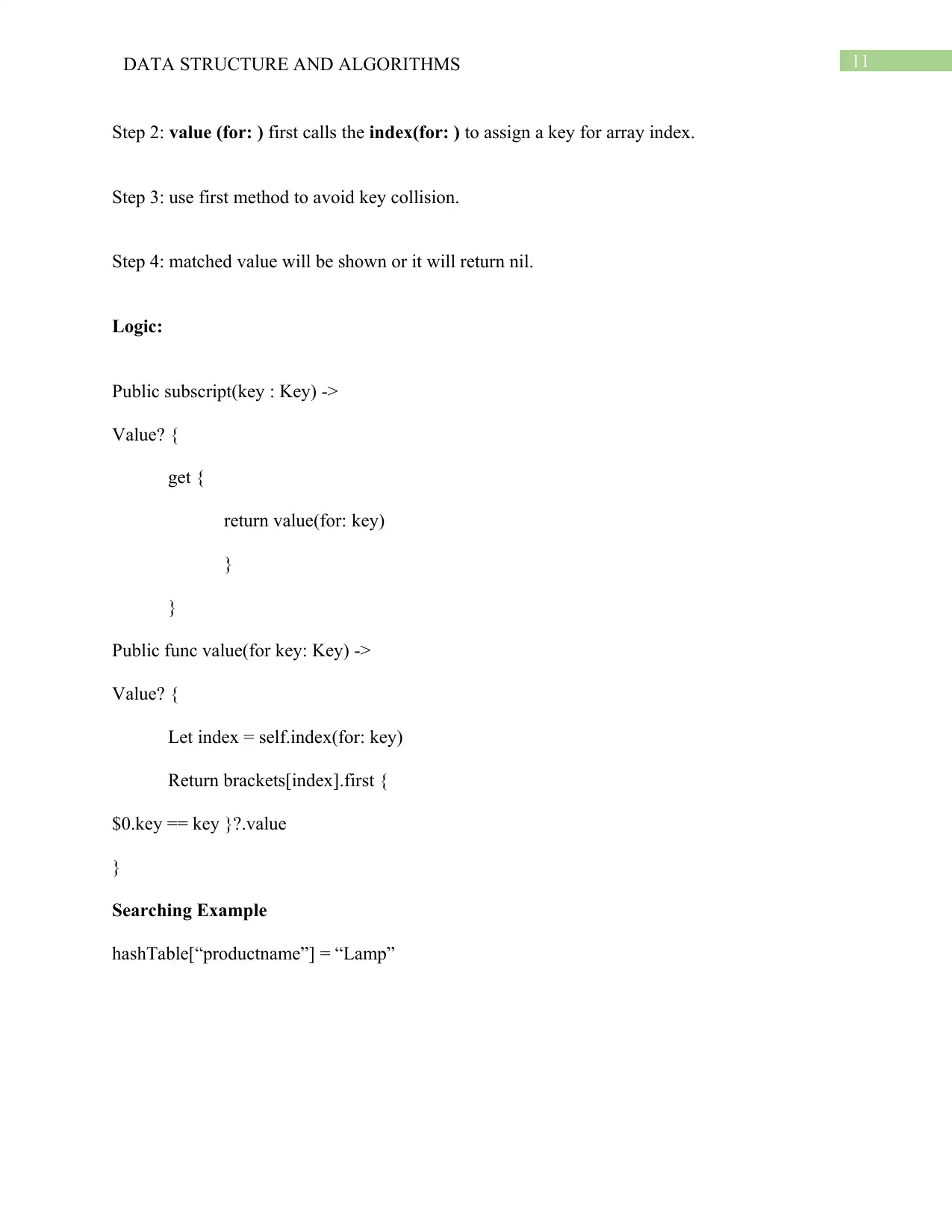
11DATA STRUCTURE AND ALGORITHMS
Step 2: value (for: ) first calls the index(for: ) to assign a key for array index.
Step 3: use first method to avoid key collision.
Step 4: matched value will be shown or it will return nil.
Logic:
Public subscript(key : Key) ->
Value? {
get {
return value(for: key)
}
}
Public func value(for key: Key) ->
Value? {
Let index = self.index(for: key)
Return brackets[index].first {
$0.key == key }?.value
}
Searching Example
hashTable[“productname”] = “Lamp”
Step 2: value (for: ) first calls the index(for: ) to assign a key for array index.
Step 3: use first method to avoid key collision.
Step 4: matched value will be shown or it will return nil.
Logic:
Public subscript(key : Key) ->
Value? {
get {
return value(for: key)
}
}
Public func value(for key: Key) ->
Value? {
Let index = self.index(for: key)
Return brackets[index].first {
$0.key == key }?.value
}
Searching Example
hashTable[“productname”] = “Lamp”
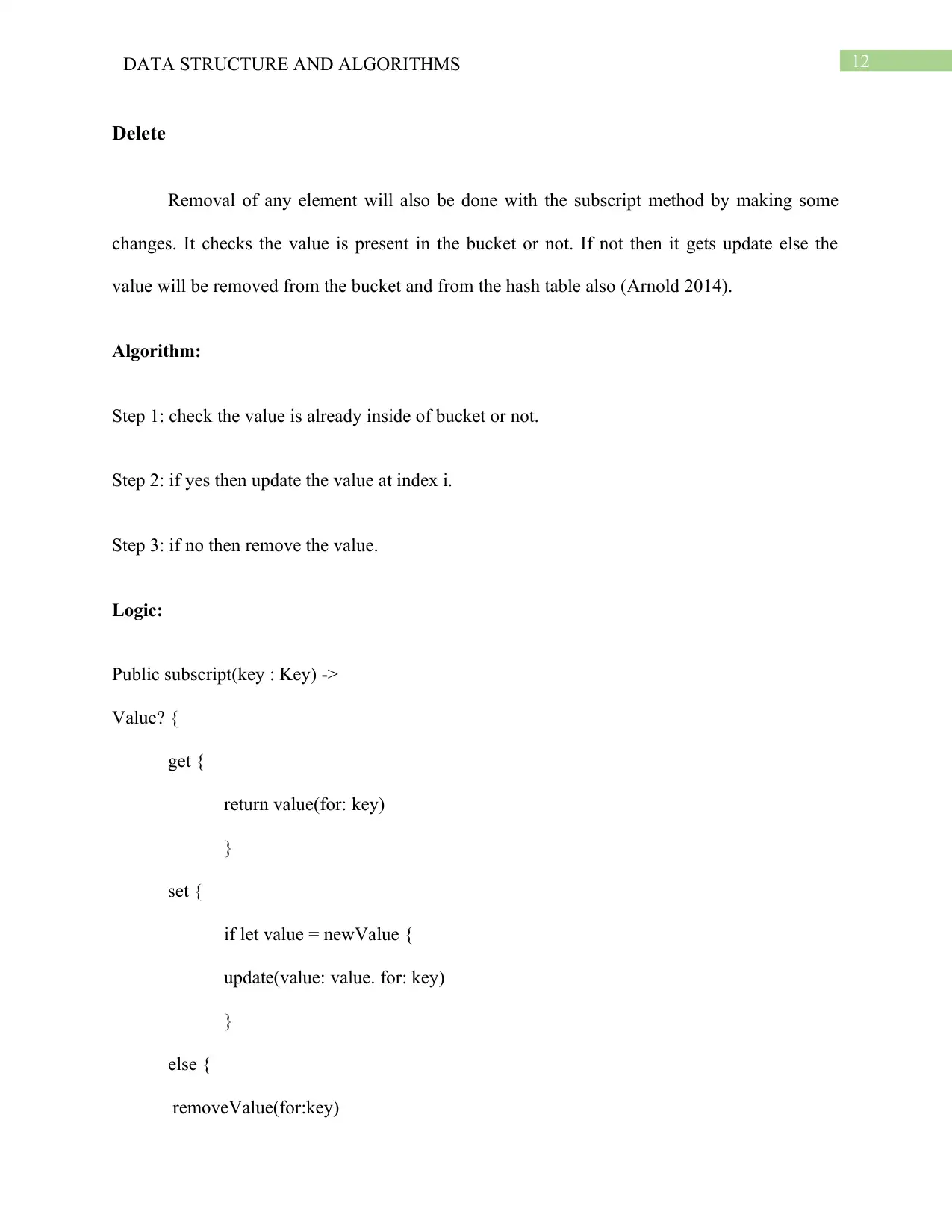
12DATA STRUCTURE AND ALGORITHMS
Delete
Removal of any element will also be done with the subscript method by making some
changes. It checks the value is present in the bucket or not. If not then it gets update else the
value will be removed from the bucket and from the hash table also (Arnold 2014).
Algorithm:
Step 1: check the value is already inside of bucket or not.
Step 2: if yes then update the value at index i.
Step 3: if no then remove the value.
Logic:
Public subscript(key : Key) ->
Value? {
get {
return value(for: key)
}
set {
if let value = newValue {
update(value: value. for: key)
}
else {
removeValue(for:key)
Delete
Removal of any element will also be done with the subscript method by making some
changes. It checks the value is present in the bucket or not. If not then it gets update else the
value will be removed from the bucket and from the hash table also (Arnold 2014).
Algorithm:
Step 1: check the value is already inside of bucket or not.
Step 2: if yes then update the value at index i.
Step 3: if no then remove the value.
Logic:
Public subscript(key : Key) ->
Value? {
get {
return value(for: key)
}
set {
if let value = newValue {
update(value: value. for: key)
}
else {
removeValue(for:key)
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
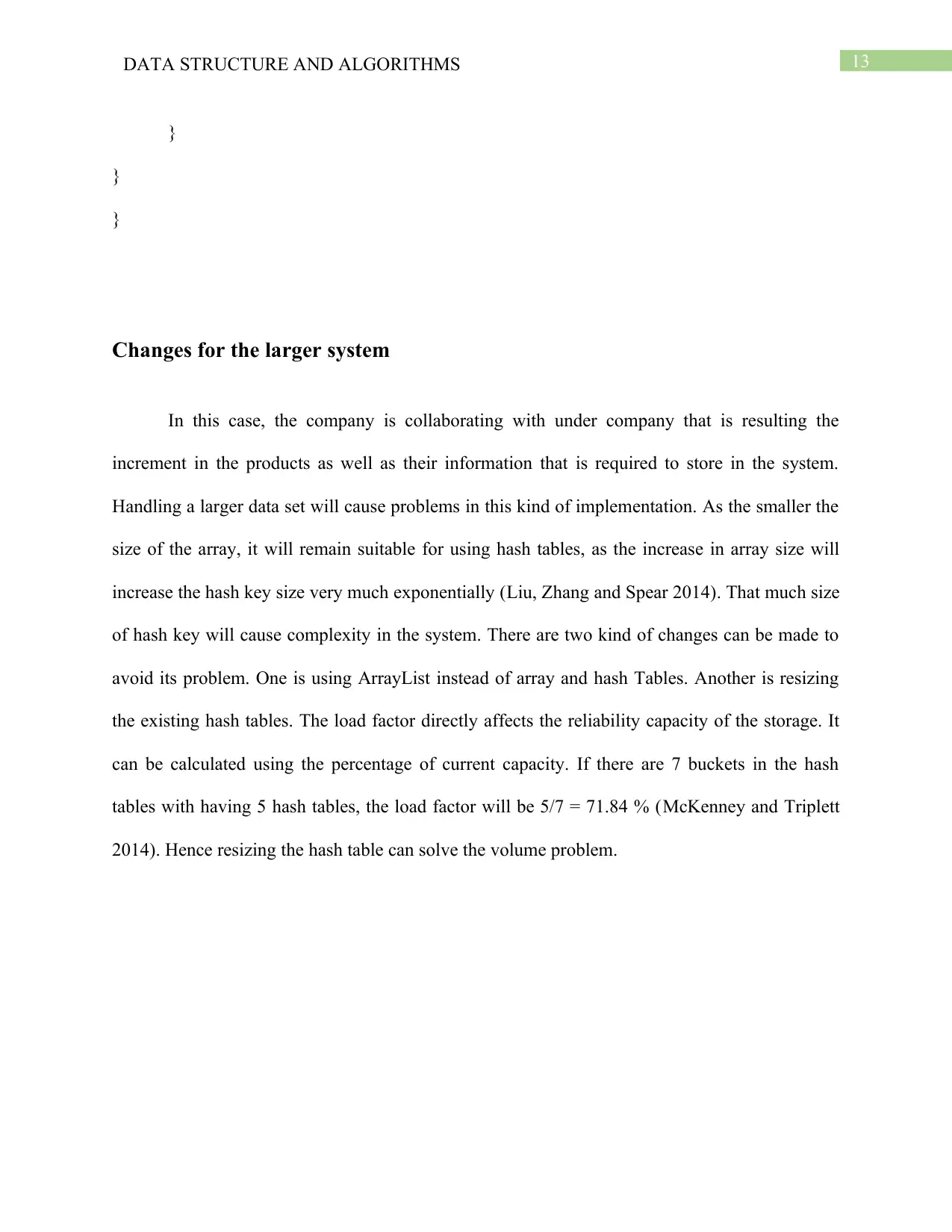
13DATA STRUCTURE AND ALGORITHMS
}
}
}
Changes for the larger system
In this case, the company is collaborating with under company that is resulting the
increment in the products as well as their information that is required to store in the system.
Handling a larger data set will cause problems in this kind of implementation. As the smaller the
size of the array, it will remain suitable for using hash tables, as the increase in array size will
increase the hash key size very much exponentially (Liu, Zhang and Spear 2014). That much size
of hash key will cause complexity in the system. There are two kind of changes can be made to
avoid its problem. One is using ArrayList instead of array and hash Tables. Another is resizing
the existing hash tables. The load factor directly affects the reliability capacity of the storage. It
can be calculated using the percentage of current capacity. If there are 7 buckets in the hash
tables with having 5 hash tables, the load factor will be 5/7 = 71.84 % (McKenney and Triplett
2014). Hence resizing the hash table can solve the volume problem.
}
}
}
Changes for the larger system
In this case, the company is collaborating with under company that is resulting the
increment in the products as well as their information that is required to store in the system.
Handling a larger data set will cause problems in this kind of implementation. As the smaller the
size of the array, it will remain suitable for using hash tables, as the increase in array size will
increase the hash key size very much exponentially (Liu, Zhang and Spear 2014). That much size
of hash key will cause complexity in the system. There are two kind of changes can be made to
avoid its problem. One is using ArrayList instead of array and hash Tables. Another is resizing
the existing hash tables. The load factor directly affects the reliability capacity of the storage. It
can be calculated using the percentage of current capacity. If there are 7 buckets in the hash
tables with having 5 hash tables, the load factor will be 5/7 = 71.84 % (McKenney and Triplett
2014). Hence resizing the hash table can solve the volume problem.
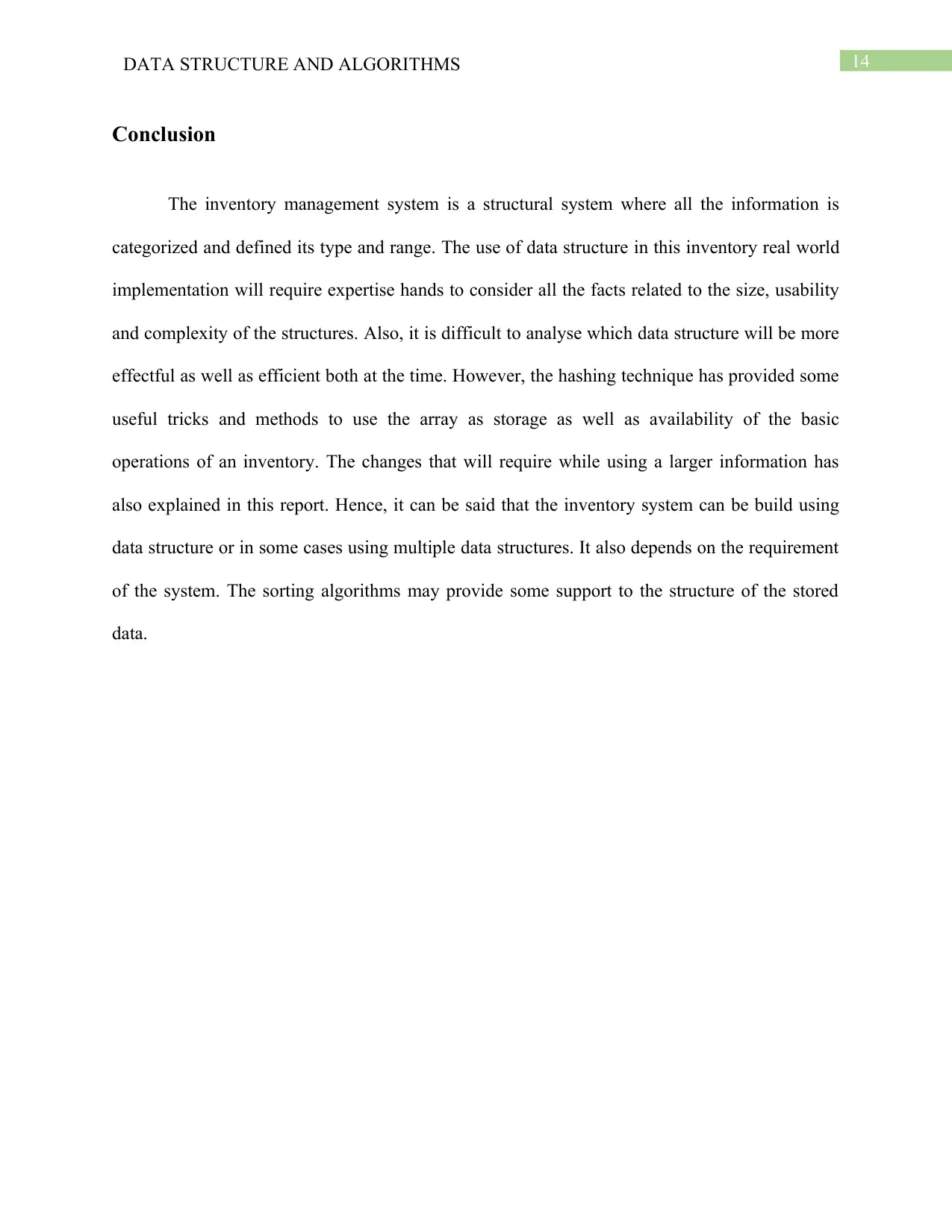
14DATA STRUCTURE AND ALGORITHMS
Conclusion
The inventory management system is a structural system where all the information is
categorized and defined its type and range. The use of data structure in this inventory real world
implementation will require expertise hands to consider all the facts related to the size, usability
and complexity of the structures. Also, it is difficult to analyse which data structure will be more
effectful as well as efficient both at the time. However, the hashing technique has provided some
useful tricks and methods to use the array as storage as well as availability of the basic
operations of an inventory. The changes that will require while using a larger information has
also explained in this report. Hence, it can be said that the inventory system can be build using
data structure or in some cases using multiple data structures. It also depends on the requirement
of the system. The sorting algorithms may provide some support to the structure of the stored
data.
Conclusion
The inventory management system is a structural system where all the information is
categorized and defined its type and range. The use of data structure in this inventory real world
implementation will require expertise hands to consider all the facts related to the size, usability
and complexity of the structures. Also, it is difficult to analyse which data structure will be more
effectful as well as efficient both at the time. However, the hashing technique has provided some
useful tricks and methods to use the array as storage as well as availability of the basic
operations of an inventory. The changes that will require while using a larger information has
also explained in this report. Hence, it can be said that the inventory system can be build using
data structure or in some cases using multiple data structures. It also depends on the requirement
of the system. The sorting algorithms may provide some support to the structure of the stored
data.
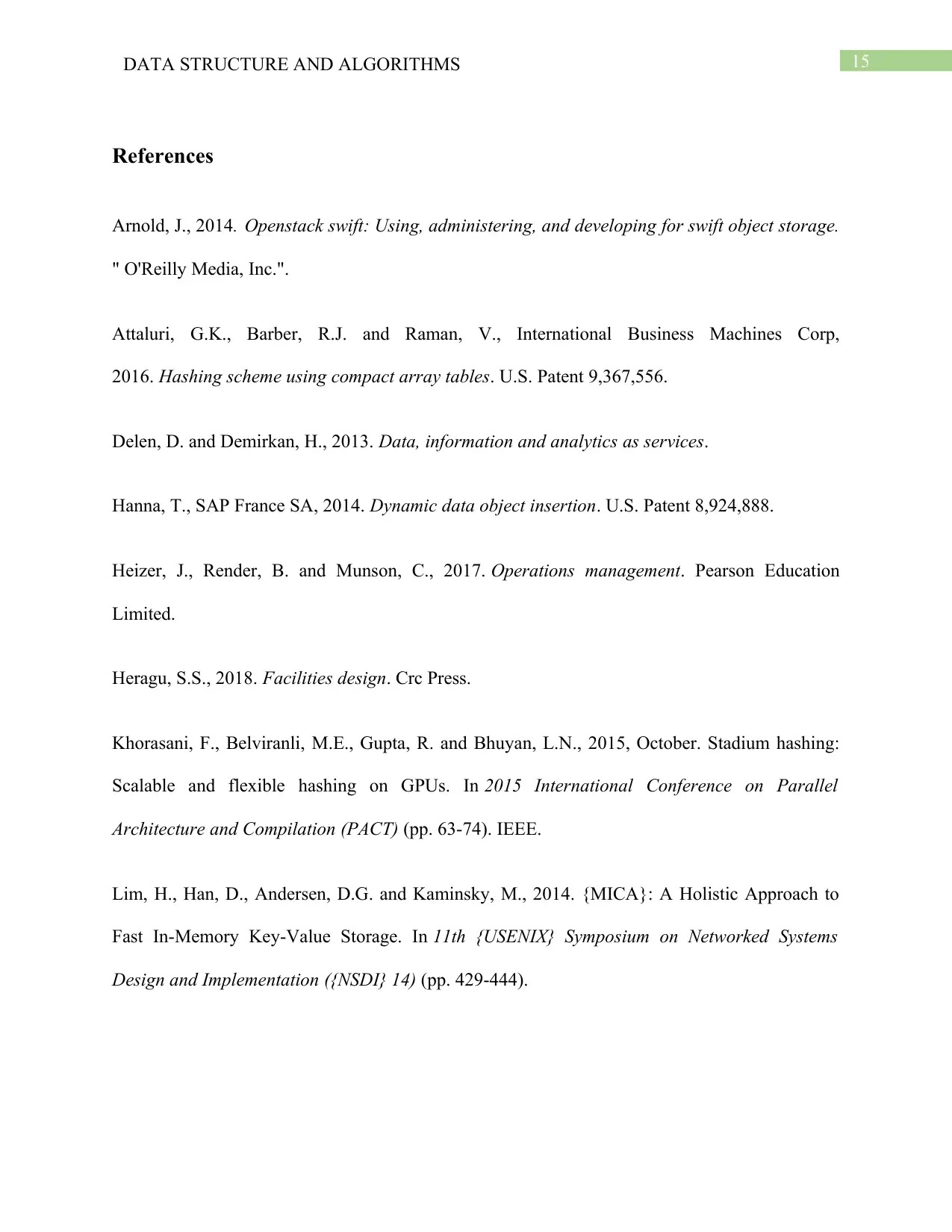
15DATA STRUCTURE AND ALGORITHMS
References
Arnold, J., 2014. Openstack swift: Using, administering, and developing for swift object storage.
" O'Reilly Media, Inc.".
Attaluri, G.K., Barber, R.J. and Raman, V., International Business Machines Corp,
2016. Hashing scheme using compact array tables. U.S. Patent 9,367,556.
Delen, D. and Demirkan, H., 2013. Data, information and analytics as services.
Hanna, T., SAP France SA, 2014. Dynamic data object insertion. U.S. Patent 8,924,888.
Heizer, J., Render, B. and Munson, C., 2017. Operations management. Pearson Education
Limited.
Heragu, S.S., 2018. Facilities design. Crc Press.
Khorasani, F., Belviranli, M.E., Gupta, R. and Bhuyan, L.N., 2015, October. Stadium hashing:
Scalable and flexible hashing on GPUs. In 2015 International Conference on Parallel
Architecture and Compilation (PACT) (pp. 63-74). IEEE.
Lim, H., Han, D., Andersen, D.G. and Kaminsky, M., 2014. {MICA}: A Holistic Approach to
Fast In-Memory Key-Value Storage. In 11th {USENIX} Symposium on Networked Systems
Design and Implementation ({NSDI} 14) (pp. 429-444).
References
Arnold, J., 2014. Openstack swift: Using, administering, and developing for swift object storage.
" O'Reilly Media, Inc.".
Attaluri, G.K., Barber, R.J. and Raman, V., International Business Machines Corp,
2016. Hashing scheme using compact array tables. U.S. Patent 9,367,556.
Delen, D. and Demirkan, H., 2013. Data, information and analytics as services.
Hanna, T., SAP France SA, 2014. Dynamic data object insertion. U.S. Patent 8,924,888.
Heizer, J., Render, B. and Munson, C., 2017. Operations management. Pearson Education
Limited.
Heragu, S.S., 2018. Facilities design. Crc Press.
Khorasani, F., Belviranli, M.E., Gupta, R. and Bhuyan, L.N., 2015, October. Stadium hashing:
Scalable and flexible hashing on GPUs. In 2015 International Conference on Parallel
Architecture and Compilation (PACT) (pp. 63-74). IEEE.
Lim, H., Han, D., Andersen, D.G. and Kaminsky, M., 2014. {MICA}: A Holistic Approach to
Fast In-Memory Key-Value Storage. In 11th {USENIX} Symposium on Networked Systems
Design and Implementation ({NSDI} 14) (pp. 429-444).
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
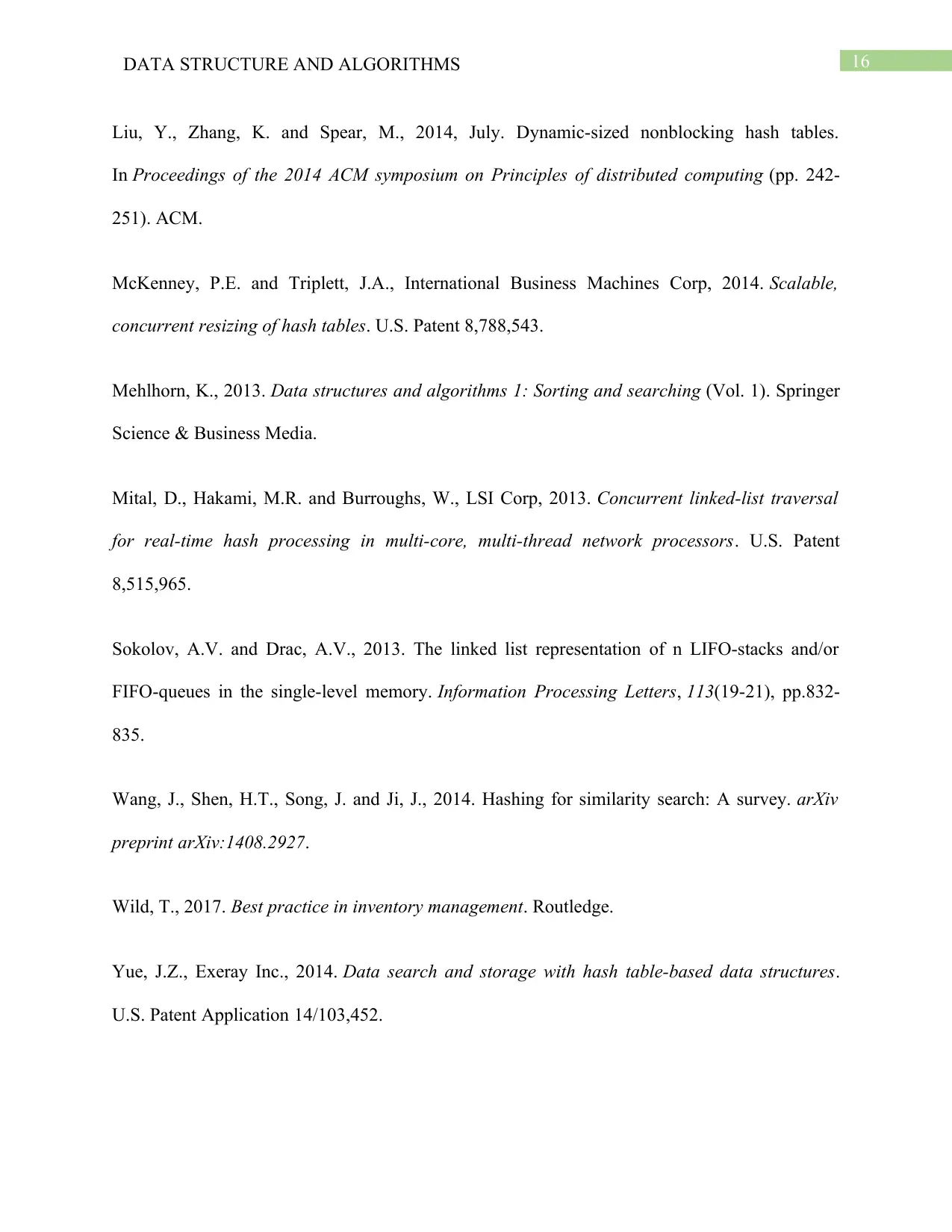
16DATA STRUCTURE AND ALGORITHMS
Liu, Y., Zhang, K. and Spear, M., 2014, July. Dynamic-sized nonblocking hash tables.
In Proceedings of the 2014 ACM symposium on Principles of distributed computing (pp. 242-
251). ACM.
McKenney, P.E. and Triplett, J.A., International Business Machines Corp, 2014. Scalable,
concurrent resizing of hash tables. U.S. Patent 8,788,543.
Mehlhorn, K., 2013. Data structures and algorithms 1: Sorting and searching (Vol. 1). Springer
Science & Business Media.
Mital, D., Hakami, M.R. and Burroughs, W., LSI Corp, 2013. Concurrent linked-list traversal
for real-time hash processing in multi-core, multi-thread network processors. U.S. Patent
8,515,965.
Sokolov, A.V. and Drac, A.V., 2013. The linked list representation of n LIFO-stacks and/or
FIFO-queues in the single-level memory. Information Processing Letters, 113(19-21), pp.832-
835.
Wang, J., Shen, H.T., Song, J. and Ji, J., 2014. Hashing for similarity search: A survey. arXiv
preprint arXiv:1408.2927.
Wild, T., 2017. Best practice in inventory management. Routledge.
Yue, J.Z., Exeray Inc., 2014. Data search and storage with hash table-based data structures.
U.S. Patent Application 14/103,452.
Liu, Y., Zhang, K. and Spear, M., 2014, July. Dynamic-sized nonblocking hash tables.
In Proceedings of the 2014 ACM symposium on Principles of distributed computing (pp. 242-
251). ACM.
McKenney, P.E. and Triplett, J.A., International Business Machines Corp, 2014. Scalable,
concurrent resizing of hash tables. U.S. Patent 8,788,543.
Mehlhorn, K., 2013. Data structures and algorithms 1: Sorting and searching (Vol. 1). Springer
Science & Business Media.
Mital, D., Hakami, M.R. and Burroughs, W., LSI Corp, 2013. Concurrent linked-list traversal
for real-time hash processing in multi-core, multi-thread network processors. U.S. Patent
8,515,965.
Sokolov, A.V. and Drac, A.V., 2013. The linked list representation of n LIFO-stacks and/or
FIFO-queues in the single-level memory. Information Processing Letters, 113(19-21), pp.832-
835.
Wang, J., Shen, H.T., Song, J. and Ji, J., 2014. Hashing for similarity search: A survey. arXiv
preprint arXiv:1408.2927.
Wild, T., 2017. Best practice in inventory management. Routledge.
Yue, J.Z., Exeray Inc., 2014. Data search and storage with hash table-based data structures.
U.S. Patent Application 14/103,452.
1 out of 17
Related Documents
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.