Solved Assignments and Coding Problems
VerifiedAdded on  2022/12/20
|7
|1222
|1
AI Summary
Desklib offers a collection of solved assignments and coding problems. Find help with coding assignments and practice coding problems.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
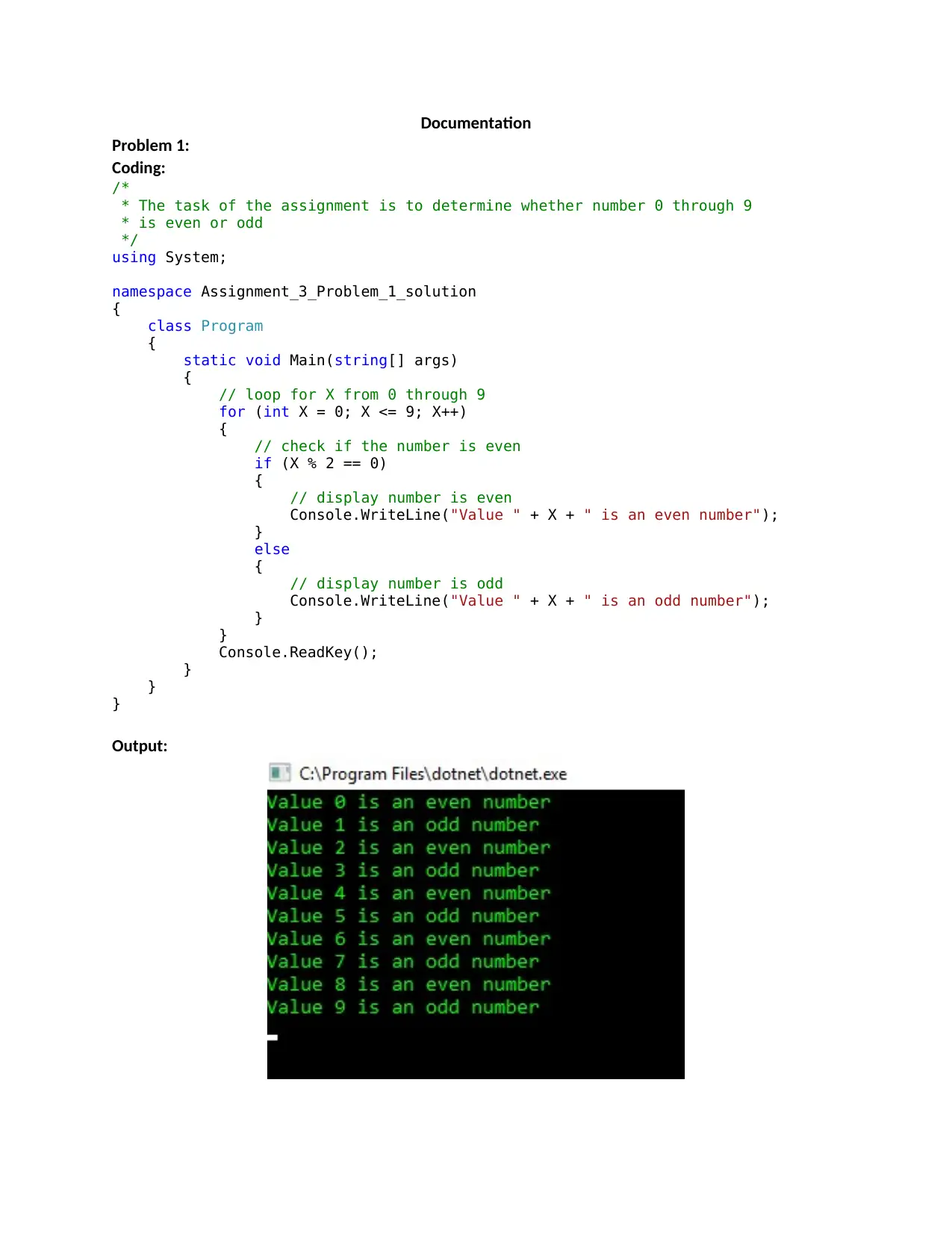
Documentation
Problem 1:
Coding:
/*
* The task of the assignment is to determine whether number 0 through 9
* is even or odd
*/
using System;
namespace Assignment_3_Problem_1_solution
{
class Program
{
static void Main(string[] args)
{
// loop for X from 0 through 9
for (int X = 0; X <= 9; X++)
{
// check if the number is even
if (X % 2 == 0)
{
// display number is even
Console.WriteLine("Value " + X + " is an even number");
}
else
{
// display number is odd
Console.WriteLine("Value " + X + " is an odd number");
}
}
Console.ReadKey();
}
}
}
Output:
Problem 1:
Coding:
/*
* The task of the assignment is to determine whether number 0 through 9
* is even or odd
*/
using System;
namespace Assignment_3_Problem_1_solution
{
class Program
{
static void Main(string[] args)
{
// loop for X from 0 through 9
for (int X = 0; X <= 9; X++)
{
// check if the number is even
if (X % 2 == 0)
{
// display number is even
Console.WriteLine("Value " + X + " is an even number");
}
else
{
// display number is odd
Console.WriteLine("Value " + X + " is an odd number");
}
}
Console.ReadKey();
}
}
}
Output:
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
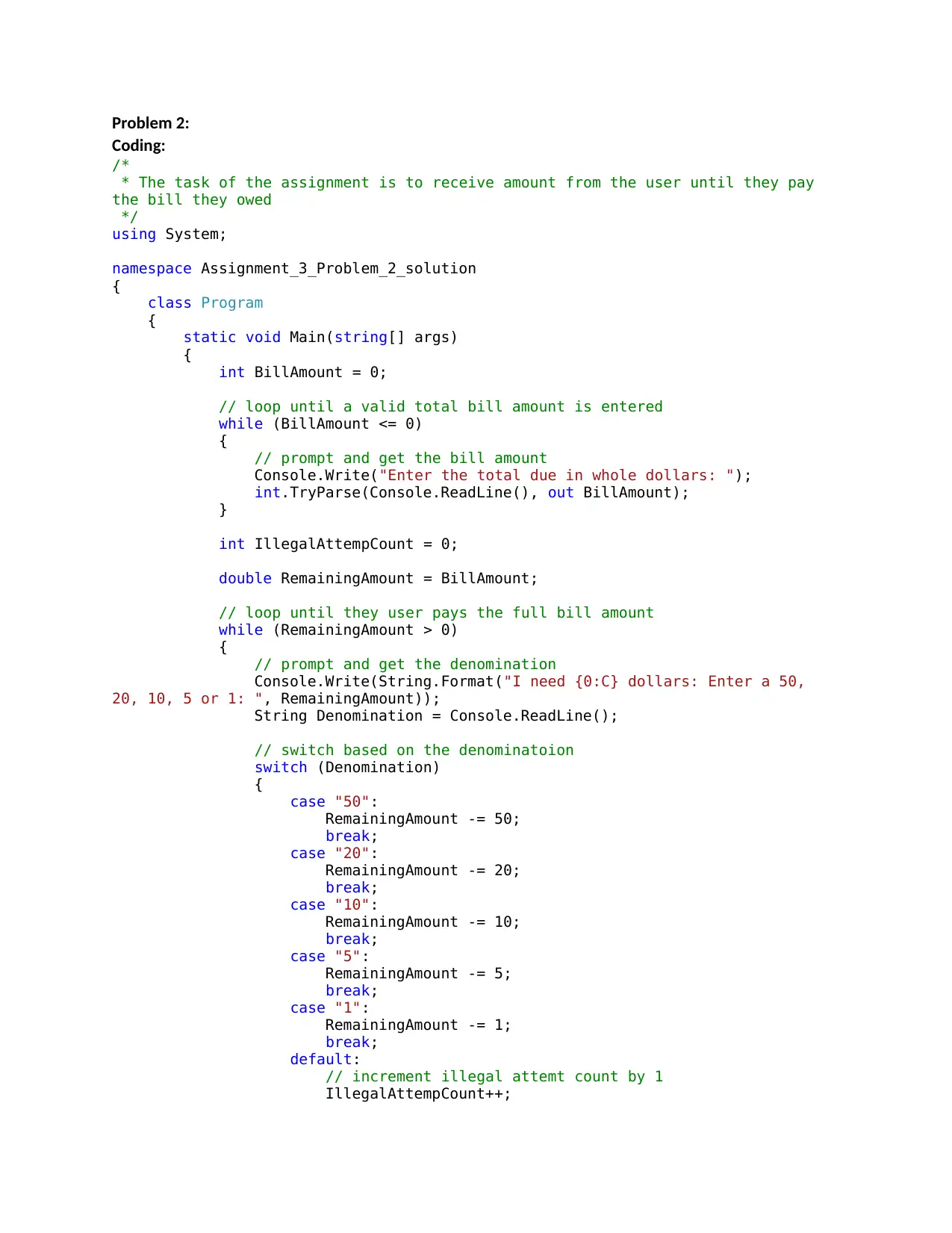
Problem 2:
Coding:
/*
* The task of the assignment is to receive amount from the user until they pay
the bill they owed
*/
using System;
namespace Assignment_3_Problem_2_solution
{
class Program
{
static void Main(string[] args)
{
int BillAmount = 0;
// loop until a valid total bill amount is entered
while (BillAmount <= 0)
{
// prompt and get the bill amount
Console.Write("Enter the total due in whole dollars: ");
int.TryParse(Console.ReadLine(), out BillAmount);
}
int IllegalAttempCount = 0;
double RemainingAmount = BillAmount;
// loop until they user pays the full bill amount
while (RemainingAmount > 0)
{
// prompt and get the denomination
Console.Write(String.Format("I need {0:C} dollars: Enter a 50,
20, 10, 5 or 1: ", RemainingAmount));
String Denomination = Console.ReadLine();
// switch based on the denominatoion
switch (Denomination)
{
case "50":
RemainingAmount -= 50;
break;
case "20":
RemainingAmount -= 20;
break;
case "10":
RemainingAmount -= 10;
break;
case "5":
RemainingAmount -= 5;
break;
case "1":
RemainingAmount -= 1;
break;
default:
// increment illegal attemt count by 1
IllegalAttempCount++;
Coding:
/*
* The task of the assignment is to receive amount from the user until they pay
the bill they owed
*/
using System;
namespace Assignment_3_Problem_2_solution
{
class Program
{
static void Main(string[] args)
{
int BillAmount = 0;
// loop until a valid total bill amount is entered
while (BillAmount <= 0)
{
// prompt and get the bill amount
Console.Write("Enter the total due in whole dollars: ");
int.TryParse(Console.ReadLine(), out BillAmount);
}
int IllegalAttempCount = 0;
double RemainingAmount = BillAmount;
// loop until they user pays the full bill amount
while (RemainingAmount > 0)
{
// prompt and get the denomination
Console.Write(String.Format("I need {0:C} dollars: Enter a 50,
20, 10, 5 or 1: ", RemainingAmount));
String Denomination = Console.ReadLine();
// switch based on the denominatoion
switch (Denomination)
{
case "50":
RemainingAmount -= 50;
break;
case "20":
RemainingAmount -= 20;
break;
case "10":
RemainingAmount -= 10;
break;
case "5":
RemainingAmount -= 5;
break;
case "1":
RemainingAmount -= 1;
break;
default:
// increment illegal attemt count by 1
IllegalAttempCount++;
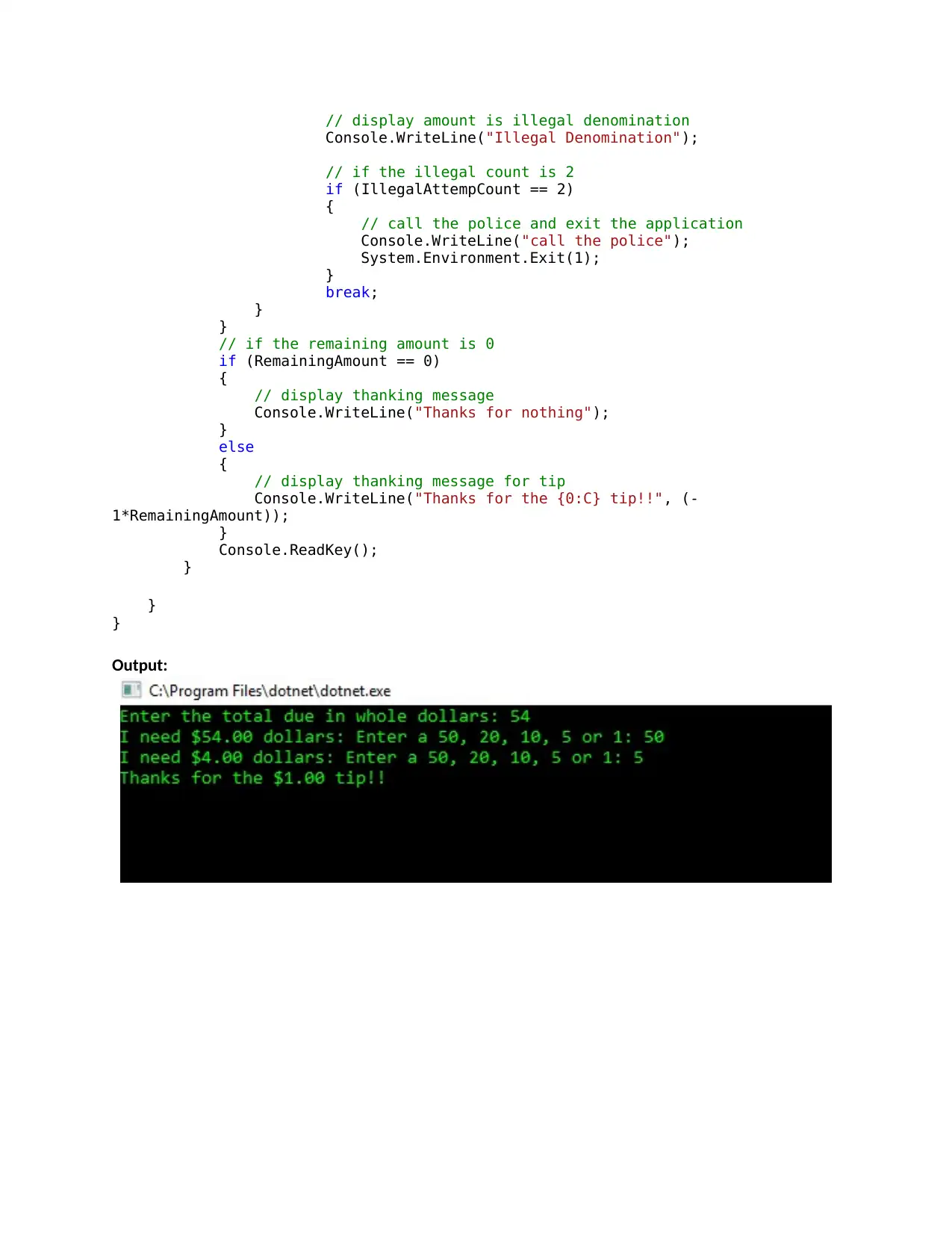
// display amount is illegal denomination
Console.WriteLine("Illegal Denomination");
// if the illegal count is 2
if (IllegalAttempCount == 2)
{
// call the police and exit the application
Console.WriteLine("call the police");
System.Environment.Exit(1);
}
break;
}
}
// if the remaining amount is 0
if (RemainingAmount == 0)
{
// display thanking message
Console.WriteLine("Thanks for nothing");
}
else
{
// display thanking message for tip
Console.WriteLine("Thanks for the {0:C} tip!!", (-
1*RemainingAmount));
}
Console.ReadKey();
}
}
}
Output:
Console.WriteLine("Illegal Denomination");
// if the illegal count is 2
if (IllegalAttempCount == 2)
{
// call the police and exit the application
Console.WriteLine("call the police");
System.Environment.Exit(1);
}
break;
}
}
// if the remaining amount is 0
if (RemainingAmount == 0)
{
// display thanking message
Console.WriteLine("Thanks for nothing");
}
else
{
// display thanking message for tip
Console.WriteLine("Thanks for the {0:C} tip!!", (-
1*RemainingAmount));
}
Console.ReadKey();
}
}
}
Output:
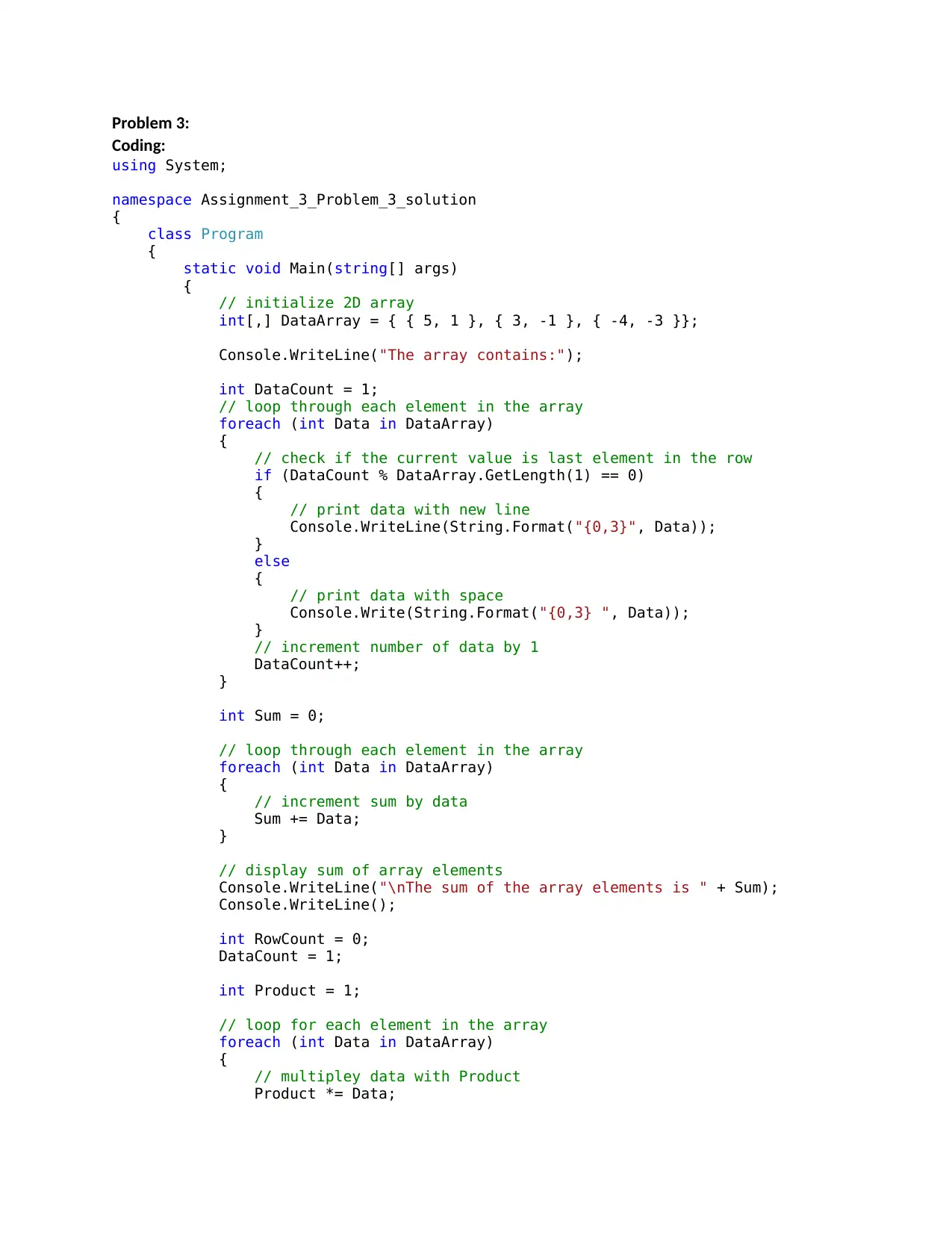
Problem 3:
Coding:
using System;
namespace Assignment_3_Problem_3_solution
{
class Program
{
static void Main(string[] args)
{
// initialize 2D array
int[,] DataArray = { { 5, 1 }, { 3, -1 }, { -4, -3 }};
Console.WriteLine("The array contains:");
int DataCount = 1;
// loop through each element in the array
foreach (int Data in DataArray)
{
// check if the current value is last element in the row
if (DataCount % DataArray.GetLength(1) == 0)
{
// print data with new line
Console.WriteLine(String.Format("{0,3}", Data));
}
else
{
// print data with space
Console.Write(String.Format("{0,3} ", Data));
}
// increment number of data by 1
DataCount++;
}
int Sum = 0;
// loop through each element in the array
foreach (int Data in DataArray)
{
// increment sum by data
Sum += Data;
}
// display sum of array elements
Console.WriteLine("\nThe sum of the array elements is " + Sum);
Console.WriteLine();
int RowCount = 0;
DataCount = 1;
int Product = 1;
// loop for each element in the array
foreach (int Data in DataArray)
{
// multipley data with Product
Product *= Data;
Coding:
using System;
namespace Assignment_3_Problem_3_solution
{
class Program
{
static void Main(string[] args)
{
// initialize 2D array
int[,] DataArray = { { 5, 1 }, { 3, -1 }, { -4, -3 }};
Console.WriteLine("The array contains:");
int DataCount = 1;
// loop through each element in the array
foreach (int Data in DataArray)
{
// check if the current value is last element in the row
if (DataCount % DataArray.GetLength(1) == 0)
{
// print data with new line
Console.WriteLine(String.Format("{0,3}", Data));
}
else
{
// print data with space
Console.Write(String.Format("{0,3} ", Data));
}
// increment number of data by 1
DataCount++;
}
int Sum = 0;
// loop through each element in the array
foreach (int Data in DataArray)
{
// increment sum by data
Sum += Data;
}
// display sum of array elements
Console.WriteLine("\nThe sum of the array elements is " + Sum);
Console.WriteLine();
int RowCount = 0;
DataCount = 1;
int Product = 1;
// loop for each element in the array
foreach (int Data in DataArray)
{
// multipley data with Product
Product *= Data;
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
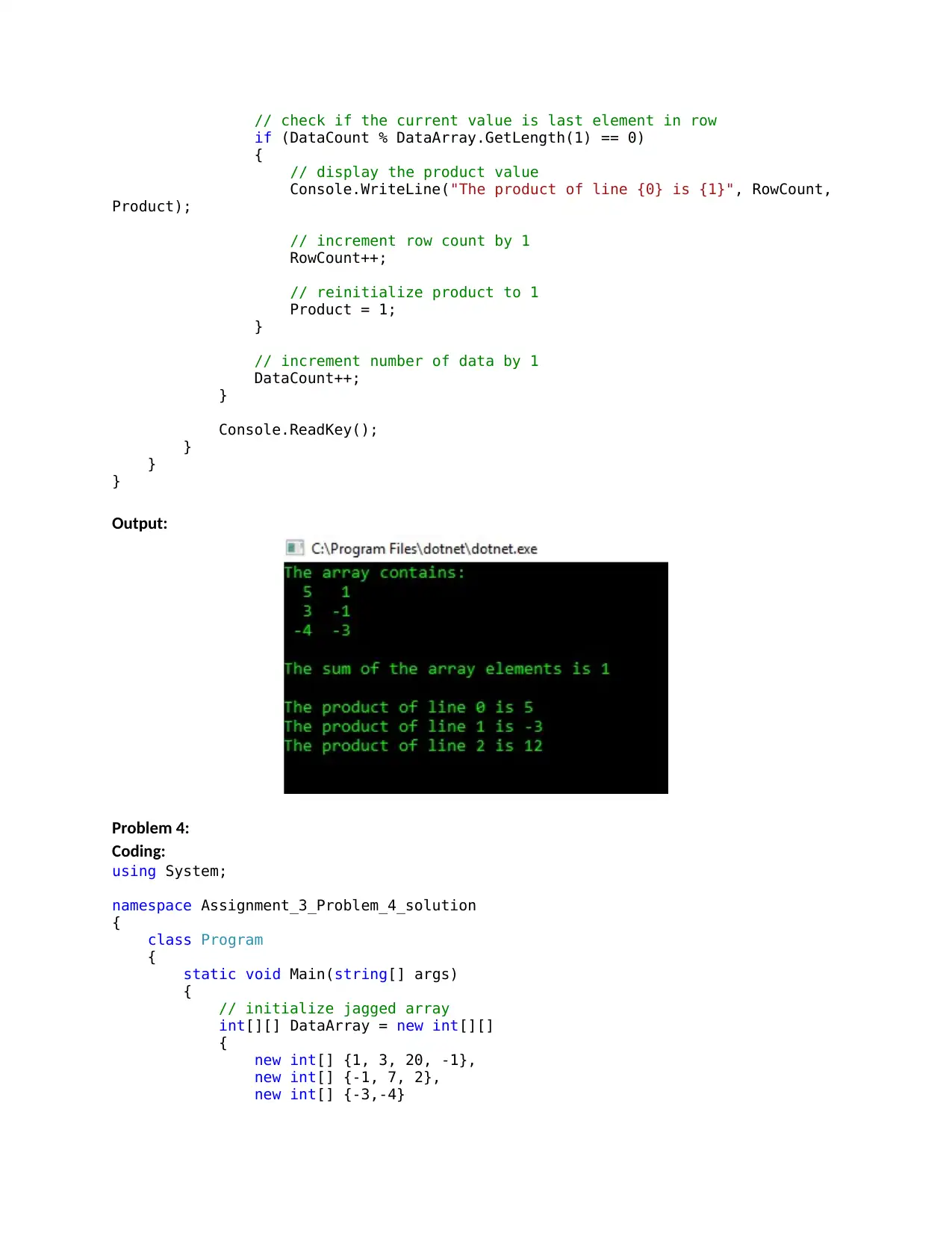
// check if the current value is last element in row
if (DataCount % DataArray.GetLength(1) == 0)
{
// display the product value
Console.WriteLine("The product of line {0} is {1}", RowCount,
Product);
// increment row count by 1
RowCount++;
// reinitialize product to 1
Product = 1;
}
// increment number of data by 1
DataCount++;
}
Console.ReadKey();
}
}
}
Output:
Problem 4:
Coding:
using System;
namespace Assignment_3_Problem_4_solution
{
class Program
{
static void Main(string[] args)
{
// initialize jagged array
int[][] DataArray = new int[][]
{
new int[] {1, 3, 20, -1},
new int[] {-1, 7, 2},
new int[] {-3,-4}
if (DataCount % DataArray.GetLength(1) == 0)
{
// display the product value
Console.WriteLine("The product of line {0} is {1}", RowCount,
Product);
// increment row count by 1
RowCount++;
// reinitialize product to 1
Product = 1;
}
// increment number of data by 1
DataCount++;
}
Console.ReadKey();
}
}
}
Output:
Problem 4:
Coding:
using System;
namespace Assignment_3_Problem_4_solution
{
class Program
{
static void Main(string[] args)
{
// initialize jagged array
int[][] DataArray = new int[][]
{
new int[] {1, 3, 20, -1},
new int[] {-1, 7, 2},
new int[] {-3,-4}
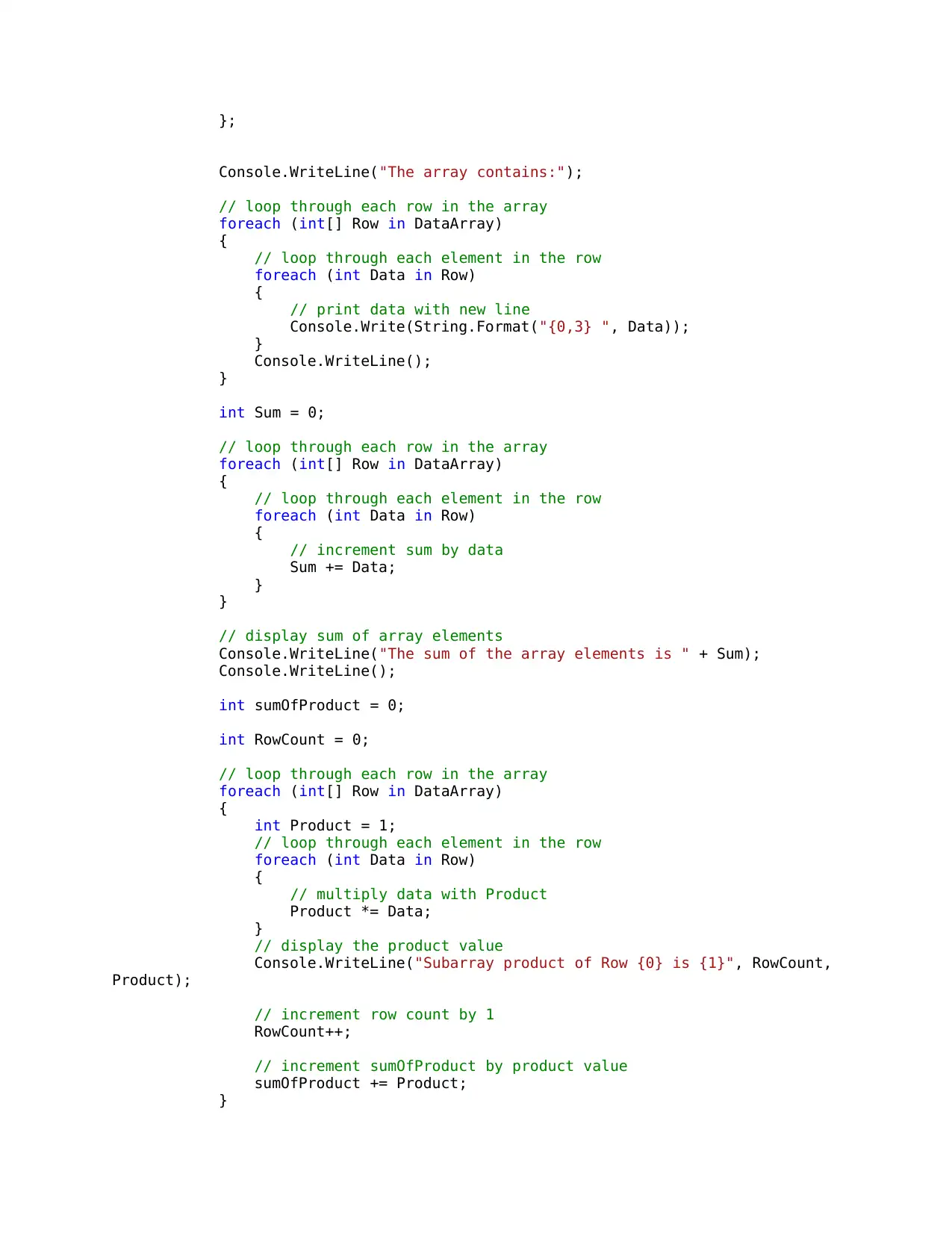
};
Console.WriteLine("The array contains:");
// loop through each row in the array
foreach (int[] Row in DataArray)
{
// loop through each element in the row
foreach (int Data in Row)
{
// print data with new line
Console.Write(String.Format("{0,3} ", Data));
}
Console.WriteLine();
}
int Sum = 0;
// loop through each row in the array
foreach (int[] Row in DataArray)
{
// loop through each element in the row
foreach (int Data in Row)
{
// increment sum by data
Sum += Data;
}
}
// display sum of array elements
Console.WriteLine("The sum of the array elements is " + Sum);
Console.WriteLine();
int sumOfProduct = 0;
int RowCount = 0;
// loop through each row in the array
foreach (int[] Row in DataArray)
{
int Product = 1;
// loop through each element in the row
foreach (int Data in Row)
{
// multiply data with Product
Product *= Data;
}
// display the product value
Console.WriteLine("Subarray product of Row {0} is {1}", RowCount,
Product);
// increment row count by 1
RowCount++;
// increment sumOfProduct by product value
sumOfProduct += Product;
}
Console.WriteLine("The array contains:");
// loop through each row in the array
foreach (int[] Row in DataArray)
{
// loop through each element in the row
foreach (int Data in Row)
{
// print data with new line
Console.Write(String.Format("{0,3} ", Data));
}
Console.WriteLine();
}
int Sum = 0;
// loop through each row in the array
foreach (int[] Row in DataArray)
{
// loop through each element in the row
foreach (int Data in Row)
{
// increment sum by data
Sum += Data;
}
}
// display sum of array elements
Console.WriteLine("The sum of the array elements is " + Sum);
Console.WriteLine();
int sumOfProduct = 0;
int RowCount = 0;
// loop through each row in the array
foreach (int[] Row in DataArray)
{
int Product = 1;
// loop through each element in the row
foreach (int Data in Row)
{
// multiply data with Product
Product *= Data;
}
// display the product value
Console.WriteLine("Subarray product of Row {0} is {1}", RowCount,
Product);
// increment row count by 1
RowCount++;
// increment sumOfProduct by product value
sumOfProduct += Product;
}

// display sum of product
Console.WriteLine("\nSum of the Products is {0}", sumOfProduct);
Console.ReadKey();
}
}
}
Output:
Console.WriteLine("\nSum of the Products is {0}", sumOfProduct);
Console.ReadKey();
}
}
}
Output:
1 out of 7
Related Documents
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
 +13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024  |  Zucol Services PVT LTD  |  All rights reserved.