Difference Between if-else and switch
VerifiedAdded on 2023/04/21
|13
|2163
|363
AI Summary
Switch and if-else both statements are the selection statements. Selection statements move the flow of the program to the particular block on specific conditions. E.g. if the condition is true, a particular block of statement will be executed otherwise another block of statement will be executed.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
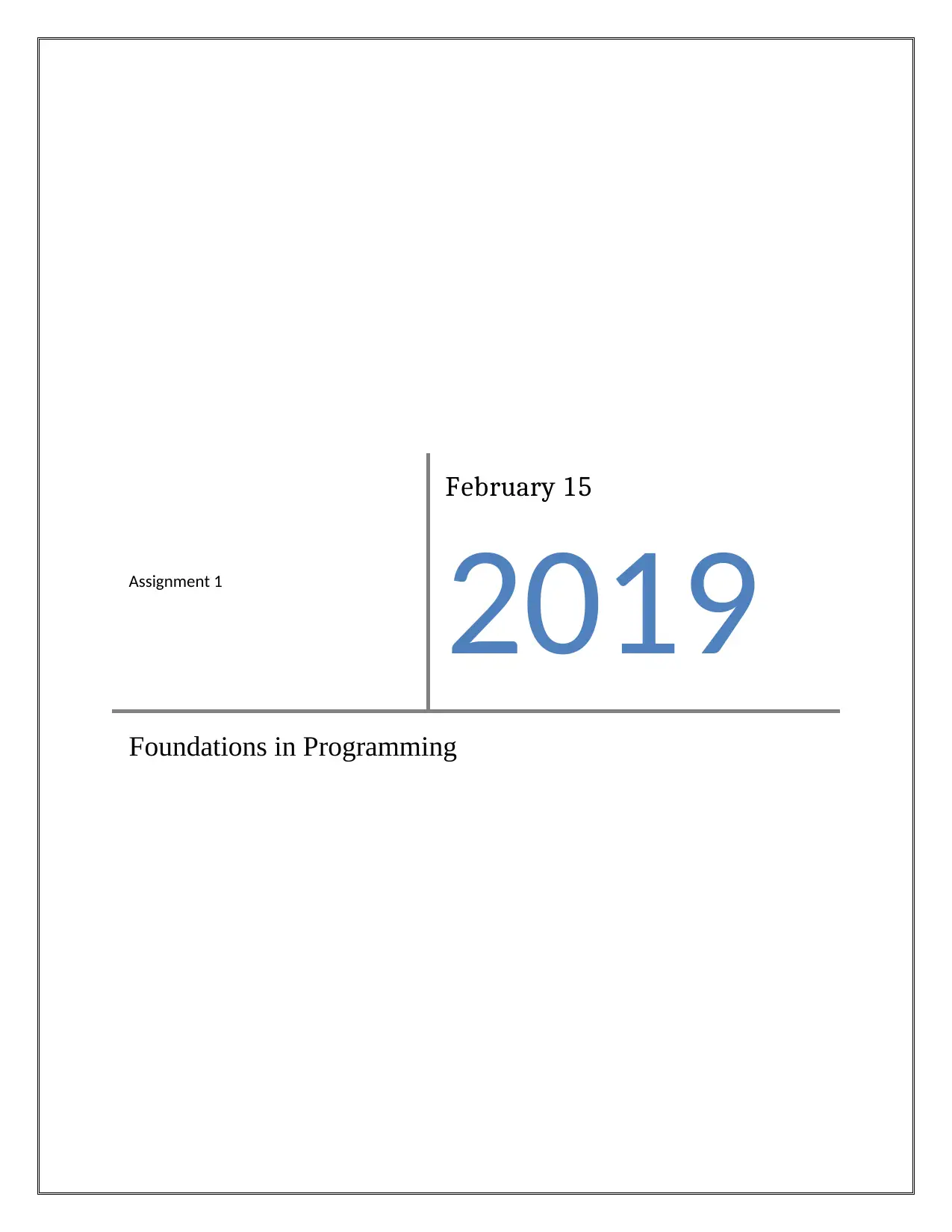
Assignment 1
February 15
2019
Foundations in Programming
February 15
2019
Foundations in Programming
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
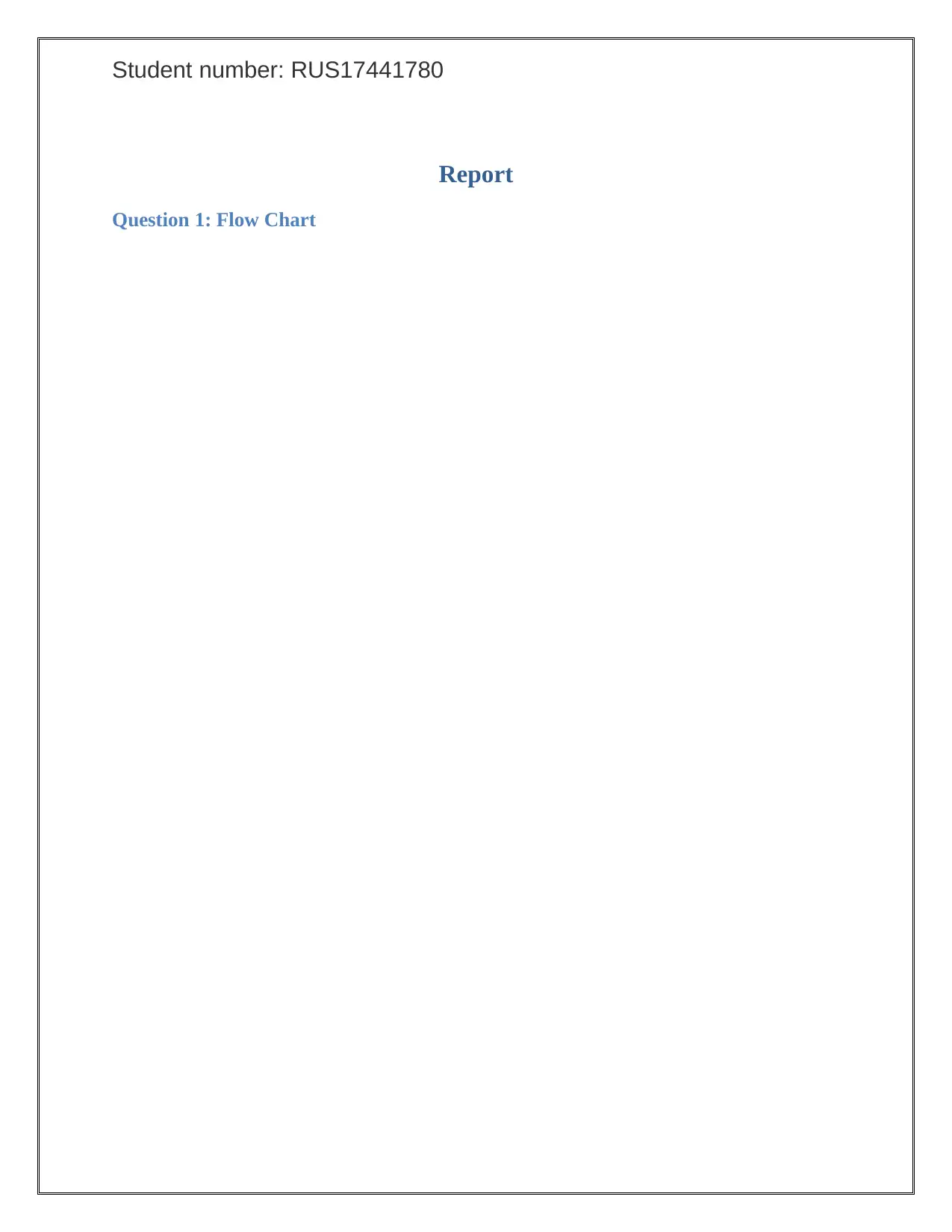
Student number: RUS17441780
Report
Question 1: Flow Chart
Report
Question 1: Flow Chart
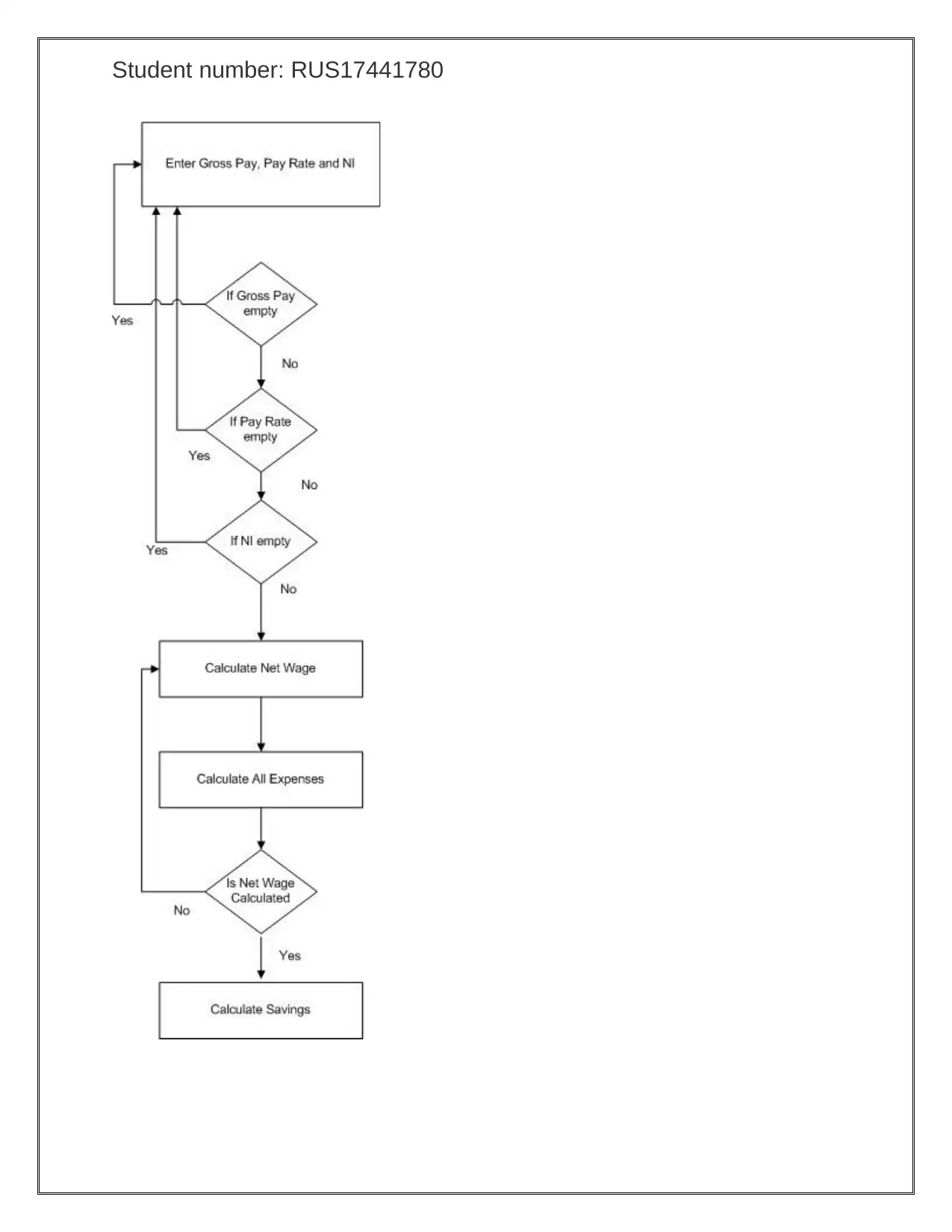
Student number: RUS17441780
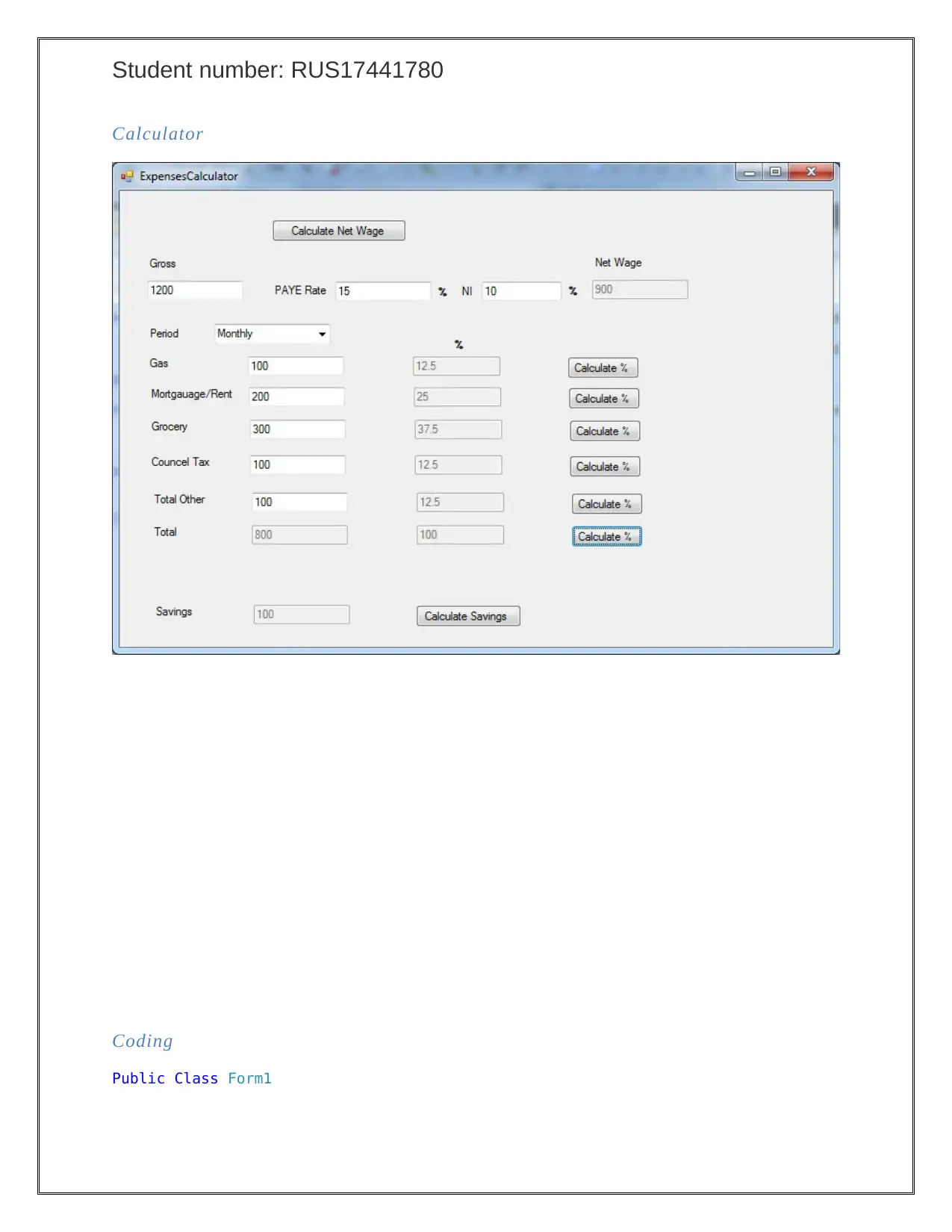
Student number: RUS17441780
Calculator
Coding
Public Class Form1
Calculator
Coding
Public Class Form1
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
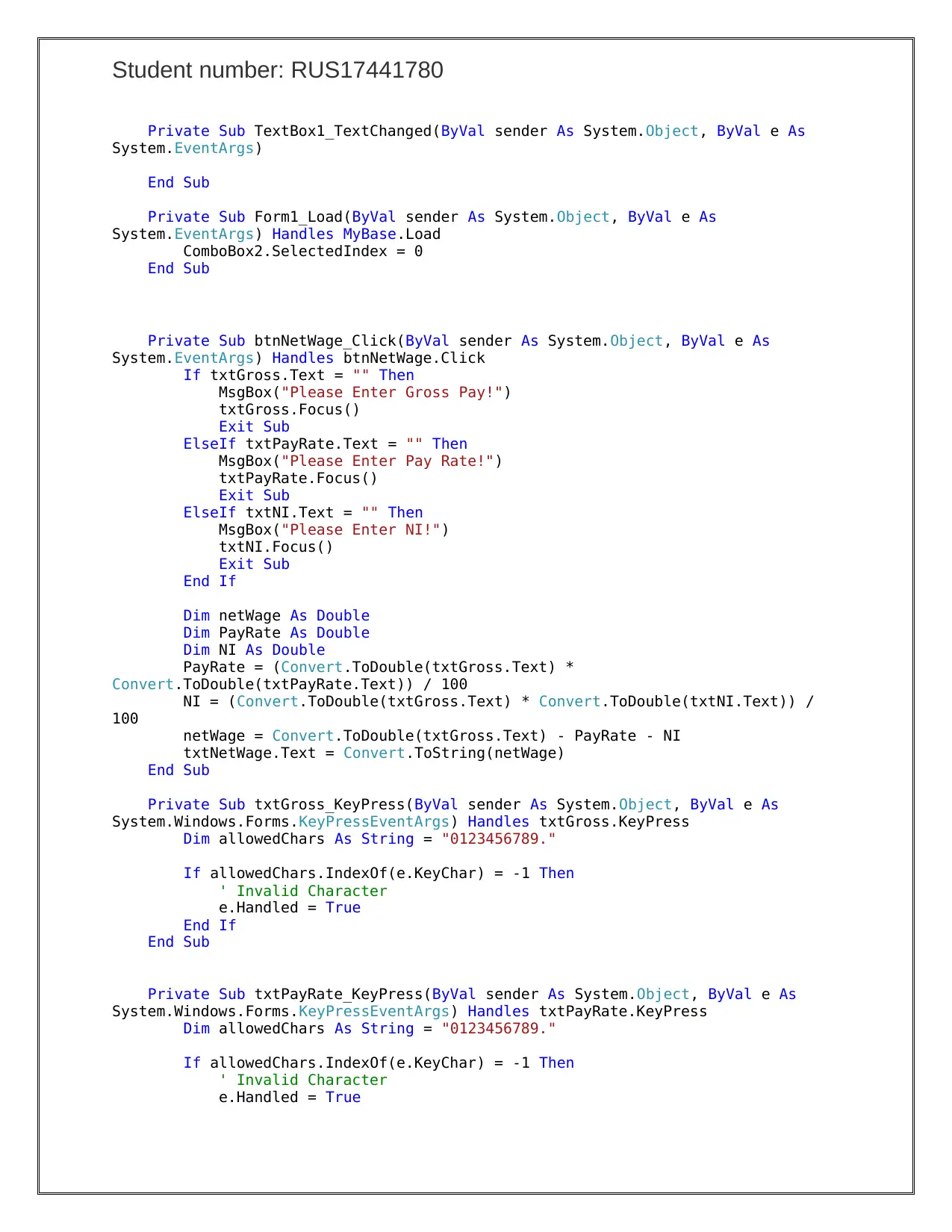
Student number: RUS17441780
Private Sub TextBox1_TextChanged(ByVal sender As System.Object, ByVal e As
System.EventArgs)
End Sub
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles MyBase.Load
ComboBox2.SelectedIndex = 0
End Sub
Private Sub btnNetWage_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles btnNetWage.Click
If txtGross.Text = "" Then
MsgBox("Please Enter Gross Pay!")
txtGross.Focus()
Exit Sub
ElseIf txtPayRate.Text = "" Then
MsgBox("Please Enter Pay Rate!")
txtPayRate.Focus()
Exit Sub
ElseIf txtNI.Text = "" Then
MsgBox("Please Enter NI!")
txtNI.Focus()
Exit Sub
End If
Dim netWage As Double
Dim PayRate As Double
Dim NI As Double
PayRate = (Convert.ToDouble(txtGross.Text) *
Convert.ToDouble(txtPayRate.Text)) / 100
NI = (Convert.ToDouble(txtGross.Text) * Convert.ToDouble(txtNI.Text)) /
100
netWage = Convert.ToDouble(txtGross.Text) - PayRate - NI
txtNetWage.Text = Convert.ToString(netWage)
End Sub
Private Sub txtGross_KeyPress(ByVal sender As System.Object, ByVal e As
System.Windows.Forms.KeyPressEventArgs) Handles txtGross.KeyPress
Dim allowedChars As String = "0123456789."
If allowedChars.IndexOf(e.KeyChar) = -1 Then
' Invalid Character
e.Handled = True
End If
End Sub
Private Sub txtPayRate_KeyPress(ByVal sender As System.Object, ByVal e As
System.Windows.Forms.KeyPressEventArgs) Handles txtPayRate.KeyPress
Dim allowedChars As String = "0123456789."
If allowedChars.IndexOf(e.KeyChar) = -1 Then
' Invalid Character
e.Handled = True
Private Sub TextBox1_TextChanged(ByVal sender As System.Object, ByVal e As
System.EventArgs)
End Sub
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles MyBase.Load
ComboBox2.SelectedIndex = 0
End Sub
Private Sub btnNetWage_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles btnNetWage.Click
If txtGross.Text = "" Then
MsgBox("Please Enter Gross Pay!")
txtGross.Focus()
Exit Sub
ElseIf txtPayRate.Text = "" Then
MsgBox("Please Enter Pay Rate!")
txtPayRate.Focus()
Exit Sub
ElseIf txtNI.Text = "" Then
MsgBox("Please Enter NI!")
txtNI.Focus()
Exit Sub
End If
Dim netWage As Double
Dim PayRate As Double
Dim NI As Double
PayRate = (Convert.ToDouble(txtGross.Text) *
Convert.ToDouble(txtPayRate.Text)) / 100
NI = (Convert.ToDouble(txtGross.Text) * Convert.ToDouble(txtNI.Text)) /
100
netWage = Convert.ToDouble(txtGross.Text) - PayRate - NI
txtNetWage.Text = Convert.ToString(netWage)
End Sub
Private Sub txtGross_KeyPress(ByVal sender As System.Object, ByVal e As
System.Windows.Forms.KeyPressEventArgs) Handles txtGross.KeyPress
Dim allowedChars As String = "0123456789."
If allowedChars.IndexOf(e.KeyChar) = -1 Then
' Invalid Character
e.Handled = True
End If
End Sub
Private Sub txtPayRate_KeyPress(ByVal sender As System.Object, ByVal e As
System.Windows.Forms.KeyPressEventArgs) Handles txtPayRate.KeyPress
Dim allowedChars As String = "0123456789."
If allowedChars.IndexOf(e.KeyChar) = -1 Then
' Invalid Character
e.Handled = True
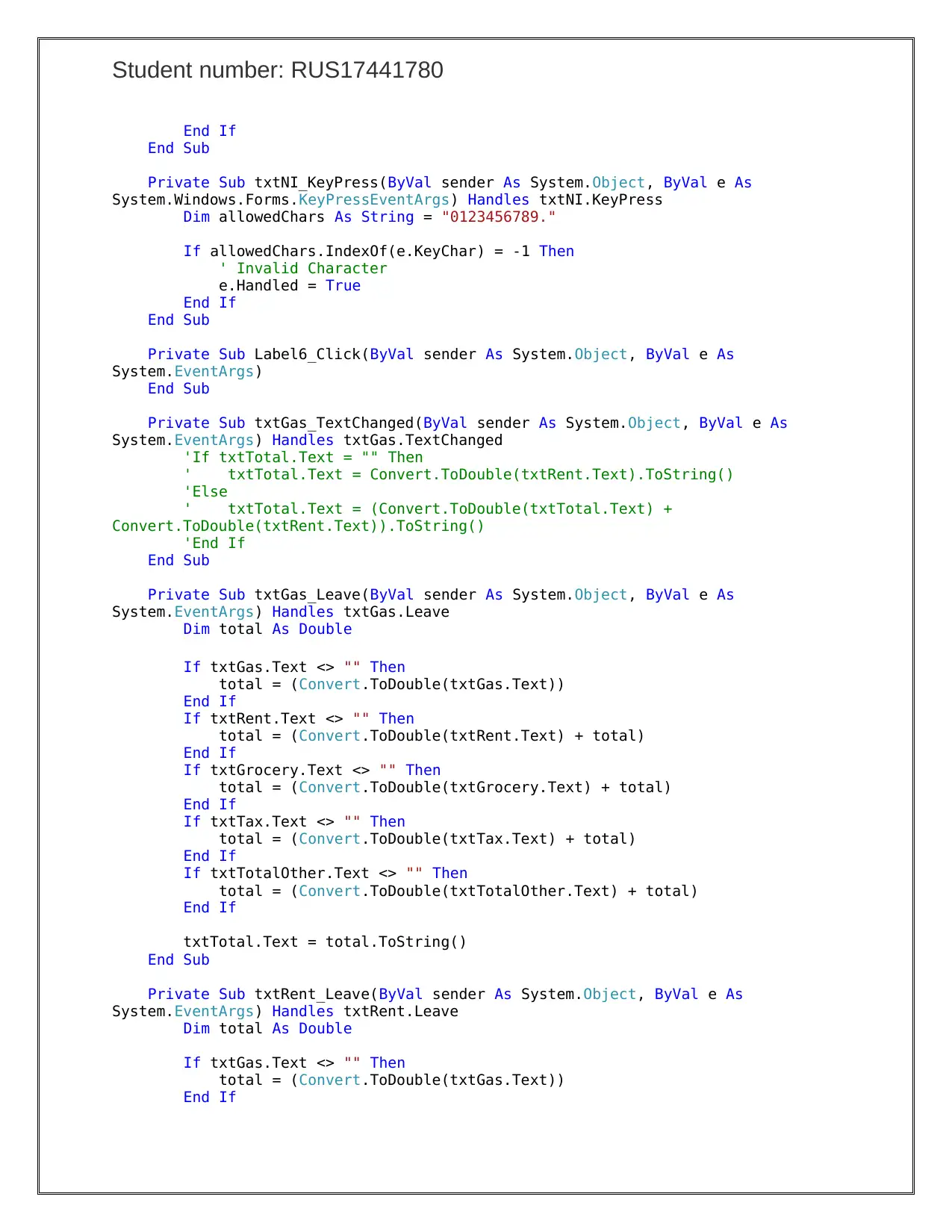
Student number: RUS17441780
End If
End Sub
Private Sub txtNI_KeyPress(ByVal sender As System.Object, ByVal e As
System.Windows.Forms.KeyPressEventArgs) Handles txtNI.KeyPress
Dim allowedChars As String = "0123456789."
If allowedChars.IndexOf(e.KeyChar) = -1 Then
' Invalid Character
e.Handled = True
End If
End Sub
Private Sub Label6_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs)
End Sub
Private Sub txtGas_TextChanged(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles txtGas.TextChanged
'If txtTotal.Text = "" Then
' txtTotal.Text = Convert.ToDouble(txtRent.Text).ToString()
'Else
' txtTotal.Text = (Convert.ToDouble(txtTotal.Text) +
Convert.ToDouble(txtRent.Text)).ToString()
'End If
End Sub
Private Sub txtGas_Leave(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles txtGas.Leave
Dim total As Double
If txtGas.Text <> "" Then
total = (Convert.ToDouble(txtGas.Text))
End If
If txtRent.Text <> "" Then
total = (Convert.ToDouble(txtRent.Text) + total)
End If
If txtGrocery.Text <> "" Then
total = (Convert.ToDouble(txtGrocery.Text) + total)
End If
If txtTax.Text <> "" Then
total = (Convert.ToDouble(txtTax.Text) + total)
End If
If txtTotalOther.Text <> "" Then
total = (Convert.ToDouble(txtTotalOther.Text) + total)
End If
txtTotal.Text = total.ToString()
End Sub
Private Sub txtRent_Leave(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles txtRent.Leave
Dim total As Double
If txtGas.Text <> "" Then
total = (Convert.ToDouble(txtGas.Text))
End If
End If
End Sub
Private Sub txtNI_KeyPress(ByVal sender As System.Object, ByVal e As
System.Windows.Forms.KeyPressEventArgs) Handles txtNI.KeyPress
Dim allowedChars As String = "0123456789."
If allowedChars.IndexOf(e.KeyChar) = -1 Then
' Invalid Character
e.Handled = True
End If
End Sub
Private Sub Label6_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs)
End Sub
Private Sub txtGas_TextChanged(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles txtGas.TextChanged
'If txtTotal.Text = "" Then
' txtTotal.Text = Convert.ToDouble(txtRent.Text).ToString()
'Else
' txtTotal.Text = (Convert.ToDouble(txtTotal.Text) +
Convert.ToDouble(txtRent.Text)).ToString()
'End If
End Sub
Private Sub txtGas_Leave(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles txtGas.Leave
Dim total As Double
If txtGas.Text <> "" Then
total = (Convert.ToDouble(txtGas.Text))
End If
If txtRent.Text <> "" Then
total = (Convert.ToDouble(txtRent.Text) + total)
End If
If txtGrocery.Text <> "" Then
total = (Convert.ToDouble(txtGrocery.Text) + total)
End If
If txtTax.Text <> "" Then
total = (Convert.ToDouble(txtTax.Text) + total)
End If
If txtTotalOther.Text <> "" Then
total = (Convert.ToDouble(txtTotalOther.Text) + total)
End If
txtTotal.Text = total.ToString()
End Sub
Private Sub txtRent_Leave(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles txtRent.Leave
Dim total As Double
If txtGas.Text <> "" Then
total = (Convert.ToDouble(txtGas.Text))
End If
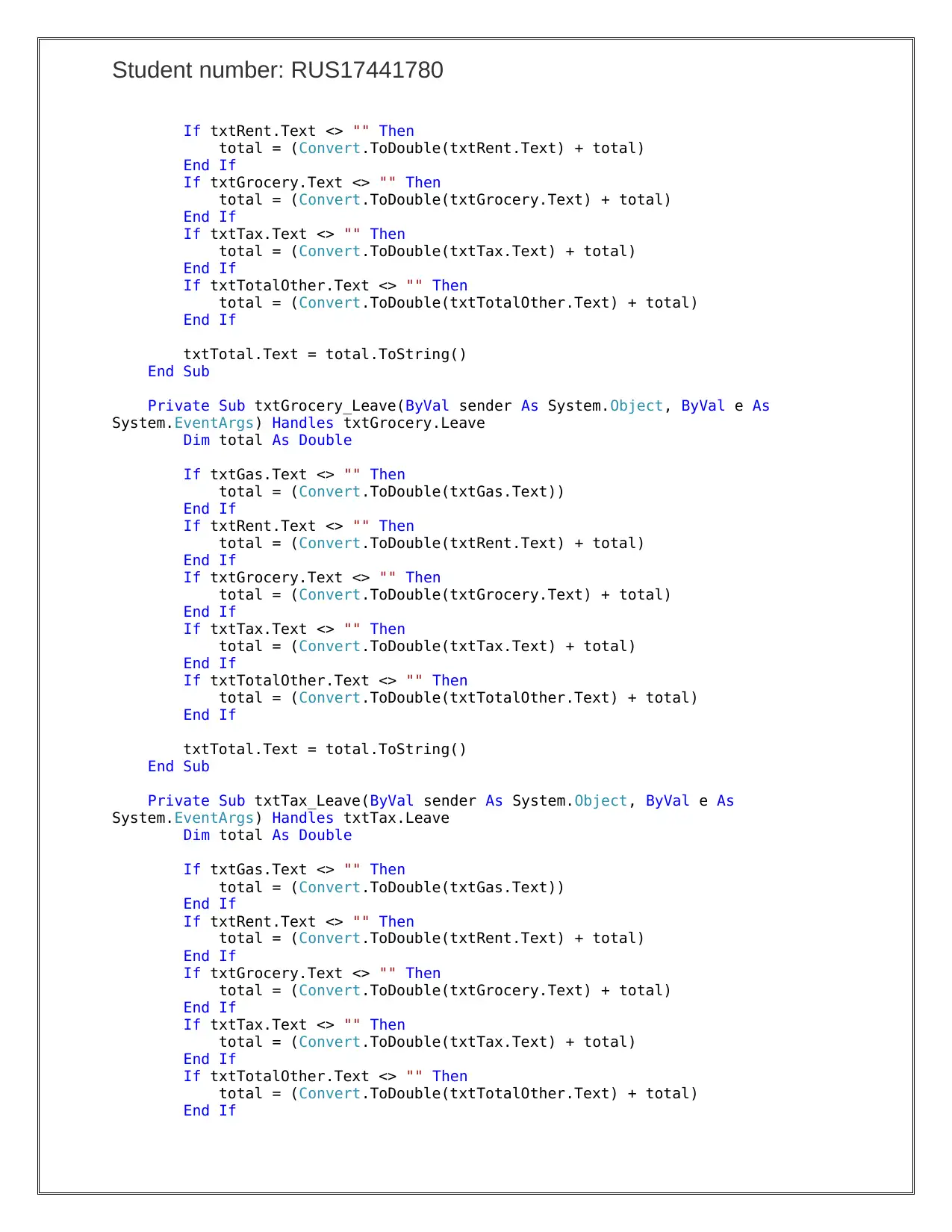
Student number: RUS17441780
If txtRent.Text <> "" Then
total = (Convert.ToDouble(txtRent.Text) + total)
End If
If txtGrocery.Text <> "" Then
total = (Convert.ToDouble(txtGrocery.Text) + total)
End If
If txtTax.Text <> "" Then
total = (Convert.ToDouble(txtTax.Text) + total)
End If
If txtTotalOther.Text <> "" Then
total = (Convert.ToDouble(txtTotalOther.Text) + total)
End If
txtTotal.Text = total.ToString()
End Sub
Private Sub txtGrocery_Leave(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles txtGrocery.Leave
Dim total As Double
If txtGas.Text <> "" Then
total = (Convert.ToDouble(txtGas.Text))
End If
If txtRent.Text <> "" Then
total = (Convert.ToDouble(txtRent.Text) + total)
End If
If txtGrocery.Text <> "" Then
total = (Convert.ToDouble(txtGrocery.Text) + total)
End If
If txtTax.Text <> "" Then
total = (Convert.ToDouble(txtTax.Text) + total)
End If
If txtTotalOther.Text <> "" Then
total = (Convert.ToDouble(txtTotalOther.Text) + total)
End If
txtTotal.Text = total.ToString()
End Sub
Private Sub txtTax_Leave(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles txtTax.Leave
Dim total As Double
If txtGas.Text <> "" Then
total = (Convert.ToDouble(txtGas.Text))
End If
If txtRent.Text <> "" Then
total = (Convert.ToDouble(txtRent.Text) + total)
End If
If txtGrocery.Text <> "" Then
total = (Convert.ToDouble(txtGrocery.Text) + total)
End If
If txtTax.Text <> "" Then
total = (Convert.ToDouble(txtTax.Text) + total)
End If
If txtTotalOther.Text <> "" Then
total = (Convert.ToDouble(txtTotalOther.Text) + total)
End If
If txtRent.Text <> "" Then
total = (Convert.ToDouble(txtRent.Text) + total)
End If
If txtGrocery.Text <> "" Then
total = (Convert.ToDouble(txtGrocery.Text) + total)
End If
If txtTax.Text <> "" Then
total = (Convert.ToDouble(txtTax.Text) + total)
End If
If txtTotalOther.Text <> "" Then
total = (Convert.ToDouble(txtTotalOther.Text) + total)
End If
txtTotal.Text = total.ToString()
End Sub
Private Sub txtGrocery_Leave(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles txtGrocery.Leave
Dim total As Double
If txtGas.Text <> "" Then
total = (Convert.ToDouble(txtGas.Text))
End If
If txtRent.Text <> "" Then
total = (Convert.ToDouble(txtRent.Text) + total)
End If
If txtGrocery.Text <> "" Then
total = (Convert.ToDouble(txtGrocery.Text) + total)
End If
If txtTax.Text <> "" Then
total = (Convert.ToDouble(txtTax.Text) + total)
End If
If txtTotalOther.Text <> "" Then
total = (Convert.ToDouble(txtTotalOther.Text) + total)
End If
txtTotal.Text = total.ToString()
End Sub
Private Sub txtTax_Leave(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles txtTax.Leave
Dim total As Double
If txtGas.Text <> "" Then
total = (Convert.ToDouble(txtGas.Text))
End If
If txtRent.Text <> "" Then
total = (Convert.ToDouble(txtRent.Text) + total)
End If
If txtGrocery.Text <> "" Then
total = (Convert.ToDouble(txtGrocery.Text) + total)
End If
If txtTax.Text <> "" Then
total = (Convert.ToDouble(txtTax.Text) + total)
End If
If txtTotalOther.Text <> "" Then
total = (Convert.ToDouble(txtTotalOther.Text) + total)
End If
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
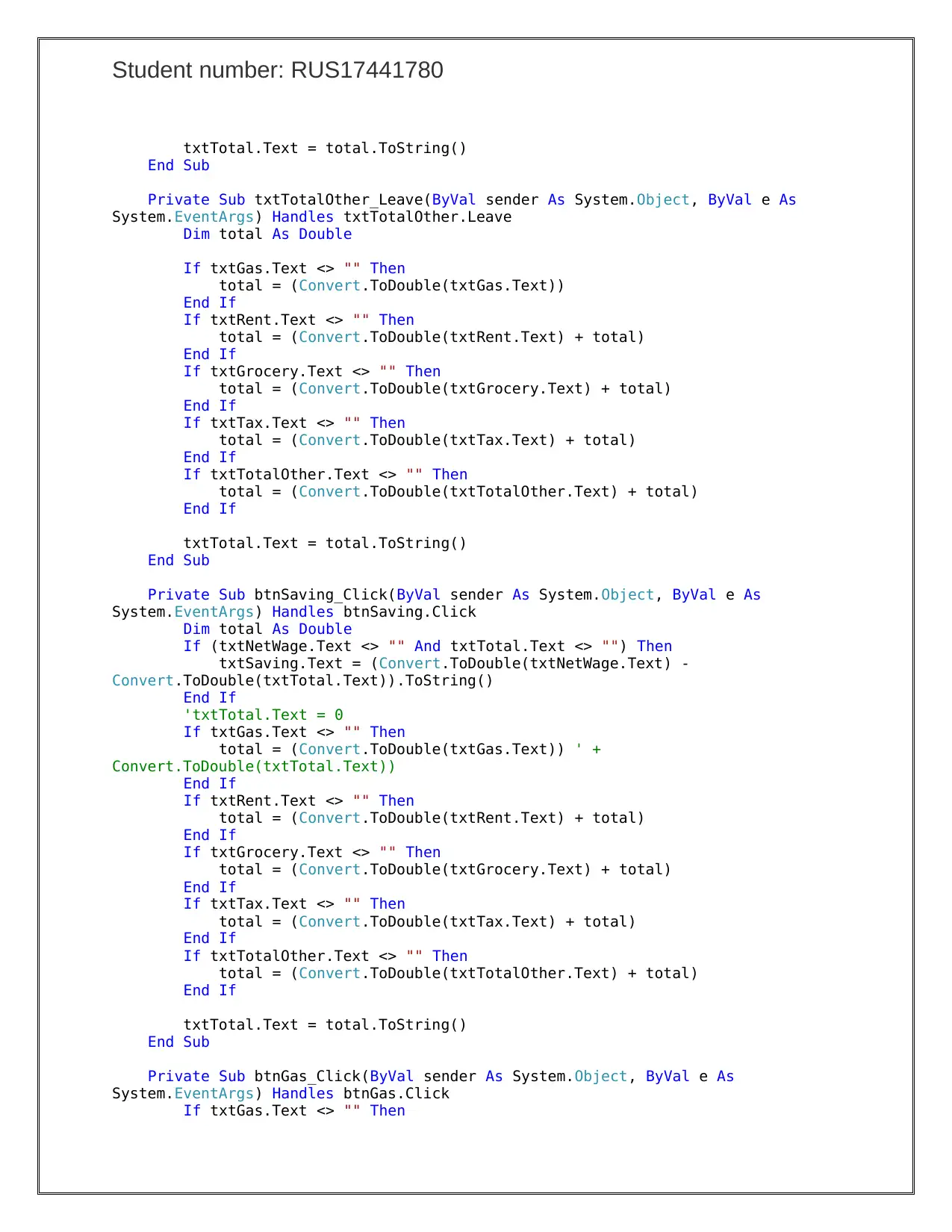
Student number: RUS17441780
txtTotal.Text = total.ToString()
End Sub
Private Sub txtTotalOther_Leave(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles txtTotalOther.Leave
Dim total As Double
If txtGas.Text <> "" Then
total = (Convert.ToDouble(txtGas.Text))
End If
If txtRent.Text <> "" Then
total = (Convert.ToDouble(txtRent.Text) + total)
End If
If txtGrocery.Text <> "" Then
total = (Convert.ToDouble(txtGrocery.Text) + total)
End If
If txtTax.Text <> "" Then
total = (Convert.ToDouble(txtTax.Text) + total)
End If
If txtTotalOther.Text <> "" Then
total = (Convert.ToDouble(txtTotalOther.Text) + total)
End If
txtTotal.Text = total.ToString()
End Sub
Private Sub btnSaving_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles btnSaving.Click
Dim total As Double
If (txtNetWage.Text <> "" And txtTotal.Text <> "") Then
txtSaving.Text = (Convert.ToDouble(txtNetWage.Text) -
Convert.ToDouble(txtTotal.Text)).ToString()
End If
'txtTotal.Text = 0
If txtGas.Text <> "" Then
total = (Convert.ToDouble(txtGas.Text)) ' +
Convert.ToDouble(txtTotal.Text))
End If
If txtRent.Text <> "" Then
total = (Convert.ToDouble(txtRent.Text) + total)
End If
If txtGrocery.Text <> "" Then
total = (Convert.ToDouble(txtGrocery.Text) + total)
End If
If txtTax.Text <> "" Then
total = (Convert.ToDouble(txtTax.Text) + total)
End If
If txtTotalOther.Text <> "" Then
total = (Convert.ToDouble(txtTotalOther.Text) + total)
End If
txtTotal.Text = total.ToString()
End Sub
Private Sub btnGas_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles btnGas.Click
If txtGas.Text <> "" Then
txtTotal.Text = total.ToString()
End Sub
Private Sub txtTotalOther_Leave(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles txtTotalOther.Leave
Dim total As Double
If txtGas.Text <> "" Then
total = (Convert.ToDouble(txtGas.Text))
End If
If txtRent.Text <> "" Then
total = (Convert.ToDouble(txtRent.Text) + total)
End If
If txtGrocery.Text <> "" Then
total = (Convert.ToDouble(txtGrocery.Text) + total)
End If
If txtTax.Text <> "" Then
total = (Convert.ToDouble(txtTax.Text) + total)
End If
If txtTotalOther.Text <> "" Then
total = (Convert.ToDouble(txtTotalOther.Text) + total)
End If
txtTotal.Text = total.ToString()
End Sub
Private Sub btnSaving_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles btnSaving.Click
Dim total As Double
If (txtNetWage.Text <> "" And txtTotal.Text <> "") Then
txtSaving.Text = (Convert.ToDouble(txtNetWage.Text) -
Convert.ToDouble(txtTotal.Text)).ToString()
End If
'txtTotal.Text = 0
If txtGas.Text <> "" Then
total = (Convert.ToDouble(txtGas.Text)) ' +
Convert.ToDouble(txtTotal.Text))
End If
If txtRent.Text <> "" Then
total = (Convert.ToDouble(txtRent.Text) + total)
End If
If txtGrocery.Text <> "" Then
total = (Convert.ToDouble(txtGrocery.Text) + total)
End If
If txtTax.Text <> "" Then
total = (Convert.ToDouble(txtTax.Text) + total)
End If
If txtTotalOther.Text <> "" Then
total = (Convert.ToDouble(txtTotalOther.Text) + total)
End If
txtTotal.Text = total.ToString()
End Sub
Private Sub btnGas_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles btnGas.Click
If txtGas.Text <> "" Then
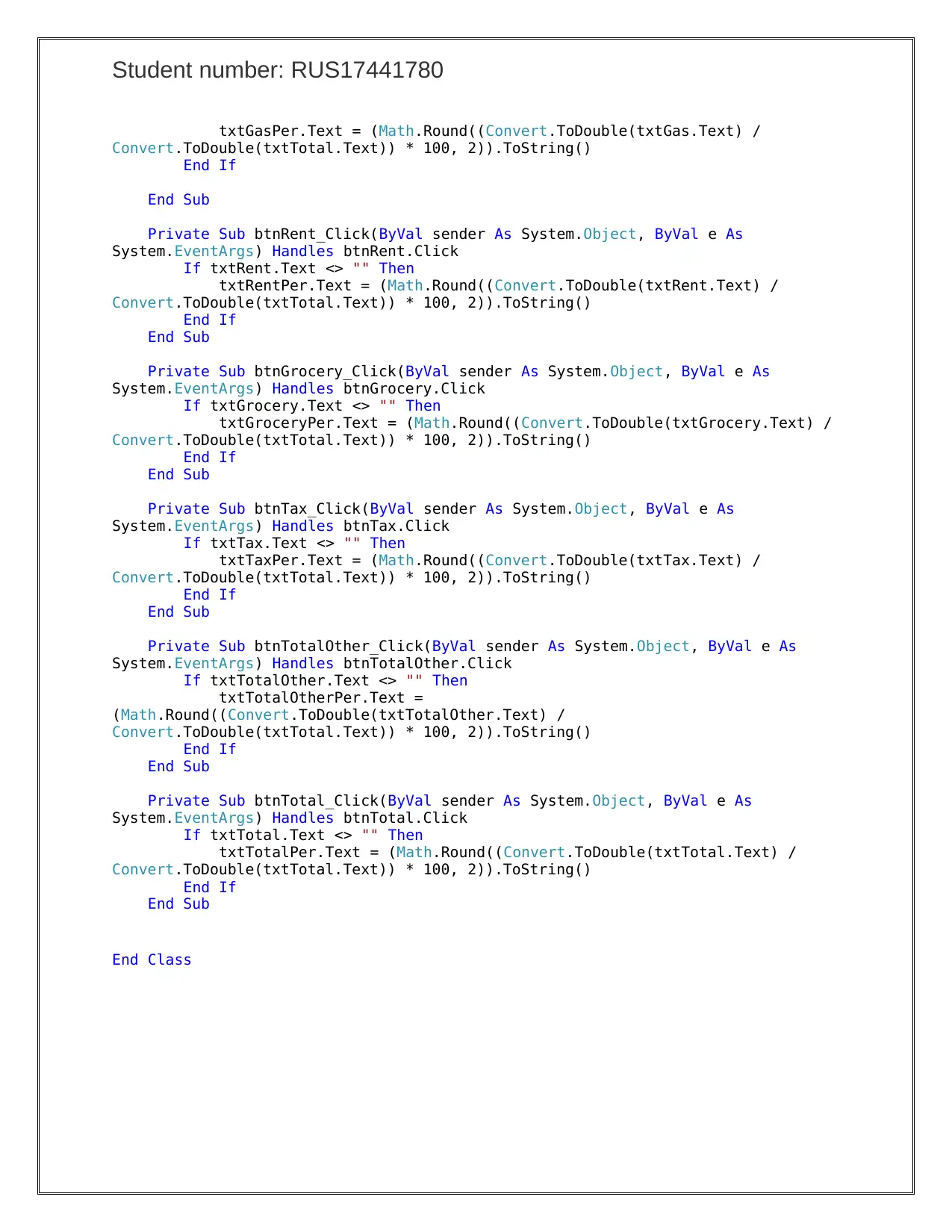
Student number: RUS17441780
txtGasPer.Text = (Math.Round((Convert.ToDouble(txtGas.Text) /
Convert.ToDouble(txtTotal.Text)) * 100, 2)).ToString()
End If
End Sub
Private Sub btnRent_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles btnRent.Click
If txtRent.Text <> "" Then
txtRentPer.Text = (Math.Round((Convert.ToDouble(txtRent.Text) /
Convert.ToDouble(txtTotal.Text)) * 100, 2)).ToString()
End If
End Sub
Private Sub btnGrocery_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles btnGrocery.Click
If txtGrocery.Text <> "" Then
txtGroceryPer.Text = (Math.Round((Convert.ToDouble(txtGrocery.Text) /
Convert.ToDouble(txtTotal.Text)) * 100, 2)).ToString()
End If
End Sub
Private Sub btnTax_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles btnTax.Click
If txtTax.Text <> "" Then
txtTaxPer.Text = (Math.Round((Convert.ToDouble(txtTax.Text) /
Convert.ToDouble(txtTotal.Text)) * 100, 2)).ToString()
End If
End Sub
Private Sub btnTotalOther_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles btnTotalOther.Click
If txtTotalOther.Text <> "" Then
txtTotalOtherPer.Text =
(Math.Round((Convert.ToDouble(txtTotalOther.Text) /
Convert.ToDouble(txtTotal.Text)) * 100, 2)).ToString()
End If
End Sub
Private Sub btnTotal_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles btnTotal.Click
If txtTotal.Text <> "" Then
txtTotalPer.Text = (Math.Round((Convert.ToDouble(txtTotal.Text) /
Convert.ToDouble(txtTotal.Text)) * 100, 2)).ToString()
End If
End Sub
End Class
txtGasPer.Text = (Math.Round((Convert.ToDouble(txtGas.Text) /
Convert.ToDouble(txtTotal.Text)) * 100, 2)).ToString()
End If
End Sub
Private Sub btnRent_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles btnRent.Click
If txtRent.Text <> "" Then
txtRentPer.Text = (Math.Round((Convert.ToDouble(txtRent.Text) /
Convert.ToDouble(txtTotal.Text)) * 100, 2)).ToString()
End If
End Sub
Private Sub btnGrocery_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles btnGrocery.Click
If txtGrocery.Text <> "" Then
txtGroceryPer.Text = (Math.Round((Convert.ToDouble(txtGrocery.Text) /
Convert.ToDouble(txtTotal.Text)) * 100, 2)).ToString()
End If
End Sub
Private Sub btnTax_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles btnTax.Click
If txtTax.Text <> "" Then
txtTaxPer.Text = (Math.Round((Convert.ToDouble(txtTax.Text) /
Convert.ToDouble(txtTotal.Text)) * 100, 2)).ToString()
End If
End Sub
Private Sub btnTotalOther_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles btnTotalOther.Click
If txtTotalOther.Text <> "" Then
txtTotalOtherPer.Text =
(Math.Round((Convert.ToDouble(txtTotalOther.Text) /
Convert.ToDouble(txtTotal.Text)) * 100, 2)).ToString()
End If
End Sub
Private Sub btnTotal_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles btnTotal.Click
If txtTotal.Text <> "" Then
txtTotalPer.Text = (Math.Round((Convert.ToDouble(txtTotal.Text) /
Convert.ToDouble(txtTotal.Text)) * 100, 2)).ToString()
End If
End Sub
End Class
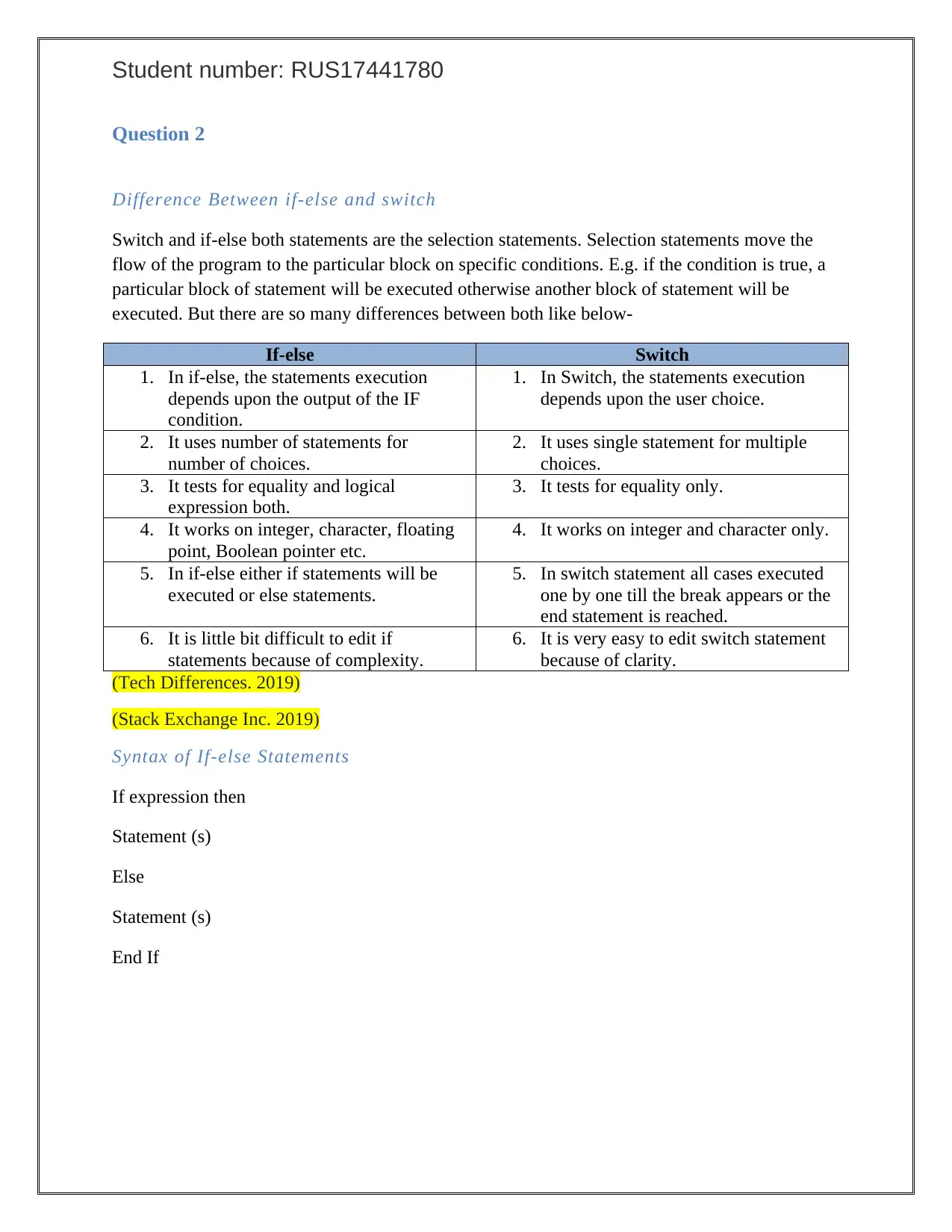
Student number: RUS17441780
Question 2
Difference Between if-else and switch
Switch and if-else both statements are the selection statements. Selection statements move the
flow of the program to the particular block on specific conditions. E.g. if the condition is true, a
particular block of statement will be executed otherwise another block of statement will be
executed. But there are so many differences between both like below-
If-else Switch
1. In if-else, the statements execution
depends upon the output of the IF
condition.
1. In Switch, the statements execution
depends upon the user choice.
2. It uses number of statements for
number of choices.
2. It uses single statement for multiple
choices.
3. It tests for equality and logical
expression both.
3. It tests for equality only.
4. It works on integer, character, floating
point, Boolean pointer etc.
4. It works on integer and character only.
5. In if-else either if statements will be
executed or else statements.
5. In switch statement all cases executed
one by one till the break appears or the
end statement is reached.
6. It is little bit difficult to edit if
statements because of complexity.
6. It is very easy to edit switch statement
because of clarity.
(Tech Differences. 2019)
(Stack Exchange Inc. 2019)
Syntax of If-else Statements
If expression then
Statement (s)
Else
Statement (s)
End If
Question 2
Difference Between if-else and switch
Switch and if-else both statements are the selection statements. Selection statements move the
flow of the program to the particular block on specific conditions. E.g. if the condition is true, a
particular block of statement will be executed otherwise another block of statement will be
executed. But there are so many differences between both like below-
If-else Switch
1. In if-else, the statements execution
depends upon the output of the IF
condition.
1. In Switch, the statements execution
depends upon the user choice.
2. It uses number of statements for
number of choices.
2. It uses single statement for multiple
choices.
3. It tests for equality and logical
expression both.
3. It tests for equality only.
4. It works on integer, character, floating
point, Boolean pointer etc.
4. It works on integer and character only.
5. In if-else either if statements will be
executed or else statements.
5. In switch statement all cases executed
one by one till the break appears or the
end statement is reached.
6. It is little bit difficult to edit if
statements because of complexity.
6. It is very easy to edit switch statement
because of clarity.
(Tech Differences. 2019)
(Stack Exchange Inc. 2019)
Syntax of If-else Statements
If expression then
Statement (s)
Else
Statement (s)
End If
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
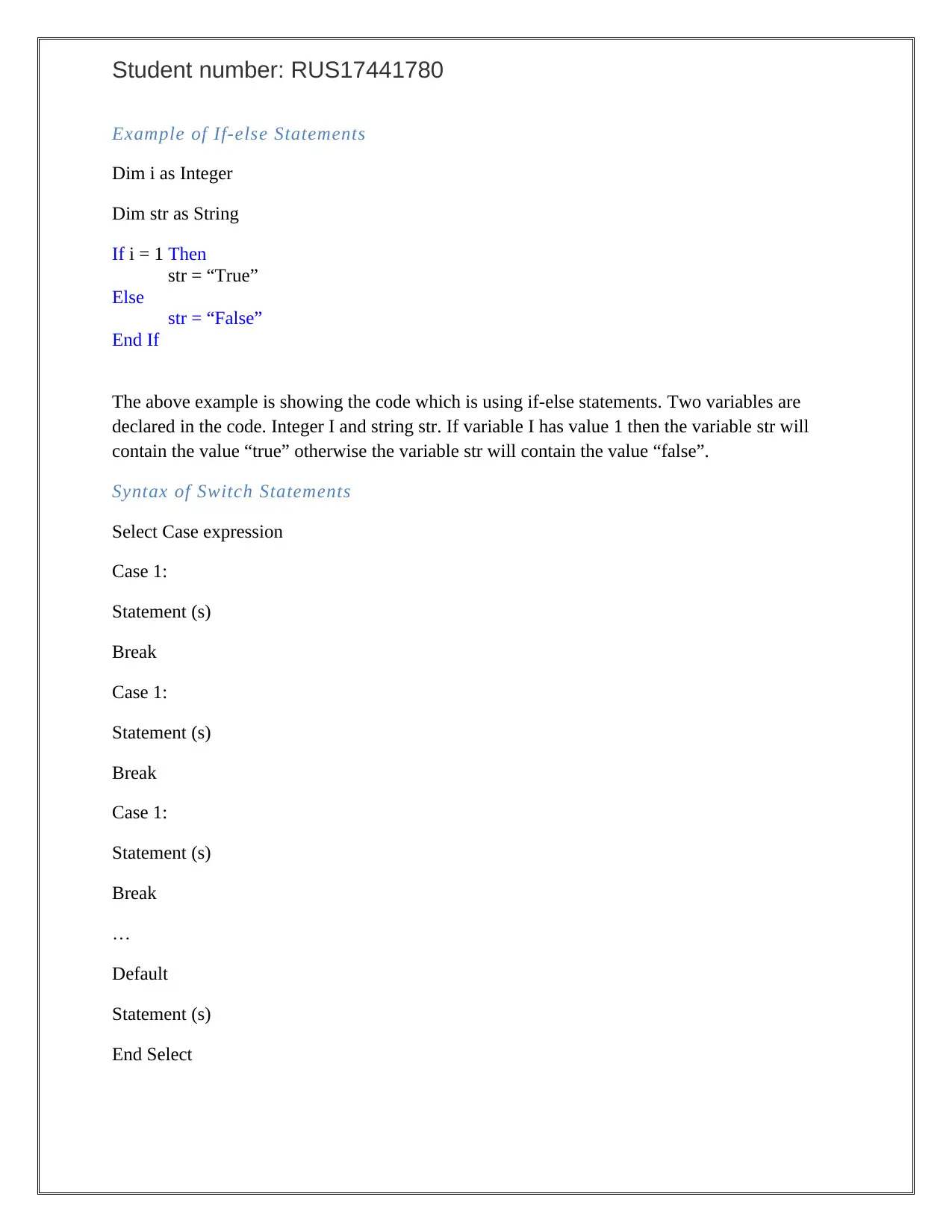
Student number: RUS17441780
Example of If-else Statements
Dim i as Integer
Dim str as String
If i = 1 Then
str = “True”
Else
str = “False”
End If
The above example is showing the code which is using if-else statements. Two variables are
declared in the code. Integer I and string str. If variable I has value 1 then the variable str will
contain the value “true” otherwise the variable str will contain the value “false”.
Syntax of Switch Statements
Select Case expression
Case 1:
Statement (s)
Break
Case 1:
Statement (s)
Break
Case 1:
Statement (s)
Break
…
Default
Statement (s)
End Select
Example of If-else Statements
Dim i as Integer
Dim str as String
If i = 1 Then
str = “True”
Else
str = “False”
End If
The above example is showing the code which is using if-else statements. Two variables are
declared in the code. Integer I and string str. If variable I has value 1 then the variable str will
contain the value “true” otherwise the variable str will contain the value “false”.
Syntax of Switch Statements
Select Case expression
Case 1:
Statement (s)
Break
Case 1:
Statement (s)
Break
Case 1:
Statement (s)
Break
…
Default
Statement (s)
End Select
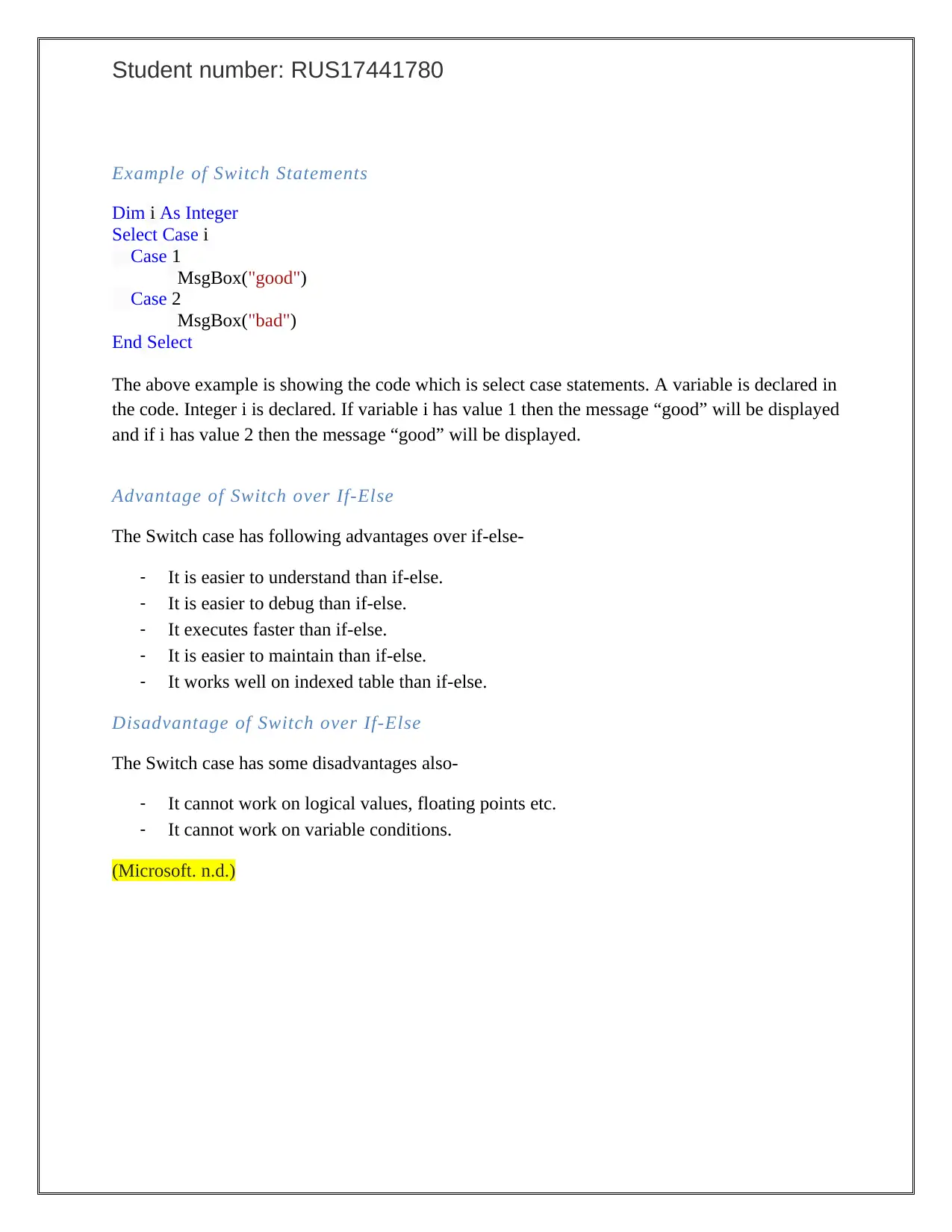
Student number: RUS17441780
Example of Switch Statements
Dim i As Integer
Select Case i
Case 1
MsgBox("good")
Case 2
MsgBox("bad")
End Select
The above example is showing the code which is select case statements. A variable is declared in
the code. Integer i is declared. If variable i has value 1 then the message “good” will be displayed
and if i has value 2 then the message “good” will be displayed.
Advantage of Switch over If-Else
The Switch case has following advantages over if-else-
- It is easier to understand than if-else.
- It is easier to debug than if-else.
- It executes faster than if-else.
- It is easier to maintain than if-else.
- It works well on indexed table than if-else.
Disadvantage of Switch over If-Else
The Switch case has some disadvantages also-
- It cannot work on logical values, floating points etc.
- It cannot work on variable conditions.
(Microsoft. n.d.)
Example of Switch Statements
Dim i As Integer
Select Case i
Case 1
MsgBox("good")
Case 2
MsgBox("bad")
End Select
The above example is showing the code which is select case statements. A variable is declared in
the code. Integer i is declared. If variable i has value 1 then the message “good” will be displayed
and if i has value 2 then the message “good” will be displayed.
Advantage of Switch over If-Else
The Switch case has following advantages over if-else-
- It is easier to understand than if-else.
- It is easier to debug than if-else.
- It executes faster than if-else.
- It is easier to maintain than if-else.
- It works well on indexed table than if-else.
Disadvantage of Switch over If-Else
The Switch case has some disadvantages also-
- It cannot work on logical values, floating points etc.
- It cannot work on variable conditions.
(Microsoft. n.d.)
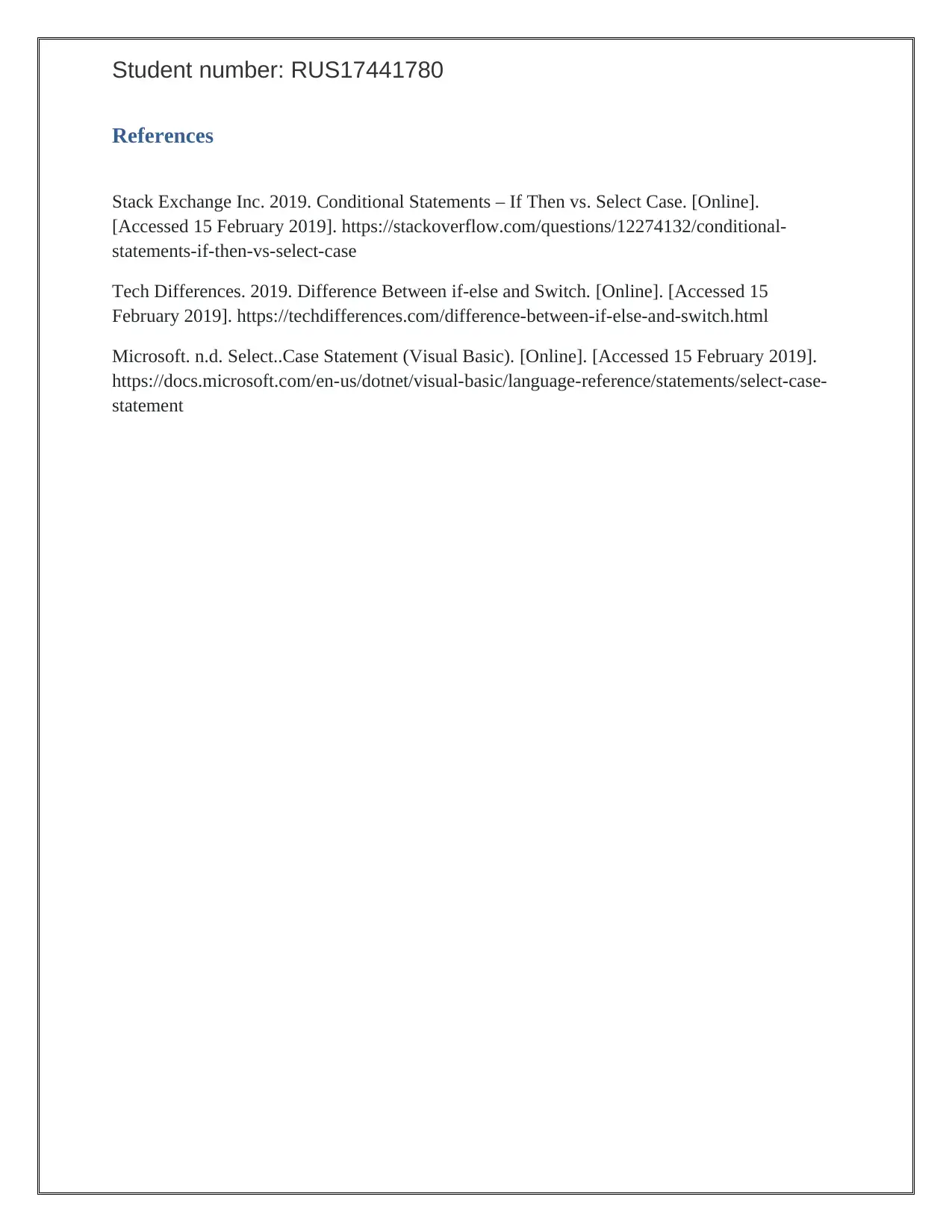
Student number: RUS17441780
References
Stack Exchange Inc. 2019. Conditional Statements – If Then vs. Select Case. [Online].
[Accessed 15 February 2019]. https://stackoverflow.com/questions/12274132/conditional-
statements-if-then-vs-select-case
Tech Differences. 2019. Difference Between if-else and Switch. [Online]. [Accessed 15
February 2019]. https://techdifferences.com/difference-between-if-else-and-switch.html
Microsoft. n.d. Select..Case Statement (Visual Basic). [Online]. [Accessed 15 February 2019].
https://docs.microsoft.com/en-us/dotnet/visual-basic/language-reference/statements/select-case-
statement
References
Stack Exchange Inc. 2019. Conditional Statements – If Then vs. Select Case. [Online].
[Accessed 15 February 2019]. https://stackoverflow.com/questions/12274132/conditional-
statements-if-then-vs-select-case
Tech Differences. 2019. Difference Between if-else and Switch. [Online]. [Accessed 15
February 2019]. https://techdifferences.com/difference-between-if-else-and-switch.html
Microsoft. n.d. Select..Case Statement (Visual Basic). [Online]. [Accessed 15 February 2019].
https://docs.microsoft.com/en-us/dotnet/visual-basic/language-reference/statements/select-case-
statement
1 out of 13
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.