User and Technical Documentation for Health Club Membership Calculator Program
VerifiedAdded on  2023/06/10
|26
|5023
|395
AI Summary
This document provides user and technical documentation for Health Club Membership Calculator program. The user documentation includes the graphical and command line interface design, program comments, and a guide to run the program. The technical documentation includes the program's name, version, programming language, purpose, source code, include files, data files, and functions. Additionally, the document provides a strategy for testing the program with normal, extreme, and exceptional test cases.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
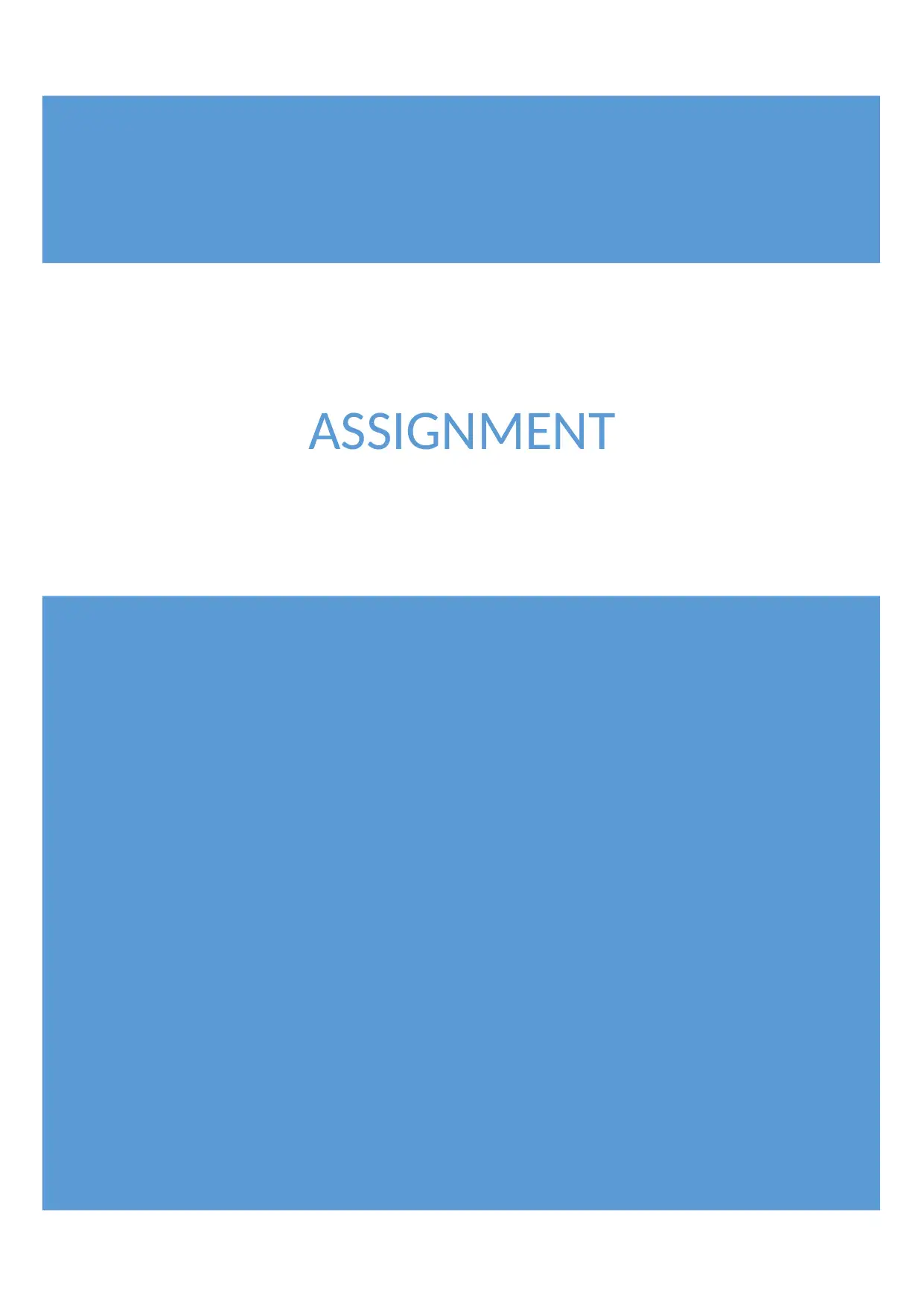
ASSIGNMENT
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
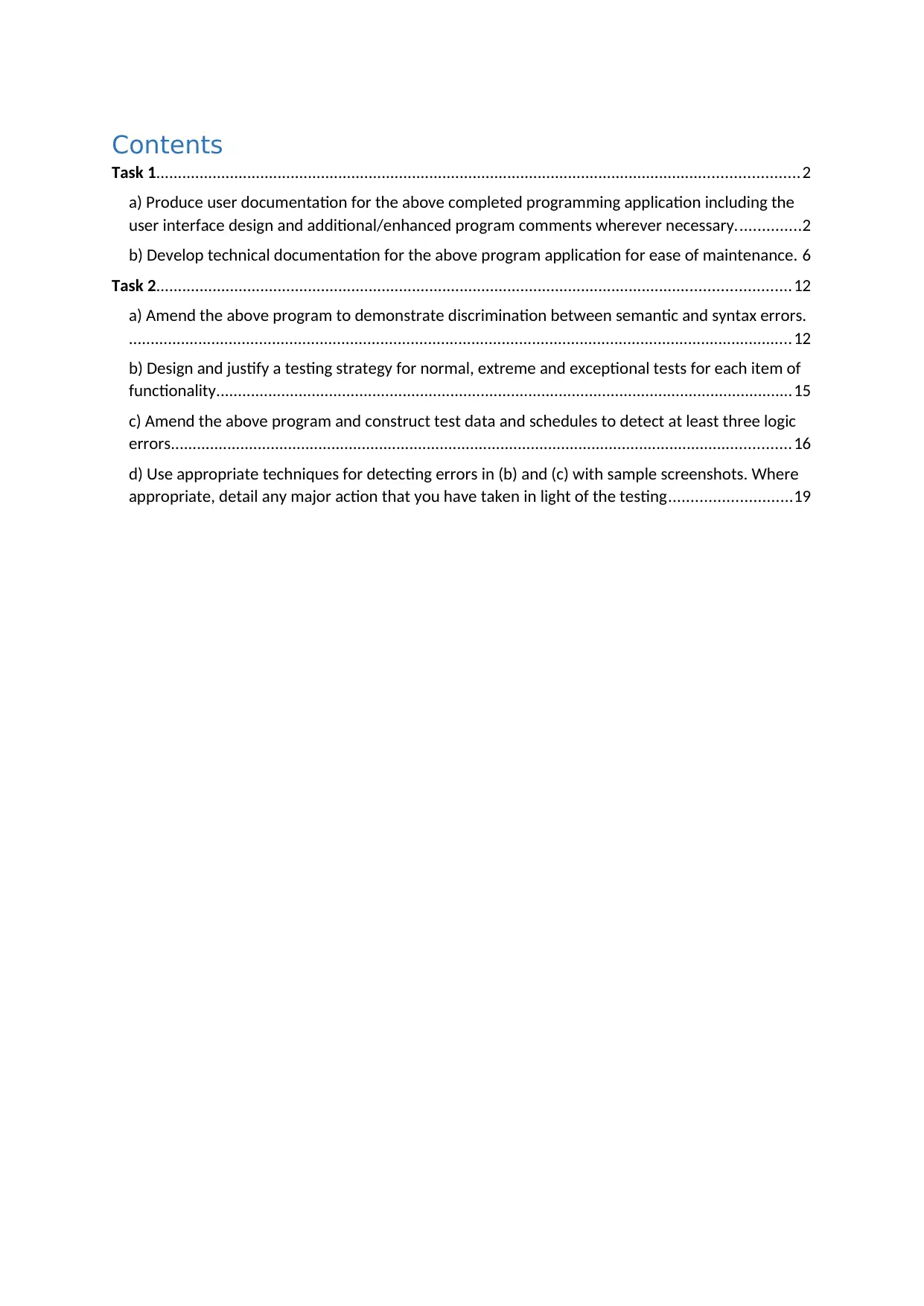
Contents
Task 1....................................................................................................................................................2
a) Produce user documentation for the above completed programming application including the
user interface design and additional/enhanced program comments wherever necessary...............2
b) Develop technical documentation for the above program application for ease of maintenance. 6
Task 2..................................................................................................................................................12
a) Amend the above program to demonstrate discrimination between semantic and syntax errors.
.........................................................................................................................................................12
b) Design and justify a testing strategy for normal, extreme and exceptional tests for each item of
functionality.....................................................................................................................................15
c) Amend the above program and construct test data and schedules to detect at least three logic
errors...............................................................................................................................................16
d) Use appropriate techniques for detecting errors in (b) and (c) with sample screenshots. Where
appropriate, detail any major action that you have taken in light of the testing............................19
Task 1....................................................................................................................................................2
a) Produce user documentation for the above completed programming application including the
user interface design and additional/enhanced program comments wherever necessary...............2
b) Develop technical documentation for the above program application for ease of maintenance. 6
Task 2..................................................................................................................................................12
a) Amend the above program to demonstrate discrimination between semantic and syntax errors.
.........................................................................................................................................................12
b) Design and justify a testing strategy for normal, extreme and exceptional tests for each item of
functionality.....................................................................................................................................15
c) Amend the above program and construct test data and schedules to detect at least three logic
errors...............................................................................................................................................16
d) Use appropriate techniques for detecting errors in (b) and (c) with sample screenshots. Where
appropriate, detail any major action that you have taken in light of the testing............................19
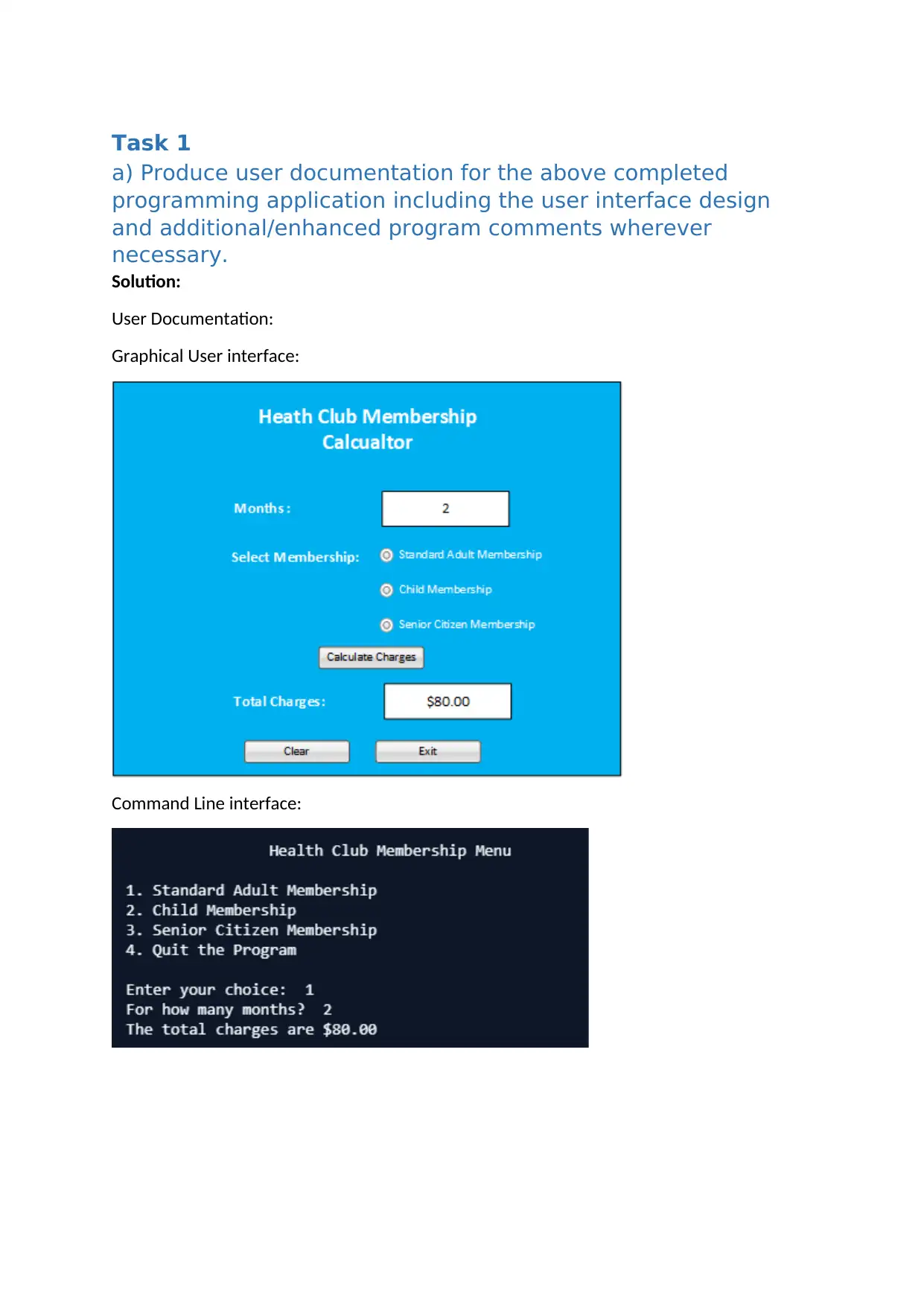
Task 1
a) Produce user documentation for the above completed
programming application including the user interface design
and additional/enhanced program comments wherever
necessary.
Solution:
User Documentation:
Graphical User interface:
Command Line interface:
a) Produce user documentation for the above completed
programming application including the user interface design
and additional/enhanced program comments wherever
necessary.
Solution:
User Documentation:
Graphical User interface:
Command Line interface:
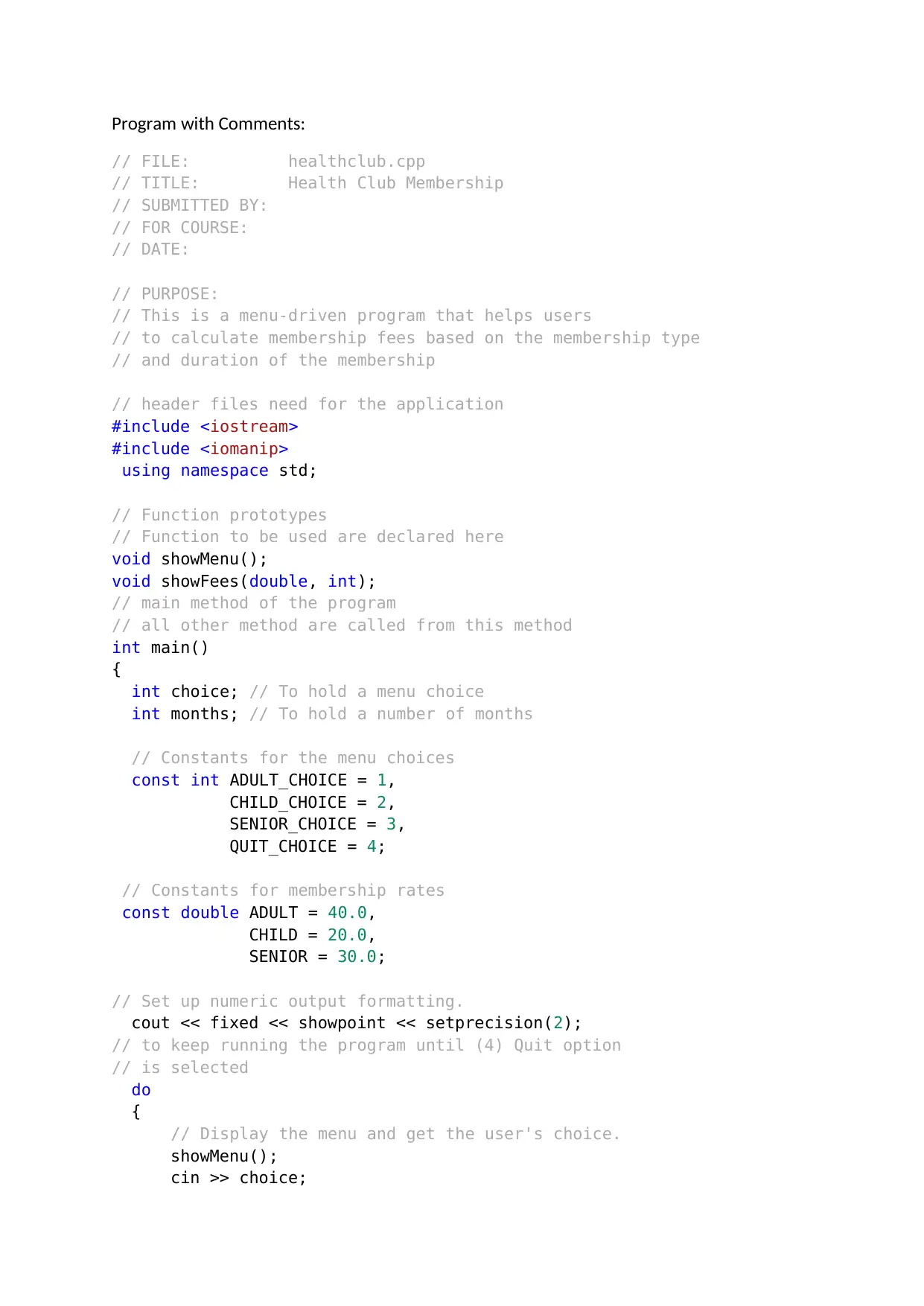
Program with Comments:
// FILE: healthclub.cpp
// TITLE: Health Club Membership
// SUBMITTED BY:
// FOR COURSE:
// DATE:
// PURPOSE:
// This is a menu-driven program that helps users
// to calculate membership fees based on the membership type
// and duration of the membership
// header files need for the application
#include <iostream>
#include <iomanip>
using namespace std;
// Function prototypes
// Function to be used are declared here
void showMenu();
void showFees(double, int);
// main method of the program
// all other method are called from this method
int main()
{
int choice; // To hold a menu choice
int months; // To hold a number of months
// Constants for the menu choices
const int ADULT_CHOICE = 1,
CHILD_CHOICE = 2,
SENIOR_CHOICE = 3,
QUIT_CHOICE = 4;
// Constants for membership rates
const double ADULT = 40.0,
CHILD = 20.0,
SENIOR = 30.0;
// Set up numeric output formatting.
cout << fixed << showpoint << setprecision(2);
// to keep running the program until (4) Quit option
// is selected
do
{
// Display the menu and get the user's choice.
showMenu();
cin >> choice;
// FILE: healthclub.cpp
// TITLE: Health Club Membership
// SUBMITTED BY:
// FOR COURSE:
// DATE:
// PURPOSE:
// This is a menu-driven program that helps users
// to calculate membership fees based on the membership type
// and duration of the membership
// header files need for the application
#include <iostream>
#include <iomanip>
using namespace std;
// Function prototypes
// Function to be used are declared here
void showMenu();
void showFees(double, int);
// main method of the program
// all other method are called from this method
int main()
{
int choice; // To hold a menu choice
int months; // To hold a number of months
// Constants for the menu choices
const int ADULT_CHOICE = 1,
CHILD_CHOICE = 2,
SENIOR_CHOICE = 3,
QUIT_CHOICE = 4;
// Constants for membership rates
const double ADULT = 40.0,
CHILD = 20.0,
SENIOR = 30.0;
// Set up numeric output formatting.
cout << fixed << showpoint << setprecision(2);
// to keep running the program until (4) Quit option
// is selected
do
{
// Display the menu and get the user's choice.
showMenu();
cin >> choice;
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
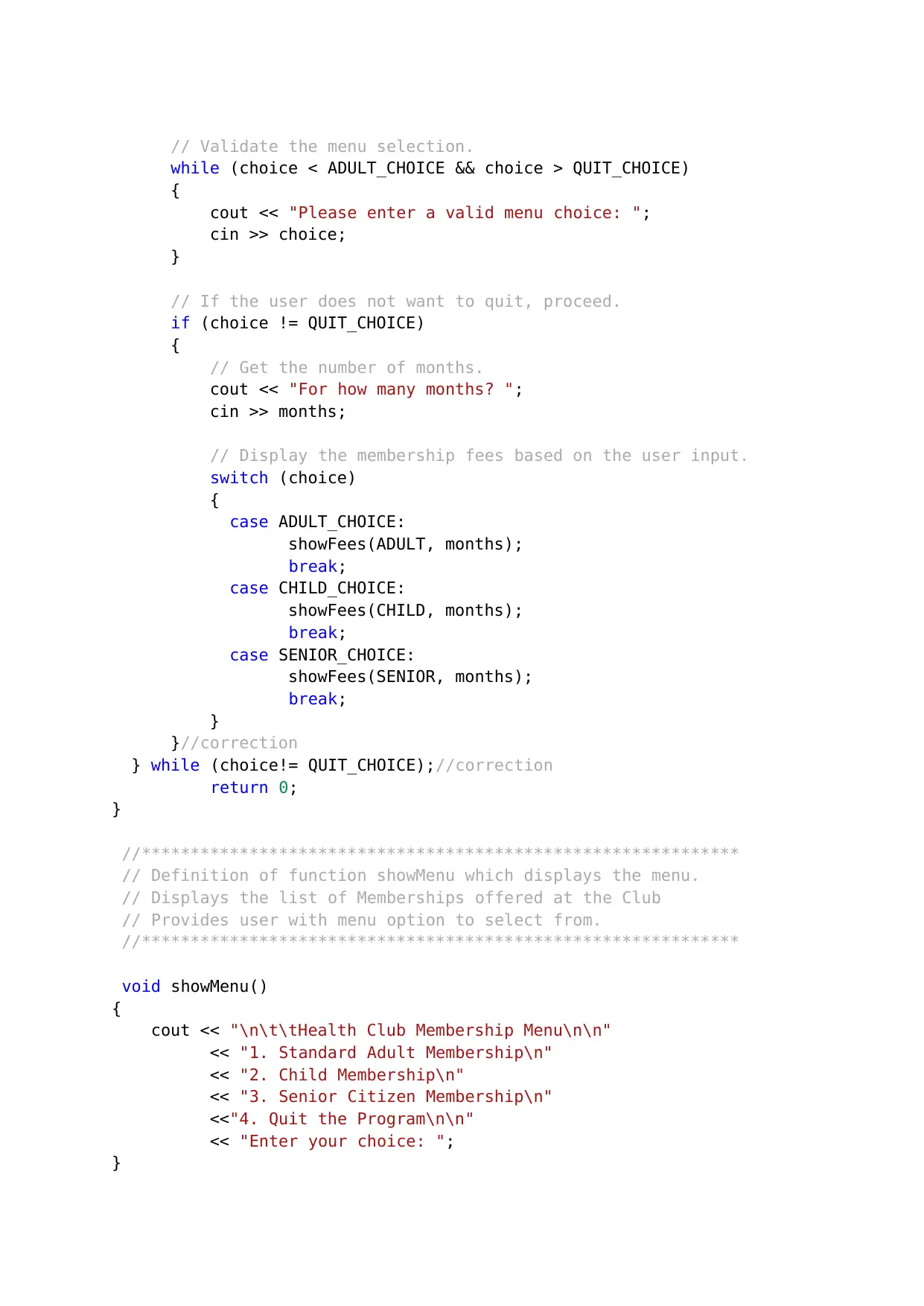
// Validate the menu selection.
while (choice < ADULT_CHOICE && choice > QUIT_CHOICE)
{
cout << "Please enter a valid menu choice: ";
cin >> choice;
}
// If the user does not want to quit, proceed.
if (choice != QUIT_CHOICE)
{
// Get the number of months.
cout << "For how many months? ";
cin >> months;
// Display the membership fees based on the user input.
switch (choice)
{
case ADULT_CHOICE:
showFees(ADULT, months);
break;
case CHILD_CHOICE:
showFees(CHILD, months);
break;
case SENIOR_CHOICE:
showFees(SENIOR, months);
break;
}
}//correction
} while (choice!= QUIT_CHOICE);//correction
return 0;
}
//*************************************************************
// Definition of function showMenu which displays the menu.
// Displays the list of Memberships offered at the Club
// Provides user with menu option to select from.
//*************************************************************
void showMenu()
{
cout << "\n\t\tHealth Club Membership Menu\n\n"
<< "1. Standard Adult Membership\n"
<< "2. Child Membership\n"
<< "3. Senior Citizen Membership\n"
<<"4. Quit the Program\n\n"
<< "Enter your choice: ";
}
while (choice < ADULT_CHOICE && choice > QUIT_CHOICE)
{
cout << "Please enter a valid menu choice: ";
cin >> choice;
}
// If the user does not want to quit, proceed.
if (choice != QUIT_CHOICE)
{
// Get the number of months.
cout << "For how many months? ";
cin >> months;
// Display the membership fees based on the user input.
switch (choice)
{
case ADULT_CHOICE:
showFees(ADULT, months);
break;
case CHILD_CHOICE:
showFees(CHILD, months);
break;
case SENIOR_CHOICE:
showFees(SENIOR, months);
break;
}
}//correction
} while (choice!= QUIT_CHOICE);//correction
return 0;
}
//*************************************************************
// Definition of function showMenu which displays the menu.
// Displays the list of Memberships offered at the Club
// Provides user with menu option to select from.
//*************************************************************
void showMenu()
{
cout << "\n\t\tHealth Club Membership Menu\n\n"
<< "1. Standard Adult Membership\n"
<< "2. Child Membership\n"
<< "3. Senior Citizen Membership\n"
<<"4. Quit the Program\n\n"
<< "Enter your choice: ";
}
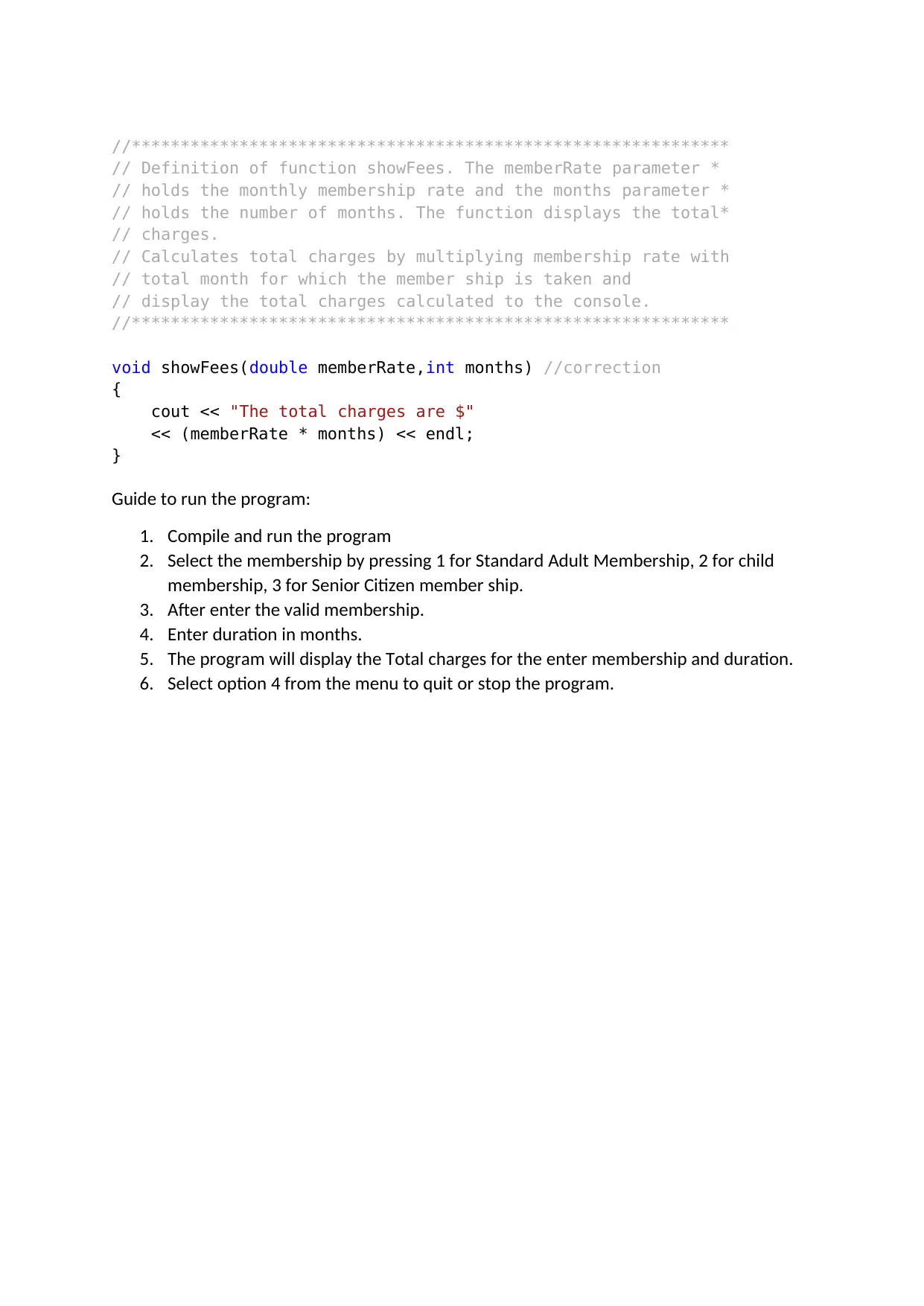
//*************************************************************
// Definition of function showFees. The memberRate parameter *
// holds the monthly membership rate and the months parameter *
// holds the number of months. The function displays the total*
// charges.
// Calculates total charges by multiplying membership rate with
// total month for which the member ship is taken and
// display the total charges calculated to the console.
//*************************************************************
void showFees(double memberRate,int months) //correction
{
cout << "The total charges are $"
<< (memberRate * months) << endl;
}
Guide to run the program:
1. Compile and run the program
2. Select the membership by pressing 1 for Standard Adult Membership, 2 for child
membership, 3 for Senior Citizen member ship.
3. After enter the valid membership.
4. Enter duration in months.
5. The program will display the Total charges for the enter membership and duration.
6. Select option 4 from the menu to quit or stop the program.
// Definition of function showFees. The memberRate parameter *
// holds the monthly membership rate and the months parameter *
// holds the number of months. The function displays the total*
// charges.
// Calculates total charges by multiplying membership rate with
// total month for which the member ship is taken and
// display the total charges calculated to the console.
//*************************************************************
void showFees(double memberRate,int months) //correction
{
cout << "The total charges are $"
<< (memberRate * months) << endl;
}
Guide to run the program:
1. Compile and run the program
2. Select the membership by pressing 1 for Standard Adult Membership, 2 for child
membership, 3 for Senior Citizen member ship.
3. After enter the valid membership.
4. Enter duration in months.
5. The program will display the Total charges for the enter membership and duration.
6. Select option 4 from the menu to quit or stop the program.
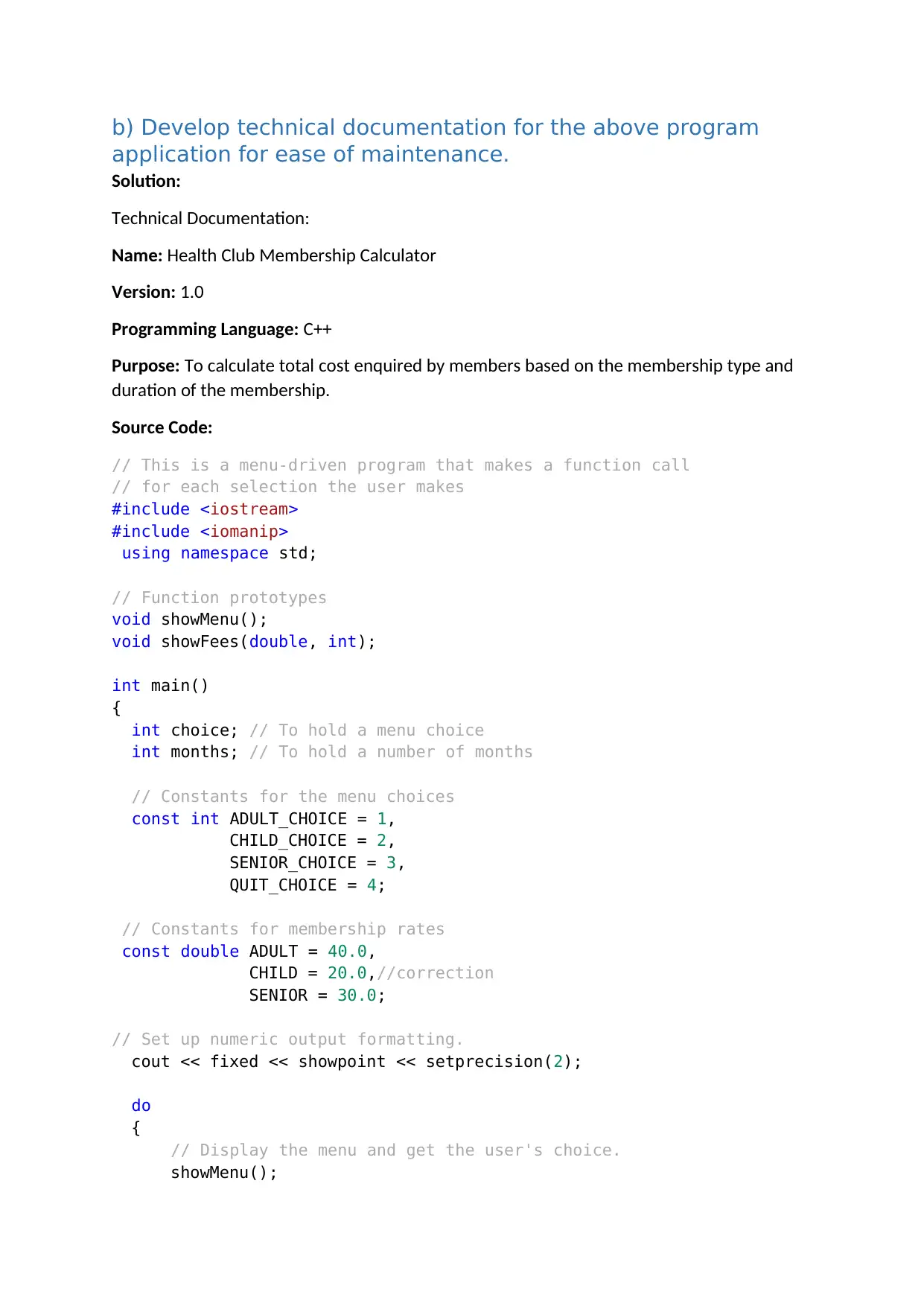
b) Develop technical documentation for the above program
application for ease of maintenance.
Solution:
Technical Documentation:
Name: Health Club Membership Calculator
Version: 1.0
Programming Language: C++
Purpose: To calculate total cost enquired by members based on the membership type and
duration of the membership.
Source Code:
// This is a menu-driven program that makes a function call
// for each selection the user makes
#include <iostream>
#include <iomanip>
using namespace std;
// Function prototypes
void showMenu();
void showFees(double, int);
int main()
{
int choice; // To hold a menu choice
int months; // To hold a number of months
// Constants for the menu choices
const int ADULT_CHOICE = 1,
CHILD_CHOICE = 2,
SENIOR_CHOICE = 3,
QUIT_CHOICE = 4;
// Constants for membership rates
const double ADULT = 40.0,
CHILD = 20.0,//correction
SENIOR = 30.0;
// Set up numeric output formatting.
cout << fixed << showpoint << setprecision(2);
do
{
// Display the menu and get the user's choice.
showMenu();
application for ease of maintenance.
Solution:
Technical Documentation:
Name: Health Club Membership Calculator
Version: 1.0
Programming Language: C++
Purpose: To calculate total cost enquired by members based on the membership type and
duration of the membership.
Source Code:
// This is a menu-driven program that makes a function call
// for each selection the user makes
#include <iostream>
#include <iomanip>
using namespace std;
// Function prototypes
void showMenu();
void showFees(double, int);
int main()
{
int choice; // To hold a menu choice
int months; // To hold a number of months
// Constants for the menu choices
const int ADULT_CHOICE = 1,
CHILD_CHOICE = 2,
SENIOR_CHOICE = 3,
QUIT_CHOICE = 4;
// Constants for membership rates
const double ADULT = 40.0,
CHILD = 20.0,//correction
SENIOR = 30.0;
// Set up numeric output formatting.
cout << fixed << showpoint << setprecision(2);
do
{
// Display the menu and get the user's choice.
showMenu();
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
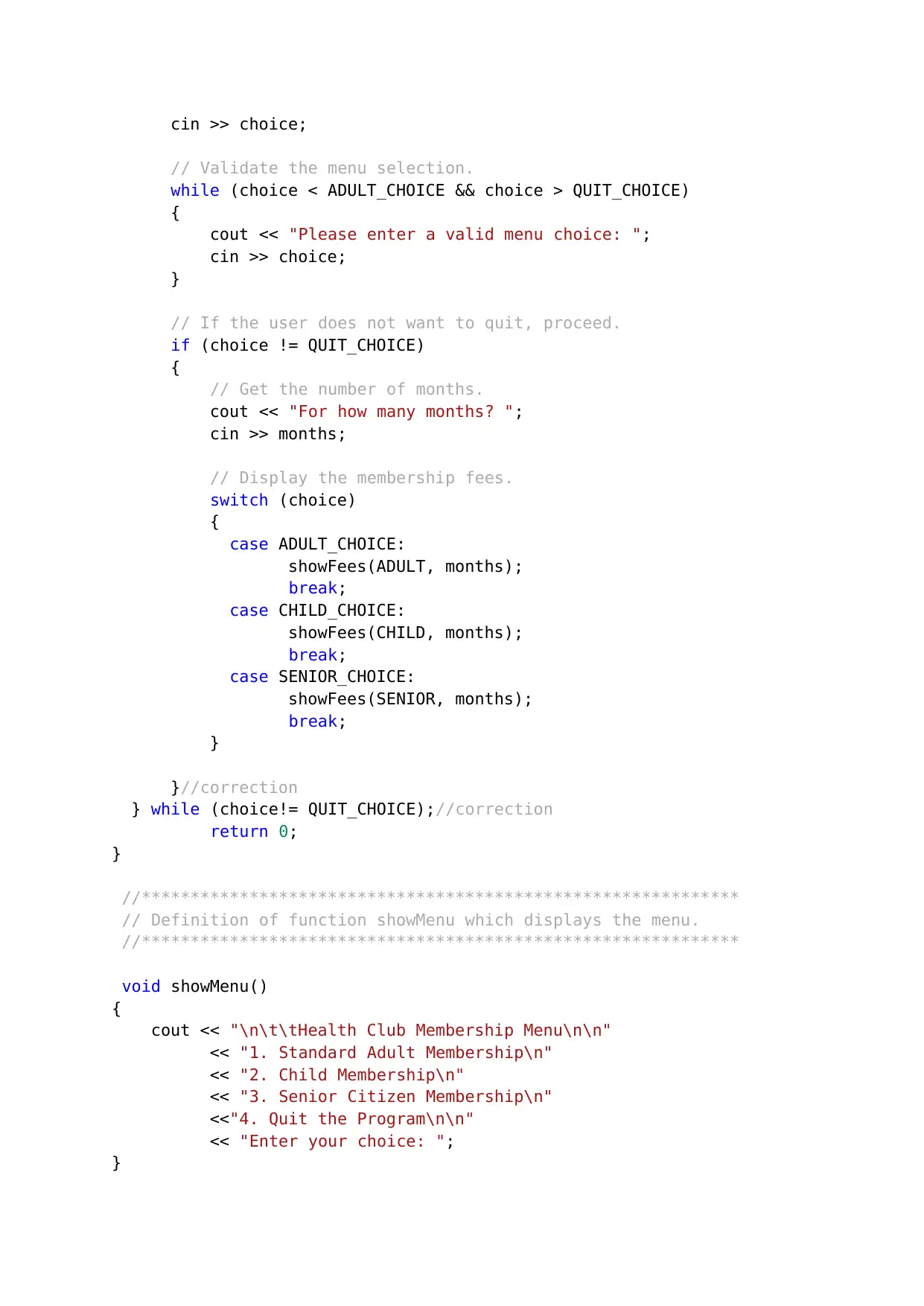
cin >> choice;
// Validate the menu selection.
while (choice < ADULT_CHOICE && choice > QUIT_CHOICE)
{
cout << "Please enter a valid menu choice: ";
cin >> choice;
}
// If the user does not want to quit, proceed.
if (choice != QUIT_CHOICE)
{
// Get the number of months.
cout << "For how many months? ";
cin >> months;
// Display the membership fees.
switch (choice)
{
case ADULT_CHOICE:
showFees(ADULT, months);
break;
case CHILD_CHOICE:
showFees(CHILD, months);
break;
case SENIOR_CHOICE:
showFees(SENIOR, months);
break;
}
}//correction
} while (choice!= QUIT_CHOICE);//correction
return 0;
}
//*************************************************************
// Definition of function showMenu which displays the menu.
//*************************************************************
void showMenu()
{
cout << "\n\t\tHealth Club Membership Menu\n\n"
<< "1. Standard Adult Membership\n"
<< "2. Child Membership\n"
<< "3. Senior Citizen Membership\n"
<<"4. Quit the Program\n\n"
<< "Enter your choice: ";
}
// Validate the menu selection.
while (choice < ADULT_CHOICE && choice > QUIT_CHOICE)
{
cout << "Please enter a valid menu choice: ";
cin >> choice;
}
// If the user does not want to quit, proceed.
if (choice != QUIT_CHOICE)
{
// Get the number of months.
cout << "For how many months? ";
cin >> months;
// Display the membership fees.
switch (choice)
{
case ADULT_CHOICE:
showFees(ADULT, months);
break;
case CHILD_CHOICE:
showFees(CHILD, months);
break;
case SENIOR_CHOICE:
showFees(SENIOR, months);
break;
}
}//correction
} while (choice!= QUIT_CHOICE);//correction
return 0;
}
//*************************************************************
// Definition of function showMenu which displays the menu.
//*************************************************************
void showMenu()
{
cout << "\n\t\tHealth Club Membership Menu\n\n"
<< "1. Standard Adult Membership\n"
<< "2. Child Membership\n"
<< "3. Senior Citizen Membership\n"
<<"4. Quit the Program\n\n"
<< "Enter your choice: ";
}
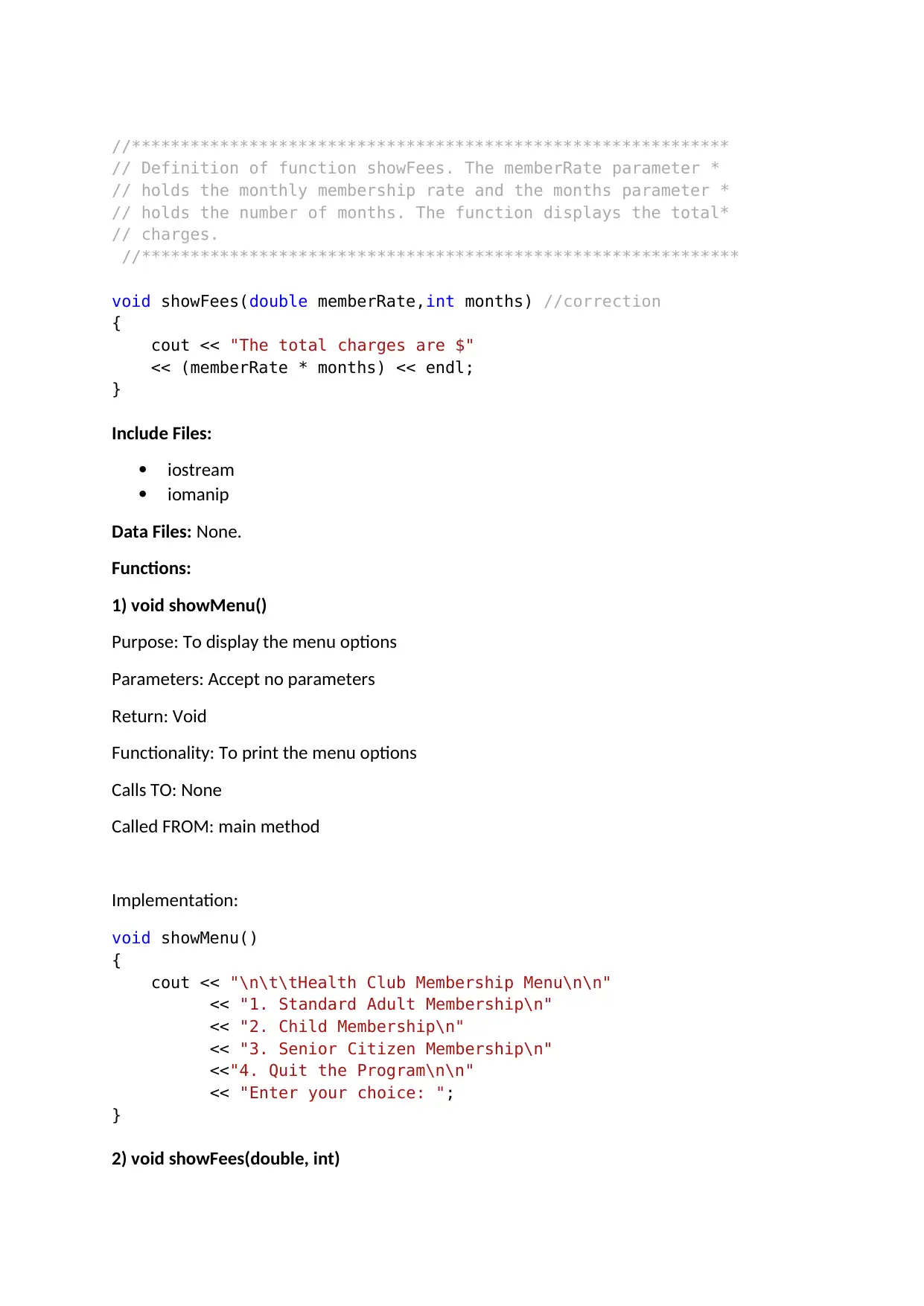
//*************************************************************
// Definition of function showFees. The memberRate parameter *
// holds the monthly membership rate and the months parameter *
// holds the number of months. The function displays the total*
// charges.
//*************************************************************
void showFees(double memberRate,int months) //correction
{
cout << "The total charges are $"
<< (memberRate * months) << endl;
}
Include Files:
ï‚· iostream
ï‚· iomanip
Data Files: None.
Functions:
1) void showMenu()
Purpose: To display the menu options
Parameters: Accept no parameters
Return: Void
Functionality: To print the menu options
Calls TO: None
Called FROM: main method
Implementation:
void showMenu()
{
cout << "\n\t\tHealth Club Membership Menu\n\n"
<< "1. Standard Adult Membership\n"
<< "2. Child Membership\n"
<< "3. Senior Citizen Membership\n"
<<"4. Quit the Program\n\n"
<< "Enter your choice: ";
}
2) void showFees(double, int)
// Definition of function showFees. The memberRate parameter *
// holds the monthly membership rate and the months parameter *
// holds the number of months. The function displays the total*
// charges.
//*************************************************************
void showFees(double memberRate,int months) //correction
{
cout << "The total charges are $"
<< (memberRate * months) << endl;
}
Include Files:
ï‚· iostream
ï‚· iomanip
Data Files: None.
Functions:
1) void showMenu()
Purpose: To display the menu options
Parameters: Accept no parameters
Return: Void
Functionality: To print the menu options
Calls TO: None
Called FROM: main method
Implementation:
void showMenu()
{
cout << "\n\t\tHealth Club Membership Menu\n\n"
<< "1. Standard Adult Membership\n"
<< "2. Child Membership\n"
<< "3. Senior Citizen Membership\n"
<<"4. Quit the Program\n\n"
<< "Enter your choice: ";
}
2) void showFees(double, int)
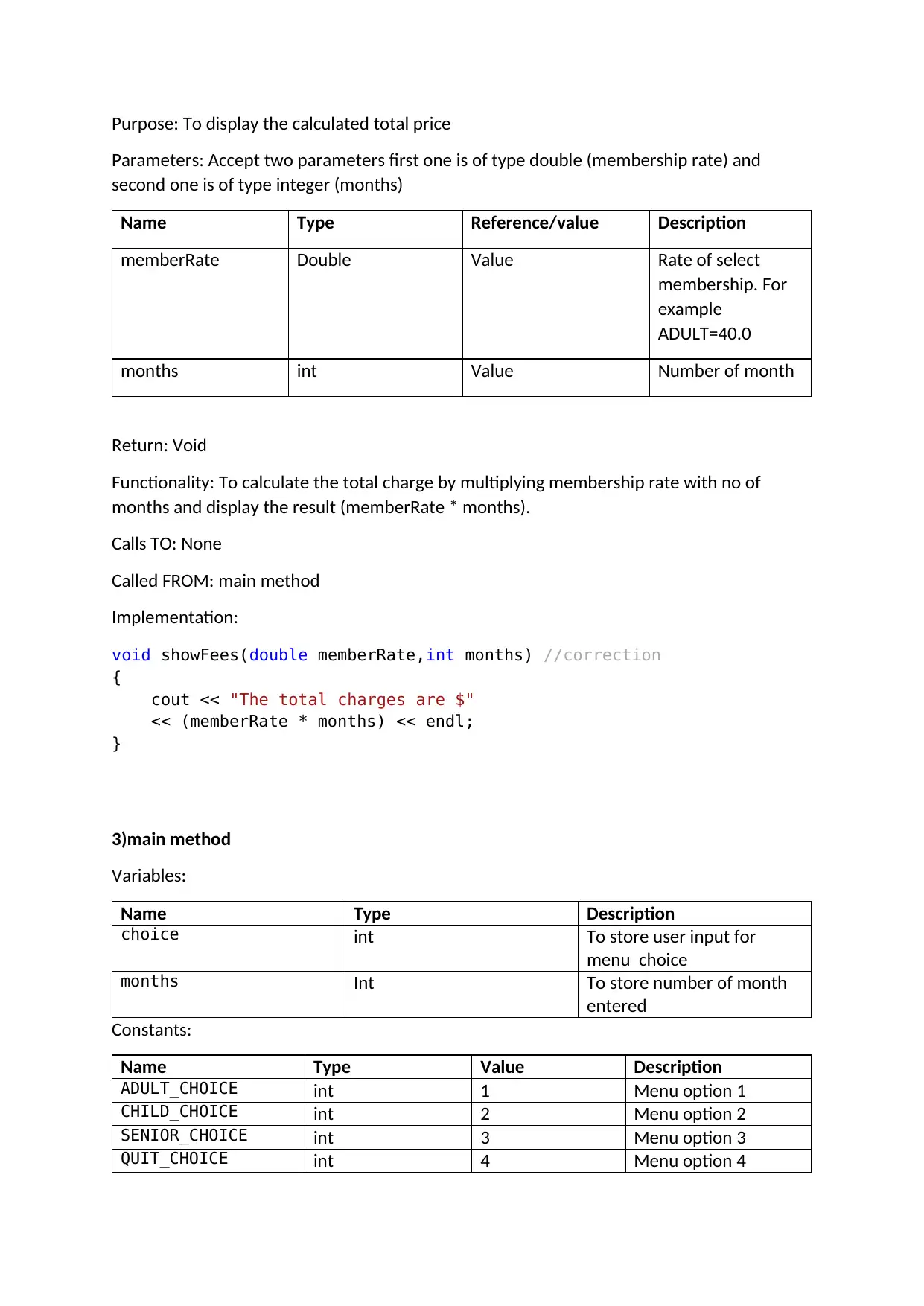
Purpose: To display the calculated total price
Parameters: Accept two parameters first one is of type double (membership rate) and
second one is of type integer (months)
Name Type Reference/value Description
memberRate Double Value Rate of select
membership. For
example
ADULT=40.0
months int Value Number of month
Return: Void
Functionality: To calculate the total charge by multiplying membership rate with no of
months and display the result (memberRate * months).
Calls TO: None
Called FROM: main method
Implementation:
void showFees(double memberRate,int months) //correction
{
cout << "The total charges are $"
<< (memberRate * months) << endl;
}
3)main method
Variables:
Name Type Description
choice int To store user input for
menu choice
months Int To store number of month
entered
Constants:
Name Type Value Description
ADULT_CHOICE int 1 Menu option 1
CHILD_CHOICE int 2 Menu option 2
SENIOR_CHOICE int 3 Menu option 3
QUIT_CHOICE int 4 Menu option 4
Parameters: Accept two parameters first one is of type double (membership rate) and
second one is of type integer (months)
Name Type Reference/value Description
memberRate Double Value Rate of select
membership. For
example
ADULT=40.0
months int Value Number of month
Return: Void
Functionality: To calculate the total charge by multiplying membership rate with no of
months and display the result (memberRate * months).
Calls TO: None
Called FROM: main method
Implementation:
void showFees(double memberRate,int months) //correction
{
cout << "The total charges are $"
<< (memberRate * months) << endl;
}
3)main method
Variables:
Name Type Description
choice int To store user input for
menu choice
months Int To store number of month
entered
Constants:
Name Type Value Description
ADULT_CHOICE int 1 Menu option 1
CHILD_CHOICE int 2 Menu option 2
SENIOR_CHOICE int 3 Menu option 3
QUIT_CHOICE int 4 Menu option 4
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
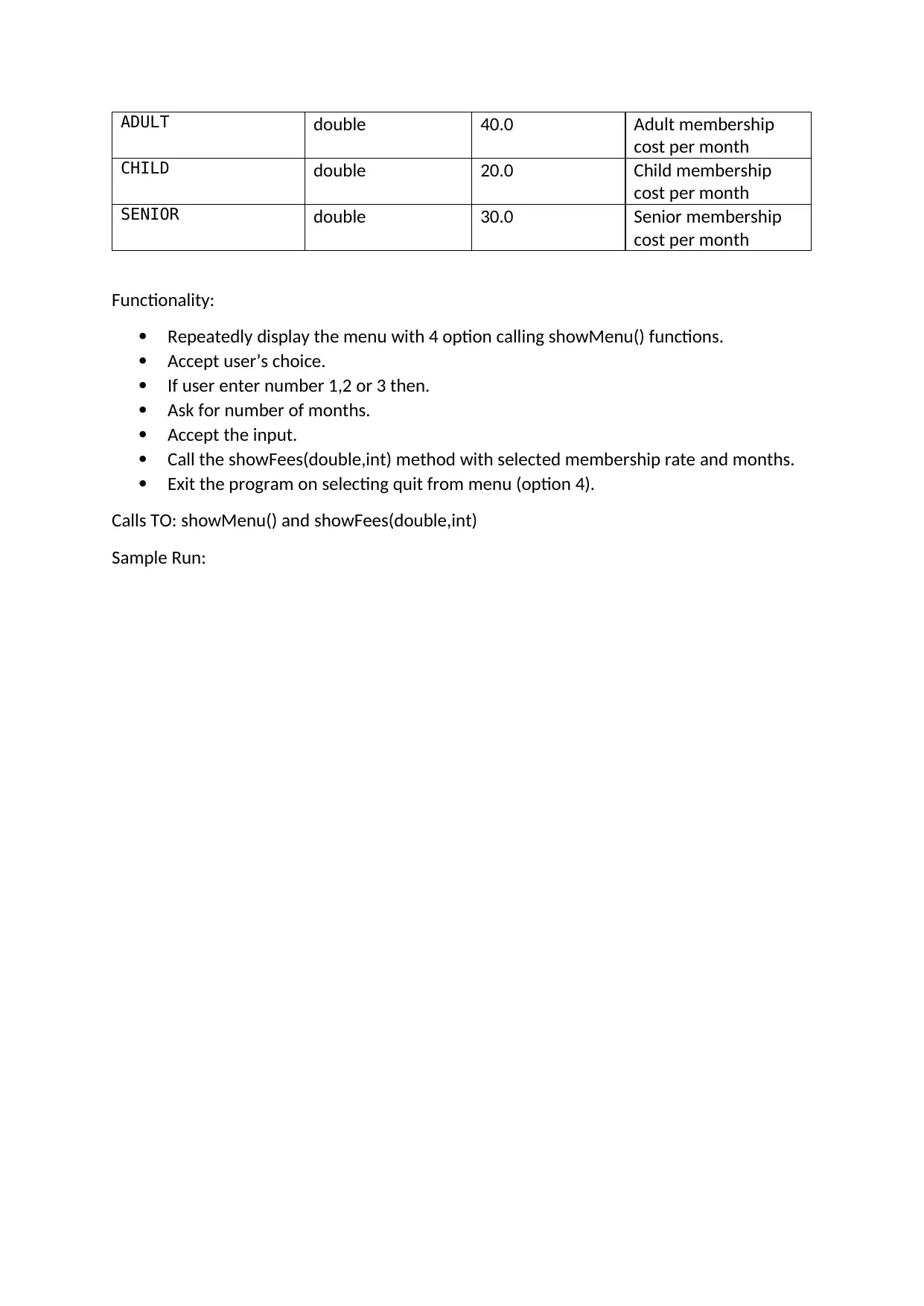
ADULT double 40.0 Adult membership
cost per month
CHILD double 20.0 Child membership
cost per month
SENIOR double 30.0 Senior membership
cost per month
Functionality:
ï‚· Repeatedly display the menu with 4 option calling showMenu() functions.
 Accept user’s choice.
ï‚· If user enter number 1,2 or 3 then.
ï‚· Ask for number of months.
ï‚· Accept the input.
ï‚· Call the showFees(double,int) method with selected membership rate and months.
ï‚· Exit the program on selecting quit from menu (option 4).
Calls TO: showMenu() and showFees(double,int)
Sample Run:
cost per month
CHILD double 20.0 Child membership
cost per month
SENIOR double 30.0 Senior membership
cost per month
Functionality:
ï‚· Repeatedly display the menu with 4 option calling showMenu() functions.
 Accept user’s choice.
ï‚· If user enter number 1,2 or 3 then.
ï‚· Ask for number of months.
ï‚· Accept the input.
ï‚· Call the showFees(double,int) method with selected membership rate and months.
ï‚· Exit the program on selecting quit from menu (option 4).
Calls TO: showMenu() and showFees(double,int)
Sample Run:
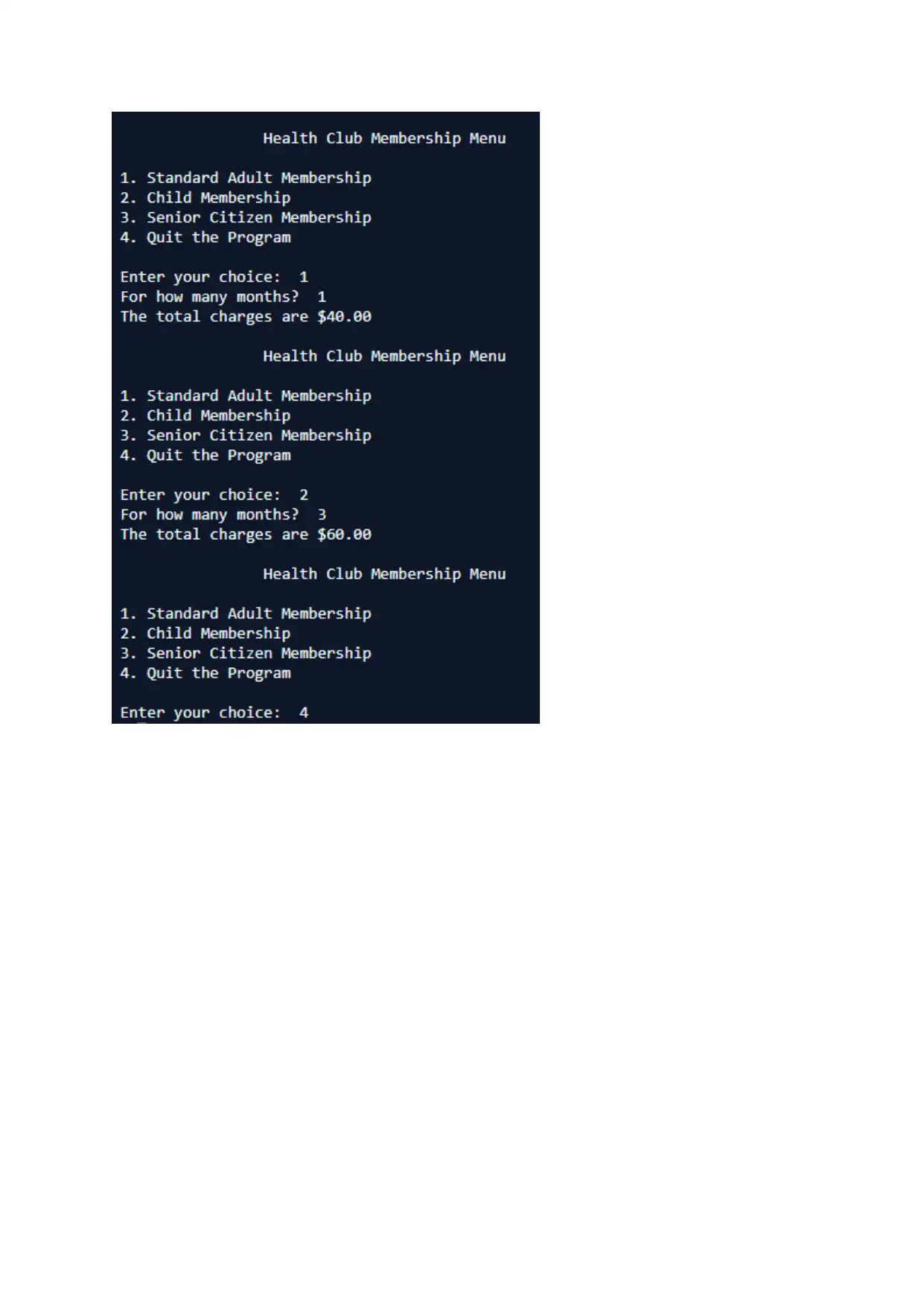
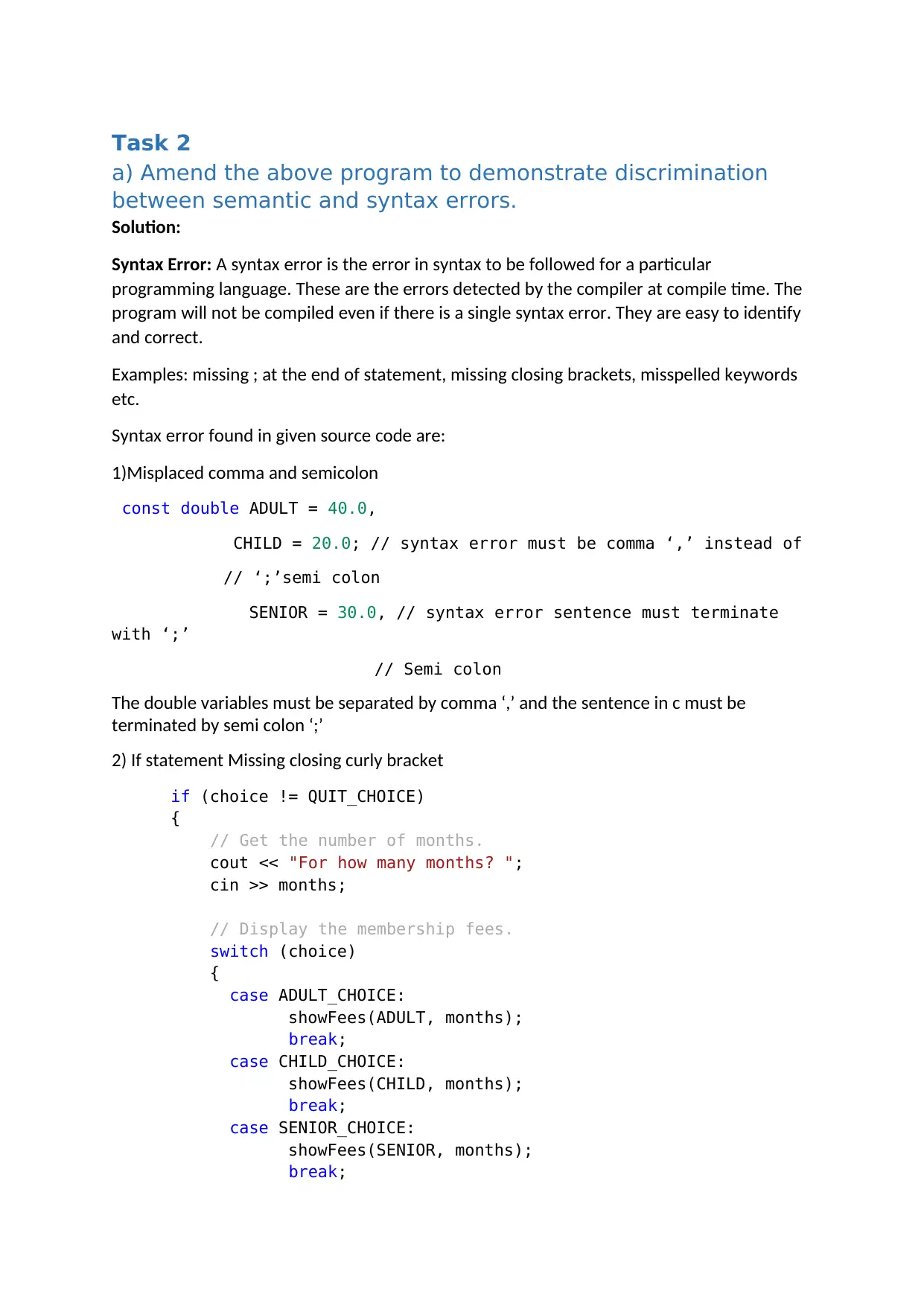
Task 2
a) Amend the above program to demonstrate discrimination
between semantic and syntax errors.
Solution:
Syntax Error: A syntax error is the error in syntax to be followed for a particular
programming language. These are the errors detected by the compiler at compile time. The
program will not be compiled even if there is a single syntax error. They are easy to identify
and correct.
Examples: missing ; at the end of statement, missing closing brackets, misspelled keywords
etc.
Syntax error found in given source code are:
1)Misplaced comma and semicolon
const double ADULT = 40.0,
CHILD = 20.0; // syntax error must be comma ‘,’ instead of
// ‘;’semi colon
SENIOR = 30.0, // syntax error sentence must terminate
with ‘;’
// Semi colon
The double variables must be separated by comma ‘,’ and the sentence in c must be
terminated by semi colon ‘;’
2) If statement Missing closing curly bracket
if (choice != QUIT_CHOICE)
{
// Get the number of months.
cout << "For how many months? ";
cin >> months;
// Display the membership fees.
switch (choice)
{
case ADULT_CHOICE:
showFees(ADULT, months);
break;
case CHILD_CHOICE:
showFees(CHILD, months);
break;
case SENIOR_CHOICE:
showFees(SENIOR, months);
break;
a) Amend the above program to demonstrate discrimination
between semantic and syntax errors.
Solution:
Syntax Error: A syntax error is the error in syntax to be followed for a particular
programming language. These are the errors detected by the compiler at compile time. The
program will not be compiled even if there is a single syntax error. They are easy to identify
and correct.
Examples: missing ; at the end of statement, missing closing brackets, misspelled keywords
etc.
Syntax error found in given source code are:
1)Misplaced comma and semicolon
const double ADULT = 40.0,
CHILD = 20.0; // syntax error must be comma ‘,’ instead of
// ‘;’semi colon
SENIOR = 30.0, // syntax error sentence must terminate
with ‘;’
// Semi colon
The double variables must be separated by comma ‘,’ and the sentence in c must be
terminated by semi colon ‘;’
2) If statement Missing closing curly bracket
if (choice != QUIT_CHOICE)
{
// Get the number of months.
cout << "For how many months? ";
cin >> months;
// Display the membership fees.
switch (choice)
{
case ADULT_CHOICE:
showFees(ADULT, months);
break;
case CHILD_CHOICE:
showFees(CHILD, months);
break;
case SENIOR_CHOICE:
showFees(SENIOR, months);
break;
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
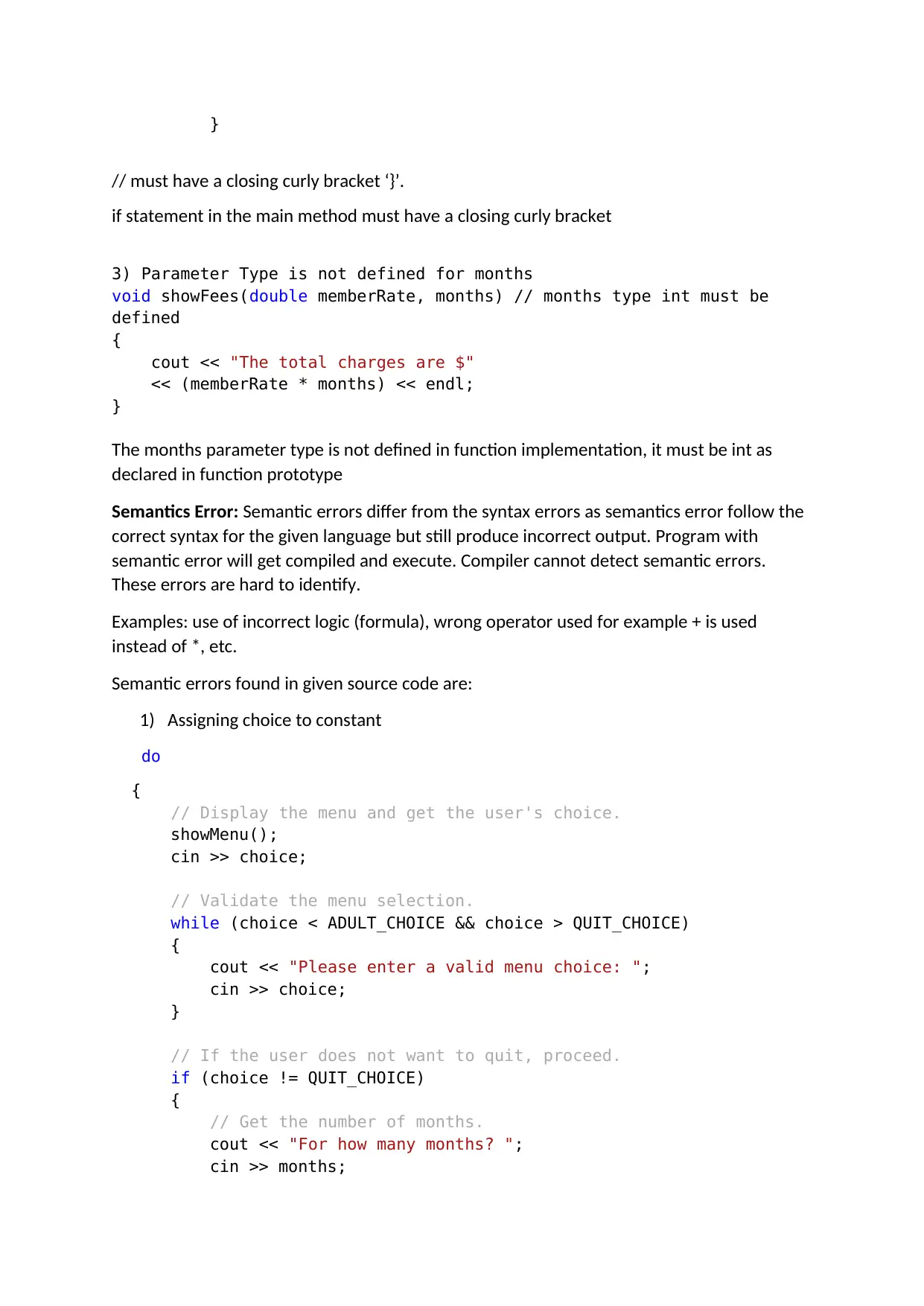
}
// must have a closing curly bracket ‘}’.
if statement in the main method must have a closing curly bracket
3) Parameter Type is not defined for months
void showFees(double memberRate, months) // months type int must be
defined
{
cout << "The total charges are $"
<< (memberRate * months) << endl;
}
The months parameter type is not defined in function implementation, it must be int as
declared in function prototype
Semantics Error: Semantic errors differ from the syntax errors as semantics error follow the
correct syntax for the given language but still produce incorrect output. Program with
semantic error will get compiled and execute. Compiler cannot detect semantic errors.
These errors are hard to identify.
Examples: use of incorrect logic (formula), wrong operator used for example + is used
instead of *, etc.
Semantic errors found in given source code are:
1) Assigning choice to constant
do
{
// Display the menu and get the user's choice.
showMenu();
cin >> choice;
// Validate the menu selection.
while (choice < ADULT_CHOICE && choice > QUIT_CHOICE)
{
cout << "Please enter a valid menu choice: ";
cin >> choice;
}
// If the user does not want to quit, proceed.
if (choice != QUIT_CHOICE)
{
// Get the number of months.
cout << "For how many months? ";
cin >> months;
// must have a closing curly bracket ‘}’.
if statement in the main method must have a closing curly bracket
3) Parameter Type is not defined for months
void showFees(double memberRate, months) // months type int must be
defined
{
cout << "The total charges are $"
<< (memberRate * months) << endl;
}
The months parameter type is not defined in function implementation, it must be int as
declared in function prototype
Semantics Error: Semantic errors differ from the syntax errors as semantics error follow the
correct syntax for the given language but still produce incorrect output. Program with
semantic error will get compiled and execute. Compiler cannot detect semantic errors.
These errors are hard to identify.
Examples: use of incorrect logic (formula), wrong operator used for example + is used
instead of *, etc.
Semantic errors found in given source code are:
1) Assigning choice to constant
do
{
// Display the menu and get the user's choice.
showMenu();
cin >> choice;
// Validate the menu selection.
while (choice < ADULT_CHOICE && choice > QUIT_CHOICE)
{
cout << "Please enter a valid menu choice: ";
cin >> choice;
}
// If the user does not want to quit, proceed.
if (choice != QUIT_CHOICE)
{
// Get the number of months.
cout << "For how many months? ";
cin >> months;
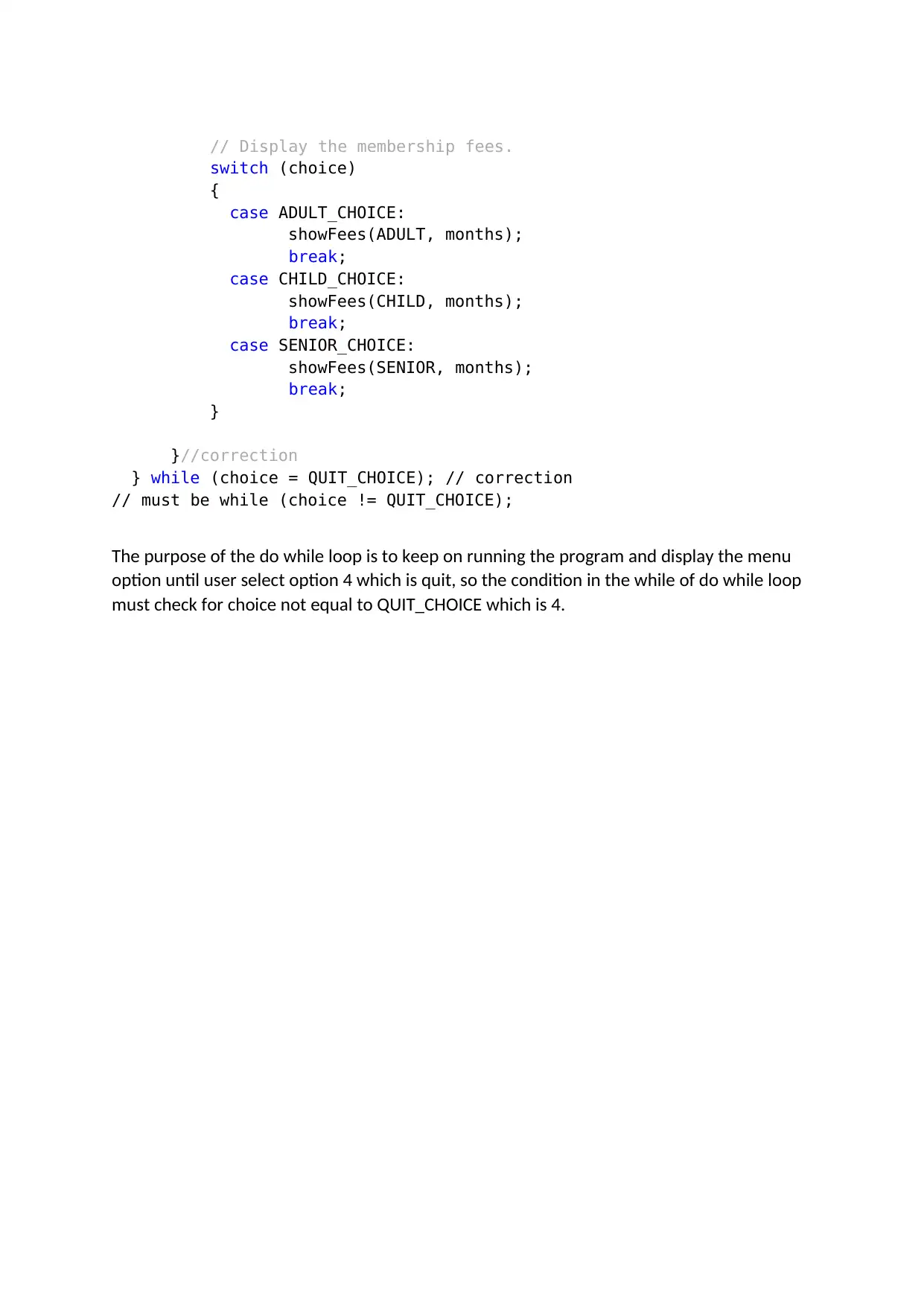
// Display the membership fees.
switch (choice)
{
case ADULT_CHOICE:
showFees(ADULT, months);
break;
case CHILD_CHOICE:
showFees(CHILD, months);
break;
case SENIOR_CHOICE:
showFees(SENIOR, months);
break;
}
}//correction
} while (choice = QUIT_CHOICE); // correction
// must be while (choice != QUIT_CHOICE);
The purpose of the do while loop is to keep on running the program and display the menu
option until user select option 4 which is quit, so the condition in the while of do while loop
must check for choice not equal to QUIT_CHOICE which is 4.
switch (choice)
{
case ADULT_CHOICE:
showFees(ADULT, months);
break;
case CHILD_CHOICE:
showFees(CHILD, months);
break;
case SENIOR_CHOICE:
showFees(SENIOR, months);
break;
}
}//correction
} while (choice = QUIT_CHOICE); // correction
// must be while (choice != QUIT_CHOICE);
The purpose of the do while loop is to keep on running the program and display the menu
option until user select option 4 which is quit, so the condition in the while of do while loop
must check for choice not equal to QUIT_CHOICE which is 4.
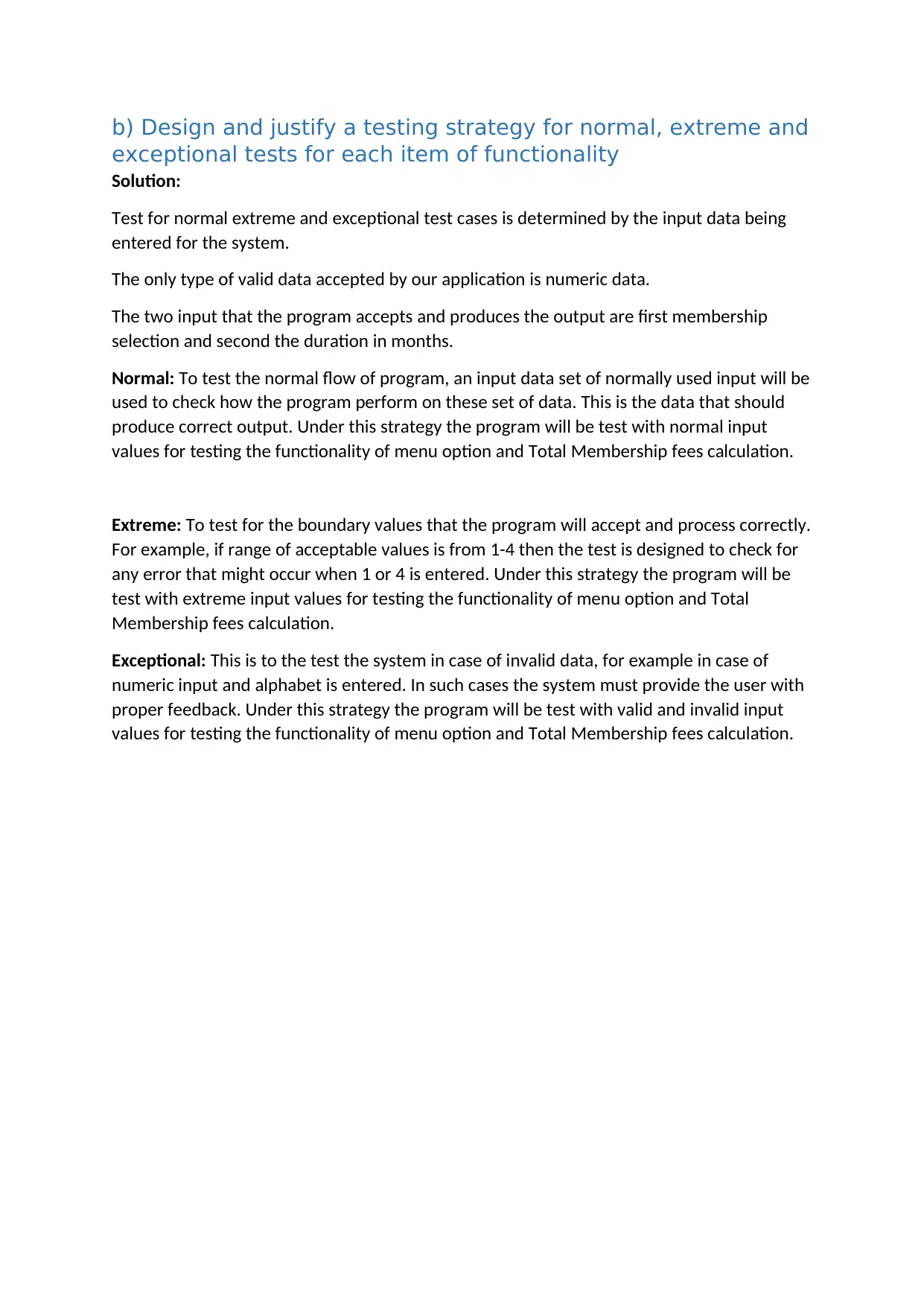
b) Design and justify a testing strategy for normal, extreme and
exceptional tests for each item of functionality
Solution:
Test for normal extreme and exceptional test cases is determined by the input data being
entered for the system.
The only type of valid data accepted by our application is numeric data.
The two input that the program accepts and produces the output are first membership
selection and second the duration in months.
Normal: To test the normal flow of program, an input data set of normally used input will be
used to check how the program perform on these set of data. This is the data that should
produce correct output. Under this strategy the program will be test with normal input
values for testing the functionality of menu option and Total Membership fees calculation.
Extreme: To test for the boundary values that the program will accept and process correctly.
For example, if range of acceptable values is from 1-4 then the test is designed to check for
any error that might occur when 1 or 4 is entered. Under this strategy the program will be
test with extreme input values for testing the functionality of menu option and Total
Membership fees calculation.
Exceptional: This is to the test the system in case of invalid data, for example in case of
numeric input and alphabet is entered. In such cases the system must provide the user with
proper feedback. Under this strategy the program will be test with valid and invalid input
values for testing the functionality of menu option and Total Membership fees calculation.
exceptional tests for each item of functionality
Solution:
Test for normal extreme and exceptional test cases is determined by the input data being
entered for the system.
The only type of valid data accepted by our application is numeric data.
The two input that the program accepts and produces the output are first membership
selection and second the duration in months.
Normal: To test the normal flow of program, an input data set of normally used input will be
used to check how the program perform on these set of data. This is the data that should
produce correct output. Under this strategy the program will be test with normal input
values for testing the functionality of menu option and Total Membership fees calculation.
Extreme: To test for the boundary values that the program will accept and process correctly.
For example, if range of acceptable values is from 1-4 then the test is designed to check for
any error that might occur when 1 or 4 is entered. Under this strategy the program will be
test with extreme input values for testing the functionality of menu option and Total
Membership fees calculation.
Exceptional: This is to the test the system in case of invalid data, for example in case of
numeric input and alphabet is entered. In such cases the system must provide the user with
proper feedback. Under this strategy the program will be test with valid and invalid input
values for testing the functionality of menu option and Total Membership fees calculation.
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
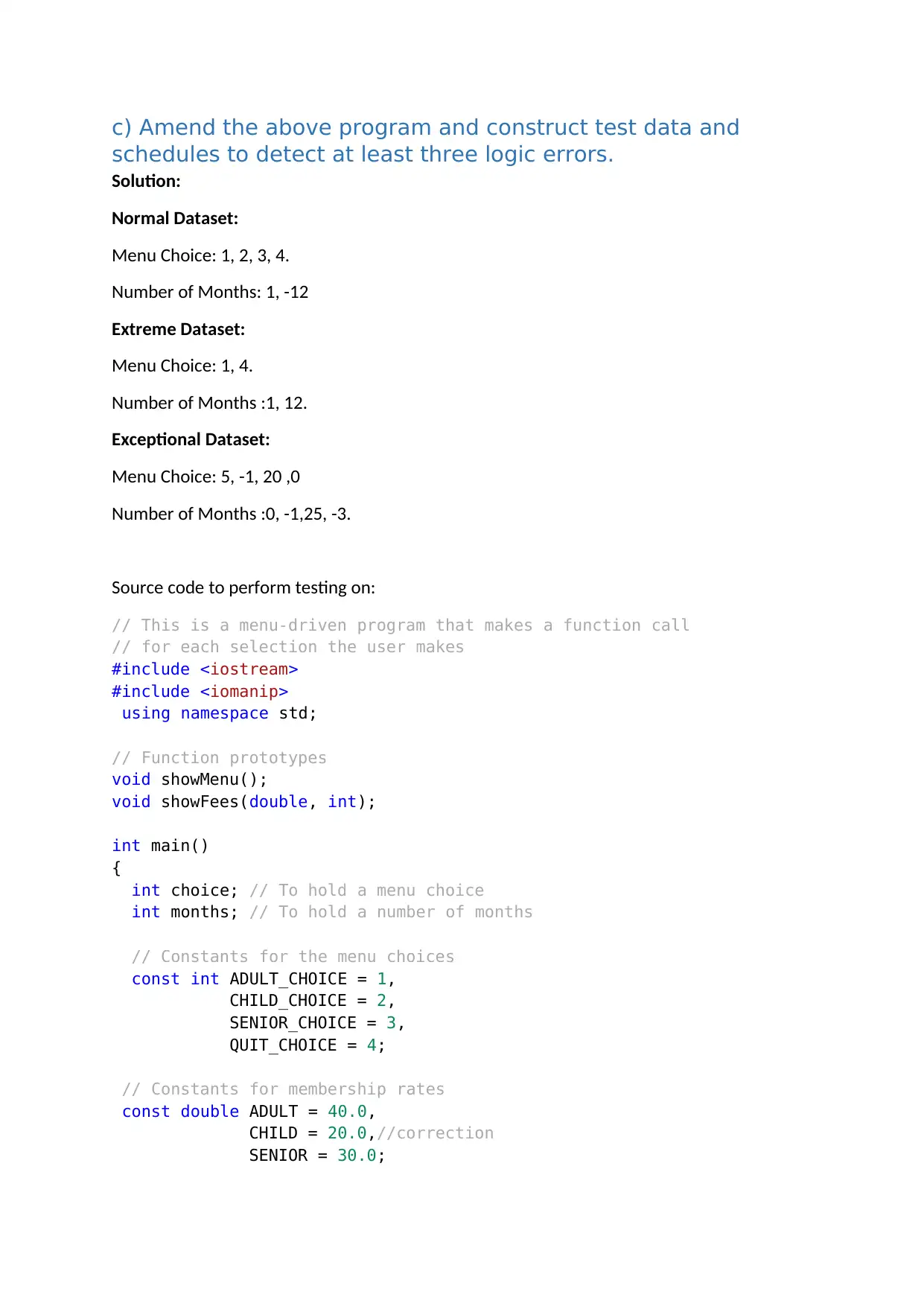
c) Amend the above program and construct test data and
schedules to detect at least three logic errors.
Solution:
Normal Dataset:
Menu Choice: 1, 2, 3, 4.
Number of Months: 1, -12
Extreme Dataset:
Menu Choice: 1, 4.
Number of Months :1, 12.
Exceptional Dataset:
Menu Choice: 5, -1, 20 ,0
Number of Months :0, -1,25, -3.
Source code to perform testing on:
// This is a menu-driven program that makes a function call
// for each selection the user makes
#include <iostream>
#include <iomanip>
using namespace std;
// Function prototypes
void showMenu();
void showFees(double, int);
int main()
{
int choice; // To hold a menu choice
int months; // To hold a number of months
// Constants for the menu choices
const int ADULT_CHOICE = 1,
CHILD_CHOICE = 2,
SENIOR_CHOICE = 3,
QUIT_CHOICE = 4;
// Constants for membership rates
const double ADULT = 40.0,
CHILD = 20.0,//correction
SENIOR = 30.0;
schedules to detect at least three logic errors.
Solution:
Normal Dataset:
Menu Choice: 1, 2, 3, 4.
Number of Months: 1, -12
Extreme Dataset:
Menu Choice: 1, 4.
Number of Months :1, 12.
Exceptional Dataset:
Menu Choice: 5, -1, 20 ,0
Number of Months :0, -1,25, -3.
Source code to perform testing on:
// This is a menu-driven program that makes a function call
// for each selection the user makes
#include <iostream>
#include <iomanip>
using namespace std;
// Function prototypes
void showMenu();
void showFees(double, int);
int main()
{
int choice; // To hold a menu choice
int months; // To hold a number of months
// Constants for the menu choices
const int ADULT_CHOICE = 1,
CHILD_CHOICE = 2,
SENIOR_CHOICE = 3,
QUIT_CHOICE = 4;
// Constants for membership rates
const double ADULT = 40.0,
CHILD = 20.0,//correction
SENIOR = 30.0;
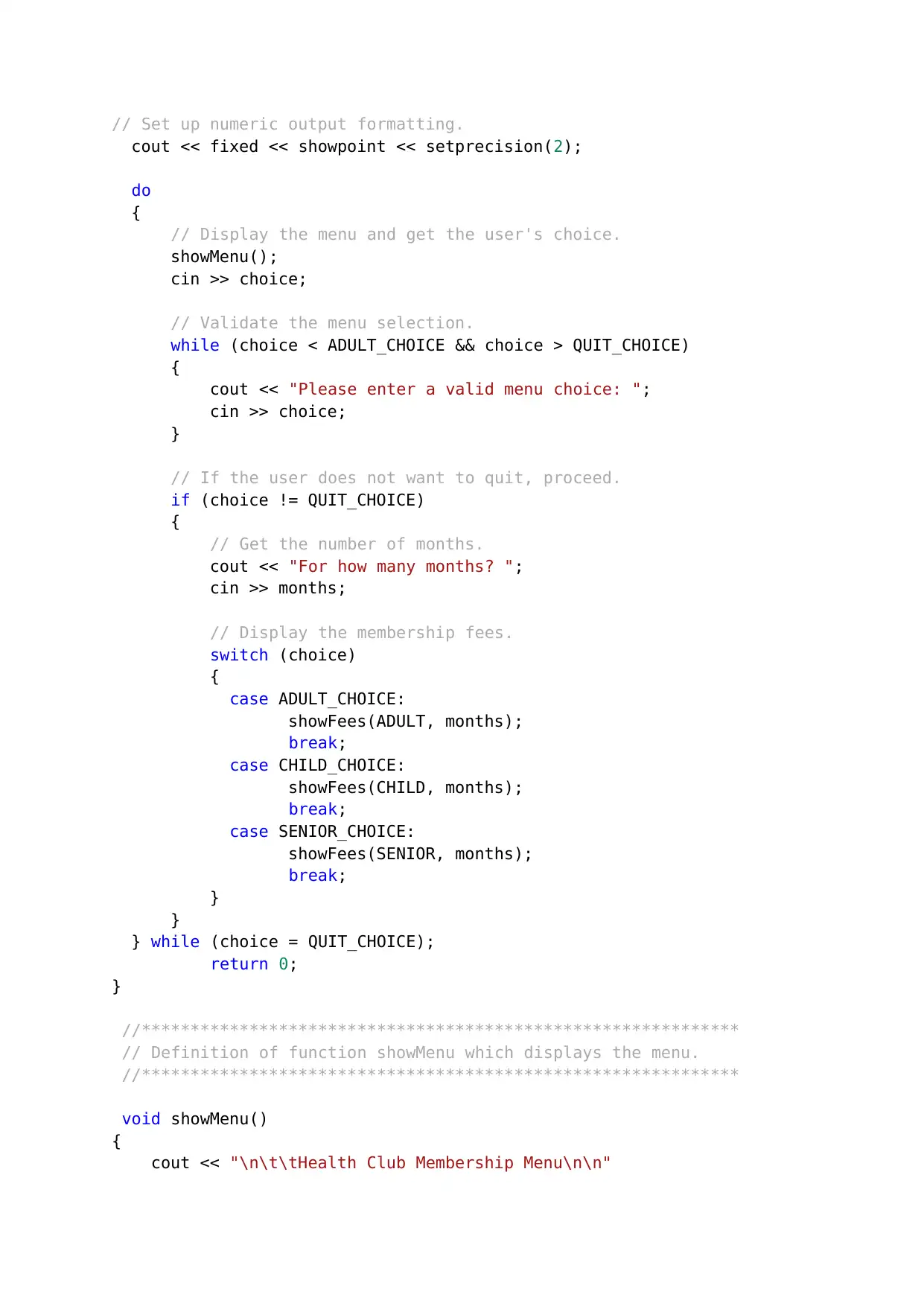
// Set up numeric output formatting.
cout << fixed << showpoint << setprecision(2);
do
{
// Display the menu and get the user's choice.
showMenu();
cin >> choice;
// Validate the menu selection.
while (choice < ADULT_CHOICE && choice > QUIT_CHOICE)
{
cout << "Please enter a valid menu choice: ";
cin >> choice;
}
// If the user does not want to quit, proceed.
if (choice != QUIT_CHOICE)
{
// Get the number of months.
cout << "For how many months? ";
cin >> months;
// Display the membership fees.
switch (choice)
{
case ADULT_CHOICE:
showFees(ADULT, months);
break;
case CHILD_CHOICE:
showFees(CHILD, months);
break;
case SENIOR_CHOICE:
showFees(SENIOR, months);
break;
}
}
} while (choice = QUIT_CHOICE);
return 0;
}
//*************************************************************
// Definition of function showMenu which displays the menu.
//*************************************************************
void showMenu()
{
cout << "\n\t\tHealth Club Membership Menu\n\n"
cout << fixed << showpoint << setprecision(2);
do
{
// Display the menu and get the user's choice.
showMenu();
cin >> choice;
// Validate the menu selection.
while (choice < ADULT_CHOICE && choice > QUIT_CHOICE)
{
cout << "Please enter a valid menu choice: ";
cin >> choice;
}
// If the user does not want to quit, proceed.
if (choice != QUIT_CHOICE)
{
// Get the number of months.
cout << "For how many months? ";
cin >> months;
// Display the membership fees.
switch (choice)
{
case ADULT_CHOICE:
showFees(ADULT, months);
break;
case CHILD_CHOICE:
showFees(CHILD, months);
break;
case SENIOR_CHOICE:
showFees(SENIOR, months);
break;
}
}
} while (choice = QUIT_CHOICE);
return 0;
}
//*************************************************************
// Definition of function showMenu which displays the menu.
//*************************************************************
void showMenu()
{
cout << "\n\t\tHealth Club Membership Menu\n\n"
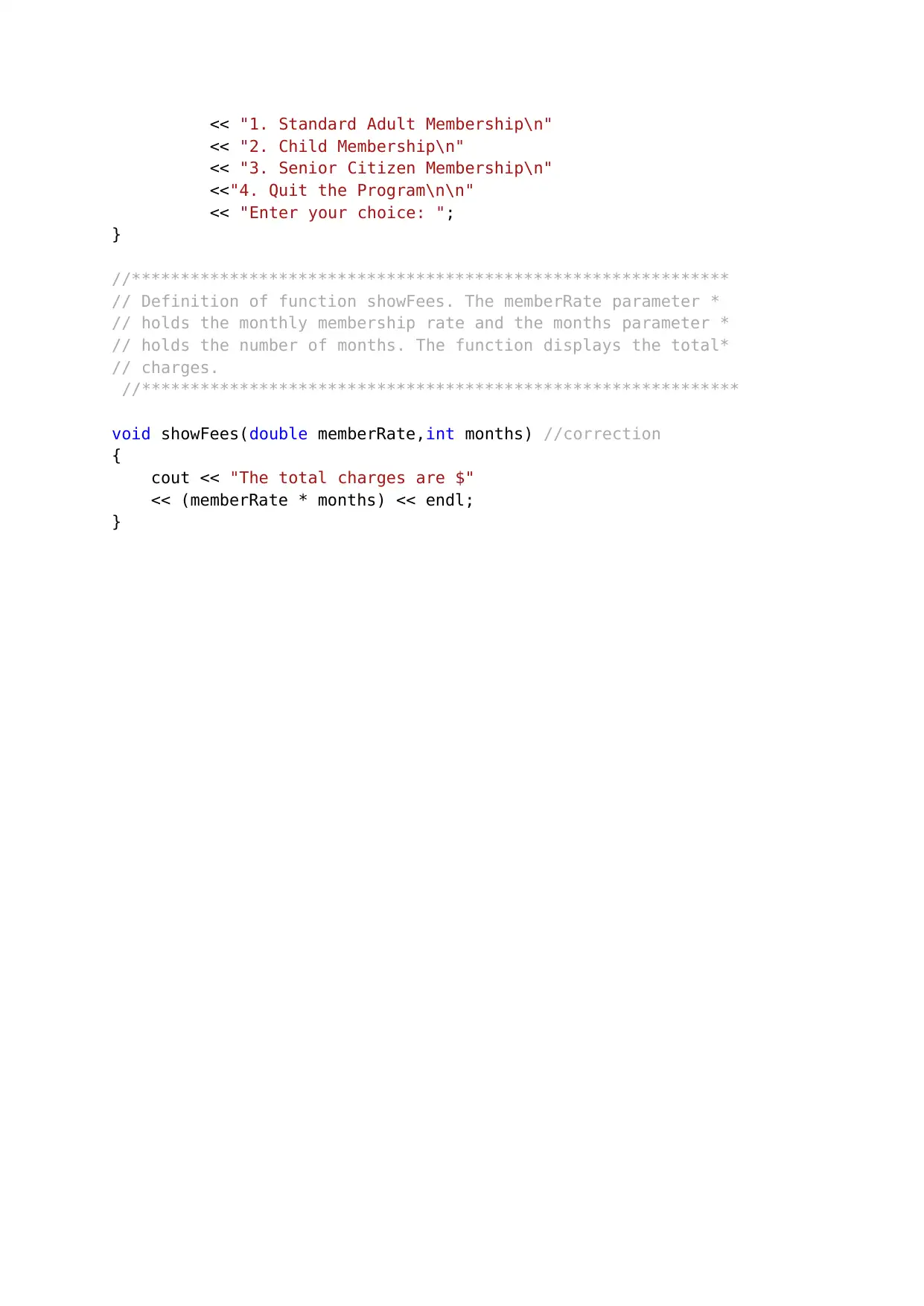
<< "1. Standard Adult Membership\n"
<< "2. Child Membership\n"
<< "3. Senior Citizen Membership\n"
<<"4. Quit the Program\n\n"
<< "Enter your choice: ";
}
//*************************************************************
// Definition of function showFees. The memberRate parameter *
// holds the monthly membership rate and the months parameter *
// holds the number of months. The function displays the total*
// charges.
//*************************************************************
void showFees(double memberRate,int months) //correction
{
cout << "The total charges are $"
<< (memberRate * months) << endl;
}
<< "2. Child Membership\n"
<< "3. Senior Citizen Membership\n"
<<"4. Quit the Program\n\n"
<< "Enter your choice: ";
}
//*************************************************************
// Definition of function showFees. The memberRate parameter *
// holds the monthly membership rate and the months parameter *
// holds the number of months. The function displays the total*
// charges.
//*************************************************************
void showFees(double memberRate,int months) //correction
{
cout << "The total charges are $"
<< (memberRate * months) << endl;
}
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
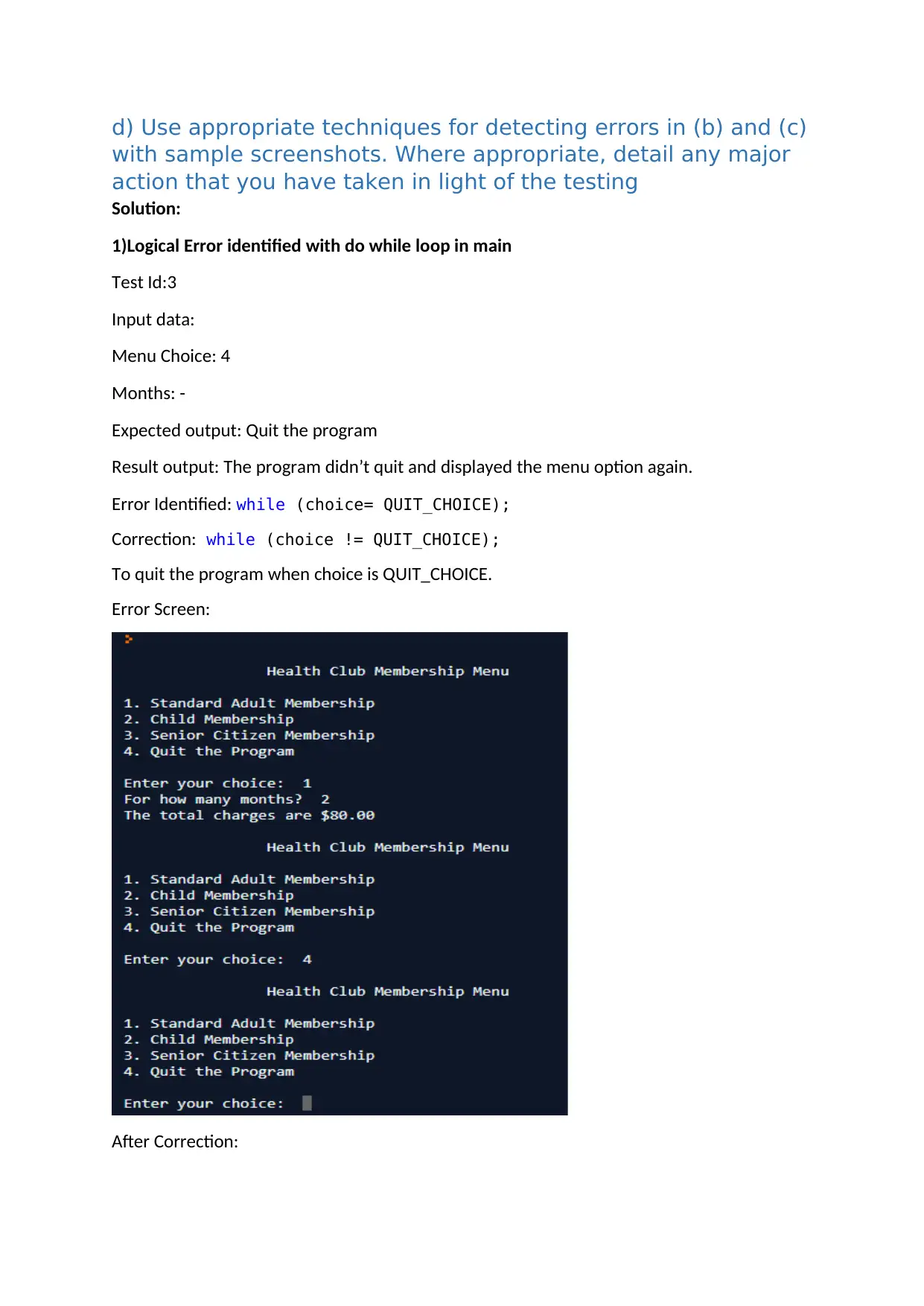
d) Use appropriate techniques for detecting errors in (b) and (c)
with sample screenshots. Where appropriate, detail any major
action that you have taken in light of the testing
Solution:
1)Logical Error identified with do while loop in main
Test Id:3
Input data:
Menu Choice: 4
Months: -
Expected output: Quit the program
Result output: The program didn’t quit and displayed the menu option again.
Error Identified: while (choice= QUIT_CHOICE);
Correction: while (choice != QUIT_CHOICE);
To quit the program when choice is QUIT_CHOICE.
Error Screen:
After Correction:
with sample screenshots. Where appropriate, detail any major
action that you have taken in light of the testing
Solution:
1)Logical Error identified with do while loop in main
Test Id:3
Input data:
Menu Choice: 4
Months: -
Expected output: Quit the program
Result output: The program didn’t quit and displayed the menu option again.
Error Identified: while (choice= QUIT_CHOICE);
Correction: while (choice != QUIT_CHOICE);
To quit the program when choice is QUIT_CHOICE.
Error Screen:
After Correction:
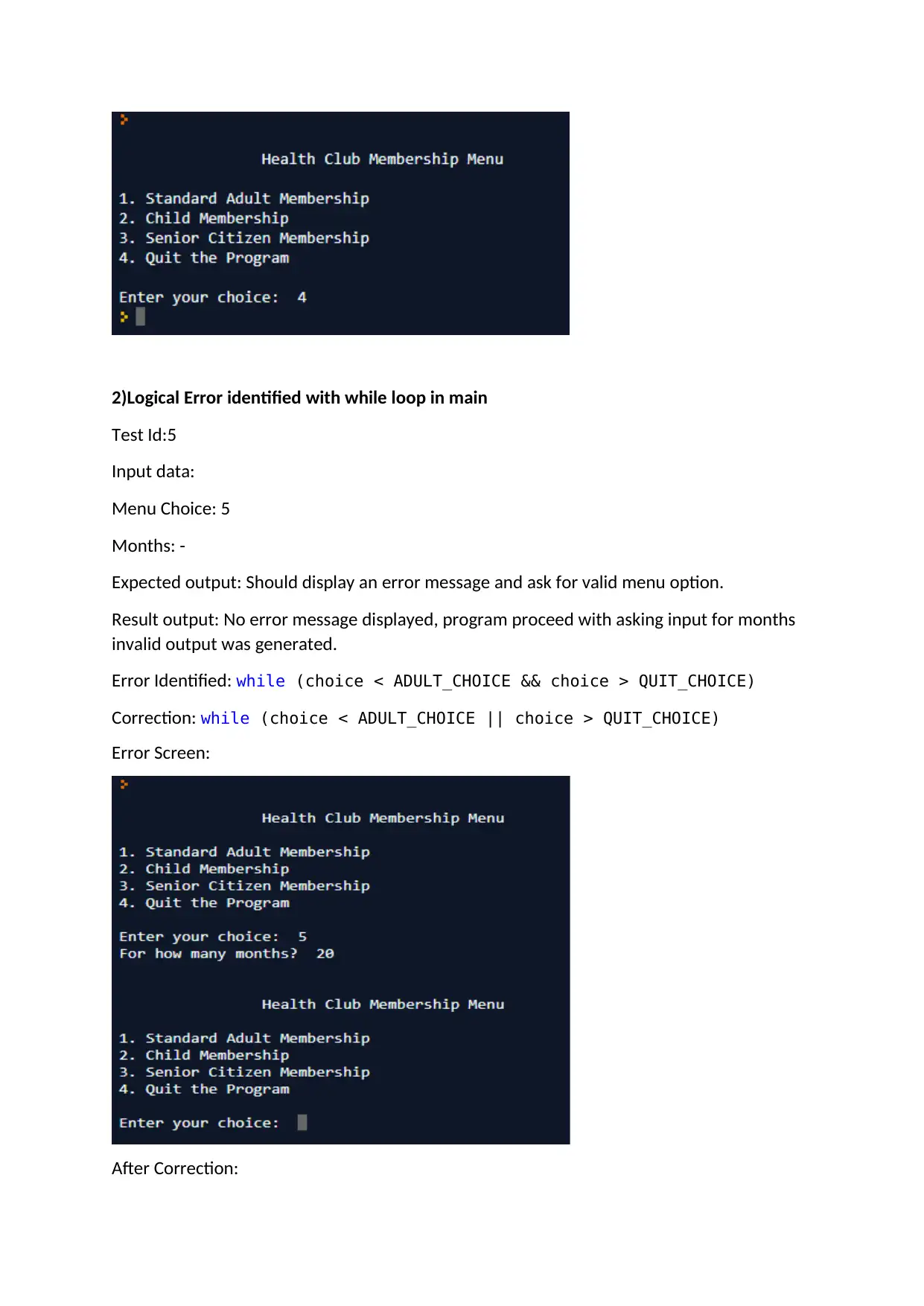
2)Logical Error identified with while loop in main
Test Id:5
Input data:
Menu Choice: 5
Months: -
Expected output: Should display an error message and ask for valid menu option.
Result output: No error message displayed, program proceed with asking input for months
invalid output was generated.
Error Identified: while (choice < ADULT_CHOICE && choice > QUIT_CHOICE)
Correction: while (choice < ADULT_CHOICE || choice > QUIT_CHOICE)
Error Screen:
After Correction:
Test Id:5
Input data:
Menu Choice: 5
Months: -
Expected output: Should display an error message and ask for valid menu option.
Result output: No error message displayed, program proceed with asking input for months
invalid output was generated.
Error Identified: while (choice < ADULT_CHOICE && choice > QUIT_CHOICE)
Correction: while (choice < ADULT_CHOICE || choice > QUIT_CHOICE)
Error Screen:
After Correction:
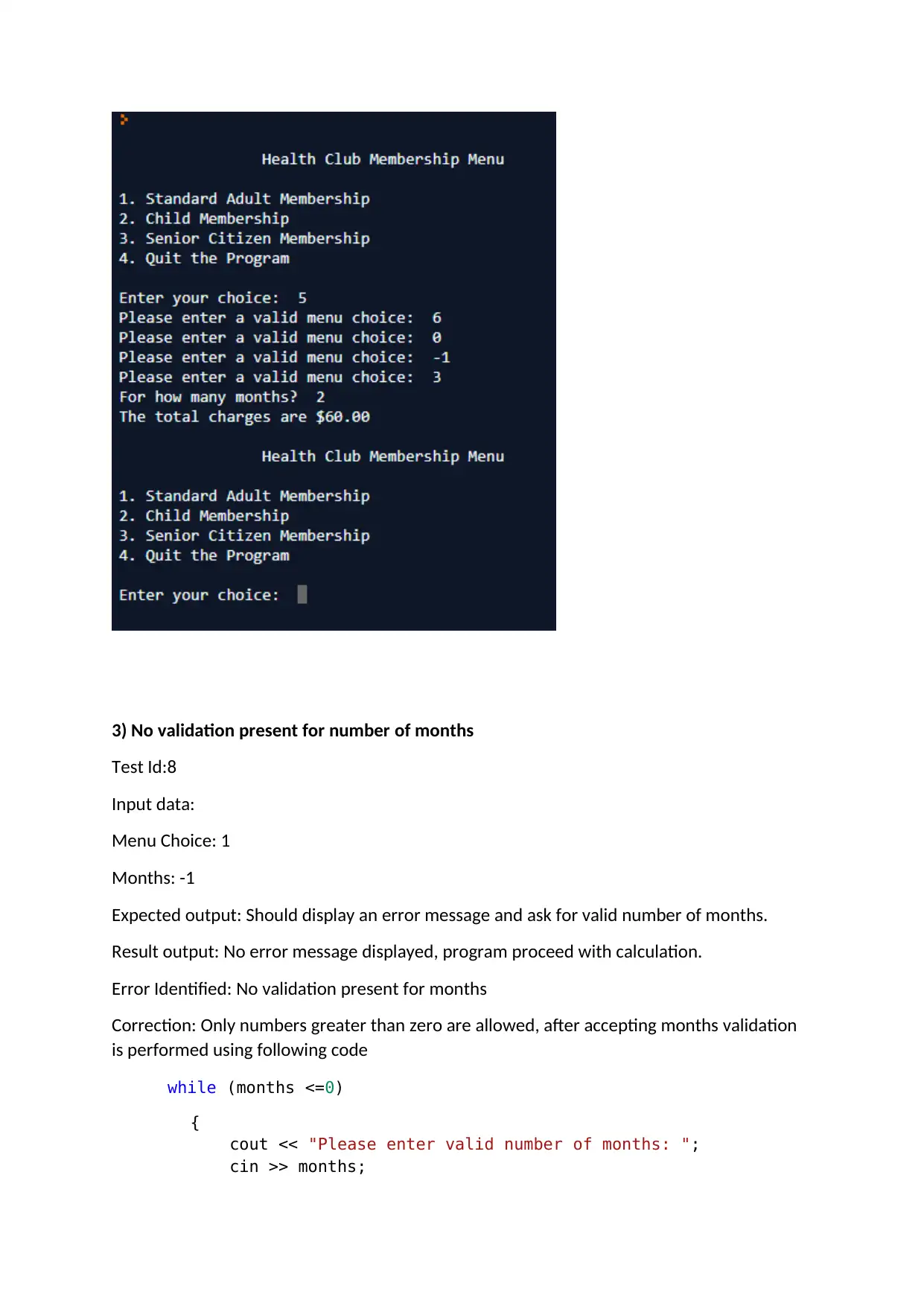
3) No validation present for number of months
Test Id:8
Input data:
Menu Choice: 1
Months: -1
Expected output: Should display an error message and ask for valid number of months.
Result output: No error message displayed, program proceed with calculation.
Error Identified: No validation present for months
Correction: Only numbers greater than zero are allowed, after accepting months validation
is performed using following code
while (months <=0)
{
cout << "Please enter valid number of months: ";
cin >> months;
Test Id:8
Input data:
Menu Choice: 1
Months: -1
Expected output: Should display an error message and ask for valid number of months.
Result output: No error message displayed, program proceed with calculation.
Error Identified: No validation present for months
Correction: Only numbers greater than zero are allowed, after accepting months validation
is performed using following code
while (months <=0)
{
cout << "Please enter valid number of months: ";
cin >> months;
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
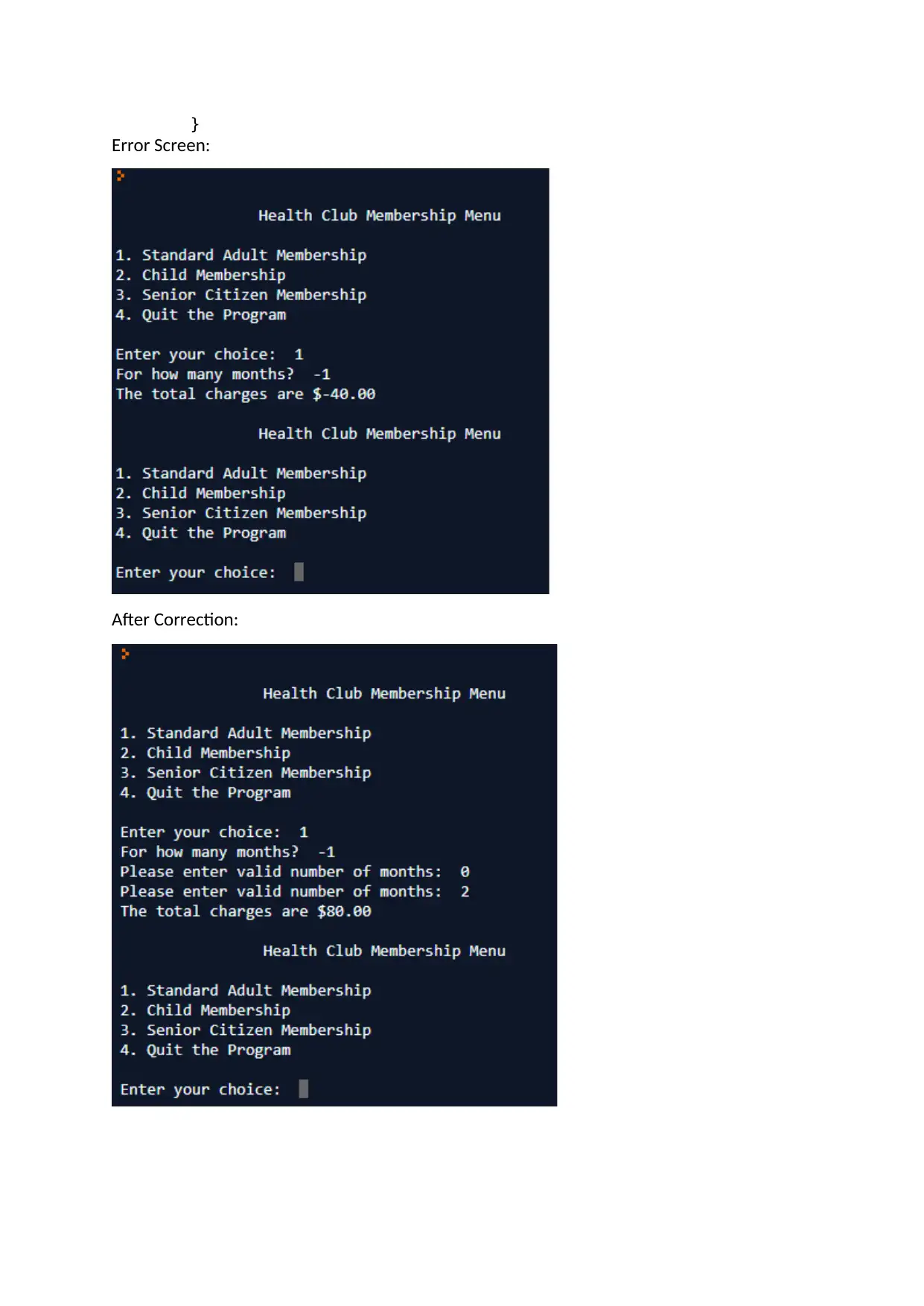
}
Error Screen:
After Correction:
Error Screen:
After Correction:
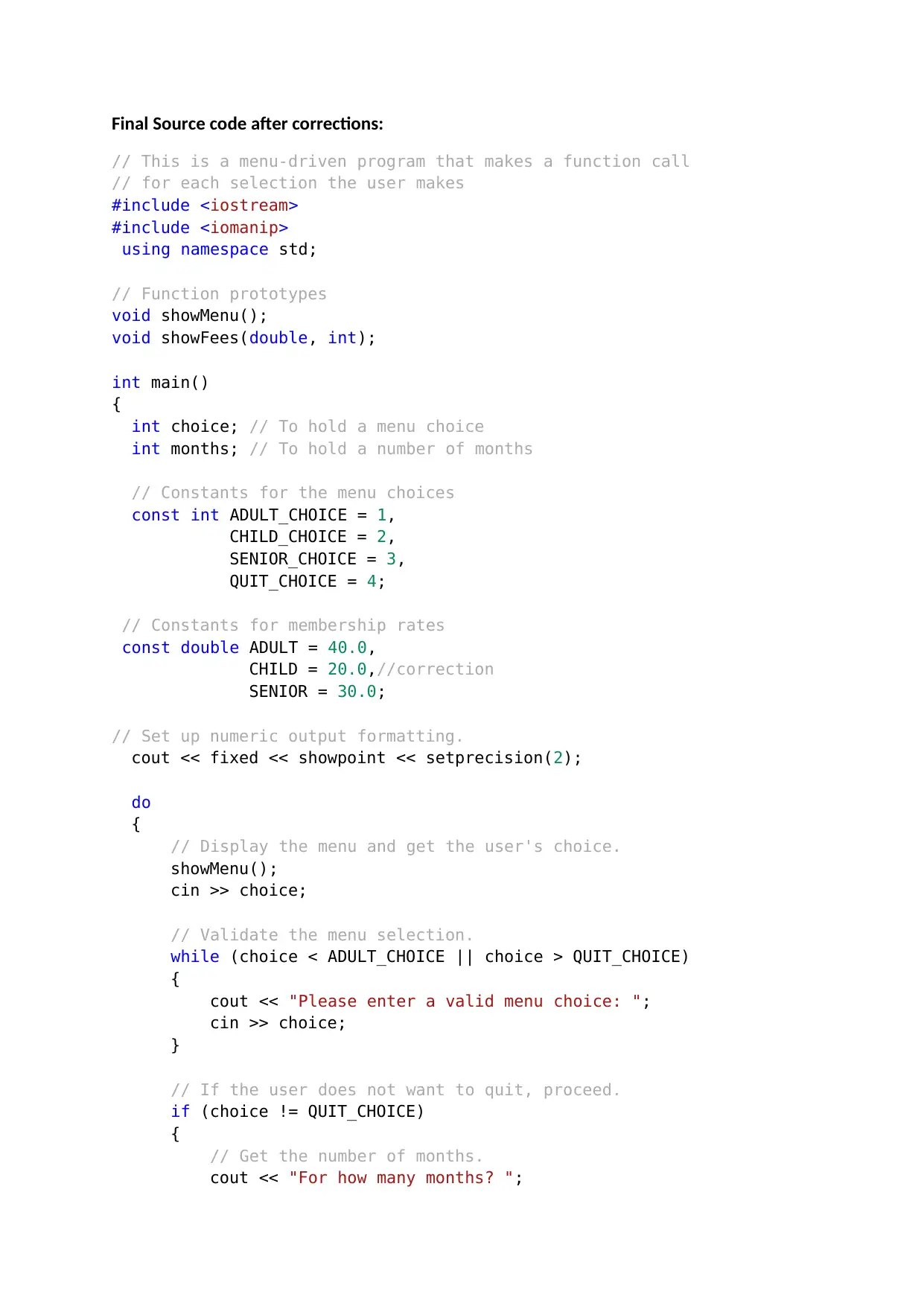
Final Source code after corrections:
// This is a menu-driven program that makes a function call
// for each selection the user makes
#include <iostream>
#include <iomanip>
using namespace std;
// Function prototypes
void showMenu();
void showFees(double, int);
int main()
{
int choice; // To hold a menu choice
int months; // To hold a number of months
// Constants for the menu choices
const int ADULT_CHOICE = 1,
CHILD_CHOICE = 2,
SENIOR_CHOICE = 3,
QUIT_CHOICE = 4;
// Constants for membership rates
const double ADULT = 40.0,
CHILD = 20.0,//correction
SENIOR = 30.0;
// Set up numeric output formatting.
cout << fixed << showpoint << setprecision(2);
do
{
// Display the menu and get the user's choice.
showMenu();
cin >> choice;
// Validate the menu selection.
while (choice < ADULT_CHOICE || choice > QUIT_CHOICE)
{
cout << "Please enter a valid menu choice: ";
cin >> choice;
}
// If the user does not want to quit, proceed.
if (choice != QUIT_CHOICE)
{
// Get the number of months.
cout << "For how many months? ";
// This is a menu-driven program that makes a function call
// for each selection the user makes
#include <iostream>
#include <iomanip>
using namespace std;
// Function prototypes
void showMenu();
void showFees(double, int);
int main()
{
int choice; // To hold a menu choice
int months; // To hold a number of months
// Constants for the menu choices
const int ADULT_CHOICE = 1,
CHILD_CHOICE = 2,
SENIOR_CHOICE = 3,
QUIT_CHOICE = 4;
// Constants for membership rates
const double ADULT = 40.0,
CHILD = 20.0,//correction
SENIOR = 30.0;
// Set up numeric output formatting.
cout << fixed << showpoint << setprecision(2);
do
{
// Display the menu and get the user's choice.
showMenu();
cin >> choice;
// Validate the menu selection.
while (choice < ADULT_CHOICE || choice > QUIT_CHOICE)
{
cout << "Please enter a valid menu choice: ";
cin >> choice;
}
// If the user does not want to quit, proceed.
if (choice != QUIT_CHOICE)
{
// Get the number of months.
cout << "For how many months? ";
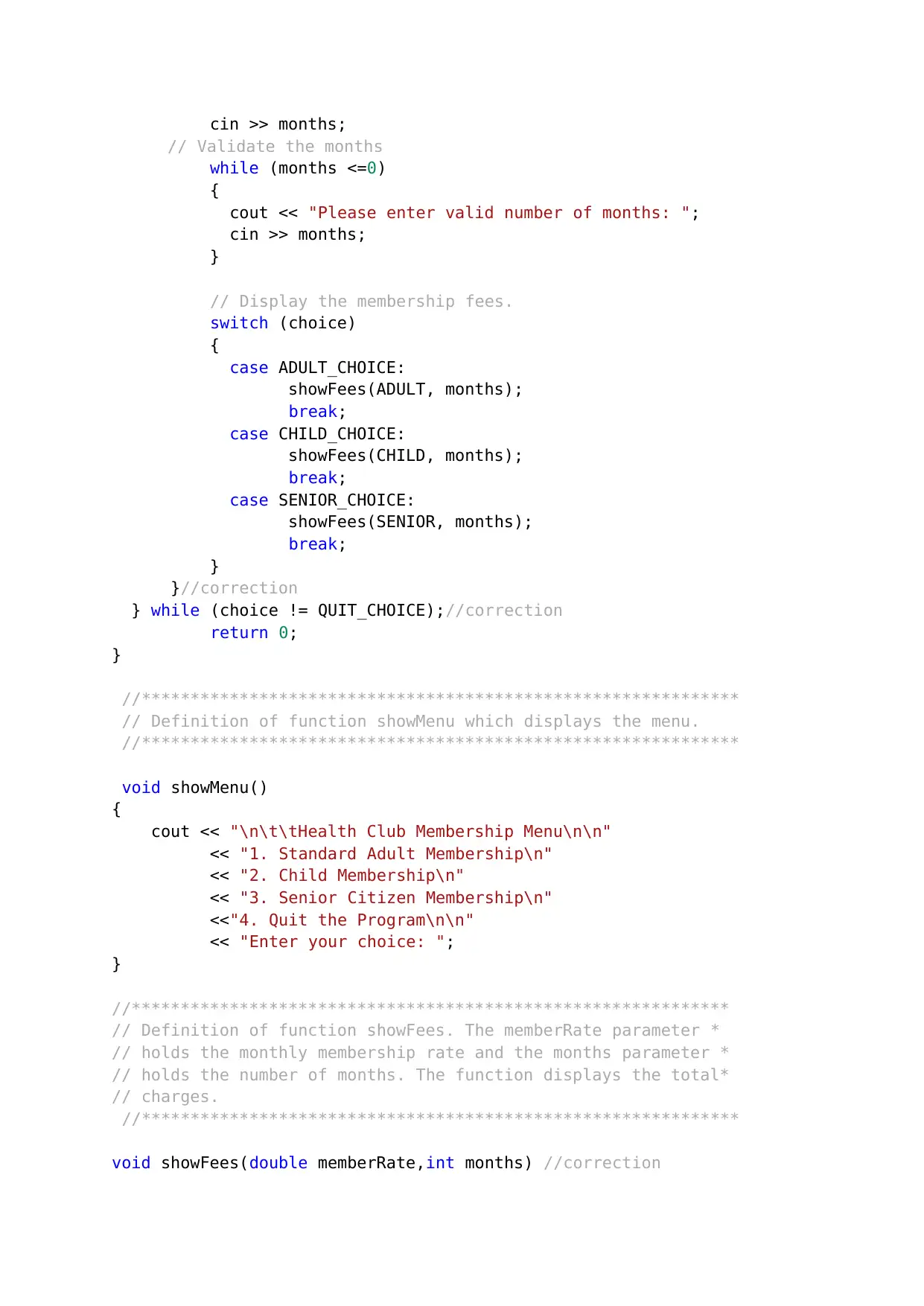
cin >> months;
// Validate the months
while (months <=0)
{
cout << "Please enter valid number of months: ";
cin >> months;
}
// Display the membership fees.
switch (choice)
{
case ADULT_CHOICE:
showFees(ADULT, months);
break;
case CHILD_CHOICE:
showFees(CHILD, months);
break;
case SENIOR_CHOICE:
showFees(SENIOR, months);
break;
}
}//correction
} while (choice != QUIT_CHOICE);//correction
return 0;
}
//*************************************************************
// Definition of function showMenu which displays the menu.
//*************************************************************
void showMenu()
{
cout << "\n\t\tHealth Club Membership Menu\n\n"
<< "1. Standard Adult Membership\n"
<< "2. Child Membership\n"
<< "3. Senior Citizen Membership\n"
<<"4. Quit the Program\n\n"
<< "Enter your choice: ";
}
//*************************************************************
// Definition of function showFees. The memberRate parameter *
// holds the monthly membership rate and the months parameter *
// holds the number of months. The function displays the total*
// charges.
//*************************************************************
void showFees(double memberRate,int months) //correction
// Validate the months
while (months <=0)
{
cout << "Please enter valid number of months: ";
cin >> months;
}
// Display the membership fees.
switch (choice)
{
case ADULT_CHOICE:
showFees(ADULT, months);
break;
case CHILD_CHOICE:
showFees(CHILD, months);
break;
case SENIOR_CHOICE:
showFees(SENIOR, months);
break;
}
}//correction
} while (choice != QUIT_CHOICE);//correction
return 0;
}
//*************************************************************
// Definition of function showMenu which displays the menu.
//*************************************************************
void showMenu()
{
cout << "\n\t\tHealth Club Membership Menu\n\n"
<< "1. Standard Adult Membership\n"
<< "2. Child Membership\n"
<< "3. Senior Citizen Membership\n"
<<"4. Quit the Program\n\n"
<< "Enter your choice: ";
}
//*************************************************************
// Definition of function showFees. The memberRate parameter *
// holds the monthly membership rate and the months parameter *
// holds the number of months. The function displays the total*
// charges.
//*************************************************************
void showFees(double memberRate,int months) //correction
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
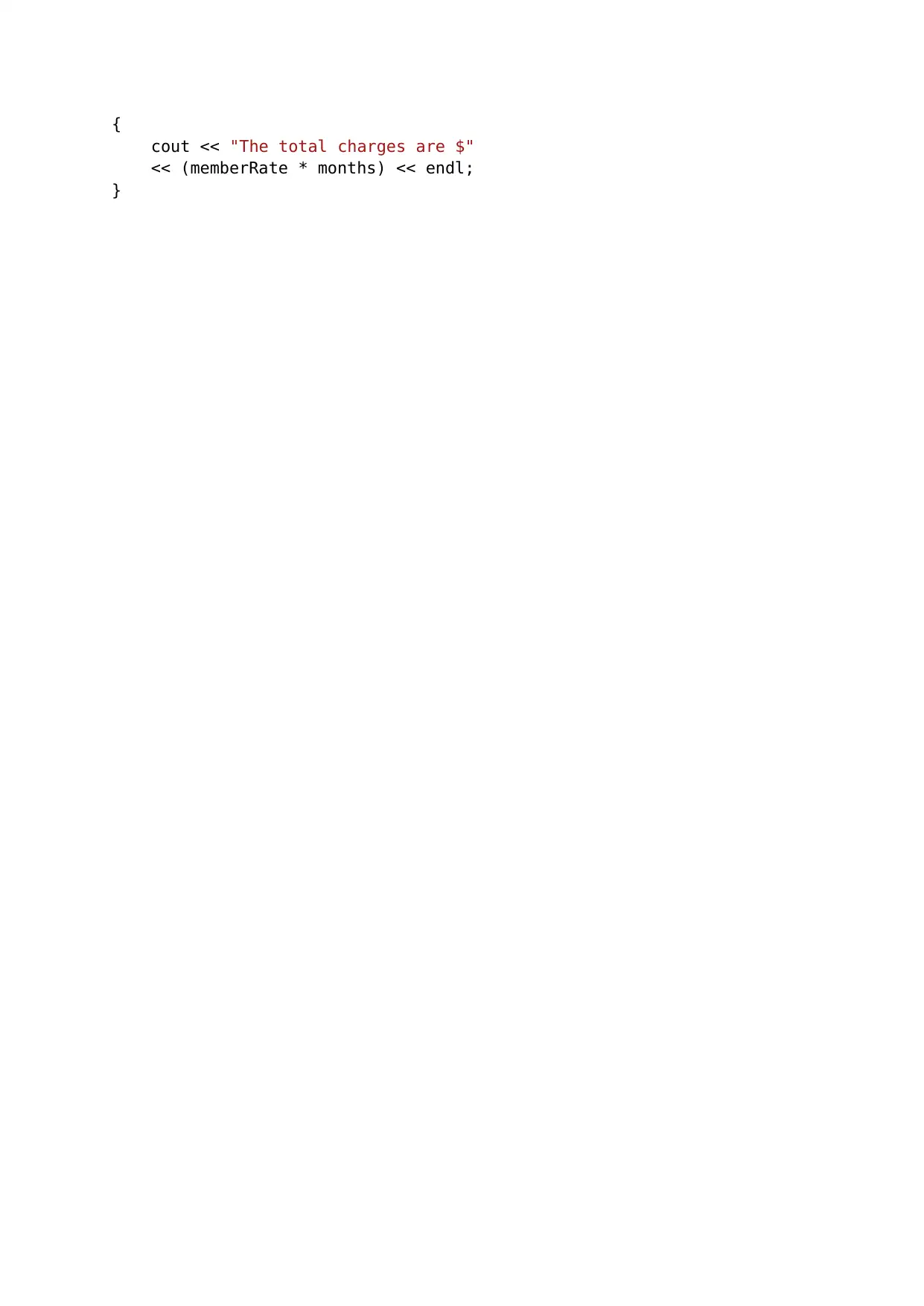
{
cout << "The total charges are $"
<< (memberRate * months) << endl;
}
cout << "The total charges are $"
<< (memberRate * months) << endl;
}
1 out of 26
Related Documents
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
 +13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024  |  Zucol Services PVT LTD  |  All rights reserved.