Error Checking And Handling
VerifiedAdded on 2022/09/06
|12
|2453
|21
AI Summary
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
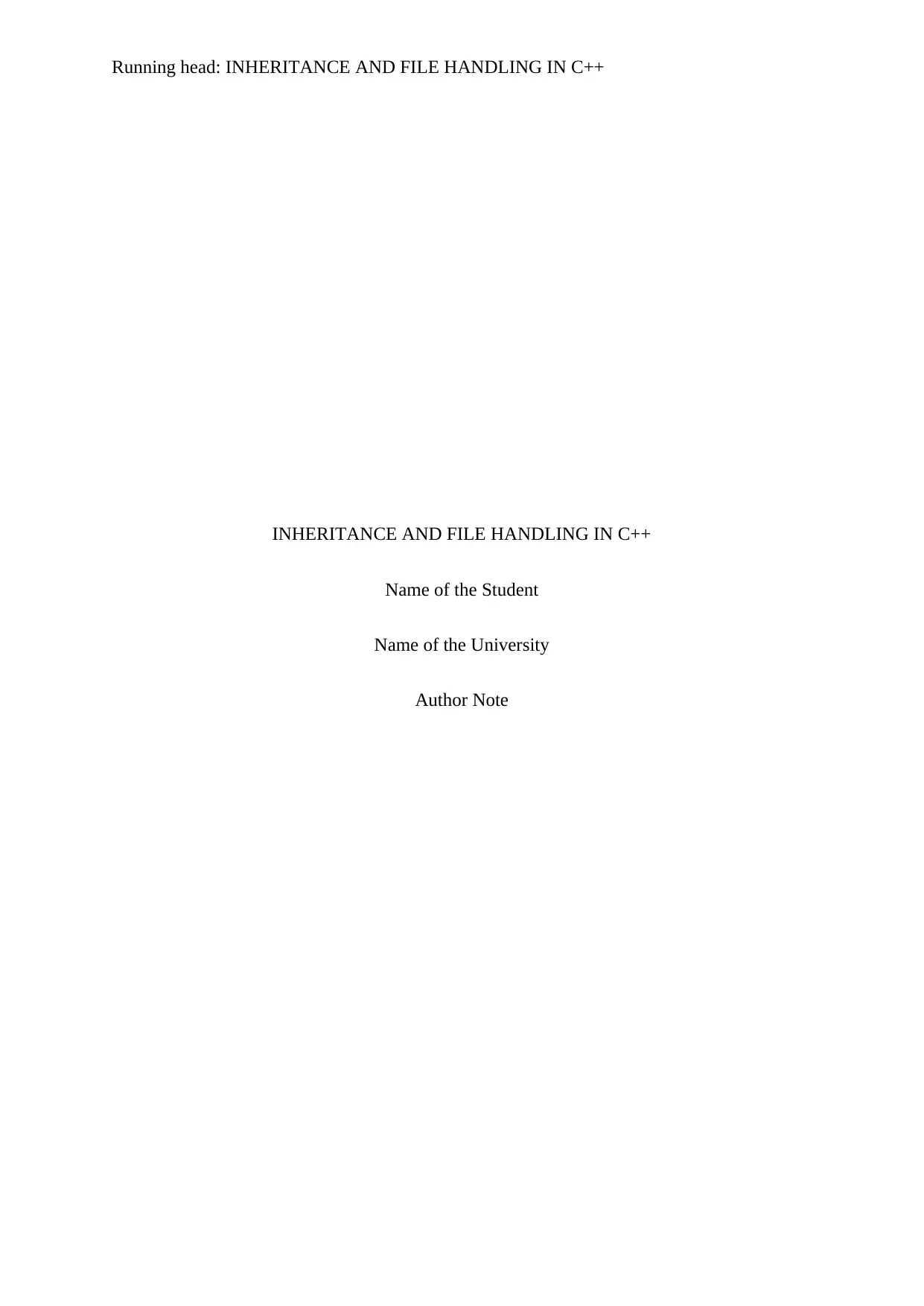
Running head: INHERITANCE AND FILE HANDLING IN C++
INHERITANCE AND FILE HANDLING IN C++
Name of the Student
Name of the University
Author Note
INHERITANCE AND FILE HANDLING IN C++
Name of the Student
Name of the University
Author Note
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
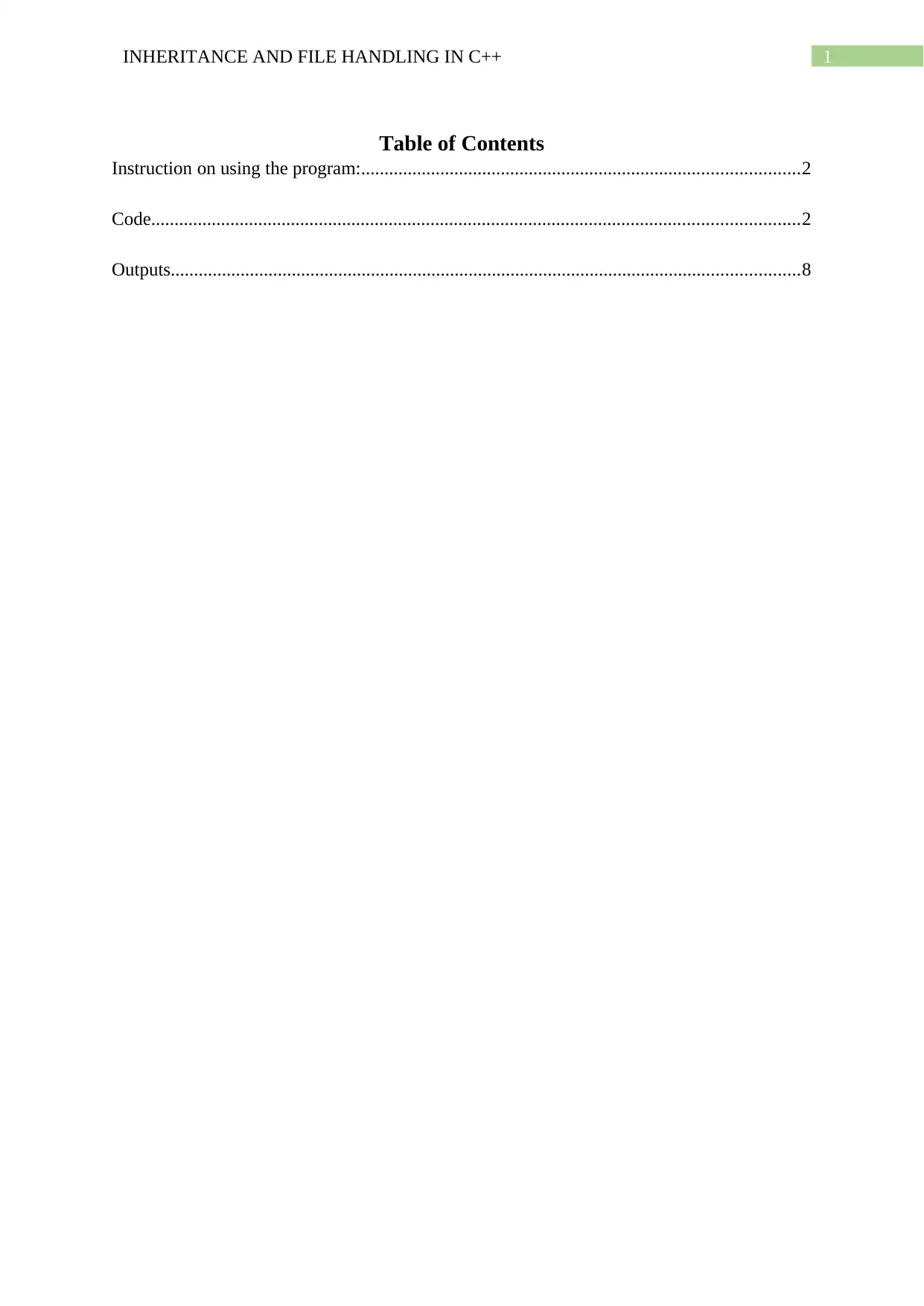
1INHERITANCE AND FILE HANDLING IN C++
Table of Contents
Instruction on using the program:..............................................................................................2
Code...........................................................................................................................................2
Outputs.......................................................................................................................................8
Table of Contents
Instruction on using the program:..............................................................................................2
Code...........................................................................................................................................2
Outputs.......................................................................................................................................8
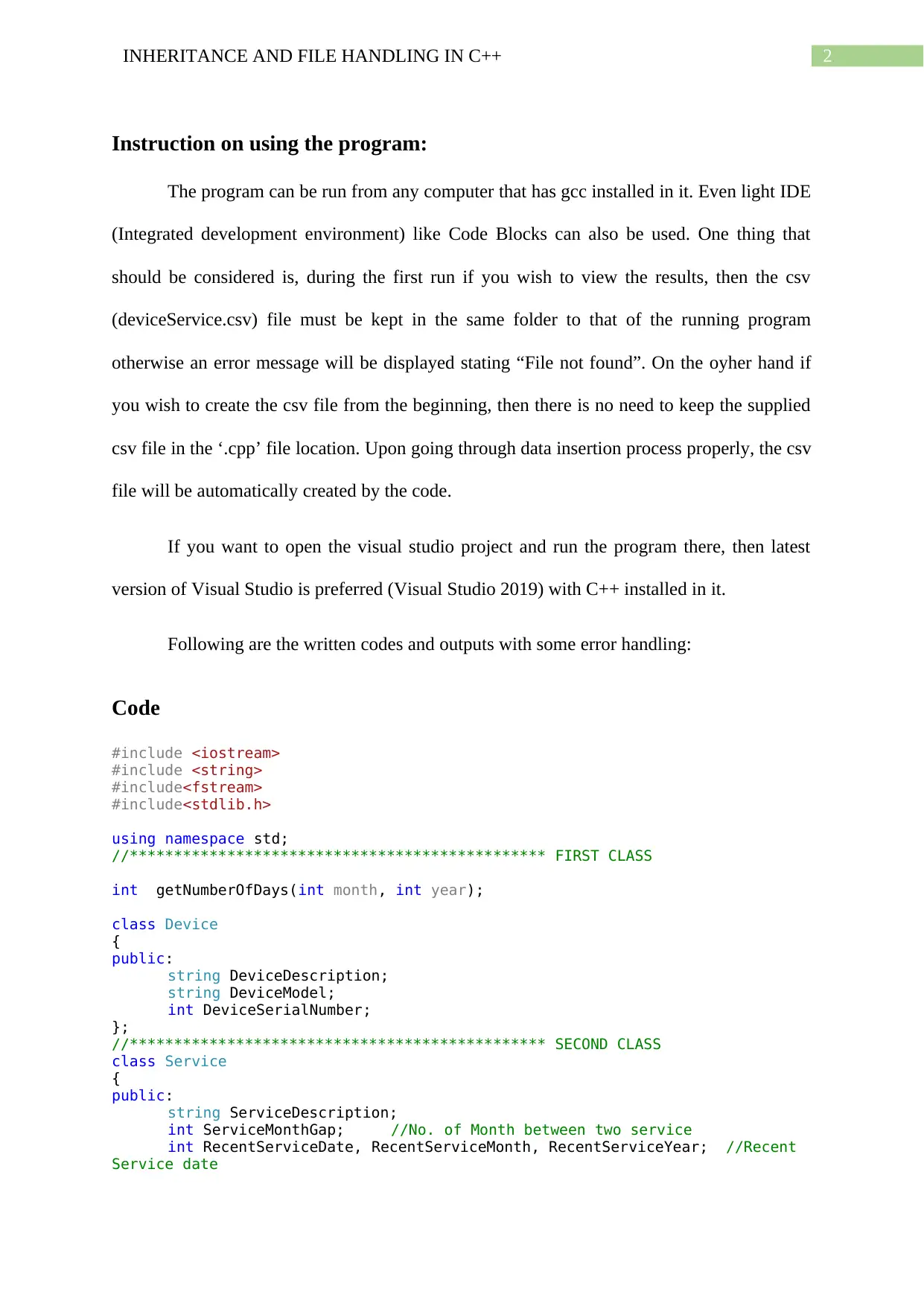
2INHERITANCE AND FILE HANDLING IN C++
Instruction on using the program:
The program can be run from any computer that has gcc installed in it. Even light IDE
(Integrated development environment) like Code Blocks can also be used. One thing that
should be considered is, during the first run if you wish to view the results, then the csv
(deviceService.csv) file must be kept in the same folder to that of the running program
otherwise an error message will be displayed stating “File not found”. On the oyher hand if
you wish to create the csv file from the beginning, then there is no need to keep the supplied
csv file in the ‘.cpp’ file location. Upon going through data insertion process properly, the csv
file will be automatically created by the code.
If you want to open the visual studio project and run the program there, then latest
version of Visual Studio is preferred (Visual Studio 2019) with C++ installed in it.
Following are the written codes and outputs with some error handling:
Code
#include <iostream>
#include <string>
#include<fstream>
#include<stdlib.h>
using namespace std;
//*********************************************** FIRST CLASS
int getNumberOfDays(int month, int year);
class Device
{
public:
string DeviceDescription;
string DeviceModel;
int DeviceSerialNumber;
};
//*********************************************** SECOND CLASS
class Service
{
public:
string ServiceDescription;
int ServiceMonthGap; //No. of Month between two service
int RecentServiceDate, RecentServiceMonth, RecentServiceYear; //Recent
Service date
Instruction on using the program:
The program can be run from any computer that has gcc installed in it. Even light IDE
(Integrated development environment) like Code Blocks can also be used. One thing that
should be considered is, during the first run if you wish to view the results, then the csv
(deviceService.csv) file must be kept in the same folder to that of the running program
otherwise an error message will be displayed stating “File not found”. On the oyher hand if
you wish to create the csv file from the beginning, then there is no need to keep the supplied
csv file in the ‘.cpp’ file location. Upon going through data insertion process properly, the csv
file will be automatically created by the code.
If you want to open the visual studio project and run the program there, then latest
version of Visual Studio is preferred (Visual Studio 2019) with C++ installed in it.
Following are the written codes and outputs with some error handling:
Code
#include <iostream>
#include <string>
#include<fstream>
#include<stdlib.h>
using namespace std;
//*********************************************** FIRST CLASS
int getNumberOfDays(int month, int year);
class Device
{
public:
string DeviceDescription;
string DeviceModel;
int DeviceSerialNumber;
};
//*********************************************** SECOND CLASS
class Service
{
public:
string ServiceDescription;
int ServiceMonthGap; //No. of Month between two service
int RecentServiceDate, RecentServiceMonth, RecentServiceYear; //Recent
Service date
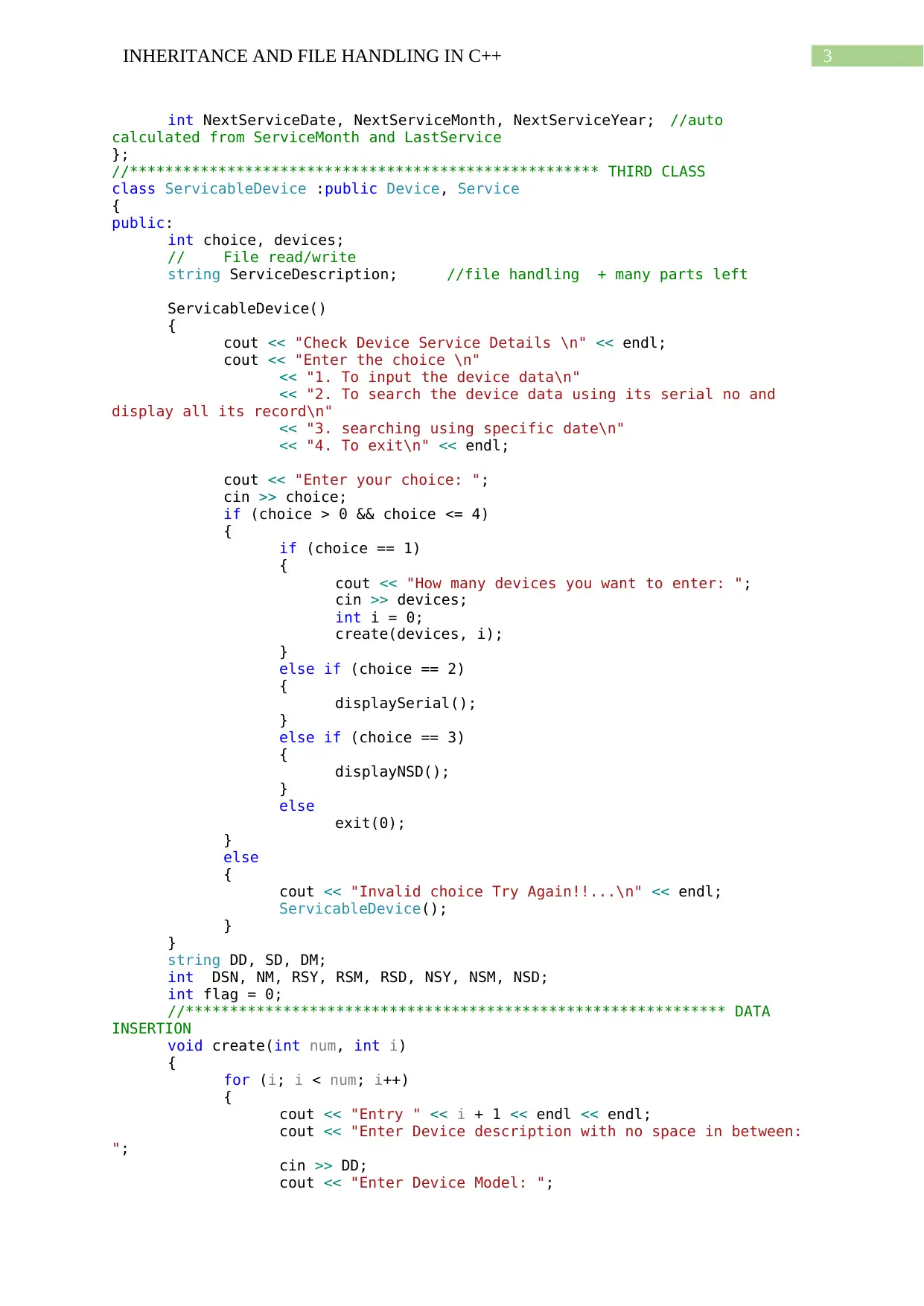
3INHERITANCE AND FILE HANDLING IN C++
int NextServiceDate, NextServiceMonth, NextServiceYear; //auto
calculated from ServiceMonth and LastService
};
//***************************************************** THIRD CLASS
class ServicableDevice :public Device, Service
{
public:
int choice, devices;
// File read/write
string ServiceDescription; //file handling + many parts left
ServicableDevice()
{
cout << "Check Device Service Details \n" << endl;
cout << "Enter the choice \n"
<< "1. To input the device data\n"
<< "2. To search the device data using its serial no and
display all its record\n"
<< "3. searching using specific date\n"
<< "4. To exit\n" << endl;
cout << "Enter your choice: ";
cin >> choice;
if (choice > 0 && choice <= 4)
{
if (choice == 1)
{
cout << "How many devices you want to enter: ";
cin >> devices;
int i = 0;
create(devices, i);
}
else if (choice == 2)
{
displaySerial();
}
else if (choice == 3)
{
displayNSD();
}
else
exit(0);
}
else
{
cout << "Invalid choice Try Again!!...\n" << endl;
ServicableDevice();
}
}
string DD, SD, DM;
int DSN, NM, RSY, RSM, RSD, NSY, NSM, NSD;
int flag = 0;
//************************************************************* DATA
INSERTION
void create(int num, int i)
{
for (i; i < num; i++)
{
cout << "Entry " << i + 1 << endl << endl;
cout << "Enter Device description with no space in between:
";
cin >> DD;
cout << "Enter Device Model: ";
int NextServiceDate, NextServiceMonth, NextServiceYear; //auto
calculated from ServiceMonth and LastService
};
//***************************************************** THIRD CLASS
class ServicableDevice :public Device, Service
{
public:
int choice, devices;
// File read/write
string ServiceDescription; //file handling + many parts left
ServicableDevice()
{
cout << "Check Device Service Details \n" << endl;
cout << "Enter the choice \n"
<< "1. To input the device data\n"
<< "2. To search the device data using its serial no and
display all its record\n"
<< "3. searching using specific date\n"
<< "4. To exit\n" << endl;
cout << "Enter your choice: ";
cin >> choice;
if (choice > 0 && choice <= 4)
{
if (choice == 1)
{
cout << "How many devices you want to enter: ";
cin >> devices;
int i = 0;
create(devices, i);
}
else if (choice == 2)
{
displaySerial();
}
else if (choice == 3)
{
displayNSD();
}
else
exit(0);
}
else
{
cout << "Invalid choice Try Again!!...\n" << endl;
ServicableDevice();
}
}
string DD, SD, DM;
int DSN, NM, RSY, RSM, RSD, NSY, NSM, NSD;
int flag = 0;
//************************************************************* DATA
INSERTION
void create(int num, int i)
{
for (i; i < num; i++)
{
cout << "Entry " << i + 1 << endl << endl;
cout << "Enter Device description with no space in between:
";
cin >> DD;
cout << "Enter Device Model: ";
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
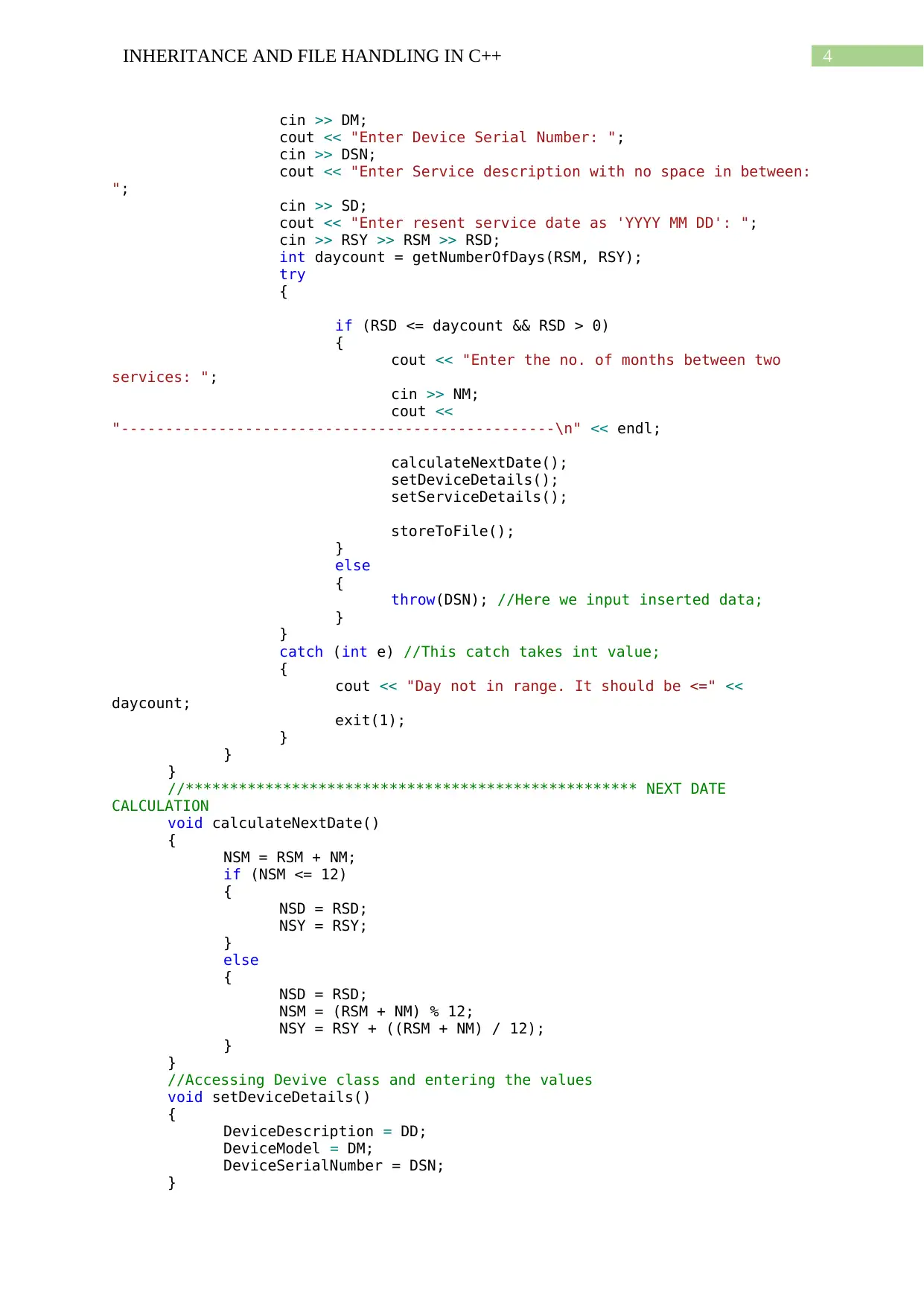
4INHERITANCE AND FILE HANDLING IN C++
cin >> DM;
cout << "Enter Device Serial Number: ";
cin >> DSN;
cout << "Enter Service description with no space in between:
";
cin >> SD;
cout << "Enter resent service date as 'YYYY MM DD': ";
cin >> RSY >> RSM >> RSD;
int daycount = getNumberOfDays(RSM, RSY);
try
{
if (RSD <= daycount && RSD > 0)
{
cout << "Enter the no. of months between two
services: ";
cin >> NM;
cout <<
"-------------------------------------------------\n" << endl;
calculateNextDate();
setDeviceDetails();
setServiceDetails();
storeToFile();
}
else
{
throw(DSN); //Here we input inserted data;
}
}
catch (int e) //This catch takes int value;
{
cout << "Day not in range. It should be <=" <<
daycount;
exit(1);
}
}
}
//*************************************************** NEXT DATE
CALCULATION
void calculateNextDate()
{
NSM = RSM + NM;
if (NSM <= 12)
{
NSD = RSD;
NSY = RSY;
}
else
{
NSD = RSD;
NSM = (RSM + NM) % 12;
NSY = RSY + ((RSM + NM) / 12);
}
}
//Accessing Devive class and entering the values
void setDeviceDetails()
{
DeviceDescription = DD;
DeviceModel = DM;
DeviceSerialNumber = DSN;
}
cin >> DM;
cout << "Enter Device Serial Number: ";
cin >> DSN;
cout << "Enter Service description with no space in between:
";
cin >> SD;
cout << "Enter resent service date as 'YYYY MM DD': ";
cin >> RSY >> RSM >> RSD;
int daycount = getNumberOfDays(RSM, RSY);
try
{
if (RSD <= daycount && RSD > 0)
{
cout << "Enter the no. of months between two
services: ";
cin >> NM;
cout <<
"-------------------------------------------------\n" << endl;
calculateNextDate();
setDeviceDetails();
setServiceDetails();
storeToFile();
}
else
{
throw(DSN); //Here we input inserted data;
}
}
catch (int e) //This catch takes int value;
{
cout << "Day not in range. It should be <=" <<
daycount;
exit(1);
}
}
}
//*************************************************** NEXT DATE
CALCULATION
void calculateNextDate()
{
NSM = RSM + NM;
if (NSM <= 12)
{
NSD = RSD;
NSY = RSY;
}
else
{
NSD = RSD;
NSM = (RSM + NM) % 12;
NSY = RSY + ((RSM + NM) / 12);
}
}
//Accessing Devive class and entering the values
void setDeviceDetails()
{
DeviceDescription = DD;
DeviceModel = DM;
DeviceSerialNumber = DSN;
}
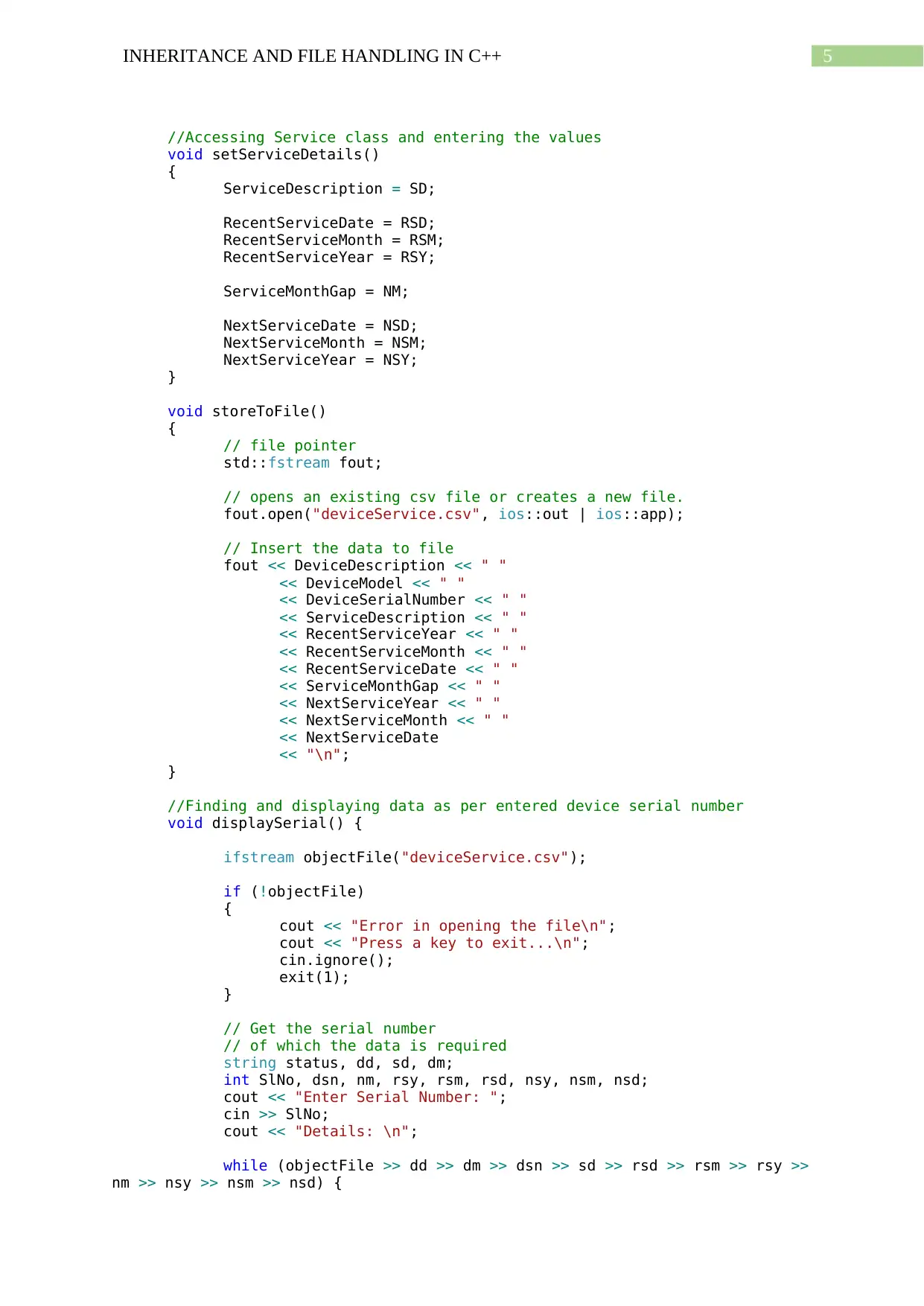
5INHERITANCE AND FILE HANDLING IN C++
//Accessing Service class and entering the values
void setServiceDetails()
{
ServiceDescription = SD;
RecentServiceDate = RSD;
RecentServiceMonth = RSM;
RecentServiceYear = RSY;
ServiceMonthGap = NM;
NextServiceDate = NSD;
NextServiceMonth = NSM;
NextServiceYear = NSY;
}
void storeToFile()
{
// file pointer
std::fstream fout;
// opens an existing csv file or creates a new file.
fout.open("deviceService.csv", ios::out | ios::app);
// Insert the data to file
fout << DeviceDescription << " "
<< DeviceModel << " "
<< DeviceSerialNumber << " "
<< ServiceDescription << " "
<< RecentServiceYear << " "
<< RecentServiceMonth << " "
<< RecentServiceDate << " "
<< ServiceMonthGap << " "
<< NextServiceYear << " "
<< NextServiceMonth << " "
<< NextServiceDate
<< "\n";
}
//Finding and displaying data as per entered device serial number
void displaySerial() {
ifstream objectFile("deviceService.csv");
if (!objectFile)
{
cout << "Error in opening the file\n";
cout << "Press a key to exit...\n";
cin.ignore();
exit(1);
}
// Get the serial number
// of which the data is required
string status, dd, sd, dm;
int SlNo, dsn, nm, rsy, rsm, rsd, nsy, nsm, nsd;
cout << "Enter Serial Number: ";
cin >> SlNo;
cout << "Details: \n";
while (objectFile >> dd >> dm >> dsn >> sd >> rsd >> rsm >> rsy >>
nm >> nsy >> nsm >> nsd) {
//Accessing Service class and entering the values
void setServiceDetails()
{
ServiceDescription = SD;
RecentServiceDate = RSD;
RecentServiceMonth = RSM;
RecentServiceYear = RSY;
ServiceMonthGap = NM;
NextServiceDate = NSD;
NextServiceMonth = NSM;
NextServiceYear = NSY;
}
void storeToFile()
{
// file pointer
std::fstream fout;
// opens an existing csv file or creates a new file.
fout.open("deviceService.csv", ios::out | ios::app);
// Insert the data to file
fout << DeviceDescription << " "
<< DeviceModel << " "
<< DeviceSerialNumber << " "
<< ServiceDescription << " "
<< RecentServiceYear << " "
<< RecentServiceMonth << " "
<< RecentServiceDate << " "
<< ServiceMonthGap << " "
<< NextServiceYear << " "
<< NextServiceMonth << " "
<< NextServiceDate
<< "\n";
}
//Finding and displaying data as per entered device serial number
void displaySerial() {
ifstream objectFile("deviceService.csv");
if (!objectFile)
{
cout << "Error in opening the file\n";
cout << "Press a key to exit...\n";
cin.ignore();
exit(1);
}
// Get the serial number
// of which the data is required
string status, dd, sd, dm;
int SlNo, dsn, nm, rsy, rsm, rsd, nsy, nsm, nsd;
cout << "Enter Serial Number: ";
cin >> SlNo;
cout << "Details: \n";
while (objectFile >> dd >> dm >> dsn >> sd >> rsd >> rsm >> rsy >>
nm >> nsy >> nsm >> nsd) {
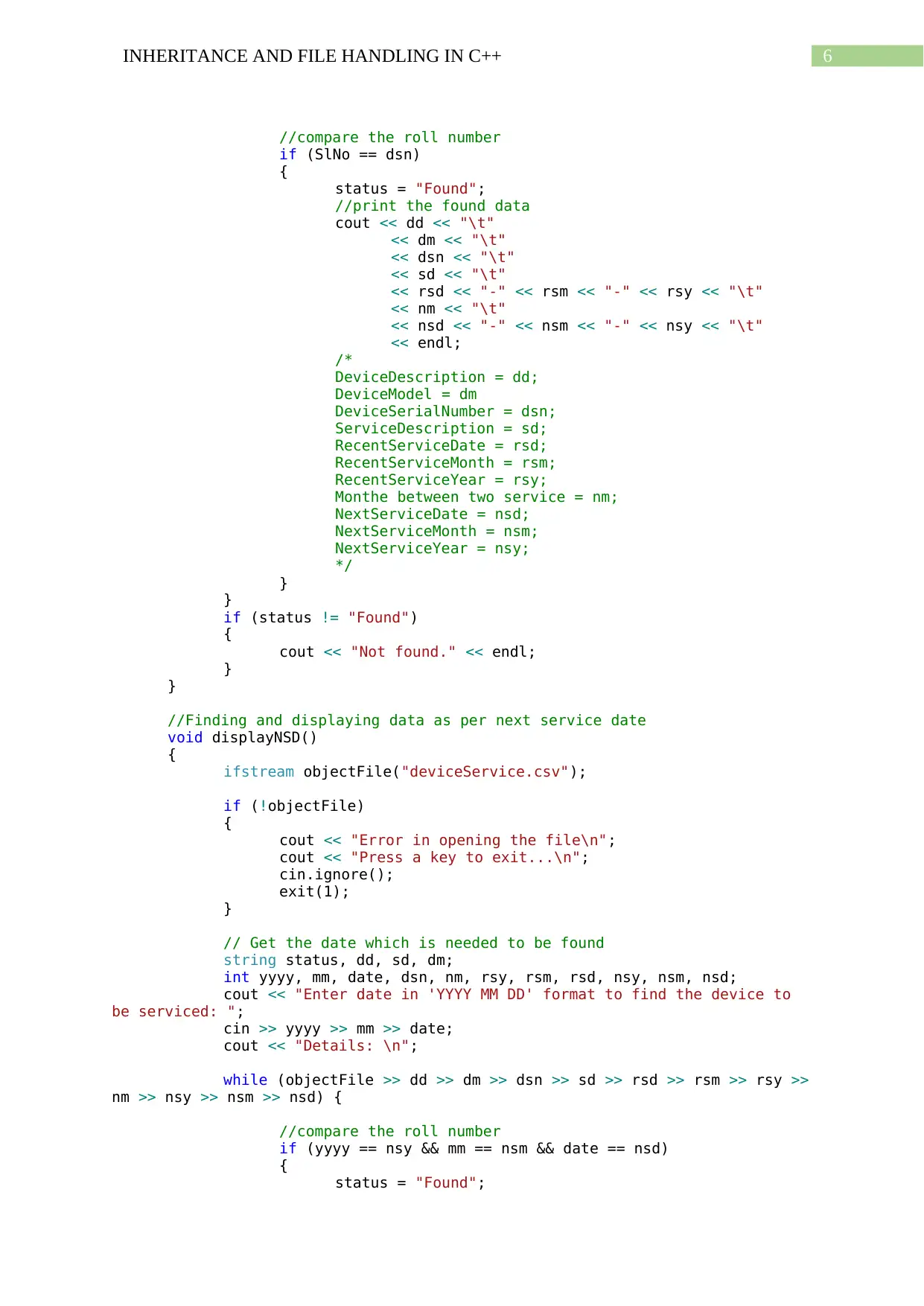
6INHERITANCE AND FILE HANDLING IN C++
//compare the roll number
if (SlNo == dsn)
{
status = "Found";
//print the found data
cout << dd << "\t"
<< dm << "\t"
<< dsn << "\t"
<< sd << "\t"
<< rsd << "-" << rsm << "-" << rsy << "\t"
<< nm << "\t"
<< nsd << "-" << nsm << "-" << nsy << "\t"
<< endl;
/*
DeviceDescription = dd;
DeviceModel = dm
DeviceSerialNumber = dsn;
ServiceDescription = sd;
RecentServiceDate = rsd;
RecentServiceMonth = rsm;
RecentServiceYear = rsy;
Monthe between two service = nm;
NextServiceDate = nsd;
NextServiceMonth = nsm;
NextServiceYear = nsy;
*/
}
}
if (status != "Found")
{
cout << "Not found." << endl;
}
}
//Finding and displaying data as per next service date
void displayNSD()
{
ifstream objectFile("deviceService.csv");
if (!objectFile)
{
cout << "Error in opening the file\n";
cout << "Press a key to exit...\n";
cin.ignore();
exit(1);
}
// Get the date which is needed to be found
string status, dd, sd, dm;
int yyyy, mm, date, dsn, nm, rsy, rsm, rsd, nsy, nsm, nsd;
cout << "Enter date in 'YYYY MM DD' format to find the device to
be serviced: ";
cin >> yyyy >> mm >> date;
cout << "Details: \n";
while (objectFile >> dd >> dm >> dsn >> sd >> rsd >> rsm >> rsy >>
nm >> nsy >> nsm >> nsd) {
//compare the roll number
if (yyyy == nsy && mm == nsm && date == nsd)
{
status = "Found";
//compare the roll number
if (SlNo == dsn)
{
status = "Found";
//print the found data
cout << dd << "\t"
<< dm << "\t"
<< dsn << "\t"
<< sd << "\t"
<< rsd << "-" << rsm << "-" << rsy << "\t"
<< nm << "\t"
<< nsd << "-" << nsm << "-" << nsy << "\t"
<< endl;
/*
DeviceDescription = dd;
DeviceModel = dm
DeviceSerialNumber = dsn;
ServiceDescription = sd;
RecentServiceDate = rsd;
RecentServiceMonth = rsm;
RecentServiceYear = rsy;
Monthe between two service = nm;
NextServiceDate = nsd;
NextServiceMonth = nsm;
NextServiceYear = nsy;
*/
}
}
if (status != "Found")
{
cout << "Not found." << endl;
}
}
//Finding and displaying data as per next service date
void displayNSD()
{
ifstream objectFile("deviceService.csv");
if (!objectFile)
{
cout << "Error in opening the file\n";
cout << "Press a key to exit...\n";
cin.ignore();
exit(1);
}
// Get the date which is needed to be found
string status, dd, sd, dm;
int yyyy, mm, date, dsn, nm, rsy, rsm, rsd, nsy, nsm, nsd;
cout << "Enter date in 'YYYY MM DD' format to find the device to
be serviced: ";
cin >> yyyy >> mm >> date;
cout << "Details: \n";
while (objectFile >> dd >> dm >> dsn >> sd >> rsd >> rsm >> rsy >>
nm >> nsy >> nsm >> nsd) {
//compare the roll number
if (yyyy == nsy && mm == nsm && date == nsd)
{
status = "Found";
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
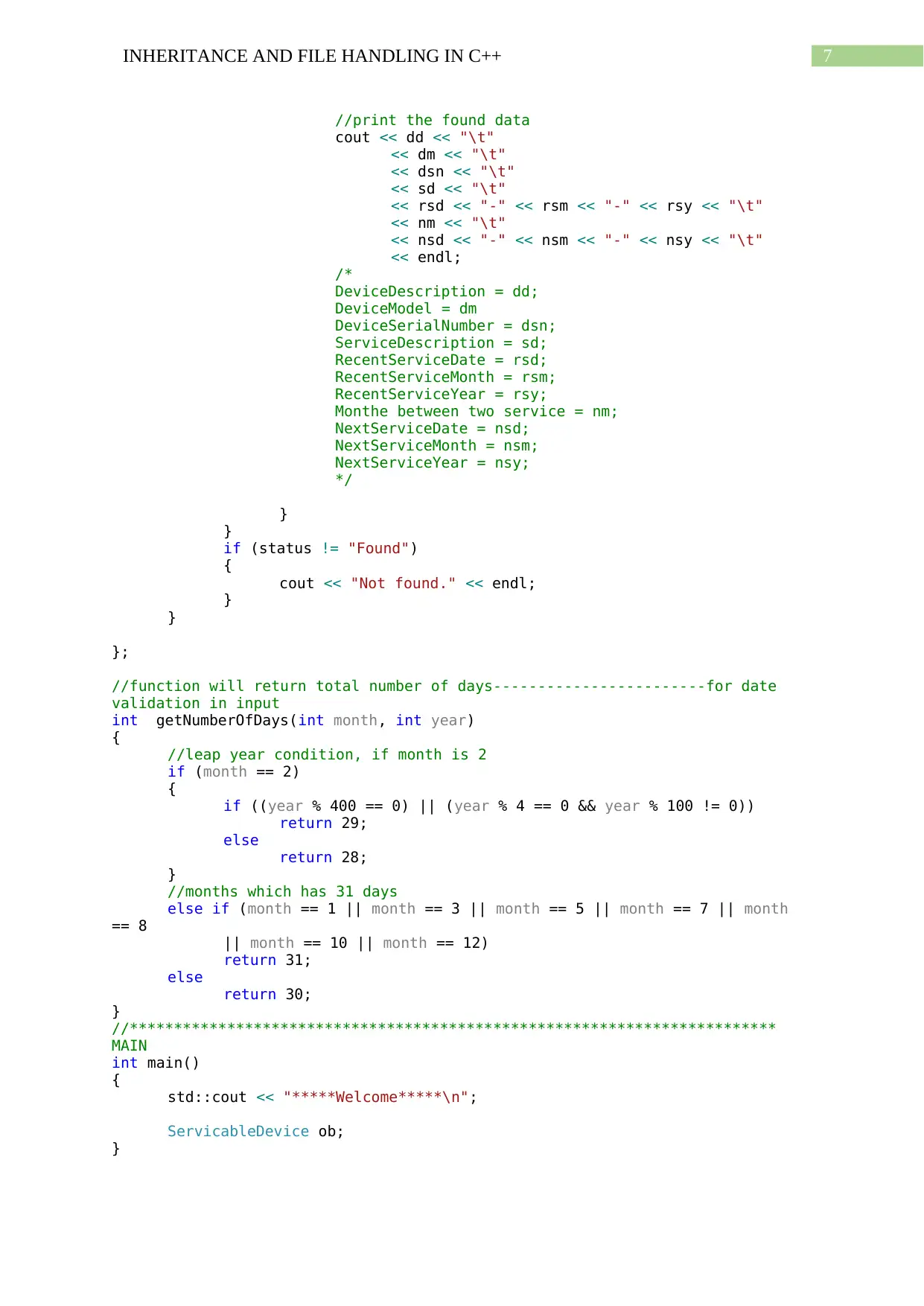
7INHERITANCE AND FILE HANDLING IN C++
//print the found data
cout << dd << "\t"
<< dm << "\t"
<< dsn << "\t"
<< sd << "\t"
<< rsd << "-" << rsm << "-" << rsy << "\t"
<< nm << "\t"
<< nsd << "-" << nsm << "-" << nsy << "\t"
<< endl;
/*
DeviceDescription = dd;
DeviceModel = dm
DeviceSerialNumber = dsn;
ServiceDescription = sd;
RecentServiceDate = rsd;
RecentServiceMonth = rsm;
RecentServiceYear = rsy;
Monthe between two service = nm;
NextServiceDate = nsd;
NextServiceMonth = nsm;
NextServiceYear = nsy;
*/
}
}
if (status != "Found")
{
cout << "Not found." << endl;
}
}
};
//function will return total number of days------------------------for date
validation in input
int getNumberOfDays(int month, int year)
{
//leap year condition, if month is 2
if (month == 2)
{
if ((year % 400 == 0) || (year % 4 == 0 && year % 100 != 0))
return 29;
else
return 28;
}
//months which has 31 days
else if (month == 1 || month == 3 || month == 5 || month == 7 || month
== 8
|| month == 10 || month == 12)
return 31;
else
return 30;
}
//*************************************************************************
MAIN
int main()
{
std::cout << "*****Welcome*****\n";
ServicableDevice ob;
}
//print the found data
cout << dd << "\t"
<< dm << "\t"
<< dsn << "\t"
<< sd << "\t"
<< rsd << "-" << rsm << "-" << rsy << "\t"
<< nm << "\t"
<< nsd << "-" << nsm << "-" << nsy << "\t"
<< endl;
/*
DeviceDescription = dd;
DeviceModel = dm
DeviceSerialNumber = dsn;
ServiceDescription = sd;
RecentServiceDate = rsd;
RecentServiceMonth = rsm;
RecentServiceYear = rsy;
Monthe between two service = nm;
NextServiceDate = nsd;
NextServiceMonth = nsm;
NextServiceYear = nsy;
*/
}
}
if (status != "Found")
{
cout << "Not found." << endl;
}
}
};
//function will return total number of days------------------------for date
validation in input
int getNumberOfDays(int month, int year)
{
//leap year condition, if month is 2
if (month == 2)
{
if ((year % 400 == 0) || (year % 4 == 0 && year % 100 != 0))
return 29;
else
return 28;
}
//months which has 31 days
else if (month == 1 || month == 3 || month == 5 || month == 7 || month
== 8
|| month == 10 || month == 12)
return 31;
else
return 30;
}
//*************************************************************************
MAIN
int main()
{
std::cout << "*****Welcome*****\n";
ServicableDevice ob;
}
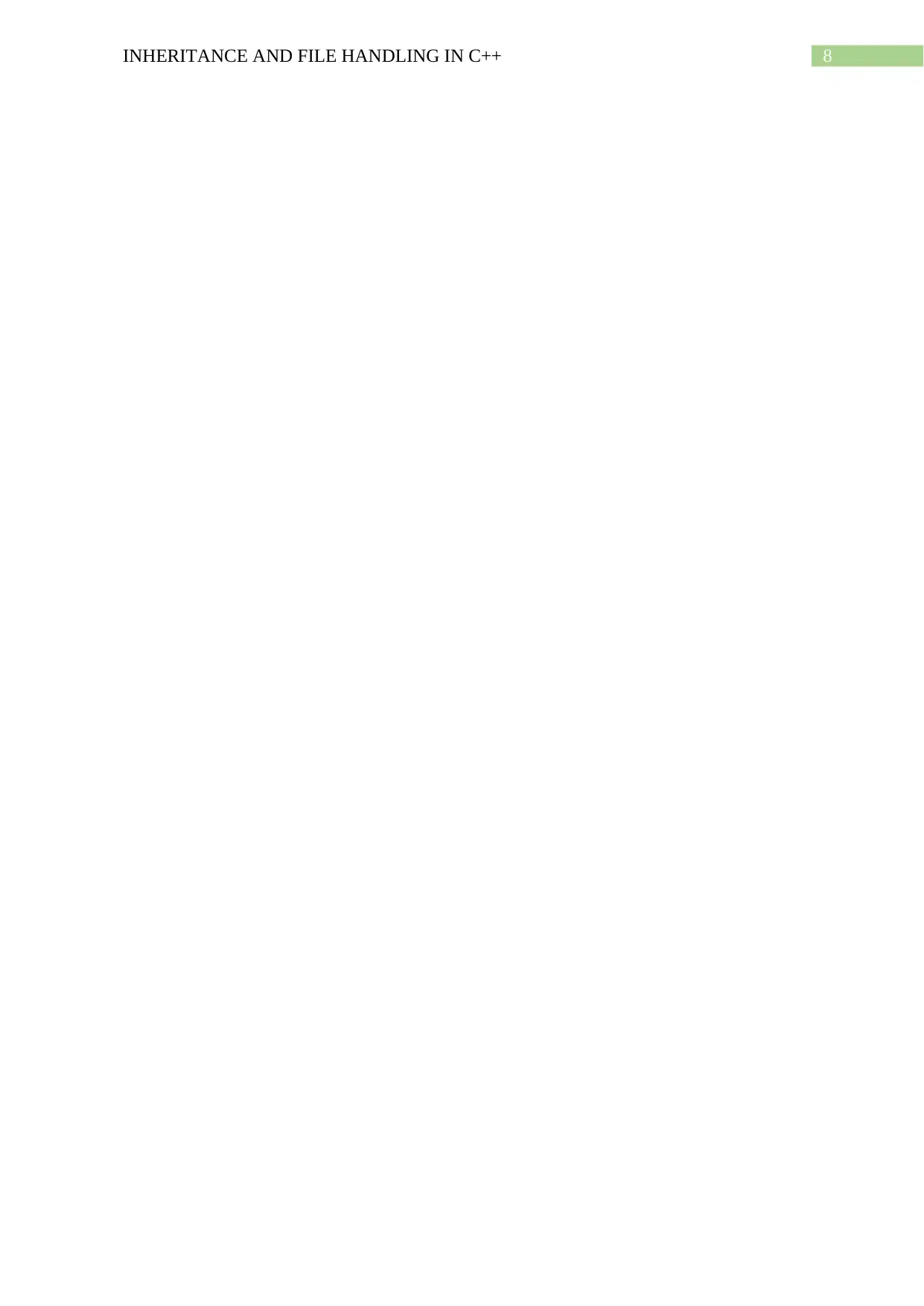
8INHERITANCE AND FILE HANDLING IN C++
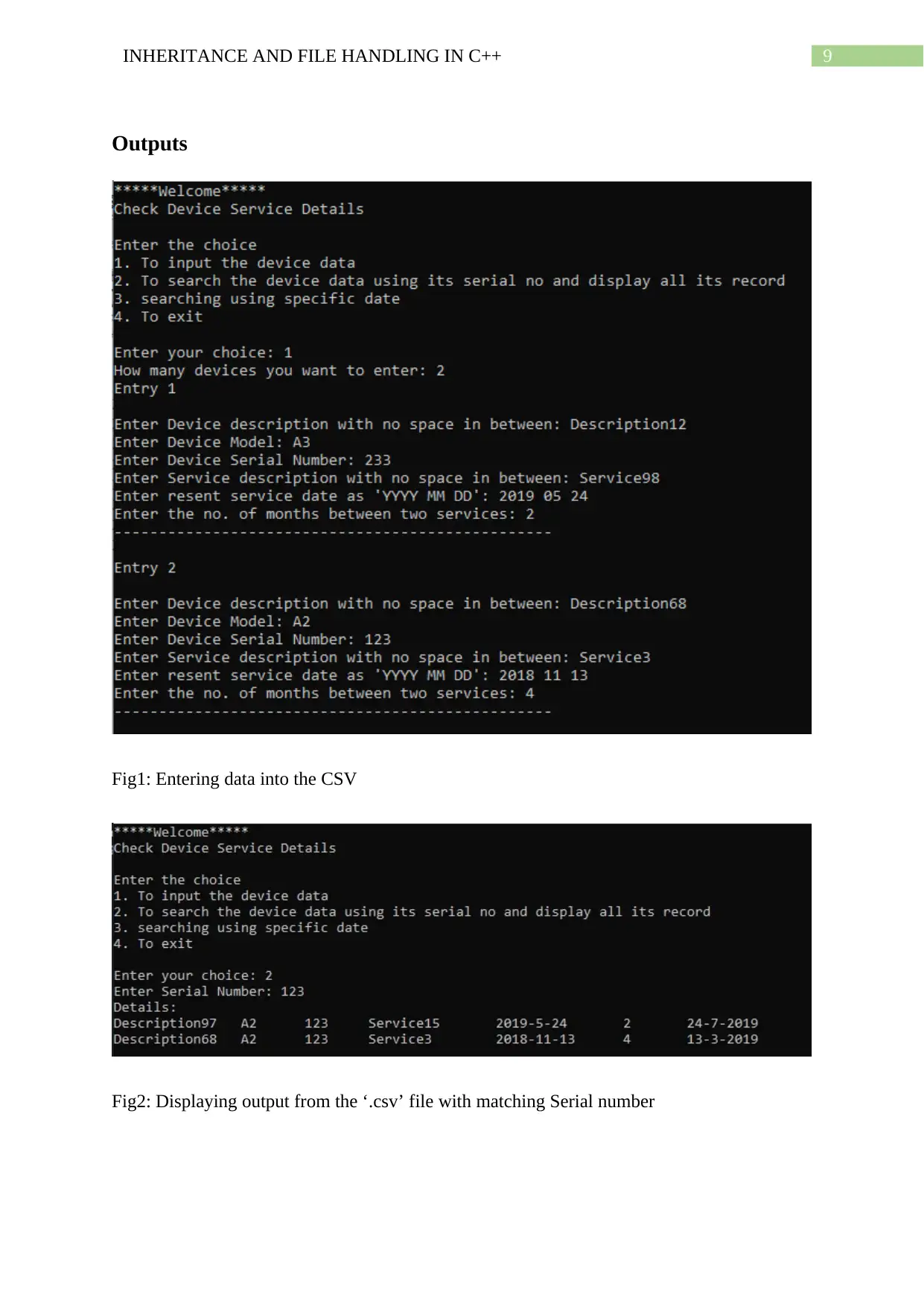
9INHERITANCE AND FILE HANDLING IN C++
Outputs
Fig1: Entering data into the CSV
Fig2: Displaying output from the ‘.csv’ file with matching Serial number
Outputs
Fig1: Entering data into the CSV
Fig2: Displaying output from the ‘.csv’ file with matching Serial number
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
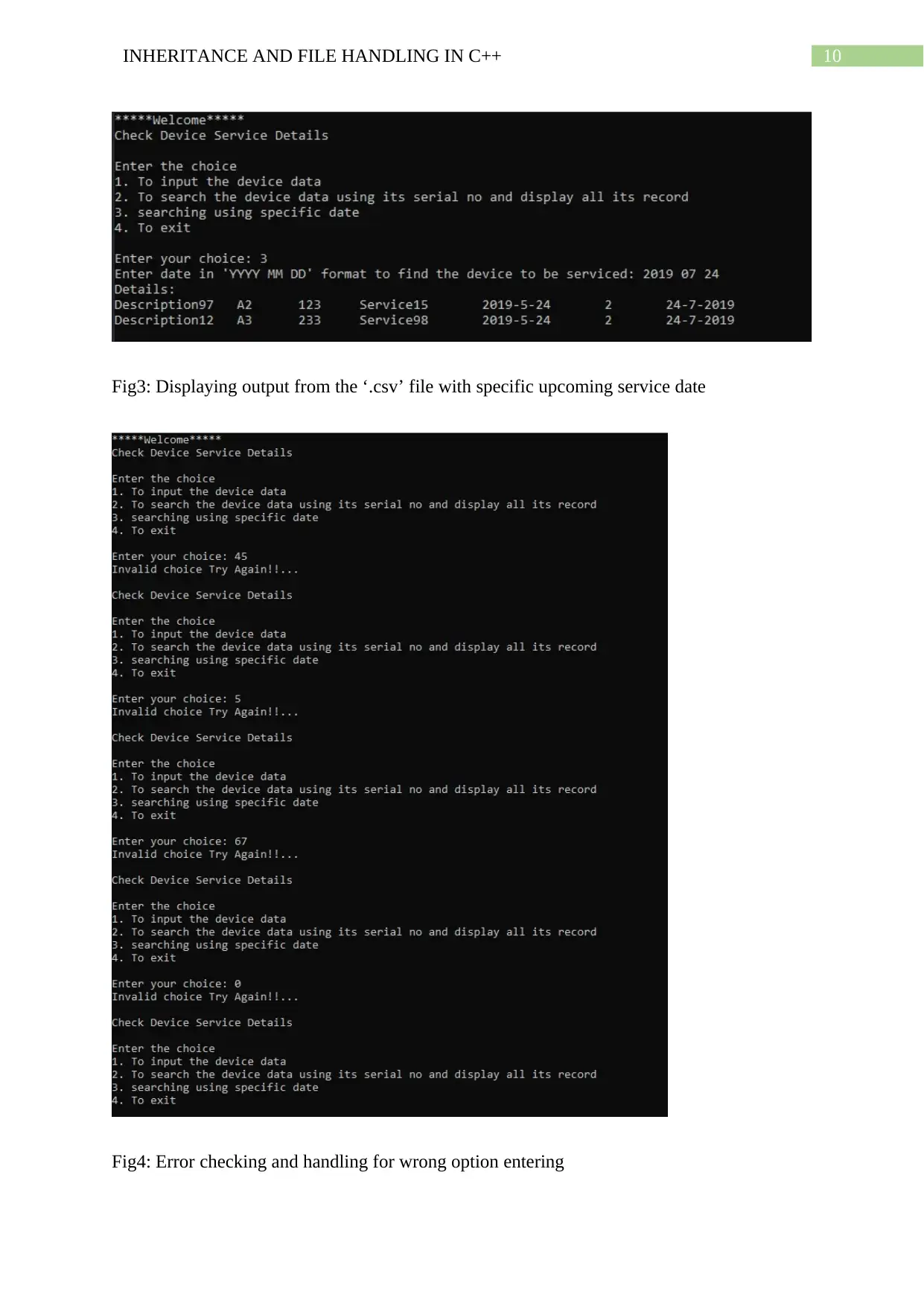
10INHERITANCE AND FILE HANDLING IN C++
Fig3: Displaying output from the ‘.csv’ file with specific upcoming service date
Fig4: Error checking and handling for wrong option entering
Fig3: Displaying output from the ‘.csv’ file with specific upcoming service date
Fig4: Error checking and handling for wrong option entering
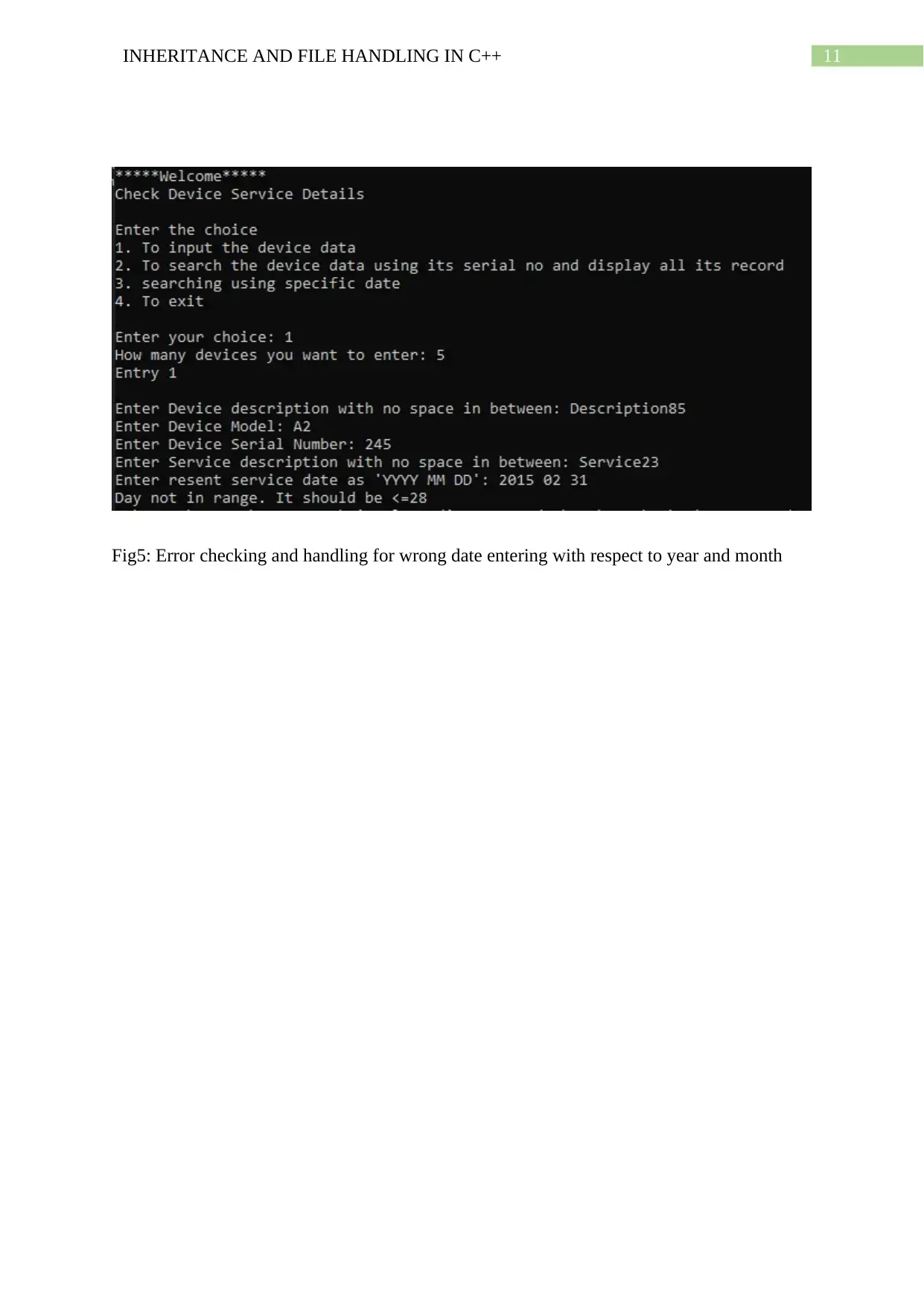
11INHERITANCE AND FILE HANDLING IN C++
Fig5: Error checking and handling for wrong date entering with respect to year and month
Fig5: Error checking and handling for wrong date entering with respect to year and month
1 out of 12
Related Documents
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.