ITECH1400 – Foundations of Programming
VerifiedAdded on 2023/04/19
|7
|1303
|94
AI Summary
This document is an assignment for ITECH1400 – Foundations of Programming. It includes details about the assignment, class design, class diagrams, activity flowchart, software implementation, code explanation, and use. The assignment focuses on creating a supermarket self-service checkout system using Python programming language.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
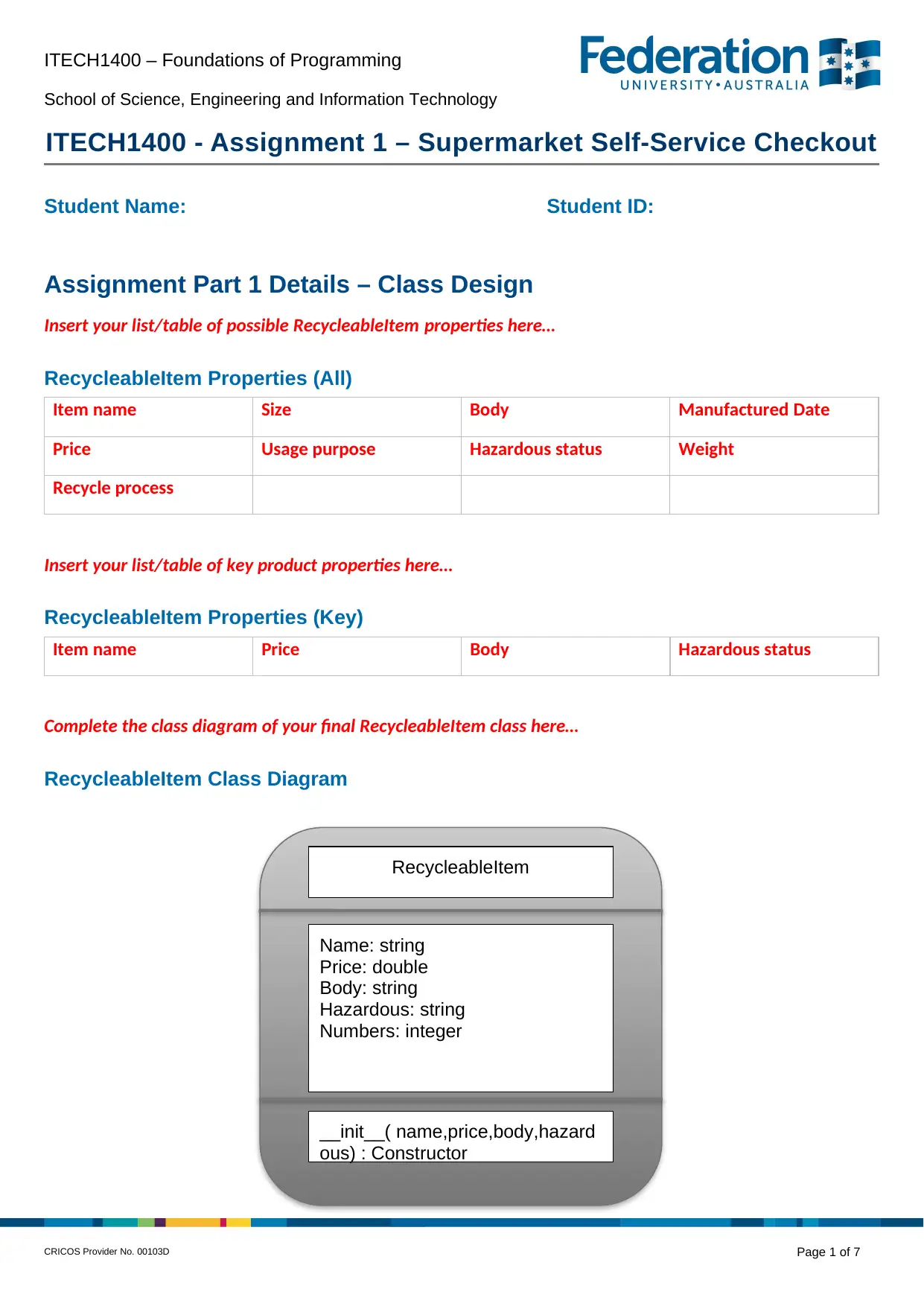
ITECH1400 – Foundations of Programming
School of Science, Engineering and Information Technology
ITECH1400 - Assignment 1 – Supermarket Self-Service Checkout
Student Name: Student ID:
Assignment Part 1 Details – Class Design
Insert your list/table of possible RecycleableItem properties here…
RecycleableItem Properties (All)
Item name Size Body Manufactured Date
Price Usage purpose Hazardous status Weight
Recycle process
Insert your list/table of key product properties here…
RecycleableItem Properties (Key)
Item name Price Body Hazardous status
Complete the class diagram of your final RecycleableItem class here…
RecycleableItem Class Diagram
RecycleableItem
Name: string
Price: double
Body: string
Hazardous: string
Numbers: integer
__init__( name,price,body,hazard
ous) : Constructor
CRICOS Provider No. 00103D Page 1 of 7
School of Science, Engineering and Information Technology
ITECH1400 - Assignment 1 – Supermarket Self-Service Checkout
Student Name: Student ID:
Assignment Part 1 Details – Class Design
Insert your list/table of possible RecycleableItem properties here…
RecycleableItem Properties (All)
Item name Size Body Manufactured Date
Price Usage purpose Hazardous status Weight
Recycle process
Insert your list/table of key product properties here…
RecycleableItem Properties (Key)
Item name Price Body Hazardous status
Complete the class diagram of your final RecycleableItem class here…
RecycleableItem Class Diagram
RecycleableItem
Name: string
Price: double
Body: string
Hazardous: string
Numbers: integer
__init__( name,price,body,hazard
ous) : Constructor
CRICOS Provider No. 00103D Page 1 of 7
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
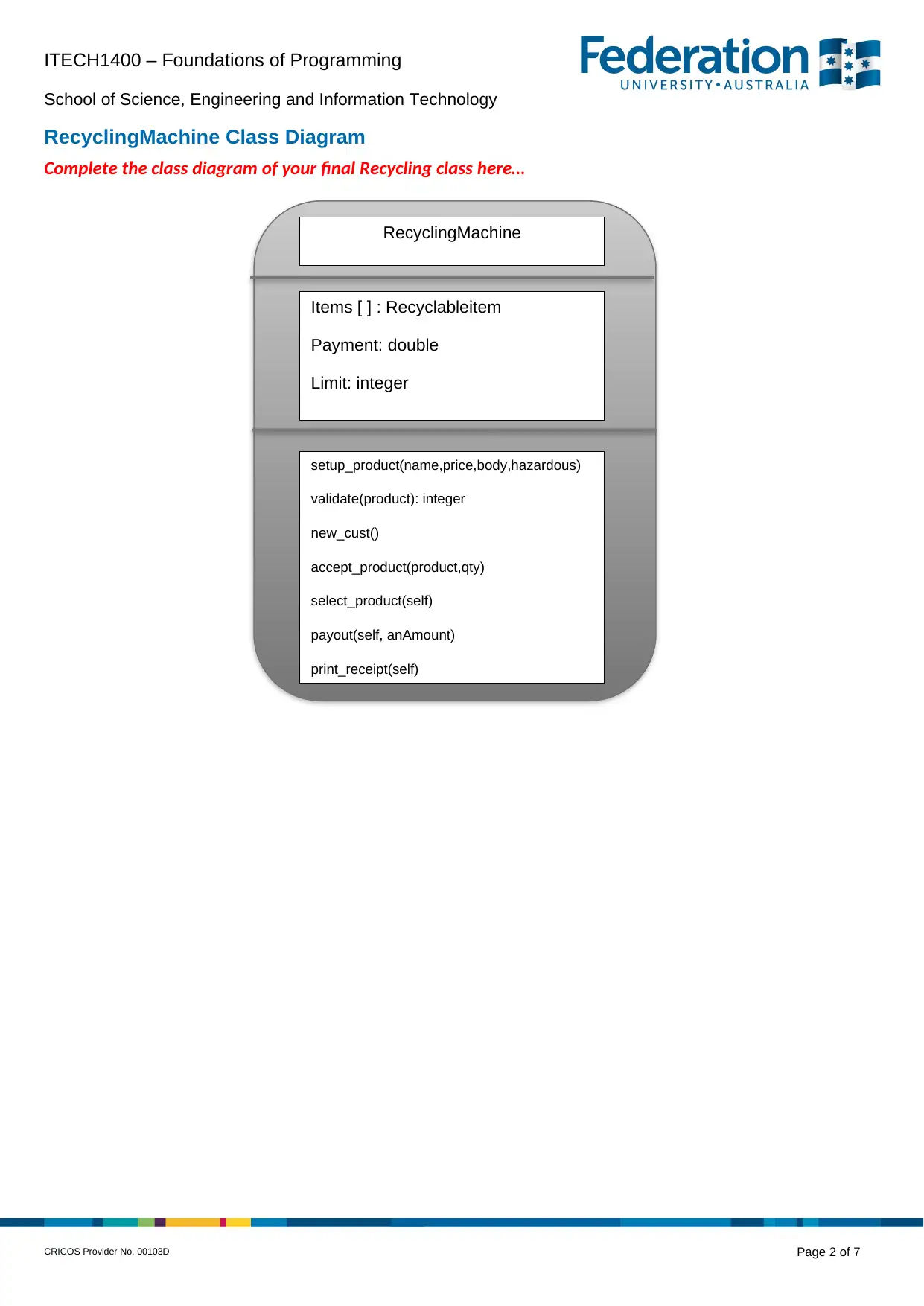
ITECH1400 – Foundations of Programming
School of Science, Engineering and Information Technology
RecyclingMachine Class Diagram
Complete the class diagram of your final Recycling class here…
RecyclingMachine
Items [ ] : Recyclableitem
Payment: double
Limit: integer
setup_product(name,price,body,hazardous)
validate(product): integer
new_cust()
accept_product(product,qty)
select_product(self)
payout(self, anAmount)
print_receipt(self)
CRICOS Provider No. 00103D Page 2 of 7
School of Science, Engineering and Information Technology
RecyclingMachine Class Diagram
Complete the class diagram of your final Recycling class here…
RecyclingMachine
Items [ ] : Recyclableitem
Payment: double
Limit: integer
setup_product(name,price,body,hazardous)
validate(product): integer
new_cust()
accept_product(product,qty)
select_product(self)
payout(self, anAmount)
print_receipt(self)
CRICOS Provider No. 00103D Page 2 of 7
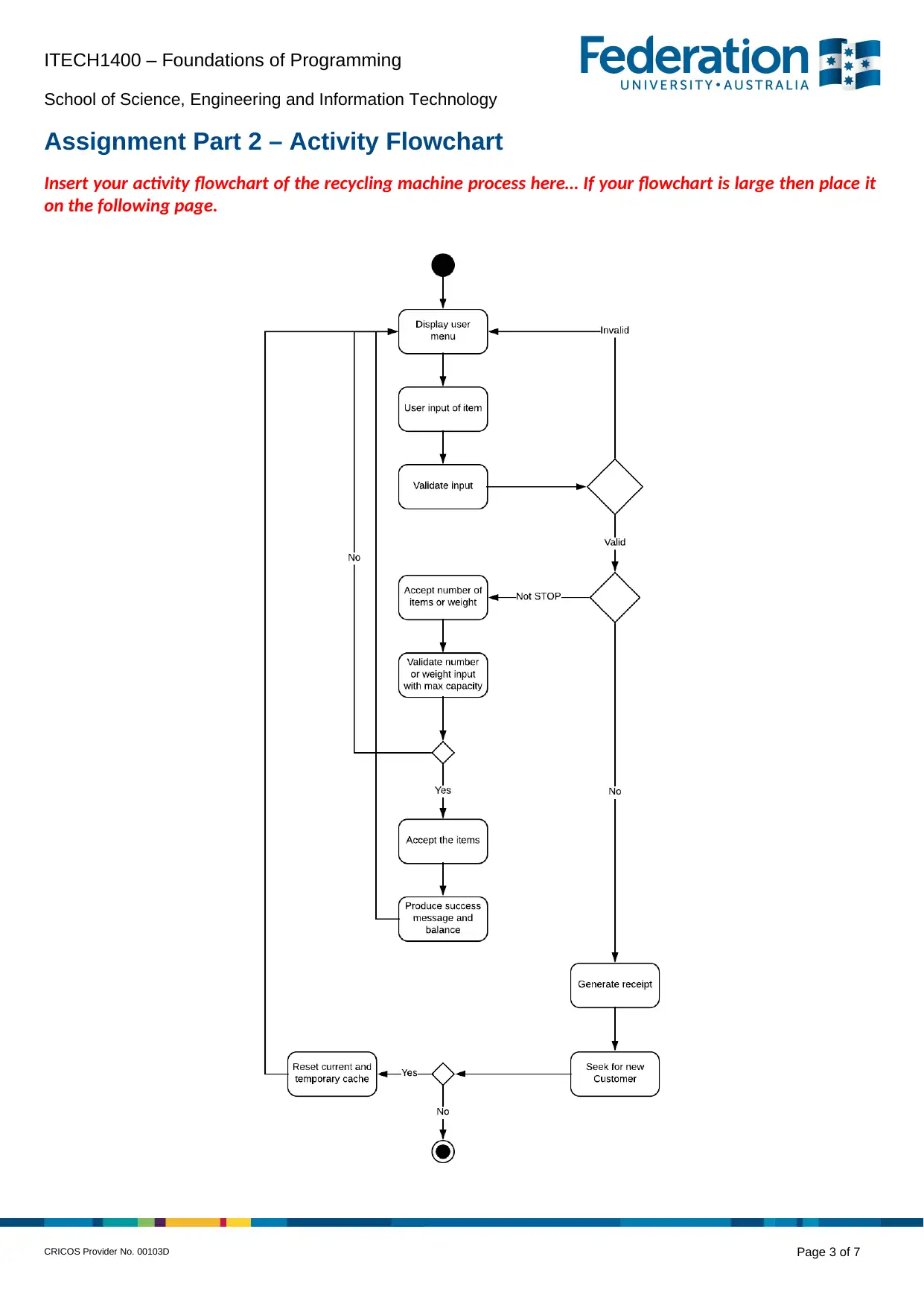
ITECH1400 – Foundations of Programming
School of Science, Engineering and Information Technology
Assignment Part 2 – Activity Flowchart
Insert your activity flowchart of the recycling machine process here… If your flowchart is large then place it
on the following page.
CRICOS Provider No. 00103D Page 3 of 7
School of Science, Engineering and Information Technology
Assignment Part 2 – Activity Flowchart
Insert your activity flowchart of the recycling machine process here… If your flowchart is large then place it
on the following page.
CRICOS Provider No. 00103D Page 3 of 7
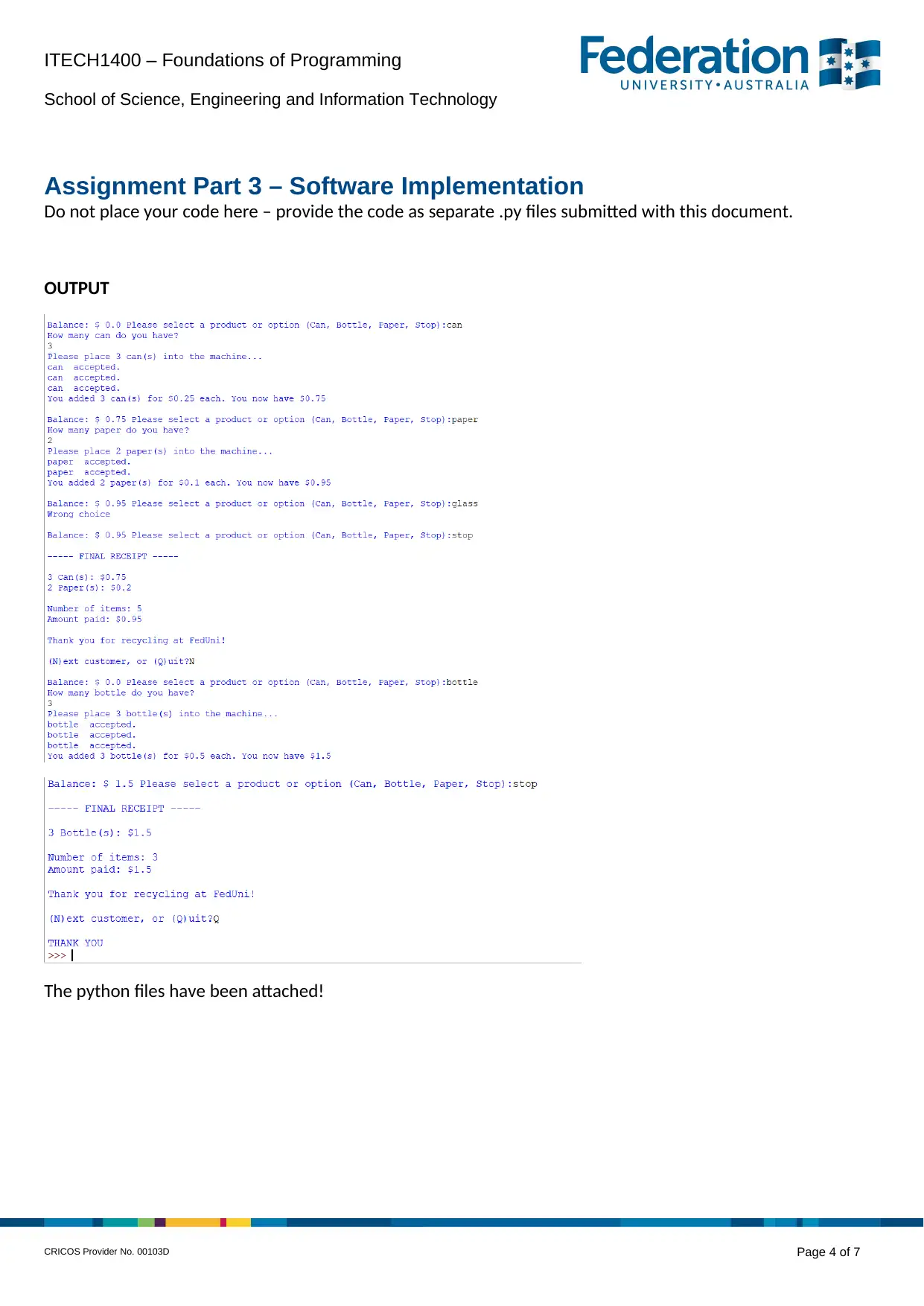
ITECH1400 – Foundations of Programming
School of Science, Engineering and Information Technology
Assignment Part 3 – Software Implementation
Do not place your code here – provide the code as separate .py files submitted with this document.
OUTPUT
The python files have been attached!
CRICOS Provider No. 00103D Page 4 of 7
School of Science, Engineering and Information Technology
Assignment Part 3 – Software Implementation
Do not place your code here – provide the code as separate .py files submitted with this document.
OUTPUT
The python files have been attached!
CRICOS Provider No. 00103D Page 4 of 7
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
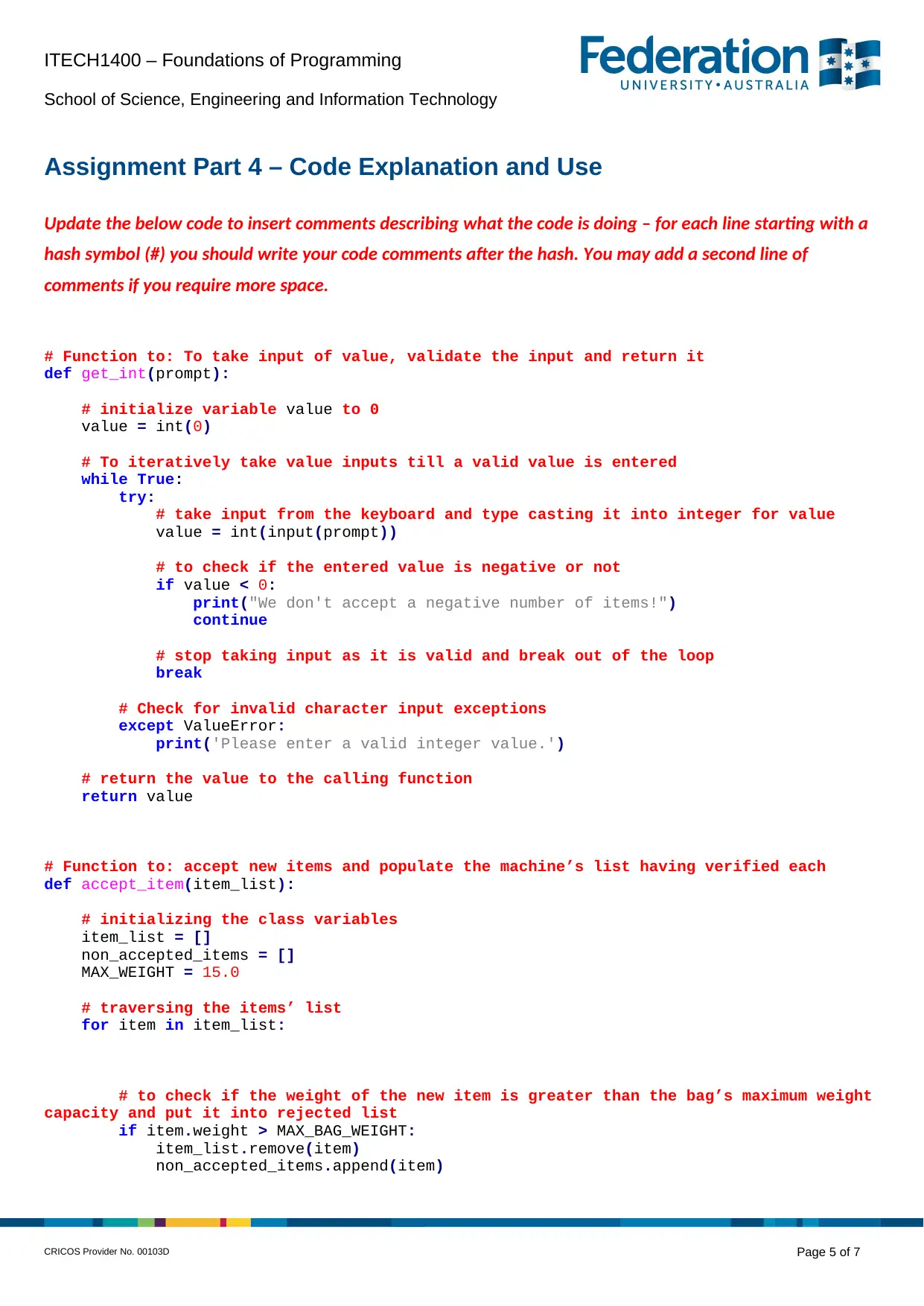
ITECH1400 – Foundations of Programming
School of Science, Engineering and Information Technology
Assignment Part 4 – Code Explanation and Use
Update the below code to insert comments describing what the code is doing – for each line starting with a
hash symbol (#) you should write your code comments after the hash. You may add a second line of
comments if you require more space.
# Function to: To take input of value, validate the input and return it
def get_int(prompt):
# initialize variable value to 0
value = int(0)
# To iteratively take value inputs till a valid value is entered
while True:
try:
# take input from the keyboard and type casting it into integer for value
value = int(input(prompt))
# to check if the entered value is negative or not
if value < 0:
print("We don't accept a negative number of items!")
continue
# stop taking input as it is valid and break out of the loop
break
# Check for invalid character input exceptions
except ValueError:
print('Please enter a valid integer value.')
# return the value to the calling function
return value
# Function to: accept new items and populate the machine’s list having verified each
def accept_item(item_list):
# initializing the class variables
item_list = []
non_accepted_items = []
MAX_WEIGHT = 15.0
# traversing the items’ list
for item in item_list:
# to check if the weight of the new item is greater than the bag’s maximum weight
capacity and put it into rejected list
if item.weight > MAX_BAG_WEIGHT:
item_list.remove(item)
non_accepted_items.append(item)
CRICOS Provider No. 00103D Page 5 of 7
School of Science, Engineering and Information Technology
Assignment Part 4 – Code Explanation and Use
Update the below code to insert comments describing what the code is doing – for each line starting with a
hash symbol (#) you should write your code comments after the hash. You may add a second line of
comments if you require more space.
# Function to: To take input of value, validate the input and return it
def get_int(prompt):
# initialize variable value to 0
value = int(0)
# To iteratively take value inputs till a valid value is entered
while True:
try:
# take input from the keyboard and type casting it into integer for value
value = int(input(prompt))
# to check if the entered value is negative or not
if value < 0:
print("We don't accept a negative number of items!")
continue
# stop taking input as it is valid and break out of the loop
break
# Check for invalid character input exceptions
except ValueError:
print('Please enter a valid integer value.')
# return the value to the calling function
return value
# Function to: accept new items and populate the machine’s list having verified each
def accept_item(item_list):
# initializing the class variables
item_list = []
non_accepted_items = []
MAX_WEIGHT = 15.0
# traversing the items’ list
for item in item_list:
# to check if the weight of the new item is greater than the bag’s maximum weight
capacity and put it into rejected list
if item.weight > MAX_BAG_WEIGHT:
item_list.remove(item)
non_accepted_items.append(item)
CRICOS Provider No. 00103D Page 5 of 7
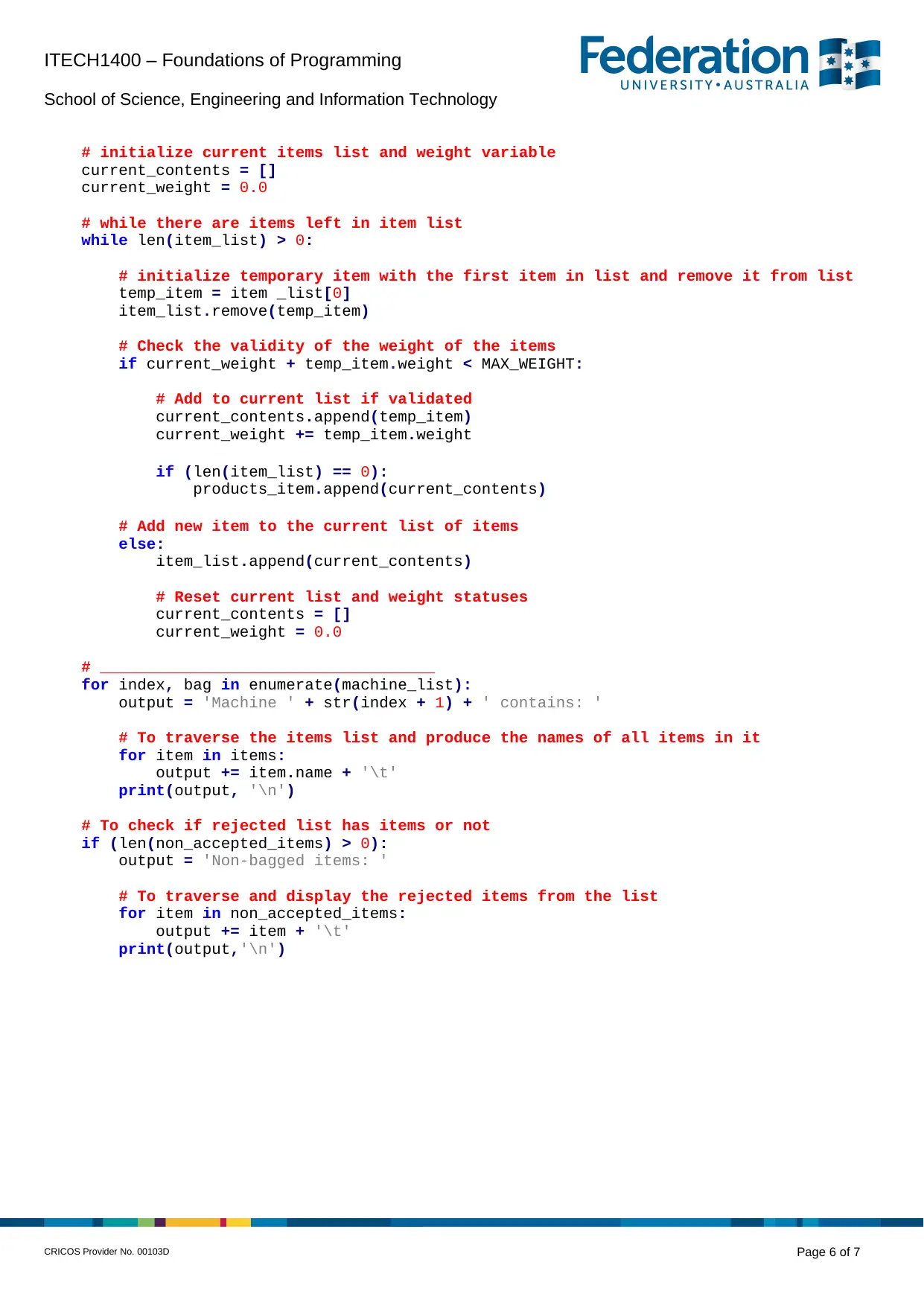
ITECH1400 – Foundations of Programming
School of Science, Engineering and Information Technology
# initialize current items list and weight variable
current_contents = []
current_weight = 0.0
# while there are items left in item list
while len(item_list) > 0:
# initialize temporary item with the first item in list and remove it from list
temp_item = item _list[0]
item_list.remove(temp_item)
# Check the validity of the weight of the items
if current_weight + temp_item.weight < MAX_WEIGHT:
# Add to current list if validated
current_contents.append(temp_item)
current_weight += temp_item.weight
if (len(item_list) == 0):
products_item.append(current_contents)
# Add new item to the current list of items
else:
item_list.append(current_contents)
# Reset current list and weight statuses
current_contents = []
current_weight = 0.0
# ____________________________________
for index, bag in enumerate(machine_list):
output = 'Machine ' + str(index + 1) + ' contains: '
# To traverse the items list and produce the names of all items in it
for item in items:
output += item.name + '\t'
print(output, '\n')
# To check if rejected list has items or not
if (len(non_accepted_items) > 0):
output = 'Non-bagged items: '
# To traverse and display the rejected items from the list
for item in non_accepted_items:
output += item + '\t'
print(output,'\n')
CRICOS Provider No. 00103D Page 6 of 7
School of Science, Engineering and Information Technology
# initialize current items list and weight variable
current_contents = []
current_weight = 0.0
# while there are items left in item list
while len(item_list) > 0:
# initialize temporary item with the first item in list and remove it from list
temp_item = item _list[0]
item_list.remove(temp_item)
# Check the validity of the weight of the items
if current_weight + temp_item.weight < MAX_WEIGHT:
# Add to current list if validated
current_contents.append(temp_item)
current_weight += temp_item.weight
if (len(item_list) == 0):
products_item.append(current_contents)
# Add new item to the current list of items
else:
item_list.append(current_contents)
# Reset current list and weight statuses
current_contents = []
current_weight = 0.0
# ____________________________________
for index, bag in enumerate(machine_list):
output = 'Machine ' + str(index + 1) + ' contains: '
# To traverse the items list and produce the names of all items in it
for item in items:
output += item.name + '\t'
print(output, '\n')
# To check if rejected list has items or not
if (len(non_accepted_items) > 0):
output = 'Non-bagged items: '
# To traverse and display the rejected items from the list
for item in non_accepted_items:
output += item + '\t'
print(output,'\n')
CRICOS Provider No. 00103D Page 6 of 7
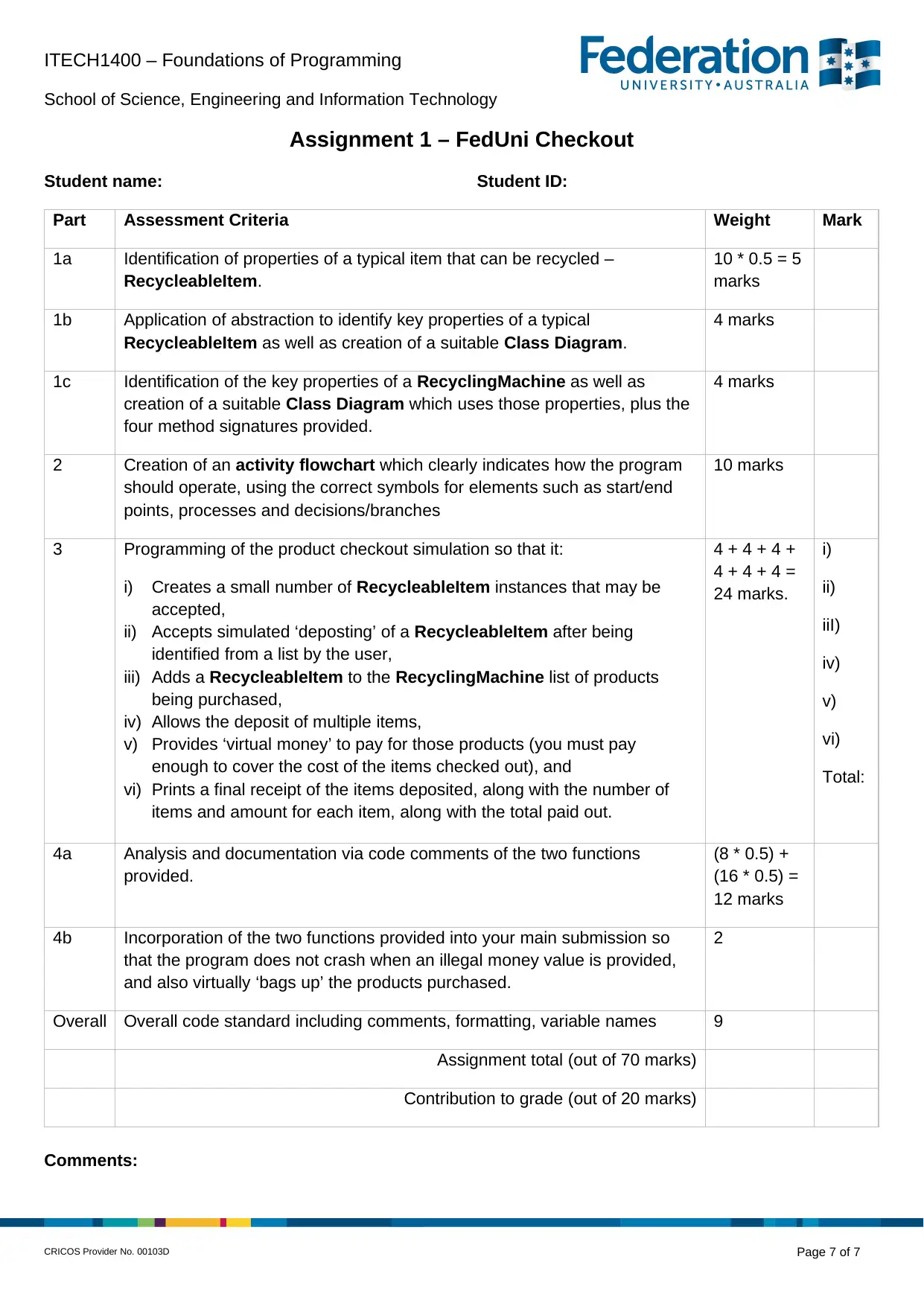
ITECH1400 – Foundations of Programming
School of Science, Engineering and Information Technology
Assignment 1 – FedUni Checkout
Student name: Student ID:
Part Assessment Criteria Weight Mark
1a Identification of properties of a typical item that can be recycled –
RecycleableItem.
10 * 0.5 = 5
marks
1b Application of abstraction to identify key properties of a typical
RecycleableItem as well as creation of a suitable Class Diagram.
4 marks
1c Identification of the key properties of a RecyclingMachine as well as
creation of a suitable Class Diagram which uses those properties, plus the
four method signatures provided.
4 marks
2 Creation of an activity flowchart which clearly indicates how the program
should operate, using the correct symbols for elements such as start/end
points, processes and decisions/branches
10 marks
3 Programming of the product checkout simulation so that it:
i) Creates a small number of RecycleableItem instances that may be
accepted,
ii) Accepts simulated ‘deposting’ of a RecycleableItem after being
identified from a list by the user,
iii) Adds a RecycleableItem to the RecyclingMachine list of products
being purchased,
iv) Allows the deposit of multiple items,
v) Provides ‘virtual money’ to pay for those products (you must pay
enough to cover the cost of the items checked out), and
vi) Prints a final receipt of the items deposited, along with the number of
items and amount for each item, along with the total paid out.
4 + 4 + 4 +
4 + 4 + 4 =
24 marks.
i)
ii)
iiI)
iv)
v)
vi)
Total:
4a Analysis and documentation via code comments of the two functions
provided.
(8 * 0.5) +
(16 * 0.5) =
12 marks
4b Incorporation of the two functions provided into your main submission so
that the program does not crash when an illegal money value is provided,
and also virtually ‘bags up’ the products purchased.
2
Overall Overall code standard including comments, formatting, variable names 9
Assignment total (out of 70 marks)
Contribution to grade (out of 20 marks)
Comments:
CRICOS Provider No. 00103D Page 7 of 7
School of Science, Engineering and Information Technology
Assignment 1 – FedUni Checkout
Student name: Student ID:
Part Assessment Criteria Weight Mark
1a Identification of properties of a typical item that can be recycled –
RecycleableItem.
10 * 0.5 = 5
marks
1b Application of abstraction to identify key properties of a typical
RecycleableItem as well as creation of a suitable Class Diagram.
4 marks
1c Identification of the key properties of a RecyclingMachine as well as
creation of a suitable Class Diagram which uses those properties, plus the
four method signatures provided.
4 marks
2 Creation of an activity flowchart which clearly indicates how the program
should operate, using the correct symbols for elements such as start/end
points, processes and decisions/branches
10 marks
3 Programming of the product checkout simulation so that it:
i) Creates a small number of RecycleableItem instances that may be
accepted,
ii) Accepts simulated ‘deposting’ of a RecycleableItem after being
identified from a list by the user,
iii) Adds a RecycleableItem to the RecyclingMachine list of products
being purchased,
iv) Allows the deposit of multiple items,
v) Provides ‘virtual money’ to pay for those products (you must pay
enough to cover the cost of the items checked out), and
vi) Prints a final receipt of the items deposited, along with the number of
items and amount for each item, along with the total paid out.
4 + 4 + 4 +
4 + 4 + 4 =
24 marks.
i)
ii)
iiI)
iv)
v)
vi)
Total:
4a Analysis and documentation via code comments of the two functions
provided.
(8 * 0.5) +
(16 * 0.5) =
12 marks
4b Incorporation of the two functions provided into your main submission so
that the program does not crash when an illegal money value is provided,
and also virtually ‘bags up’ the products purchased.
2
Overall Overall code standard including comments, formatting, variable names 9
Assignment total (out of 70 marks)
Contribution to grade (out of 20 marks)
Comments:
CRICOS Provider No. 00103D Page 7 of 7
1 out of 7
Related Documents

Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.