JAVA ASSIGNMENT
VerifiedAdded on  2024/04/11
|18
|1815
|327
AI Summary
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
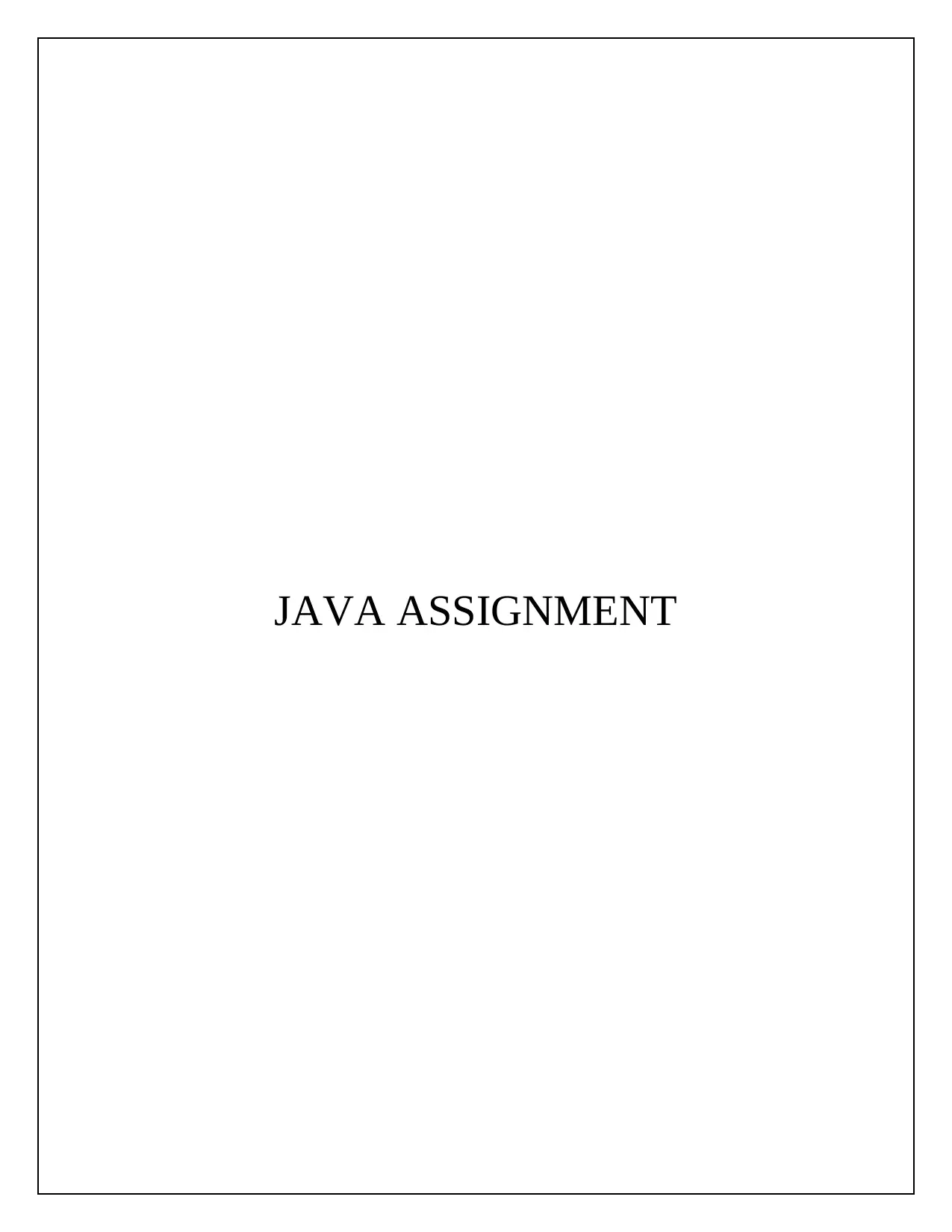
JAVA ASSIGNMENT
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
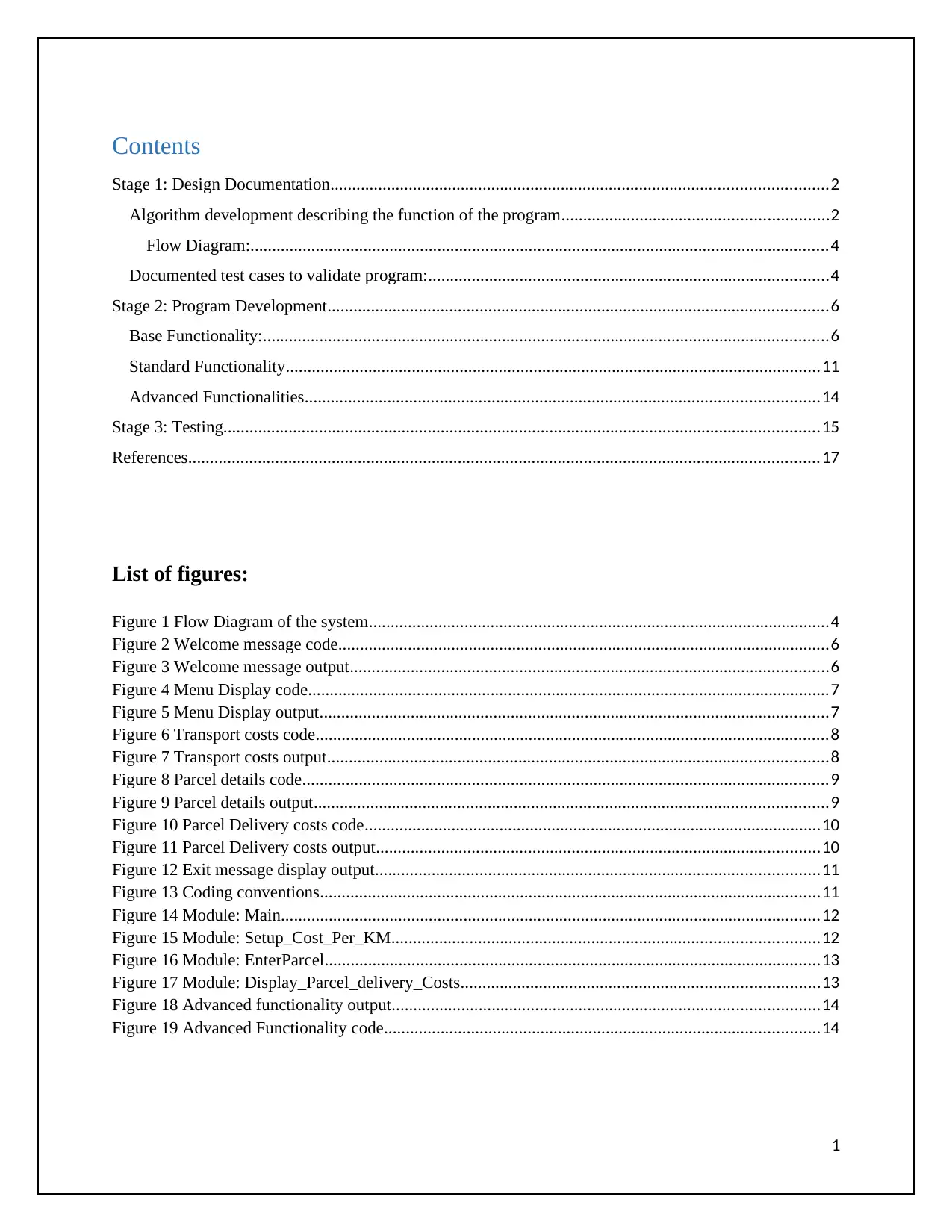
Contents
Stage 1: Design Documentation..................................................................................................................2
Algorithm development describing the function of the program.............................................................2
Flow Diagram:.....................................................................................................................................4
Documented test cases to validate program:............................................................................................4
Stage 2: Program Development...................................................................................................................6
Base Functionality:..................................................................................................................................6
Standard Functionality...........................................................................................................................11
Advanced Functionalities......................................................................................................................14
Stage 3: Testing.........................................................................................................................................15
References.................................................................................................................................................17
List of figures:
Figure 1 Flow Diagram of the system..........................................................................................................4
Figure 2 Welcome message code.................................................................................................................6
Figure 3 Welcome message output..............................................................................................................6
Figure 4 Menu Display code........................................................................................................................7
Figure 5 Menu Display output.....................................................................................................................7
Figure 6 Transport costs code......................................................................................................................8
Figure 7 Transport costs output...................................................................................................................8
Figure 8 Parcel details code.........................................................................................................................9
Figure 9 Parcel details output......................................................................................................................9
Figure 10 Parcel Delivery costs code.........................................................................................................10
Figure 11 Parcel Delivery costs output......................................................................................................10
Figure 12 Exit message display output......................................................................................................11
Figure 13 Coding conventions...................................................................................................................11
Figure 14 Module: Main............................................................................................................................12
Figure 15 Module: Setup_Cost_Per_KM..................................................................................................12
Figure 16 Module: EnterParcel..................................................................................................................13
Figure 17 Module: Display_Parcel_delivery_Costs..................................................................................13
Figure 18 Advanced functionality output..................................................................................................14
Figure 19 Advanced Functionality code....................................................................................................14
1
Stage 1: Design Documentation..................................................................................................................2
Algorithm development describing the function of the program.............................................................2
Flow Diagram:.....................................................................................................................................4
Documented test cases to validate program:............................................................................................4
Stage 2: Program Development...................................................................................................................6
Base Functionality:..................................................................................................................................6
Standard Functionality...........................................................................................................................11
Advanced Functionalities......................................................................................................................14
Stage 3: Testing.........................................................................................................................................15
References.................................................................................................................................................17
List of figures:
Figure 1 Flow Diagram of the system..........................................................................................................4
Figure 2 Welcome message code.................................................................................................................6
Figure 3 Welcome message output..............................................................................................................6
Figure 4 Menu Display code........................................................................................................................7
Figure 5 Menu Display output.....................................................................................................................7
Figure 6 Transport costs code......................................................................................................................8
Figure 7 Transport costs output...................................................................................................................8
Figure 8 Parcel details code.........................................................................................................................9
Figure 9 Parcel details output......................................................................................................................9
Figure 10 Parcel Delivery costs code.........................................................................................................10
Figure 11 Parcel Delivery costs output......................................................................................................10
Figure 12 Exit message display output......................................................................................................11
Figure 13 Coding conventions...................................................................................................................11
Figure 14 Module: Main............................................................................................................................12
Figure 15 Module: Setup_Cost_Per_KM..................................................................................................12
Figure 16 Module: EnterParcel..................................................................................................................13
Figure 17 Module: Display_Parcel_delivery_Costs..................................................................................13
Figure 18 Advanced functionality output..................................................................................................14
Figure 19 Advanced Functionality code....................................................................................................14
1
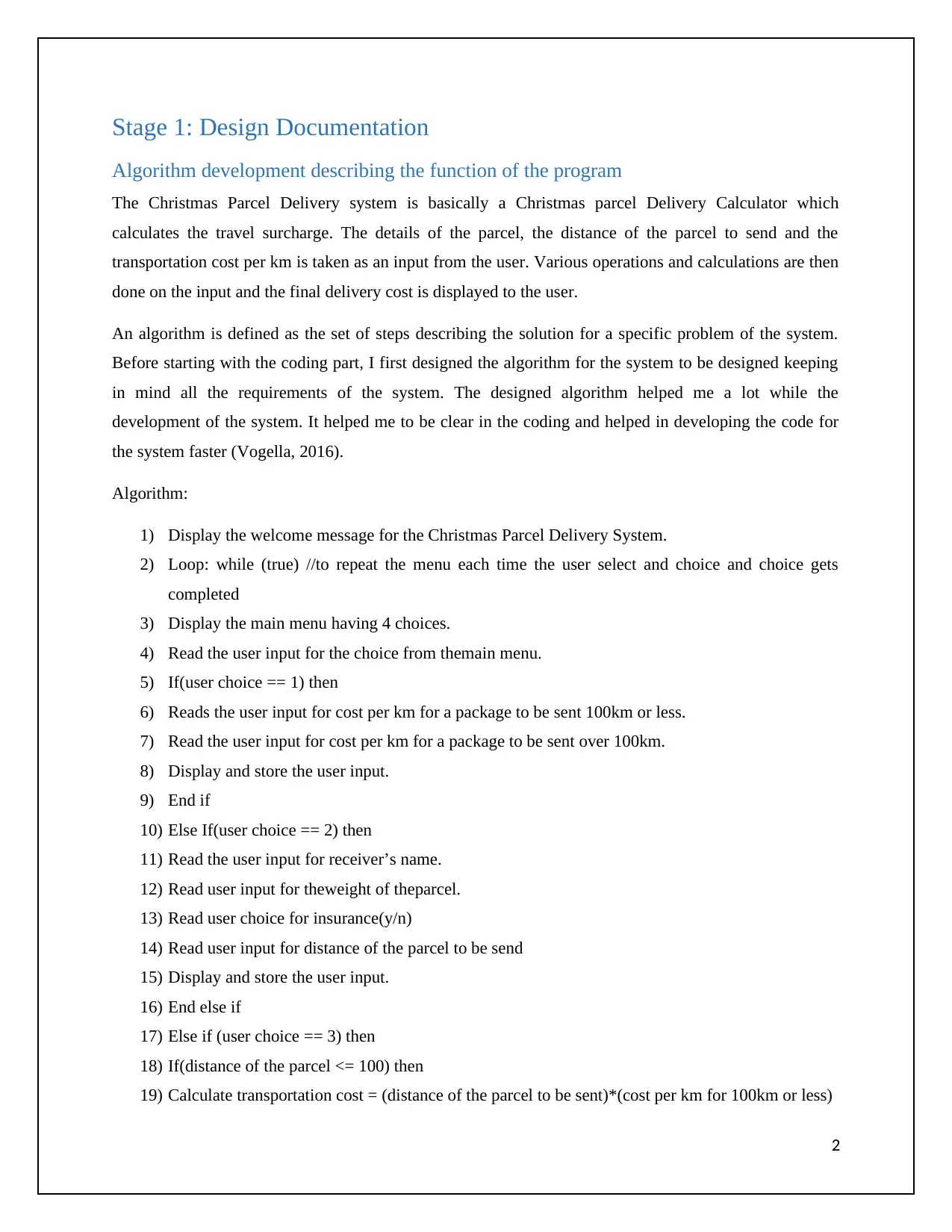
Stage 1: Design Documentation
Algorithm development describing the function of the program
The Christmas Parcel Delivery system is basically a Christmas parcel Delivery Calculator which
calculates the travel surcharge. The details of the parcel, the distance of the parcel to send and the
transportation cost per km is taken as an input from the user. Various operations and calculations are then
done on the input and the final delivery cost is displayed to the user.
An algorithm is defined as the set of steps describing the solution for a specific problem of the system.
Before starting with the coding part, I first designed the algorithm for the system to be designed keeping
in mind all the requirements of the system. The designed algorithm helped me a lot while the
development of the system. It helped me to be clear in the coding and helped in developing the code for
the system faster (Vogella, 2016).
Algorithm:
1) Display the welcome message for the Christmas Parcel Delivery System.
2) Loop: while (true) //to repeat the menu each time the user select and choice and choice gets
completed
3) Display the main menu having 4 choices.
4) Read the user input for the choice from themain menu.
5) If(user choice == 1) then
6) Reads the user input for cost per km for a package to be sent 100km or less.
7) Read the user input for cost per km for a package to be sent over 100km.
8) Display and store the user input.
9) End if
10) Else If(user choice == 2) then
11) Read the user input for receiver’s name.
12) Read user input for theweight of theparcel.
13) Read user choice for insurance(y/n)
14) Read user input for distance of the parcel to be send
15) Display and store the user input.
16) End else if
17) Else if (user choice == 3) then
18) If(distance of the parcel <= 100) then
19) Calculate transportation cost = (distance of the parcel to be sent)*(cost per km for 100km or less)
2
Algorithm development describing the function of the program
The Christmas Parcel Delivery system is basically a Christmas parcel Delivery Calculator which
calculates the travel surcharge. The details of the parcel, the distance of the parcel to send and the
transportation cost per km is taken as an input from the user. Various operations and calculations are then
done on the input and the final delivery cost is displayed to the user.
An algorithm is defined as the set of steps describing the solution for a specific problem of the system.
Before starting with the coding part, I first designed the algorithm for the system to be designed keeping
in mind all the requirements of the system. The designed algorithm helped me a lot while the
development of the system. It helped me to be clear in the coding and helped in developing the code for
the system faster (Vogella, 2016).
Algorithm:
1) Display the welcome message for the Christmas Parcel Delivery System.
2) Loop: while (true) //to repeat the menu each time the user select and choice and choice gets
completed
3) Display the main menu having 4 choices.
4) Read the user input for the choice from themain menu.
5) If(user choice == 1) then
6) Reads the user input for cost per km for a package to be sent 100km or less.
7) Read the user input for cost per km for a package to be sent over 100km.
8) Display and store the user input.
9) End if
10) Else If(user choice == 2) then
11) Read the user input for receiver’s name.
12) Read user input for theweight of theparcel.
13) Read user choice for insurance(y/n)
14) Read user input for distance of the parcel to be send
15) Display and store the user input.
16) End else if
17) Else if (user choice == 3) then
18) If(distance of the parcel <= 100) then
19) Calculate transportation cost = (distance of the parcel to be sent)*(cost per km for 100km or less)
2
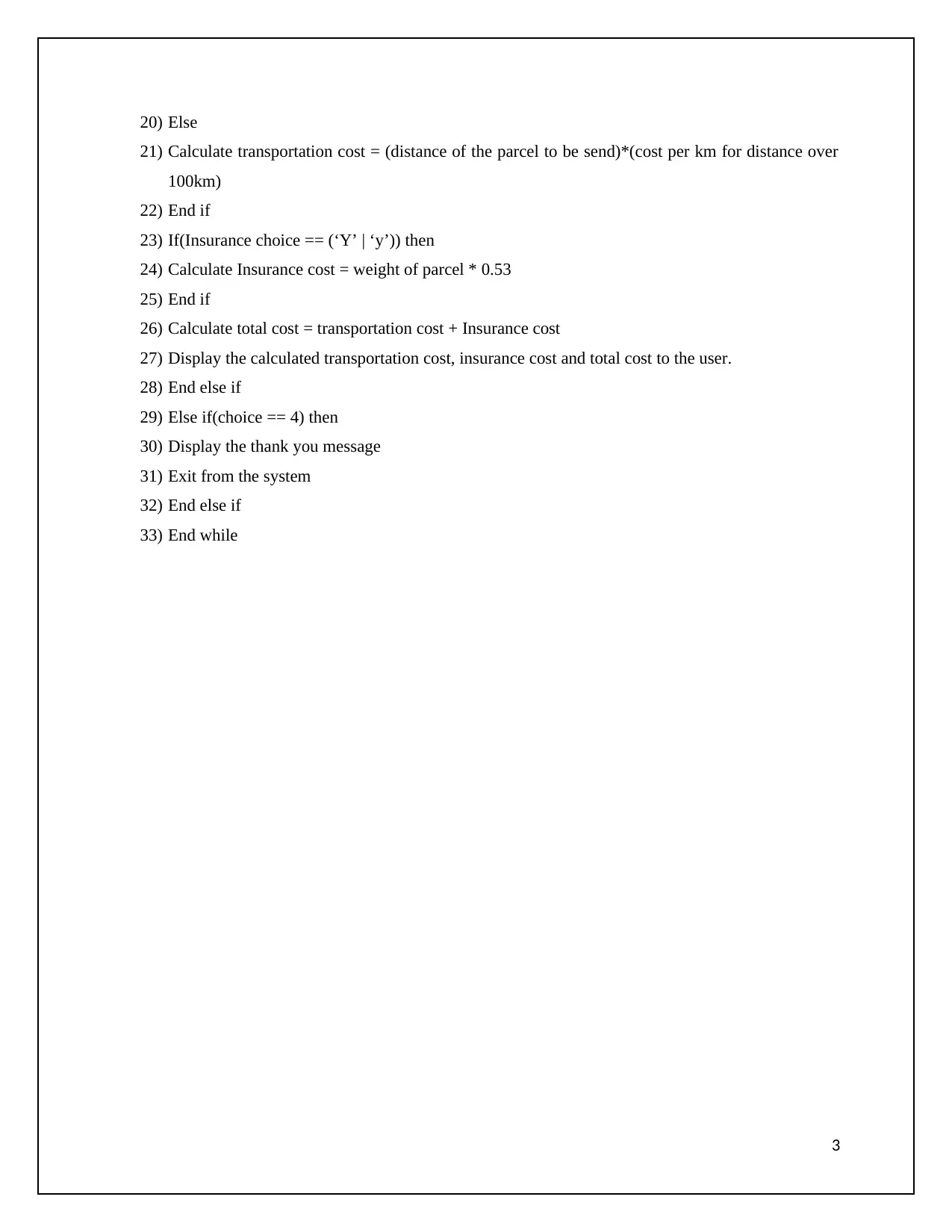
20) Else
21) Calculate transportation cost = (distance of the parcel to be send)*(cost per km for distance over
100km)
22) End if
23) If(Insurance choice == (‘Y’ | ‘y’)) then
24) Calculate Insurance cost = weight of parcel * 0.53
25) End if
26) Calculate total cost = transportation cost + Insurance cost
27) Display the calculated transportation cost, insurance cost and total cost to the user.
28) End else if
29) Else if(choice == 4) then
30) Display the thank you message
31) Exit from the system
32) End else if
33) End while
3
21) Calculate transportation cost = (distance of the parcel to be send)*(cost per km for distance over
100km)
22) End if
23) If(Insurance choice == (‘Y’ | ‘y’)) then
24) Calculate Insurance cost = weight of parcel * 0.53
25) End if
26) Calculate total cost = transportation cost + Insurance cost
27) Display the calculated transportation cost, insurance cost and total cost to the user.
28) End else if
29) Else if(choice == 4) then
30) Display the thank you message
31) Exit from the system
32) End else if
33) End while
3
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
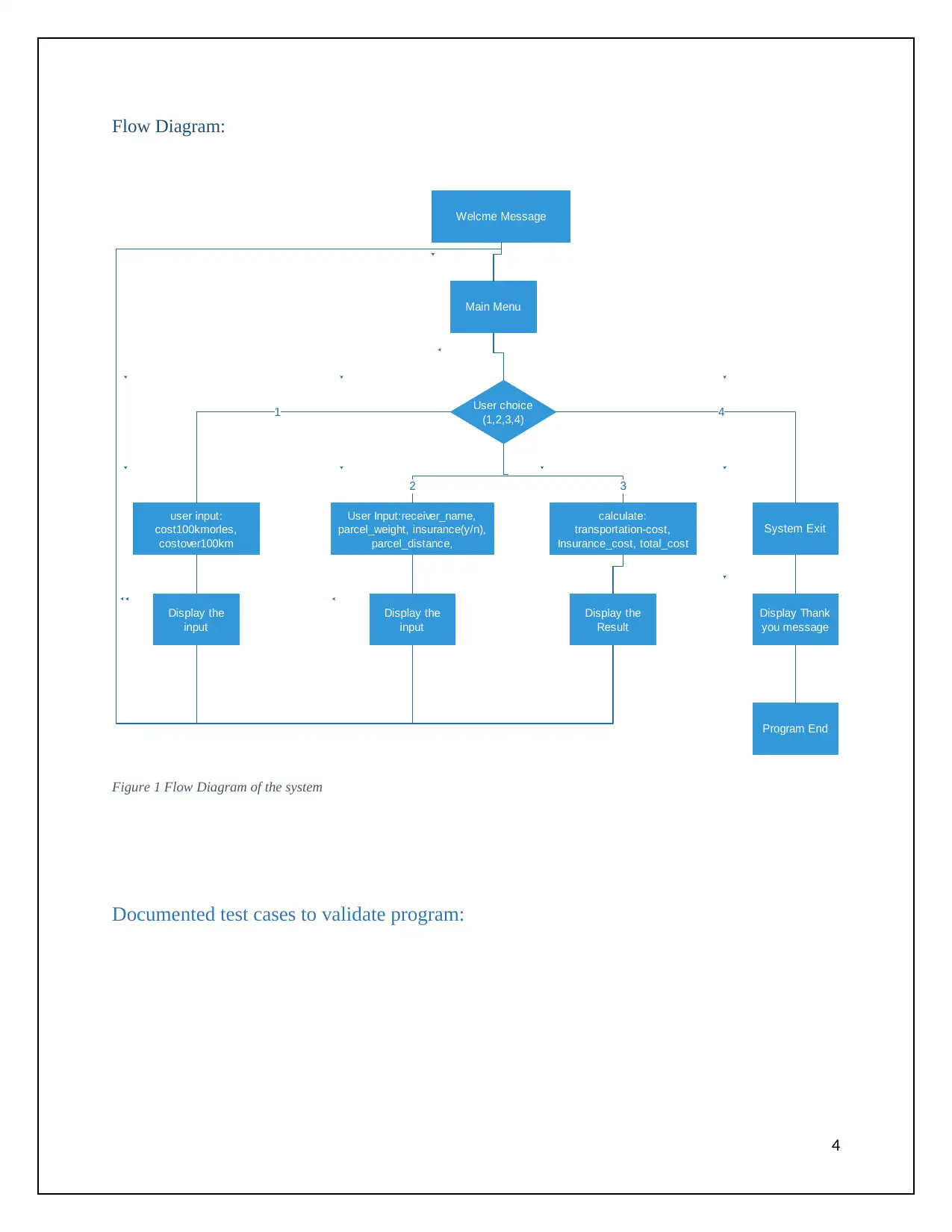
Flow Diagram:
Welcme Message
Main Menu
User choice
(1,2,3,4)
user input:
cost100kmorles,
costover100km
User Input:receiver_name,
parcel_weight, insurance(y/n),
parcel_distance,
calculate:
transportation-cost,
Insurance_cost, total_cost
System Exit
Display the
input
Display the
input
Display the
Result
Display Thank
you message
1
32
4
Program End
Figure 1 Flow Diagram of the system
Documented test cases to validate program:
4
Welcme Message
Main Menu
User choice
(1,2,3,4)
user input:
cost100kmorles,
costover100km
User Input:receiver_name,
parcel_weight, insurance(y/n),
parcel_distance,
calculate:
transportation-cost,
Insurance_cost, total_cost
System Exit
Display the
input
Display the
input
Display the
Result
Display Thank
you message
1
32
4
Program End
Figure 1 Flow Diagram of the system
Documented test cases to validate program:
4
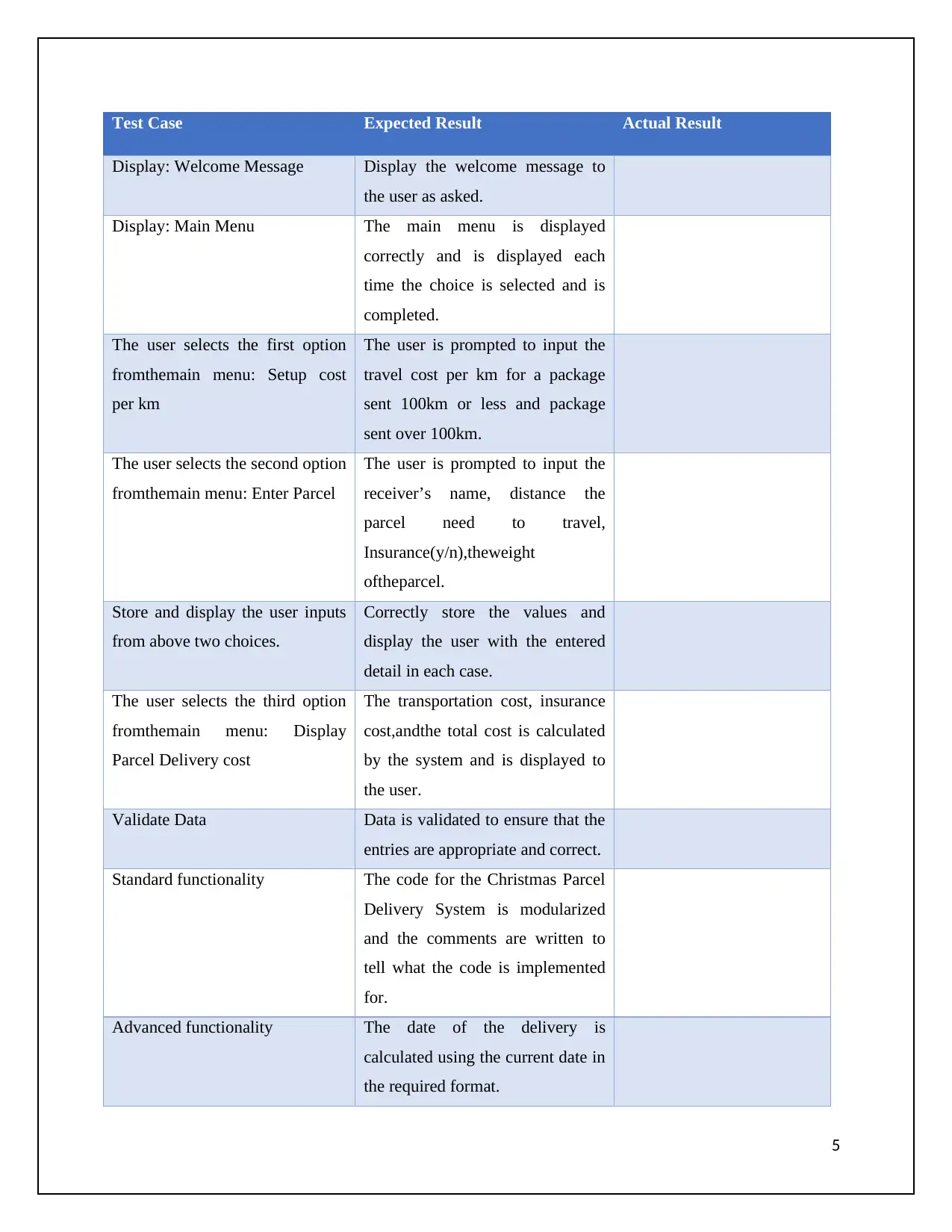
Test Case Expected Result Actual Result
Display: Welcome Message Display the welcome message to
the user as asked.
Display: Main Menu The main menu is displayed
correctly and is displayed each
time the choice is selected and is
completed.
The user selects the first option
fromthemain menu: Setup cost
per km
The user is prompted to input the
travel cost per km for a package
sent 100km or less and package
sent over 100km.
The user selects the second option
fromthemain menu: Enter Parcel
The user is prompted to input the
receiver’s name, distance the
parcel need to travel,
Insurance(y/n),theweight
oftheparcel.
Store and display the user inputs
from above two choices.
Correctly store the values and
display the user with the entered
detail in each case.
The user selects the third option
fromthemain menu: Display
Parcel Delivery cost
The transportation cost, insurance
cost,andthe total cost is calculated
by the system and is displayed to
the user.
Validate Data Data is validated to ensure that the
entries are appropriate and correct.
Standard functionality The code for the Christmas Parcel
Delivery System is modularized
and the comments are written to
tell what the code is implemented
for.
Advanced functionality The date of the delivery is
calculated using the current date in
the required format.
5
Display: Welcome Message Display the welcome message to
the user as asked.
Display: Main Menu The main menu is displayed
correctly and is displayed each
time the choice is selected and is
completed.
The user selects the first option
fromthemain menu: Setup cost
per km
The user is prompted to input the
travel cost per km for a package
sent 100km or less and package
sent over 100km.
The user selects the second option
fromthemain menu: Enter Parcel
The user is prompted to input the
receiver’s name, distance the
parcel need to travel,
Insurance(y/n),theweight
oftheparcel.
Store and display the user inputs
from above two choices.
Correctly store the values and
display the user with the entered
detail in each case.
The user selects the third option
fromthemain menu: Display
Parcel Delivery cost
The transportation cost, insurance
cost,andthe total cost is calculated
by the system and is displayed to
the user.
Validate Data Data is validated to ensure that the
entries are appropriate and correct.
Standard functionality The code for the Christmas Parcel
Delivery System is modularized
and the comments are written to
tell what the code is implemented
for.
Advanced functionality The date of the delivery is
calculated using the current date in
the required format.
5
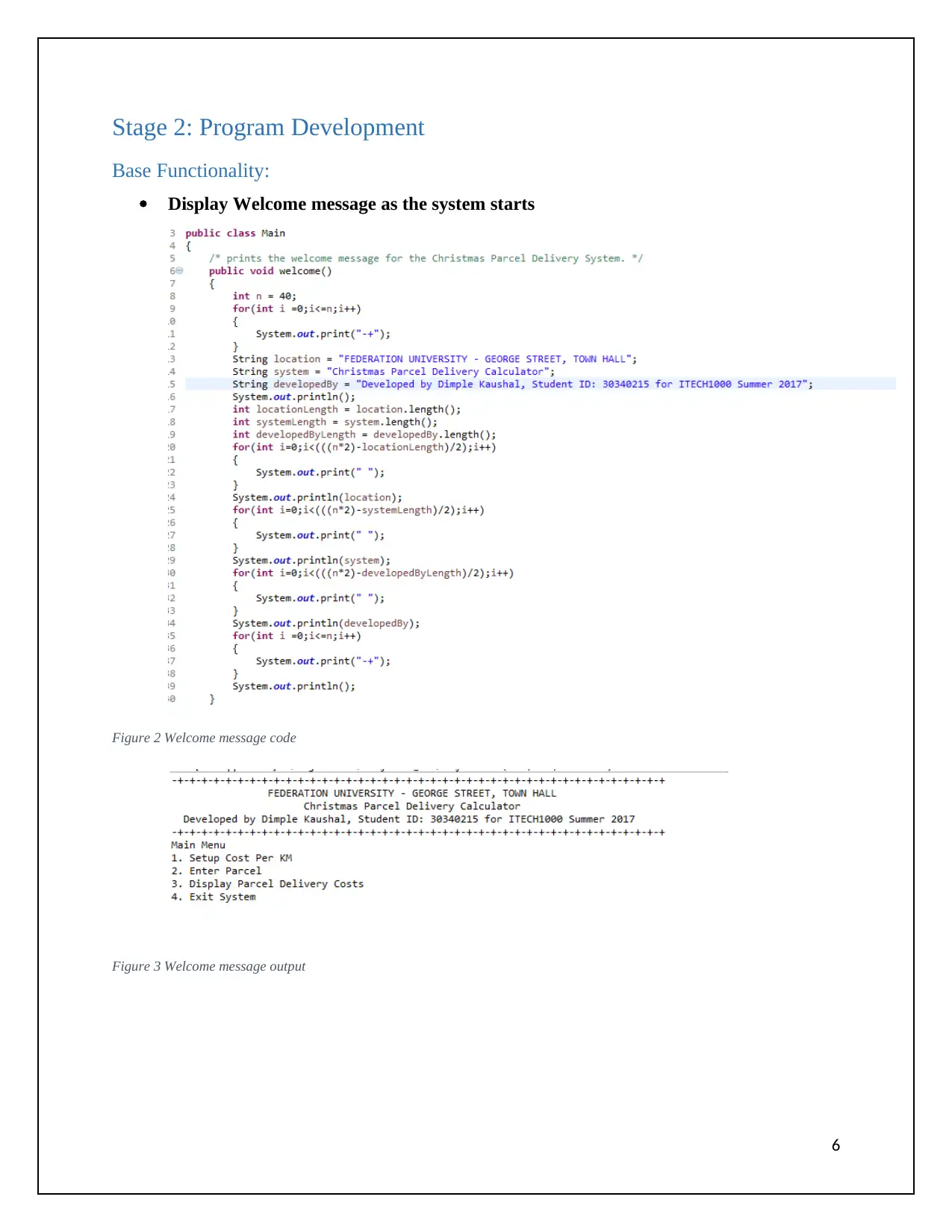
Stage 2: Program Development
Base Functionality:
ï‚· Display Welcome message as the system starts
Figure 2 Welcome message code
Figure 3 Welcome message output
6
Base Functionality:
ï‚· Display Welcome message as the system starts
Figure 2 Welcome message code
Figure 3 Welcome message output
6
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
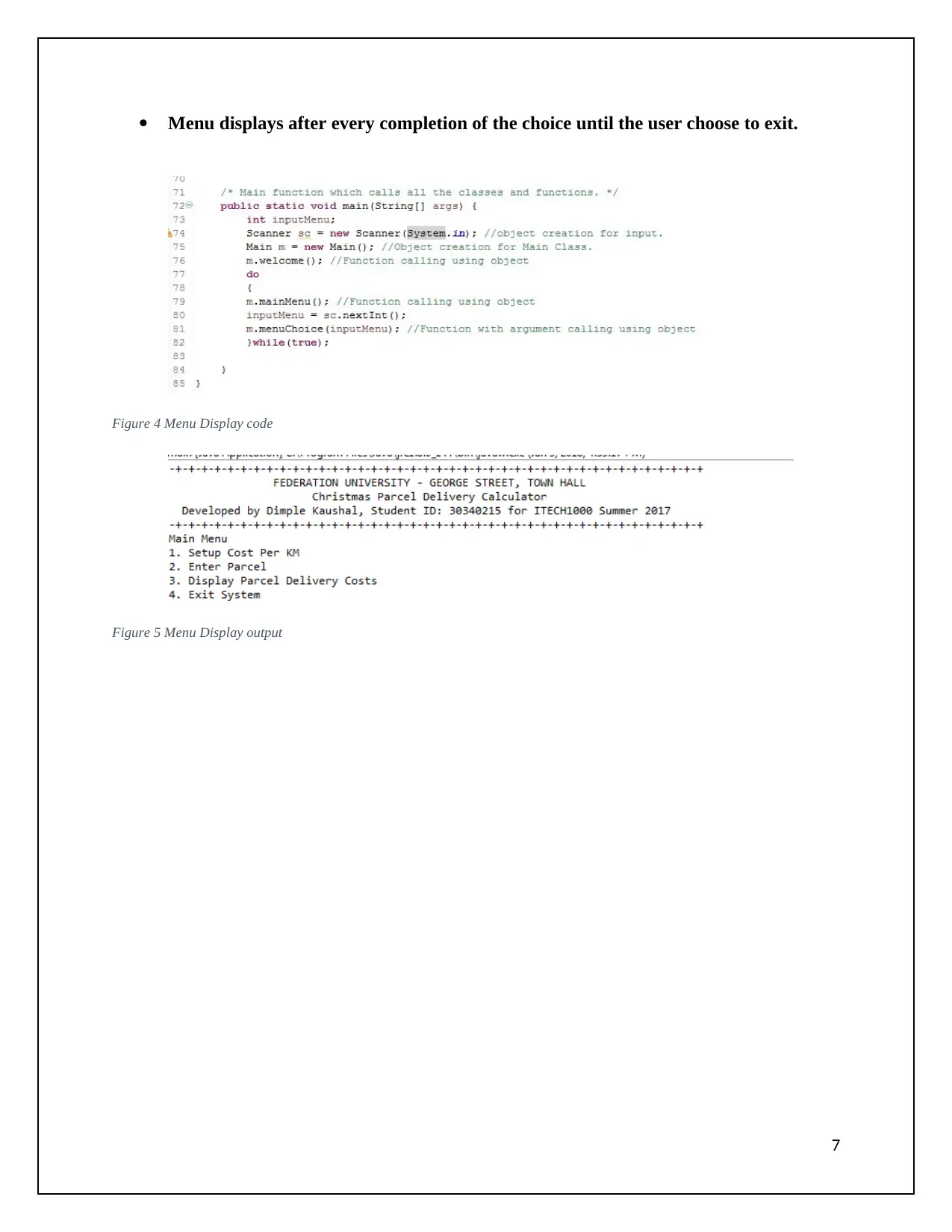
ï‚· Menu displays after every completion of the choice until the user choose to exit.
Figure 4 Menu Display code
Figure 5 Menu Display output
7
Figure 4 Menu Display code
Figure 5 Menu Display output
7
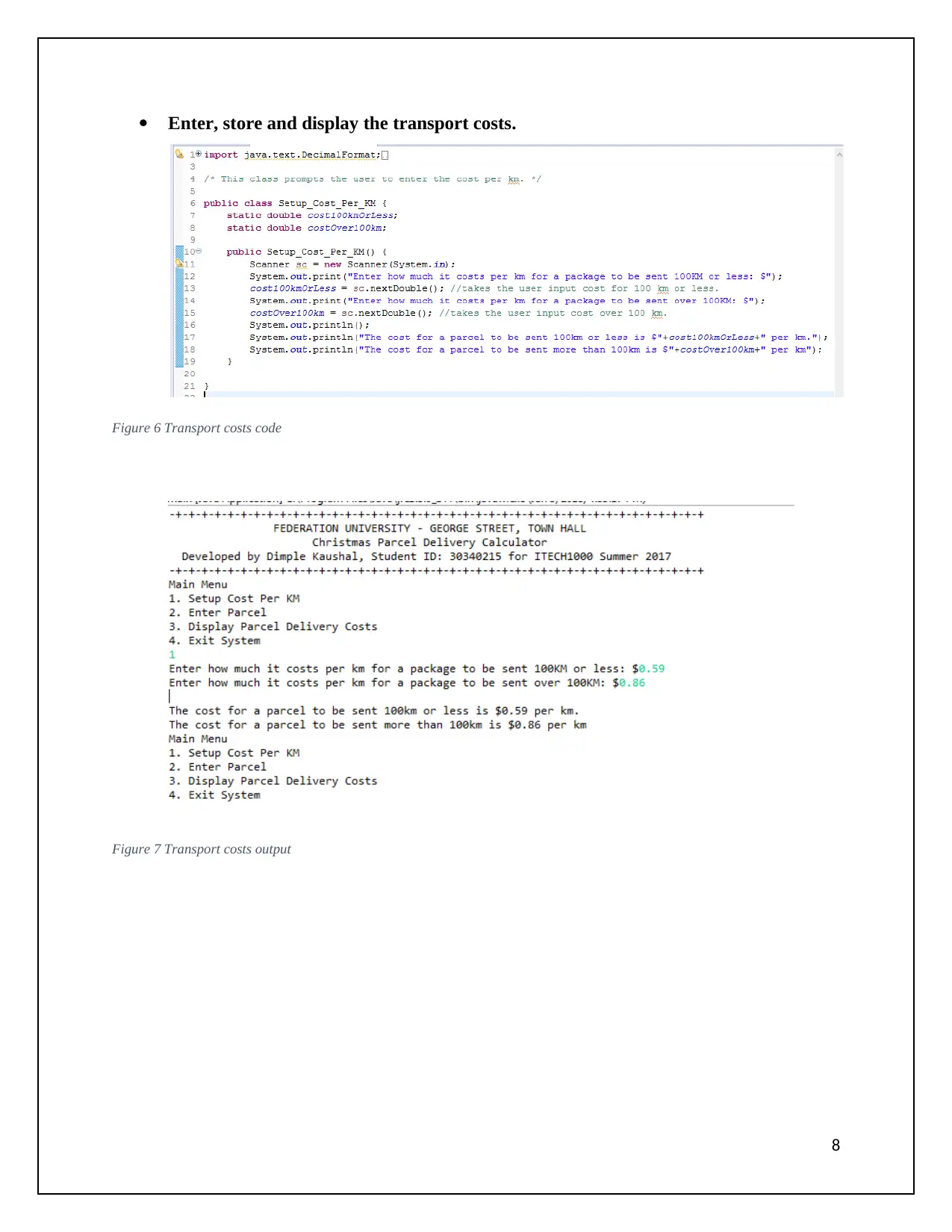
ï‚· Enter, store and display the transport costs.
Figure 6 Transport costs code
Figure 7 Transport costs output
8
Figure 6 Transport costs code
Figure 7 Transport costs output
8
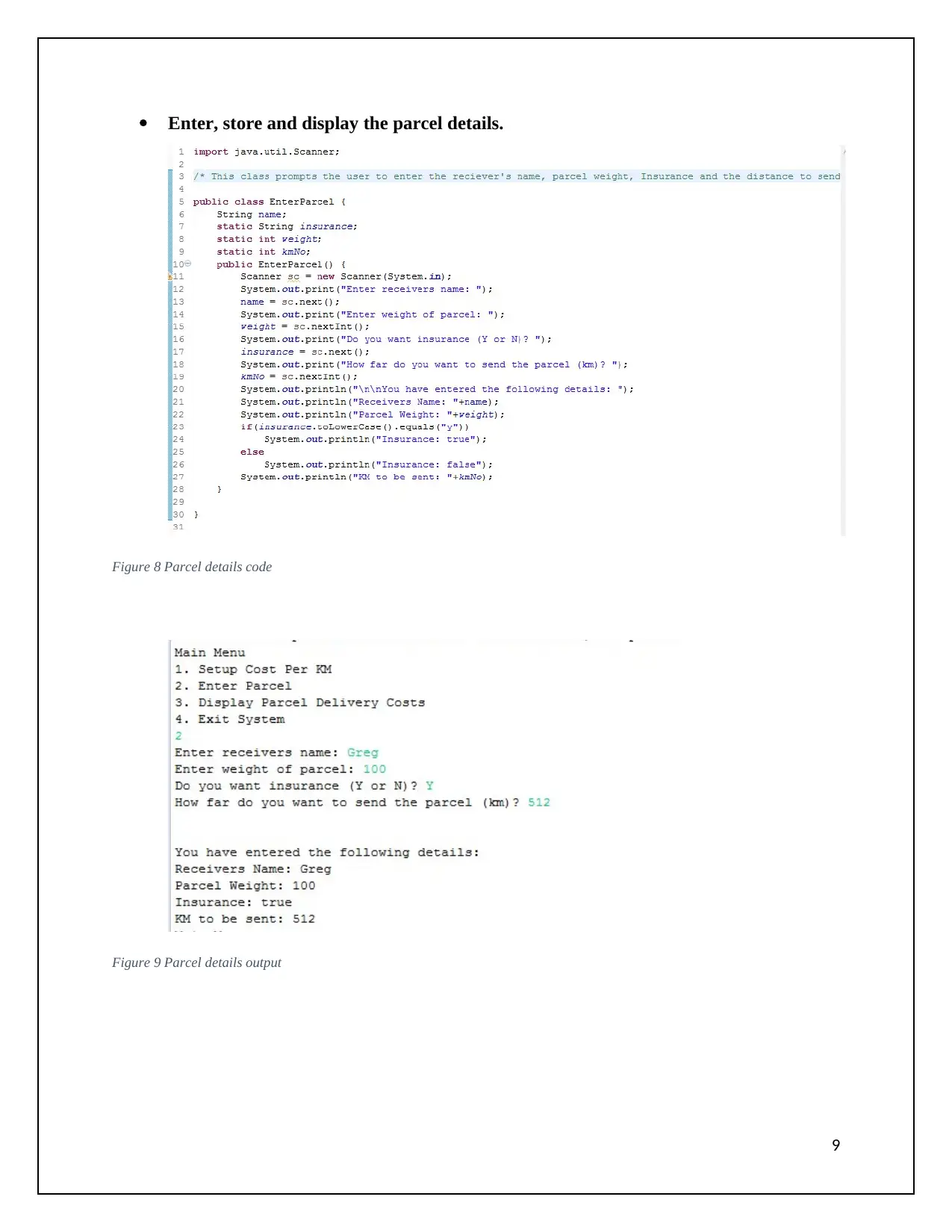
ï‚· Enter, store and display the parcel details.
Figure 8 Parcel details code
Figure 9 Parcel details output
9
Figure 8 Parcel details code
Figure 9 Parcel details output
9
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
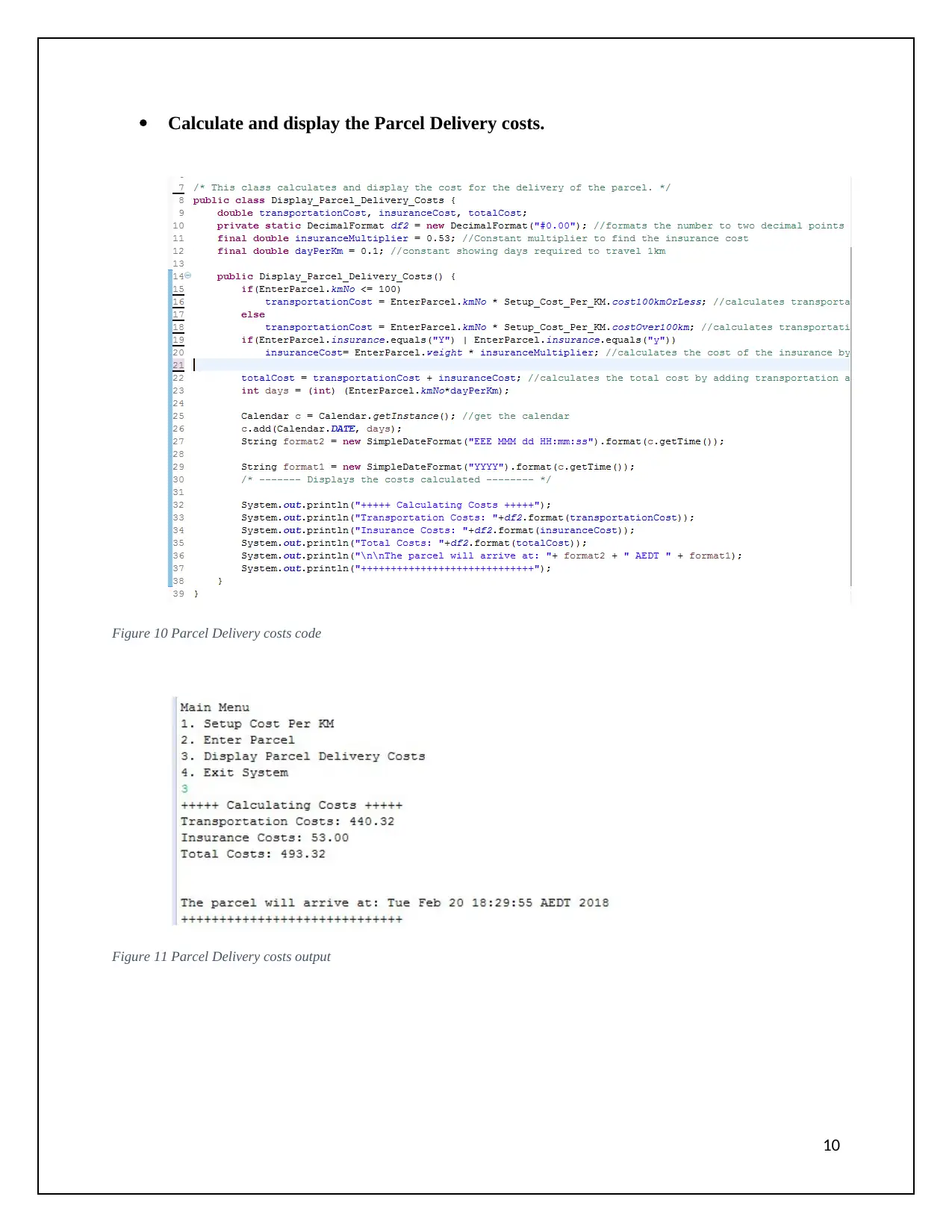
ï‚· Calculate and display the Parcel Delivery costs.
Figure 10 Parcel Delivery costs code
Figure 11 Parcel Delivery costs output
10
Figure 10 Parcel Delivery costs code
Figure 11 Parcel Delivery costs output
10
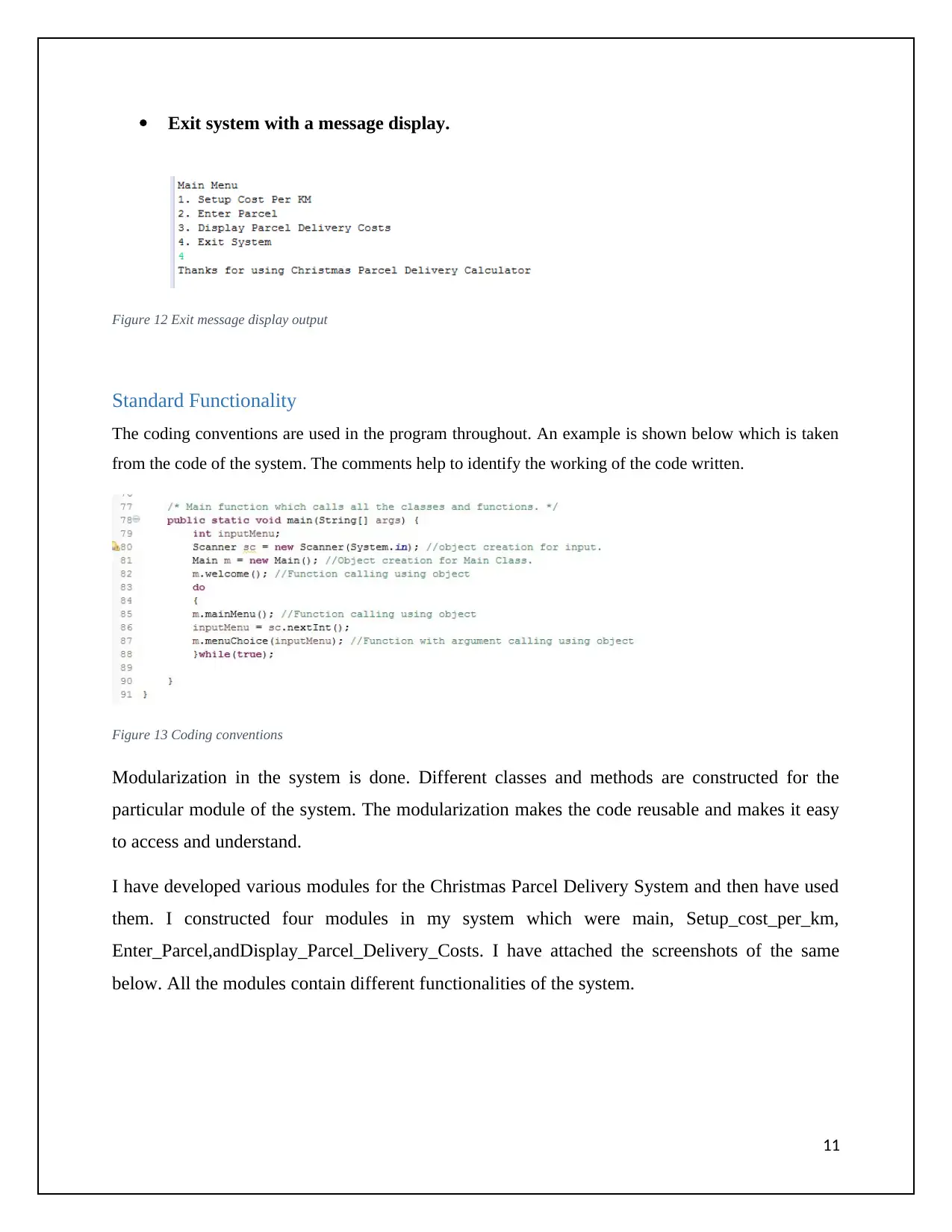
ï‚· Exit system with a message display.
Figure 12 Exit message display output
Standard Functionality
The coding conventions are used in the program throughout. An example is shown below which is taken
from the code of the system. The comments help to identify the working of the code written.
Figure 13 Coding conventions
Modularization in the system is done. Different classes and methods are constructed for the
particular module of the system. The modularization makes the code reusable and makes it easy
to access and understand.
I have developed various modules for the Christmas Parcel Delivery System and then have used
them. I constructed four modules in my system which were main, Setup_cost_per_km,
Enter_Parcel,andDisplay_Parcel_Delivery_Costs. I have attached the screenshots of the same
below. All the modules contain different functionalities of the system.
11
Figure 12 Exit message display output
Standard Functionality
The coding conventions are used in the program throughout. An example is shown below which is taken
from the code of the system. The comments help to identify the working of the code written.
Figure 13 Coding conventions
Modularization in the system is done. Different classes and methods are constructed for the
particular module of the system. The modularization makes the code reusable and makes it easy
to access and understand.
I have developed various modules for the Christmas Parcel Delivery System and then have used
them. I constructed four modules in my system which were main, Setup_cost_per_km,
Enter_Parcel,andDisplay_Parcel_Delivery_Costs. I have attached the screenshots of the same
below. All the modules contain different functionalities of the system.
11
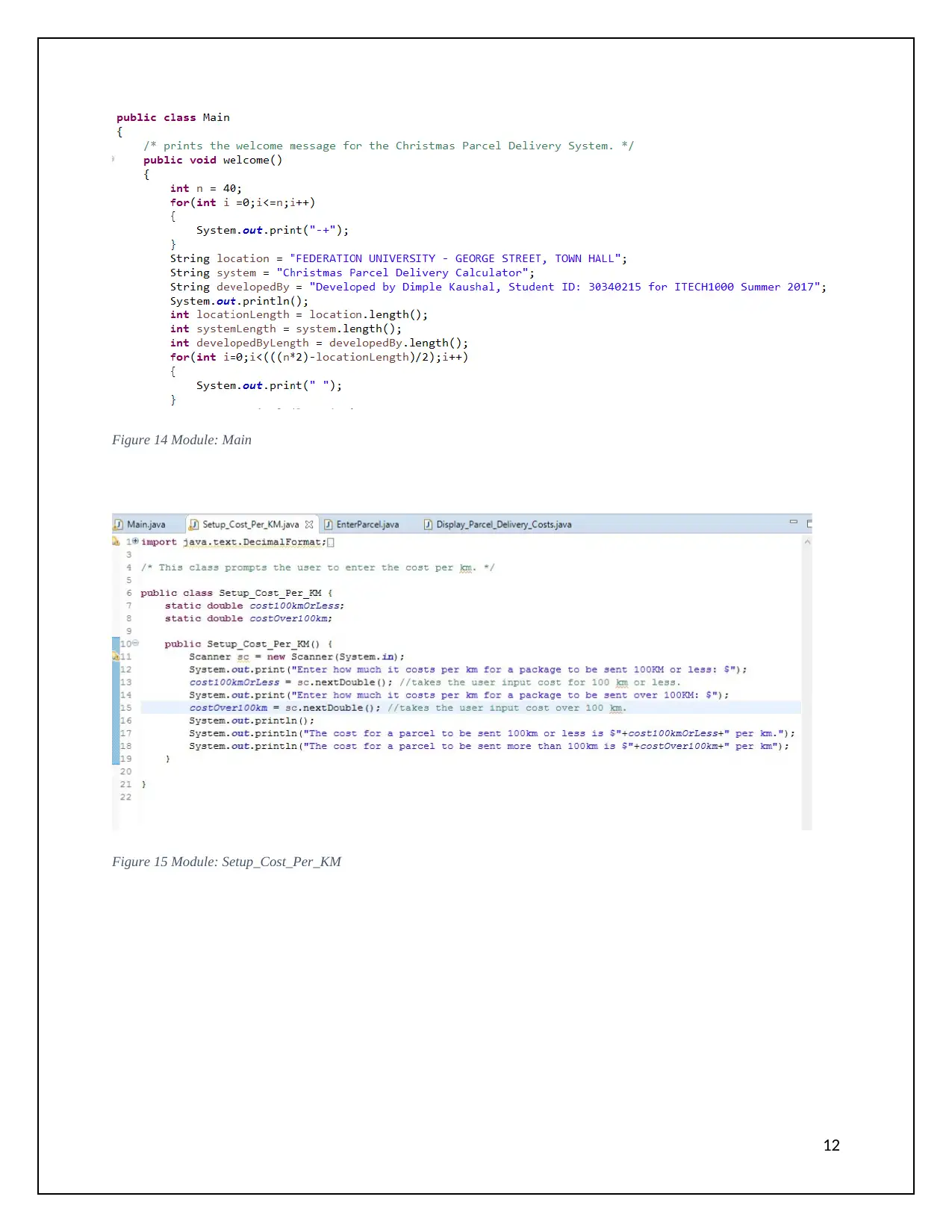
Figure 14 Module: Main
Figure 15 Module: Setup_Cost_Per_KM
12
Figure 15 Module: Setup_Cost_Per_KM
12
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
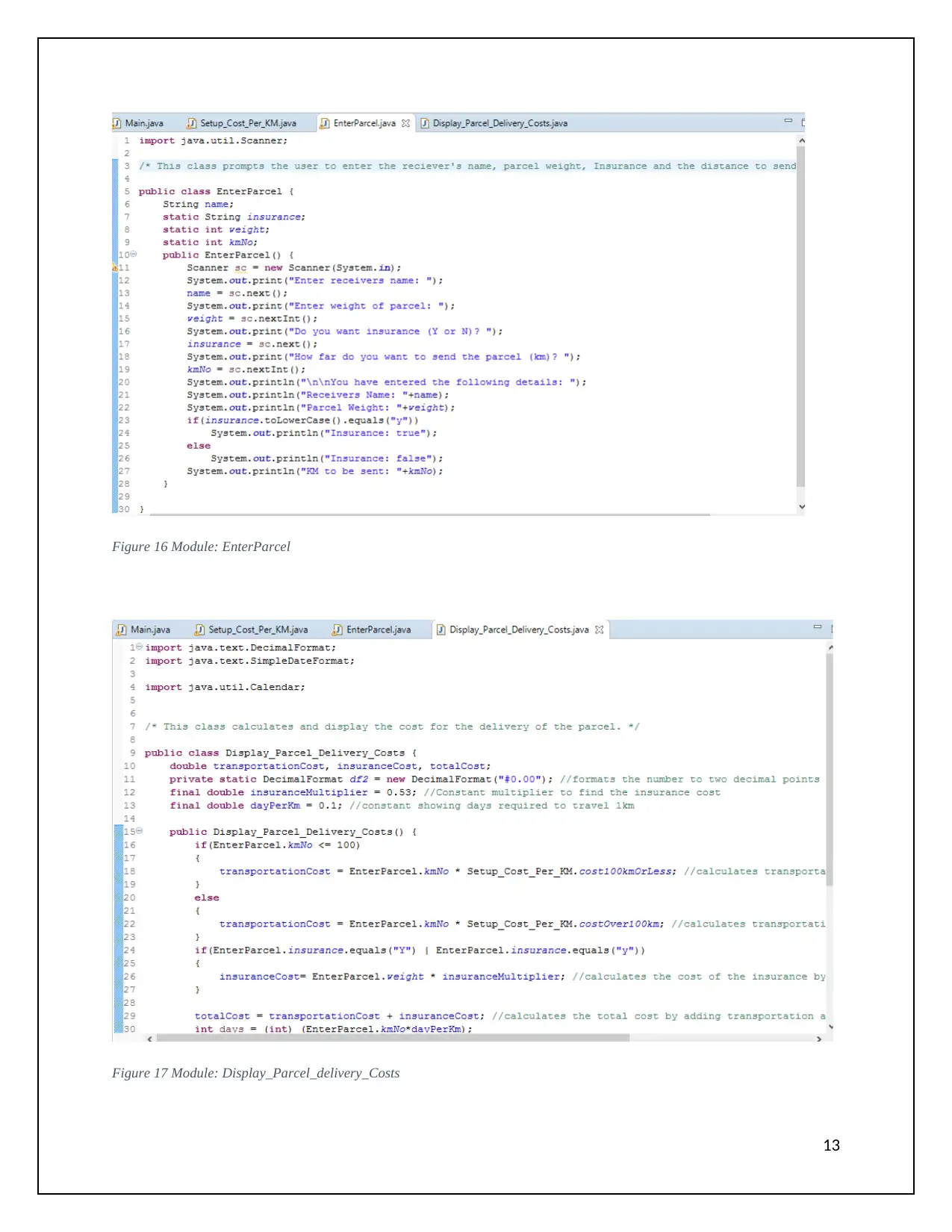
Figure 16 Module: EnterParcel
Figure 17 Module: Display_Parcel_delivery_Costs
13
Figure 17 Module: Display_Parcel_delivery_Costs
13
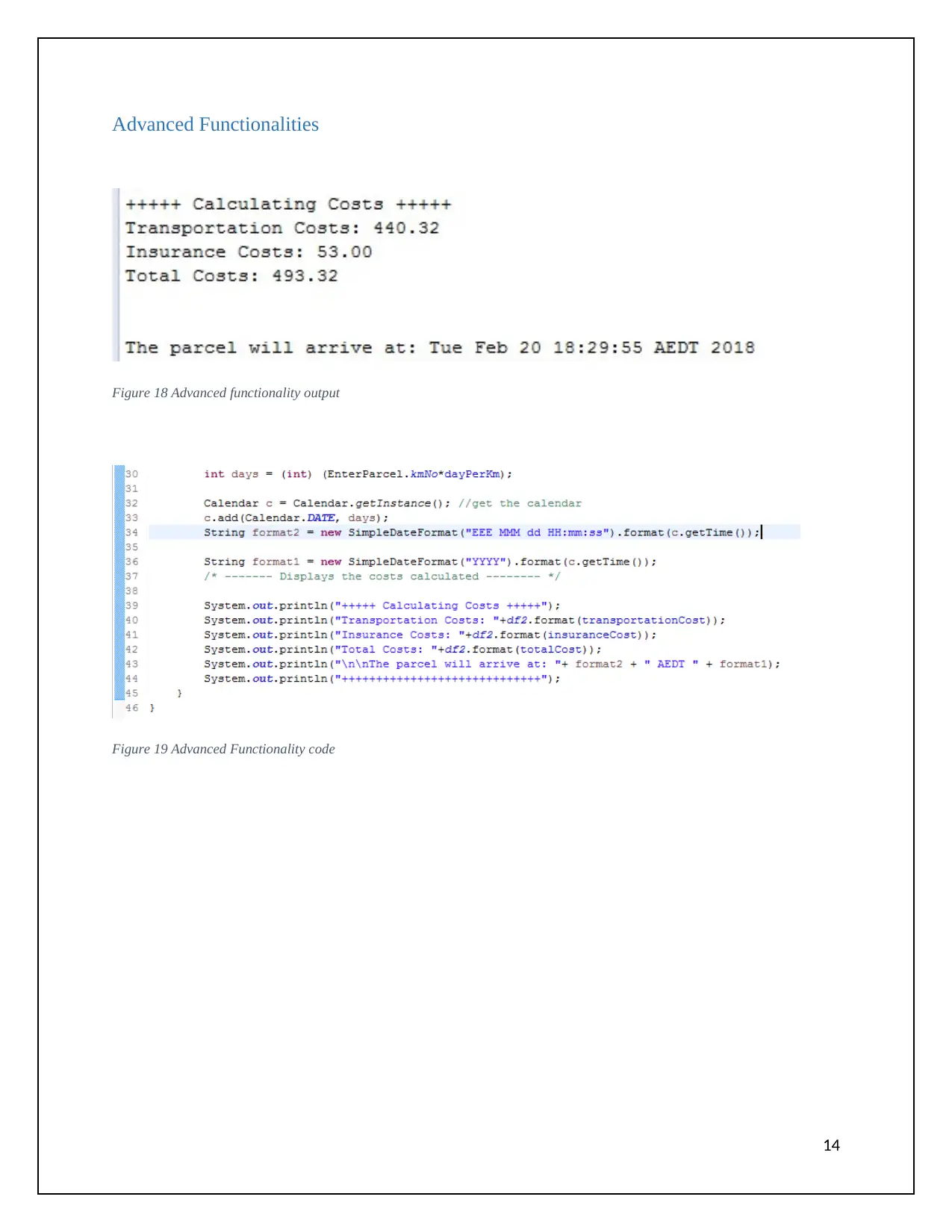
Advanced Functionalities
Figure 18 Advanced functionality output
Figure 19 Advanced Functionality code
14
Figure 18 Advanced functionality output
Figure 19 Advanced Functionality code
14
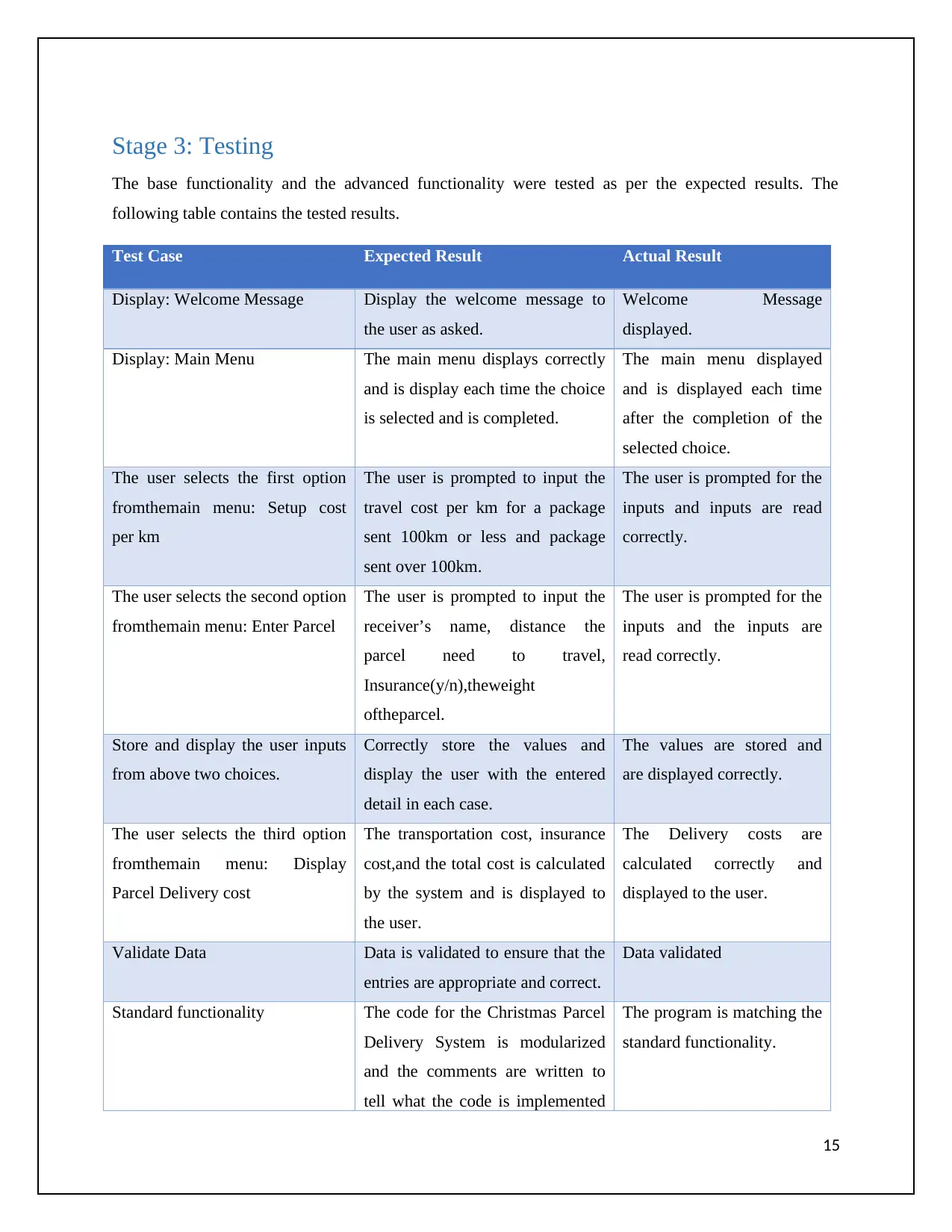
Stage 3: Testing
The base functionality and the advanced functionality were tested as per the expected results. The
following table contains the tested results.
Test Case Expected Result Actual Result
Display: Welcome Message Display the welcome message to
the user as asked.
Welcome Message
displayed.
Display: Main Menu The main menu displays correctly
and is display each time the choice
is selected and is completed.
The main menu displayed
and is displayed each time
after the completion of the
selected choice.
The user selects the first option
fromthemain menu: Setup cost
per km
The user is prompted to input the
travel cost per km for a package
sent 100km or less and package
sent over 100km.
The user is prompted for the
inputs and inputs are read
correctly.
The user selects the second option
fromthemain menu: Enter Parcel
The user is prompted to input the
receiver’s name, distance the
parcel need to travel,
Insurance(y/n),theweight
oftheparcel.
The user is prompted for the
inputs and the inputs are
read correctly.
Store and display the user inputs
from above two choices.
Correctly store the values and
display the user with the entered
detail in each case.
The values are stored and
are displayed correctly.
The user selects the third option
fromthemain menu: Display
Parcel Delivery cost
The transportation cost, insurance
cost,and the total cost is calculated
by the system and is displayed to
the user.
The Delivery costs are
calculated correctly and
displayed to the user.
Validate Data Data is validated to ensure that the
entries are appropriate and correct.
Data validated
Standard functionality The code for the Christmas Parcel
Delivery System is modularized
and the comments are written to
tell what the code is implemented
The program is matching the
standard functionality.
15
The base functionality and the advanced functionality were tested as per the expected results. The
following table contains the tested results.
Test Case Expected Result Actual Result
Display: Welcome Message Display the welcome message to
the user as asked.
Welcome Message
displayed.
Display: Main Menu The main menu displays correctly
and is display each time the choice
is selected and is completed.
The main menu displayed
and is displayed each time
after the completion of the
selected choice.
The user selects the first option
fromthemain menu: Setup cost
per km
The user is prompted to input the
travel cost per km for a package
sent 100km or less and package
sent over 100km.
The user is prompted for the
inputs and inputs are read
correctly.
The user selects the second option
fromthemain menu: Enter Parcel
The user is prompted to input the
receiver’s name, distance the
parcel need to travel,
Insurance(y/n),theweight
oftheparcel.
The user is prompted for the
inputs and the inputs are
read correctly.
Store and display the user inputs
from above two choices.
Correctly store the values and
display the user with the entered
detail in each case.
The values are stored and
are displayed correctly.
The user selects the third option
fromthemain menu: Display
Parcel Delivery cost
The transportation cost, insurance
cost,and the total cost is calculated
by the system and is displayed to
the user.
The Delivery costs are
calculated correctly and
displayed to the user.
Validate Data Data is validated to ensure that the
entries are appropriate and correct.
Data validated
Standard functionality The code for the Christmas Parcel
Delivery System is modularized
and the comments are written to
tell what the code is implemented
The program is matching the
standard functionality.
15
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
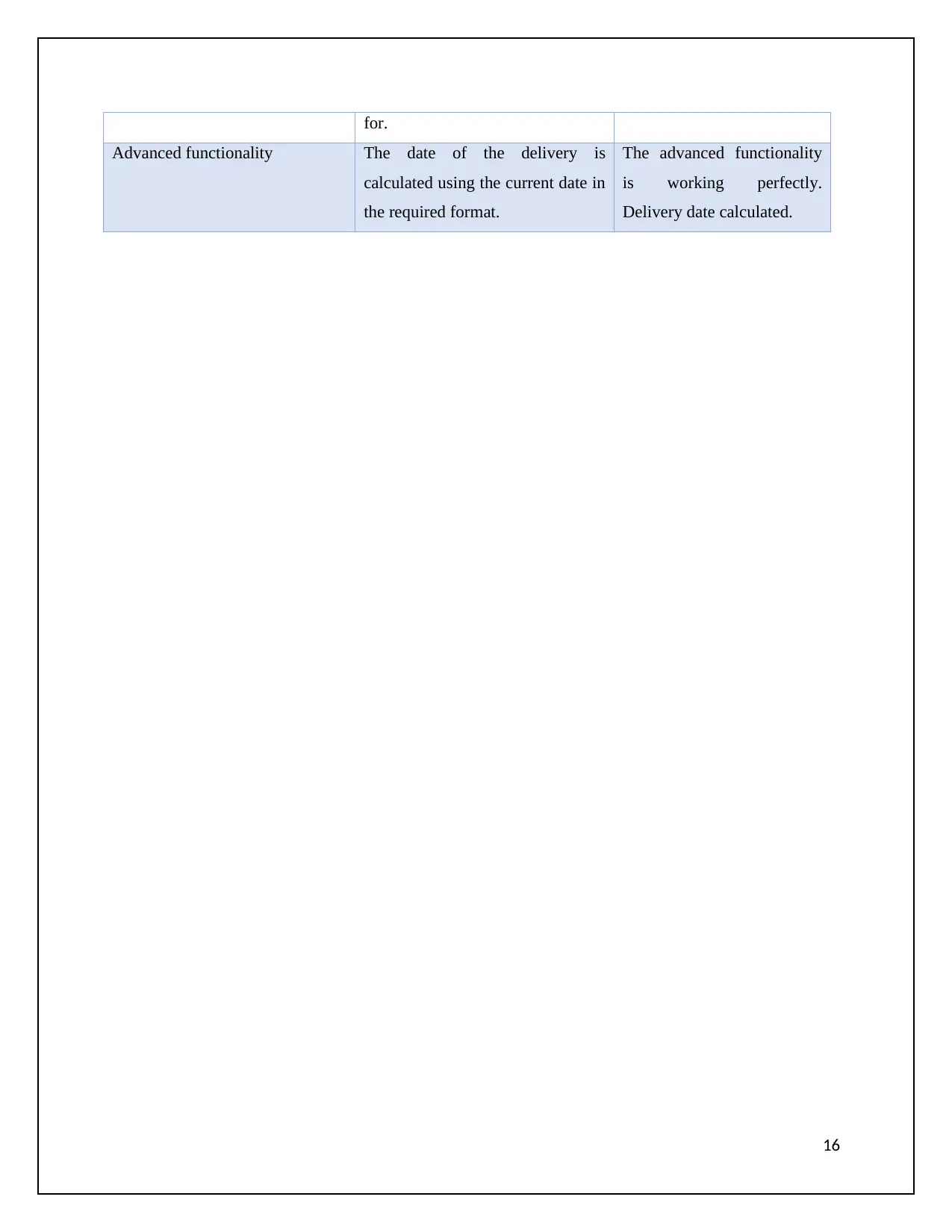
for.
Advanced functionality The date of the delivery is
calculated using the current date in
the required format.
The advanced functionality
is working perfectly.
Delivery date calculated.
16
Advanced functionality The date of the delivery is
calculated using the current date in
the required format.
The advanced functionality
is working perfectly.
Delivery date calculated.
16
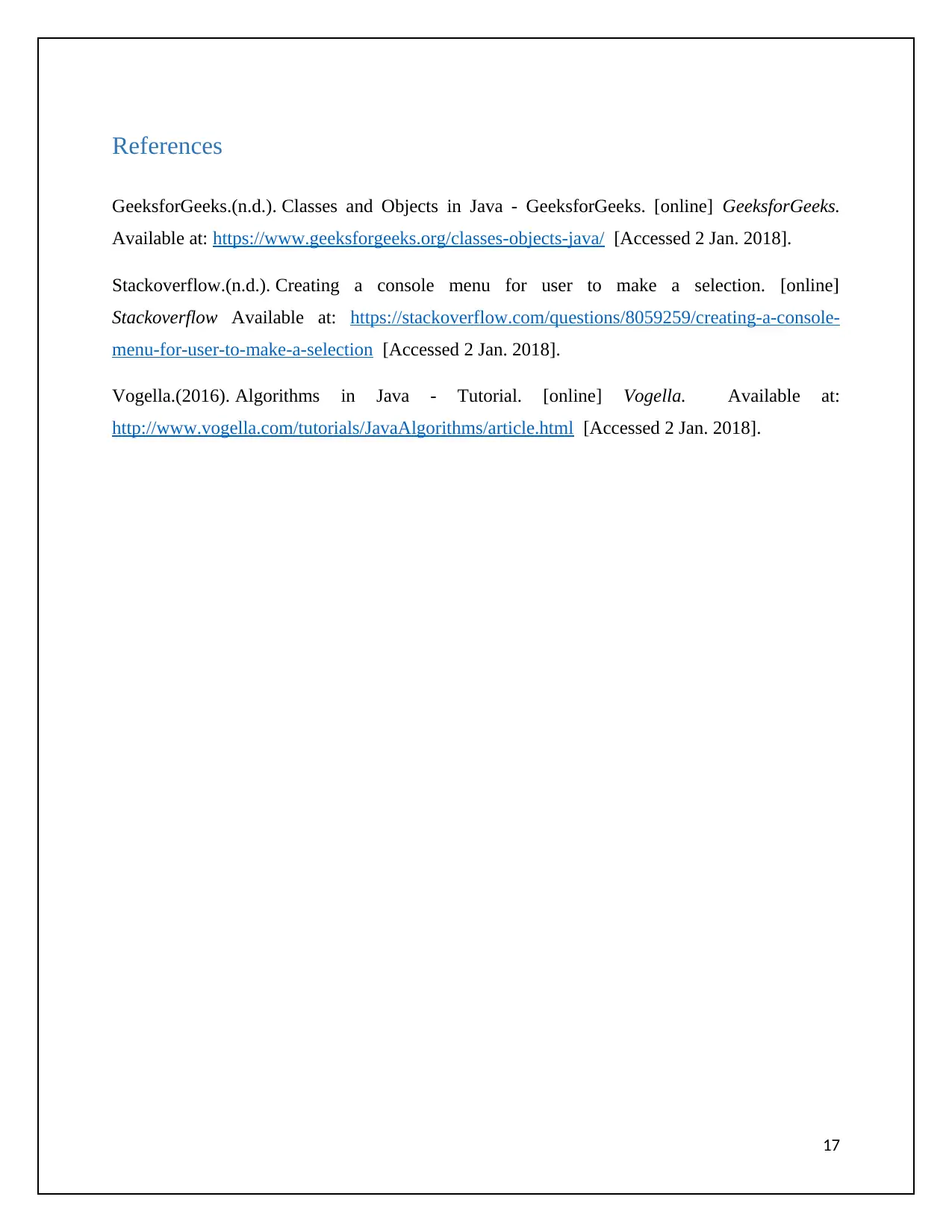
References
GeeksforGeeks.(n.d.). Classes and Objects in Java - GeeksforGeeks. [online] GeeksforGeeks.
Available at: https://www.geeksforgeeks.org/classes-objects-java/ [Accessed 2 Jan. 2018].
Stackoverflow.(n.d.). Creating a console menu for user to make a selection. [online]
Stackoverflow Available at: https://stackoverflow.com/questions/8059259/creating-a-console-
menu-for-user-to-make-a-selection [Accessed 2 Jan. 2018].
Vogella.(2016). Algorithms in Java - Tutorial. [online] Vogella. Available at:
http://www.vogella.com/tutorials/JavaAlgorithms/article.html [Accessed 2 Jan. 2018].
17
GeeksforGeeks.(n.d.). Classes and Objects in Java - GeeksforGeeks. [online] GeeksforGeeks.
Available at: https://www.geeksforgeeks.org/classes-objects-java/ [Accessed 2 Jan. 2018].
Stackoverflow.(n.d.). Creating a console menu for user to make a selection. [online]
Stackoverflow Available at: https://stackoverflow.com/questions/8059259/creating-a-console-
menu-for-user-to-make-a-selection [Accessed 2 Jan. 2018].
Vogella.(2016). Algorithms in Java - Tutorial. [online] Vogella. Available at:
http://www.vogella.com/tutorials/JavaAlgorithms/article.html [Accessed 2 Jan. 2018].
17
1 out of 18
Related Documents
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
 +13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024  |  Zucol Services PVT LTD  |  All rights reserved.