Java Programs for Infix, Postfix and Interpreter
VerifiedAdded on  2023/06/11
|88
|9426
|328
AI Summary
This article contains Java programs for infix, postfix and interpreter. The programs include Infix to Postfix conversion, Interpreter Demo, Postfix to Infix conversion, Euclid algorithm, IdentToken and InputData. These programs are useful for students studying computer science and related fields. The article also provides solved assignments, essays, dissertations and more related to these programs at Desklib. Students can get expert assistance and improve their grades.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
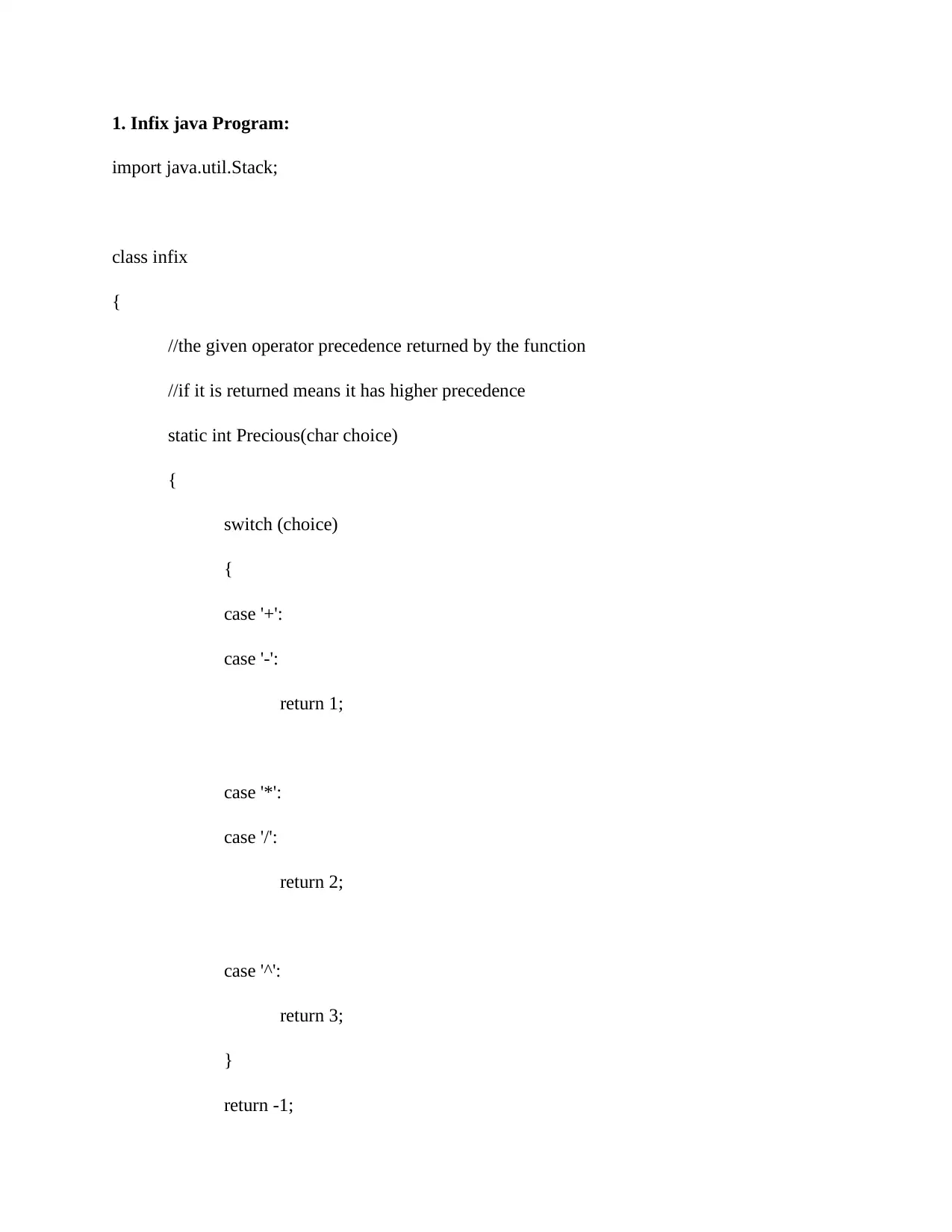
1. Infix java Program:
import java.util.Stack;
class infix
{
//the given operator precedence returned by the function
//if it is returned means it has higher precedence
static int Precious(char choice)
{
switch (choice)
{
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
case '^':
return 3;
}
return -1;
import java.util.Stack;
class infix
{
//the given operator precedence returned by the function
//if it is returned means it has higher precedence
static int Precious(char choice)
{
switch (choice)
{
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
case '^':
return 3;
}
return -1;
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
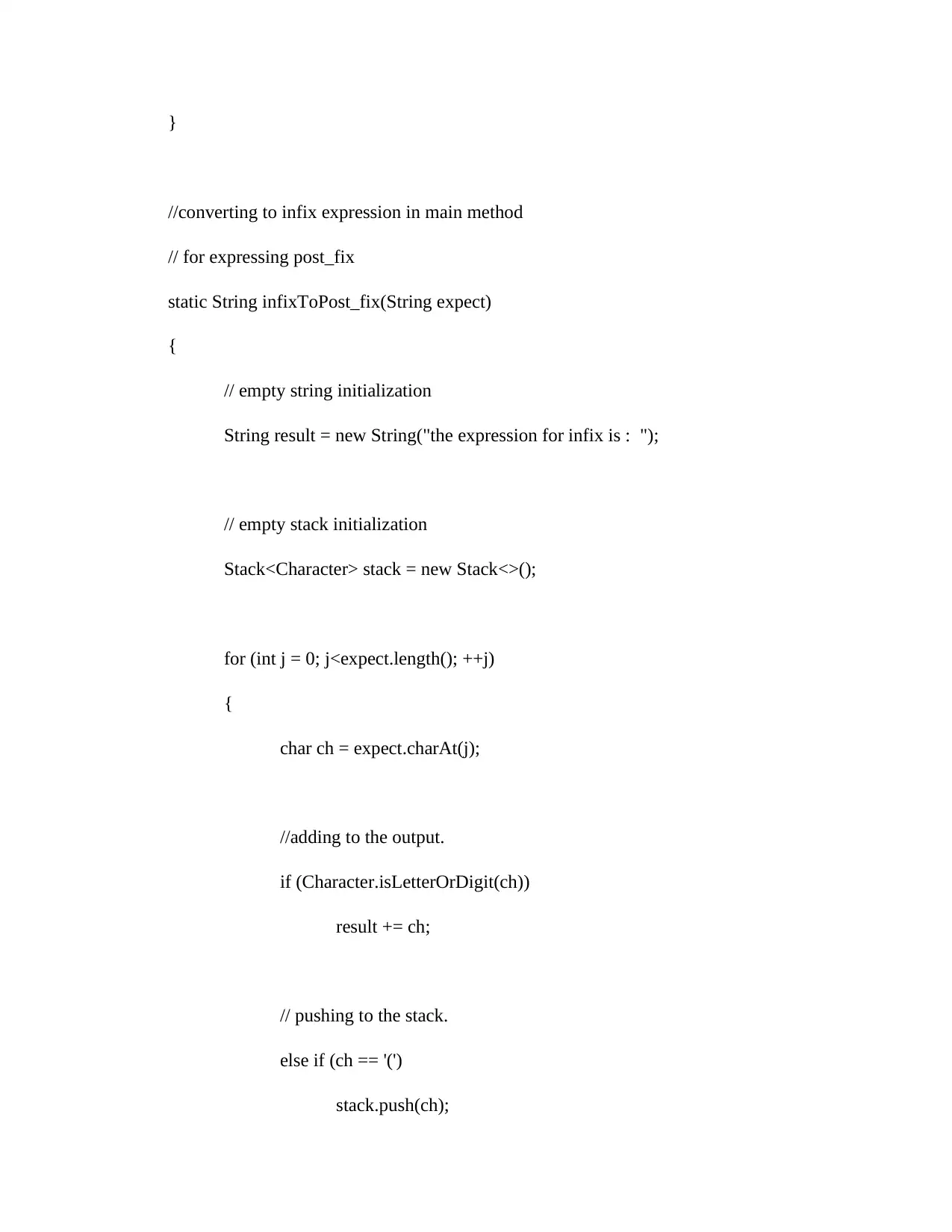
}
//converting to infix expression in main method
// for expressing post_fix
static String infixToPost_fix(String expect)
{
// empty string initialization
String result = new String("the expression for infix is : ");
// empty stack initialization
Stack<Character> stack = new Stack<>();
for (int j = 0; j<expect.length(); ++j)
{
char ch = expect.charAt(j);
//adding to the output.
if (Character.isLetterOrDigit(ch))
result += ch;
// pushing to the stack.
else if (ch == '(')
stack.push(ch);
//converting to infix expression in main method
// for expressing post_fix
static String infixToPost_fix(String expect)
{
// empty string initialization
String result = new String("the expression for infix is : ");
// empty stack initialization
Stack<Character> stack = new Stack<>();
for (int j = 0; j<expect.length(); ++j)
{
char ch = expect.charAt(j);
//adding to the output.
if (Character.isLetterOrDigit(ch))
result += ch;
// pushing to the stack.
else if (ch == '(')
stack.push(ch);
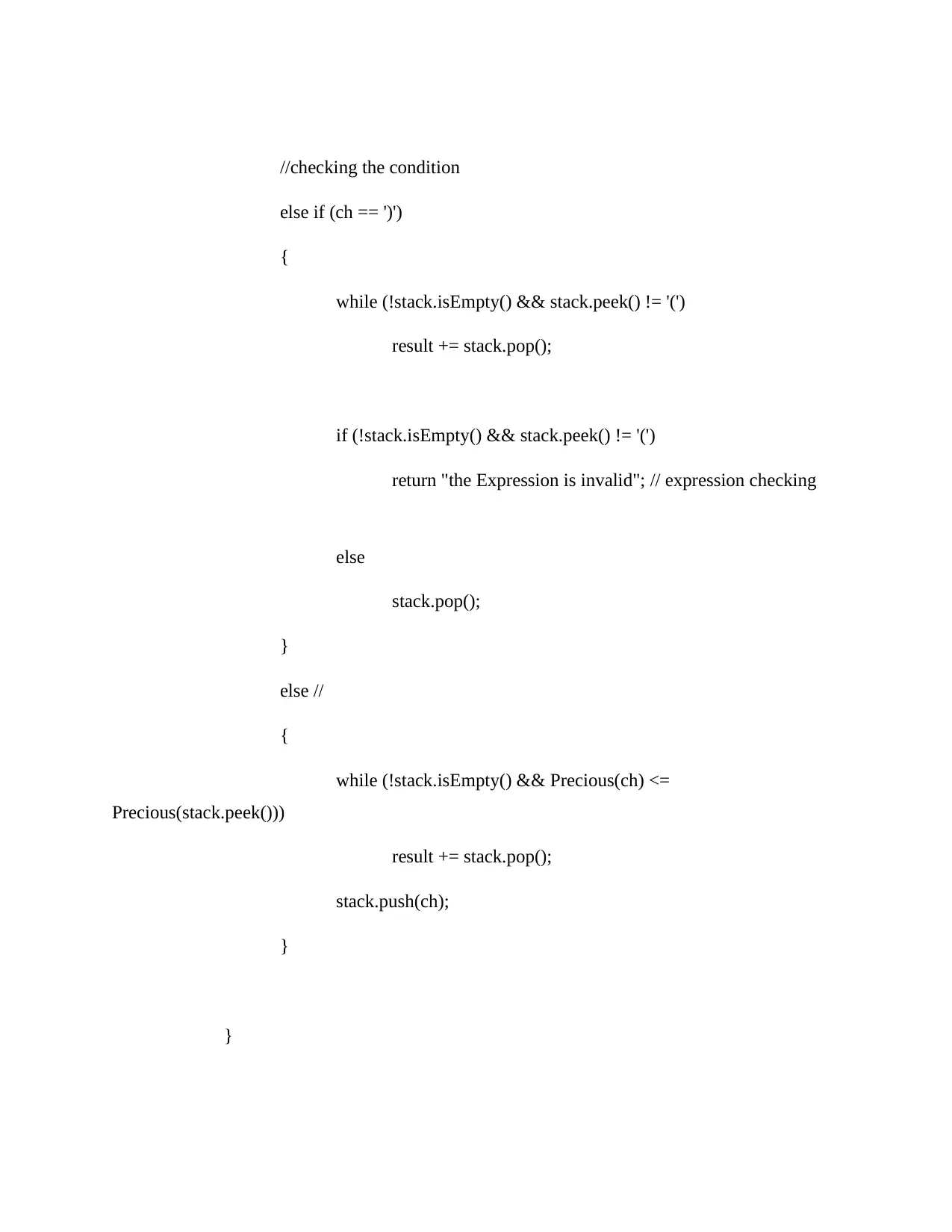
//checking the condition
else if (ch == ')')
{
while (!stack.isEmpty() && stack.peek() != '(')
result += stack.pop();
if (!stack.isEmpty() && stack.peek() != '(')
return "the Expression is invalid"; // expression checking
else
stack.pop();
}
else //
{
while (!stack.isEmpty() && Precious(ch) <=
Precious(stack.peek()))
result += stack.pop();
stack.push(ch);
}
}
else if (ch == ')')
{
while (!stack.isEmpty() && stack.peek() != '(')
result += stack.pop();
if (!stack.isEmpty() && stack.peek() != '(')
return "the Expression is invalid"; // expression checking
else
stack.pop();
}
else //
{
while (!stack.isEmpty() && Precious(ch) <=
Precious(stack.peek()))
result += stack.pop();
stack.push(ch);
}
}
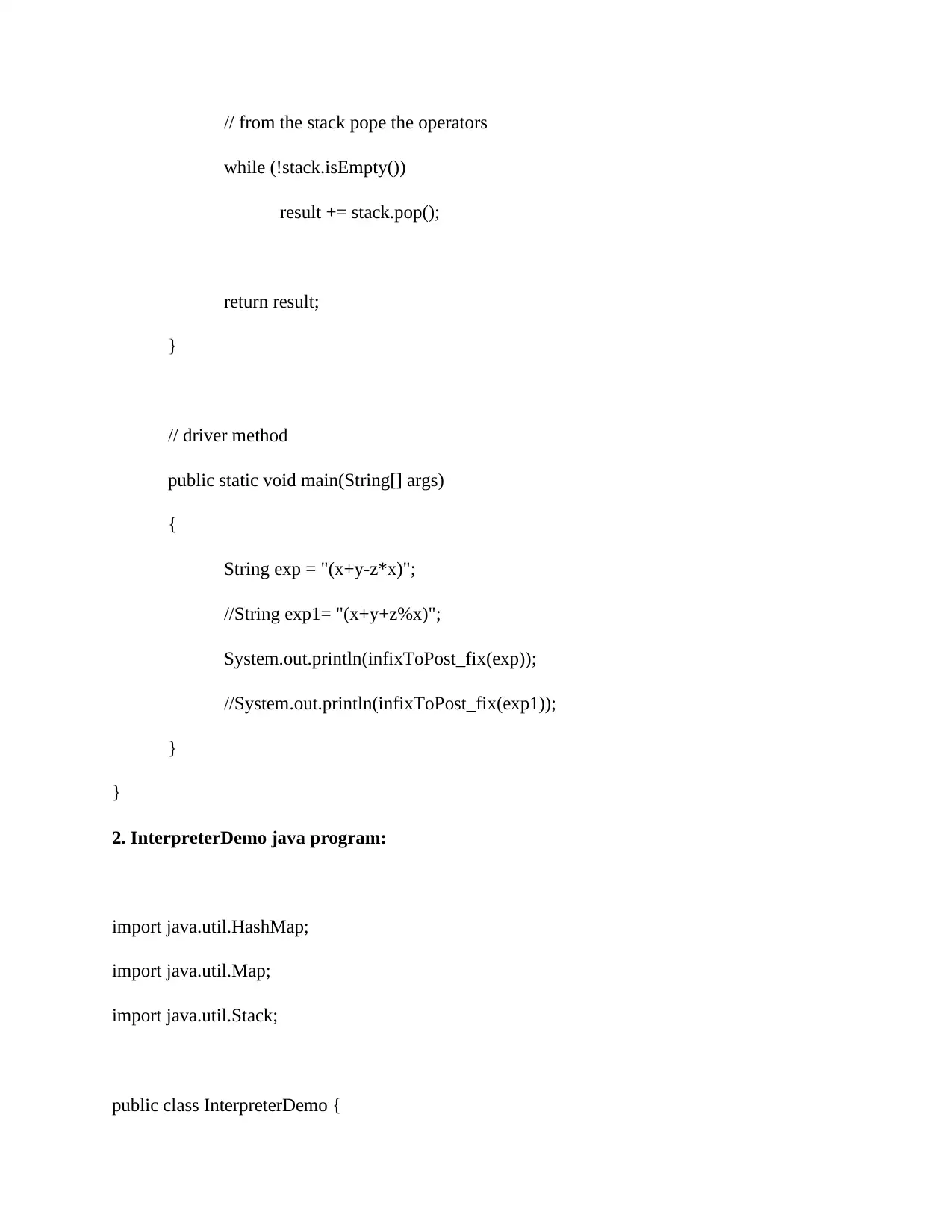
// from the stack pope the operators
while (!stack.isEmpty())
result += stack.pop();
return result;
}
// driver method
public static void main(String[] args)
{
String exp = "(x+y-z*x)";
//String exp1= "(x+y+z%x)";
System.out.println(infixToPost_fix(exp));
//System.out.println(infixToPost_fix(exp1));
}
}
2. InterpreterDemo java program:
import java.util.HashMap;
import java.util.Map;
import java.util.Stack;
public class InterpreterDemo {
while (!stack.isEmpty())
result += stack.pop();
return result;
}
// driver method
public static void main(String[] args)
{
String exp = "(x+y-z*x)";
//String exp1= "(x+y+z%x)";
System.out.println(infixToPost_fix(exp));
//System.out.println(infixToPost_fix(exp1));
}
}
2. InterpreterDemo java program:
import java.util.HashMap;
import java.util.Map;
import java.util.Stack;
public class InterpreterDemo {
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
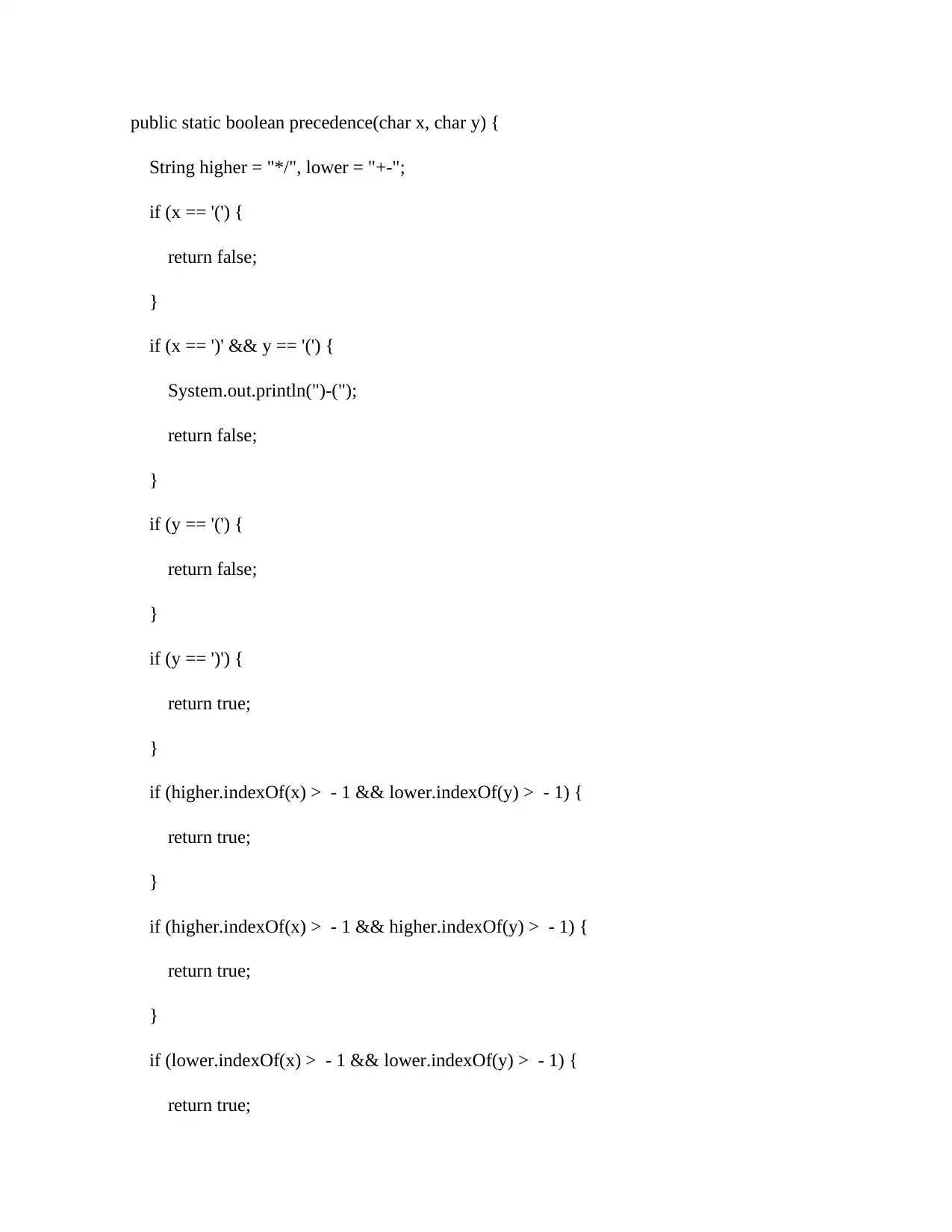
public static boolean precedence(char x, char y) {
String higher = "*/", lower = "+-";
if (x == '(') {
return false;
}
if (x == ')' && y == '(') {
System.out.println(")-(");
return false;
}
if (y == '(') {
return false;
}
if (y == ')') {
return true;
}
if (higher.indexOf(x) > - 1 && lower.indexOf(y) > - 1) {
return true;
}
if (higher.indexOf(x) > - 1 && higher.indexOf(y) > - 1) {
return true;
}
if (lower.indexOf(x) > - 1 && lower.indexOf(y) > - 1) {
return true;
String higher = "*/", lower = "+-";
if (x == '(') {
return false;
}
if (x == ')' && y == '(') {
System.out.println(")-(");
return false;
}
if (y == '(') {
return false;
}
if (y == ')') {
return true;
}
if (higher.indexOf(x) > - 1 && lower.indexOf(y) > - 1) {
return true;
}
if (higher.indexOf(x) > - 1 && higher.indexOf(y) > - 1) {
return true;
}
if (lower.indexOf(x) > - 1 && lower.indexOf(y) > - 1) {
return true;
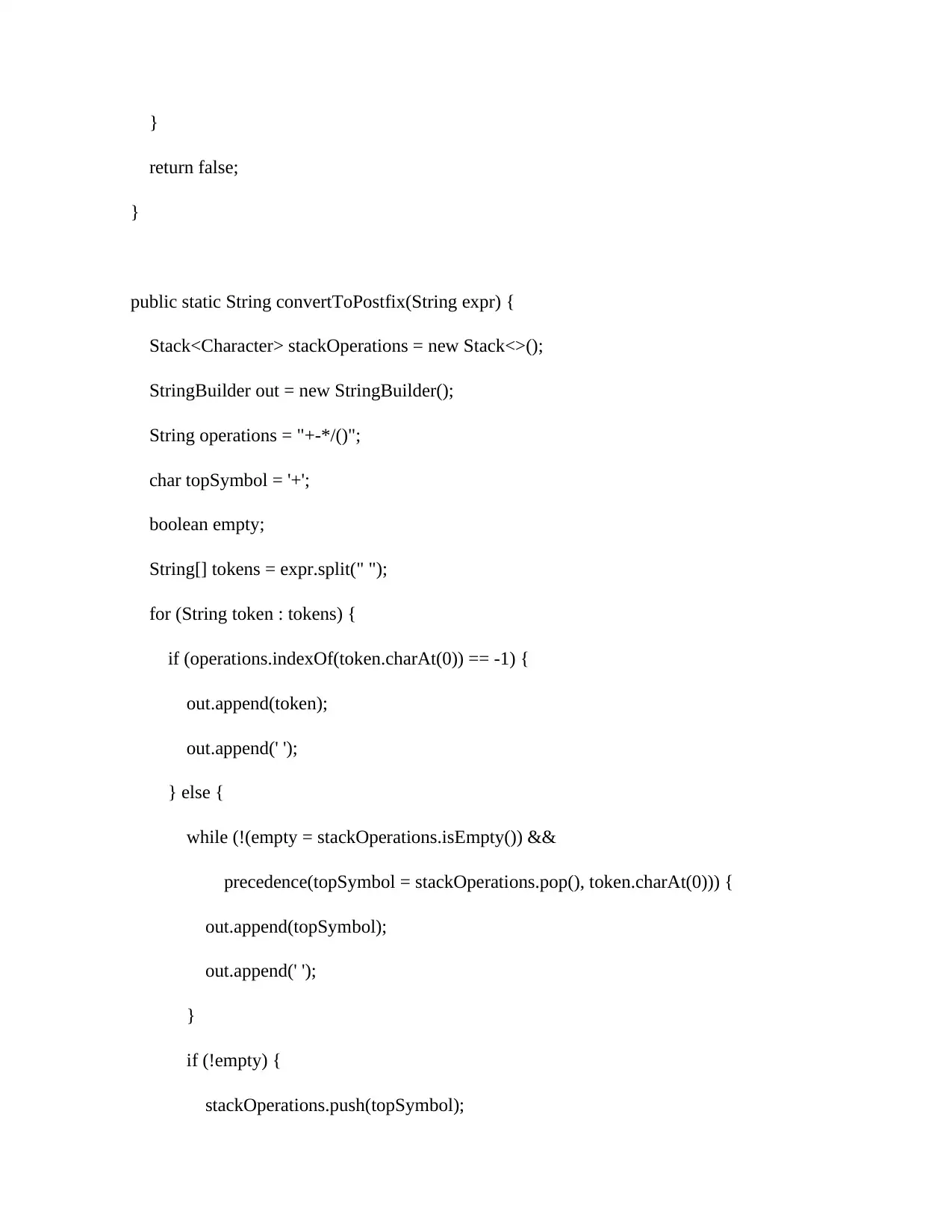
}
return false;
}
public static String convertToPostfix(String expr) {
Stack<Character> stackOperations = new Stack<>();
StringBuilder out = new StringBuilder();
String operations = "+-*/()";
char topSymbol = '+';
boolean empty;
String[] tokens = expr.split(" ");
for (String token : tokens) {
if (operations.indexOf(token.charAt(0)) == -1) {
out.append(token);
out.append(' ');
} else {
while (!(empty = stackOperations.isEmpty()) &&
precedence(topSymbol = stackOperations.pop(), token.charAt(0))) {
out.append(topSymbol);
out.append(' ');
}
if (!empty) {
stackOperations.push(topSymbol);
return false;
}
public static String convertToPostfix(String expr) {
Stack<Character> stackOperations = new Stack<>();
StringBuilder out = new StringBuilder();
String operations = "+-*/()";
char topSymbol = '+';
boolean empty;
String[] tokens = expr.split(" ");
for (String token : tokens) {
if (operations.indexOf(token.charAt(0)) == -1) {
out.append(token);
out.append(' ');
} else {
while (!(empty = stackOperations.isEmpty()) &&
precedence(topSymbol = stackOperations.pop(), token.charAt(0))) {
out.append(topSymbol);
out.append(' ');
}
if (!empty) {
stackOperations.push(topSymbol);
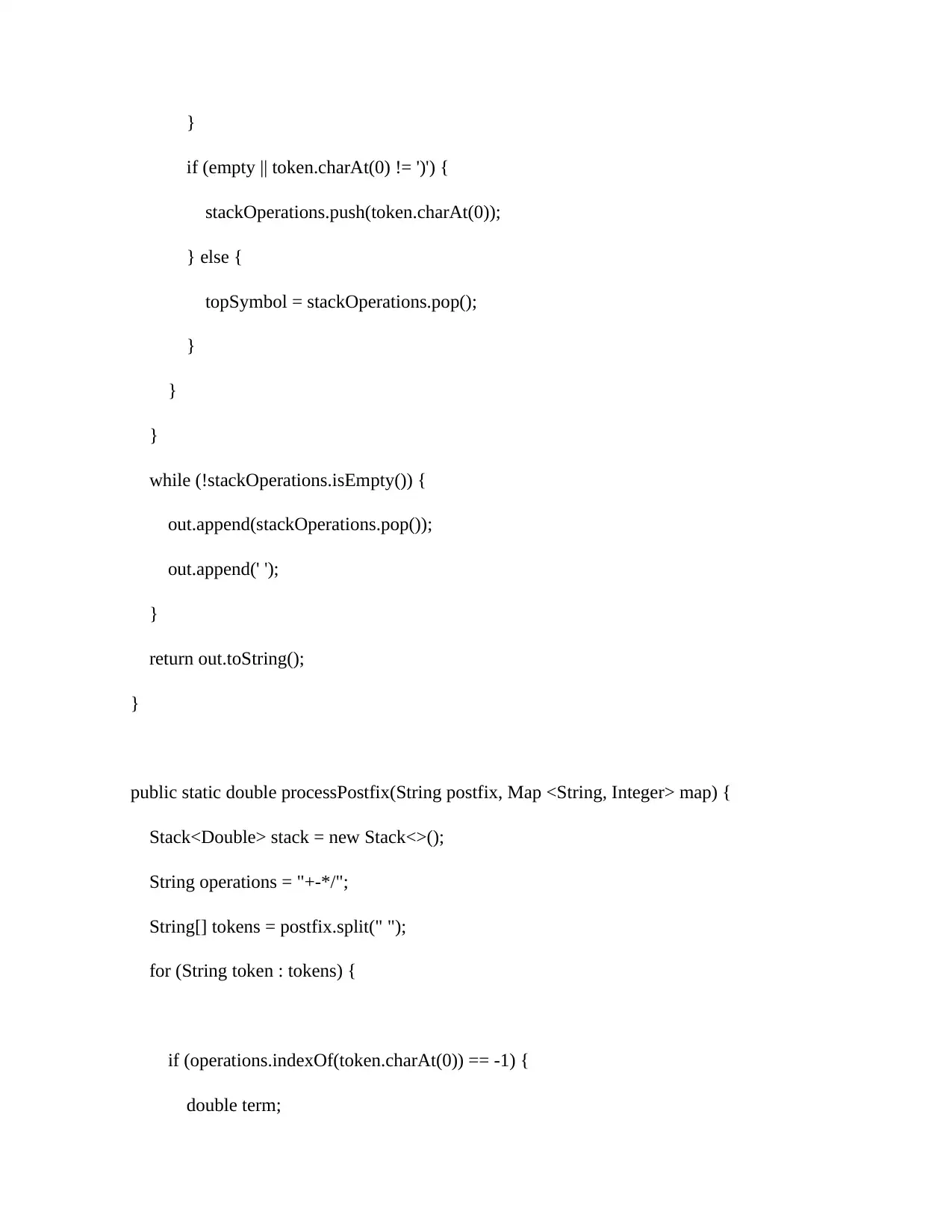
}
if (empty || token.charAt(0) != ')') {
stackOperations.push(token.charAt(0));
} else {
topSymbol = stackOperations.pop();
}
}
}
while (!stackOperations.isEmpty()) {
out.append(stackOperations.pop());
out.append(' ');
}
return out.toString();
}
public static double processPostfix(String postfix, Map <String, Integer> map) {
Stack<Double> stack = new Stack<>();
String operations = "+-*/";
String[] tokens = postfix.split(" ");
for (String token : tokens) {
if (operations.indexOf(token.charAt(0)) == -1) {
double term;
if (empty || token.charAt(0) != ')') {
stackOperations.push(token.charAt(0));
} else {
topSymbol = stackOperations.pop();
}
}
}
while (!stackOperations.isEmpty()) {
out.append(stackOperations.pop());
out.append(' ');
}
return out.toString();
}
public static double processPostfix(String postfix, Map <String, Integer> map) {
Stack<Double> stack = new Stack<>();
String operations = "+-*/";
String[] tokens = postfix.split(" ");
for (String token : tokens) {
if (operations.indexOf(token.charAt(0)) == -1) {
double term;
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
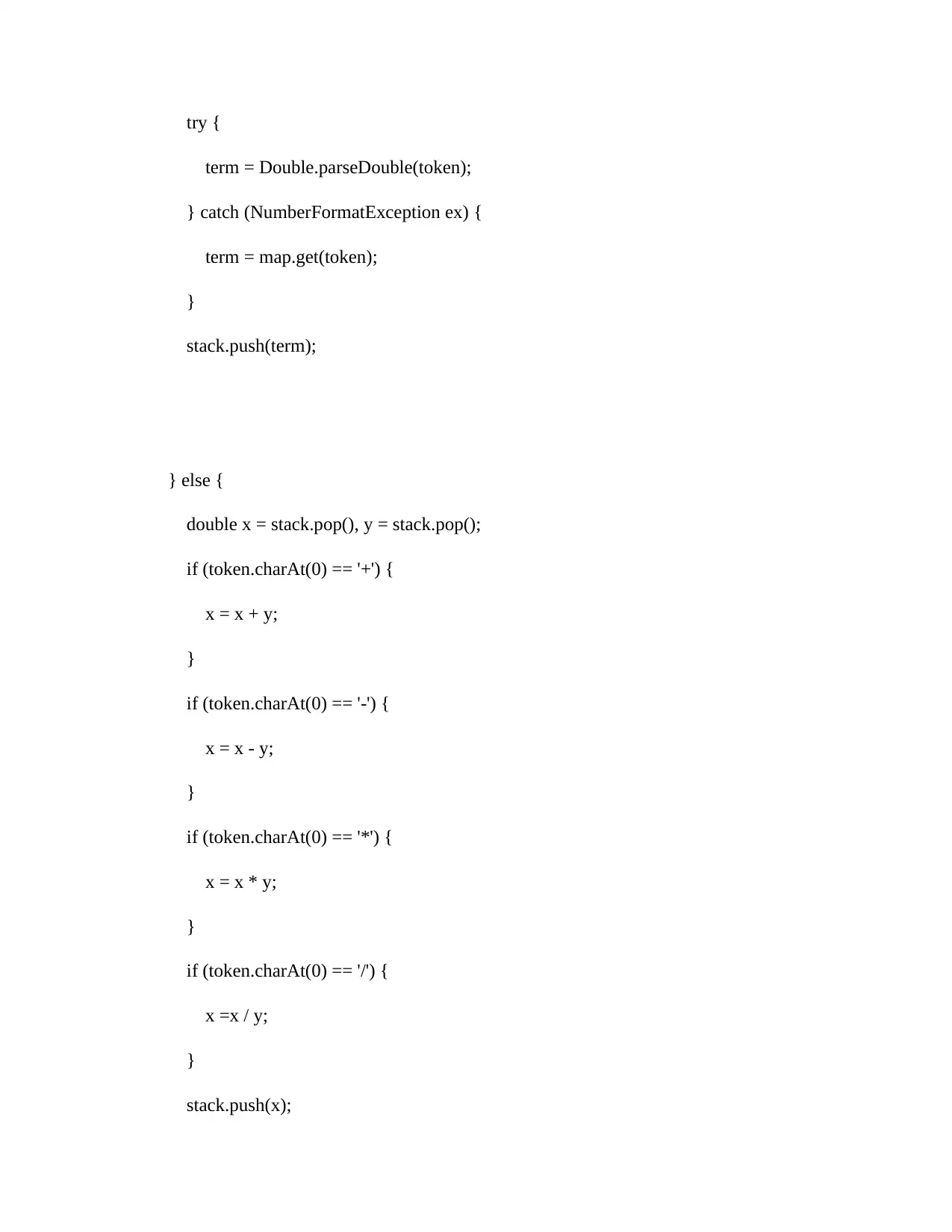
try {
term = Double.parseDouble(token);
} catch (NumberFormatException ex) {
term = map.get(token);
}
stack.push(term);
} else {
double x = stack.pop(), y = stack.pop();
if (token.charAt(0) == '+') {
x = x + y;
}
if (token.charAt(0) == '-') {
x = x - y;
}
if (token.charAt(0) == '*') {
x = x * y;
}
if (token.charAt(0) == '/') {
x =x / y;
}
stack.push(x);
term = Double.parseDouble(token);
} catch (NumberFormatException ex) {
term = map.get(token);
}
stack.push(term);
} else {
double x = stack.pop(), y = stack.pop();
if (token.charAt(0) == '+') {
x = x + y;
}
if (token.charAt(0) == '-') {
x = x - y;
}
if (token.charAt(0) == '*') {
x = x * y;
}
if (token.charAt(0) == '/') {
x =x / y;
}
stack.push(x);
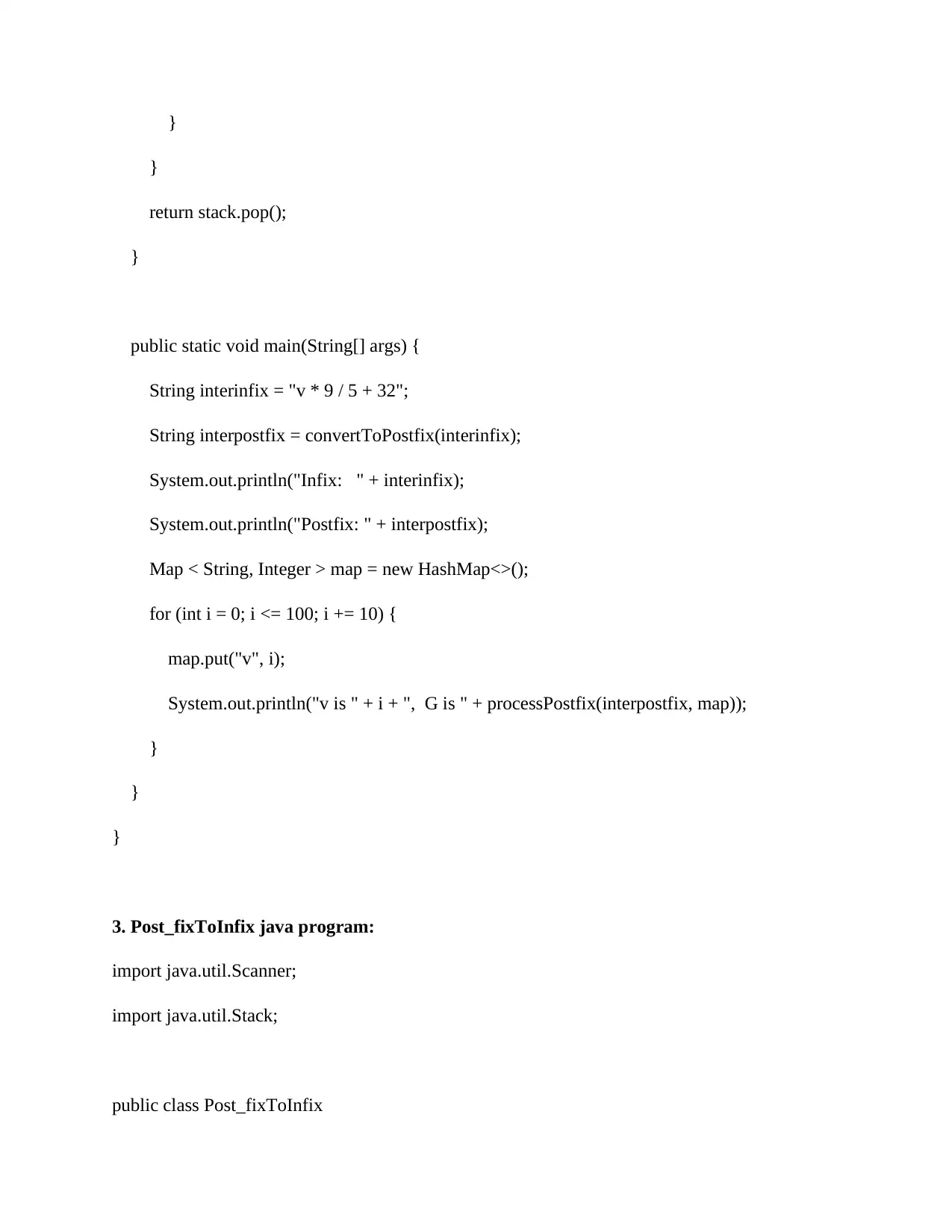
}
}
return stack.pop();
}
public static void main(String[] args) {
String interinfix = "v * 9 / 5 + 32";
String interpostfix = convertToPostfix(interinfix);
System.out.println("Infix: " + interinfix);
System.out.println("Postfix: " + interpostfix);
Map < String, Integer > map = new HashMap<>();
for (int i = 0; i <= 100; i += 10) {
map.put("v", i);
System.out.println("v is " + i + ", G is " + processPostfix(interpostfix, map));
}
}
}
3. Post_fixToInfix java program:
import java.util.Scanner;
import java.util.Stack;
public class Post_fixToInfix
}
return stack.pop();
}
public static void main(String[] args) {
String interinfix = "v * 9 / 5 + 32";
String interpostfix = convertToPostfix(interinfix);
System.out.println("Infix: " + interinfix);
System.out.println("Postfix: " + interpostfix);
Map < String, Integer > map = new HashMap<>();
for (int i = 0; i <= 100; i += 10) {
map.put("v", i);
System.out.println("v is " + i + ", G is " + processPostfix(interpostfix, map));
}
}
}
3. Post_fixToInfix java program:
import java.util.Scanner;
import java.util.Stack;
public class Post_fixToInfix
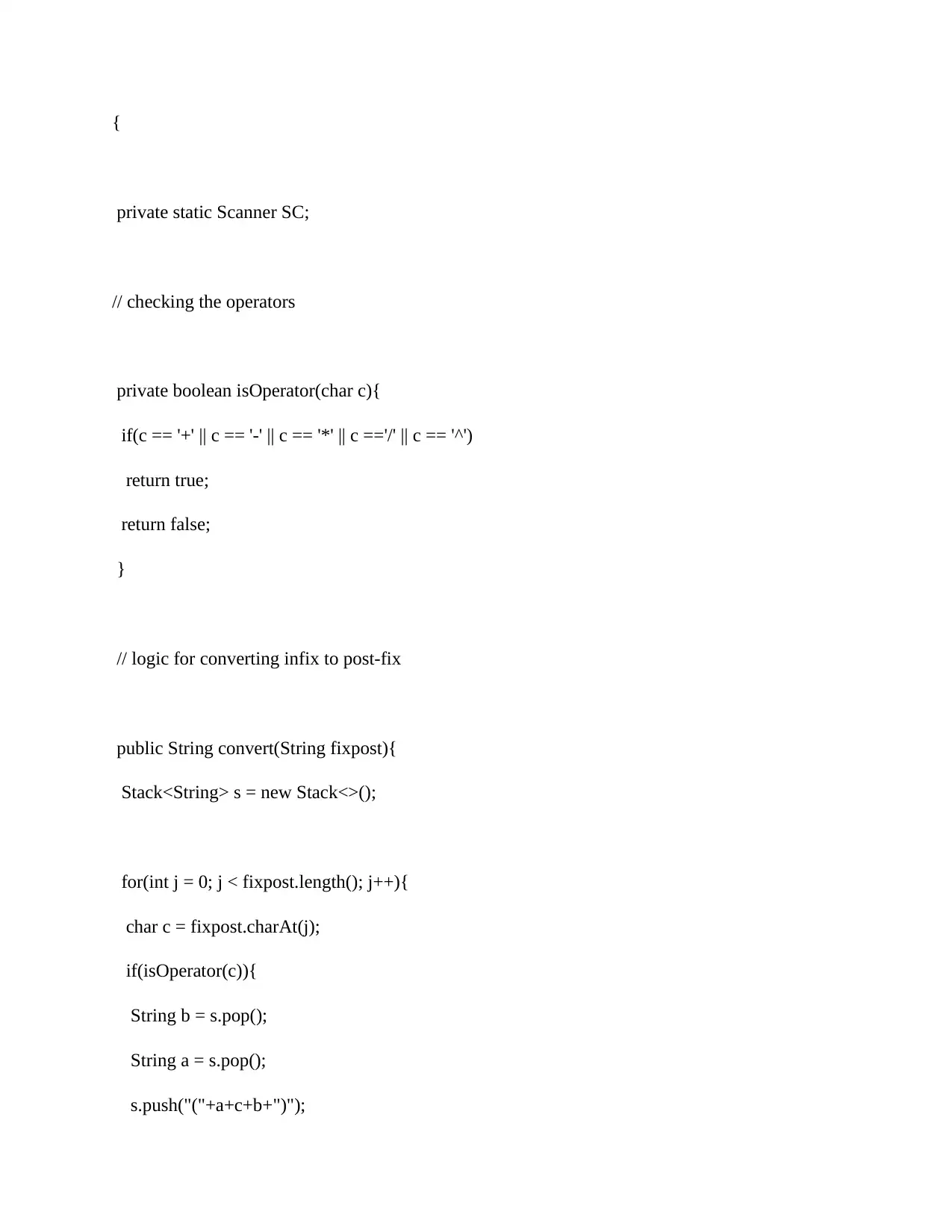
{
private static Scanner SC;
// checking the operators
private boolean isOperator(char c){
if(c == '+' || c == '-' || c == '*' || c =='/' || c == '^')
return true;
return false;
}
// logic for converting infix to post-fix
public String convert(String fixpost){
Stack<String> s = new Stack<>();
for(int j = 0; j < fixpost.length(); j++){
char c = fixpost.charAt(j);
if(isOperator(c)){
String b = s.pop();
String a = s.pop();
s.push("("+a+c+b+")");
private static Scanner SC;
// checking the operators
private boolean isOperator(char c){
if(c == '+' || c == '-' || c == '*' || c =='/' || c == '^')
return true;
return false;
}
// logic for converting infix to post-fix
public String convert(String fixpost){
Stack<String> s = new Stack<>();
for(int j = 0; j < fixpost.length(); j++){
char c = fixpost.charAt(j);
if(isOperator(c)){
String b = s.pop();
String a = s.pop();
s.push("("+a+c+b+")");
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
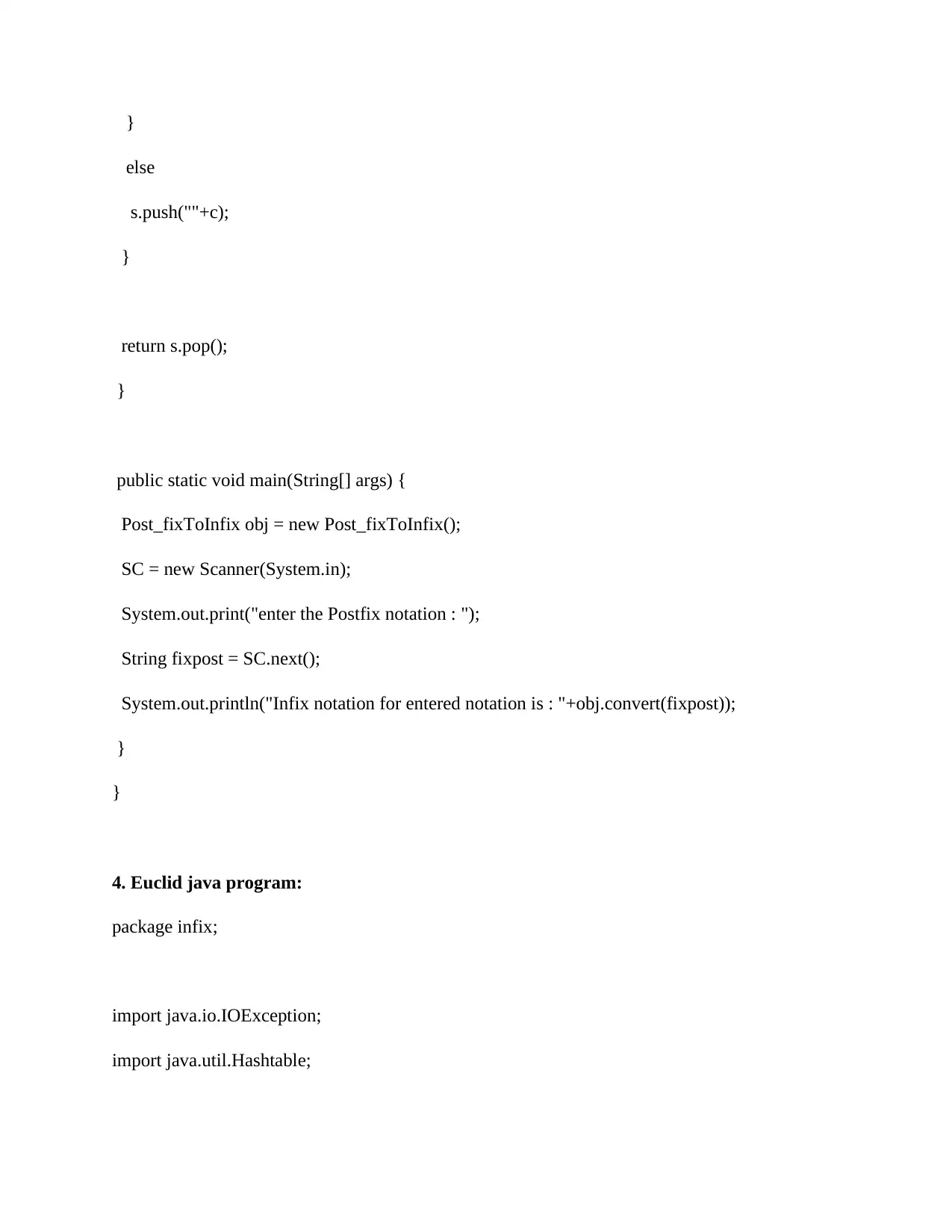
}
else
s.push(""+c);
}
return s.pop();
}
public static void main(String[] args) {
Post_fixToInfix obj = new Post_fixToInfix();
SC = new Scanner(System.in);
System.out.print("enter the Postfix notation : ");
String fixpost = SC.next();
System.out.println("Infix notation for entered notation is : "+obj.convert(fixpost));
}
}
4. Euclid java program:
package infix;
import java.io.IOException;
import java.util.Hashtable;
else
s.push(""+c);
}
return s.pop();
}
public static void main(String[] args) {
Post_fixToInfix obj = new Post_fixToInfix();
SC = new Scanner(System.in);
System.out.print("enter the Postfix notation : ");
String fixpost = SC.next();
System.out.println("Infix notation for entered notation is : "+obj.convert(fixpost));
}
}
4. Euclid java program:
package infix;
import java.io.IOException;
import java.util.Hashtable;
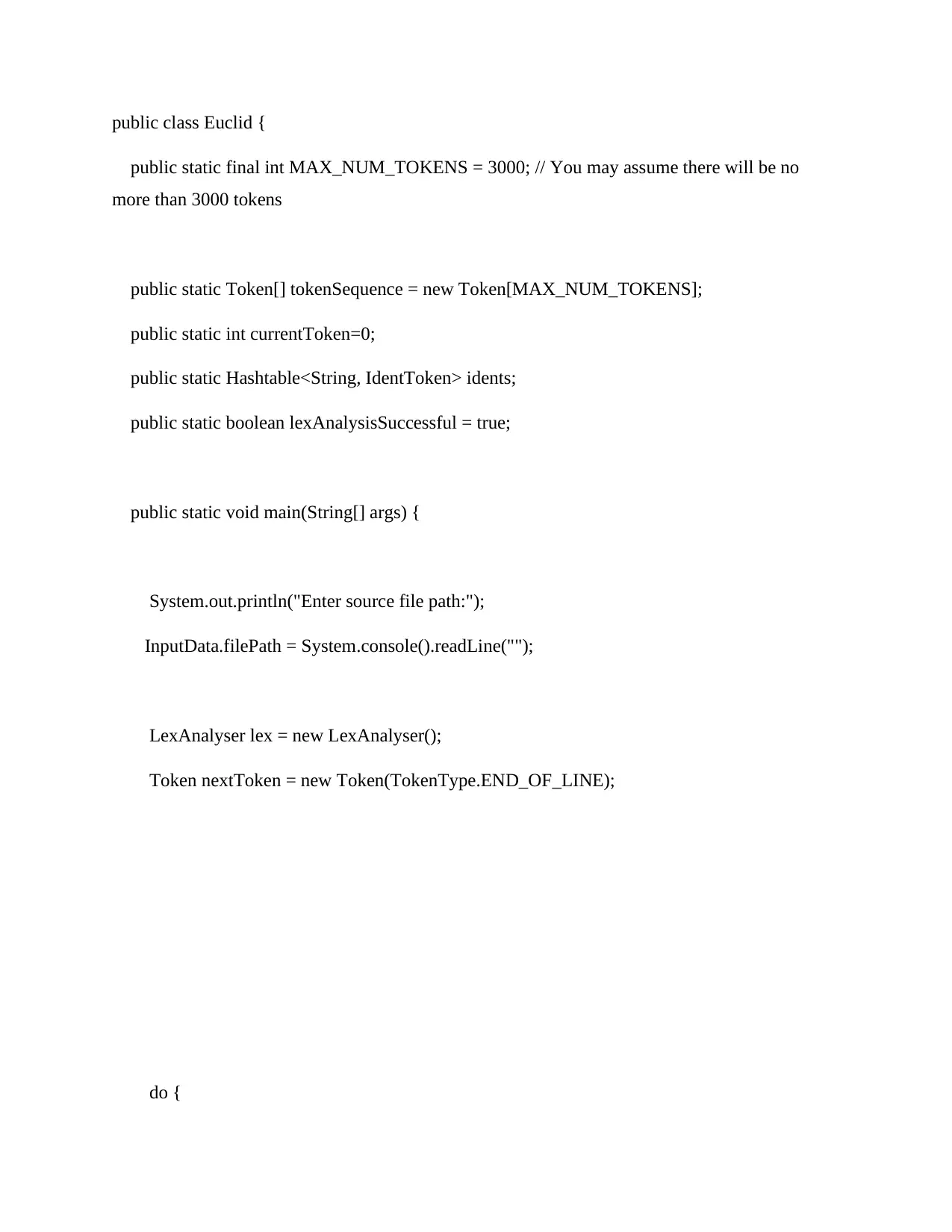
public class Euclid {
public static final int MAX_NUM_TOKENS = 3000; // You may assume there will be no
more than 3000 tokens
public static Token[] tokenSequence = new Token[MAX_NUM_TOKENS];
public static int currentToken=0;
public static Hashtable<String, IdentToken> idents;
public static boolean lexAnalysisSuccessful = true;
public static void main(String[] args) {
System.out.println("Enter source file path:");
InputData.filePath = System.console().readLine("");
LexAnalyser lex = new LexAnalyser();
Token nextToken = new Token(TokenType.END_OF_LINE);
do {
public static final int MAX_NUM_TOKENS = 3000; // You may assume there will be no
more than 3000 tokens
public static Token[] tokenSequence = new Token[MAX_NUM_TOKENS];
public static int currentToken=0;
public static Hashtable<String, IdentToken> idents;
public static boolean lexAnalysisSuccessful = true;
public static void main(String[] args) {
System.out.println("Enter source file path:");
InputData.filePath = System.console().readLine("");
LexAnalyser lex = new LexAnalyser();
Token nextToken = new Token(TokenType.END_OF_LINE);
do {
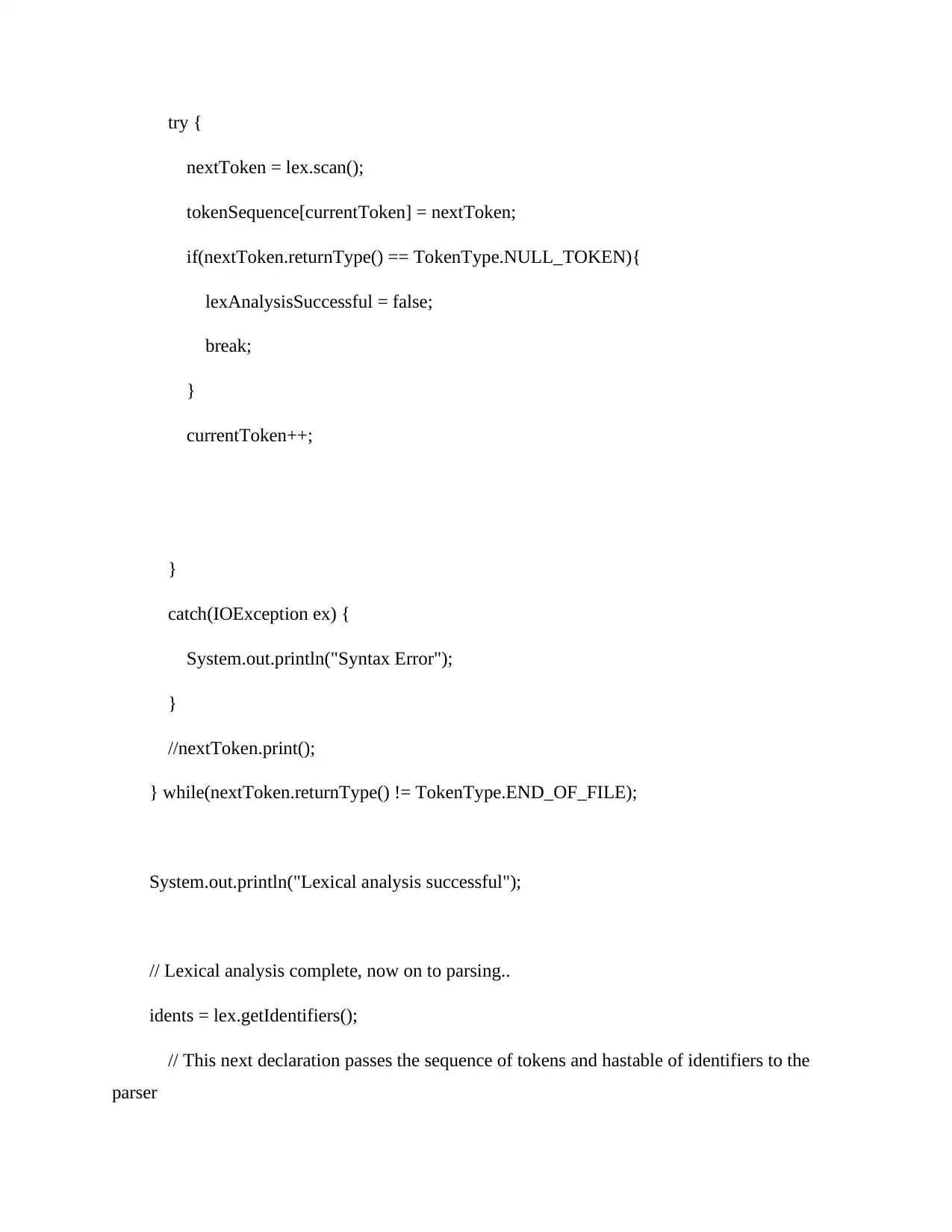
try {
nextToken = lex.scan();
tokenSequence[currentToken] = nextToken;
if(nextToken.returnType() == TokenType.NULL_TOKEN){
lexAnalysisSuccessful = false;
break;
}
currentToken++;
}
catch(IOException ex) {
System.out.println("Syntax Error");
}
//nextToken.print();
} while(nextToken.returnType() != TokenType.END_OF_FILE);
System.out.println("Lexical analysis successful");
// Lexical analysis complete, now on to parsing..
idents = lex.getIdentifiers();
// This next declaration passes the sequence of tokens and hastable of identifiers to the
parser
nextToken = lex.scan();
tokenSequence[currentToken] = nextToken;
if(nextToken.returnType() == TokenType.NULL_TOKEN){
lexAnalysisSuccessful = false;
break;
}
currentToken++;
}
catch(IOException ex) {
System.out.println("Syntax Error");
}
//nextToken.print();
} while(nextToken.returnType() != TokenType.END_OF_FILE);
System.out.println("Lexical analysis successful");
// Lexical analysis complete, now on to parsing..
idents = lex.getIdentifiers();
// This next declaration passes the sequence of tokens and hastable of identifiers to the
parser
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
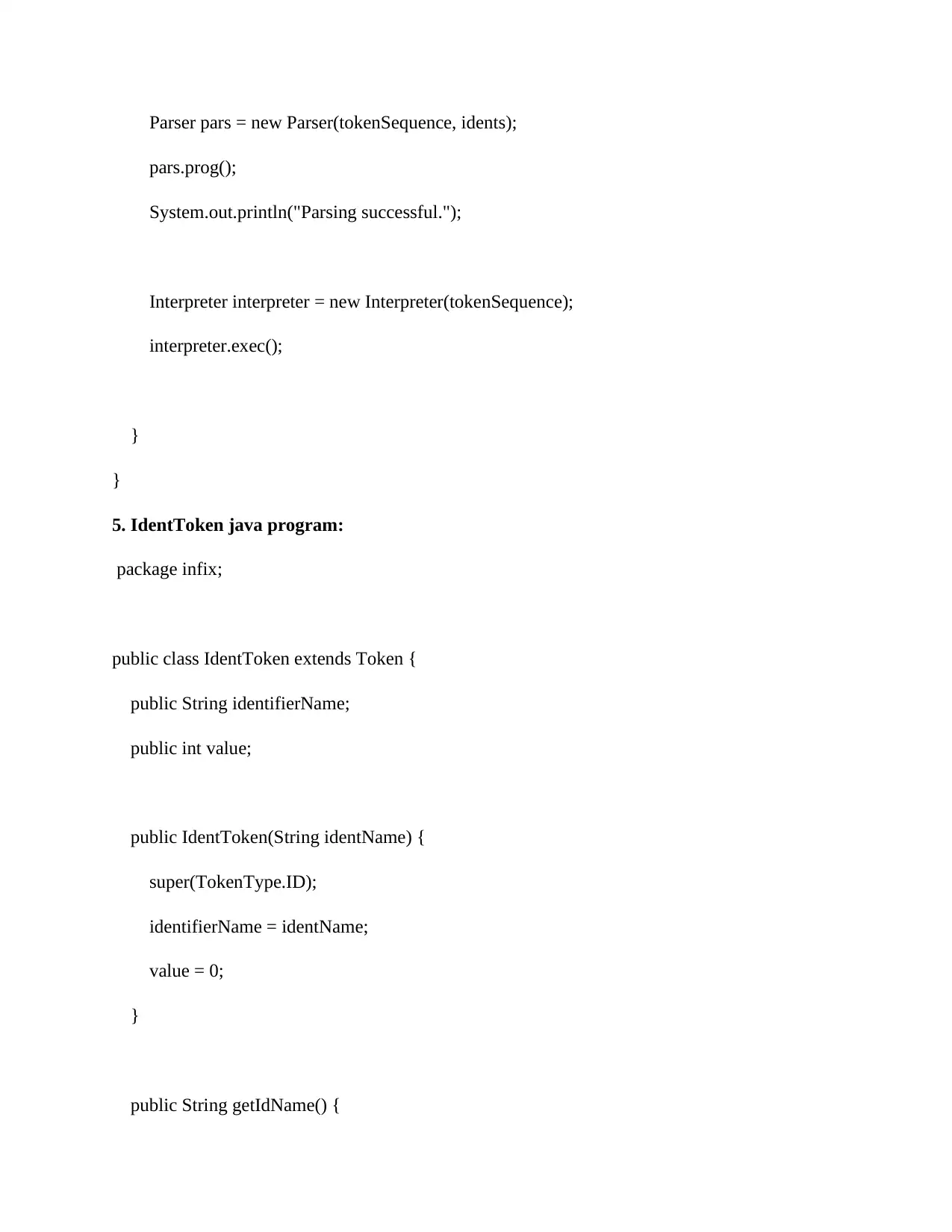
Parser pars = new Parser(tokenSequence, idents);
pars.prog();
System.out.println("Parsing successful.");
Interpreter interpreter = new Interpreter(tokenSequence);
interpreter.exec();
}
}
5. IdentToken java program:
package infix;
public class IdentToken extends Token {
public String identifierName;
public int value;
public IdentToken(String identName) {
super(TokenType.ID);
identifierName = identName;
value = 0;
}
public String getIdName() {
pars.prog();
System.out.println("Parsing successful.");
Interpreter interpreter = new Interpreter(tokenSequence);
interpreter.exec();
}
}
5. IdentToken java program:
package infix;
public class IdentToken extends Token {
public String identifierName;
public int value;
public IdentToken(String identName) {
super(TokenType.ID);
identifierName = identName;
value = 0;
}
public String getIdName() {
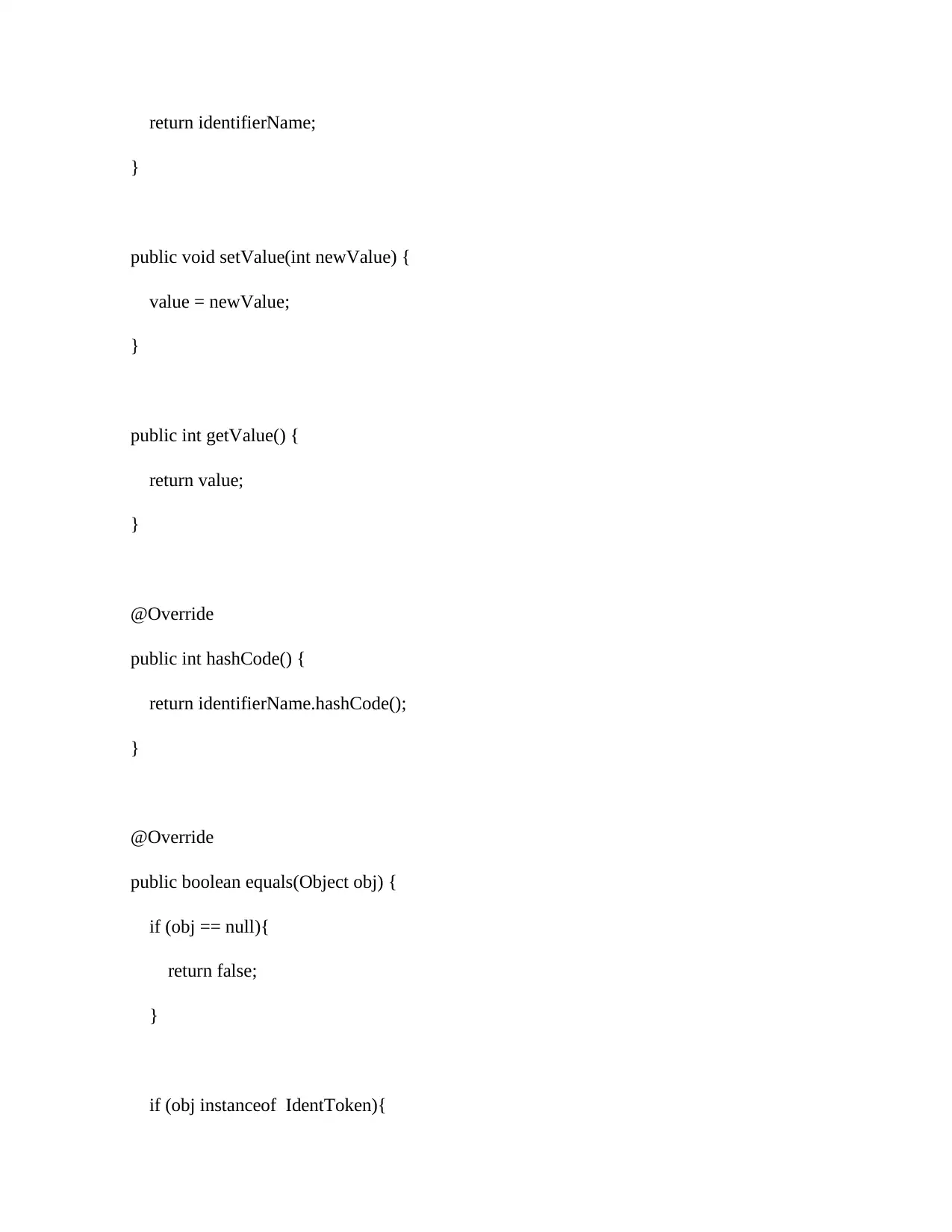
return identifierName;
}
public void setValue(int newValue) {
value = newValue;
}
public int getValue() {
return value;
}
@Override
public int hashCode() {
return identifierName.hashCode();
}
@Override
public boolean equals(Object obj) {
if (obj == null){
return false;
}
if (obj instanceof IdentToken){
}
public void setValue(int newValue) {
value = newValue;
}
public int getValue() {
return value;
}
@Override
public int hashCode() {
return identifierName.hashCode();
}
@Override
public boolean equals(Object obj) {
if (obj == null){
return false;
}
if (obj instanceof IdentToken){
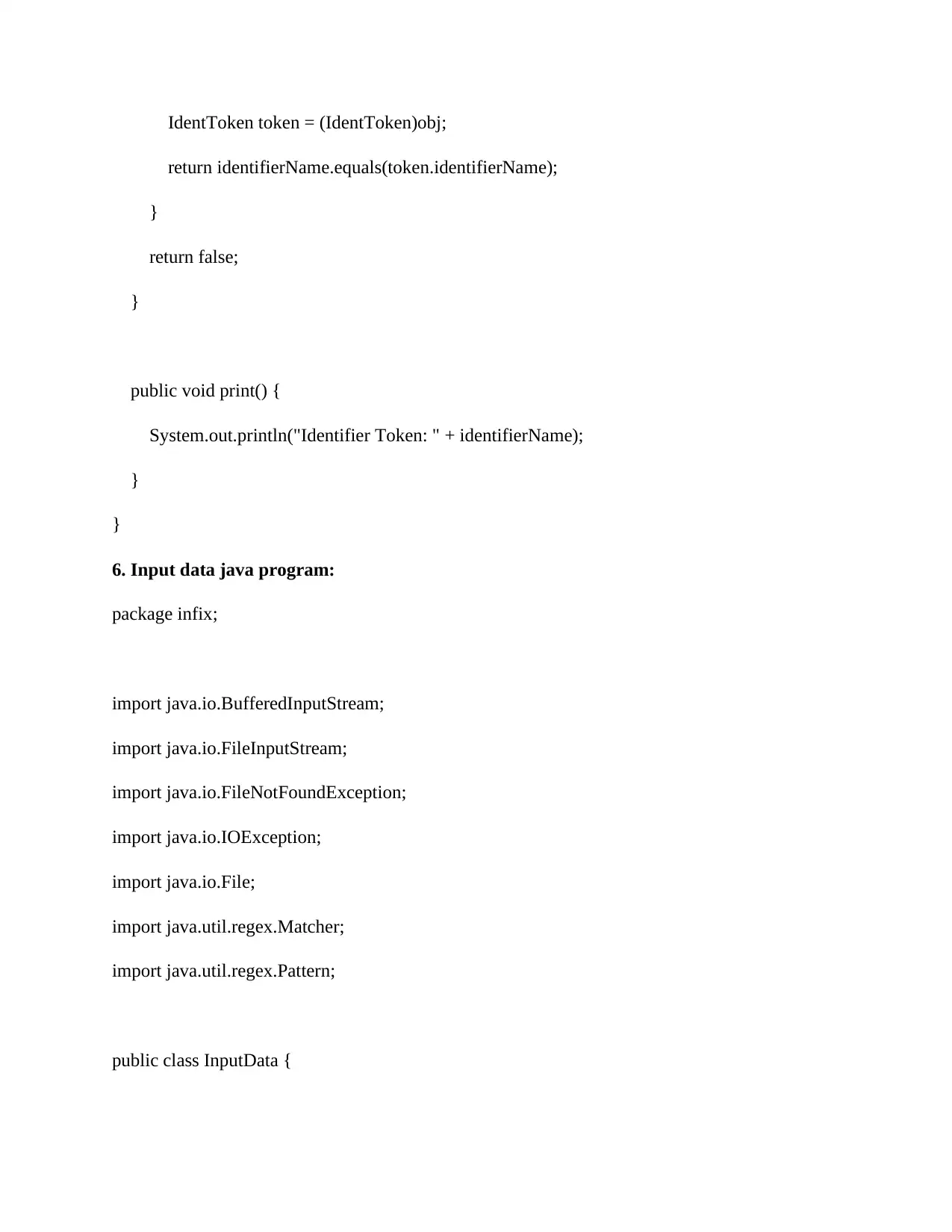
IdentToken token = (IdentToken)obj;
return identifierName.equals(token.identifierName);
}
return false;
}
public void print() {
System.out.println("Identifier Token: " + identifierName);
}
}
6. Input data java program:
package infix;
import java.io.BufferedInputStream;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.File;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class InputData {
return identifierName.equals(token.identifierName);
}
return false;
}
public void print() {
System.out.println("Identifier Token: " + identifierName);
}
}
6. Input data java program:
package infix;
import java.io.BufferedInputStream;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.File;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class InputData {
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
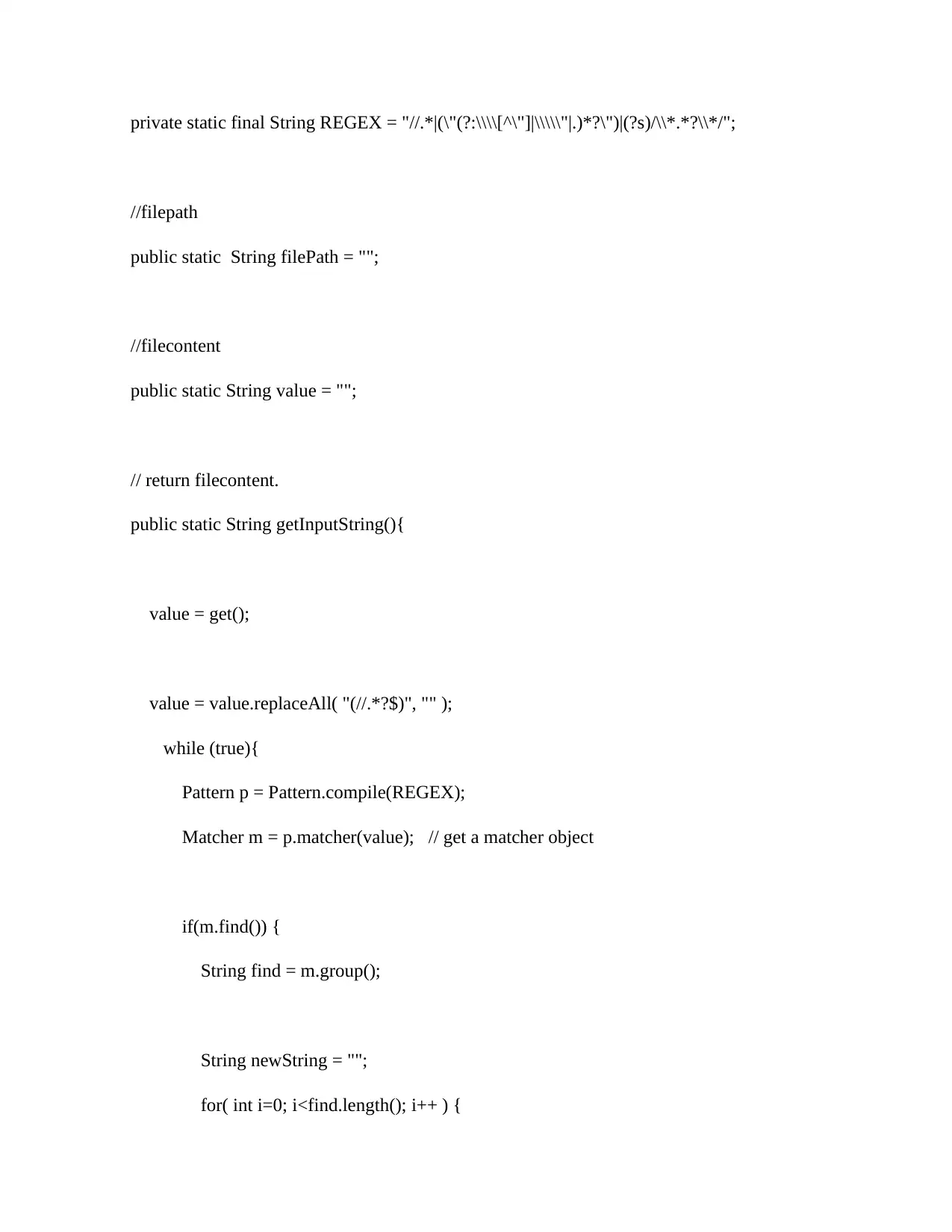
private static final String REGEX = "//.*|(\"(?:\\\\[^\"]|\\\\\"|.)*?\")|(?s)/\\*.*?\\*/";
//filepath
public static String filePath = "";
//filecontent
public static String value = "";
// return filecontent.
public static String getInputString(){
value = get();
value = value.replaceAll( "(//.*?$)", "" );
while (true){
Pattern p = Pattern.compile(REGEX);
Matcher m = p.matcher(value); // get a matcher object
if(m.find()) {
String find = m.group();
String newString = "";
for( int i=0; i<find.length(); i++ ) {
//filepath
public static String filePath = "";
//filecontent
public static String value = "";
// return filecontent.
public static String getInputString(){
value = get();
value = value.replaceAll( "(//.*?$)", "" );
while (true){
Pattern p = Pattern.compile(REGEX);
Matcher m = p.matcher(value); // get a matcher object
if(m.find()) {
String find = m.group();
String newString = "";
for( int i=0; i<find.length(); i++ ) {
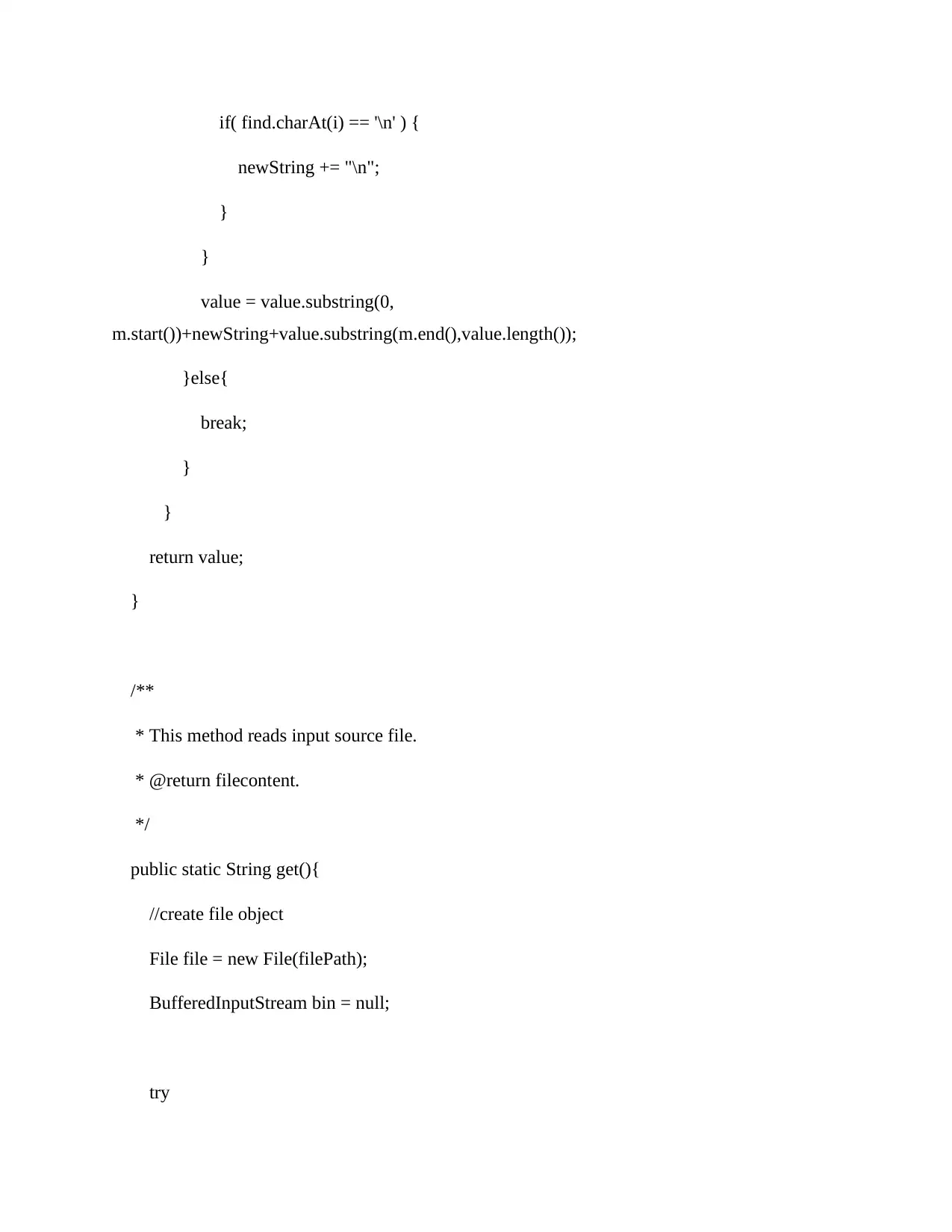
if( find.charAt(i) == '\n' ) {
newString += "\n";
}
}
value = value.substring(0,
m.start())+newString+value.substring(m.end(),value.length());
}else{
break;
}
}
return value;
}
/**
* This method reads input source file.
* @return filecontent.
*/
public static String get(){
//create file object
File file = new File(filePath);
BufferedInputStream bin = null;
try
newString += "\n";
}
}
value = value.substring(0,
m.start())+newString+value.substring(m.end(),value.length());
}else{
break;
}
}
return value;
}
/**
* This method reads input source file.
* @return filecontent.
*/
public static String get(){
//create file object
File file = new File(filePath);
BufferedInputStream bin = null;
try
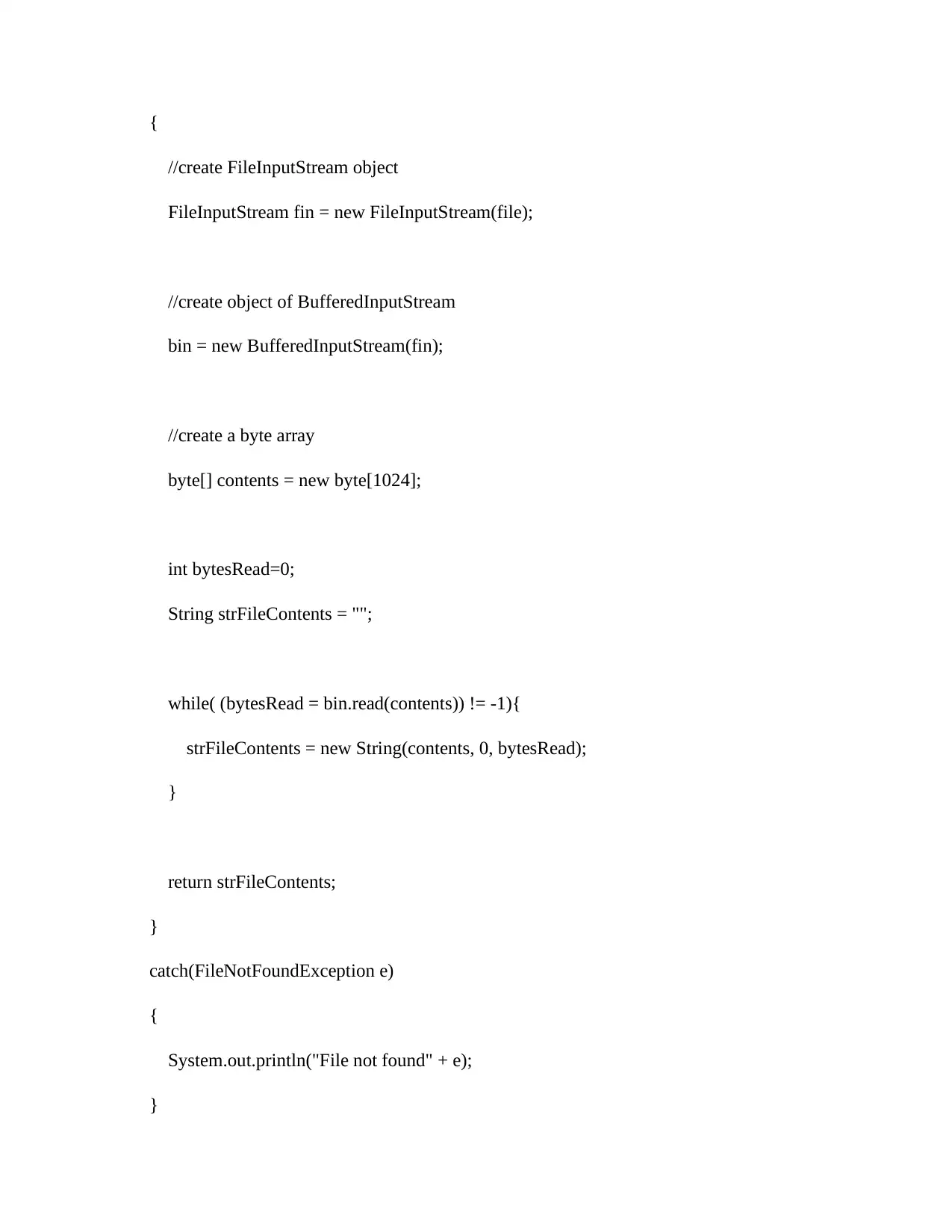
{
//create FileInputStream object
FileInputStream fin = new FileInputStream(file);
//create object of BufferedInputStream
bin = new BufferedInputStream(fin);
//create a byte array
byte[] contents = new byte[1024];
int bytesRead=0;
String strFileContents = "";
while( (bytesRead = bin.read(contents)) != -1){
strFileContents = new String(contents, 0, bytesRead);
}
return strFileContents;
}
catch(FileNotFoundException e)
{
System.out.println("File not found" + e);
}
//create FileInputStream object
FileInputStream fin = new FileInputStream(file);
//create object of BufferedInputStream
bin = new BufferedInputStream(fin);
//create a byte array
byte[] contents = new byte[1024];
int bytesRead=0;
String strFileContents = "";
while( (bytesRead = bin.read(contents)) != -1){
strFileContents = new String(contents, 0, bytesRead);
}
return strFileContents;
}
catch(FileNotFoundException e)
{
System.out.println("File not found" + e);
}
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
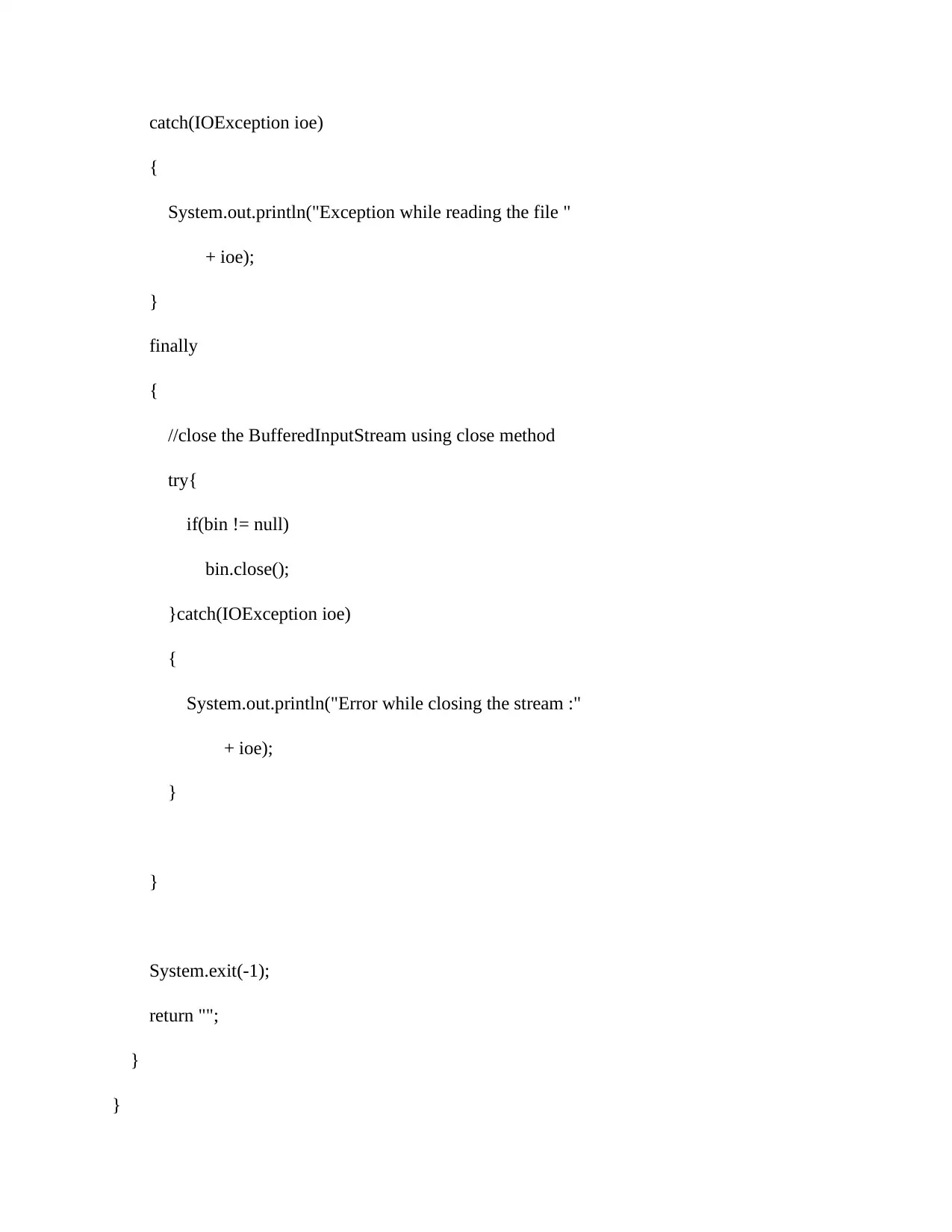
catch(IOException ioe)
{
System.out.println("Exception while reading the file "
+ ioe);
}
finally
{
//close the BufferedInputStream using close method
try{
if(bin != null)
bin.close();
}catch(IOException ioe)
{
System.out.println("Error while closing the stream :"
+ ioe);
}
}
System.exit(-1);
return "";
}
}
{
System.out.println("Exception while reading the file "
+ ioe);
}
finally
{
//close the BufferedInputStream using close method
try{
if(bin != null)
bin.close();
}catch(IOException ioe)
{
System.out.println("Error while closing the stream :"
+ ioe);
}
}
System.exit(-1);
return "";
}
}
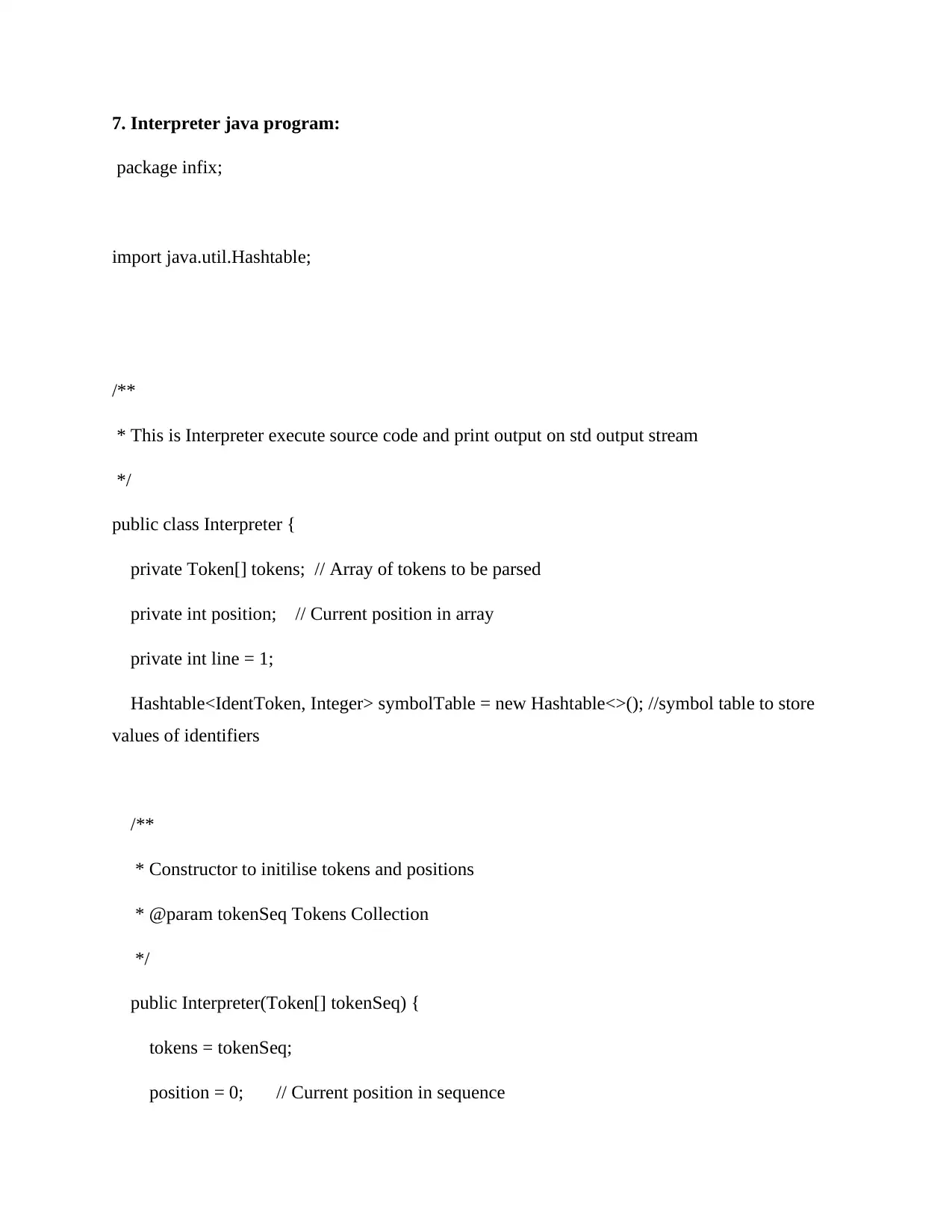
7. Interpreter java program:
package infix;
import java.util.Hashtable;
/**
* This is Interpreter execute source code and print output on std output stream
*/
public class Interpreter {
private Token[] tokens; // Array of tokens to be parsed
private int position; // Current position in array
private int line = 1;
Hashtable<IdentToken, Integer> symbolTable = new Hashtable<>(); //symbol table to store
values of identifiers
/**
* Constructor to initilise tokens and positions
* @param tokenSeq Tokens Collection
*/
public Interpreter(Token[] tokenSeq) {
tokens = tokenSeq;
position = 0; // Current position in sequence
package infix;
import java.util.Hashtable;
/**
* This is Interpreter execute source code and print output on std output stream
*/
public class Interpreter {
private Token[] tokens; // Array of tokens to be parsed
private int position; // Current position in array
private int line = 1;
Hashtable<IdentToken, Integer> symbolTable = new Hashtable<>(); //symbol table to store
values of identifiers
/**
* Constructor to initilise tokens and positions
* @param tokenSeq Tokens Collection
*/
public Interpreter(Token[] tokenSeq) {
tokens = tokenSeq;
position = 0; // Current position in sequence
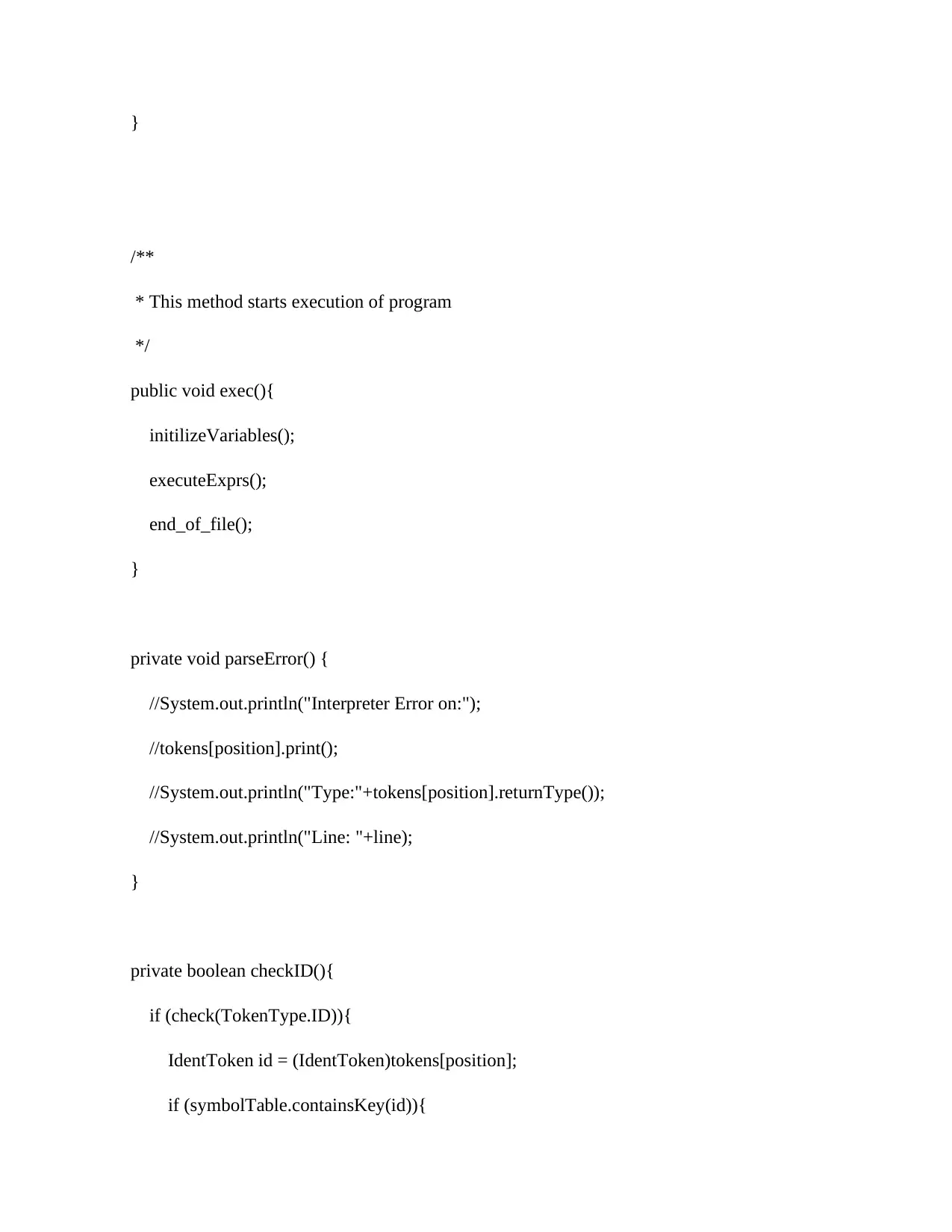
}
/**
* This method starts execution of program
*/
public void exec(){
initilizeVariables();
executeExprs();
end_of_file();
}
private void parseError() {
//System.out.println("Interpreter Error on:");
//tokens[position].print();
//System.out.println("Type:"+tokens[position].returnType());
//System.out.println("Line: "+line);
}
private boolean checkID(){
if (check(TokenType.ID)){
IdentToken id = (IdentToken)tokens[position];
if (symbolTable.containsKey(id)){
/**
* This method starts execution of program
*/
public void exec(){
initilizeVariables();
executeExprs();
end_of_file();
}
private void parseError() {
//System.out.println("Interpreter Error on:");
//tokens[position].print();
//System.out.println("Type:"+tokens[position].returnType());
//System.out.println("Line: "+line);
}
private boolean checkID(){
if (check(TokenType.ID)){
IdentToken id = (IdentToken)tokens[position];
if (symbolTable.containsKey(id)){
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
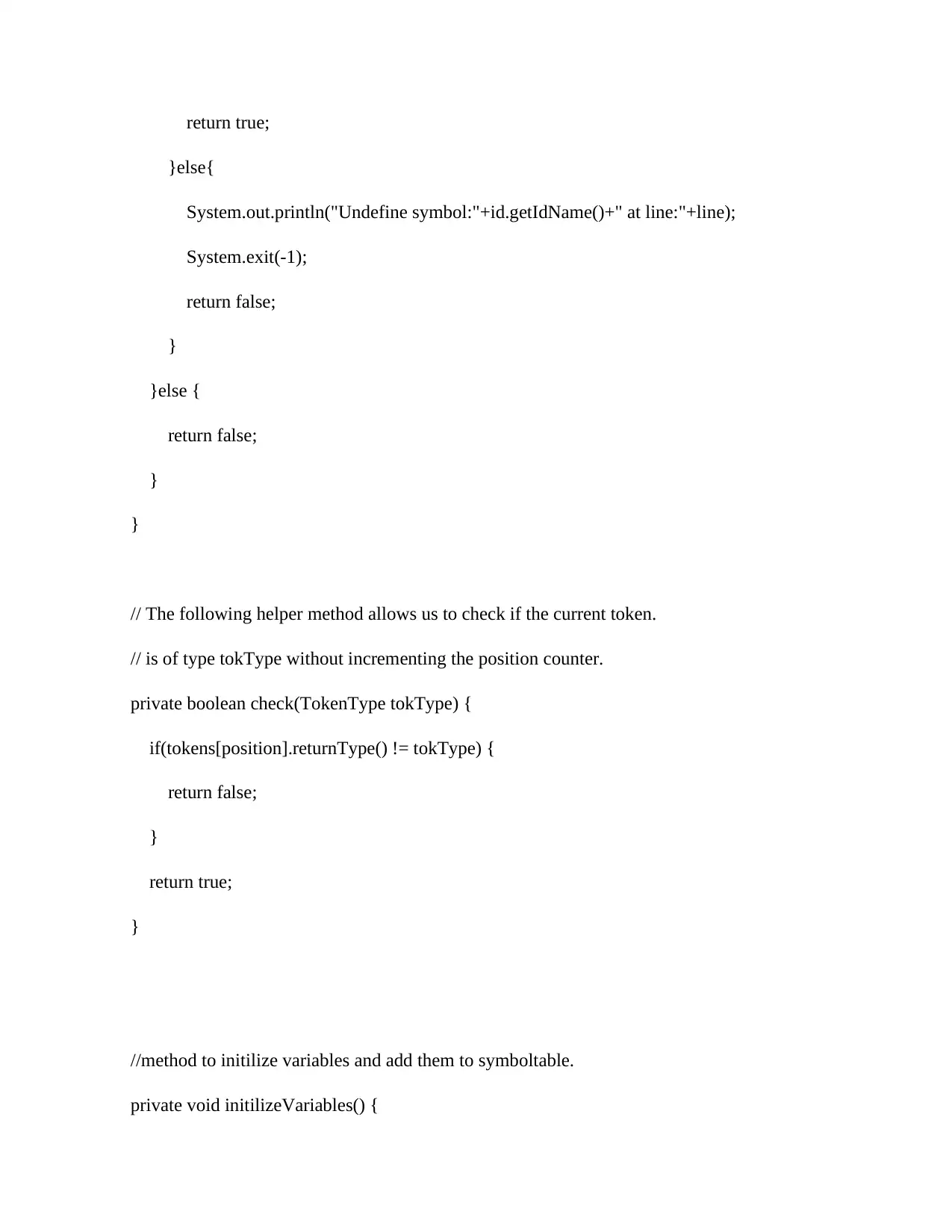
return true;
}else{
System.out.println("Undefine symbol:"+id.getIdName()+" at line:"+line);
System.exit(-1);
return false;
}
}else {
return false;
}
}
// The following helper method allows us to check if the current token.
// is of type tokType without incrementing the position counter.
private boolean check(TokenType tokType) {
if(tokens[position].returnType() != tokType) {
return false;
}
return true;
}
//method to initilize variables and add them to symboltable.
private void initilizeVariables() {
}else{
System.out.println("Undefine symbol:"+id.getIdName()+" at line:"+line);
System.exit(-1);
return false;
}
}else {
return false;
}
}
// The following helper method allows us to check if the current token.
// is of type tokType without incrementing the position counter.
private boolean check(TokenType tokType) {
if(tokens[position].returnType() != tokType) {
return false;
}
return true;
}
//method to initilize variables and add them to symboltable.
private void initilizeVariables() {
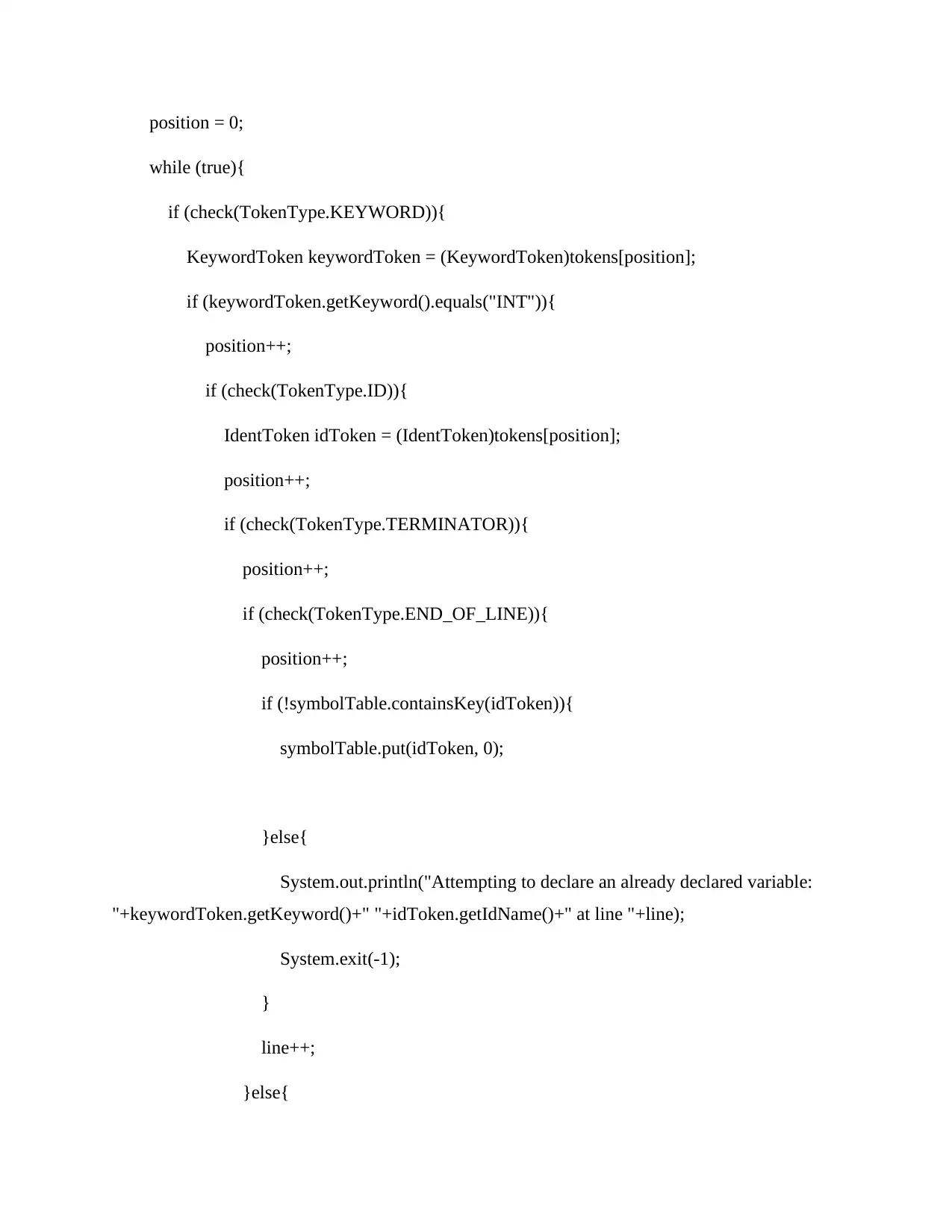
position = 0;
while (true){
if (check(TokenType.KEYWORD)){
KeywordToken keywordToken = (KeywordToken)tokens[position];
if (keywordToken.getKeyword().equals("INT")){
position++;
if (check(TokenType.ID)){
IdentToken idToken = (IdentToken)tokens[position];
position++;
if (check(TokenType.TERMINATOR)){
position++;
if (check(TokenType.END_OF_LINE)){
position++;
if (!symbolTable.containsKey(idToken)){
symbolTable.put(idToken, 0);
}else{
System.out.println("Attempting to declare an already declared variable:
"+keywordToken.getKeyword()+" "+idToken.getIdName()+" at line "+line);
System.exit(-1);
}
line++;
}else{
while (true){
if (check(TokenType.KEYWORD)){
KeywordToken keywordToken = (KeywordToken)tokens[position];
if (keywordToken.getKeyword().equals("INT")){
position++;
if (check(TokenType.ID)){
IdentToken idToken = (IdentToken)tokens[position];
position++;
if (check(TokenType.TERMINATOR)){
position++;
if (check(TokenType.END_OF_LINE)){
position++;
if (!symbolTable.containsKey(idToken)){
symbolTable.put(idToken, 0);
}else{
System.out.println("Attempting to declare an already declared variable:
"+keywordToken.getKeyword()+" "+idToken.getIdName()+" at line "+line);
System.exit(-1);
}
line++;
}else{
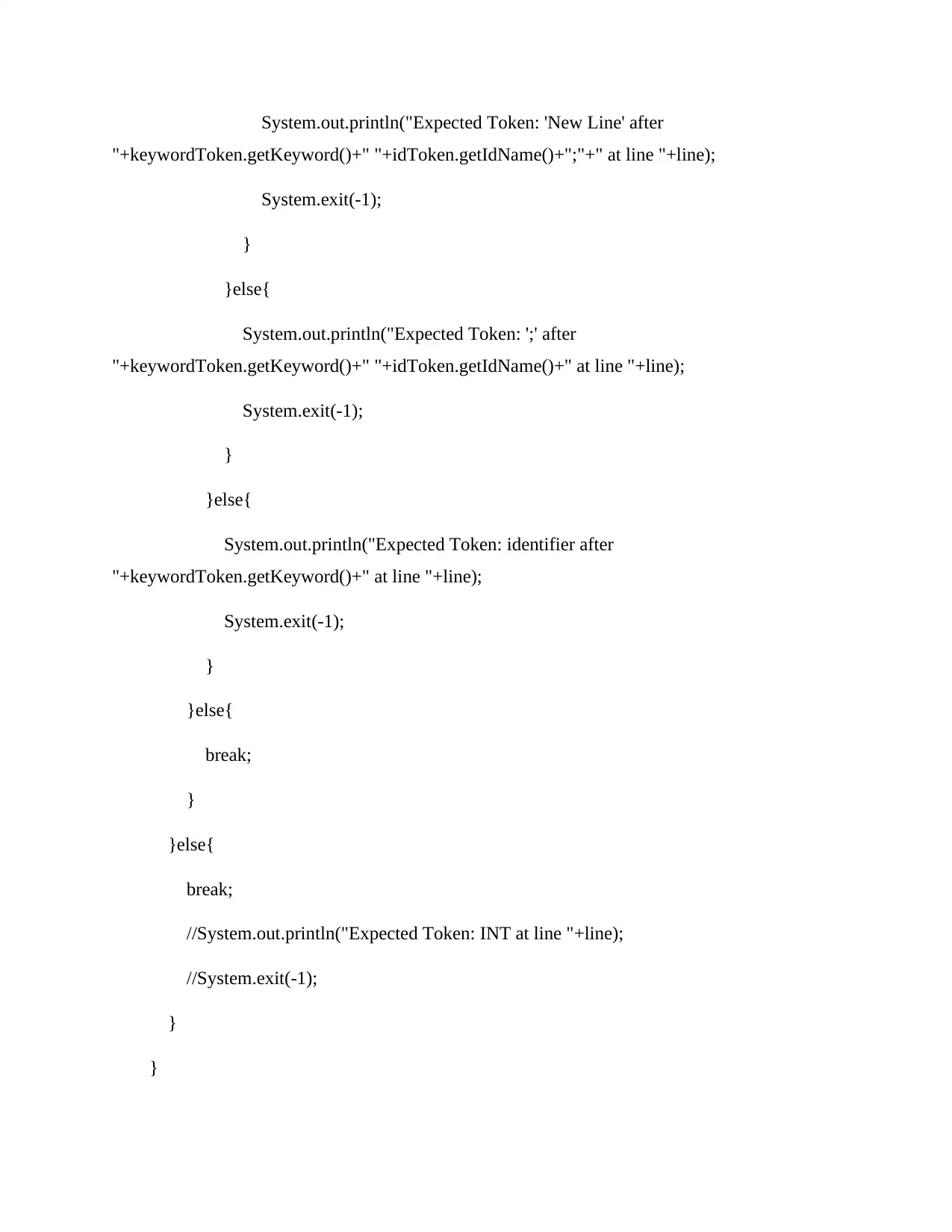
System.out.println("Expected Token: 'New Line' after
"+keywordToken.getKeyword()+" "+idToken.getIdName()+";"+" at line "+line);
System.exit(-1);
}
}else{
System.out.println("Expected Token: ';' after
"+keywordToken.getKeyword()+" "+idToken.getIdName()+" at line "+line);
System.exit(-1);
}
}else{
System.out.println("Expected Token: identifier after
"+keywordToken.getKeyword()+" at line "+line);
System.exit(-1);
}
}else{
break;
}
}else{
break;
//System.out.println("Expected Token: INT at line "+line);
//System.exit(-1);
}
}
"+keywordToken.getKeyword()+" "+idToken.getIdName()+";"+" at line "+line);
System.exit(-1);
}
}else{
System.out.println("Expected Token: ';' after
"+keywordToken.getKeyword()+" "+idToken.getIdName()+" at line "+line);
System.exit(-1);
}
}else{
System.out.println("Expected Token: identifier after
"+keywordToken.getKeyword()+" at line "+line);
System.exit(-1);
}
}else{
break;
}
}else{
break;
//System.out.println("Expected Token: INT at line "+line);
//System.exit(-1);
}
}
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
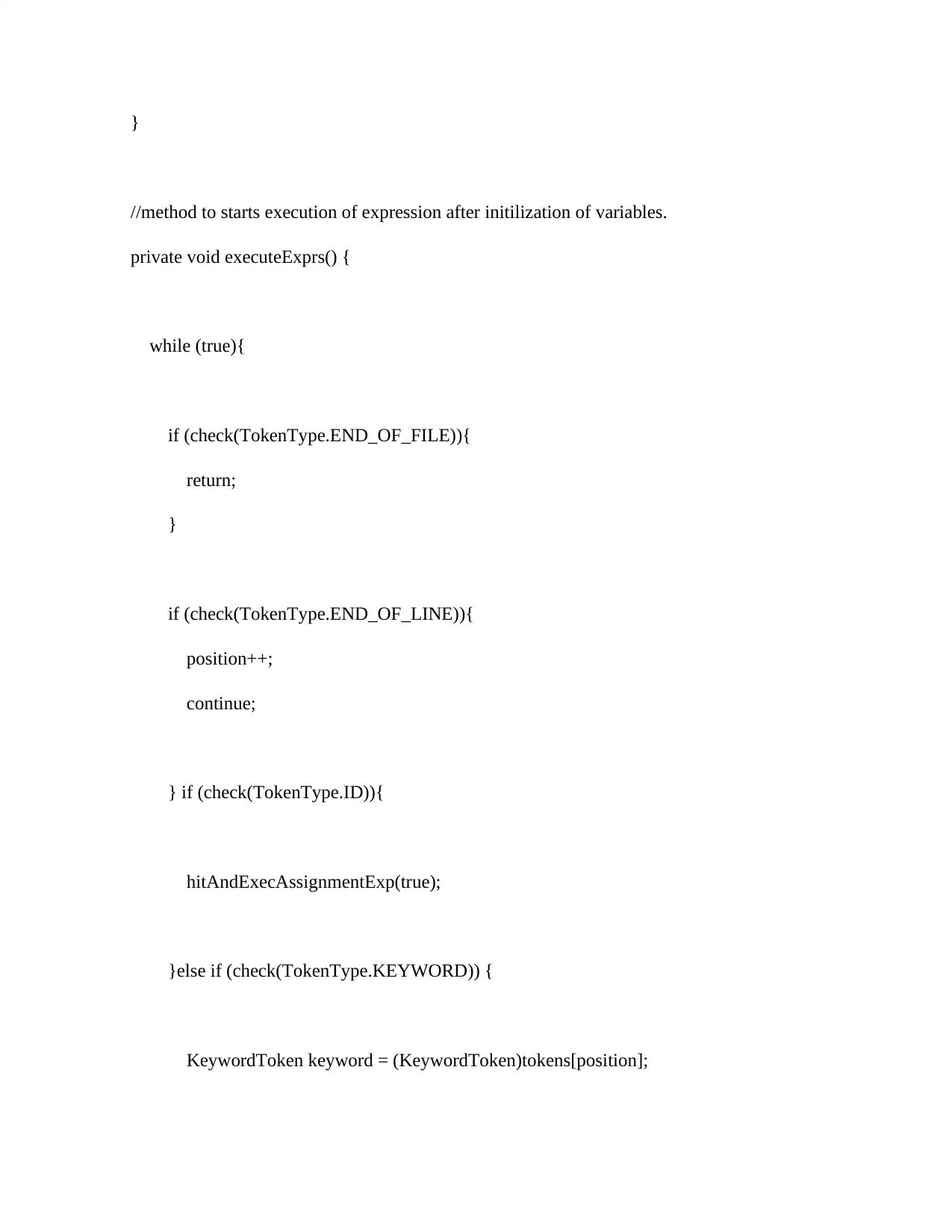
}
//method to starts execution of expression after initilization of variables.
private void executeExprs() {
while (true){
if (check(TokenType.END_OF_FILE)){
return;
}
if (check(TokenType.END_OF_LINE)){
position++;
continue;
} if (check(TokenType.ID)){
hitAndExecAssignmentExp(true);
}else if (check(TokenType.KEYWORD)) {
KeywordToken keyword = (KeywordToken)tokens[position];
//method to starts execution of expression after initilization of variables.
private void executeExprs() {
while (true){
if (check(TokenType.END_OF_FILE)){
return;
}
if (check(TokenType.END_OF_LINE)){
position++;
continue;
} if (check(TokenType.ID)){
hitAndExecAssignmentExp(true);
}else if (check(TokenType.KEYWORD)) {
KeywordToken keyword = (KeywordToken)tokens[position];
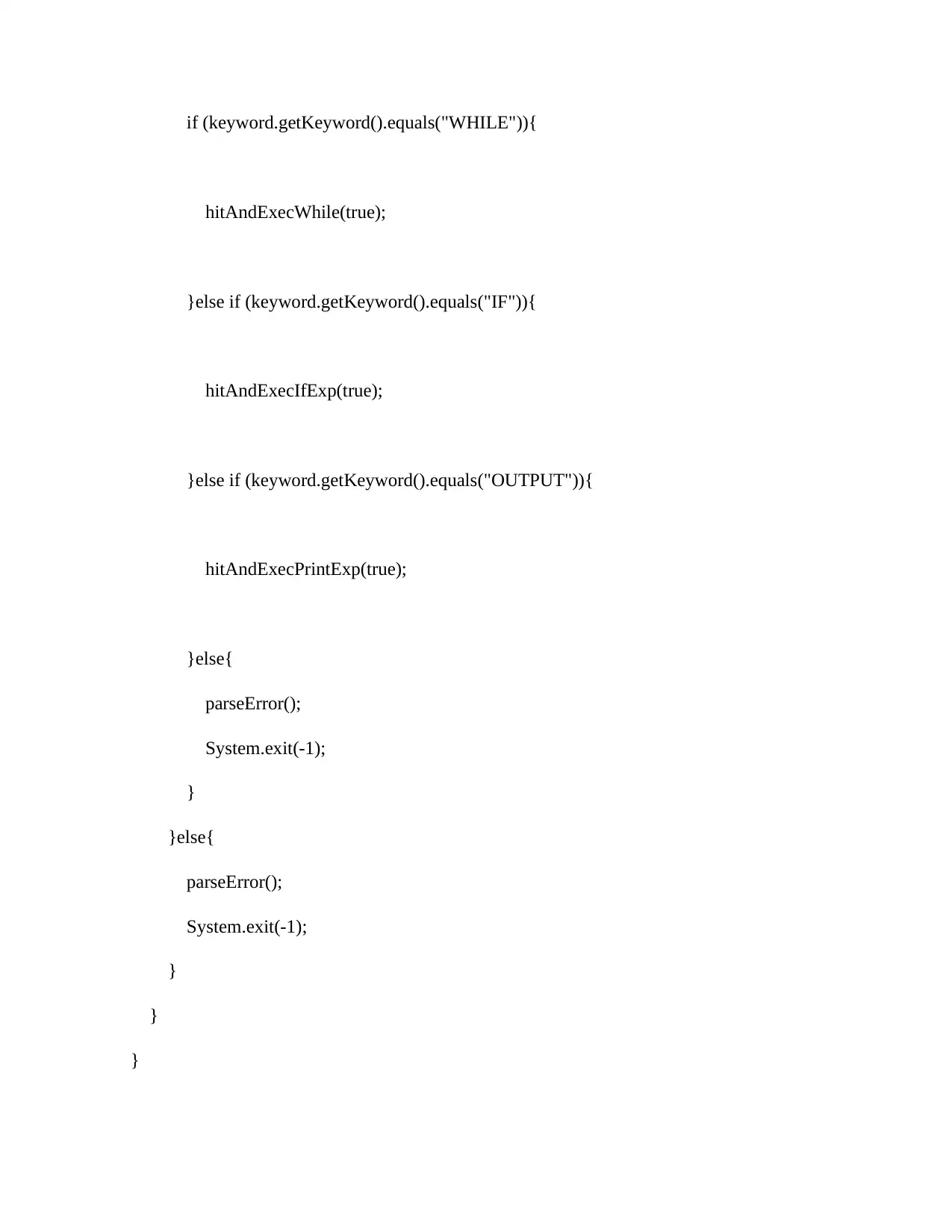
if (keyword.getKeyword().equals("WHILE")){
hitAndExecWhile(true);
}else if (keyword.getKeyword().equals("IF")){
hitAndExecIfExp(true);
}else if (keyword.getKeyword().equals("OUTPUT")){
hitAndExecPrintExp(true);
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}
}
hitAndExecWhile(true);
}else if (keyword.getKeyword().equals("IF")){
hitAndExecIfExp(true);
}else if (keyword.getKeyword().equals("OUTPUT")){
hitAndExecPrintExp(true);
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}
}
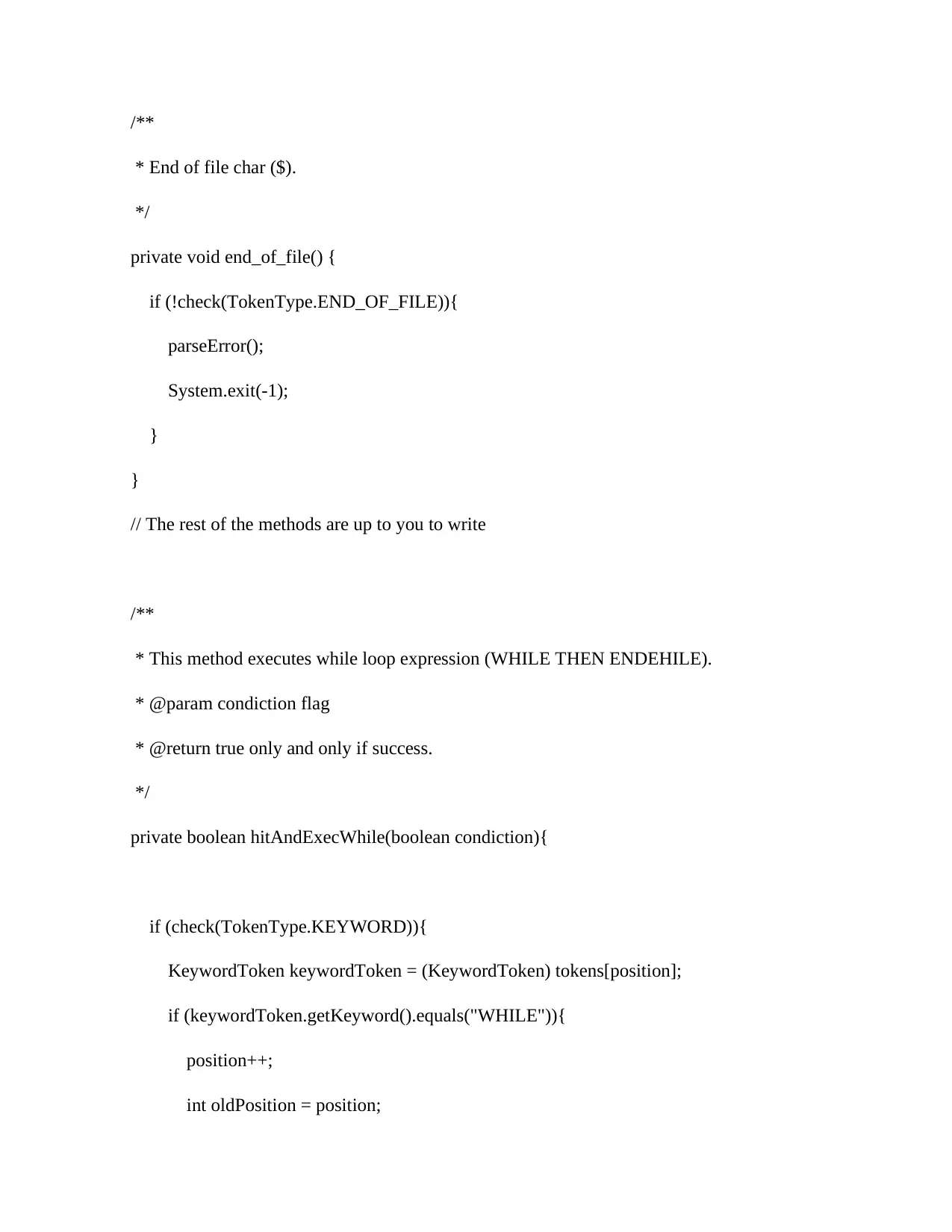
/**
* End of file char ($).
*/
private void end_of_file() {
if (!check(TokenType.END_OF_FILE)){
parseError();
System.exit(-1);
}
}
// The rest of the methods are up to you to write
/**
* This method executes while loop expression (WHILE THEN ENDEHILE).
* @param condiction flag
* @return true only and only if success.
*/
private boolean hitAndExecWhile(boolean condiction){
if (check(TokenType.KEYWORD)){
KeywordToken keywordToken = (KeywordToken) tokens[position];
if (keywordToken.getKeyword().equals("WHILE")){
position++;
int oldPosition = position;
* End of file char ($).
*/
private void end_of_file() {
if (!check(TokenType.END_OF_FILE)){
parseError();
System.exit(-1);
}
}
// The rest of the methods are up to you to write
/**
* This method executes while loop expression (WHILE THEN ENDEHILE).
* @param condiction flag
* @return true only and only if success.
*/
private boolean hitAndExecWhile(boolean condiction){
if (check(TokenType.KEYWORD)){
KeywordToken keywordToken = (KeywordToken) tokens[position];
if (keywordToken.getKeyword().equals("WHILE")){
position++;
int oldPosition = position;
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
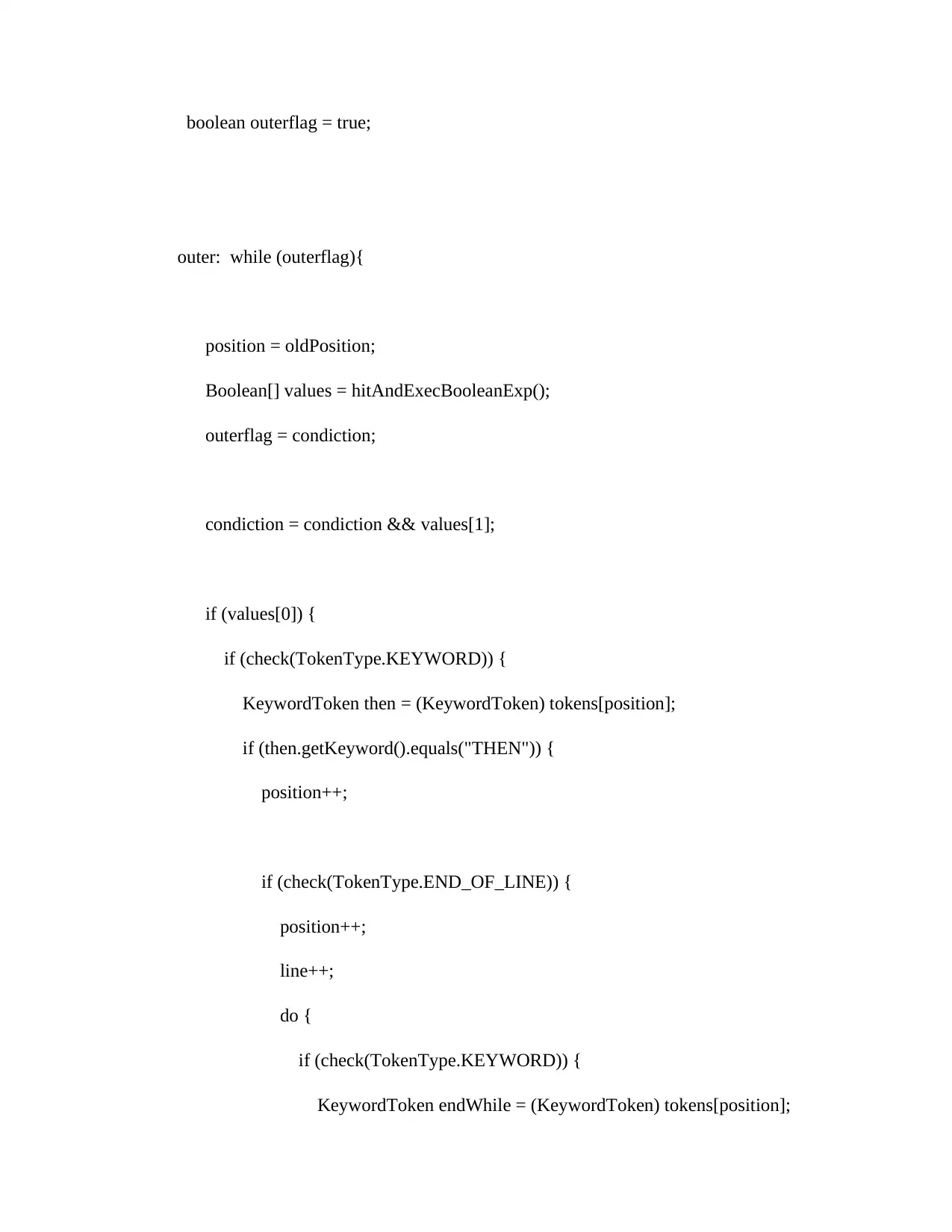
boolean outerflag = true;
outer: while (outerflag){
position = oldPosition;
Boolean[] values = hitAndExecBooleanExp();
outerflag = condiction;
condiction = condiction && values[1];
if (values[0]) {
if (check(TokenType.KEYWORD)) {
KeywordToken then = (KeywordToken) tokens[position];
if (then.getKeyword().equals("THEN")) {
position++;
if (check(TokenType.END_OF_LINE)) {
position++;
line++;
do {
if (check(TokenType.KEYWORD)) {
KeywordToken endWhile = (KeywordToken) tokens[position];
outer: while (outerflag){
position = oldPosition;
Boolean[] values = hitAndExecBooleanExp();
outerflag = condiction;
condiction = condiction && values[1];
if (values[0]) {
if (check(TokenType.KEYWORD)) {
KeywordToken then = (KeywordToken) tokens[position];
if (then.getKeyword().equals("THEN")) {
position++;
if (check(TokenType.END_OF_LINE)) {
position++;
line++;
do {
if (check(TokenType.KEYWORD)) {
KeywordToken endWhile = (KeywordToken) tokens[position];
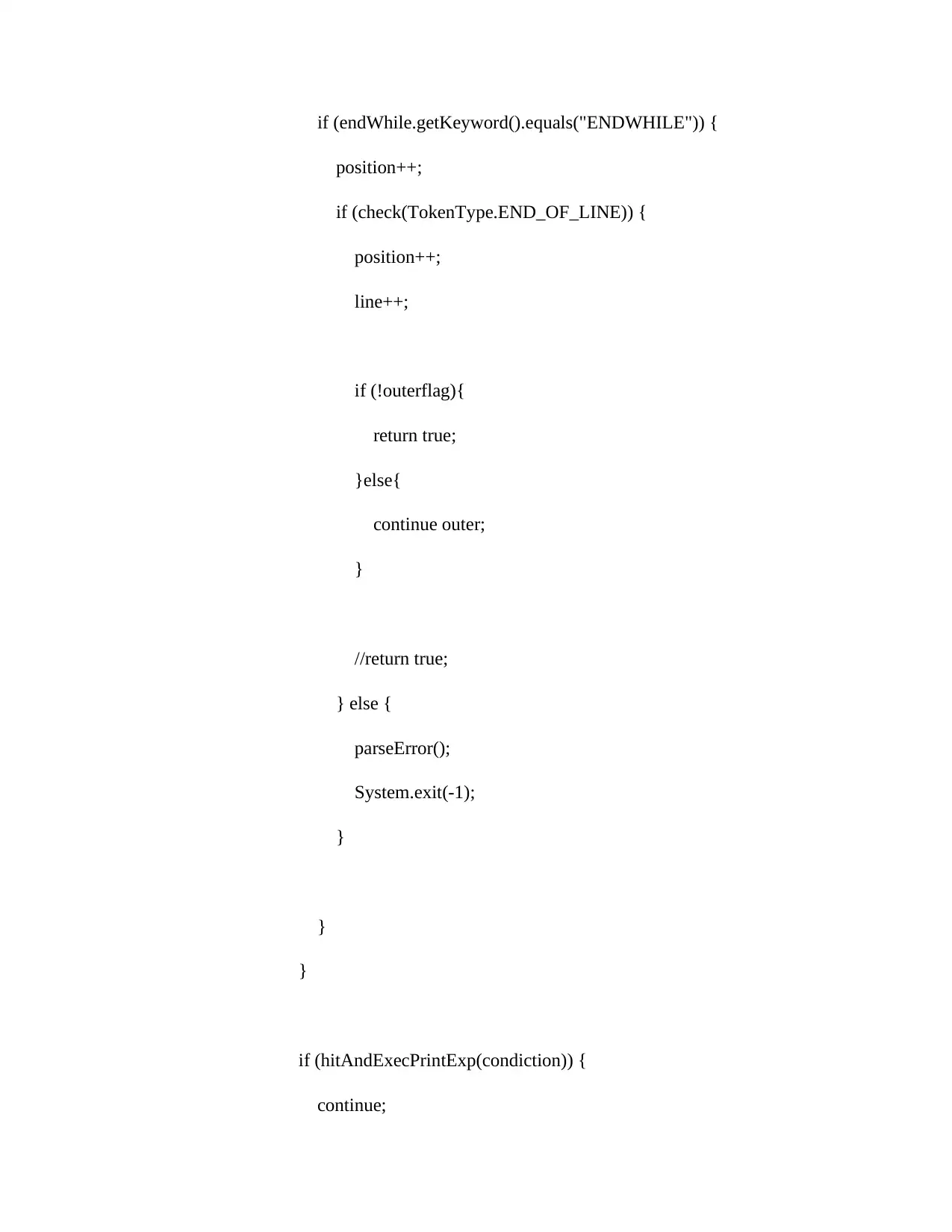
if (endWhile.getKeyword().equals("ENDWHILE")) {
position++;
if (check(TokenType.END_OF_LINE)) {
position++;
line++;
if (!outerflag){
return true;
}else{
continue outer;
}
//return true;
} else {
parseError();
System.exit(-1);
}
}
}
if (hitAndExecPrintExp(condiction)) {
continue;
position++;
if (check(TokenType.END_OF_LINE)) {
position++;
line++;
if (!outerflag){
return true;
}else{
continue outer;
}
//return true;
} else {
parseError();
System.exit(-1);
}
}
}
if (hitAndExecPrintExp(condiction)) {
continue;
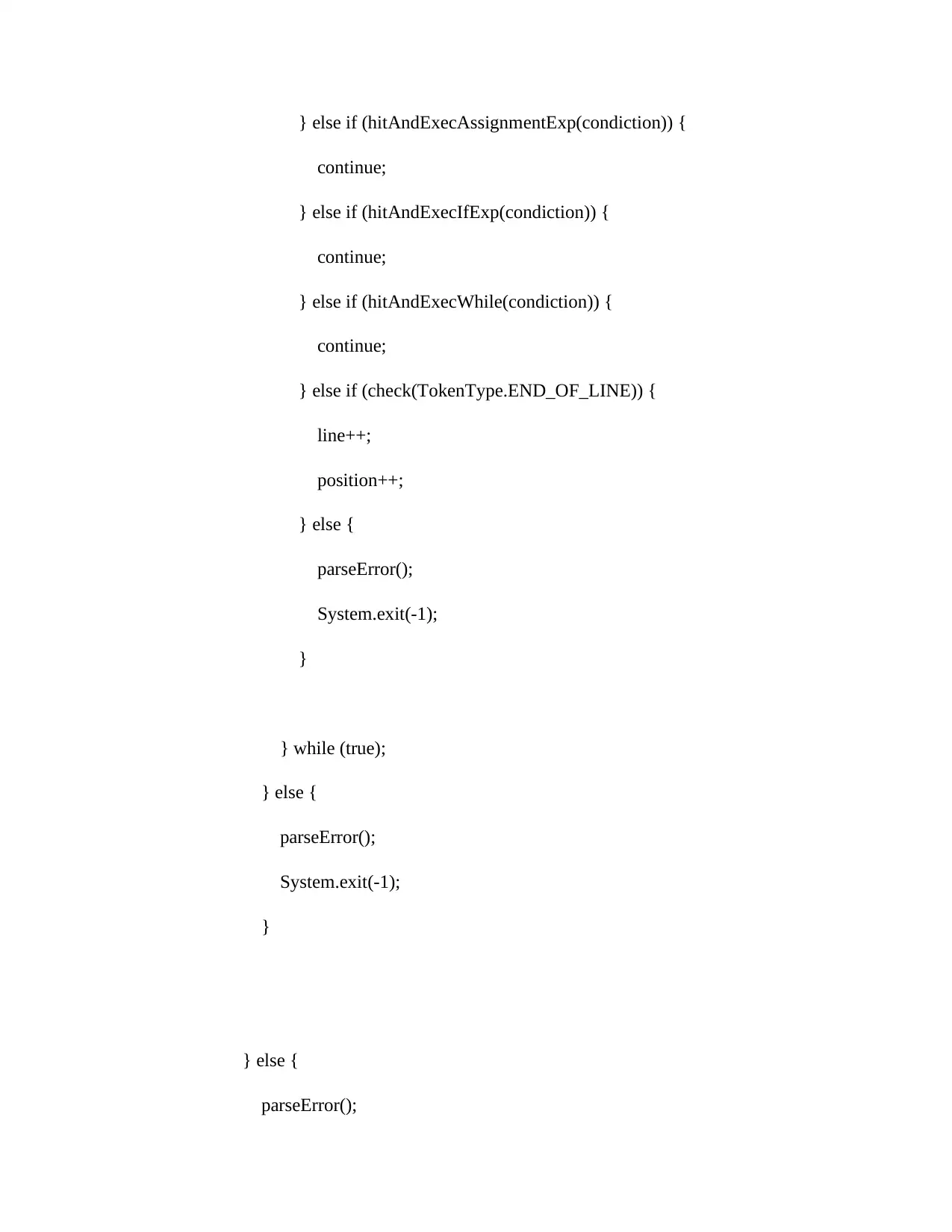
} else if (hitAndExecAssignmentExp(condiction)) {
continue;
} else if (hitAndExecIfExp(condiction)) {
continue;
} else if (hitAndExecWhile(condiction)) {
continue;
} else if (check(TokenType.END_OF_LINE)) {
line++;
position++;
} else {
parseError();
System.exit(-1);
}
} while (true);
} else {
parseError();
System.exit(-1);
}
} else {
parseError();
continue;
} else if (hitAndExecIfExp(condiction)) {
continue;
} else if (hitAndExecWhile(condiction)) {
continue;
} else if (check(TokenType.END_OF_LINE)) {
line++;
position++;
} else {
parseError();
System.exit(-1);
}
} while (true);
} else {
parseError();
System.exit(-1);
}
} else {
parseError();
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
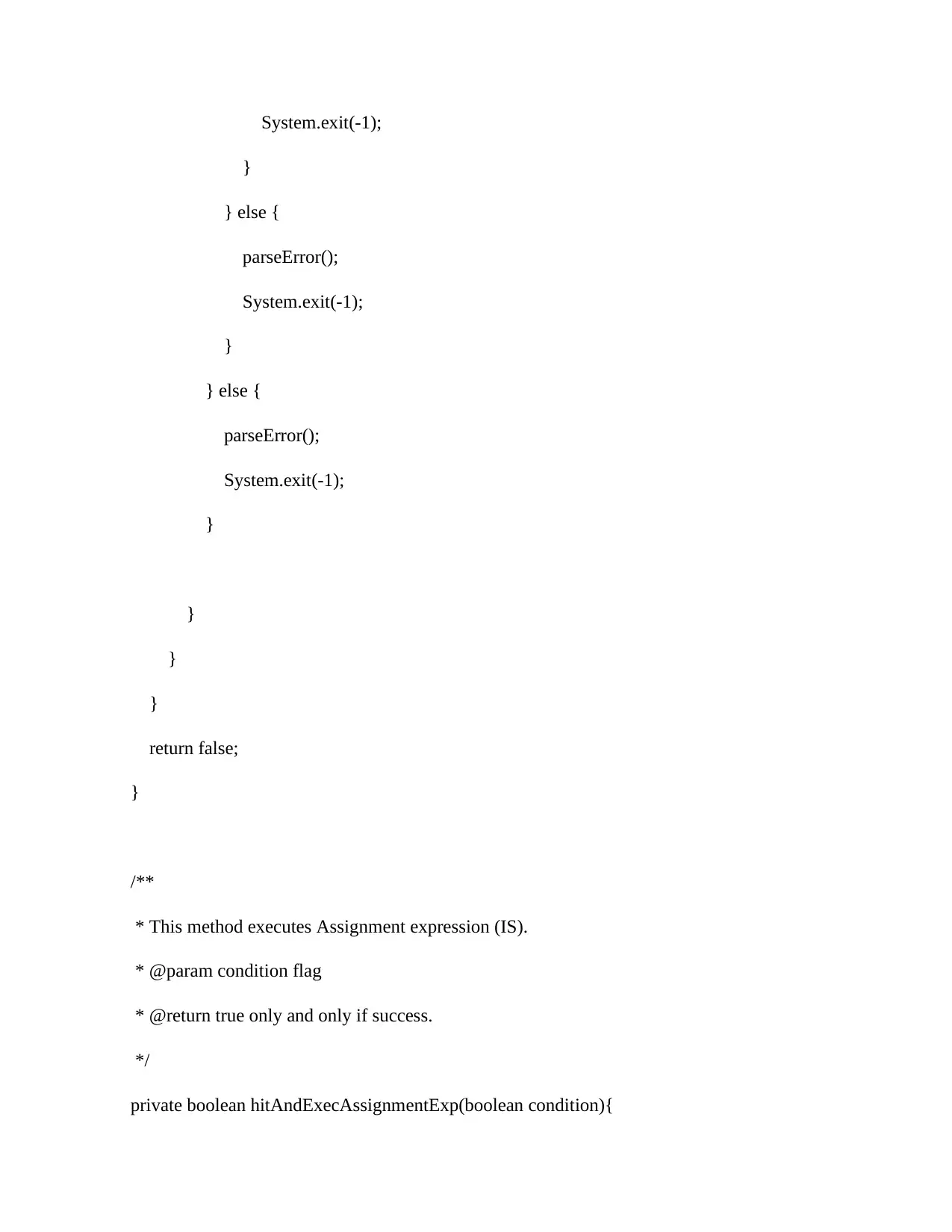
System.exit(-1);
}
} else {
parseError();
System.exit(-1);
}
} else {
parseError();
System.exit(-1);
}
}
}
}
return false;
}
/**
* This method executes Assignment expression (IS).
* @param condition flag
* @return true only and only if success.
*/
private boolean hitAndExecAssignmentExp(boolean condition){
}
} else {
parseError();
System.exit(-1);
}
} else {
parseError();
System.exit(-1);
}
}
}
}
return false;
}
/**
* This method executes Assignment expression (IS).
* @param condition flag
* @return true only and only if success.
*/
private boolean hitAndExecAssignmentExp(boolean condition){
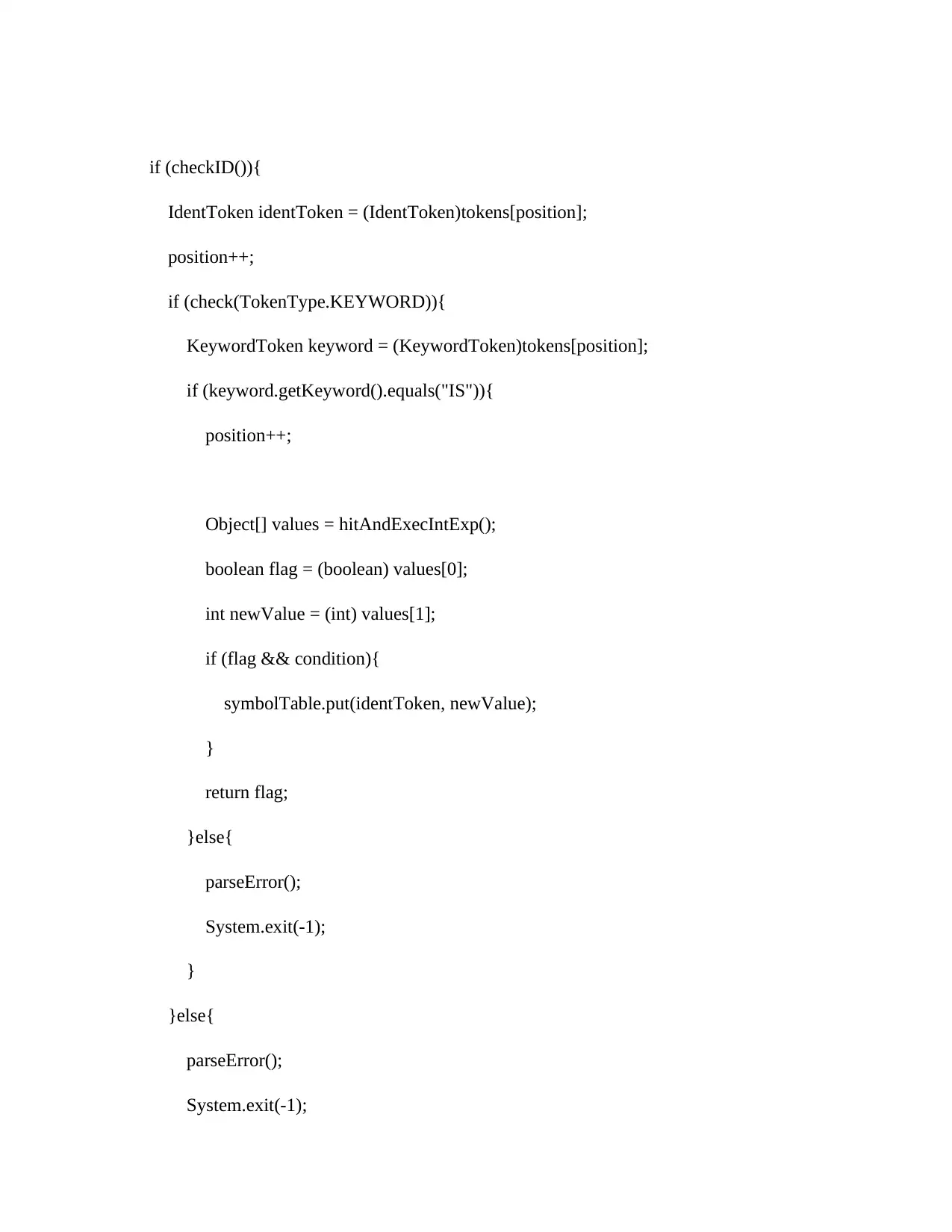
if (checkID()){
IdentToken identToken = (IdentToken)tokens[position];
position++;
if (check(TokenType.KEYWORD)){
KeywordToken keyword = (KeywordToken)tokens[position];
if (keyword.getKeyword().equals("IS")){
position++;
Object[] values = hitAndExecIntExp();
boolean flag = (boolean) values[0];
int newValue = (int) values[1];
if (flag && condition){
symbolTable.put(identToken, newValue);
}
return flag;
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
IdentToken identToken = (IdentToken)tokens[position];
position++;
if (check(TokenType.KEYWORD)){
KeywordToken keyword = (KeywordToken)tokens[position];
if (keyword.getKeyword().equals("IS")){
position++;
Object[] values = hitAndExecIntExp();
boolean flag = (boolean) values[0];
int newValue = (int) values[1];
if (flag && condition){
symbolTable.put(identToken, newValue);
}
return flag;
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
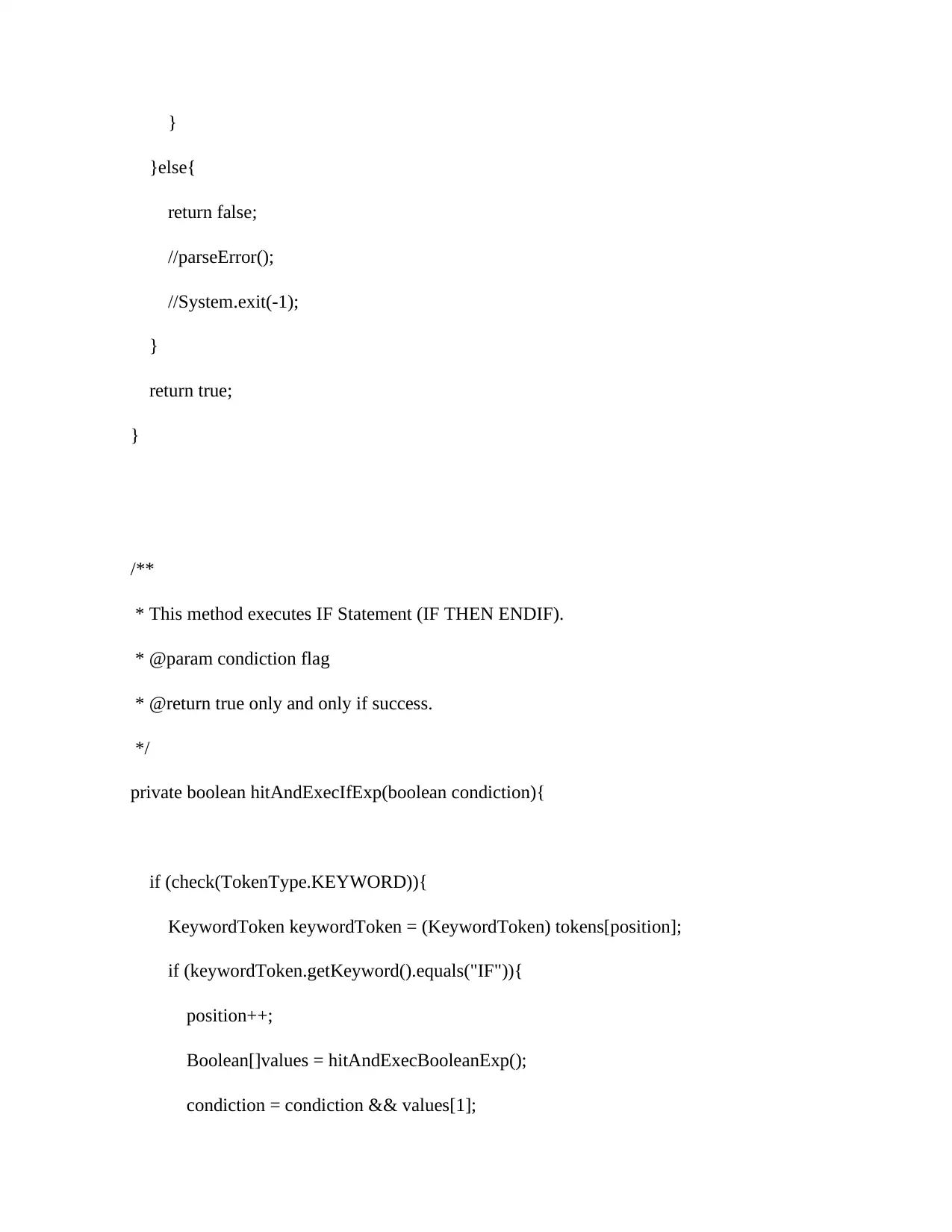
}
}else{
return false;
//parseError();
//System.exit(-1);
}
return true;
}
/**
* This method executes IF Statement (IF THEN ENDIF).
* @param condiction flag
* @return true only and only if success.
*/
private boolean hitAndExecIfExp(boolean condiction){
if (check(TokenType.KEYWORD)){
KeywordToken keywordToken = (KeywordToken) tokens[position];
if (keywordToken.getKeyword().equals("IF")){
position++;
Boolean[]values = hitAndExecBooleanExp();
condiction = condiction && values[1];
}else{
return false;
//parseError();
//System.exit(-1);
}
return true;
}
/**
* This method executes IF Statement (IF THEN ENDIF).
* @param condiction flag
* @return true only and only if success.
*/
private boolean hitAndExecIfExp(boolean condiction){
if (check(TokenType.KEYWORD)){
KeywordToken keywordToken = (KeywordToken) tokens[position];
if (keywordToken.getKeyword().equals("IF")){
position++;
Boolean[]values = hitAndExecBooleanExp();
condiction = condiction && values[1];
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
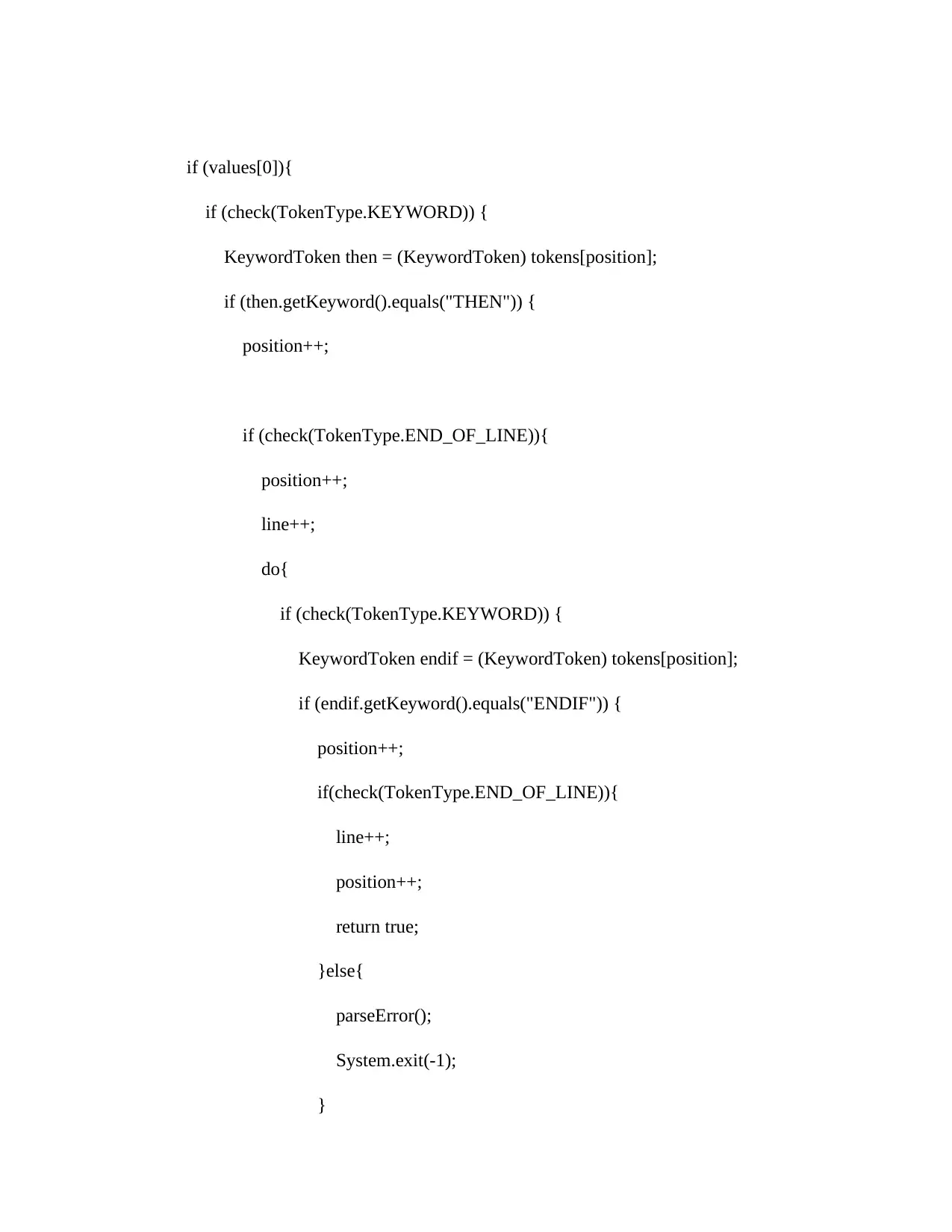
if (values[0]){
if (check(TokenType.KEYWORD)) {
KeywordToken then = (KeywordToken) tokens[position];
if (then.getKeyword().equals("THEN")) {
position++;
if (check(TokenType.END_OF_LINE)){
position++;
line++;
do{
if (check(TokenType.KEYWORD)) {
KeywordToken endif = (KeywordToken) tokens[position];
if (endif.getKeyword().equals("ENDIF")) {
position++;
if(check(TokenType.END_OF_LINE)){
line++;
position++;
return true;
}else{
parseError();
System.exit(-1);
}
if (check(TokenType.KEYWORD)) {
KeywordToken then = (KeywordToken) tokens[position];
if (then.getKeyword().equals("THEN")) {
position++;
if (check(TokenType.END_OF_LINE)){
position++;
line++;
do{
if (check(TokenType.KEYWORD)) {
KeywordToken endif = (KeywordToken) tokens[position];
if (endif.getKeyword().equals("ENDIF")) {
position++;
if(check(TokenType.END_OF_LINE)){
line++;
position++;
return true;
}else{
parseError();
System.exit(-1);
}
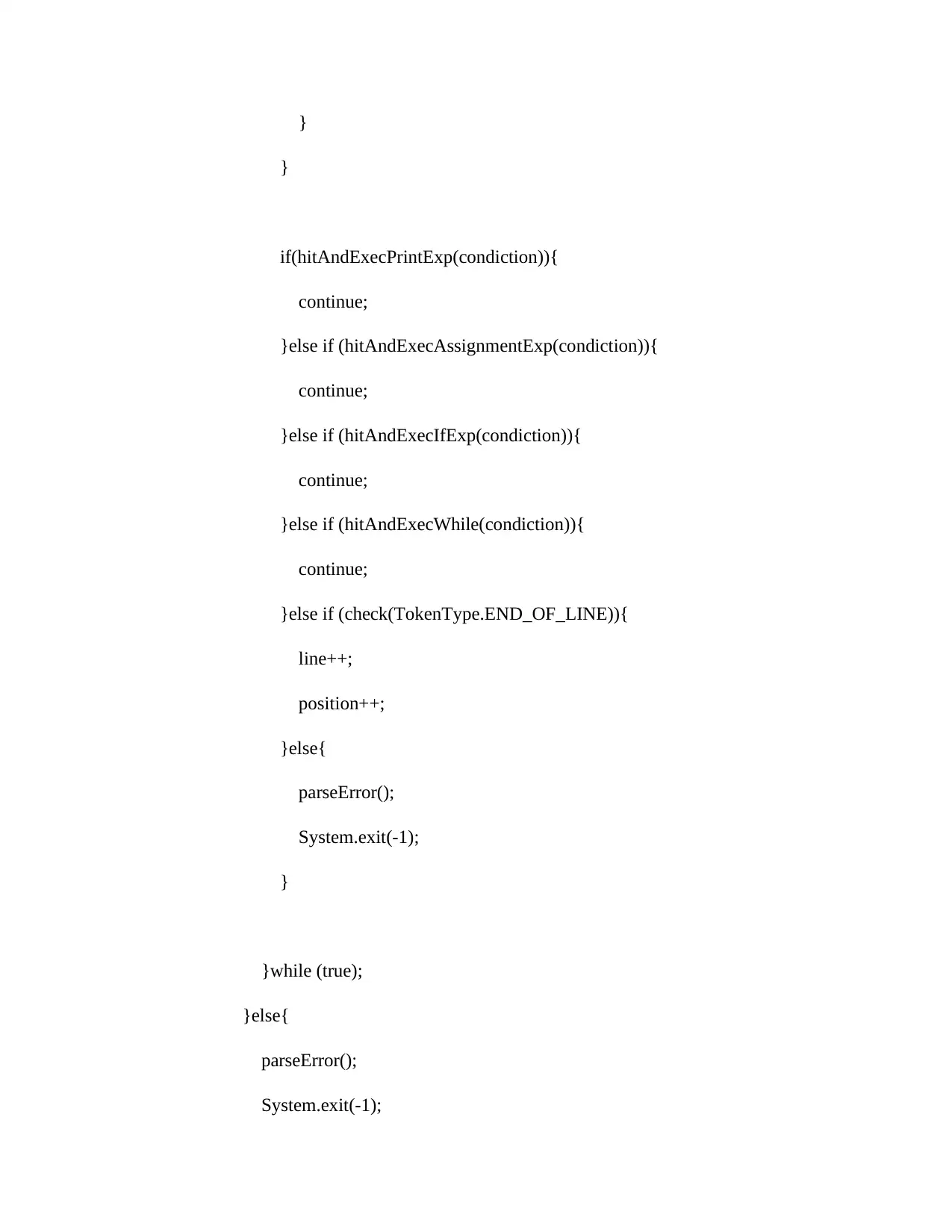
}
}
if(hitAndExecPrintExp(condiction)){
continue;
}else if (hitAndExecAssignmentExp(condiction)){
continue;
}else if (hitAndExecIfExp(condiction)){
continue;
}else if (hitAndExecWhile(condiction)){
continue;
}else if (check(TokenType.END_OF_LINE)){
line++;
position++;
}else{
parseError();
System.exit(-1);
}
}while (true);
}else{
parseError();
System.exit(-1);
}
if(hitAndExecPrintExp(condiction)){
continue;
}else if (hitAndExecAssignmentExp(condiction)){
continue;
}else if (hitAndExecIfExp(condiction)){
continue;
}else if (hitAndExecWhile(condiction)){
continue;
}else if (check(TokenType.END_OF_LINE)){
line++;
position++;
}else{
parseError();
System.exit(-1);
}
}while (true);
}else{
parseError();
System.exit(-1);
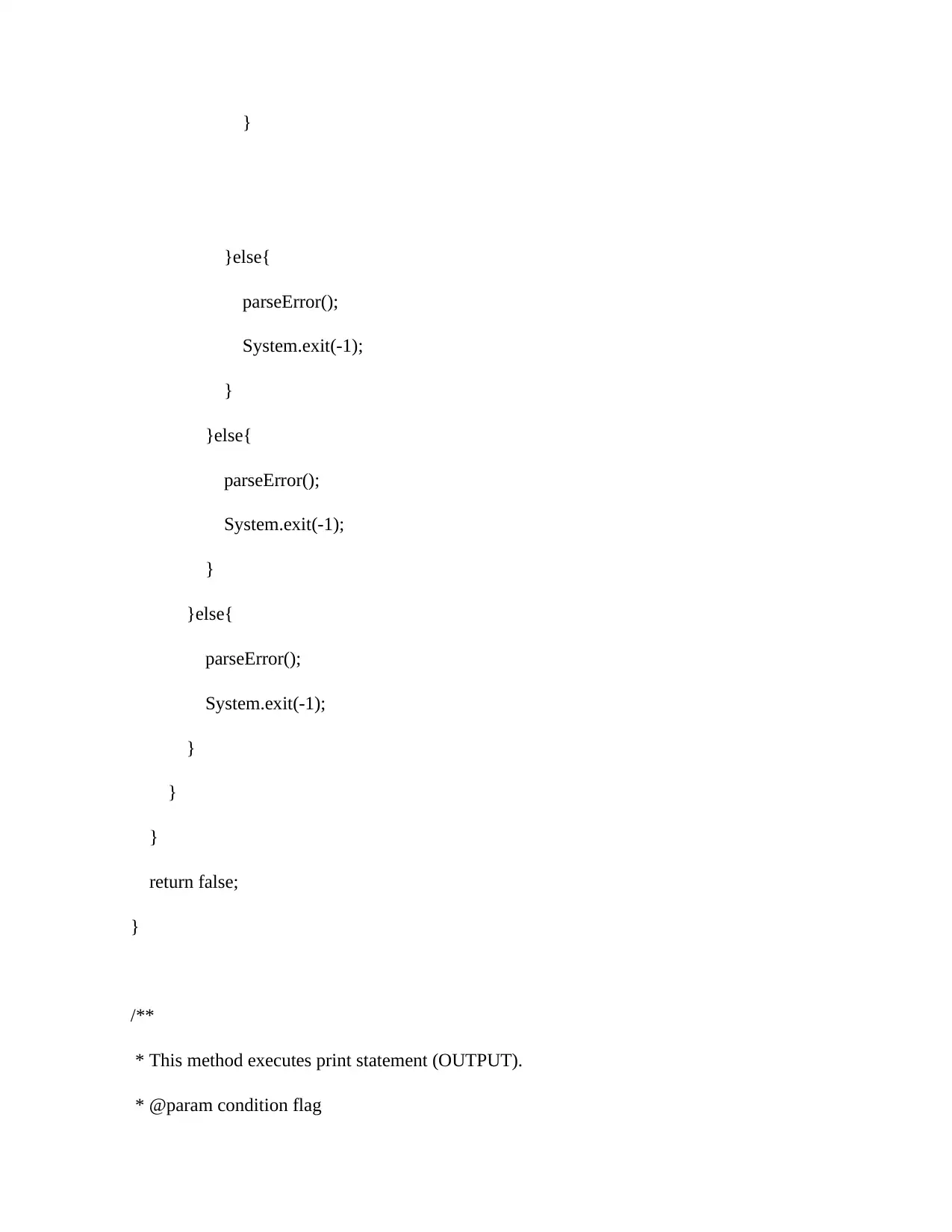
}
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}
}
return false;
}
/**
* This method executes print statement (OUTPUT).
* @param condition flag
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}
}
return false;
}
/**
* This method executes print statement (OUTPUT).
* @param condition flag
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
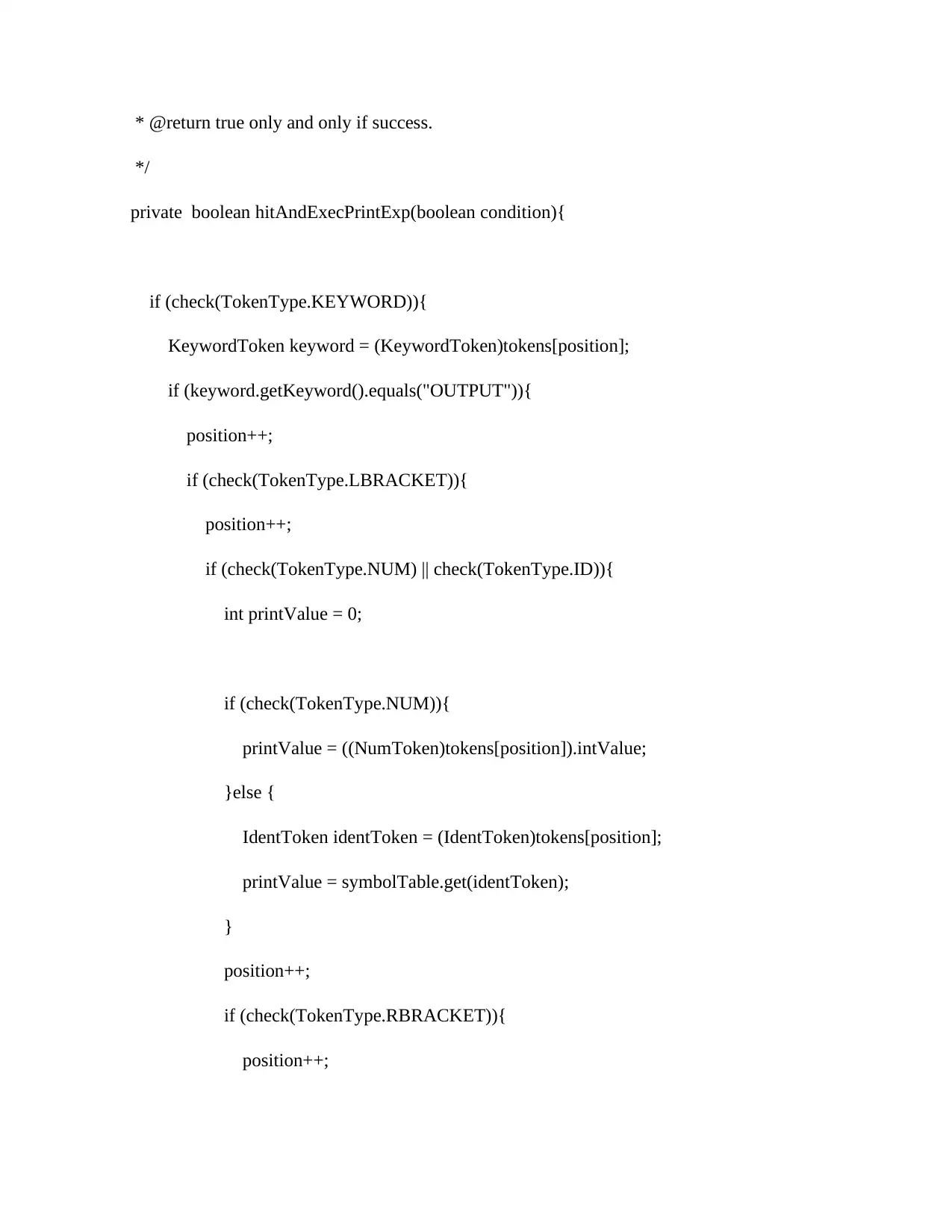
* @return true only and only if success.
*/
private boolean hitAndExecPrintExp(boolean condition){
if (check(TokenType.KEYWORD)){
KeywordToken keyword = (KeywordToken)tokens[position];
if (keyword.getKeyword().equals("OUTPUT")){
position++;
if (check(TokenType.LBRACKET)){
position++;
if (check(TokenType.NUM) || check(TokenType.ID)){
int printValue = 0;
if (check(TokenType.NUM)){
printValue = ((NumToken)tokens[position]).intValue;
}else {
IdentToken identToken = (IdentToken)tokens[position];
printValue = symbolTable.get(identToken);
}
position++;
if (check(TokenType.RBRACKET)){
position++;
*/
private boolean hitAndExecPrintExp(boolean condition){
if (check(TokenType.KEYWORD)){
KeywordToken keyword = (KeywordToken)tokens[position];
if (keyword.getKeyword().equals("OUTPUT")){
position++;
if (check(TokenType.LBRACKET)){
position++;
if (check(TokenType.NUM) || check(TokenType.ID)){
int printValue = 0;
if (check(TokenType.NUM)){
printValue = ((NumToken)tokens[position]).intValue;
}else {
IdentToken identToken = (IdentToken)tokens[position];
printValue = symbolTable.get(identToken);
}
position++;
if (check(TokenType.RBRACKET)){
position++;
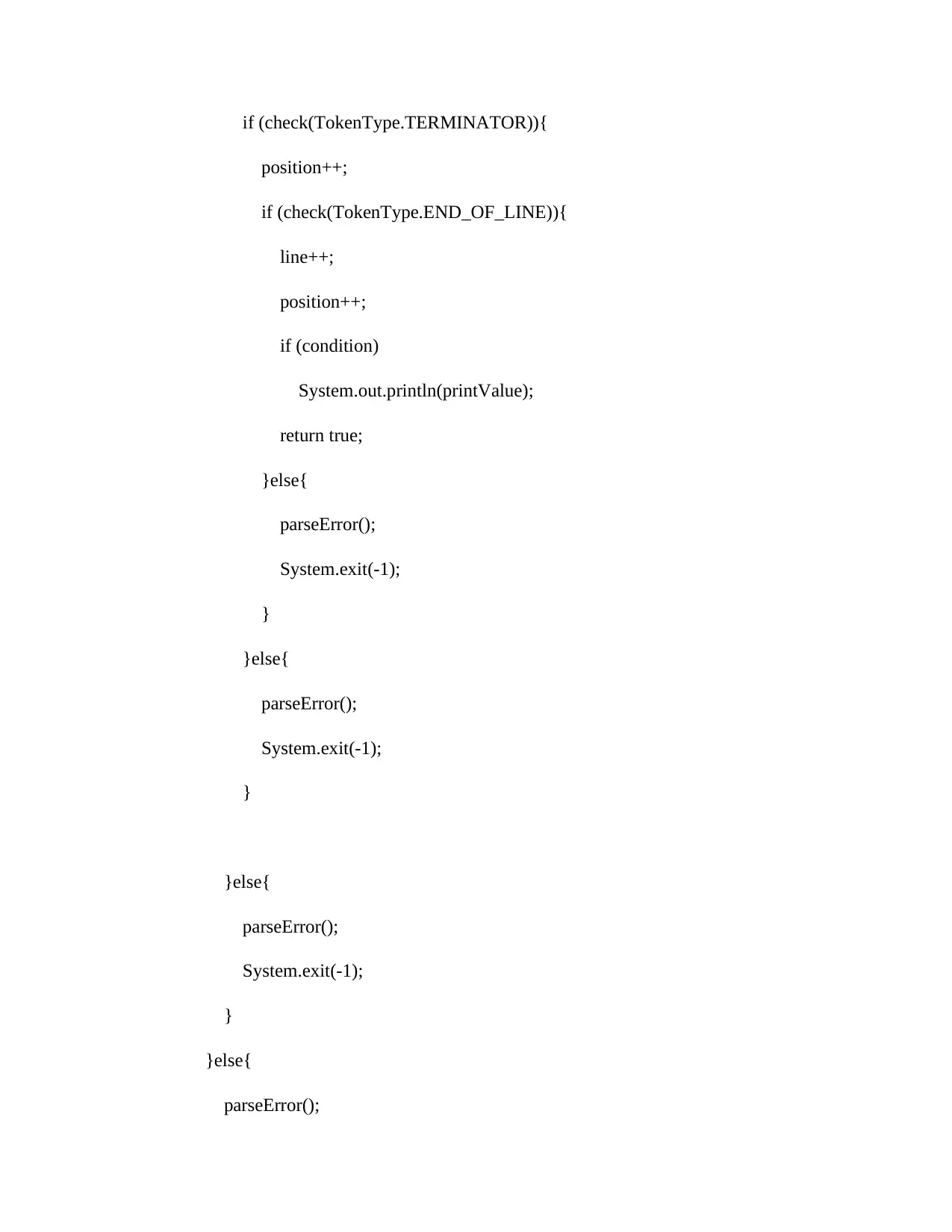
if (check(TokenType.TERMINATOR)){
position++;
if (check(TokenType.END_OF_LINE)){
line++;
position++;
if (condition)
System.out.println(printValue);
return true;
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
position++;
if (check(TokenType.END_OF_LINE)){
line++;
position++;
if (condition)
System.out.println(printValue);
return true;
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
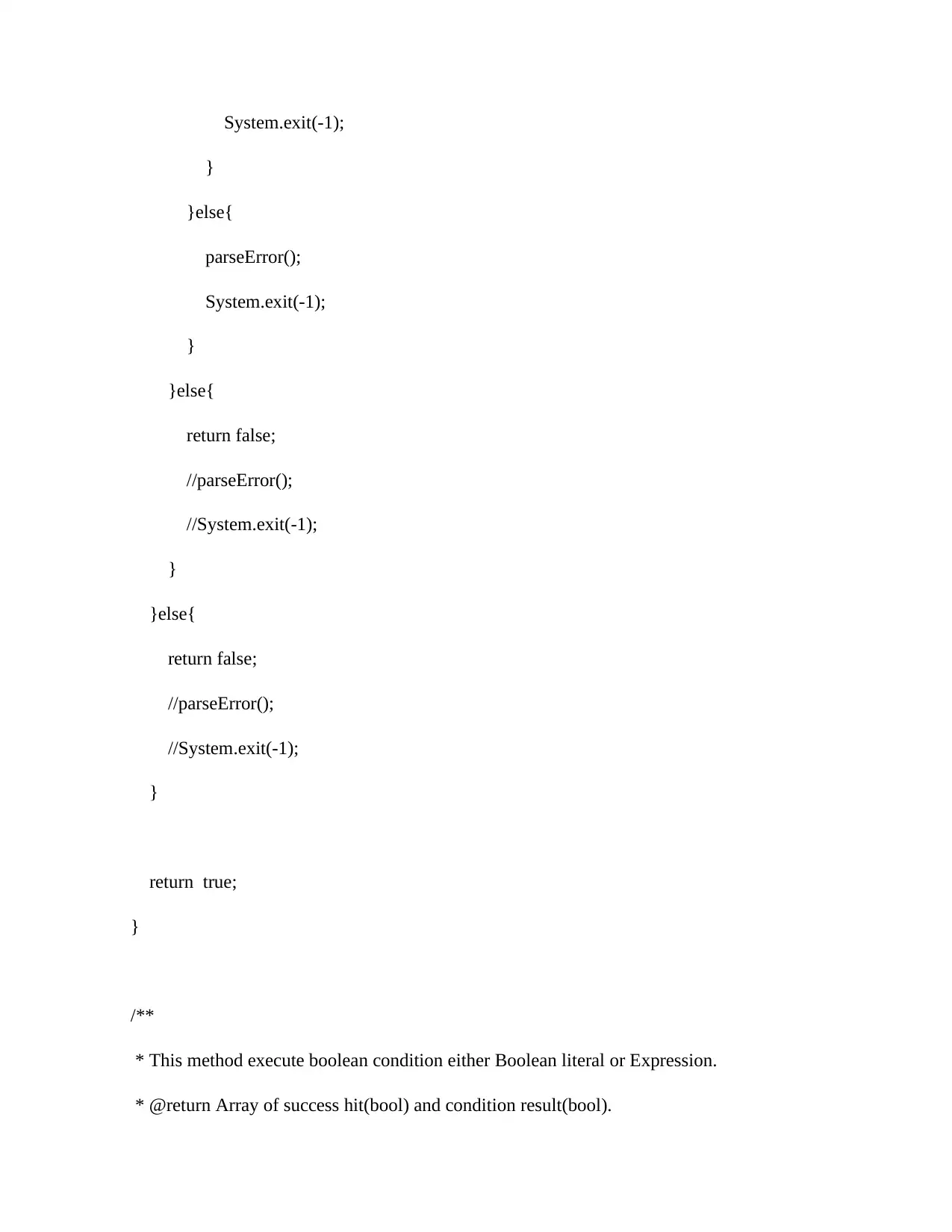
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
return false;
//parseError();
//System.exit(-1);
}
}else{
return false;
//parseError();
//System.exit(-1);
}
return true;
}
/**
* This method execute boolean condition either Boolean literal or Expression.
* @return Array of success hit(bool) and condition result(bool).
}
}else{
parseError();
System.exit(-1);
}
}else{
return false;
//parseError();
//System.exit(-1);
}
}else{
return false;
//parseError();
//System.exit(-1);
}
return true;
}
/**
* This method execute boolean condition either Boolean literal or Expression.
* @return Array of success hit(bool) and condition result(bool).
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
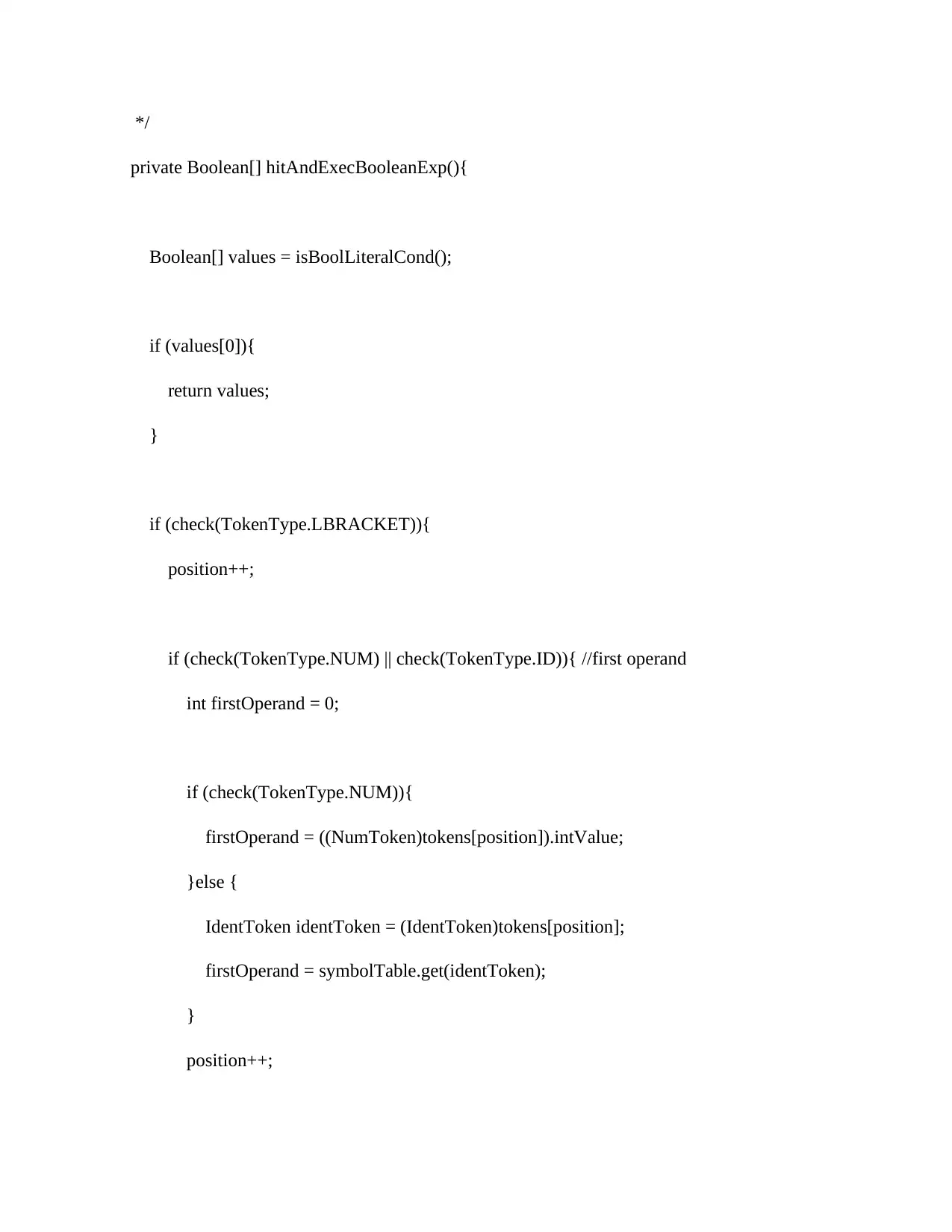
*/
private Boolean[] hitAndExecBooleanExp(){
Boolean[] values = isBoolLiteralCond();
if (values[0]){
return values;
}
if (check(TokenType.LBRACKET)){
position++;
if (check(TokenType.NUM) || check(TokenType.ID)){ //first operand
int firstOperand = 0;
if (check(TokenType.NUM)){
firstOperand = ((NumToken)tokens[position]).intValue;
}else {
IdentToken identToken = (IdentToken)tokens[position];
firstOperand = symbolTable.get(identToken);
}
position++;
private Boolean[] hitAndExecBooleanExp(){
Boolean[] values = isBoolLiteralCond();
if (values[0]){
return values;
}
if (check(TokenType.LBRACKET)){
position++;
if (check(TokenType.NUM) || check(TokenType.ID)){ //first operand
int firstOperand = 0;
if (check(TokenType.NUM)){
firstOperand = ((NumToken)tokens[position]).intValue;
}else {
IdentToken identToken = (IdentToken)tokens[position];
firstOperand = symbolTable.get(identToken);
}
position++;
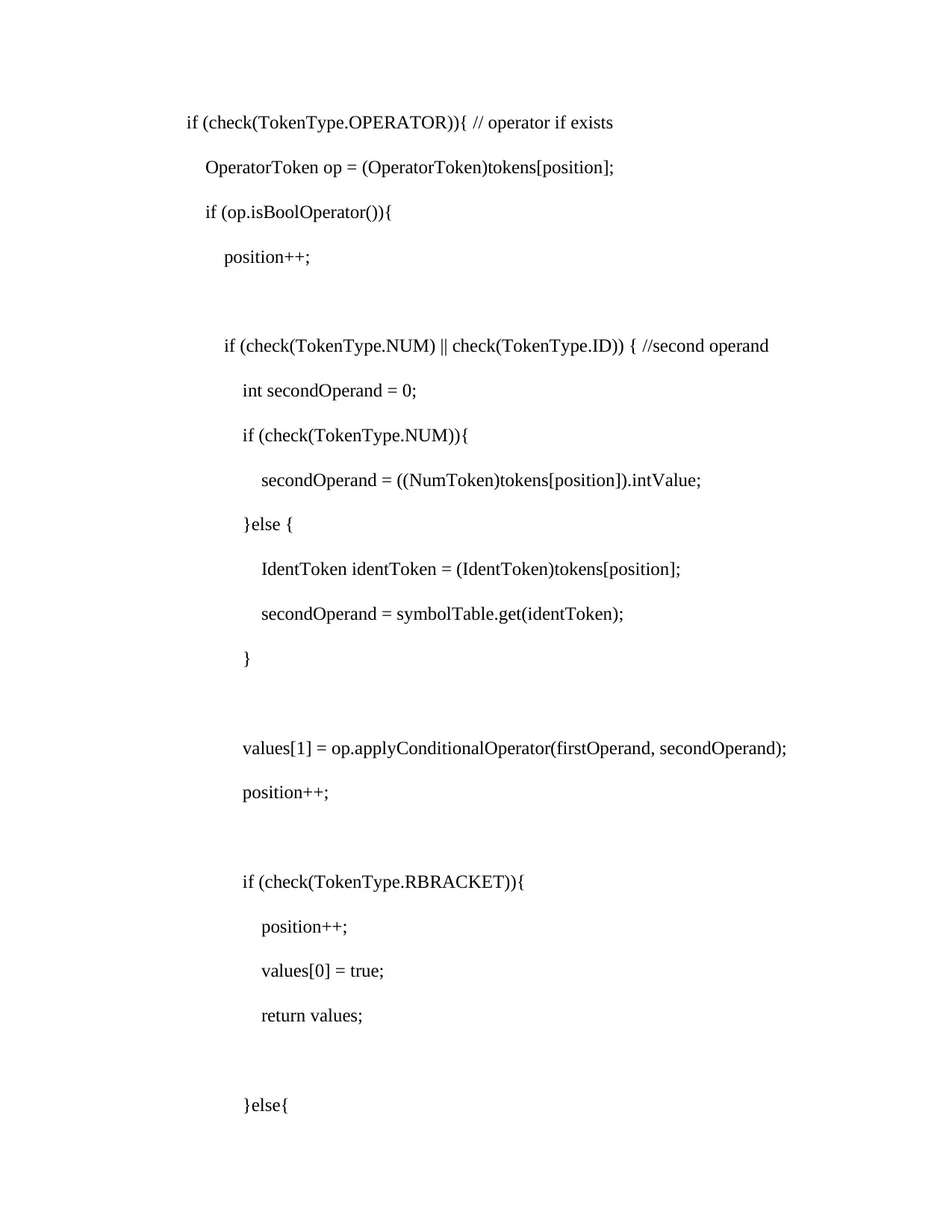
if (check(TokenType.OPERATOR)){ // operator if exists
OperatorToken op = (OperatorToken)tokens[position];
if (op.isBoolOperator()){
position++;
if (check(TokenType.NUM) || check(TokenType.ID)) { //second operand
int secondOperand = 0;
if (check(TokenType.NUM)){
secondOperand = ((NumToken)tokens[position]).intValue;
}else {
IdentToken identToken = (IdentToken)tokens[position];
secondOperand = symbolTable.get(identToken);
}
values[1] = op.applyConditionalOperator(firstOperand, secondOperand);
position++;
if (check(TokenType.RBRACKET)){
position++;
values[0] = true;
return values;
}else{
OperatorToken op = (OperatorToken)tokens[position];
if (op.isBoolOperator()){
position++;
if (check(TokenType.NUM) || check(TokenType.ID)) { //second operand
int secondOperand = 0;
if (check(TokenType.NUM)){
secondOperand = ((NumToken)tokens[position]).intValue;
}else {
IdentToken identToken = (IdentToken)tokens[position];
secondOperand = symbolTable.get(identToken);
}
values[1] = op.applyConditionalOperator(firstOperand, secondOperand);
position++;
if (check(TokenType.RBRACKET)){
position++;
values[0] = true;
return values;
}else{
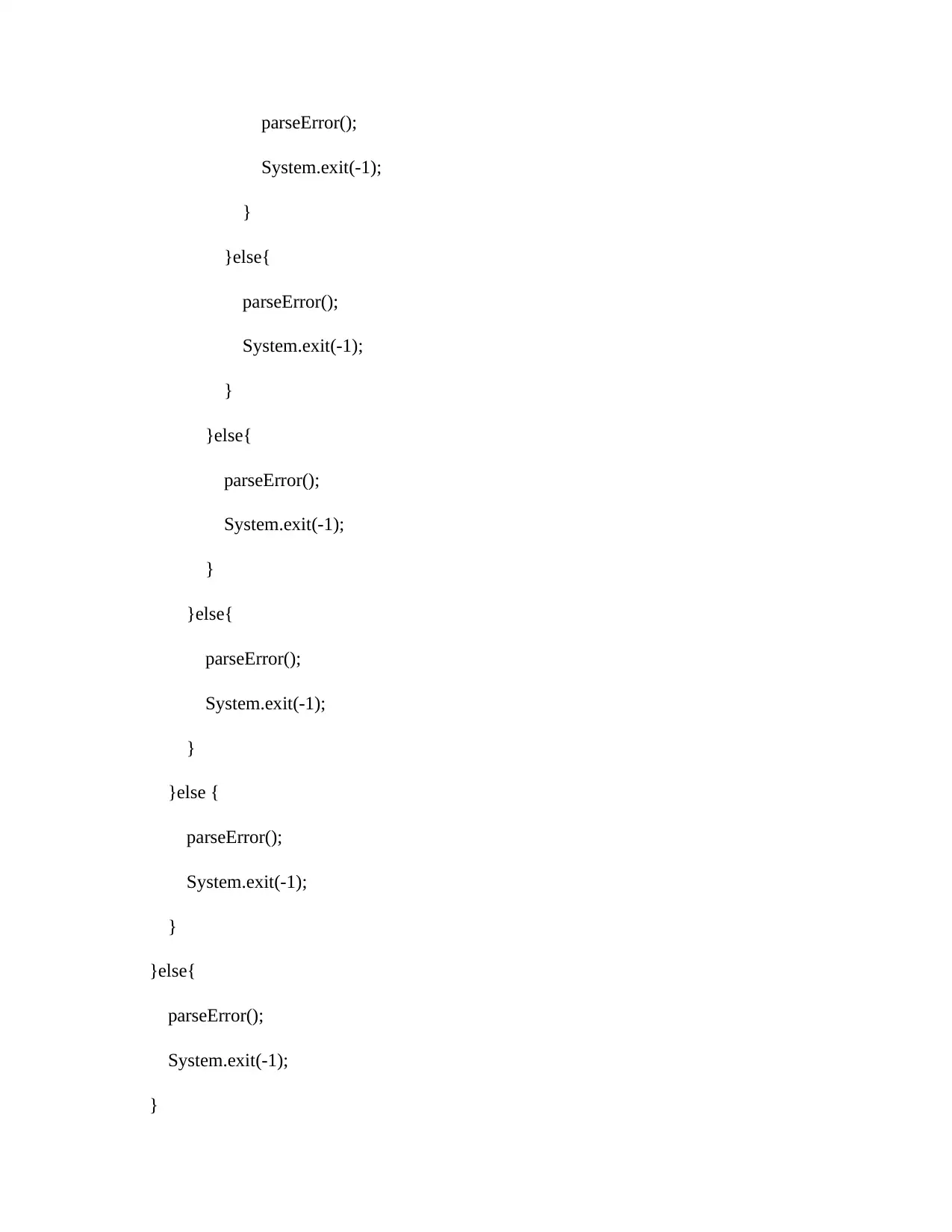
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else {
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else {
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
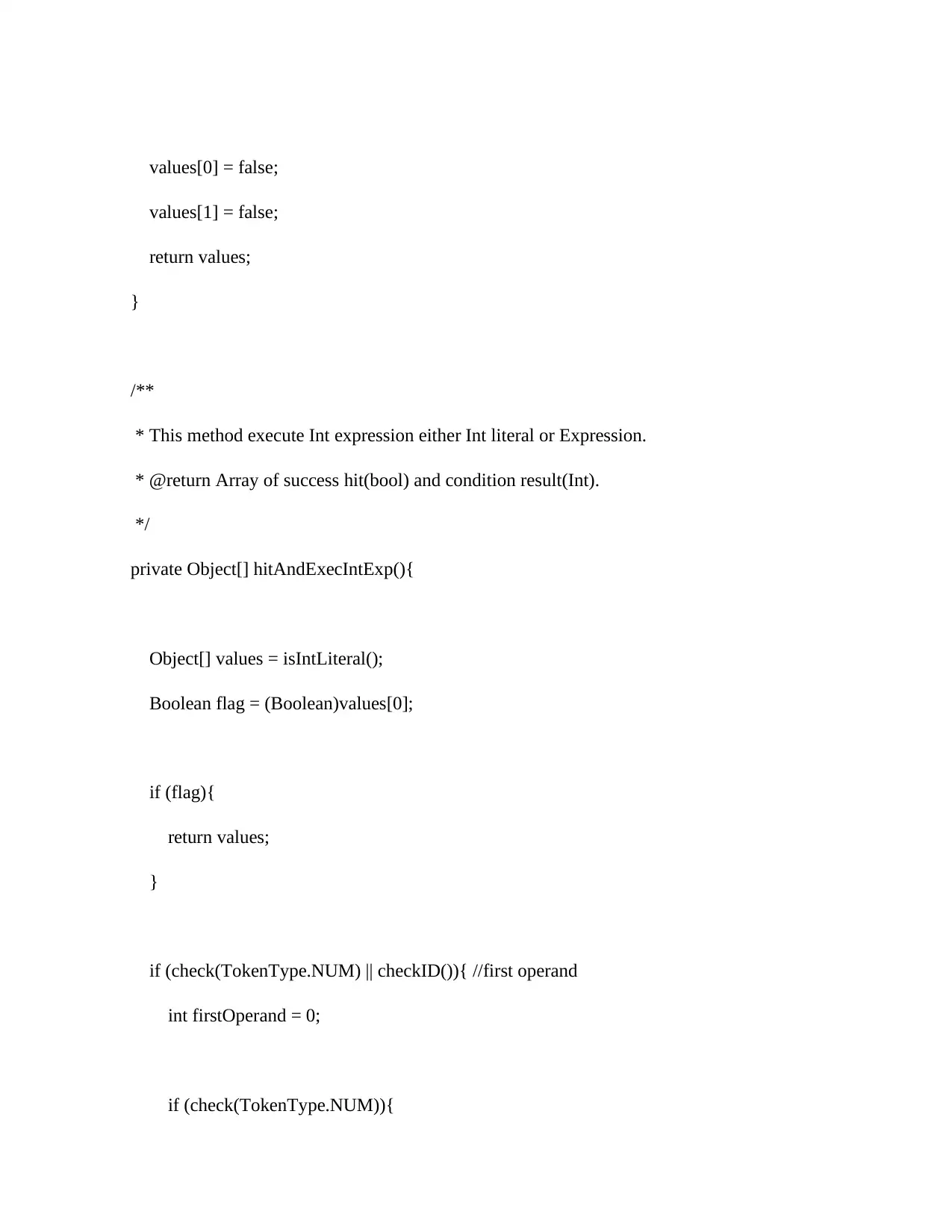
values[0] = false;
values[1] = false;
return values;
}
/**
* This method execute Int expression either Int literal or Expression.
* @return Array of success hit(bool) and condition result(Int).
*/
private Object[] hitAndExecIntExp(){
Object[] values = isIntLiteral();
Boolean flag = (Boolean)values[0];
if (flag){
return values;
}
if (check(TokenType.NUM) || checkID()){ //first operand
int firstOperand = 0;
if (check(TokenType.NUM)){
values[1] = false;
return values;
}
/**
* This method execute Int expression either Int literal or Expression.
* @return Array of success hit(bool) and condition result(Int).
*/
private Object[] hitAndExecIntExp(){
Object[] values = isIntLiteral();
Boolean flag = (Boolean)values[0];
if (flag){
return values;
}
if (check(TokenType.NUM) || checkID()){ //first operand
int firstOperand = 0;
if (check(TokenType.NUM)){
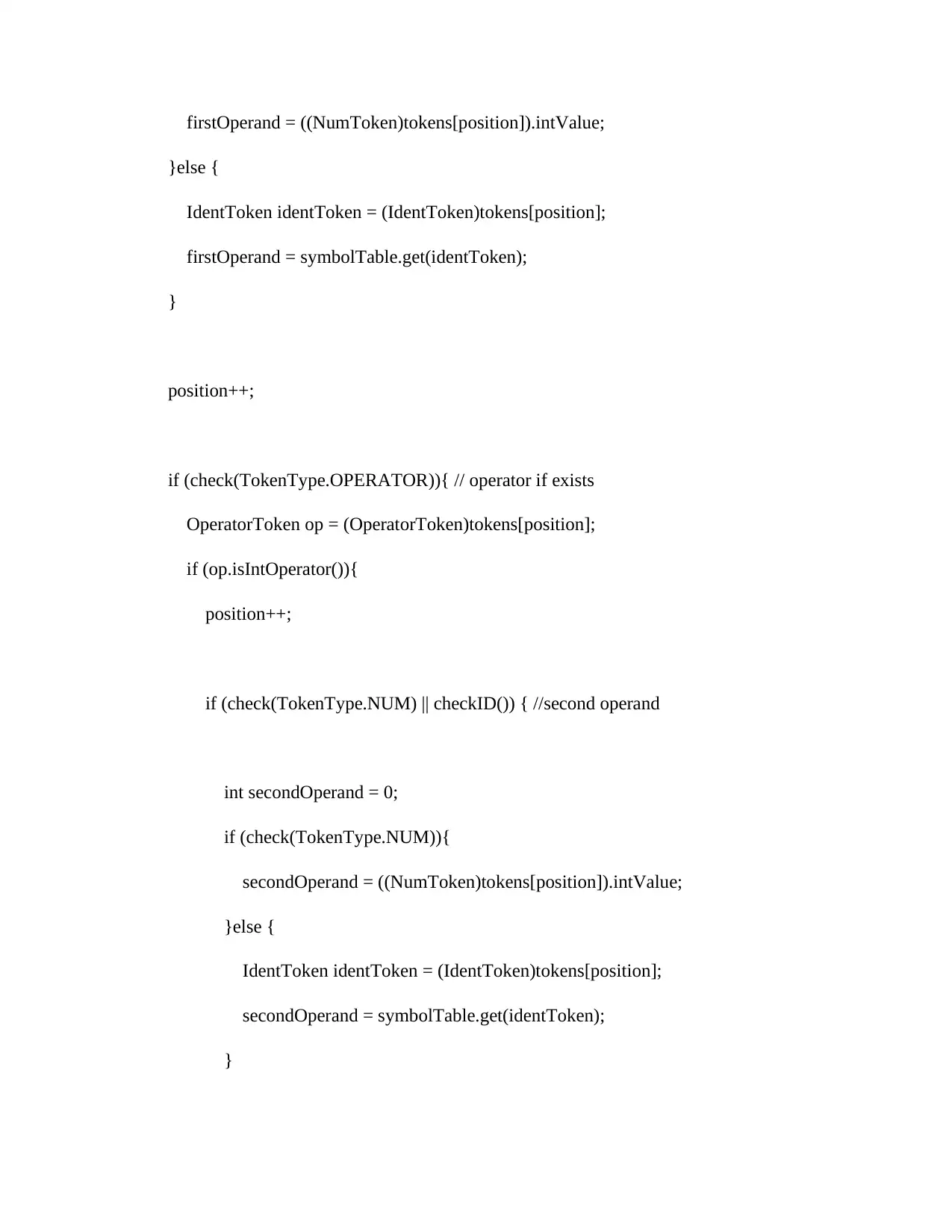
firstOperand = ((NumToken)tokens[position]).intValue;
}else {
IdentToken identToken = (IdentToken)tokens[position];
firstOperand = symbolTable.get(identToken);
}
position++;
if (check(TokenType.OPERATOR)){ // operator if exists
OperatorToken op = (OperatorToken)tokens[position];
if (op.isIntOperator()){
position++;
if (check(TokenType.NUM) || checkID()) { //second operand
int secondOperand = 0;
if (check(TokenType.NUM)){
secondOperand = ((NumToken)tokens[position]).intValue;
}else {
IdentToken identToken = (IdentToken)tokens[position];
secondOperand = symbolTable.get(identToken);
}
}else {
IdentToken identToken = (IdentToken)tokens[position];
firstOperand = symbolTable.get(identToken);
}
position++;
if (check(TokenType.OPERATOR)){ // operator if exists
OperatorToken op = (OperatorToken)tokens[position];
if (op.isIntOperator()){
position++;
if (check(TokenType.NUM) || checkID()) { //second operand
int secondOperand = 0;
if (check(TokenType.NUM)){
secondOperand = ((NumToken)tokens[position]).intValue;
}else {
IdentToken identToken = (IdentToken)tokens[position];
secondOperand = symbolTable.get(identToken);
}
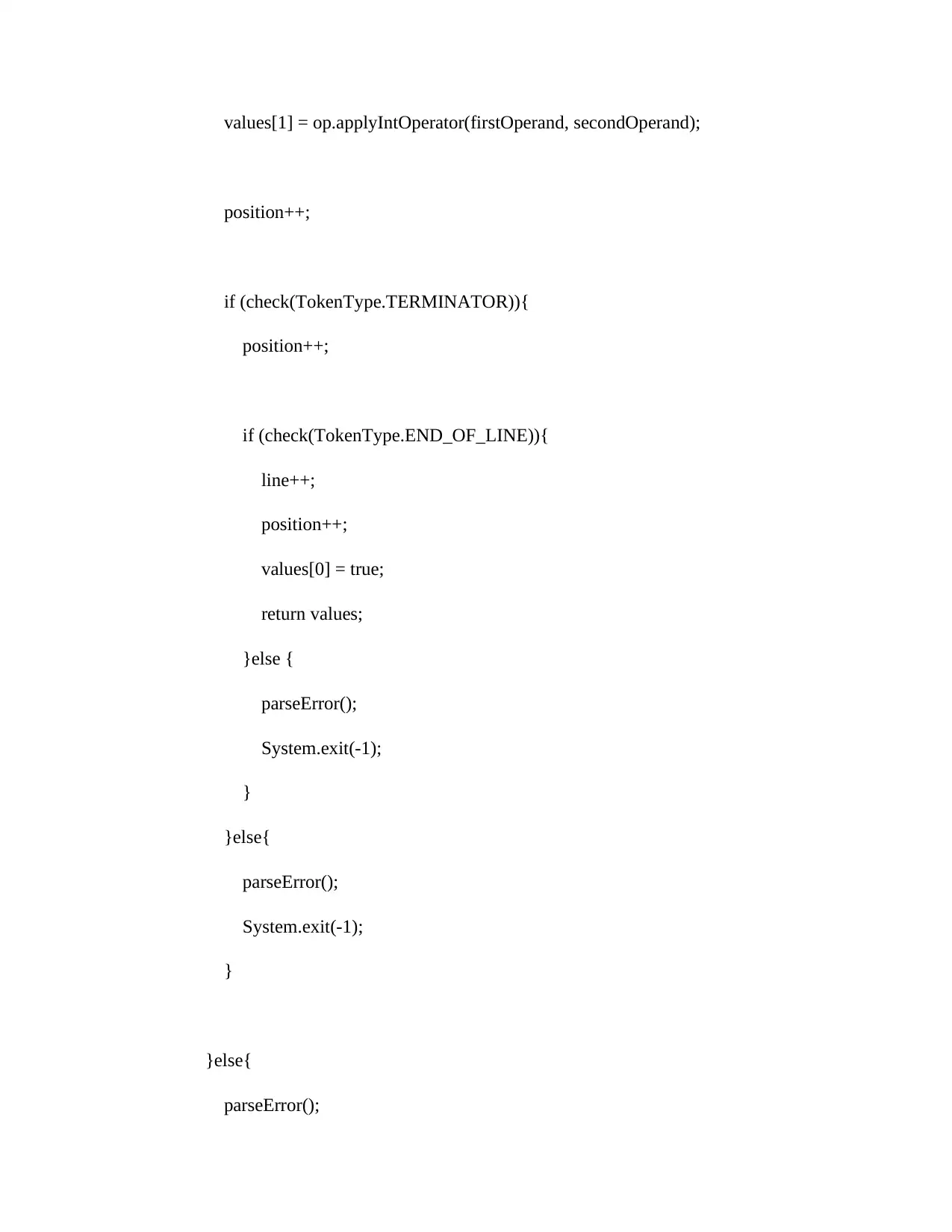
values[1] = op.applyIntOperator(firstOperand, secondOperand);
position++;
if (check(TokenType.TERMINATOR)){
position++;
if (check(TokenType.END_OF_LINE)){
line++;
position++;
values[0] = true;
return values;
}else {
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
position++;
if (check(TokenType.TERMINATOR)){
position++;
if (check(TokenType.END_OF_LINE)){
line++;
position++;
values[0] = true;
return values;
}else {
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
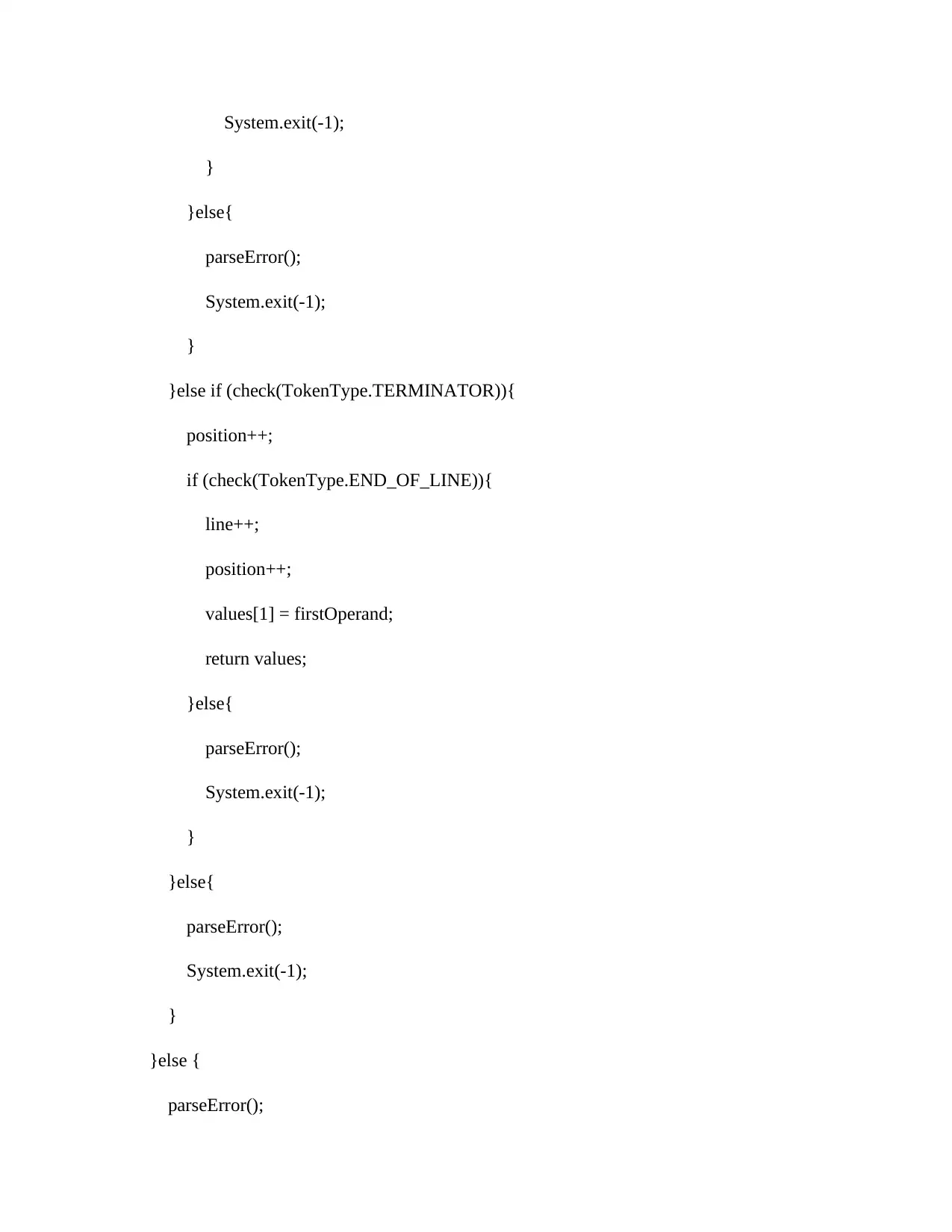
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else if (check(TokenType.TERMINATOR)){
position++;
if (check(TokenType.END_OF_LINE)){
line++;
position++;
values[1] = firstOperand;
return values;
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else {
parseError();
}
}else{
parseError();
System.exit(-1);
}
}else if (check(TokenType.TERMINATOR)){
position++;
if (check(TokenType.END_OF_LINE)){
line++;
position++;
values[1] = firstOperand;
return values;
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else {
parseError();
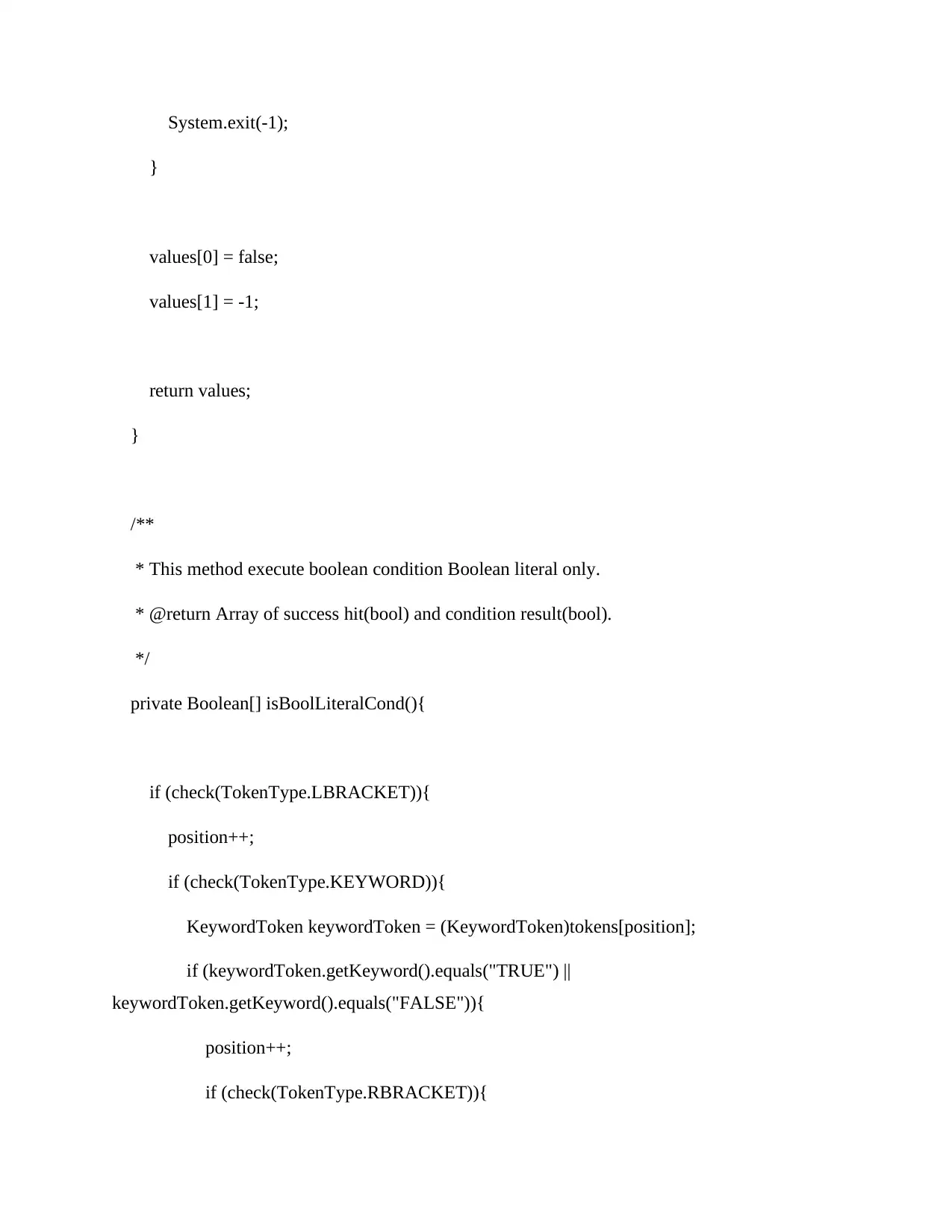
System.exit(-1);
}
values[0] = false;
values[1] = -1;
return values;
}
/**
* This method execute boolean condition Boolean literal only.
* @return Array of success hit(bool) and condition result(bool).
*/
private Boolean[] isBoolLiteralCond(){
if (check(TokenType.LBRACKET)){
position++;
if (check(TokenType.KEYWORD)){
KeywordToken keywordToken = (KeywordToken)tokens[position];
if (keywordToken.getKeyword().equals("TRUE") ||
keywordToken.getKeyword().equals("FALSE")){
position++;
if (check(TokenType.RBRACKET)){
}
values[0] = false;
values[1] = -1;
return values;
}
/**
* This method execute boolean condition Boolean literal only.
* @return Array of success hit(bool) and condition result(bool).
*/
private Boolean[] isBoolLiteralCond(){
if (check(TokenType.LBRACKET)){
position++;
if (check(TokenType.KEYWORD)){
KeywordToken keywordToken = (KeywordToken)tokens[position];
if (keywordToken.getKeyword().equals("TRUE") ||
keywordToken.getKeyword().equals("FALSE")){
position++;
if (check(TokenType.RBRACKET)){
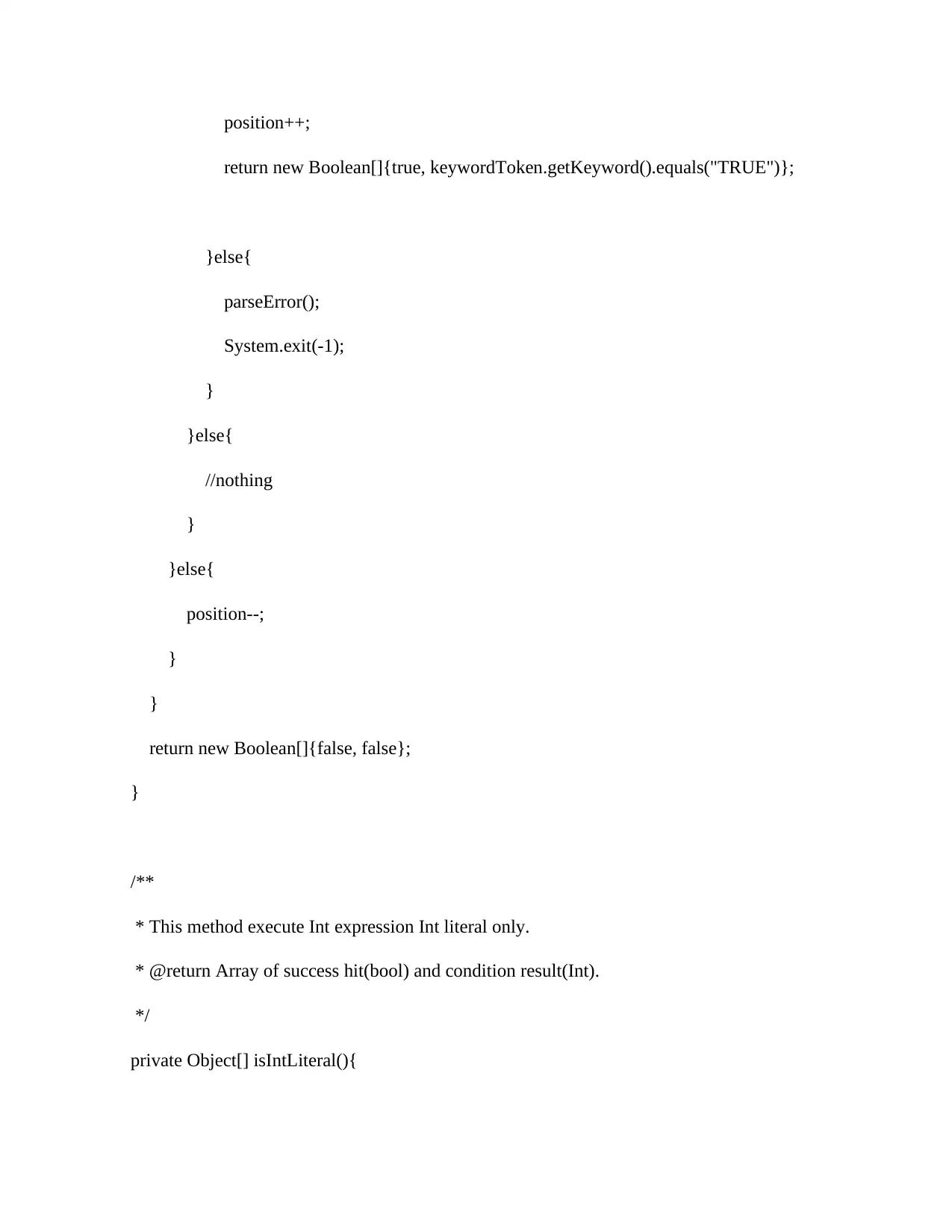
position++;
return new Boolean[]{true, keywordToken.getKeyword().equals("TRUE")};
}else{
parseError();
System.exit(-1);
}
}else{
//nothing
}
}else{
position--;
}
}
return new Boolean[]{false, false};
}
/**
* This method execute Int expression Int literal only.
* @return Array of success hit(bool) and condition result(Int).
*/
private Object[] isIntLiteral(){
return new Boolean[]{true, keywordToken.getKeyword().equals("TRUE")};
}else{
parseError();
System.exit(-1);
}
}else{
//nothing
}
}else{
position--;
}
}
return new Boolean[]{false, false};
}
/**
* This method execute Int expression Int literal only.
* @return Array of success hit(bool) and condition result(Int).
*/
private Object[] isIntLiteral(){
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
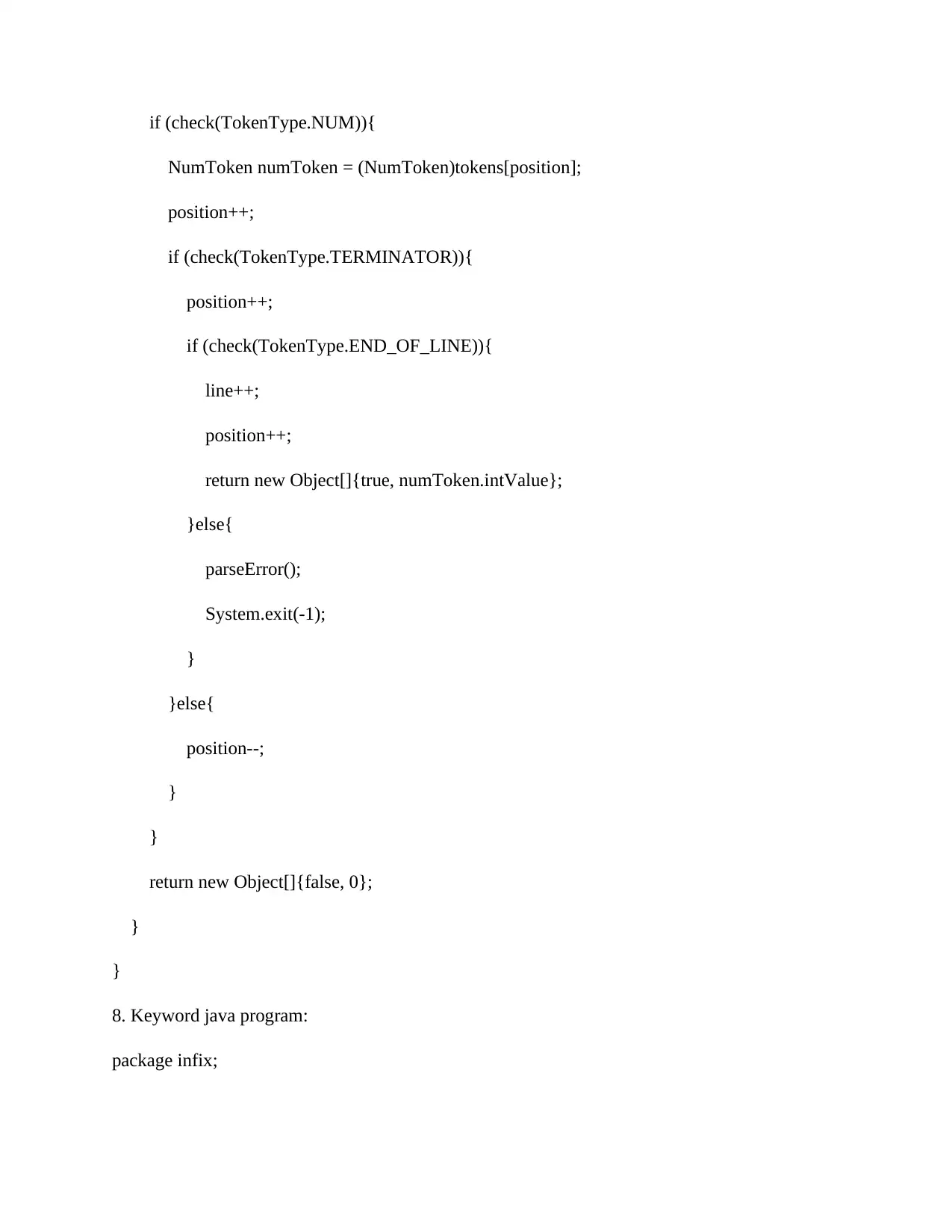
if (check(TokenType.NUM)){
NumToken numToken = (NumToken)tokens[position];
position++;
if (check(TokenType.TERMINATOR)){
position++;
if (check(TokenType.END_OF_LINE)){
line++;
position++;
return new Object[]{true, numToken.intValue};
}else{
parseError();
System.exit(-1);
}
}else{
position--;
}
}
return new Object[]{false, 0};
}
}
8. Keyword java program:
package infix;
NumToken numToken = (NumToken)tokens[position];
position++;
if (check(TokenType.TERMINATOR)){
position++;
if (check(TokenType.END_OF_LINE)){
line++;
position++;
return new Object[]{true, numToken.intValue};
}else{
parseError();
System.exit(-1);
}
}else{
position--;
}
}
return new Object[]{false, 0};
}
}
8. Keyword java program:
package infix;
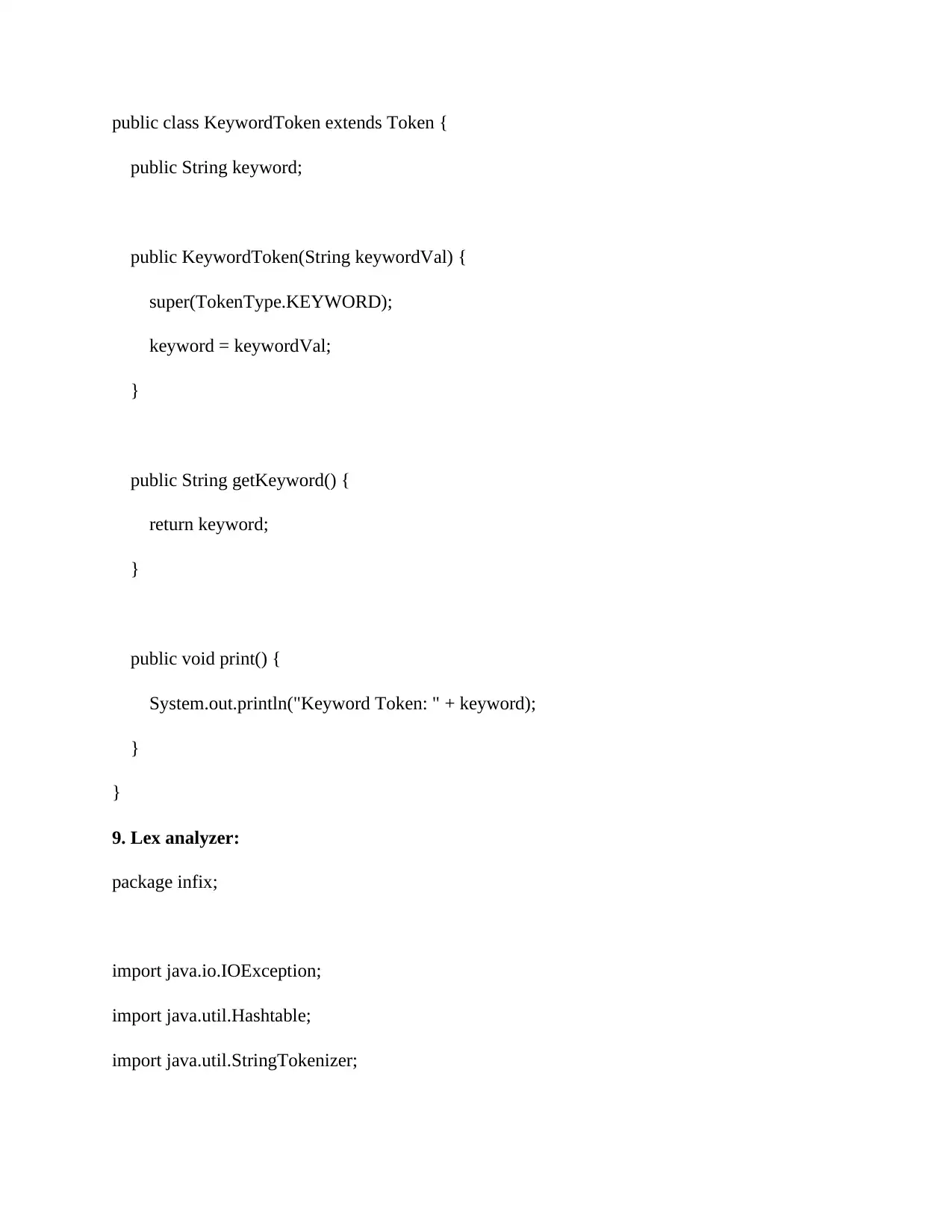
public class KeywordToken extends Token {
public String keyword;
public KeywordToken(String keywordVal) {
super(TokenType.KEYWORD);
keyword = keywordVal;
}
public String getKeyword() {
return keyword;
}
public void print() {
System.out.println("Keyword Token: " + keyword);
}
}
9. Lex analyzer:
package infix;
import java.io.IOException;
import java.util.Hashtable;
import java.util.StringTokenizer;
public String keyword;
public KeywordToken(String keywordVal) {
super(TokenType.KEYWORD);
keyword = keywordVal;
}
public String getKeyword() {
return keyword;
}
public void print() {
System.out.println("Keyword Token: " + keyword);
}
}
9. Lex analyzer:
package infix;
import java.io.IOException;
import java.util.Hashtable;
import java.util.StringTokenizer;
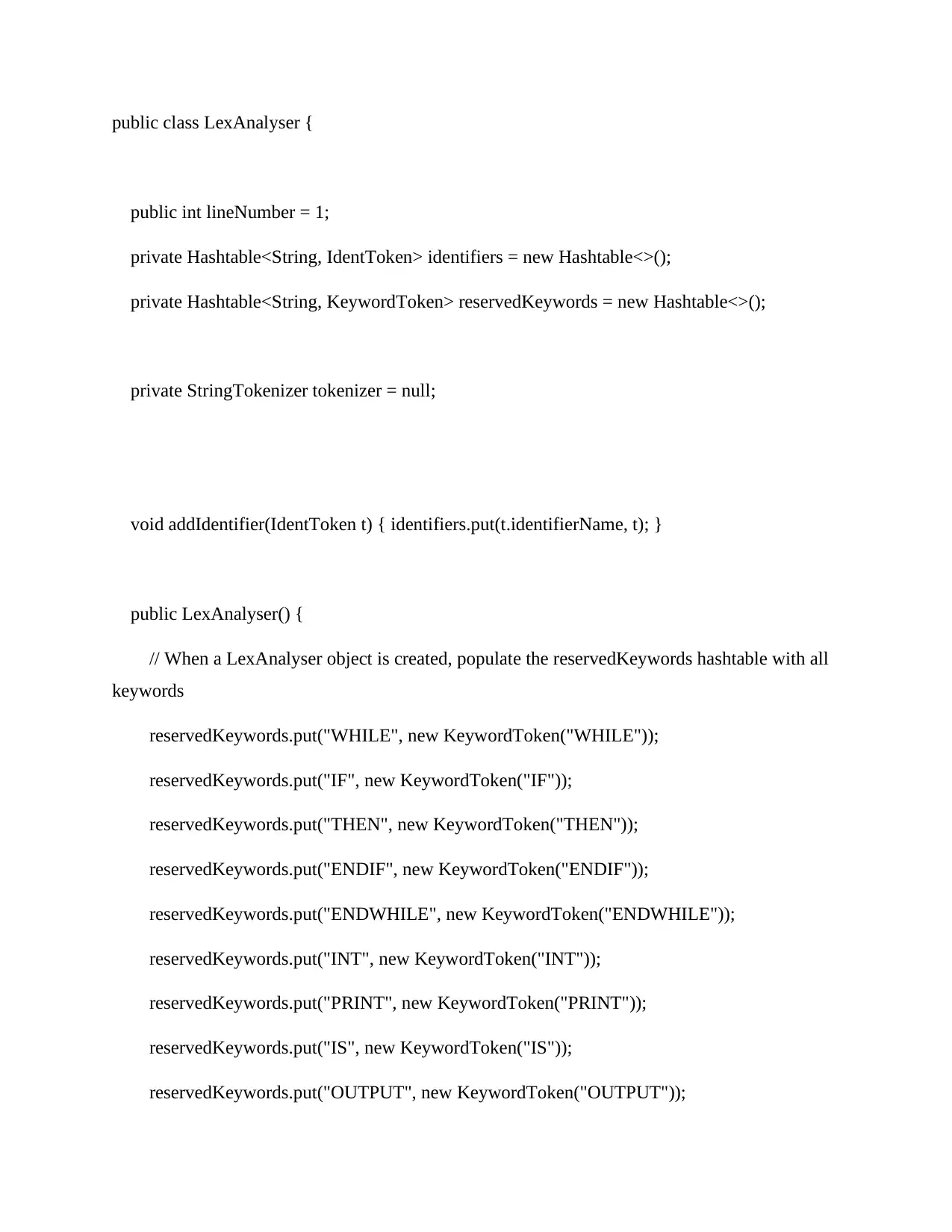
public class LexAnalyser {
public int lineNumber = 1;
private Hashtable<String, IdentToken> identifiers = new Hashtable<>();
private Hashtable<String, KeywordToken> reservedKeywords = new Hashtable<>();
private StringTokenizer tokenizer = null;
void addIdentifier(IdentToken t) { identifiers.put(t.identifierName, t); }
public LexAnalyser() {
// When a LexAnalyser object is created, populate the reservedKeywords hashtable with all
keywords
reservedKeywords.put("WHILE", new KeywordToken("WHILE"));
reservedKeywords.put("IF", new KeywordToken("IF"));
reservedKeywords.put("THEN", new KeywordToken("THEN"));
reservedKeywords.put("ENDIF", new KeywordToken("ENDIF"));
reservedKeywords.put("ENDWHILE", new KeywordToken("ENDWHILE"));
reservedKeywords.put("INT", new KeywordToken("INT"));
reservedKeywords.put("PRINT", new KeywordToken("PRINT"));
reservedKeywords.put("IS", new KeywordToken("IS"));
reservedKeywords.put("OUTPUT", new KeywordToken("OUTPUT"));
public int lineNumber = 1;
private Hashtable<String, IdentToken> identifiers = new Hashtable<>();
private Hashtable<String, KeywordToken> reservedKeywords = new Hashtable<>();
private StringTokenizer tokenizer = null;
void addIdentifier(IdentToken t) { identifiers.put(t.identifierName, t); }
public LexAnalyser() {
// When a LexAnalyser object is created, populate the reservedKeywords hashtable with all
keywords
reservedKeywords.put("WHILE", new KeywordToken("WHILE"));
reservedKeywords.put("IF", new KeywordToken("IF"));
reservedKeywords.put("THEN", new KeywordToken("THEN"));
reservedKeywords.put("ENDIF", new KeywordToken("ENDIF"));
reservedKeywords.put("ENDWHILE", new KeywordToken("ENDWHILE"));
reservedKeywords.put("INT", new KeywordToken("INT"));
reservedKeywords.put("PRINT", new KeywordToken("PRINT"));
reservedKeywords.put("IS", new KeywordToken("IS"));
reservedKeywords.put("OUTPUT", new KeywordToken("OUTPUT"));
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
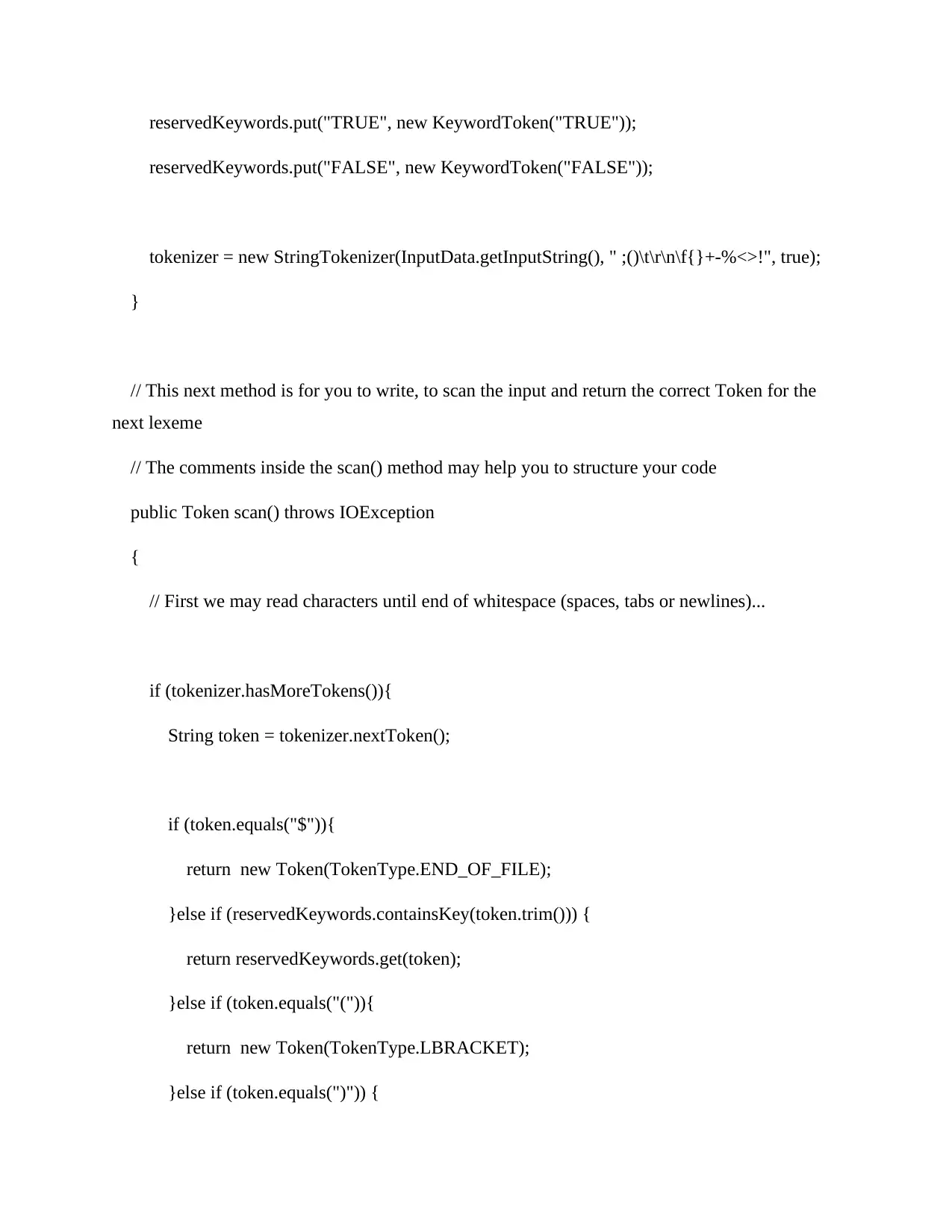
reservedKeywords.put("TRUE", new KeywordToken("TRUE"));
reservedKeywords.put("FALSE", new KeywordToken("FALSE"));
tokenizer = new StringTokenizer(InputData.getInputString(), " ;()\t\r\n\f{}+-%<>!", true);
}
// This next method is for you to write, to scan the input and return the correct Token for the
next lexeme
// The comments inside the scan() method may help you to structure your code
public Token scan() throws IOException
{
// First we may read characters until end of whitespace (spaces, tabs or newlines)...
if (tokenizer.hasMoreTokens()){
String token = tokenizer.nextToken();
if (token.equals("$")){
return new Token(TokenType.END_OF_FILE);
}else if (reservedKeywords.containsKey(token.trim())) {
return reservedKeywords.get(token);
}else if (token.equals("(")){
return new Token(TokenType.LBRACKET);
}else if (token.equals(")")) {
reservedKeywords.put("FALSE", new KeywordToken("FALSE"));
tokenizer = new StringTokenizer(InputData.getInputString(), " ;()\t\r\n\f{}+-%<>!", true);
}
// This next method is for you to write, to scan the input and return the correct Token for the
next lexeme
// The comments inside the scan() method may help you to structure your code
public Token scan() throws IOException
{
// First we may read characters until end of whitespace (spaces, tabs or newlines)...
if (tokenizer.hasMoreTokens()){
String token = tokenizer.nextToken();
if (token.equals("$")){
return new Token(TokenType.END_OF_FILE);
}else if (reservedKeywords.containsKey(token.trim())) {
return reservedKeywords.get(token);
}else if (token.equals("(")){
return new Token(TokenType.LBRACKET);
}else if (token.equals(")")) {
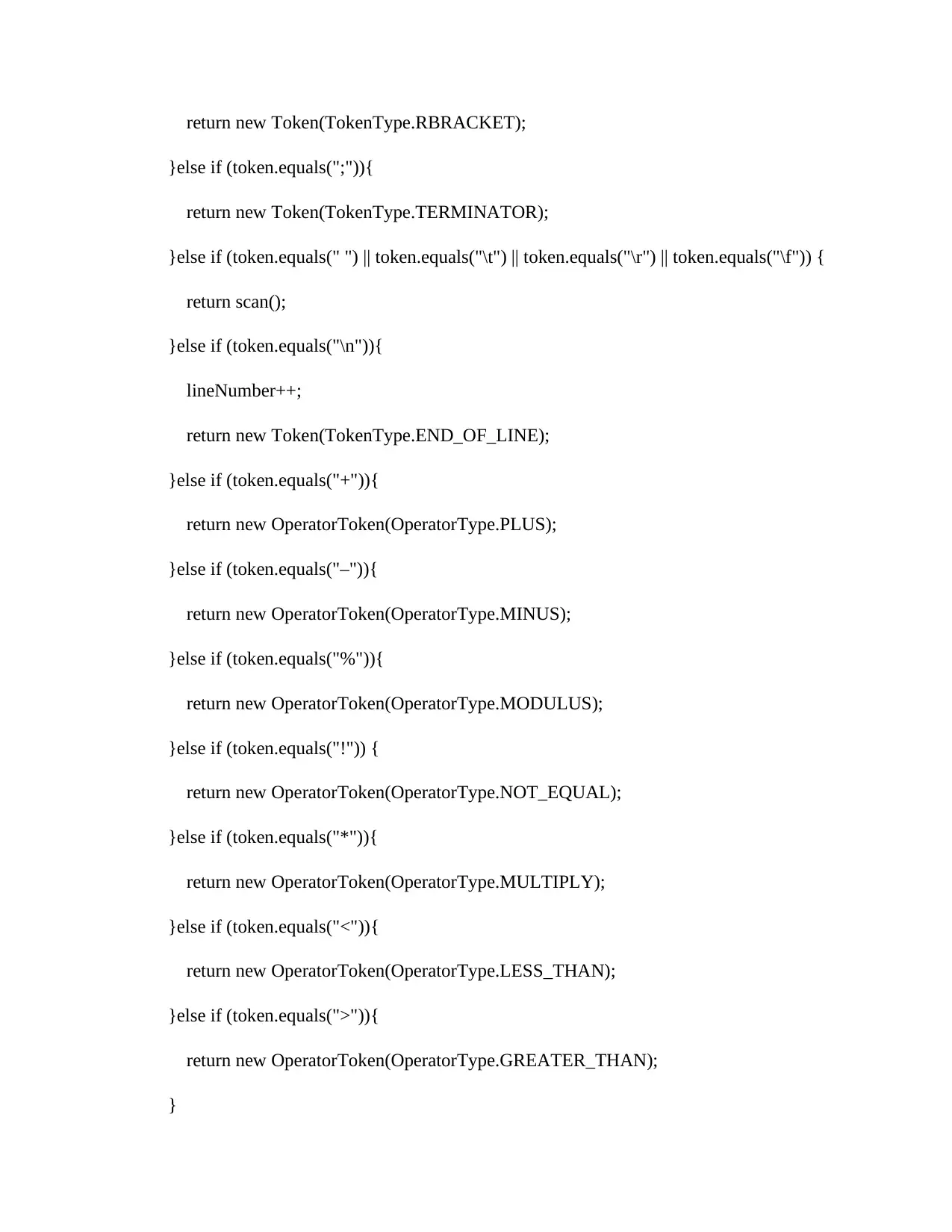
return new Token(TokenType.RBRACKET);
}else if (token.equals(";")){
return new Token(TokenType.TERMINATOR);
}else if (token.equals(" ") || token.equals("\t") || token.equals("\r") || token.equals("\f")) {
return scan();
}else if (token.equals("\n")){
lineNumber++;
return new Token(TokenType.END_OF_LINE);
}else if (token.equals("+")){
return new OperatorToken(OperatorType.PLUS);
}else if (token.equals("–")){
return new OperatorToken(OperatorType.MINUS);
}else if (token.equals("%")){
return new OperatorToken(OperatorType.MODULUS);
}else if (token.equals("!")) {
return new OperatorToken(OperatorType.NOT_EQUAL);
}else if (token.equals("*")){
return new OperatorToken(OperatorType.MULTIPLY);
}else if (token.equals("<")){
return new OperatorToken(OperatorType.LESS_THAN);
}else if (token.equals(">")){
return new OperatorToken(OperatorType.GREATER_THAN);
}
}else if (token.equals(";")){
return new Token(TokenType.TERMINATOR);
}else if (token.equals(" ") || token.equals("\t") || token.equals("\r") || token.equals("\f")) {
return scan();
}else if (token.equals("\n")){
lineNumber++;
return new Token(TokenType.END_OF_LINE);
}else if (token.equals("+")){
return new OperatorToken(OperatorType.PLUS);
}else if (token.equals("–")){
return new OperatorToken(OperatorType.MINUS);
}else if (token.equals("%")){
return new OperatorToken(OperatorType.MODULUS);
}else if (token.equals("!")) {
return new OperatorToken(OperatorType.NOT_EQUAL);
}else if (token.equals("*")){
return new OperatorToken(OperatorType.MULTIPLY);
}else if (token.equals("<")){
return new OperatorToken(OperatorType.LESS_THAN);
}else if (token.equals(">")){
return new OperatorToken(OperatorType.GREATER_THAN);
}
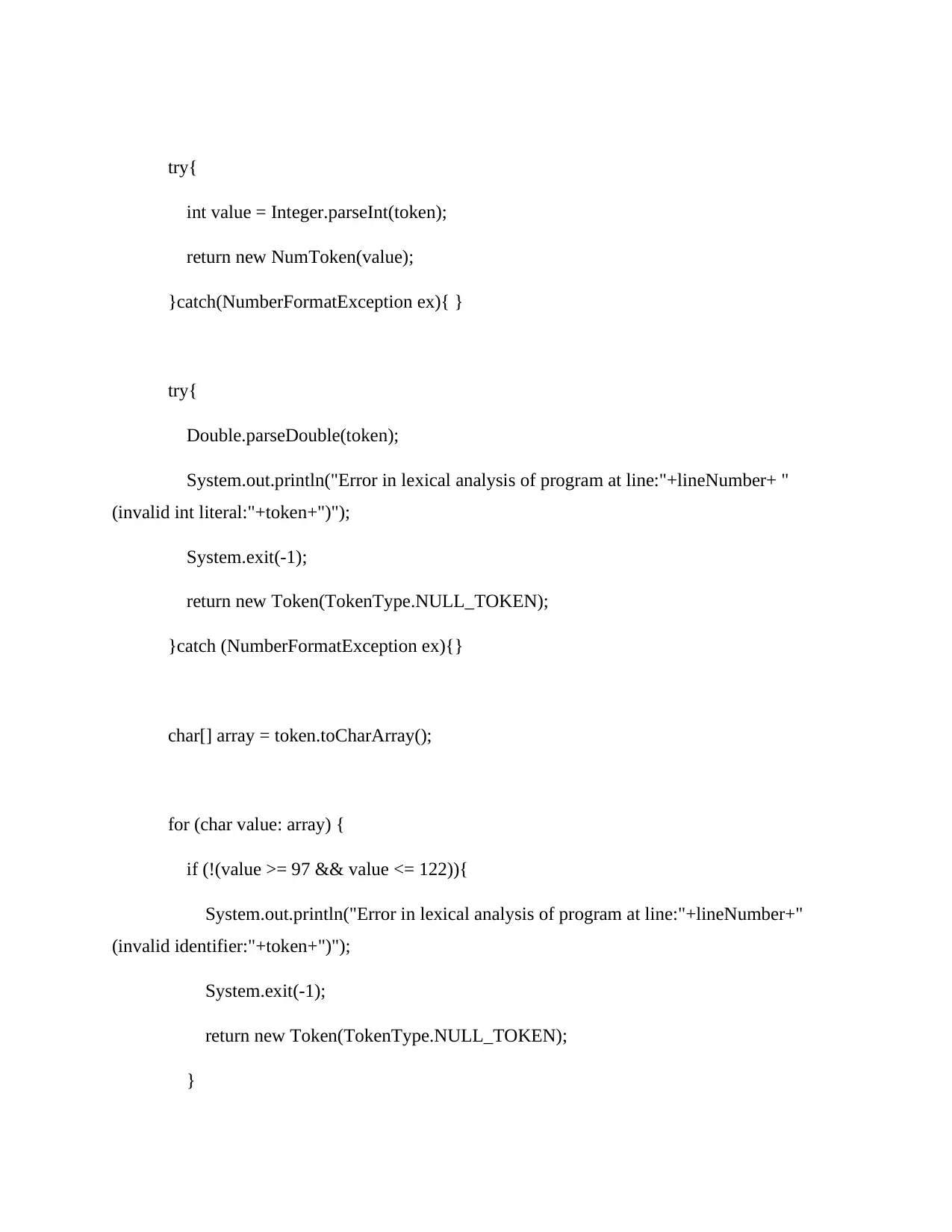
try{
int value = Integer.parseInt(token);
return new NumToken(value);
}catch(NumberFormatException ex){ }
try{
Double.parseDouble(token);
System.out.println("Error in lexical analysis of program at line:"+lineNumber+ "
(invalid int literal:"+token+")");
System.exit(-1);
return new Token(TokenType.NULL_TOKEN);
}catch (NumberFormatException ex){}
char[] array = token.toCharArray();
for (char value: array) {
if (!(value >= 97 && value <= 122)){
System.out.println("Error in lexical analysis of program at line:"+lineNumber+"
(invalid identifier:"+token+")");
System.exit(-1);
return new Token(TokenType.NULL_TOKEN);
}
int value = Integer.parseInt(token);
return new NumToken(value);
}catch(NumberFormatException ex){ }
try{
Double.parseDouble(token);
System.out.println("Error in lexical analysis of program at line:"+lineNumber+ "
(invalid int literal:"+token+")");
System.exit(-1);
return new Token(TokenType.NULL_TOKEN);
}catch (NumberFormatException ex){}
char[] array = token.toCharArray();
for (char value: array) {
if (!(value >= 97 && value <= 122)){
System.out.println("Error in lexical analysis of program at line:"+lineNumber+"
(invalid identifier:"+token+")");
System.exit(-1);
return new Token(TokenType.NULL_TOKEN);
}
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
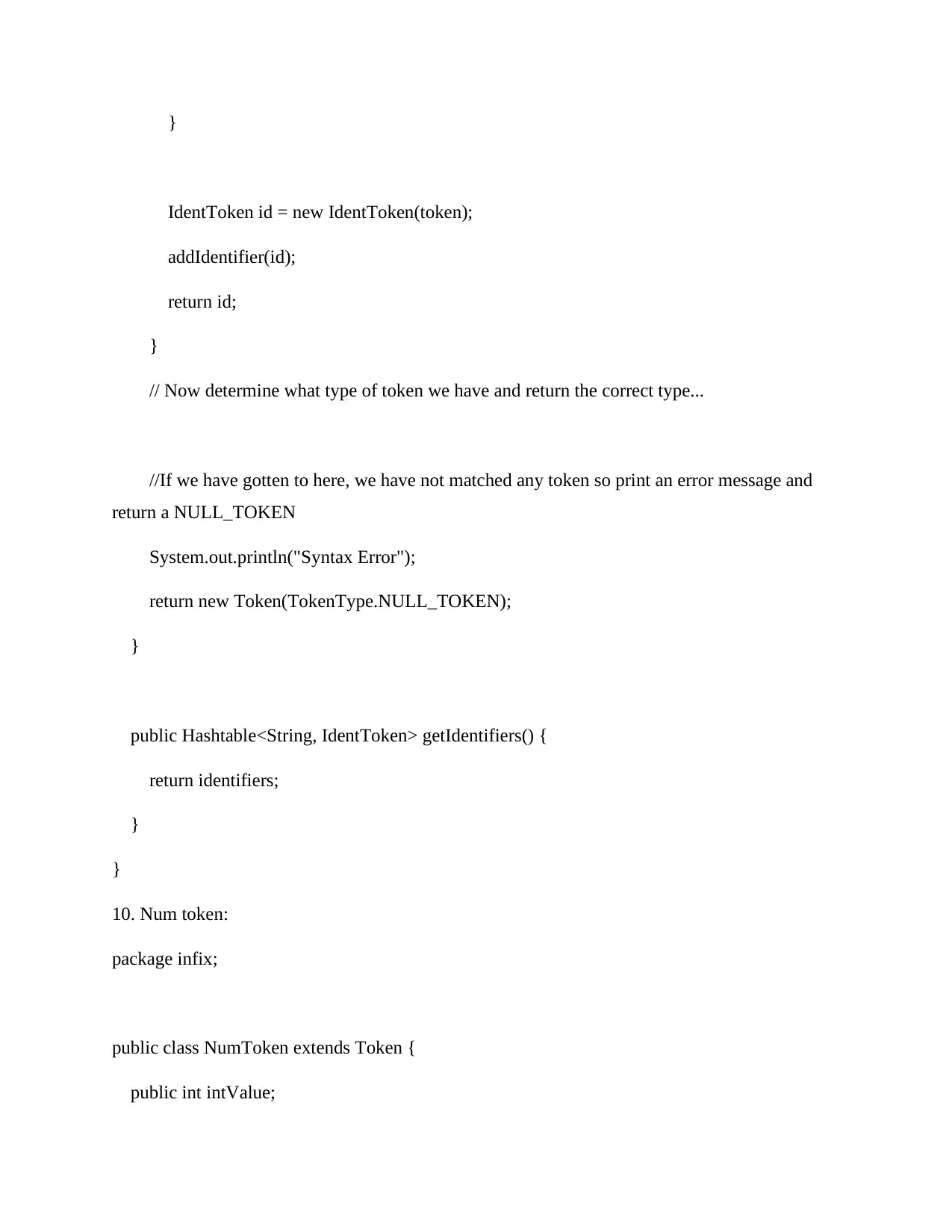
}
IdentToken id = new IdentToken(token);
addIdentifier(id);
return id;
}
// Now determine what type of token we have and return the correct type...
//If we have gotten to here, we have not matched any token so print an error message and
return a NULL_TOKEN
System.out.println("Syntax Error");
return new Token(TokenType.NULL_TOKEN);
}
public Hashtable<String, IdentToken> getIdentifiers() {
return identifiers;
}
}
10. Num token:
package infix;
public class NumToken extends Token {
public int intValue;
IdentToken id = new IdentToken(token);
addIdentifier(id);
return id;
}
// Now determine what type of token we have and return the correct type...
//If we have gotten to here, we have not matched any token so print an error message and
return a NULL_TOKEN
System.out.println("Syntax Error");
return new Token(TokenType.NULL_TOKEN);
}
public Hashtable<String, IdentToken> getIdentifiers() {
return identifiers;
}
}
10. Num token:
package infix;
public class NumToken extends Token {
public int intValue;
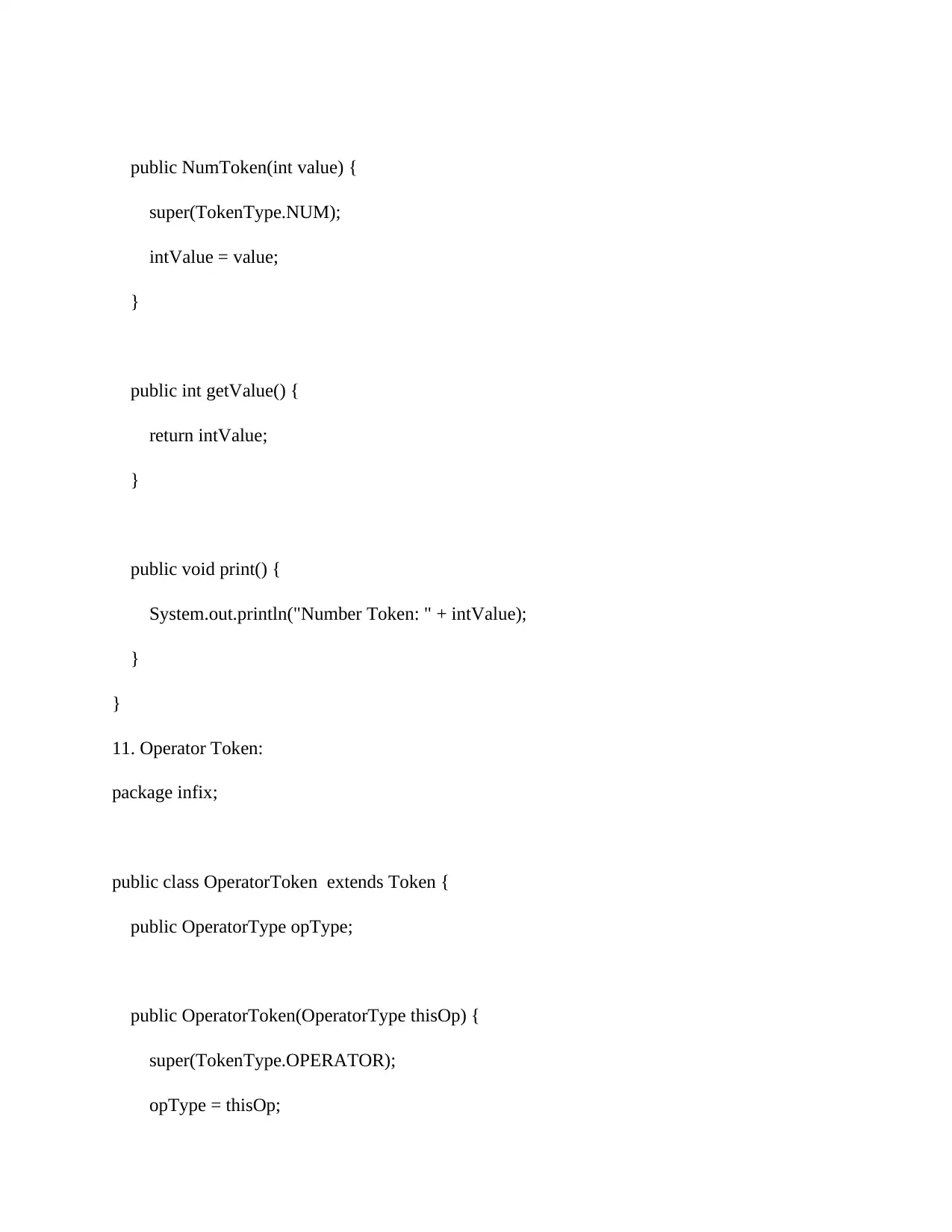
public NumToken(int value) {
super(TokenType.NUM);
intValue = value;
}
public int getValue() {
return intValue;
}
public void print() {
System.out.println("Number Token: " + intValue);
}
}
11. Operator Token:
package infix;
public class OperatorToken extends Token {
public OperatorType opType;
public OperatorToken(OperatorType thisOp) {
super(TokenType.OPERATOR);
opType = thisOp;
super(TokenType.NUM);
intValue = value;
}
public int getValue() {
return intValue;
}
public void print() {
System.out.println("Number Token: " + intValue);
}
}
11. Operator Token:
package infix;
public class OperatorToken extends Token {
public OperatorType opType;
public OperatorToken(OperatorType thisOp) {
super(TokenType.OPERATOR);
opType = thisOp;
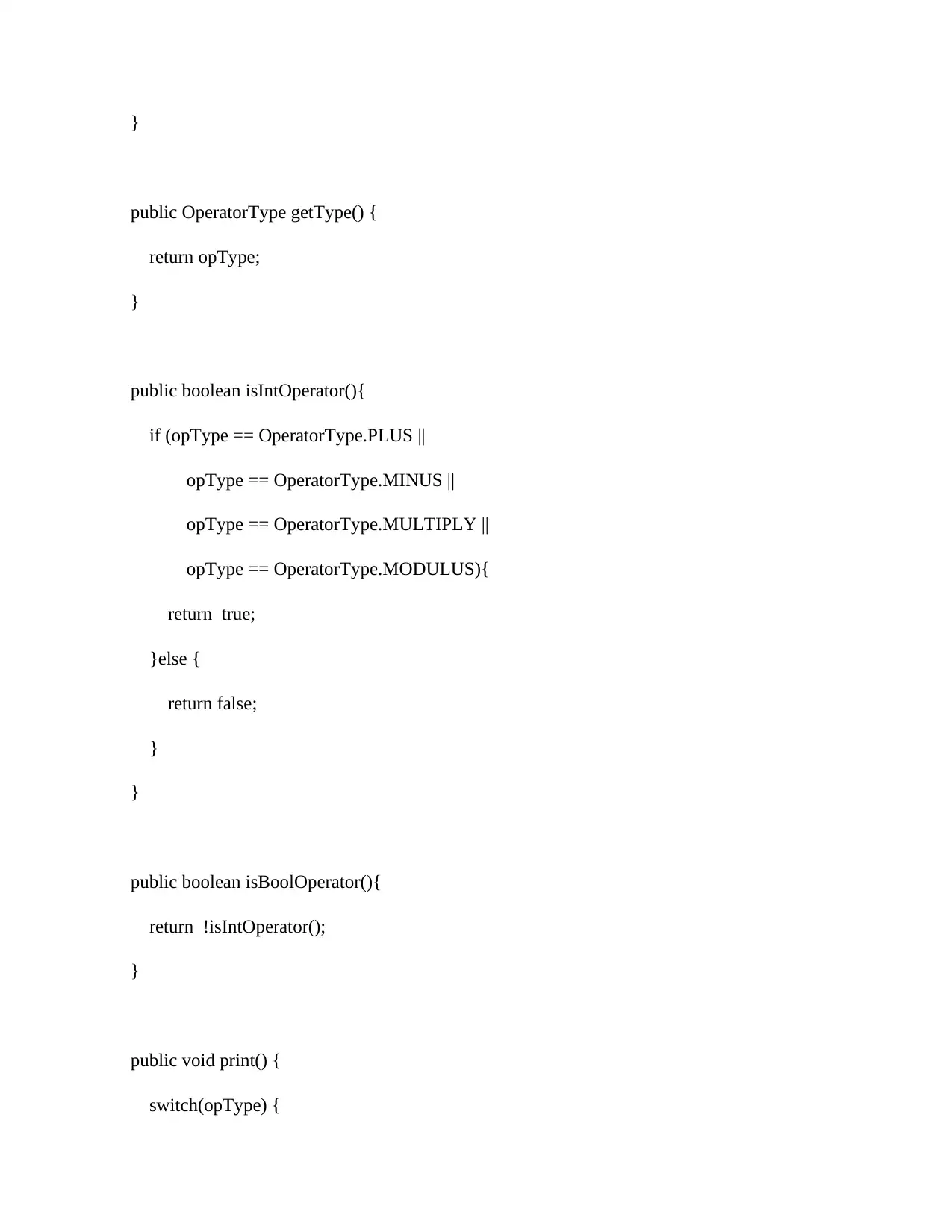
}
public OperatorType getType() {
return opType;
}
public boolean isIntOperator(){
if (opType == OperatorType.PLUS ||
opType == OperatorType.MINUS ||
opType == OperatorType.MULTIPLY ||
opType == OperatorType.MODULUS){
return true;
}else {
return false;
}
}
public boolean isBoolOperator(){
return !isIntOperator();
}
public void print() {
switch(opType) {
public OperatorType getType() {
return opType;
}
public boolean isIntOperator(){
if (opType == OperatorType.PLUS ||
opType == OperatorType.MINUS ||
opType == OperatorType.MULTIPLY ||
opType == OperatorType.MODULUS){
return true;
}else {
return false;
}
}
public boolean isBoolOperator(){
return !isIntOperator();
}
public void print() {
switch(opType) {
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
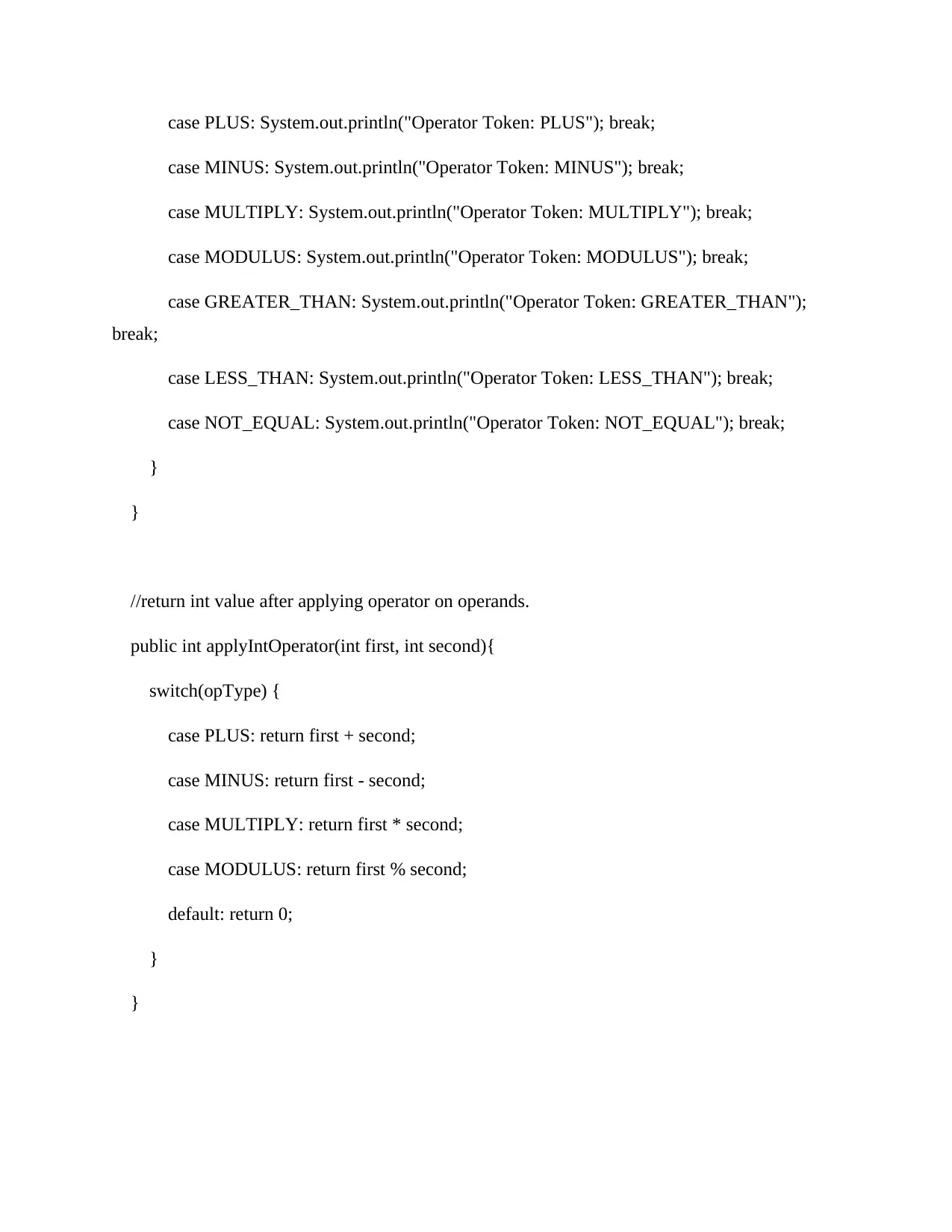
case PLUS: System.out.println("Operator Token: PLUS"); break;
case MINUS: System.out.println("Operator Token: MINUS"); break;
case MULTIPLY: System.out.println("Operator Token: MULTIPLY"); break;
case MODULUS: System.out.println("Operator Token: MODULUS"); break;
case GREATER_THAN: System.out.println("Operator Token: GREATER_THAN");
break;
case LESS_THAN: System.out.println("Operator Token: LESS_THAN"); break;
case NOT_EQUAL: System.out.println("Operator Token: NOT_EQUAL"); break;
}
}
//return int value after applying operator on operands.
public int applyIntOperator(int first, int second){
switch(opType) {
case PLUS: return first + second;
case MINUS: return first - second;
case MULTIPLY: return first * second;
case MODULUS: return first % second;
default: return 0;
}
}
case MINUS: System.out.println("Operator Token: MINUS"); break;
case MULTIPLY: System.out.println("Operator Token: MULTIPLY"); break;
case MODULUS: System.out.println("Operator Token: MODULUS"); break;
case GREATER_THAN: System.out.println("Operator Token: GREATER_THAN");
break;
case LESS_THAN: System.out.println("Operator Token: LESS_THAN"); break;
case NOT_EQUAL: System.out.println("Operator Token: NOT_EQUAL"); break;
}
}
//return int value after applying operator on operands.
public int applyIntOperator(int first, int second){
switch(opType) {
case PLUS: return first + second;
case MINUS: return first - second;
case MULTIPLY: return first * second;
case MODULUS: return first % second;
default: return 0;
}
}
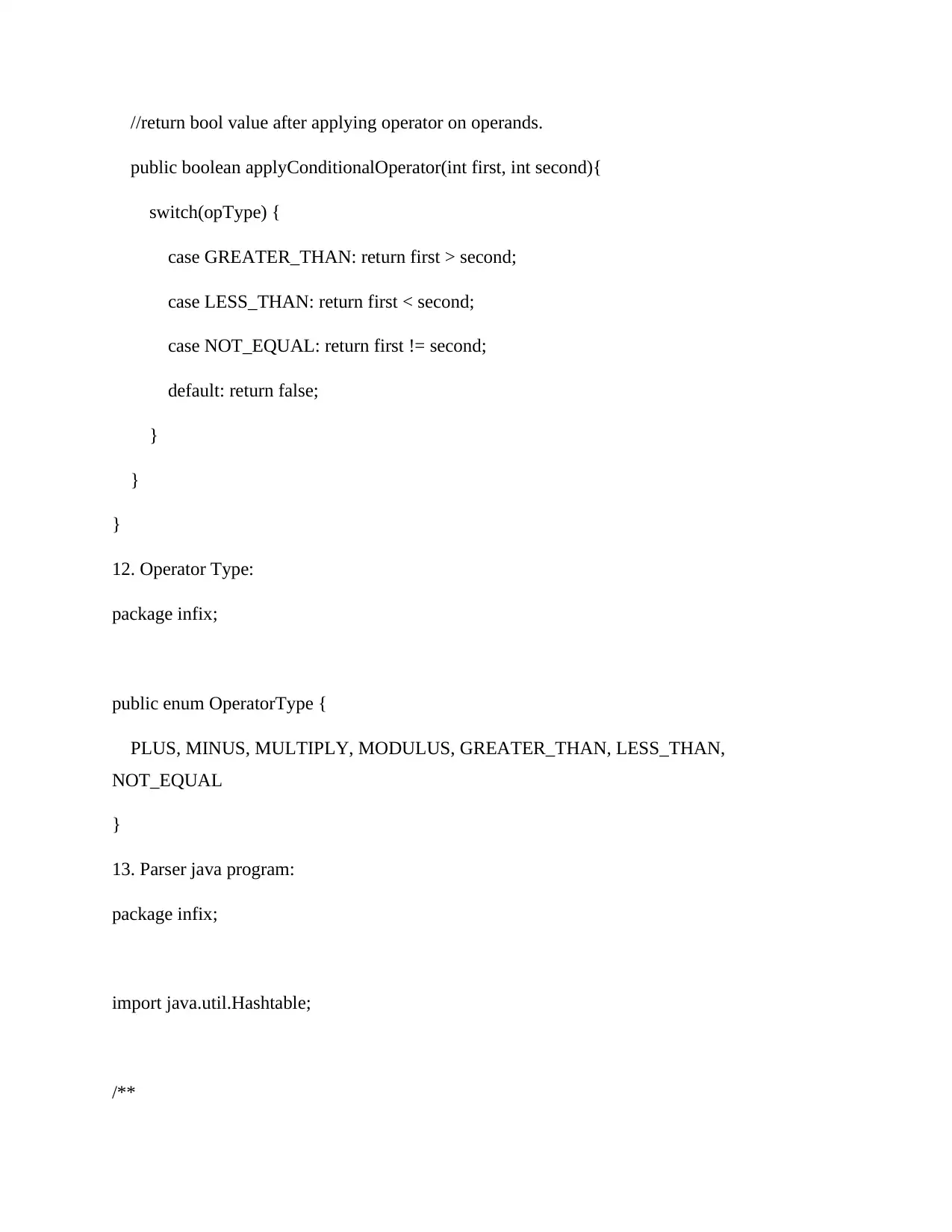
//return bool value after applying operator on operands.
public boolean applyConditionalOperator(int first, int second){
switch(opType) {
case GREATER_THAN: return first > second;
case LESS_THAN: return first < second;
case NOT_EQUAL: return first != second;
default: return false;
}
}
}
12. Operator Type:
package infix;
public enum OperatorType {
PLUS, MINUS, MULTIPLY, MODULUS, GREATER_THAN, LESS_THAN,
NOT_EQUAL
}
13. Parser java program:
package infix;
import java.util.Hashtable;
/**
public boolean applyConditionalOperator(int first, int second){
switch(opType) {
case GREATER_THAN: return first > second;
case LESS_THAN: return first < second;
case NOT_EQUAL: return first != second;
default: return false;
}
}
}
12. Operator Type:
package infix;
public enum OperatorType {
PLUS, MINUS, MULTIPLY, MODULUS, GREATER_THAN, LESS_THAN,
NOT_EQUAL
}
13. Parser java program:
package infix;
import java.util.Hashtable;
/**
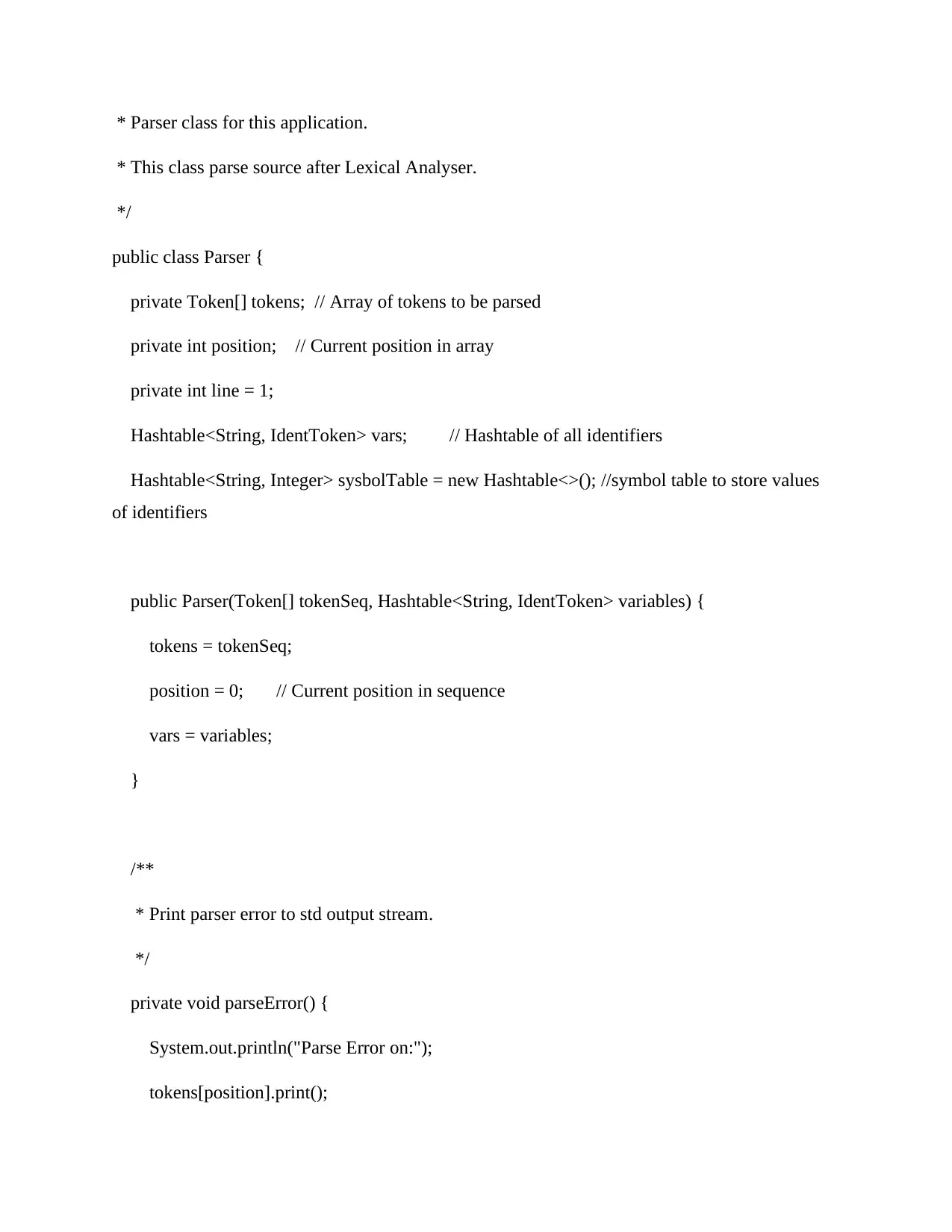
* Parser class for this application.
* This class parse source after Lexical Analyser.
*/
public class Parser {
private Token[] tokens; // Array of tokens to be parsed
private int position; // Current position in array
private int line = 1;
Hashtable<String, IdentToken> vars; // Hashtable of all identifiers
Hashtable<String, Integer> sysbolTable = new Hashtable<>(); //symbol table to store values
of identifiers
public Parser(Token[] tokenSeq, Hashtable<String, IdentToken> variables) {
tokens = tokenSeq;
position = 0; // Current position in sequence
vars = variables;
}
/**
* Print parser error to std output stream.
*/
private void parseError() {
System.out.println("Parse Error on:");
tokens[position].print();
* This class parse source after Lexical Analyser.
*/
public class Parser {
private Token[] tokens; // Array of tokens to be parsed
private int position; // Current position in array
private int line = 1;
Hashtable<String, IdentToken> vars; // Hashtable of all identifiers
Hashtable<String, Integer> sysbolTable = new Hashtable<>(); //symbol table to store values
of identifiers
public Parser(Token[] tokenSeq, Hashtable<String, IdentToken> variables) {
tokens = tokenSeq;
position = 0; // Current position in sequence
vars = variables;
}
/**
* Print parser error to std output stream.
*/
private void parseError() {
System.out.println("Parse Error on:");
tokens[position].print();
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
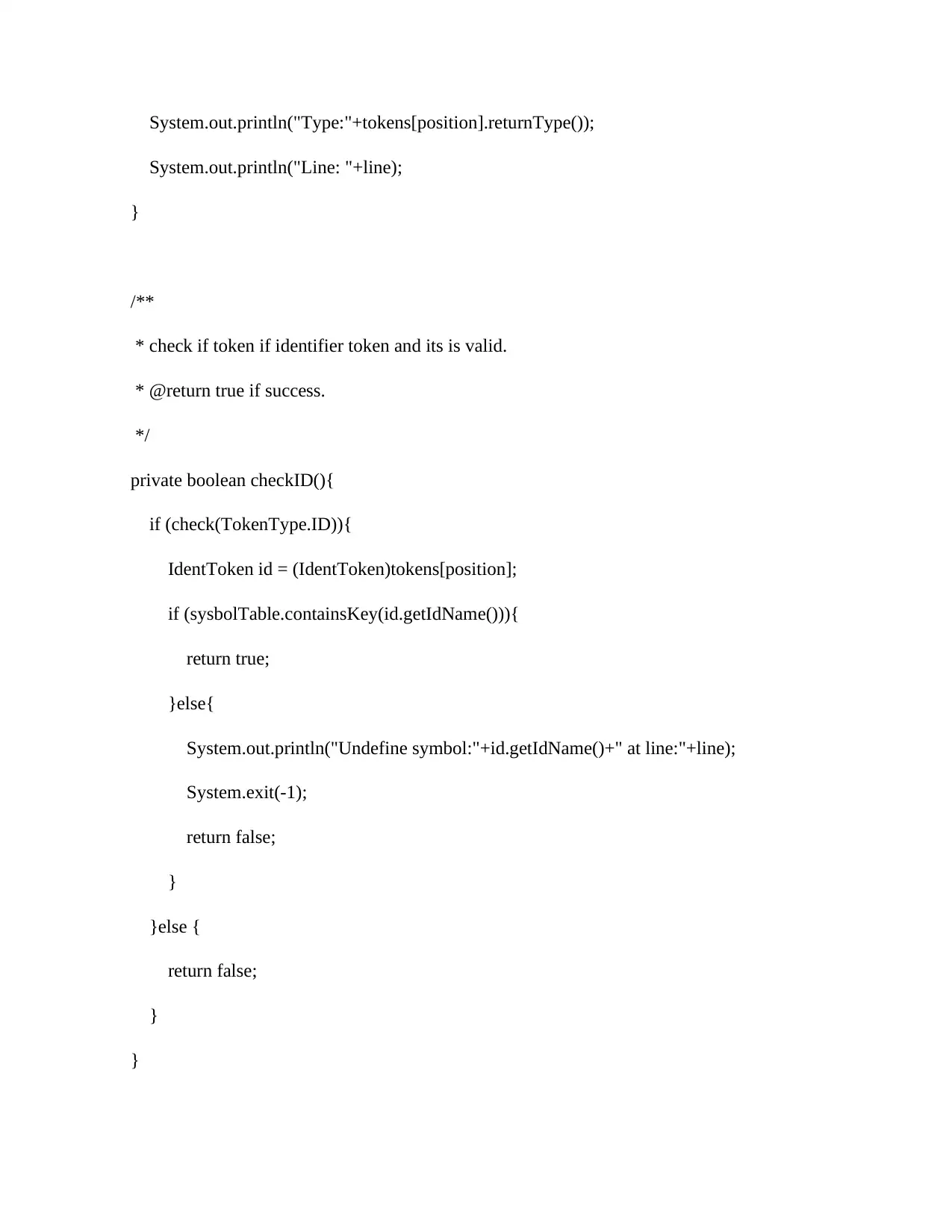
System.out.println("Type:"+tokens[position].returnType());
System.out.println("Line: "+line);
}
/**
* check if token if identifier token and its is valid.
* @return true if success.
*/
private boolean checkID(){
if (check(TokenType.ID)){
IdentToken id = (IdentToken)tokens[position];
if (sysbolTable.containsKey(id.getIdName())){
return true;
}else{
System.out.println("Undefine symbol:"+id.getIdName()+" at line:"+line);
System.exit(-1);
return false;
}
}else {
return false;
}
}
System.out.println("Line: "+line);
}
/**
* check if token if identifier token and its is valid.
* @return true if success.
*/
private boolean checkID(){
if (check(TokenType.ID)){
IdentToken id = (IdentToken)tokens[position];
if (sysbolTable.containsKey(id.getIdName())){
return true;
}else{
System.out.println("Undefine symbol:"+id.getIdName()+" at line:"+line);
System.exit(-1);
return false;
}
}else {
return false;
}
}
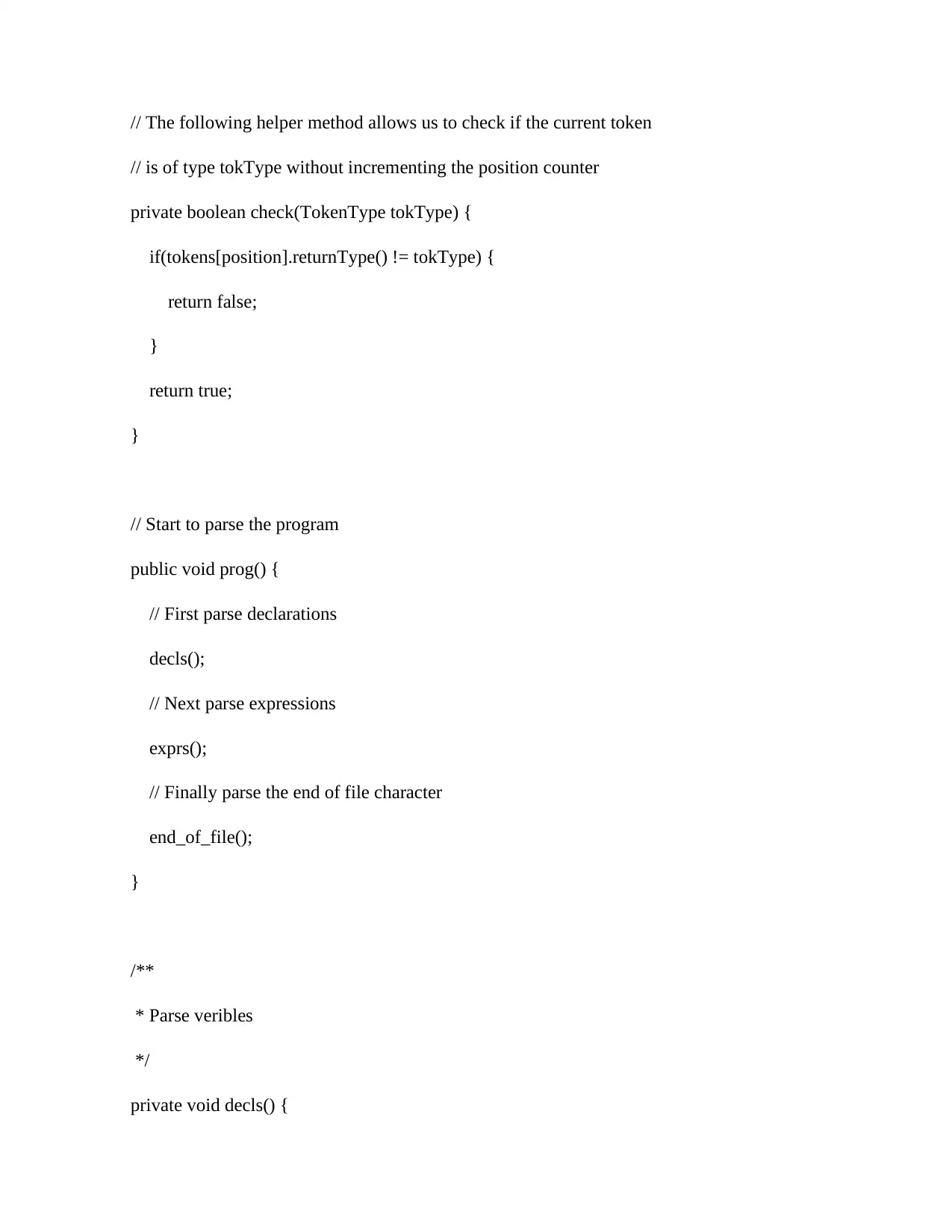
// The following helper method allows us to check if the current token
// is of type tokType without incrementing the position counter
private boolean check(TokenType tokType) {
if(tokens[position].returnType() != tokType) {
return false;
}
return true;
}
// Start to parse the program
public void prog() {
// First parse declarations
decls();
// Next parse expressions
exprs();
// Finally parse the end of file character
end_of_file();
}
/**
* Parse veribles
*/
private void decls() {
// is of type tokType without incrementing the position counter
private boolean check(TokenType tokType) {
if(tokens[position].returnType() != tokType) {
return false;
}
return true;
}
// Start to parse the program
public void prog() {
// First parse declarations
decls();
// Next parse expressions
exprs();
// Finally parse the end of file character
end_of_file();
}
/**
* Parse veribles
*/
private void decls() {
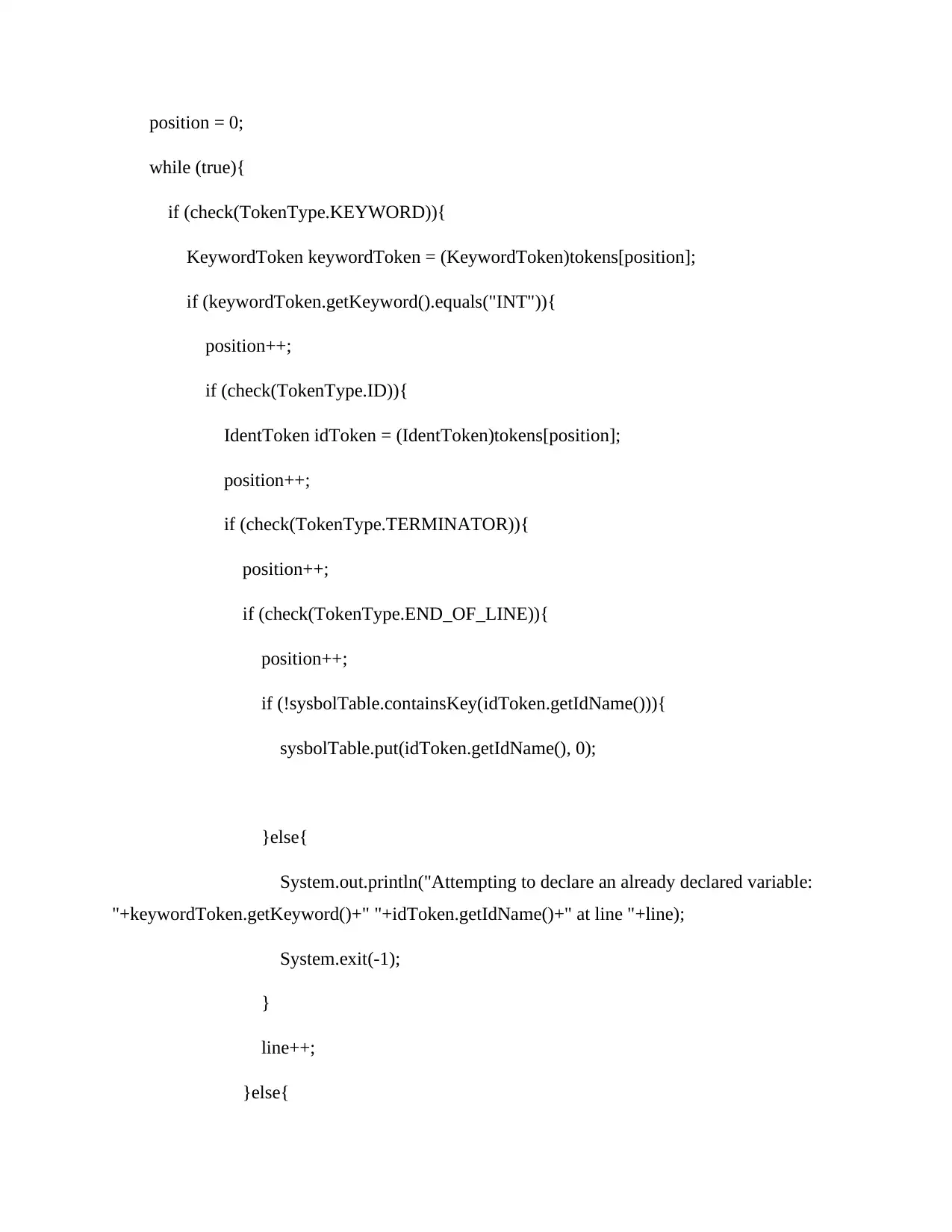
position = 0;
while (true){
if (check(TokenType.KEYWORD)){
KeywordToken keywordToken = (KeywordToken)tokens[position];
if (keywordToken.getKeyword().equals("INT")){
position++;
if (check(TokenType.ID)){
IdentToken idToken = (IdentToken)tokens[position];
position++;
if (check(TokenType.TERMINATOR)){
position++;
if (check(TokenType.END_OF_LINE)){
position++;
if (!sysbolTable.containsKey(idToken.getIdName())){
sysbolTable.put(idToken.getIdName(), 0);
}else{
System.out.println("Attempting to declare an already declared variable:
"+keywordToken.getKeyword()+" "+idToken.getIdName()+" at line "+line);
System.exit(-1);
}
line++;
}else{
while (true){
if (check(TokenType.KEYWORD)){
KeywordToken keywordToken = (KeywordToken)tokens[position];
if (keywordToken.getKeyword().equals("INT")){
position++;
if (check(TokenType.ID)){
IdentToken idToken = (IdentToken)tokens[position];
position++;
if (check(TokenType.TERMINATOR)){
position++;
if (check(TokenType.END_OF_LINE)){
position++;
if (!sysbolTable.containsKey(idToken.getIdName())){
sysbolTable.put(idToken.getIdName(), 0);
}else{
System.out.println("Attempting to declare an already declared variable:
"+keywordToken.getKeyword()+" "+idToken.getIdName()+" at line "+line);
System.exit(-1);
}
line++;
}else{
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
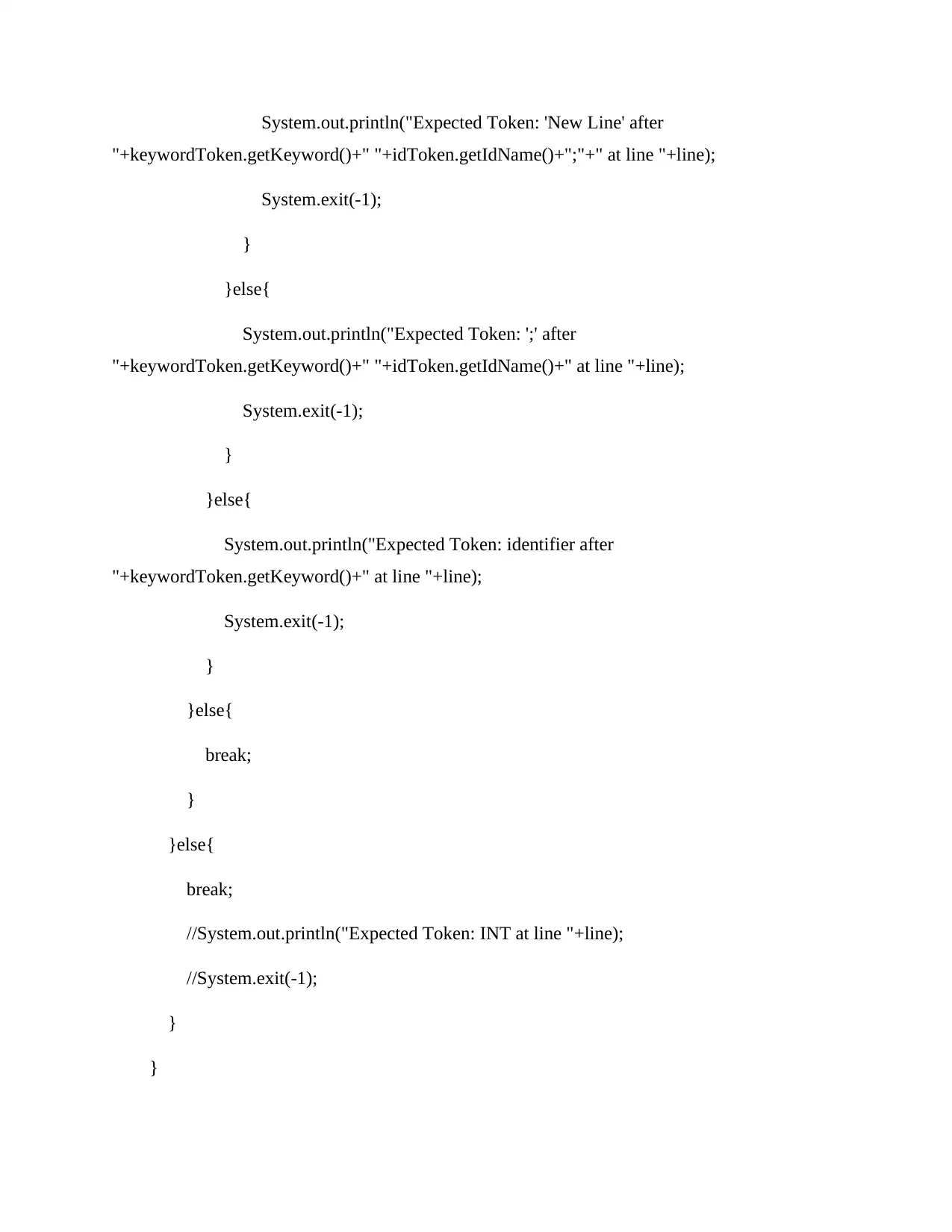
System.out.println("Expected Token: 'New Line' after
"+keywordToken.getKeyword()+" "+idToken.getIdName()+";"+" at line "+line);
System.exit(-1);
}
}else{
System.out.println("Expected Token: ';' after
"+keywordToken.getKeyword()+" "+idToken.getIdName()+" at line "+line);
System.exit(-1);
}
}else{
System.out.println("Expected Token: identifier after
"+keywordToken.getKeyword()+" at line "+line);
System.exit(-1);
}
}else{
break;
}
}else{
break;
//System.out.println("Expected Token: INT at line "+line);
//System.exit(-1);
}
}
"+keywordToken.getKeyword()+" "+idToken.getIdName()+";"+" at line "+line);
System.exit(-1);
}
}else{
System.out.println("Expected Token: ';' after
"+keywordToken.getKeyword()+" "+idToken.getIdName()+" at line "+line);
System.exit(-1);
}
}else{
System.out.println("Expected Token: identifier after
"+keywordToken.getKeyword()+" at line "+line);
System.exit(-1);
}
}else{
break;
}
}else{
break;
//System.out.println("Expected Token: INT at line "+line);
//System.exit(-1);
}
}
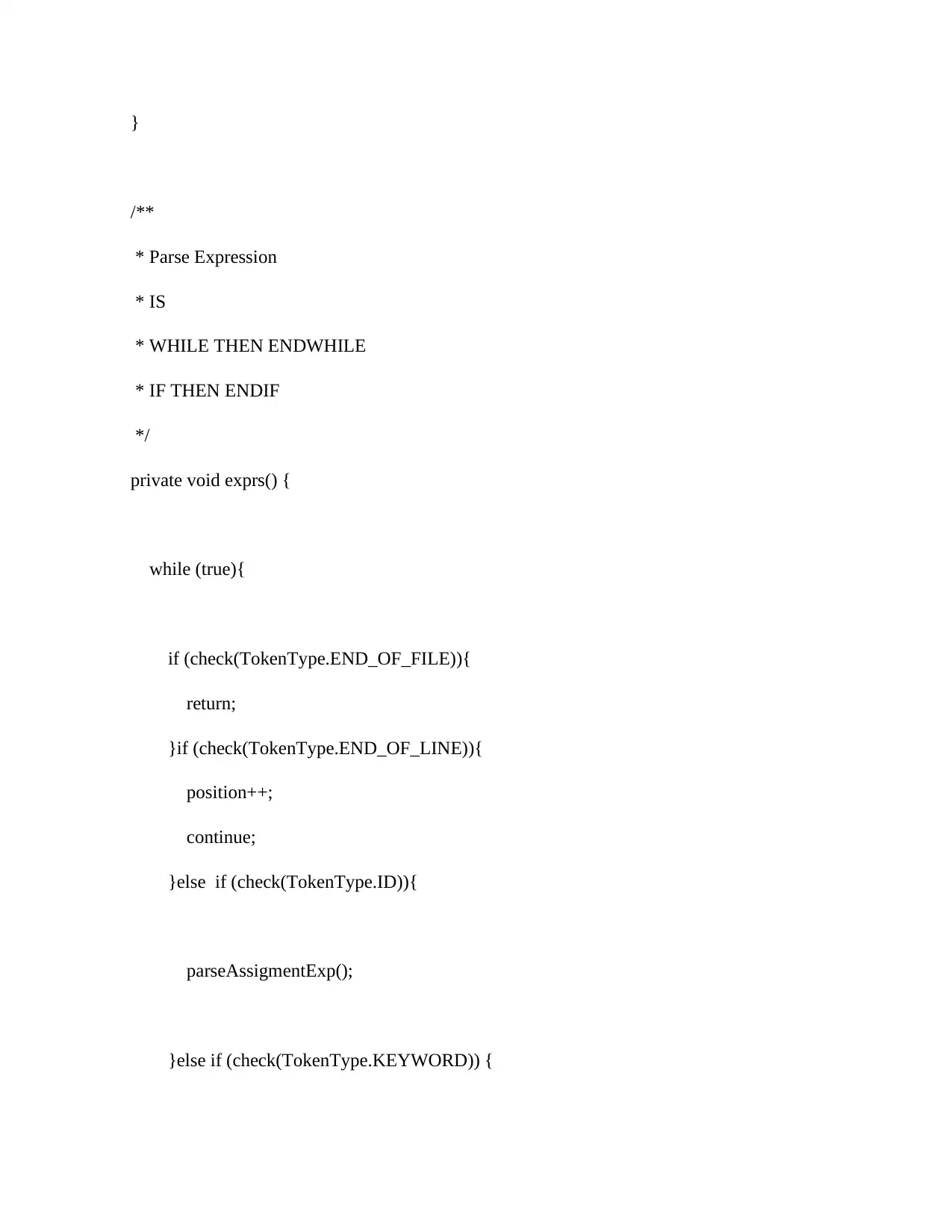
}
/**
* Parse Expression
* IS
* WHILE THEN ENDWHILE
* IF THEN ENDIF
*/
private void exprs() {
while (true){
if (check(TokenType.END_OF_FILE)){
return;
}if (check(TokenType.END_OF_LINE)){
position++;
continue;
}else if (check(TokenType.ID)){
parseAssigmentExp();
}else if (check(TokenType.KEYWORD)) {
/**
* Parse Expression
* IS
* WHILE THEN ENDWHILE
* IF THEN ENDIF
*/
private void exprs() {
while (true){
if (check(TokenType.END_OF_FILE)){
return;
}if (check(TokenType.END_OF_LINE)){
position++;
continue;
}else if (check(TokenType.ID)){
parseAssigmentExp();
}else if (check(TokenType.KEYWORD)) {
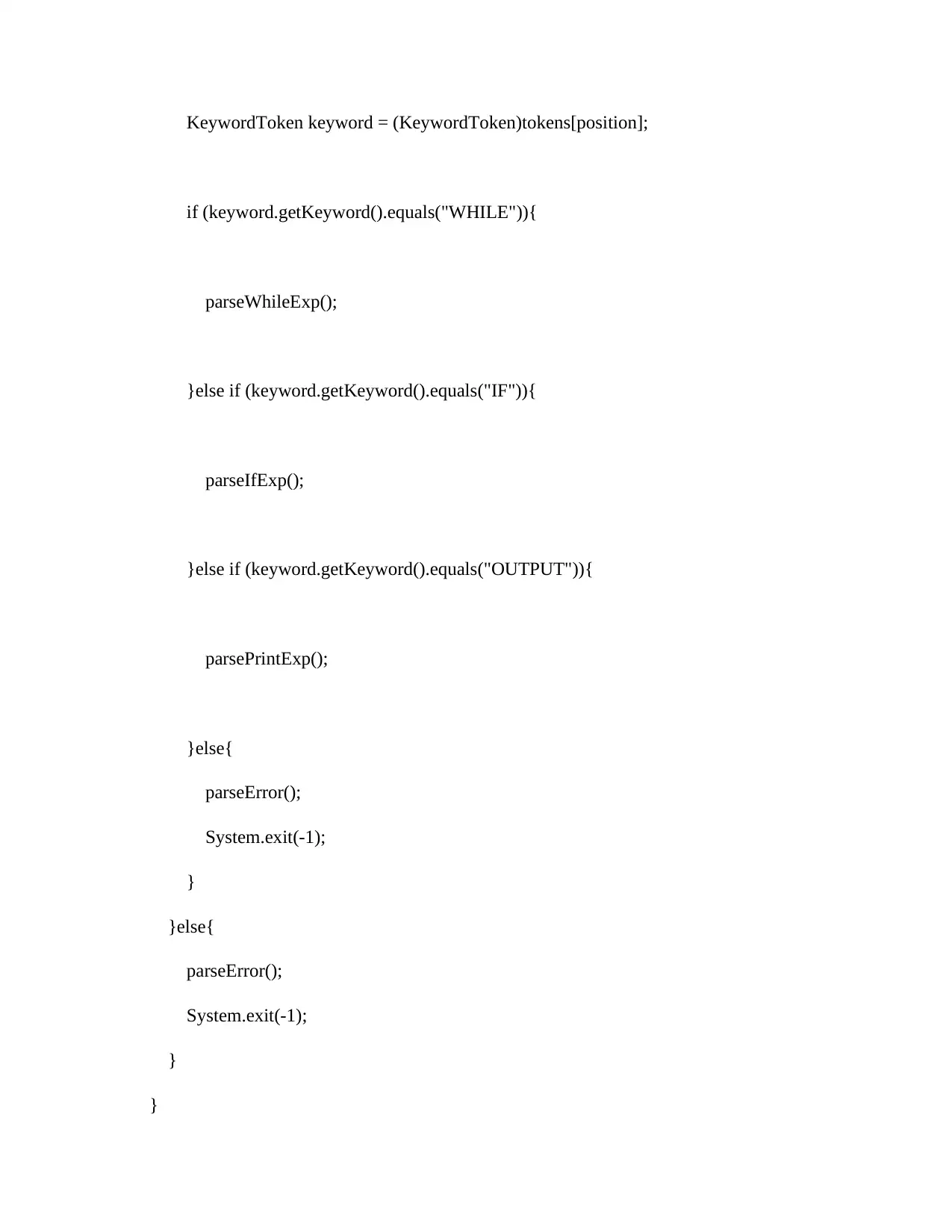
KeywordToken keyword = (KeywordToken)tokens[position];
if (keyword.getKeyword().equals("WHILE")){
parseWhileExp();
}else if (keyword.getKeyword().equals("IF")){
parseIfExp();
}else if (keyword.getKeyword().equals("OUTPUT")){
parsePrintExp();
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}
if (keyword.getKeyword().equals("WHILE")){
parseWhileExp();
}else if (keyword.getKeyword().equals("IF")){
parseIfExp();
}else if (keyword.getKeyword().equals("OUTPUT")){
parsePrintExp();
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
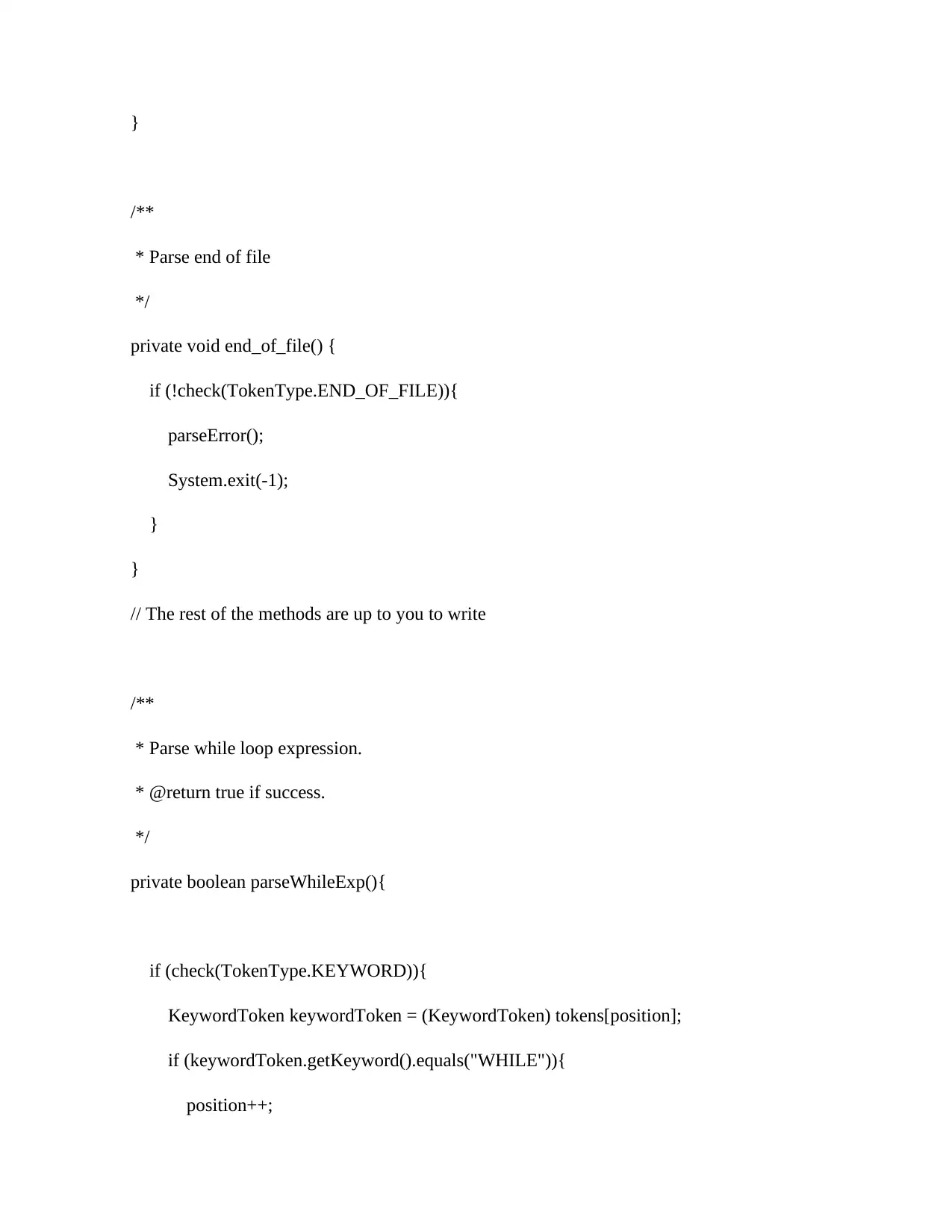
}
/**
* Parse end of file
*/
private void end_of_file() {
if (!check(TokenType.END_OF_FILE)){
parseError();
System.exit(-1);
}
}
// The rest of the methods are up to you to write
/**
* Parse while loop expression.
* @return true if success.
*/
private boolean parseWhileExp(){
if (check(TokenType.KEYWORD)){
KeywordToken keywordToken = (KeywordToken) tokens[position];
if (keywordToken.getKeyword().equals("WHILE")){
position++;
/**
* Parse end of file
*/
private void end_of_file() {
if (!check(TokenType.END_OF_FILE)){
parseError();
System.exit(-1);
}
}
// The rest of the methods are up to you to write
/**
* Parse while loop expression.
* @return true if success.
*/
private boolean parseWhileExp(){
if (check(TokenType.KEYWORD)){
KeywordToken keywordToken = (KeywordToken) tokens[position];
if (keywordToken.getKeyword().equals("WHILE")){
position++;
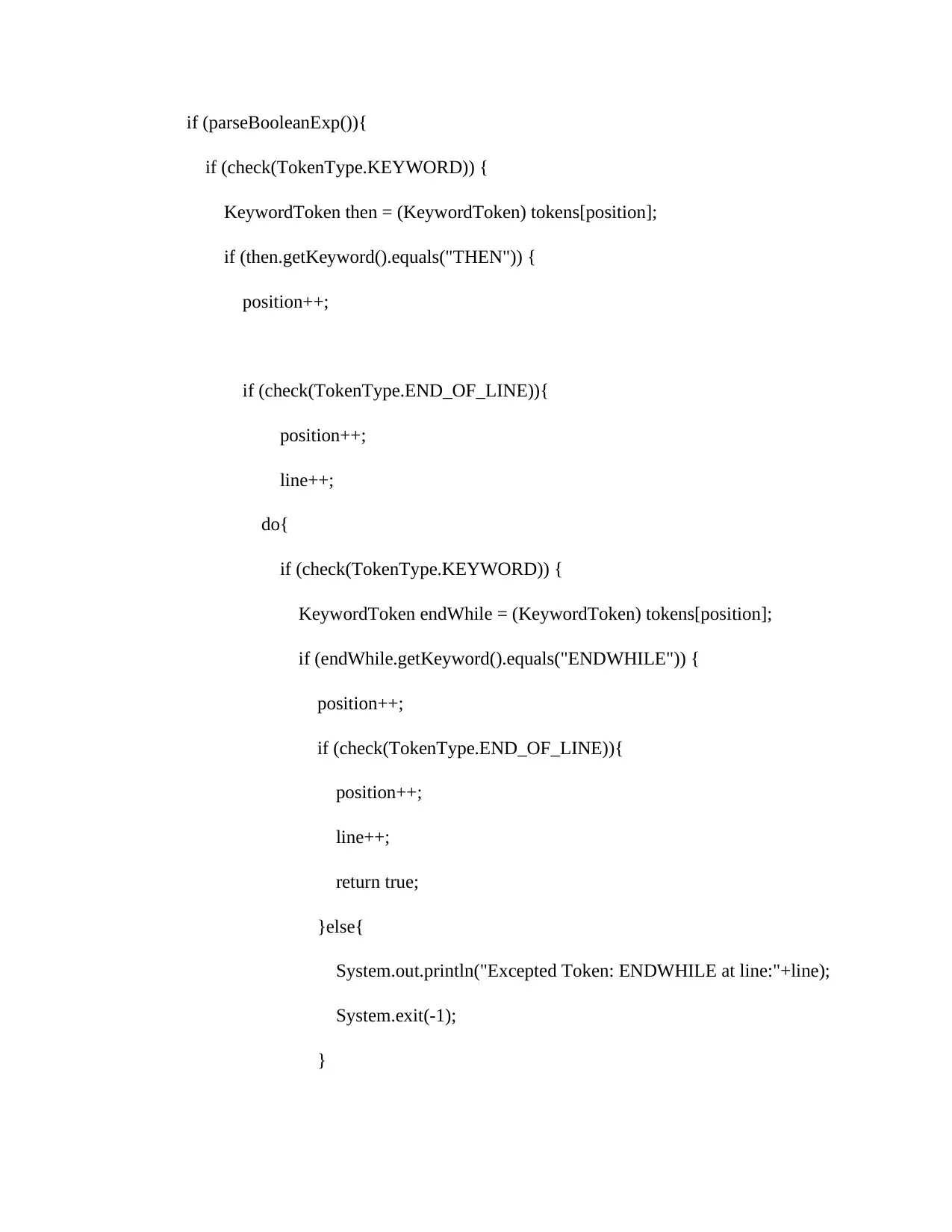
if (parseBooleanExp()){
if (check(TokenType.KEYWORD)) {
KeywordToken then = (KeywordToken) tokens[position];
if (then.getKeyword().equals("THEN")) {
position++;
if (check(TokenType.END_OF_LINE)){
position++;
line++;
do{
if (check(TokenType.KEYWORD)) {
KeywordToken endWhile = (KeywordToken) tokens[position];
if (endWhile.getKeyword().equals("ENDWHILE")) {
position++;
if (check(TokenType.END_OF_LINE)){
position++;
line++;
return true;
}else{
System.out.println("Excepted Token: ENDWHILE at line:"+line);
System.exit(-1);
}
if (check(TokenType.KEYWORD)) {
KeywordToken then = (KeywordToken) tokens[position];
if (then.getKeyword().equals("THEN")) {
position++;
if (check(TokenType.END_OF_LINE)){
position++;
line++;
do{
if (check(TokenType.KEYWORD)) {
KeywordToken endWhile = (KeywordToken) tokens[position];
if (endWhile.getKeyword().equals("ENDWHILE")) {
position++;
if (check(TokenType.END_OF_LINE)){
position++;
line++;
return true;
}else{
System.out.println("Excepted Token: ENDWHILE at line:"+line);
System.exit(-1);
}
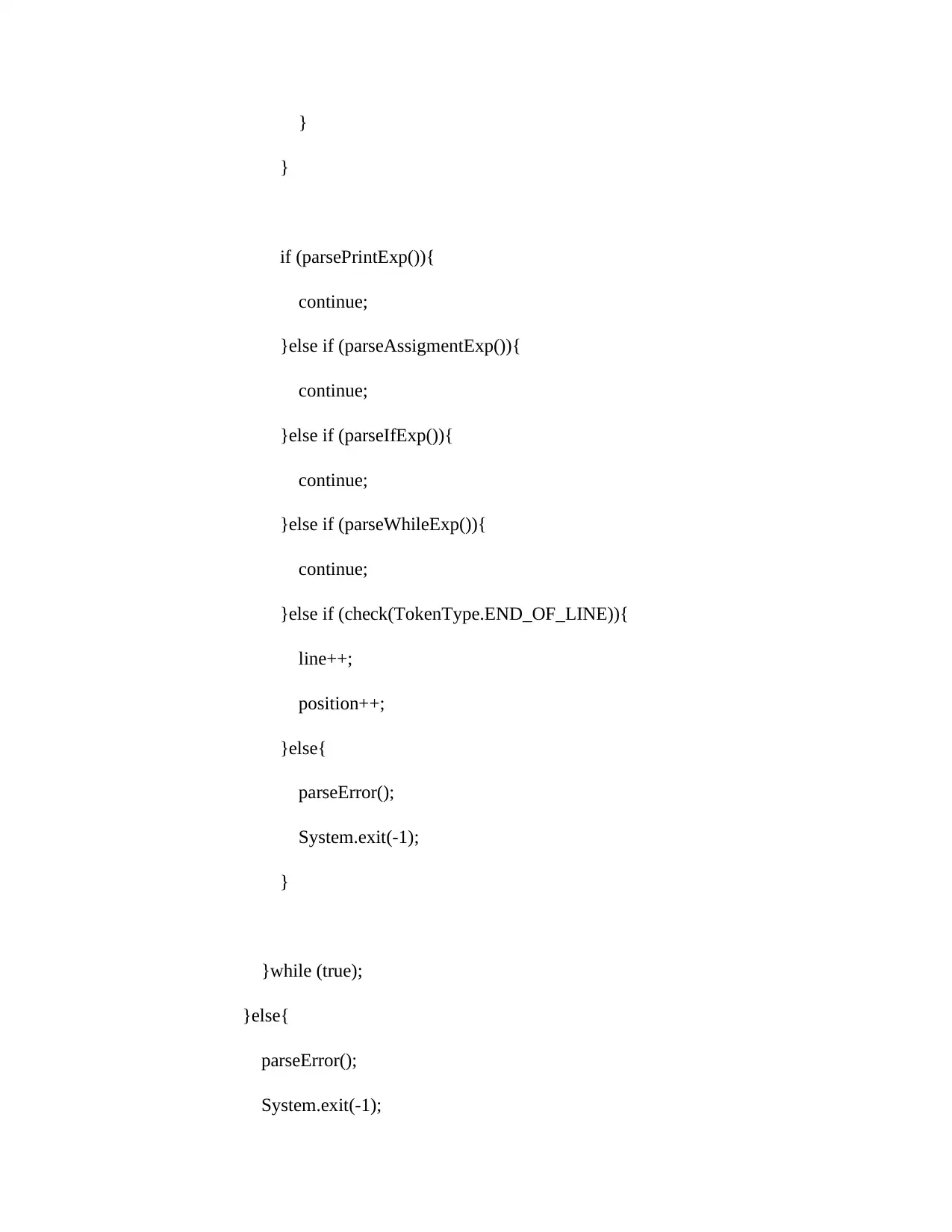
}
}
if (parsePrintExp()){
continue;
}else if (parseAssigmentExp()){
continue;
}else if (parseIfExp()){
continue;
}else if (parseWhileExp()){
continue;
}else if (check(TokenType.END_OF_LINE)){
line++;
position++;
}else{
parseError();
System.exit(-1);
}
}while (true);
}else{
parseError();
System.exit(-1);
}
if (parsePrintExp()){
continue;
}else if (parseAssigmentExp()){
continue;
}else if (parseIfExp()){
continue;
}else if (parseWhileExp()){
continue;
}else if (check(TokenType.END_OF_LINE)){
line++;
position++;
}else{
parseError();
System.exit(-1);
}
}while (true);
}else{
parseError();
System.exit(-1);
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
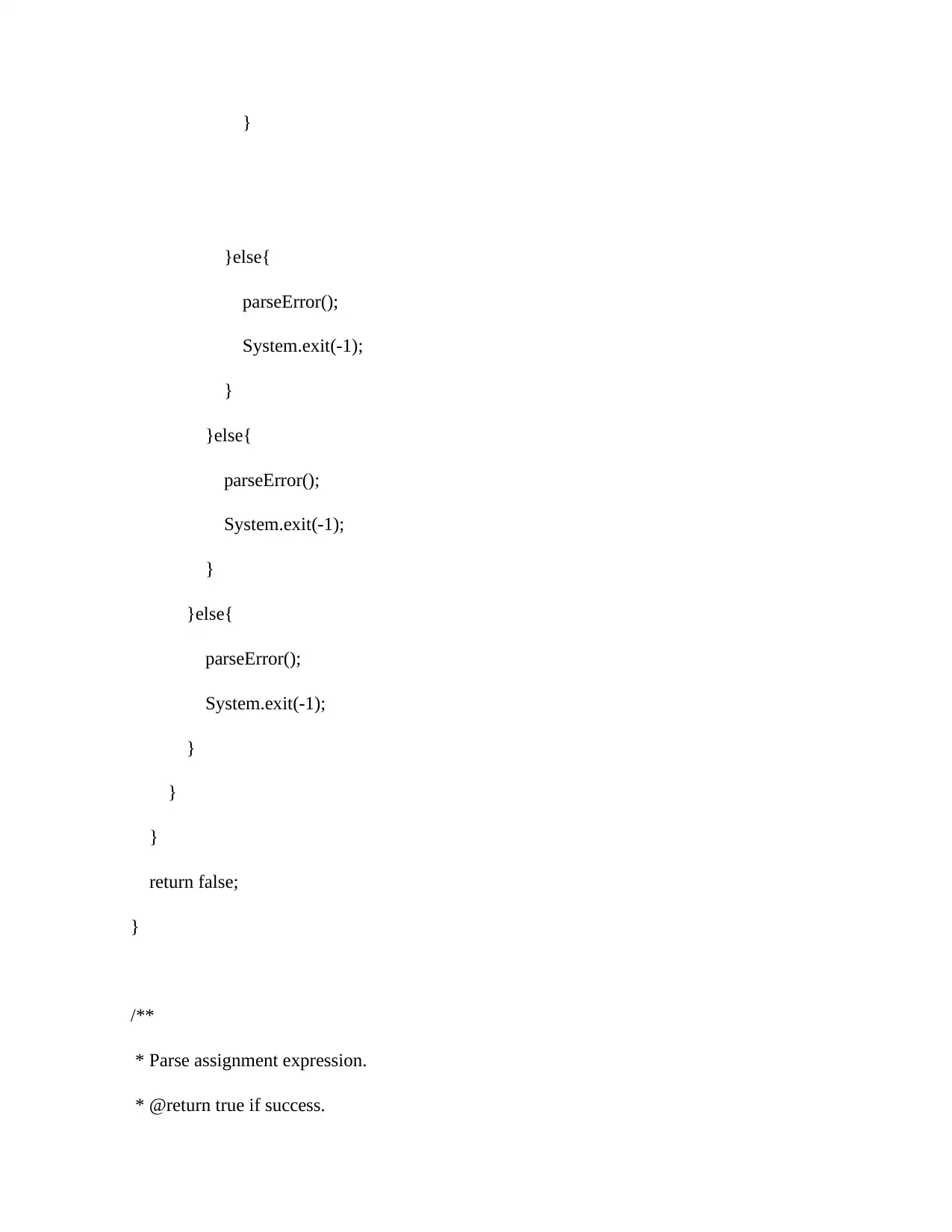
}
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}
}
return false;
}
/**
* Parse assignment expression.
* @return true if success.
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}
}
return false;
}
/**
* Parse assignment expression.
* @return true if success.
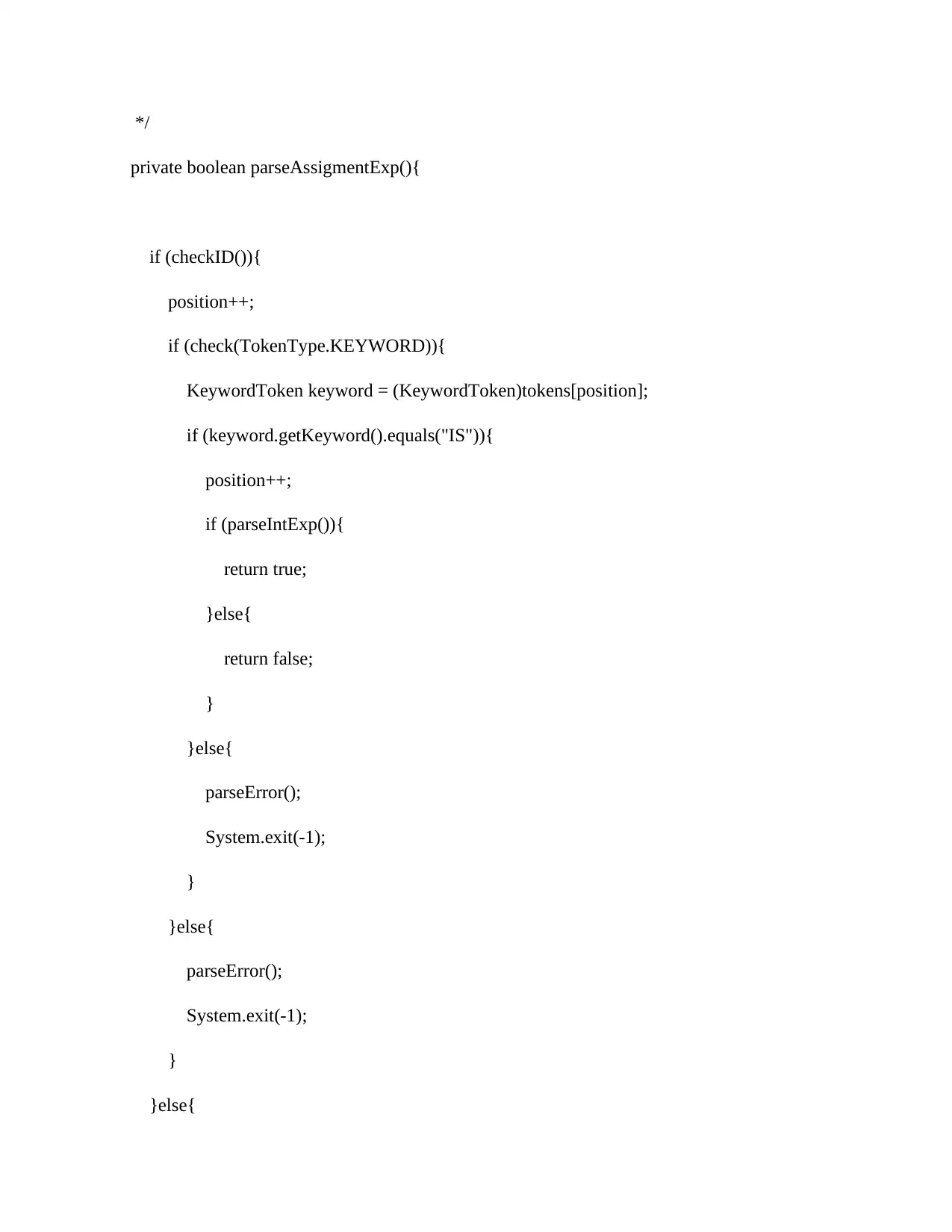
*/
private boolean parseAssigmentExp(){
if (checkID()){
position++;
if (check(TokenType.KEYWORD)){
KeywordToken keyword = (KeywordToken)tokens[position];
if (keyword.getKeyword().equals("IS")){
position++;
if (parseIntExp()){
return true;
}else{
return false;
}
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
private boolean parseAssigmentExp(){
if (checkID()){
position++;
if (check(TokenType.KEYWORD)){
KeywordToken keyword = (KeywordToken)tokens[position];
if (keyword.getKeyword().equals("IS")){
position++;
if (parseIntExp()){
return true;
}else{
return false;
}
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
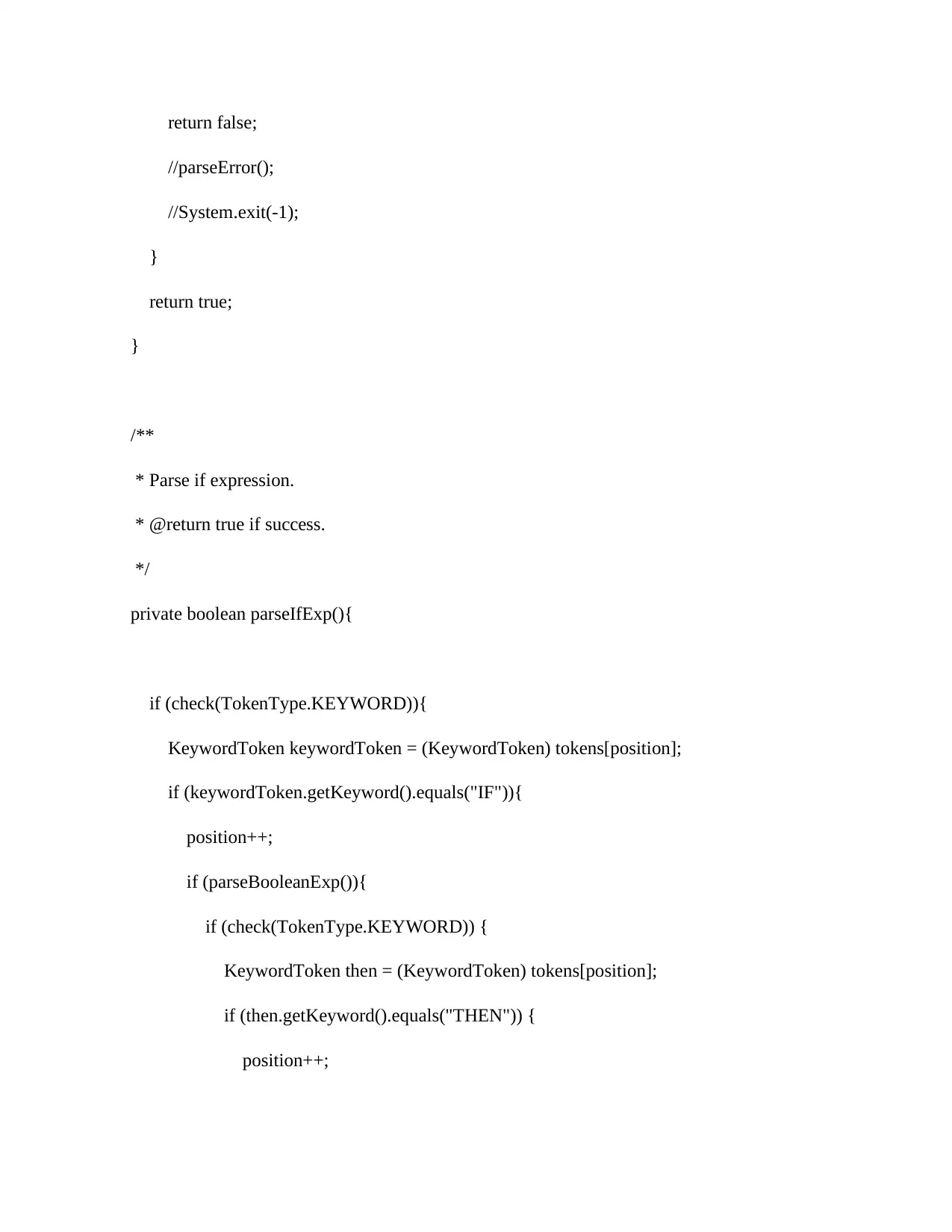
return false;
//parseError();
//System.exit(-1);
}
return true;
}
/**
* Parse if expression.
* @return true if success.
*/
private boolean parseIfExp(){
if (check(TokenType.KEYWORD)){
KeywordToken keywordToken = (KeywordToken) tokens[position];
if (keywordToken.getKeyword().equals("IF")){
position++;
if (parseBooleanExp()){
if (check(TokenType.KEYWORD)) {
KeywordToken then = (KeywordToken) tokens[position];
if (then.getKeyword().equals("THEN")) {
position++;
//parseError();
//System.exit(-1);
}
return true;
}
/**
* Parse if expression.
* @return true if success.
*/
private boolean parseIfExp(){
if (check(TokenType.KEYWORD)){
KeywordToken keywordToken = (KeywordToken) tokens[position];
if (keywordToken.getKeyword().equals("IF")){
position++;
if (parseBooleanExp()){
if (check(TokenType.KEYWORD)) {
KeywordToken then = (KeywordToken) tokens[position];
if (then.getKeyword().equals("THEN")) {
position++;
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
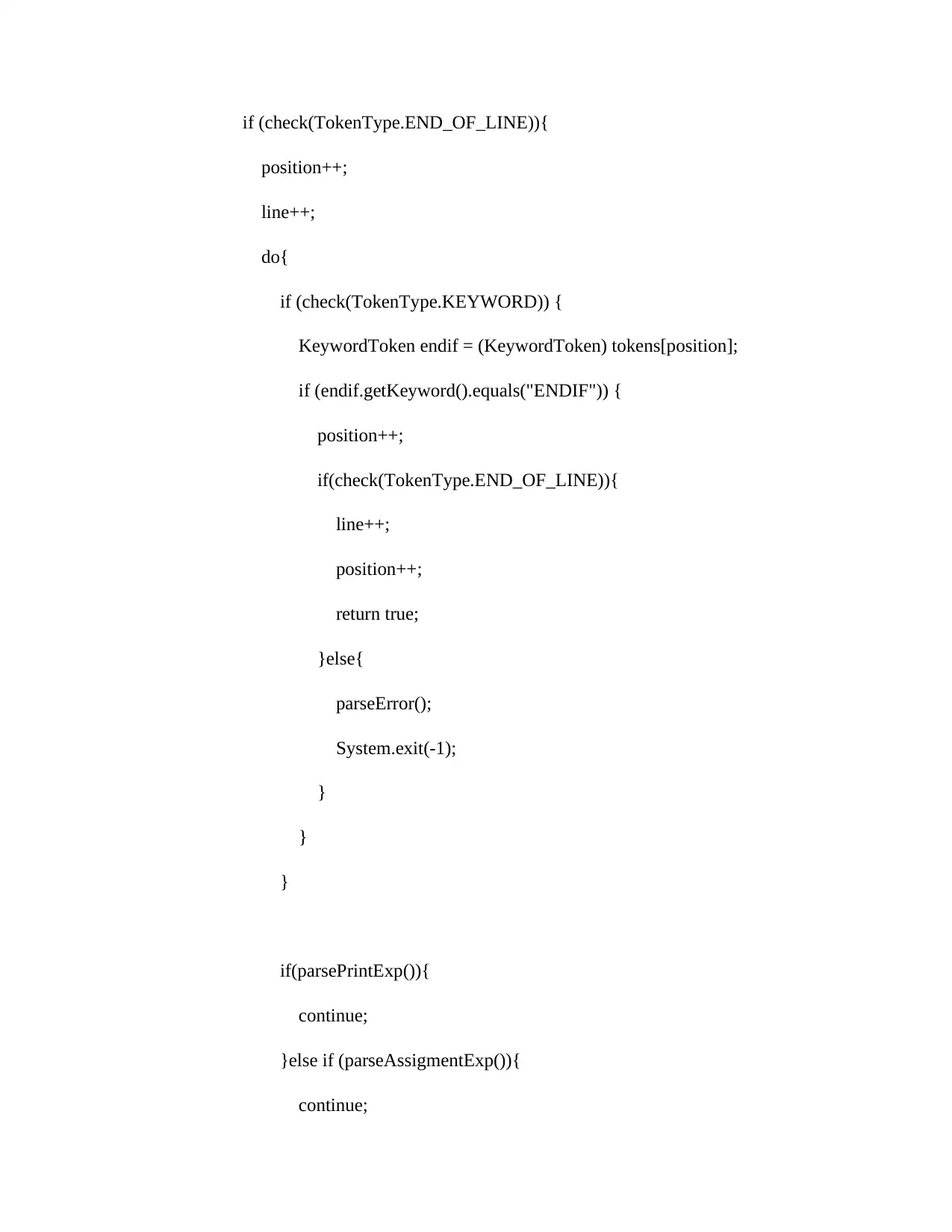
if (check(TokenType.END_OF_LINE)){
position++;
line++;
do{
if (check(TokenType.KEYWORD)) {
KeywordToken endif = (KeywordToken) tokens[position];
if (endif.getKeyword().equals("ENDIF")) {
position++;
if(check(TokenType.END_OF_LINE)){
line++;
position++;
return true;
}else{
parseError();
System.exit(-1);
}
}
}
if(parsePrintExp()){
continue;
}else if (parseAssigmentExp()){
continue;
position++;
line++;
do{
if (check(TokenType.KEYWORD)) {
KeywordToken endif = (KeywordToken) tokens[position];
if (endif.getKeyword().equals("ENDIF")) {
position++;
if(check(TokenType.END_OF_LINE)){
line++;
position++;
return true;
}else{
parseError();
System.exit(-1);
}
}
}
if(parsePrintExp()){
continue;
}else if (parseAssigmentExp()){
continue;
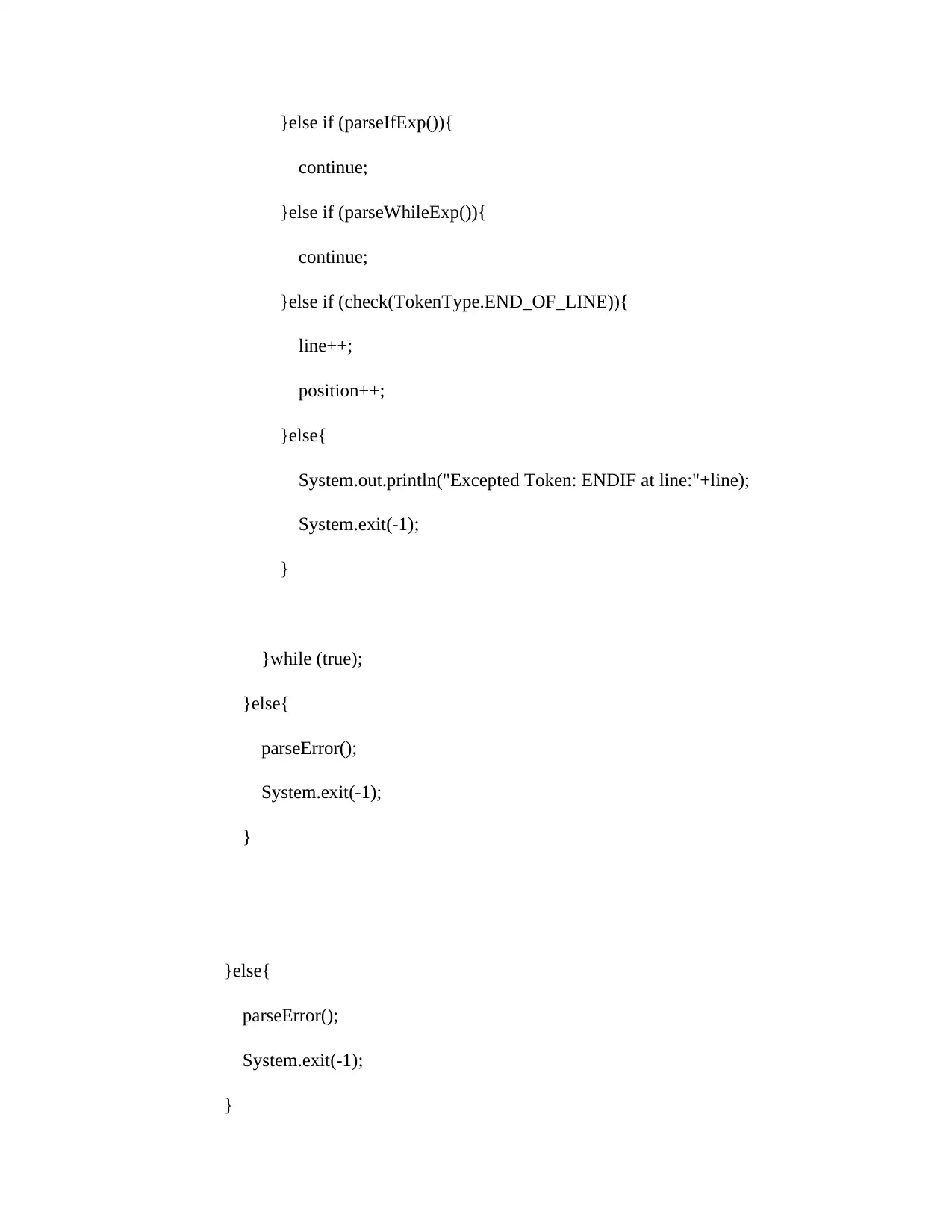
}else if (parseIfExp()){
continue;
}else if (parseWhileExp()){
continue;
}else if (check(TokenType.END_OF_LINE)){
line++;
position++;
}else{
System.out.println("Excepted Token: ENDIF at line:"+line);
System.exit(-1);
}
}while (true);
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
continue;
}else if (parseWhileExp()){
continue;
}else if (check(TokenType.END_OF_LINE)){
line++;
position++;
}else{
System.out.println("Excepted Token: ENDIF at line:"+line);
System.exit(-1);
}
}while (true);
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
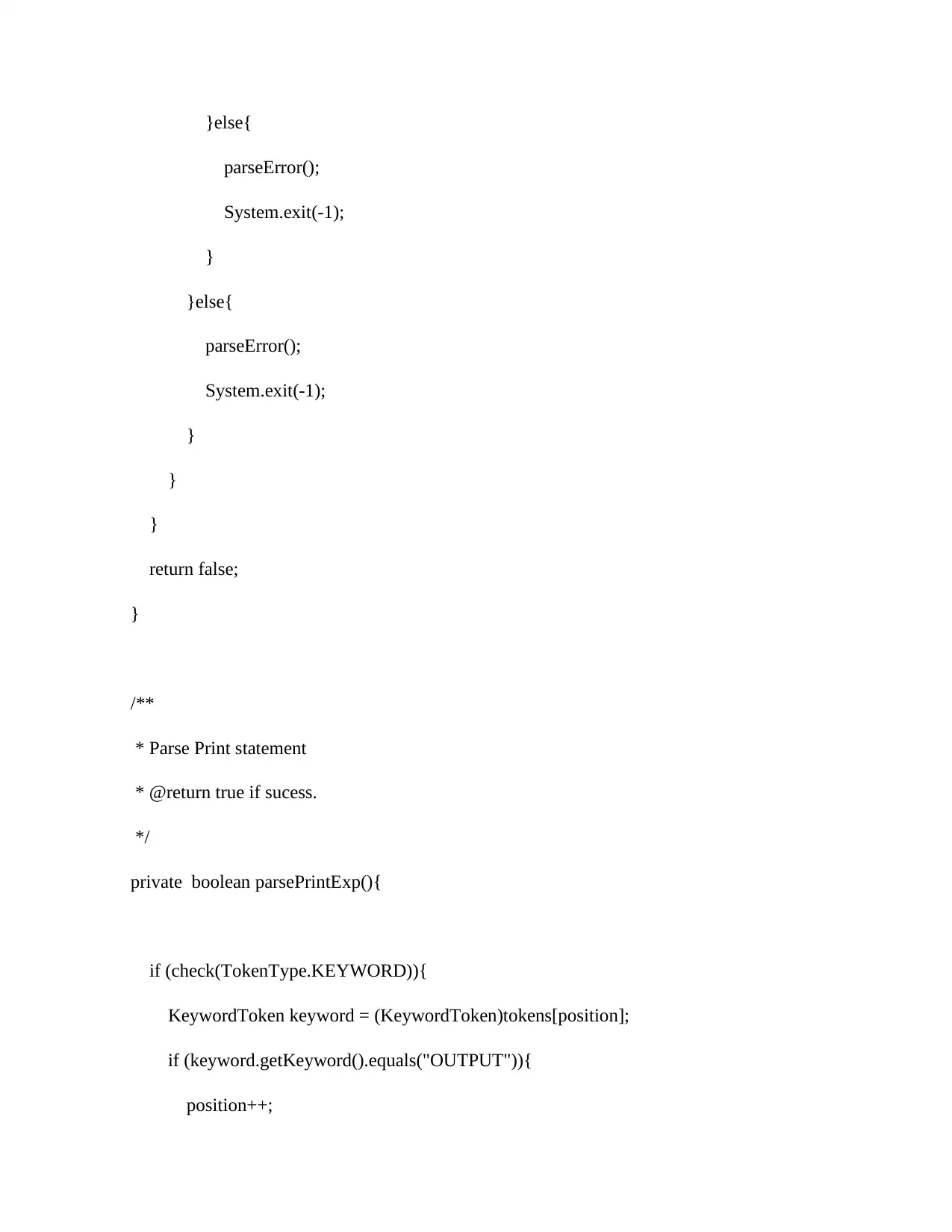
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}
}
return false;
}
/**
* Parse Print statement
* @return true if sucess.
*/
private boolean parsePrintExp(){
if (check(TokenType.KEYWORD)){
KeywordToken keyword = (KeywordToken)tokens[position];
if (keyword.getKeyword().equals("OUTPUT")){
position++;
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}
}
return false;
}
/**
* Parse Print statement
* @return true if sucess.
*/
private boolean parsePrintExp(){
if (check(TokenType.KEYWORD)){
KeywordToken keyword = (KeywordToken)tokens[position];
if (keyword.getKeyword().equals("OUTPUT")){
position++;
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
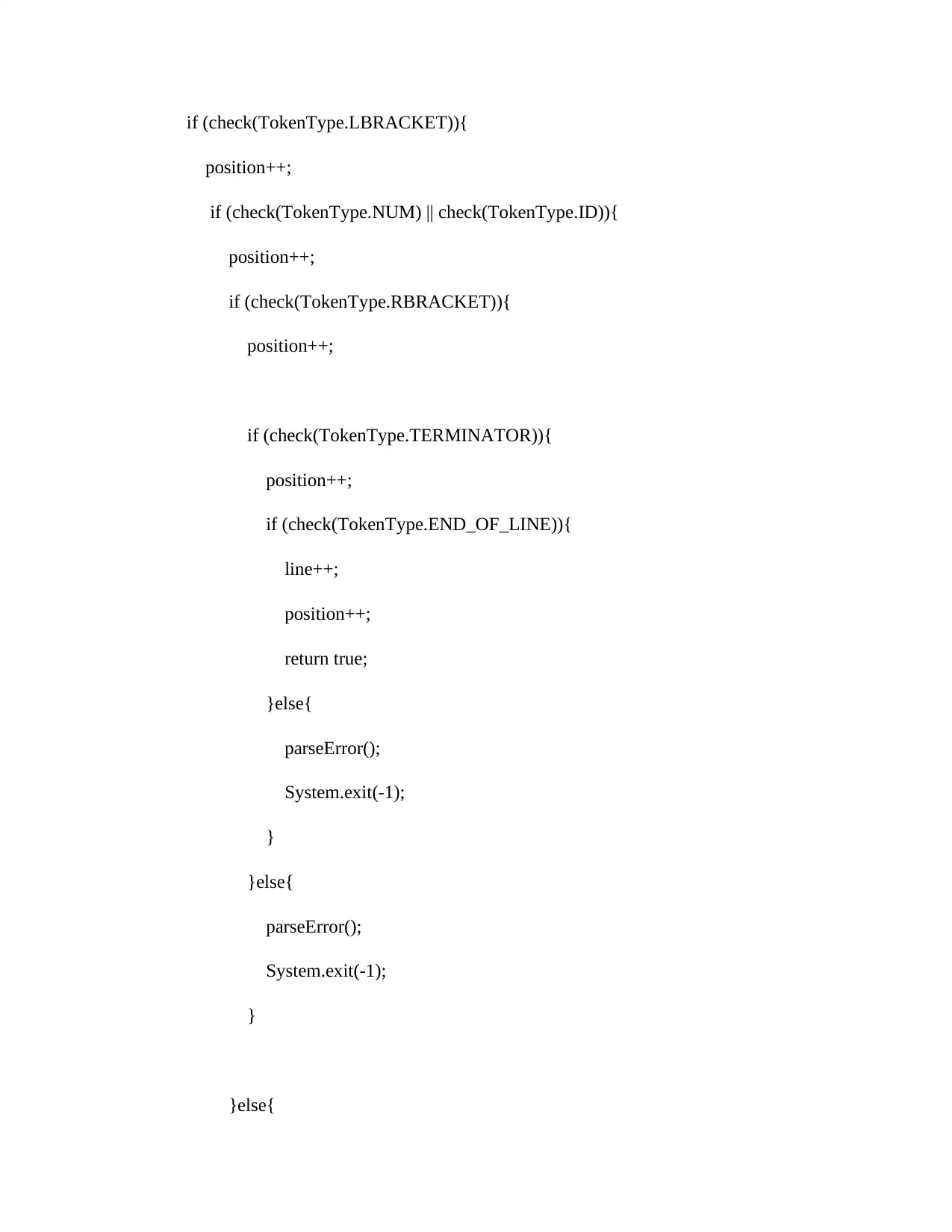
if (check(TokenType.LBRACKET)){
position++;
if (check(TokenType.NUM) || check(TokenType.ID)){
position++;
if (check(TokenType.RBRACKET)){
position++;
if (check(TokenType.TERMINATOR)){
position++;
if (check(TokenType.END_OF_LINE)){
line++;
position++;
return true;
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
position++;
if (check(TokenType.NUM) || check(TokenType.ID)){
position++;
if (check(TokenType.RBRACKET)){
position++;
if (check(TokenType.TERMINATOR)){
position++;
if (check(TokenType.END_OF_LINE)){
line++;
position++;
return true;
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
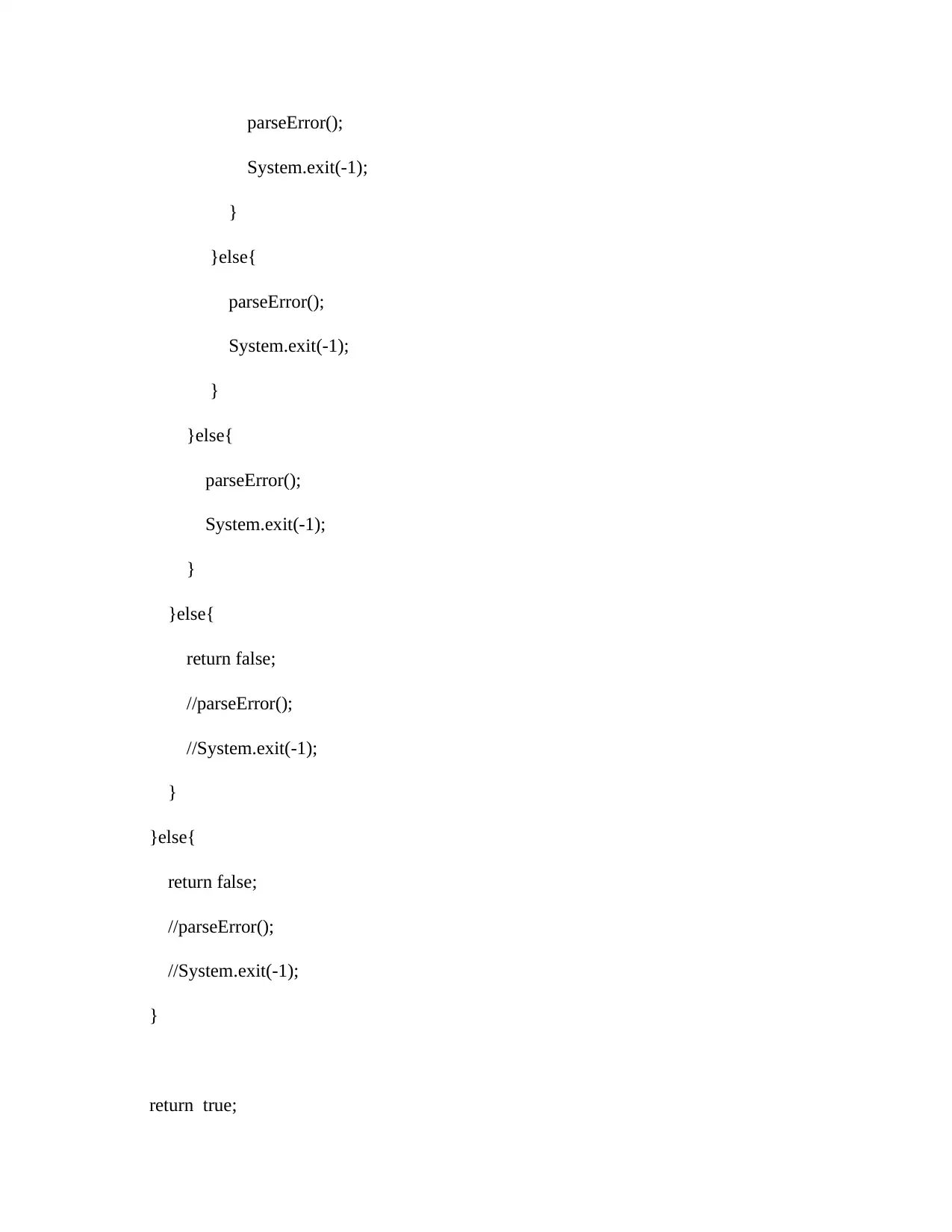
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
return false;
//parseError();
//System.exit(-1);
}
}else{
return false;
//parseError();
//System.exit(-1);
}
return true;
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
return false;
//parseError();
//System.exit(-1);
}
}else{
return false;
//parseError();
//System.exit(-1);
}
return true;
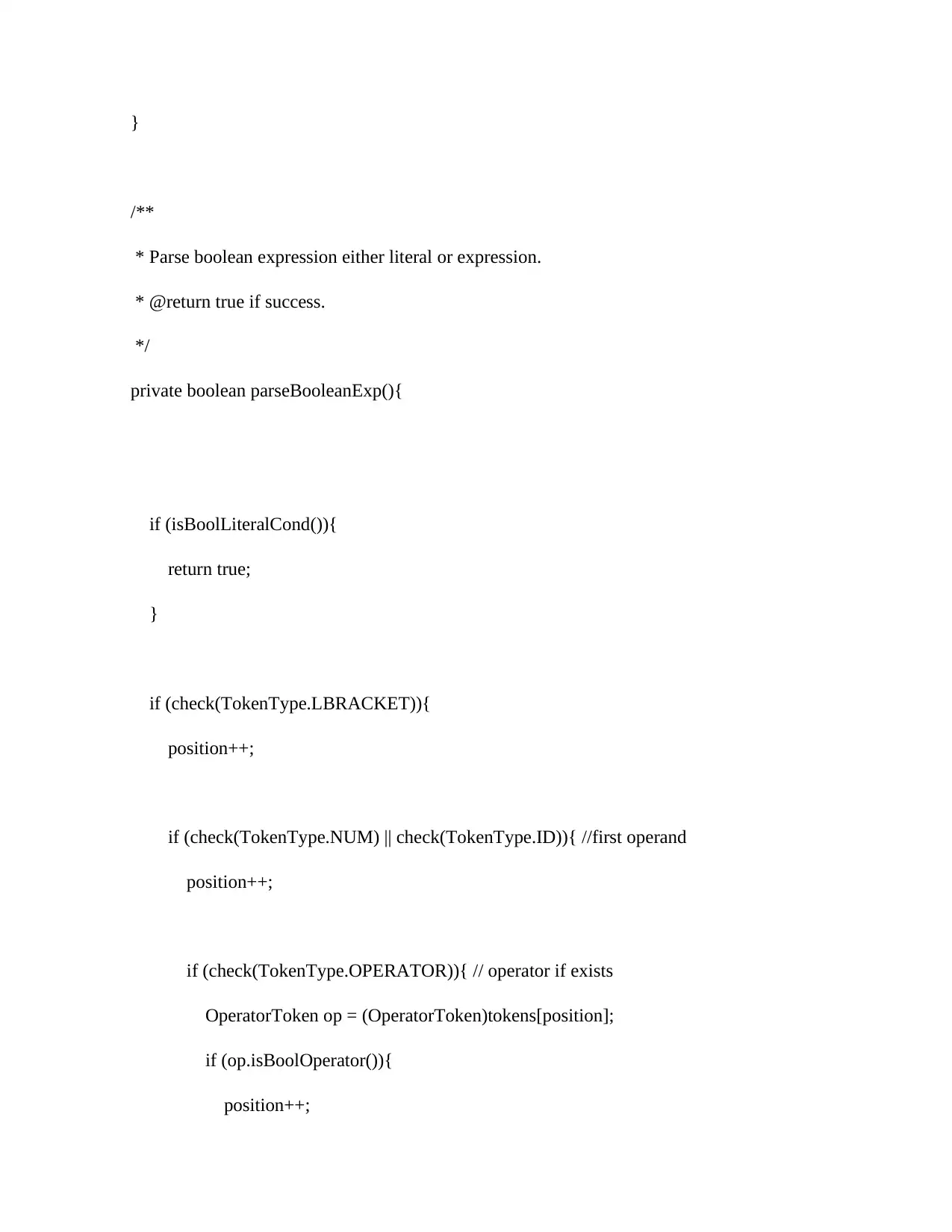
}
/**
* Parse boolean expression either literal or expression.
* @return true if success.
*/
private boolean parseBooleanExp(){
if (isBoolLiteralCond()){
return true;
}
if (check(TokenType.LBRACKET)){
position++;
if (check(TokenType.NUM) || check(TokenType.ID)){ //first operand
position++;
if (check(TokenType.OPERATOR)){ // operator if exists
OperatorToken op = (OperatorToken)tokens[position];
if (op.isBoolOperator()){
position++;
/**
* Parse boolean expression either literal or expression.
* @return true if success.
*/
private boolean parseBooleanExp(){
if (isBoolLiteralCond()){
return true;
}
if (check(TokenType.LBRACKET)){
position++;
if (check(TokenType.NUM) || check(TokenType.ID)){ //first operand
position++;
if (check(TokenType.OPERATOR)){ // operator if exists
OperatorToken op = (OperatorToken)tokens[position];
if (op.isBoolOperator()){
position++;
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
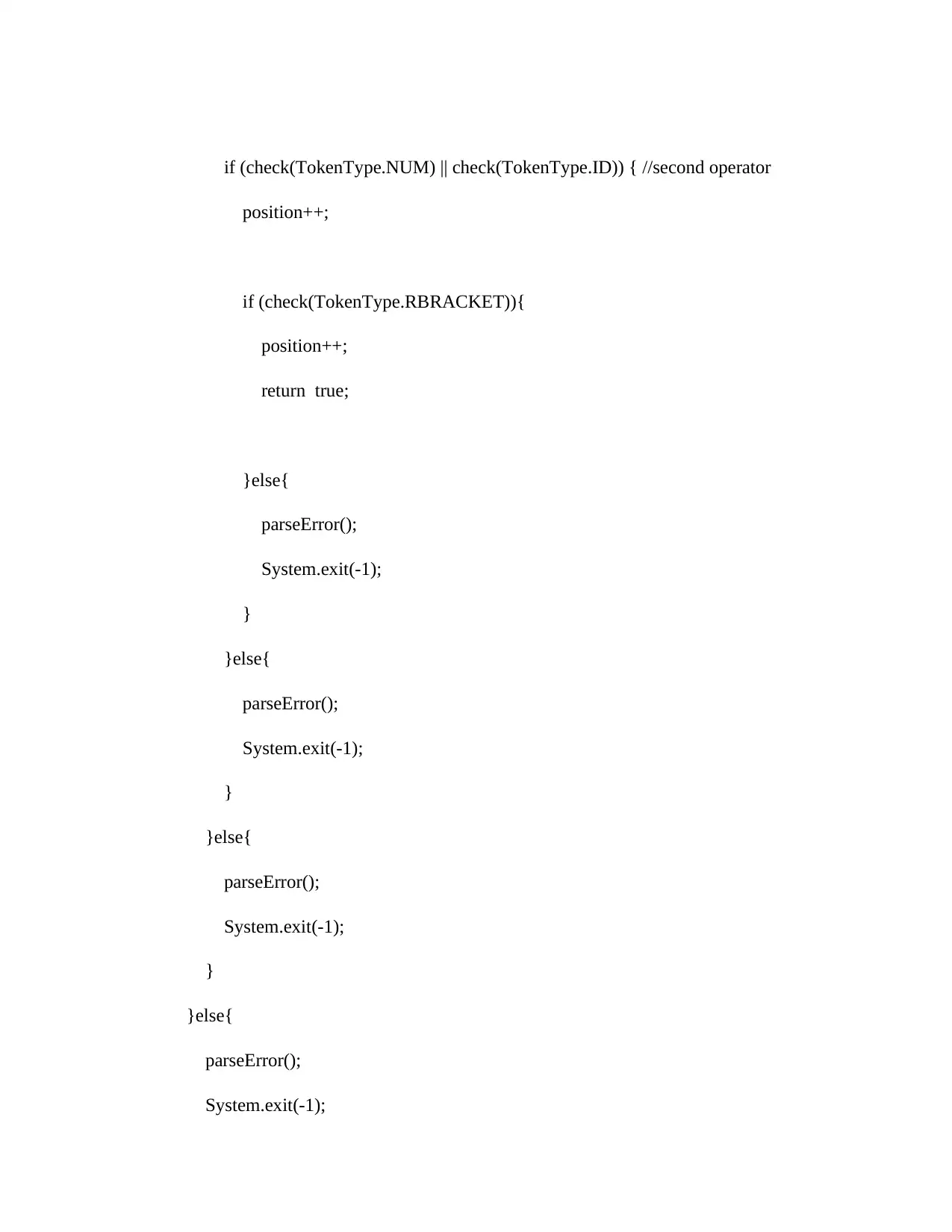
if (check(TokenType.NUM) || check(TokenType.ID)) { //second operator
position++;
if (check(TokenType.RBRACKET)){
position++;
return true;
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
position++;
if (check(TokenType.RBRACKET)){
position++;
return true;
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
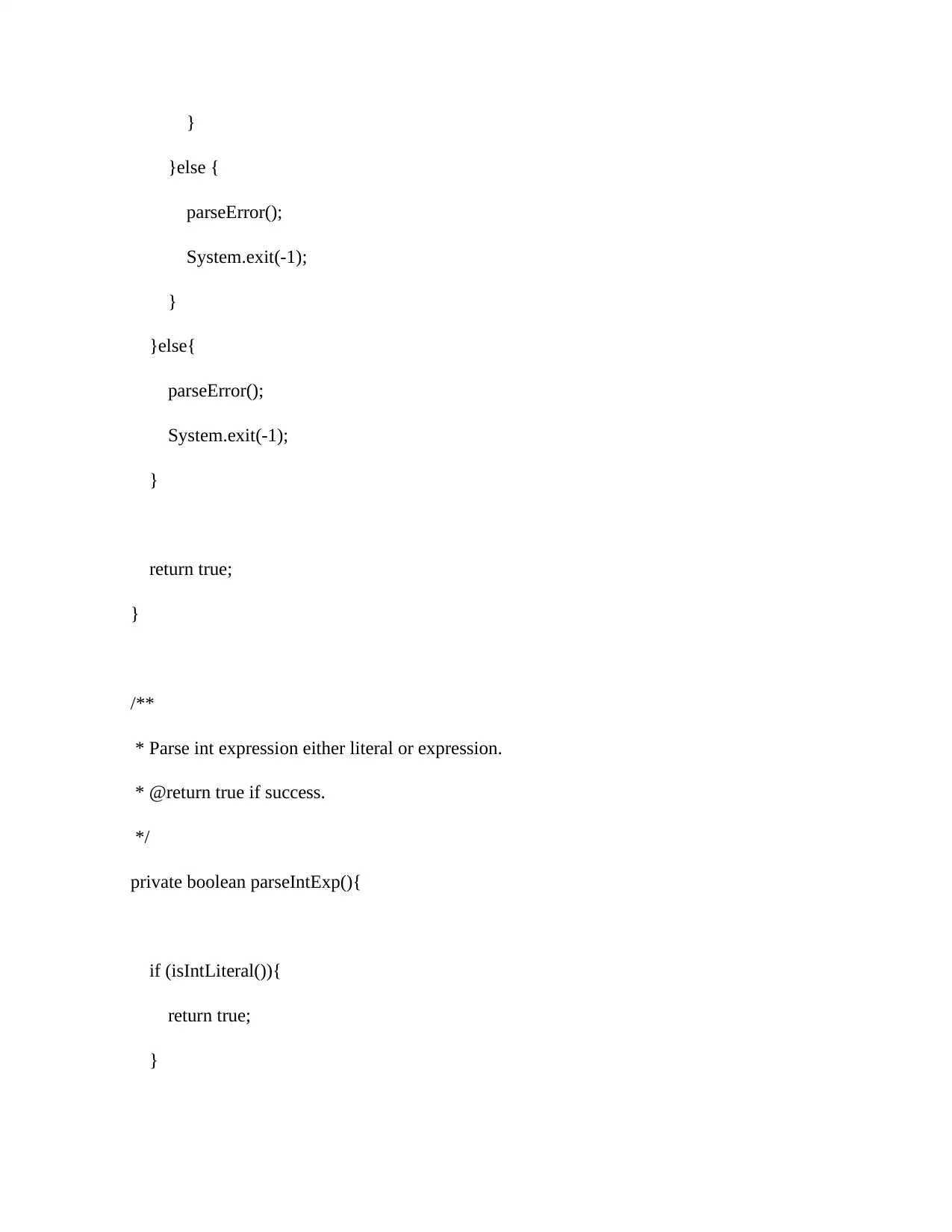
}
}else {
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
return true;
}
/**
* Parse int expression either literal or expression.
* @return true if success.
*/
private boolean parseIntExp(){
if (isIntLiteral()){
return true;
}
}else {
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
return true;
}
/**
* Parse int expression either literal or expression.
* @return true if success.
*/
private boolean parseIntExp(){
if (isIntLiteral()){
return true;
}
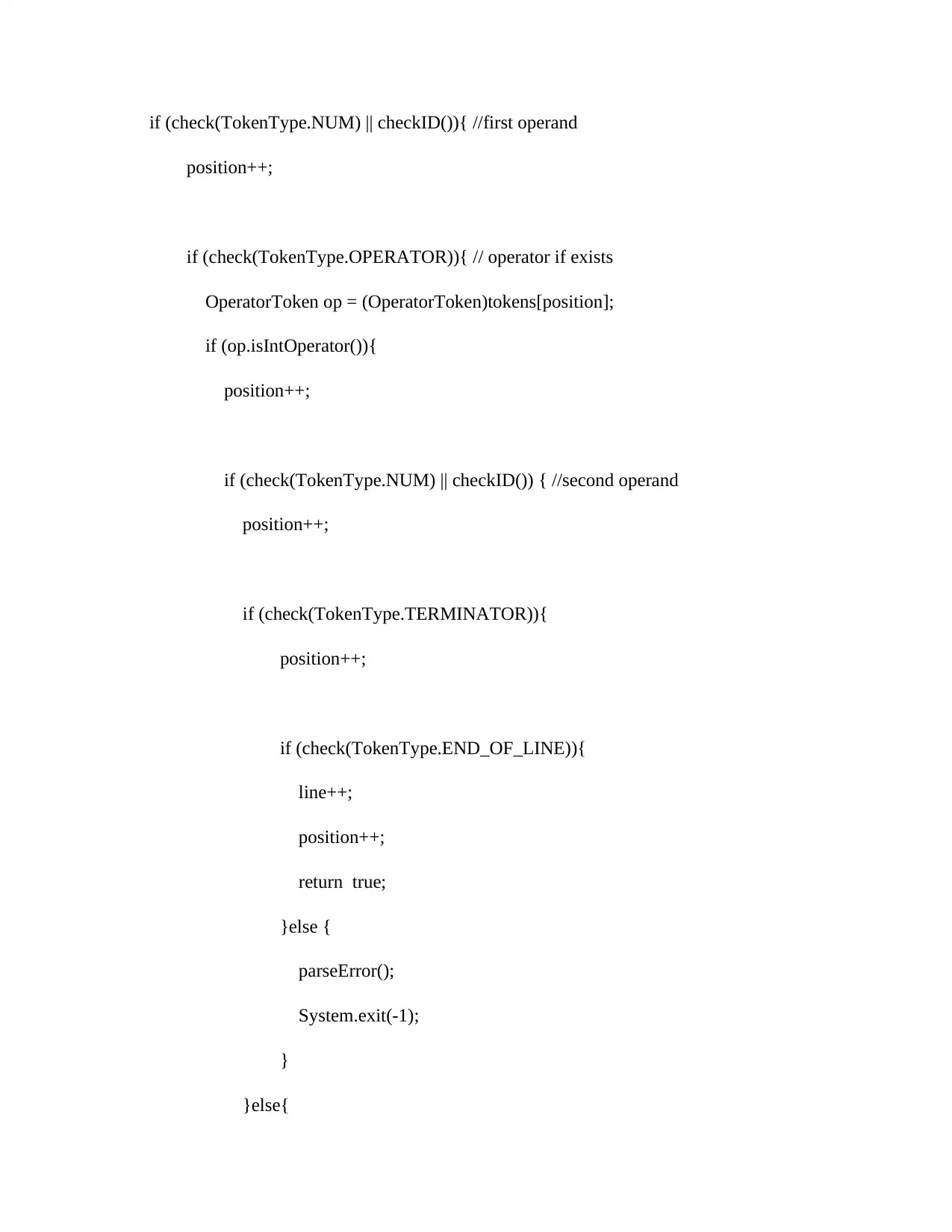
if (check(TokenType.NUM) || checkID()){ //first operand
position++;
if (check(TokenType.OPERATOR)){ // operator if exists
OperatorToken op = (OperatorToken)tokens[position];
if (op.isIntOperator()){
position++;
if (check(TokenType.NUM) || checkID()) { //second operand
position++;
if (check(TokenType.TERMINATOR)){
position++;
if (check(TokenType.END_OF_LINE)){
line++;
position++;
return true;
}else {
parseError();
System.exit(-1);
}
}else{
position++;
if (check(TokenType.OPERATOR)){ // operator if exists
OperatorToken op = (OperatorToken)tokens[position];
if (op.isIntOperator()){
position++;
if (check(TokenType.NUM) || checkID()) { //second operand
position++;
if (check(TokenType.TERMINATOR)){
position++;
if (check(TokenType.END_OF_LINE)){
line++;
position++;
return true;
}else {
parseError();
System.exit(-1);
}
}else{
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
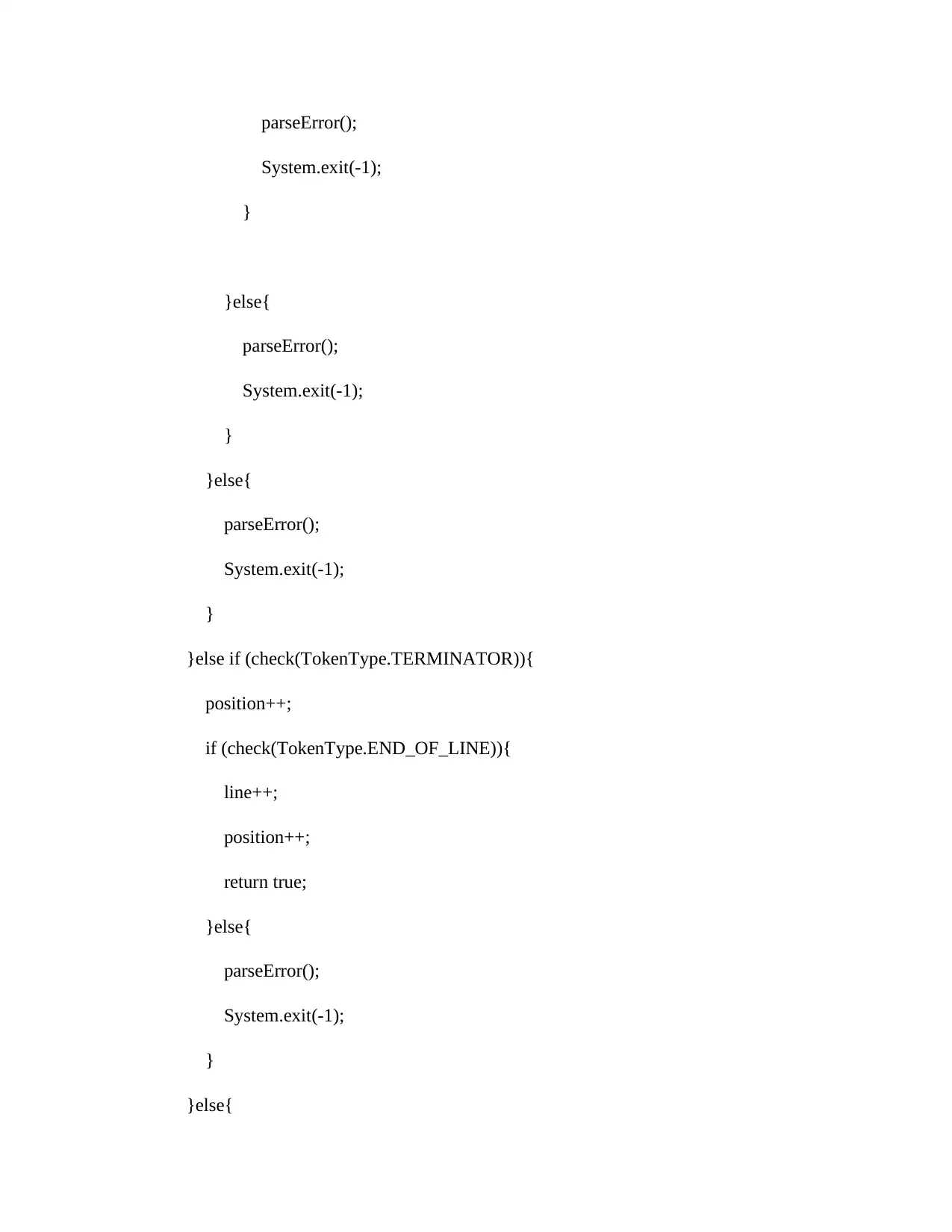
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else if (check(TokenType.TERMINATOR)){
position++;
if (check(TokenType.END_OF_LINE)){
line++;
position++;
return true;
}else{
parseError();
System.exit(-1);
}
}else{
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else{
parseError();
System.exit(-1);
}
}else if (check(TokenType.TERMINATOR)){
position++;
if (check(TokenType.END_OF_LINE)){
line++;
position++;
return true;
}else{
parseError();
System.exit(-1);
}
}else{
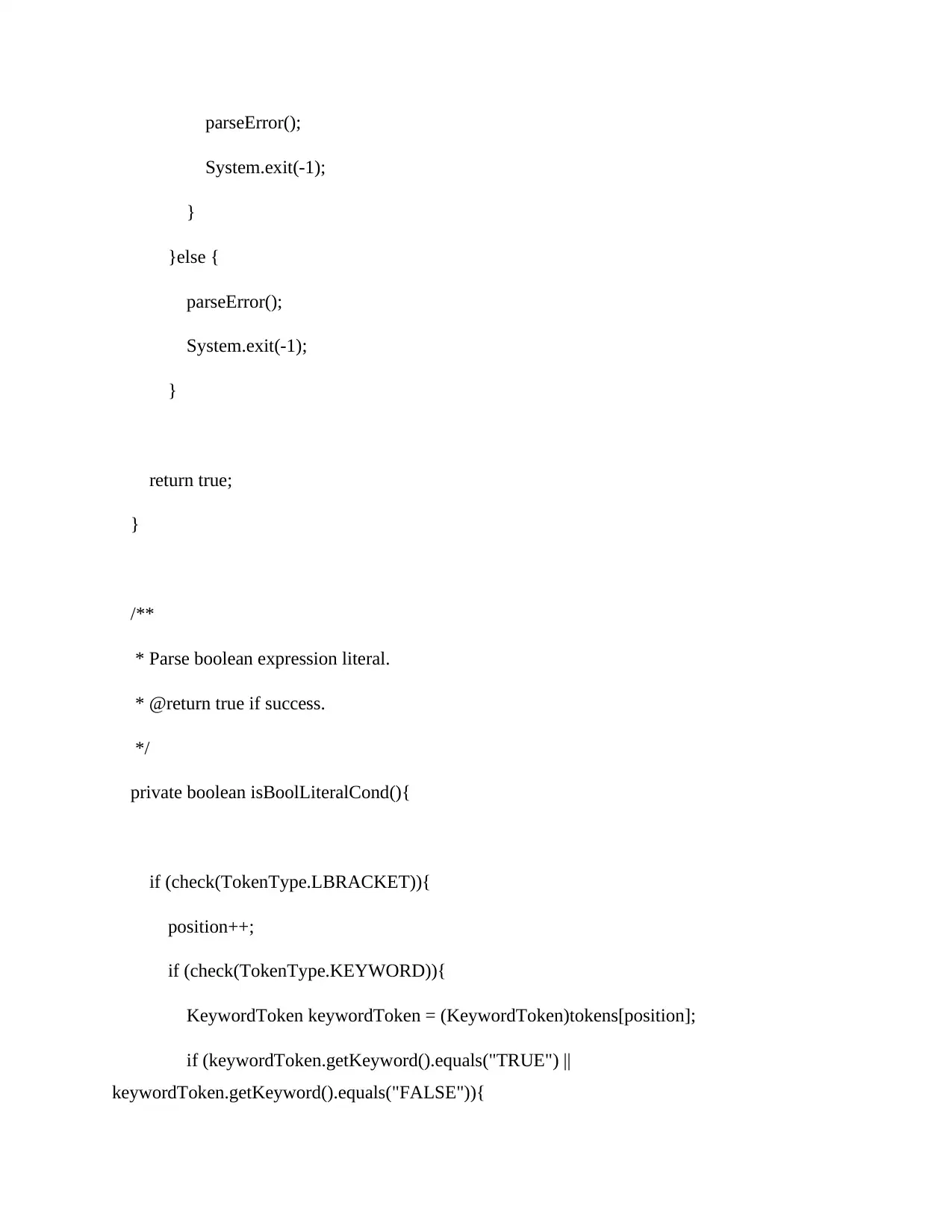
parseError();
System.exit(-1);
}
}else {
parseError();
System.exit(-1);
}
return true;
}
/**
* Parse boolean expression literal.
* @return true if success.
*/
private boolean isBoolLiteralCond(){
if (check(TokenType.LBRACKET)){
position++;
if (check(TokenType.KEYWORD)){
KeywordToken keywordToken = (KeywordToken)tokens[position];
if (keywordToken.getKeyword().equals("TRUE") ||
keywordToken.getKeyword().equals("FALSE")){
System.exit(-1);
}
}else {
parseError();
System.exit(-1);
}
return true;
}
/**
* Parse boolean expression literal.
* @return true if success.
*/
private boolean isBoolLiteralCond(){
if (check(TokenType.LBRACKET)){
position++;
if (check(TokenType.KEYWORD)){
KeywordToken keywordToken = (KeywordToken)tokens[position];
if (keywordToken.getKeyword().equals("TRUE") ||
keywordToken.getKeyword().equals("FALSE")){
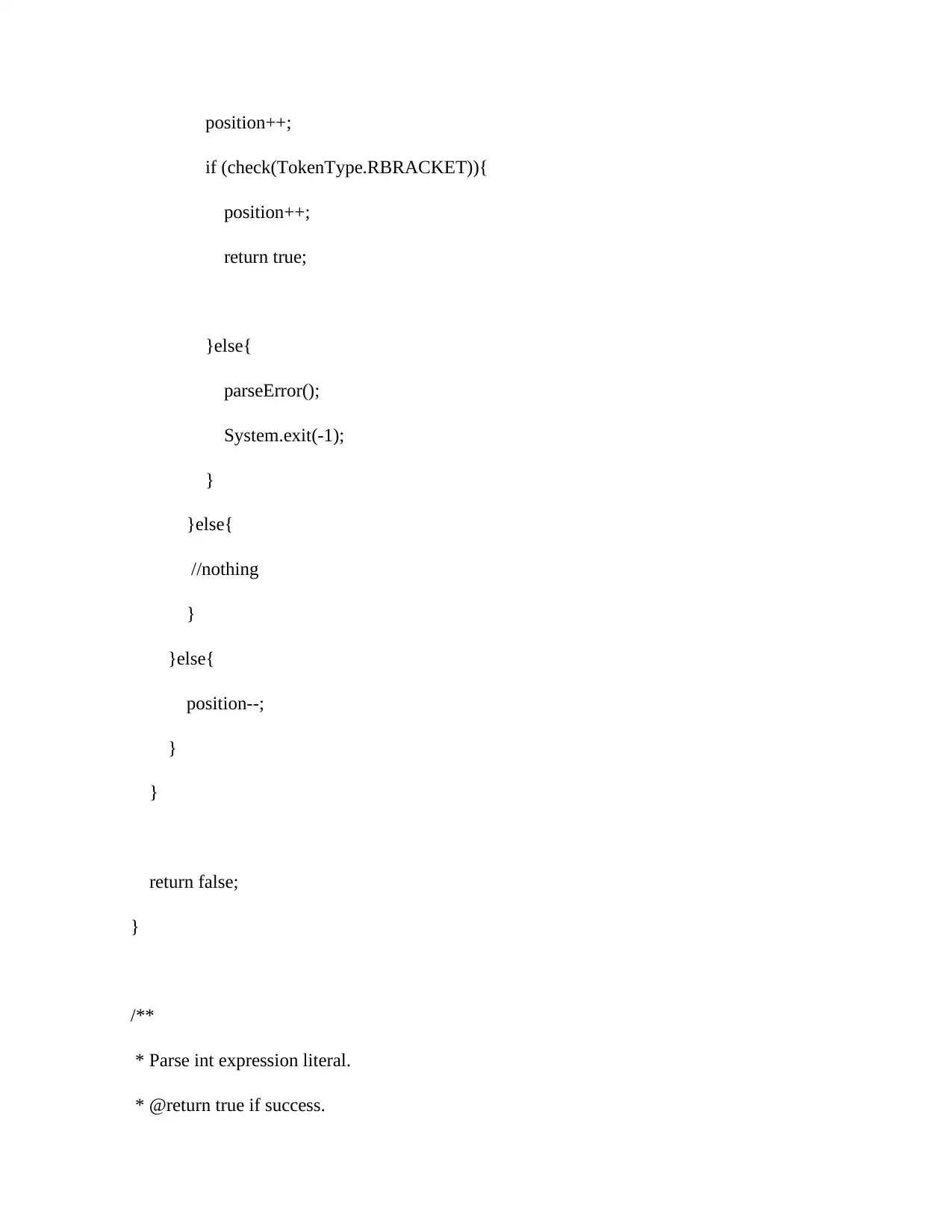
position++;
if (check(TokenType.RBRACKET)){
position++;
return true;
}else{
parseError();
System.exit(-1);
}
}else{
//nothing
}
}else{
position--;
}
}
return false;
}
/**
* Parse int expression literal.
* @return true if success.
if (check(TokenType.RBRACKET)){
position++;
return true;
}else{
parseError();
System.exit(-1);
}
}else{
//nothing
}
}else{
position--;
}
}
return false;
}
/**
* Parse int expression literal.
* @return true if success.
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
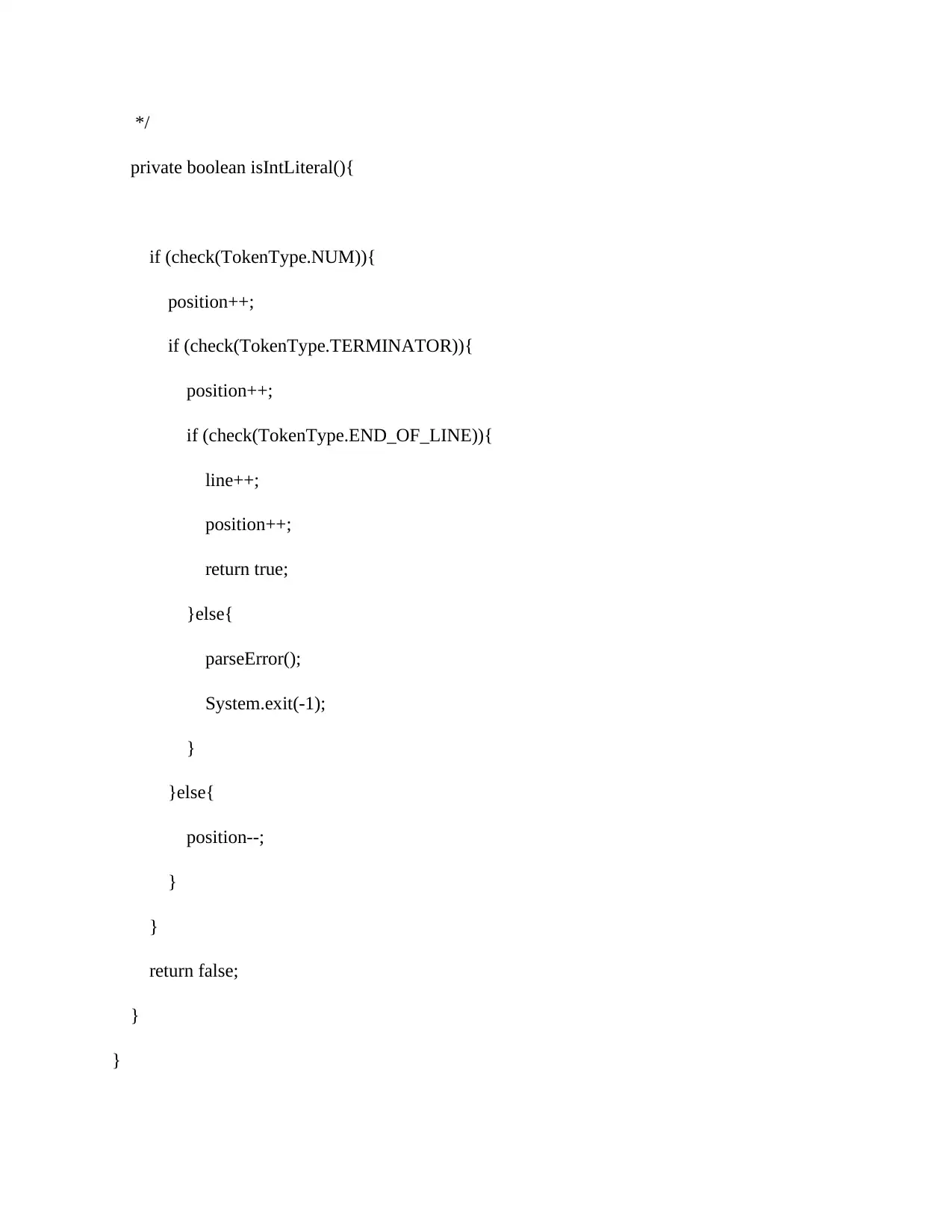
*/
private boolean isIntLiteral(){
if (check(TokenType.NUM)){
position++;
if (check(TokenType.TERMINATOR)){
position++;
if (check(TokenType.END_OF_LINE)){
line++;
position++;
return true;
}else{
parseError();
System.exit(-1);
}
}else{
position--;
}
}
return false;
}
}
private boolean isIntLiteral(){
if (check(TokenType.NUM)){
position++;
if (check(TokenType.TERMINATOR)){
position++;
if (check(TokenType.END_OF_LINE)){
line++;
position++;
return true;
}else{
parseError();
System.exit(-1);
}
}else{
position--;
}
}
return false;
}
}
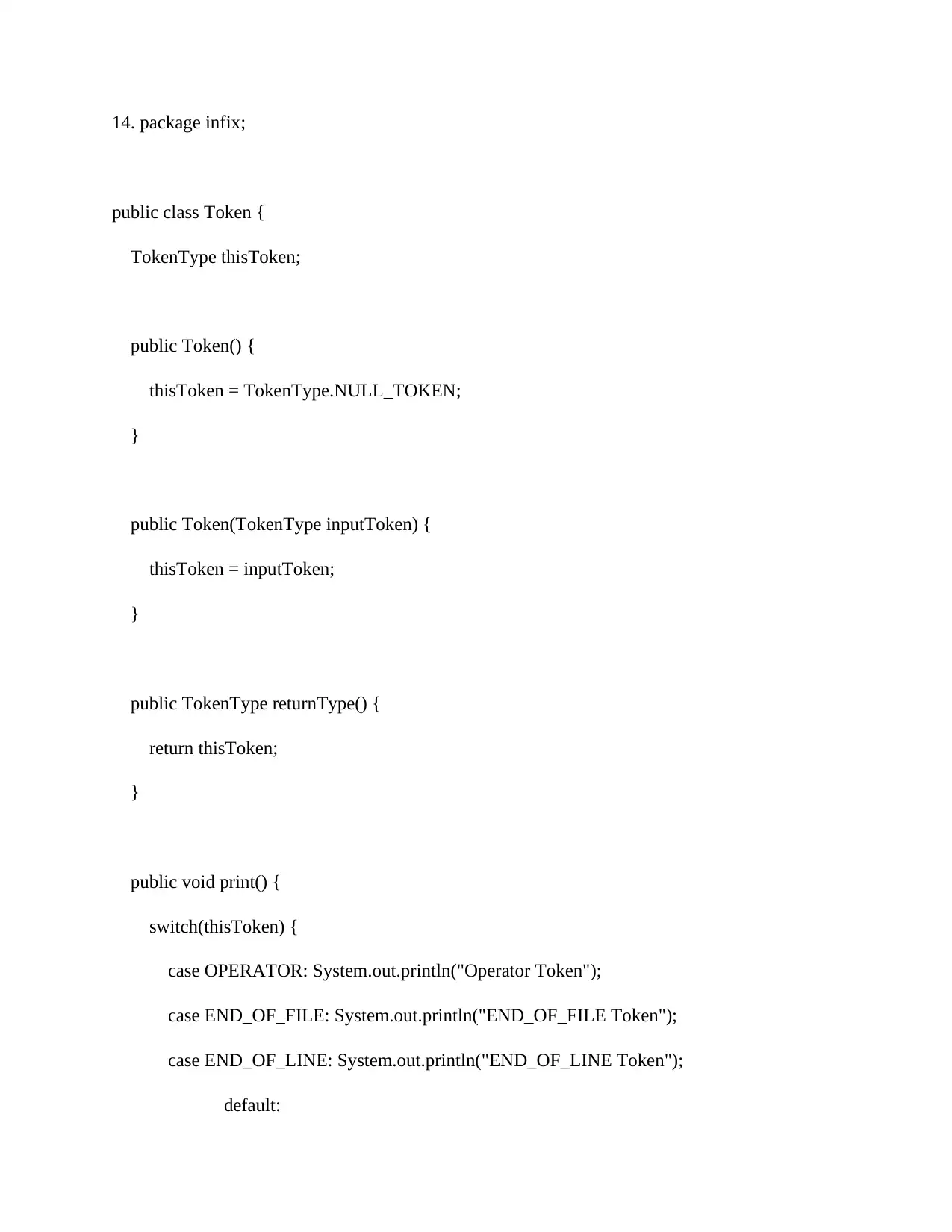
14. package infix;
public class Token {
TokenType thisToken;
public Token() {
thisToken = TokenType.NULL_TOKEN;
}
public Token(TokenType inputToken) {
thisToken = inputToken;
}
public TokenType returnType() {
return thisToken;
}
public void print() {
switch(thisToken) {
case OPERATOR: System.out.println("Operator Token");
case END_OF_FILE: System.out.println("END_OF_FILE Token");
case END_OF_LINE: System.out.println("END_OF_LINE Token");
default:
public class Token {
TokenType thisToken;
public Token() {
thisToken = TokenType.NULL_TOKEN;
}
public Token(TokenType inputToken) {
thisToken = inputToken;
}
public TokenType returnType() {
return thisToken;
}
public void print() {
switch(thisToken) {
case OPERATOR: System.out.println("Operator Token");
case END_OF_FILE: System.out.println("END_OF_FILE Token");
case END_OF_LINE: System.out.println("END_OF_LINE Token");
default:
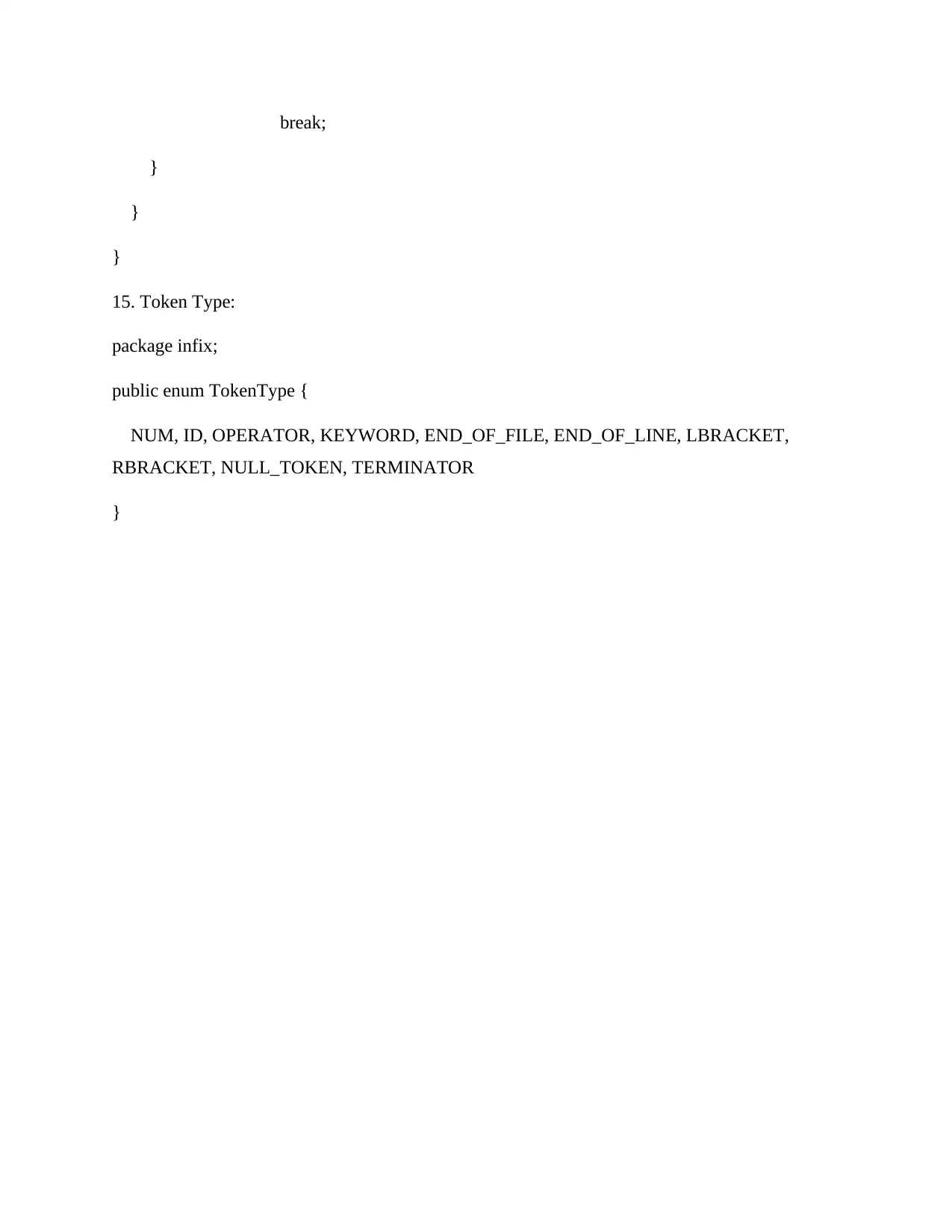
break;
}
}
}
15. Token Type:
package infix;
public enum TokenType {
NUM, ID, OPERATOR, KEYWORD, END_OF_FILE, END_OF_LINE, LBRACKET,
RBRACKET, NULL_TOKEN, TERMINATOR
}
}
}
}
15. Token Type:
package infix;
public enum TokenType {
NUM, ID, OPERATOR, KEYWORD, END_OF_FILE, END_OF_LINE, LBRACKET,
RBRACKET, NULL_TOKEN, TERMINATOR
}
1 out of 88
Related Documents
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
 +13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024  |  Zucol Services PVT LTD  |  All rights reserved.