NIM Game Playing Interface in Java
VerifiedAdded on  2023/06/11
|8
|2250
|180
AI Summary
This Java code implements the NIM game where players take turns to remove stones from a pile. The player who removes the last stone wins. The code includes a playing interface for both human and computer moves.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
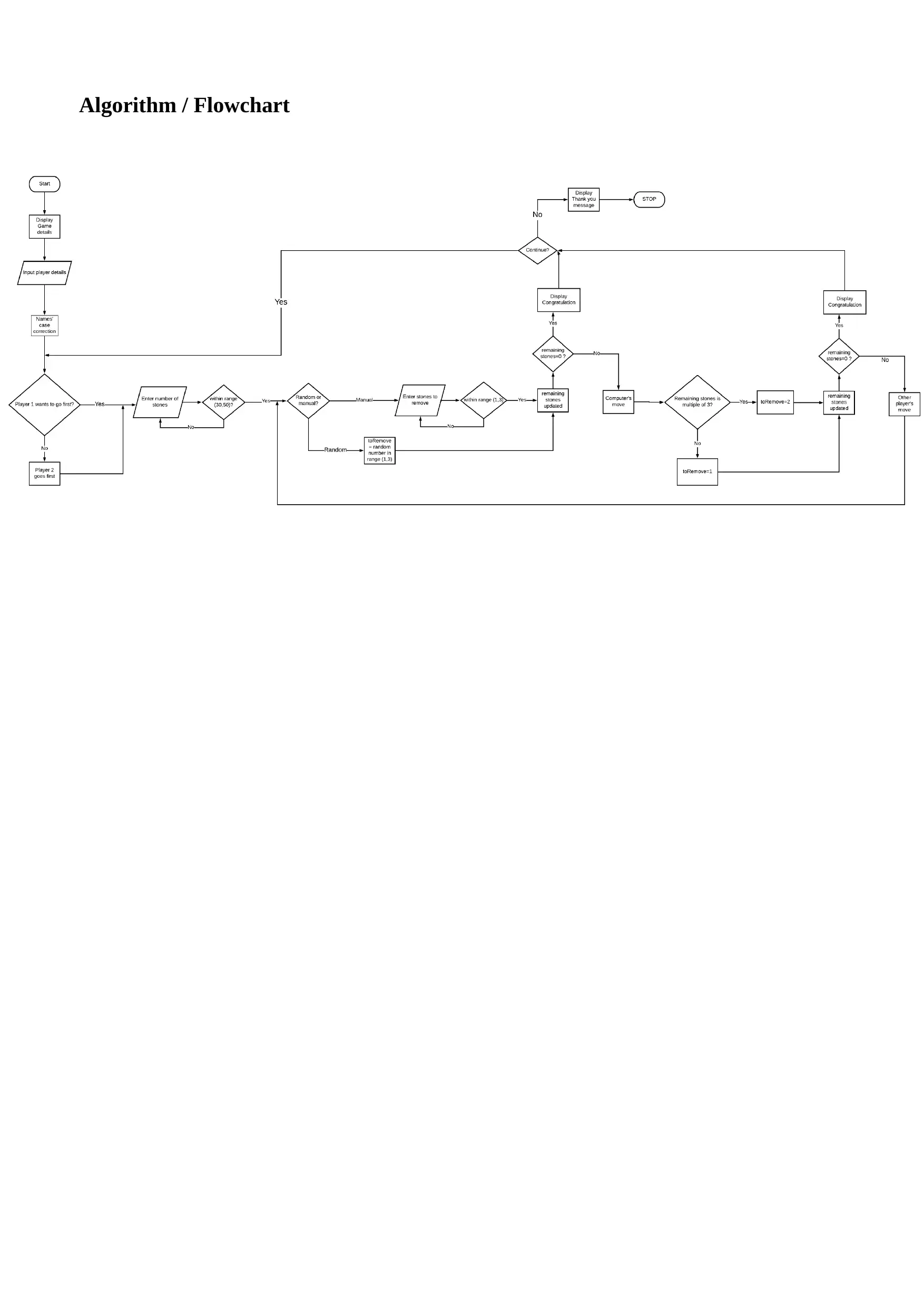
Algorithm / Flowchart
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
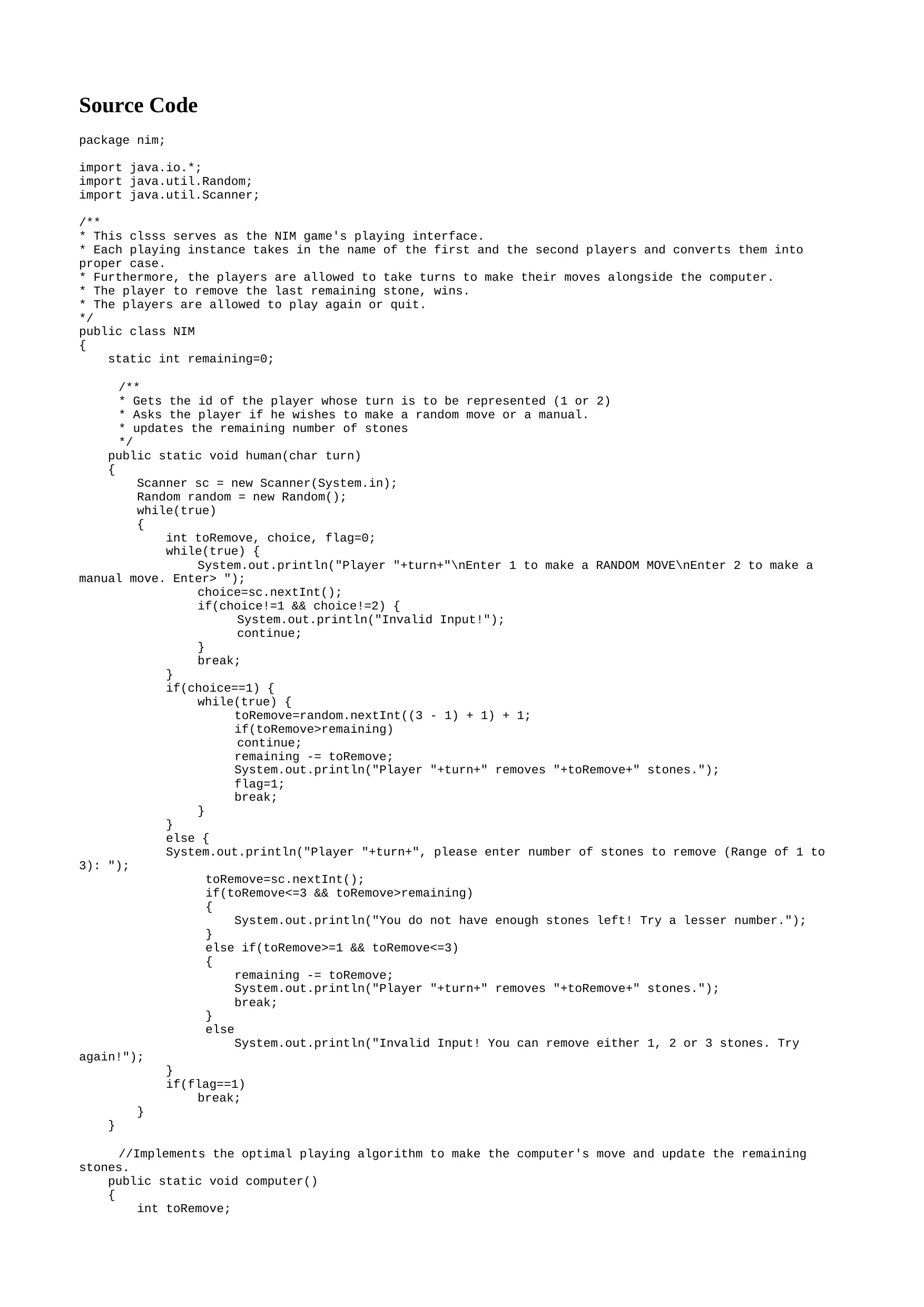
Source Code
package nim;
import java.io.*;
import java.util.Random;
import java.util.Scanner;
/**
* This clsss serves as the NIM game's playing interface.
* Each playing instance takes in the name of the first and the second players and converts them into
proper case.
* Furthermore, the players are allowed to take turns to make their moves alongside the computer.
* The player to remove the last remaining stone, wins.
* The players are allowed to play again or quit.
*/
public class NIM
{
static int remaining=0;
/**
* Gets the id of the player whose turn is to be represented (1 or 2)
* Asks the player if he wishes to make a random move or a manual.
* updates the remaining number of stones
*/
public static void human(char turn)
{
Scanner sc = new Scanner(System.in);
Random random = new Random();
while(true)
{
int toRemove, choice, flag=0;
while(true) {
System.out.println("Player "+turn+"\nEnter 1 to make a RANDOM MOVE\nEnter 2 to make a
manual move. Enter> ");
choice=sc.nextInt();
if(choice!=1 && choice!=2) {
System.out.println("Invalid Input!");
continue;
}
break;
}
if(choice==1) {
while(true) {
toRemove=random.nextInt((3 - 1) + 1) + 1;
if(toRemove>remaining)
continue;
remaining -= toRemove;
System.out.println("Player "+turn+" removes "+toRemove+" stones.");
flag=1;
break;
}
}
else {
System.out.println("Player "+turn+", please enter number of stones to remove (Range of 1 to
3): ");
toRemove=sc.nextInt();
if(toRemove<=3 && toRemove>remaining)
{
System.out.println("You do not have enough stones left! Try a lesser number.");
}
else if(toRemove>=1 && toRemove<=3)
{
remaining -= toRemove;
System.out.println("Player "+turn+" removes "+toRemove+" stones.");
break;
}
else
System.out.println("Invalid Input! You can remove either 1, 2 or 3 stones. Try
again!");
}
if(flag==1)
break;
}
}
//Implements the optimal playing algorithm to make the computer's move and update the remaining
stones.
public static void computer()
{
int toRemove;
package nim;
import java.io.*;
import java.util.Random;
import java.util.Scanner;
/**
* This clsss serves as the NIM game's playing interface.
* Each playing instance takes in the name of the first and the second players and converts them into
proper case.
* Furthermore, the players are allowed to take turns to make their moves alongside the computer.
* The player to remove the last remaining stone, wins.
* The players are allowed to play again or quit.
*/
public class NIM
{
static int remaining=0;
/**
* Gets the id of the player whose turn is to be represented (1 or 2)
* Asks the player if he wishes to make a random move or a manual.
* updates the remaining number of stones
*/
public static void human(char turn)
{
Scanner sc = new Scanner(System.in);
Random random = new Random();
while(true)
{
int toRemove, choice, flag=0;
while(true) {
System.out.println("Player "+turn+"\nEnter 1 to make a RANDOM MOVE\nEnter 2 to make a
manual move. Enter> ");
choice=sc.nextInt();
if(choice!=1 && choice!=2) {
System.out.println("Invalid Input!");
continue;
}
break;
}
if(choice==1) {
while(true) {
toRemove=random.nextInt((3 - 1) + 1) + 1;
if(toRemove>remaining)
continue;
remaining -= toRemove;
System.out.println("Player "+turn+" removes "+toRemove+" stones.");
flag=1;
break;
}
}
else {
System.out.println("Player "+turn+", please enter number of stones to remove (Range of 1 to
3): ");
toRemove=sc.nextInt();
if(toRemove<=3 && toRemove>remaining)
{
System.out.println("You do not have enough stones left! Try a lesser number.");
}
else if(toRemove>=1 && toRemove<=3)
{
remaining -= toRemove;
System.out.println("Player "+turn+" removes "+toRemove+" stones.");
break;
}
else
System.out.println("Invalid Input! You can remove either 1, 2 or 3 stones. Try
again!");
}
if(flag==1)
break;
}
}
//Implements the optimal playing algorithm to make the computer's move and update the remaining
stones.
public static void computer()
{
int toRemove;
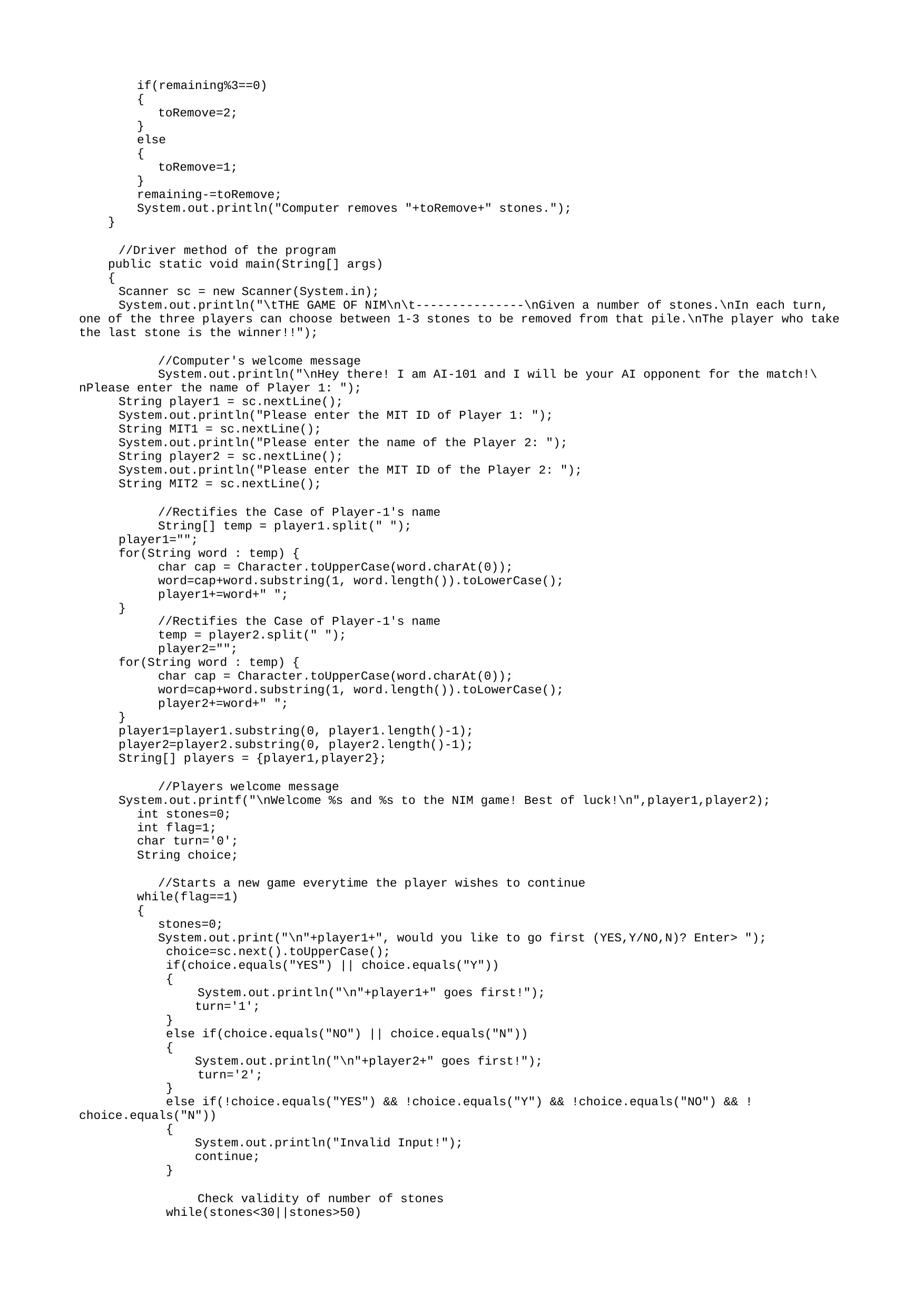
if(remaining%3==0)
{
toRemove=2;
}
else
{
toRemove=1;
}
remaining-=toRemove;
System.out.println("Computer removes "+toRemove+" stones.");
}
//Driver method of the program
public static void main(String[] args)
{
Scanner sc = new Scanner(System.in);
System.out.println("\tTHE GAME OF NIM\n\t---------------\nGiven a number of stones.\nIn each turn,
one of the three players can choose between 1-3 stones to be removed from that pile.\nThe player who take
the last stone is the winner!!");
//Computer's welcome message
System.out.println("\nHey there! I am AI-101 and I will be your AI opponent for the match!\
nPlease enter the name of Player 1: ");
String player1 = sc.nextLine();
System.out.println("Please enter the MIT ID of Player 1: ");
String MIT1 = sc.nextLine();
System.out.println("Please enter the name of the Player 2: ");
String player2 = sc.nextLine();
System.out.println("Please enter the MIT ID of the Player 2: ");
String MIT2 = sc.nextLine();
//Rectifies the Case of Player-1's name
String[] temp = player1.split(" ");
player1="";
for(String word : temp) {
char cap = Character.toUpperCase(word.charAt(0));
word=cap+word.substring(1, word.length()).toLowerCase();
player1+=word+" ";
}
//Rectifies the Case of Player-1's name
temp = player2.split(" ");
player2="";
for(String word : temp) {
char cap = Character.toUpperCase(word.charAt(0));
word=cap+word.substring(1, word.length()).toLowerCase();
player2+=word+" ";
}
player1=player1.substring(0, player1.length()-1);
player2=player2.substring(0, player2.length()-1);
String[] players = {player1,player2};
//Players welcome message
System.out.printf("\nWelcome %s and %s to the NIM game! Best of luck!\n",player1,player2);
int stones=0;
int flag=1;
char turn='0';
String choice;
//Starts a new game everytime the player wishes to continue
while(flag==1)
{
stones=0;
System.out.print("\n"+player1+", would you like to go first (YES,Y/NO,N)? Enter> ");
choice=sc.next().toUpperCase();
if(choice.equals("YES") || choice.equals("Y"))
{
System.out.println("\n"+player1+" goes first!");
turn='1';
}
else if(choice.equals("NO") || choice.equals("N"))
{
System.out.println("\n"+player2+" goes first!");
turn='2';
}
else if(!choice.equals("YES") && !choice.equals("Y") && !choice.equals("NO") && !
choice.equals("N"))
{
System.out.println("Invalid Input!");
continue;
}
Check validity of number of stones
while(stones<30||stones>50)
{
toRemove=2;
}
else
{
toRemove=1;
}
remaining-=toRemove;
System.out.println("Computer removes "+toRemove+" stones.");
}
//Driver method of the program
public static void main(String[] args)
{
Scanner sc = new Scanner(System.in);
System.out.println("\tTHE GAME OF NIM\n\t---------------\nGiven a number of stones.\nIn each turn,
one of the three players can choose between 1-3 stones to be removed from that pile.\nThe player who take
the last stone is the winner!!");
//Computer's welcome message
System.out.println("\nHey there! I am AI-101 and I will be your AI opponent for the match!\
nPlease enter the name of Player 1: ");
String player1 = sc.nextLine();
System.out.println("Please enter the MIT ID of Player 1: ");
String MIT1 = sc.nextLine();
System.out.println("Please enter the name of the Player 2: ");
String player2 = sc.nextLine();
System.out.println("Please enter the MIT ID of the Player 2: ");
String MIT2 = sc.nextLine();
//Rectifies the Case of Player-1's name
String[] temp = player1.split(" ");
player1="";
for(String word : temp) {
char cap = Character.toUpperCase(word.charAt(0));
word=cap+word.substring(1, word.length()).toLowerCase();
player1+=word+" ";
}
//Rectifies the Case of Player-1's name
temp = player2.split(" ");
player2="";
for(String word : temp) {
char cap = Character.toUpperCase(word.charAt(0));
word=cap+word.substring(1, word.length()).toLowerCase();
player2+=word+" ";
}
player1=player1.substring(0, player1.length()-1);
player2=player2.substring(0, player2.length()-1);
String[] players = {player1,player2};
//Players welcome message
System.out.printf("\nWelcome %s and %s to the NIM game! Best of luck!\n",player1,player2);
int stones=0;
int flag=1;
char turn='0';
String choice;
//Starts a new game everytime the player wishes to continue
while(flag==1)
{
stones=0;
System.out.print("\n"+player1+", would you like to go first (YES,Y/NO,N)? Enter> ");
choice=sc.next().toUpperCase();
if(choice.equals("YES") || choice.equals("Y"))
{
System.out.println("\n"+player1+" goes first!");
turn='1';
}
else if(choice.equals("NO") || choice.equals("N"))
{
System.out.println("\n"+player2+" goes first!");
turn='2';
}
else if(!choice.equals("YES") && !choice.equals("Y") && !choice.equals("NO") && !
choice.equals("N"))
{
System.out.println("Invalid Input!");
continue;
}
Check validity of number of stones
while(stones<30||stones>50)
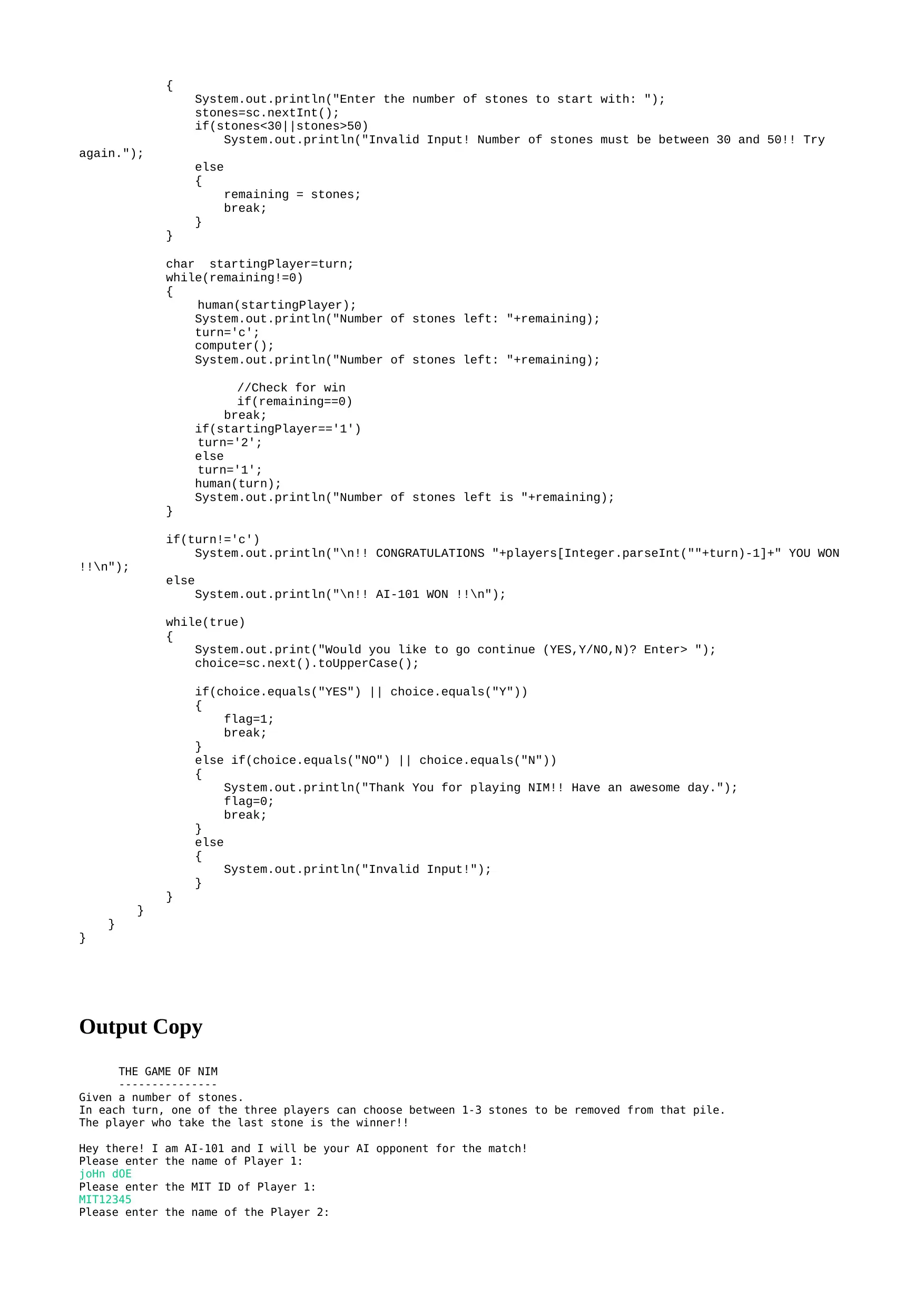
{
System.out.println("Enter the number of stones to start with: ");
stones=sc.nextInt();
if(stones<30||stones>50)
System.out.println("Invalid Input! Number of stones must be between 30 and 50!! Try
again.");
else
{
remaining = stones;
break;
}
}
char startingPlayer=turn;
while(remaining!=0)
{
human(startingPlayer);
System.out.println("Number of stones left: "+remaining);
turn='c';
computer();
System.out.println("Number of stones left: "+remaining);
//Check for win
if(remaining==0)
break;
if(startingPlayer=='1')
turn='2';
else
turn='1';
human(turn);
System.out.println("Number of stones left is "+remaining);
}
if(turn!='c')
System.out.println("\n!! CONGRATULATIONS "+players[Integer.parseInt(""+turn)-1]+" YOU WON
!!\n");
else
System.out.println("\n!! AI-101 WON !!\n");
while(true)
{
System.out.print("Would you like to go continue (YES,Y/NO,N)? Enter> ");
choice=sc.next().toUpperCase();
if(choice.equals("YES") || choice.equals("Y"))
{
flag=1;
break;
}
else if(choice.equals("NO") || choice.equals("N"))
{
System.out.println("Thank You for playing NIM!! Have an awesome day.");
flag=0;
break;
}
else
{
System.out.println("Invalid Input!");
}
}
}
}
}
Output Copy
THE GAME OF NIM
---------------
Given a number of stones.
In each turn, one of the three players can choose between 1-3 stones to be removed from that pile.
The player who take the last stone is the winner!!
Hey there! I am AI-101 and I will be your AI opponent for the match!
Please enter the name of Player 1:
joHn dOE
Please enter the MIT ID of Player 1:
MIT12345
Please enter the name of the Player 2:
System.out.println("Enter the number of stones to start with: ");
stones=sc.nextInt();
if(stones<30||stones>50)
System.out.println("Invalid Input! Number of stones must be between 30 and 50!! Try
again.");
else
{
remaining = stones;
break;
}
}
char startingPlayer=turn;
while(remaining!=0)
{
human(startingPlayer);
System.out.println("Number of stones left: "+remaining);
turn='c';
computer();
System.out.println("Number of stones left: "+remaining);
//Check for win
if(remaining==0)
break;
if(startingPlayer=='1')
turn='2';
else
turn='1';
human(turn);
System.out.println("Number of stones left is "+remaining);
}
if(turn!='c')
System.out.println("\n!! CONGRATULATIONS "+players[Integer.parseInt(""+turn)-1]+" YOU WON
!!\n");
else
System.out.println("\n!! AI-101 WON !!\n");
while(true)
{
System.out.print("Would you like to go continue (YES,Y/NO,N)? Enter> ");
choice=sc.next().toUpperCase();
if(choice.equals("YES") || choice.equals("Y"))
{
flag=1;
break;
}
else if(choice.equals("NO") || choice.equals("N"))
{
System.out.println("Thank You for playing NIM!! Have an awesome day.");
flag=0;
break;
}
else
{
System.out.println("Invalid Input!");
}
}
}
}
}
Output Copy
THE GAME OF NIM
---------------
Given a number of stones.
In each turn, one of the three players can choose between 1-3 stones to be removed from that pile.
The player who take the last stone is the winner!!
Hey there! I am AI-101 and I will be your AI opponent for the match!
Please enter the name of Player 1:
joHn dOE
Please enter the MIT ID of Player 1:
MIT12345
Please enter the name of the Player 2:
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
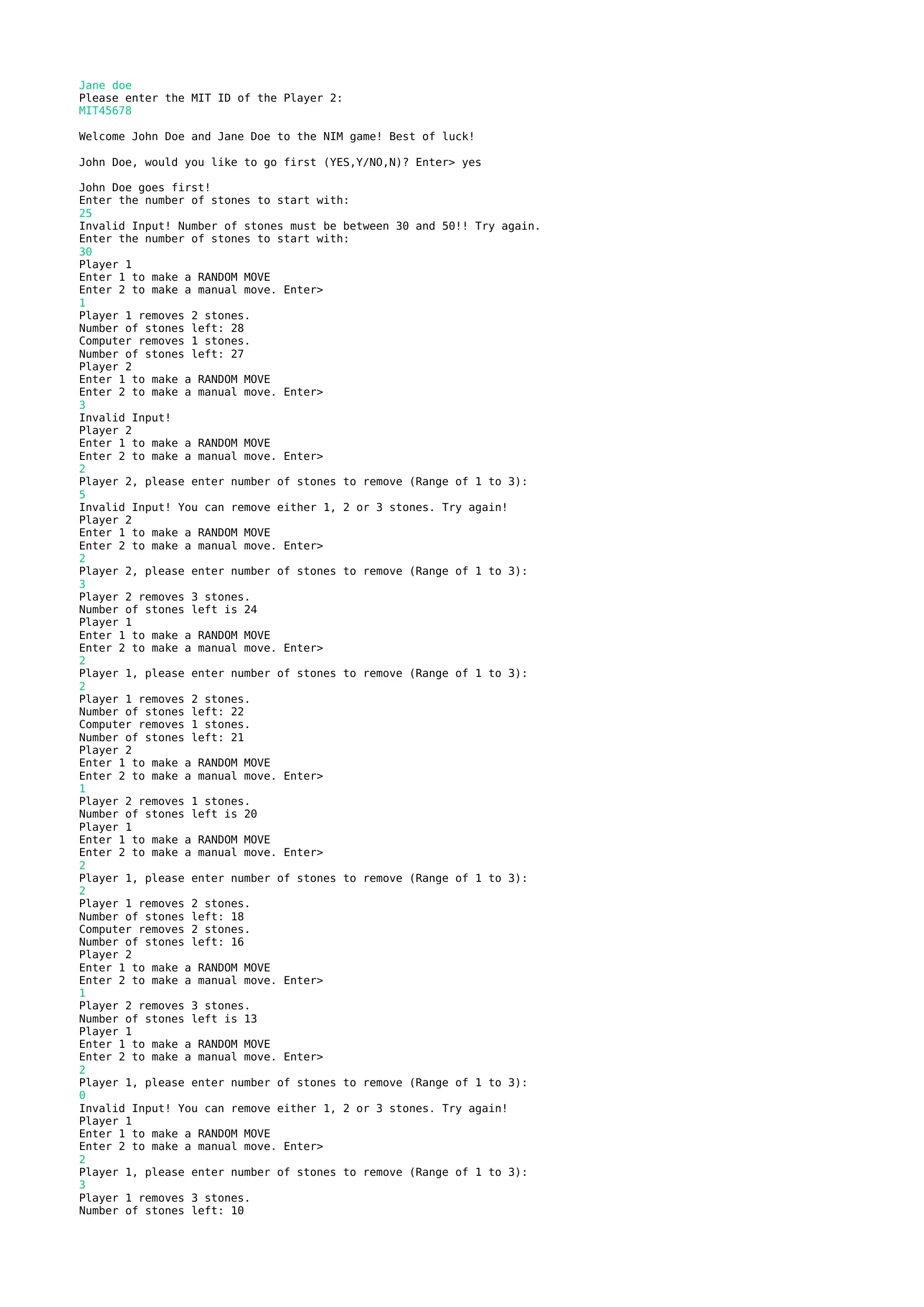
Jane doe
Please enter the MIT ID of the Player 2:
MIT45678
Welcome John Doe and Jane Doe to the NIM game! Best of luck!
John Doe, would you like to go first (YES,Y/NO,N)? Enter> yes
John Doe goes first!
Enter the number of stones to start with:
25
Invalid Input! Number of stones must be between 30 and 50!! Try again.
Enter the number of stones to start with:
30
Player 1
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
1
Player 1 removes 2 stones.
Number of stones left: 28
Computer removes 1 stones.
Number of stones left: 27
Player 2
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
3
Invalid Input!
Player 2
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
2
Player 2, please enter number of stones to remove (Range of 1 to 3):
5
Invalid Input! You can remove either 1, 2 or 3 stones. Try again!
Player 2
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
2
Player 2, please enter number of stones to remove (Range of 1 to 3):
3
Player 2 removes 3 stones.
Number of stones left is 24
Player 1
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
2
Player 1, please enter number of stones to remove (Range of 1 to 3):
2
Player 1 removes 2 stones.
Number of stones left: 22
Computer removes 1 stones.
Number of stones left: 21
Player 2
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
1
Player 2 removes 1 stones.
Number of stones left is 20
Player 1
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
2
Player 1, please enter number of stones to remove (Range of 1 to 3):
2
Player 1 removes 2 stones.
Number of stones left: 18
Computer removes 2 stones.
Number of stones left: 16
Player 2
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
1
Player 2 removes 3 stones.
Number of stones left is 13
Player 1
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
2
Player 1, please enter number of stones to remove (Range of 1 to 3):
0
Invalid Input! You can remove either 1, 2 or 3 stones. Try again!
Player 1
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
2
Player 1, please enter number of stones to remove (Range of 1 to 3):
3
Player 1 removes 3 stones.
Number of stones left: 10
Please enter the MIT ID of the Player 2:
MIT45678
Welcome John Doe and Jane Doe to the NIM game! Best of luck!
John Doe, would you like to go first (YES,Y/NO,N)? Enter> yes
John Doe goes first!
Enter the number of stones to start with:
25
Invalid Input! Number of stones must be between 30 and 50!! Try again.
Enter the number of stones to start with:
30
Player 1
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
1
Player 1 removes 2 stones.
Number of stones left: 28
Computer removes 1 stones.
Number of stones left: 27
Player 2
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
3
Invalid Input!
Player 2
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
2
Player 2, please enter number of stones to remove (Range of 1 to 3):
5
Invalid Input! You can remove either 1, 2 or 3 stones. Try again!
Player 2
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
2
Player 2, please enter number of stones to remove (Range of 1 to 3):
3
Player 2 removes 3 stones.
Number of stones left is 24
Player 1
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
2
Player 1, please enter number of stones to remove (Range of 1 to 3):
2
Player 1 removes 2 stones.
Number of stones left: 22
Computer removes 1 stones.
Number of stones left: 21
Player 2
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
1
Player 2 removes 1 stones.
Number of stones left is 20
Player 1
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
2
Player 1, please enter number of stones to remove (Range of 1 to 3):
2
Player 1 removes 2 stones.
Number of stones left: 18
Computer removes 2 stones.
Number of stones left: 16
Player 2
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
1
Player 2 removes 3 stones.
Number of stones left is 13
Player 1
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
2
Player 1, please enter number of stones to remove (Range of 1 to 3):
0
Invalid Input! You can remove either 1, 2 or 3 stones. Try again!
Player 1
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
2
Player 1, please enter number of stones to remove (Range of 1 to 3):
3
Player 1 removes 3 stones.
Number of stones left: 10
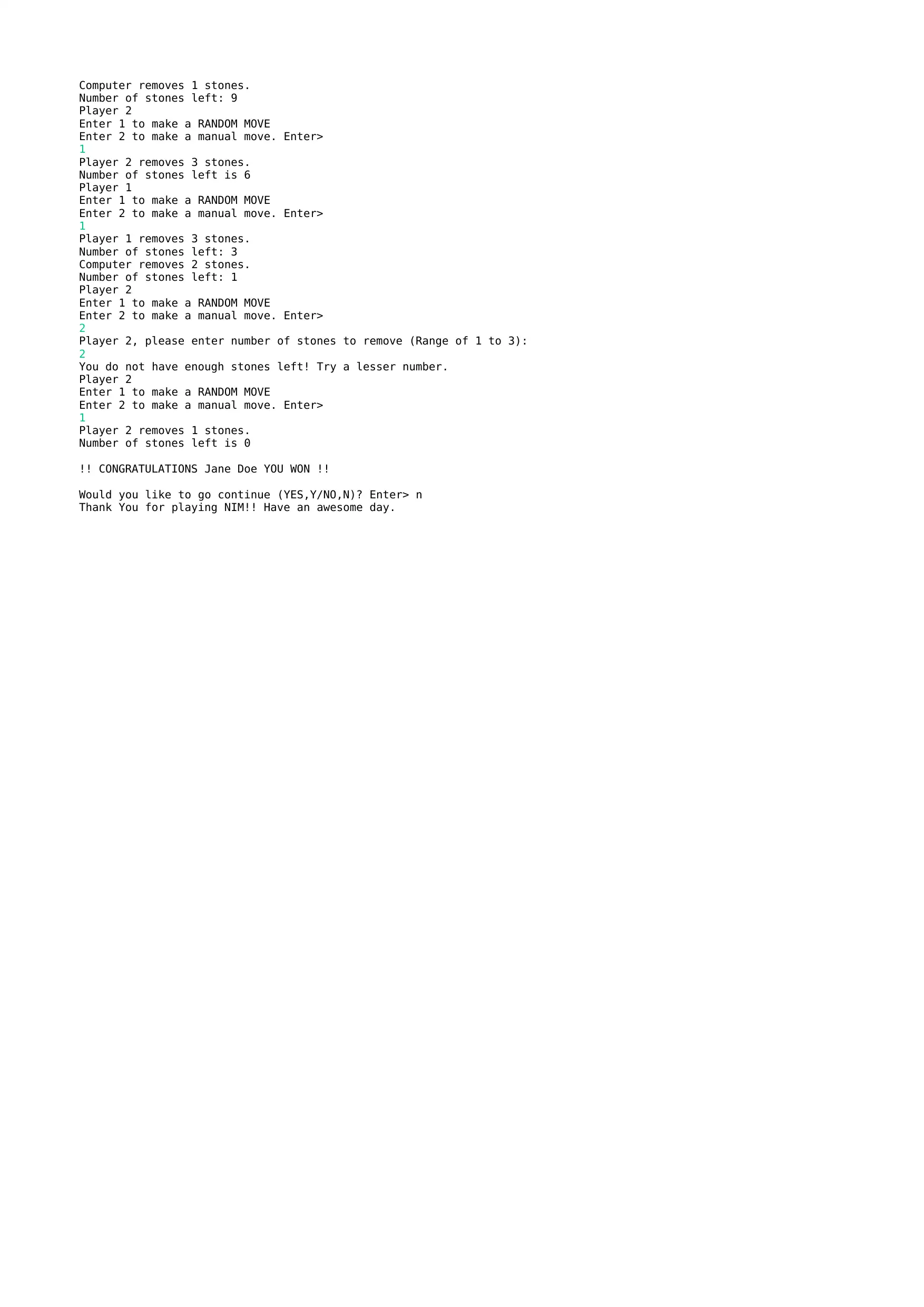
Computer removes 1 stones.
Number of stones left: 9
Player 2
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
1
Player 2 removes 3 stones.
Number of stones left is 6
Player 1
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
1
Player 1 removes 3 stones.
Number of stones left: 3
Computer removes 2 stones.
Number of stones left: 1
Player 2
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
2
Player 2, please enter number of stones to remove (Range of 1 to 3):
2
You do not have enough stones left! Try a lesser number.
Player 2
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
1
Player 2 removes 1 stones.
Number of stones left is 0
!! CONGRATULATIONS Jane Doe YOU WON !!
Would you like to go continue (YES,Y/NO,N)? Enter> n
Thank You for playing NIM!! Have an awesome day.
Number of stones left: 9
Player 2
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
1
Player 2 removes 3 stones.
Number of stones left is 6
Player 1
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
1
Player 1 removes 3 stones.
Number of stones left: 3
Computer removes 2 stones.
Number of stones left: 1
Player 2
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
2
Player 2, please enter number of stones to remove (Range of 1 to 3):
2
You do not have enough stones left! Try a lesser number.
Player 2
Enter 1 to make a RANDOM MOVE
Enter 2 to make a manual move. Enter>
1
Player 2 removes 1 stones.
Number of stones left is 0
!! CONGRATULATIONS Jane Doe YOU WON !!
Would you like to go continue (YES,Y/NO,N)? Enter> n
Thank You for playing NIM!! Have an awesome day.
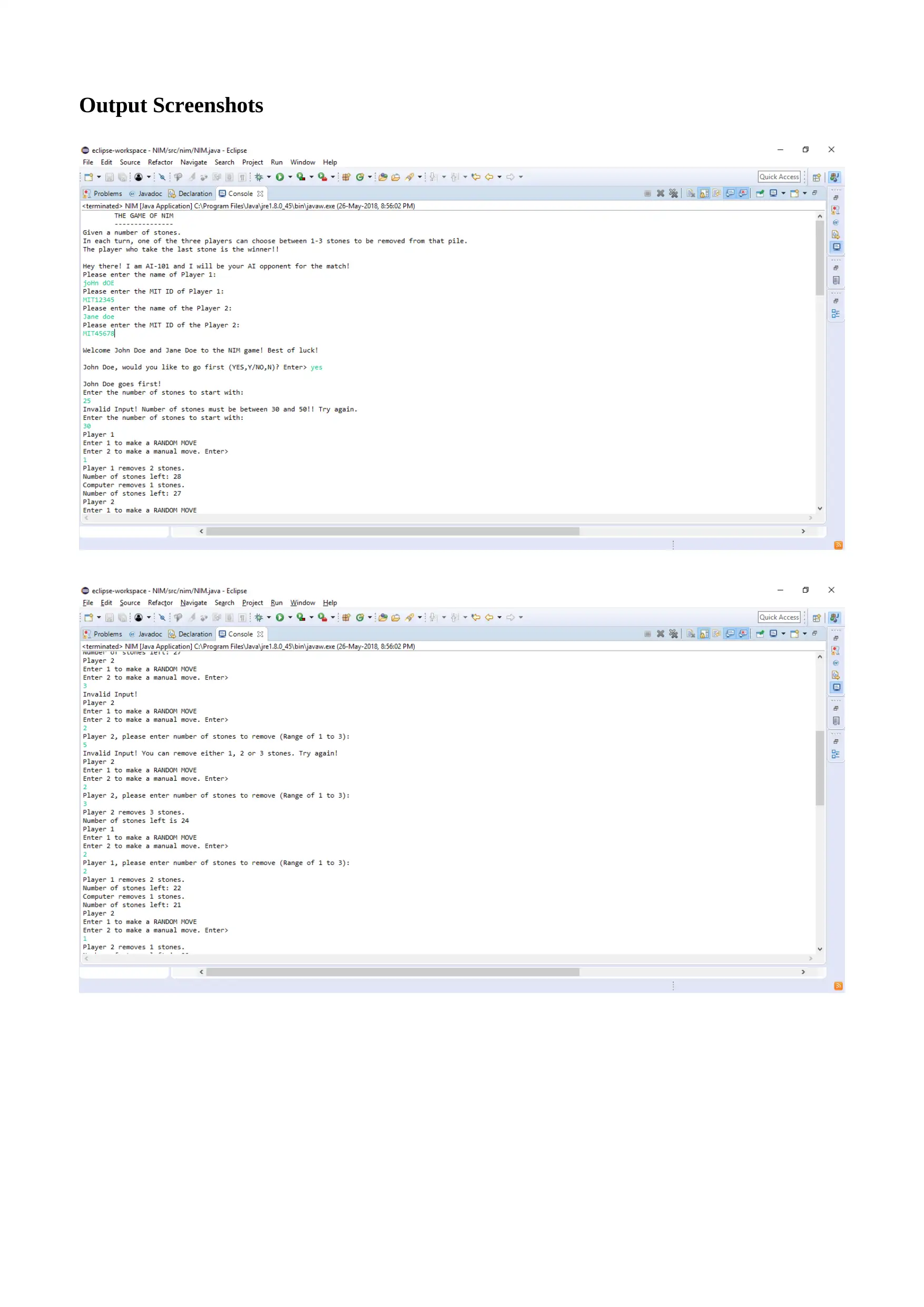
Output Screenshots
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
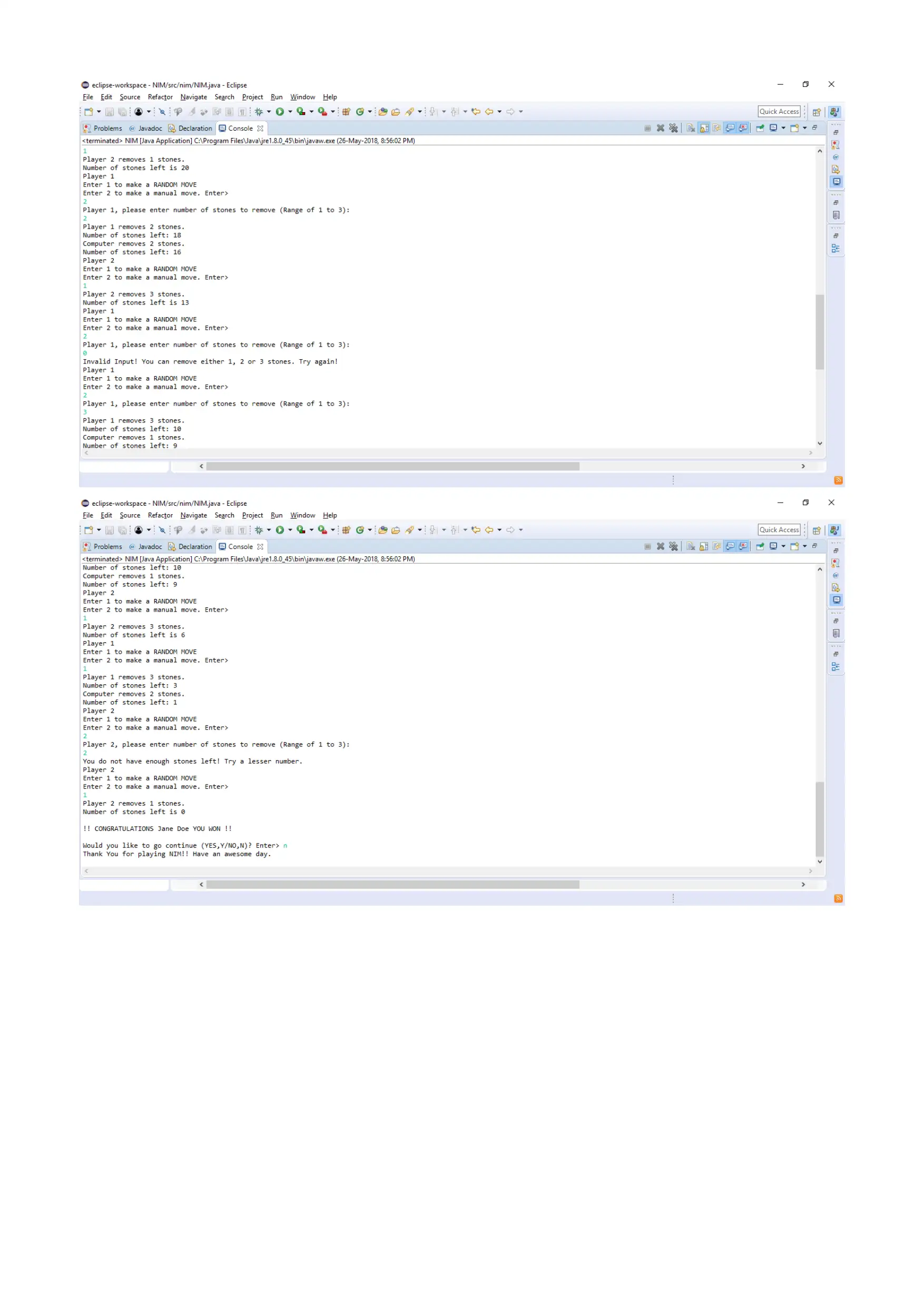
1 out of 8
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
 +13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024  |  Zucol Services PVT LTD  |  All rights reserved.