Object Oriented Programming using Java
VerifiedAdded on  2023/04/10
|14
|585
|389
AI Summary
This document provides information about Object Oriented Programming using Java. It includes code examples and explanations of concepts. The document also includes solved assignments and essays related to the topic. It is a valuable resource for students studying programming and Java.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
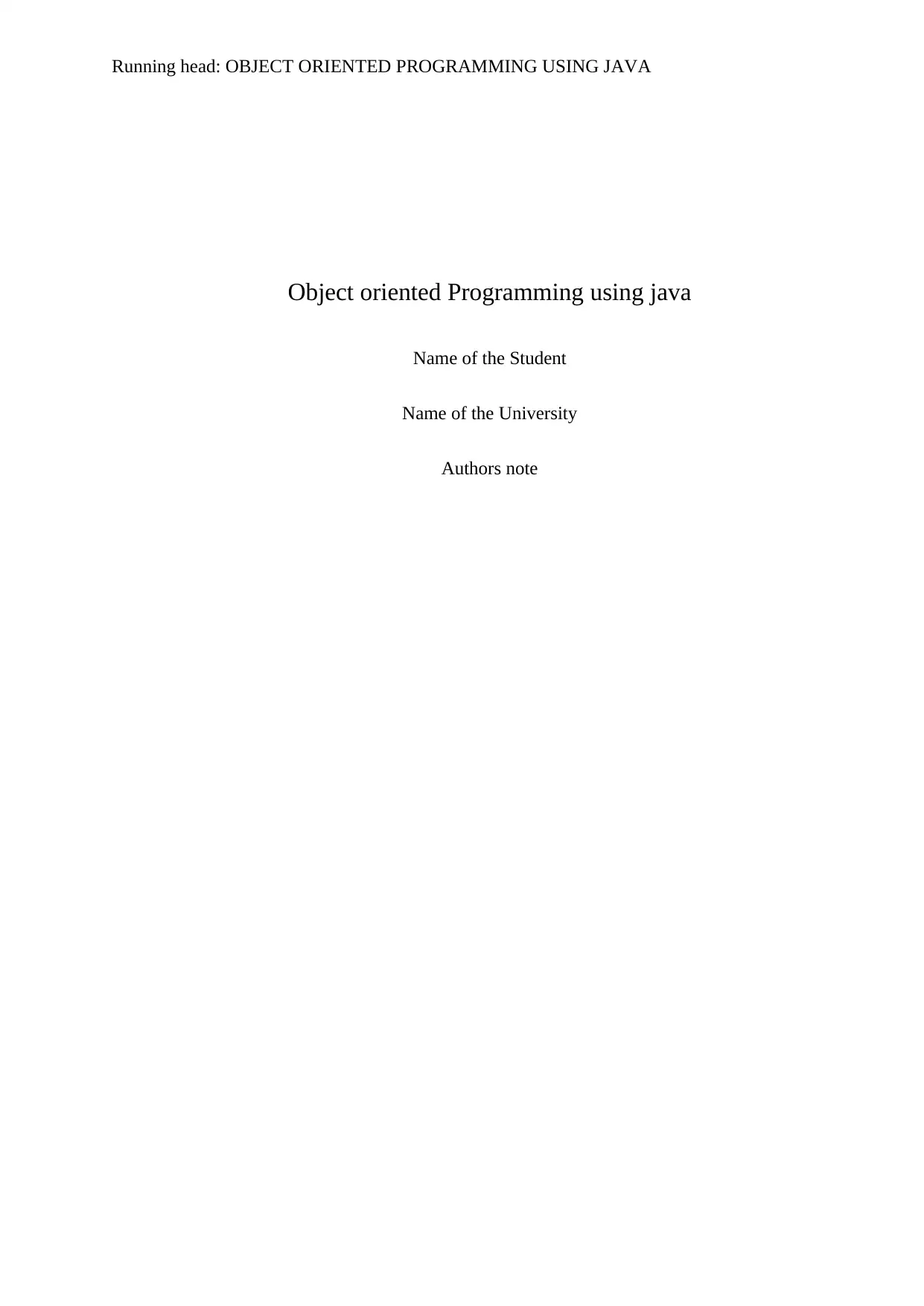
Running head: OBJECT ORIENTED PROGRAMMING USING JAVA
Object oriented Programming using java
Name of the Student
Name of the University
Authors note
Object oriented Programming using java
Name of the Student
Name of the University
Authors note
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
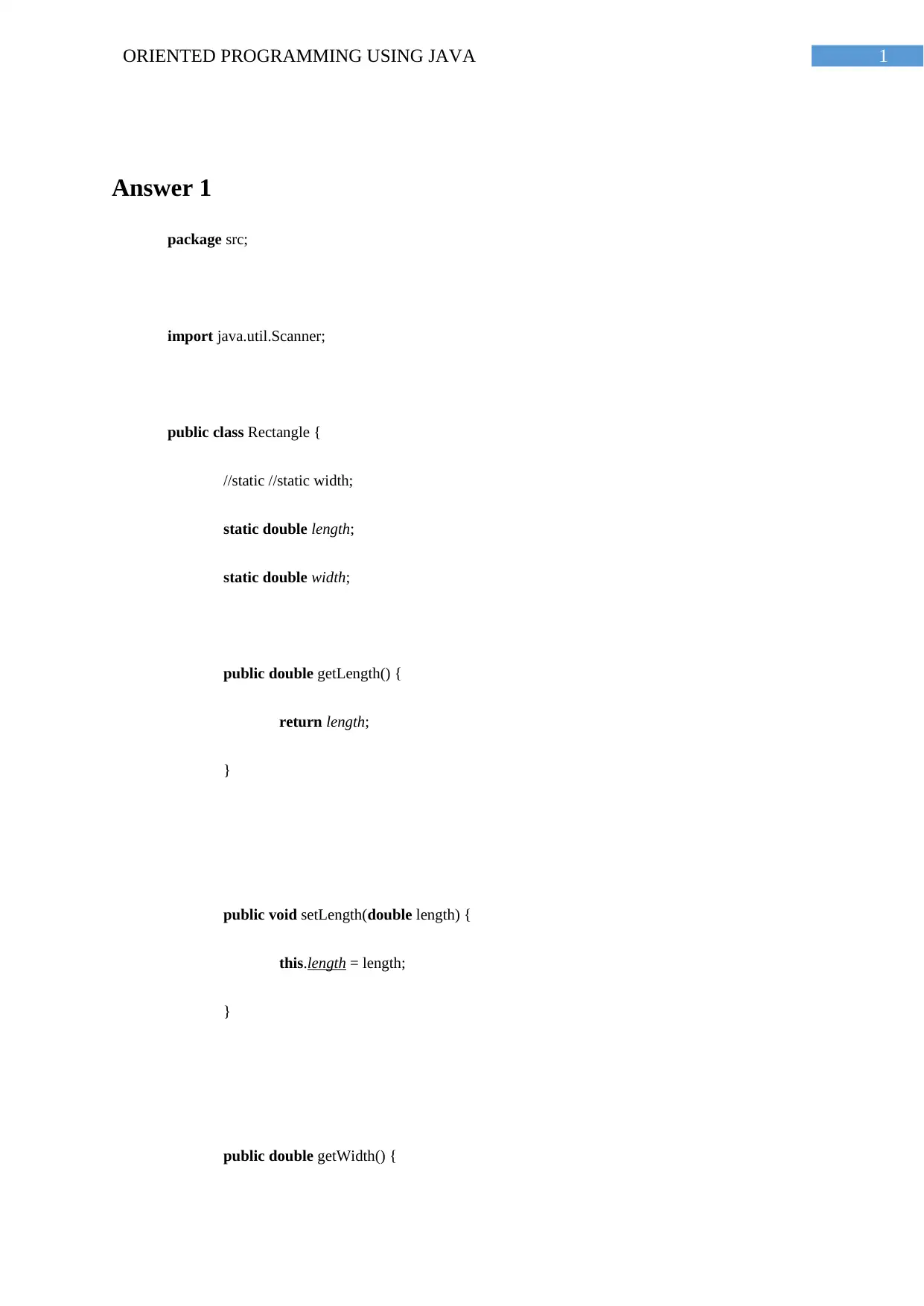
1ORIENTED PROGRAMMING USING JAVA
Answer 1
package src;
import java.util.Scanner;
public class Rectangle {
//static //static width;
static double length;
static double width;
public double getLength() {
return length;
}
public void setLength(double length) {
this.length = length;
}
public double getWidth() {
Answer 1
package src;
import java.util.Scanner;
public class Rectangle {
//static //static width;
static double length;
static double width;
public double getLength() {
return length;
}
public void setLength(double length) {
this.length = length;
}
public double getWidth() {
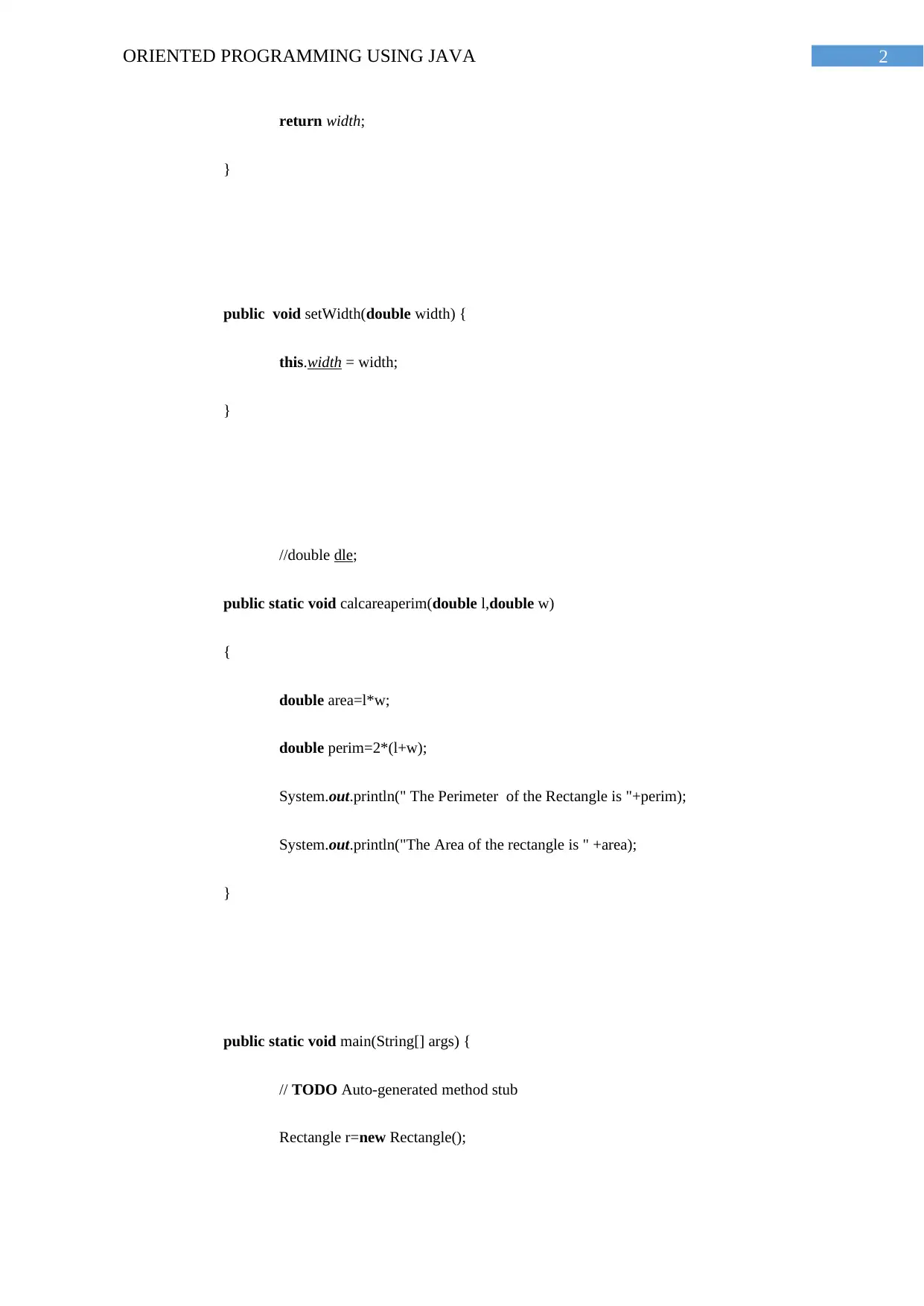
2ORIENTED PROGRAMMING USING JAVA
return width;
}
public void setWidth(double width) {
this.width = width;
}
//double dle;
public static void calcareaperim(double l,double w)
{
double area=l*w;
double perim=2*(l+w);
System.out.println(" The Perimeter of the Rectangle is "+perim);
System.out.println("The Area of the rectangle is " +area);
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Rectangle r=new Rectangle();
return width;
}
public void setWidth(double width) {
this.width = width;
}
//double dle;
public static void calcareaperim(double l,double w)
{
double area=l*w;
double perim=2*(l+w);
System.out.println(" The Perimeter of the Rectangle is "+perim);
System.out.println("The Area of the rectangle is " +area);
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Rectangle r=new Rectangle();
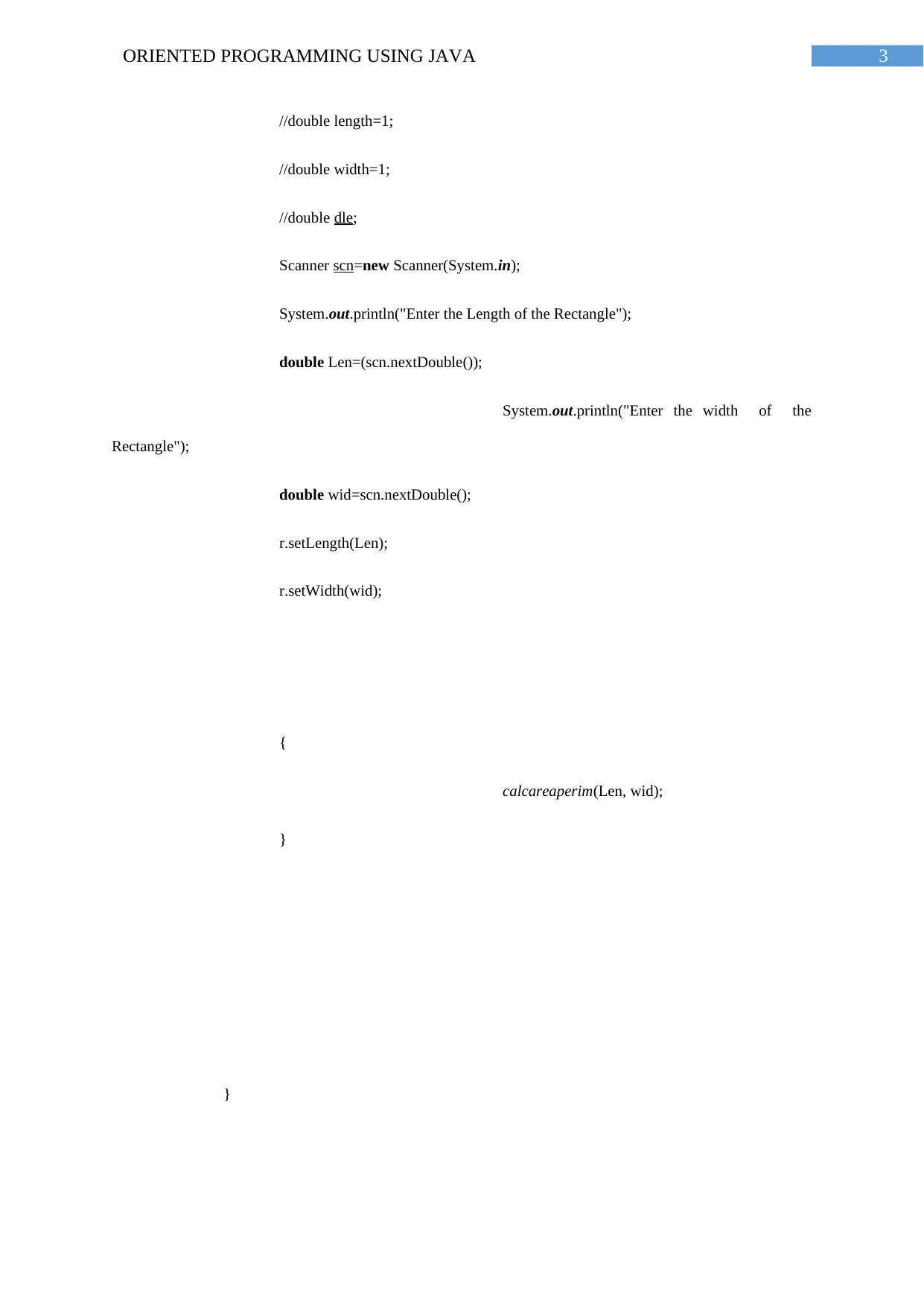
3ORIENTED PROGRAMMING USING JAVA
//double length=1;
//double width=1;
//double dle;
Scanner scn=new Scanner(System.in);
System.out.println("Enter the Length of the Rectangle");
double Len=(scn.nextDouble());
System.out.println("Enter the width of the
Rectangle");
double wid=scn.nextDouble();
r.setLength(Len);
r.setWidth(wid);
{
calcareaperim(Len, wid);
}
}
//double length=1;
//double width=1;
//double dle;
Scanner scn=new Scanner(System.in);
System.out.println("Enter the Length of the Rectangle");
double Len=(scn.nextDouble());
System.out.println("Enter the width of the
Rectangle");
double wid=scn.nextDouble();
r.setLength(Len);
r.setWidth(wid);
{
calcareaperim(Len, wid);
}
}
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
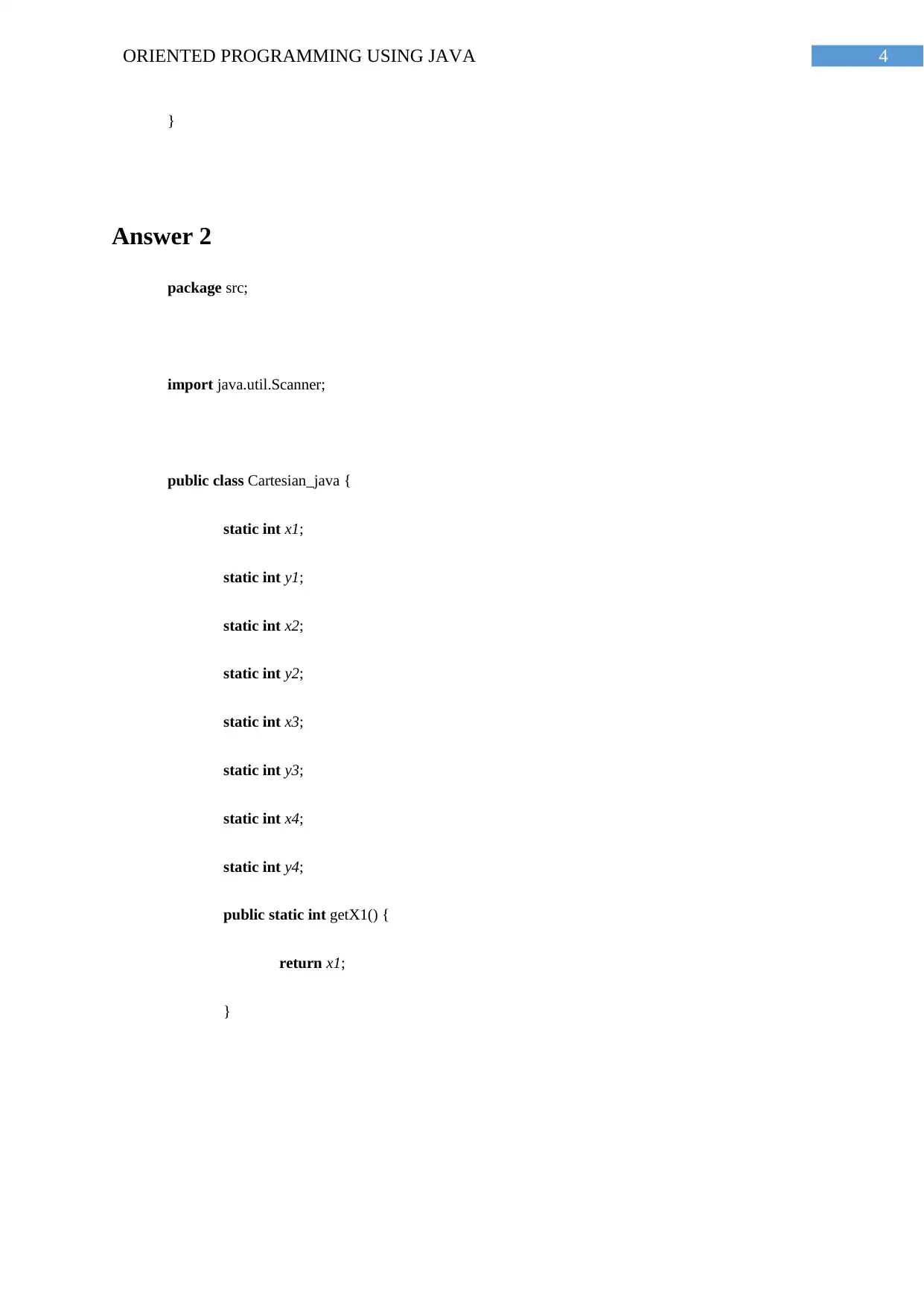
4ORIENTED PROGRAMMING USING JAVA
}
Answer 2
package src;
import java.util.Scanner;
public class Cartesian_java {
static int x1;
static int y1;
static int x2;
static int y2;
static int x3;
static int y3;
static int x4;
static int y4;
public static int getX1() {
return x1;
}
}
Answer 2
package src;
import java.util.Scanner;
public class Cartesian_java {
static int x1;
static int y1;
static int x2;
static int y2;
static int x3;
static int y3;
static int x4;
static int y4;
public static int getX1() {
return x1;
}
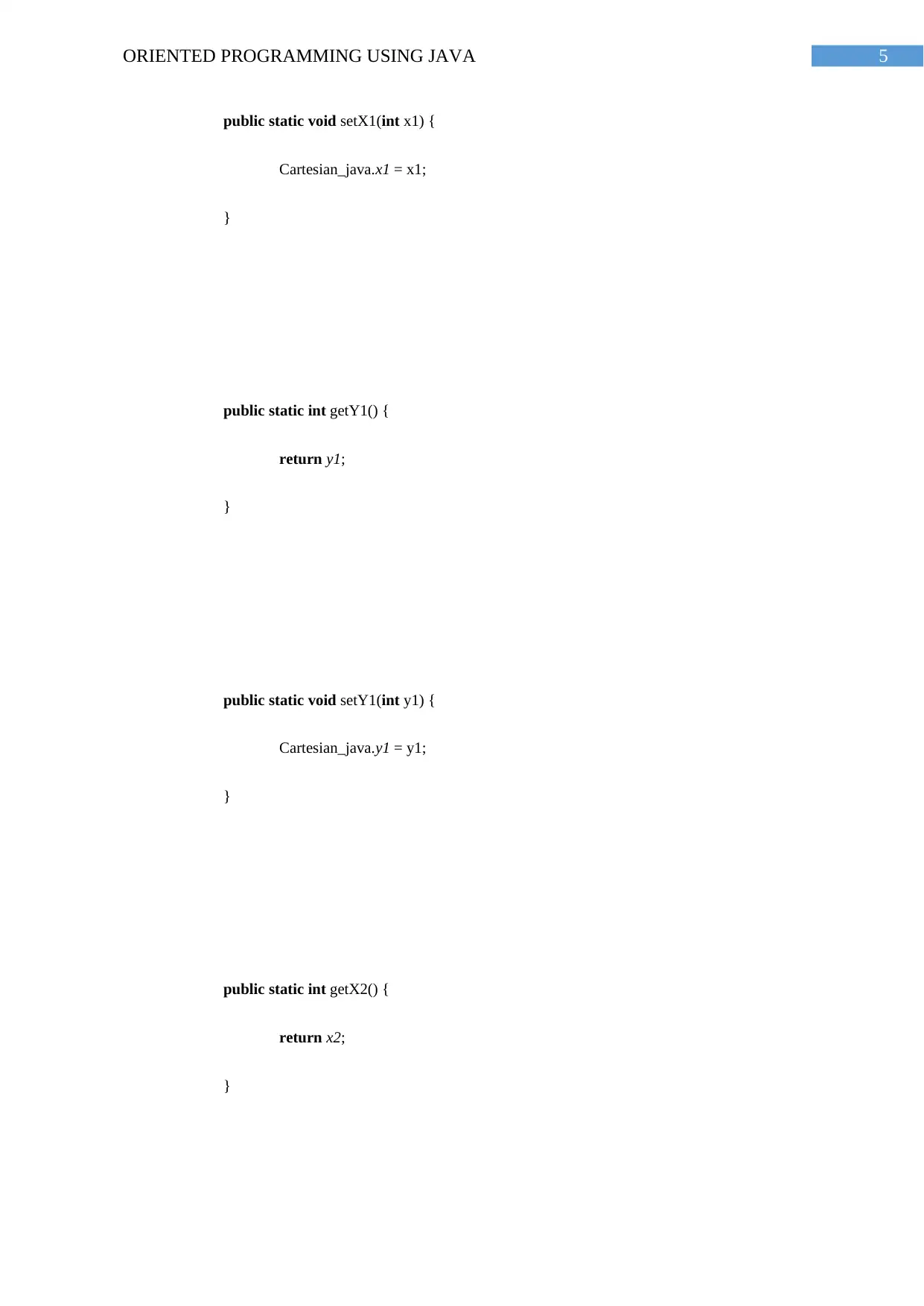
5ORIENTED PROGRAMMING USING JAVA
public static void setX1(int x1) {
Cartesian_java.x1 = x1;
}
public static int getY1() {
return y1;
}
public static void setY1(int y1) {
Cartesian_java.y1 = y1;
}
public static int getX2() {
return x2;
}
public static void setX1(int x1) {
Cartesian_java.x1 = x1;
}
public static int getY1() {
return y1;
}
public static void setY1(int y1) {
Cartesian_java.y1 = y1;
}
public static int getX2() {
return x2;
}
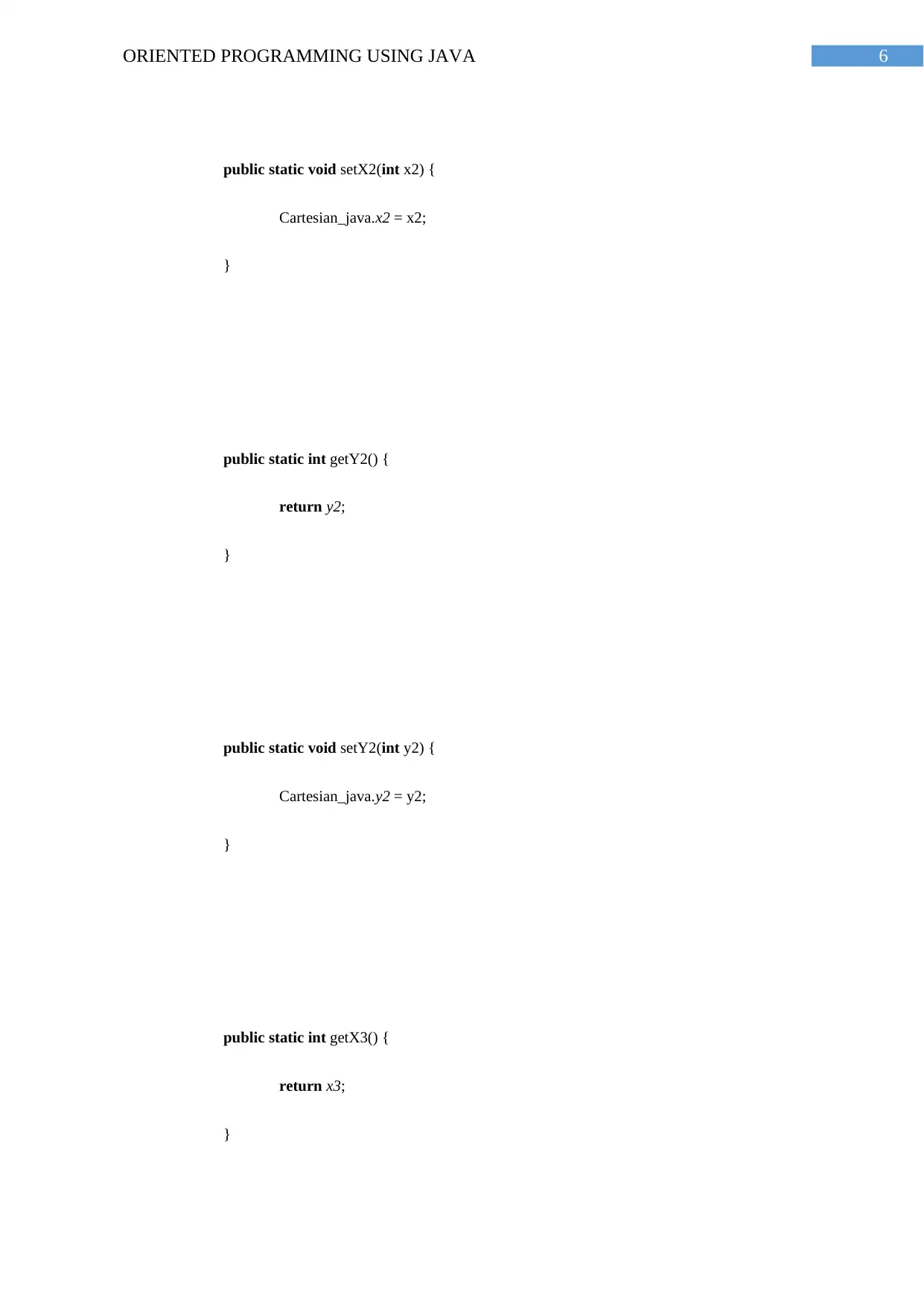
6ORIENTED PROGRAMMING USING JAVA
public static void setX2(int x2) {
Cartesian_java.x2 = x2;
}
public static int getY2() {
return y2;
}
public static void setY2(int y2) {
Cartesian_java.y2 = y2;
}
public static int getX3() {
return x3;
}
public static void setX2(int x2) {
Cartesian_java.x2 = x2;
}
public static int getY2() {
return y2;
}
public static void setY2(int y2) {
Cartesian_java.y2 = y2;
}
public static int getX3() {
return x3;
}
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
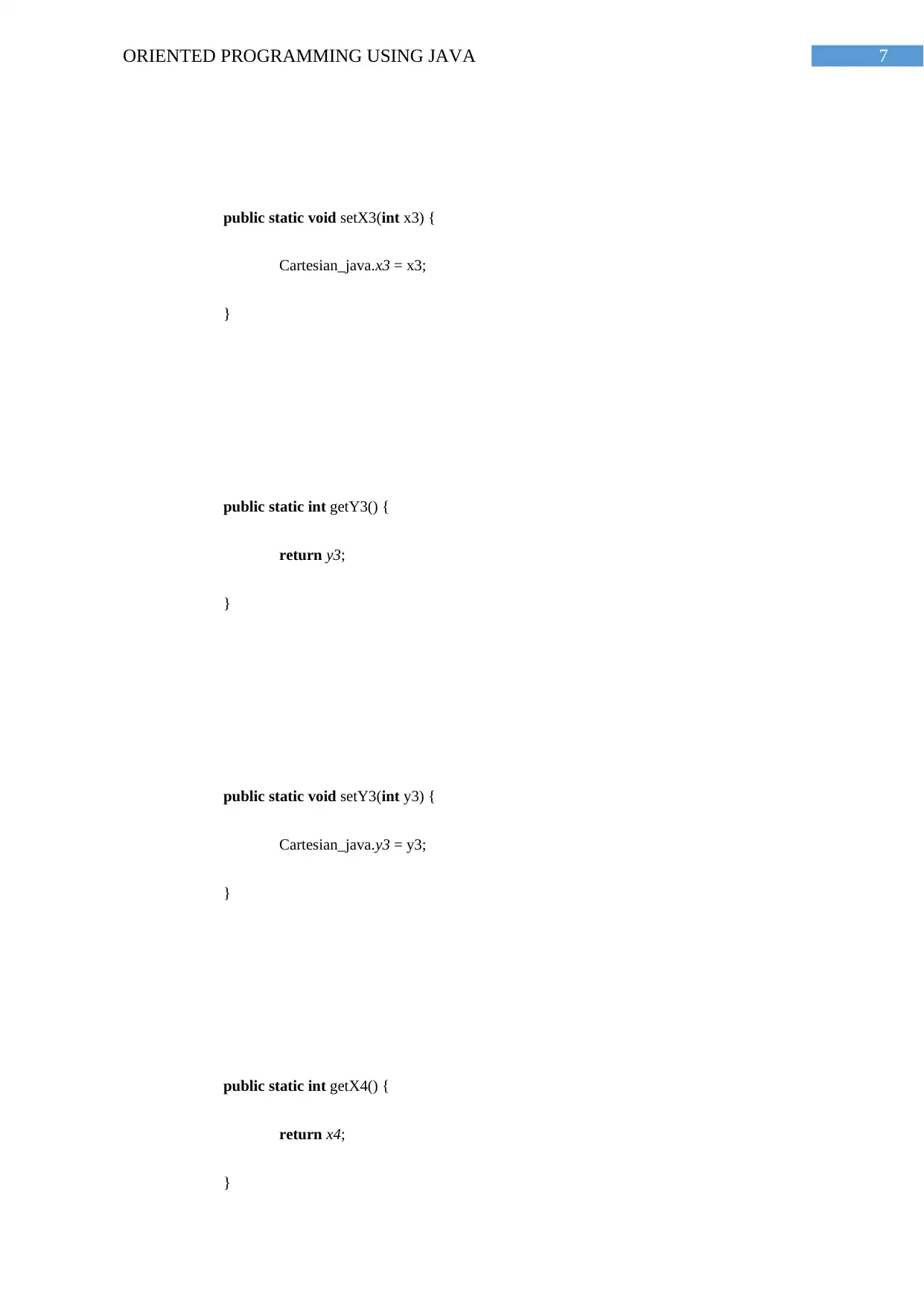
7ORIENTED PROGRAMMING USING JAVA
public static void setX3(int x3) {
Cartesian_java.x3 = x3;
}
public static int getY3() {
return y3;
}
public static void setY3(int y3) {
Cartesian_java.y3 = y3;
}
public static int getX4() {
return x4;
}
public static void setX3(int x3) {
Cartesian_java.x3 = x3;
}
public static int getY3() {
return y3;
}
public static void setY3(int y3) {
Cartesian_java.y3 = y3;
}
public static int getX4() {
return x4;
}
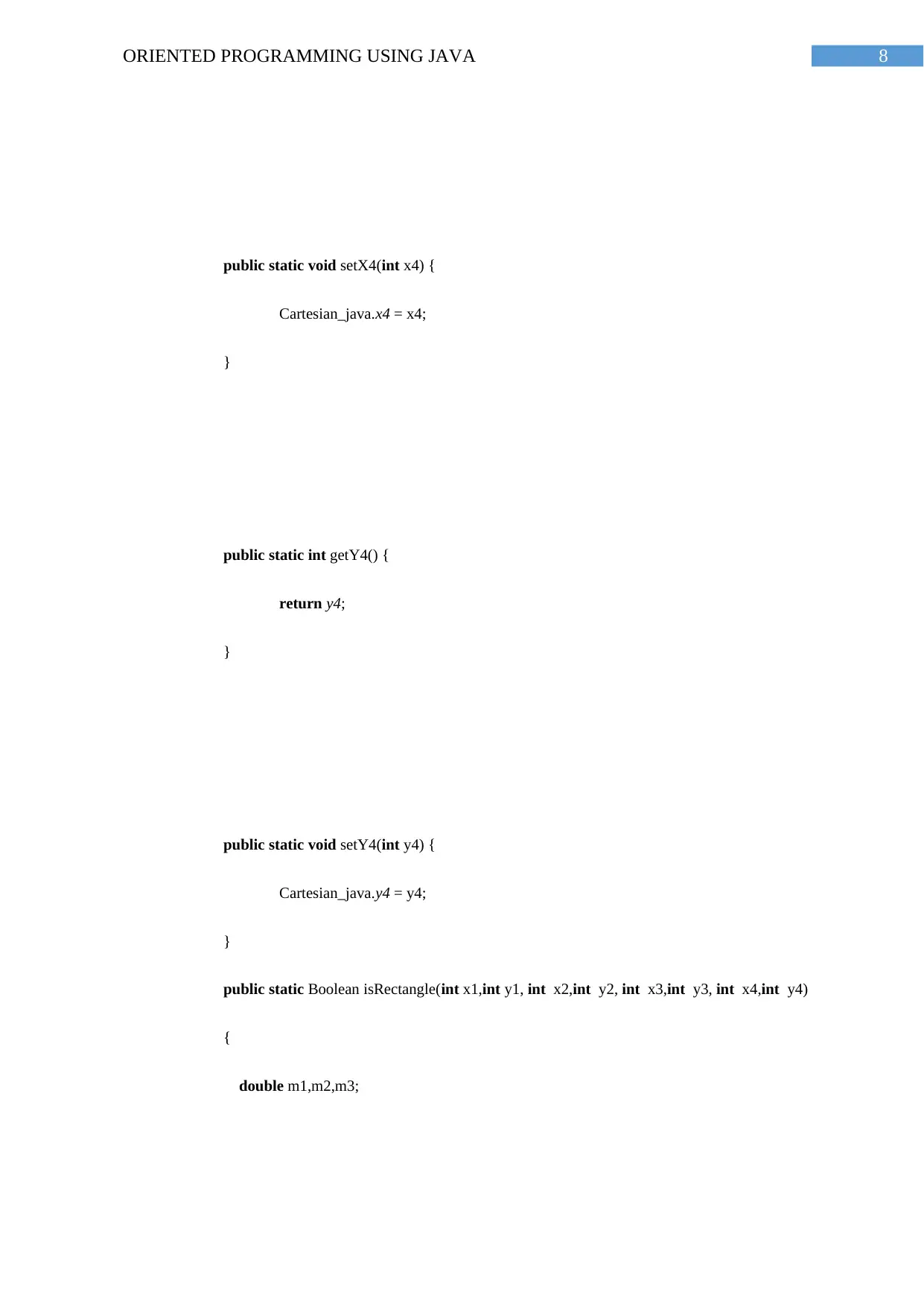
8ORIENTED PROGRAMMING USING JAVA
public static void setX4(int x4) {
Cartesian_java.x4 = x4;
}
public static int getY4() {
return y4;
}
public static void setY4(int y4) {
Cartesian_java.y4 = y4;
}
public static Boolean isRectangle(int x1,int y1, int x2,int y2, int x3,int y3, int x4,int y4)
{
double m1,m2,m3;
public static void setX4(int x4) {
Cartesian_java.x4 = x4;
}
public static int getY4() {
return y4;
}
public static void setY4(int y4) {
Cartesian_java.y4 = y4;
}
public static Boolean isRectangle(int x1,int y1, int x2,int y2, int x3,int y3, int x4,int y4)
{
double m1,m2,m3;
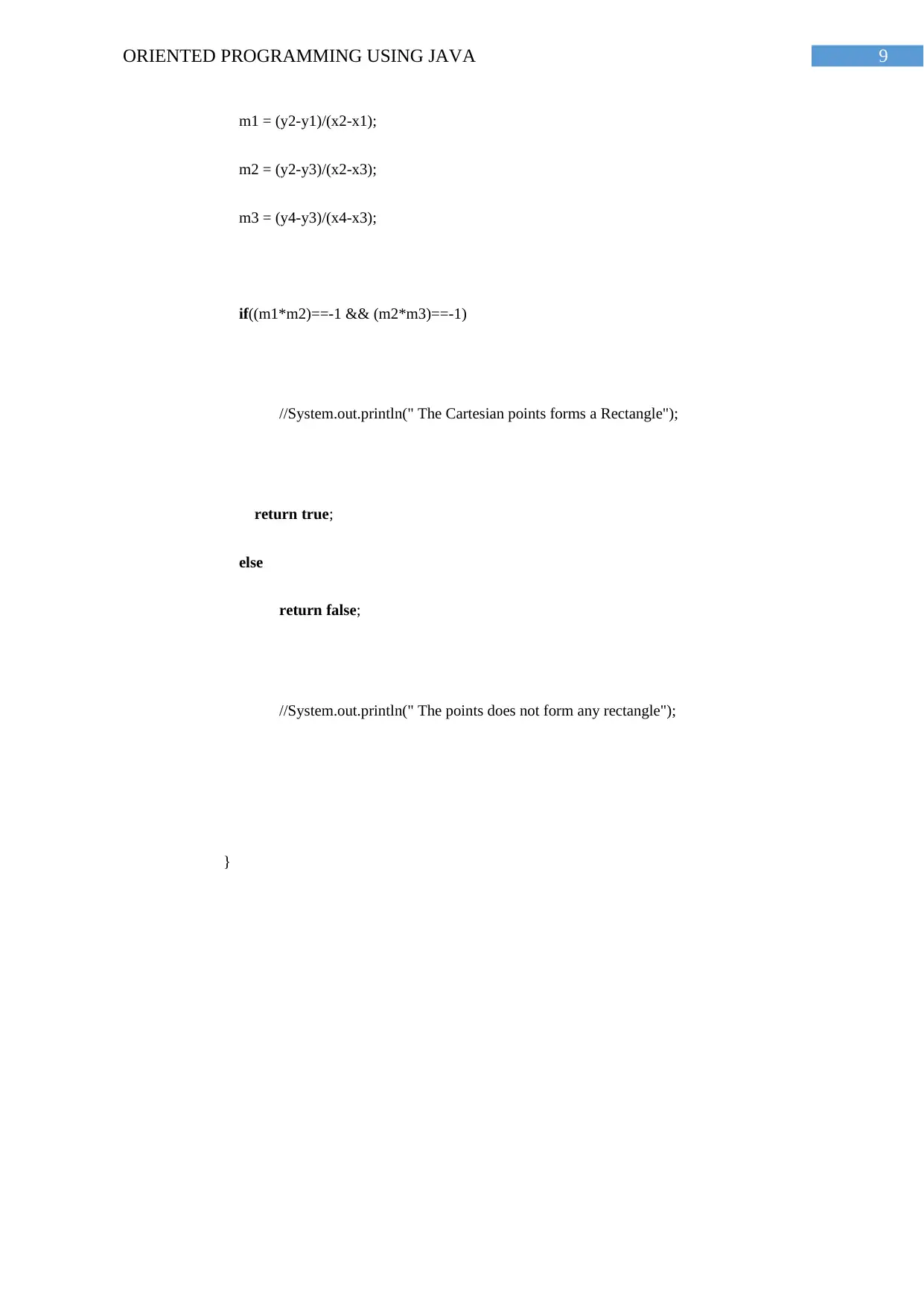
9ORIENTED PROGRAMMING USING JAVA
m1 = (y2-y1)/(x2-x1);
m2 = (y2-y3)/(x2-x3);
m3 = (y4-y3)/(x4-x3);
if((m1*m2)==-1 && (m2*m3)==-1)
//System.out.println(" The Cartesian points forms a Rectangle");
return true;
else
return false;
//System.out.println(" The points does not form any rectangle");
}
m1 = (y2-y1)/(x2-x1);
m2 = (y2-y3)/(x2-x3);
m3 = (y4-y3)/(x4-x3);
if((m1*m2)==-1 && (m2*m3)==-1)
//System.out.println(" The Cartesian points forms a Rectangle");
return true;
else
return false;
//System.out.println(" The points does not form any rectangle");
}
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
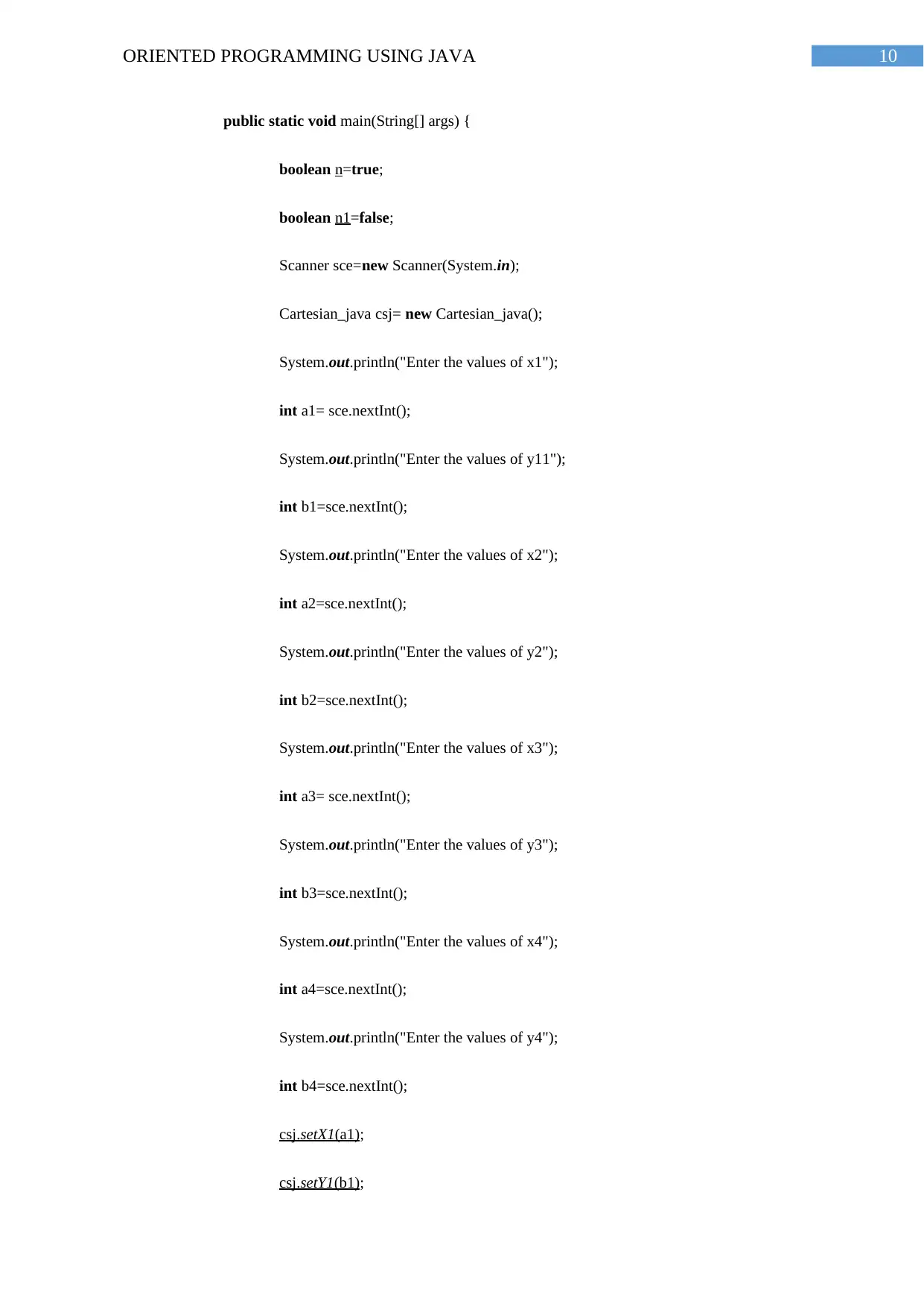
10ORIENTED PROGRAMMING USING JAVA
public static void main(String[] args) {
boolean n=true;
boolean n1=false;
Scanner sce=new Scanner(System.in);
Cartesian_java csj= new Cartesian_java();
System.out.println("Enter the values of x1");
int a1= sce.nextInt();
System.out.println("Enter the values of y11");
int b1=sce.nextInt();
System.out.println("Enter the values of x2");
int a2=sce.nextInt();
System.out.println("Enter the values of y2");
int b2=sce.nextInt();
System.out.println("Enter the values of x3");
int a3= sce.nextInt();
System.out.println("Enter the values of y3");
int b3=sce.nextInt();
System.out.println("Enter the values of x4");
int a4=sce.nextInt();
System.out.println("Enter the values of y4");
int b4=sce.nextInt();
csj.setX1(a1);
csj.setY1(b1);
public static void main(String[] args) {
boolean n=true;
boolean n1=false;
Scanner sce=new Scanner(System.in);
Cartesian_java csj= new Cartesian_java();
System.out.println("Enter the values of x1");
int a1= sce.nextInt();
System.out.println("Enter the values of y11");
int b1=sce.nextInt();
System.out.println("Enter the values of x2");
int a2=sce.nextInt();
System.out.println("Enter the values of y2");
int b2=sce.nextInt();
System.out.println("Enter the values of x3");
int a3= sce.nextInt();
System.out.println("Enter the values of y3");
int b3=sce.nextInt();
System.out.println("Enter the values of x4");
int a4=sce.nextInt();
System.out.println("Enter the values of y4");
int b4=sce.nextInt();
csj.setX1(a1);
csj.setY1(b1);
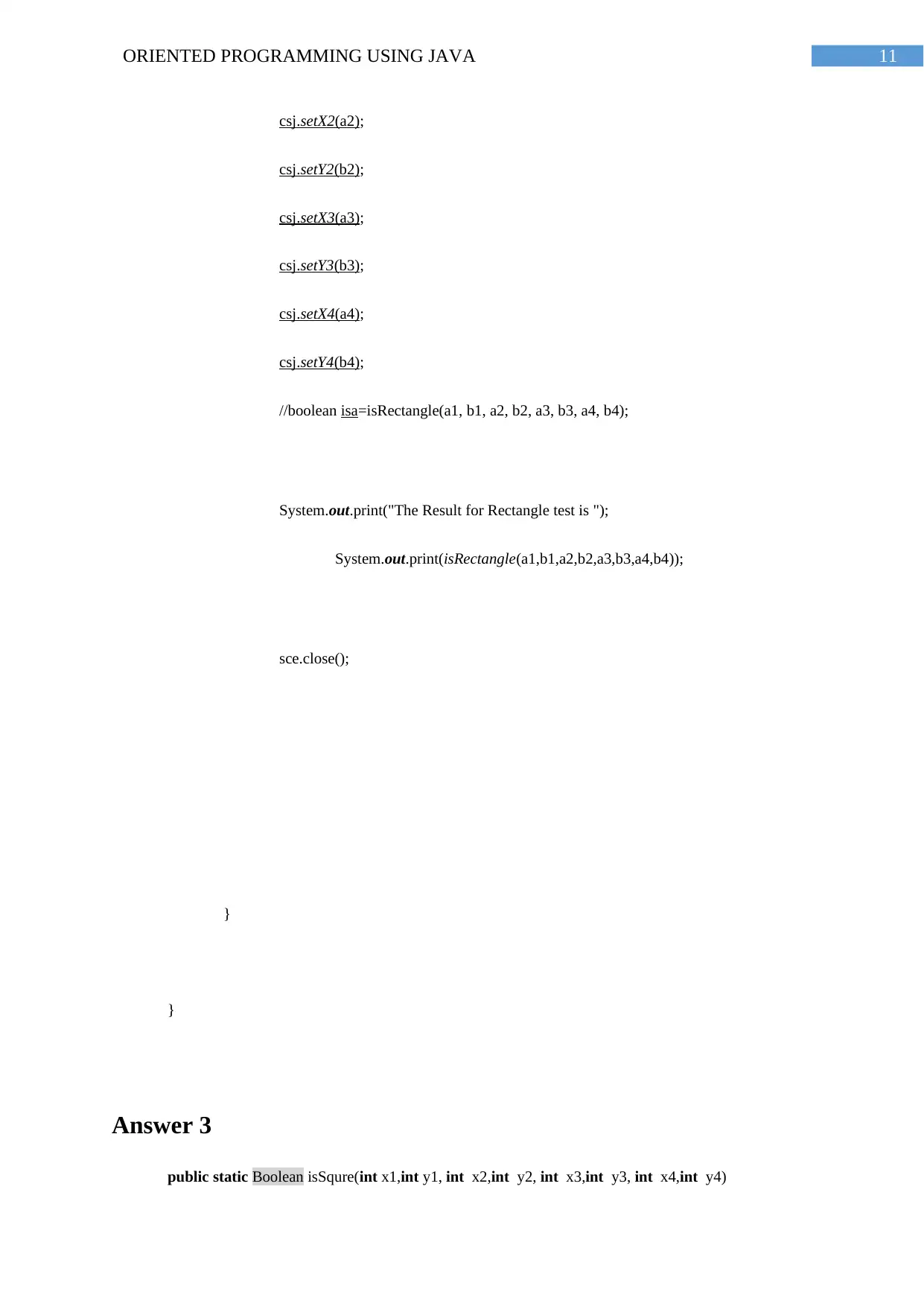
11ORIENTED PROGRAMMING USING JAVA
csj.setX2(a2);
csj.setY2(b2);
csj.setX3(a3);
csj.setY3(b3);
csj.setX4(a4);
csj.setY4(b4);
//boolean isa=isRectangle(a1, b1, a2, b2, a3, b3, a4, b4);
System.out.print("The Result for Rectangle test is ");
System.out.print(isRectangle(a1,b1,a2,b2,a3,b3,a4,b4));
sce.close();
}
}
Answer 3
public static Boolean isSqure(int x1,int y1, int x2,int y2, int x3,int y3, int x4,int y4)
csj.setX2(a2);
csj.setY2(b2);
csj.setX3(a3);
csj.setY3(b3);
csj.setX4(a4);
csj.setY4(b4);
//boolean isa=isRectangle(a1, b1, a2, b2, a3, b3, a4, b4);
System.out.print("The Result for Rectangle test is ");
System.out.print(isRectangle(a1,b1,a2,b2,a3,b3,a4,b4));
sce.close();
}
}
Answer 3
public static Boolean isSqure(int x1,int y1, int x2,int y2, int x3,int y3, int x4,int y4)
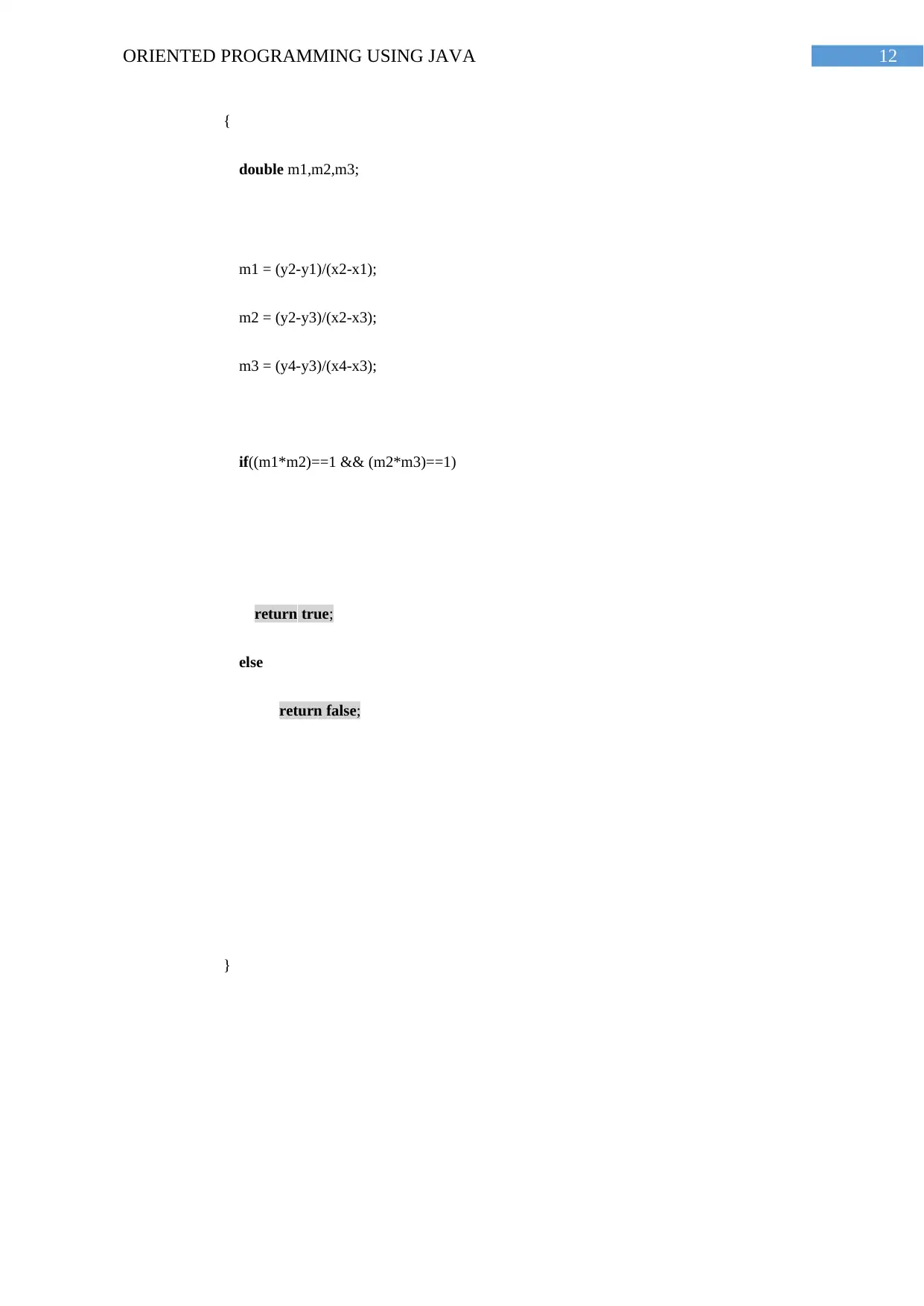
12ORIENTED PROGRAMMING USING JAVA
{
double m1,m2,m3;
m1 = (y2-y1)/(x2-x1);
m2 = (y2-y3)/(x2-x3);
m3 = (y4-y3)/(x4-x3);
if((m1*m2)==1 && (m2*m3)==1)
return true;
else
return false;
}
{
double m1,m2,m3;
m1 = (y2-y1)/(x2-x1);
m2 = (y2-y3)/(x2-x3);
m3 = (y4-y3)/(x4-x3);
if((m1*m2)==1 && (m2*m3)==1)
return true;
else
return false;
}
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
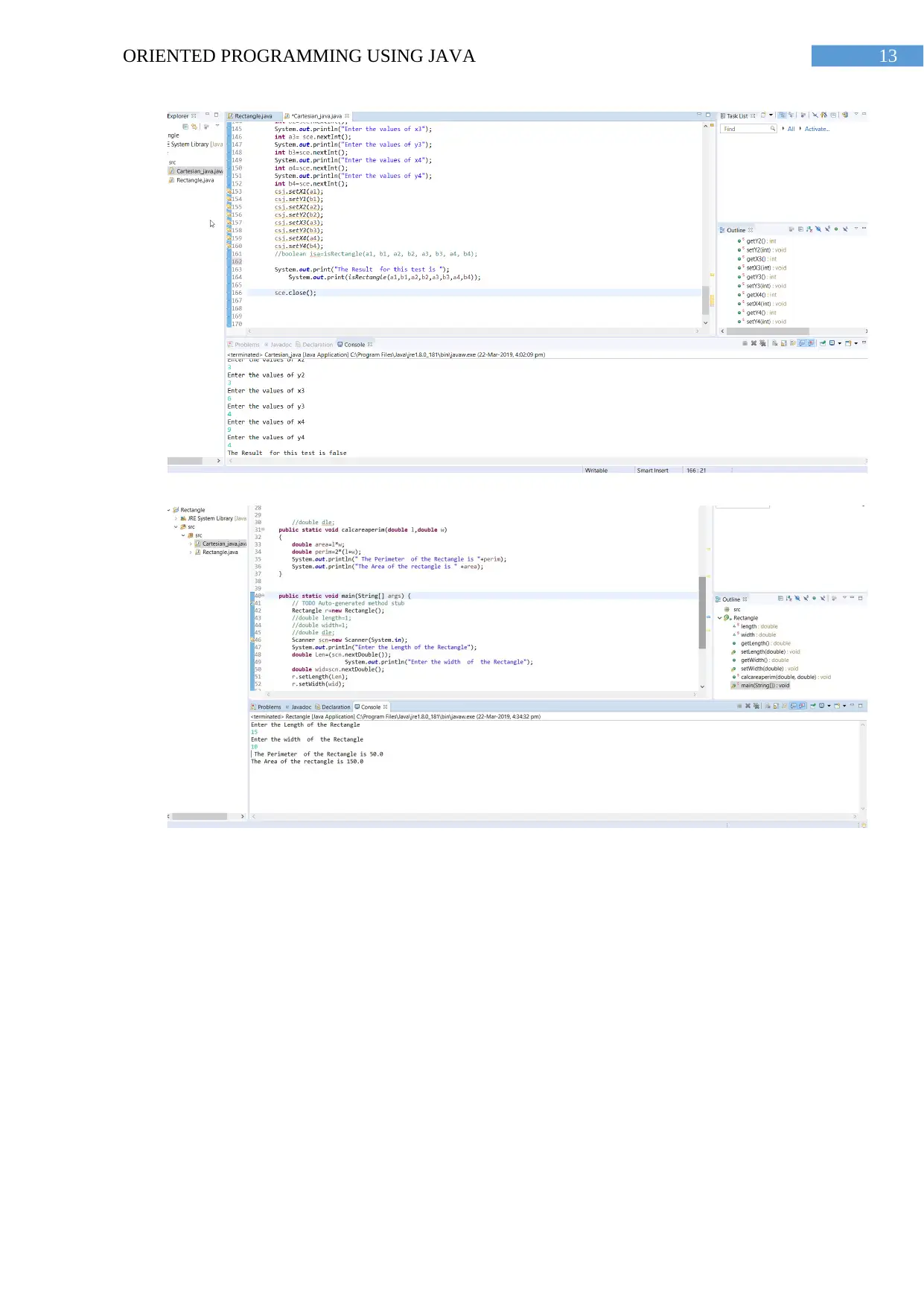
13ORIENTED PROGRAMMING USING JAVA
1 out of 14
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
 +13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024  |  Zucol Services PVT LTD  |  All rights reserved.