COMP9812 - Computer Networks And Operating Systems
Added on 2021-10-07
34 Pages3084 Words208 Views
Operating System
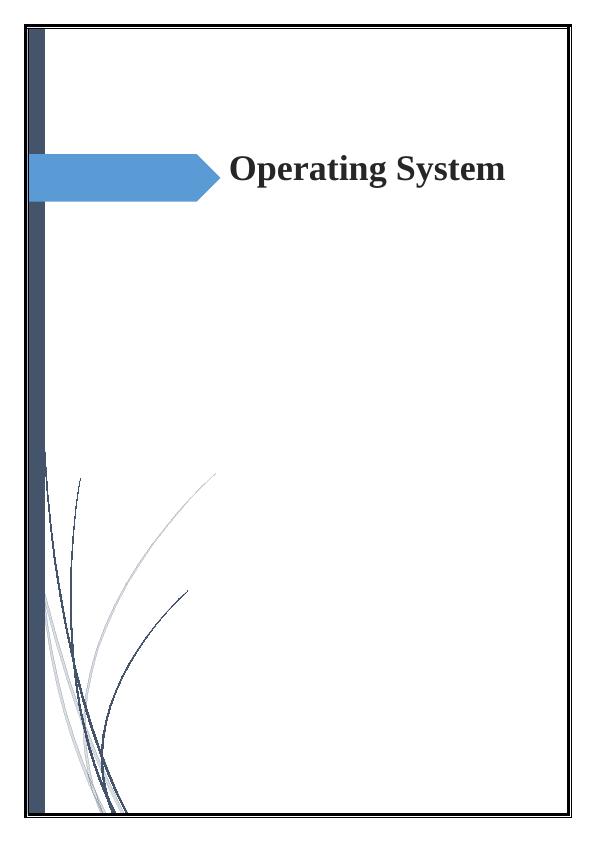
Table of Contents
1. Introduction.................................................................................................................................2
Task 1: Creating and Destroying the Segment of Shared Memory.................................................2
Task 2: Sharing only One Message between the Producer and Consumer.....................................4
Task 3: Sharing a series of Messages between the Producer and Consumer..................................6
Task 4: Delaying the Message Sent by the Producer........................................................................8
Task 5: Implementing Semaphores..................................................................................................10
Task 6: Character-based circular buffer.........................................................................................13
2. Conclusion..................................................................................................................................14
References..........................................................................................................................................15
1. Introduction.................................................................................................................................2
Task 1: Creating and Destroying the Segment of Shared Memory.................................................2
Task 2: Sharing only One Message between the Producer and Consumer.....................................4
Task 3: Sharing a series of Messages between the Producer and Consumer..................................6
Task 4: Delaying the Message Sent by the Producer........................................................................8
Task 5: Implementing Semaphores..................................................................................................10
Task 6: Character-based circular buffer.........................................................................................13
2. Conclusion..................................................................................................................................14
References..........................................................................................................................................15
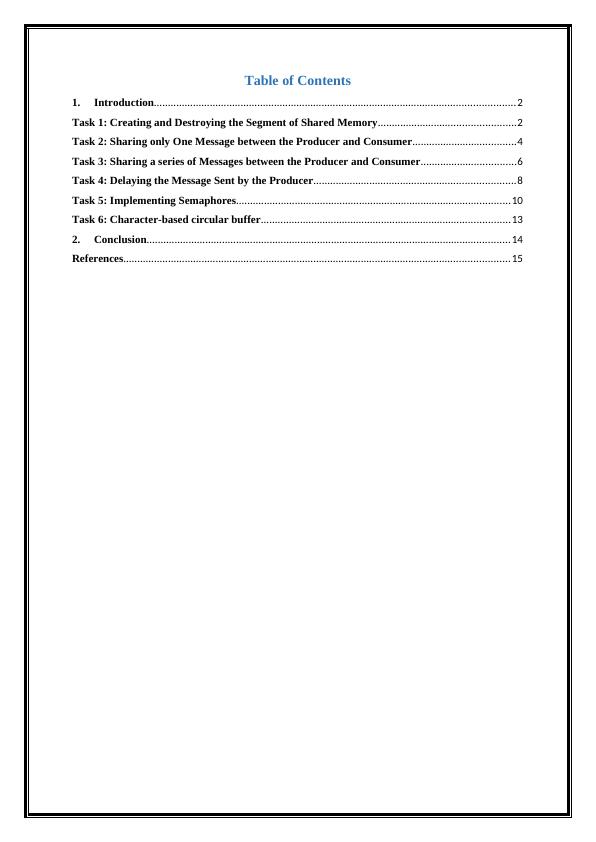
1. Introduction
In today’s modern applications, inter-process communication is widely spread. This
report sheds light on shared memory between the producer and the consumer. It is difficult to
use shared memory transportation, but this method provides more control and speed.
However, there will be behaviour difference in different operating systems, which can be
dealt easily. Here, the system refers to message queue, which contains two-way
communication channels for the producer and the consumer to exchange messages. The
shared memory will be developed here with the help of C language, in the Linux operating
system.
The objective of this report is to accomplish six tasks such as, creating and destroying
the segment of shared memory, sending one message from the producer to the consumer,
sending series of messages from the producer to the consumer, delaying the message sent by
the producer, implementing semaphores and Character-based circular buffer. All these
objectives will be met in the following sections of the report.
Task 1: Creating and Destroying the Segment of Shared Memory
Here, the task refers to developing a segment of shared memory for the producer and
consumer. We create producer for sending the message and sharing the memory. There is
memory space for the producer so that it is possible for the producer to share the memory
with the consumer. This segment contains, owner name, bytes, shared memory and status.
The program is used to check the remaining memory space of the producer. The producer can
easily send the message to the consumer. Once the memory of the producer is checked, the
memory will be decreased and it will be shared with the consumer (Ahmad, 2010).
Program
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
#include<sys/types.h>
#include<sys/ipc.h>
In today’s modern applications, inter-process communication is widely spread. This
report sheds light on shared memory between the producer and the consumer. It is difficult to
use shared memory transportation, but this method provides more control and speed.
However, there will be behaviour difference in different operating systems, which can be
dealt easily. Here, the system refers to message queue, which contains two-way
communication channels for the producer and the consumer to exchange messages. The
shared memory will be developed here with the help of C language, in the Linux operating
system.
The objective of this report is to accomplish six tasks such as, creating and destroying
the segment of shared memory, sending one message from the producer to the consumer,
sending series of messages from the producer to the consumer, delaying the message sent by
the producer, implementing semaphores and Character-based circular buffer. All these
objectives will be met in the following sections of the report.
Task 1: Creating and Destroying the Segment of Shared Memory
Here, the task refers to developing a segment of shared memory for the producer and
consumer. We create producer for sending the message and sharing the memory. There is
memory space for the producer so that it is possible for the producer to share the memory
with the consumer. This segment contains, owner name, bytes, shared memory and status.
The program is used to check the remaining memory space of the producer. The producer can
easily send the message to the consumer. Once the memory of the producer is checked, the
memory will be decreased and it will be shared with the consumer (Ahmad, 2010).
Program
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
#include<sys/types.h>
#include<sys/ipc.h>
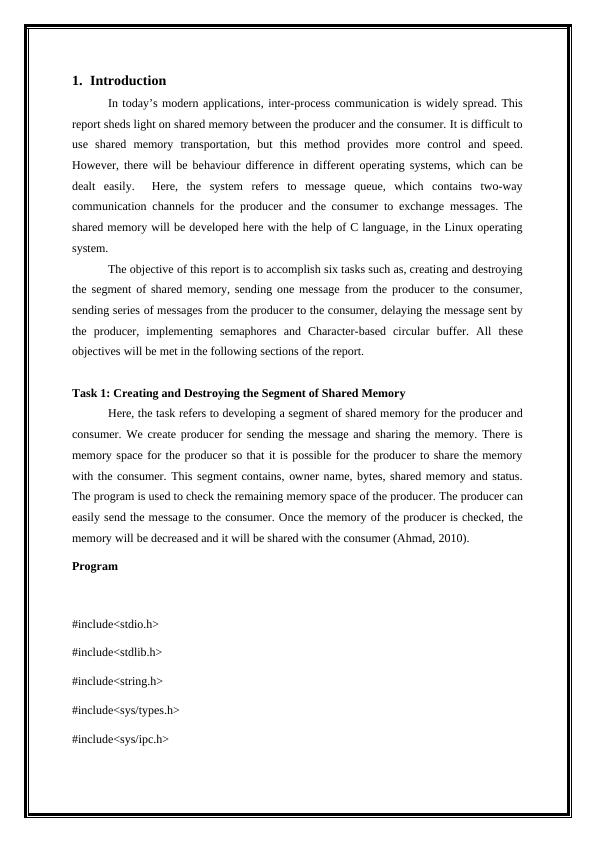
#include<sys/shm.h>
#define SHSIZE 100
int main(int argc,char*argv[])
{
int shmid;
key_t key;
char * shm;
char*s;
key=9876;
shmid=shmget(key,SHSIZE,IPC_CREAT | 0666);
if(shmid<0)
{
perror("shmget");
exit(1);
}
shm=shmat(shmid,NULL,0);
if(shm==(char*)-1)
{
perror("shmat");
exit(1);
}
memcpy(shm,"hello world",11);
#define SHSIZE 100
int main(int argc,char*argv[])
{
int shmid;
key_t key;
char * shm;
char*s;
key=9876;
shmid=shmget(key,SHSIZE,IPC_CREAT | 0666);
if(shmid<0)
{
perror("shmget");
exit(1);
}
shm=shmat(shmid,NULL,0);
if(shm==(char*)-1)
{
perror("shmat");
exit(1);
}
memcpy(shm,"hello world",11);
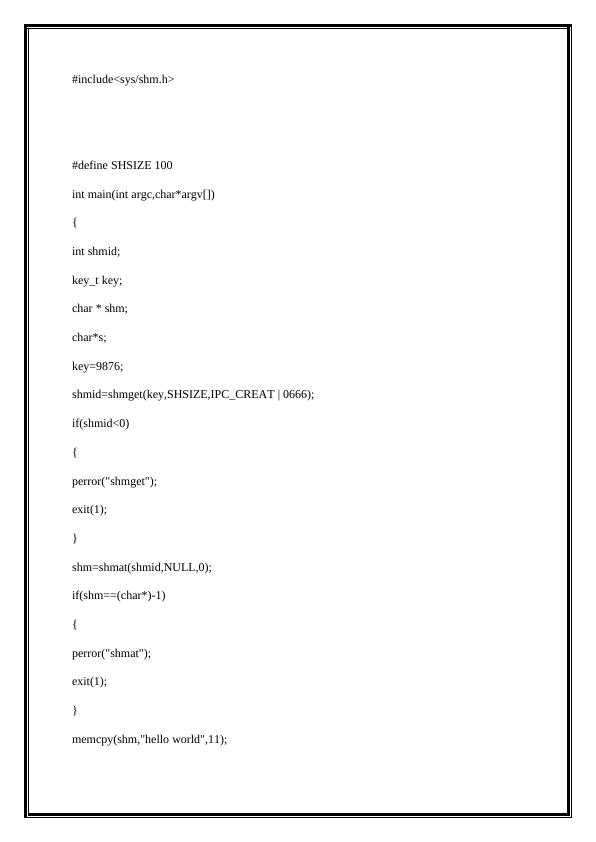
s = shm;
s += 11;
*s = 0;
while(*shm != '*')
sleep (1);
return 0;
}
Output
s += 11;
*s = 0;
while(*shm != '*')
sleep (1);
return 0;
}
Output
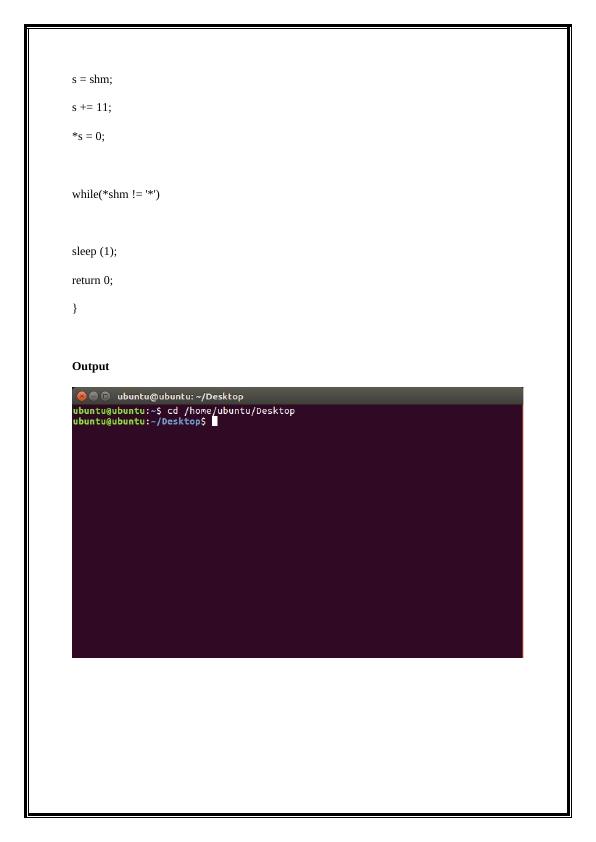
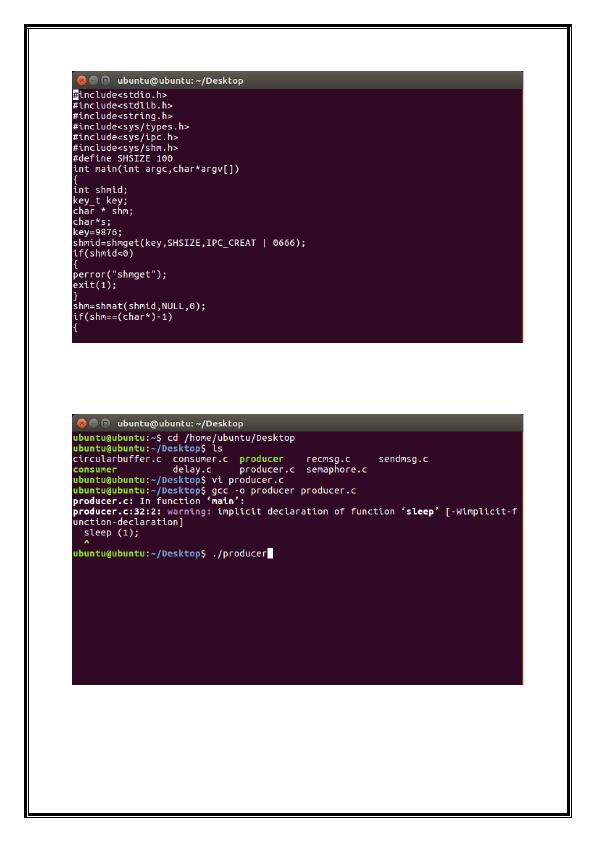
Task 2: Sharing only One Message between the Producer and Consumer
Here, the task refers to sharing only one message that is sent from the producer to the
consumer, where the producer is referred as the sender and the receiver as the consumer.
After the message is received, it is displayed on the page of the consumer (Hogan and
Epping, 2014).
Program
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
#include<sys/types.h>
#include<sys/ipc.h>
#include<sys/shm.h>
#define SHSIZE 100
int main(int argc,char*argv[])
{
int shmid;
Here, the task refers to sharing only one message that is sent from the producer to the
consumer, where the producer is referred as the sender and the receiver as the consumer.
After the message is received, it is displayed on the page of the consumer (Hogan and
Epping, 2014).
Program
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
#include<sys/types.h>
#include<sys/ipc.h>
#include<sys/shm.h>
#define SHSIZE 100
int main(int argc,char*argv[])
{
int shmid;
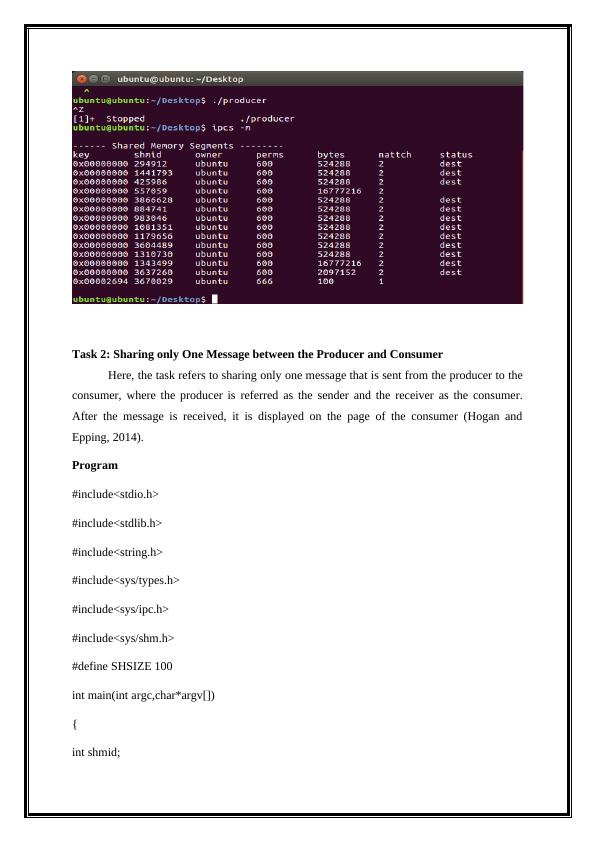
key_t key;
char * shm;
char*s;
key=9876;
shmid=shmget(key,SHSIZE,IPC_CREAT | 0666);
if(shmid<0)
{
perror("shmget");
exit(1);
}
shm=shmat(shmid,NULL,0);
if(shm==(char*)-1)
{
perror("shmat");
exit(1);
}
for(s =shm; *s !=0; s++)
printf("%c", *s);
printf("\n");
*shm = '*';
return 0;
char * shm;
char*s;
key=9876;
shmid=shmget(key,SHSIZE,IPC_CREAT | 0666);
if(shmid<0)
{
perror("shmget");
exit(1);
}
shm=shmat(shmid,NULL,0);
if(shm==(char*)-1)
{
perror("shmat");
exit(1);
}
for(s =shm; *s !=0; s++)
printf("%c", *s);
printf("\n");
*shm = '*';
return 0;
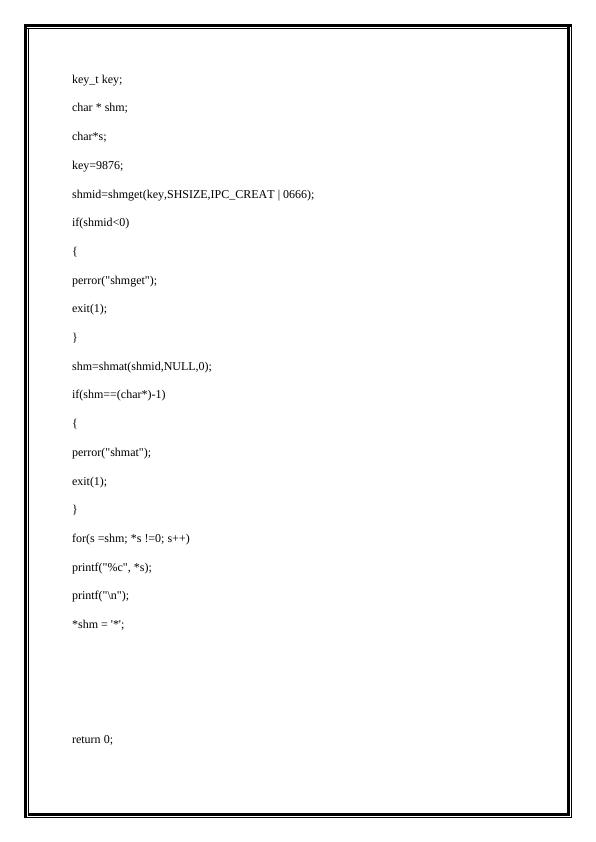
End of preview
Want to access all the pages? Upload your documents or become a member.
Related Documents
Operating Systemlg...
|30
|2055
|230
Shared Memory and Inter-Process Communication in Operating Systemslg...
|34
|3181
|51
Understanding Buffer Overflow: Stack and Heap Buffer Overflows, Exploiting Buffer Overflow, JOP and ROPlg...
|11
|1080
|283
Shared Memory Segment Creation and Destruction in C Programminglg...
|29
|2258
|453
Desklib - Online Library for Study Materiallg...
|11
|2075
|276
Inter-process Communication and Synchronisationlg...
|11
|2268
|343