Student Information Management System
VerifiedAdded on 2021/04/21
|14
|2251
|265
AI Summary
The provided document is an assignment on developing a student information management system. It outlines the requirements for implementing a menu system using switch-case statements, utilizing linked lists for data storage, and incorporating file I/O operations to generate reports. The development aims to improve performance, maintainability, and feature additions such as validation of user input and limiting concurrent updates.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
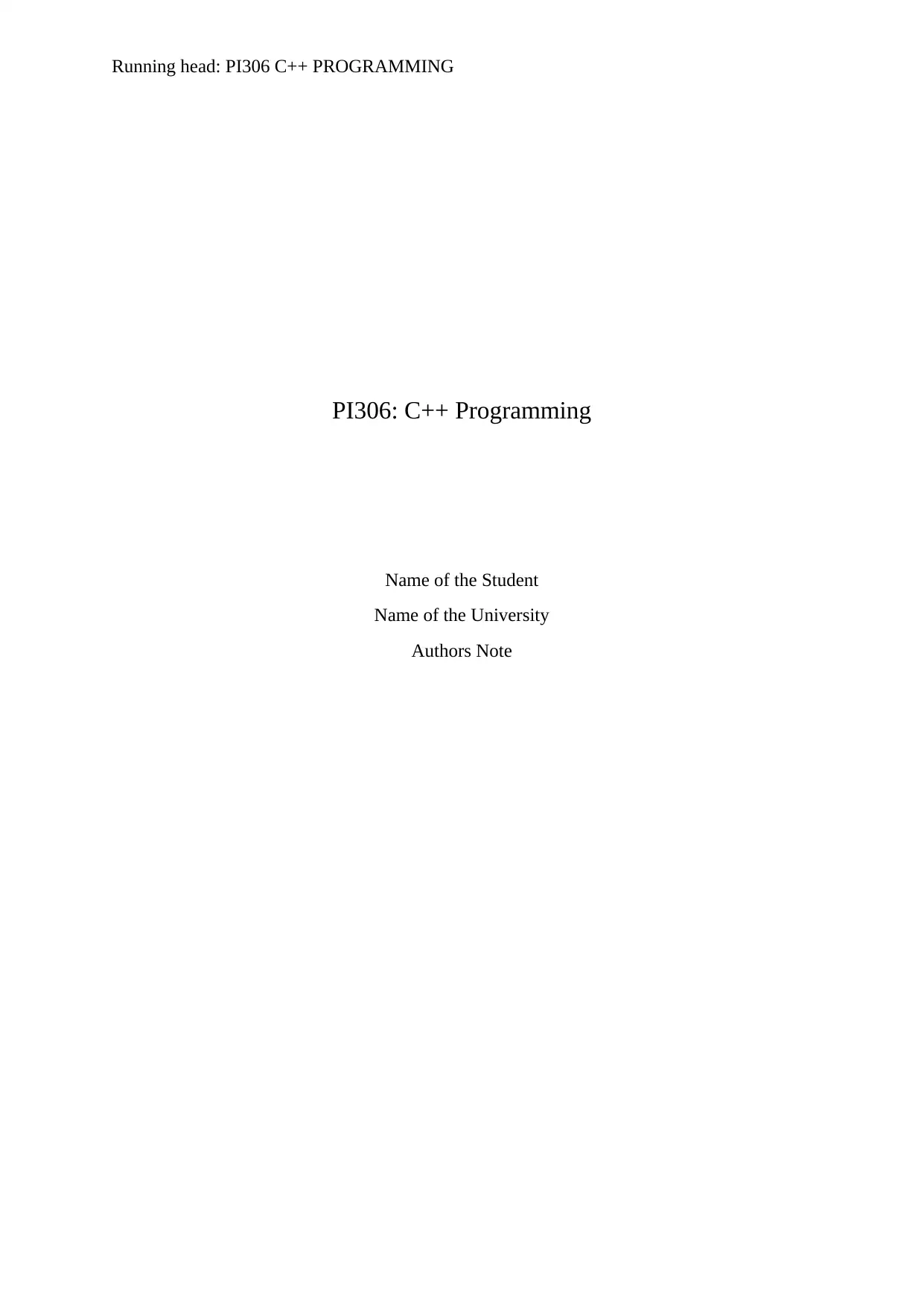
Running head: PI306 C++ PROGRAMMING
PI306: C++ Programming
Name of the Student
Name of the University
Authors Note
PI306: C++ Programming
Name of the Student
Name of the University
Authors Note
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
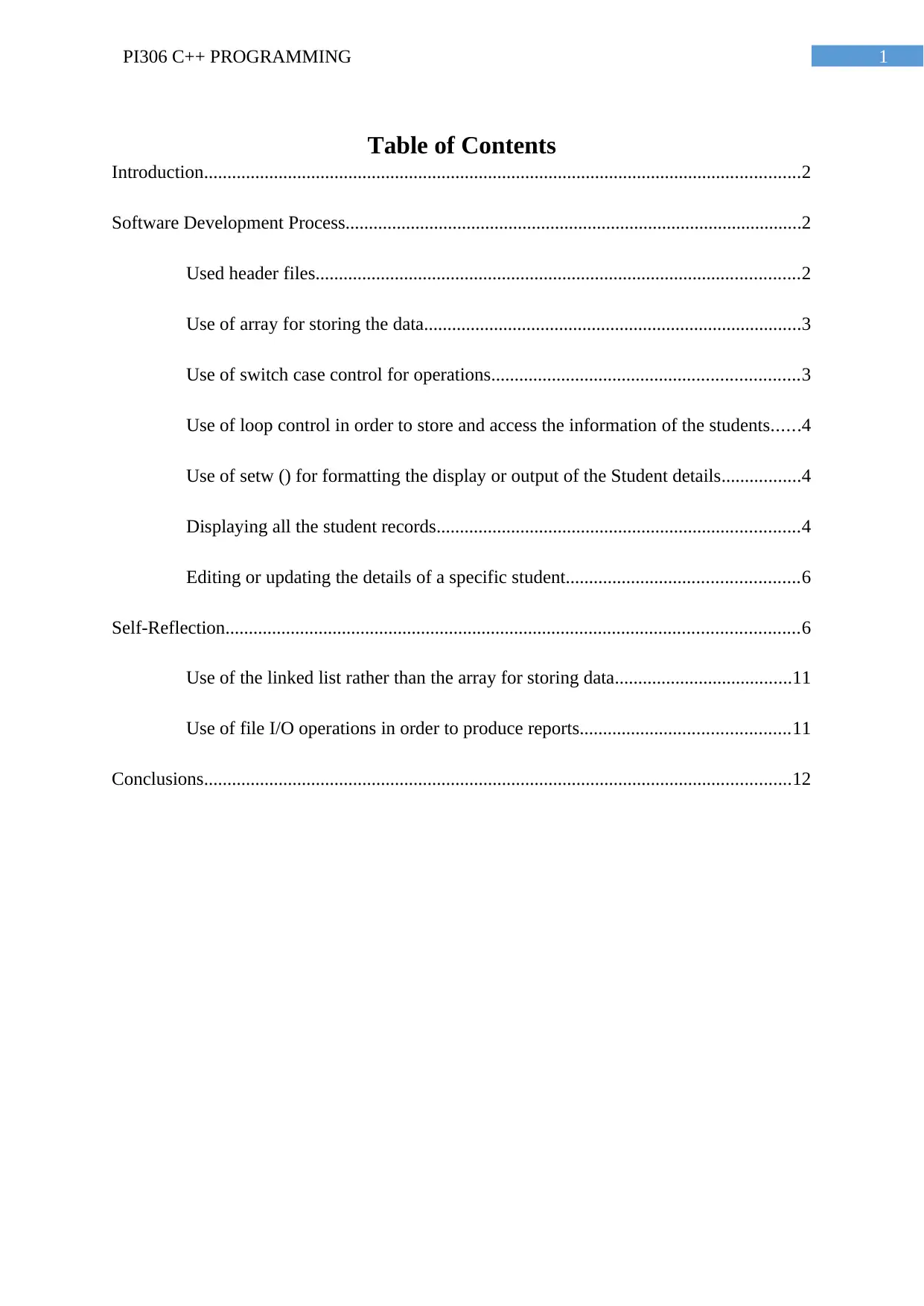
1PI306 C++ PROGRAMMING
Table of Contents
Introduction................................................................................................................................2
Software Development Process..................................................................................................2
Used header files........................................................................................................2
Use of array for storing the data.................................................................................3
Use of switch case control for operations..................................................................3
Use of loop control in order to store and access the information of the students......4
Use of setw () for formatting the display or output of the Student details.................4
Displaying all the student records..............................................................................4
Editing or updating the details of a specific student..................................................6
Self-Reflection...........................................................................................................................6
Use of the linked list rather than the array for storing data......................................11
Use of file I/O operations in order to produce reports.............................................11
Conclusions..............................................................................................................................12
Table of Contents
Introduction................................................................................................................................2
Software Development Process..................................................................................................2
Used header files........................................................................................................2
Use of array for storing the data.................................................................................3
Use of switch case control for operations..................................................................3
Use of loop control in order to store and access the information of the students......4
Use of setw () for formatting the display or output of the Student details.................4
Displaying all the student records..............................................................................4
Editing or updating the details of a specific student..................................................6
Self-Reflection...........................................................................................................................6
Use of the linked list rather than the array for storing data......................................11
Use of file I/O operations in order to produce reports.............................................11
Conclusions..............................................................................................................................12
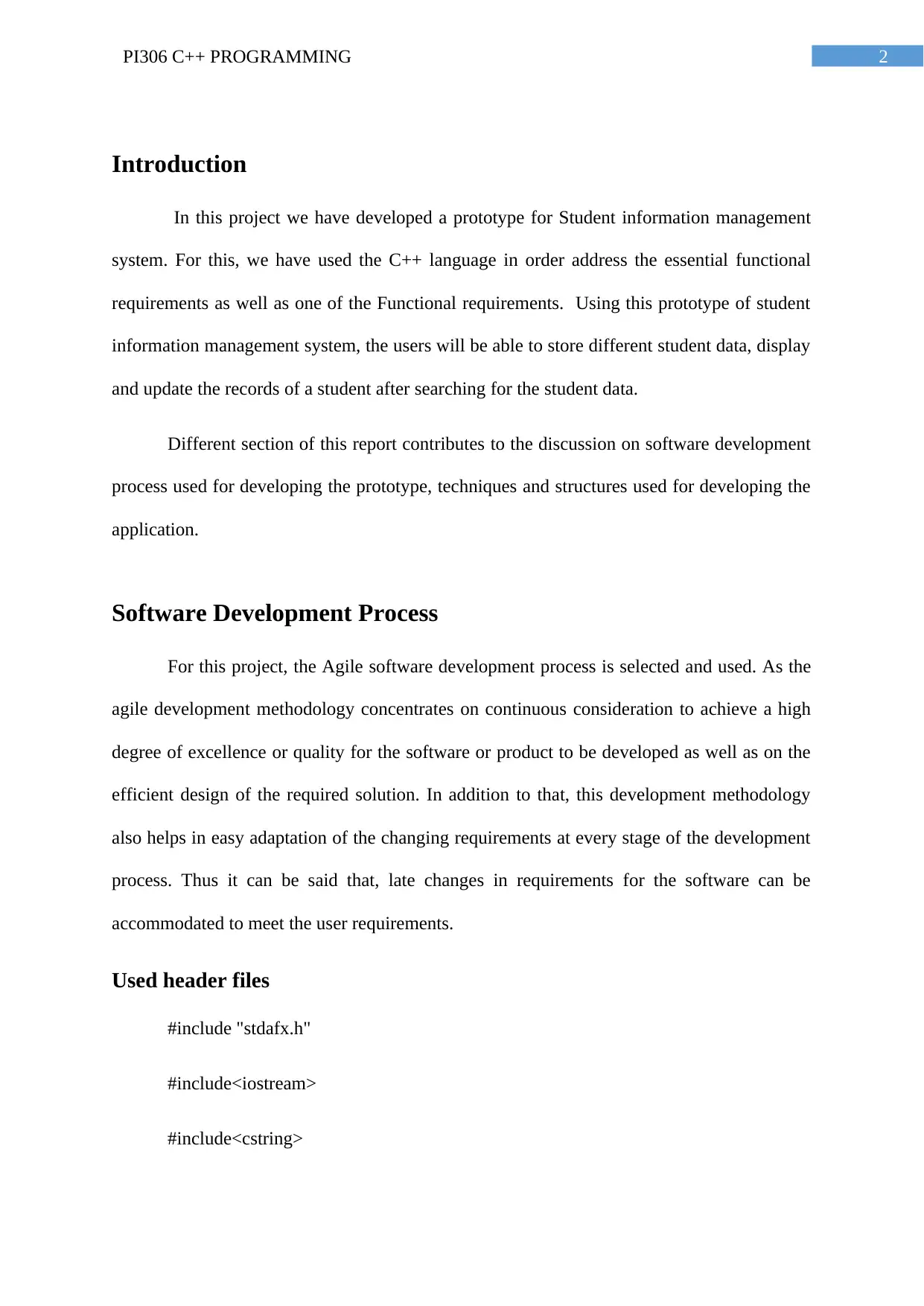
2PI306 C++ PROGRAMMING
Introduction
In this project we have developed a prototype for Student information management
system. For this, we have used the C++ language in order address the essential functional
requirements as well as one of the Functional requirements. Using this prototype of student
information management system, the users will be able to store different student data, display
and update the records of a student after searching for the student data.
Different section of this report contributes to the discussion on software development
process used for developing the prototype, techniques and structures used for developing the
application.
Software Development Process
For this project, the Agile software development process is selected and used. As the
agile development methodology concentrates on continuous consideration to achieve a high
degree of excellence or quality for the software or product to be developed as well as on the
efficient design of the required solution. In addition to that, this development methodology
also helps in easy adaptation of the changing requirements at every stage of the development
process. Thus it can be said that, late changes in requirements for the software can be
accommodated to meet the user requirements.
Used header files
#include "stdafx.h"
#include<iostream>
#include<cstring>
Introduction
In this project we have developed a prototype for Student information management
system. For this, we have used the C++ language in order address the essential functional
requirements as well as one of the Functional requirements. Using this prototype of student
information management system, the users will be able to store different student data, display
and update the records of a student after searching for the student data.
Different section of this report contributes to the discussion on software development
process used for developing the prototype, techniques and structures used for developing the
application.
Software Development Process
For this project, the Agile software development process is selected and used. As the
agile development methodology concentrates on continuous consideration to achieve a high
degree of excellence or quality for the software or product to be developed as well as on the
efficient design of the required solution. In addition to that, this development methodology
also helps in easy adaptation of the changing requirements at every stage of the development
process. Thus it can be said that, late changes in requirements for the software can be
accommodated to meet the user requirements.
Used header files
#include "stdafx.h"
#include<iostream>
#include<cstring>
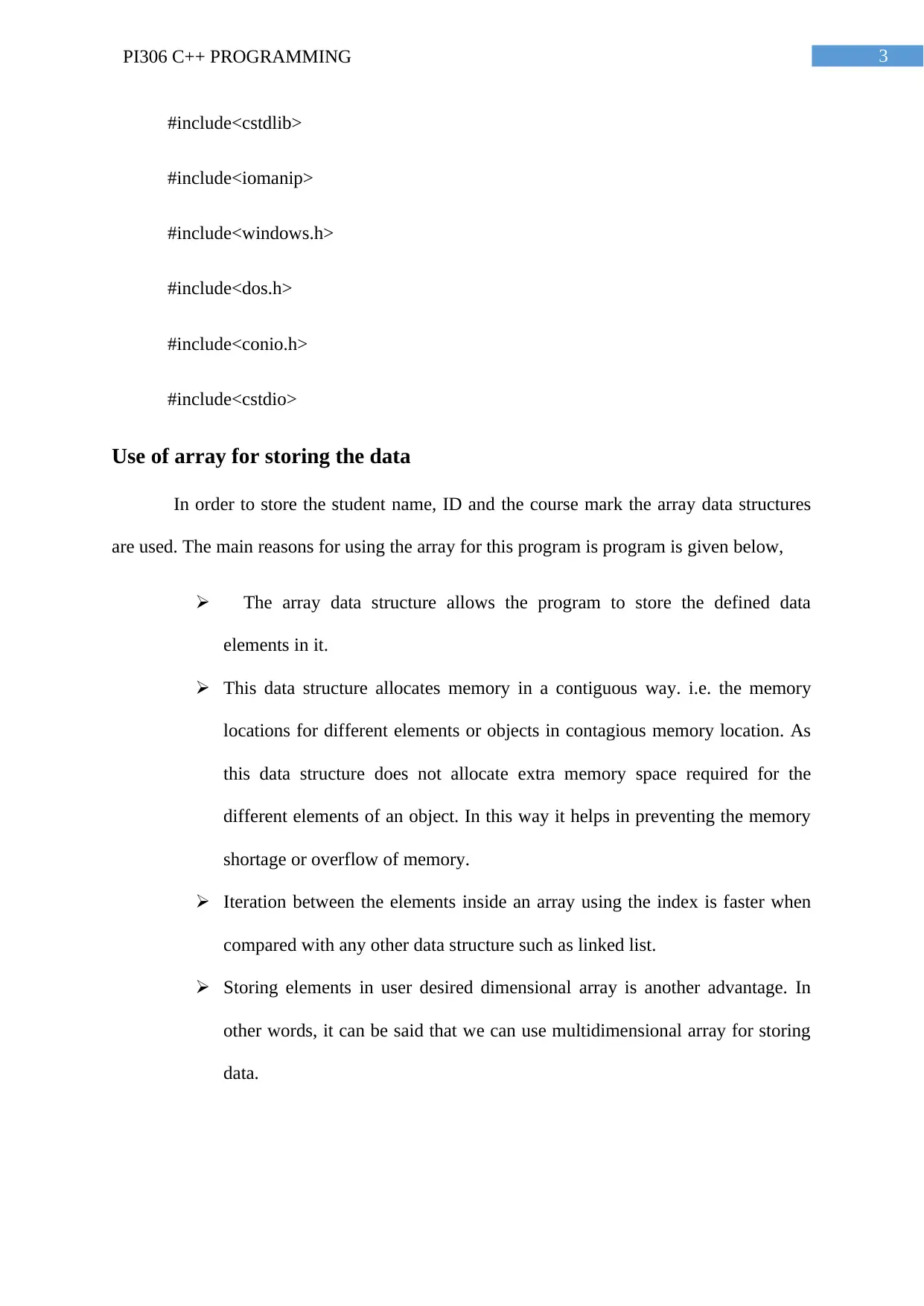
3PI306 C++ PROGRAMMING
#include<cstdlib>
#include<iomanip>
#include<windows.h>
#include<dos.h>
#include<conio.h>
#include<cstdio>
Use of array for storing the data
In order to store the student name, ID and the course mark the array data structures
are used. The main reasons for using the array for this program is program is given below,
The array data structure allows the program to store the defined data
elements in it.
This data structure allocates memory in a contiguous way. i.e. the memory
locations for different elements or objects in contagious memory location. As
this data structure does not allocate extra memory space required for the
different elements of an object. In this way it helps in preventing the memory
shortage or overflow of memory.
Iteration between the elements inside an array using the index is faster when
compared with any other data structure such as linked list.
Storing elements in user desired dimensional array is another advantage. In
other words, it can be said that we can use multidimensional array for storing
data.
#include<cstdlib>
#include<iomanip>
#include<windows.h>
#include<dos.h>
#include<conio.h>
#include<cstdio>
Use of array for storing the data
In order to store the student name, ID and the course mark the array data structures
are used. The main reasons for using the array for this program is program is given below,
The array data structure allows the program to store the defined data
elements in it.
This data structure allocates memory in a contiguous way. i.e. the memory
locations for different elements or objects in contagious memory location. As
this data structure does not allocate extra memory space required for the
different elements of an object. In this way it helps in preventing the memory
shortage or overflow of memory.
Iteration between the elements inside an array using the index is faster when
compared with any other data structure such as linked list.
Storing elements in user desired dimensional array is another advantage. In
other words, it can be said that we can use multidimensional array for storing
data.
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
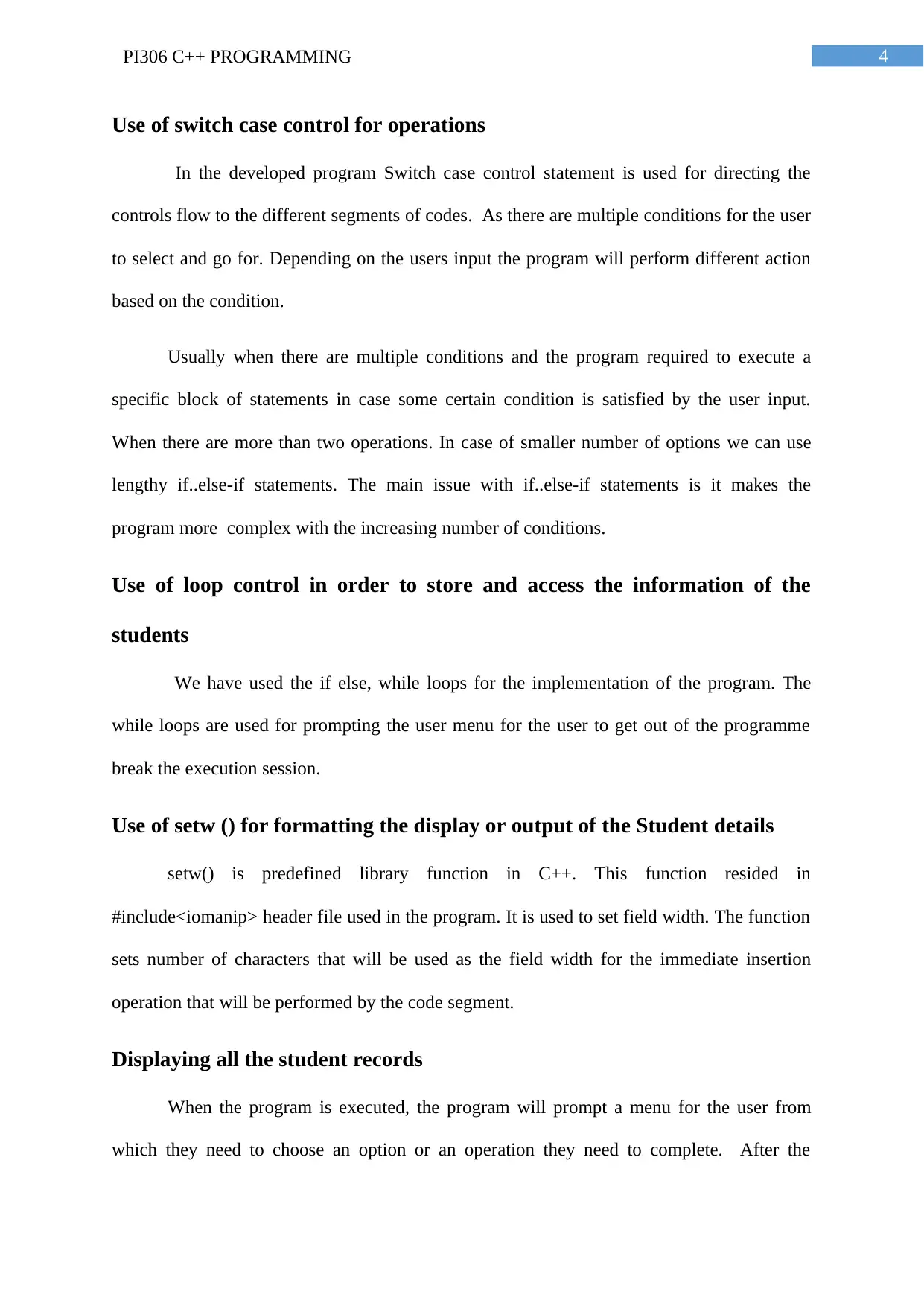
4PI306 C++ PROGRAMMING
Use of switch case control for operations
In the developed program Switch case control statement is used for directing the
controls flow to the different segments of codes. As there are multiple conditions for the user
to select and go for. Depending on the users input the program will perform different action
based on the condition.
Usually when there are multiple conditions and the program required to execute a
specific block of statements in case some certain condition is satisfied by the user input.
When there are more than two operations. In case of smaller number of options we can use
lengthy if..else-if statements. The main issue with if..else-if statements is it makes the
program more complex with the increasing number of conditions.
Use of loop control in order to store and access the information of the
students
We have used the if else, while loops for the implementation of the program. The
while loops are used for prompting the user menu for the user to get out of the programme
break the execution session.
Use of setw () for formatting the display or output of the Student details
setw() is predefined library function in C++. This function resided in
#include<iomanip> header file used in the program. It is used to set field width. The function
sets number of characters that will be used as the field width for the immediate insertion
operation that will be performed by the code segment.
Displaying all the student records
When the program is executed, the program will prompt a menu for the user from
which they need to choose an option or an operation they need to complete. After the
Use of switch case control for operations
In the developed program Switch case control statement is used for directing the
controls flow to the different segments of codes. As there are multiple conditions for the user
to select and go for. Depending on the users input the program will perform different action
based on the condition.
Usually when there are multiple conditions and the program required to execute a
specific block of statements in case some certain condition is satisfied by the user input.
When there are more than two operations. In case of smaller number of options we can use
lengthy if..else-if statements. The main issue with if..else-if statements is it makes the
program more complex with the increasing number of conditions.
Use of loop control in order to store and access the information of the
students
We have used the if else, while loops for the implementation of the program. The
while loops are used for prompting the user menu for the user to get out of the programme
break the execution session.
Use of setw () for formatting the display or output of the Student details
setw() is predefined library function in C++. This function resided in
#include<iomanip> header file used in the program. It is used to set field width. The function
sets number of characters that will be used as the field width for the immediate insertion
operation that will be performed by the code segment.
Displaying all the student records
When the program is executed, the program will prompt a menu for the user from
which they need to choose an option or an operation they need to complete. After the
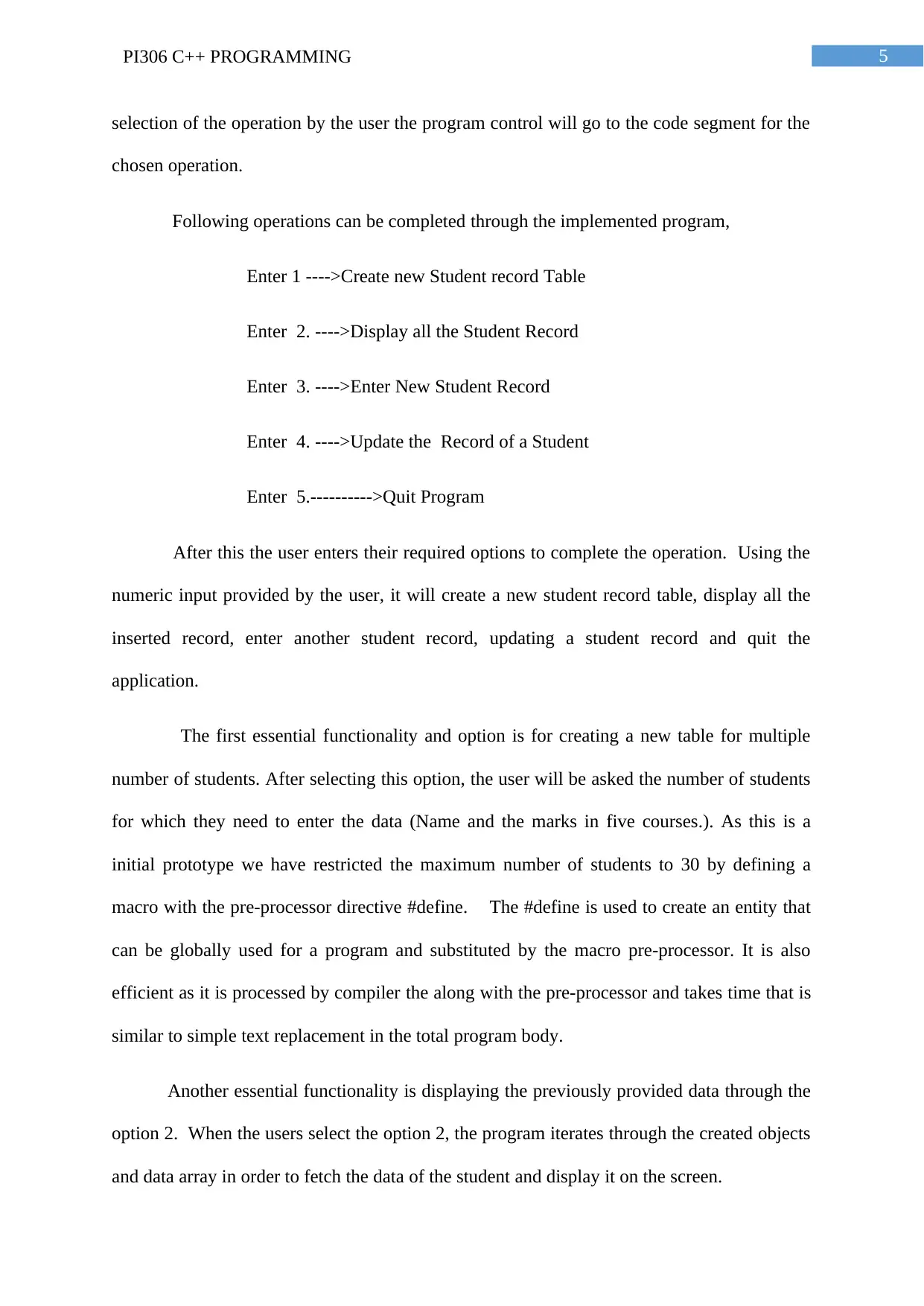
5PI306 C++ PROGRAMMING
selection of the operation by the user the program control will go to the code segment for the
chosen operation.
Following operations can be completed through the implemented program,
Enter 1 ---->Create new Student record Table
Enter 2. ---->Display all the Student Record
Enter 3. ---->Enter New Student Record
Enter 4. ---->Update the Record of a Student
Enter 5.---------->Quit Program
After this the user enters their required options to complete the operation. Using the
numeric input provided by the user, it will create a new student record table, display all the
inserted record, enter another student record, updating a student record and quit the
application.
The first essential functionality and option is for creating a new table for multiple
number of students. After selecting this option, the user will be asked the number of students
for which they need to enter the data (Name and the marks in five courses.). As this is a
initial prototype we have restricted the maximum number of students to 30 by defining a
macro with the pre-processor directive #define. The #define is used to create an entity that
can be globally used for a program and substituted by the macro pre-processor. It is also
efficient as it is processed by compiler the along with the pre-processor and takes time that is
similar to simple text replacement in the total program body.
Another essential functionality is displaying the previously provided data through the
option 2. When the users select the option 2, the program iterates through the created objects
and data array in order to fetch the data of the student and display it on the screen.
selection of the operation by the user the program control will go to the code segment for the
chosen operation.
Following operations can be completed through the implemented program,
Enter 1 ---->Create new Student record Table
Enter 2. ---->Display all the Student Record
Enter 3. ---->Enter New Student Record
Enter 4. ---->Update the Record of a Student
Enter 5.---------->Quit Program
After this the user enters their required options to complete the operation. Using the
numeric input provided by the user, it will create a new student record table, display all the
inserted record, enter another student record, updating a student record and quit the
application.
The first essential functionality and option is for creating a new table for multiple
number of students. After selecting this option, the user will be asked the number of students
for which they need to enter the data (Name and the marks in five courses.). As this is a
initial prototype we have restricted the maximum number of students to 30 by defining a
macro with the pre-processor directive #define. The #define is used to create an entity that
can be globally used for a program and substituted by the macro pre-processor. It is also
efficient as it is processed by compiler the along with the pre-processor and takes time that is
similar to simple text replacement in the total program body.
Another essential functionality is displaying the previously provided data through the
option 2. When the users select the option 2, the program iterates through the created objects
and data array in order to fetch the data of the student and display it on the screen.
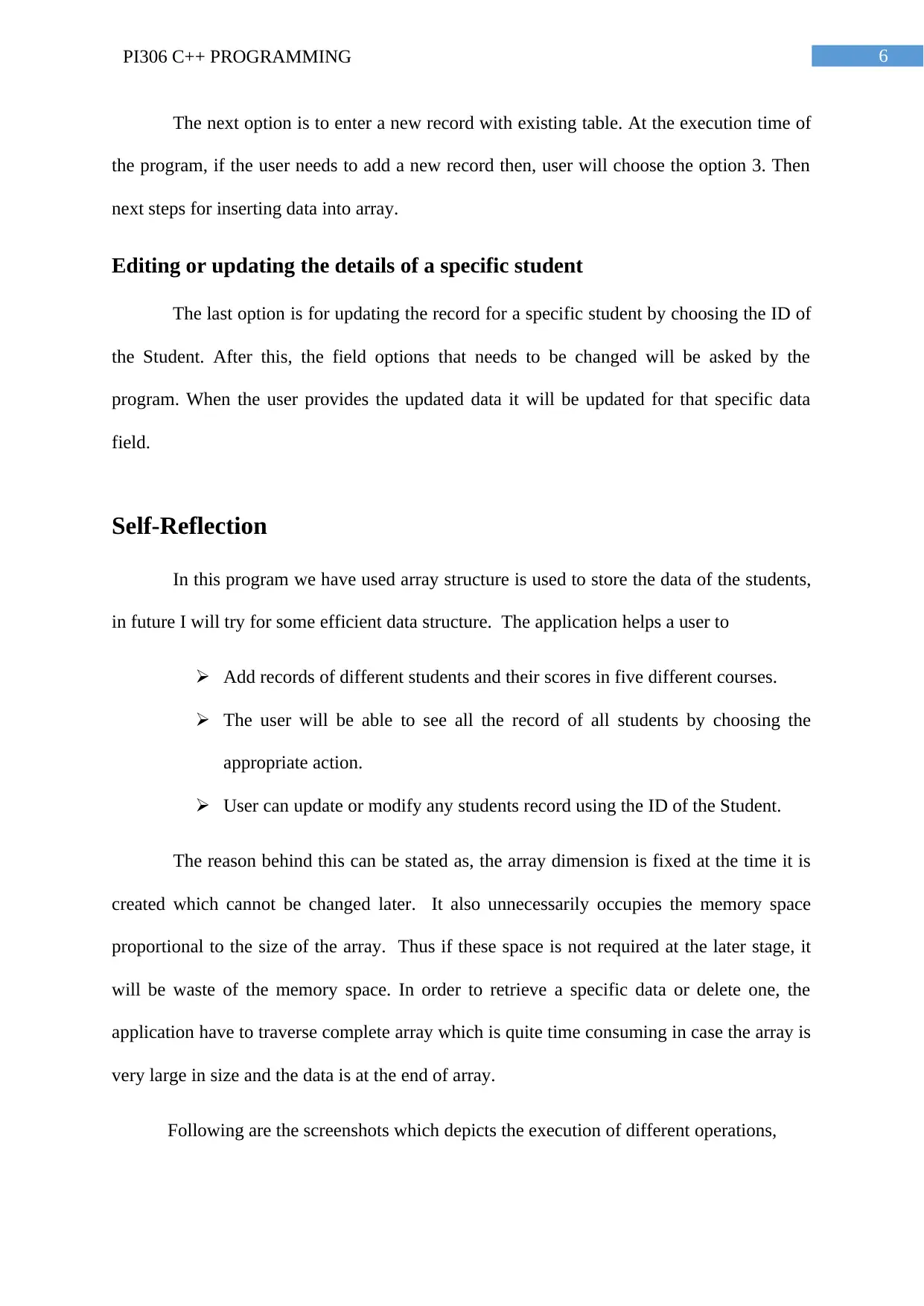
6PI306 C++ PROGRAMMING
The next option is to enter a new record with existing table. At the execution time of
the program, if the user needs to add a new record then, user will choose the option 3. Then
next steps for inserting data into array.
Editing or updating the details of a specific student
The last option is for updating the record for a specific student by choosing the ID of
the Student. After this, the field options that needs to be changed will be asked by the
program. When the user provides the updated data it will be updated for that specific data
field.
Self-Reflection
In this program we have used array structure is used to store the data of the students,
in future I will try for some efficient data structure. The application helps a user to
Add records of different students and their scores in five different courses.
The user will be able to see all the record of all students by choosing the
appropriate action.
User can update or modify any students record using the ID of the Student.
The reason behind this can be stated as, the array dimension is fixed at the time it is
created which cannot be changed later. It also unnecessarily occupies the memory space
proportional to the size of the array. Thus if these space is not required at the later stage, it
will be waste of the memory space. In order to retrieve a specific data or delete one, the
application have to traverse complete array which is quite time consuming in case the array is
very large in size and the data is at the end of array.
Following are the screenshots which depicts the execution of different operations,
The next option is to enter a new record with existing table. At the execution time of
the program, if the user needs to add a new record then, user will choose the option 3. Then
next steps for inserting data into array.
Editing or updating the details of a specific student
The last option is for updating the record for a specific student by choosing the ID of
the Student. After this, the field options that needs to be changed will be asked by the
program. When the user provides the updated data it will be updated for that specific data
field.
Self-Reflection
In this program we have used array structure is used to store the data of the students,
in future I will try for some efficient data structure. The application helps a user to
Add records of different students and their scores in five different courses.
The user will be able to see all the record of all students by choosing the
appropriate action.
User can update or modify any students record using the ID of the Student.
The reason behind this can be stated as, the array dimension is fixed at the time it is
created which cannot be changed later. It also unnecessarily occupies the memory space
proportional to the size of the array. Thus if these space is not required at the later stage, it
will be waste of the memory space. In order to retrieve a specific data or delete one, the
application have to traverse complete array which is quite time consuming in case the array is
very large in size and the data is at the end of array.
Following are the screenshots which depicts the execution of different operations,
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
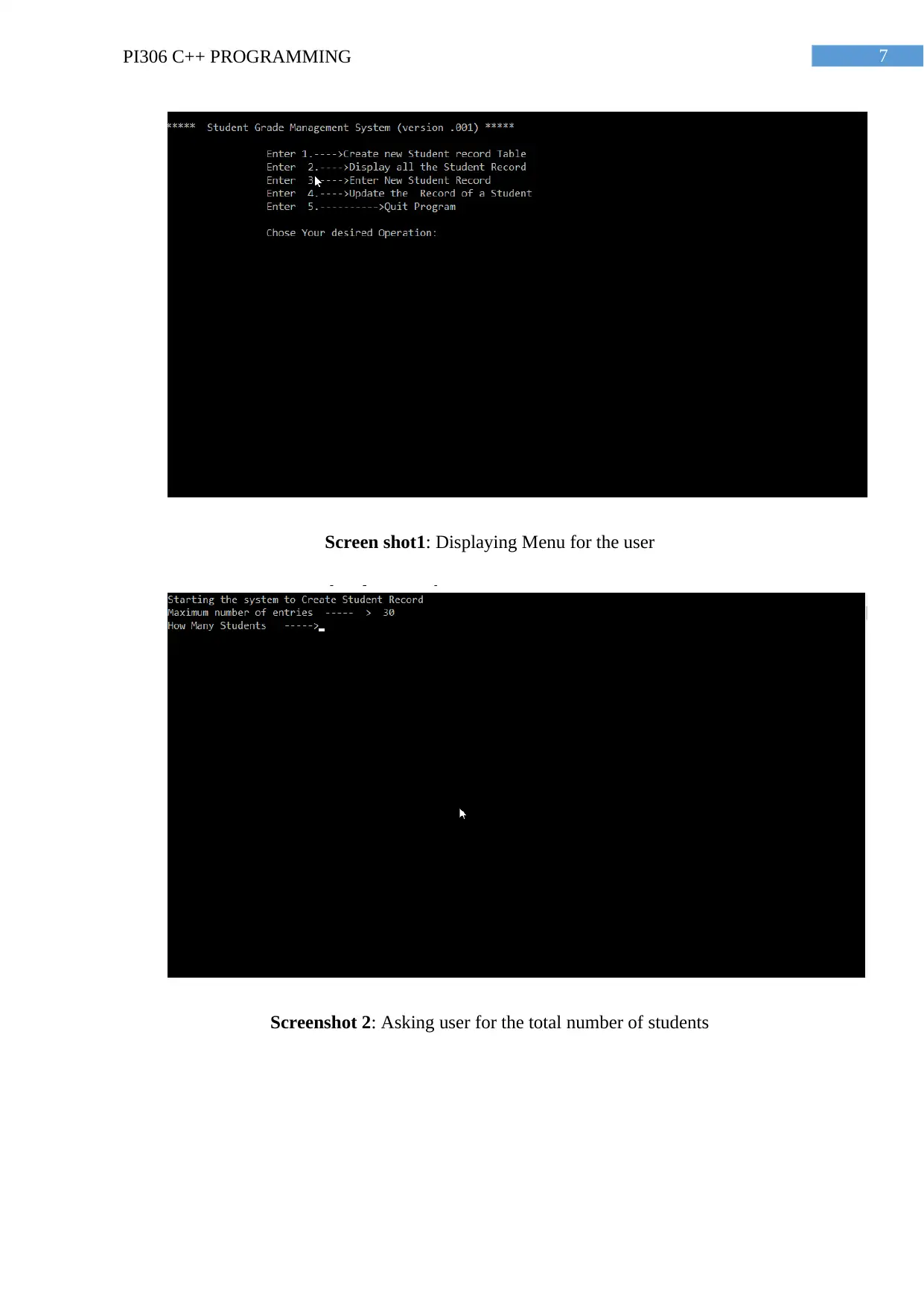
7PI306 C++ PROGRAMMING
Screen shot1: Displaying Menu for the user
Screenshot 2: Asking user for the total number of students
Screen shot1: Displaying Menu for the user
Screenshot 2: Asking user for the total number of students
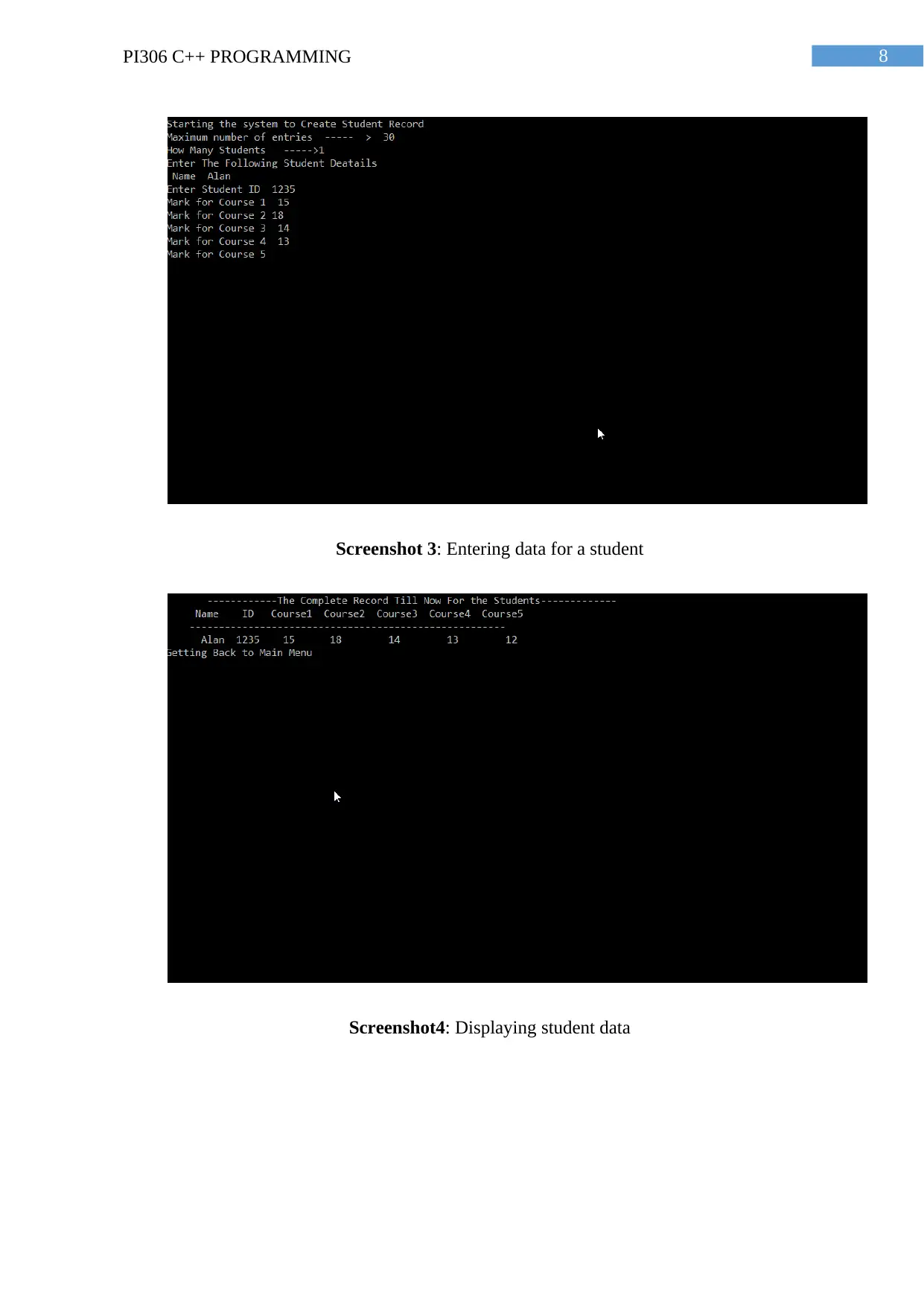
8PI306 C++ PROGRAMMING
Screenshot 3: Entering data for a student
Screenshot4: Displaying student data
Screenshot 3: Entering data for a student
Screenshot4: Displaying student data
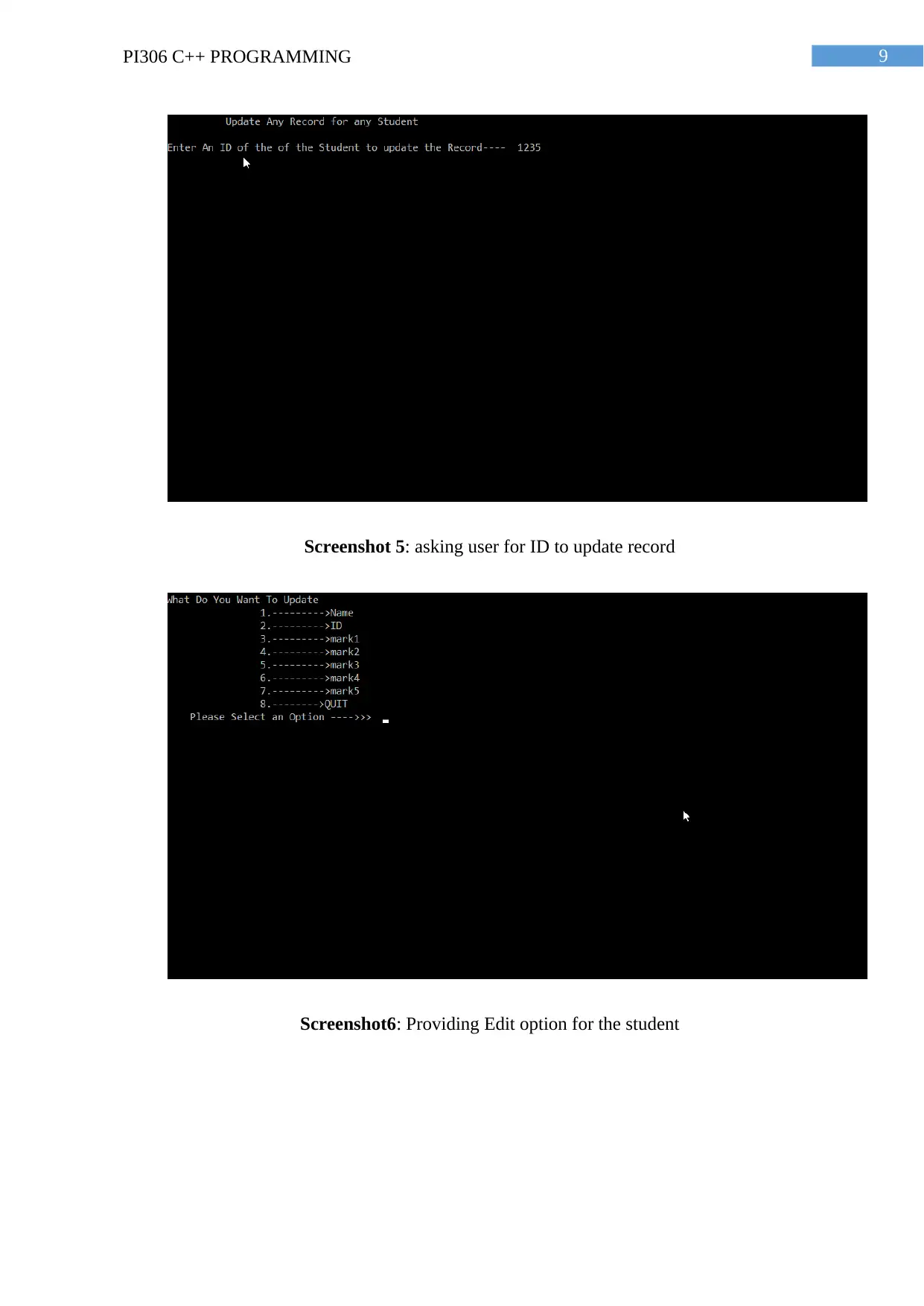
9PI306 C++ PROGRAMMING
Screenshot 5: asking user for ID to update record
Screenshot6: Providing Edit option for the student
Screenshot 5: asking user for ID to update record
Screenshot6: Providing Edit option for the student
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
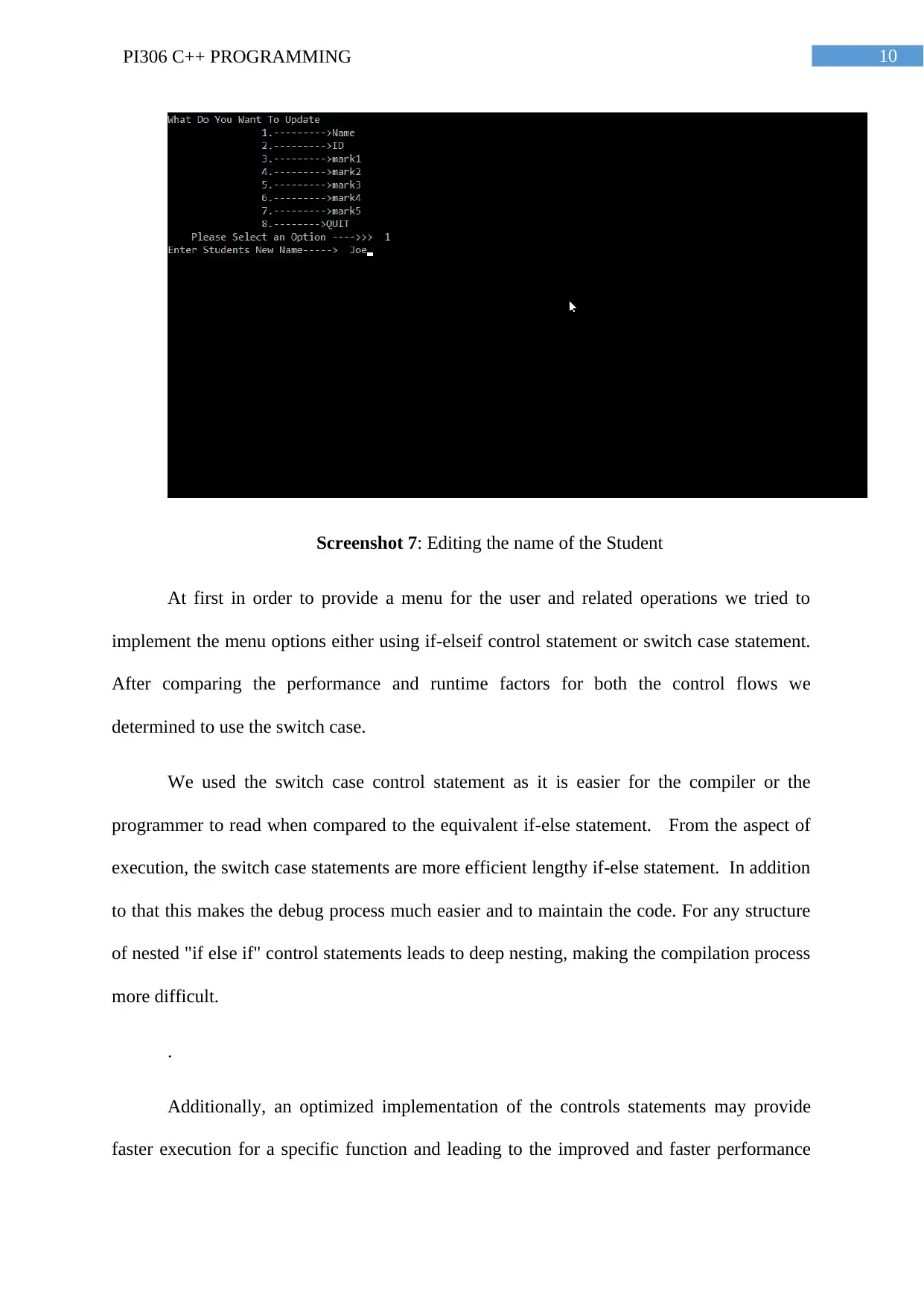
10PI306 C++ PROGRAMMING
Screenshot 7: Editing the name of the Student
At first in order to provide a menu for the user and related operations we tried to
implement the menu options either using if-elseif control statement or switch case statement.
After comparing the performance and runtime factors for both the control flows we
determined to use the switch case.
We used the switch case control statement as it is easier for the compiler or the
programmer to read when compared to the equivalent if-else statement. From the aspect of
execution, the switch case statements are more efficient lengthy if-else statement. In addition
to that this makes the debug process much easier and to maintain the code. For any structure
of nested "if else if" control statements leads to deep nesting, making the compilation process
more difficult.
.
Additionally, an optimized implementation of the controls statements may provide
faster execution for a specific function and leading to the improved and faster performance
Screenshot 7: Editing the name of the Student
At first in order to provide a menu for the user and related operations we tried to
implement the menu options either using if-elseif control statement or switch case statement.
After comparing the performance and runtime factors for both the control flows we
determined to use the switch case.
We used the switch case control statement as it is easier for the compiler or the
programmer to read when compared to the equivalent if-else statement. From the aspect of
execution, the switch case statements are more efficient lengthy if-else statement. In addition
to that this makes the debug process much easier and to maintain the code. For any structure
of nested "if else if" control statements leads to deep nesting, making the compilation process
more difficult.
.
Additionally, an optimized implementation of the controls statements may provide
faster execution for a specific function and leading to the improved and faster performance
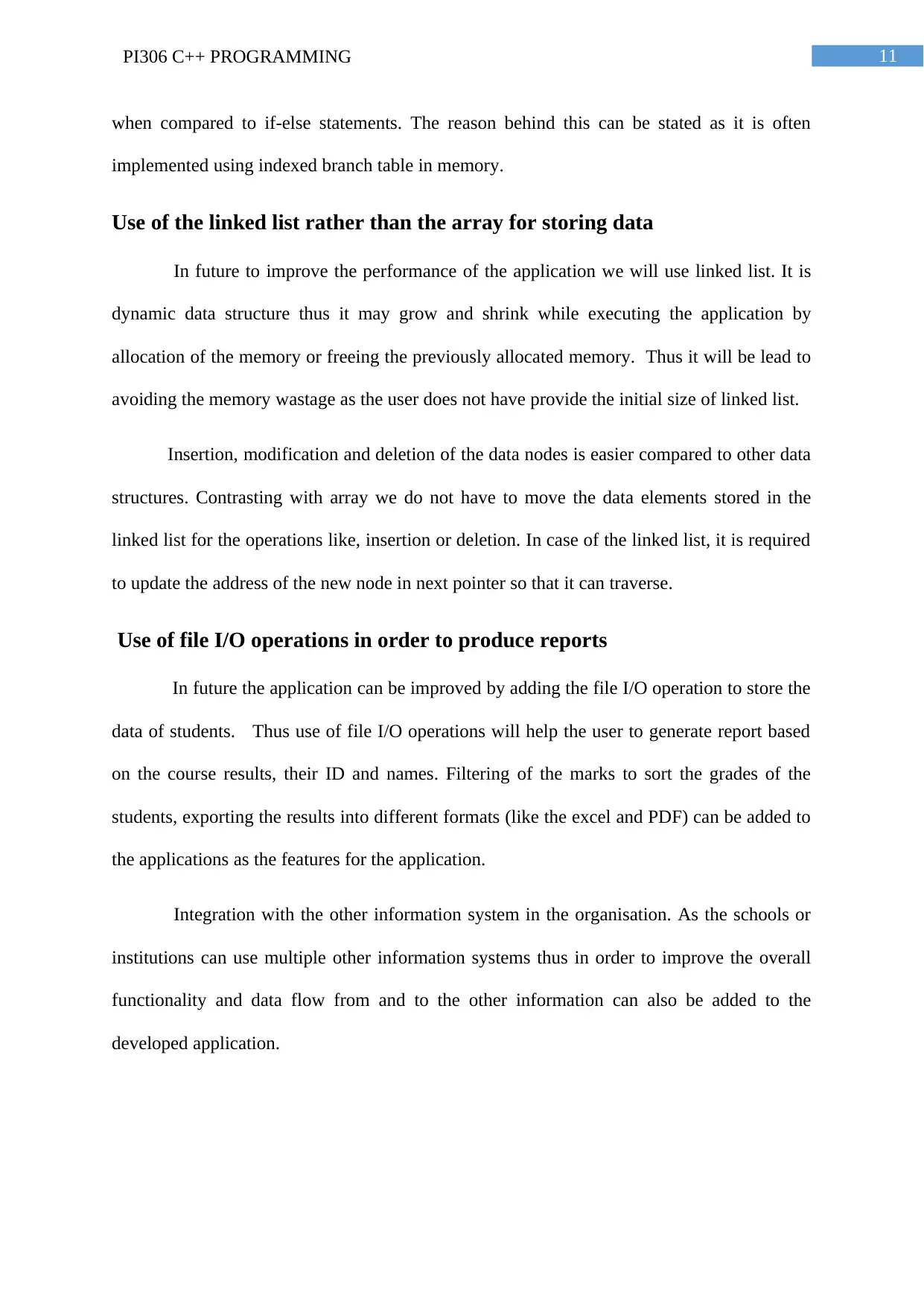
11PI306 C++ PROGRAMMING
when compared to if-else statements. The reason behind this can be stated as it is often
implemented using indexed branch table in memory.
Use of the linked list rather than the array for storing data
In future to improve the performance of the application we will use linked list. It is
dynamic data structure thus it may grow and shrink while executing the application by
allocation of the memory or freeing the previously allocated memory. Thus it will be lead to
avoiding the memory wastage as the user does not have provide the initial size of linked list.
Insertion, modification and deletion of the data nodes is easier compared to other data
structures. Contrasting with array we do not have to move the data elements stored in the
linked list for the operations like, insertion or deletion. In case of the linked list, it is required
to update the address of the new node in next pointer so that it can traverse.
Use of file I/O operations in order to produce reports
In future the application can be improved by adding the file I/O operation to store the
data of students. Thus use of file I/O operations will help the user to generate report based
on the course results, their ID and names. Filtering of the marks to sort the grades of the
students, exporting the results into different formats (like the excel and PDF) can be added to
the applications as the features for the application.
Integration with the other information system in the organisation. As the schools or
institutions can use multiple other information systems thus in order to improve the overall
functionality and data flow from and to the other information can also be added to the
developed application.
when compared to if-else statements. The reason behind this can be stated as it is often
implemented using indexed branch table in memory.
Use of the linked list rather than the array for storing data
In future to improve the performance of the application we will use linked list. It is
dynamic data structure thus it may grow and shrink while executing the application by
allocation of the memory or freeing the previously allocated memory. Thus it will be lead to
avoiding the memory wastage as the user does not have provide the initial size of linked list.
Insertion, modification and deletion of the data nodes is easier compared to other data
structures. Contrasting with array we do not have to move the data elements stored in the
linked list for the operations like, insertion or deletion. In case of the linked list, it is required
to update the address of the new node in next pointer so that it can traverse.
Use of file I/O operations in order to produce reports
In future the application can be improved by adding the file I/O operation to store the
data of students. Thus use of file I/O operations will help the user to generate report based
on the course results, their ID and names. Filtering of the marks to sort the grades of the
students, exporting the results into different formats (like the excel and PDF) can be added to
the applications as the features for the application.
Integration with the other information system in the organisation. As the schools or
institutions can use multiple other information systems thus in order to improve the overall
functionality and data flow from and to the other information can also be added to the
developed application.
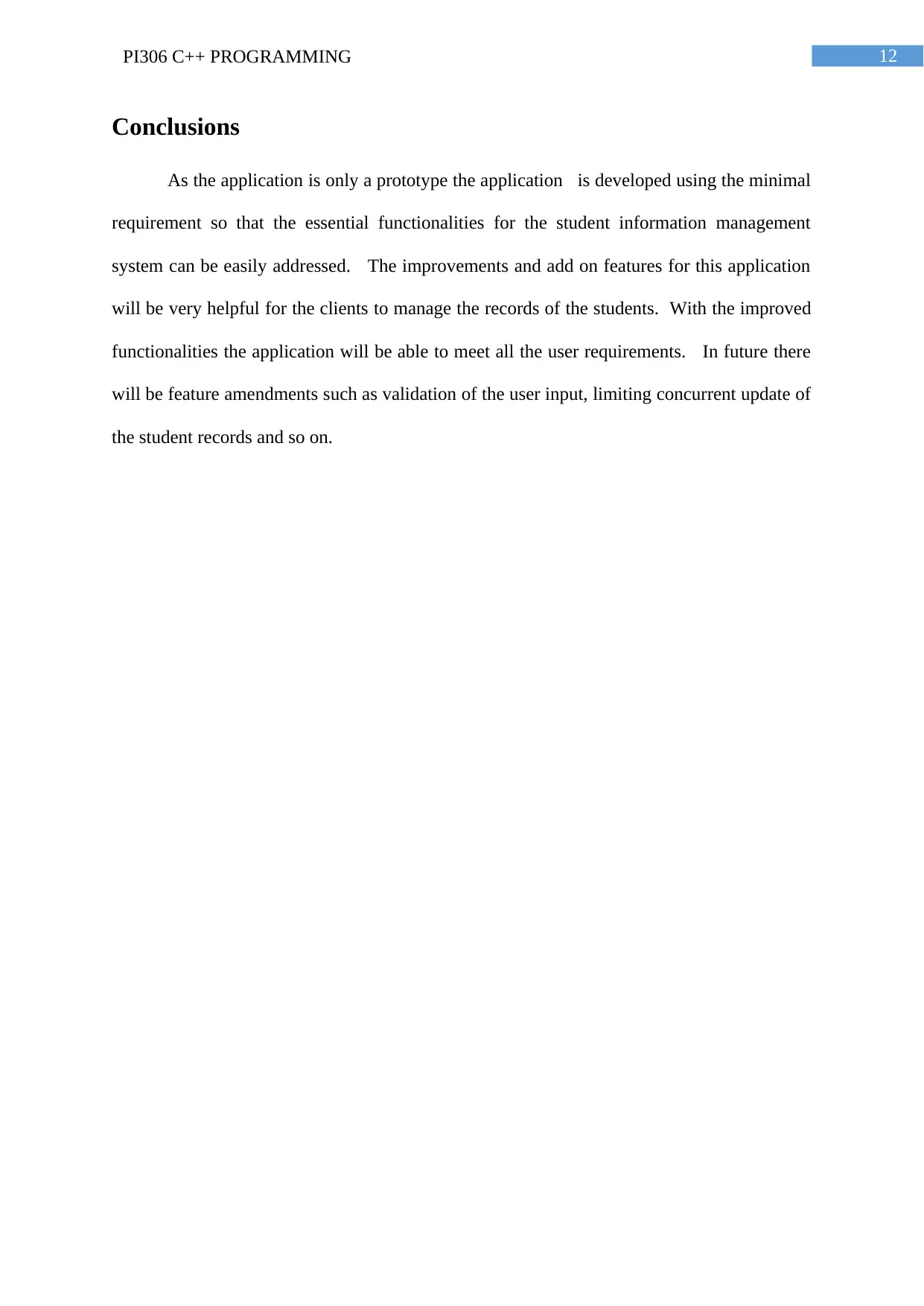
12PI306 C++ PROGRAMMING
Conclusions
As the application is only a prototype the application is developed using the minimal
requirement so that the essential functionalities for the student information management
system can be easily addressed. The improvements and add on features for this application
will be very helpful for the clients to manage the records of the students. With the improved
functionalities the application will be able to meet all the user requirements. In future there
will be feature amendments such as validation of the user input, limiting concurrent update of
the student records and so on.
Conclusions
As the application is only a prototype the application is developed using the minimal
requirement so that the essential functionalities for the student information management
system can be easily addressed. The improvements and add on features for this application
will be very helpful for the clients to manage the records of the students. With the improved
functionalities the application will be able to meet all the user requirements. In future there
will be feature amendments such as validation of the user input, limiting concurrent update of
the student records and so on.
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
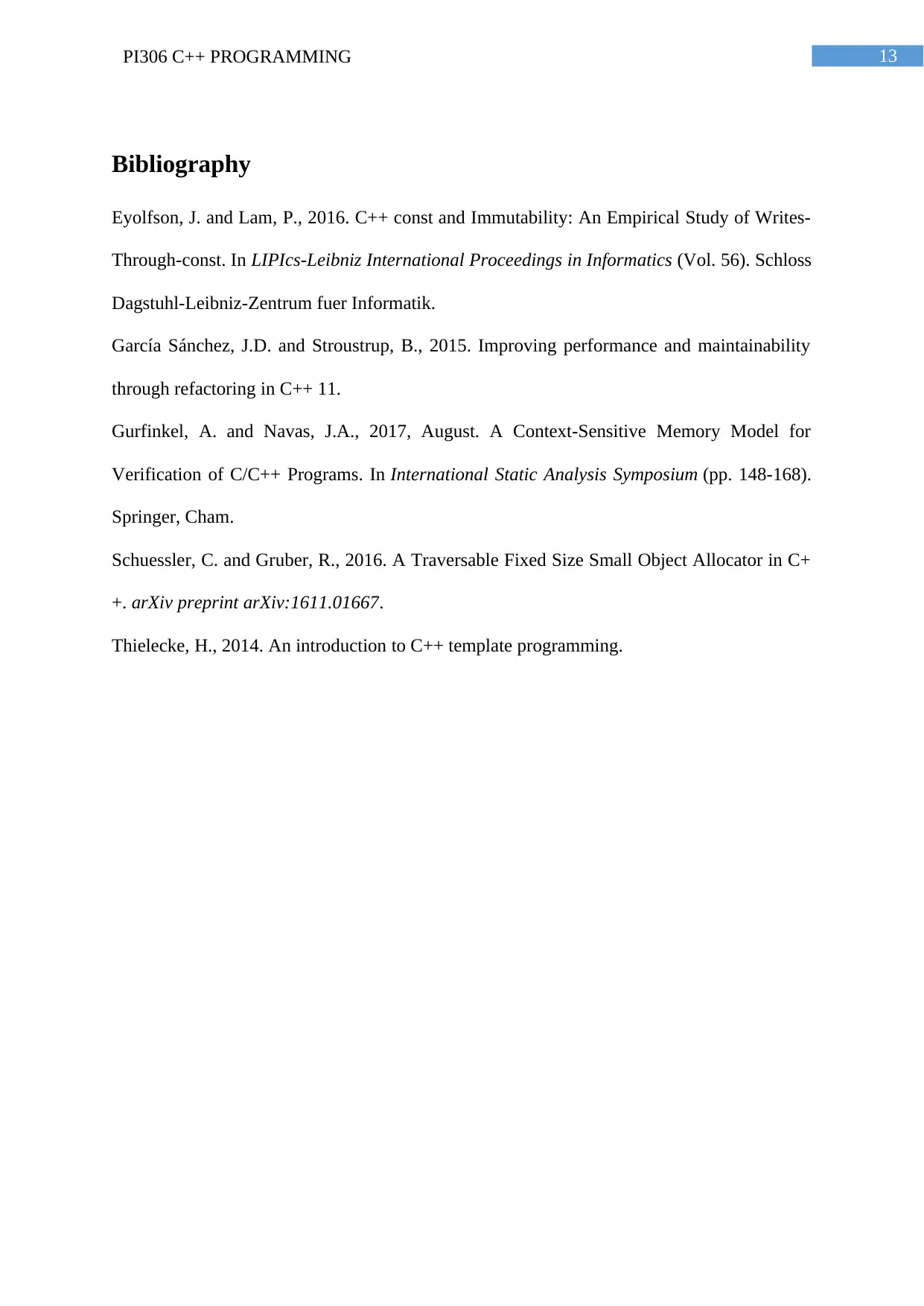
13PI306 C++ PROGRAMMING
Bibliography
Eyolfson, J. and Lam, P., 2016. C++ const and Immutability: An Empirical Study of Writes-
Through-const. In LIPIcs-Leibniz International Proceedings in Informatics (Vol. 56). Schloss
Dagstuhl-Leibniz-Zentrum fuer Informatik.
García Sánchez, J.D. and Stroustrup, B., 2015. Improving performance and maintainability
through refactoring in C++ 11.
Gurfinkel, A. and Navas, J.A., 2017, August. A Context-Sensitive Memory Model for
Verification of C/C++ Programs. In International Static Analysis Symposium (pp. 148-168).
Springer, Cham.
Schuessler, C. and Gruber, R., 2016. A Traversable Fixed Size Small Object Allocator in C+
+. arXiv preprint arXiv:1611.01667.
Thielecke, H., 2014. An introduction to C++ template programming.
Bibliography
Eyolfson, J. and Lam, P., 2016. C++ const and Immutability: An Empirical Study of Writes-
Through-const. In LIPIcs-Leibniz International Proceedings in Informatics (Vol. 56). Schloss
Dagstuhl-Leibniz-Zentrum fuer Informatik.
García Sánchez, J.D. and Stroustrup, B., 2015. Improving performance and maintainability
through refactoring in C++ 11.
Gurfinkel, A. and Navas, J.A., 2017, August. A Context-Sensitive Memory Model for
Verification of C/C++ Programs. In International Static Analysis Symposium (pp. 148-168).
Springer, Cham.
Schuessler, C. and Gruber, R., 2016. A Traversable Fixed Size Small Object Allocator in C+
+. arXiv preprint arXiv:1611.01667.
Thielecke, H., 2014. An introduction to C++ template programming.
1 out of 14
Related Documents
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.