Word Puzzle Game
VerifiedAdded on  2019/10/18
|8
|2069
|314
Report
AI Summary
The assignment content is related to a word search game where the user needs to find the words on a puzzle board. The program generates the puzzle board and displays it along with the list of selected words. It then enters a loop where the user can enter the word they want to find, or if they choose to quit. If the user enters a correct word, they need to specify the cells that contain the first letter of the word. If both cell selections are correct, the program marks the word as found. The game continues until all words are found or the user decides to quit.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
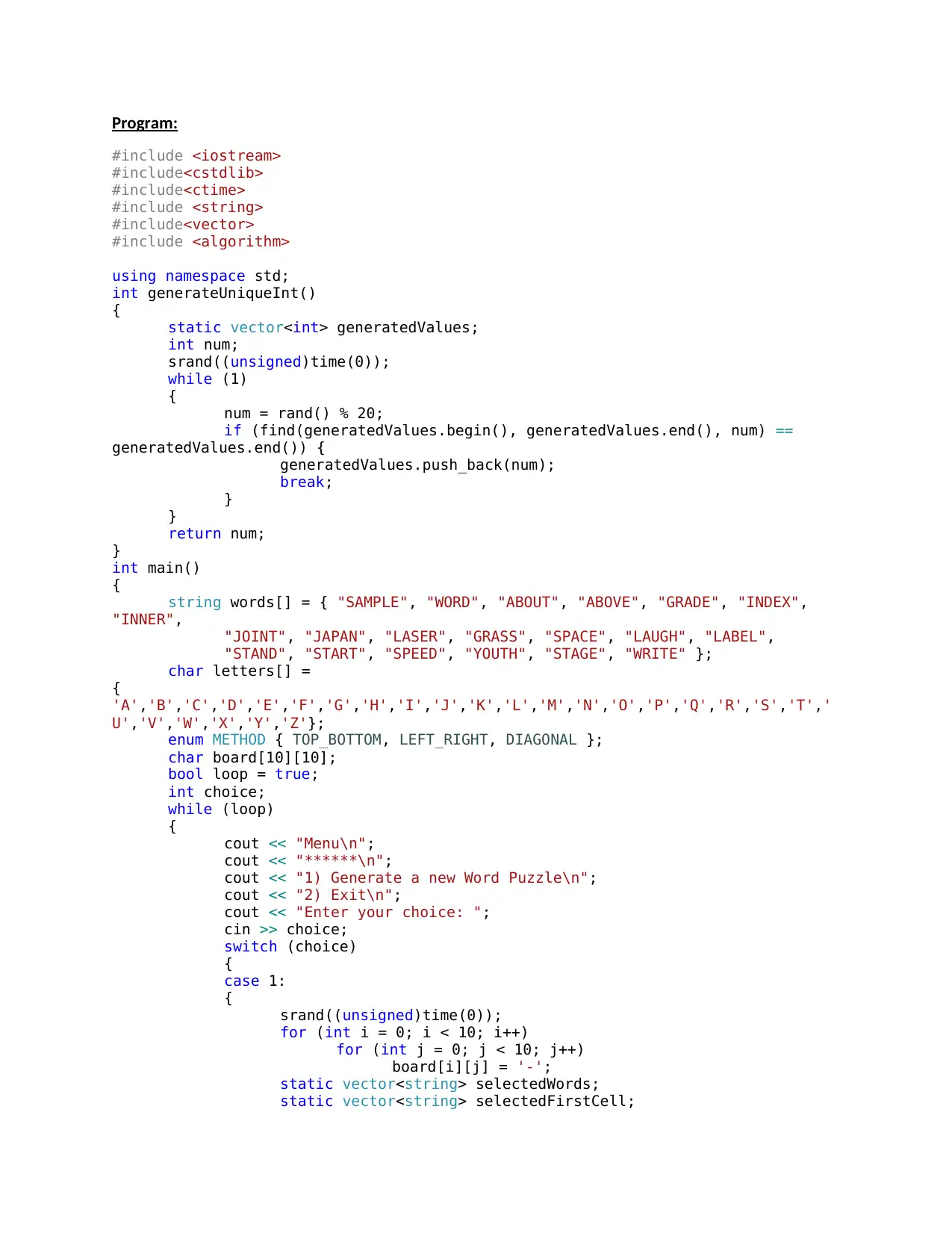
Program:
#include <iostream>
#include<cstdlib>
#include<ctime>
#include <string>
#include<vector>
#include <algorithm>
using namespace std;
int generateUniqueInt()
{
static vector<int> generatedValues;
int num;
srand((unsigned)time(0));
while (1)
{
num = rand() % 20;
if (find(generatedValues.begin(), generatedValues.end(), num) ==
generatedValues.end()) {
generatedValues.push_back(num);
break;
}
}
return num;
}
int main()
{
string words[] = { "SAMPLE", "WORD", "ABOUT", "ABOVE", "GRADE", "INDEX",
"INNER",
"JOINT", "JAPAN", "LASER", "GRASS", "SPACE", "LAUGH", "LABEL",
"STAND", "START", "SPEED", "YOUTH", "STAGE", "WRITE" };
char letters[] =
{
'A','B','C','D','E','F','G','H','I','J','K','L','M','N','O','P','Q','R','S','T','
U','V','W','X','Y','Z'};
enum METHOD { TOP_BOTTOM, LEFT_RIGHT, DIAGONAL };
char board[10][10];
bool loop = true;
int choice;
while (loop)
{
cout << "Menu\n";
cout << "******\n";
cout << "1) Generate a new Word Puzzle\n";
cout << "2) Exit\n";
cout << "Enter your choice: ";
cin >> choice;
switch (choice)
{
case 1:
{
srand((unsigned)time(0));
for (int i = 0; i < 10; i++)
for (int j = 0; j < 10; j++)
board[i][j] = '-';
static vector<string> selectedWords;
static vector<string> selectedFirstCell;
#include <iostream>
#include<cstdlib>
#include<ctime>
#include <string>
#include<vector>
#include <algorithm>
using namespace std;
int generateUniqueInt()
{
static vector<int> generatedValues;
int num;
srand((unsigned)time(0));
while (1)
{
num = rand() % 20;
if (find(generatedValues.begin(), generatedValues.end(), num) ==
generatedValues.end()) {
generatedValues.push_back(num);
break;
}
}
return num;
}
int main()
{
string words[] = { "SAMPLE", "WORD", "ABOUT", "ABOVE", "GRADE", "INDEX",
"INNER",
"JOINT", "JAPAN", "LASER", "GRASS", "SPACE", "LAUGH", "LABEL",
"STAND", "START", "SPEED", "YOUTH", "STAGE", "WRITE" };
char letters[] =
{
'A','B','C','D','E','F','G','H','I','J','K','L','M','N','O','P','Q','R','S','T','
U','V','W','X','Y','Z'};
enum METHOD { TOP_BOTTOM, LEFT_RIGHT, DIAGONAL };
char board[10][10];
bool loop = true;
int choice;
while (loop)
{
cout << "Menu\n";
cout << "******\n";
cout << "1) Generate a new Word Puzzle\n";
cout << "2) Exit\n";
cout << "Enter your choice: ";
cin >> choice;
switch (choice)
{
case 1:
{
srand((unsigned)time(0));
for (int i = 0; i < 10; i++)
for (int j = 0; j < 10; j++)
board[i][j] = '-';
static vector<string> selectedWords;
static vector<string> selectedFirstCell;
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
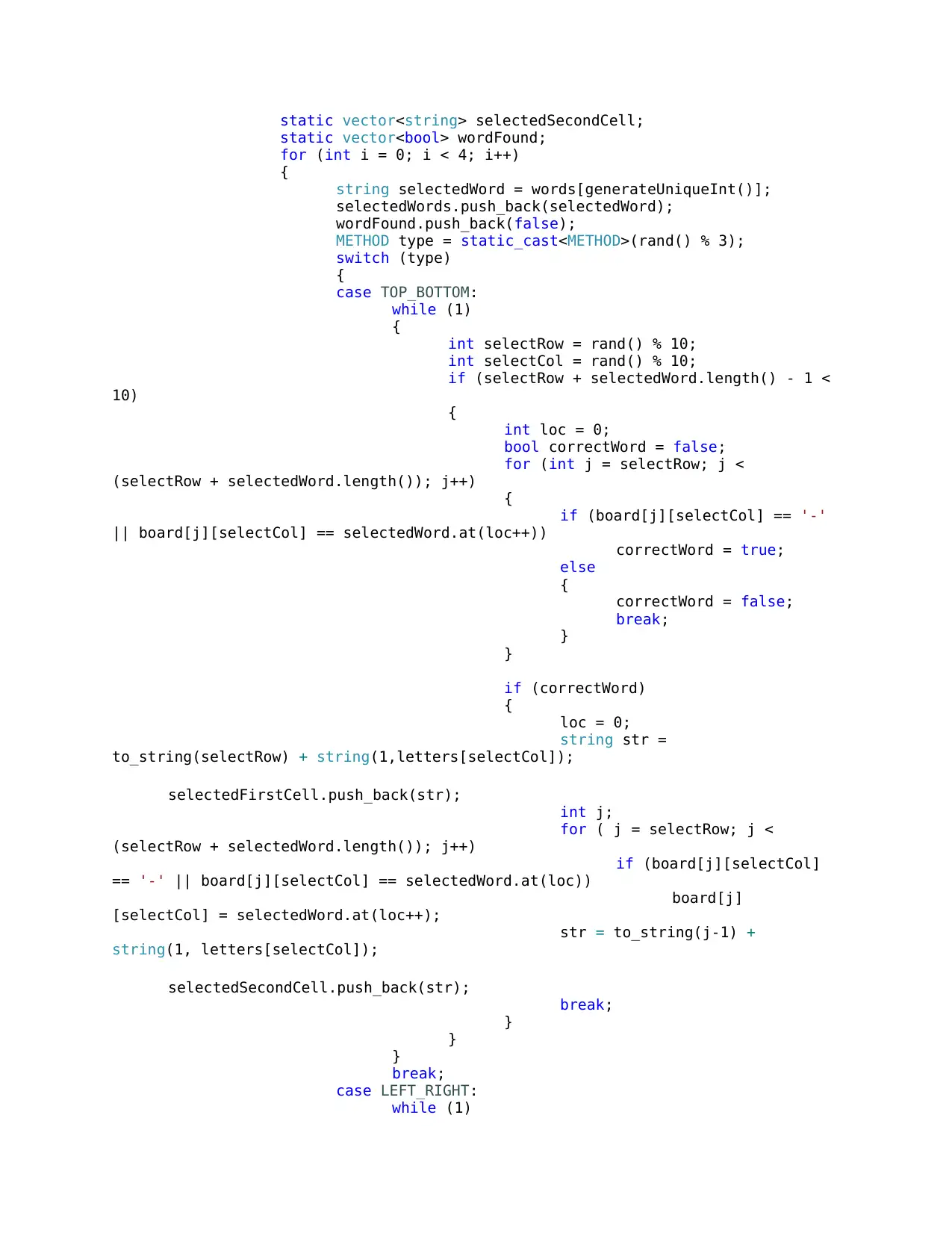
static vector<string> selectedSecondCell;
static vector<bool> wordFound;
for (int i = 0; i < 4; i++)
{
string selectedWord = words[generateUniqueInt()];
selectedWords.push_back(selectedWord);
wordFound.push_back(false);
METHOD type = static_cast<METHOD>(rand() % 3);
switch (type)
{
case TOP_BOTTOM:
while (1)
{
int selectRow = rand() % 10;
int selectCol = rand() % 10;
if (selectRow + selectedWord.length() - 1 <
10)
{
int loc = 0;
bool correctWord = false;
for (int j = selectRow; j <
(selectRow + selectedWord.length()); j++)
{
if (board[j][selectCol] == '-'
|| board[j][selectCol] == selectedWord.at(loc++))
correctWord = true;
else
{
correctWord = false;
break;
}
}
if (correctWord)
{
loc = 0;
string str =
to_string(selectRow) + string(1,letters[selectCol]);
selectedFirstCell.push_back(str);
int j;
for ( j = selectRow; j <
(selectRow + selectedWord.length()); j++)
if (board[j][selectCol]
== '-' || board[j][selectCol] == selectedWord.at(loc))
board[j]
[selectCol] = selectedWord.at(loc++);
str = to_string(j-1) +
string(1, letters[selectCol]);
selectedSecondCell.push_back(str);
break;
}
}
}
break;
case LEFT_RIGHT:
while (1)
static vector<bool> wordFound;
for (int i = 0; i < 4; i++)
{
string selectedWord = words[generateUniqueInt()];
selectedWords.push_back(selectedWord);
wordFound.push_back(false);
METHOD type = static_cast<METHOD>(rand() % 3);
switch (type)
{
case TOP_BOTTOM:
while (1)
{
int selectRow = rand() % 10;
int selectCol = rand() % 10;
if (selectRow + selectedWord.length() - 1 <
10)
{
int loc = 0;
bool correctWord = false;
for (int j = selectRow; j <
(selectRow + selectedWord.length()); j++)
{
if (board[j][selectCol] == '-'
|| board[j][selectCol] == selectedWord.at(loc++))
correctWord = true;
else
{
correctWord = false;
break;
}
}
if (correctWord)
{
loc = 0;
string str =
to_string(selectRow) + string(1,letters[selectCol]);
selectedFirstCell.push_back(str);
int j;
for ( j = selectRow; j <
(selectRow + selectedWord.length()); j++)
if (board[j][selectCol]
== '-' || board[j][selectCol] == selectedWord.at(loc))
board[j]
[selectCol] = selectedWord.at(loc++);
str = to_string(j-1) +
string(1, letters[selectCol]);
selectedSecondCell.push_back(str);
break;
}
}
}
break;
case LEFT_RIGHT:
while (1)
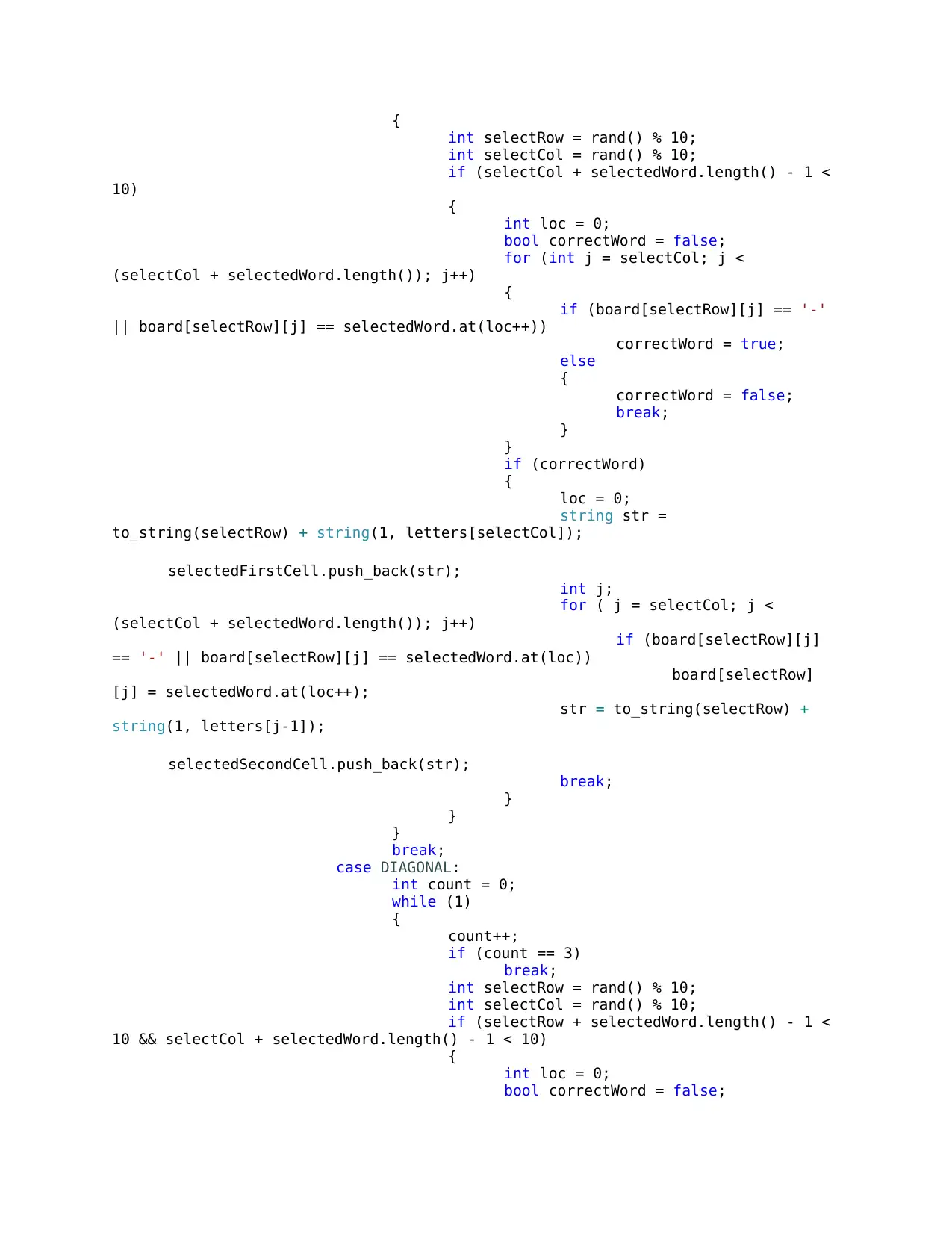
{
int selectRow = rand() % 10;
int selectCol = rand() % 10;
if (selectCol + selectedWord.length() - 1 <
10)
{
int loc = 0;
bool correctWord = false;
for (int j = selectCol; j <
(selectCol + selectedWord.length()); j++)
{
if (board[selectRow][j] == '-'
|| board[selectRow][j] == selectedWord.at(loc++))
correctWord = true;
else
{
correctWord = false;
break;
}
}
if (correctWord)
{
loc = 0;
string str =
to_string(selectRow) + string(1, letters[selectCol]);
selectedFirstCell.push_back(str);
int j;
for ( j = selectCol; j <
(selectCol + selectedWord.length()); j++)
if (board[selectRow][j]
== '-' || board[selectRow][j] == selectedWord.at(loc))
board[selectRow]
[j] = selectedWord.at(loc++);
str = to_string(selectRow) +
string(1, letters[j-1]);
selectedSecondCell.push_back(str);
break;
}
}
}
break;
case DIAGONAL:
int count = 0;
while (1)
{
count++;
if (count == 3)
break;
int selectRow = rand() % 10;
int selectCol = rand() % 10;
if (selectRow + selectedWord.length() - 1 <
10 && selectCol + selectedWord.length() - 1 < 10)
{
int loc = 0;
bool correctWord = false;
int selectRow = rand() % 10;
int selectCol = rand() % 10;
if (selectCol + selectedWord.length() - 1 <
10)
{
int loc = 0;
bool correctWord = false;
for (int j = selectCol; j <
(selectCol + selectedWord.length()); j++)
{
if (board[selectRow][j] == '-'
|| board[selectRow][j] == selectedWord.at(loc++))
correctWord = true;
else
{
correctWord = false;
break;
}
}
if (correctWord)
{
loc = 0;
string str =
to_string(selectRow) + string(1, letters[selectCol]);
selectedFirstCell.push_back(str);
int j;
for ( j = selectCol; j <
(selectCol + selectedWord.length()); j++)
if (board[selectRow][j]
== '-' || board[selectRow][j] == selectedWord.at(loc))
board[selectRow]
[j] = selectedWord.at(loc++);
str = to_string(selectRow) +
string(1, letters[j-1]);
selectedSecondCell.push_back(str);
break;
}
}
}
break;
case DIAGONAL:
int count = 0;
while (1)
{
count++;
if (count == 3)
break;
int selectRow = rand() % 10;
int selectCol = rand() % 10;
if (selectRow + selectedWord.length() - 1 <
10 && selectCol + selectedWord.length() - 1 < 10)
{
int loc = 0;
bool correctWord = false;
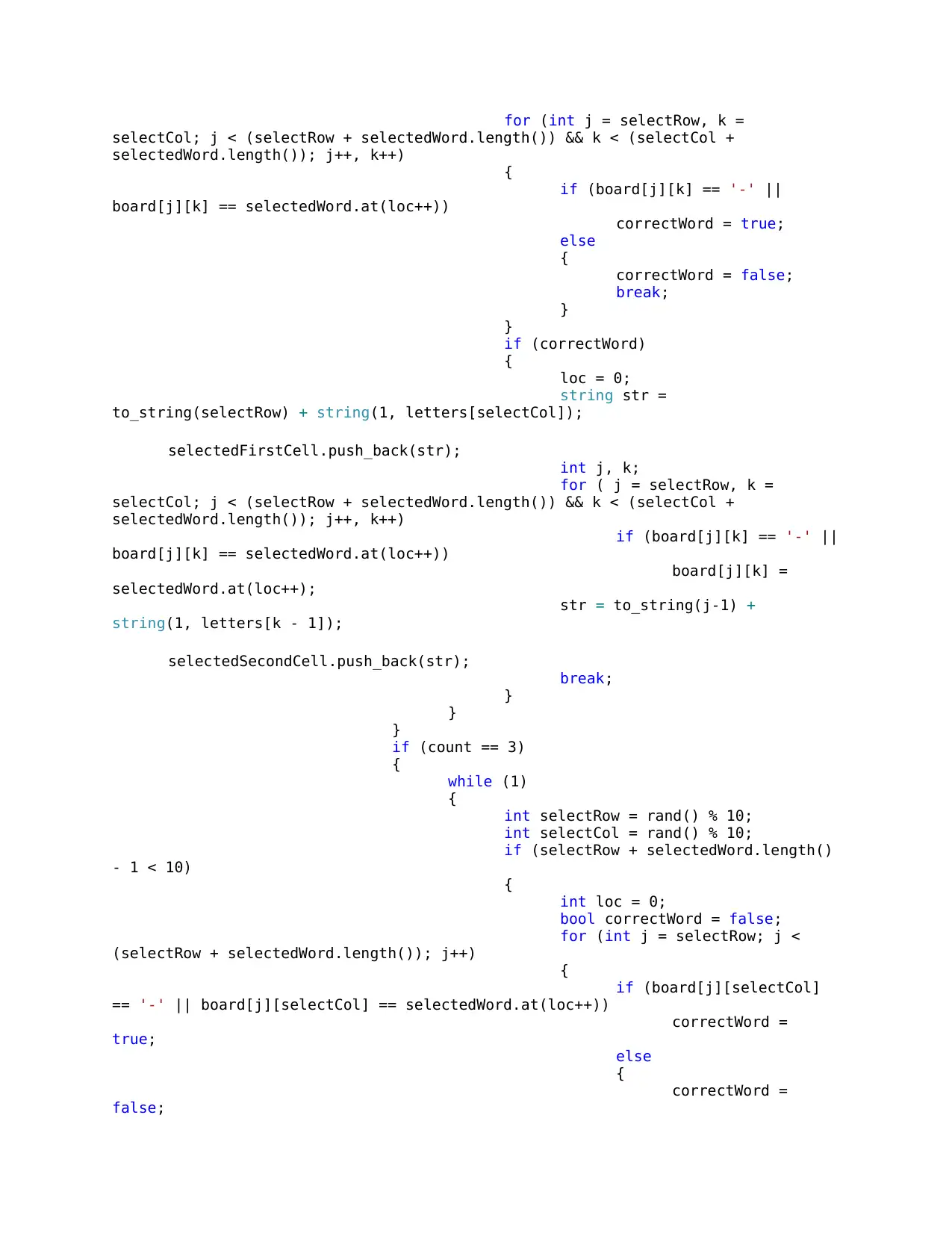
for (int j = selectRow, k =
selectCol; j < (selectRow + selectedWord.length()) && k < (selectCol +
selectedWord.length()); j++, k++)
{
if (board[j][k] == '-' ||
board[j][k] == selectedWord.at(loc++))
correctWord = true;
else
{
correctWord = false;
break;
}
}
if (correctWord)
{
loc = 0;
string str =
to_string(selectRow) + string(1, letters[selectCol]);
selectedFirstCell.push_back(str);
int j, k;
for ( j = selectRow, k =
selectCol; j < (selectRow + selectedWord.length()) && k < (selectCol +
selectedWord.length()); j++, k++)
if (board[j][k] == '-' ||
board[j][k] == selectedWord.at(loc++))
board[j][k] =
selectedWord.at(loc++);
str = to_string(j-1) +
string(1, letters[k - 1]);
selectedSecondCell.push_back(str);
break;
}
}
}
if (count == 3)
{
while (1)
{
int selectRow = rand() % 10;
int selectCol = rand() % 10;
if (selectRow + selectedWord.length()
- 1 < 10)
{
int loc = 0;
bool correctWord = false;
for (int j = selectRow; j <
(selectRow + selectedWord.length()); j++)
{
if (board[j][selectCol]
== '-' || board[j][selectCol] == selectedWord.at(loc++))
correctWord =
true;
else
{
correctWord =
false;
selectCol; j < (selectRow + selectedWord.length()) && k < (selectCol +
selectedWord.length()); j++, k++)
{
if (board[j][k] == '-' ||
board[j][k] == selectedWord.at(loc++))
correctWord = true;
else
{
correctWord = false;
break;
}
}
if (correctWord)
{
loc = 0;
string str =
to_string(selectRow) + string(1, letters[selectCol]);
selectedFirstCell.push_back(str);
int j, k;
for ( j = selectRow, k =
selectCol; j < (selectRow + selectedWord.length()) && k < (selectCol +
selectedWord.length()); j++, k++)
if (board[j][k] == '-' ||
board[j][k] == selectedWord.at(loc++))
board[j][k] =
selectedWord.at(loc++);
str = to_string(j-1) +
string(1, letters[k - 1]);
selectedSecondCell.push_back(str);
break;
}
}
}
if (count == 3)
{
while (1)
{
int selectRow = rand() % 10;
int selectCol = rand() % 10;
if (selectRow + selectedWord.length()
- 1 < 10)
{
int loc = 0;
bool correctWord = false;
for (int j = selectRow; j <
(selectRow + selectedWord.length()); j++)
{
if (board[j][selectCol]
== '-' || board[j][selectCol] == selectedWord.at(loc++))
correctWord =
true;
else
{
correctWord =
false;
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
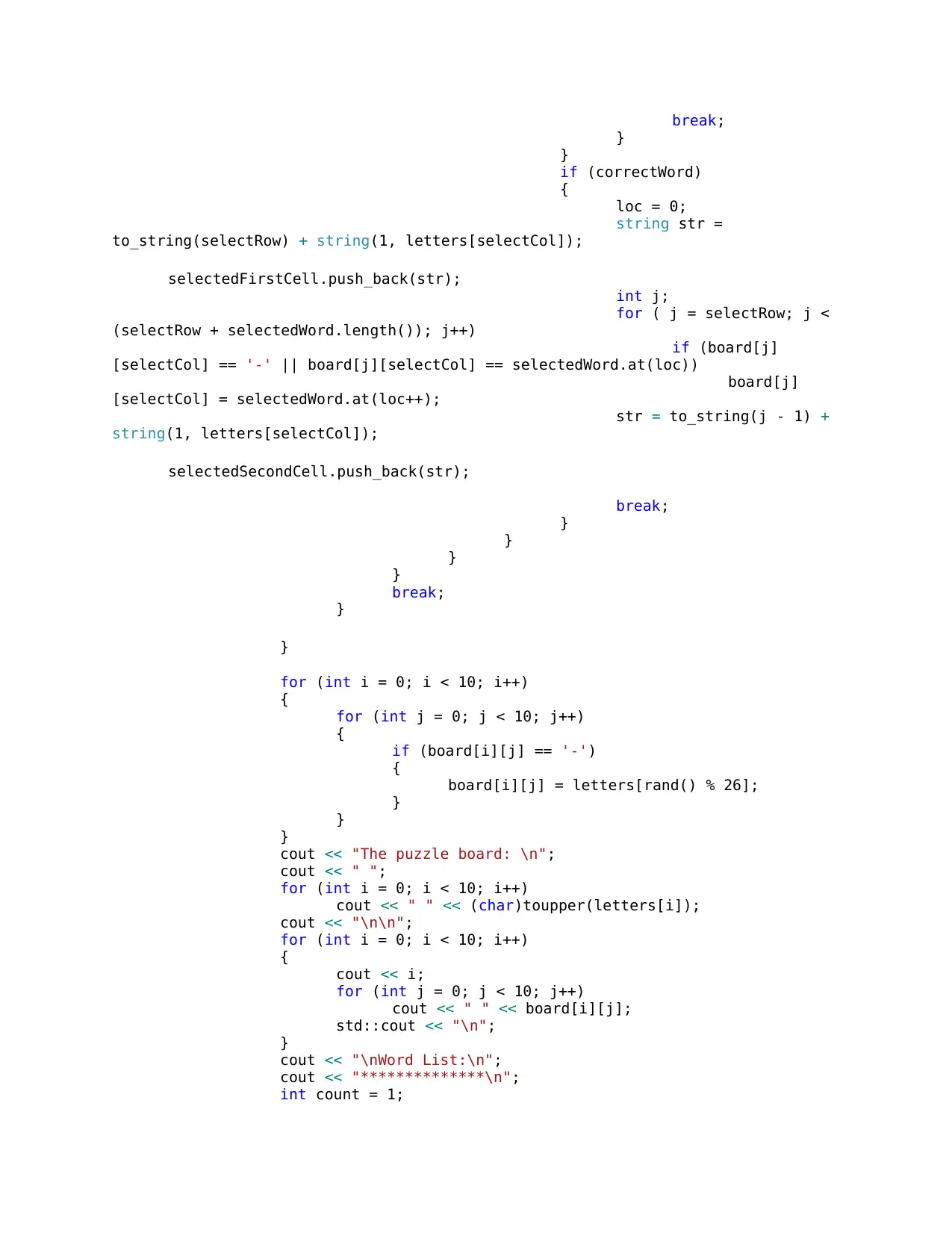
break;
}
}
if (correctWord)
{
loc = 0;
string str =
to_string(selectRow) + string(1, letters[selectCol]);
selectedFirstCell.push_back(str);
int j;
for ( j = selectRow; j <
(selectRow + selectedWord.length()); j++)
if (board[j]
[selectCol] == '-' || board[j][selectCol] == selectedWord.at(loc))
board[j]
[selectCol] = selectedWord.at(loc++);
str = to_string(j - 1) +
string(1, letters[selectCol]);
selectedSecondCell.push_back(str);
break;
}
}
}
}
break;
}
}
for (int i = 0; i < 10; i++)
{
for (int j = 0; j < 10; j++)
{
if (board[i][j] == '-')
{
board[i][j] = letters[rand() % 26];
}
}
}
cout << "The puzzle board: \n";
cout << " ";
for (int i = 0; i < 10; i++)
cout << " " << (char)toupper(letters[i]);
cout << "\n\n";
for (int i = 0; i < 10; i++)
{
cout << i;
for (int j = 0; j < 10; j++)
cout << " " << board[i][j];
std::cout << "\n";
}
cout << "\nWord List:\n";
cout << "**************\n";
int count = 1;
}
}
if (correctWord)
{
loc = 0;
string str =
to_string(selectRow) + string(1, letters[selectCol]);
selectedFirstCell.push_back(str);
int j;
for ( j = selectRow; j <
(selectRow + selectedWord.length()); j++)
if (board[j]
[selectCol] == '-' || board[j][selectCol] == selectedWord.at(loc))
board[j]
[selectCol] = selectedWord.at(loc++);
str = to_string(j - 1) +
string(1, letters[selectCol]);
selectedSecondCell.push_back(str);
break;
}
}
}
}
break;
}
}
for (int i = 0; i < 10; i++)
{
for (int j = 0; j < 10; j++)
{
if (board[i][j] == '-')
{
board[i][j] = letters[rand() % 26];
}
}
}
cout << "The puzzle board: \n";
cout << " ";
for (int i = 0; i < 10; i++)
cout << " " << (char)toupper(letters[i]);
cout << "\n\n";
for (int i = 0; i < 10; i++)
{
cout << i;
for (int j = 0; j < 10; j++)
cout << " " << board[i][j];
std::cout << "\n";
}
cout << "\nWord List:\n";
cout << "**************\n";
int count = 1;
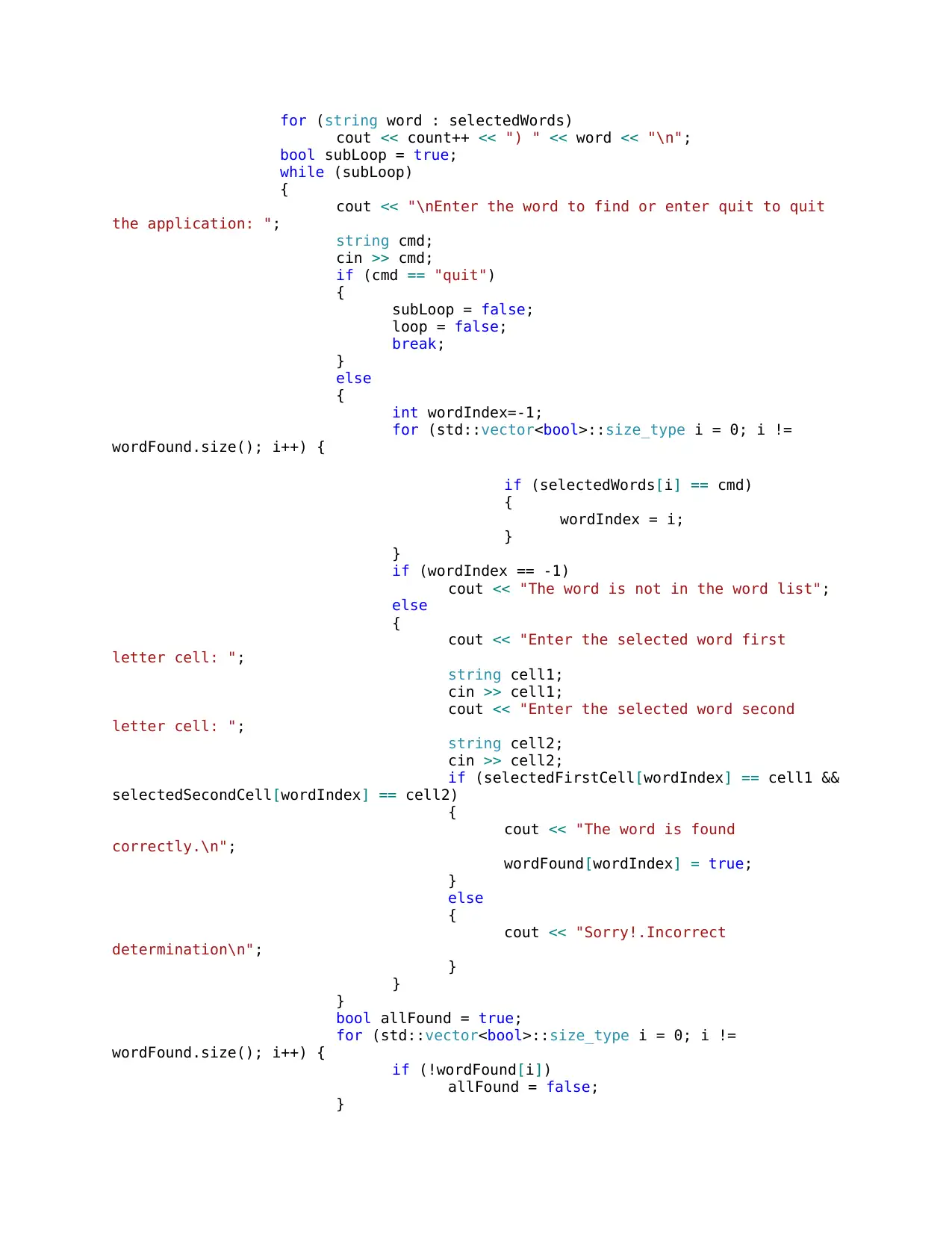
for (string word : selectedWords)
cout << count++ << ") " << word << "\n";
bool subLoop = true;
while (subLoop)
{
cout << "\nEnter the word to find or enter quit to quit
the application: ";
string cmd;
cin >> cmd;
if (cmd == "quit")
{
subLoop = false;
loop = false;
break;
}
else
{
int wordIndex=-1;
for (std::vector<bool>::size_type i = 0; i !=
wordFound.size(); i++) {
if (selectedWords[i] == cmd)
{
wordIndex = i;
}
}
if (wordIndex == -1)
cout << "The word is not in the word list";
else
{
cout << "Enter the selected word first
letter cell: ";
string cell1;
cin >> cell1;
cout << "Enter the selected word second
letter cell: ";
string cell2;
cin >> cell2;
if (selectedFirstCell[wordIndex] == cell1 &&
selectedSecondCell[wordIndex] == cell2)
{
cout << "The word is found
correctly.\n";
wordFound[wordIndex] = true;
}
else
{
cout << "Sorry!.Incorrect
determination\n";
}
}
}
bool allFound = true;
for (std::vector<bool>::size_type i = 0; i !=
wordFound.size(); i++) {
if (!wordFound[i])
allFound = false;
}
cout << count++ << ") " << word << "\n";
bool subLoop = true;
while (subLoop)
{
cout << "\nEnter the word to find or enter quit to quit
the application: ";
string cmd;
cin >> cmd;
if (cmd == "quit")
{
subLoop = false;
loop = false;
break;
}
else
{
int wordIndex=-1;
for (std::vector<bool>::size_type i = 0; i !=
wordFound.size(); i++) {
if (selectedWords[i] == cmd)
{
wordIndex = i;
}
}
if (wordIndex == -1)
cout << "The word is not in the word list";
else
{
cout << "Enter the selected word first
letter cell: ";
string cell1;
cin >> cell1;
cout << "Enter the selected word second
letter cell: ";
string cell2;
cin >> cell2;
if (selectedFirstCell[wordIndex] == cell1 &&
selectedSecondCell[wordIndex] == cell2)
{
cout << "The word is found
correctly.\n";
wordFound[wordIndex] = true;
}
else
{
cout << "Sorry!.Incorrect
determination\n";
}
}
}
bool allFound = true;
for (std::vector<bool>::size_type i = 0; i !=
wordFound.size(); i++) {
if (!wordFound[i])
allFound = false;
}
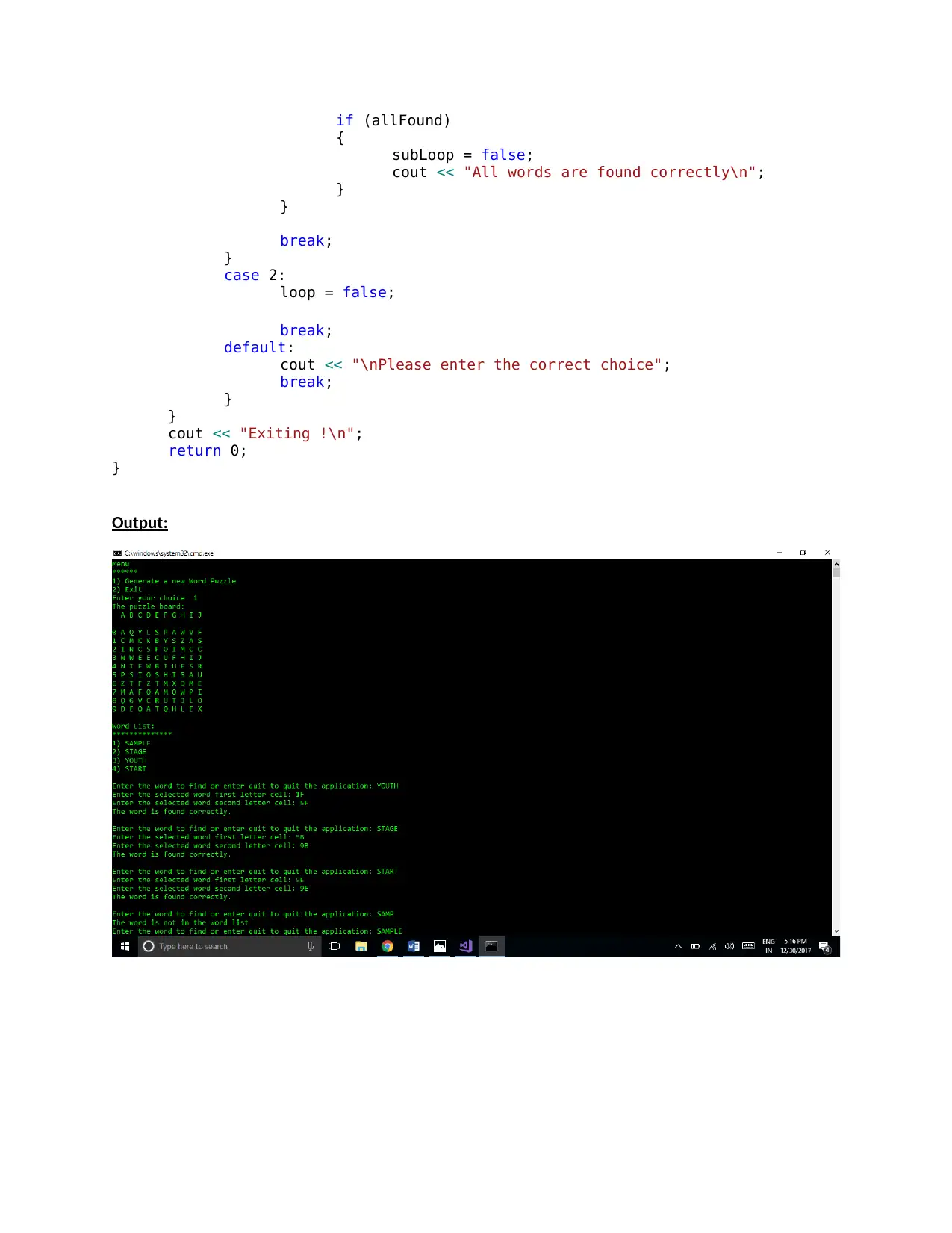
if (allFound)
{
subLoop = false;
cout << "All words are found correctly\n";
}
}
break;
}
case 2:
loop = false;
break;
default:
cout << "\nPlease enter the correct choice";
break;
}
}
cout << "Exiting !\n";
return 0;
}
Output:
{
subLoop = false;
cout << "All words are found correctly\n";
}
}
break;
}
case 2:
loop = false;
break;
default:
cout << "\nPlease enter the correct choice";
break;
}
}
cout << "Exiting !\n";
return 0;
}
Output:
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
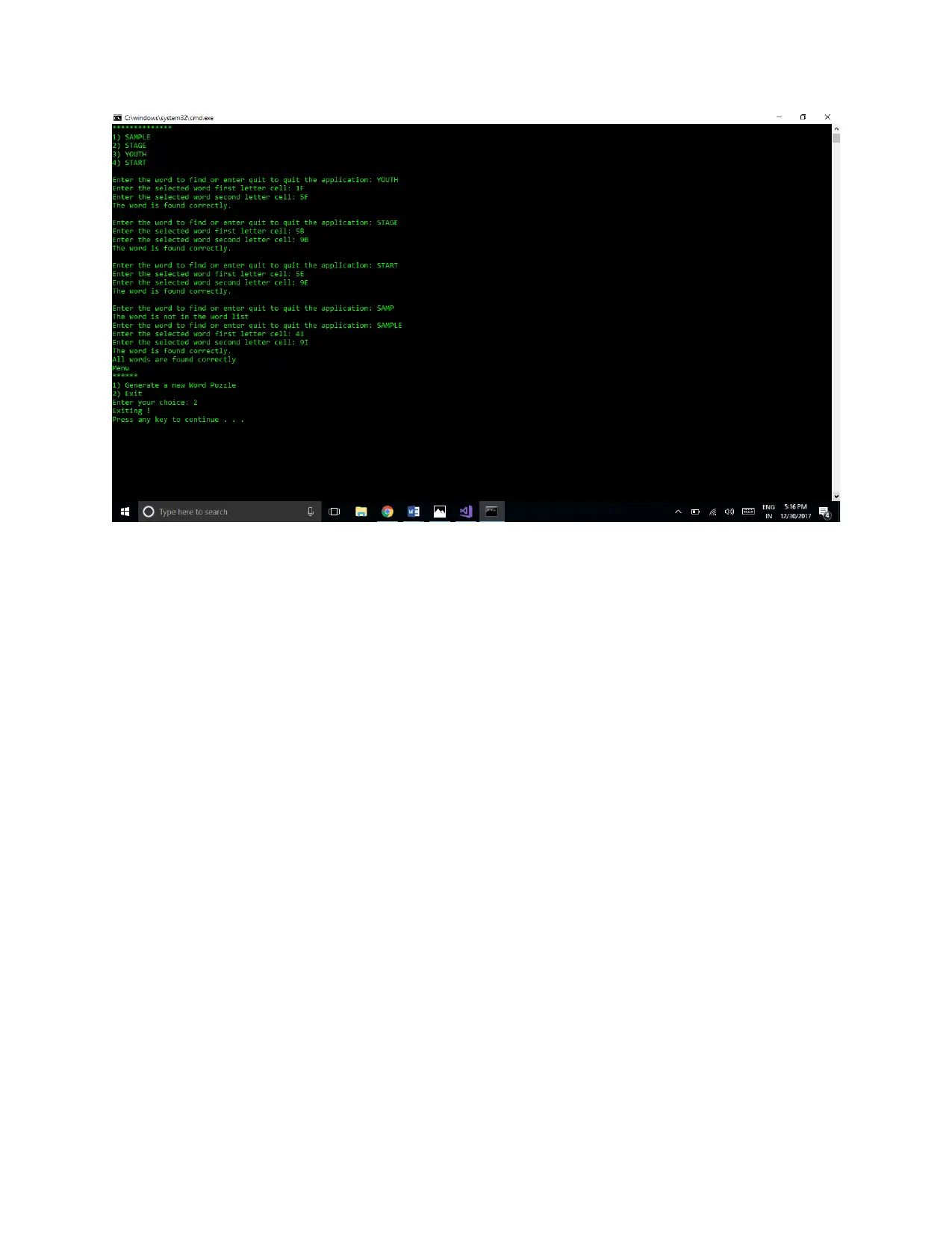
1 out of 8
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
 +13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024  |  Zucol Services PVT LTD  |  All rights reserved.