Python Programming Assignment: Implementing Basic Algorithms and Debugging Techniques
VerifiedAdded on 2024/05/21
|36
|4030
|413
AI Summary
This assignment explores fundamental programming concepts using the Python language. It covers defining algorithms, implementing them in code using IDLE, and understanding the debugging process. The report analyzes the characteristics of procedural, object-oriented, and event-driven programming paradigms, highlighting the strengths of Python's object-oriented approach. The assignment also delves into the importance of coding standards and demonstrates how to apply them for better code readability and maintainability.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
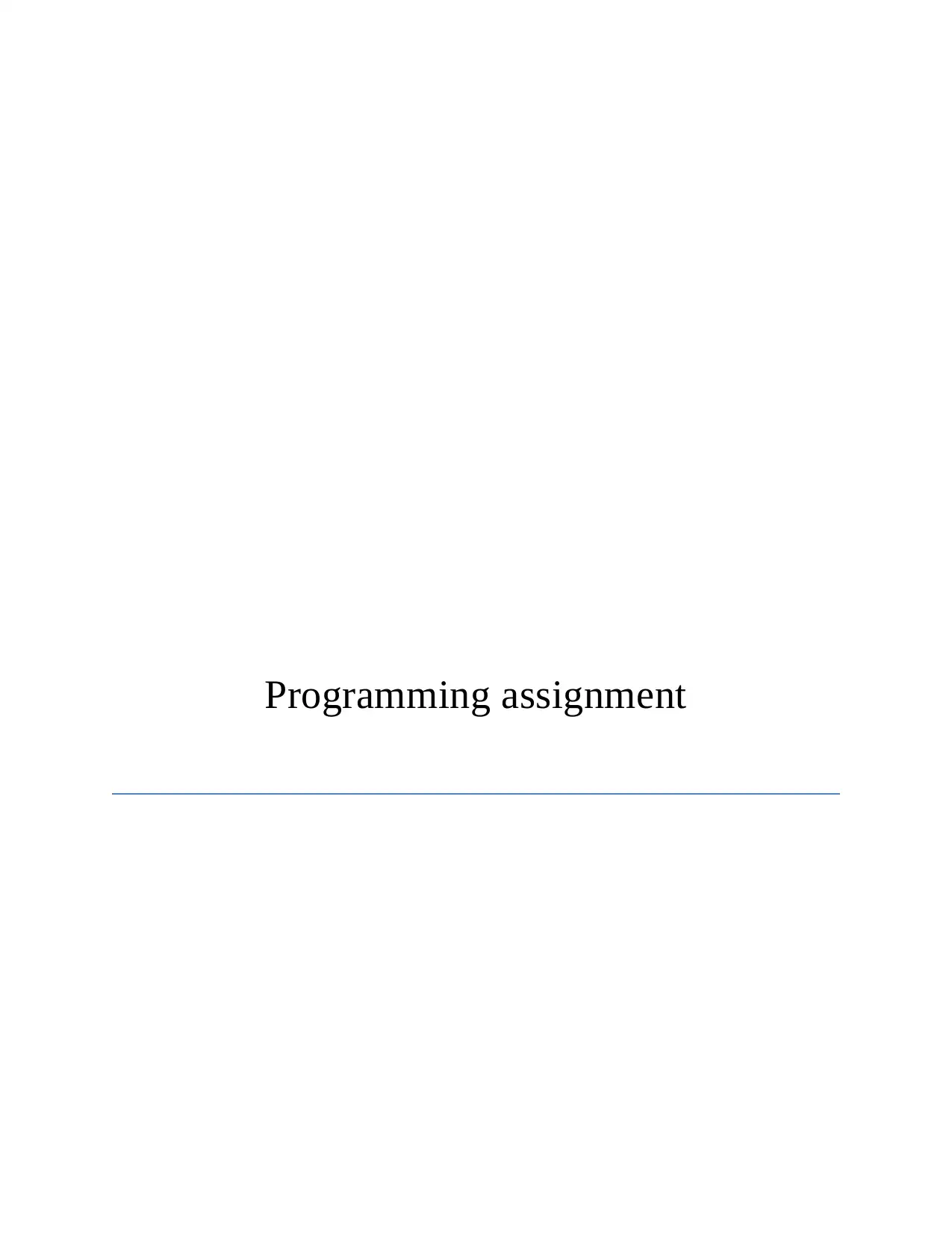
Programming assignment
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
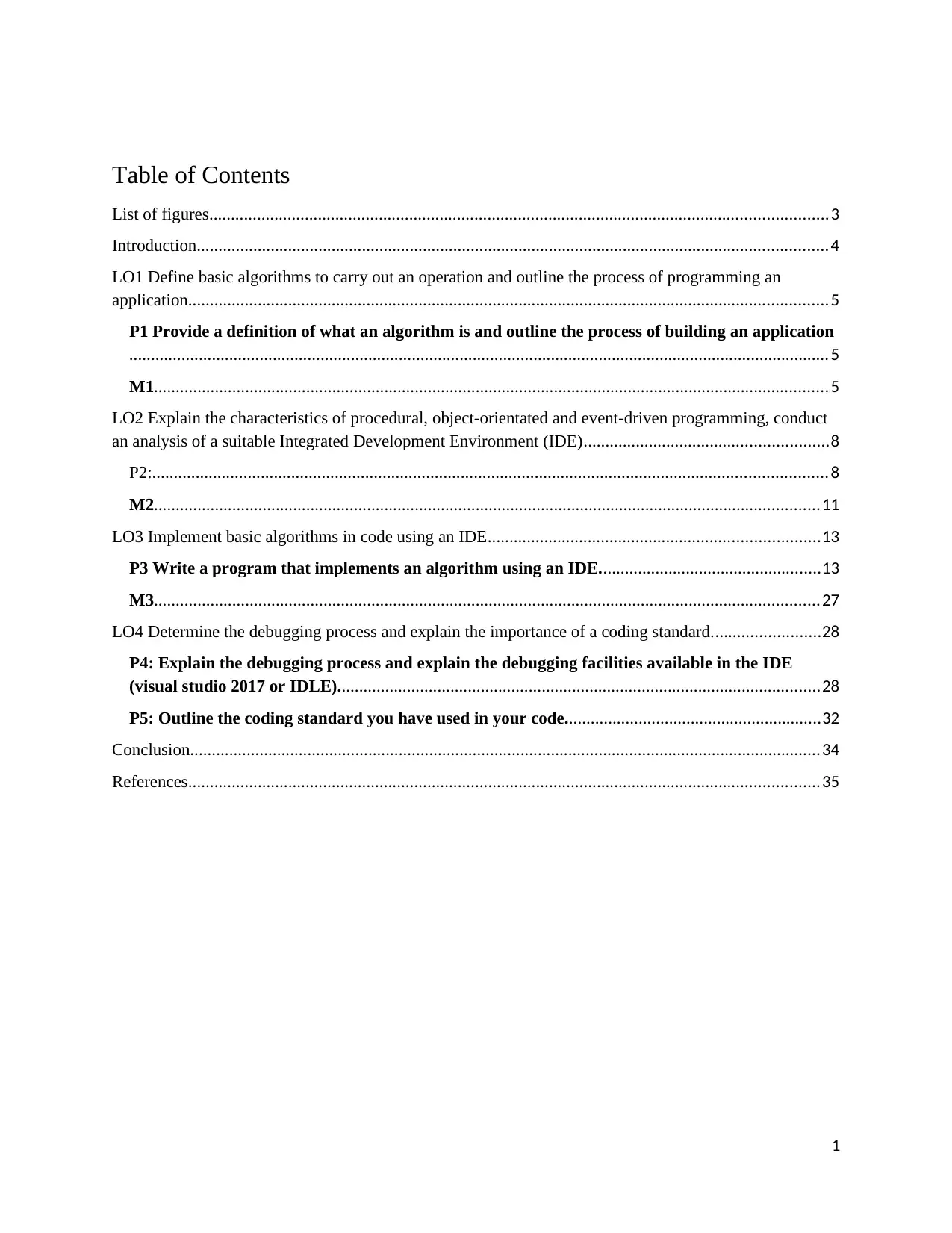
Table of Contents
List of figures..............................................................................................................................................3
Introduction.................................................................................................................................................4
LO1 Define basic algorithms to carry out an operation and outline the process of programming an
application...................................................................................................................................................5
P1 Provide a definition of what an algorithm is and outline the process of building an application
.................................................................................................................................................................5
M1...........................................................................................................................................................5
LO2 Explain the characteristics of procedural, object-orientated and event-driven programming, conduct
an analysis of a suitable Integrated Development Environment (IDE)........................................................8
P2:...........................................................................................................................................................8
M2.........................................................................................................................................................11
LO3 Implement basic algorithms in code using an IDE............................................................................13
P3 Write a program that implements an algorithm using an IDE...................................................13
M3.........................................................................................................................................................27
LO4 Determine the debugging process and explain the importance of a coding standard.........................28
P4: Explain the debugging process and explain the debugging facilities available in the IDE
(visual studio 2017 or IDLE)...............................................................................................................28
P5: Outline the coding standard you have used in your code...........................................................32
Conclusion.................................................................................................................................................34
References.................................................................................................................................................35
1
List of figures..............................................................................................................................................3
Introduction.................................................................................................................................................4
LO1 Define basic algorithms to carry out an operation and outline the process of programming an
application...................................................................................................................................................5
P1 Provide a definition of what an algorithm is and outline the process of building an application
.................................................................................................................................................................5
M1...........................................................................................................................................................5
LO2 Explain the characteristics of procedural, object-orientated and event-driven programming, conduct
an analysis of a suitable Integrated Development Environment (IDE)........................................................8
P2:...........................................................................................................................................................8
M2.........................................................................................................................................................11
LO3 Implement basic algorithms in code using an IDE............................................................................13
P3 Write a program that implements an algorithm using an IDE...................................................13
M3.........................................................................................................................................................27
LO4 Determine the debugging process and explain the importance of a coding standard.........................28
P4: Explain the debugging process and explain the debugging facilities available in the IDE
(visual studio 2017 or IDLE)...............................................................................................................28
P5: Outline the coding standard you have used in your code...........................................................32
Conclusion.................................................................................................................................................34
References.................................................................................................................................................35
1
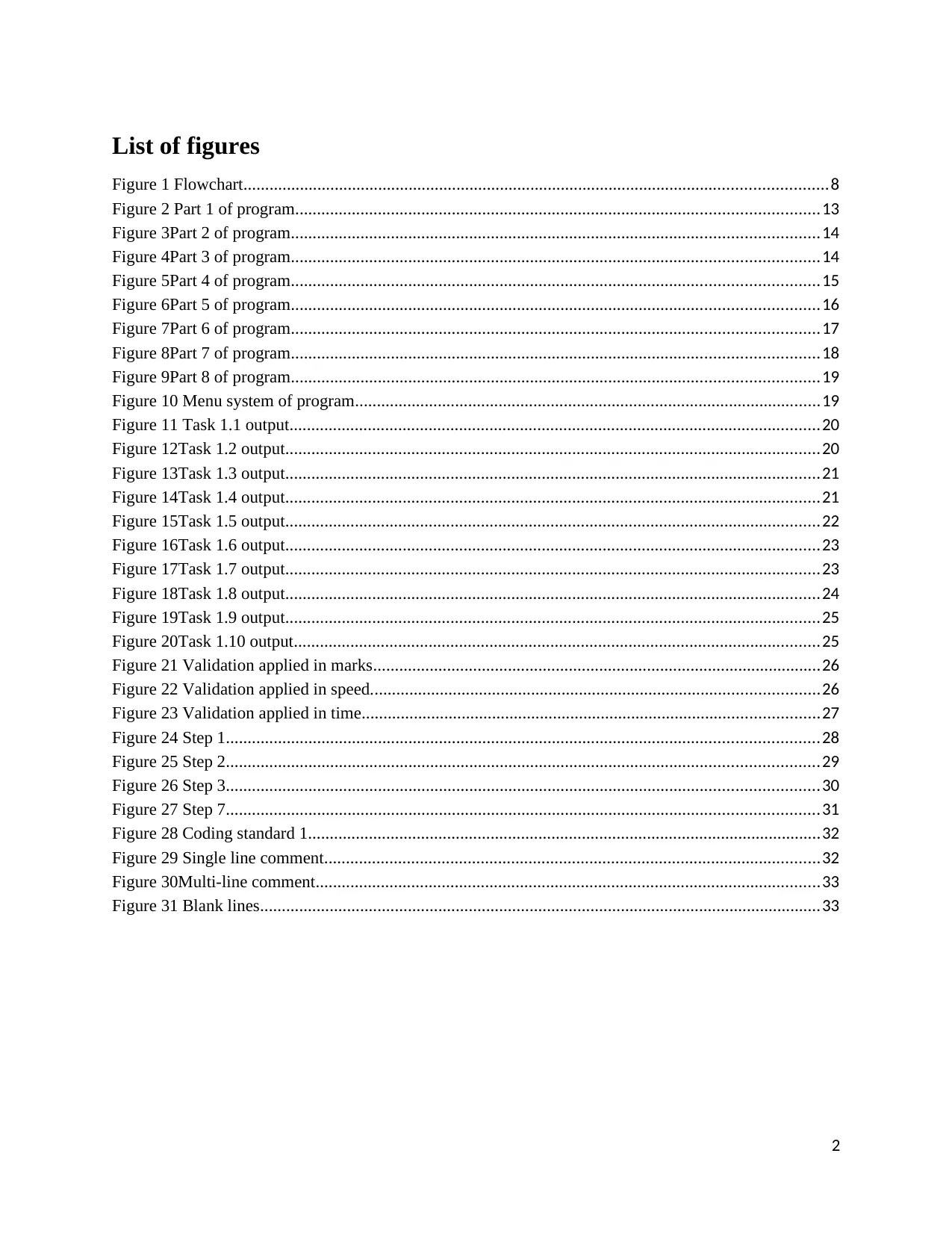
List of figures
Figure 1 Flowchart......................................................................................................................................8
Figure 2 Part 1 of program........................................................................................................................13
Figure 3Part 2 of program.........................................................................................................................14
Figure 4Part 3 of program.........................................................................................................................14
Figure 5Part 4 of program.........................................................................................................................15
Figure 6Part 5 of program.........................................................................................................................16
Figure 7Part 6 of program.........................................................................................................................17
Figure 8Part 7 of program.........................................................................................................................18
Figure 9Part 8 of program.........................................................................................................................19
Figure 10 Menu system of program...........................................................................................................19
Figure 11 Task 1.1 output..........................................................................................................................20
Figure 12Task 1.2 output...........................................................................................................................20
Figure 13Task 1.3 output...........................................................................................................................21
Figure 14Task 1.4 output...........................................................................................................................21
Figure 15Task 1.5 output...........................................................................................................................22
Figure 16Task 1.6 output...........................................................................................................................23
Figure 17Task 1.7 output...........................................................................................................................23
Figure 18Task 1.8 output...........................................................................................................................24
Figure 19Task 1.9 output...........................................................................................................................25
Figure 20Task 1.10 output.........................................................................................................................25
Figure 21 Validation applied in marks.......................................................................................................26
Figure 22 Validation applied in speed.......................................................................................................26
Figure 23 Validation applied in time.........................................................................................................27
Figure 24 Step 1........................................................................................................................................28
Figure 25 Step 2........................................................................................................................................29
Figure 26 Step 3........................................................................................................................................30
Figure 27 Step 7........................................................................................................................................31
Figure 28 Coding standard 1......................................................................................................................32
Figure 29 Single line comment..................................................................................................................32
Figure 30Multi-line comment....................................................................................................................33
Figure 31 Blank lines.................................................................................................................................33
2
Figure 1 Flowchart......................................................................................................................................8
Figure 2 Part 1 of program........................................................................................................................13
Figure 3Part 2 of program.........................................................................................................................14
Figure 4Part 3 of program.........................................................................................................................14
Figure 5Part 4 of program.........................................................................................................................15
Figure 6Part 5 of program.........................................................................................................................16
Figure 7Part 6 of program.........................................................................................................................17
Figure 8Part 7 of program.........................................................................................................................18
Figure 9Part 8 of program.........................................................................................................................19
Figure 10 Menu system of program...........................................................................................................19
Figure 11 Task 1.1 output..........................................................................................................................20
Figure 12Task 1.2 output...........................................................................................................................20
Figure 13Task 1.3 output...........................................................................................................................21
Figure 14Task 1.4 output...........................................................................................................................21
Figure 15Task 1.5 output...........................................................................................................................22
Figure 16Task 1.6 output...........................................................................................................................23
Figure 17Task 1.7 output...........................................................................................................................23
Figure 18Task 1.8 output...........................................................................................................................24
Figure 19Task 1.9 output...........................................................................................................................25
Figure 20Task 1.10 output.........................................................................................................................25
Figure 21 Validation applied in marks.......................................................................................................26
Figure 22 Validation applied in speed.......................................................................................................26
Figure 23 Validation applied in time.........................................................................................................27
Figure 24 Step 1........................................................................................................................................28
Figure 25 Step 2........................................................................................................................................29
Figure 26 Step 3........................................................................................................................................30
Figure 27 Step 7........................................................................................................................................31
Figure 28 Coding standard 1......................................................................................................................32
Figure 29 Single line comment..................................................................................................................32
Figure 30Multi-line comment....................................................................................................................33
Figure 31 Blank lines.................................................................................................................................33
2
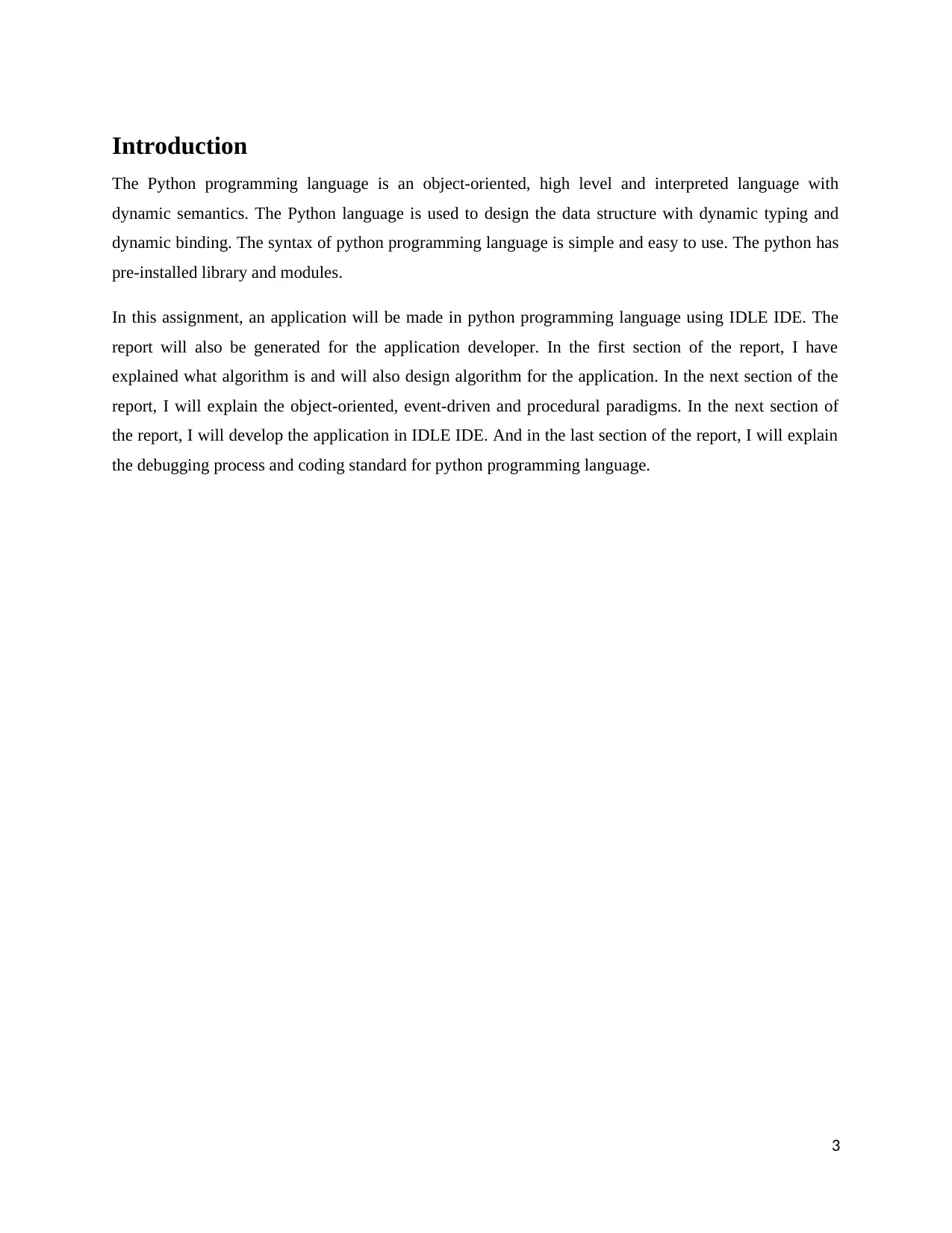
Introduction
The Python programming language is an object-oriented, high level and interpreted language with
dynamic semantics. The Python language is used to design the data structure with dynamic typing and
dynamic binding. The syntax of python programming language is simple and easy to use. The python has
pre-installed library and modules.
In this assignment, an application will be made in python programming language using IDLE IDE. The
report will also be generated for the application developer. In the first section of the report, I have
explained what algorithm is and will also design algorithm for the application. In the next section of the
report, I will explain the object-oriented, event-driven and procedural paradigms. In the next section of
the report, I will develop the application in IDLE IDE. And in the last section of the report, I will explain
the debugging process and coding standard for python programming language.
3
The Python programming language is an object-oriented, high level and interpreted language with
dynamic semantics. The Python language is used to design the data structure with dynamic typing and
dynamic binding. The syntax of python programming language is simple and easy to use. The python has
pre-installed library and modules.
In this assignment, an application will be made in python programming language using IDLE IDE. The
report will also be generated for the application developer. In the first section of the report, I have
explained what algorithm is and will also design algorithm for the application. In the next section of the
report, I will explain the object-oriented, event-driven and procedural paradigms. In the next section of
the report, I will develop the application in IDLE IDE. And in the last section of the report, I will explain
the debugging process and coding standard for python programming language.
3
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
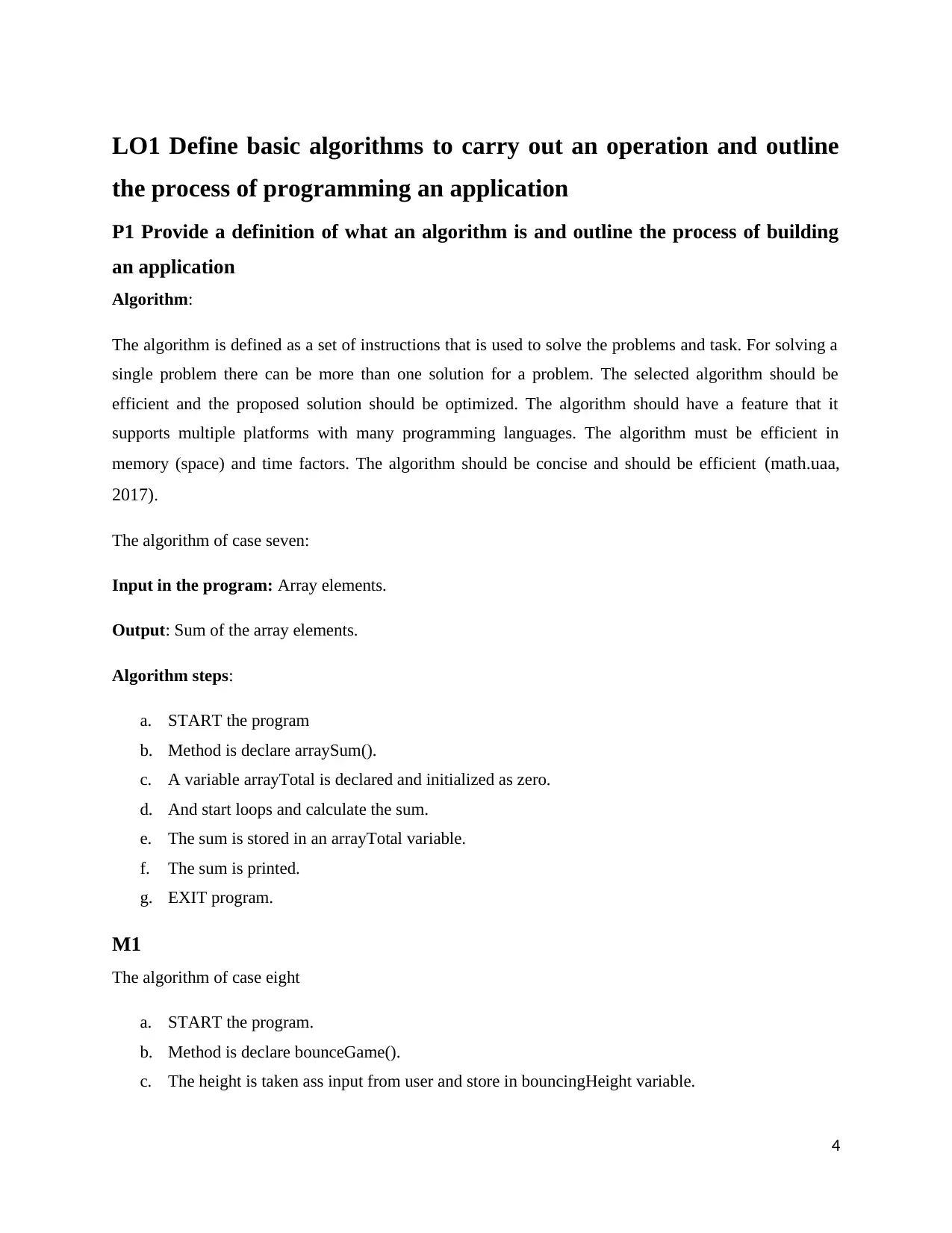
LO1 Define basic algorithms to carry out an operation and outline
the process of programming an application
P1 Provide a definition of what an algorithm is and outline the process of building
an application
Algorithm:
The algorithm is defined as a set of instructions that is used to solve the problems and task. For solving a
single problem there can be more than one solution for a problem. The selected algorithm should be
efficient and the proposed solution should be optimized. The algorithm should have a feature that it
supports multiple platforms with many programming languages. The algorithm must be efficient in
memory (space) and time factors. The algorithm should be concise and should be efficient (math.uaa,
2017).
The algorithm of case seven:
Input in the program: Array elements.
Output: Sum of the array elements.
Algorithm steps:
a. START the program
b. Method is declare arraySum().
c. A variable arrayTotal is declared and initialized as zero.
d. And start loops and calculate the sum.
e. The sum is stored in an arrayTotal variable.
f. The sum is printed.
g. EXIT program.
M1
The algorithm of case eight
a. START the program.
b. Method is declare bounceGame().
c. The height is taken ass input from user and store in bouncingHeight variable.
4
the process of programming an application
P1 Provide a definition of what an algorithm is and outline the process of building
an application
Algorithm:
The algorithm is defined as a set of instructions that is used to solve the problems and task. For solving a
single problem there can be more than one solution for a problem. The selected algorithm should be
efficient and the proposed solution should be optimized. The algorithm should have a feature that it
supports multiple platforms with many programming languages. The algorithm must be efficient in
memory (space) and time factors. The algorithm should be concise and should be efficient (math.uaa,
2017).
The algorithm of case seven:
Input in the program: Array elements.
Output: Sum of the array elements.
Algorithm steps:
a. START the program
b. Method is declare arraySum().
c. A variable arrayTotal is declared and initialized as zero.
d. And start loops and calculate the sum.
e. The sum is stored in an arrayTotal variable.
f. The sum is printed.
g. EXIT program.
M1
The algorithm of case eight
a. START the program.
b. Method is declare bounceGame().
c. The height is taken ass input from user and store in bouncingHeight variable.
4
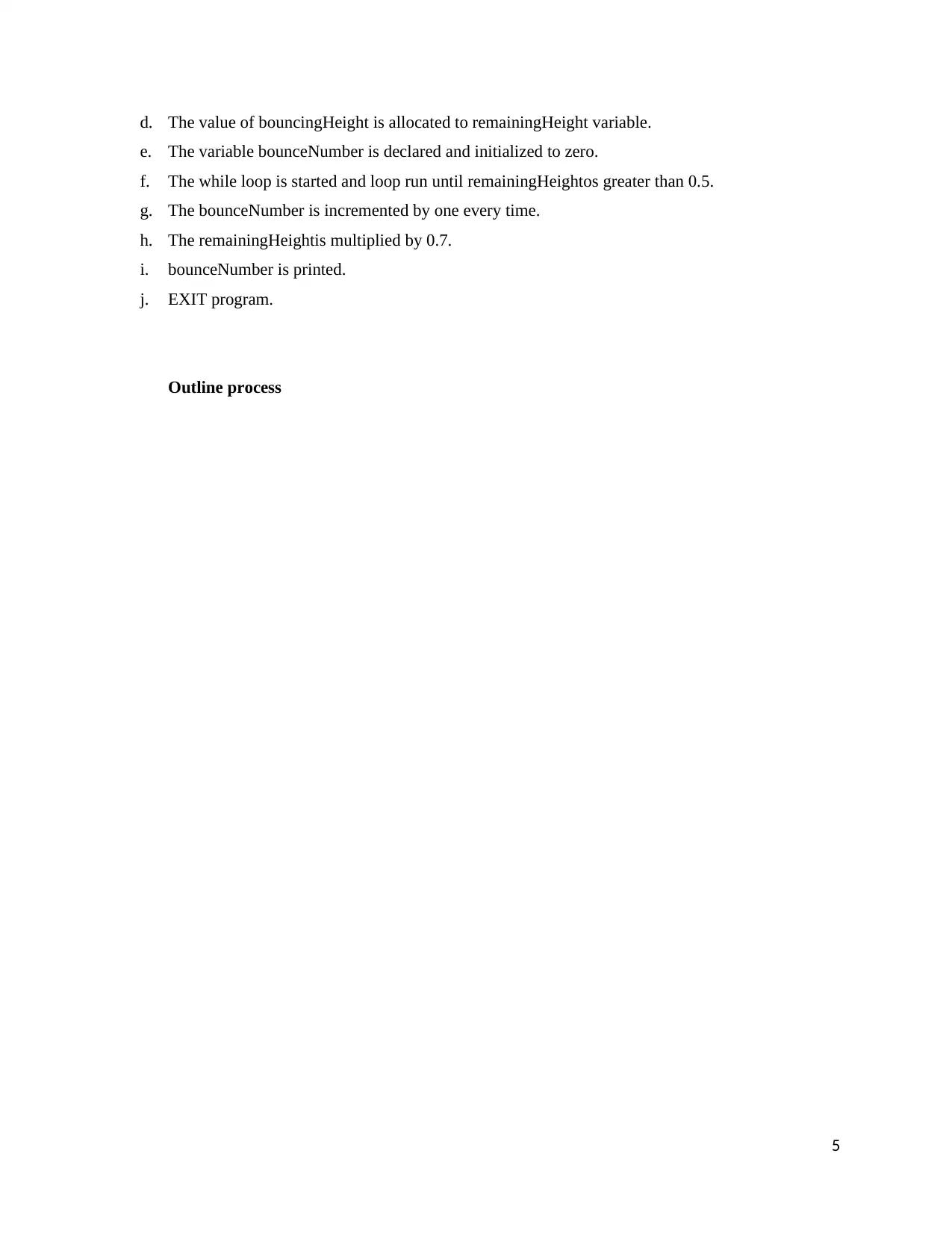
d. The value of bouncingHeight is allocated to remainingHeight variable.
e. The variable bounceNumber is declared and initialized to zero.
f. The while loop is started and loop run until remainingHeightos greater than 0.5.
g. The bounceNumber is incremented by one every time.
h. The remainingHeightis multiplied by 0.7.
i. bounceNumber is printed.
j. EXIT program.
Outline process
5
e. The variable bounceNumber is declared and initialized to zero.
f. The while loop is started and loop run until remainingHeightos greater than 0.5.
g. The bounceNumber is incremented by one every time.
h. The remainingHeightis multiplied by 0.7.
i. bounceNumber is printed.
j. EXIT program.
Outline process
5
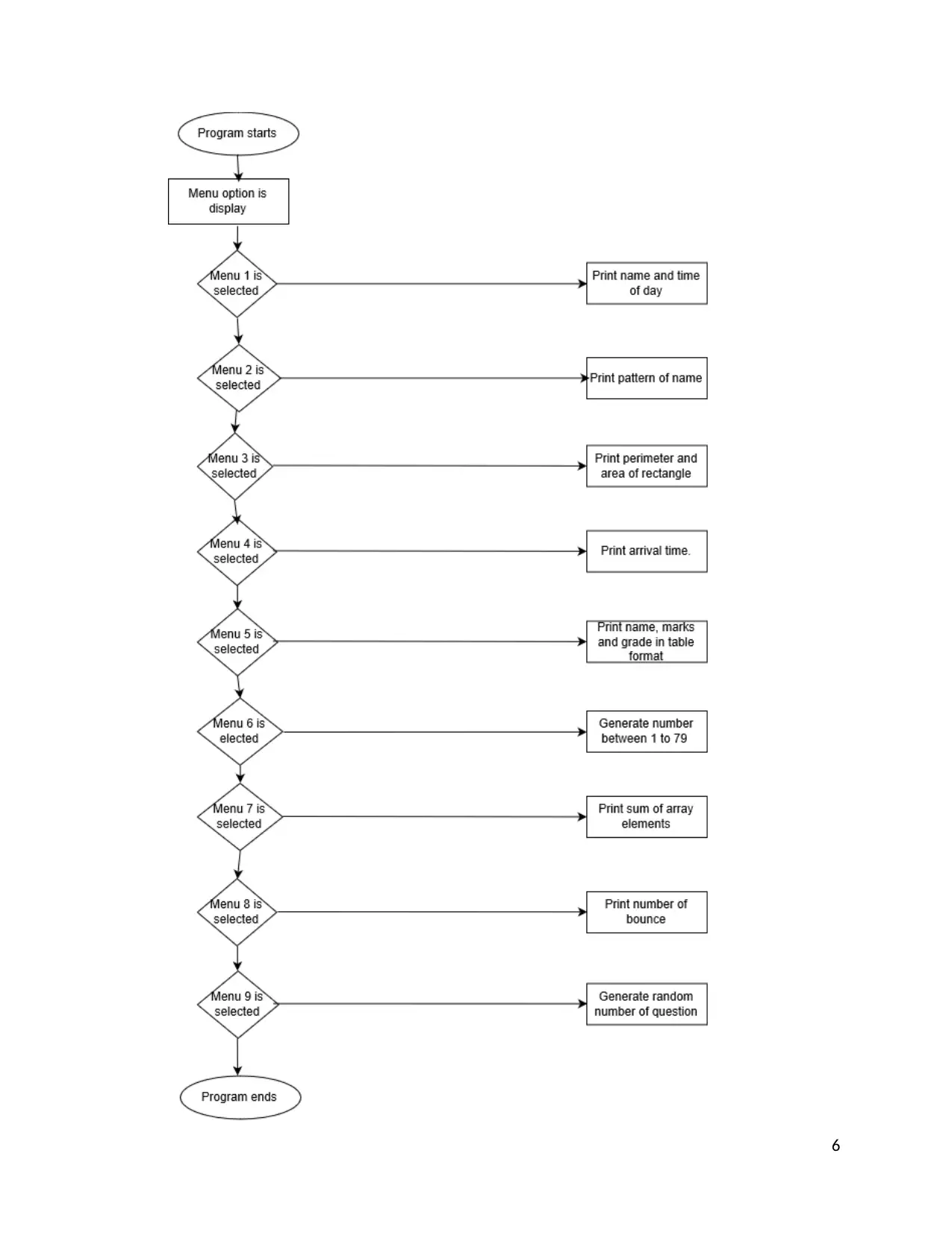
6
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
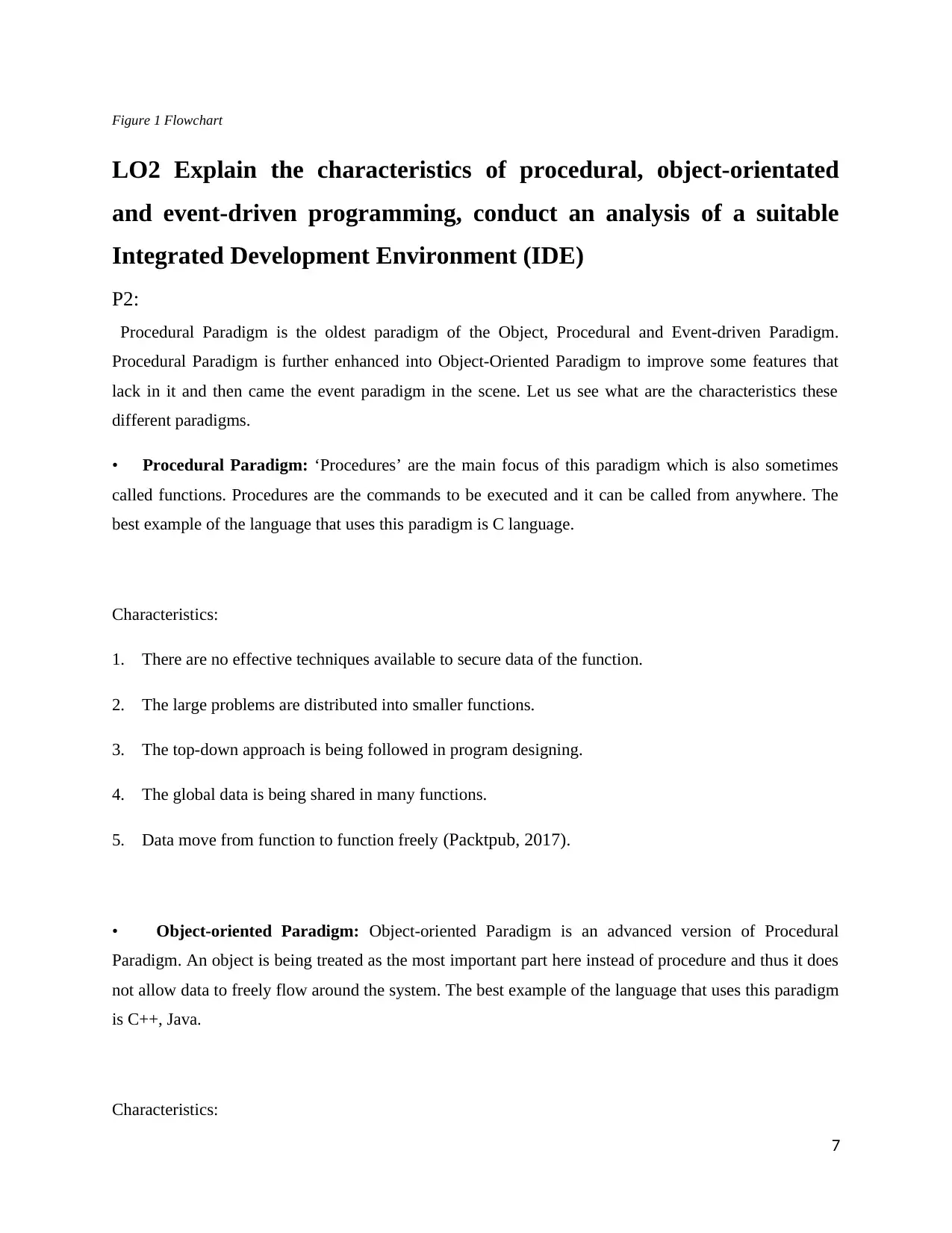
Figure 1 Flowchart
LO2 Explain the characteristics of procedural, object-orientated
and event-driven programming, conduct an analysis of a suitable
Integrated Development Environment (IDE)
P2:
Procedural Paradigm is the oldest paradigm of the Object, Procedural and Event-driven Paradigm.
Procedural Paradigm is further enhanced into Object-Oriented Paradigm to improve some features that
lack in it and then came the event paradigm in the scene. Let us see what are the characteristics these
different paradigms.
• Procedural Paradigm: ‘Procedures’ are the main focus of this paradigm which is also sometimes
called functions. Procedures are the commands to be executed and it can be called from anywhere. The
best example of the language that uses this paradigm is C language.
Characteristics:
1. There are no effective techniques available to secure data of the function.
2. The large problems are distributed into smaller functions.
3. The top-down approach is being followed in program designing.
4. The global data is being shared in many functions.
5. Data move from function to function freely (Packtpub, 2017).
• Object-oriented Paradigm: Object-oriented Paradigm is an advanced version of Procedural
Paradigm. An object is being treated as the most important part here instead of procedure and thus it does
not allow data to freely flow around the system. The best example of the language that uses this paradigm
is C++, Java.
Characteristics:
7
LO2 Explain the characteristics of procedural, object-orientated
and event-driven programming, conduct an analysis of a suitable
Integrated Development Environment (IDE)
P2:
Procedural Paradigm is the oldest paradigm of the Object, Procedural and Event-driven Paradigm.
Procedural Paradigm is further enhanced into Object-Oriented Paradigm to improve some features that
lack in it and then came the event paradigm in the scene. Let us see what are the characteristics these
different paradigms.
• Procedural Paradigm: ‘Procedures’ are the main focus of this paradigm which is also sometimes
called functions. Procedures are the commands to be executed and it can be called from anywhere. The
best example of the language that uses this paradigm is C language.
Characteristics:
1. There are no effective techniques available to secure data of the function.
2. The large problems are distributed into smaller functions.
3. The top-down approach is being followed in program designing.
4. The global data is being shared in many functions.
5. Data move from function to function freely (Packtpub, 2017).
• Object-oriented Paradigm: Object-oriented Paradigm is an advanced version of Procedural
Paradigm. An object is being treated as the most important part here instead of procedure and thus it does
not allow data to freely flow around the system. The best example of the language that uses this paradigm
is C++, Java.
Characteristics:
7
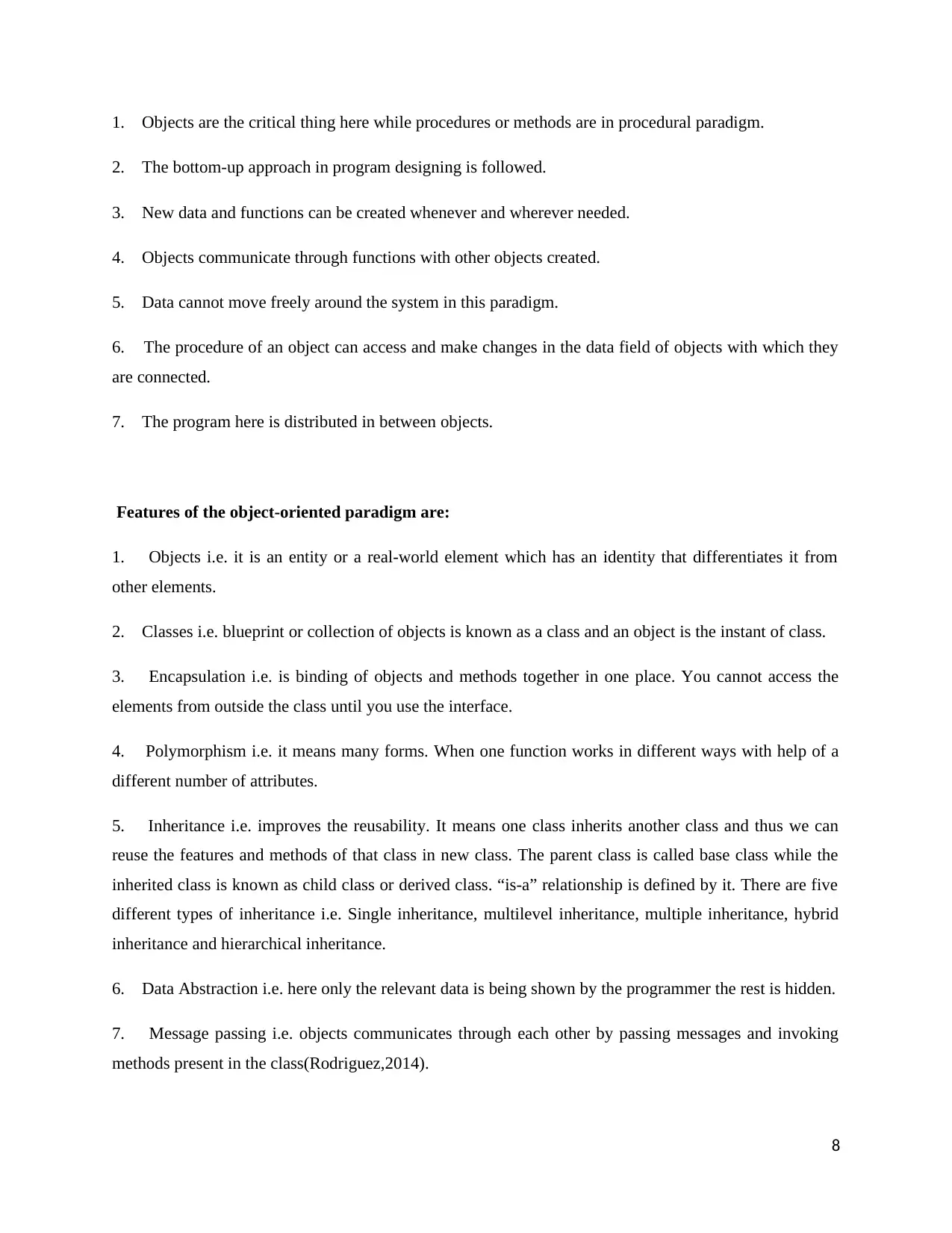
1. Objects are the critical thing here while procedures or methods are in procedural paradigm.
2. The bottom-up approach in program designing is followed.
3. New data and functions can be created whenever and wherever needed.
4. Objects communicate through functions with other objects created.
5. Data cannot move freely around the system in this paradigm.
6. The procedure of an object can access and make changes in the data field of objects with which they
are connected.
7. The program here is distributed in between objects.
Features of the object-oriented paradigm are:
1. Objects i.e. it is an entity or a real-world element which has an identity that differentiates it from
other elements.
2. Classes i.e. blueprint or collection of objects is known as a class and an object is the instant of class.
3. Encapsulation i.e. is binding of objects and methods together in one place. You cannot access the
elements from outside the class until you use the interface.
4. Polymorphism i.e. it means many forms. When one function works in different ways with help of a
different number of attributes.
5. Inheritance i.e. improves the reusability. It means one class inherits another class and thus we can
reuse the features and methods of that class in new class. The parent class is called base class while the
inherited class is known as child class or derived class. “is-a” relationship is defined by it. There are five
different types of inheritance i.e. Single inheritance, multilevel inheritance, multiple inheritance, hybrid
inheritance and hierarchical inheritance.
6. Data Abstraction i.e. here only the relevant data is being shown by the programmer the rest is hidden.
7. Message passing i.e. objects communicates through each other by passing messages and invoking
methods present in the class(Rodriguez,2014).
8
2. The bottom-up approach in program designing is followed.
3. New data and functions can be created whenever and wherever needed.
4. Objects communicate through functions with other objects created.
5. Data cannot move freely around the system in this paradigm.
6. The procedure of an object can access and make changes in the data field of objects with which they
are connected.
7. The program here is distributed in between objects.
Features of the object-oriented paradigm are:
1. Objects i.e. it is an entity or a real-world element which has an identity that differentiates it from
other elements.
2. Classes i.e. blueprint or collection of objects is known as a class and an object is the instant of class.
3. Encapsulation i.e. is binding of objects and methods together in one place. You cannot access the
elements from outside the class until you use the interface.
4. Polymorphism i.e. it means many forms. When one function works in different ways with help of a
different number of attributes.
5. Inheritance i.e. improves the reusability. It means one class inherits another class and thus we can
reuse the features and methods of that class in new class. The parent class is called base class while the
inherited class is known as child class or derived class. “is-a” relationship is defined by it. There are five
different types of inheritance i.e. Single inheritance, multilevel inheritance, multiple inheritance, hybrid
inheritance and hierarchical inheritance.
6. Data Abstraction i.e. here only the relevant data is being shown by the programmer the rest is hidden.
7. Message passing i.e. objects communicates through each other by passing messages and invoking
methods present in the class(Rodriguez,2014).
8
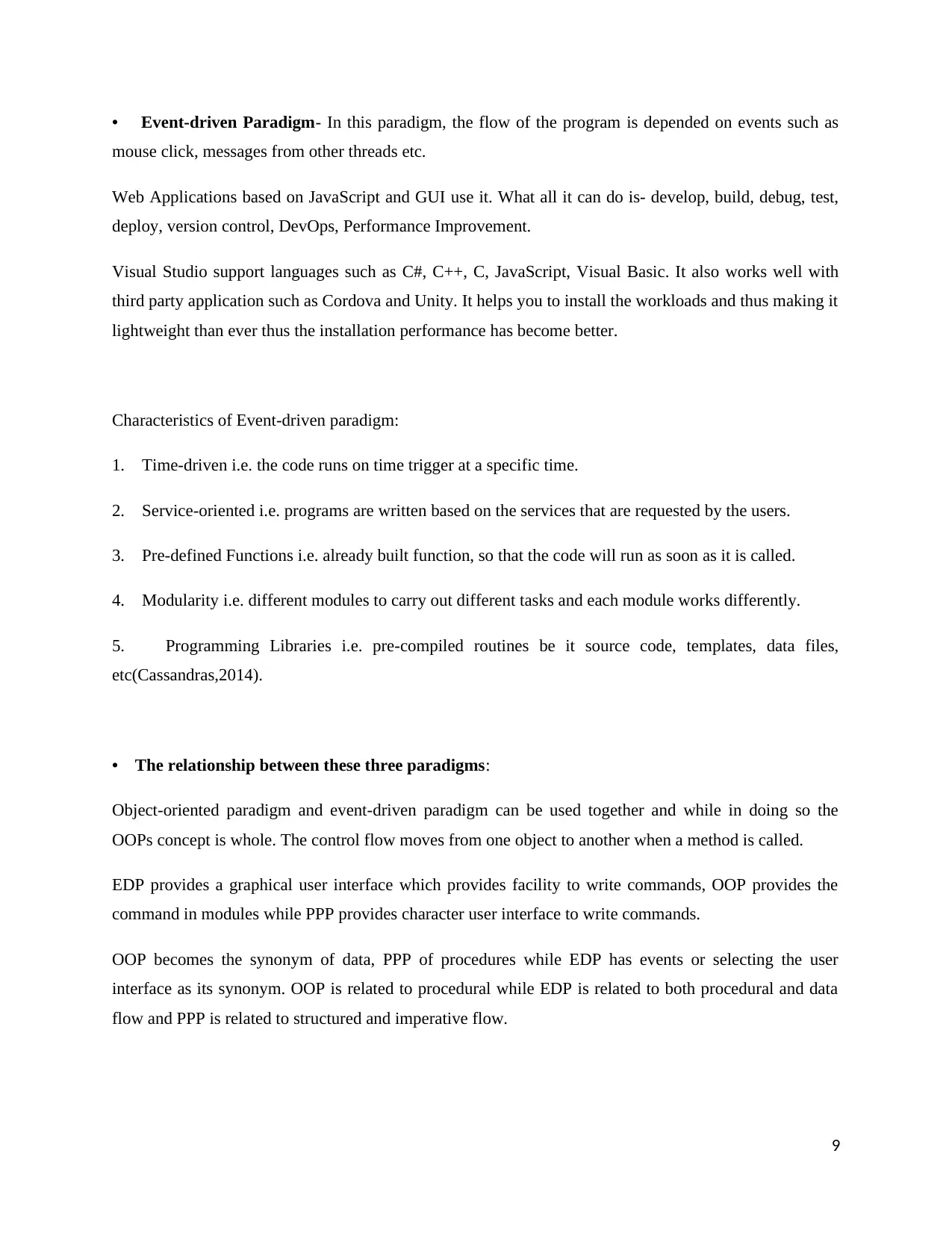
• Event-driven Paradigm- In this paradigm, the flow of the program is depended on events such as
mouse click, messages from other threads etc.
Web Applications based on JavaScript and GUI use it. What all it can do is- develop, build, debug, test,
deploy, version control, DevOps, Performance Improvement.
Visual Studio support languages such as C#, C++, C, JavaScript, Visual Basic. It also works well with
third party application such as Cordova and Unity. It helps you to install the workloads and thus making it
lightweight than ever thus the installation performance has become better.
Characteristics of Event-driven paradigm:
1. Time-driven i.e. the code runs on time trigger at a specific time.
2. Service-oriented i.e. programs are written based on the services that are requested by the users.
3. Pre-defined Functions i.e. already built function, so that the code will run as soon as it is called.
4. Modularity i.e. different modules to carry out different tasks and each module works differently.
5. Programming Libraries i.e. pre-compiled routines be it source code, templates, data files,
etc(Cassandras,2014).
• The relationship between these three paradigms:
Object-oriented paradigm and event-driven paradigm can be used together and while in doing so the
OOPs concept is whole. The control flow moves from one object to another when a method is called.
EDP provides a graphical user interface which provides facility to write commands, OOP provides the
command in modules while PPP provides character user interface to write commands.
OOP becomes the synonym of data, PPP of procedures while EDP has events or selecting the user
interface as its synonym. OOP is related to procedural while EDP is related to both procedural and data
flow and PPP is related to structured and imperative flow.
9
mouse click, messages from other threads etc.
Web Applications based on JavaScript and GUI use it. What all it can do is- develop, build, debug, test,
deploy, version control, DevOps, Performance Improvement.
Visual Studio support languages such as C#, C++, C, JavaScript, Visual Basic. It also works well with
third party application such as Cordova and Unity. It helps you to install the workloads and thus making it
lightweight than ever thus the installation performance has become better.
Characteristics of Event-driven paradigm:
1. Time-driven i.e. the code runs on time trigger at a specific time.
2. Service-oriented i.e. programs are written based on the services that are requested by the users.
3. Pre-defined Functions i.e. already built function, so that the code will run as soon as it is called.
4. Modularity i.e. different modules to carry out different tasks and each module works differently.
5. Programming Libraries i.e. pre-compiled routines be it source code, templates, data files,
etc(Cassandras,2014).
• The relationship between these three paradigms:
Object-oriented paradigm and event-driven paradigm can be used together and while in doing so the
OOPs concept is whole. The control flow moves from one object to another when a method is called.
EDP provides a graphical user interface which provides facility to write commands, OOP provides the
command in modules while PPP provides character user interface to write commands.
OOP becomes the synonym of data, PPP of procedures while EDP has events or selecting the user
interface as its synonym. OOP is related to procedural while EDP is related to both procedural and data
flow and PPP is related to structured and imperative flow.
9
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
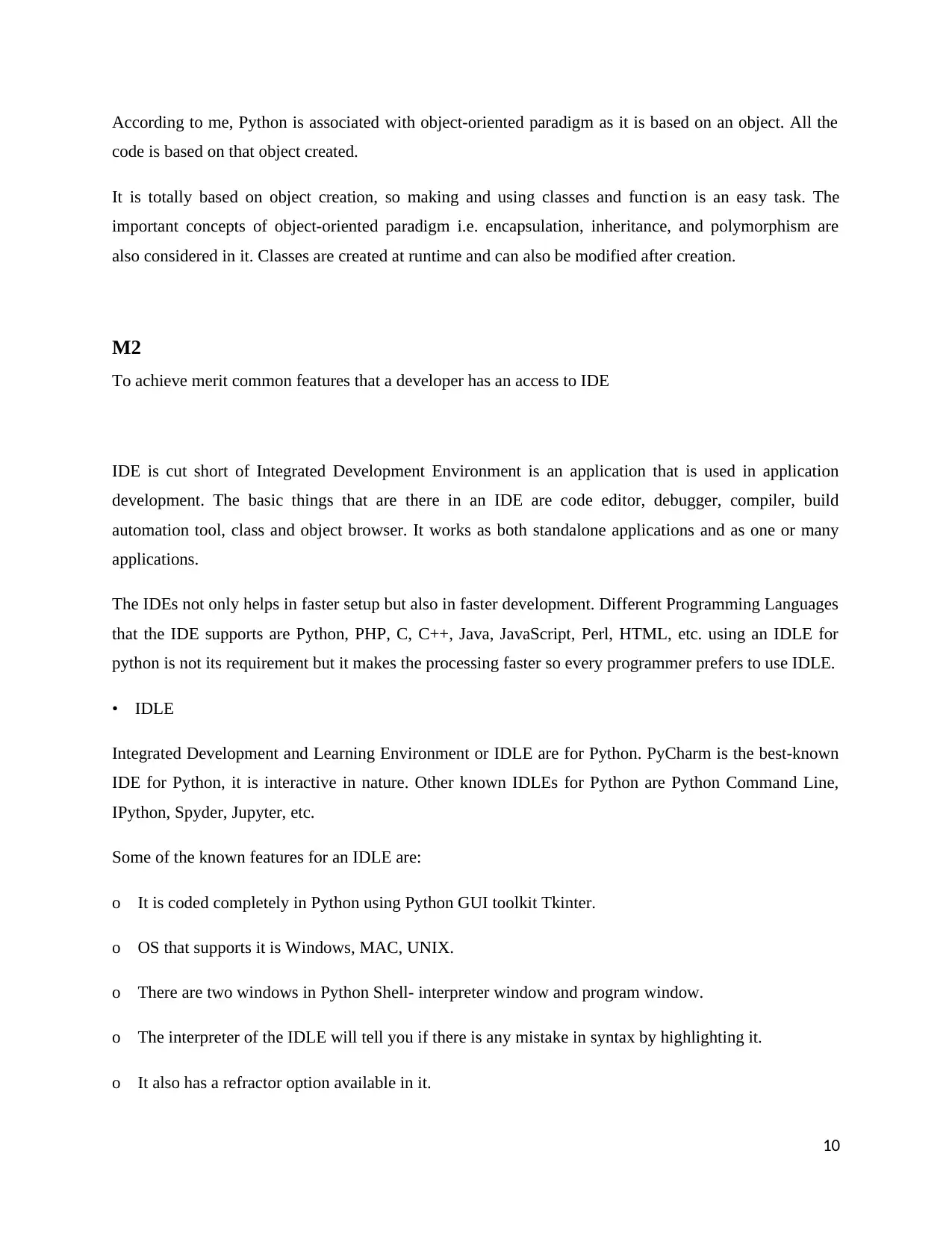
According to me, Python is associated with object-oriented paradigm as it is based on an object. All the
code is based on that object created.
It is totally based on object creation, so making and using classes and functi on is an easy task. The
important concepts of object-oriented paradigm i.e. encapsulation, inheritance, and polymorphism are
also considered in it. Classes are created at runtime and can also be modified after creation.
M2
To achieve merit common features that a developer has an access to IDE
IDE is cut short of Integrated Development Environment is an application that is used in application
development. The basic things that are there in an IDE are code editor, debugger, compiler, build
automation tool, class and object browser. It works as both standalone applications and as one or many
applications.
The IDEs not only helps in faster setup but also in faster development. Different Programming Languages
that the IDE supports are Python, PHP, C, C++, Java, JavaScript, Perl, HTML, etc. using an IDLE for
python is not its requirement but it makes the processing faster so every programmer prefers to use IDLE.
• IDLE
Integrated Development and Learning Environment or IDLE are for Python. PyCharm is the best-known
IDE for Python, it is interactive in nature. Other known IDLEs for Python are Python Command Line,
IPython, Spyder, Jupyter, etc.
Some of the known features for an IDLE are:
o It is coded completely in Python using Python GUI toolkit Tkinter.
o OS that supports it is Windows, MAC, UNIX.
o There are two windows in Python Shell- interpreter window and program window.
o The interpreter of the IDLE will tell you if there is any mistake in syntax by highlighting it.
o It also has a refractor option available in it.
10
code is based on that object created.
It is totally based on object creation, so making and using classes and functi on is an easy task. The
important concepts of object-oriented paradigm i.e. encapsulation, inheritance, and polymorphism are
also considered in it. Classes are created at runtime and can also be modified after creation.
M2
To achieve merit common features that a developer has an access to IDE
IDE is cut short of Integrated Development Environment is an application that is used in application
development. The basic things that are there in an IDE are code editor, debugger, compiler, build
automation tool, class and object browser. It works as both standalone applications and as one or many
applications.
The IDEs not only helps in faster setup but also in faster development. Different Programming Languages
that the IDE supports are Python, PHP, C, C++, Java, JavaScript, Perl, HTML, etc. using an IDLE for
python is not its requirement but it makes the processing faster so every programmer prefers to use IDLE.
• IDLE
Integrated Development and Learning Environment or IDLE are for Python. PyCharm is the best-known
IDE for Python, it is interactive in nature. Other known IDLEs for Python are Python Command Line,
IPython, Spyder, Jupyter, etc.
Some of the known features for an IDLE are:
o It is coded completely in Python using Python GUI toolkit Tkinter.
o OS that supports it is Windows, MAC, UNIX.
o There are two windows in Python Shell- interpreter window and program window.
o The interpreter of the IDLE will tell you if there is any mistake in syntax by highlighting it.
o It also has a refractor option available in it.
10
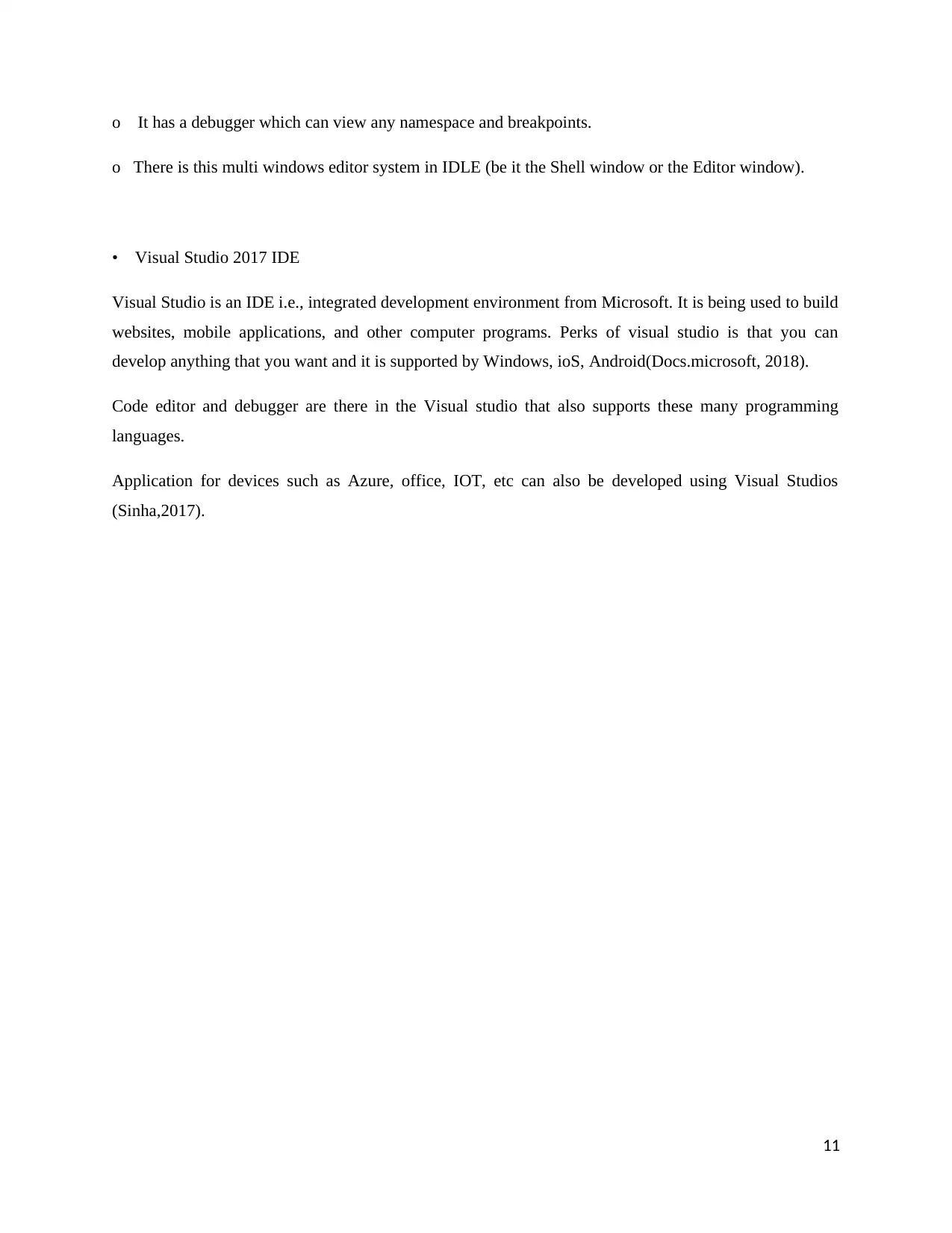
o It has a debugger which can view any namespace and breakpoints.
o There is this multi windows editor system in IDLE (be it the Shell window or the Editor window).
• Visual Studio 2017 IDE
Visual Studio is an IDE i.e., integrated development environment from Microsoft. It is being used to build
websites, mobile applications, and other computer programs. Perks of visual studio is that you can
develop anything that you want and it is supported by Windows, ioS, Android(Docs.microsoft, 2018).
Code editor and debugger are there in the Visual studio that also supports these many programming
languages.
Application for devices such as Azure, office, IOT, etc can also be developed using Visual Studios
(Sinha,2017).
11
o There is this multi windows editor system in IDLE (be it the Shell window or the Editor window).
• Visual Studio 2017 IDE
Visual Studio is an IDE i.e., integrated development environment from Microsoft. It is being used to build
websites, mobile applications, and other computer programs. Perks of visual studio is that you can
develop anything that you want and it is supported by Windows, ioS, Android(Docs.microsoft, 2018).
Code editor and debugger are there in the Visual studio that also supports these many programming
languages.
Application for devices such as Azure, office, IOT, etc can also be developed using Visual Studios
(Sinha,2017).
11
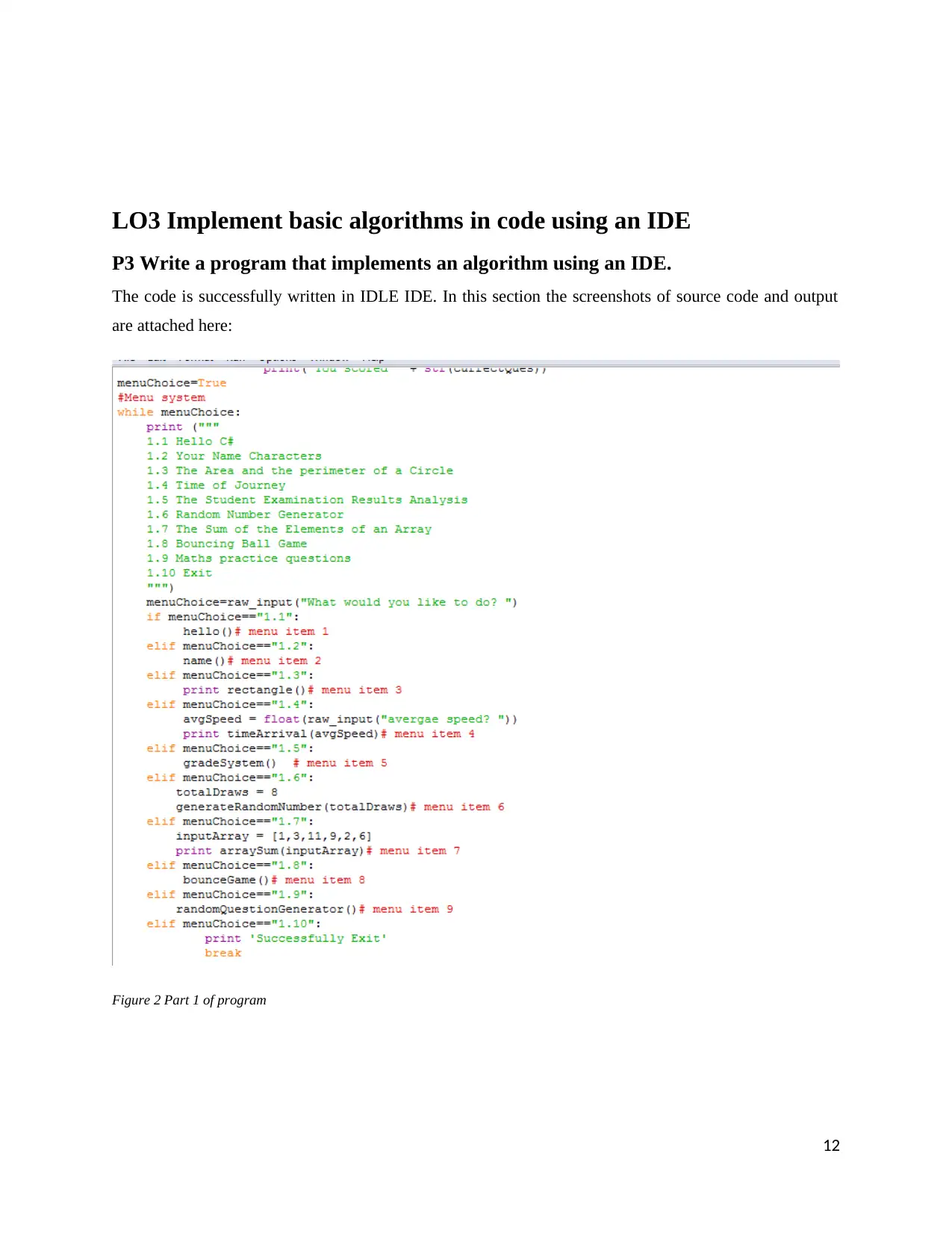
LO3 Implement basic algorithms in code using an IDE
P3 Write a program that implements an algorithm using an IDE.
The code is successfully written in IDLE IDE. In this section the screenshots of source code and output
are attached here:
Figure 2 Part 1 of program
12
P3 Write a program that implements an algorithm using an IDE.
The code is successfully written in IDLE IDE. In this section the screenshots of source code and output
are attached here:
Figure 2 Part 1 of program
12
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
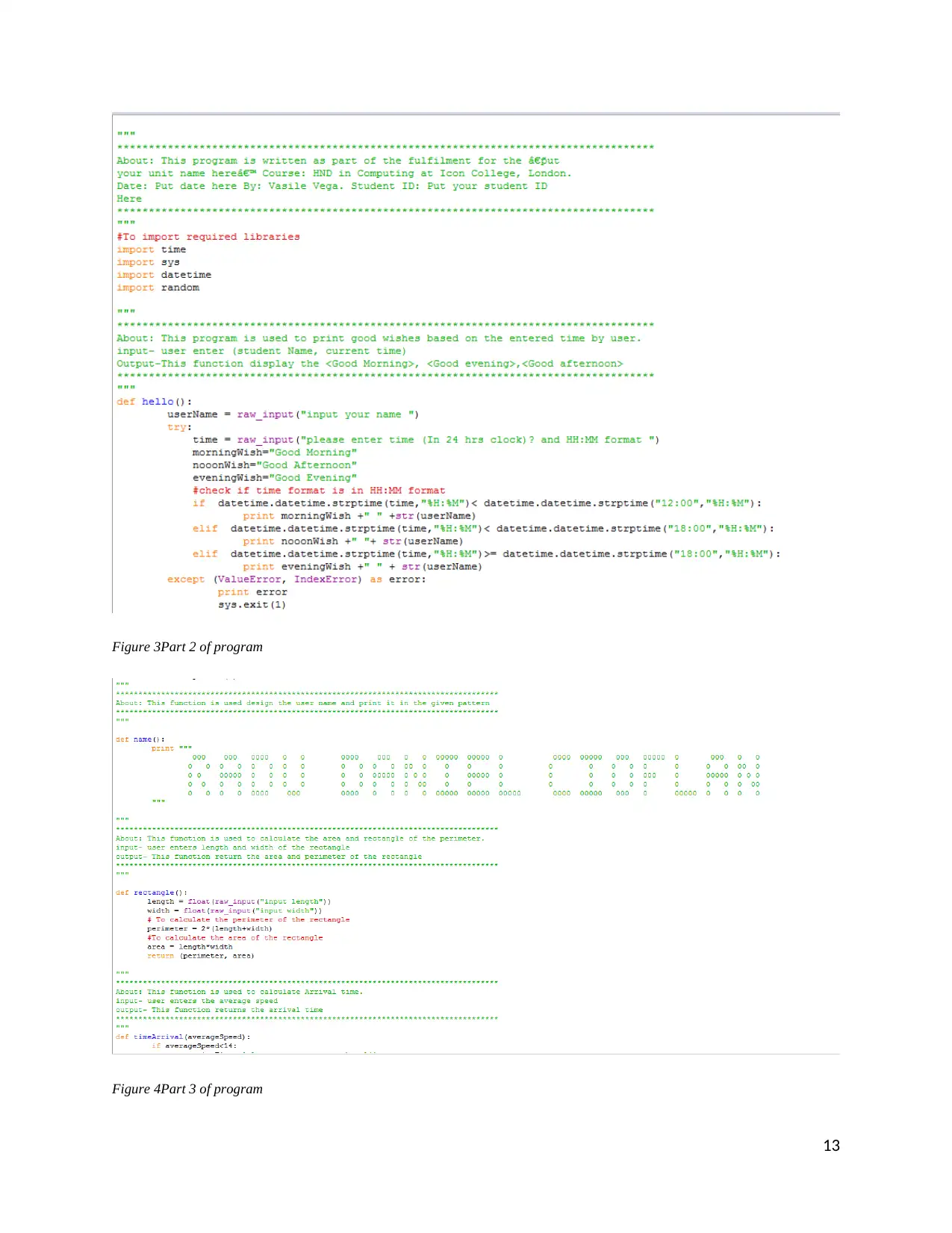
Figure 3Part 2 of program
Figure 4Part 3 of program
13
Figure 4Part 3 of program
13
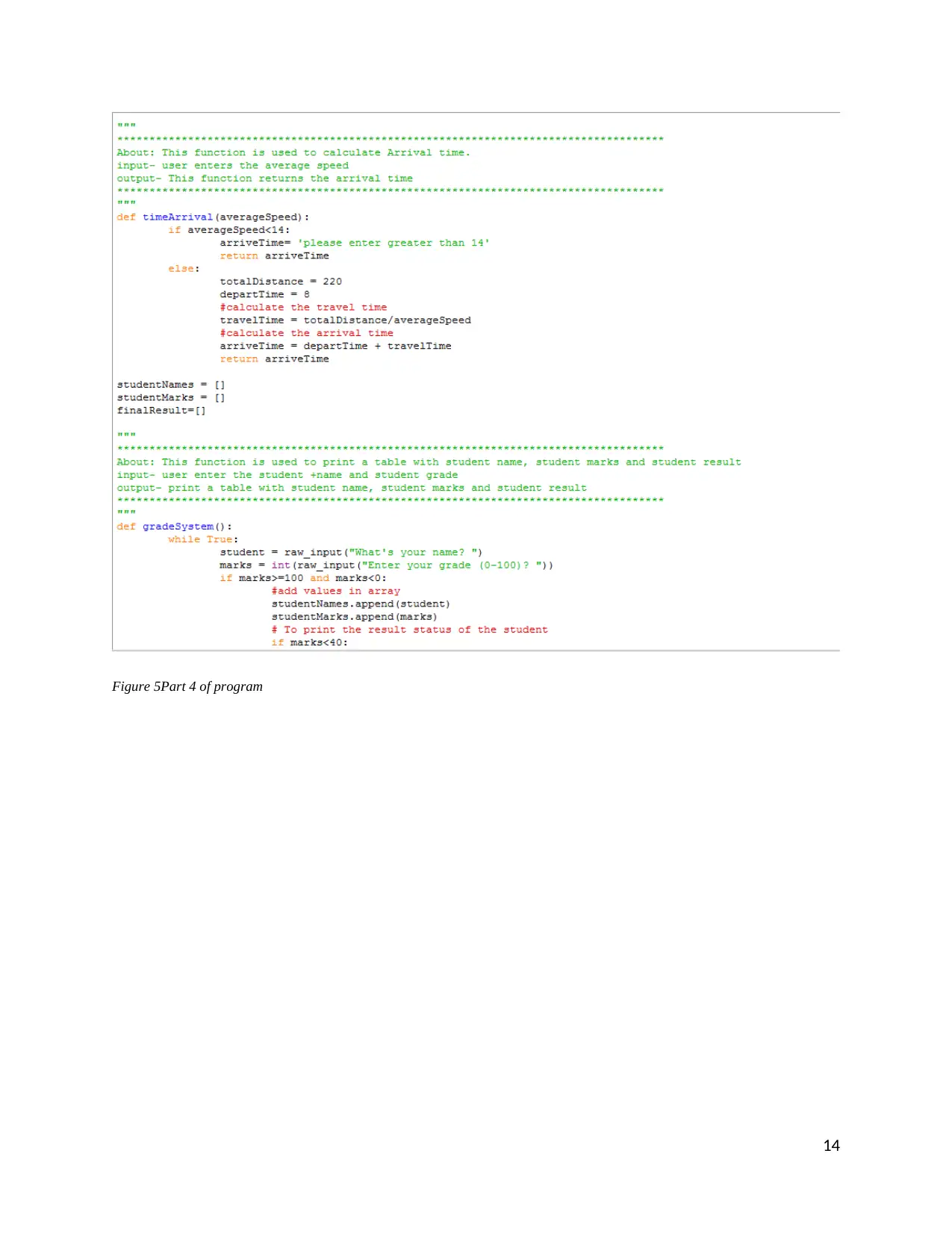
Figure 5Part 4 of program
14
14
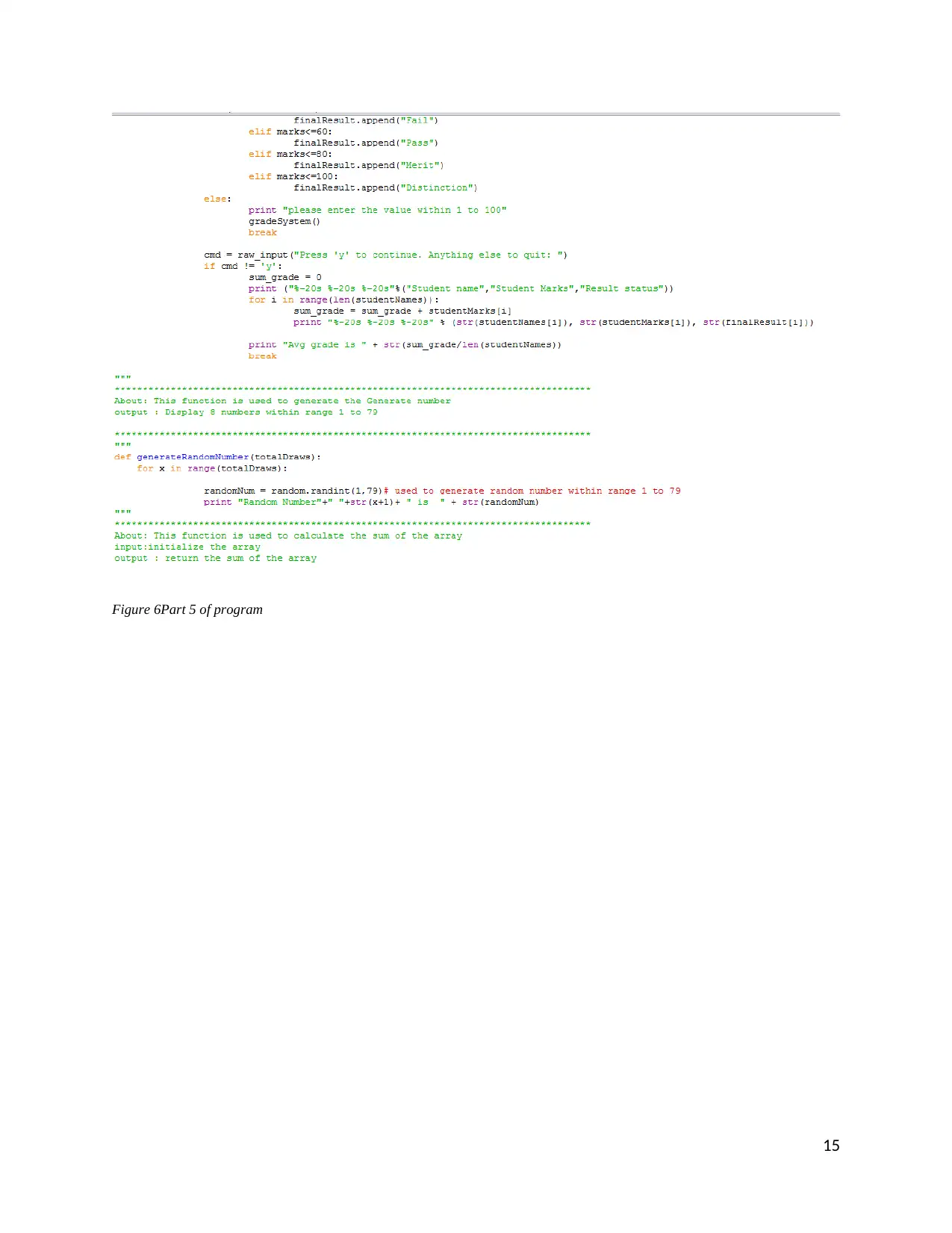
Figure 6Part 5 of program
15
15
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.

Figure 7Part 6 of program
16
16
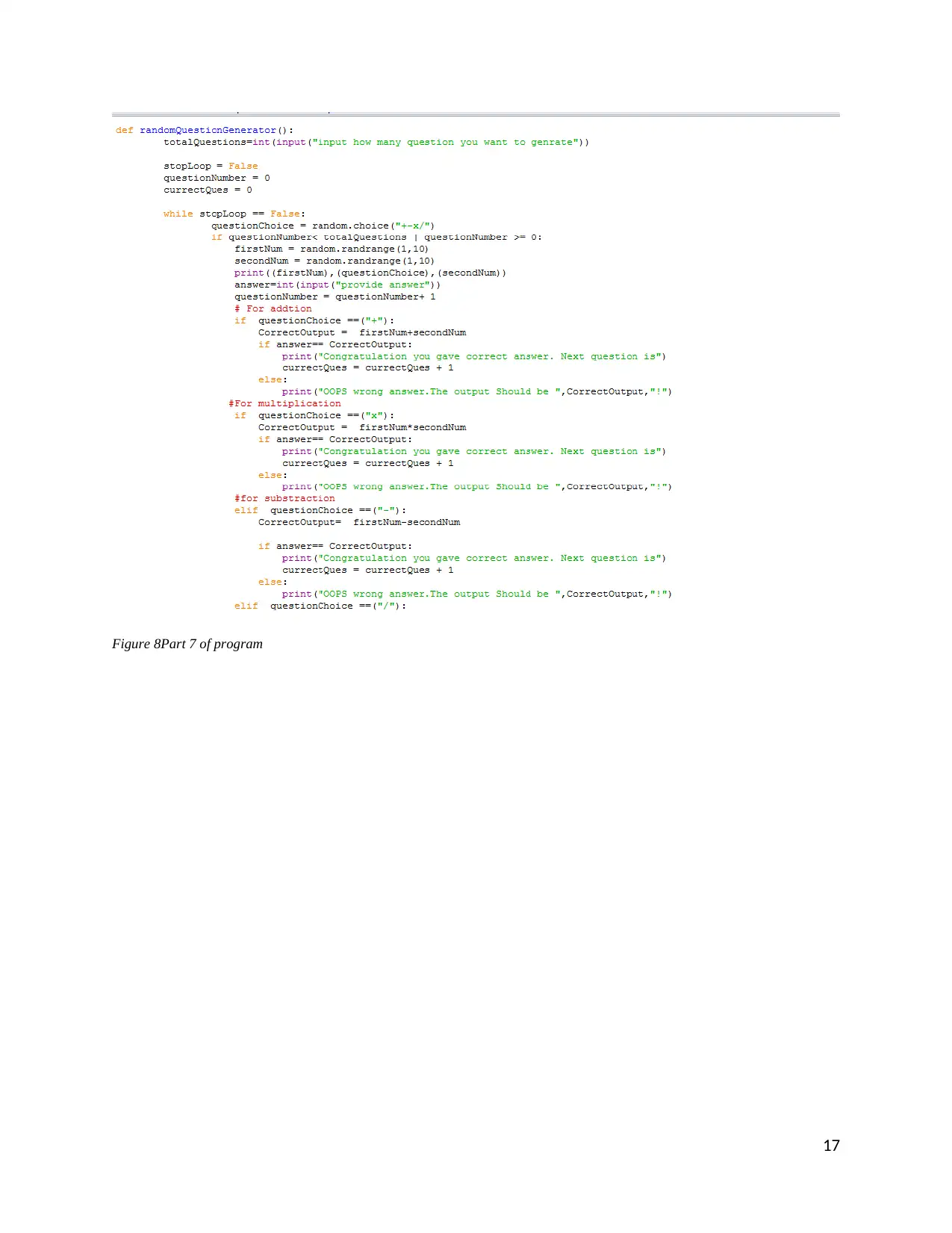
Figure 8Part 7 of program
17
17
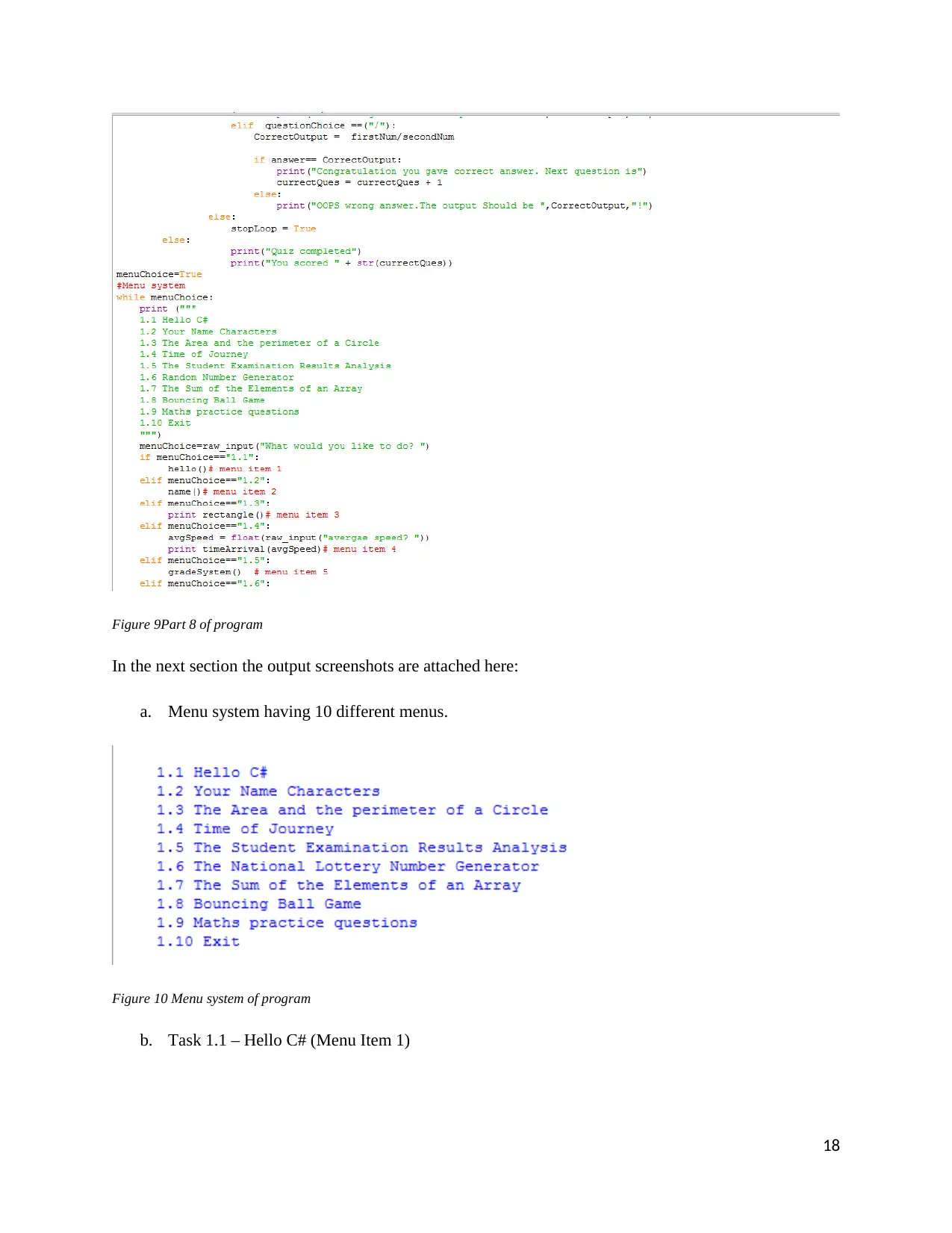
Figure 9Part 8 of program
In the next section the output screenshots are attached here:
a. Menu system having 10 different menus.
Figure 10 Menu system of program
b. Task 1.1 – Hello C# (Menu Item 1)
18
In the next section the output screenshots are attached here:
a. Menu system having 10 different menus.
Figure 10 Menu system of program
b. Task 1.1 – Hello C# (Menu Item 1)
18
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
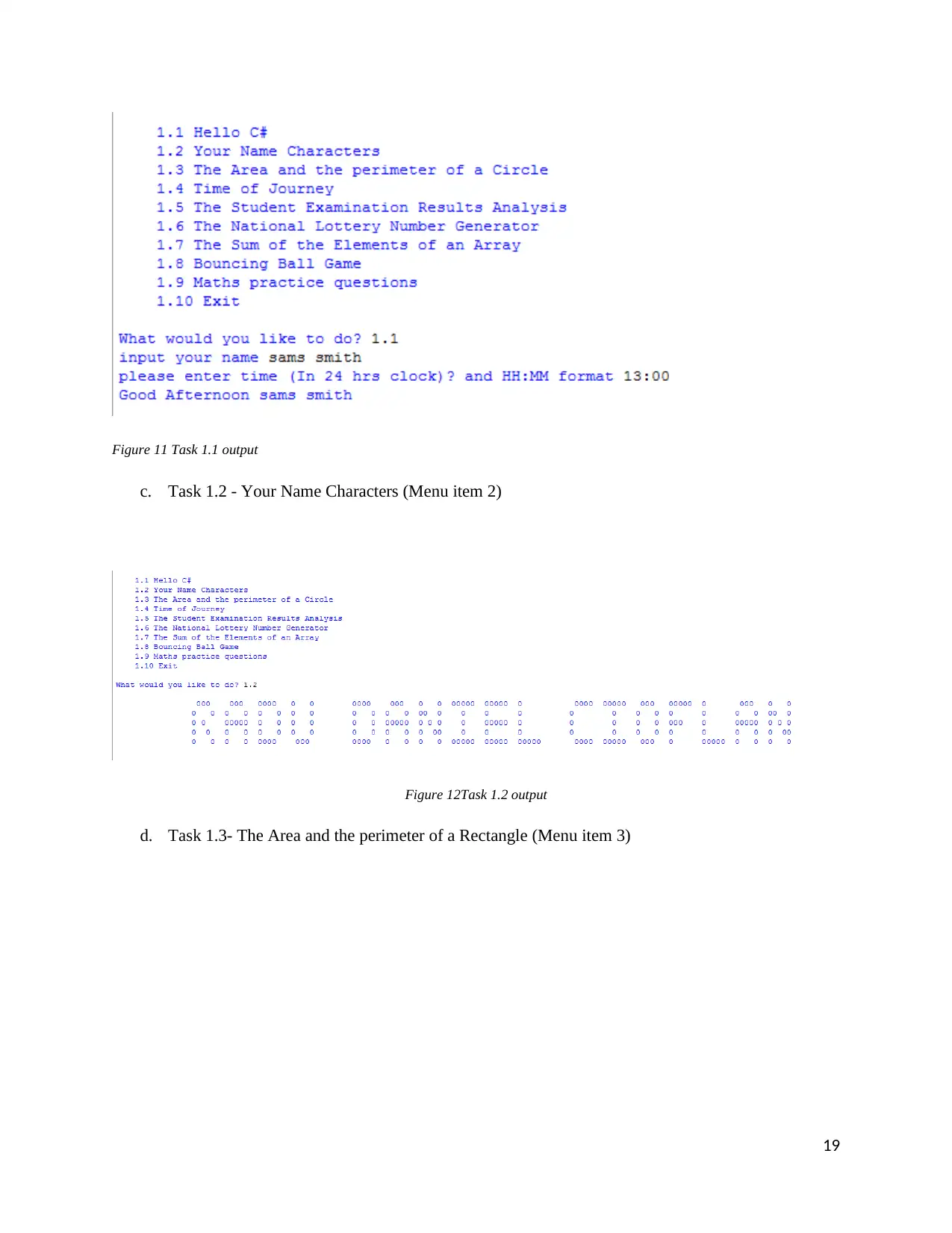
Figure 11 Task 1.1 output
c. Task 1.2 - Your Name Characters (Menu item 2)
Figure 12Task 1.2 output
d. Task 1.3- The Area and the perimeter of a Rectangle (Menu item 3)
19
c. Task 1.2 - Your Name Characters (Menu item 2)
Figure 12Task 1.2 output
d. Task 1.3- The Area and the perimeter of a Rectangle (Menu item 3)
19
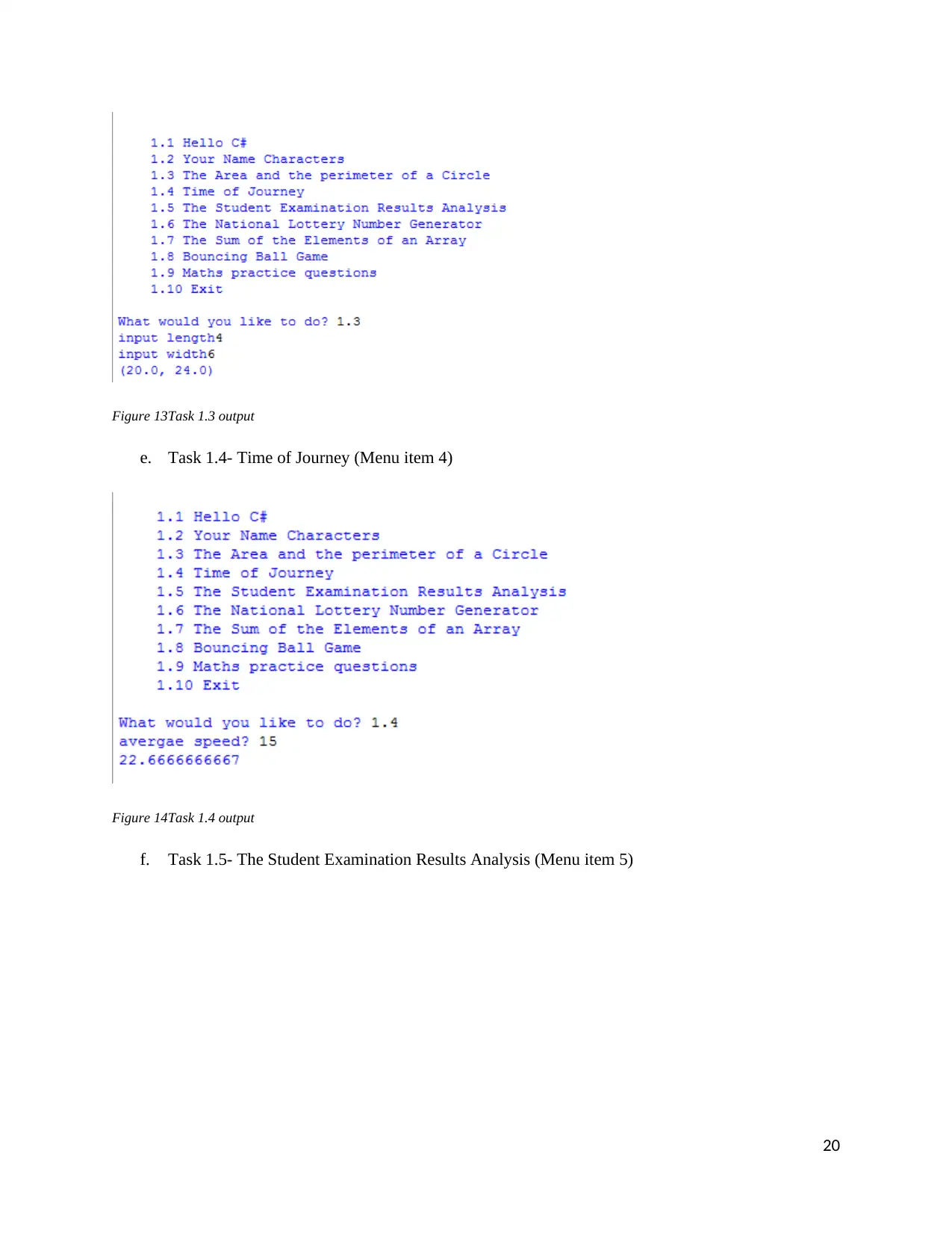
Figure 13Task 1.3 output
e. Task 1.4- Time of Journey (Menu item 4)
Figure 14Task 1.4 output
f. Task 1.5- The Student Examination Results Analysis (Menu item 5)
20
e. Task 1.4- Time of Journey (Menu item 4)
Figure 14Task 1.4 output
f. Task 1.5- The Student Examination Results Analysis (Menu item 5)
20
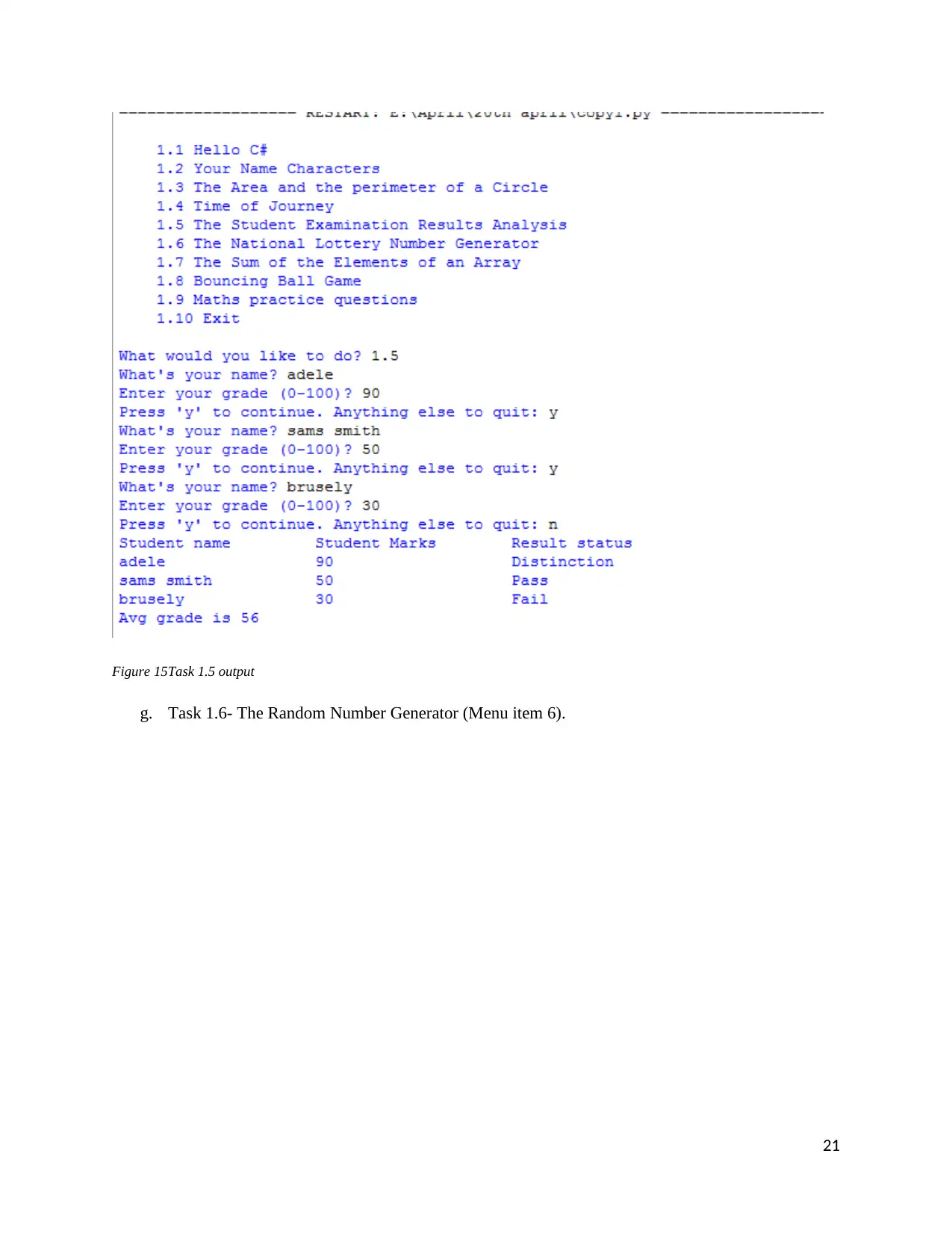
Figure 15Task 1.5 output
g. Task 1.6- The Random Number Generator (Menu item 6).
21
g. Task 1.6- The Random Number Generator (Menu item 6).
21
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
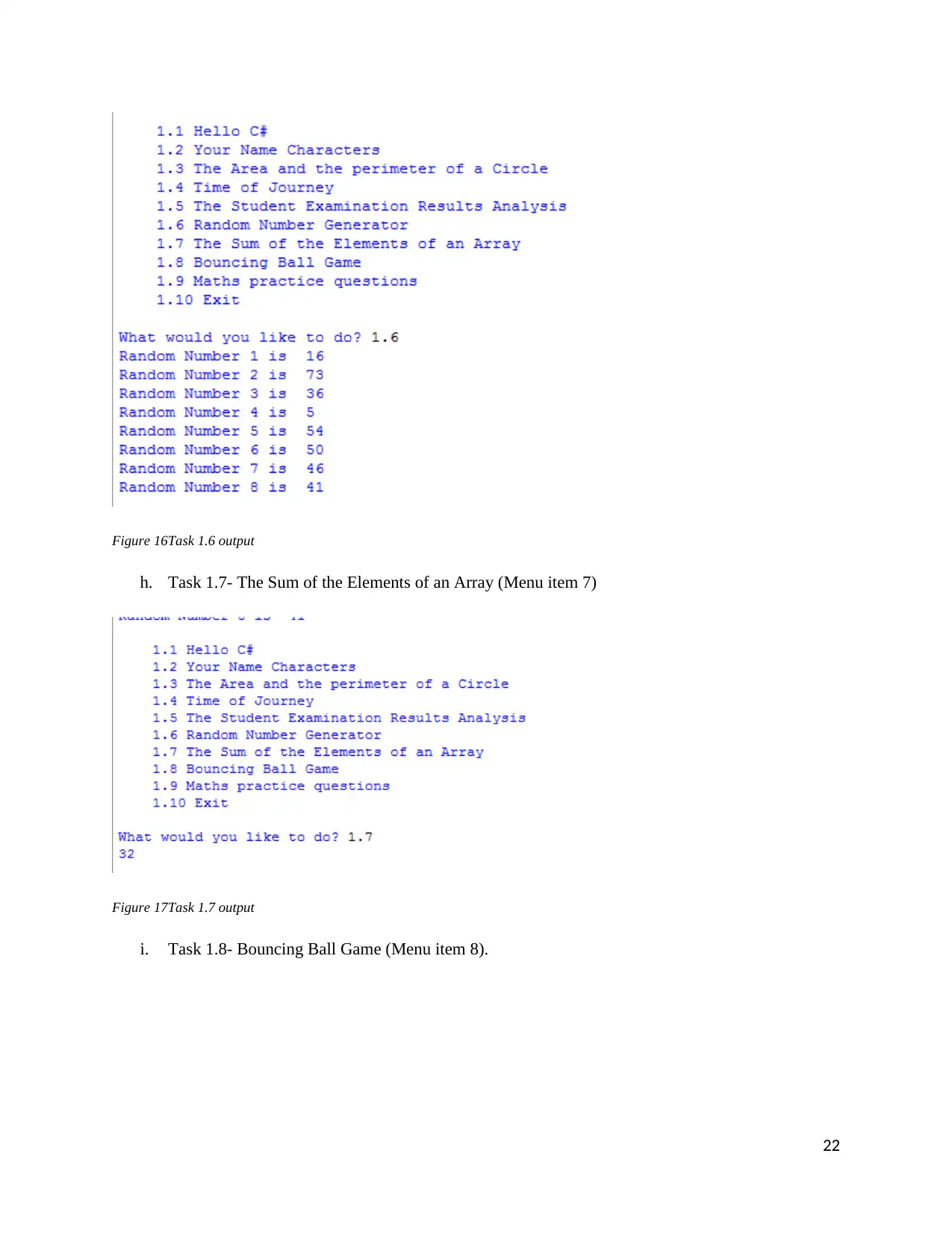
Figure 16Task 1.6 output
h. Task 1.7- The Sum of the Elements of an Array (Menu item 7)
Figure 17Task 1.7 output
i. Task 1.8- Bouncing Ball Game (Menu item 8).
22
h. Task 1.7- The Sum of the Elements of an Array (Menu item 7)
Figure 17Task 1.7 output
i. Task 1.8- Bouncing Ball Game (Menu item 8).
22
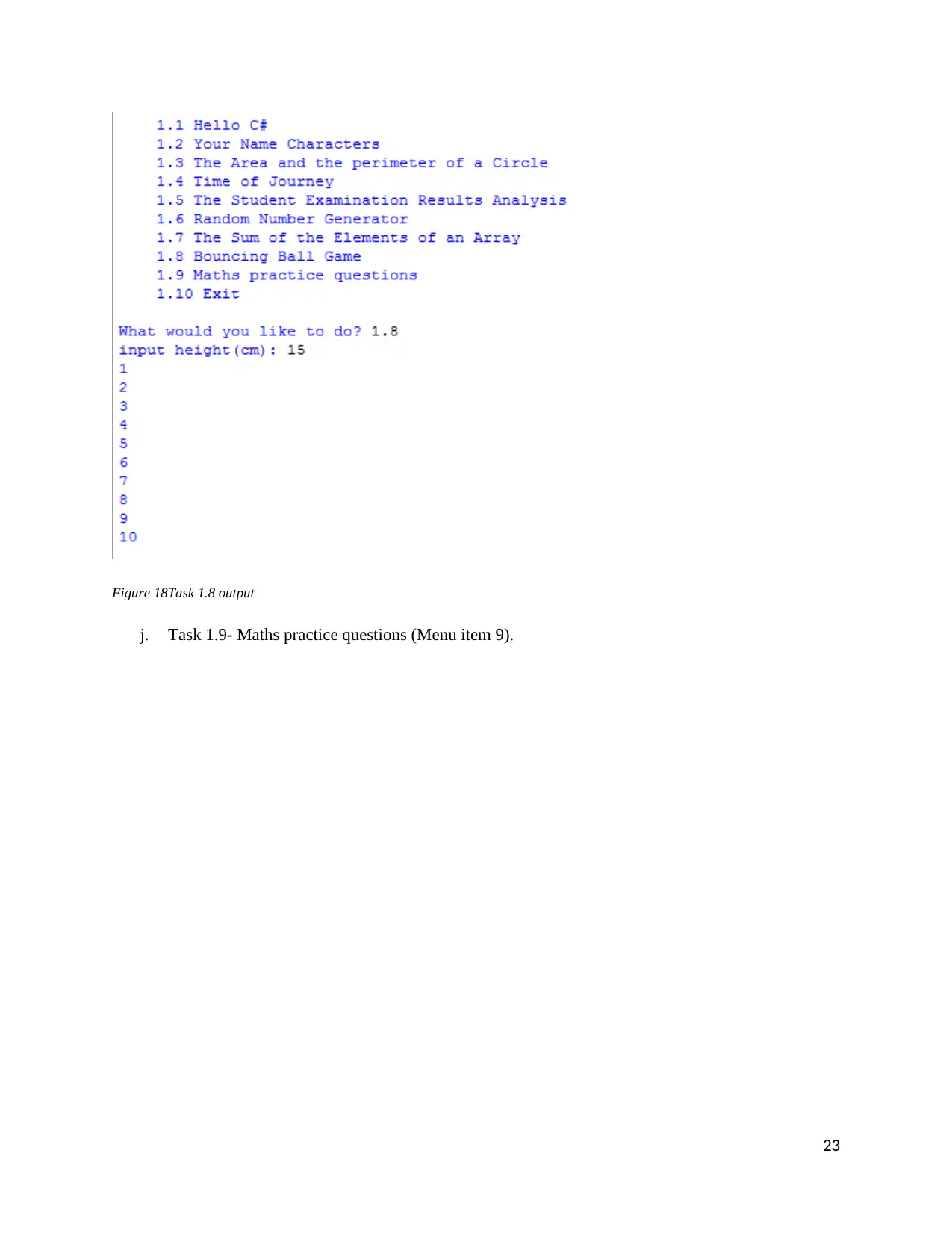
Figure 18Task 1.8 output
j. Task 1.9- Maths practice questions (Menu item 9).
23
j. Task 1.9- Maths practice questions (Menu item 9).
23
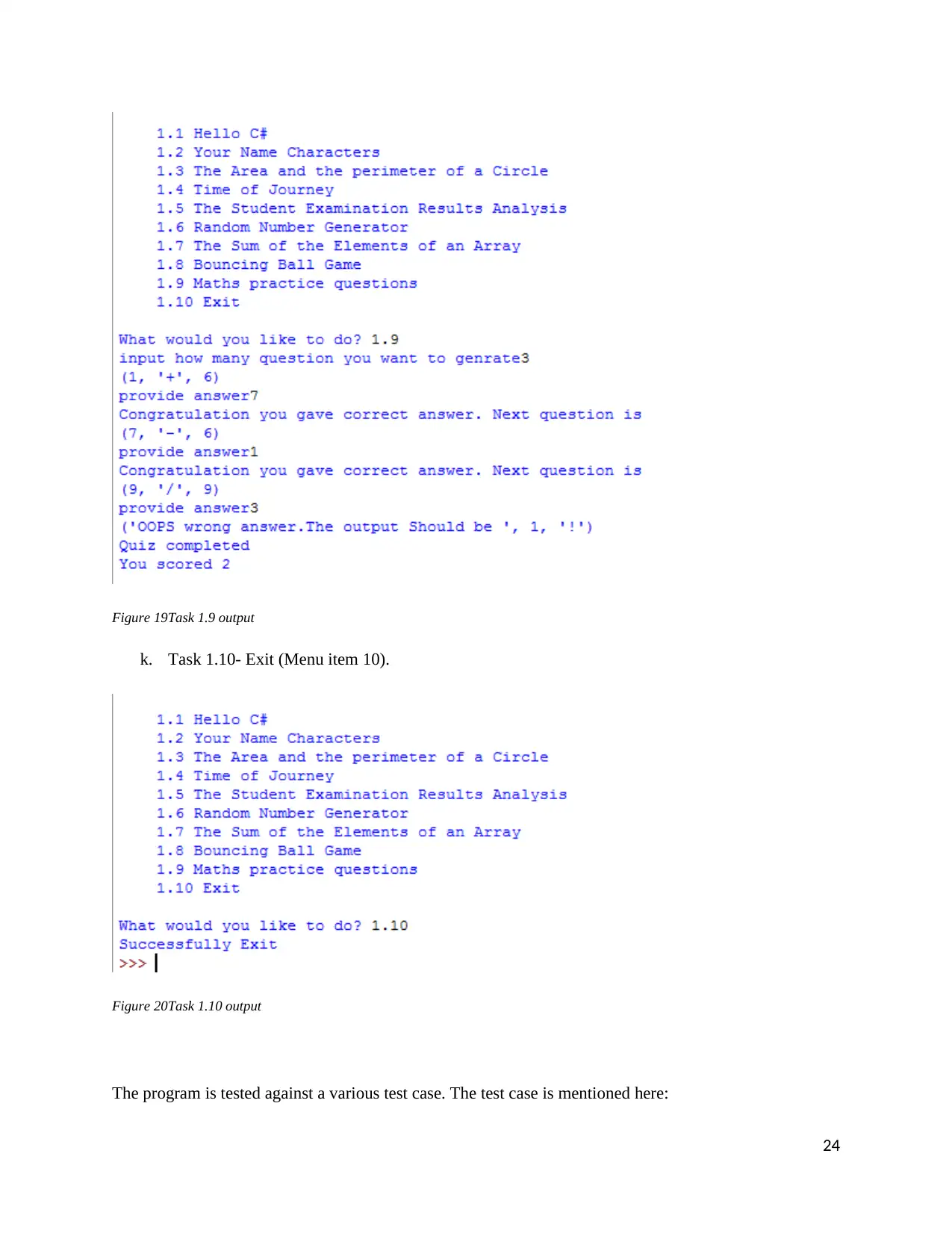
Figure 19Task 1.9 output
k. Task 1.10- Exit (Menu item 10).
Figure 20Task 1.10 output
The program is tested against a various test case. The test case is mentioned here:
24
k. Task 1.10- Exit (Menu item 10).
Figure 20Task 1.10 output
The program is tested against a various test case. The test case is mentioned here:
24
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
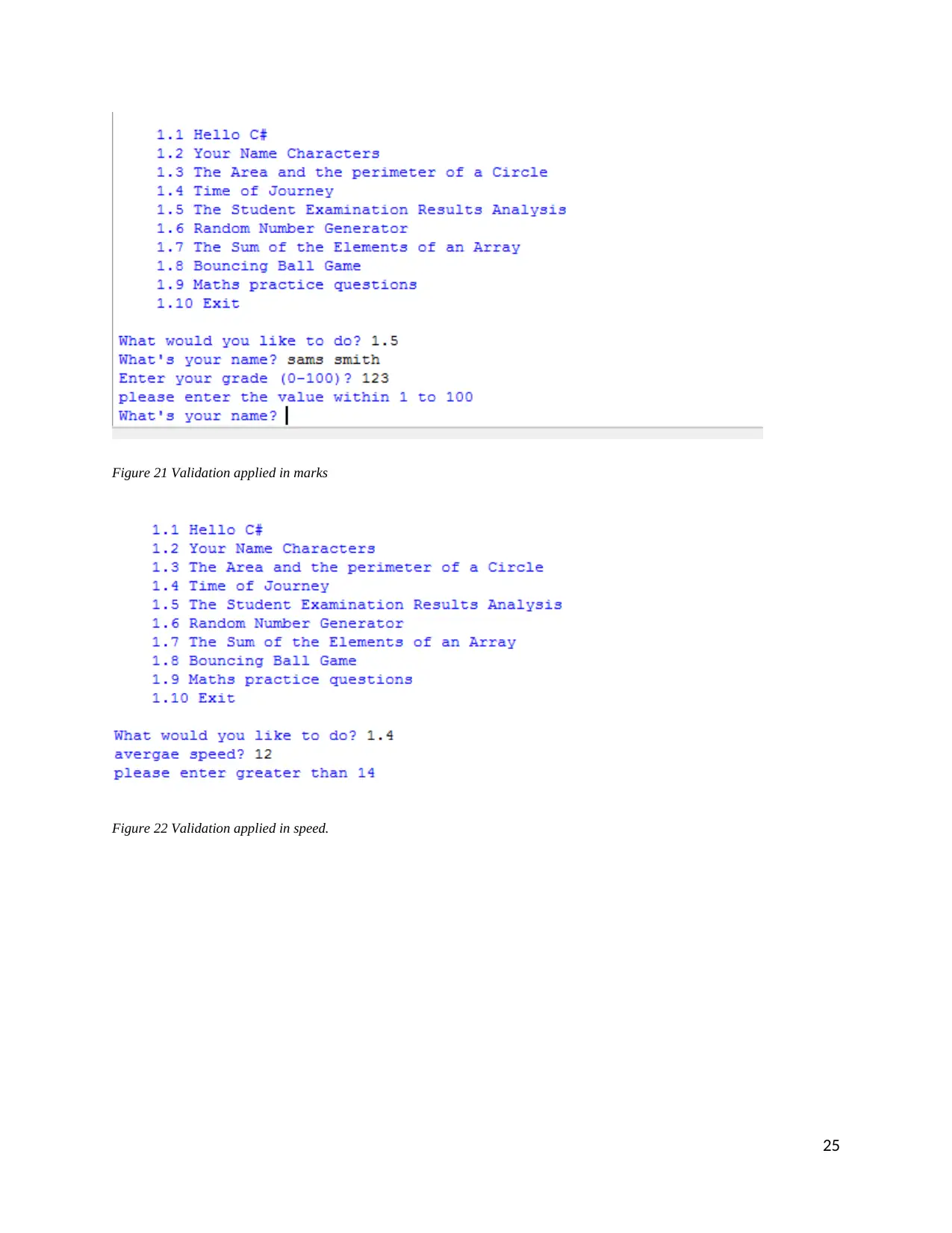
Figure 21 Validation applied in marks
Figure 22 Validation applied in speed.
25
Figure 22 Validation applied in speed.
25
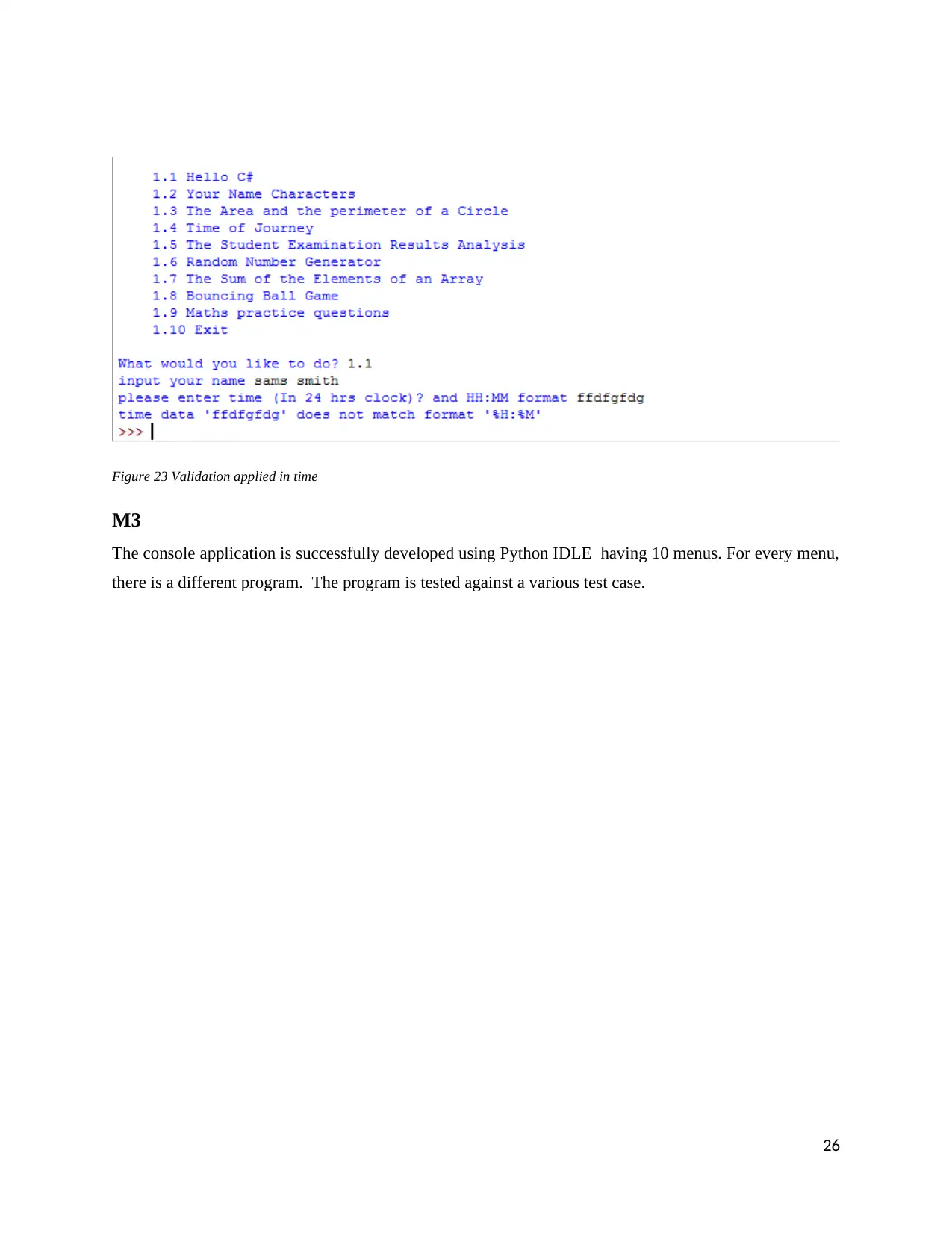
Figure 23 Validation applied in time
M3
The console application is successfully developed using Python IDLE having 10 menus. For every menu,
there is a different program. The program is tested against a various test case.
26
M3
The console application is successfully developed using Python IDLE having 10 menus. For every menu,
there is a different program. The program is tested against a various test case.
26
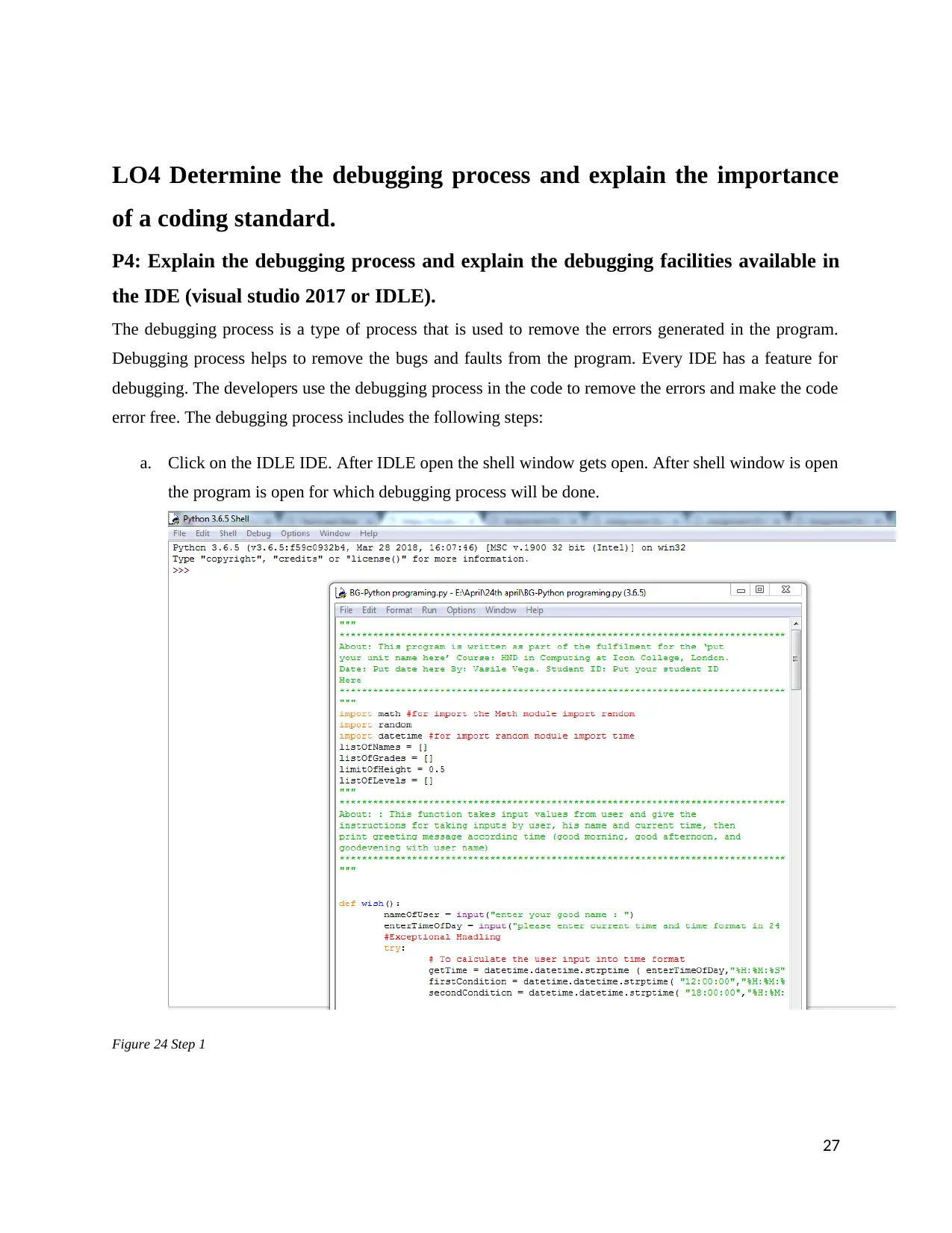
LO4 Determine the debugging process and explain the importance
of a coding standard.
P4: Explain the debugging process and explain the debugging facilities available in
the IDE (visual studio 2017 or IDLE).
The debugging process is a type of process that is used to remove the errors generated in the program.
Debugging process helps to remove the bugs and faults from the program. Every IDE has a feature for
debugging. The developers use the debugging process in the code to remove the errors and make the code
error free. The debugging process includes the following steps:
a. Click on the IDLE IDE. After IDLE open the shell window gets open. After shell window is open
the program is open for which debugging process will be done.
Figure 24 Step 1
27
of a coding standard.
P4: Explain the debugging process and explain the debugging facilities available in
the IDE (visual studio 2017 or IDLE).
The debugging process is a type of process that is used to remove the errors generated in the program.
Debugging process helps to remove the bugs and faults from the program. Every IDE has a feature for
debugging. The developers use the debugging process in the code to remove the errors and make the code
error free. The debugging process includes the following steps:
a. Click on the IDLE IDE. After IDLE open the shell window gets open. After shell window is open
the program is open for which debugging process will be done.
Figure 24 Step 1
27
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
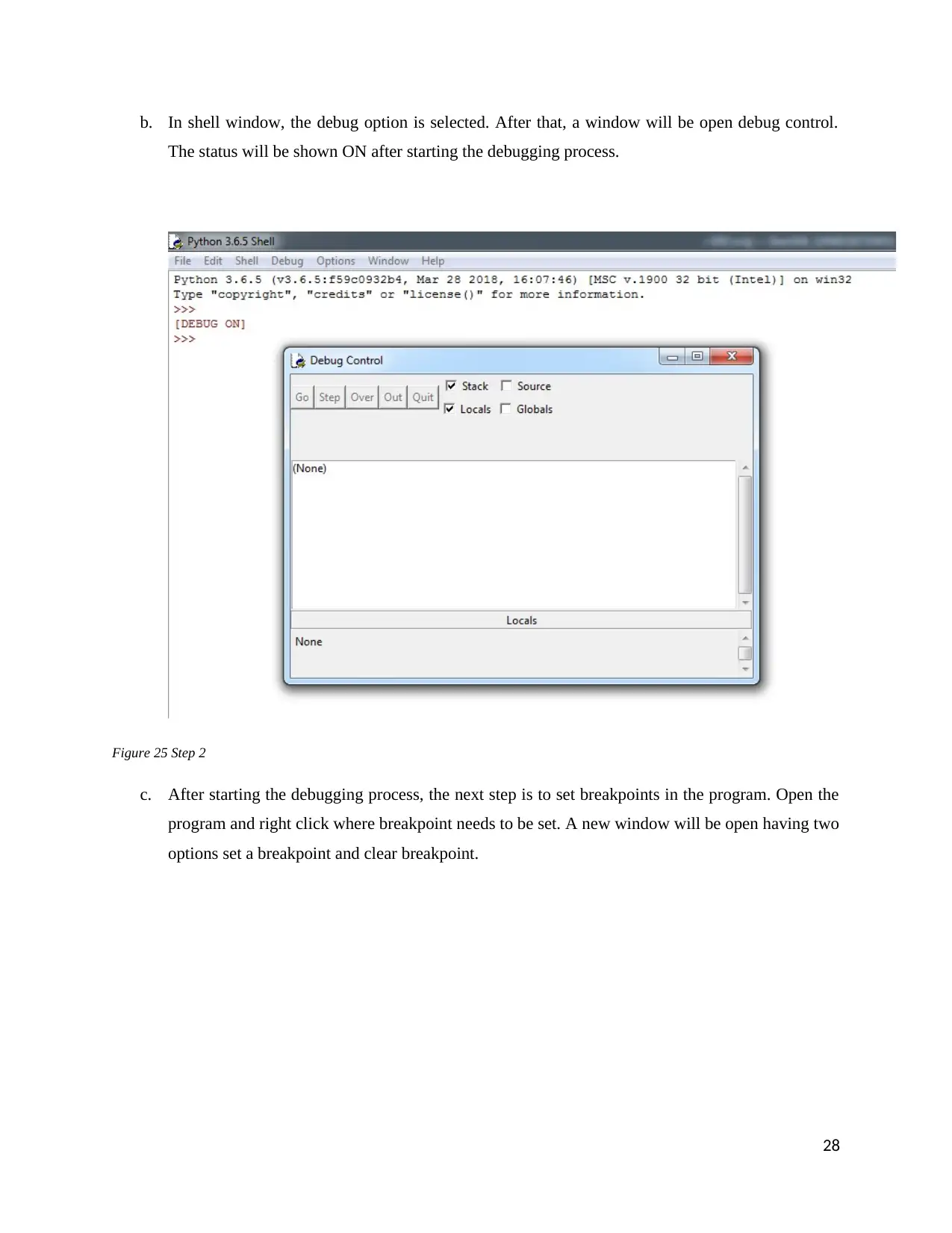
b. In shell window, the debug option is selected. After that, a window will be open debug control.
The status will be shown ON after starting the debugging process.
Figure 25 Step 2
c. After starting the debugging process, the next step is to set breakpoints in the program. Open the
program and right click where breakpoint needs to be set. A new window will be open having two
options set a breakpoint and clear breakpoint.
28
The status will be shown ON after starting the debugging process.
Figure 25 Step 2
c. After starting the debugging process, the next step is to set breakpoints in the program. Open the
program and right click where breakpoint needs to be set. A new window will be open having two
options set a breakpoint and clear breakpoint.
28
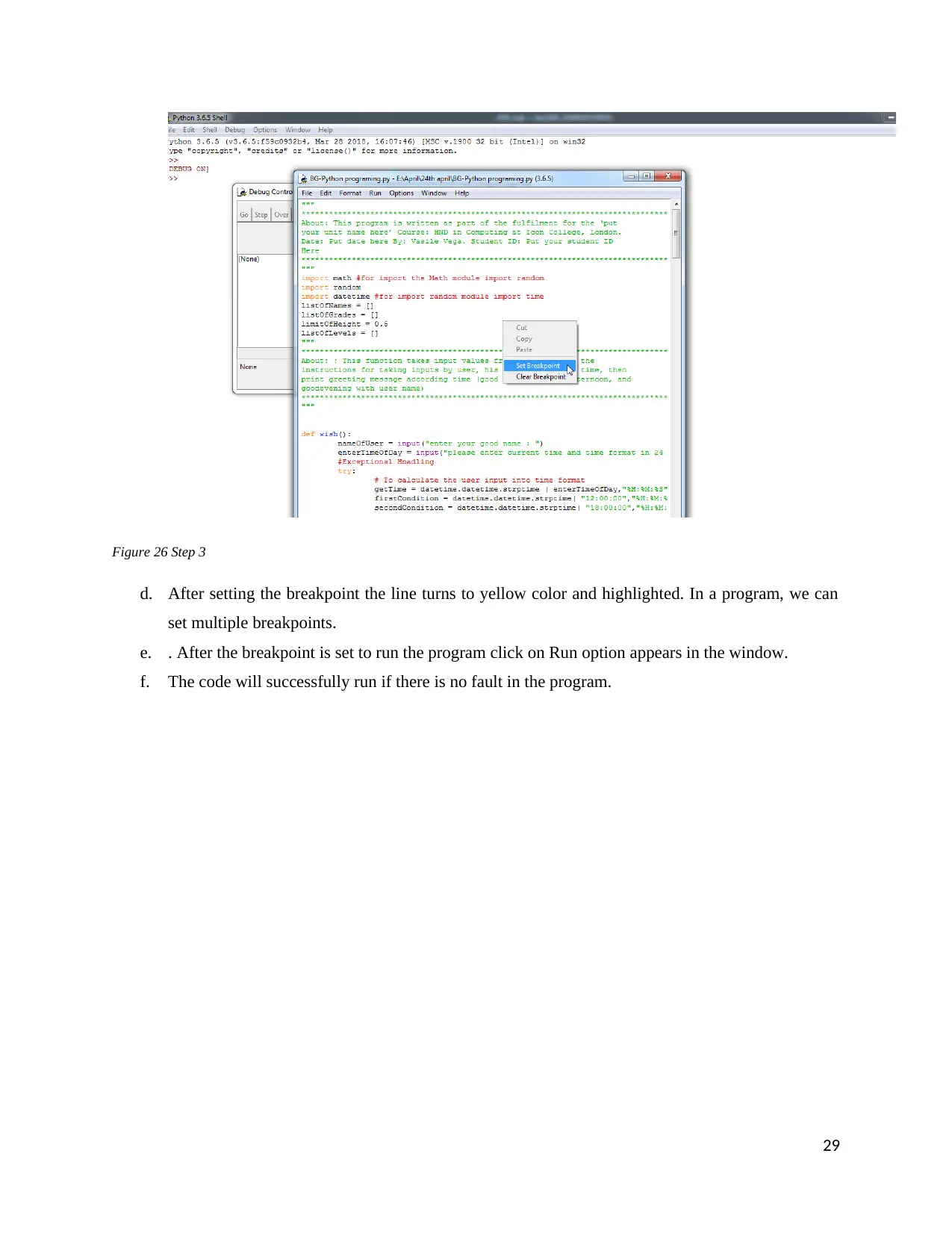
Figure 26 Step 3
d. After setting the breakpoint the line turns to yellow color and highlighted. In a program, we can
set multiple breakpoints.
e. . After the breakpoint is set to run the program click on Run option appears in the window.
f. The code will successfully run if there is no fault in the program.
29
d. After setting the breakpoint the line turns to yellow color and highlighted. In a program, we can
set multiple breakpoints.
e. . After the breakpoint is set to run the program click on Run option appears in the window.
f. The code will successfully run if there is no fault in the program.
29
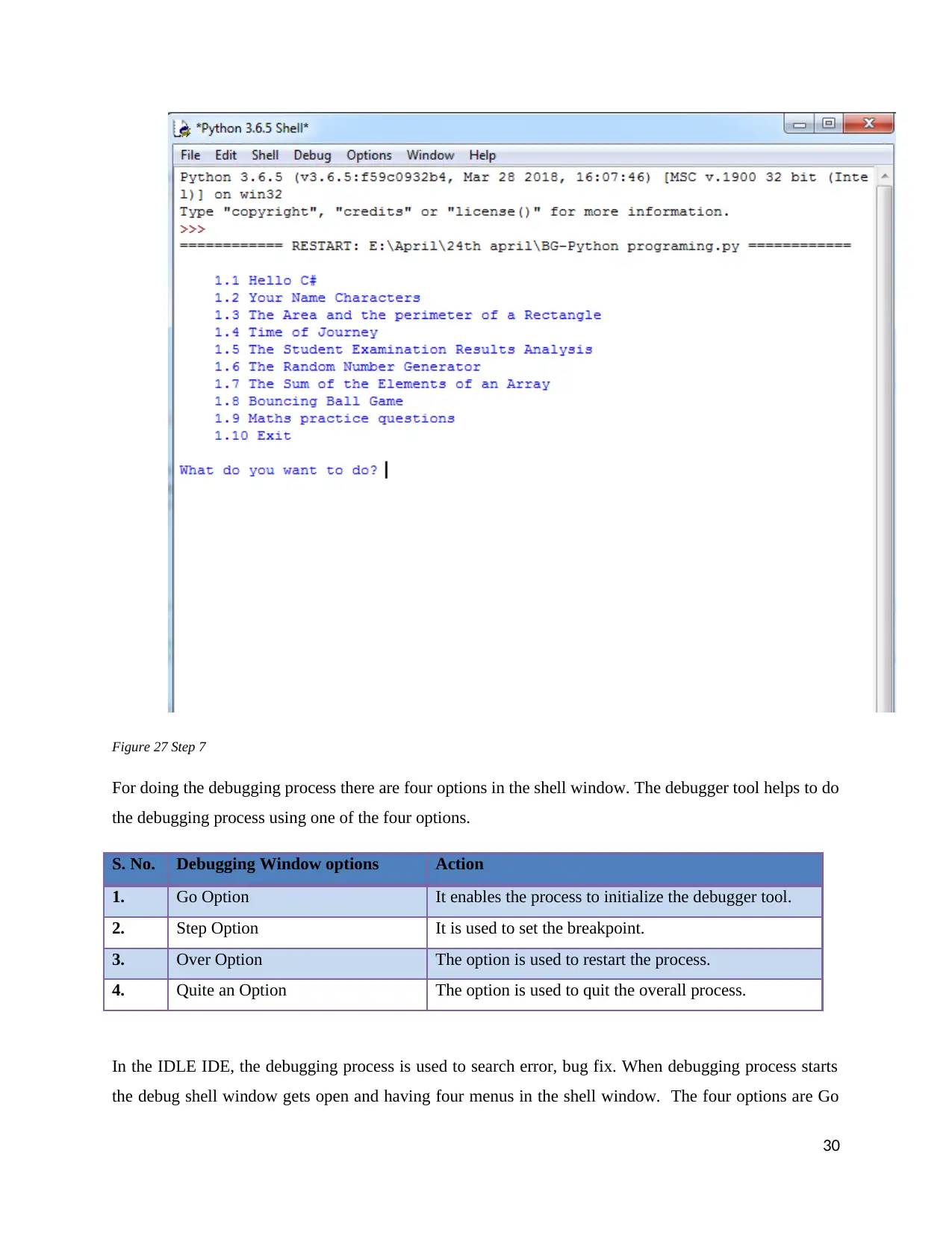
Figure 27 Step 7
For doing the debugging process there are four options in the shell window. The debugger tool helps to do
the debugging process using one of the four options.
S. No. Debugging Window options Action
1. Go Option It enables the process to initialize the debugger tool.
2. Step Option It is used to set the breakpoint.
3. Over Option The option is used to restart the process.
4. Quite an Option The option is used to quit the overall process.
In the IDLE IDE, the debugging process is used to search error, bug fix. When debugging process starts
the debug shell window gets open and having four menus in the shell window. The four options are Go
30
For doing the debugging process there are four options in the shell window. The debugger tool helps to do
the debugging process using one of the four options.
S. No. Debugging Window options Action
1. Go Option It enables the process to initialize the debugger tool.
2. Step Option It is used to set the breakpoint.
3. Over Option The option is used to restart the process.
4. Quite an Option The option is used to quit the overall process.
In the IDLE IDE, the debugging process is used to search error, bug fix. When debugging process starts
the debug shell window gets open and having four menus in the shell window. The four options are Go
30
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
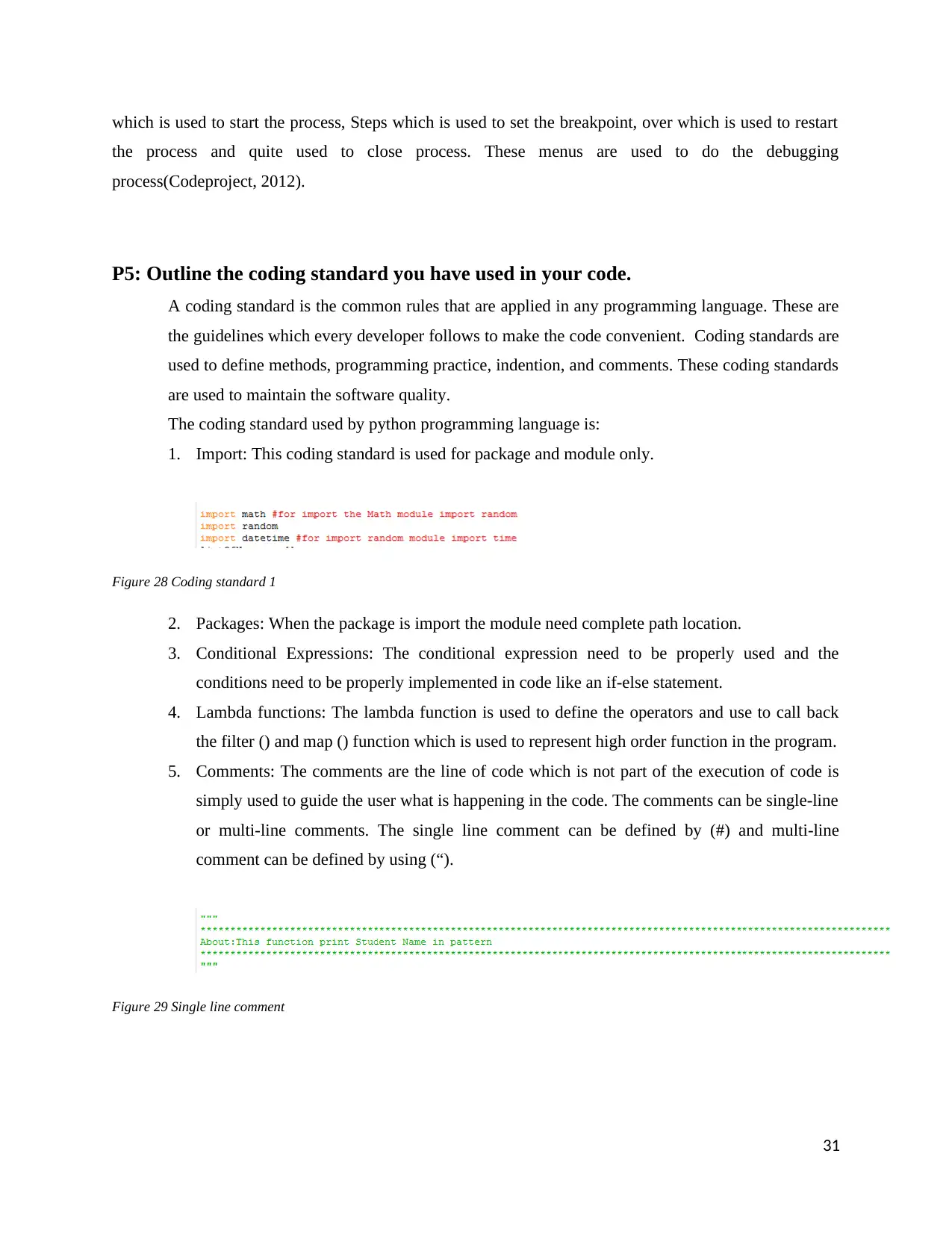
which is used to start the process, Steps which is used to set the breakpoint, over which is used to restart
the process and quite used to close process. These menus are used to do the debugging
process(Codeproject, 2012).
P5: Outline the coding standard you have used in your code.
A coding standard is the common rules that are applied in any programming language. These are
the guidelines which every developer follows to make the code convenient. Coding standards are
used to define methods, programming practice, indention, and comments. These coding standards
are used to maintain the software quality.
The coding standard used by python programming language is:
1. Import: This coding standard is used for package and module only.
Figure 28 Coding standard 1
2. Packages: When the package is import the module need complete path location.
3. Conditional Expressions: The conditional expression need to be properly used and the
conditions need to be properly implemented in code like an if-else statement.
4. Lambda functions: The lambda function is used to define the operators and use to call back
the filter () and map () function which is used to represent high order function in the program.
5. Comments: The comments are the line of code which is not part of the execution of code is
simply used to guide the user what is happening in the code. The comments can be single-line
or multi-line comments. The single line comment can be defined by (#) and multi-line
comment can be defined by using (“).
Figure 29 Single line comment
31
the process and quite used to close process. These menus are used to do the debugging
process(Codeproject, 2012).
P5: Outline the coding standard you have used in your code.
A coding standard is the common rules that are applied in any programming language. These are
the guidelines which every developer follows to make the code convenient. Coding standards are
used to define methods, programming practice, indention, and comments. These coding standards
are used to maintain the software quality.
The coding standard used by python programming language is:
1. Import: This coding standard is used for package and module only.
Figure 28 Coding standard 1
2. Packages: When the package is import the module need complete path location.
3. Conditional Expressions: The conditional expression need to be properly used and the
conditions need to be properly implemented in code like an if-else statement.
4. Lambda functions: The lambda function is used to define the operators and use to call back
the filter () and map () function which is used to represent high order function in the program.
5. Comments: The comments are the line of code which is not part of the execution of code is
simply used to guide the user what is happening in the code. The comments can be single-line
or multi-line comments. The single line comment can be defined by (#) and multi-line
comment can be defined by using (“).
Figure 29 Single line comment
31
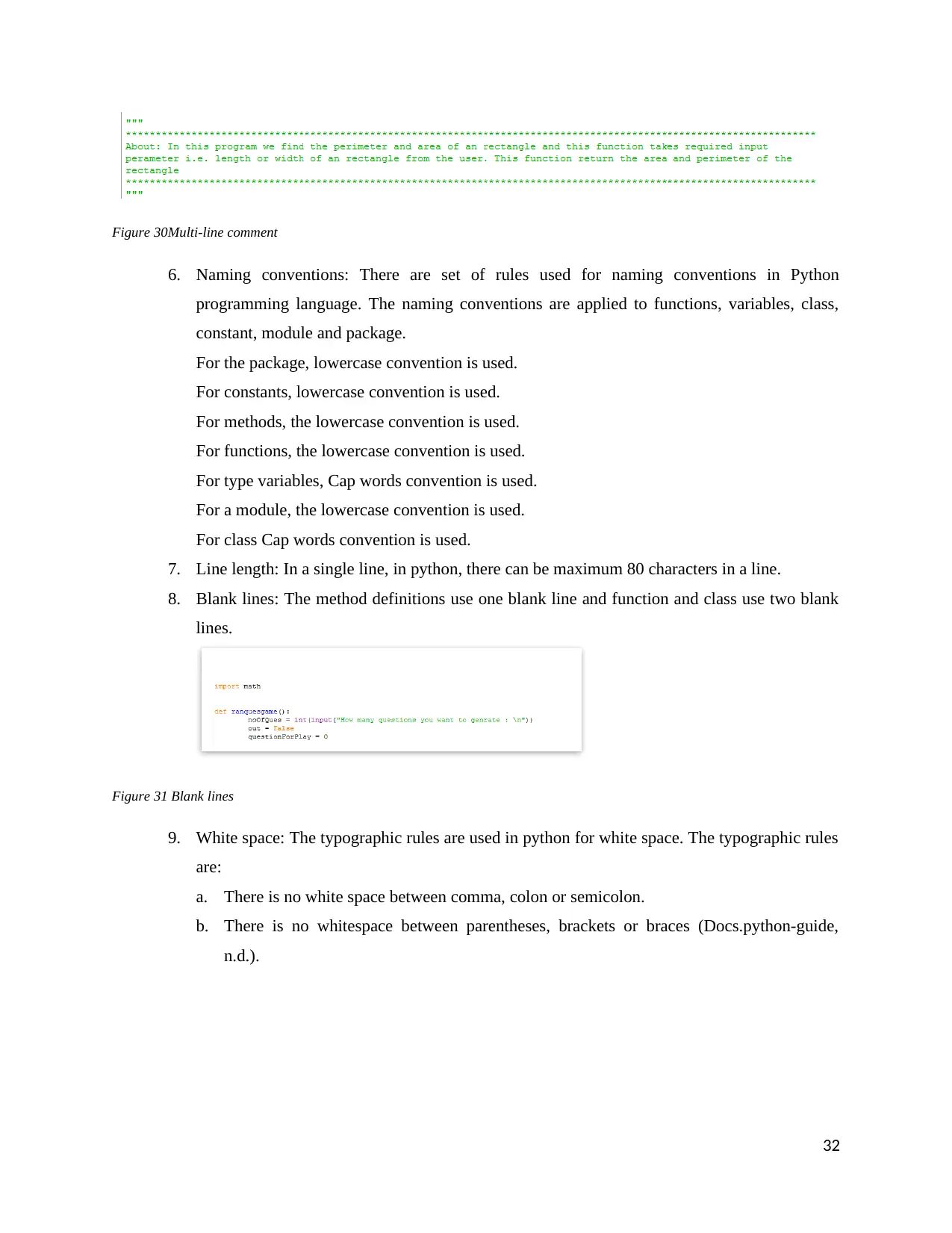
Figure 30Multi-line comment
6. Naming conventions: There are set of rules used for naming conventions in Python
programming language. The naming conventions are applied to functions, variables, class,
constant, module and package.
For the package, lowercase convention is used.
For constants, lowercase convention is used.
For methods, the lowercase convention is used.
For functions, the lowercase convention is used.
For type variables, Cap words convention is used.
For a module, the lowercase convention is used.
For class Cap words convention is used.
7. Line length: In a single line, in python, there can be maximum 80 characters in a line.
8. Blank lines: The method definitions use one blank line and function and class use two blank
lines.
Figure 31 Blank lines
9. White space: The typographic rules are used in python for white space. The typographic rules
are:
a. There is no white space between comma, colon or semicolon.
b. There is no whitespace between parentheses, brackets or braces (Docs.python-guide,
n.d.).
32
6. Naming conventions: There are set of rules used for naming conventions in Python
programming language. The naming conventions are applied to functions, variables, class,
constant, module and package.
For the package, lowercase convention is used.
For constants, lowercase convention is used.
For methods, the lowercase convention is used.
For functions, the lowercase convention is used.
For type variables, Cap words convention is used.
For a module, the lowercase convention is used.
For class Cap words convention is used.
7. Line length: In a single line, in python, there can be maximum 80 characters in a line.
8. Blank lines: The method definitions use one blank line and function and class use two blank
lines.
Figure 31 Blank lines
9. White space: The typographic rules are used in python for white space. The typographic rules
are:
a. There is no white space between comma, colon or semicolon.
b. There is no whitespace between parentheses, brackets or braces (Docs.python-guide,
n.d.).
32
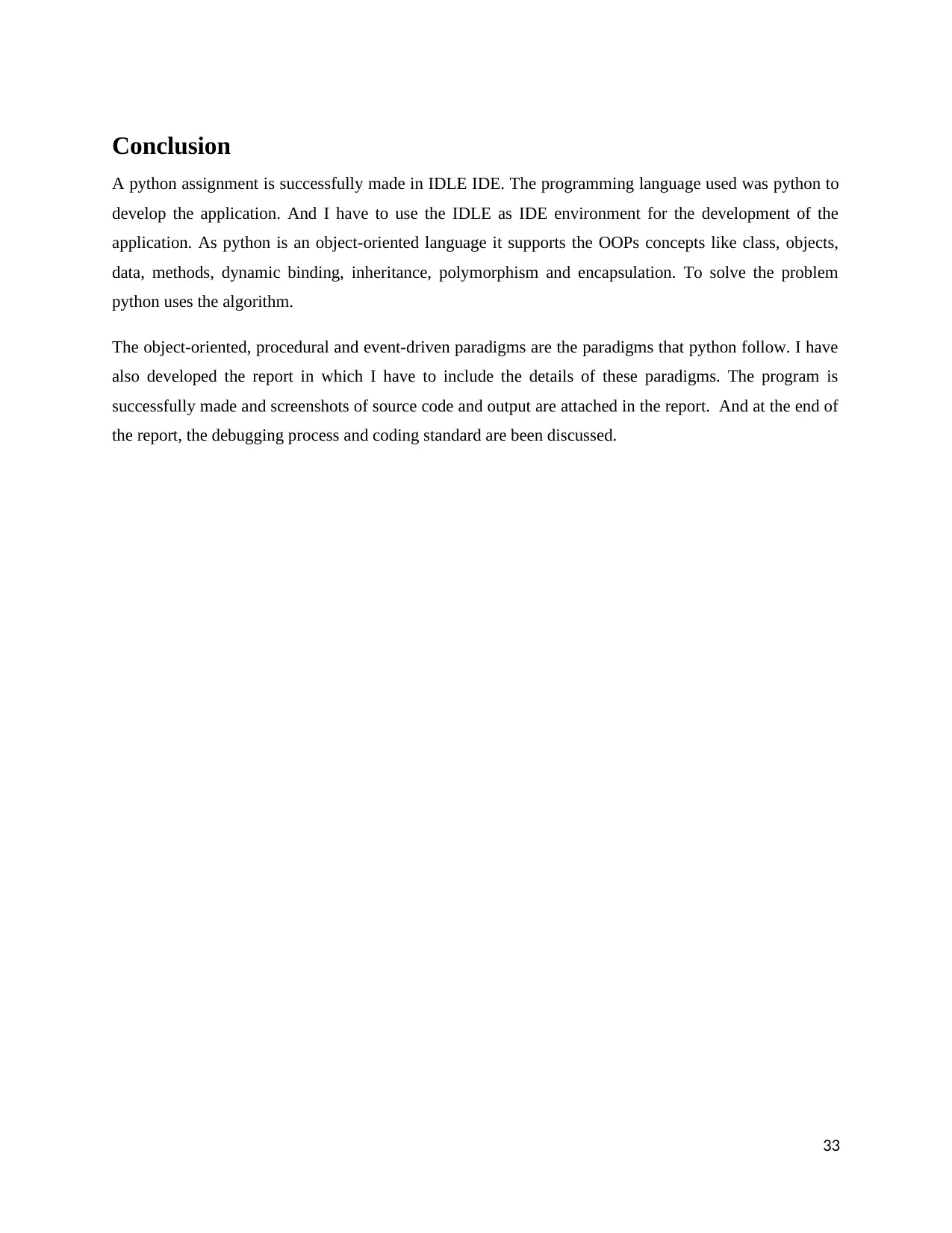
Conclusion
A python assignment is successfully made in IDLE IDE. The programming language used was python to
develop the application. And I have to use the IDLE as IDE environment for the development of the
application. As python is an object-oriented language it supports the OOPs concepts like class, objects,
data, methods, dynamic binding, inheritance, polymorphism and encapsulation. To solve the problem
python uses the algorithm.
The object-oriented, procedural and event-driven paradigms are the paradigms that python follow. I have
also developed the report in which I have to include the details of these paradigms. The program is
successfully made and screenshots of source code and output are attached in the report. And at the end of
the report, the debugging process and coding standard are been discussed.
33
A python assignment is successfully made in IDLE IDE. The programming language used was python to
develop the application. And I have to use the IDLE as IDE environment for the development of the
application. As python is an object-oriented language it supports the OOPs concepts like class, objects,
data, methods, dynamic binding, inheritance, polymorphism and encapsulation. To solve the problem
python uses the algorithm.
The object-oriented, procedural and event-driven paradigms are the paradigms that python follow. I have
also developed the report in which I have to include the details of these paradigms. The program is
successfully made and screenshots of source code and output are attached in the report. And at the end of
the report, the debugging process and coding standard are been discussed.
33
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
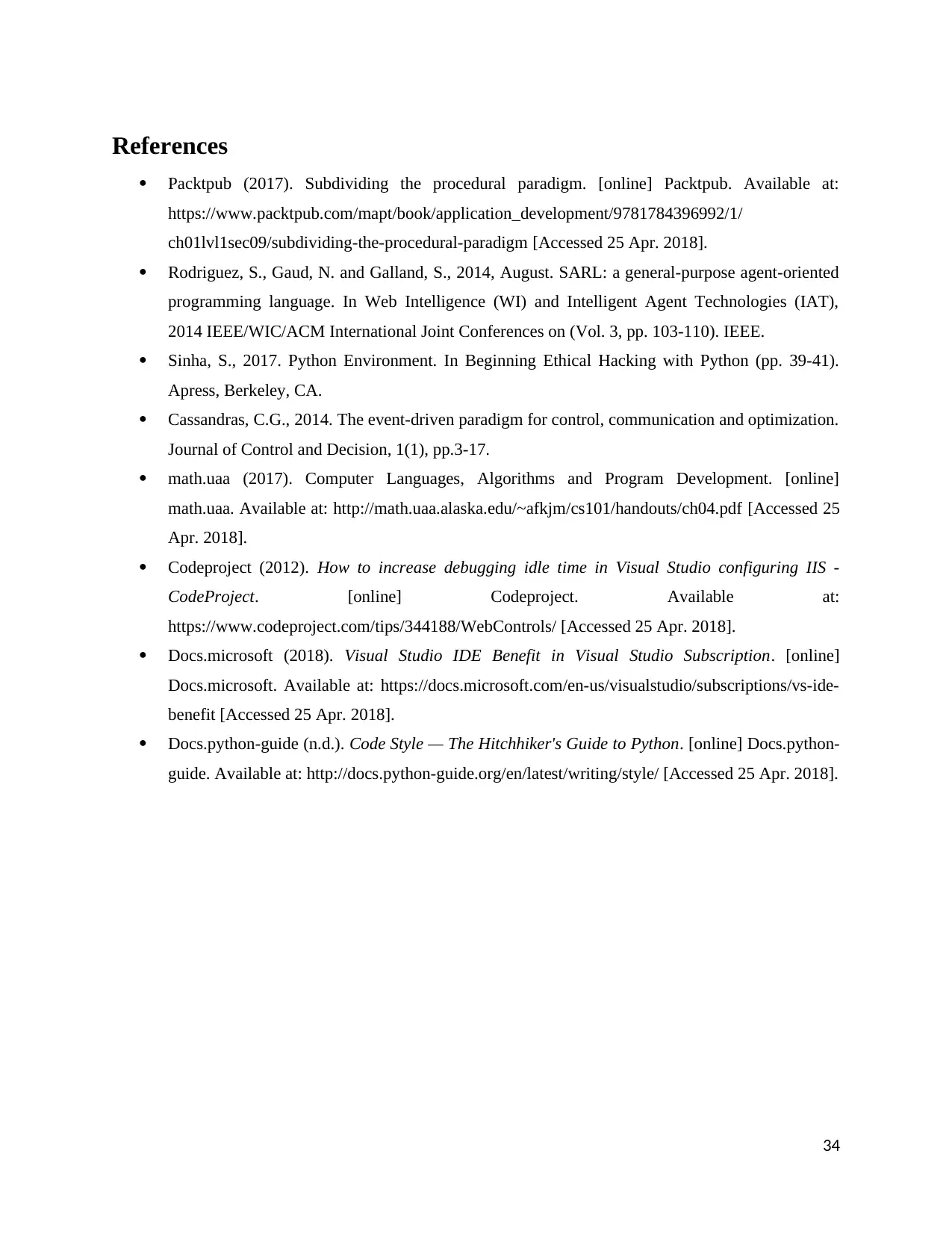
References
Packtpub (2017). Subdividing the procedural paradigm. [online] Packtpub. Available at:
https://www.packtpub.com/mapt/book/application_development/9781784396992/1/
ch01lvl1sec09/subdividing-the-procedural-paradigm [Accessed 25 Apr. 2018].
Rodriguez, S., Gaud, N. and Galland, S., 2014, August. SARL: a general-purpose agent-oriented
programming language. In Web Intelligence (WI) and Intelligent Agent Technologies (IAT),
2014 IEEE/WIC/ACM International Joint Conferences on (Vol. 3, pp. 103-110). IEEE.
Sinha, S., 2017. Python Environment. In Beginning Ethical Hacking with Python (pp. 39-41).
Apress, Berkeley, CA.
Cassandras, C.G., 2014. The event-driven paradigm for control, communication and optimization.
Journal of Control and Decision, 1(1), pp.3-17.
math.uaa (2017). Computer Languages, Algorithms and Program Development. [online]
math.uaa. Available at: http://math.uaa.alaska.edu/~afkjm/cs101/handouts/ch04.pdf [Accessed 25
Apr. 2018].
Codeproject (2012). How to increase debugging idle time in Visual Studio configuring IIS -
CodeProject. [online] Codeproject. Available at:
https://www.codeproject.com/tips/344188/WebControls/ [Accessed 25 Apr. 2018].
Docs.microsoft (2018). Visual Studio IDE Benefit in Visual Studio Subscription. [online]
Docs.microsoft. Available at: https://docs.microsoft.com/en-us/visualstudio/subscriptions/vs-ide-
benefit [Accessed 25 Apr. 2018].
Docs.python-guide (n.d.). Code Style — The Hitchhiker's Guide to Python. [online] Docs.python-
guide. Available at: http://docs.python-guide.org/en/latest/writing/style/ [Accessed 25 Apr. 2018].
34
Packtpub (2017). Subdividing the procedural paradigm. [online] Packtpub. Available at:
https://www.packtpub.com/mapt/book/application_development/9781784396992/1/
ch01lvl1sec09/subdividing-the-procedural-paradigm [Accessed 25 Apr. 2018].
Rodriguez, S., Gaud, N. and Galland, S., 2014, August. SARL: a general-purpose agent-oriented
programming language. In Web Intelligence (WI) and Intelligent Agent Technologies (IAT),
2014 IEEE/WIC/ACM International Joint Conferences on (Vol. 3, pp. 103-110). IEEE.
Sinha, S., 2017. Python Environment. In Beginning Ethical Hacking with Python (pp. 39-41).
Apress, Berkeley, CA.
Cassandras, C.G., 2014. The event-driven paradigm for control, communication and optimization.
Journal of Control and Decision, 1(1), pp.3-17.
math.uaa (2017). Computer Languages, Algorithms and Program Development. [online]
math.uaa. Available at: http://math.uaa.alaska.edu/~afkjm/cs101/handouts/ch04.pdf [Accessed 25
Apr. 2018].
Codeproject (2012). How to increase debugging idle time in Visual Studio configuring IIS -
CodeProject. [online] Codeproject. Available at:
https://www.codeproject.com/tips/344188/WebControls/ [Accessed 25 Apr. 2018].
Docs.microsoft (2018). Visual Studio IDE Benefit in Visual Studio Subscription. [online]
Docs.microsoft. Available at: https://docs.microsoft.com/en-us/visualstudio/subscriptions/vs-ide-
benefit [Accessed 25 Apr. 2018].
Docs.python-guide (n.d.). Code Style — The Hitchhiker's Guide to Python. [online] Docs.python-
guide. Available at: http://docs.python-guide.org/en/latest/writing/style/ [Accessed 25 Apr. 2018].
34
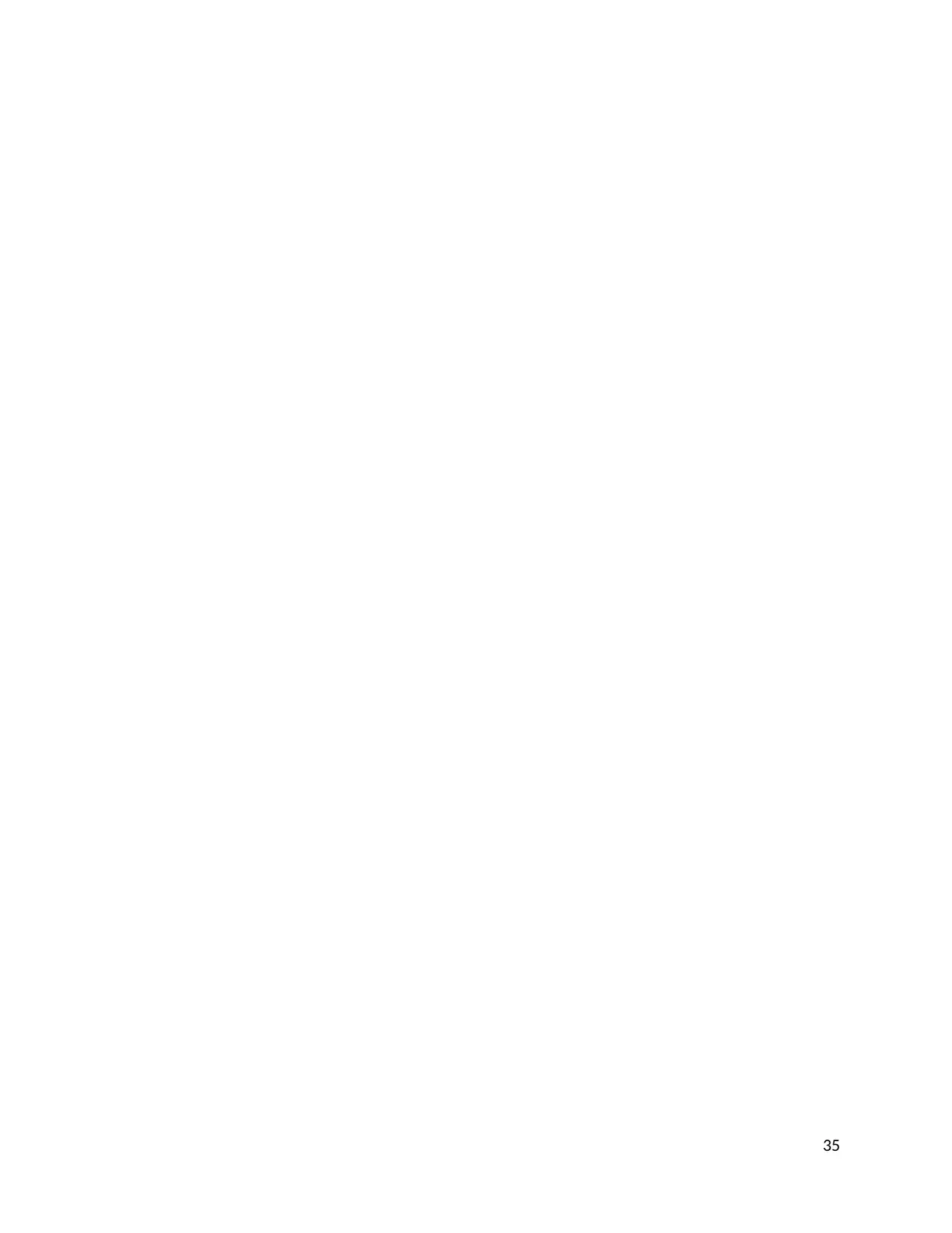
35
1 out of 36
Related Documents
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.