Design and Testing of Bank Account and Loan Account Classes in Java
VerifiedAdded on  2023/01/17
|8
|1340
|24
AI Summary
This document provides a detailed explanation of the design and testing of Bank Account and Loan Account classes in Java. It includes the code for the classes, along with explanations of the methods and their functionalities. The document also includes screenshots of the code and references for further reading.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
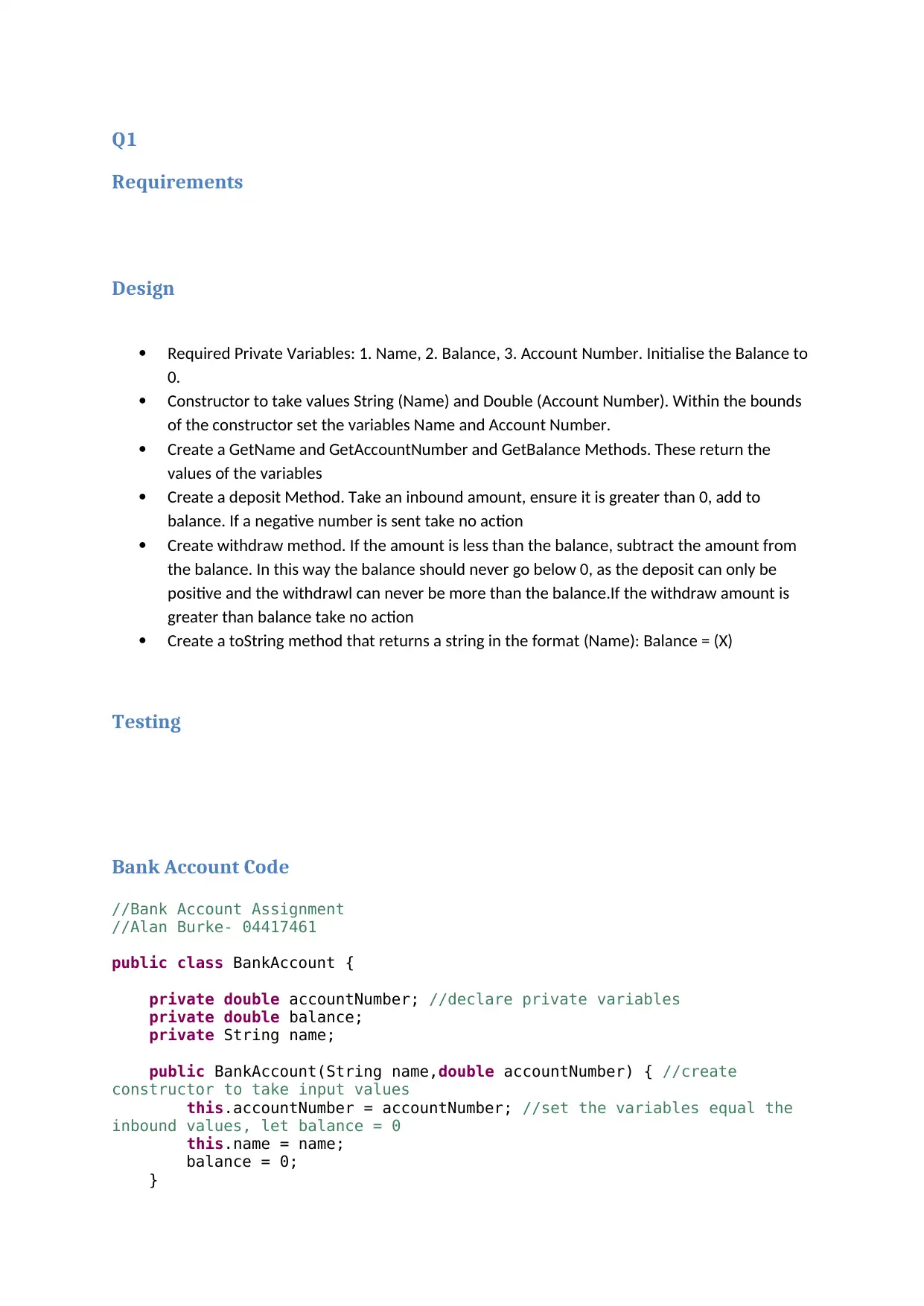
Q1
Requirements
Design
ï‚· Required Private Variables: 1. Name, 2. Balance, 3. Account Number. Initialise the Balance to
0.
ï‚· Constructor to take values String (Name) and Double (Account Number). Within the bounds
of the constructor set the variables Name and Account Number.
ï‚· Create a GetName and GetAccountNumber and GetBalance Methods. These return the
values of the variables
ï‚· Create a deposit Method. Take an inbound amount, ensure it is greater than 0, add to
balance. If a negative number is sent take no action
ï‚· Create withdraw method. If the amount is less than the balance, subtract the amount from
the balance. In this way the balance should never go below 0, as the deposit can only be
positive and the withdrawl can never be more than the balance.If the withdraw amount is
greater than balance take no action
ï‚· Create a toString method that returns a string in the format (Name): Balance = (X)
Testing
Bank Account Code
//Bank Account Assignment
//Alan Burke- 04417461
public class BankAccount {
private double accountNumber; //declare private variables
private double balance;
private String name;
public BankAccount(String name,double accountNumber) { //create
constructor to take input values
this.accountNumber = accountNumber; //set the variables equal the
inbound values, let balance = 0
this.name = name;
balance = 0;
}
Requirements
Design
ï‚· Required Private Variables: 1. Name, 2. Balance, 3. Account Number. Initialise the Balance to
0.
ï‚· Constructor to take values String (Name) and Double (Account Number). Within the bounds
of the constructor set the variables Name and Account Number.
ï‚· Create a GetName and GetAccountNumber and GetBalance Methods. These return the
values of the variables
ï‚· Create a deposit Method. Take an inbound amount, ensure it is greater than 0, add to
balance. If a negative number is sent take no action
ï‚· Create withdraw method. If the amount is less than the balance, subtract the amount from
the balance. In this way the balance should never go below 0, as the deposit can only be
positive and the withdrawl can never be more than the balance.If the withdraw amount is
greater than balance take no action
ï‚· Create a toString method that returns a string in the format (Name): Balance = (X)
Testing
Bank Account Code
//Bank Account Assignment
//Alan Burke- 04417461
public class BankAccount {
private double accountNumber; //declare private variables
private double balance;
private String name;
public BankAccount(String name,double accountNumber) { //create
constructor to take input values
this.accountNumber = accountNumber; //set the variables equal the
inbound values, let balance = 0
this.name = name;
balance = 0;
}
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
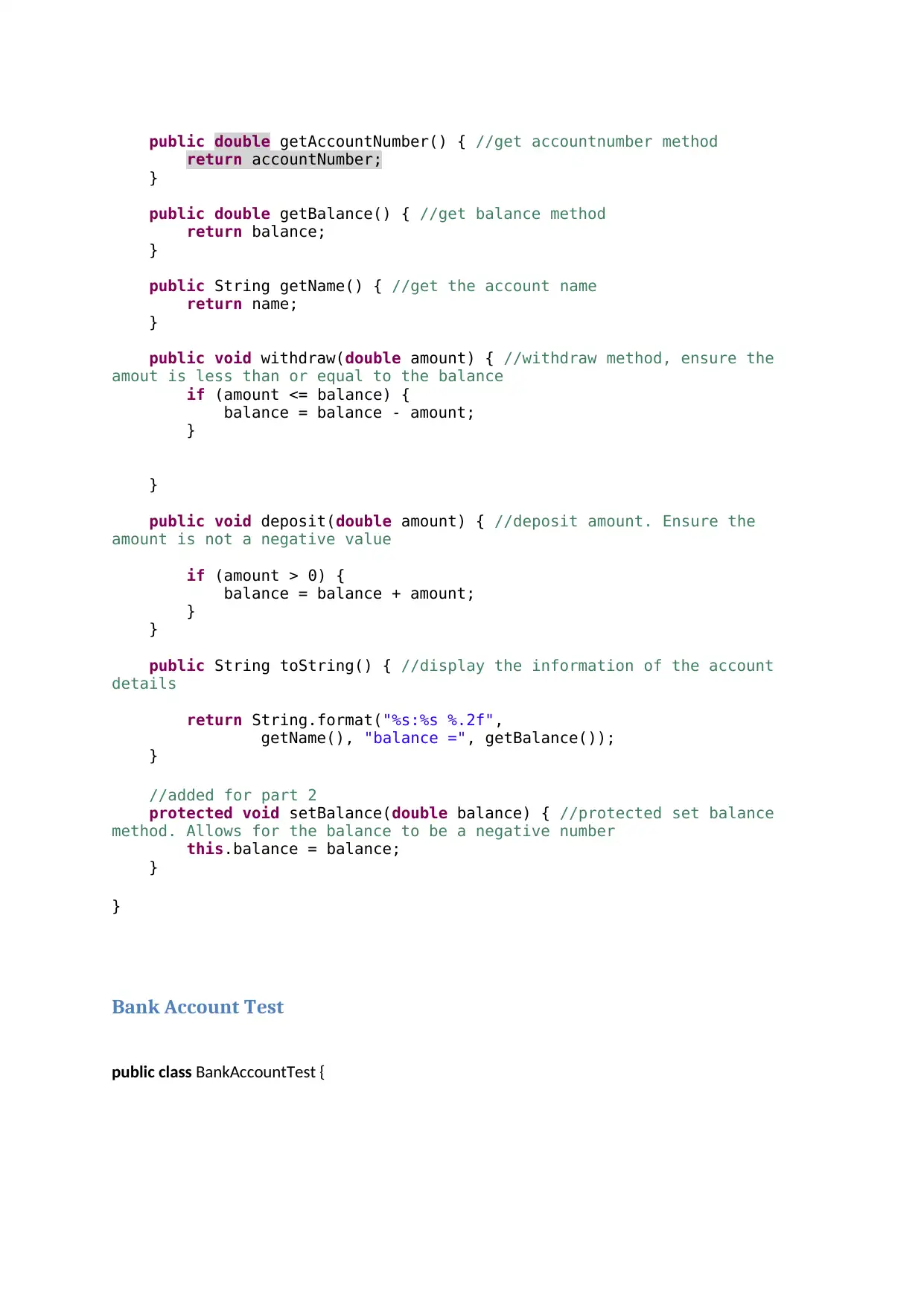
public double getAccountNumber() { //get accountnumber method
return accountNumber;
}
public double getBalance() { //get balance method
return balance;
}
public String getName() { //get the account name
return name;
}
public void withdraw(double amount) { //withdraw method, ensure the
amout is less than or equal to the balance
if (amount <= balance) {
balance = balance - amount;
}
}
public void deposit(double amount) { //deposit amount. Ensure the
amount is not a negative value
if (amount > 0) {
balance = balance + amount;
}
}
public String toString() { //display the information of the account
details
return String.format("%s:%s %.2f",
getName(), "balance =", getBalance());
}
//added for part 2
protected void setBalance(double balance) { //protected set balance
method. Allows for the balance to be a negative number
this.balance = balance;
}
}
Bank Account Test
public class BankAccountTest {
return accountNumber;
}
public double getBalance() { //get balance method
return balance;
}
public String getName() { //get the account name
return name;
}
public void withdraw(double amount) { //withdraw method, ensure the
amout is less than or equal to the balance
if (amount <= balance) {
balance = balance - amount;
}
}
public void deposit(double amount) { //deposit amount. Ensure the
amount is not a negative value
if (amount > 0) {
balance = balance + amount;
}
}
public String toString() { //display the information of the account
details
return String.format("%s:%s %.2f",
getName(), "balance =", getBalance());
}
//added for part 2
protected void setBalance(double balance) { //protected set balance
method. Allows for the balance to be a negative number
this.balance = balance;
}
}
Bank Account Test
public class BankAccountTest {
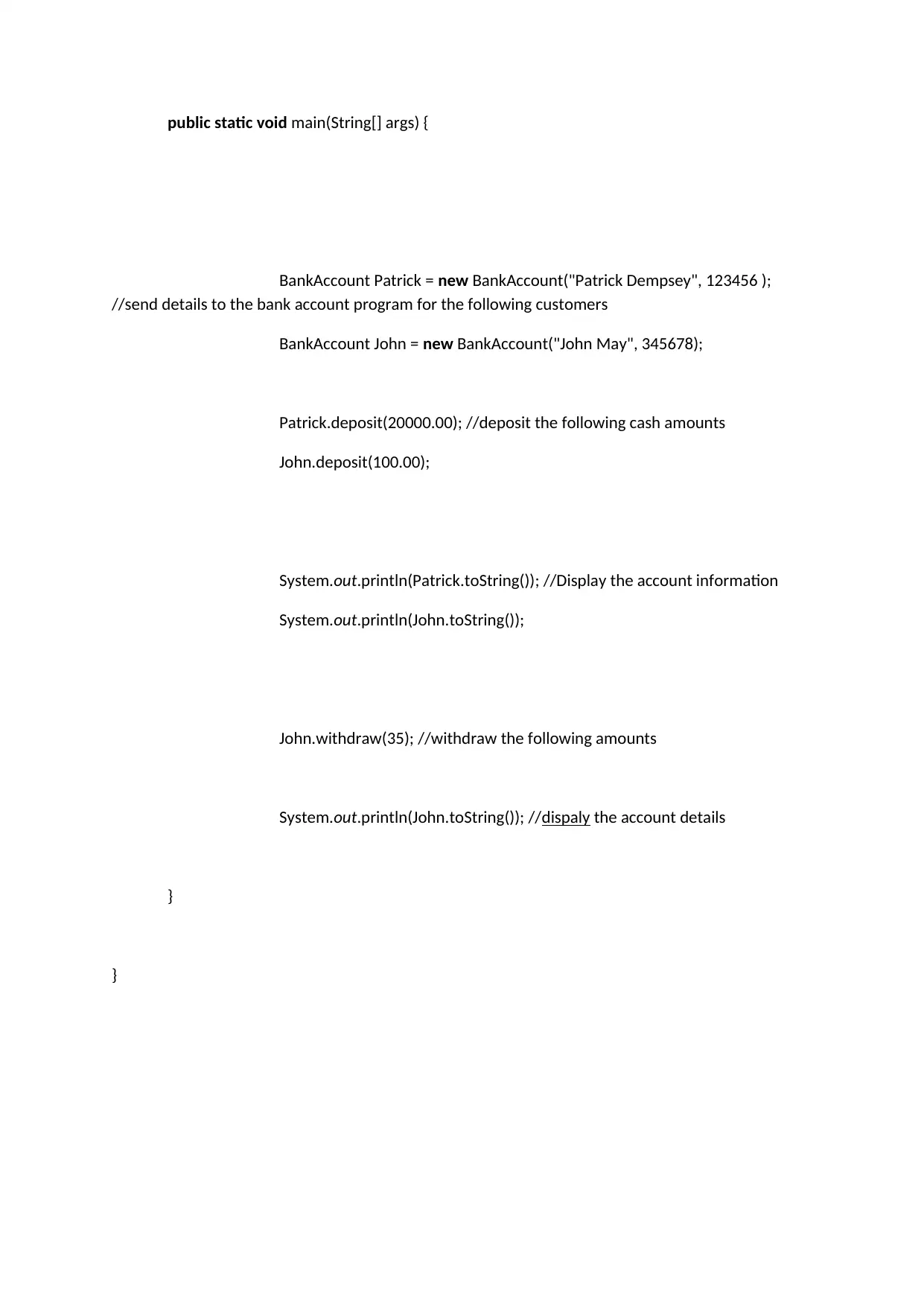
public static void main(String[] args) {
BankAccount Patrick = new BankAccount("Patrick Dempsey", 123456 );
//send details to the bank account program for the following customers
BankAccount John = new BankAccount("John May", 345678);
Patrick.deposit(20000.00); //deposit the following cash amounts
John.deposit(100.00);
System.out.println(Patrick.toString()); //Display the account information
System.out.println(John.toString());
John.withdraw(35); //withdraw the following amounts
System.out.println(John.toString()); //dispaly the account details
}
}
BankAccount Patrick = new BankAccount("Patrick Dempsey", 123456 );
//send details to the bank account program for the following customers
BankAccount John = new BankAccount("John May", 345678);
Patrick.deposit(20000.00); //deposit the following cash amounts
John.deposit(100.00);
System.out.println(Patrick.toString()); //Display the account information
System.out.println(John.toString());
John.withdraw(35); //withdraw the following amounts
System.out.println(John.toString()); //dispaly the account details
}
}
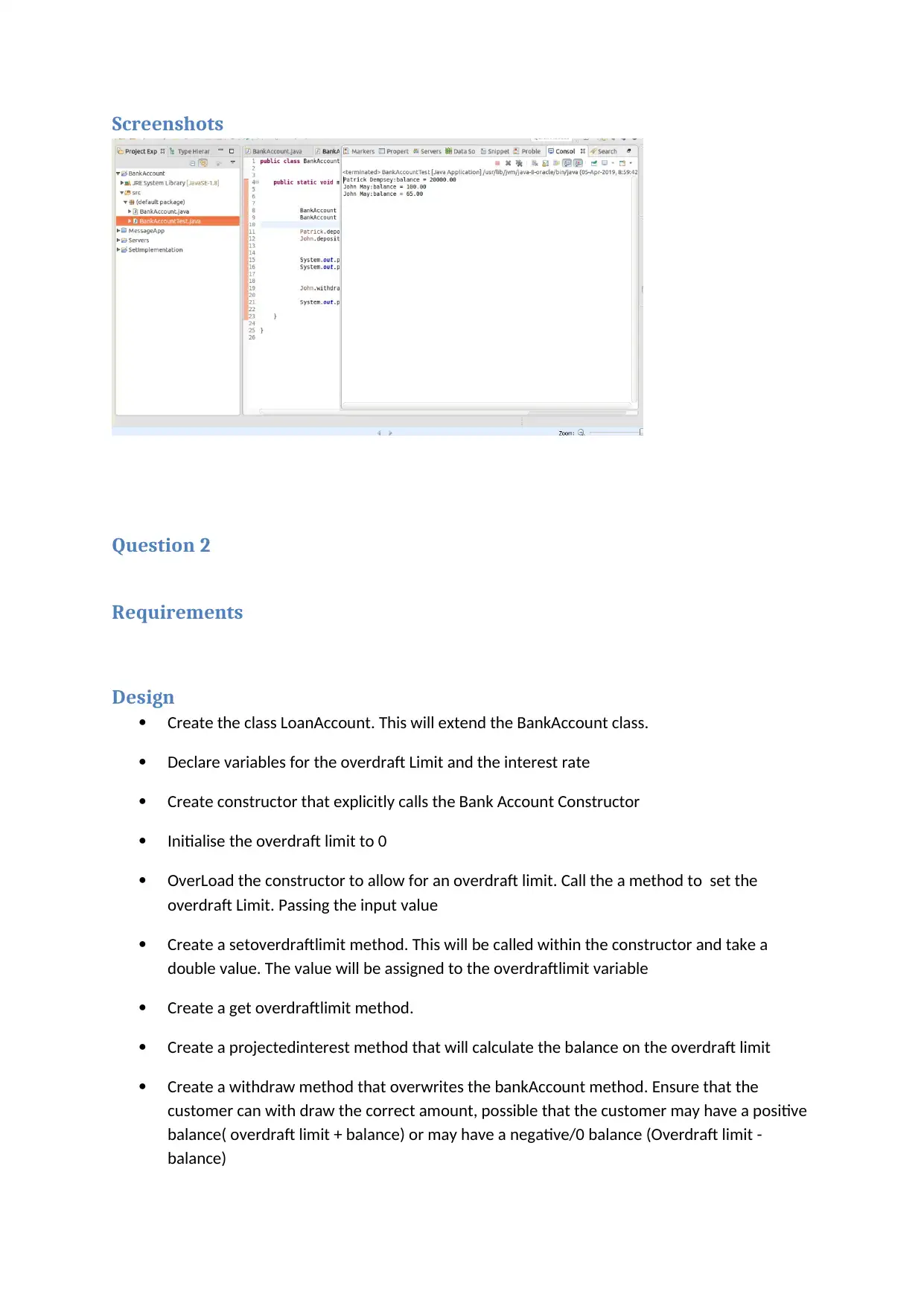
Screenshots
Question 2
Requirements
Design
ï‚· Create the class LoanAccount. This will extend the BankAccount class.
ï‚· Declare variables for the overdraft Limit and the interest rate
ï‚· Create constructor that explicitly calls the Bank Account Constructor
ï‚· Initialise the overdraft limit to 0
ï‚· OverLoad the constructor to allow for an overdraft limit. Call the a method to set the
overdraft Limit. Passing the input value
ï‚· Create a setoverdraftlimit method. This will be called within the constructor and take a
double value. The value will be assigned to the overdraftlimit variable
ï‚· Create a get overdraftlimit method.
ï‚· Create a projectedinterest method that will calculate the balance on the overdraft limit
ï‚· Create a withdraw method that overwrites the bankAccount method. Ensure that the
customer can with draw the correct amount, possible that the customer may have a positive
balance( overdraft limit + balance) or may have a negative/0 balance (Overdraft limit -
balance)
Question 2
Requirements
Design
ï‚· Create the class LoanAccount. This will extend the BankAccount class.
ï‚· Declare variables for the overdraft Limit and the interest rate
ï‚· Create constructor that explicitly calls the Bank Account Constructor
ï‚· Initialise the overdraft limit to 0
ï‚· OverLoad the constructor to allow for an overdraft limit. Call the a method to set the
overdraft Limit. Passing the input value
ï‚· Create a setoverdraftlimit method. This will be called within the constructor and take a
double value. The value will be assigned to the overdraftlimit variable
ï‚· Create a get overdraftlimit method.
ï‚· Create a projectedinterest method that will calculate the balance on the overdraft limit
ï‚· Create a withdraw method that overwrites the bankAccount method. Ensure that the
customer can with draw the correct amount, possible that the customer may have a positive
balance( overdraft limit + balance) or may have a negative/0 balance (Overdraft limit -
balance)
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
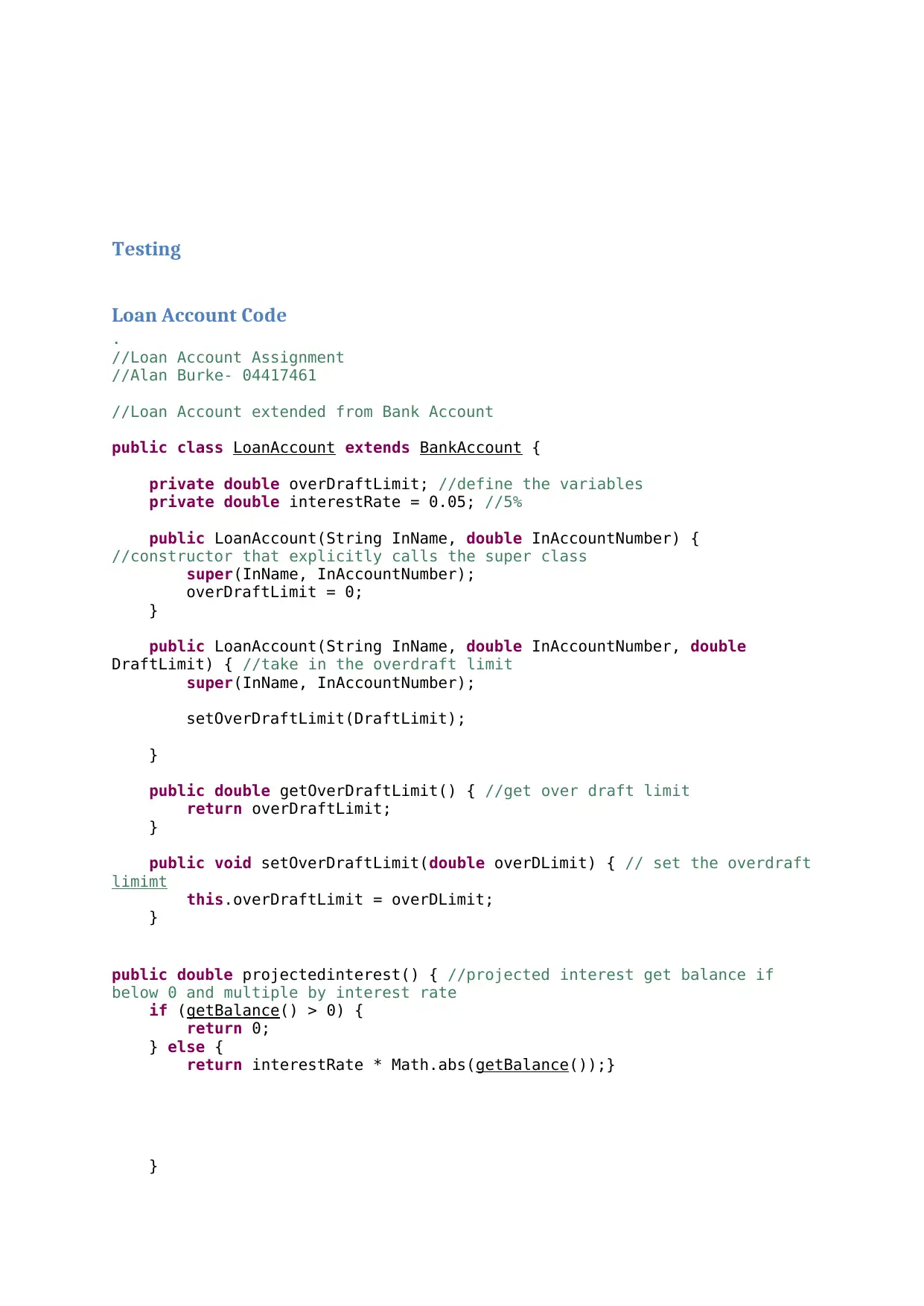
Testing
Loan Account Code
.
//Loan Account Assignment
//Alan Burke- 04417461
//Loan Account extended from Bank Account
public class LoanAccount extends BankAccount {
private double overDraftLimit; //define the variables
private double interestRate = 0.05; //5%
public LoanAccount(String InName, double InAccountNumber) {
//constructor that explicitly calls the super class
super(InName, InAccountNumber);
overDraftLimit = 0;
}
public LoanAccount(String InName, double InAccountNumber, double
DraftLimit) { //take in the overdraft limit
super(InName, InAccountNumber);
setOverDraftLimit(DraftLimit);
}
public double getOverDraftLimit() { //get over draft limit
return overDraftLimit;
}
public void setOverDraftLimit(double overDLimit) { // set the overdraft
limimt
this.overDraftLimit = overDLimit;
}
public double projectedinterest() { //projected interest get balance if
below 0 and multiple by interest rate
if (getBalance() > 0) {
return 0;
} else {
return interestRate * Math.abs(getBalance());}
}
Loan Account Code
.
//Loan Account Assignment
//Alan Burke- 04417461
//Loan Account extended from Bank Account
public class LoanAccount extends BankAccount {
private double overDraftLimit; //define the variables
private double interestRate = 0.05; //5%
public LoanAccount(String InName, double InAccountNumber) {
//constructor that explicitly calls the super class
super(InName, InAccountNumber);
overDraftLimit = 0;
}
public LoanAccount(String InName, double InAccountNumber, double
DraftLimit) { //take in the overdraft limit
super(InName, InAccountNumber);
setOverDraftLimit(DraftLimit);
}
public double getOverDraftLimit() { //get over draft limit
return overDraftLimit;
}
public void setOverDraftLimit(double overDLimit) { // set the overdraft
limimt
this.overDraftLimit = overDLimit;
}
public double projectedinterest() { //projected interest get balance if
below 0 and multiple by interest rate
if (getBalance() > 0) {
return 0;
} else {
return interestRate * Math.abs(getBalance());}
}
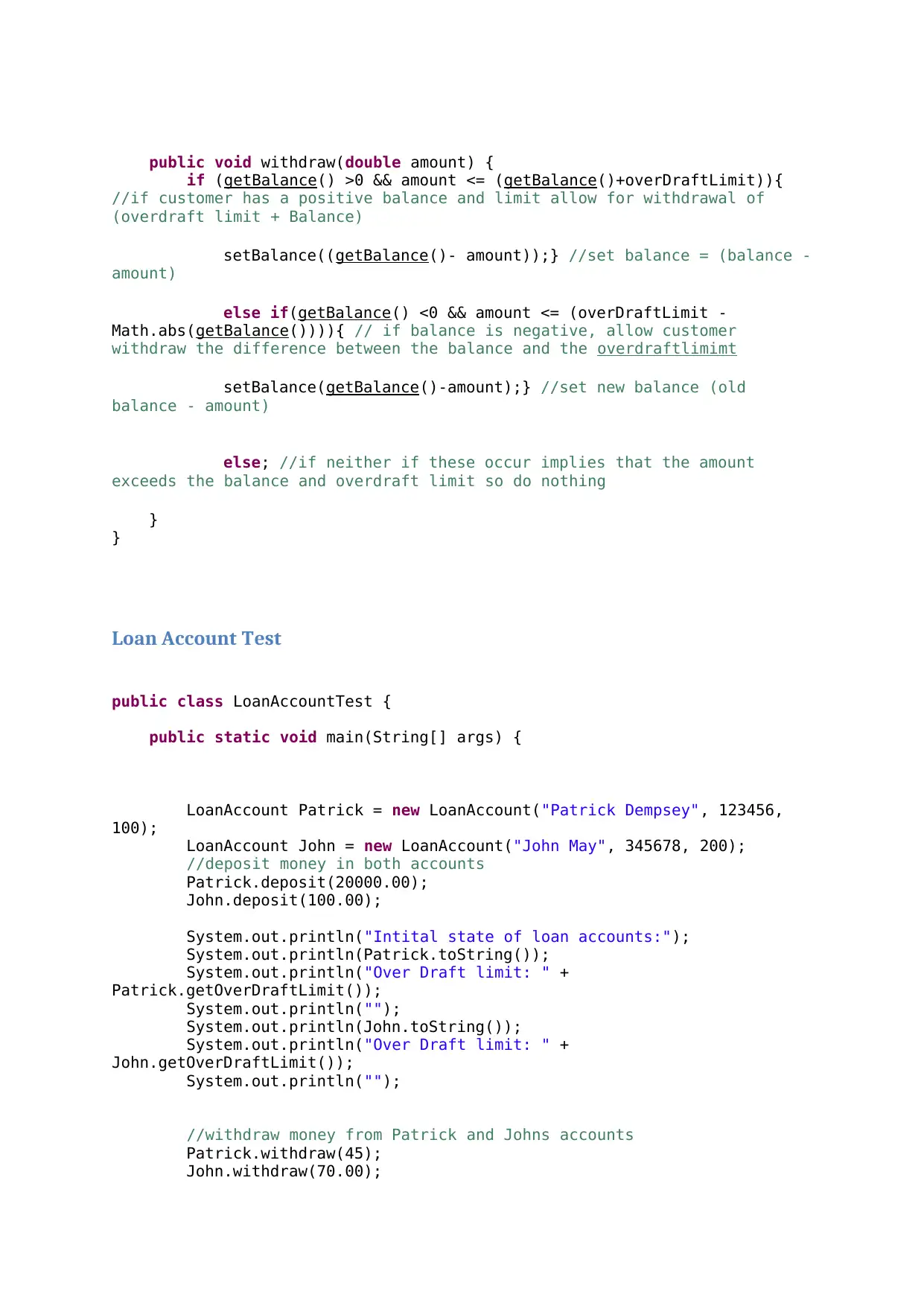
public void withdraw(double amount) {
if (getBalance() >0 && amount <= (getBalance()+overDraftLimit)){
//if customer has a positive balance and limit allow for withdrawal of
(overdraft limit + Balance)
setBalance((getBalance()- amount));} //set balance = (balance -
amount)
else if(getBalance() <0 && amount <= (overDraftLimit -
Math.abs(getBalance()))){ // if balance is negative, allow customer
withdraw the difference between the balance and the overdraftlimimt
setBalance(getBalance()-amount);} //set new balance (old
balance - amount)
else; //if neither if these occur implies that the amount
exceeds the balance and overdraft limit so do nothing
}
}
Loan Account Test
public class LoanAccountTest {
public static void main(String[] args) {
LoanAccount Patrick = new LoanAccount("Patrick Dempsey", 123456,
100);
LoanAccount John = new LoanAccount("John May", 345678, 200);
//deposit money in both accounts
Patrick.deposit(20000.00);
John.deposit(100.00);
System.out.println("Intital state of loan accounts:");
System.out.println(Patrick.toString());
System.out.println("Over Draft limit: " +
Patrick.getOverDraftLimit());
System.out.println("");
System.out.println(John.toString());
System.out.println("Over Draft limit: " +
John.getOverDraftLimit());
System.out.println("");
//withdraw money from Patrick and Johns accounts
Patrick.withdraw(45);
John.withdraw(70.00);
if (getBalance() >0 && amount <= (getBalance()+overDraftLimit)){
//if customer has a positive balance and limit allow for withdrawal of
(overdraft limit + Balance)
setBalance((getBalance()- amount));} //set balance = (balance -
amount)
else if(getBalance() <0 && amount <= (overDraftLimit -
Math.abs(getBalance()))){ // if balance is negative, allow customer
withdraw the difference between the balance and the overdraftlimimt
setBalance(getBalance()-amount);} //set new balance (old
balance - amount)
else; //if neither if these occur implies that the amount
exceeds the balance and overdraft limit so do nothing
}
}
Loan Account Test
public class LoanAccountTest {
public static void main(String[] args) {
LoanAccount Patrick = new LoanAccount("Patrick Dempsey", 123456,
100);
LoanAccount John = new LoanAccount("John May", 345678, 200);
//deposit money in both accounts
Patrick.deposit(20000.00);
John.deposit(100.00);
System.out.println("Intital state of loan accounts:");
System.out.println(Patrick.toString());
System.out.println("Over Draft limit: " +
Patrick.getOverDraftLimit());
System.out.println("");
System.out.println(John.toString());
System.out.println("Over Draft limit: " +
John.getOverDraftLimit());
System.out.println("");
//withdraw money from Patrick and Johns accounts
Patrick.withdraw(45);
John.withdraw(70.00);
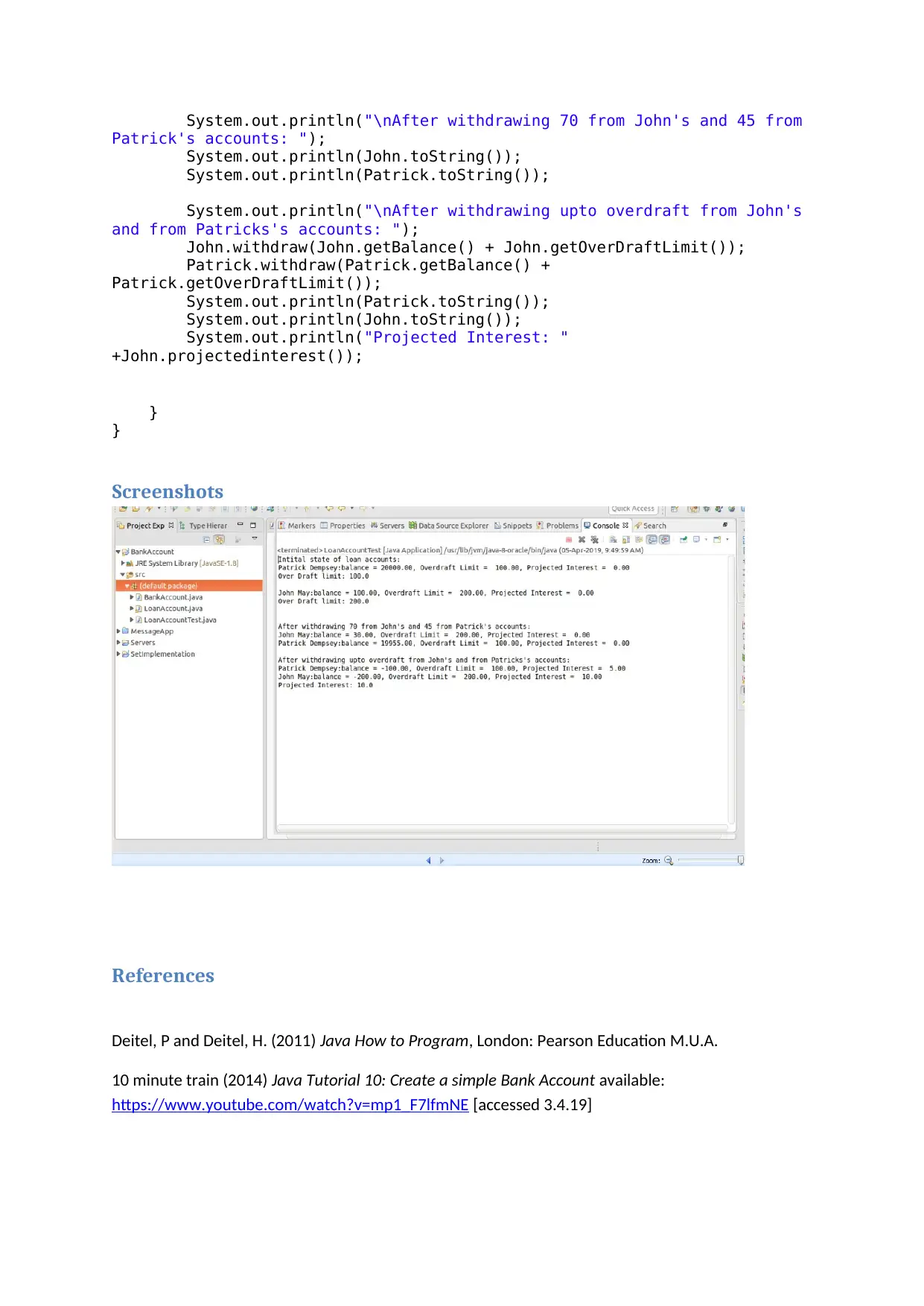
System.out.println("\nAfter withdrawing 70 from John's and 45 from
Patrick's accounts: ");
System.out.println(John.toString());
System.out.println(Patrick.toString());
System.out.println("\nAfter withdrawing upto overdraft from John's
and from Patricks's accounts: ");
John.withdraw(John.getBalance() + John.getOverDraftLimit());
Patrick.withdraw(Patrick.getBalance() +
Patrick.getOverDraftLimit());
System.out.println(Patrick.toString());
System.out.println(John.toString());
System.out.println("Projected Interest: "
+John.projectedinterest());
}
}
Screenshots
References
Deitel, P and Deitel, H. (2011) Java How to Program, London: Pearson Education M.U.A.
10 minute train (2014) Java Tutorial 10: Create a simple Bank Account available:
https://www.youtube.com/watch?v=mp1_F7lfmNE [accessed 3.4.19]
Patrick's accounts: ");
System.out.println(John.toString());
System.out.println(Patrick.toString());
System.out.println("\nAfter withdrawing upto overdraft from John's
and from Patricks's accounts: ");
John.withdraw(John.getBalance() + John.getOverDraftLimit());
Patrick.withdraw(Patrick.getBalance() +
Patrick.getOverDraftLimit());
System.out.println(Patrick.toString());
System.out.println(John.toString());
System.out.println("Projected Interest: "
+John.projectedinterest());
}
}
Screenshots
References
Deitel, P and Deitel, H. (2011) Java How to Program, London: Pearson Education M.U.A.
10 minute train (2014) Java Tutorial 10: Create a simple Bank Account available:
https://www.youtube.com/watch?v=mp1_F7lfmNE [accessed 3.4.19]
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
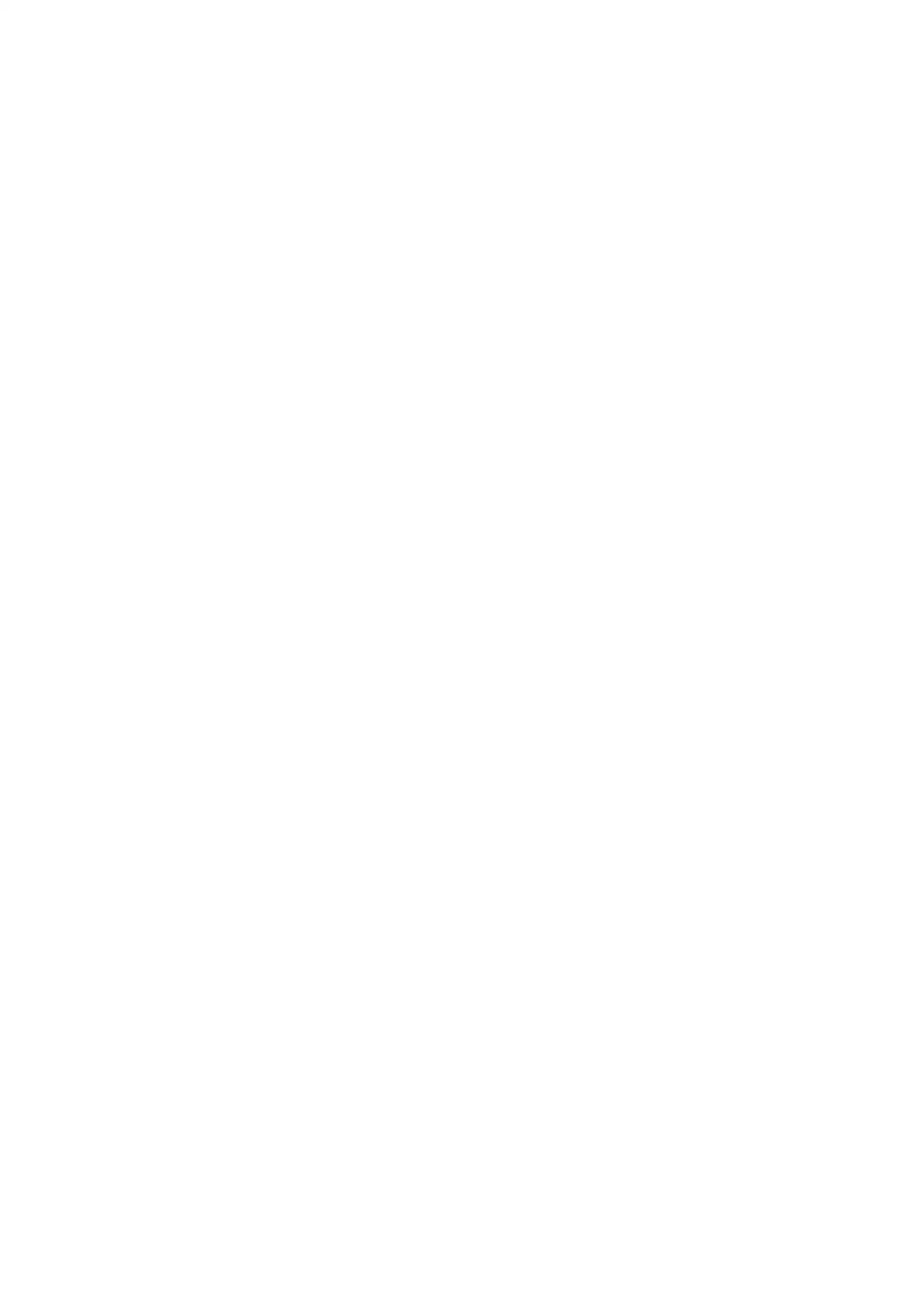
1 out of 8
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
 +13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024  |  Zucol Services PVT LTD  |  All rights reserved.