Common Errors in C Programming and How to Fix Them
VerifiedAdded on 2022/11/29
|6
|1243
|342
AI Summary
This document discusses common errors in C programming and provides solutions for fixing them. It covers missing include statements, insufficient array length, unknown type name, missing cast to char pointer, and off by one errors. Each error is explained in detail, along with the steps to resolve it.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
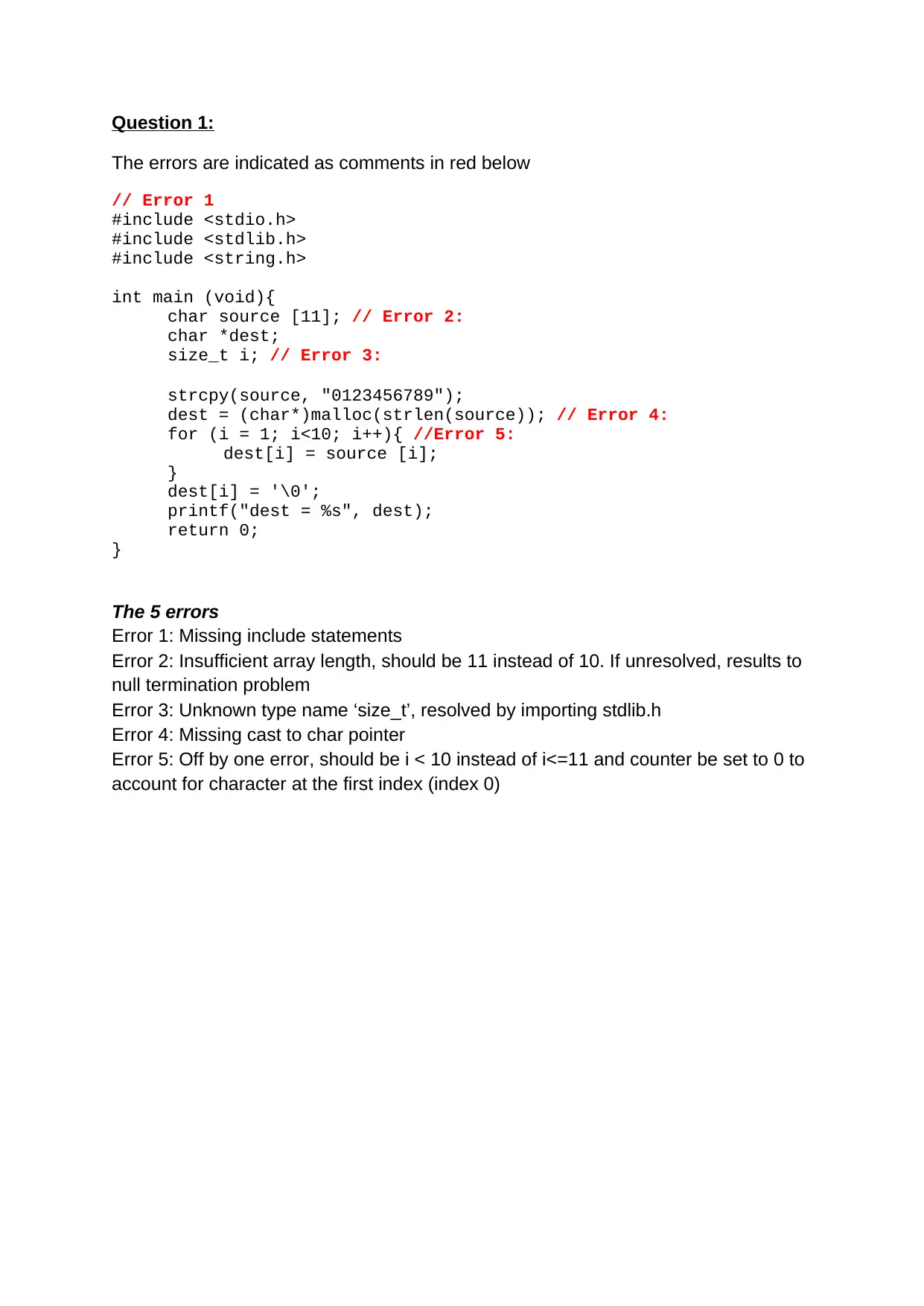
Question 1:
The errors are indicated as comments in red below
// Error 1
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main (void){
char source [11]; // Error 2:
char *dest;
size_t i; // Error 3:
strcpy(source, "0123456789");
dest = (char*)malloc(strlen(source)); // Error 4:
for (i = 1; i<10; i++){ //Error 5:
dest[i] = source [i];
}
dest[i] = '\0';
printf("dest = %s", dest);
return 0;
}
The 5 errors
Error 1: Missing include statements
Error 2: Insufficient array length, should be 11 instead of 10. If unresolved, results to
null termination problem
Error 3: Unknown type name ‘size_t’, resolved by importing stdlib.h
Error 4: Missing cast to char pointer
Error 5: Off by one error, should be i < 10 instead of i<=11 and counter be set to 0 to
account for character at the first index (index 0)
The errors are indicated as comments in red below
// Error 1
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main (void){
char source [11]; // Error 2:
char *dest;
size_t i; // Error 3:
strcpy(source, "0123456789");
dest = (char*)malloc(strlen(source)); // Error 4:
for (i = 1; i<10; i++){ //Error 5:
dest[i] = source [i];
}
dest[i] = '\0';
printf("dest = %s", dest);
return 0;
}
The 5 errors
Error 1: Missing include statements
Error 2: Insufficient array length, should be 11 instead of 10. If unresolved, results to
null termination problem
Error 3: Unknown type name ‘size_t’, resolved by importing stdlib.h
Error 4: Missing cast to char pointer
Error 5: Off by one error, should be i < 10 instead of i<=11 and counter be set to 0 to
account for character at the first index (index 0)
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
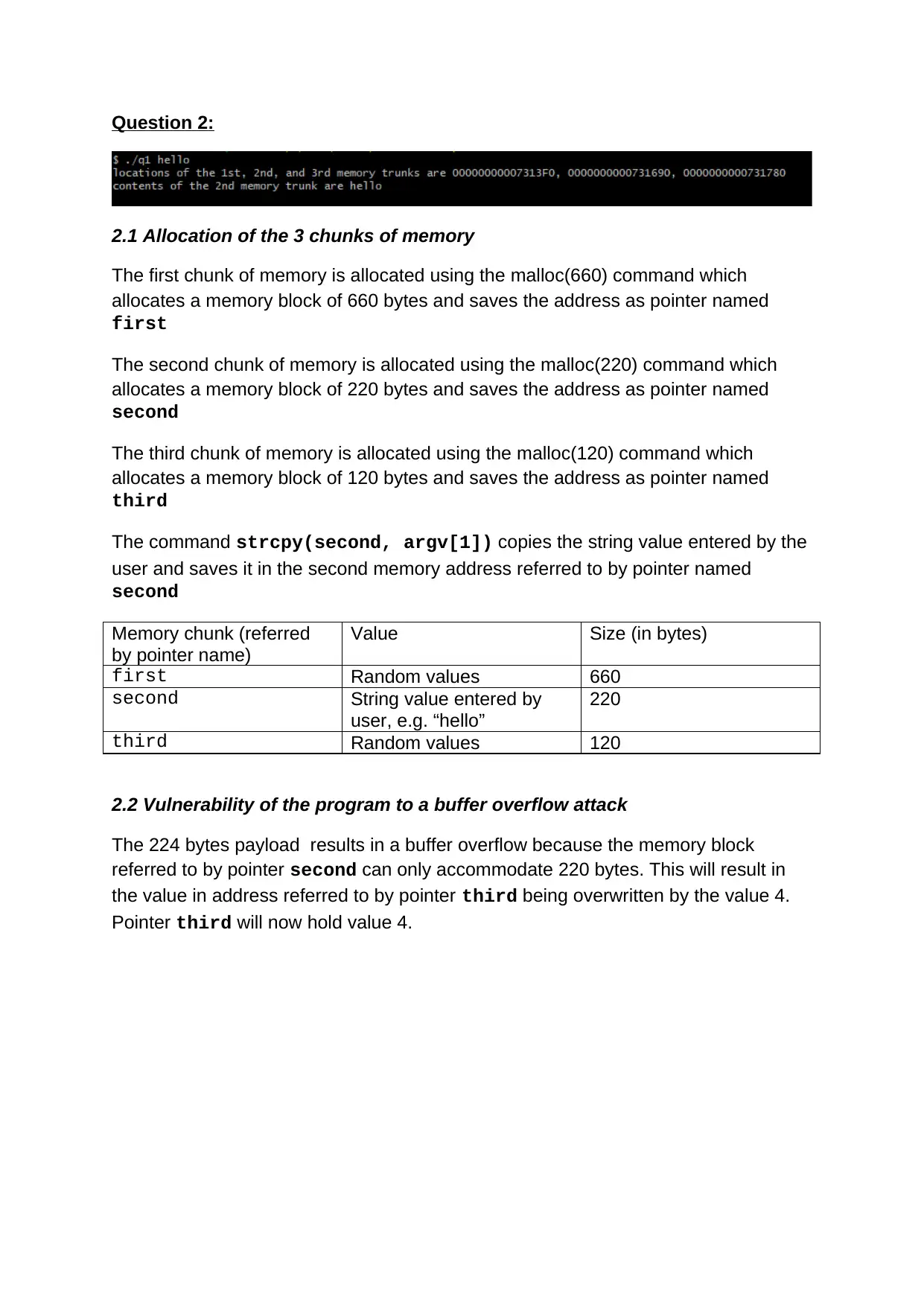
Question 2:
2.1 Allocation of the 3 chunks of memory
The first chunk of memory is allocated using the malloc(660) command which
allocates a memory block of 660 bytes and saves the address as pointer named
first
The second chunk of memory is allocated using the malloc(220) command which
allocates a memory block of 220 bytes and saves the address as pointer named
second
The third chunk of memory is allocated using the malloc(120) command which
allocates a memory block of 120 bytes and saves the address as pointer named
third
The command strcpy(second, argv[1]) copies the string value entered by the
user and saves it in the second memory address referred to by pointer named
second
Memory chunk (referred
by pointer name)
Value Size (in bytes)
first Random values 660
second String value entered by
user, e.g. “hello”
220
third Random values 120
2.2 Vulnerability of the program to a buffer overflow attack
The 224 bytes payload results in a buffer overflow because the memory block
referred to by pointer second can only accommodate 220 bytes. This will result in
the value in address referred to by pointer third being overwritten by the value 4.
Pointer third will now hold value 4.
2.1 Allocation of the 3 chunks of memory
The first chunk of memory is allocated using the malloc(660) command which
allocates a memory block of 660 bytes and saves the address as pointer named
first
The second chunk of memory is allocated using the malloc(220) command which
allocates a memory block of 220 bytes and saves the address as pointer named
second
The third chunk of memory is allocated using the malloc(120) command which
allocates a memory block of 120 bytes and saves the address as pointer named
third
The command strcpy(second, argv[1]) copies the string value entered by the
user and saves it in the second memory address referred to by pointer named
second
Memory chunk (referred
by pointer name)
Value Size (in bytes)
first Random values 660
second String value entered by
user, e.g. “hello”
220
third Random values 120
2.2 Vulnerability of the program to a buffer overflow attack
The 224 bytes payload results in a buffer overflow because the memory block
referred to by pointer second can only accommodate 220 bytes. This will result in
the value in address referred to by pointer third being overwritten by the value 4.
Pointer third will now hold value 4.
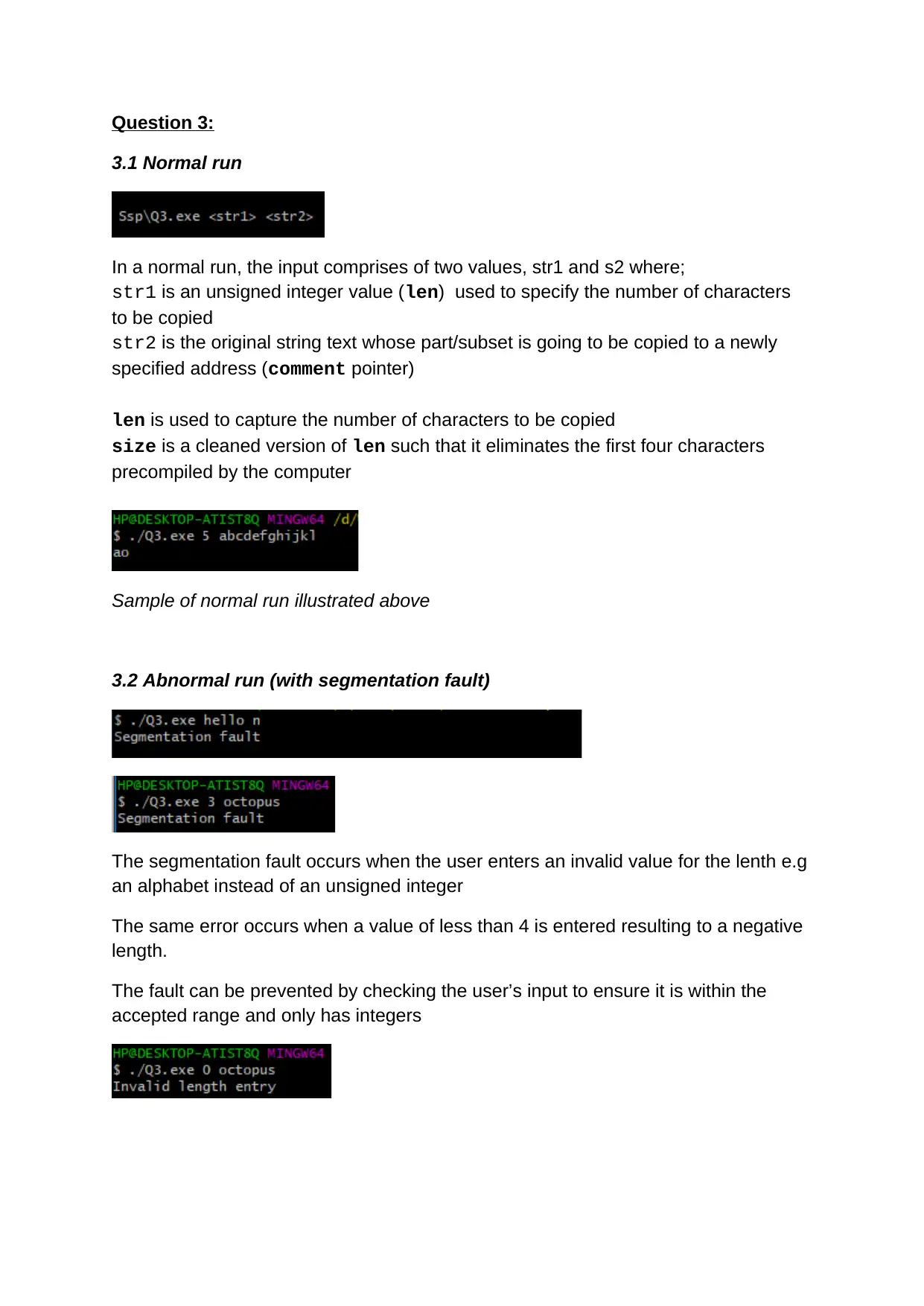
Question 3:
3.1 Normal run
In a normal run, the input comprises of two values, str1 and s2 where;
str1 is an unsigned integer value (len) used to specify the number of characters
to be copied
str2 is the original string text whose part/subset is going to be copied to a newly
specified address (comment pointer)
len is used to capture the number of characters to be copied
size is a cleaned version of len such that it eliminates the first four characters
precompiled by the computer
Sample of normal run illustrated above
3.2 Abnormal run (with segmentation fault)
The segmentation fault occurs when the user enters an invalid value for the lenth e.g
an alphabet instead of an unsigned integer
The same error occurs when a value of less than 4 is entered resulting to a negative
length.
The fault can be prevented by checking the user’s input to ensure it is within the
accepted range and only has integers
3.1 Normal run
In a normal run, the input comprises of two values, str1 and s2 where;
str1 is an unsigned integer value (len) used to specify the number of characters
to be copied
str2 is the original string text whose part/subset is going to be copied to a newly
specified address (comment pointer)
len is used to capture the number of characters to be copied
size is a cleaned version of len such that it eliminates the first four characters
precompiled by the computer
Sample of normal run illustrated above
3.2 Abnormal run (with segmentation fault)
The segmentation fault occurs when the user enters an invalid value for the lenth e.g
an alphabet instead of an unsigned integer
The same error occurs when a value of less than 4 is entered resulting to a negative
length.
The fault can be prevented by checking the user’s input to ensure it is within the
accepted range and only has integers
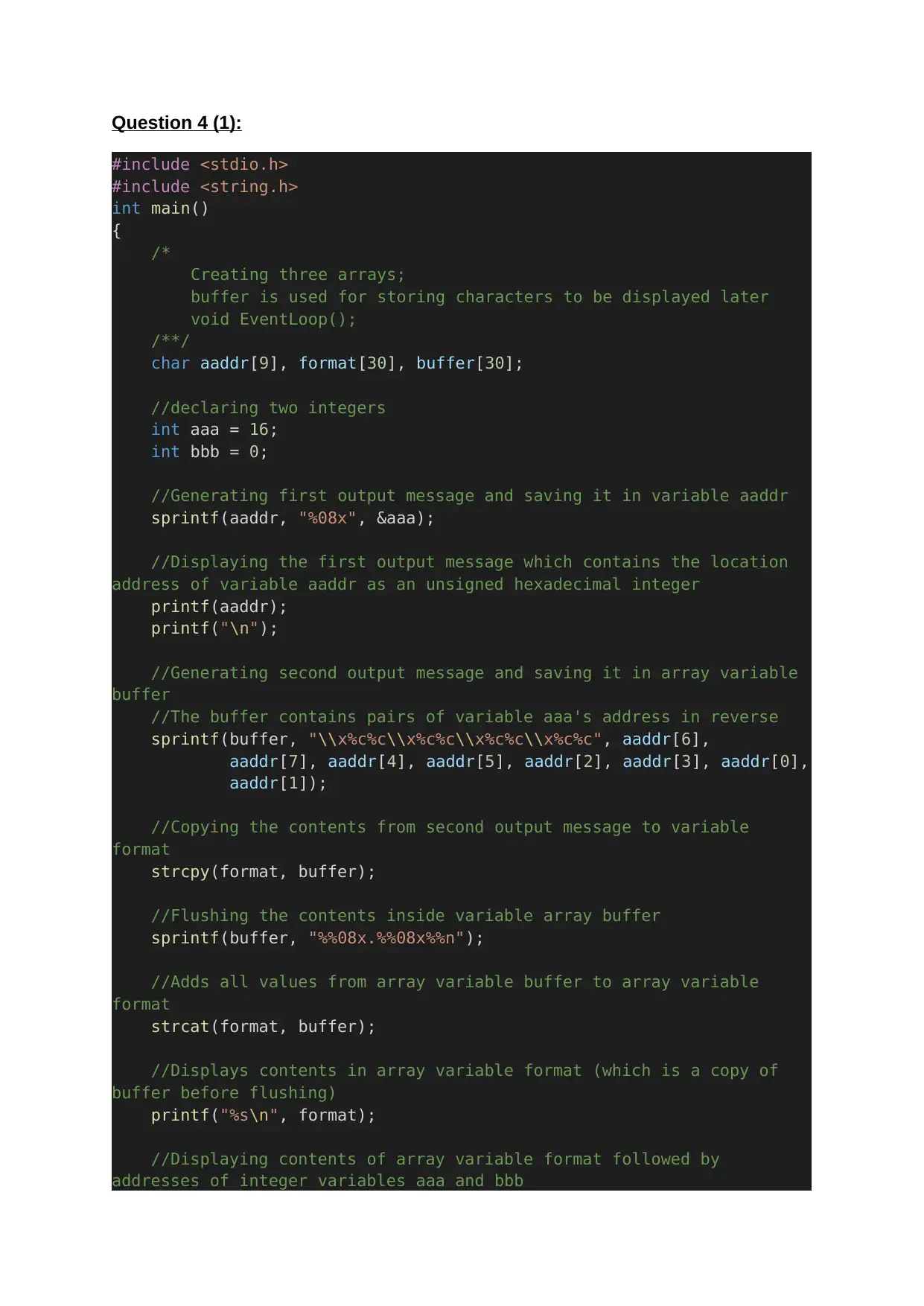
Question 4 (1):
#include <stdio.h>
#include <string.h>
int main()
{
/*
Creating three arrays;
buffer is used for storing characters to be displayed later
void EventLoop();
/**/
char aaddr[9], format[30], buffer[30];
//declaring two integers
int aaa = 16;
int bbb = 0;
//Generating first output message and saving it in variable aaddr
sprintf(aaddr, "%08x", &aaa);
//Displaying the first output message which contains the location
address of variable aaddr as an unsigned hexadecimal integer
printf(aaddr);
printf("\n");
//Generating second output message and saving it in array variable
buffer
//The buffer contains pairs of variable aaa's address in reverse
sprintf(buffer, "\\x%c%c\\x%c%c\\x%c%c\\x%c%c", aaddr[6],
aaddr[7], aaddr[4], aaddr[5], aaddr[2], aaddr[3], aaddr[0],
aaddr[1]);
//Copying the contents from second output message to variable
format
strcpy(format, buffer);
//Flushing the contents inside variable array buffer
sprintf(buffer, "%%08x.%%08x%%n");
//Adds all values from array variable buffer to array variable
format
strcat(format, buffer);
//Displays contents in array variable format (which is a copy of
buffer before flushing)
printf("%s\n", format);
//Displaying contents of array variable format followed by
addresses of integer variables aaa and bbb
#include <stdio.h>
#include <string.h>
int main()
{
/*
Creating three arrays;
buffer is used for storing characters to be displayed later
void EventLoop();
/**/
char aaddr[9], format[30], buffer[30];
//declaring two integers
int aaa = 16;
int bbb = 0;
//Generating first output message and saving it in variable aaddr
sprintf(aaddr, "%08x", &aaa);
//Displaying the first output message which contains the location
address of variable aaddr as an unsigned hexadecimal integer
printf(aaddr);
printf("\n");
//Generating second output message and saving it in array variable
buffer
//The buffer contains pairs of variable aaa's address in reverse
sprintf(buffer, "\\x%c%c\\x%c%c\\x%c%c\\x%c%c", aaddr[6],
aaddr[7], aaddr[4], aaddr[5], aaddr[2], aaddr[3], aaddr[0],
aaddr[1]);
//Copying the contents from second output message to variable
format
strcpy(format, buffer);
//Flushing the contents inside variable array buffer
sprintf(buffer, "%%08x.%%08x%%n");
//Adds all values from array variable buffer to array variable
format
strcat(format, buffer);
//Displays contents in array variable format (which is a copy of
buffer before flushing)
printf("%s\n", format);
//Displaying contents of array variable format followed by
addresses of integer variables aaa and bbb
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
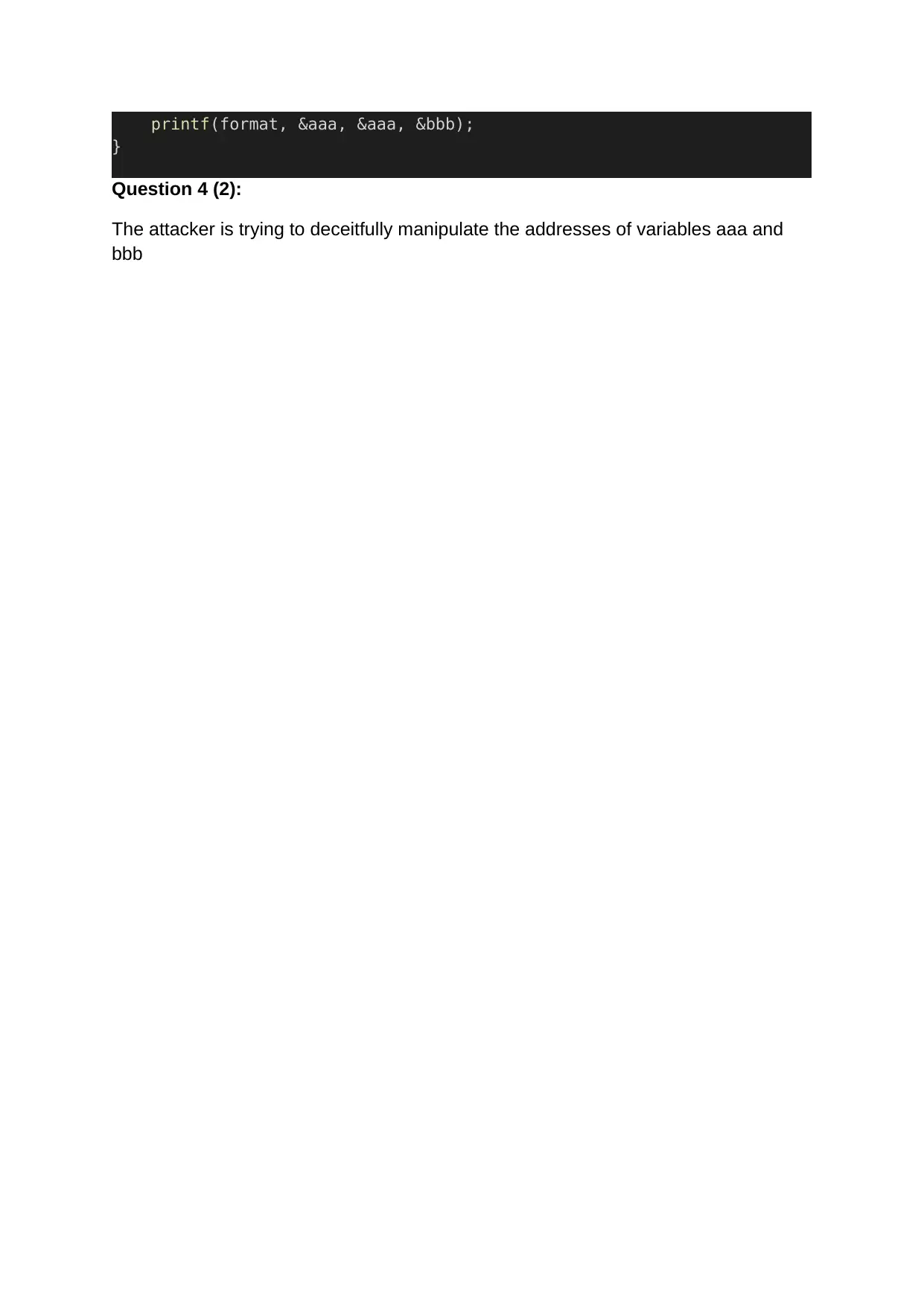
printf(format, &aaa, &aaa, &bbb);
}
Question 4 (2):
The attacker is trying to deceitfully manipulate the addresses of variables aaa and
bbb
}
Question 4 (2):
The attacker is trying to deceitfully manipulate the addresses of variables aaa and
bbb
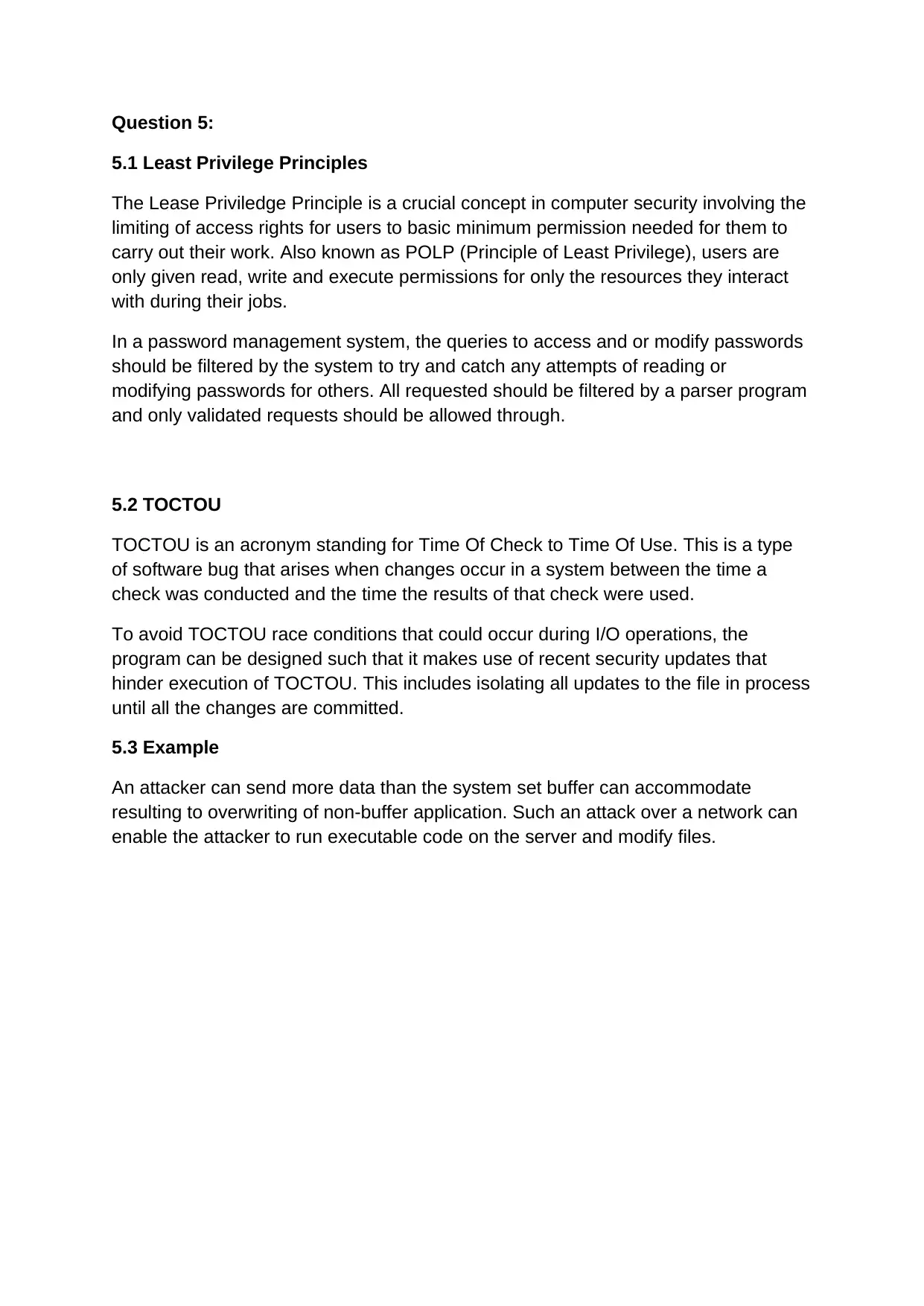
Question 5:
5.1 Least Privilege Principles
The Lease Priviledge Principle is a crucial concept in computer security involving the
limiting of access rights for users to basic minimum permission needed for them to
carry out their work. Also known as POLP (Principle of Least Privilege), users are
only given read, write and execute permissions for only the resources they interact
with during their jobs.
In a password management system, the queries to access and or modify passwords
should be filtered by the system to try and catch any attempts of reading or
modifying passwords for others. All requested should be filtered by a parser program
and only validated requests should be allowed through.
5.2 TOCTOU
TOCTOU is an acronym standing for Time Of Check to Time Of Use. This is a type
of software bug that arises when changes occur in a system between the time a
check was conducted and the time the results of that check were used.
To avoid TOCTOU race conditions that could occur during I/O operations, the
program can be designed such that it makes use of recent security updates that
hinder execution of TOCTOU. This includes isolating all updates to the file in process
until all the changes are committed.
5.3 Example
An attacker can send more data than the system set buffer can accommodate
resulting to overwriting of non-buffer application. Such an attack over a network can
enable the attacker to run executable code on the server and modify files.
5.1 Least Privilege Principles
The Lease Priviledge Principle is a crucial concept in computer security involving the
limiting of access rights for users to basic minimum permission needed for them to
carry out their work. Also known as POLP (Principle of Least Privilege), users are
only given read, write and execute permissions for only the resources they interact
with during their jobs.
In a password management system, the queries to access and or modify passwords
should be filtered by the system to try and catch any attempts of reading or
modifying passwords for others. All requested should be filtered by a parser program
and only validated requests should be allowed through.
5.2 TOCTOU
TOCTOU is an acronym standing for Time Of Check to Time Of Use. This is a type
of software bug that arises when changes occur in a system between the time a
check was conducted and the time the results of that check were used.
To avoid TOCTOU race conditions that could occur during I/O operations, the
program can be designed such that it makes use of recent security updates that
hinder execution of TOCTOU. This includes isolating all updates to the file in process
until all the changes are committed.
5.3 Example
An attacker can send more data than the system set buffer can accommodate
resulting to overwriting of non-buffer application. Such an attack over a network can
enable the attacker to run executable code on the server and modify files.
1 out of 6
Related Documents
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.