Common Errors in C Programming and How to Fix Them
Added on 2022-11-29
6 Pages1243 Words342 Views
Question 1:
The errors are indicated as comments in red below
// Error 1
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main (void){
char source [11]; // Error 2:
char *dest;
size_t i; // Error 3:
strcpy(source, "0123456789");
dest = (char*)malloc(strlen(source)); // Error 4:
for (i = 1; i<10; i++){ //Error 5:
dest[i] = source [i];
}
dest[i] = '\0';
printf("dest = %s", dest);
return 0;
}
The 5 errors
Error 1: Missing include statements
Error 2: Insufficient array length, should be 11 instead of 10. If unresolved, results to
null termination problem
Error 3: Unknown type name ‘size_t’, resolved by importing stdlib.h
Error 4: Missing cast to char pointer
Error 5: Off by one error, should be i < 10 instead of i<=11 and counter be set to 0 to
account for character at the first index (index 0)
The errors are indicated as comments in red below
// Error 1
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main (void){
char source [11]; // Error 2:
char *dest;
size_t i; // Error 3:
strcpy(source, "0123456789");
dest = (char*)malloc(strlen(source)); // Error 4:
for (i = 1; i<10; i++){ //Error 5:
dest[i] = source [i];
}
dest[i] = '\0';
printf("dest = %s", dest);
return 0;
}
The 5 errors
Error 1: Missing include statements
Error 2: Insufficient array length, should be 11 instead of 10. If unresolved, results to
null termination problem
Error 3: Unknown type name ‘size_t’, resolved by importing stdlib.h
Error 4: Missing cast to char pointer
Error 5: Off by one error, should be i < 10 instead of i<=11 and counter be set to 0 to
account for character at the first index (index 0)
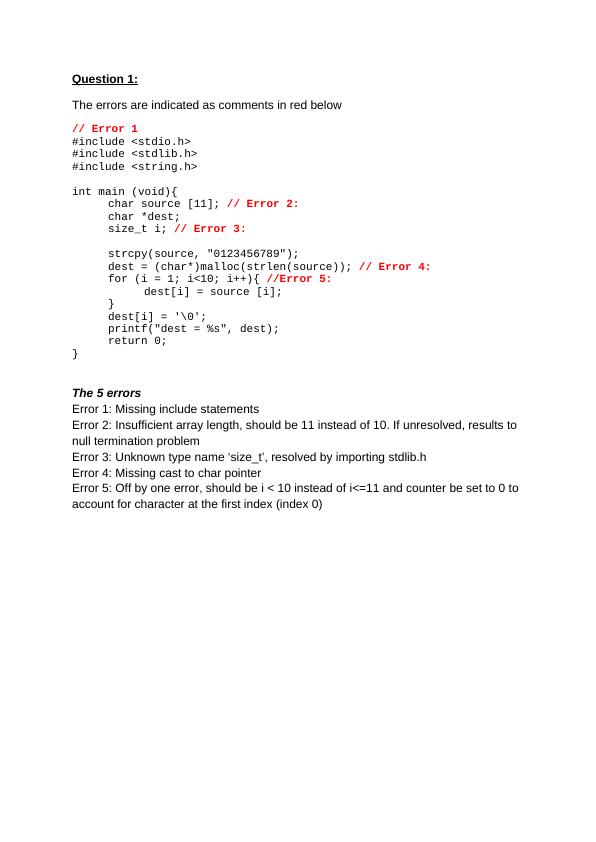
Question 2:
2.1 Allocation of the 3 chunks of memory
The first chunk of memory is allocated using the malloc(660) command which
allocates a memory block of 660 bytes and saves the address as pointer named
first
The second chunk of memory is allocated using the malloc(220) command which
allocates a memory block of 220 bytes and saves the address as pointer named
second
The third chunk of memory is allocated using the malloc(120) command which
allocates a memory block of 120 bytes and saves the address as pointer named
third
The command strcpy(second, argv[1]) copies the string value entered by the
user and saves it in the second memory address referred to by pointer named
second
Memory chunk (referred
by pointer name)
Value Size (in bytes)
first Random values 660
second String value entered by
user, e.g. “hello”
220
third Random values 120
2.2 Vulnerability of the program to a buffer overflow attack
The 224 bytes payload results in a buffer overflow because the memory block
referred to by pointer second can only accommodate 220 bytes. This will result in
the value in address referred to by pointer third being overwritten by the value 4.
Pointer third will now hold value 4.
2.1 Allocation of the 3 chunks of memory
The first chunk of memory is allocated using the malloc(660) command which
allocates a memory block of 660 bytes and saves the address as pointer named
first
The second chunk of memory is allocated using the malloc(220) command which
allocates a memory block of 220 bytes and saves the address as pointer named
second
The third chunk of memory is allocated using the malloc(120) command which
allocates a memory block of 120 bytes and saves the address as pointer named
third
The command strcpy(second, argv[1]) copies the string value entered by the
user and saves it in the second memory address referred to by pointer named
second
Memory chunk (referred
by pointer name)
Value Size (in bytes)
first Random values 660
second String value entered by
user, e.g. “hello”
220
third Random values 120
2.2 Vulnerability of the program to a buffer overflow attack
The 224 bytes payload results in a buffer overflow because the memory block
referred to by pointer second can only accommodate 220 bytes. This will result in
the value in address referred to by pointer third being overwritten by the value 4.
Pointer third will now hold value 4.
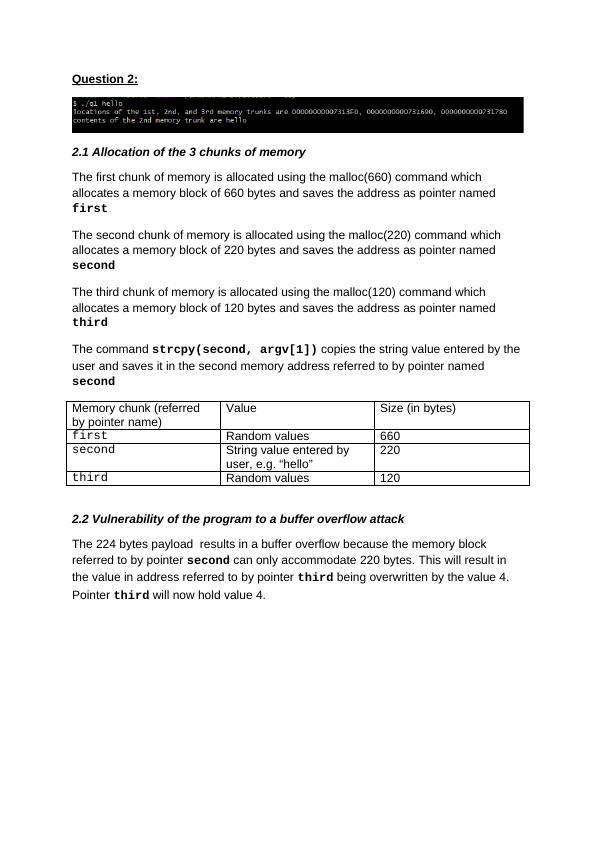
Question 3:
3.1 Normal run
In a normal run, the input comprises of two values, str1 and s2 where;
str1 is an unsigned integer value (len) used to specify the number of characters
to be copied
str2 is the original string text whose part/subset is going to be copied to a newly
specified address (comment pointer)
len is used to capture the number of characters to be copied
size is a cleaned version of len such that it eliminates the first four characters
precompiled by the computer
Sample of normal run illustrated above
3.2 Abnormal run (with segmentation fault)
The segmentation fault occurs when the user enters an invalid value for the lenth e.g
an alphabet instead of an unsigned integer
The same error occurs when a value of less than 4 is entered resulting to a negative
length.
The fault can be prevented by checking the user’s input to ensure it is within the
accepted range and only has integers
3.1 Normal run
In a normal run, the input comprises of two values, str1 and s2 where;
str1 is an unsigned integer value (len) used to specify the number of characters
to be copied
str2 is the original string text whose part/subset is going to be copied to a newly
specified address (comment pointer)
len is used to capture the number of characters to be copied
size is a cleaned version of len such that it eliminates the first four characters
precompiled by the computer
Sample of normal run illustrated above
3.2 Abnormal run (with segmentation fault)
The segmentation fault occurs when the user enters an invalid value for the lenth e.g
an alphabet instead of an unsigned integer
The same error occurs when a value of less than 4 is entered resulting to a negative
length.
The fault can be prevented by checking the user’s input to ensure it is within the
accepted range and only has integers
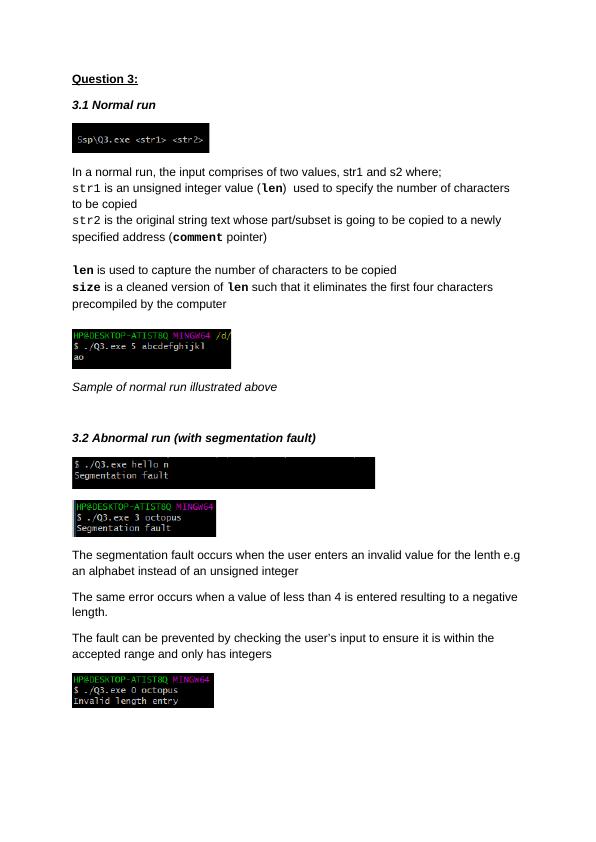
End of preview
Want to access all the pages? Upload your documents or become a member.
Related Documents
Secure Systems Programming: Coursework 2lg...
|10
|2518
|90
Secure Systems Programminglg...
|13
|1507
|3
Understanding Buffer Overflow: Stack and Heap Buffer Overflows, Exploiting Buffer Overflow, JOP and ROPlg...
|11
|1080
|283
Secure Programming in Clg...
|14
|2158
|367
Solution Question 1: 1) 2) 3) 4).lg...
|2
|340
|68