Safe-Driving Practice Simulator
VerifiedAdded on  2023/06/15
|14
|973
|476
AI Summary
Desklib offers a Safe-Driving Practice Simulator for students to experience safe-driving. The simulator includes a racing board, UML diagram, flow chart, model view, and source code for the AcceleratorMonitor event handler. The simulator also includes 3D graphics for rotating geometry and graphics operation.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
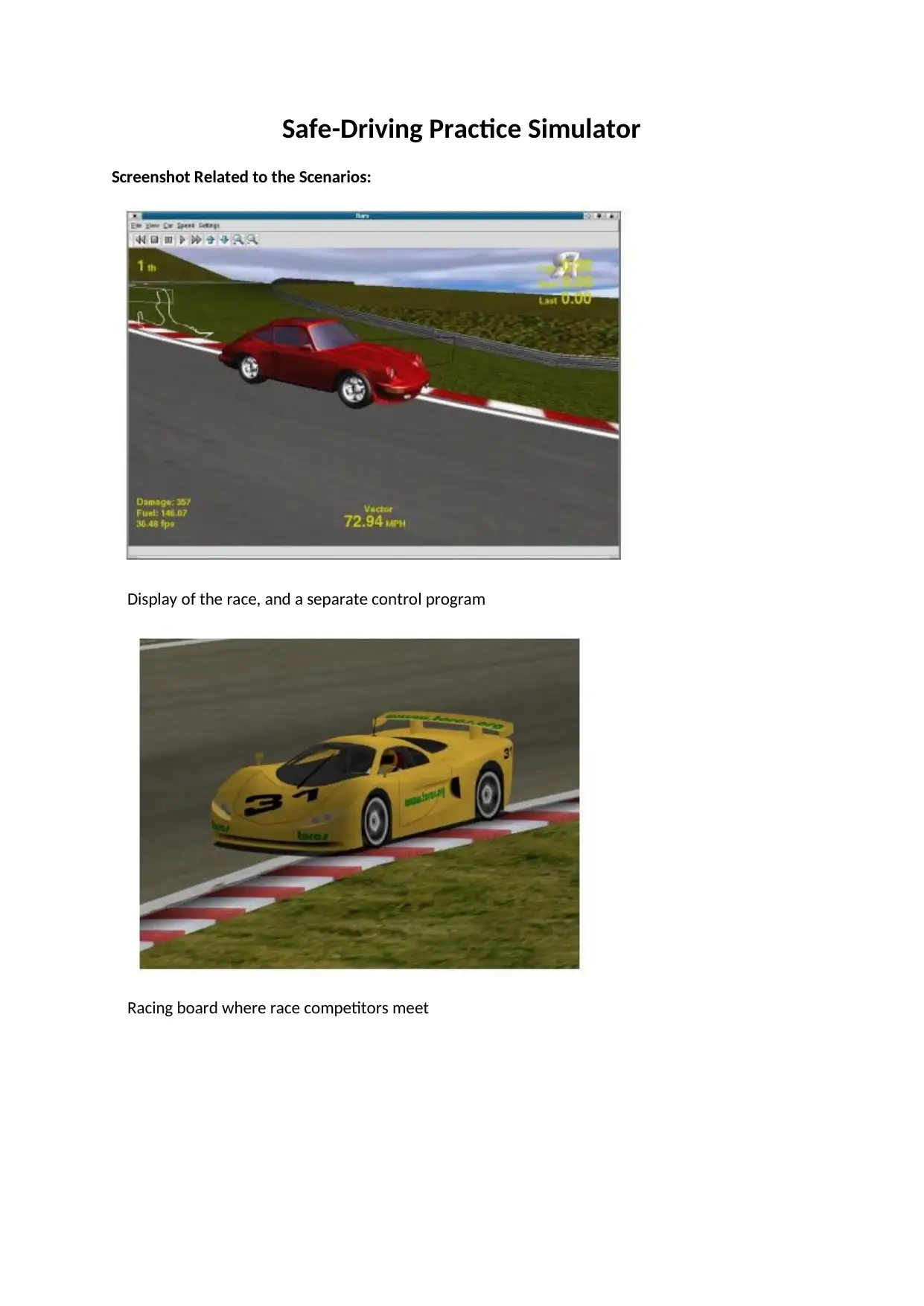
Safe-Driving Practice Simulator
Screenshot Related to the Scenarios:
Display of the race, and a separate control program
Racing board where race competitors meet
Screenshot Related to the Scenarios:
Display of the race, and a separate control program
Racing board where race competitors meet
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
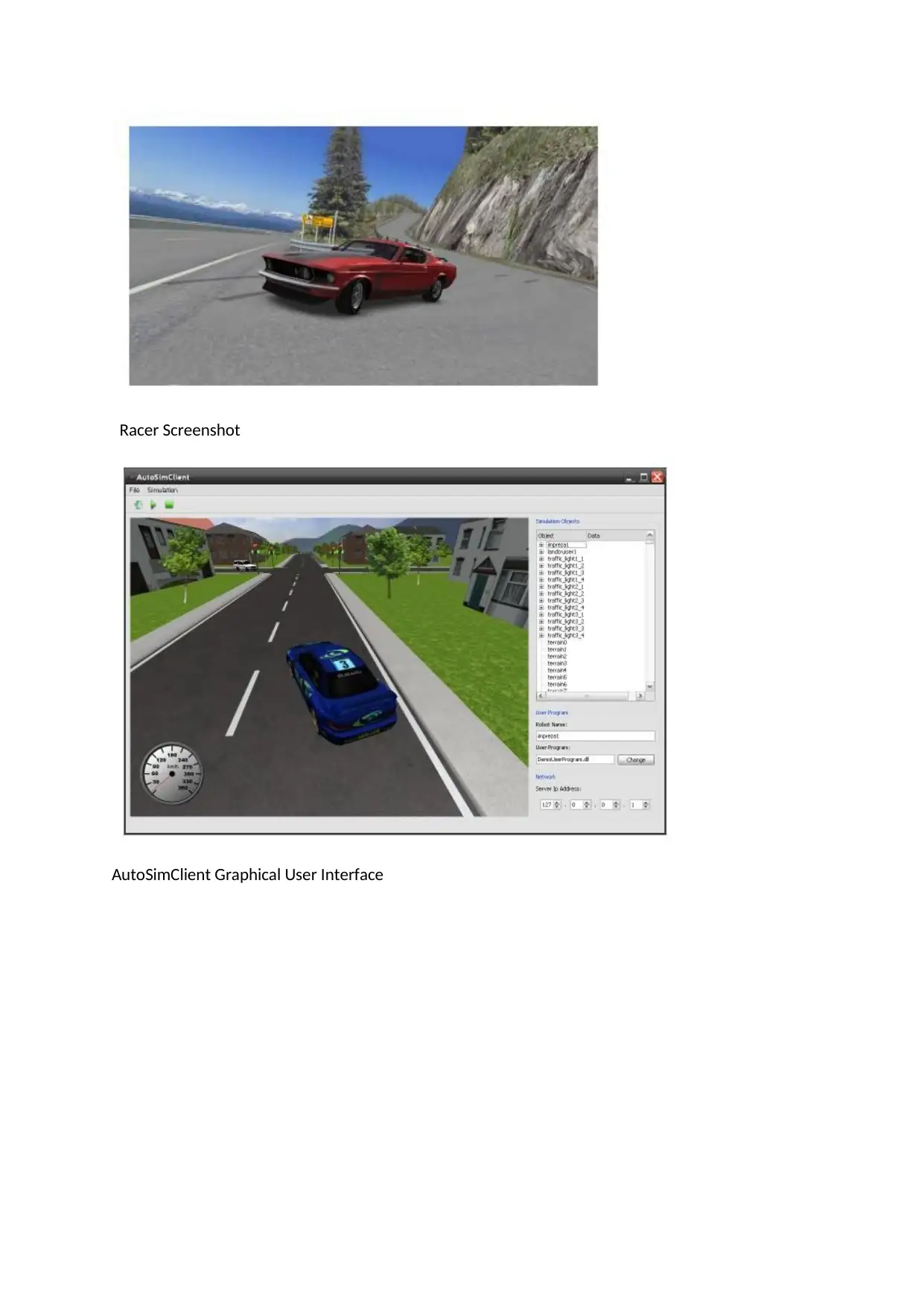
Racer Screenshot
AutoSimClient Graphical User Interface
AutoSimClient Graphical User Interface
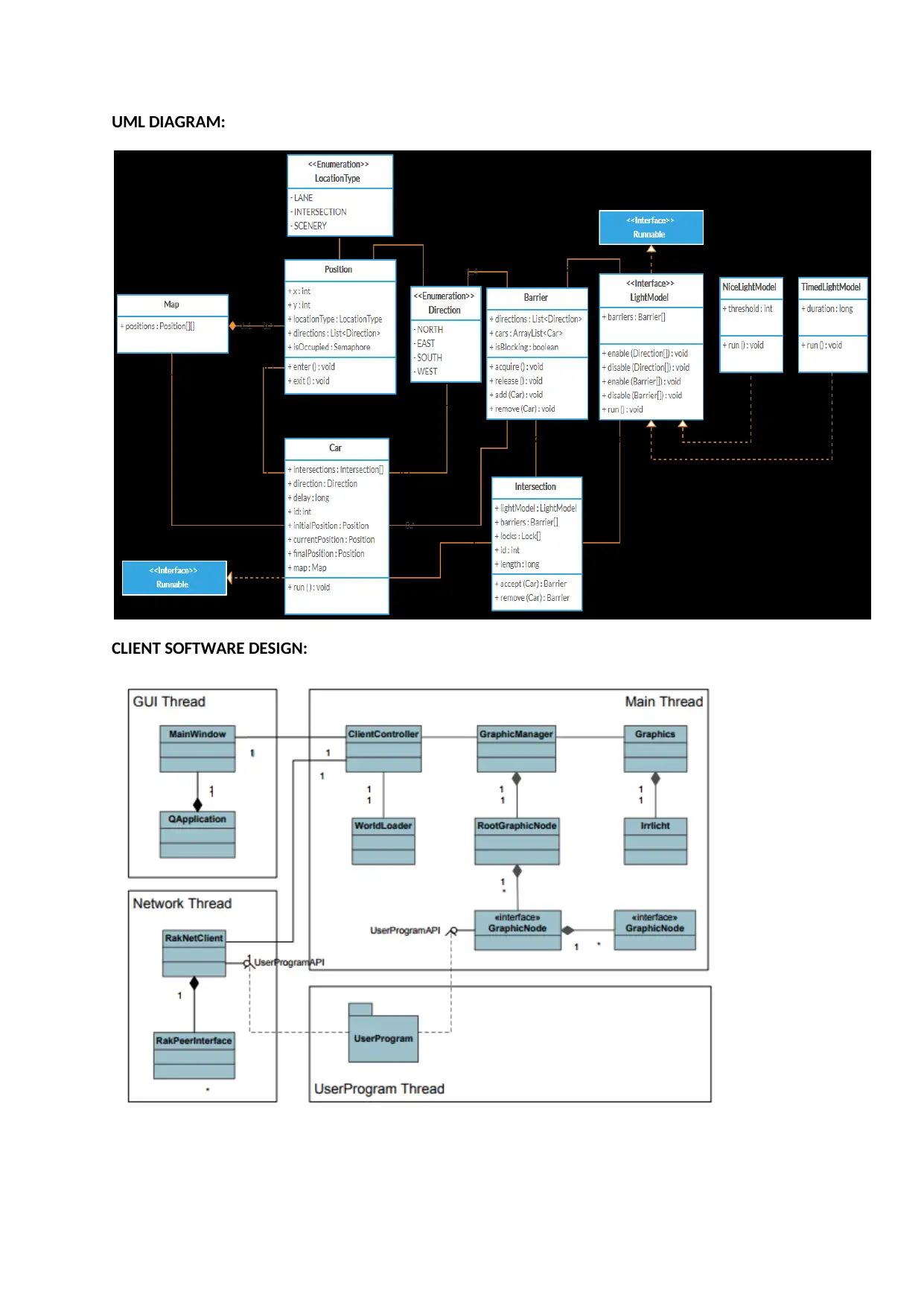
UML DIAGRAM:
CLIENT SOFTWARE DESIGN:
CLIENT SOFTWARE DESIGN:
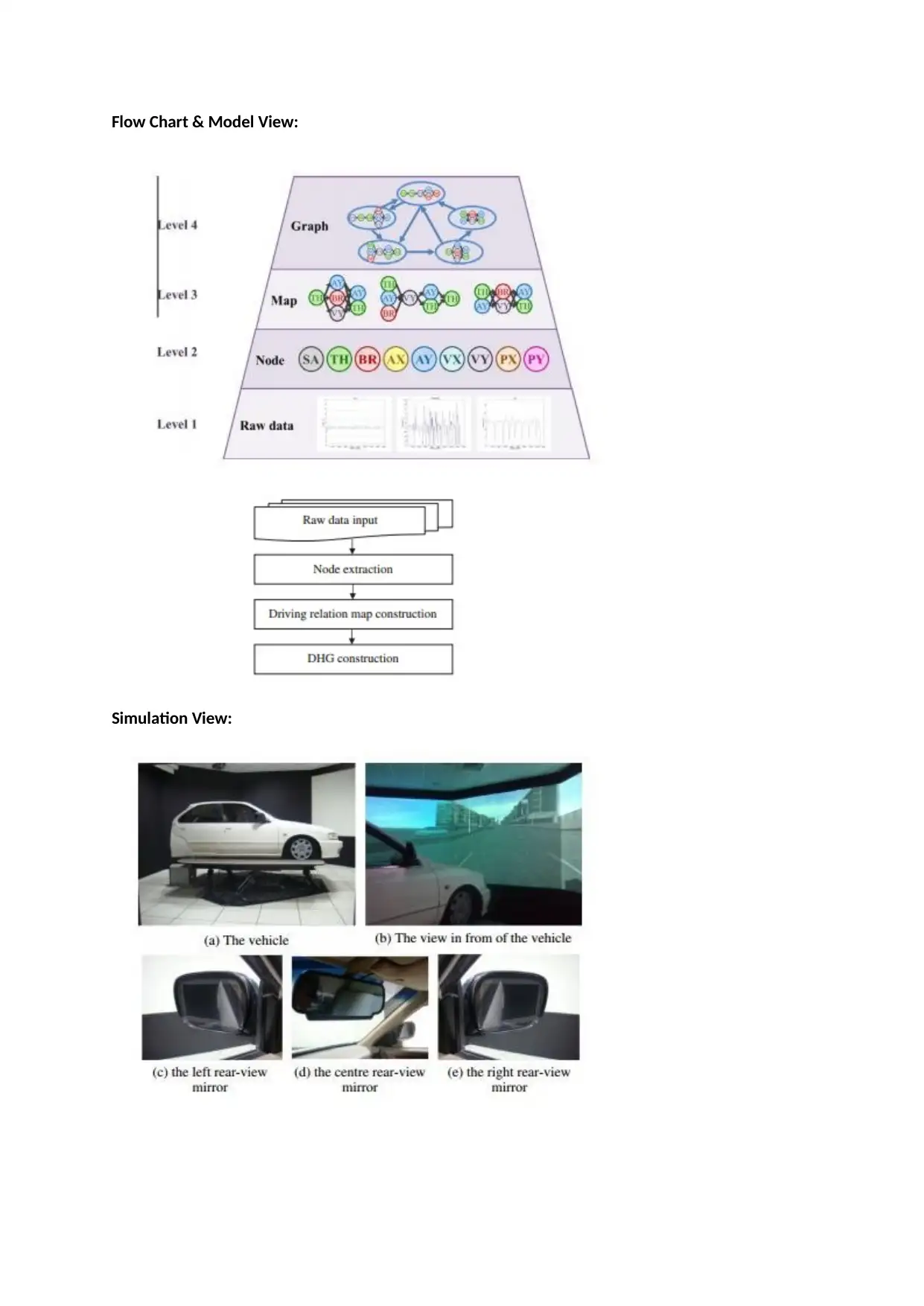
Flow Chart & Model View:
Simulation View:
Simulation View:
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
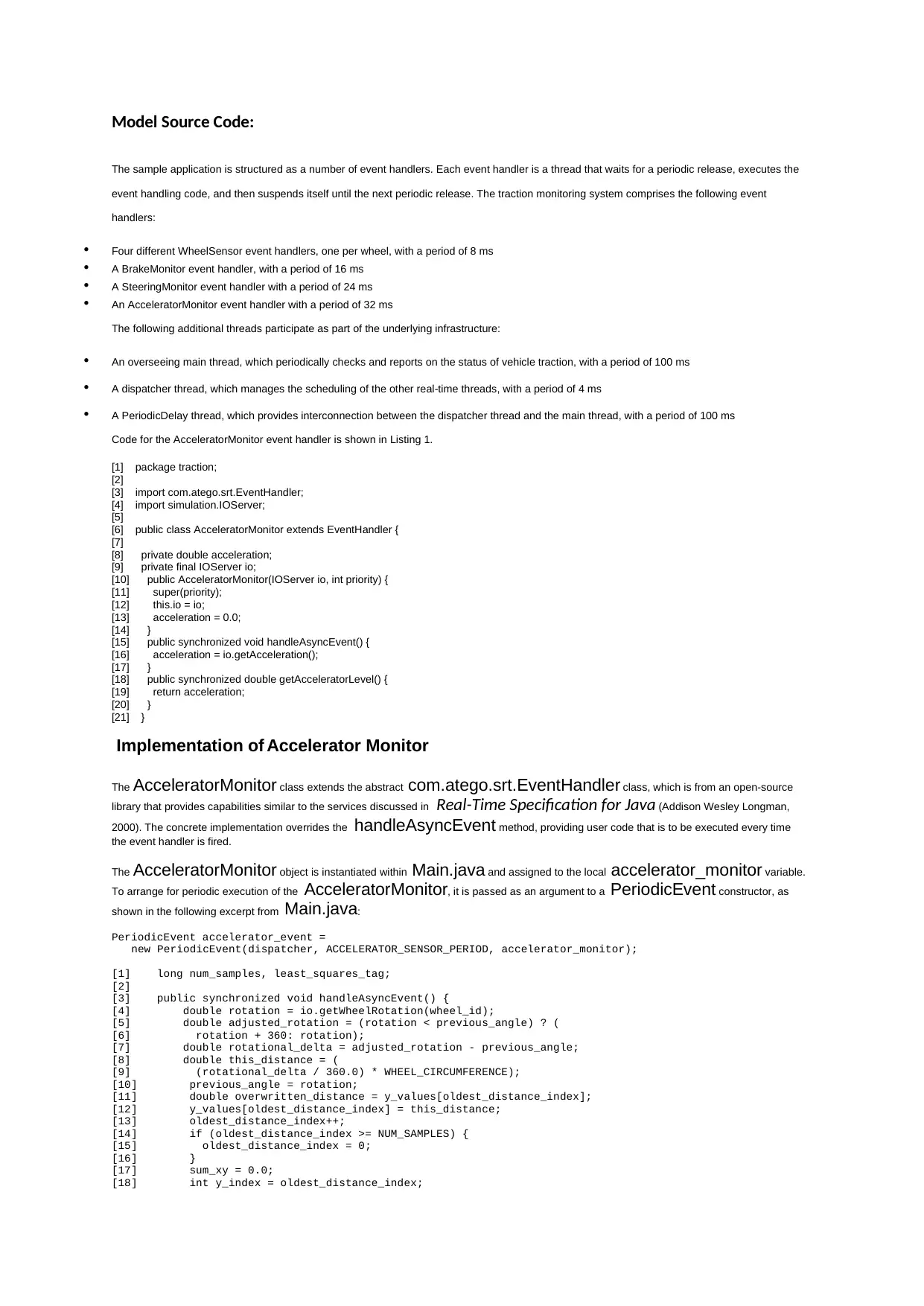
Model Source Code:
The sample application is structured as a number of event handlers. Each event handler is a thread that waits for a periodic release, executes the
event handling code, and then suspends itself until the next periodic release. The traction monitoring system comprises the following event
handlers:
• Four different WheelSensor event handlers, one per wheel, with a period of 8 ms
• A BrakeMonitor event handler, with a period of 16 ms
• A SteeringMonitor event handler with a period of 24 ms
• An AcceleratorMonitor event handler with a period of 32 ms
The following additional threads participate as part of the underlying infrastructure:
• An overseeing main thread, which periodically checks and reports on the status of vehicle traction, with a period of 100 ms
• A dispatcher thread, which manages the scheduling of the other real-time threads, with a period of 4 ms
• A PeriodicDelay thread, which provides interconnection between the dispatcher thread and the main thread, with a period of 100 ms
Code for the AcceleratorMonitor event handler is shown in Listing 1.
[1] package traction;
[2]
[3] import com.atego.srt.EventHandler;
[4] import simulation.IOServer;
[5]
[6] public class AcceleratorMonitor extends EventHandler {
[7]
[8] private double acceleration;
[9] private final IOServer io;
[10] public AcceleratorMonitor(IOServer io, int priority) {
[11] super(priority);
[12] this.io = io;
[13] acceleration = 0.0;
[14] }
[15] public synchronized void handleAsyncEvent() {
[16] acceleration = io.getAcceleration();
[17] }
[18] public synchronized double getAcceleratorLevel() {
[19] return acceleration;
[20] }
[21] }
Implementation of Accelerator Monitor
The AcceleratorMonitor class extends the abstract com.atego.srt.EventHandler class, which is from an open-source
library that provides capabilities similar to the services discussed in Real-Time Specification for Java (Addison Wesley Longman,
2000). The concrete implementation overrides the handleAsyncEvent method, providing user code that is to be executed every time
the event handler is fired.
The AcceleratorMonitor object is instantiated within Main.java and assigned to the local accelerator_monitor variable.
To arrange for periodic execution of the AcceleratorMonitor, it is passed as an argument to a PeriodicEvent constructor, as
shown in the following excerpt from Main.java:
PeriodicEvent accelerator_event =
new PeriodicEvent(dispatcher, ACCELERATOR_SENSOR_PERIOD, accelerator_monitor);
[1] long num_samples, least_squares_tag;
[2]
[3] public synchronized void handleAsyncEvent() {
[4] double rotation = io.getWheelRotation(wheel_id);
[5] double adjusted_rotation = (rotation < previous_angle) ? (
[6] rotation + 360: rotation);
[7] double rotational_delta = adjusted_rotation - previous_angle;
[8] double this_distance = (
[9] (rotational_delta / 360.0) * WHEEL_CIRCUMFERENCE);
[10] previous_angle = rotation;
[11] double overwritten_distance = y_values[oldest_distance_index];
[12] y_values[oldest_distance_index] = this_distance;
[13] oldest_distance_index++;
[14] if (oldest_distance_index >= NUM_SAMPLES) {
[15] oldest_distance_index = 0;
[16] }
[17] sum_xy = 0.0;
[18] int y_index = oldest_distance_index;
The sample application is structured as a number of event handlers. Each event handler is a thread that waits for a periodic release, executes the
event handling code, and then suspends itself until the next periodic release. The traction monitoring system comprises the following event
handlers:
• Four different WheelSensor event handlers, one per wheel, with a period of 8 ms
• A BrakeMonitor event handler, with a period of 16 ms
• A SteeringMonitor event handler with a period of 24 ms
• An AcceleratorMonitor event handler with a period of 32 ms
The following additional threads participate as part of the underlying infrastructure:
• An overseeing main thread, which periodically checks and reports on the status of vehicle traction, with a period of 100 ms
• A dispatcher thread, which manages the scheduling of the other real-time threads, with a period of 4 ms
• A PeriodicDelay thread, which provides interconnection between the dispatcher thread and the main thread, with a period of 100 ms
Code for the AcceleratorMonitor event handler is shown in Listing 1.
[1] package traction;
[2]
[3] import com.atego.srt.EventHandler;
[4] import simulation.IOServer;
[5]
[6] public class AcceleratorMonitor extends EventHandler {
[7]
[8] private double acceleration;
[9] private final IOServer io;
[10] public AcceleratorMonitor(IOServer io, int priority) {
[11] super(priority);
[12] this.io = io;
[13] acceleration = 0.0;
[14] }
[15] public synchronized void handleAsyncEvent() {
[16] acceleration = io.getAcceleration();
[17] }
[18] public synchronized double getAcceleratorLevel() {
[19] return acceleration;
[20] }
[21] }
Implementation of Accelerator Monitor
The AcceleratorMonitor class extends the abstract com.atego.srt.EventHandler class, which is from an open-source
library that provides capabilities similar to the services discussed in Real-Time Specification for Java (Addison Wesley Longman,
2000). The concrete implementation overrides the handleAsyncEvent method, providing user code that is to be executed every time
the event handler is fired.
The AcceleratorMonitor object is instantiated within Main.java and assigned to the local accelerator_monitor variable.
To arrange for periodic execution of the AcceleratorMonitor, it is passed as an argument to a PeriodicEvent constructor, as
shown in the following excerpt from Main.java:
PeriodicEvent accelerator_event =
new PeriodicEvent(dispatcher, ACCELERATOR_SENSOR_PERIOD, accelerator_monitor);
[1] long num_samples, least_squares_tag;
[2]
[3] public synchronized void handleAsyncEvent() {
[4] double rotation = io.getWheelRotation(wheel_id);
[5] double adjusted_rotation = (rotation < previous_angle) ? (
[6] rotation + 360: rotation);
[7] double rotational_delta = adjusted_rotation - previous_angle;
[8] double this_distance = (
[9] (rotational_delta / 360.0) * WHEEL_CIRCUMFERENCE);
[10] previous_angle = rotation;
[11] double overwritten_distance = y_values[oldest_distance_index];
[12] y_values[oldest_distance_index] = this_distance;
[13] oldest_distance_index++;
[14] if (oldest_distance_index >= NUM_SAMPLES) {
[15] oldest_distance_index = 0;
[16] }
[17] sum_xy = 0.0;
[18] int y_index = oldest_distance_index;
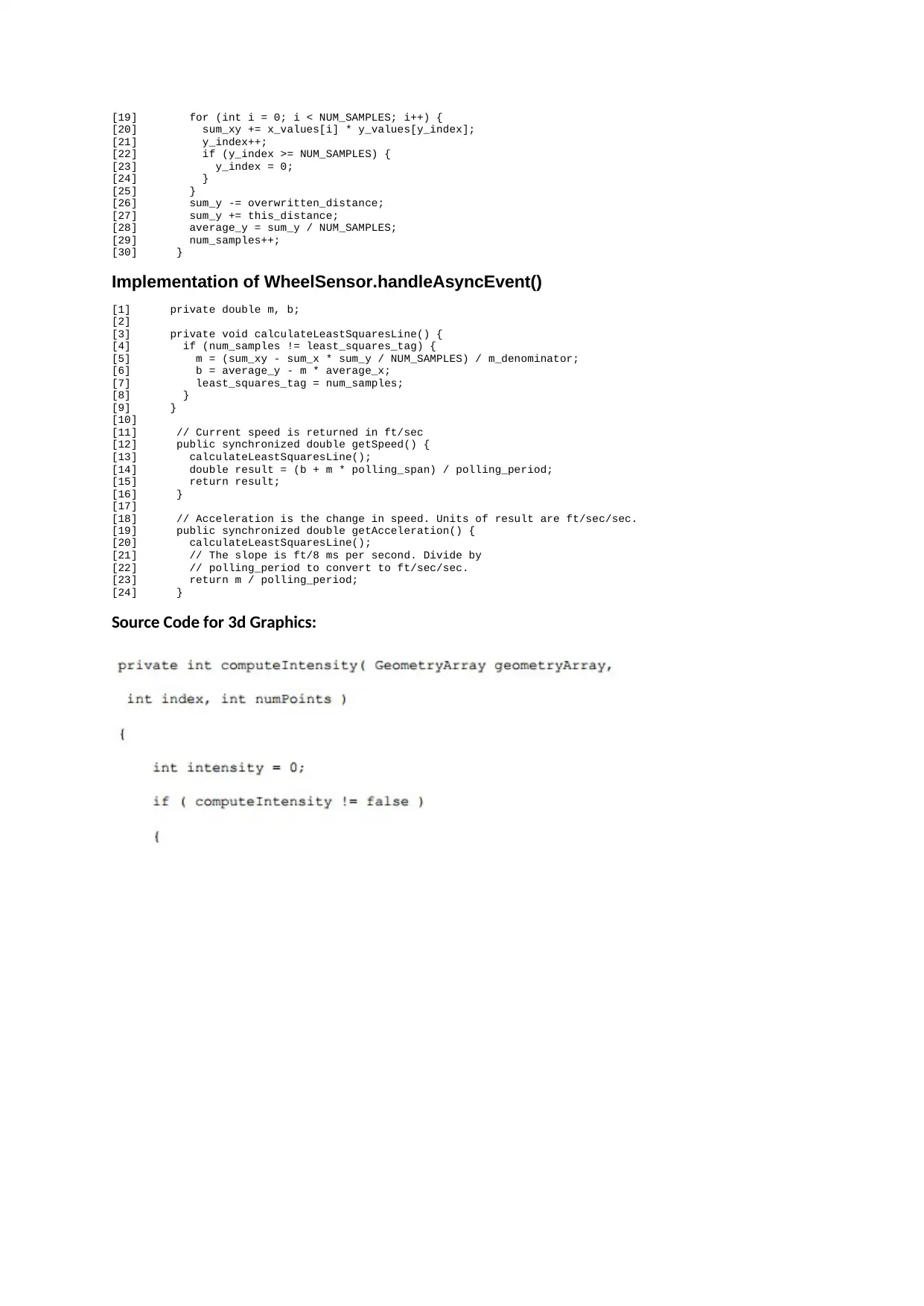
[19] for (int i = 0; i < NUM_SAMPLES; i++) {
[20] sum_xy += x_values[i] * y_values[y_index];
[21] y_index++;
[22] if (y_index >= NUM_SAMPLES) {
[23] y_index = 0;
[24] }
[25] }
[26] sum_y -= overwritten_distance;
[27] sum_y += this_distance;
[28] average_y = sum_y / NUM_SAMPLES;
[29] num_samples++;
[30] }
Implementation of WheelSensor.handleAsyncEvent()
[1] private double m, b;
[2]
[3] private void calculateLeastSquaresLine() {
[4] if (num_samples != least_squares_tag) {
[5] m = (sum_xy - sum_x * sum_y / NUM_SAMPLES) / m_denominator;
[6] b = average_y - m * average_x;
[7] least_squares_tag = num_samples;
[8] }
[9] }
[10]
[11] // Current speed is returned in ft/sec
[12] public synchronized double getSpeed() {
[13] calculateLeastSquaresLine();
[14] double result = (b + m * polling_span) / polling_period;
[15] return result;
[16] }
[17]
[18] // Acceleration is the change in speed. Units of result are ft/sec/sec.
[19] public synchronized double getAcceleration() {
[20] calculateLeastSquaresLine();
[21] // The slope is ft/8 ms per second. Divide by
[22] // polling_period to convert to ft/sec/sec.
[23] return m / polling_period;
[24] }
Source Code for 3d Graphics:
[20] sum_xy += x_values[i] * y_values[y_index];
[21] y_index++;
[22] if (y_index >= NUM_SAMPLES) {
[23] y_index = 0;
[24] }
[25] }
[26] sum_y -= overwritten_distance;
[27] sum_y += this_distance;
[28] average_y = sum_y / NUM_SAMPLES;
[29] num_samples++;
[30] }
Implementation of WheelSensor.handleAsyncEvent()
[1] private double m, b;
[2]
[3] private void calculateLeastSquaresLine() {
[4] if (num_samples != least_squares_tag) {
[5] m = (sum_xy - sum_x * sum_y / NUM_SAMPLES) / m_denominator;
[6] b = average_y - m * average_x;
[7] least_squares_tag = num_samples;
[8] }
[9] }
[10]
[11] // Current speed is returned in ft/sec
[12] public synchronized double getSpeed() {
[13] calculateLeastSquaresLine();
[14] double result = (b + m * polling_span) / polling_period;
[15] return result;
[16] }
[17]
[18] // Acceleration is the change in speed. Units of result are ft/sec/sec.
[19] public synchronized double getAcceleration() {
[20] calculateLeastSquaresLine();
[21] // The slope is ft/8 ms per second. Divide by
[22] // polling_period to convert to ft/sec/sec.
[23] return m / polling_period;
[24] }
Source Code for 3d Graphics:
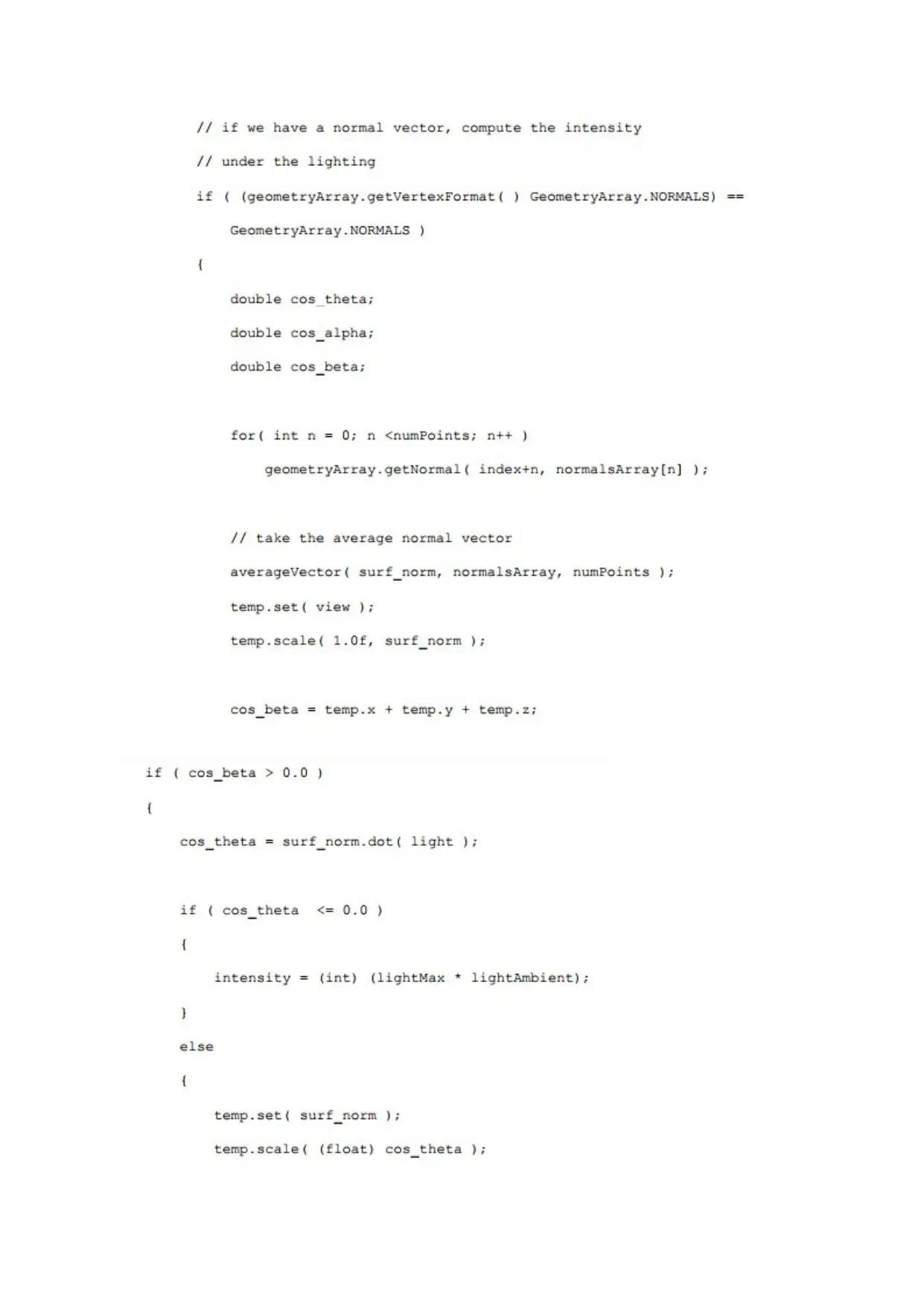
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
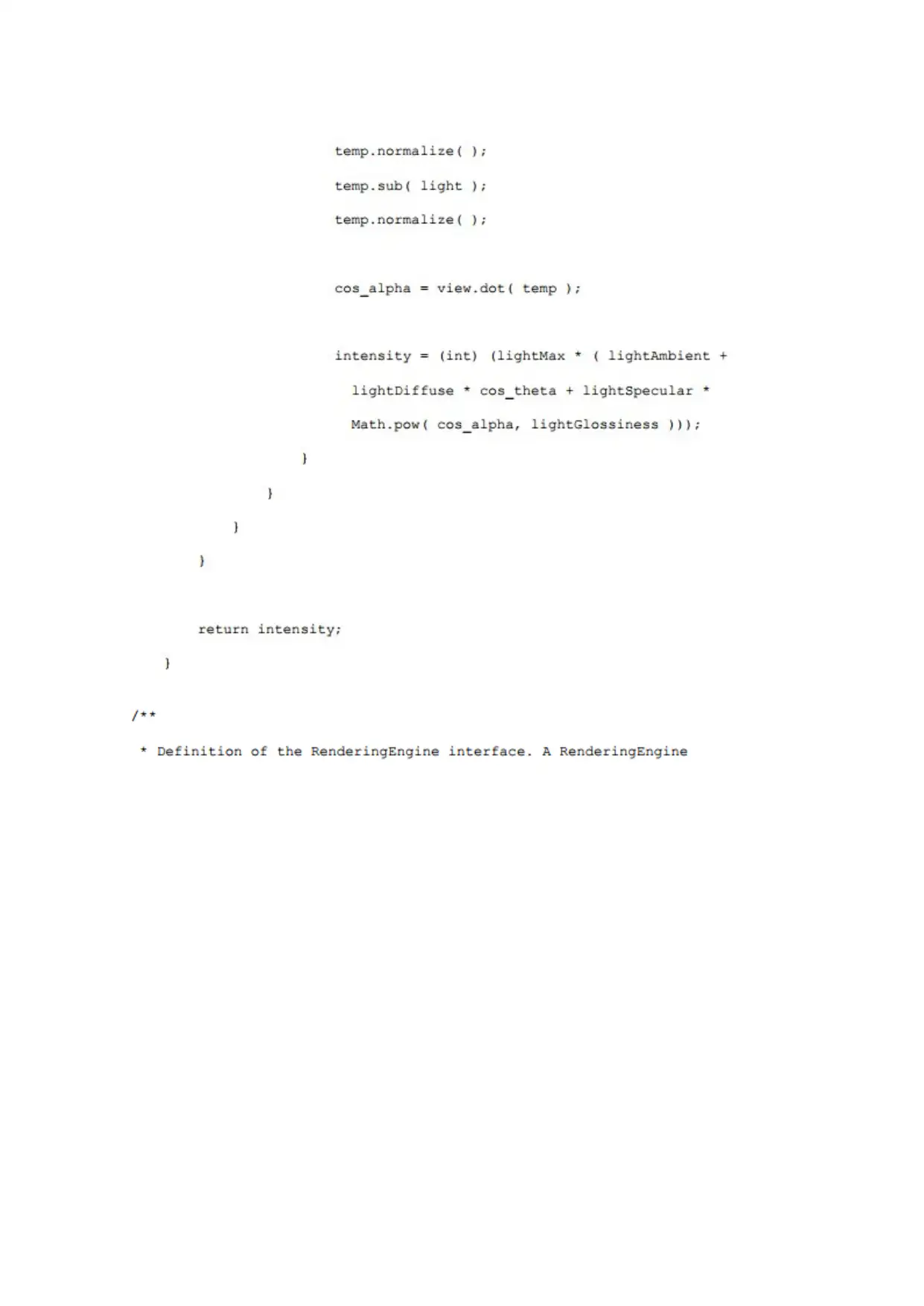
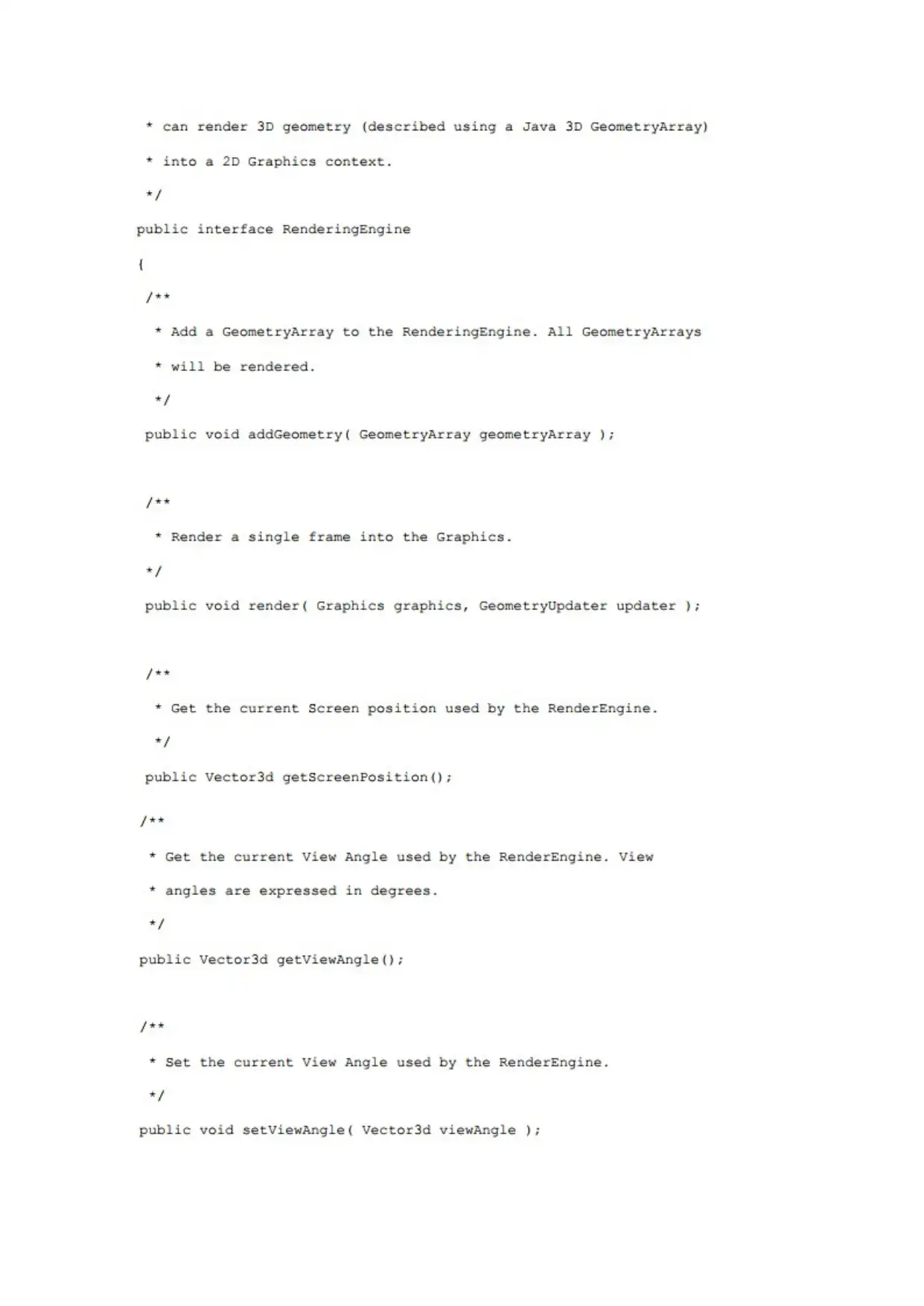
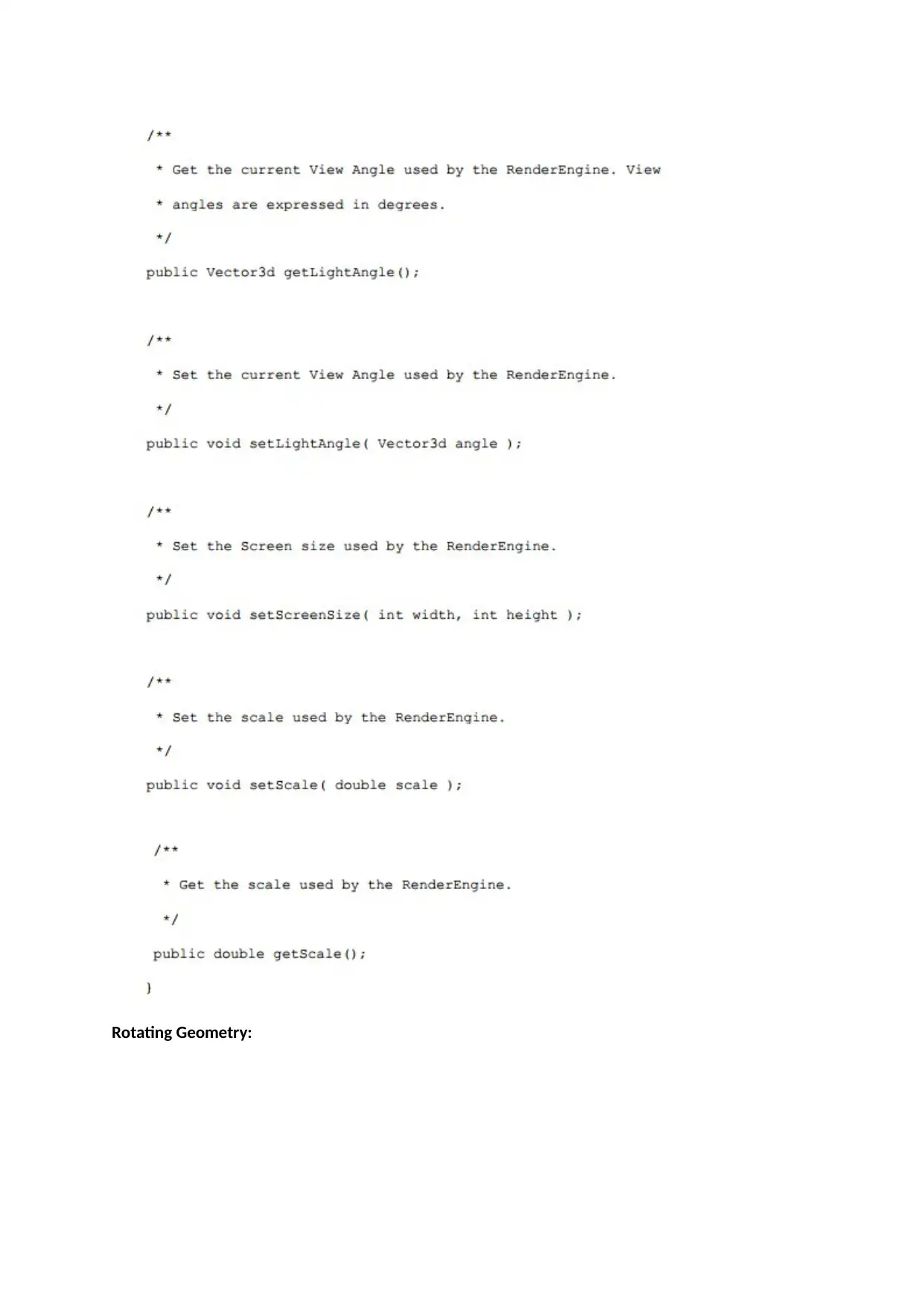
Rotating Geometry:
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
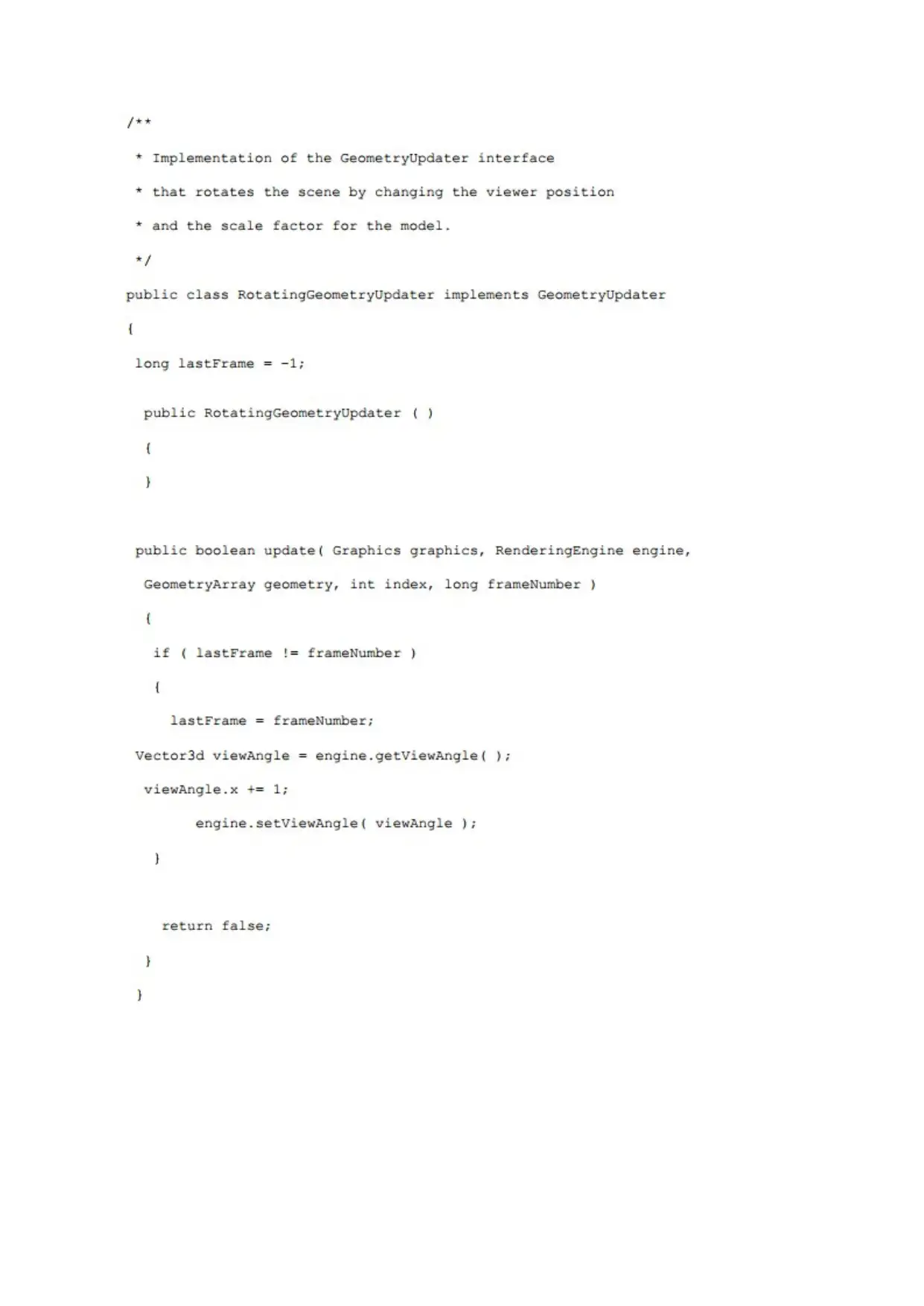
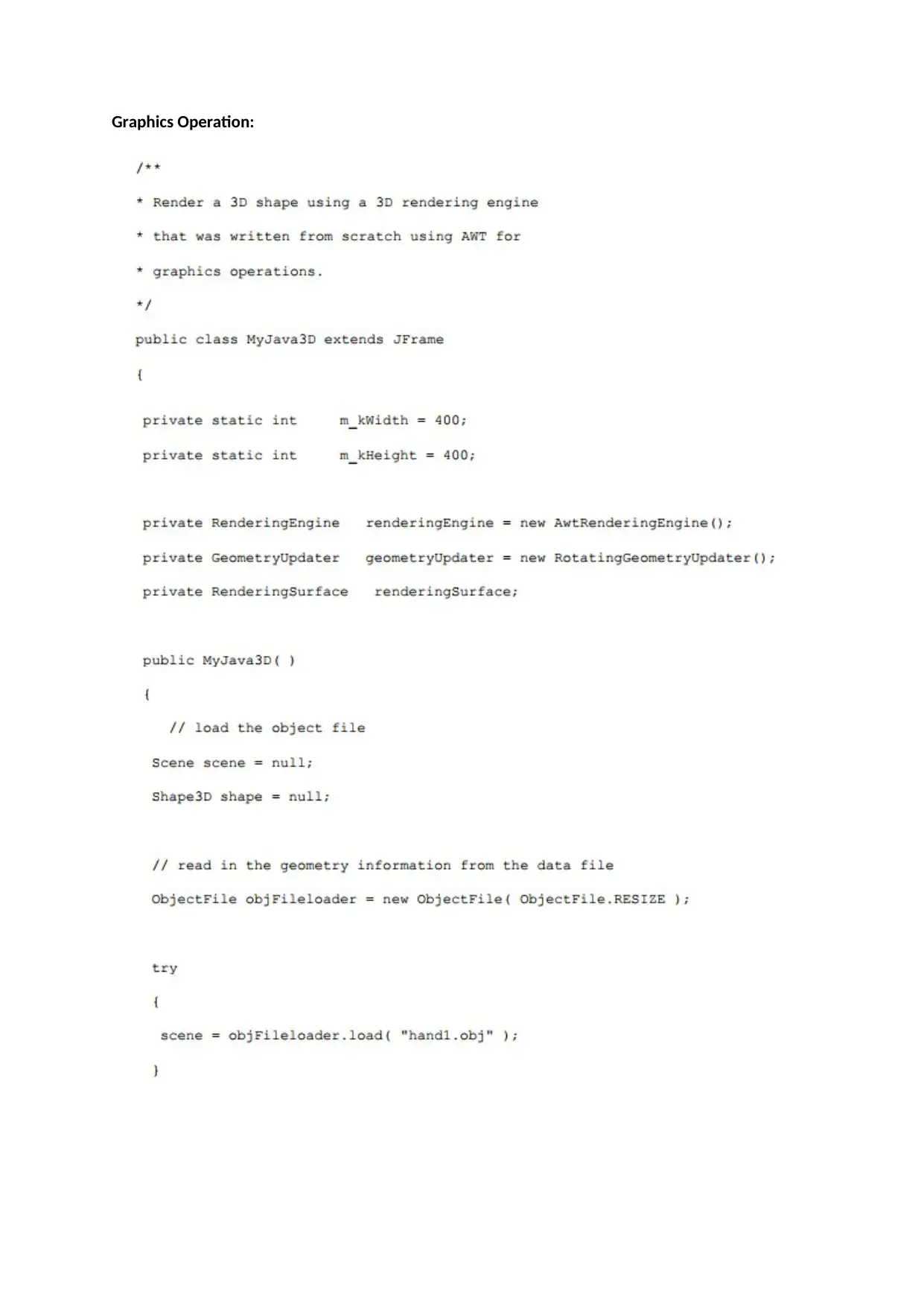
Graphics Operation:
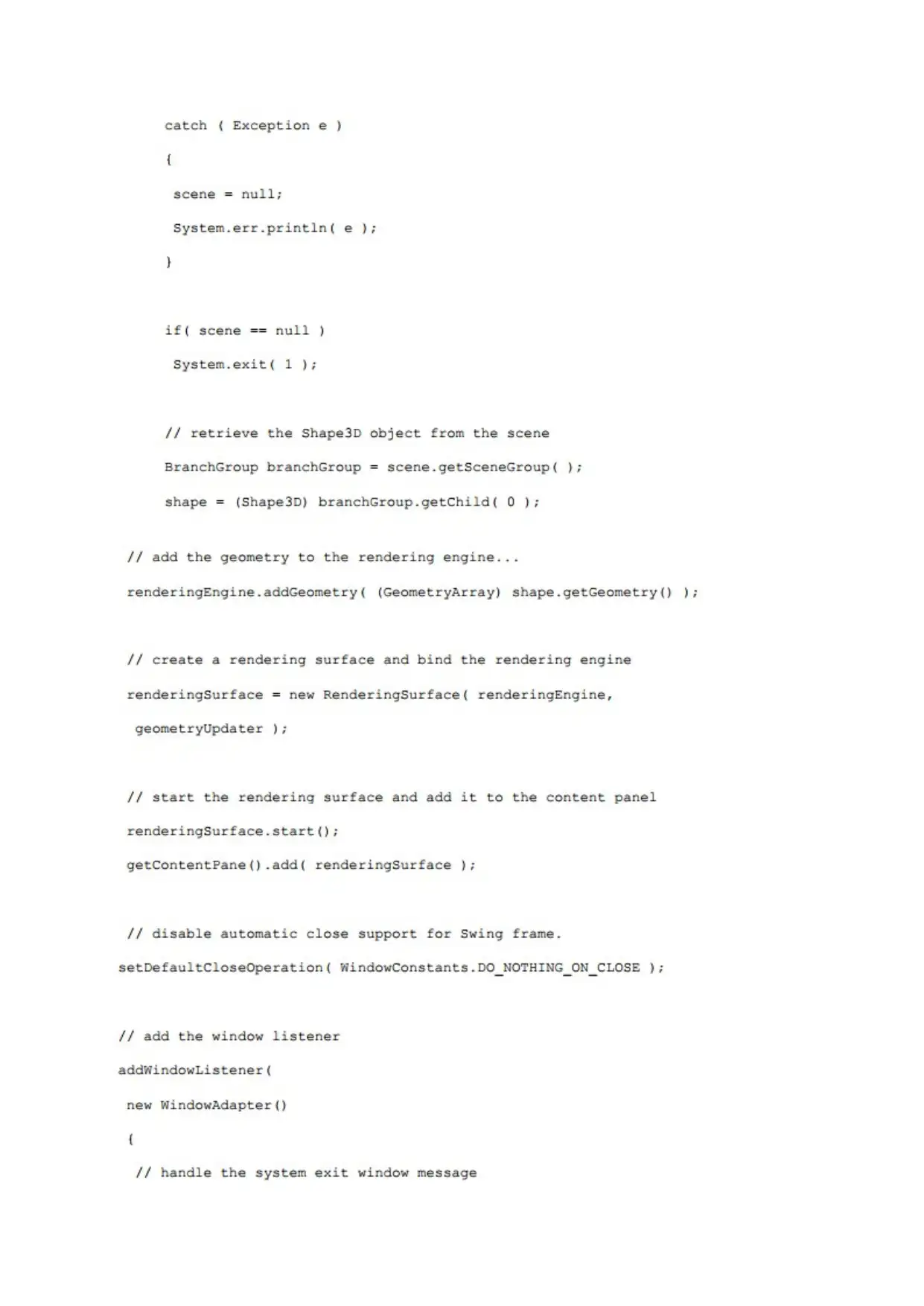
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
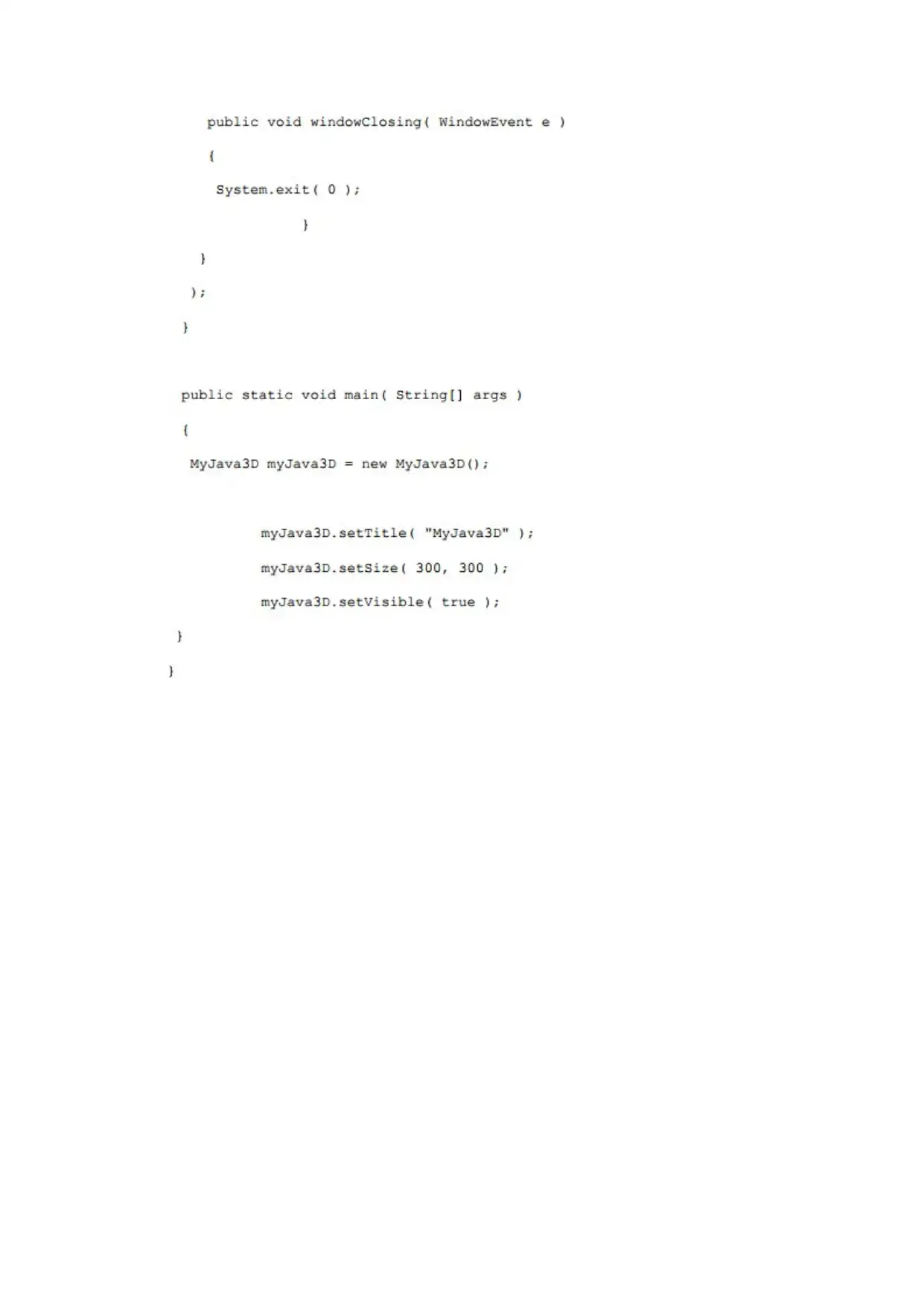
1 out of 14
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
 +13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024  |  Zucol Services PVT LTD  |  All rights reserved.