Viewing Each Album in Detail
VerifiedAdded on 2019/09/19
|4
|1500
|649
Report
AI Summary
The assignment requires you to design an object-oriented album/track class and create a C# application to demonstrate the class's functionality, including creating, modifying, and viewing album and track details. You must use AlbumLength() and AmazonLink() methods in your solution. The submission should be in a zipped folder named 'roll_ number_yourname-ASS-1.zip'.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
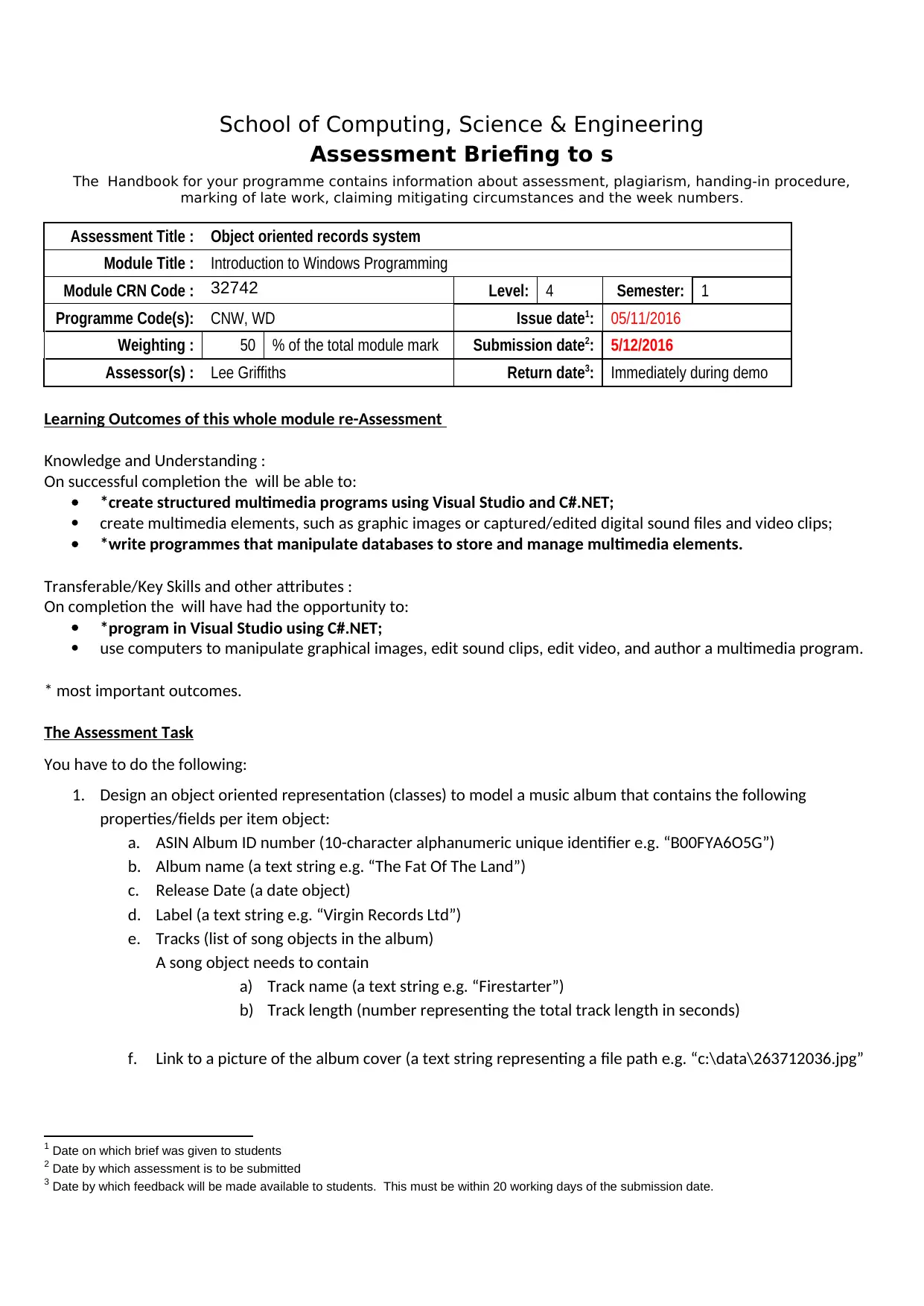
School of Computing, Science & Engineering
Assessment Briefing to s
The Handbook for your programme contains information about assessment, plagiarism, handing-in procedure,
marking of late work, claiming mitigating circumstances and the week numbers.
Assessment Title : Object oriented records system
Module Title : Introduction to Windows Programming
Module CRN Code : 32742 Level: 4 Semester: 1
Programme Code(s): CNW, WD Issue date1: 05/11/2016
Weighting : 50 % of the total module mark Submission date2: 5/12/2016
Assessor(s) : Lee Griffiths Return date3: Immediately during demo
Learning Outcomes of this whole module re-Assessment
Knowledge and Understanding :
On successful completion the will be able to:
*create structured multimedia programs using Visual Studio and C#.NET;
create multimedia elements, such as graphic images or captured/edited digital sound files and video clips;
*write programmes that manipulate databases to store and manage multimedia elements.
Transferable/Key Skills and other attributes :
On completion the will have had the opportunity to:
*program in Visual Studio using C#.NET;
use computers to manipulate graphical images, edit sound clips, edit video, and author a multimedia program.
* most important outcomes.
The Assessment Task
You have to do the following:
1. Design an object oriented representation (classes) to model a music album that contains the following
properties/fields per item object:
a. ASIN Album ID number (10-character alphanumeric unique identifier e.g. “B00FYA6O5G”)
b. Album name (a text string e.g. “The Fat Of The Land”)
c. Release Date (a date object)
d. Label (a text string e.g. “Virgin Records Ltd”)
e. Tracks (list of song objects in the album)
A song object needs to contain
a) Track name (a text string e.g. “Firestarter”)
b) Track length (number representing the total track length in seconds)
f. Link to a picture of the album cover (a text string representing a file path e.g. “c:\data\263712036.jpg”
1 Date on which brief was given to students
2 Date by which assessment is to be submitted
3 Date by which feedback will be made available to students. This must be within 20 working days of the submission date.
Assessment Briefing to s
The Handbook for your programme contains information about assessment, plagiarism, handing-in procedure,
marking of late work, claiming mitigating circumstances and the week numbers.
Assessment Title : Object oriented records system
Module Title : Introduction to Windows Programming
Module CRN Code : 32742 Level: 4 Semester: 1
Programme Code(s): CNW, WD Issue date1: 05/11/2016
Weighting : 50 % of the total module mark Submission date2: 5/12/2016
Assessor(s) : Lee Griffiths Return date3: Immediately during demo
Learning Outcomes of this whole module re-Assessment
Knowledge and Understanding :
On successful completion the will be able to:
*create structured multimedia programs using Visual Studio and C#.NET;
create multimedia elements, such as graphic images or captured/edited digital sound files and video clips;
*write programmes that manipulate databases to store and manage multimedia elements.
Transferable/Key Skills and other attributes :
On completion the will have had the opportunity to:
*program in Visual Studio using C#.NET;
use computers to manipulate graphical images, edit sound clips, edit video, and author a multimedia program.
* most important outcomes.
The Assessment Task
You have to do the following:
1. Design an object oriented representation (classes) to model a music album that contains the following
properties/fields per item object:
a. ASIN Album ID number (10-character alphanumeric unique identifier e.g. “B00FYA6O5G”)
b. Album name (a text string e.g. “The Fat Of The Land”)
c. Release Date (a date object)
d. Label (a text string e.g. “Virgin Records Ltd”)
e. Tracks (list of song objects in the album)
A song object needs to contain
a) Track name (a text string e.g. “Firestarter”)
b) Track length (number representing the total track length in seconds)
f. Link to a picture of the album cover (a text string representing a file path e.g. “c:\data\263712036.jpg”
1 Date on which brief was given to students
2 Date by which assessment is to be submitted
3 Date by which feedback will be made available to students. This must be within 20 working days of the submission date.
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
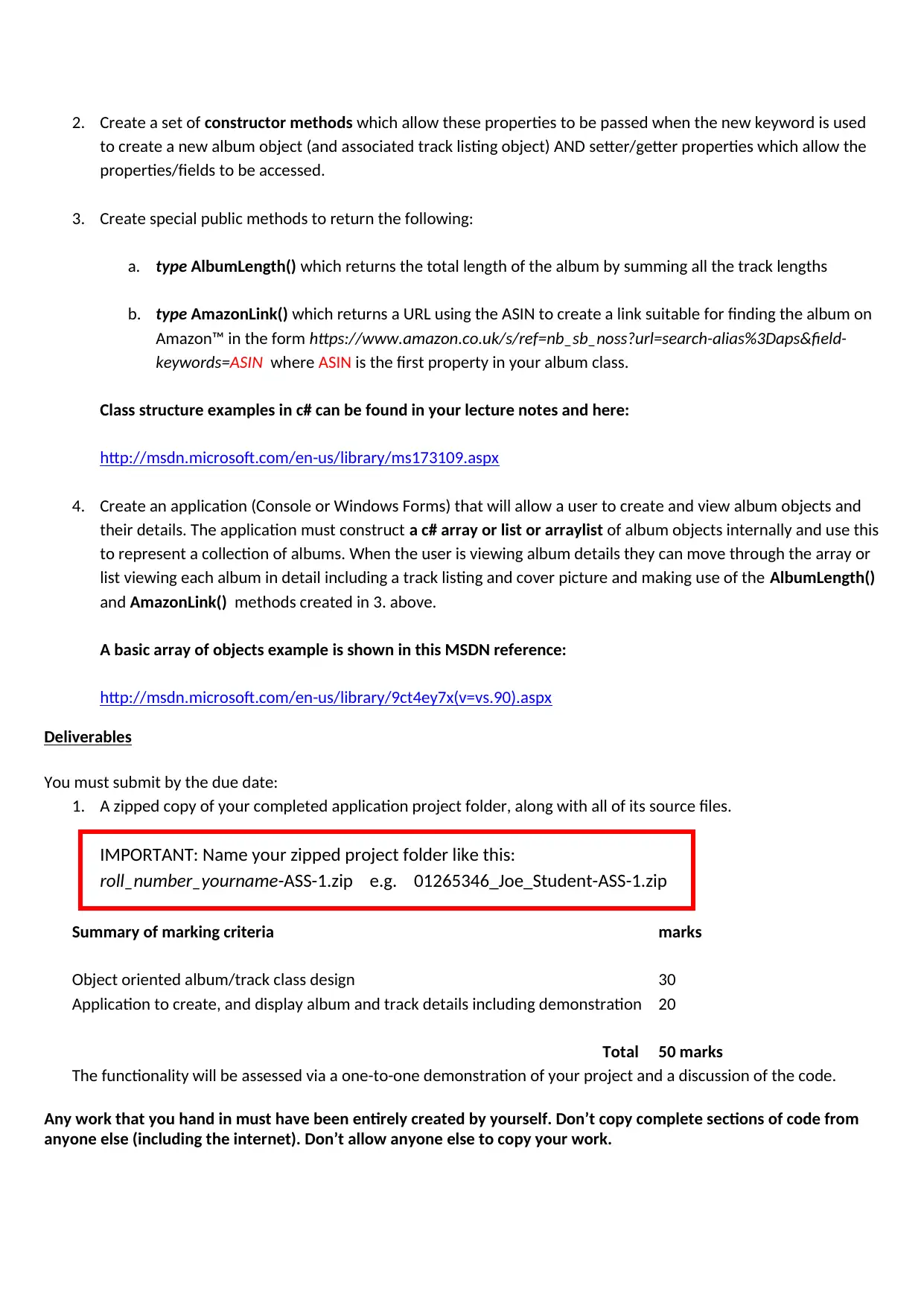
2. Create a set of constructor methods which allow these properties to be passed when the new keyword is used
to create a new album object (and associated track listing object) AND setter/getter properties which allow the
properties/fields to be accessed.
3. Create special public methods to return the following:
a. type AlbumLength() which returns the total length of the album by summing all the track lengths
b. type AmazonLink() which returns a URL using the ASIN to create a link suitable for finding the album on
Amazon™ in the form https://www.amazon.co.uk/s/ref=nb_sb_noss?url=search-alias%3Daps&field-
keywords=ASIN where ASIN is the first property in your album class.
Class structure examples in c# can be found in your lecture notes and here:
http://msdn.microsoft.com/en-us/library/ms173109.aspx
4. Create an application (Console or Windows Forms) that will allow a user to create and view album objects and
their details. The application must construct a c# array or list or arraylist of album objects internally and use this
to represent a collection of albums. When the user is viewing album details they can move through the array or
list viewing each album in detail including a track listing and cover picture and making use of the AlbumLength()
and AmazonLink() methods created in 3. above.
A basic array of objects example is shown in this MSDN reference:
http://msdn.microsoft.com/en-us/library/9ct4ey7x(v=vs.90).aspx
Deliverables
You must submit by the due date:
1. A zipped copy of your completed application project folder, along with all of its source files.
IMPORTANT: Name your zipped project folder like this:
roll_number_yourname-ASS-1.zip e.g. 01265346_Joe_Student-ASS-1.zip
Summary of marking criteria marks
Object oriented album/track class design 30
Application to create, and display album and track details including demonstration 20
Total 50 marks
The functionality will be assessed via a one-to-one demonstration of your project and a discussion of the code.
Any work that you hand in must have been entirely created by yourself. Don’t copy complete sections of code from
anyone else (including the internet). Don’t allow anyone else to copy your work.
to create a new album object (and associated track listing object) AND setter/getter properties which allow the
properties/fields to be accessed.
3. Create special public methods to return the following:
a. type AlbumLength() which returns the total length of the album by summing all the track lengths
b. type AmazonLink() which returns a URL using the ASIN to create a link suitable for finding the album on
Amazon™ in the form https://www.amazon.co.uk/s/ref=nb_sb_noss?url=search-alias%3Daps&field-
keywords=ASIN where ASIN is the first property in your album class.
Class structure examples in c# can be found in your lecture notes and here:
http://msdn.microsoft.com/en-us/library/ms173109.aspx
4. Create an application (Console or Windows Forms) that will allow a user to create and view album objects and
their details. The application must construct a c# array or list or arraylist of album objects internally and use this
to represent a collection of albums. When the user is viewing album details they can move through the array or
list viewing each album in detail including a track listing and cover picture and making use of the AlbumLength()
and AmazonLink() methods created in 3. above.
A basic array of objects example is shown in this MSDN reference:
http://msdn.microsoft.com/en-us/library/9ct4ey7x(v=vs.90).aspx
Deliverables
You must submit by the due date:
1. A zipped copy of your completed application project folder, along with all of its source files.
IMPORTANT: Name your zipped project folder like this:
roll_number_yourname-ASS-1.zip e.g. 01265346_Joe_Student-ASS-1.zip
Summary of marking criteria marks
Object oriented album/track class design 30
Application to create, and display album and track details including demonstration 20
Total 50 marks
The functionality will be assessed via a one-to-one demonstration of your project and a discussion of the code.
Any work that you hand in must have been entirely created by yourself. Don’t copy complete sections of code from
anyone else (including the internet). Don’t allow anyone else to copy your work.
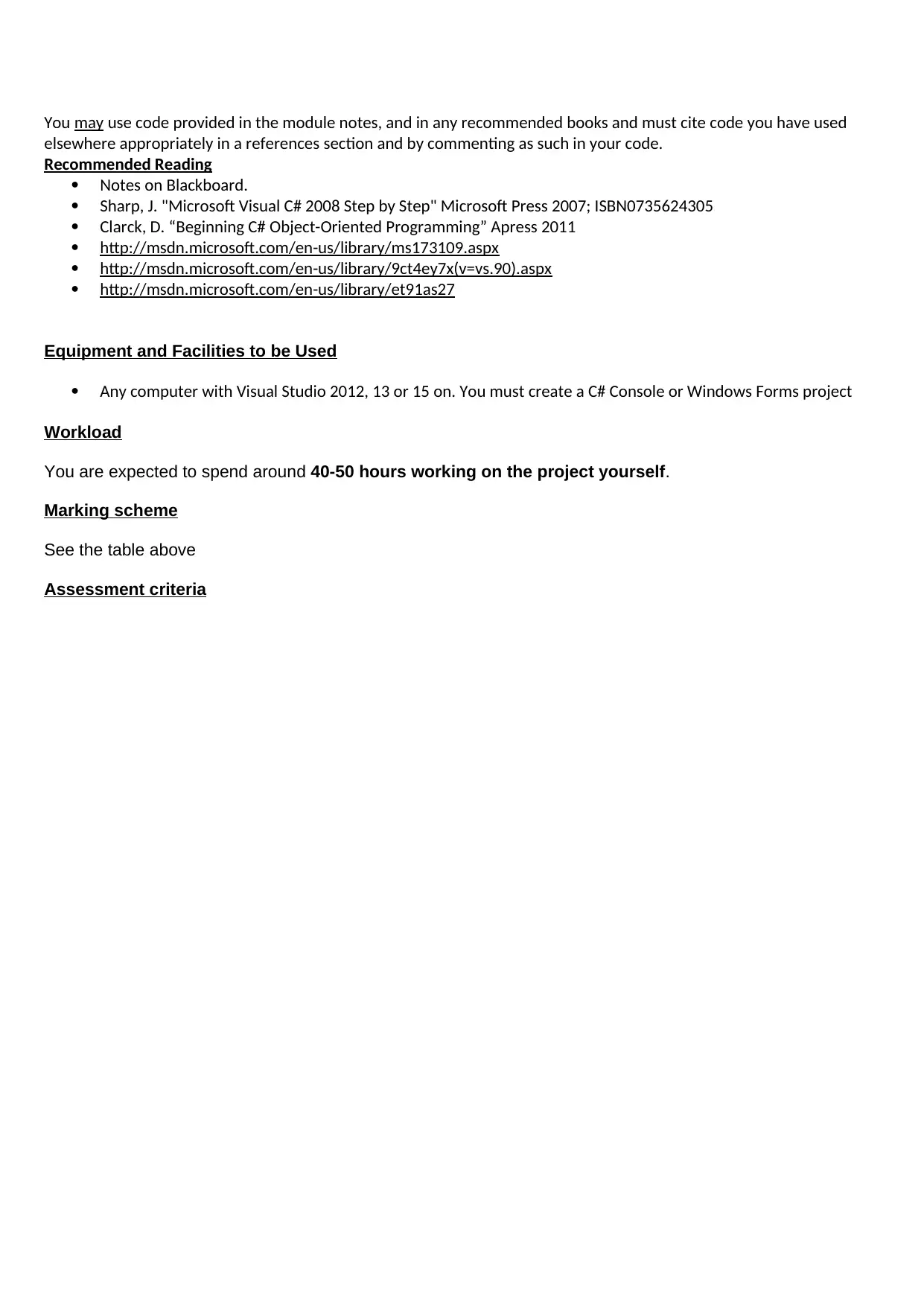
You may use code provided in the module notes, and in any recommended books and must cite code you have used
elsewhere appropriately in a references section and by commenting as such in your code.
Recommended Reading
Notes on Blackboard.
Sharp, J. "Microsoft Visual C# 2008 Step by Step" Microsoft Press 2007; ISBN0735624305
Clarck, D. “Beginning C# Object-Oriented Programming” Apress 2011
http://msdn.microsoft.com/en-us/library/ms173109.aspx
http://msdn.microsoft.com/en-us/library/9ct4ey7x(v=vs.90).aspx
http://msdn.microsoft.com/en-us/library/et91as27
Equipment and Facilities to be Used
Any computer with Visual Studio 2012, 13 or 15 on. You must create a C# Console or Windows Forms project
Workload
You are expected to spend around 40-50 hours working on the project yourself.
Marking scheme
See the table above
Assessment criteria
elsewhere appropriately in a references section and by commenting as such in your code.
Recommended Reading
Notes on Blackboard.
Sharp, J. "Microsoft Visual C# 2008 Step by Step" Microsoft Press 2007; ISBN0735624305
Clarck, D. “Beginning C# Object-Oriented Programming” Apress 2011
http://msdn.microsoft.com/en-us/library/ms173109.aspx
http://msdn.microsoft.com/en-us/library/9ct4ey7x(v=vs.90).aspx
http://msdn.microsoft.com/en-us/library/et91as27
Equipment and Facilities to be Used
Any computer with Visual Studio 2012, 13 or 15 on. You must create a C# Console or Windows Forms project
Workload
You are expected to spend around 40-50 hours working on the project yourself.
Marking scheme
See the table above
Assessment criteria
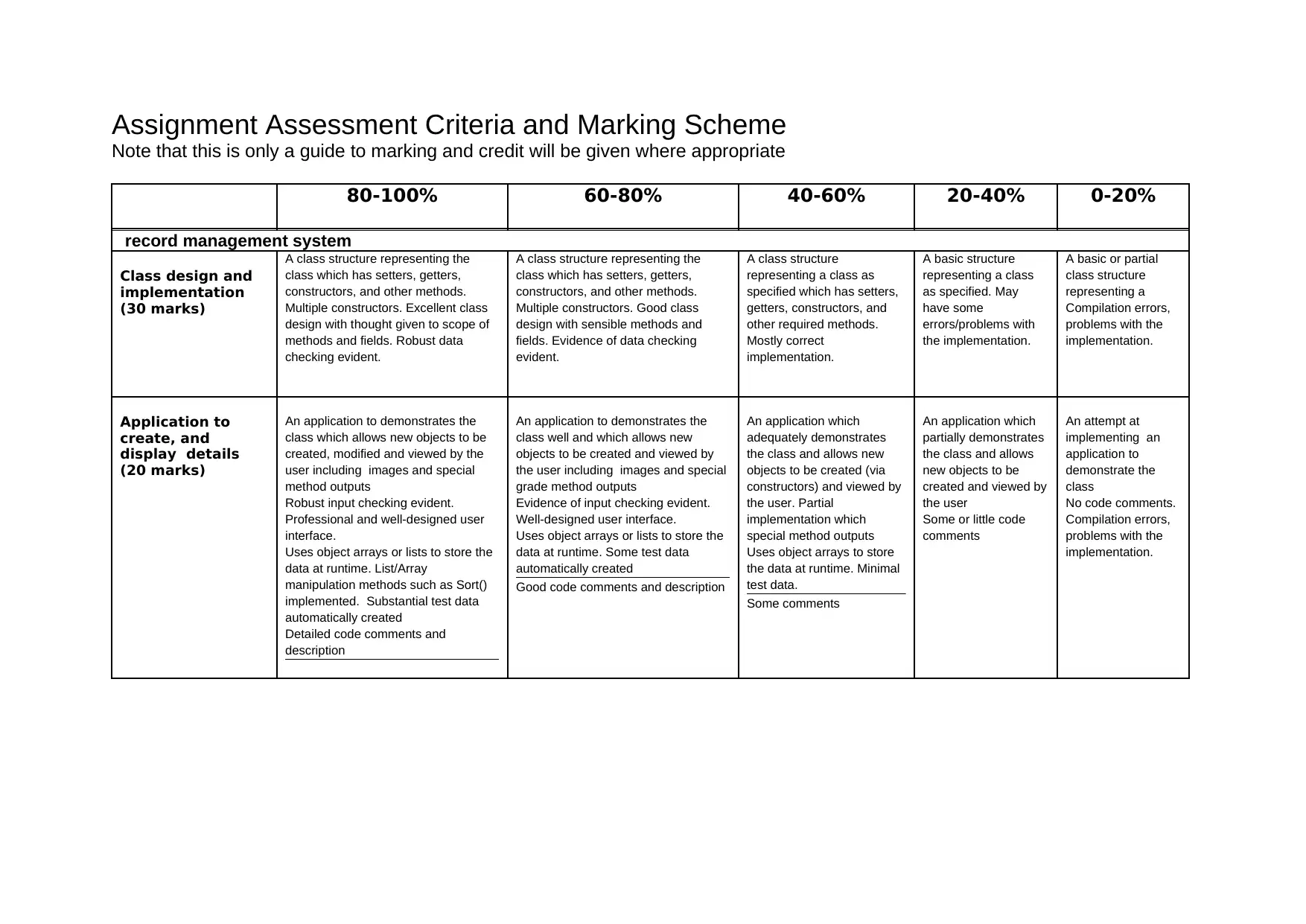
Assignment Assessment Criteria and Marking Scheme
Note that this is only a guide to marking and credit will be given where appropriate
80-100% 60-80% 40-60% 20-40% 0-20%
record management system
Class design and
implementation
(30 marks)
A class structure representing the
class which has setters, getters,
constructors, and other methods.
Multiple constructors. Excellent class
design with thought given to scope of
methods and fields. Robust data
checking evident.
A class structure representing the
class which has setters, getters,
constructors, and other methods.
Multiple constructors. Good class
design with sensible methods and
fields. Evidence of data checking
evident.
A class structure
representing a class as
specified which has setters,
getters, constructors, and
other required methods.
Mostly correct
implementation.
A basic structure
representing a class
as specified. May
have some
errors/problems with
the implementation.
A basic or partial
class structure
representing a
Compilation errors,
problems with the
implementation.
Application to
create, and
display details
(20 marks)
An application to demonstrates the
class which allows new objects to be
created, modified and viewed by the
user including images and special
method outputs
Robust input checking evident.
Professional and well-designed user
interface.
Uses object arrays or lists to store the
data at runtime. List/Array
manipulation methods such as Sort()
implemented. Substantial test data
automatically created
Detailed code comments and
description
An application to demonstrates the
class well and which allows new
objects to be created and viewed by
the user including images and special
grade method outputs
Evidence of input checking evident.
Well-designed user interface.
Uses object arrays or lists to store the
data at runtime. Some test data
automatically created
Good code comments and description
An application which
adequately demonstrates
the class and allows new
objects to be created (via
constructors) and viewed by
the user. Partial
implementation which
special method outputs
Uses object arrays to store
the data at runtime. Minimal
test data.
Some comments
An application which
partially demonstrates
the class and allows
new objects to be
created and viewed by
the user
Some or little code
comments
An attempt at
implementing an
application to
demonstrate the
class
No code comments.
Compilation errors,
problems with the
implementation.
Note that this is only a guide to marking and credit will be given where appropriate
80-100% 60-80% 40-60% 20-40% 0-20%
record management system
Class design and
implementation
(30 marks)
A class structure representing the
class which has setters, getters,
constructors, and other methods.
Multiple constructors. Excellent class
design with thought given to scope of
methods and fields. Robust data
checking evident.
A class structure representing the
class which has setters, getters,
constructors, and other methods.
Multiple constructors. Good class
design with sensible methods and
fields. Evidence of data checking
evident.
A class structure
representing a class as
specified which has setters,
getters, constructors, and
other required methods.
Mostly correct
implementation.
A basic structure
representing a class
as specified. May
have some
errors/problems with
the implementation.
A basic or partial
class structure
representing a
Compilation errors,
problems with the
implementation.
Application to
create, and
display details
(20 marks)
An application to demonstrates the
class which allows new objects to be
created, modified and viewed by the
user including images and special
method outputs
Robust input checking evident.
Professional and well-designed user
interface.
Uses object arrays or lists to store the
data at runtime. List/Array
manipulation methods such as Sort()
implemented. Substantial test data
automatically created
Detailed code comments and
description
An application to demonstrates the
class well and which allows new
objects to be created and viewed by
the user including images and special
grade method outputs
Evidence of input checking evident.
Well-designed user interface.
Uses object arrays or lists to store the
data at runtime. Some test data
automatically created
Good code comments and description
An application which
adequately demonstrates
the class and allows new
objects to be created (via
constructors) and viewed by
the user. Partial
implementation which
special method outputs
Uses object arrays to store
the data at runtime. Minimal
test data.
Some comments
An application which
partially demonstrates
the class and allows
new objects to be
created and viewed by
the user
Some or little code
comments
An attempt at
implementing an
application to
demonstrate the
class
No code comments.
Compilation errors,
problems with the
implementation.
1 out of 4
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.