Introduction to Software Development - Desklib
41 Pages6553 Words184 Views
Added on 2023-06-15
About This Document
This article covers the basics of software development, including code repositories, software layers, algorithms, and Python programming. It also includes practical sessions with tasks and solutions. Module code: CP30681E
Introduction to Software Development - Desklib
Added on 2023-06-15
ShareRelated Documents
INTRODUCTION TO SOFTWARE DEVELOPMENT
MODULE CODE: CP30681E
LOG BOOK
STUDENT ID: XXXXXXXXXXX
SCHOOL OF COMPUTING AND ENGINEERING
UNIVERSITY OF WEST LONDON
MODULE CODE: CP30681E
LOG BOOK
STUDENT ID: XXXXXXXXXXX
SCHOOL OF COMPUTING AND ENGINEERING
UNIVERSITY OF WEST LONDON
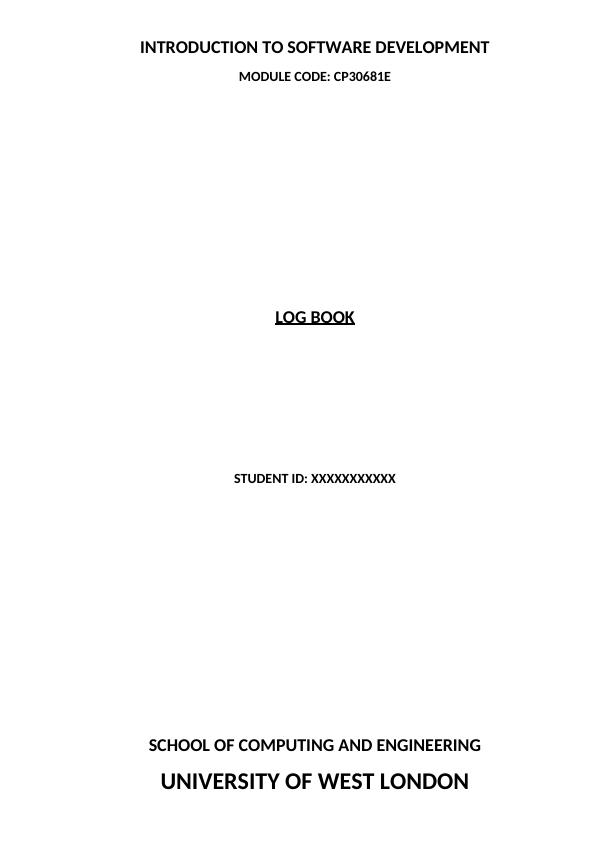
ISD lab sheet week 1
In the first week, we learnt about the various uses of a code repository and other
programming tools. We were also introduced to various software and hardware modules, different
application layers and some crucial programming languages.
Questions:
1. What is a code repository (often also called version control system) used for?
Ans. A code repository is a virtual location where one can store his or her codes and
documentations for various programs or applications. A general repository tool or software
provides the techniques to add and maintain several versions of a program, while it still is in its
development stage. One can easily keep track of the changes that have been made over time.
Here, the online code-repository Github has been used to manage the coding tasks for the
assignment and learn a handful from them.
2. Why is it advantageous to use a code repository?
Ans. The advantages of using code repositories are:
i) It enables the collaboration of various team members to work together in a
project, by communicating easily. This allows the different members to download
the modifications or advancements made to the codes and proceed accordingly.
ii) It helps to backup and restore projects.
iii) As it helps to keep track of all the versions of a project throughout its
development phase, one can easily get back codes from the past and use
accordingly.
iv) The documentation feature helps a lot in the understanding of the projects that
are stored there.
3. Describe the different “layers” of Software that exist on a typical computer and explain why
there are different layers of software.
Ans. The different layers of software, which exist in a typical computer, are System Software
and Application software. The application software level helps the program or software to
interact with the user. The user can put in inputs, view instructions and get output on the
application layer of a software. Whereas, the system layer helps the application layer to
In the first week, we learnt about the various uses of a code repository and other
programming tools. We were also introduced to various software and hardware modules, different
application layers and some crucial programming languages.
Questions:
1. What is a code repository (often also called version control system) used for?
Ans. A code repository is a virtual location where one can store his or her codes and
documentations for various programs or applications. A general repository tool or software
provides the techniques to add and maintain several versions of a program, while it still is in its
development stage. One can easily keep track of the changes that have been made over time.
Here, the online code-repository Github has been used to manage the coding tasks for the
assignment and learn a handful from them.
2. Why is it advantageous to use a code repository?
Ans. The advantages of using code repositories are:
i) It enables the collaboration of various team members to work together in a
project, by communicating easily. This allows the different members to download
the modifications or advancements made to the codes and proceed accordingly.
ii) It helps to backup and restore projects.
iii) As it helps to keep track of all the versions of a project throughout its
development phase, one can easily get back codes from the past and use
accordingly.
iv) The documentation feature helps a lot in the understanding of the projects that
are stored there.
3. Describe the different “layers” of Software that exist on a typical computer and explain why
there are different layers of software.
Ans. The different layers of software, which exist in a typical computer, are System Software
and Application software. The application software level helps the program or software to
interact with the user. The user can put in inputs, view instructions and get output on the
application layer of a software. Whereas, the system layer helps the application layer to
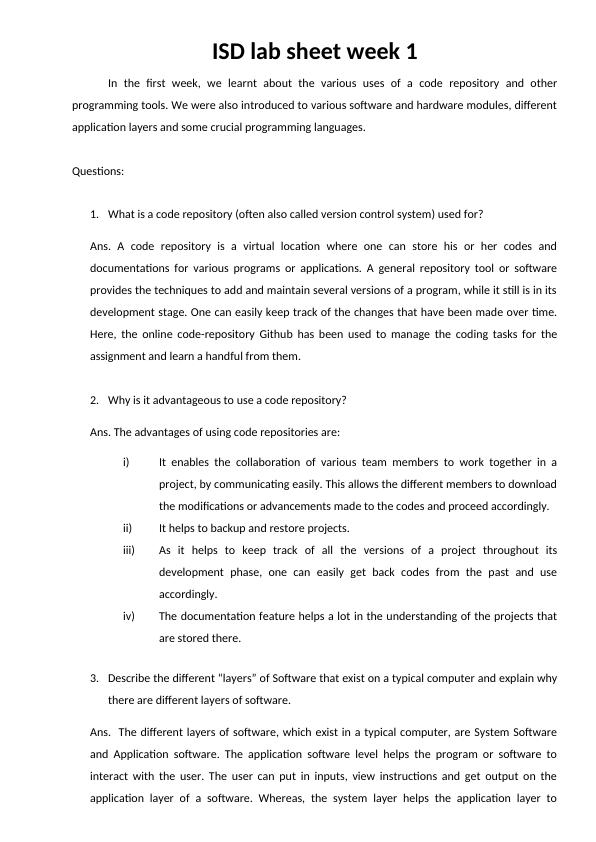
communicate with the hardware. It converts the user’s inputs in the application layer into
machine language and helps the hardware components to compute them. Further, after
computation this data is changed to user readable language and is communicated back to the
application layer as output.
4. Describe what an algorithm is and explain why it is a useful “tool” to translate from a
human level problem (we can think of) to a computer program.
Ans. Algorithm can be defined as a useful technique that can be used to write down the logic
behind the programs in the write order. It lists the correct order of the instructions or steps
that are to be followed by any program in order to produce the desired output. These are
generally written in plain English language or pseudo codes or via flow-chart diagrams.
It is easier to understand. Novice programmers or people who have no knowledge of
programming can easily understand the logics and instructions behind the execution of the
programs.
machine language and helps the hardware components to compute them. Further, after
computation this data is changed to user readable language and is communicated back to the
application layer as output.
4. Describe what an algorithm is and explain why it is a useful “tool” to translate from a
human level problem (we can think of) to a computer program.
Ans. Algorithm can be defined as a useful technique that can be used to write down the logic
behind the programs in the write order. It lists the correct order of the instructions or steps
that are to be followed by any program in order to produce the desired output. These are
generally written in plain English language or pseudo codes or via flow-chart diagrams.
It is easier to understand. Novice programmers or people who have no knowledge of
programming can easily understand the logics and instructions behind the execution of the
programs.
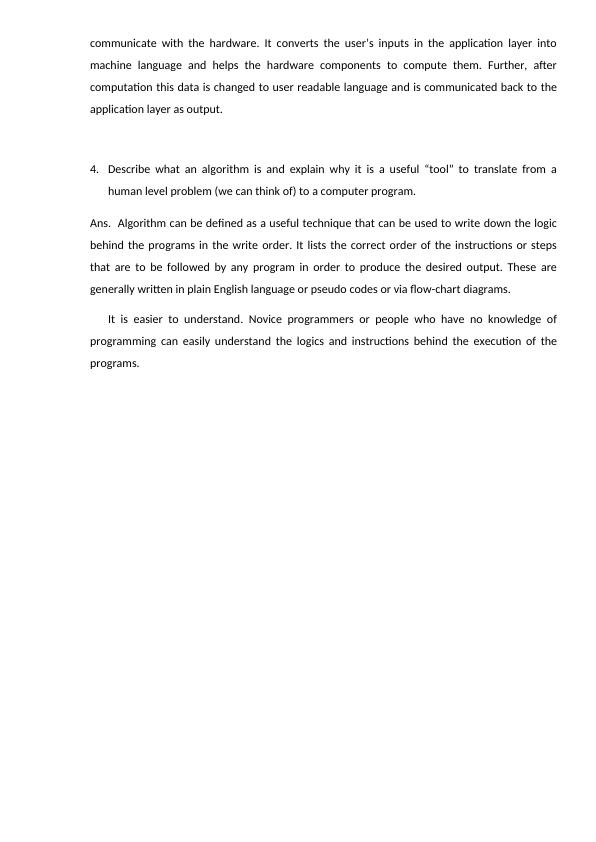
WEEK 2 - PRACTICAL SESSION 2
In week-2, we were taught about the details of computer programming. We came to know
about the keywords, identifiers, variables, operators and syntaxes that enrich any programming
language and helps in performing the desired operations. We were also taught about high-level
programming languages are converted into machine codes for the computer to understand. Then
we learnt about algorithms, their uses and importance in the field of software development.
Compilers and interpreters were among the other new topics that were taught to us. Then we were
introduced to the basics of the PYTHON language, its history, and language shell that is called as
IDLE.
Finally, we were taught about the various PYTHON keywords the correct ways to implement
each in order to solve regular coding problems.
TASKS:
1) Write an algorithm that describes how to make scrambled eggs, try to use control
words, like IF, WHEN, UNTIL, WHILE, WAIT, AND, OR.
Ans. :
1. Take a few number of eggs.
2. Break them and then pour them in a bowl.
3. Put in some black pepper and salt.
4. Batter the egg yolk for a minute inside the bowl until it becomes fluffy.
5. Now, take a clean frying pan.
6. Put oil in it.
7. Heat the pan.
8. Wait for the oil to get heated up.
9. Put the battered egg into the pan
10.While egg is not cooked,
a. stir the eggs slowly in the frying pan
b. End while
11.If any other ingredients are needed
a. Add other ingredients like red chillies, cheese etc.
12.End if
13. Put the scrambled egg in a plate and serve.
2) Is Idle (the Python language shell) an Interpreter or an Compiler or both? Explain
your answer.
Ans. Python’s language shell – IDLE is an interpreter. It takes one command or
instruction at a time, execute it, and retreats to the Development Environment in
In week-2, we were taught about the details of computer programming. We came to know
about the keywords, identifiers, variables, operators and syntaxes that enrich any programming
language and helps in performing the desired operations. We were also taught about high-level
programming languages are converted into machine codes for the computer to understand. Then
we learnt about algorithms, their uses and importance in the field of software development.
Compilers and interpreters were among the other new topics that were taught to us. Then we were
introduced to the basics of the PYTHON language, its history, and language shell that is called as
IDLE.
Finally, we were taught about the various PYTHON keywords the correct ways to implement
each in order to solve regular coding problems.
TASKS:
1) Write an algorithm that describes how to make scrambled eggs, try to use control
words, like IF, WHEN, UNTIL, WHILE, WAIT, AND, OR.
Ans. :
1. Take a few number of eggs.
2. Break them and then pour them in a bowl.
3. Put in some black pepper and salt.
4. Batter the egg yolk for a minute inside the bowl until it becomes fluffy.
5. Now, take a clean frying pan.
6. Put oil in it.
7. Heat the pan.
8. Wait for the oil to get heated up.
9. Put the battered egg into the pan
10.While egg is not cooked,
a. stir the eggs slowly in the frying pan
b. End while
11.If any other ingredients are needed
a. Add other ingredients like red chillies, cheese etc.
12.End if
13. Put the scrambled egg in a plate and serve.
2) Is Idle (the Python language shell) an Interpreter or an Compiler or both? Explain
your answer.
Ans. Python’s language shell – IDLE is an interpreter. It takes one command or
instruction at a time, execute it, and retreats to the Development Environment in
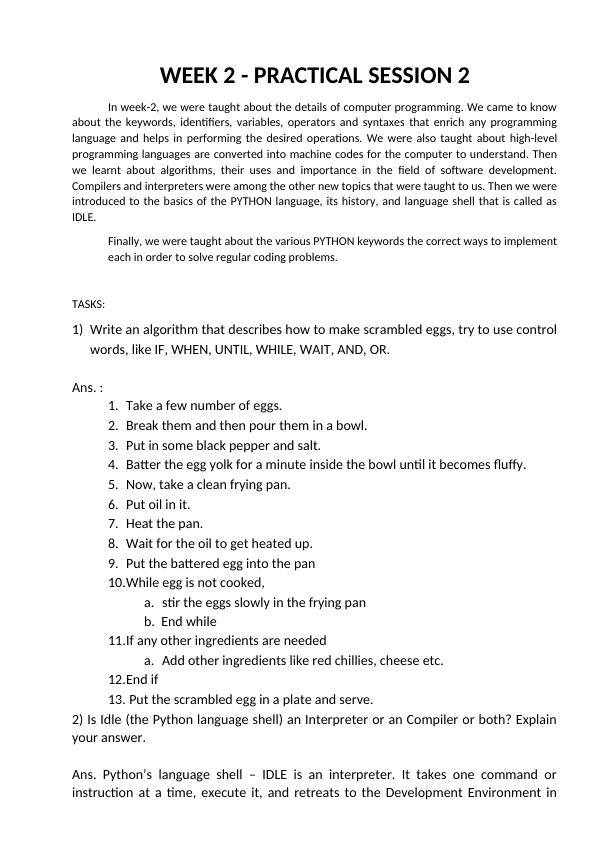
case any error is come across in any of the line. It waits to produce further
compilation report till the earlier error is solved.
3) Write a command in the Idle shell that says “Hello world”
4) Write a program that produces the following output:
Hello World
I am in my ISD class right now
Ans.
Program :
# -*- coding: utf-8 -*-
"""
Created on
@author:
"""
print("Hello World") # print command display output on screen
print("I am in my ISD class right now")
compilation report till the earlier error is solved.
3) Write a command in the Idle shell that says “Hello world”
4) Write a program that produces the following output:
Hello World
I am in my ISD class right now
Ans.
Program :
# -*- coding: utf-8 -*-
"""
Created on
@author:
"""
print("Hello World") # print command display output on screen
print("I am in my ISD class right now")
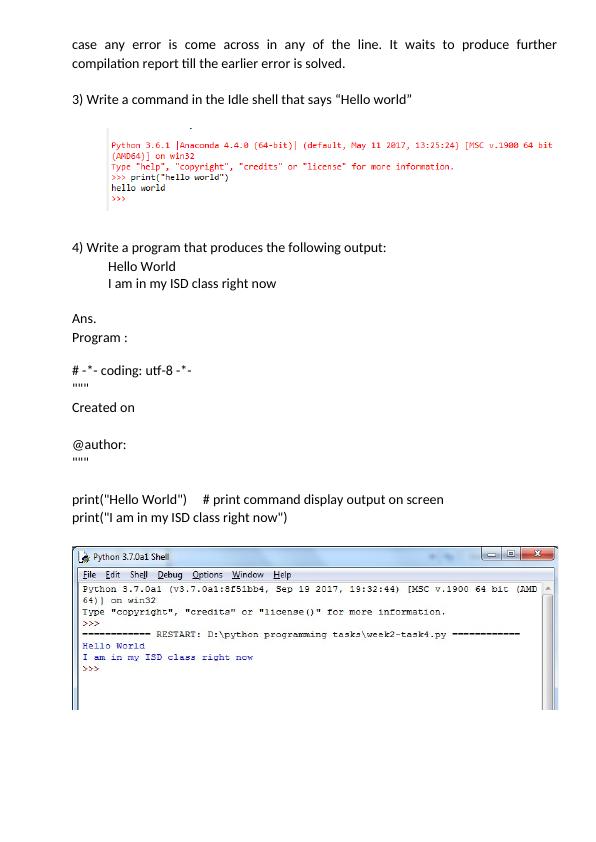
5) Write a program that asks the user for his/her name and produces an output
like:
Hi there, what is your name?
>User input to be read<
Hello
“User name”
How are you?
Ans. :
PROGRAM :
# -*- coding: utf-8 -*-
"""
Created on
@author:
"""
name = input("Hi there, what is your name? ") #input takes input from the keyboard
print("Hello")
print(name)
print("How are you?")
like:
Hi there, what is your name?
>User input to be read<
Hello
“User name”
How are you?
Ans. :
PROGRAM :
# -*- coding: utf-8 -*-
"""
Created on
@author:
"""
name = input("Hi there, what is your name? ") #input takes input from the keyboard
print("Hello")
print(name)
print("How are you?")
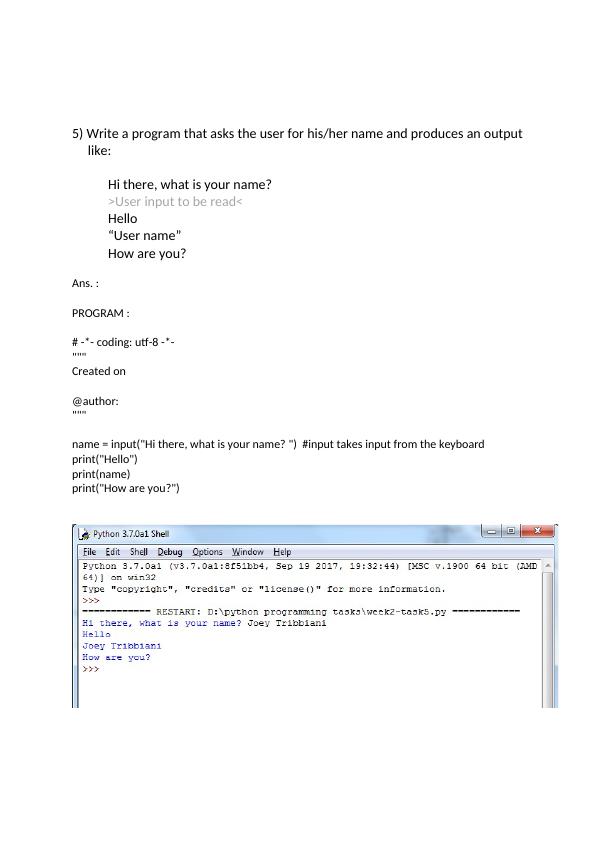
WEEK 3 – PRACTICAL SESSION 3
This week we learnt about the basics of the Python language. We learnt how to use # or hash to
write a single line comment. Then, we were taught to write programs, save them, compile them,
debug the errors and finally execute the programs.
Next we learnt about variable, data types of variable in python and how to convert values to
different data types using type casting commands like int(), float(), str().
Finally we learnt to create account the github.com website and upload programs in it.
TASKS:
1) Write a program that asks for two numbers (Python has all the basic
mathematical functions in place, like +,- etc.), adds them up and displays the
result.
Ans.:
PROGRAM:
# -*- coding: utf-8 -*-
"""
Created on
@author:
"""
number1 = int(input("Enter First number: "))
number2 = int(input("Enter Second number: "))
sum = number1 + number2
print("The sum = ", sum)
This week we learnt about the basics of the Python language. We learnt how to use # or hash to
write a single line comment. Then, we were taught to write programs, save them, compile them,
debug the errors and finally execute the programs.
Next we learnt about variable, data types of variable in python and how to convert values to
different data types using type casting commands like int(), float(), str().
Finally we learnt to create account the github.com website and upload programs in it.
TASKS:
1) Write a program that asks for two numbers (Python has all the basic
mathematical functions in place, like +,- etc.), adds them up and displays the
result.
Ans.:
PROGRAM:
# -*- coding: utf-8 -*-
"""
Created on
@author:
"""
number1 = int(input("Enter First number: "))
number2 = int(input("Enter Second number: "))
sum = number1 + number2
print("The sum = ", sum)
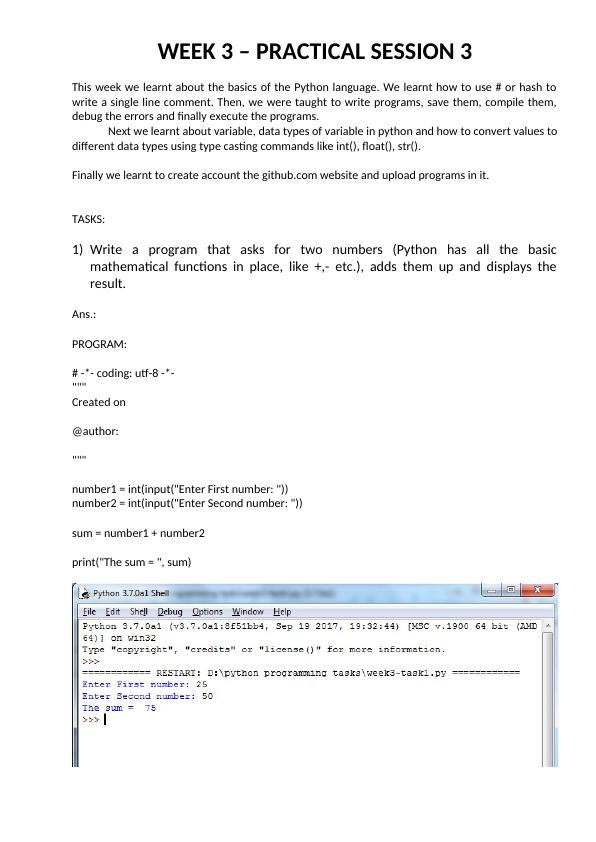
2) Answer the questions by implementing the code and run it.
a) What will the output be from the following code?
num = 4
num*=2
num1=num+2
num1+=3
print(num1)
Ans. Output: 13
b) What do the following lines of code output? Why do they give a different
answer?
print(2 / 3)
print(2 // 3)
Ans.:
0.6666666666666666
0
‘/’ does normal division by producing results in decimal values.
‘//’ does integer division only and therefore returns the floor value of the divided result.
3) All of the variable names below can be used. But which of these is the better
variable name to use?
A a Area AREA area areaOfRectangle AreaOfRectangle
Ans. areaOfRectangle is the best option to use as it uses the valid camel case variable
naming notation. Further, it conveyes the entire task that the variable is ought to
perform.
4) Which of these variables names are not allowed in Python? (More than one might
be wrong.)
apple APPLE Apple2 1Apple account number account_number
account.number accountNumber fred Fred return return_value
5Return GreatBigVariable greatBigVariable great_big_variable
great.big.variable
a) What will the output be from the following code?
num = 4
num*=2
num1=num+2
num1+=3
print(num1)
Ans. Output: 13
b) What do the following lines of code output? Why do they give a different
answer?
print(2 / 3)
print(2 // 3)
Ans.:
0.6666666666666666
0
‘/’ does normal division by producing results in decimal values.
‘//’ does integer division only and therefore returns the floor value of the divided result.
3) All of the variable names below can be used. But which of these is the better
variable name to use?
A a Area AREA area areaOfRectangle AreaOfRectangle
Ans. areaOfRectangle is the best option to use as it uses the valid camel case variable
naming notation. Further, it conveyes the entire task that the variable is ought to
perform.
4) Which of these variables names are not allowed in Python? (More than one might
be wrong.)
apple APPLE Apple2 1Apple account number account_number
account.number accountNumber fred Fred return return_value
5Return GreatBigVariable greatBigVariable great_big_variable
great.big.variable
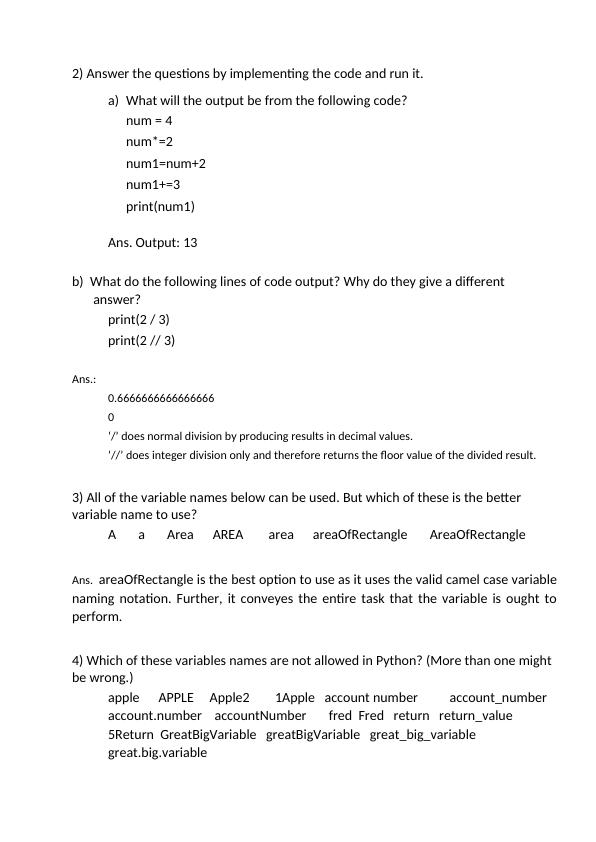
End of preview
Want to access all the pages? Upload your documents or become a member.
Related Documents
CP30681E: Introduction to Software Developmentlg...
|38
|6072
|250
Definition of Algorithm and Process of Building an Applicationlg...
|8
|1804
|83
Lecture on Problem Solving & Flowchartslg...
|36
|1691
|24
IT Support for Business: Hardware, Software and Health Issueslg...
|10
|2040
|421
Assignment - Girvan-Newman Algorithm | Spark Frameworklg...
|5
|1250
|54
(PDF) Creating Your Own Programslg...
|23
|8028
|191