Lab 7.1 - Functions and Pseudocode
Added on 2023-04-20
12 Pages2833 Words111 Views
Starting Out with Programming Logic and Design 1
Practice Exercise 6: Functions
This lab accompanies Chapter 6 of Starting Out with Programming Logic & Design.
Name: ___________________________
Lab 7.1 – Functions and Pseudocode
Critical Review
You have been coding with modules in pseudocode and functions when using Python.
You modules in pseudocode can be made into functions by returning a value.
A function is a special type of module that returns a value back to the part of the program
that called it.
Most programming languages provide a library of prewritten functions that perform
commonly needed tasks.
Library functions are built into the programming language and you can call them as
needed. They are commonly performed tasks.
Help Video: Double click the file to view video
Writing Your Own Function that Returns an Integer
Step 1: A function contains three parts: a header, a body, and a return statement. The
first is a function header which specifies the data type of the value that is to be returned,
the name of the function, and any parameter variables used by the function to accept
arguments. The body is comprised of one or more statements that are executed when the
function is called. In the following space, complete the following: (Reference: Writing
Your Own Functions, page 225).
a. Write a function with the header named addTen.
b. The function will accept an Integer variable named number.
c. The function body will ask the user to enter a number and the add 10 to the
number. The answer will be stored in the variable number.
d. The return statement will return the value of number.
Function a.__________ a.____________ (b.______________)
Display “Enter a number:”
Input c._________________
Set c._____________ = number + 10
Practice Exercise 6: Functions
This lab accompanies Chapter 6 of Starting Out with Programming Logic & Design.
Name: ___________________________
Lab 7.1 – Functions and Pseudocode
Critical Review
You have been coding with modules in pseudocode and functions when using Python.
You modules in pseudocode can be made into functions by returning a value.
A function is a special type of module that returns a value back to the part of the program
that called it.
Most programming languages provide a library of prewritten functions that perform
commonly needed tasks.
Library functions are built into the programming language and you can call them as
needed. They are commonly performed tasks.
Help Video: Double click the file to view video
Writing Your Own Function that Returns an Integer
Step 1: A function contains three parts: a header, a body, and a return statement. The
first is a function header which specifies the data type of the value that is to be returned,
the name of the function, and any parameter variables used by the function to accept
arguments. The body is comprised of one or more statements that are executed when the
function is called. In the following space, complete the following: (Reference: Writing
Your Own Functions, page 225).
a. Write a function with the header named addTen.
b. The function will accept an Integer variable named number.
c. The function body will ask the user to enter a number and the add 10 to the
number. The answer will be stored in the variable number.
d. The return statement will return the value of number.
Function a.__________ a.____________ (b.______________)
Display “Enter a number:”
Input c._________________
Set c._____________ = number + 10
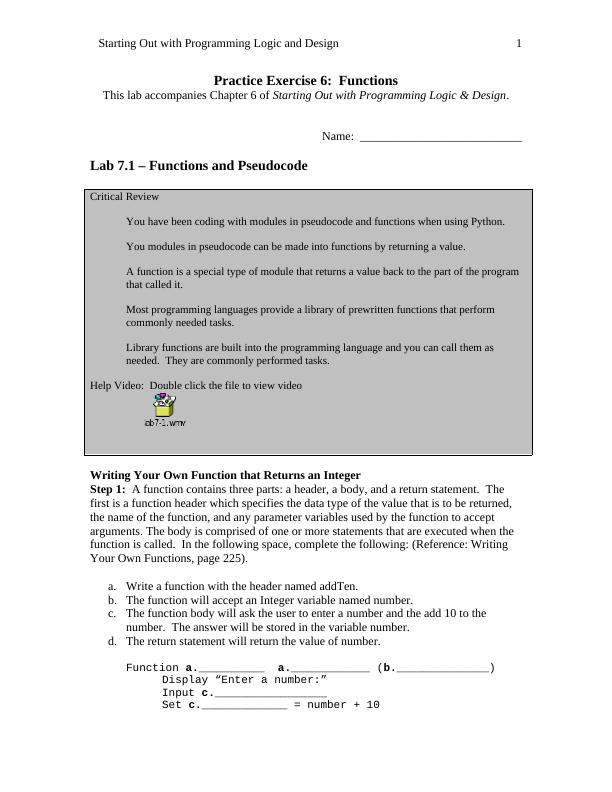
Starting Out with Programming Logic and Design 2
Return d.___________________
Step 2: In the following space, write a function call to your function from Step 1.
Set number = ____________________ (__________________)
Writing Your Own Function that Returns a Boolean Value
Step 1: A Boolean function will either return a true or a false value. You can use these
functions to test a condition. They are useful for simplifying complex conditions that are
tested in decision and repetition structures. In the following space, complete the
following: (Reference: Returning Boolean Values, page 238).
a. Write a function with the header named gender.
b. The function will accept a Boolean variable named answer.
c. The function body will ask the user to enter their gender into the variable type and
then determine if they are male or female with an if statement.
d. The return statement will return the value of answer.
Function a.__________ a.____________ (b.______________)
Declare String type
Display “Enter your gender (male or female):”
Input c._________________
If (c.___________ == “male”) then
answer = False
Else
answer = True
End If
Return d.___________________
Step 2: In the following space, write a function call to your function from Step 1.
Set answer = ____________________ (__________________)
Using Mathematical Library Function: sqrt
Step 1: The sqrt function accepts an argument and returns the square root of the
argument. In the following space, complete the following: (Reference: The sqrt
Function, page 240).
a. Declare a variable named myNumber and a variable named squareRoot of the
data type Real.
b. Ask the user to enter a number of which they want to find the square root. Store
the input in myNumber.
c. Call the sqrt function to determine the square root of myNumber.
d. Display the square root to the screen.
Declare Integer a.___________________
Declare Real a.______________________
Return d.___________________
Step 2: In the following space, write a function call to your function from Step 1.
Set number = ____________________ (__________________)
Writing Your Own Function that Returns a Boolean Value
Step 1: A Boolean function will either return a true or a false value. You can use these
functions to test a condition. They are useful for simplifying complex conditions that are
tested in decision and repetition structures. In the following space, complete the
following: (Reference: Returning Boolean Values, page 238).
a. Write a function with the header named gender.
b. The function will accept a Boolean variable named answer.
c. The function body will ask the user to enter their gender into the variable type and
then determine if they are male or female with an if statement.
d. The return statement will return the value of answer.
Function a.__________ a.____________ (b.______________)
Declare String type
Display “Enter your gender (male or female):”
Input c._________________
If (c.___________ == “male”) then
answer = False
Else
answer = True
End If
Return d.___________________
Step 2: In the following space, write a function call to your function from Step 1.
Set answer = ____________________ (__________________)
Using Mathematical Library Function: sqrt
Step 1: The sqrt function accepts an argument and returns the square root of the
argument. In the following space, complete the following: (Reference: The sqrt
Function, page 240).
a. Declare a variable named myNumber and a variable named squareRoot of the
data type Real.
b. Ask the user to enter a number of which they want to find the square root. Store
the input in myNumber.
c. Call the sqrt function to determine the square root of myNumber.
d. Display the square root to the screen.
Declare Integer a.___________________
Declare Real a.______________________
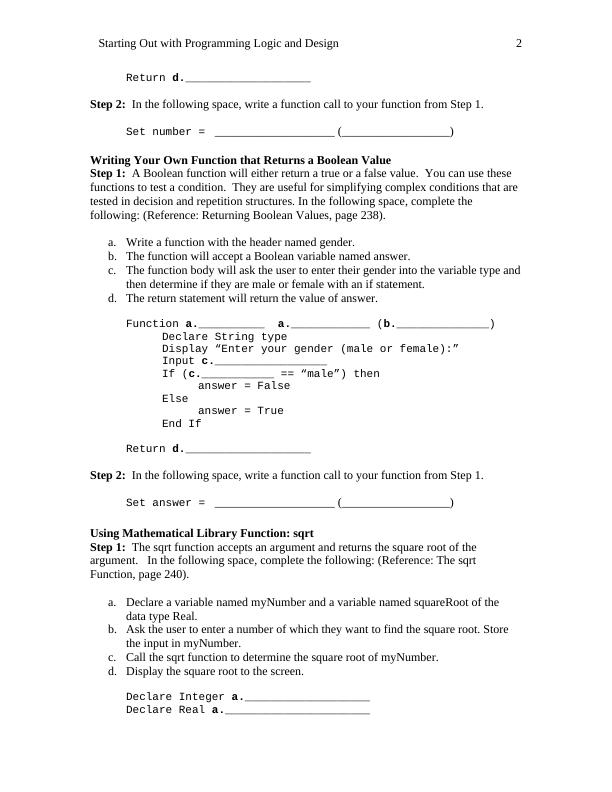
Starting Out with Programming Logic and Design 3
Display “Enter a number:”
Input b._________________________
Set c.______________ = _______________________
Display “The square root is”, d.____________________
Using Formatting Functions
Step 1: Most languages provide one or more functions that format numbers in some
way. A common use of formatting functions is to format numbers as currency amounts.
While a specific programming language will have its own name for formatting currency,
use the function currencyFormat for pseudocode. In the following space, complete the
following: (Reference: Formatting Functions, page 246).
a. Declare a variable named subtotal, a constant variable named tax set to the rate
of .06, and a variable named total.
b. Ask the user to enter the subtotal. Store the input in subtotal.
c. Calculate the total as subtotal + subtotal * tax.
d. Make a call to the currencyFormat function and pass it total. Since you are not
displaying it on this line, simply set the return value to total.
e. Display the total to the screen.
Declare Real a.___________________
Declare Constant Real a.______________________
Declare Real a.____________________________
Display “Enter the subtotal:”
Input b._________________________
Set c.______________ = _______________________
total = d.___________________(________________)
Display “The total is $”, e.____________________
Display “Enter a number:”
Input b._________________________
Set c.______________ = _______________________
Display “The square root is”, d.____________________
Using Formatting Functions
Step 1: Most languages provide one or more functions that format numbers in some
way. A common use of formatting functions is to format numbers as currency amounts.
While a specific programming language will have its own name for formatting currency,
use the function currencyFormat for pseudocode. In the following space, complete the
following: (Reference: Formatting Functions, page 246).
a. Declare a variable named subtotal, a constant variable named tax set to the rate
of .06, and a variable named total.
b. Ask the user to enter the subtotal. Store the input in subtotal.
c. Calculate the total as subtotal + subtotal * tax.
d. Make a call to the currencyFormat function and pass it total. Since you are not
displaying it on this line, simply set the return value to total.
e. Display the total to the screen.
Declare Real a.___________________
Declare Constant Real a.______________________
Declare Real a.____________________________
Display “Enter the subtotal:”
Input b._________________________
Set c.______________ = _______________________
total = d.___________________(________________)
Display “The total is $”, e.____________________
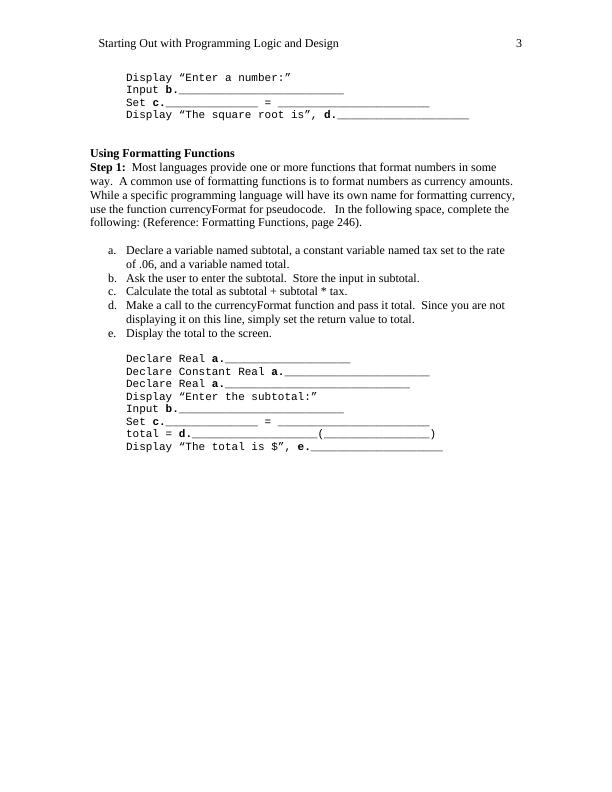
Starting Out with Programming Logic and Design 4
Lab 7.2 – Functions and Flowcharts
Critical Review
When creating a flowchart for a program that has functions, draw a separate flowchart for
each function.
The starting terminal symbol usually shows the name of the function, along with any
parameters that the function has.
The ending terminal symbol reads Return, followed by the value or expression being
returned.
In Raptor, there are built-in procedures and functions that perform a wide variety of tasks
on the programmer's behalf, saving development time and reducing the chance for errors.
Raptor's buily-in functions can return values, but modules made by the user do not have
that ability.
Raptor has the following built-in functions.
basic math: rem, mod, sqrt, log, abs, ceiling, floor
trigonometry: sin, cos, tan, cot, arcsin, arcos, arctan, arccot
miscellaneous: random, Length_of
If you want to learn what each of these functions do, use the Help menu in Raptor and
search for the function name.
While ceiling and floor round a number to the nearest integer, there is no function
in Raptor that will round a number just to two decimal places.
The random function in Raptor takes no arguments. To generate a random integer from
1 to n, use floor((random*n) + 1). For example, you can simulate the roll of a
die (random number from 1 to 6) with floor((random * 6) + 1).
Help Video: Double click the file to view video
This lab requires you to create the flowchart from page 222 on Using Random Numbers
using the RANDOM function. Use an application such as Raptor or Visio.
Step 1: Start by reading the pseudocode on page 221 and 222 of your textbook on Using
Random Numbers. In addition to simply displaying the random values, your program
will also meet the following requirements:
Allow the two players of the dice game to enter their names in variables named
playerOne and playerTwo.
Lab 7.2 – Functions and Flowcharts
Critical Review
When creating a flowchart for a program that has functions, draw a separate flowchart for
each function.
The starting terminal symbol usually shows the name of the function, along with any
parameters that the function has.
The ending terminal symbol reads Return, followed by the value or expression being
returned.
In Raptor, there are built-in procedures and functions that perform a wide variety of tasks
on the programmer's behalf, saving development time and reducing the chance for errors.
Raptor's buily-in functions can return values, but modules made by the user do not have
that ability.
Raptor has the following built-in functions.
basic math: rem, mod, sqrt, log, abs, ceiling, floor
trigonometry: sin, cos, tan, cot, arcsin, arcos, arctan, arccot
miscellaneous: random, Length_of
If you want to learn what each of these functions do, use the Help menu in Raptor and
search for the function name.
While ceiling and floor round a number to the nearest integer, there is no function
in Raptor that will round a number just to two decimal places.
The random function in Raptor takes no arguments. To generate a random integer from
1 to n, use floor((random*n) + 1). For example, you can simulate the roll of a
die (random number from 1 to 6) with floor((random * 6) + 1).
Help Video: Double click the file to view video
This lab requires you to create the flowchart from page 222 on Using Random Numbers
using the RANDOM function. Use an application such as Raptor or Visio.
Step 1: Start by reading the pseudocode on page 221 and 222 of your textbook on Using
Random Numbers. In addition to simply displaying the random values, your program
will also meet the following requirements:
Allow the two players of the dice game to enter their names in variables named
playerOne and playerTwo.
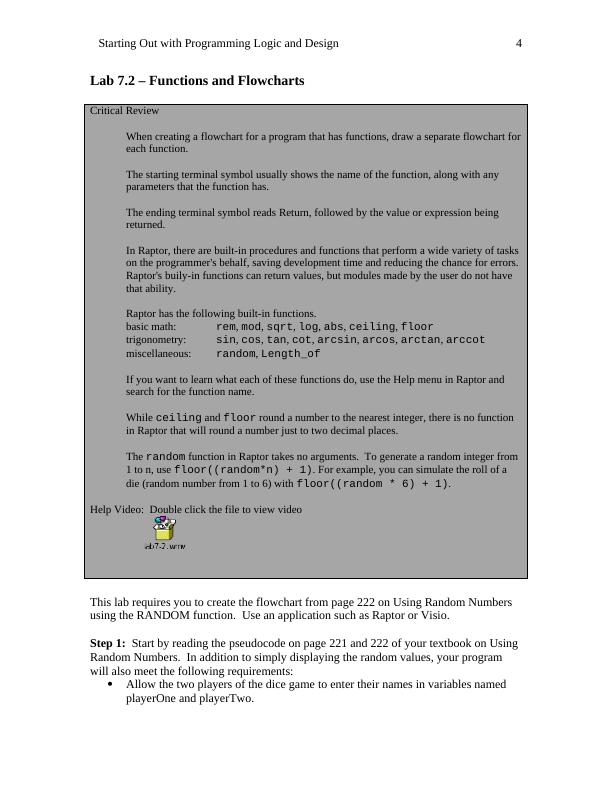
End of preview
Want to access all the pages? Upload your documents or become a member.
Related Documents
Fundamental Data Type Used to Build a Linked Listlg...
|2
|448
|58
C++ Programming Tasks for Desklib Online Librarylg...
|20
|976
|199
Designing and Implementing Algorithms for Media Management and Mutable String ADTlg...
|4
|1019
|453
Programming Language | Question and Answerlg...
|7
|889
|19
ROBOTICS C++. 1. Robotics C++ Name of Student Institutilg...
|5
|482
|24
ITECH1400 – Foundations of Programminglg...
|7
|1303
|94