Robot Class Design and Implementation
VerifiedAdded on 2020/04/21
|6
|864
|309
AI Summary
This assignment focuses on designing and implementing an abstract Robot class in Java. The Robot class incorporates methods for rotating and grasping arms using subclasses with specific implementations. The example highlights the use of abstract methods to define common functionalities that must be implemented by concrete robot types.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
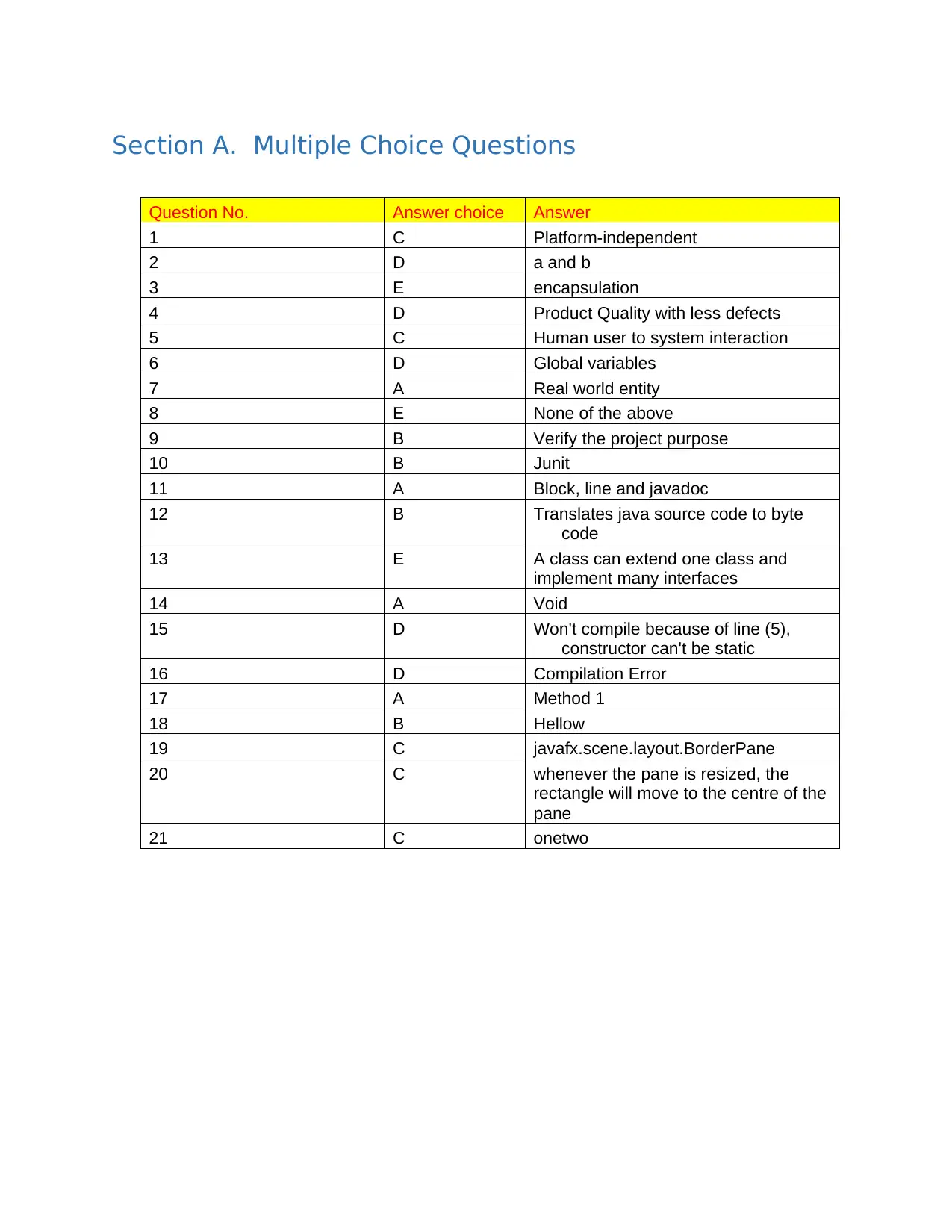
Section A. Multiple Choice Questions
Question No. Answer choice Answer
1 C Platform-independent
2 D a and b
3 E encapsulation
4 D Product Quality with less defects
5 C Human user to system interaction
6 D Global variables
7 A Real world entity
8 E None of the above
9 B Verify the project purpose
10 B Junit
11 A Block, line and javadoc
12 B Translates java source code to byte
code
13 E A class can extend one class and
implement many interfaces
14 A Void
15 D Won't compile because of line (5),
constructor can't be static
16 D Compilation Error
17 A Method 1
18 B Hellow
19 C javafx.scene.layout.BorderPane
20 C whenever the pane is resized, the
rectangle will move to the centre of the
pane
21 C onetwo
Question No. Answer choice Answer
1 C Platform-independent
2 D a and b
3 E encapsulation
4 D Product Quality with less defects
5 C Human user to system interaction
6 D Global variables
7 A Real world entity
8 E None of the above
9 B Verify the project purpose
10 B Junit
11 A Block, line and javadoc
12 B Translates java source code to byte
code
13 E A class can extend one class and
implement many interfaces
14 A Void
15 D Won't compile because of line (5),
constructor can't be static
16 D Compilation Error
17 A Method 1
18 B Hellow
19 C javafx.scene.layout.BorderPane
20 C whenever the pane is resized, the
rectangle will move to the centre of the
pane
21 C onetwo
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
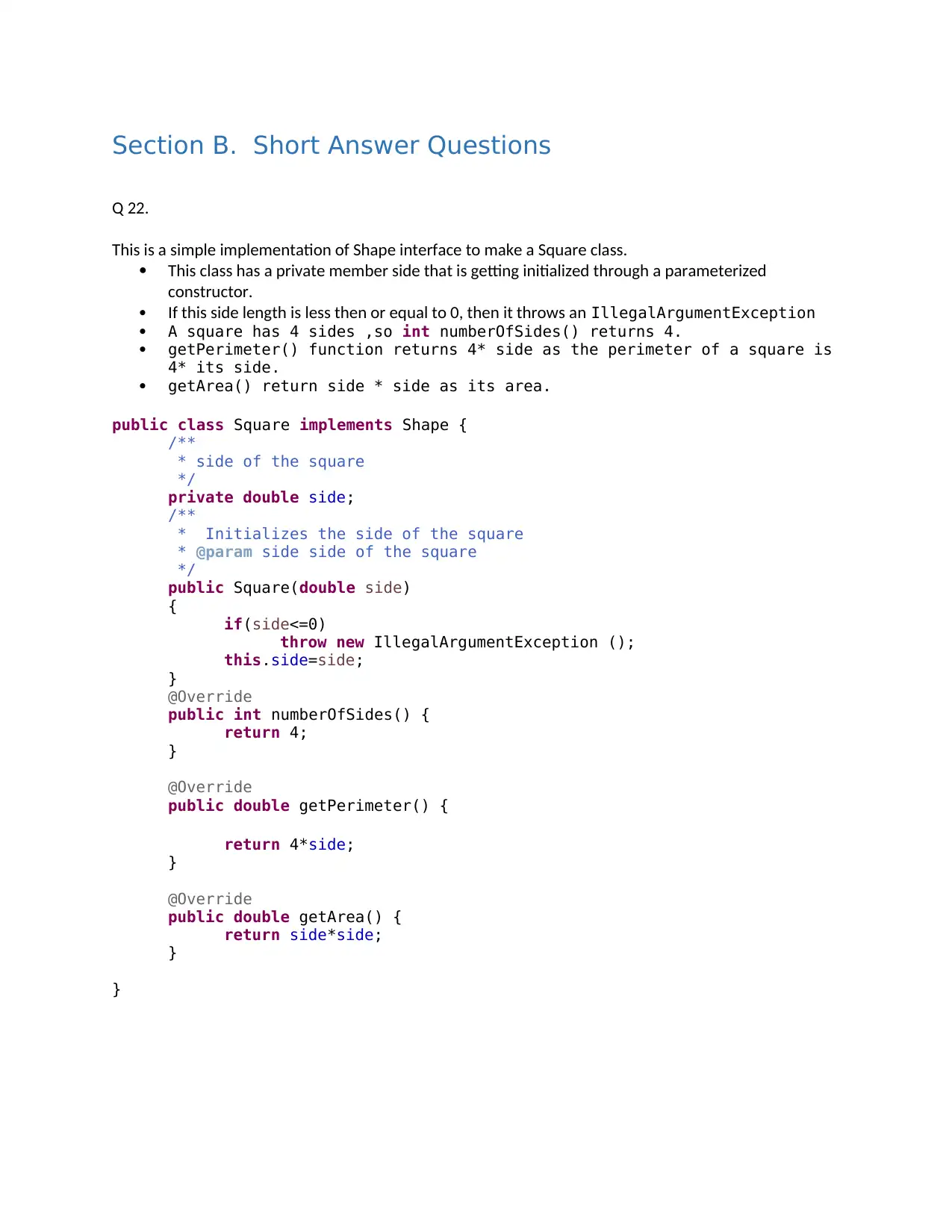
Section B. Short Answer Questions
Q 22.
This is a simple implementation of Shape interface to make a Square class.
This class has a private member side that is getting initialized through a parameterized
constructor.
If this side length is less then or equal to 0, then it throws an IllegalArgumentException
A square has 4 sides ,so int numberOfSides() returns 4.
getPerimeter() function returns 4* side as the perimeter of a square is
4* its side.
getArea() return side * side as its area.
public class Square implements Shape {
/**
* side of the square
*/
private double side;
/**
* Initializes the side of the square
* @param side side of the square
*/
public Square(double side)
{
if(side<=0)
throw new IllegalArgumentException ();
this.side=side;
}
@Override
public int numberOfSides() {
return 4;
}
@Override
public double getPerimeter() {
return 4*side;
}
@Override
public double getArea() {
return side*side;
}
}
Q 22.
This is a simple implementation of Shape interface to make a Square class.
This class has a private member side that is getting initialized through a parameterized
constructor.
If this side length is less then or equal to 0, then it throws an IllegalArgumentException
A square has 4 sides ,so int numberOfSides() returns 4.
getPerimeter() function returns 4* side as the perimeter of a square is
4* its side.
getArea() return side * side as its area.
public class Square implements Shape {
/**
* side of the square
*/
private double side;
/**
* Initializes the side of the square
* @param side side of the square
*/
public Square(double side)
{
if(side<=0)
throw new IllegalArgumentException ();
this.side=side;
}
@Override
public int numberOfSides() {
return 4;
}
@Override
public double getPerimeter() {
return 4*side;
}
@Override
public double getArea() {
return side*side;
}
}
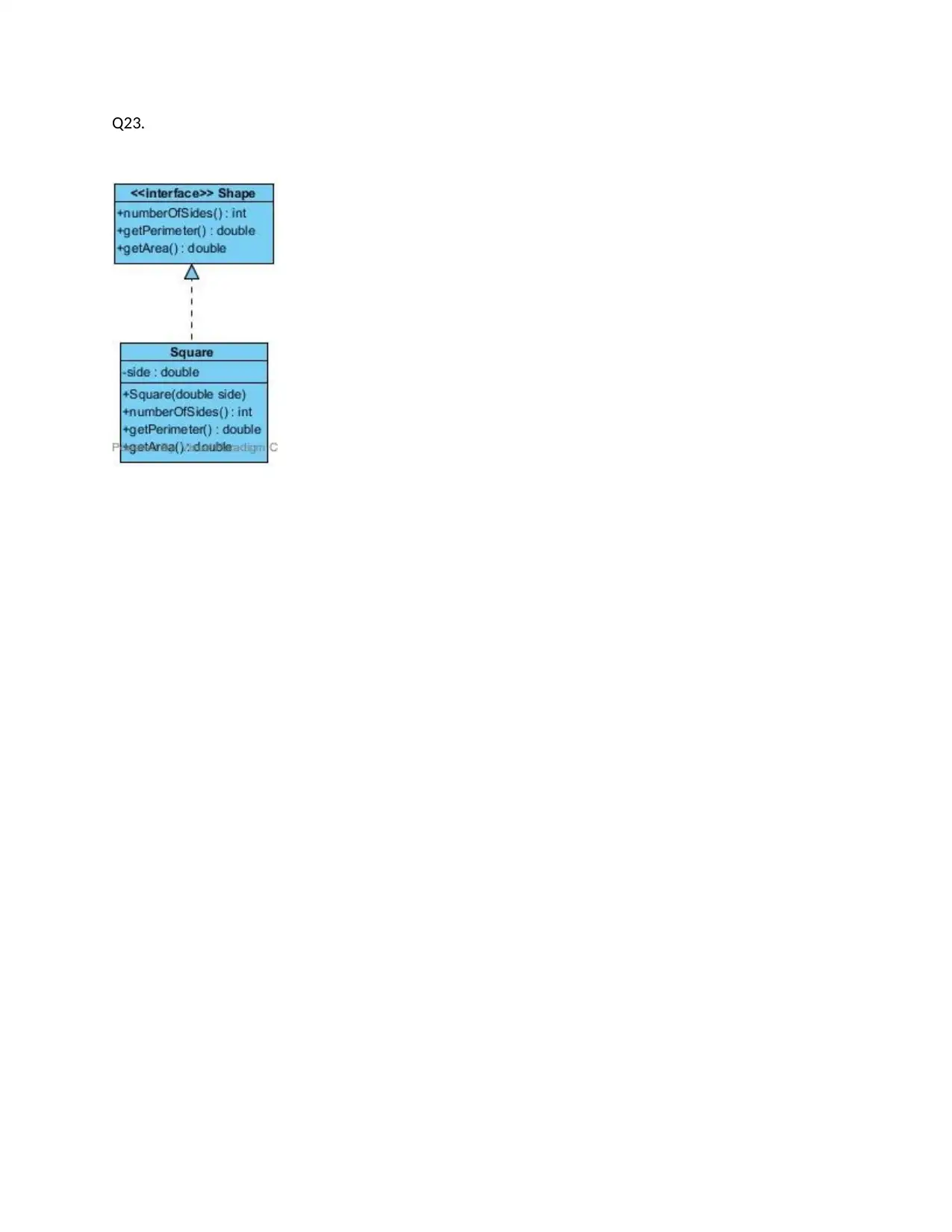
Q23.
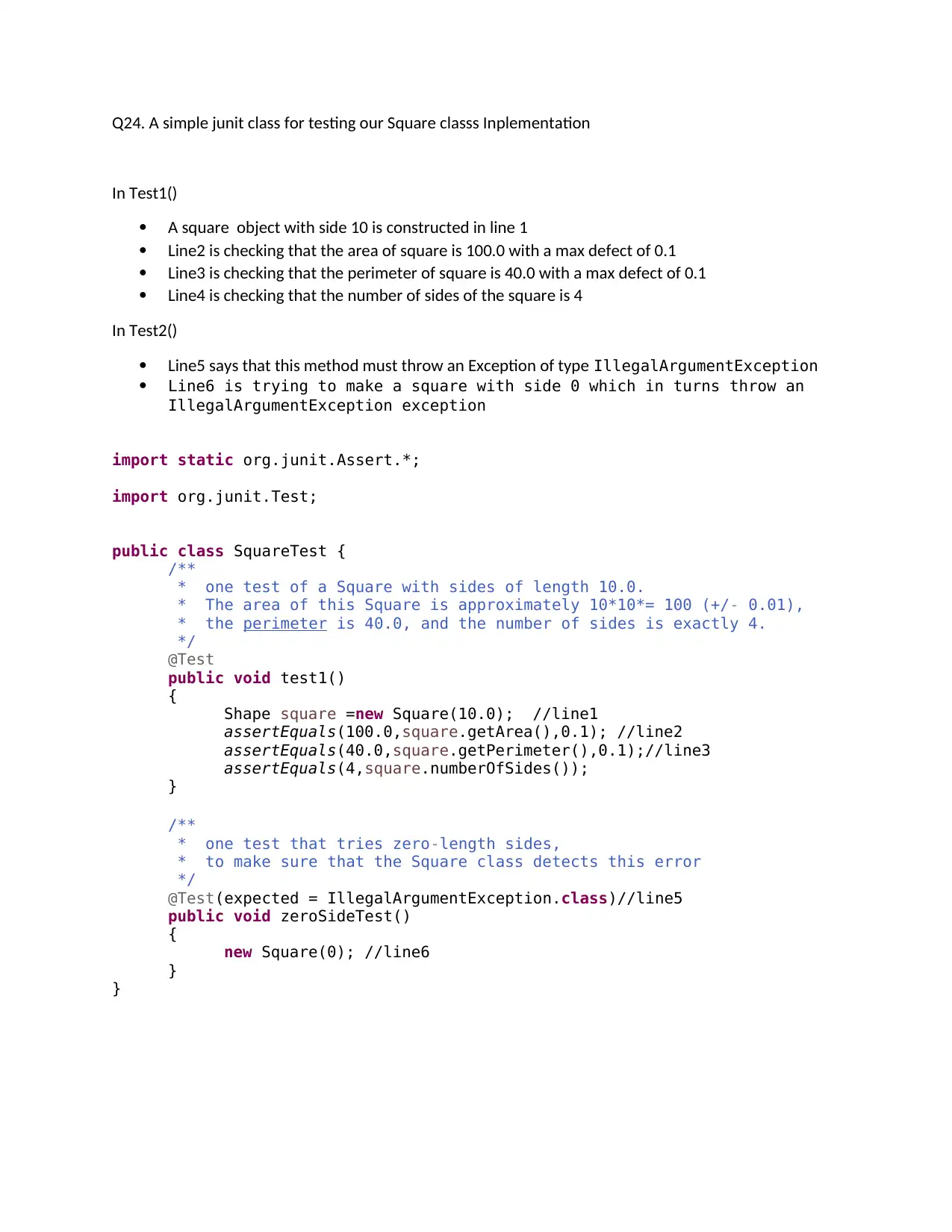
Q24. A simple junit class for testing our Square classs Inplementation
In Test1()
A square object with side 10 is constructed in line 1
Line2 is checking that the area of square is 100.0 with a max defect of 0.1
Line3 is checking that the perimeter of square is 40.0 with a max defect of 0.1
Line4 is checking that the number of sides of the square is 4
In Test2()
Line5 says that this method must throw an Exception of type IllegalArgumentException
Line6 is trying to make a square with side 0 which in turns throw an
IllegalArgumentException exception
import static org.junit.Assert.*;
import org.junit.Test;
public class SquareTest {
/**
* one test of a Square with sides of length 10.0.
* The area of this Square is approximately 10*10*= 100 (+/- 0.01),
* the perimeter is 40.0, and the number of sides is exactly 4.
*/
@Test
public void test1()
{
Shape square =new Square(10.0); //line1
assertEquals(100.0,square.getArea(),0.1); //line2
assertEquals(40.0,square.getPerimeter(),0.1);//line3
assertEquals(4,square.numberOfSides());
}
/**
* one test that tries zero-length sides,
* to make sure that the Square class detects this error
*/
@Test(expected = IllegalArgumentException.class)//line5
public void zeroSideTest()
{
new Square(0); //line6
}
}
In Test1()
A square object with side 10 is constructed in line 1
Line2 is checking that the area of square is 100.0 with a max defect of 0.1
Line3 is checking that the perimeter of square is 40.0 with a max defect of 0.1
Line4 is checking that the number of sides of the square is 4
In Test2()
Line5 says that this method must throw an Exception of type IllegalArgumentException
Line6 is trying to make a square with side 0 which in turns throw an
IllegalArgumentException exception
import static org.junit.Assert.*;
import org.junit.Test;
public class SquareTest {
/**
* one test of a Square with sides of length 10.0.
* The area of this Square is approximately 10*10*= 100 (+/- 0.01),
* the perimeter is 40.0, and the number of sides is exactly 4.
*/
@Test
public void test1()
{
Shape square =new Square(10.0); //line1
assertEquals(100.0,square.getArea(),0.1); //line2
assertEquals(40.0,square.getPerimeter(),0.1);//line3
assertEquals(4,square.numberOfSides());
}
/**
* one test that tries zero-length sides,
* to make sure that the Square class detects this error
*/
@Test(expected = IllegalArgumentException.class)//line5
public void zeroSideTest()
{
new Square(0); //line6
}
}
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
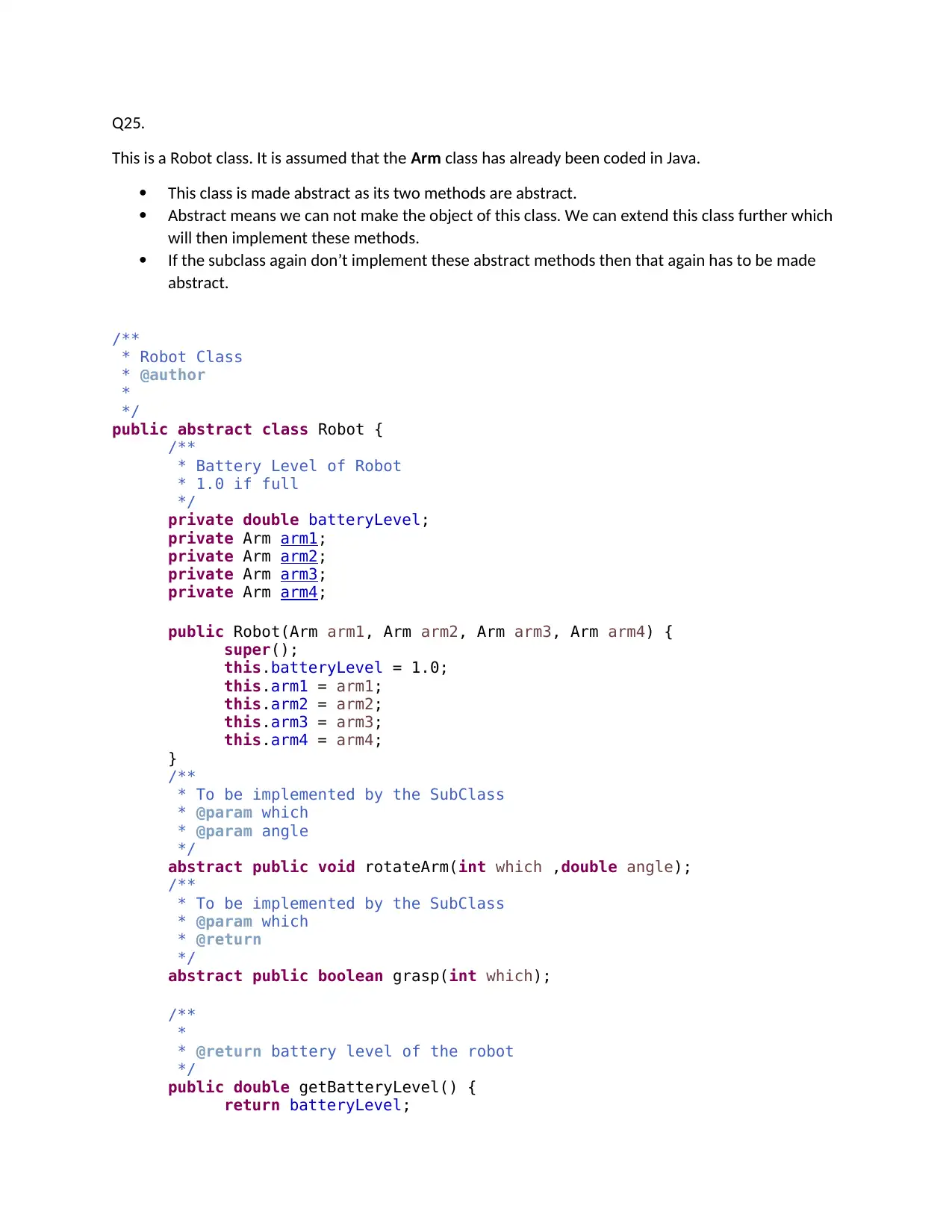
Q25.
This is a Robot class. It is assumed that the Arm class has already been coded in Java.
This class is made abstract as its two methods are abstract.
Abstract means we can not make the object of this class. We can extend this class further which
will then implement these methods.
If the subclass again don’t implement these abstract methods then that again has to be made
abstract.
/**
* Robot Class
* @author
*
*/
public abstract class Robot {
/**
* Battery Level of Robot
* 1.0 if full
*/
private double batteryLevel;
private Arm arm1;
private Arm arm2;
private Arm arm3;
private Arm arm4;
public Robot(Arm arm1, Arm arm2, Arm arm3, Arm arm4) {
super();
this.batteryLevel = 1.0;
this.arm1 = arm1;
this.arm2 = arm2;
this.arm3 = arm3;
this.arm4 = arm4;
}
/**
* To be implemented by the SubClass
* @param which
* @param angle
*/
abstract public void rotateArm(int which ,double angle);
/**
* To be implemented by the SubClass
* @param which
* @return
*/
abstract public boolean grasp(int which);
/**
*
* @return battery level of the robot
*/
public double getBatteryLevel() {
return batteryLevel;
This is a Robot class. It is assumed that the Arm class has already been coded in Java.
This class is made abstract as its two methods are abstract.
Abstract means we can not make the object of this class. We can extend this class further which
will then implement these methods.
If the subclass again don’t implement these abstract methods then that again has to be made
abstract.
/**
* Robot Class
* @author
*
*/
public abstract class Robot {
/**
* Battery Level of Robot
* 1.0 if full
*/
private double batteryLevel;
private Arm arm1;
private Arm arm2;
private Arm arm3;
private Arm arm4;
public Robot(Arm arm1, Arm arm2, Arm arm3, Arm arm4) {
super();
this.batteryLevel = 1.0;
this.arm1 = arm1;
this.arm2 = arm2;
this.arm3 = arm3;
this.arm4 = arm4;
}
/**
* To be implemented by the SubClass
* @param which
* @param angle
*/
abstract public void rotateArm(int which ,double angle);
/**
* To be implemented by the SubClass
* @param which
* @return
*/
abstract public boolean grasp(int which);
/**
*
* @return battery level of the robot
*/
public double getBatteryLevel() {
return batteryLevel;
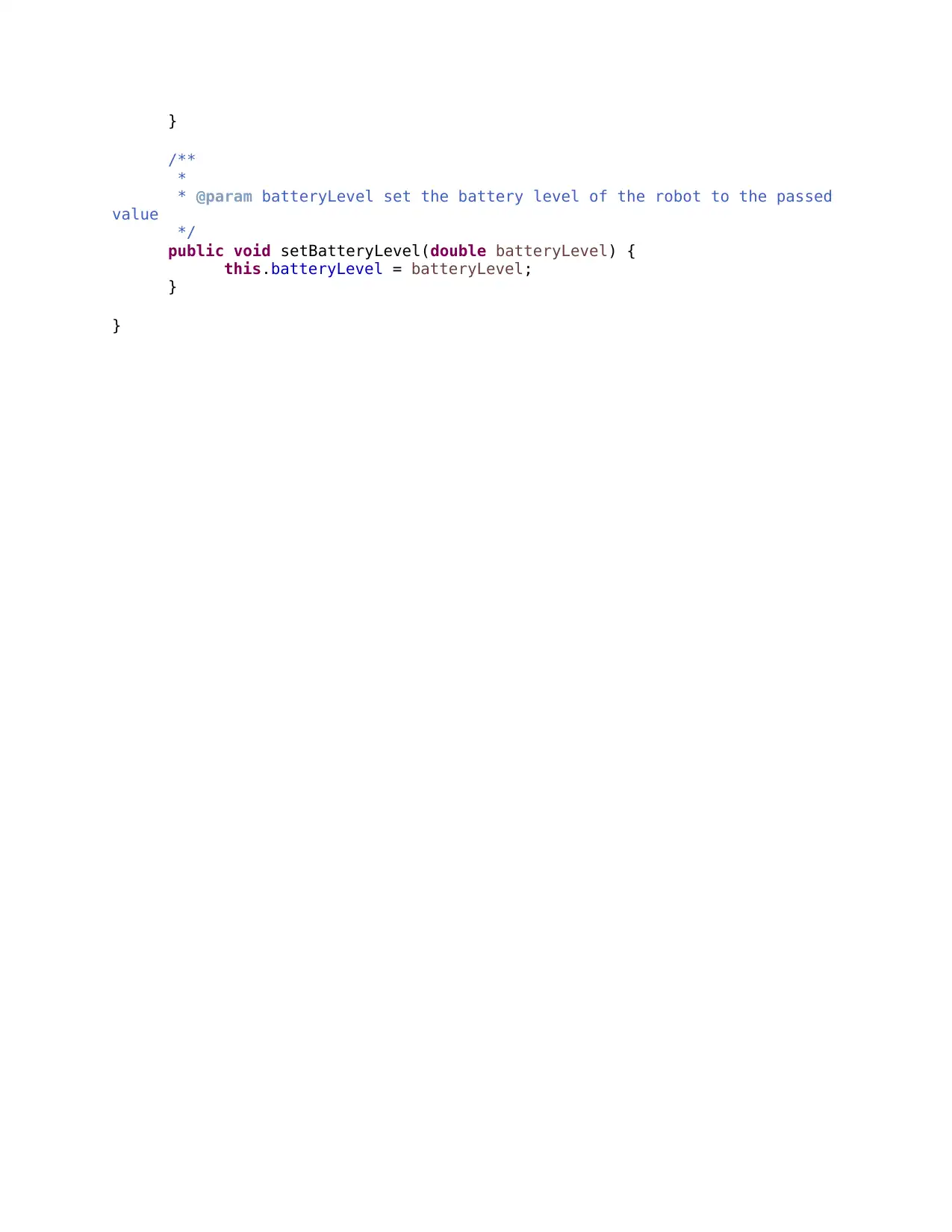
}
/**
*
* @param batteryLevel set the battery level of the robot to the passed
value
*/
public void setBatteryLevel(double batteryLevel) {
this.batteryLevel = batteryLevel;
}
}
/**
*
* @param batteryLevel set the battery level of the robot to the passed
value
*/
public void setBatteryLevel(double batteryLevel) {
this.batteryLevel = batteryLevel;
}
}
1 out of 6
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.