Python Score Function
VerifiedAdded on 2019/09/16
|11
|2022
|1178
Report
AI Summary
The assignment involves writing Python programs to prompt users for input, validate the input, and then compute a corresponding output based on predefined rules. In Exercise 1, the program prompts for a score between 0.0 and 1.0, checks if it's within the range, and then prints a grade according to a predetermined table. Exercises 2-6 involve defining functions that take parameters, perform computations, and return values. The programs are designed to handle errors and invalid inputs by using try-except blocks. The assignment requires students to submit their Python code files and Word documents showing screenshots of testing conditions.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
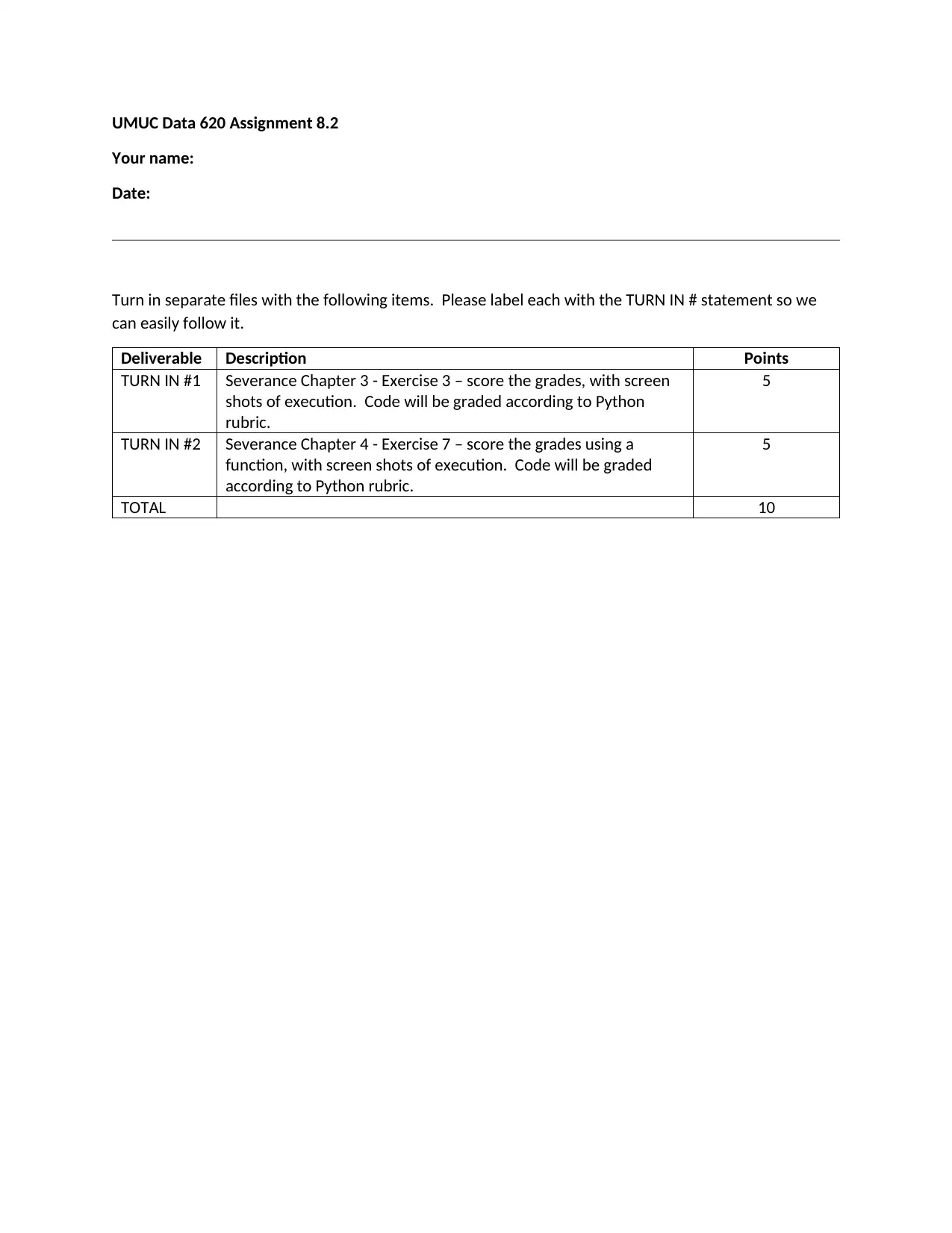
UMUC Data 620 Assignment 8.2
Your name:
Date:
Turn in separate files with the following items. Please label each with the TURN IN # statement so we
can easily follow it.
Deliverable Description Points
TURN IN #1 Severance Chapter 3 - Exercise 3 – score the grades, with screen
shots of execution. Code will be graded according to Python
rubric.
5
TURN IN #2 Severance Chapter 4 - Exercise 7 – score the grades using a
function, with screen shots of execution. Code will be graded
according to Python rubric.
5
TOTAL 10
Your name:
Date:
Turn in separate files with the following items. Please label each with the TURN IN # statement so we
can easily follow it.
Deliverable Description Points
TURN IN #1 Severance Chapter 3 - Exercise 3 – score the grades, with screen
shots of execution. Code will be graded according to Python
rubric.
5
TURN IN #2 Severance Chapter 4 - Exercise 7 – score the grades using a
function, with screen shots of execution. Code will be graded
according to Python rubric.
5
TOTAL 10
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
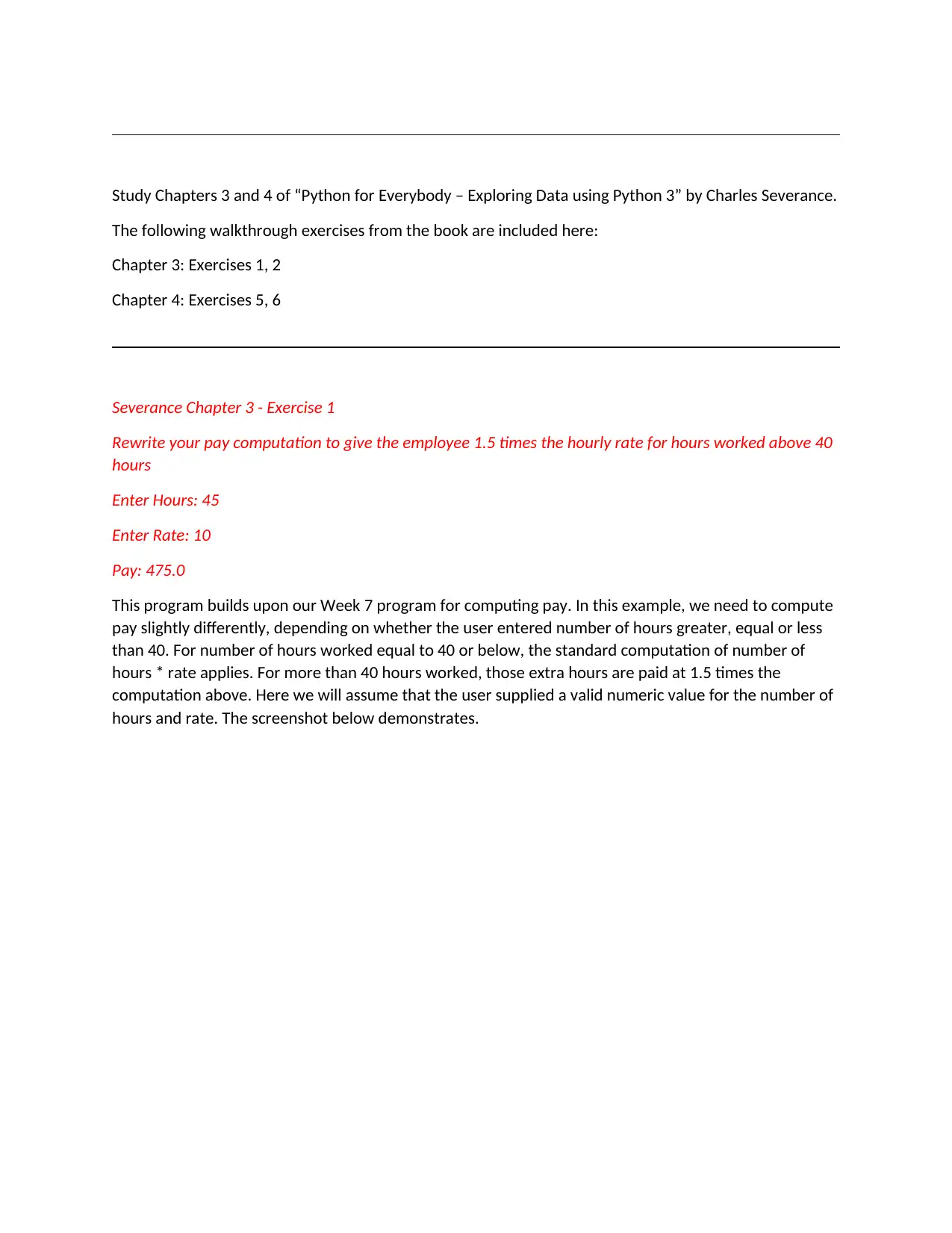
Study Chapters 3 and 4 of “Python for Everybody – Exploring Data using Python 3” by Charles Severance.
The following walkthrough exercises from the book are included here:
Chapter 3: Exercises 1, 2
Chapter 4: Exercises 5, 6
Severance Chapter 3 - Exercise 1
Rewrite your pay computation to give the employee 1.5 times the hourly rate for hours worked above 40
hours
Enter Hours: 45
Enter Rate: 10
Pay: 475.0
This program builds upon our Week 7 program for computing pay. In this example, we need to compute
pay slightly differently, depending on whether the user entered number of hours greater, equal or less
than 40. For number of hours worked equal to 40 or below, the standard computation of number of
hours * rate applies. For more than 40 hours worked, those extra hours are paid at 1.5 times the
computation above. Here we will assume that the user supplied a valid numeric value for the number of
hours and rate. The screenshot below demonstrates.
The following walkthrough exercises from the book are included here:
Chapter 3: Exercises 1, 2
Chapter 4: Exercises 5, 6
Severance Chapter 3 - Exercise 1
Rewrite your pay computation to give the employee 1.5 times the hourly rate for hours worked above 40
hours
Enter Hours: 45
Enter Rate: 10
Pay: 475.0
This program builds upon our Week 7 program for computing pay. In this example, we need to compute
pay slightly differently, depending on whether the user entered number of hours greater, equal or less
than 40. For number of hours worked equal to 40 or below, the standard computation of number of
hours * rate applies. For more than 40 hours worked, those extra hours are paid at 1.5 times the
computation above. Here we will assume that the user supplied a valid numeric value for the number of
hours and rate. The screenshot below demonstrates.
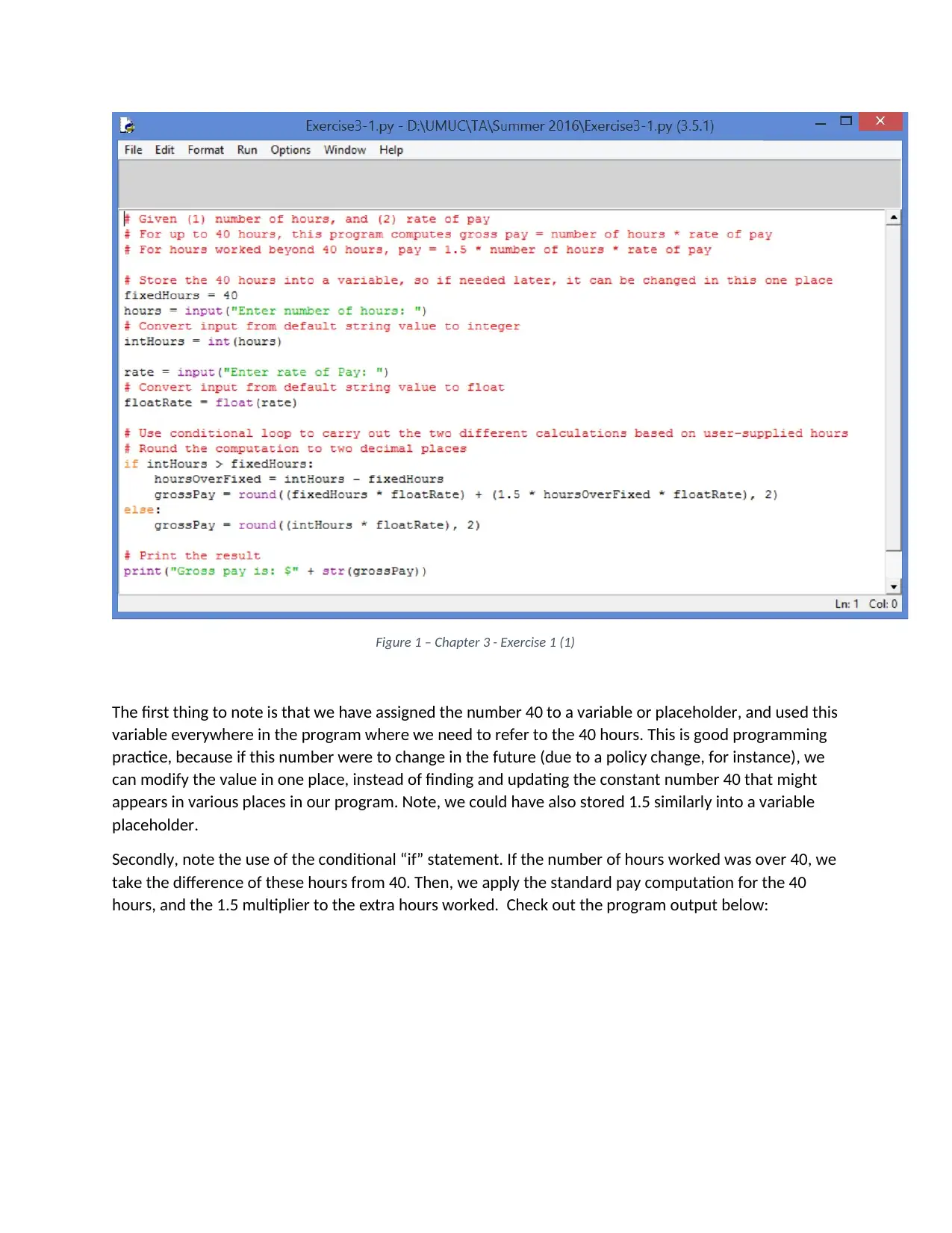
Figure 1 – Chapter 3 - Exercise 1 (1)
The first thing to note is that we have assigned the number 40 to a variable or placeholder, and used this
variable everywhere in the program where we need to refer to the 40 hours. This is good programming
practice, because if this number were to change in the future (due to a policy change, for instance), we
can modify the value in one place, instead of finding and updating the constant number 40 that might
appears in various places in our program. Note, we could have also stored 1.5 similarly into a variable
placeholder.
Secondly, note the use of the conditional “if” statement. If the number of hours worked was over 40, we
take the difference of these hours from 40. Then, we apply the standard pay computation for the 40
hours, and the 1.5 multiplier to the extra hours worked. Check out the program output below:
The first thing to note is that we have assigned the number 40 to a variable or placeholder, and used this
variable everywhere in the program where we need to refer to the 40 hours. This is good programming
practice, because if this number were to change in the future (due to a policy change, for instance), we
can modify the value in one place, instead of finding and updating the constant number 40 that might
appears in various places in our program. Note, we could have also stored 1.5 similarly into a variable
placeholder.
Secondly, note the use of the conditional “if” statement. If the number of hours worked was over 40, we
take the difference of these hours from 40. Then, we apply the standard pay computation for the 40
hours, and the 1.5 multiplier to the extra hours worked. Check out the program output below:
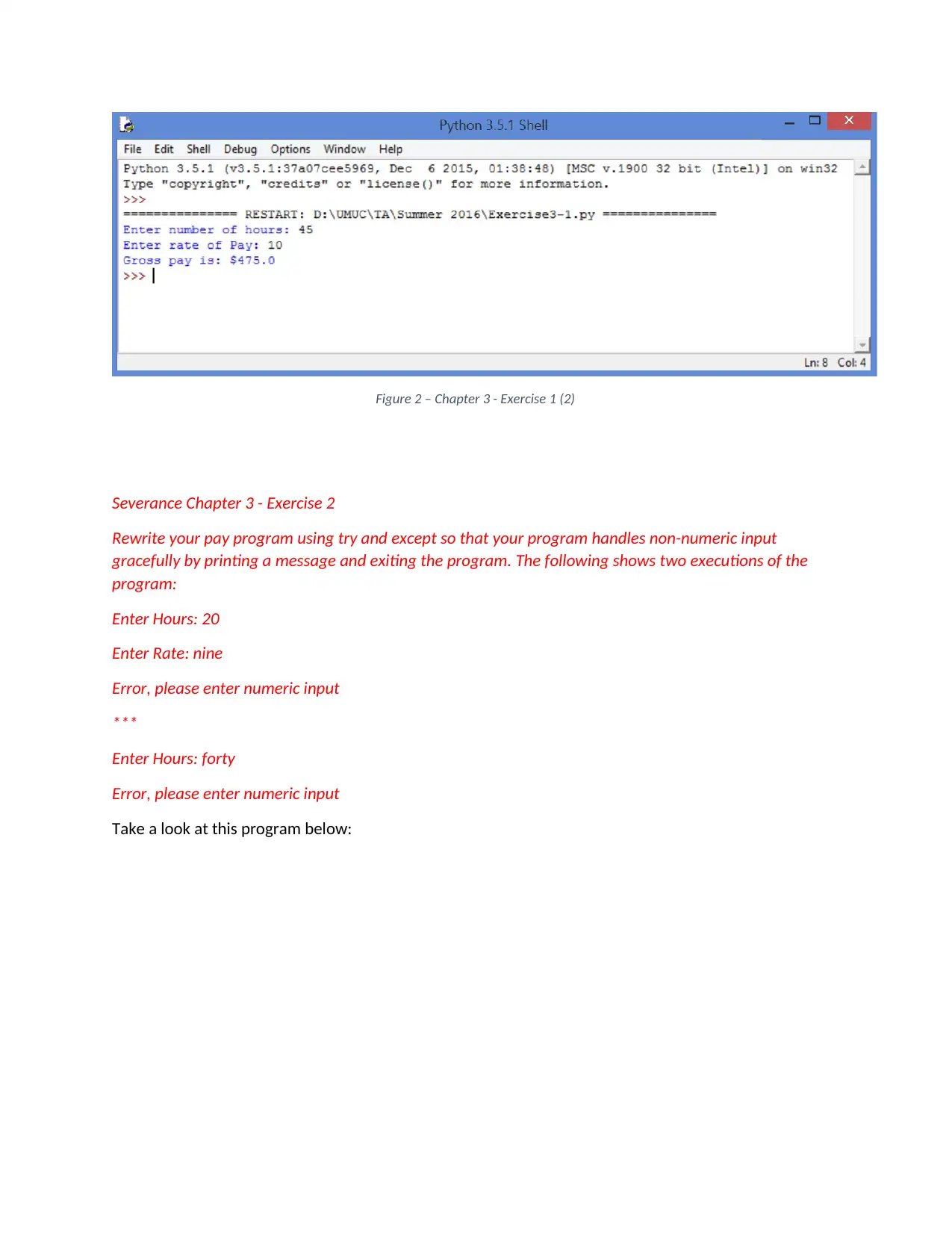
Figure 2 – Chapter 3 - Exercise 1 (2)
Severance Chapter 3 - Exercise 2
Rewrite your pay program using try and except so that your program handles non-numeric input
gracefully by printing a message and exiting the program. The following shows two executions of the
program:
Enter Hours: 20
Enter Rate: nine
Error, please enter numeric input
***
Enter Hours: forty
Error, please enter numeric input
Take a look at this program below:
Severance Chapter 3 - Exercise 2
Rewrite your pay program using try and except so that your program handles non-numeric input
gracefully by printing a message and exiting the program. The following shows two executions of the
program:
Enter Hours: 20
Enter Rate: nine
Error, please enter numeric input
***
Enter Hours: forty
Error, please enter numeric input
Take a look at this program below:
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
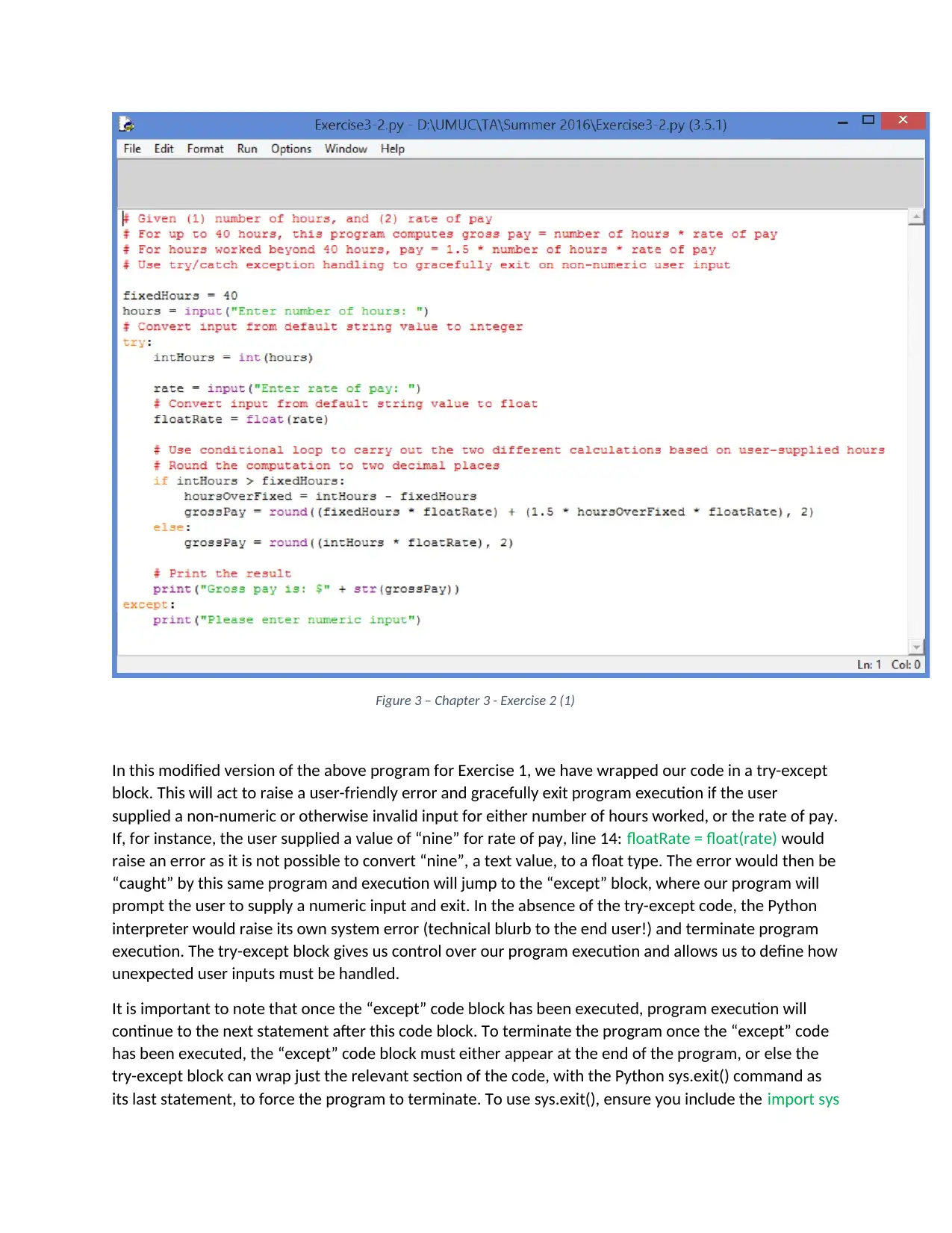
Figure 3 – Chapter 3 - Exercise 2 (1)
In this modified version of the above program for Exercise 1, we have wrapped our code in a try-except
block. This will act to raise a user-friendly error and gracefully exit program execution if the user
supplied a non-numeric or otherwise invalid input for either number of hours worked, or the rate of pay.
If, for instance, the user supplied a value of “nine” for rate of pay, line 14: floatRate = float(rate) would
raise an error as it is not possible to convert “nine”, a text value, to a float type. The error would then be
“caught” by this same program and execution will jump to the “except” block, where our program will
prompt the user to supply a numeric input and exit. In the absence of the try-except code, the Python
interpreter would raise its own system error (technical blurb to the end user!) and terminate program
execution. The try-except block gives us control over our program execution and allows us to define how
unexpected user inputs must be handled.
It is important to note that once the “except” code block has been executed, program execution will
continue to the next statement after this code block. To terminate the program once the “except” code
has been executed, the “except” code block must either appear at the end of the program, or else the
try-except block can wrap just the relevant section of the code, with the Python sys.exit() command as
its last statement, to force the program to terminate. To use sys.exit(), ensure you include the import sys
In this modified version of the above program for Exercise 1, we have wrapped our code in a try-except
block. This will act to raise a user-friendly error and gracefully exit program execution if the user
supplied a non-numeric or otherwise invalid input for either number of hours worked, or the rate of pay.
If, for instance, the user supplied a value of “nine” for rate of pay, line 14: floatRate = float(rate) would
raise an error as it is not possible to convert “nine”, a text value, to a float type. The error would then be
“caught” by this same program and execution will jump to the “except” block, where our program will
prompt the user to supply a numeric input and exit. In the absence of the try-except code, the Python
interpreter would raise its own system error (technical blurb to the end user!) and terminate program
execution. The try-except block gives us control over our program execution and allows us to define how
unexpected user inputs must be handled.
It is important to note that once the “except” code block has been executed, program execution will
continue to the next statement after this code block. To terminate the program once the “except” code
has been executed, the “except” code block must either appear at the end of the program, or else the
try-except block can wrap just the relevant section of the code, with the Python sys.exit() command as
its last statement, to force the program to terminate. To use sys.exit(), ensure you include the import sys
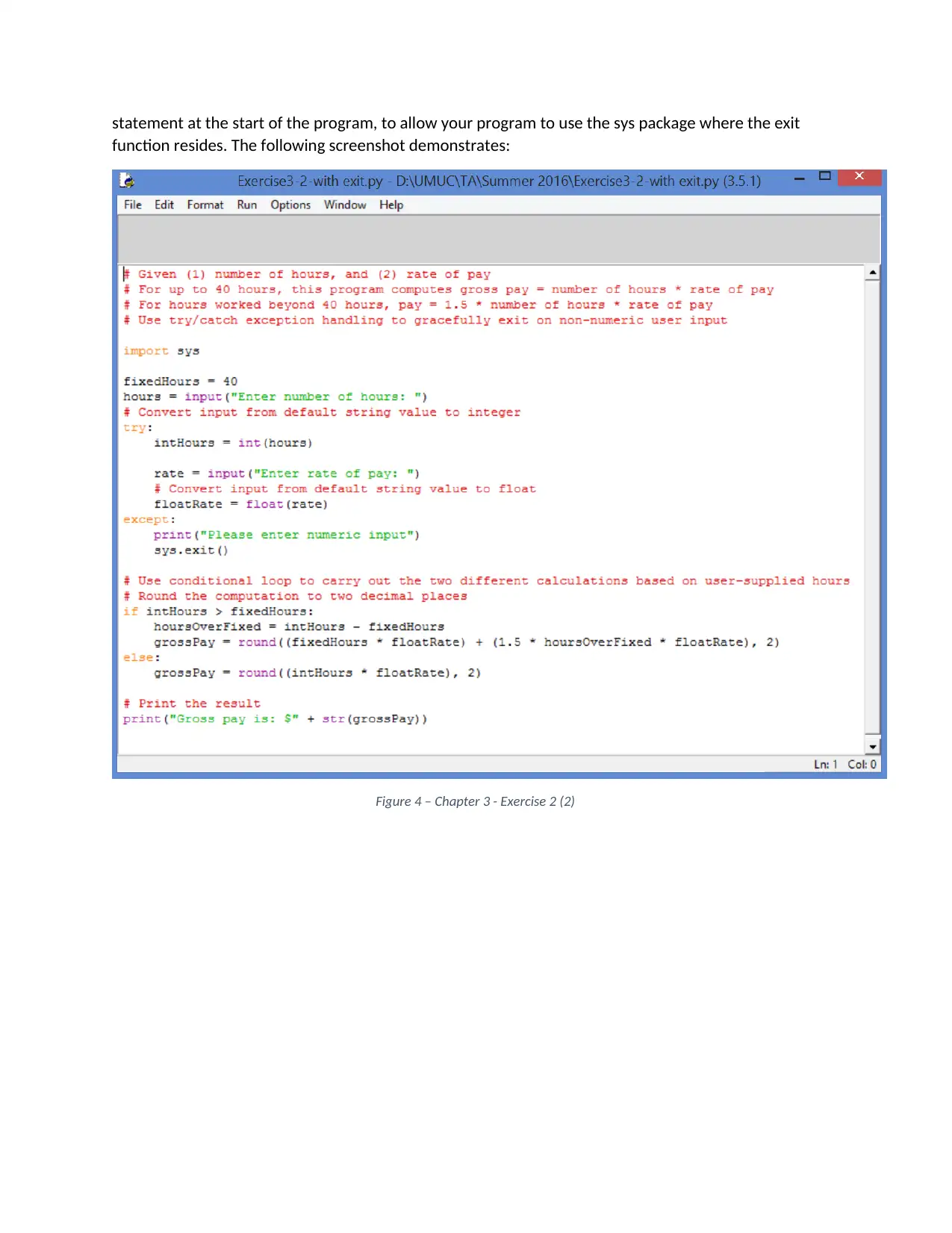
statement at the start of the program, to allow your program to use the sys package where the exit
function resides. The following screenshot demonstrates:
Figure 4 – Chapter 3 - Exercise 2 (2)
function resides. The following screenshot demonstrates:
Figure 4 – Chapter 3 - Exercise 2 (2)
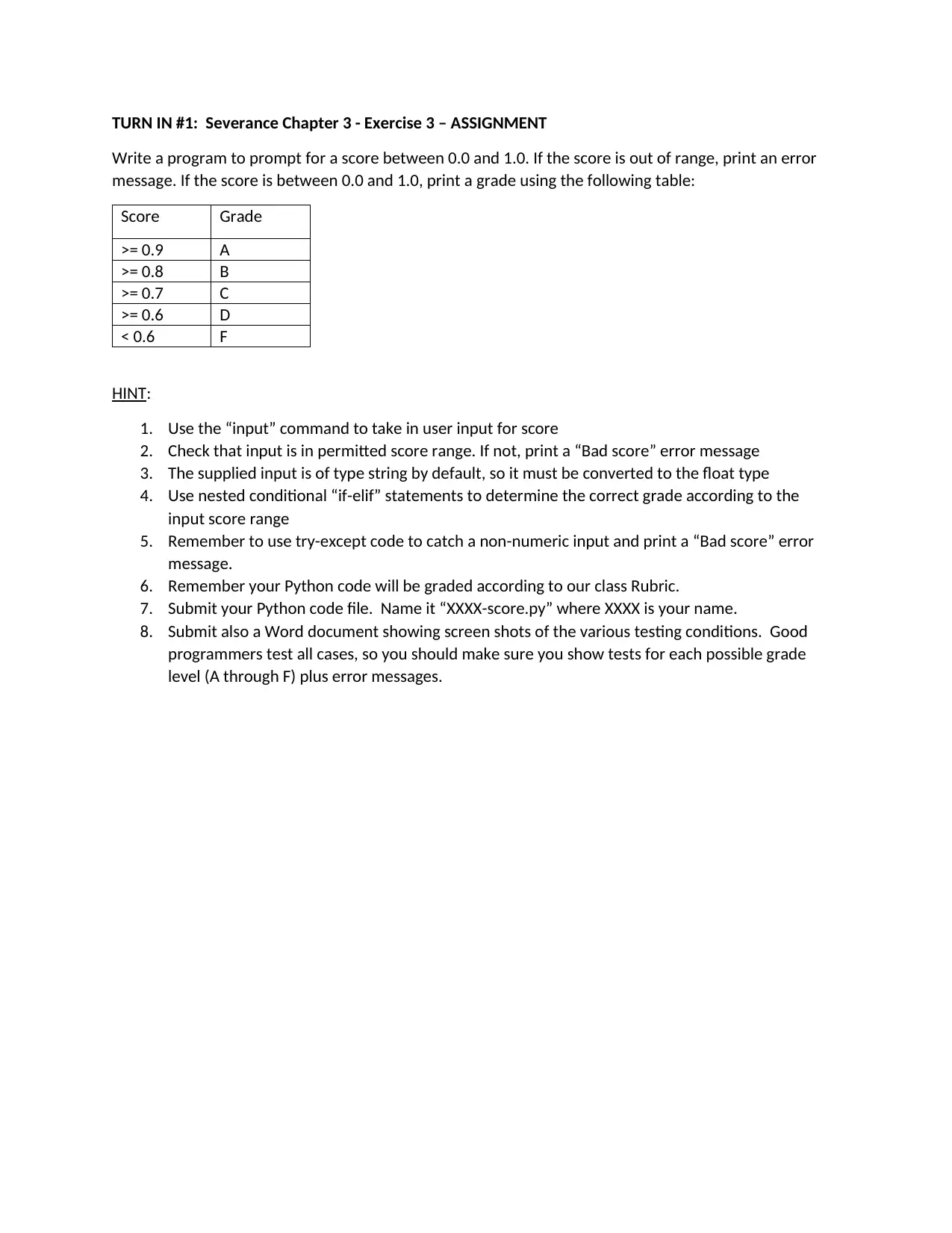
TURN IN #1: Severance Chapter 3 - Exercise 3 – ASSIGNMENT
Write a program to prompt for a score between 0.0 and 1.0. If the score is out of range, print an error
message. If the score is between 0.0 and 1.0, print a grade using the following table:
Score Grade
>= 0.9 A
>= 0.8 B
>= 0.7 C
>= 0.6 D
< 0.6 F
HINT:
1. Use the “input” command to take in user input for score
2. Check that input is in permitted score range. If not, print a “Bad score” error message
3. The supplied input is of type string by default, so it must be converted to the float type
4. Use nested conditional “if-elif” statements to determine the correct grade according to the
input score range
5. Remember to use try-except code to catch a non-numeric input and print a “Bad score” error
message.
6. Remember your Python code will be graded according to our class Rubric.
7. Submit your Python code file. Name it “XXXX-score.py” where XXXX is your name.
8. Submit also a Word document showing screen shots of the various testing conditions. Good
programmers test all cases, so you should make sure you show tests for each possible grade
level (A through F) plus error messages.
Write a program to prompt for a score between 0.0 and 1.0. If the score is out of range, print an error
message. If the score is between 0.0 and 1.0, print a grade using the following table:
Score Grade
>= 0.9 A
>= 0.8 B
>= 0.7 C
>= 0.6 D
< 0.6 F
HINT:
1. Use the “input” command to take in user input for score
2. Check that input is in permitted score range. If not, print a “Bad score” error message
3. The supplied input is of type string by default, so it must be converted to the float type
4. Use nested conditional “if-elif” statements to determine the correct grade according to the
input score range
5. Remember to use try-except code to catch a non-numeric input and print a “Bad score” error
message.
6. Remember your Python code will be graded according to our class Rubric.
7. Submit your Python code file. Name it “XXXX-score.py” where XXXX is your name.
8. Submit also a Word document showing screen shots of the various testing conditions. Good
programmers test all cases, so you should make sure you show tests for each possible grade
level (A through F) plus error messages.
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
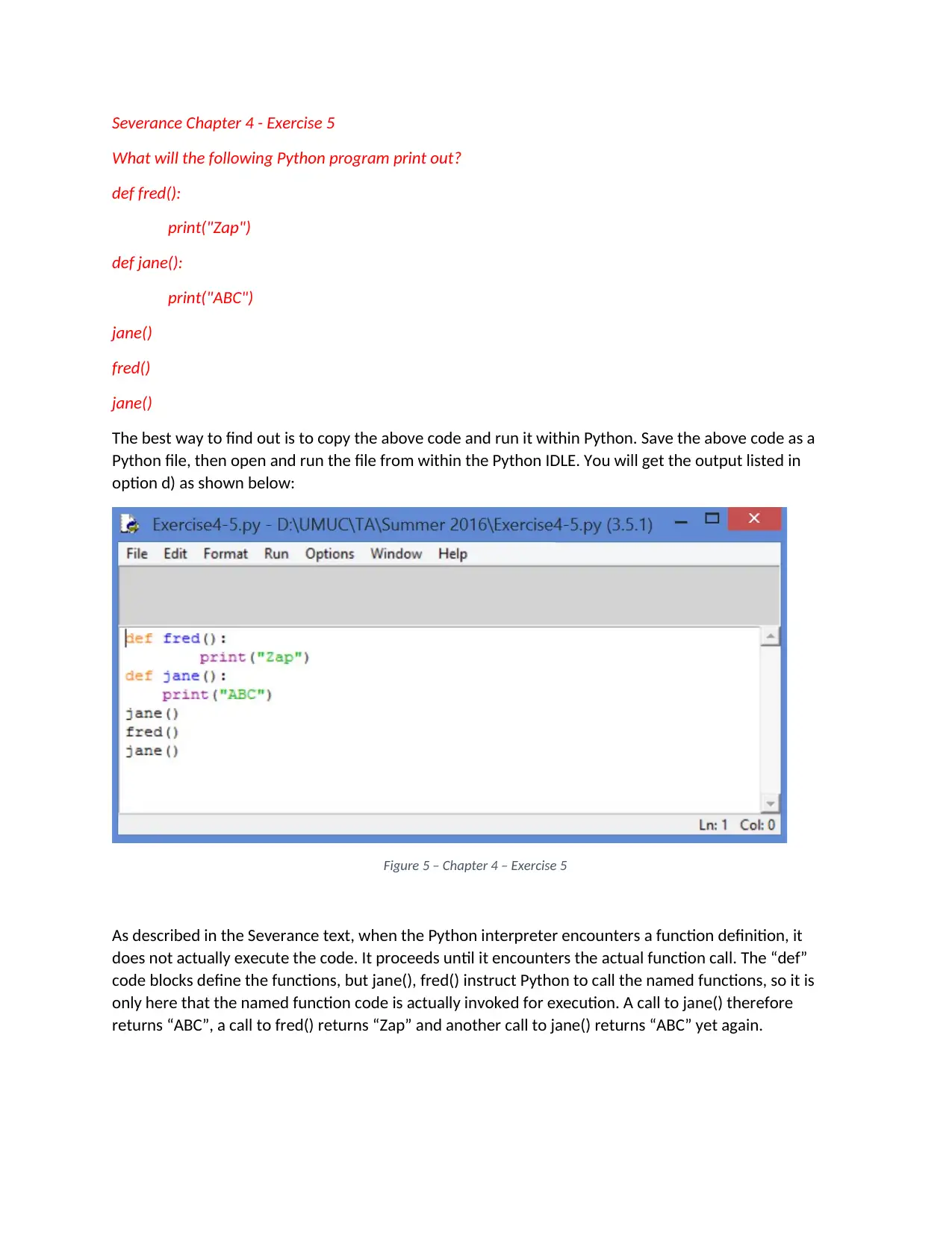
Severance Chapter 4 - Exercise 5
What will the following Python program print out?
def fred():
print("Zap")
def jane():
print("ABC")
jane()
fred()
jane()
The best way to find out is to copy the above code and run it within Python. Save the above code as a
Python file, then open and run the file from within the Python IDLE. You will get the output listed in
option d) as shown below:
Figure 5 – Chapter 4 – Exercise 5
As described in the Severance text, when the Python interpreter encounters a function definition, it
does not actually execute the code. It proceeds until it encounters the actual function call. The “def”
code blocks define the functions, but jane(), fred() instruct Python to call the named functions, so it is
only here that the named function code is actually invoked for execution. A call to jane() therefore
returns “ABC”, a call to fred() returns “Zap” and another call to jane() returns “ABC” yet again.
What will the following Python program print out?
def fred():
print("Zap")
def jane():
print("ABC")
jane()
fred()
jane()
The best way to find out is to copy the above code and run it within Python. Save the above code as a
Python file, then open and run the file from within the Python IDLE. You will get the output listed in
option d) as shown below:
Figure 5 – Chapter 4 – Exercise 5
As described in the Severance text, when the Python interpreter encounters a function definition, it
does not actually execute the code. It proceeds until it encounters the actual function call. The “def”
code blocks define the functions, but jane(), fred() instruct Python to call the named functions, so it is
only here that the named function code is actually invoked for execution. A call to jane() therefore
returns “ABC”, a call to fred() returns “Zap” and another call to jane() returns “ABC” yet again.
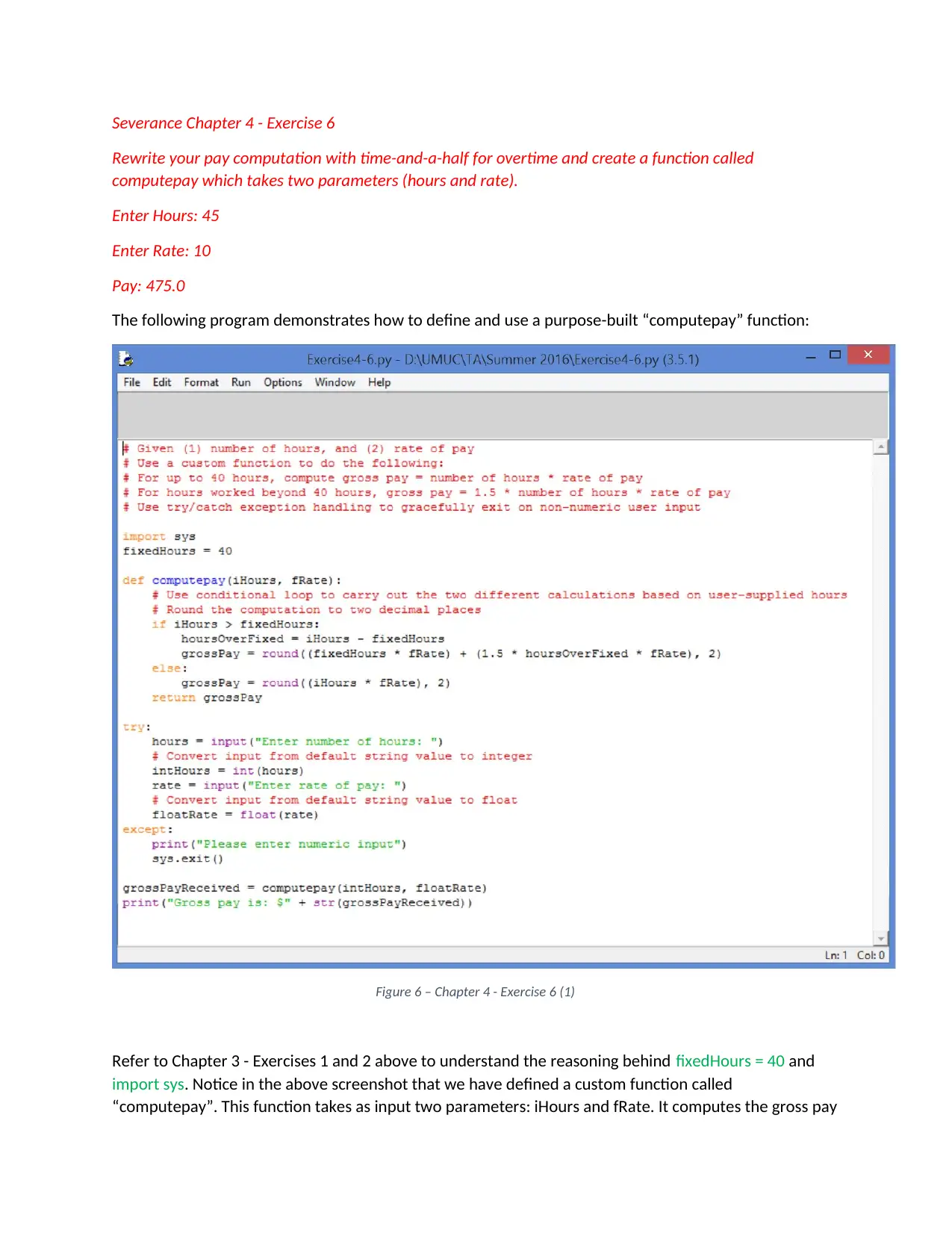
Severance Chapter 4 - Exercise 6
Rewrite your pay computation with time-and-a-half for overtime and create a function called
computepay which takes two parameters (hours and rate).
Enter Hours: 45
Enter Rate: 10
Pay: 475.0
The following program demonstrates how to define and use a purpose-built “computepay” function:
Figure 6 – Chapter 4 - Exercise 6 (1)
Refer to Chapter 3 - Exercises 1 and 2 above to understand the reasoning behind fixedHours = 40 and
import sys. Notice in the above screenshot that we have defined a custom function called
“computepay”. This function takes as input two parameters: iHours and fRate. It computes the gross pay
Rewrite your pay computation with time-and-a-half for overtime and create a function called
computepay which takes two parameters (hours and rate).
Enter Hours: 45
Enter Rate: 10
Pay: 475.0
The following program demonstrates how to define and use a purpose-built “computepay” function:
Figure 6 – Chapter 4 - Exercise 6 (1)
Refer to Chapter 3 - Exercises 1 and 2 above to understand the reasoning behind fixedHours = 40 and
import sys. Notice in the above screenshot that we have defined a custom function called
“computepay”. This function takes as input two parameters: iHours and fRate. It computes the gross pay
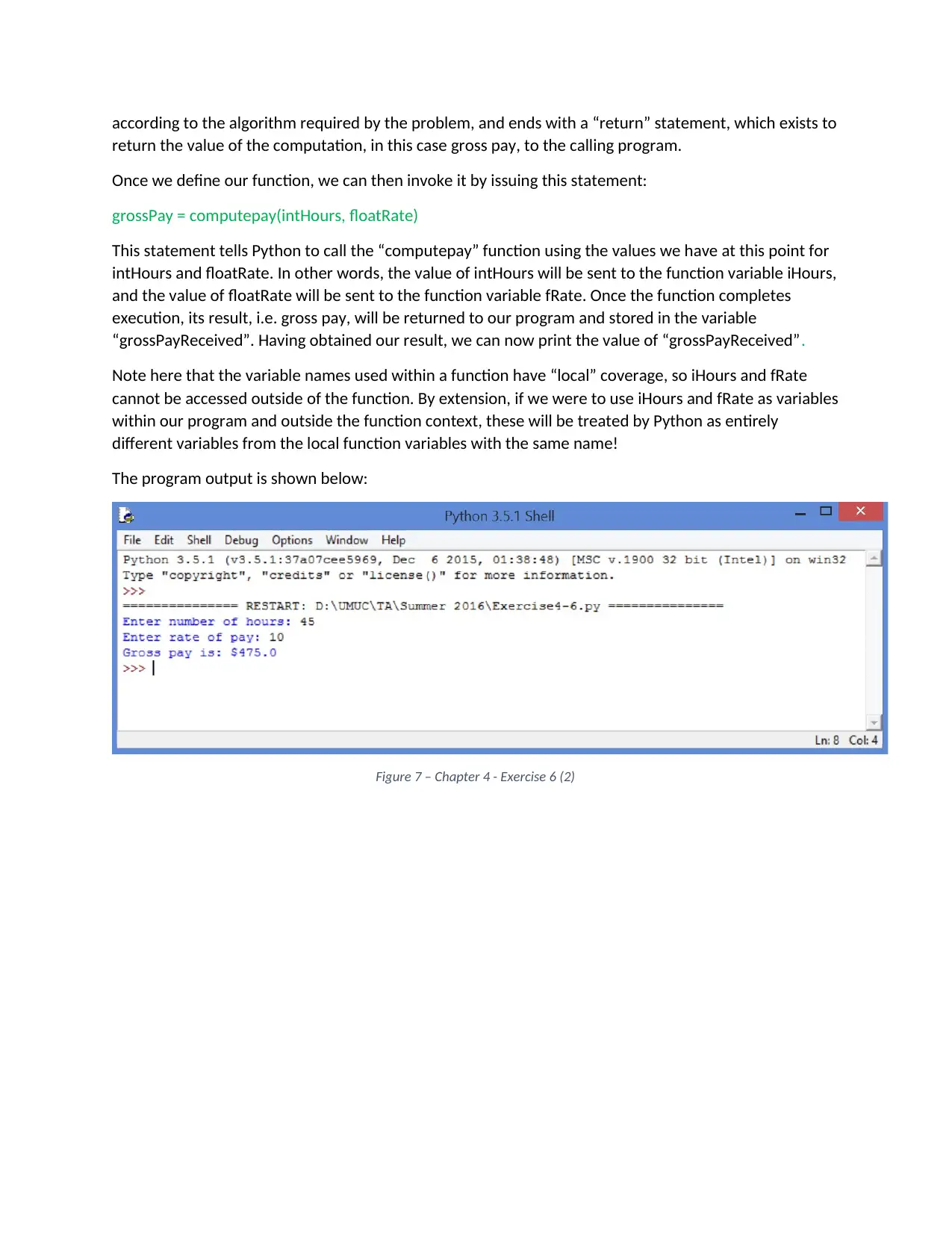
according to the algorithm required by the problem, and ends with a “return” statement, which exists to
return the value of the computation, in this case gross pay, to the calling program.
Once we define our function, we can then invoke it by issuing this statement:
grossPay = computepay(intHours, floatRate)
This statement tells Python to call the “computepay” function using the values we have at this point for
intHours and floatRate. In other words, the value of intHours will be sent to the function variable iHours,
and the value of floatRate will be sent to the function variable fRate. Once the function completes
execution, its result, i.e. gross pay, will be returned to our program and stored in the variable
“grossPayReceived”. Having obtained our result, we can now print the value of “grossPayReceived”.
Note here that the variable names used within a function have “local” coverage, so iHours and fRate
cannot be accessed outside of the function. By extension, if we were to use iHours and fRate as variables
within our program and outside the function context, these will be treated by Python as entirely
different variables from the local function variables with the same name!
The program output is shown below:
Figure 7 – Chapter 4 - Exercise 6 (2)
return the value of the computation, in this case gross pay, to the calling program.
Once we define our function, we can then invoke it by issuing this statement:
grossPay = computepay(intHours, floatRate)
This statement tells Python to call the “computepay” function using the values we have at this point for
intHours and floatRate. In other words, the value of intHours will be sent to the function variable iHours,
and the value of floatRate will be sent to the function variable fRate. Once the function completes
execution, its result, i.e. gross pay, will be returned to our program and stored in the variable
“grossPayReceived”. Having obtained our result, we can now print the value of “grossPayReceived”.
Note here that the variable names used within a function have “local” coverage, so iHours and fRate
cannot be accessed outside of the function. By extension, if we were to use iHours and fRate as variables
within our program and outside the function context, these will be treated by Python as entirely
different variables from the local function variables with the same name!
The program output is shown below:
Figure 7 – Chapter 4 - Exercise 6 (2)
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
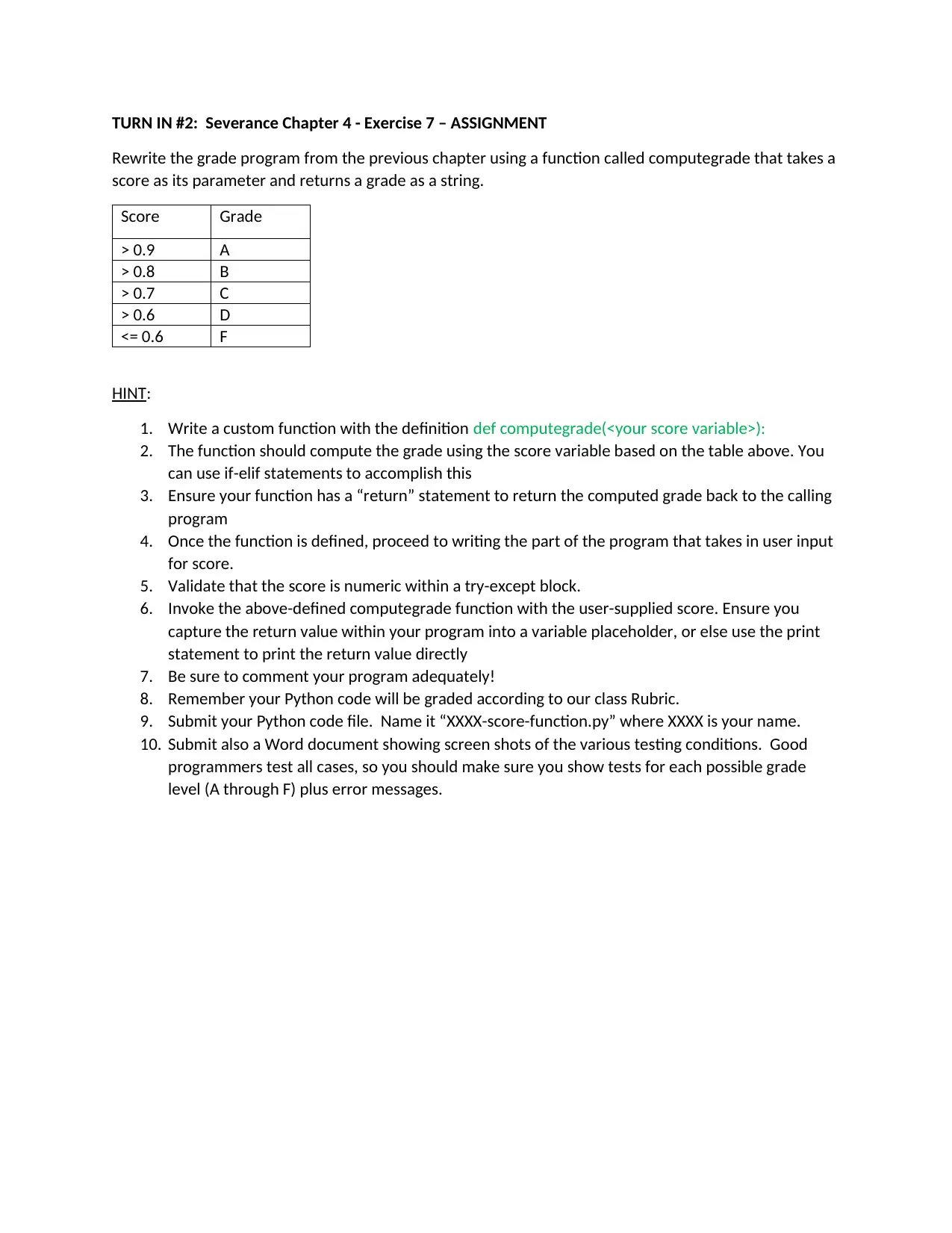
TURN IN #2: Severance Chapter 4 - Exercise 7 – ASSIGNMENT
Rewrite the grade program from the previous chapter using a function called computegrade that takes a
score as its parameter and returns a grade as a string.
Score Grade
> 0.9 A
> 0.8 B
> 0.7 C
> 0.6 D
<= 0.6 F
HINT:
1. Write a custom function with the definition def computegrade(<your score variable>):
2. The function should compute the grade using the score variable based on the table above. You
can use if-elif statements to accomplish this
3. Ensure your function has a “return” statement to return the computed grade back to the calling
program
4. Once the function is defined, proceed to writing the part of the program that takes in user input
for score.
5. Validate that the score is numeric within a try-except block.
6. Invoke the above-defined computegrade function with the user-supplied score. Ensure you
capture the return value within your program into a variable placeholder, or else use the print
statement to print the return value directly
7. Be sure to comment your program adequately!
8. Remember your Python code will be graded according to our class Rubric.
9. Submit your Python code file. Name it “XXXX-score-function.py” where XXXX is your name.
10. Submit also a Word document showing screen shots of the various testing conditions. Good
programmers test all cases, so you should make sure you show tests for each possible grade
level (A through F) plus error messages.
Rewrite the grade program from the previous chapter using a function called computegrade that takes a
score as its parameter and returns a grade as a string.
Score Grade
> 0.9 A
> 0.8 B
> 0.7 C
> 0.6 D
<= 0.6 F
HINT:
1. Write a custom function with the definition def computegrade(<your score variable>):
2. The function should compute the grade using the score variable based on the table above. You
can use if-elif statements to accomplish this
3. Ensure your function has a “return” statement to return the computed grade back to the calling
program
4. Once the function is defined, proceed to writing the part of the program that takes in user input
for score.
5. Validate that the score is numeric within a try-except block.
6. Invoke the above-defined computegrade function with the user-supplied score. Ensure you
capture the return value within your program into a variable placeholder, or else use the print
statement to print the return value directly
7. Be sure to comment your program adequately!
8. Remember your Python code will be graded according to our class Rubric.
9. Submit your Python code file. Name it “XXXX-score-function.py” where XXXX is your name.
10. Submit also a Word document showing screen shots of the various testing conditions. Good
programmers test all cases, so you should make sure you show tests for each possible grade
level (A through F) plus error messages.
1 out of 11
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.