Task 5 - Identifying Inheritance
VerifiedAdded on 2019/09/16
|8
|2037
|334
Report
AI Summary
The provided assignment content is a set of programming exercises that aim to teach object-oriented programming concepts, specifically inheritance and polymorphism. The tasks are divided into several parts, starting with identifying shared attributes and behavior between two classes (Customer and Video) and moving them to a parent class called Record. Then, the focus shifts to creating a video store system, which involves designing classes for Video, Customer, and Store, as well as implementing methods for adding videos and customers, renting videos, and displaying summaries. The assignment also includes tasks on building inheritance, testing polymorphism, and creating a menu-driven interface.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
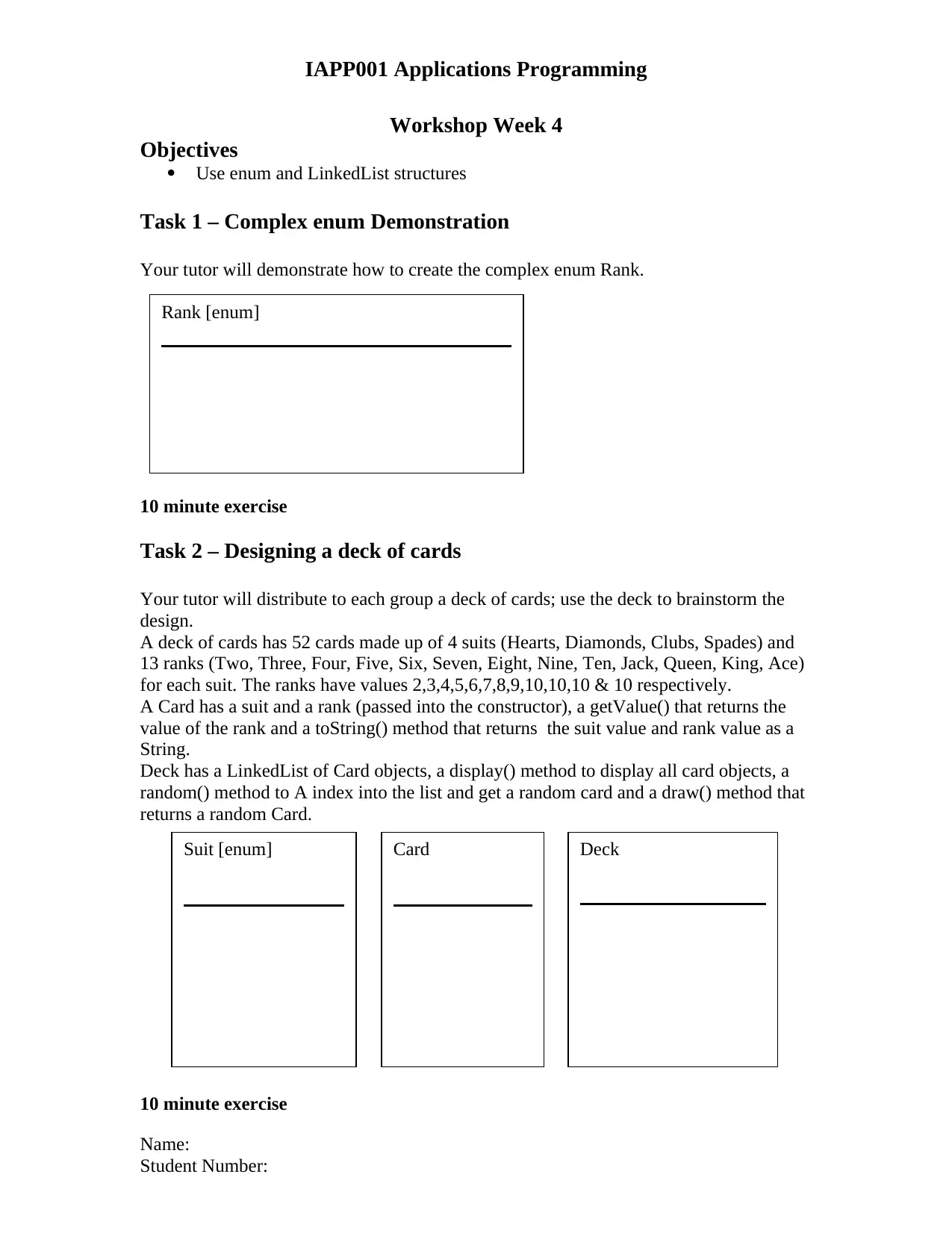
IAPP001 Applications Programming
Workshop Week 4
Objectives
Use enum and LinkedList structures
Task 1 – Complex enum Demonstration
Your tutor will demonstrate how to create the complex enum Rank.
10 minute exercise
Task 2 – Designing a deck of cards
Your tutor will distribute to each group a deck of cards; use the deck to brainstorm the
design.
A deck of cards has 52 cards made up of 4 suits (Hearts, Diamonds, Clubs, Spades) and
13 ranks (Two, Three, Four, Five, Six, Seven, Eight, Nine, Ten, Jack, Queen, King, Ace)
for each suit. The ranks have values 2,3,4,5,6,7,8,9,10,10,10 & 10 respectively.
A Card has a suit and a rank (passed into the constructor), a getValue() that returns the
value of the rank and a toString() method that returns the suit value and rank value as a
String.
Deck has a LinkedList of Card objects, a display() method to display all card objects, a
random() method to A index into the list and get a random card and a draw() method that
returns a random Card.
10 minute exercise
Name:
Student Number:
DeckCardSuit [enum]
Rank [enum]
Workshop Week 4
Objectives
Use enum and LinkedList structures
Task 1 – Complex enum Demonstration
Your tutor will demonstrate how to create the complex enum Rank.
10 minute exercise
Task 2 – Designing a deck of cards
Your tutor will distribute to each group a deck of cards; use the deck to brainstorm the
design.
A deck of cards has 52 cards made up of 4 suits (Hearts, Diamonds, Clubs, Spades) and
13 ranks (Two, Three, Four, Five, Six, Seven, Eight, Nine, Ten, Jack, Queen, King, Ace)
for each suit. The ranks have values 2,3,4,5,6,7,8,9,10,10,10 & 10 respectively.
A Card has a suit and a rank (passed into the constructor), a getValue() that returns the
value of the rank and a toString() method that returns the suit value and rank value as a
String.
Deck has a LinkedList of Card objects, a display() method to display all card objects, a
random() method to A index into the list and get a random card and a draw() method that
returns a random Card.
10 minute exercise
Name:
Student Number:
DeckCardSuit [enum]
Rank [enum]
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
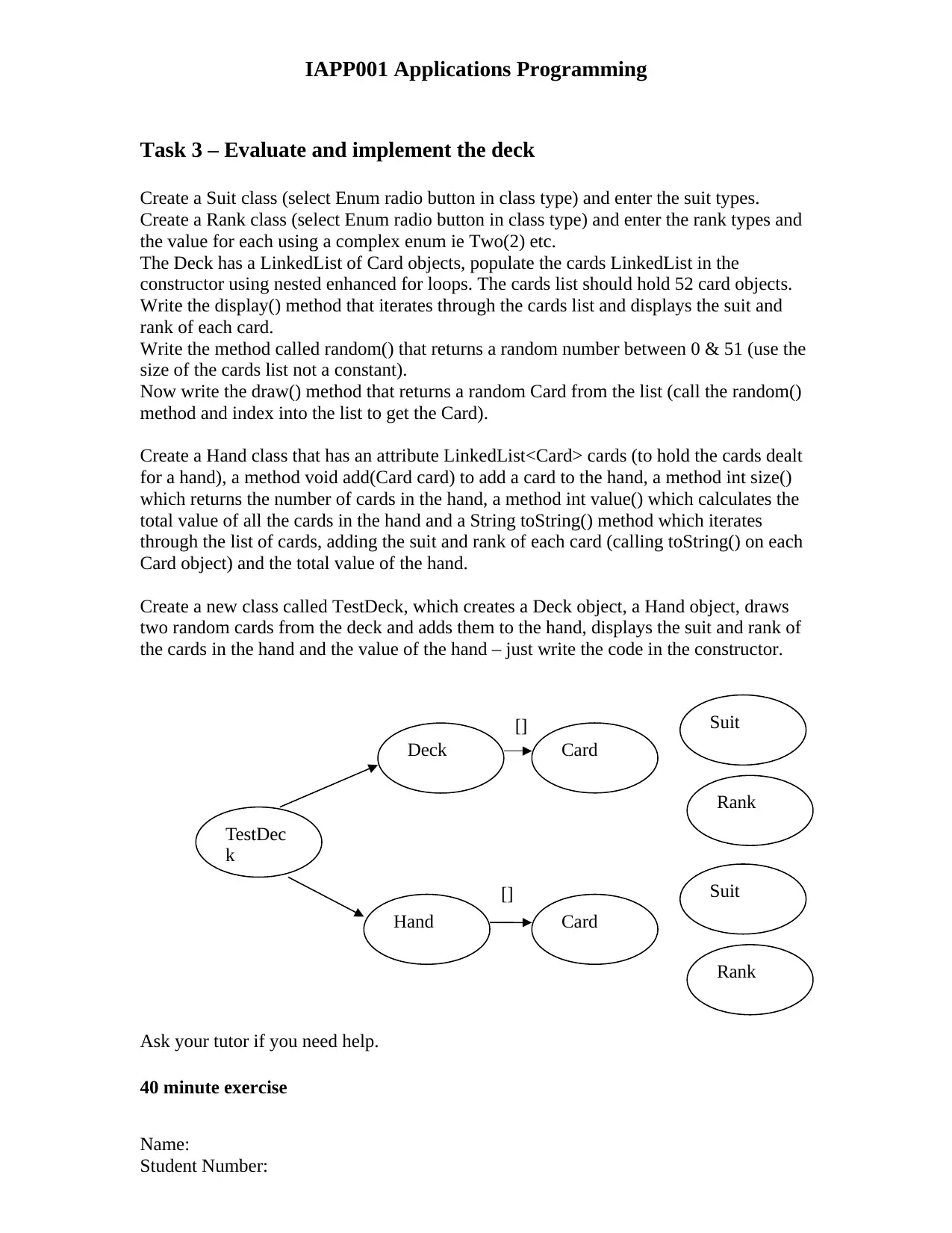
IAPP001 Applications Programming
Task 3 – Evaluate and implement the deck
Create a Suit class (select Enum radio button in class type) and enter the suit types.
Create a Rank class (select Enum radio button in class type) and enter the rank types and
the value for each using a complex enum ie Two(2) etc.
The Deck has a LinkedList of Card objects, populate the cards LinkedList in the
constructor using nested enhanced for loops. The cards list should hold 52 card objects.
Write the display() method that iterates through the cards list and displays the suit and
rank of each card.
Write the method called random() that returns a random number between 0 & 51 (use the
size of the cards list not a constant).
Now write the draw() method that returns a random Card from the list (call the random()
method and index into the list to get the Card).
Create a Hand class that has an attribute LinkedList<Card> cards (to hold the cards dealt
for a hand), a method void add(Card card) to add a card to the hand, a method int size()
which returns the number of cards in the hand, a method int value() which calculates the
total value of all the cards in the hand and a String toString() method which iterates
through the list of cards, adding the suit and rank of each card (calling toString() on each
Card object) and the total value of the hand.
Create a new class called TestDeck, which creates a Deck object, a Hand object, draws
two random cards from the deck and adds them to the hand, displays the suit and rank of
the cards in the hand and the value of the hand – just write the code in the constructor.
Ask your tutor if you need help.
40 minute exercise
Name:
Student Number:
[]
[]
Deck Card
Hand
TestDec
k
Card
Rank
Suit
Rank
Suit
Task 3 – Evaluate and implement the deck
Create a Suit class (select Enum radio button in class type) and enter the suit types.
Create a Rank class (select Enum radio button in class type) and enter the rank types and
the value for each using a complex enum ie Two(2) etc.
The Deck has a LinkedList of Card objects, populate the cards LinkedList in the
constructor using nested enhanced for loops. The cards list should hold 52 card objects.
Write the display() method that iterates through the cards list and displays the suit and
rank of each card.
Write the method called random() that returns a random number between 0 & 51 (use the
size of the cards list not a constant).
Now write the draw() method that returns a random Card from the list (call the random()
method and index into the list to get the Card).
Create a Hand class that has an attribute LinkedList<Card> cards (to hold the cards dealt
for a hand), a method void add(Card card) to add a card to the hand, a method int size()
which returns the number of cards in the hand, a method int value() which calculates the
total value of all the cards in the hand and a String toString() method which iterates
through the list of cards, adding the suit and rank of each card (calling toString() on each
Card object) and the total value of the hand.
Create a new class called TestDeck, which creates a Deck object, a Hand object, draws
two random cards from the deck and adds them to the hand, displays the suit and rank of
the cards in the hand and the value of the hand – just write the code in the constructor.
Ask your tutor if you need help.
40 minute exercise
Name:
Student Number:
[]
[]
Deck Card
Hand
TestDec
k
Card
Rank
Suit
Rank
Suit
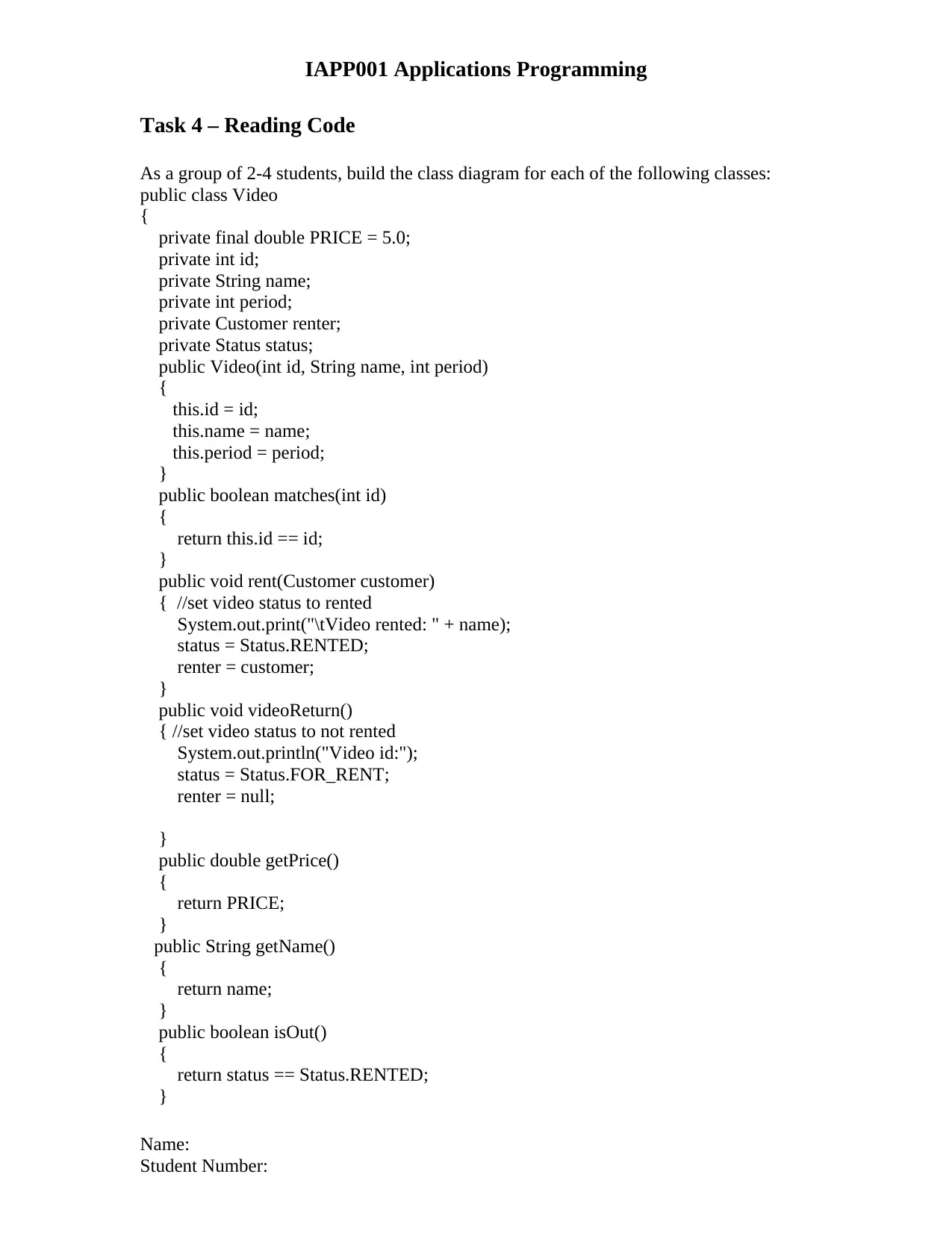
IAPP001 Applications Programming
Task 4 – Reading Code
As a group of 2-4 students, build the class diagram for each of the following classes:
public class Video
{
private final double PRICE = 5.0;
private int id;
private String name;
private int period;
private Customer renter;
private Status status;
public Video(int id, String name, int period)
{
this.id = id;
this.name = name;
this.period = period;
}
public boolean matches(int id)
{
return this.id == id;
}
public void rent(Customer customer)
{ //set video status to rented
System.out.print("\tVideo rented: " + name);
status = Status.RENTED;
renter = customer;
}
public void videoReturn()
{ //set video status to not rented
System.out.println("Video id:");
status = Status.FOR_RENT;
renter = null;
}
public double getPrice()
{
return PRICE;
}
public String getName()
{
return name;
}
public boolean isOut()
{
return status == Status.RENTED;
}
Name:
Student Number:
Task 4 – Reading Code
As a group of 2-4 students, build the class diagram for each of the following classes:
public class Video
{
private final double PRICE = 5.0;
private int id;
private String name;
private int period;
private Customer renter;
private Status status;
public Video(int id, String name, int period)
{
this.id = id;
this.name = name;
this.period = period;
}
public boolean matches(int id)
{
return this.id == id;
}
public void rent(Customer customer)
{ //set video status to rented
System.out.print("\tVideo rented: " + name);
status = Status.RENTED;
renter = customer;
}
public void videoReturn()
{ //set video status to not rented
System.out.println("Video id:");
status = Status.FOR_RENT;
renter = null;
}
public double getPrice()
{
return PRICE;
}
public String getName()
{
return name;
}
public boolean isOut()
{
return status == Status.RENTED;
}
Name:
Student Number:
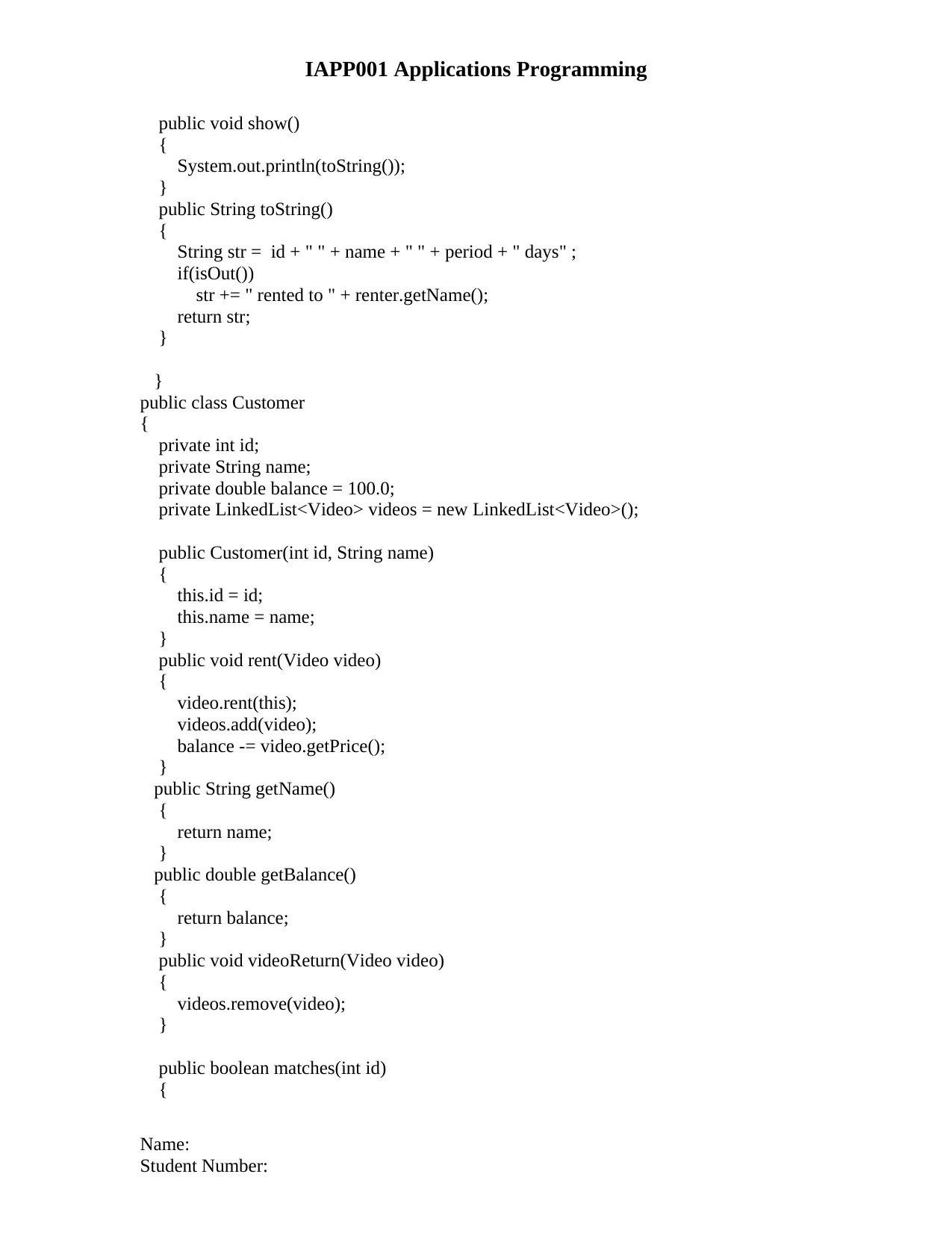
IAPP001 Applications Programming
public void show()
{
System.out.println(toString());
}
public String toString()
{
String str = id + " " + name + " " + period + " days" ;
if(isOut())
str += " rented to " + renter.getName();
return str;
}
}
public class Customer
{
private int id;
private String name;
private double balance = 100.0;
private LinkedList<Video> videos = new LinkedList<Video>();
public Customer(int id, String name)
{
this.id = id;
this.name = name;
}
public void rent(Video video)
{
video.rent(this);
videos.add(video);
balance -= video.getPrice();
}
public String getName()
{
return name;
}
public double getBalance()
{
return balance;
}
public void videoReturn(Video video)
{
videos.remove(video);
}
public boolean matches(int id)
{
Name:
Student Number:
public void show()
{
System.out.println(toString());
}
public String toString()
{
String str = id + " " + name + " " + period + " days" ;
if(isOut())
str += " rented to " + renter.getName();
return str;
}
}
public class Customer
{
private int id;
private String name;
private double balance = 100.0;
private LinkedList<Video> videos = new LinkedList<Video>();
public Customer(int id, String name)
{
this.id = id;
this.name = name;
}
public void rent(Video video)
{
video.rent(this);
videos.add(video);
balance -= video.getPrice();
}
public String getName()
{
return name;
}
public double getBalance()
{
return balance;
}
public void videoReturn(Video video)
{
videos.remove(video);
}
public boolean matches(int id)
{
Name:
Student Number:
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
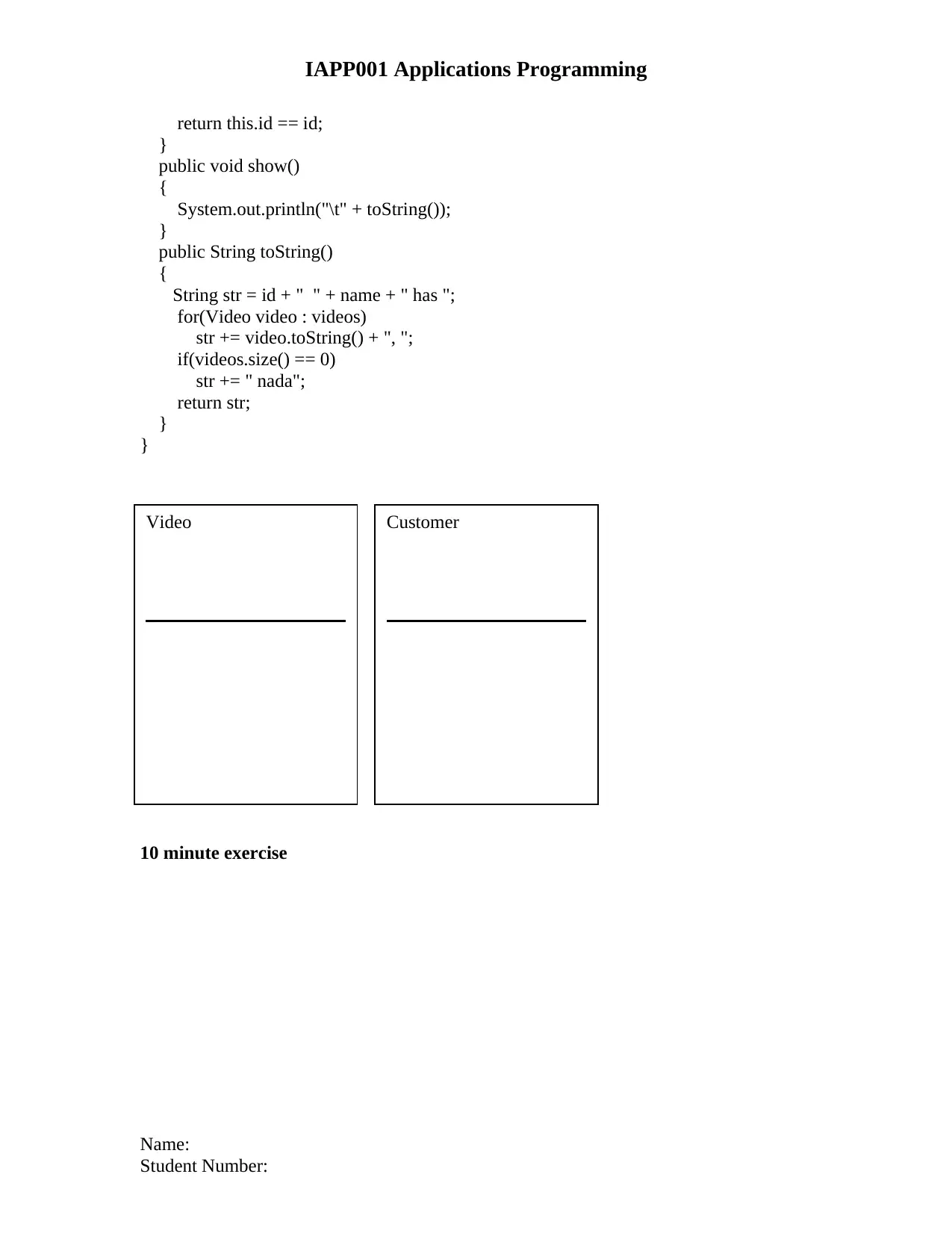
IAPP001 Applications Programming
return this.id == id;
}
public void show()
{
System.out.println("\t" + toString());
}
public String toString()
{
String str = id + " " + name + " has ";
for(Video video : videos)
str += video.toString() + ", ";
if(videos.size() == 0)
str += " nada";
return str;
}
}
10 minute exercise
Name:
Student Number:
CustomerVideo
return this.id == id;
}
public void show()
{
System.out.println("\t" + toString());
}
public String toString()
{
String str = id + " " + name + " has ";
for(Video video : videos)
str += video.toString() + ", ";
if(videos.size() == 0)
str += " nada";
return str;
}
}
10 minute exercise
Name:
Student Number:
CustomerVideo
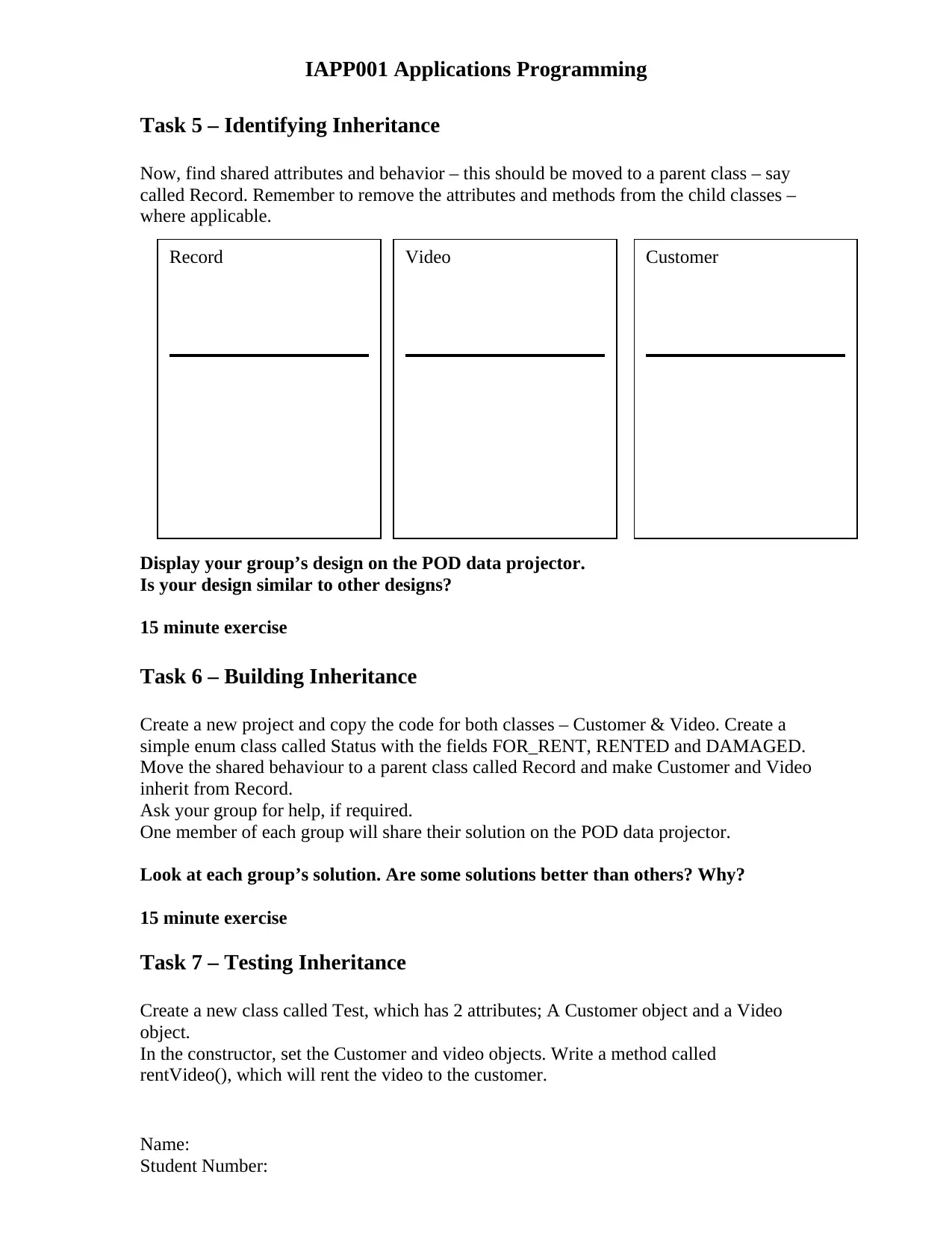
IAPP001 Applications Programming
Task 5 – Identifying Inheritance
Now, find shared attributes and behavior – this should be moved to a parent class – say
called Record. Remember to remove the attributes and methods from the child classes –
where applicable.
Display your group’s design on the POD data projector.
Is your design similar to other designs?
15 minute exercise
Task 6 – Building Inheritance
Create a new project and copy the code for both classes – Customer & Video. Create a
simple enum class called Status with the fields FOR_RENT, RENTED and DAMAGED.
Move the shared behaviour to a parent class called Record and make Customer and Video
inherit from Record.
Ask your group for help, if required.
One member of each group will share their solution on the POD data projector.
Look at each group’s solution. Are some solutions better than others? Why?
15 minute exercise
Task 7 – Testing Inheritance
Create a new class called Test, which has 2 attributes; A Customer object and a Video
object.
In the constructor, set the Customer and video objects. Write a method called
rentVideo(), which will rent the video to the customer.
Name:
Student Number:
Record Video Customer
Task 5 – Identifying Inheritance
Now, find shared attributes and behavior – this should be moved to a parent class – say
called Record. Remember to remove the attributes and methods from the child classes –
where applicable.
Display your group’s design on the POD data projector.
Is your design similar to other designs?
15 minute exercise
Task 6 – Building Inheritance
Create a new project and copy the code for both classes – Customer & Video. Create a
simple enum class called Status with the fields FOR_RENT, RENTED and DAMAGED.
Move the shared behaviour to a parent class called Record and make Customer and Video
inherit from Record.
Ask your group for help, if required.
One member of each group will share their solution on the POD data projector.
Look at each group’s solution. Are some solutions better than others? Why?
15 minute exercise
Task 7 – Testing Inheritance
Create a new class called Test, which has 2 attributes; A Customer object and a Video
object.
In the constructor, set the Customer and video objects. Write a method called
rentVideo(), which will rent the video to the customer.
Name:
Student Number:
Record Video Customer
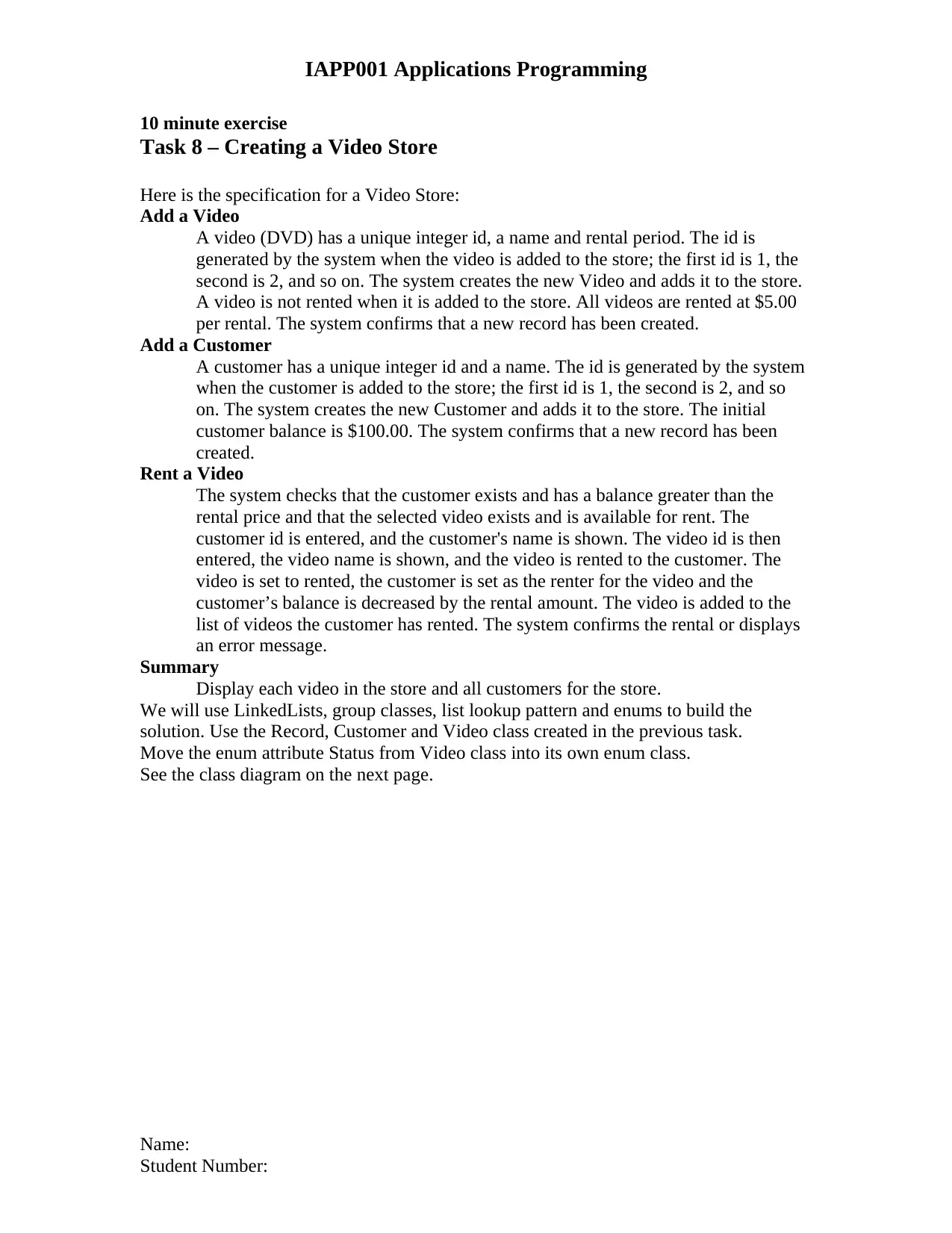
IAPP001 Applications Programming
10 minute exercise
Task 8 – Creating a Video Store
Here is the specification for a Video Store:
Add a Video
A video (DVD) has a unique integer id, a name and rental period. The id is
generated by the system when the video is added to the store; the first id is 1, the
second is 2, and so on. The system creates the new Video and adds it to the store.
A video is not rented when it is added to the store. All videos are rented at $5.00
per rental. The system confirms that a new record has been created.
Add a Customer
A customer has a unique integer id and a name. The id is generated by the system
when the customer is added to the store; the first id is 1, the second is 2, and so
on. The system creates the new Customer and adds it to the store. The initial
customer balance is $100.00. The system confirms that a new record has been
created.
Rent a Video
The system checks that the customer exists and has a balance greater than the
rental price and that the selected video exists and is available for rent. The
customer id is entered, and the customer's name is shown. The video id is then
entered, the video name is shown, and the video is rented to the customer. The
video is set to rented, the customer is set as the renter for the video and the
customer’s balance is decreased by the rental amount. The video is added to the
list of videos the customer has rented. The system confirms the rental or displays
an error message.
Summary
Display each video in the store and all customers for the store.
We will use LinkedLists, group classes, list lookup pattern and enums to build the
solution. Use the Record, Customer and Video class created in the previous task.
Move the enum attribute Status from Video class into its own enum class.
See the class diagram on the next page.
Name:
Student Number:
10 minute exercise
Task 8 – Creating a Video Store
Here is the specification for a Video Store:
Add a Video
A video (DVD) has a unique integer id, a name and rental period. The id is
generated by the system when the video is added to the store; the first id is 1, the
second is 2, and so on. The system creates the new Video and adds it to the store.
A video is not rented when it is added to the store. All videos are rented at $5.00
per rental. The system confirms that a new record has been created.
Add a Customer
A customer has a unique integer id and a name. The id is generated by the system
when the customer is added to the store; the first id is 1, the second is 2, and so
on. The system creates the new Customer and adds it to the store. The initial
customer balance is $100.00. The system confirms that a new record has been
created.
Rent a Video
The system checks that the customer exists and has a balance greater than the
rental price and that the selected video exists and is available for rent. The
customer id is entered, and the customer's name is shown. The video id is then
entered, the video name is shown, and the video is rented to the customer. The
video is set to rented, the customer is set as the renter for the video and the
customer’s balance is decreased by the rental amount. The video is added to the
list of videos the customer has rented. The system confirms the rental or displays
an error message.
Summary
Display each video in the store and all customers for the store.
We will use LinkedLists, group classes, list lookup pattern and enums to build the
solution. Use the Record, Customer and Video class created in the previous task.
Move the enum attribute Status from Video class into its own enum class.
See the class diagram on the next page.
Name:
Student Number:
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
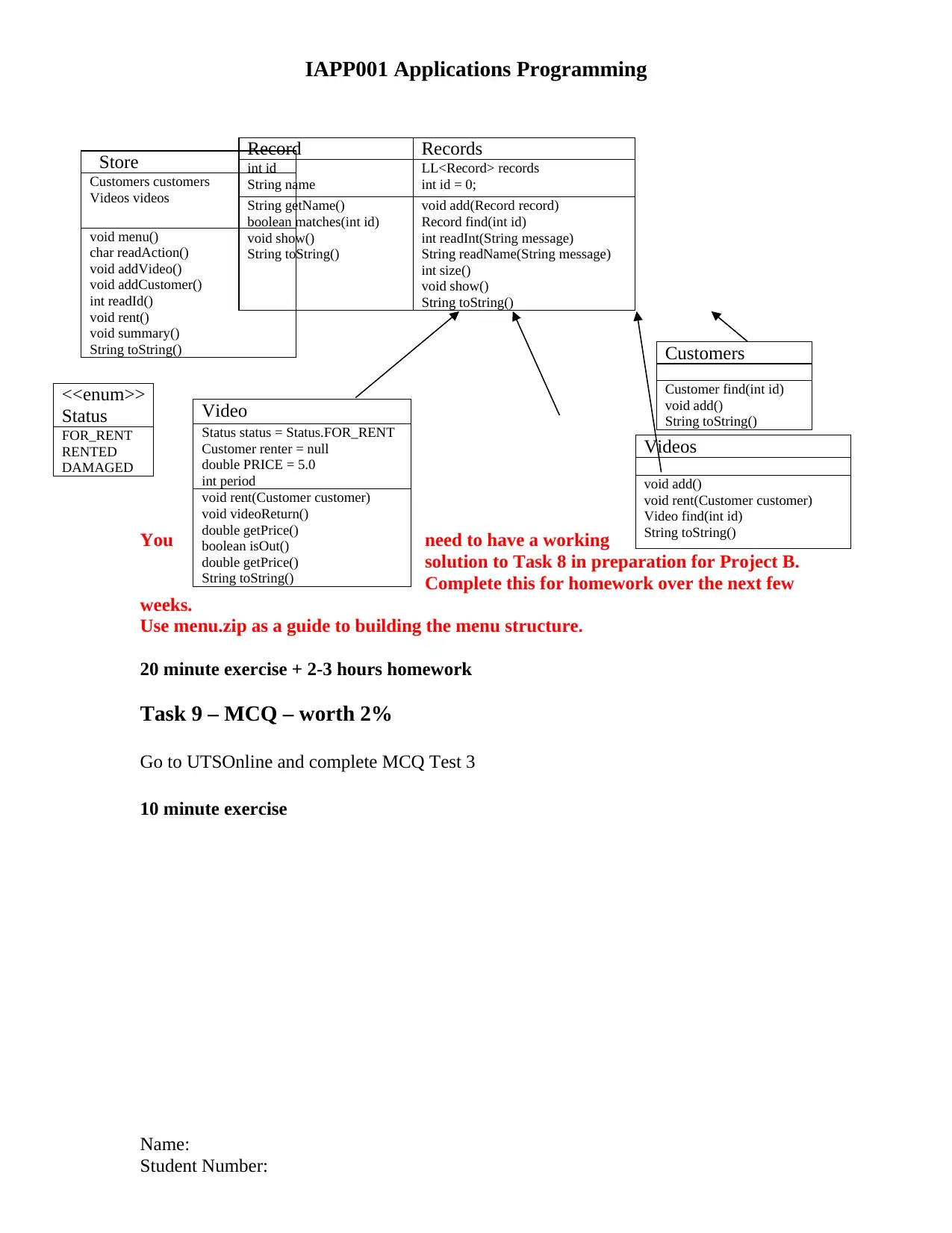
IAPP001 Applications Programming
Record Records
int id
String name
LL<Record> records
int id = 0;
String getName()
boolean matches(int id)
void show()
String toString()
void add(Record record)
Record find(int id)
int readInt(String message)
String readName(String message)
int size()
void show()
String toString()
You need to have a working
solution to Task 8 in preparation for Project B.
Complete this for homework over the next few
weeks.
Use menu.zip as a guide to building the menu structure.
20 minute exercise + 2-3 hours homework
Task 9 – MCQ – worth 2%
Go to UTSOnline and complete MCQ Test 3
10 minute exercise
Name:
Student Number:
Store
Customers customers
Videos videos
void menu()
char readAction()
void addVideo()
void addCustomer()
int readId()
void rent()
void summary()
String toString() Customers
Customer find(int id)
void add()
String toString()
Video
Status status = Status.FOR_RENT
Customer renter = null
double PRICE = 5.0
int period
void rent(Customer customer)
void videoReturn()
double getPrice()
boolean isOut()
double getPrice()
String toString()
<<enum>>
Status
FOR_RENT
RENTED
DAMAGED
Videos
void add()
void rent(Customer customer)
Video find(int id)
String toString()
Record Records
int id
String name
LL<Record> records
int id = 0;
String getName()
boolean matches(int id)
void show()
String toString()
void add(Record record)
Record find(int id)
int readInt(String message)
String readName(String message)
int size()
void show()
String toString()
You need to have a working
solution to Task 8 in preparation for Project B.
Complete this for homework over the next few
weeks.
Use menu.zip as a guide to building the menu structure.
20 minute exercise + 2-3 hours homework
Task 9 – MCQ – worth 2%
Go to UTSOnline and complete MCQ Test 3
10 minute exercise
Name:
Student Number:
Store
Customers customers
Videos videos
void menu()
char readAction()
void addVideo()
void addCustomer()
int readId()
void rent()
void summary()
String toString() Customers
Customer find(int id)
void add()
String toString()
Video
Status status = Status.FOR_RENT
Customer renter = null
double PRICE = 5.0
int period
void rent(Customer customer)
void videoReturn()
double getPrice()
boolean isOut()
double getPrice()
String toString()
<<enum>>
Status
FOR_RENT
RENTED
DAMAGED
Videos
void add()
void rent(Customer customer)
Video find(int id)
String toString()
1 out of 8
Related Documents
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.