Advanced Programming Assignment 2: Design Patterns and Application
VerifiedAdded on 2021/11/23
|28
|4015
|256
Report
AI Summary
This report presents a comprehensive solution to an advanced programming assignment. The first part of the assignment involves building an application for a Product Management System, derived from a UML class diagram. The application, developed using Java, includes features to manage technology and houseware products, such as adding, displaying, updating, and deleting product information. The second part of the report delves into design patterns, explaining their purpose and categorization, with a focus on creational, structural, and behavioral patterns. Each pattern is defined, and its applications and benefits are detailed, accompanied by relevant examples to illustrate their practical implementation and use cases within software engineering, covering patterns like Factory, Singleton, Observer and many more. The report concludes with a discussion on the overall benefits of design patterns in creating flexible, reusable, and maintainable code.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
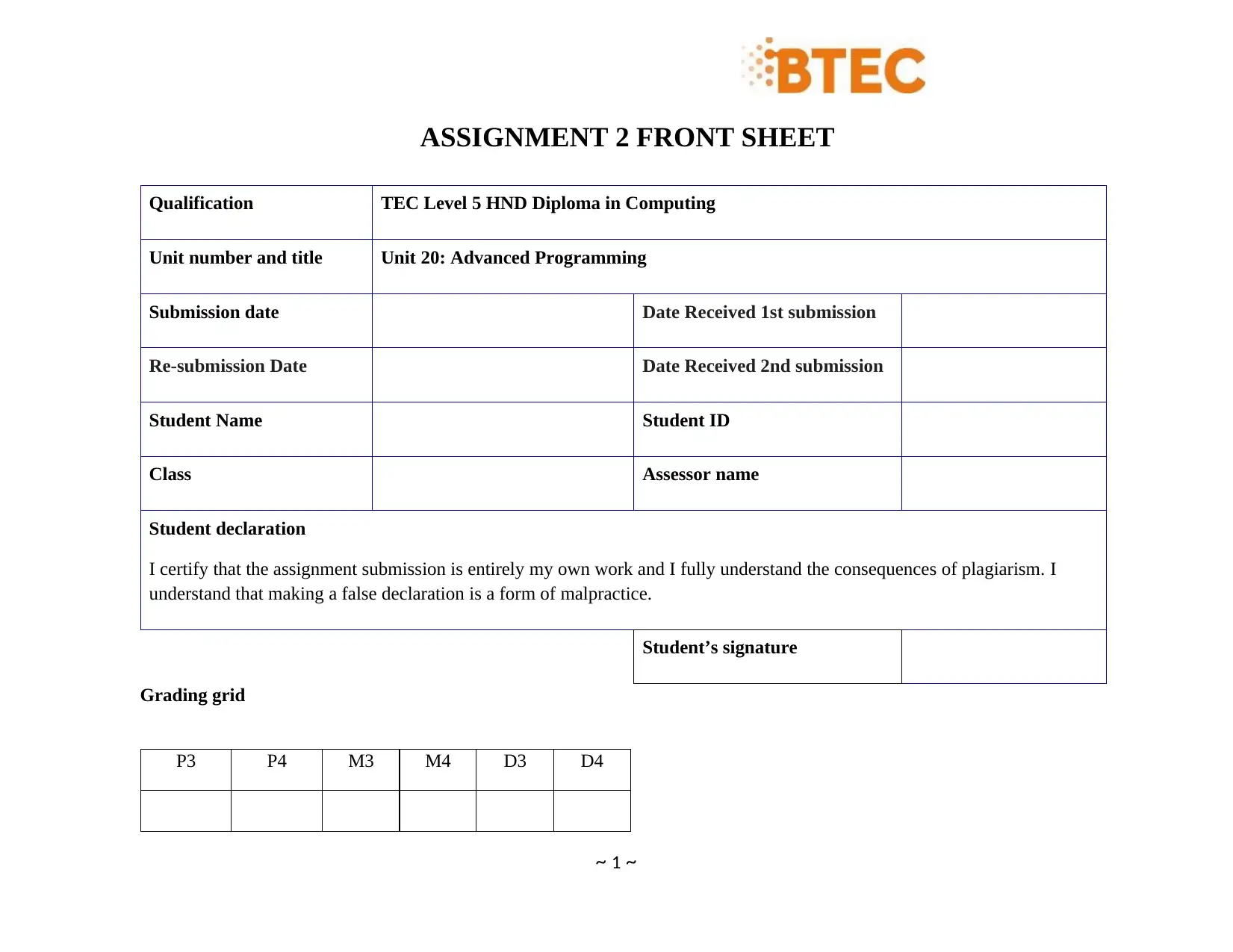
ASSIGNMENT 2 FRONT SHEET
Qualification TEC Level 5 HND Diploma in Computing
Unit number and title Unit 20: Advanced Programming
Submission date Date Received 1st submission
Re-submission Date Date Received 2nd submission
Student Name Student ID
Class Assessor name
Student declaration
I certify that the assignment submission is entirely my own work and I fully understand the consequences of plagiarism. I
understand that making a false declaration is a form of malpractice.
Student’s signature
Grading grid
P3 P4 M3 M4 D3 D4
~ 1 ~
Qualification TEC Level 5 HND Diploma in Computing
Unit number and title Unit 20: Advanced Programming
Submission date Date Received 1st submission
Re-submission Date Date Received 2nd submission
Student Name Student ID
Class Assessor name
Student declaration
I certify that the assignment submission is entirely my own work and I fully understand the consequences of plagiarism. I
understand that making a false declaration is a form of malpractice.
Student’s signature
Grading grid
P3 P4 M3 M4 D3 D4
~ 1 ~
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
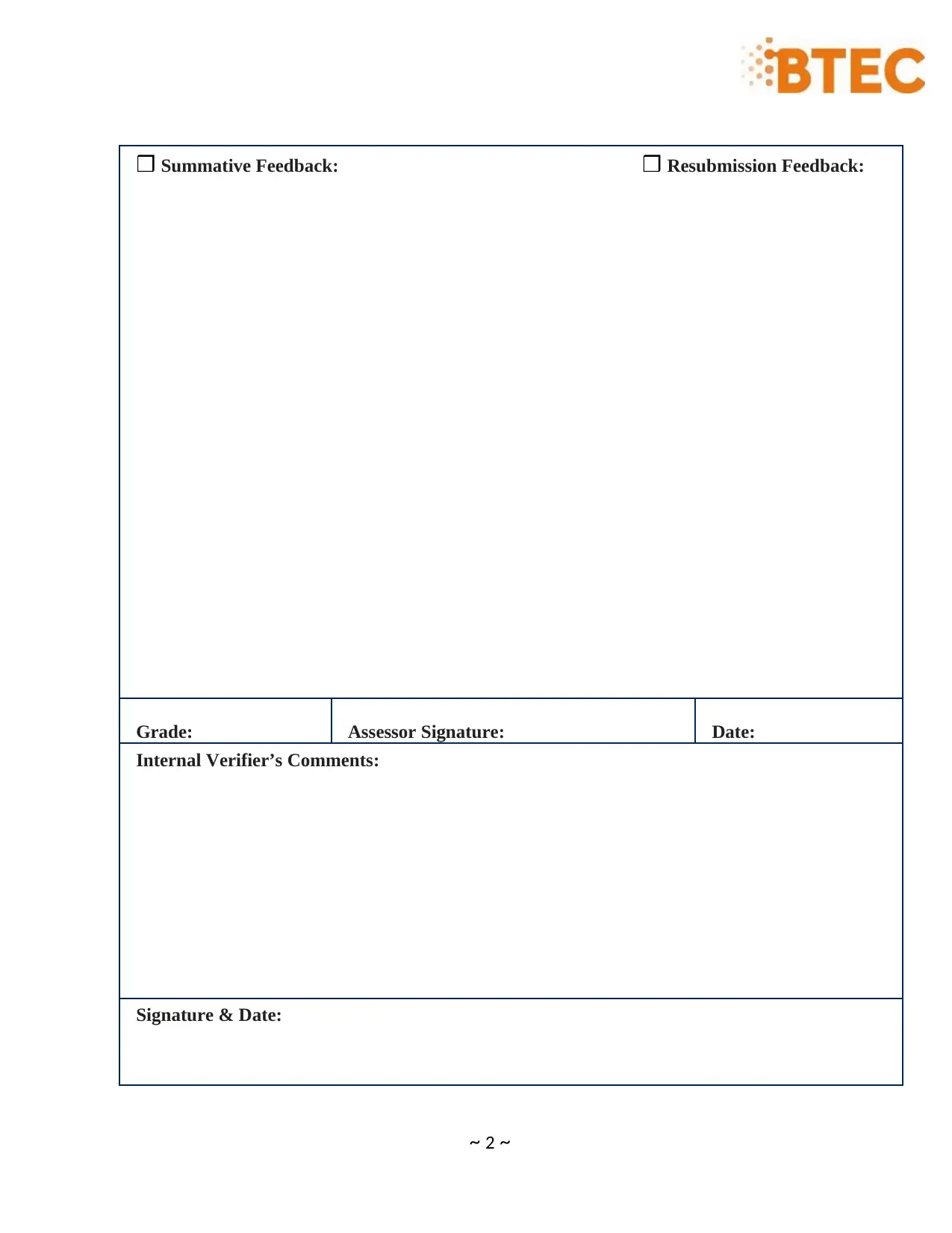
❒ Summative Feedback: ❒ Resubmission Feedback:
Grade: Assessor Signature: Date:
Internal Verifier’s Comments:
Signature & Date:
~ 2 ~
Grade: Assessor Signature: Date:
Internal Verifier’s Comments:
Signature & Date:
~ 2 ~

~ 3 ~
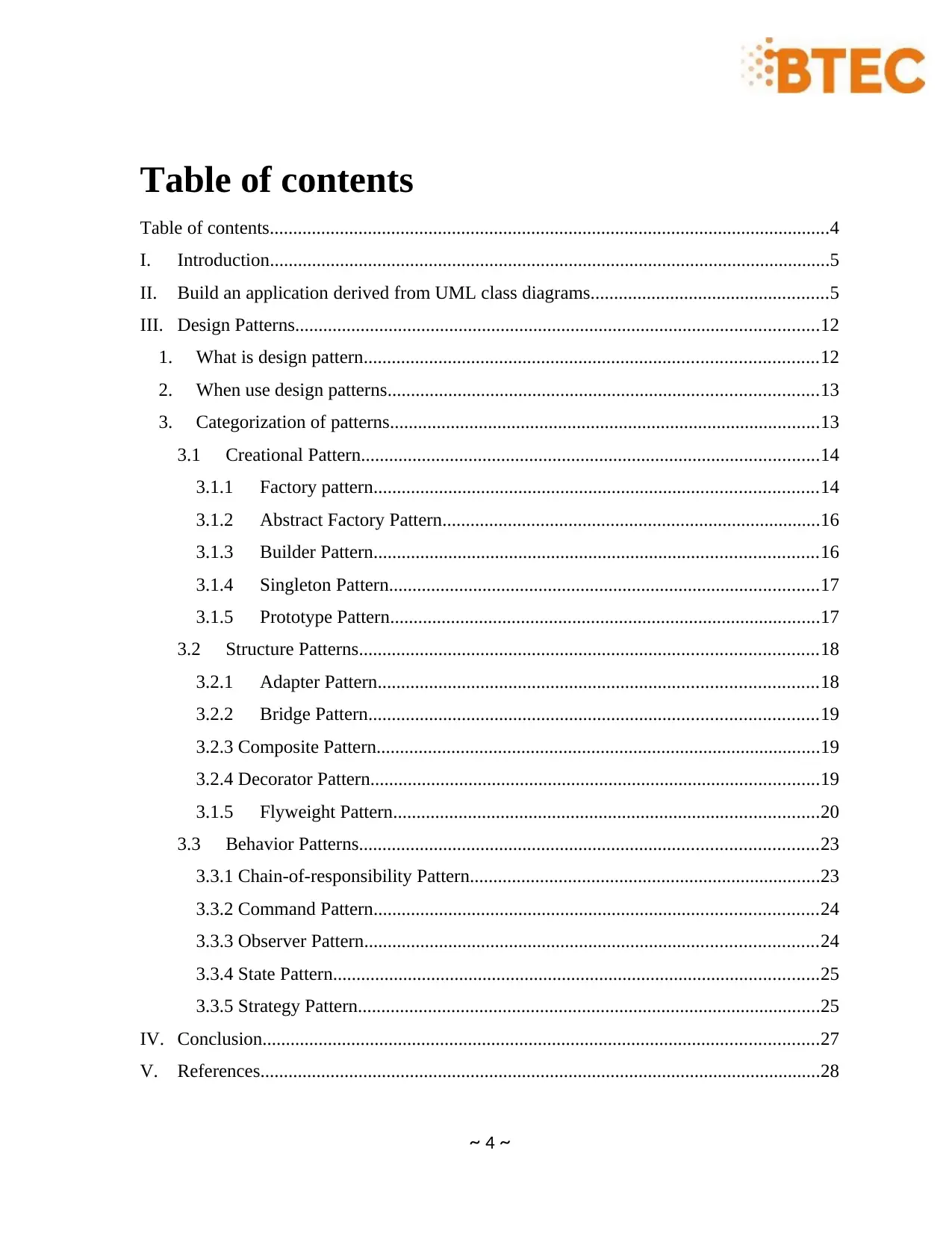
Table of contents
Table of contents........................................................................................................................4
I. Introduction........................................................................................................................5
II. Build an application derived from UML class diagrams...................................................5
III. Design Patterns................................................................................................................12
1. What is design pattern.................................................................................................12
2. When use design patterns............................................................................................13
3. Categorization of patterns............................................................................................13
3.1 Creational Pattern..................................................................................................14
3.1.1 Factory pattern...............................................................................................14
3.1.2 Abstract Factory Pattern.................................................................................16
3.1.3 Builder Pattern...............................................................................................16
3.1.4 Singleton Pattern............................................................................................17
3.1.5 Prototype Pattern............................................................................................17
3.2 Structure Patterns..................................................................................................18
3.2.1 Adapter Pattern..............................................................................................18
3.2.2 Bridge Pattern................................................................................................19
3.2.3 Composite Pattern...............................................................................................19
3.2.4 Decorator Pattern................................................................................................19
3.1.5 Flyweight Pattern...........................................................................................20
3.3 Behavior Patterns..................................................................................................23
3.3.1 Chain-of-responsibility Pattern...........................................................................23
3.3.2 Command Pattern...............................................................................................24
3.3.3 Observer Pattern.................................................................................................24
3.3.4 State Pattern........................................................................................................25
3.3.5 Strategy Pattern...................................................................................................25
IV. Conclusion.......................................................................................................................27
V. References........................................................................................................................28
~ 4 ~
Table of contents........................................................................................................................4
I. Introduction........................................................................................................................5
II. Build an application derived from UML class diagrams...................................................5
III. Design Patterns................................................................................................................12
1. What is design pattern.................................................................................................12
2. When use design patterns............................................................................................13
3. Categorization of patterns............................................................................................13
3.1 Creational Pattern..................................................................................................14
3.1.1 Factory pattern...............................................................................................14
3.1.2 Abstract Factory Pattern.................................................................................16
3.1.3 Builder Pattern...............................................................................................16
3.1.4 Singleton Pattern............................................................................................17
3.1.5 Prototype Pattern............................................................................................17
3.2 Structure Patterns..................................................................................................18
3.2.1 Adapter Pattern..............................................................................................18
3.2.2 Bridge Pattern................................................................................................19
3.2.3 Composite Pattern...............................................................................................19
3.2.4 Decorator Pattern................................................................................................19
3.1.5 Flyweight Pattern...........................................................................................20
3.3 Behavior Patterns..................................................................................................23
3.3.1 Chain-of-responsibility Pattern...........................................................................23
3.3.2 Command Pattern...............................................................................................24
3.3.3 Observer Pattern.................................................................................................24
3.3.4 State Pattern........................................................................................................25
3.3.5 Strategy Pattern...................................................................................................25
IV. Conclusion.......................................................................................................................27
V. References........................................................................................................................28
~ 4 ~
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
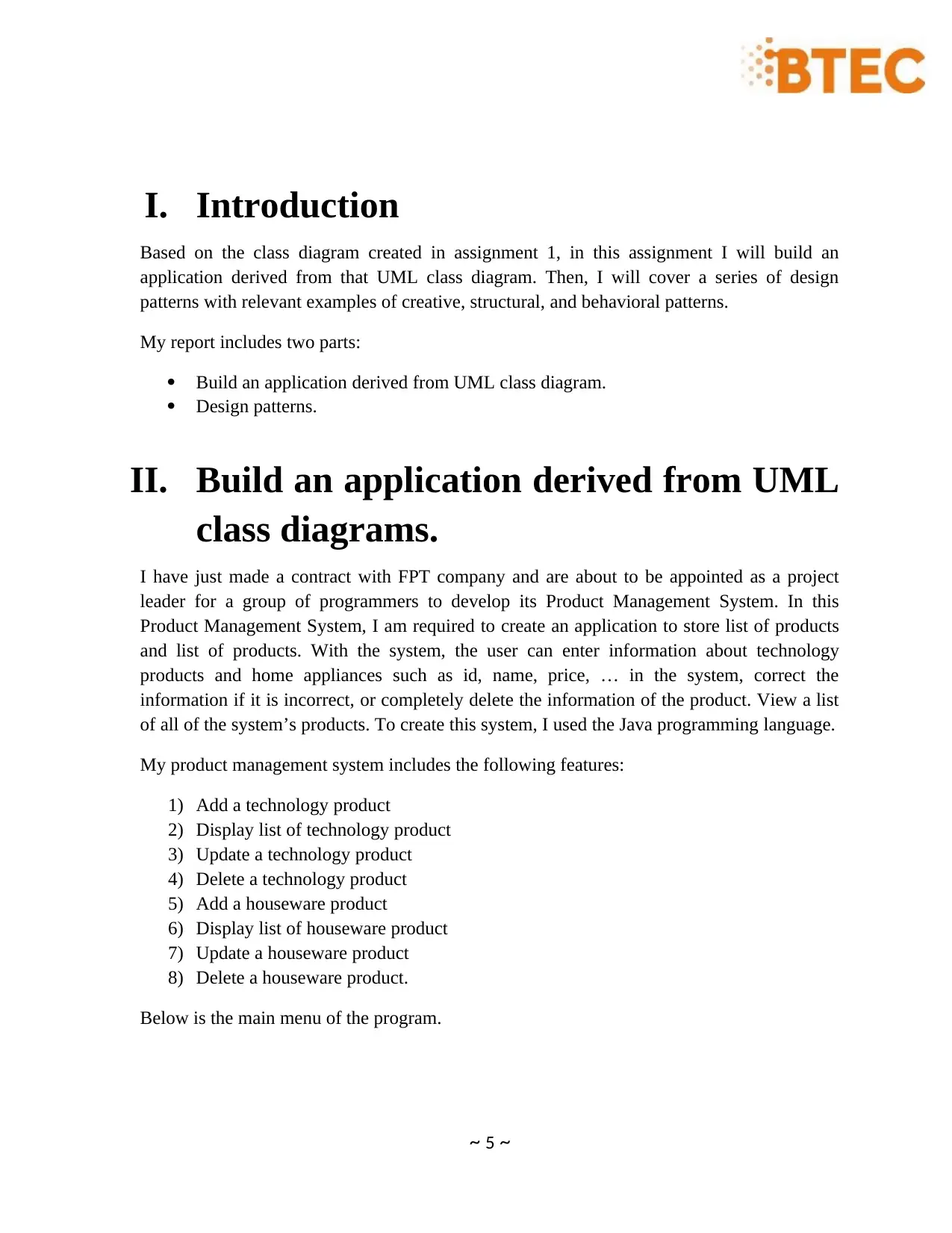
I. Introduction
Based on the class diagram created in assignment 1, in this assignment I will build an
application derived from that UML class diagram. Then, I will cover a series of design
patterns with relevant examples of creative, structural, and behavioral patterns.
My report includes two parts:
Build an application derived from UML class diagram.
Design patterns.
II. Build an application derived from UML
class diagrams.
I have just made a contract with FPT company and are about to be appointed as a project
leader for a group of programmers to develop its Product Management System. In this
Product Management System, I am required to create an application to store list of products
and list of products. With the system, the user can enter information about technology
products and home appliances such as id, name, price, … in the system, correct the
information if it is incorrect, or completely delete the information of the product. View a list
of all of the system’s products. To create this system, I used the Java programming language.
My product management system includes the following features:
1) Add a technology product
2) Display list of technology product
3) Update a technology product
4) Delete a technology product
5) Add a houseware product
6) Display list of houseware product
7) Update a houseware product
8) Delete a houseware product.
Below is the main menu of the program.
~ 5 ~
Based on the class diagram created in assignment 1, in this assignment I will build an
application derived from that UML class diagram. Then, I will cover a series of design
patterns with relevant examples of creative, structural, and behavioral patterns.
My report includes two parts:
Build an application derived from UML class diagram.
Design patterns.
II. Build an application derived from UML
class diagrams.
I have just made a contract with FPT company and are about to be appointed as a project
leader for a group of programmers to develop its Product Management System. In this
Product Management System, I am required to create an application to store list of products
and list of products. With the system, the user can enter information about technology
products and home appliances such as id, name, price, … in the system, correct the
information if it is incorrect, or completely delete the information of the product. View a list
of all of the system’s products. To create this system, I used the Java programming language.
My product management system includes the following features:
1) Add a technology product
2) Display list of technology product
3) Update a technology product
4) Delete a technology product
5) Add a houseware product
6) Display list of houseware product
7) Update a houseware product
8) Delete a houseware product.
Below is the main menu of the program.
~ 5 ~
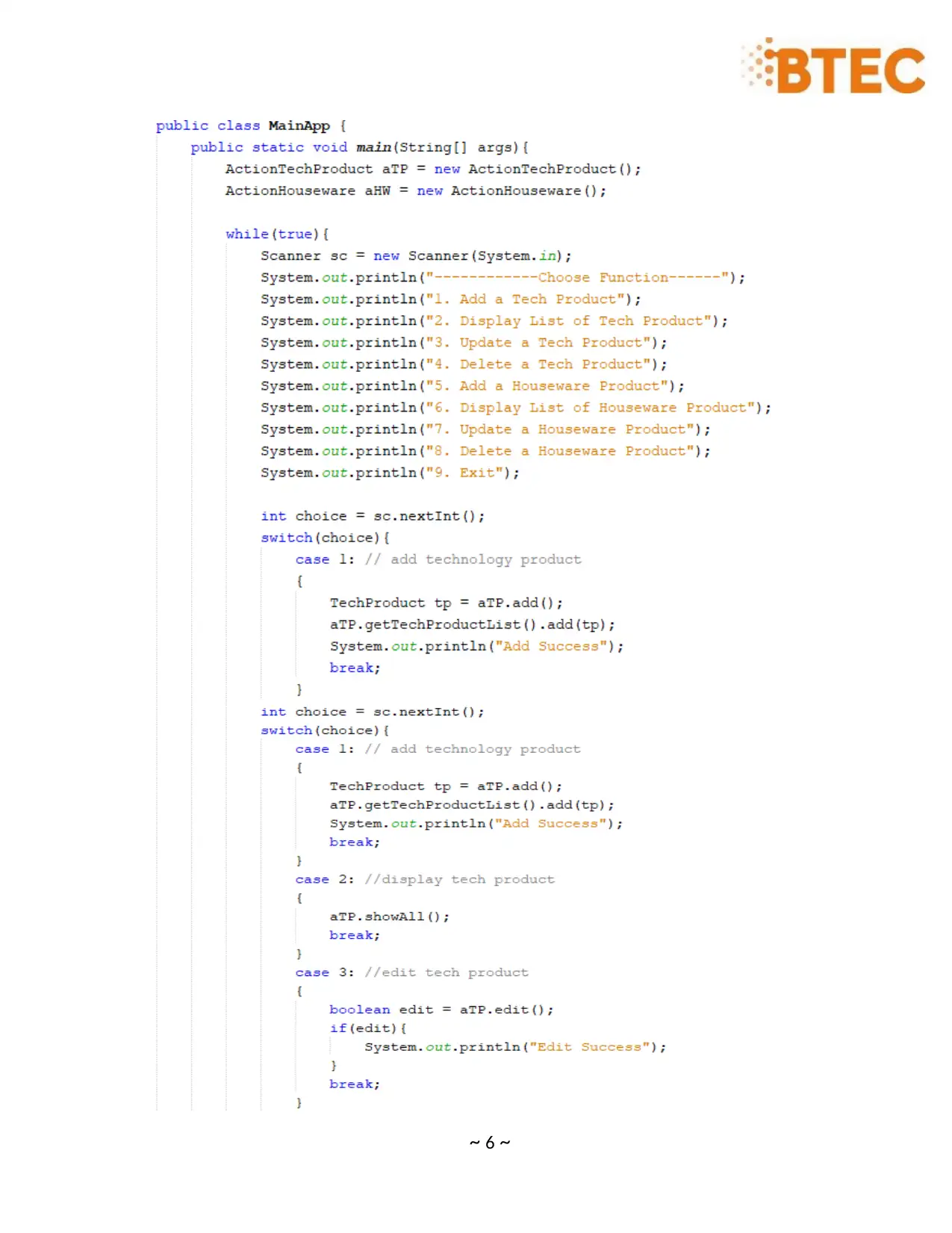
~ 6 ~
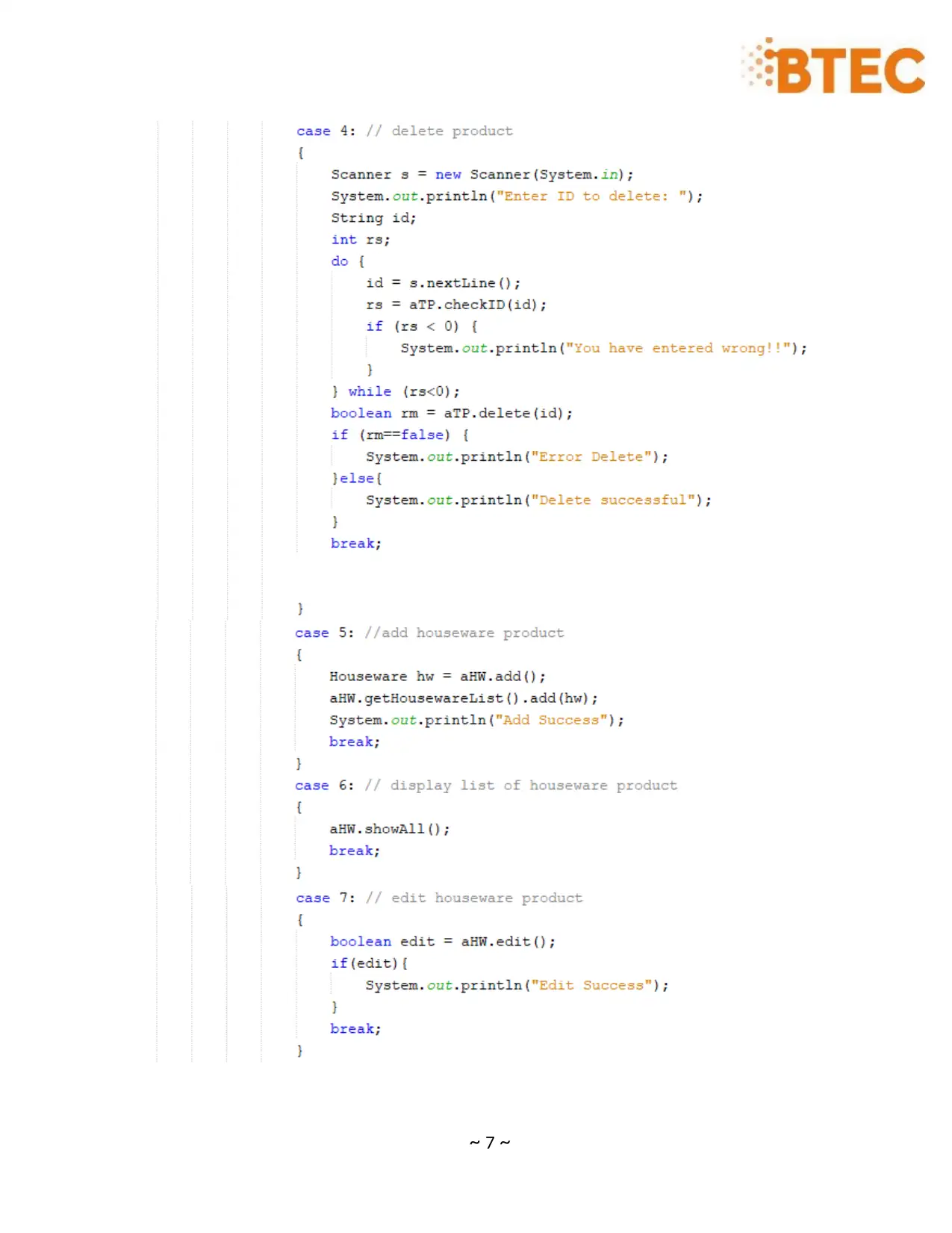
~ 7 ~
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
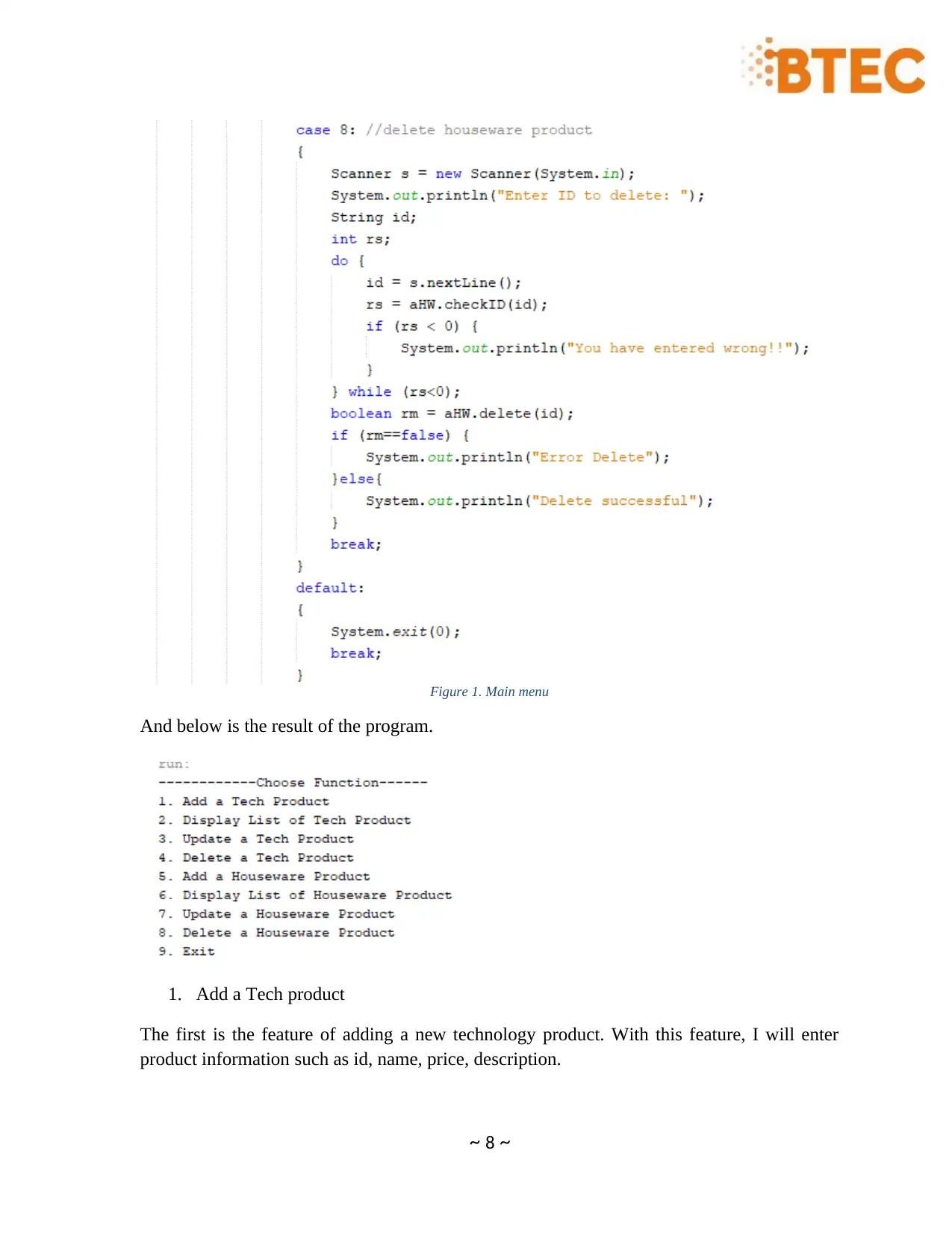
Figure 1. Main menu
And below is the result of the program.
1. Add a Tech product
The first is the feature of adding a new technology product. With this feature, I will enter
product information such as id, name, price, description.
~ 8 ~
And below is the result of the program.
1. Add a Tech product
The first is the feature of adding a new technology product. With this feature, I will enter
product information such as id, name, price, description.
~ 8 ~
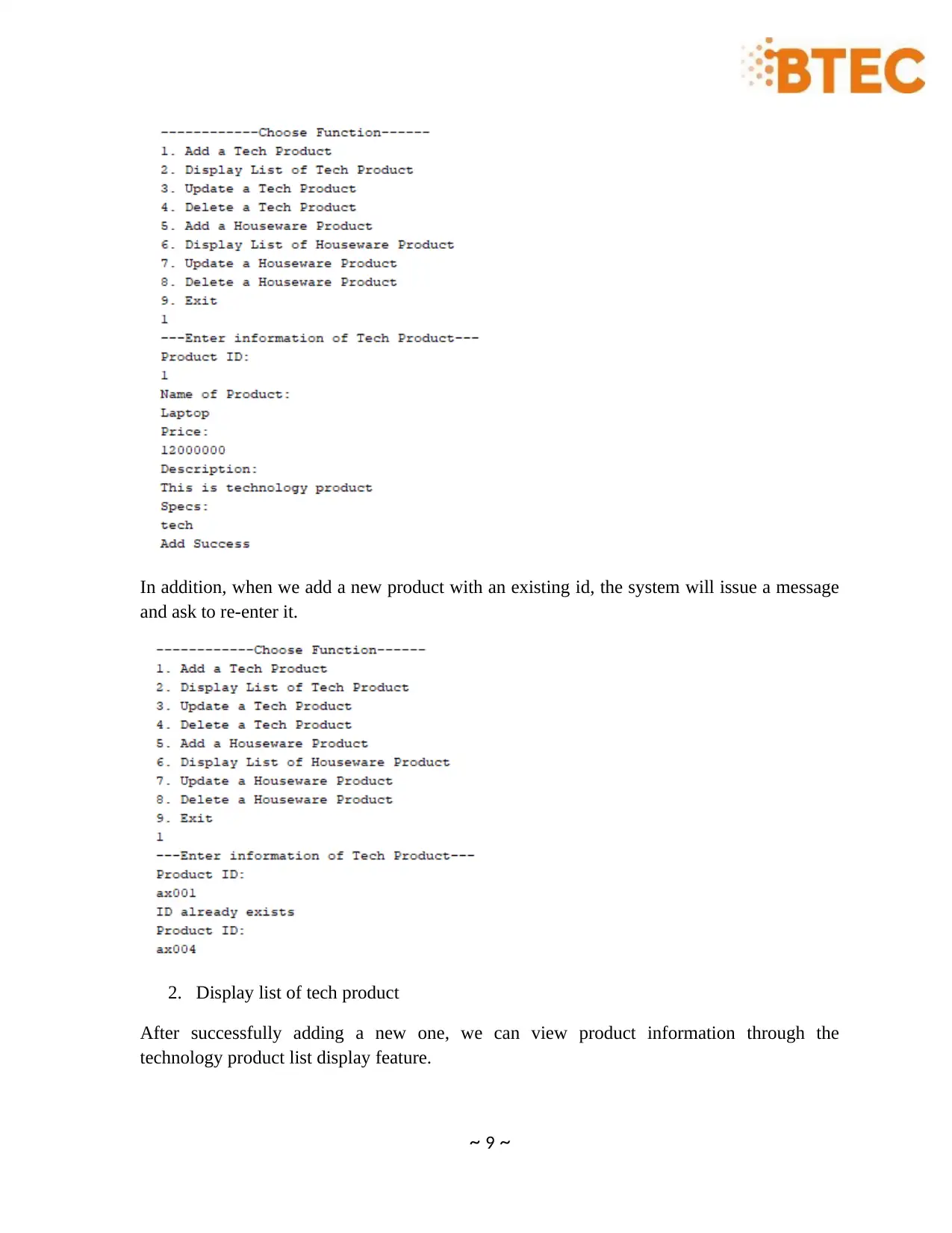
In addition, when we add a new product with an existing id, the system will issue a message
and ask to re-enter it.
2. Display list of tech product
After successfully adding a new one, we can view product information through the
technology product list display feature.
~ 9 ~
and ask to re-enter it.
2. Display list of tech product
After successfully adding a new one, we can view product information through the
technology product list display feature.
~ 9 ~
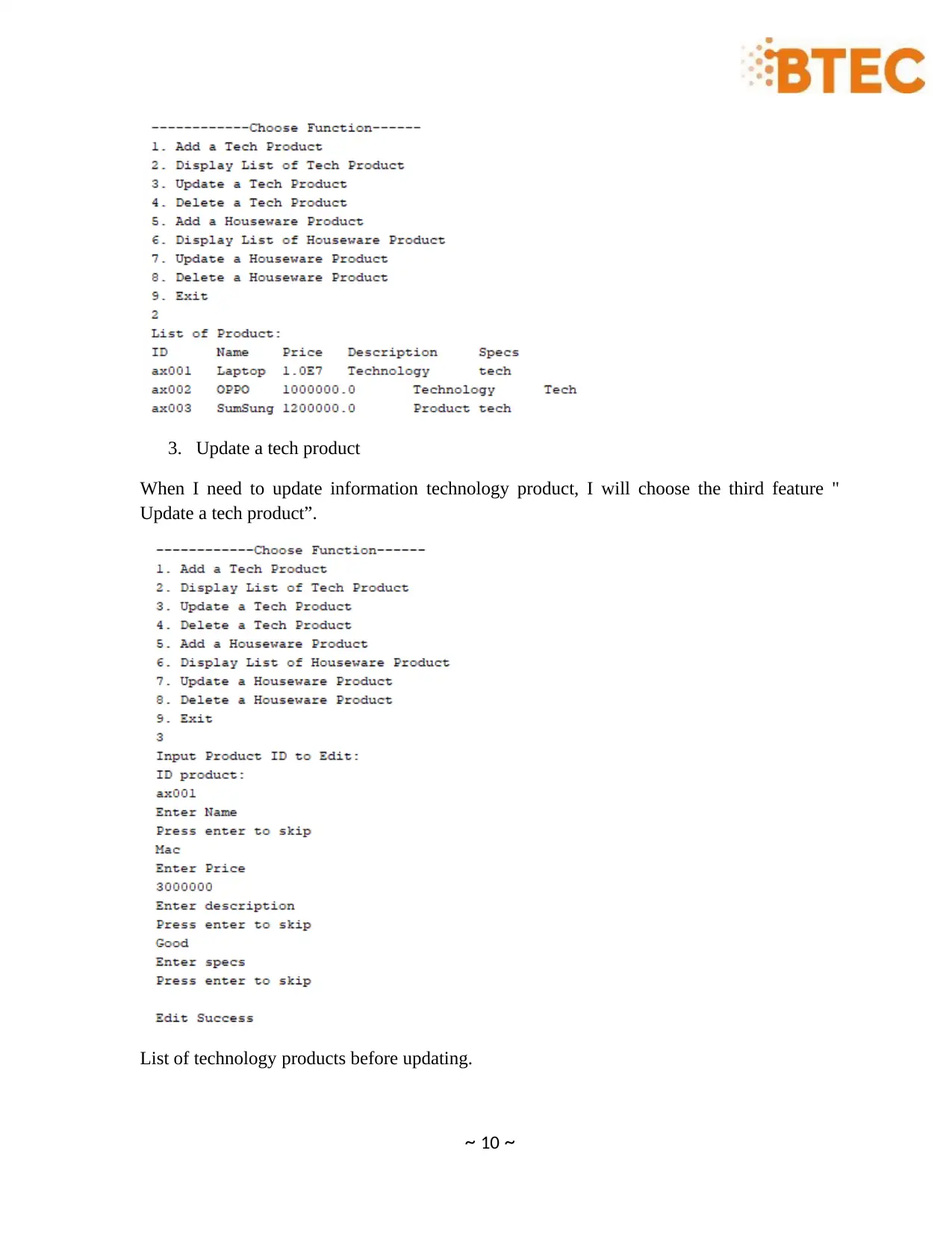
3. Update a tech product
When I need to update information technology product, I will choose the third feature "
Update a tech product”.
List of technology products before updating.
~ 10 ~
When I need to update information technology product, I will choose the third feature "
Update a tech product”.
List of technology products before updating.
~ 10 ~
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
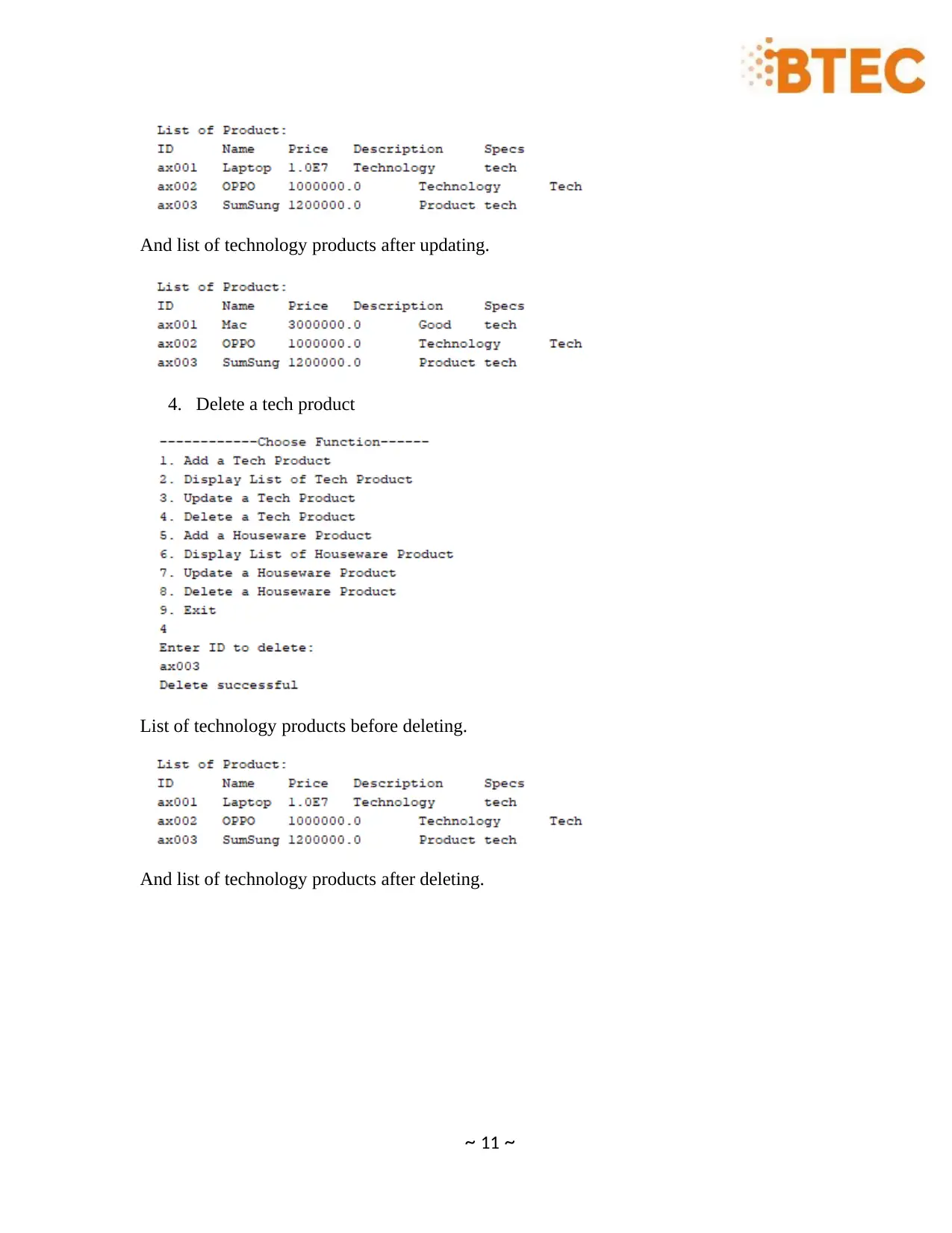
And list of technology products after updating.
4. Delete a tech product
List of technology products before deleting.
And list of technology products after deleting.
~ 11 ~
4. Delete a tech product
List of technology products before deleting.
And list of technology products after deleting.
~ 11 ~
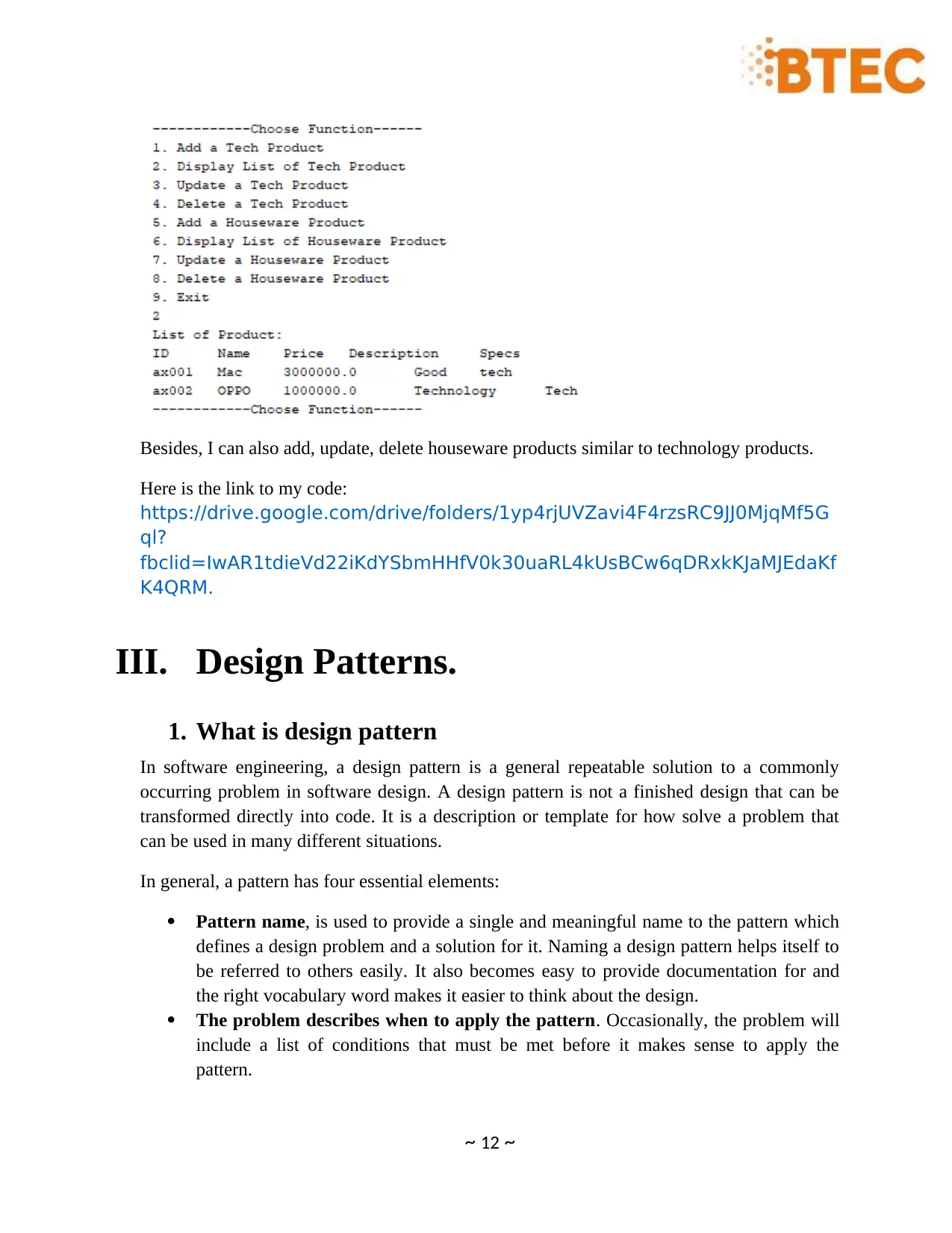
Besides, I can also add, update, delete houseware products similar to technology products.
Here is the link to my code:
https://drive.google.com/drive/folders/1yp4rjUVZavi4F4rzsRC9JJ0MjqMf5G
ql?
fbclid=IwAR1tdieVd22iKdYSbmHHfV0k30uaRL4kUsBCw6qDRxkKJaMJEdaKf
K4QRM.
III. Design Patterns.
1. What is design pattern
In software engineering, a design pattern is a general repeatable solution to a commonly
occurring problem in software design. A design pattern is not a finished design that can be
transformed directly into code. It is a description or template for how solve a problem that
can be used in many different situations.
In general, a pattern has four essential elements:
Pattern name, is used to provide a single and meaningful name to the pattern which
defines a design problem and a solution for it. Naming a design pattern helps itself to
be referred to others easily. It also becomes easy to provide documentation for and
the right vocabulary word makes it easier to think about the design.
The problem describes when to apply the pattern. Occasionally, the problem will
include a list of conditions that must be met before it makes sense to apply the
pattern.
~ 12 ~
Here is the link to my code:
https://drive.google.com/drive/folders/1yp4rjUVZavi4F4rzsRC9JJ0MjqMf5G
ql?
fbclid=IwAR1tdieVd22iKdYSbmHHfV0k30uaRL4kUsBCw6qDRxkKJaMJEdaKf
K4QRM.
III. Design Patterns.
1. What is design pattern
In software engineering, a design pattern is a general repeatable solution to a commonly
occurring problem in software design. A design pattern is not a finished design that can be
transformed directly into code. It is a description or template for how solve a problem that
can be used in many different situations.
In general, a pattern has four essential elements:
Pattern name, is used to provide a single and meaningful name to the pattern which
defines a design problem and a solution for it. Naming a design pattern helps itself to
be referred to others easily. It also becomes easy to provide documentation for and
the right vocabulary word makes it easier to think about the design.
The problem describes when to apply the pattern. Occasionally, the problem will
include a list of conditions that must be met before it makes sense to apply the
pattern.
~ 12 ~
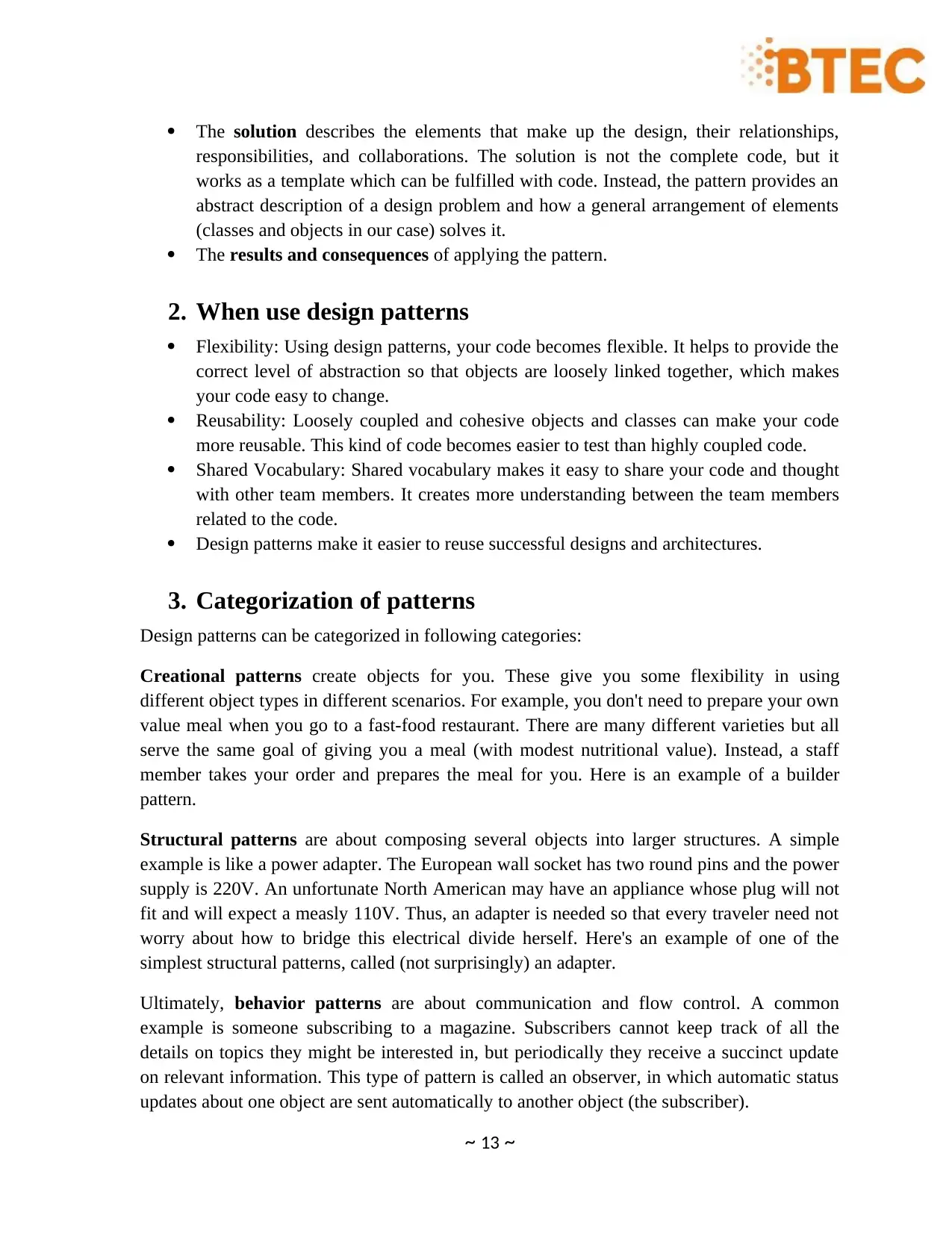
The solution describes the elements that make up the design, their relationships,
responsibilities, and collaborations. The solution is not the complete code, but it
works as a template which can be fulfilled with code. Instead, the pattern provides an
abstract description of a design problem and how a general arrangement of elements
(classes and objects in our case) solves it.
The results and consequences of applying the pattern.
2. When use design patterns
Flexibility: Using design patterns, your code becomes flexible. It helps to provide the
correct level of abstraction so that objects are loosely linked together, which makes
your code easy to change.
Reusability: Loosely coupled and cohesive objects and classes can make your code
more reusable. This kind of code becomes easier to test than highly coupled code.
Shared Vocabulary: Shared vocabulary makes it easy to share your code and thought
with other team members. It creates more understanding between the team members
related to the code.
Design patterns make it easier to reuse successful designs and architectures.
3. Categorization of patterns
Design patterns can be categorized in following categories:
Creational patterns create objects for you. These give you some flexibility in using
different object types in different scenarios. For example, you don't need to prepare your own
value meal when you go to a fast-food restaurant. There are many different varieties but all
serve the same goal of giving you a meal (with modest nutritional value). Instead, a staff
member takes your order and prepares the meal for you. Here is an example of a builder
pattern.
Structural patterns are about composing several objects into larger structures. A simple
example is like a power adapter. The European wall socket has two round pins and the power
supply is 220V. An unfortunate North American may have an appliance whose plug will not
fit and will expect a measly 110V. Thus, an adapter is needed so that every traveler need not
worry about how to bridge this electrical divide herself. Here's an example of one of the
simplest structural patterns, called (not surprisingly) an adapter.
Ultimately, behavior patterns are about communication and flow control. A common
example is someone subscribing to a magazine. Subscribers cannot keep track of all the
details on topics they might be interested in, but periodically they receive a succinct update
on relevant information. This type of pattern is called an observer, in which automatic status
updates about one object are sent automatically to another object (the subscriber).
~ 13 ~
responsibilities, and collaborations. The solution is not the complete code, but it
works as a template which can be fulfilled with code. Instead, the pattern provides an
abstract description of a design problem and how a general arrangement of elements
(classes and objects in our case) solves it.
The results and consequences of applying the pattern.
2. When use design patterns
Flexibility: Using design patterns, your code becomes flexible. It helps to provide the
correct level of abstraction so that objects are loosely linked together, which makes
your code easy to change.
Reusability: Loosely coupled and cohesive objects and classes can make your code
more reusable. This kind of code becomes easier to test than highly coupled code.
Shared Vocabulary: Shared vocabulary makes it easy to share your code and thought
with other team members. It creates more understanding between the team members
related to the code.
Design patterns make it easier to reuse successful designs and architectures.
3. Categorization of patterns
Design patterns can be categorized in following categories:
Creational patterns create objects for you. These give you some flexibility in using
different object types in different scenarios. For example, you don't need to prepare your own
value meal when you go to a fast-food restaurant. There are many different varieties but all
serve the same goal of giving you a meal (with modest nutritional value). Instead, a staff
member takes your order and prepares the meal for you. Here is an example of a builder
pattern.
Structural patterns are about composing several objects into larger structures. A simple
example is like a power adapter. The European wall socket has two round pins and the power
supply is 220V. An unfortunate North American may have an appliance whose plug will not
fit and will expect a measly 110V. Thus, an adapter is needed so that every traveler need not
worry about how to bridge this electrical divide herself. Here's an example of one of the
simplest structural patterns, called (not surprisingly) an adapter.
Ultimately, behavior patterns are about communication and flow control. A common
example is someone subscribing to a magazine. Subscribers cannot keep track of all the
details on topics they might be interested in, but periodically they receive a succinct update
on relevant information. This type of pattern is called an observer, in which automatic status
updates about one object are sent automatically to another object (the subscriber).
~ 13 ~
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
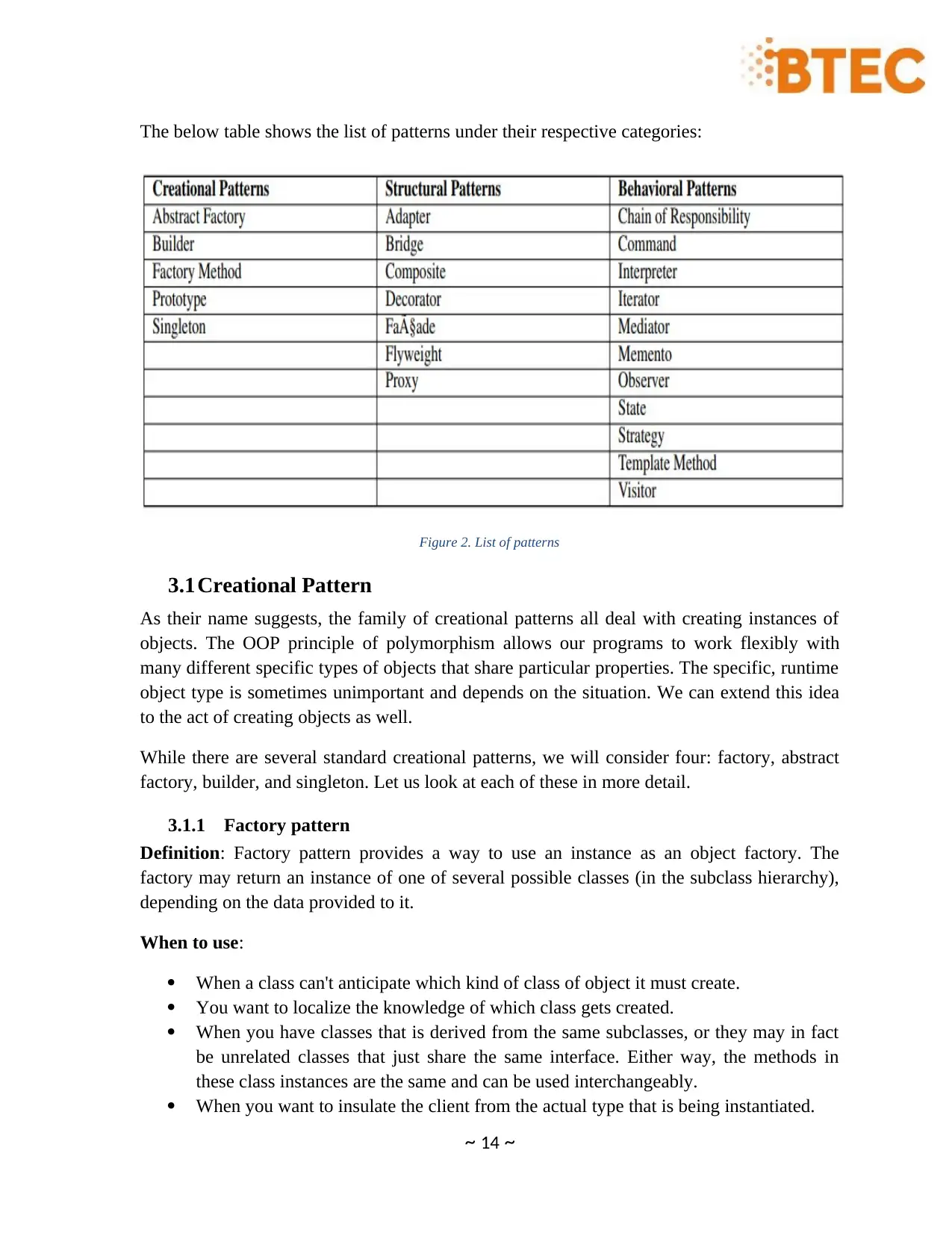
The below table shows the list of patterns under their respective categories:
Figure 2. List of patterns
3.1Creational Pattern
As their name suggests, the family of creational patterns all deal with creating instances of
objects. The OOP principle of polymorphism allows our programs to work flexibly with
many different specific types of objects that share particular properties. The specific, runtime
object type is sometimes unimportant and depends on the situation. We can extend this idea
to the act of creating objects as well.
While there are several standard creational patterns, we will consider four: factory, abstract
factory, builder, and singleton. Let us look at each of these in more detail.
3.1.1 Factory pattern
Definition: Factory pattern provides a way to use an instance as an object factory. The
factory may return an instance of one of several possible classes (in the subclass hierarchy),
depending on the data provided to it.
When to use:
When a class can't anticipate which kind of class of object it must create.
You want to localize the knowledge of which class gets created.
When you have classes that is derived from the same subclasses, or they may in fact
be unrelated classes that just share the same interface. Either way, the methods in
these class instances are the same and can be used interchangeably.
When you want to insulate the client from the actual type that is being instantiated.
~ 14 ~
Figure 2. List of patterns
3.1Creational Pattern
As their name suggests, the family of creational patterns all deal with creating instances of
objects. The OOP principle of polymorphism allows our programs to work flexibly with
many different specific types of objects that share particular properties. The specific, runtime
object type is sometimes unimportant and depends on the situation. We can extend this idea
to the act of creating objects as well.
While there are several standard creational patterns, we will consider four: factory, abstract
factory, builder, and singleton. Let us look at each of these in more detail.
3.1.1 Factory pattern
Definition: Factory pattern provides a way to use an instance as an object factory. The
factory may return an instance of one of several possible classes (in the subclass hierarchy),
depending on the data provided to it.
When to use:
When a class can't anticipate which kind of class of object it must create.
You want to localize the knowledge of which class gets created.
When you have classes that is derived from the same subclasses, or they may in fact
be unrelated classes that just share the same interface. Either way, the methods in
these class instances are the same and can be used interchangeably.
When you want to insulate the client from the actual type that is being instantiated.
~ 14 ~
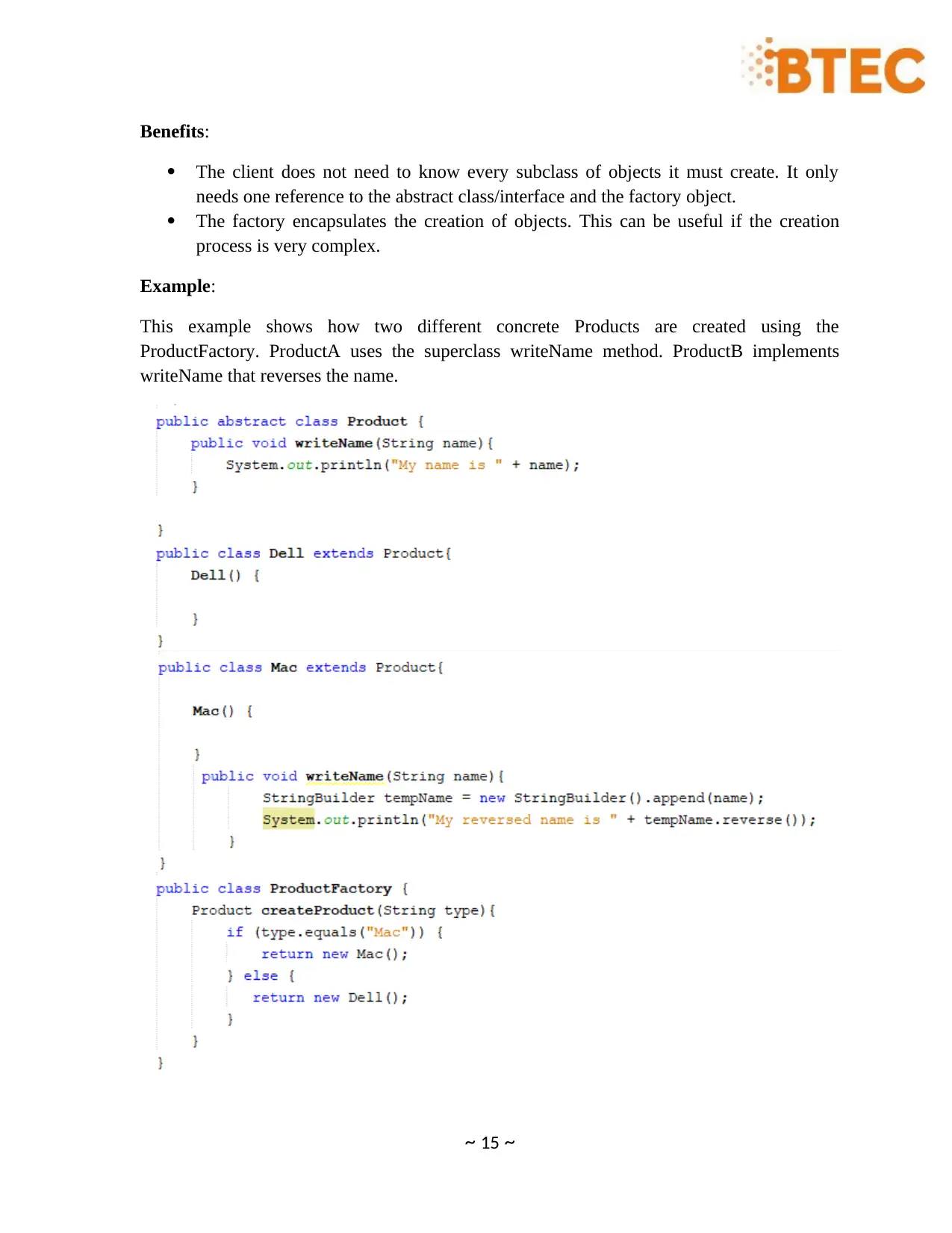
Benefits:
The client does not need to know every subclass of objects it must create. It only
needs one reference to the abstract class/interface and the factory object.
The factory encapsulates the creation of objects. This can be useful if the creation
process is very complex.
Example:
This example shows how two different concrete Products are created using the
ProductFactory. ProductA uses the superclass writeName method. ProductB implements
writeName that reverses the name.
~ 15 ~
The client does not need to know every subclass of objects it must create. It only
needs one reference to the abstract class/interface and the factory object.
The factory encapsulates the creation of objects. This can be useful if the creation
process is very complex.
Example:
This example shows how two different concrete Products are created using the
ProductFactory. ProductA uses the superclass writeName method. ProductB implements
writeName that reverses the name.
~ 15 ~
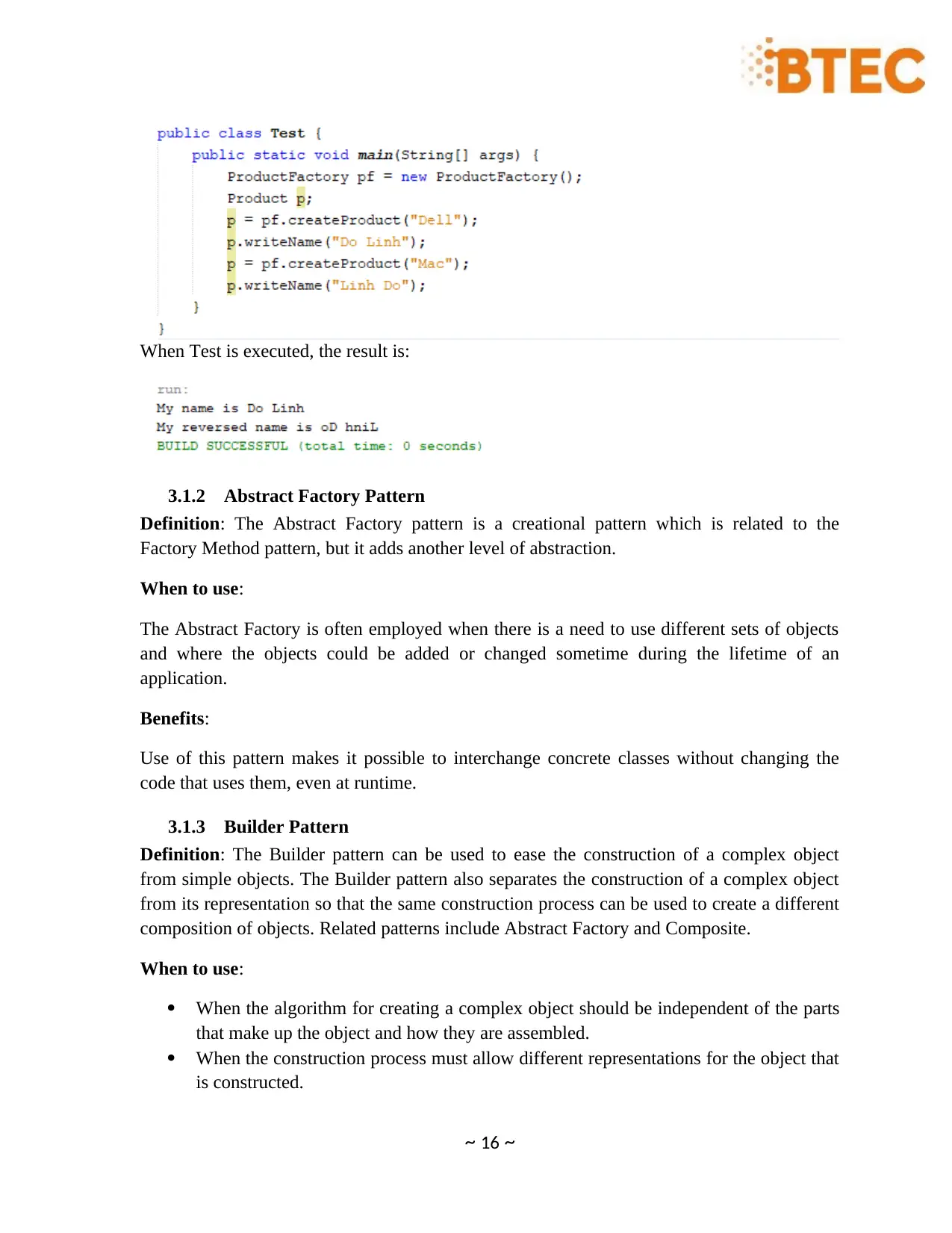
When Test is executed, the result is:
3.1.2 Abstract Factory Pattern
Definition: The Abstract Factory pattern is a creational pattern which is related to the
Factory Method pattern, but it adds another level of abstraction.
When to use:
The Abstract Factory is often employed when there is a need to use different sets of objects
and where the objects could be added or changed sometime during the lifetime of an
application.
Benefits:
Use of this pattern makes it possible to interchange concrete classes without changing the
code that uses them, even at runtime.
3.1.3 Builder Pattern
Definition: The Builder pattern can be used to ease the construction of a complex object
from simple objects. The Builder pattern also separates the construction of a complex object
from its representation so that the same construction process can be used to create a different
composition of objects. Related patterns include Abstract Factory and Composite.
When to use:
When the algorithm for creating a complex object should be independent of the parts
that make up the object and how they are assembled.
When the construction process must allow different representations for the object that
is constructed.
~ 16 ~
3.1.2 Abstract Factory Pattern
Definition: The Abstract Factory pattern is a creational pattern which is related to the
Factory Method pattern, but it adds another level of abstraction.
When to use:
The Abstract Factory is often employed when there is a need to use different sets of objects
and where the objects could be added or changed sometime during the lifetime of an
application.
Benefits:
Use of this pattern makes it possible to interchange concrete classes without changing the
code that uses them, even at runtime.
3.1.3 Builder Pattern
Definition: The Builder pattern can be used to ease the construction of a complex object
from simple objects. The Builder pattern also separates the construction of a complex object
from its representation so that the same construction process can be used to create a different
composition of objects. Related patterns include Abstract Factory and Composite.
When to use:
When the algorithm for creating a complex object should be independent of the parts
that make up the object and how they are assembled.
When the construction process must allow different representations for the object that
is constructed.
~ 16 ~
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
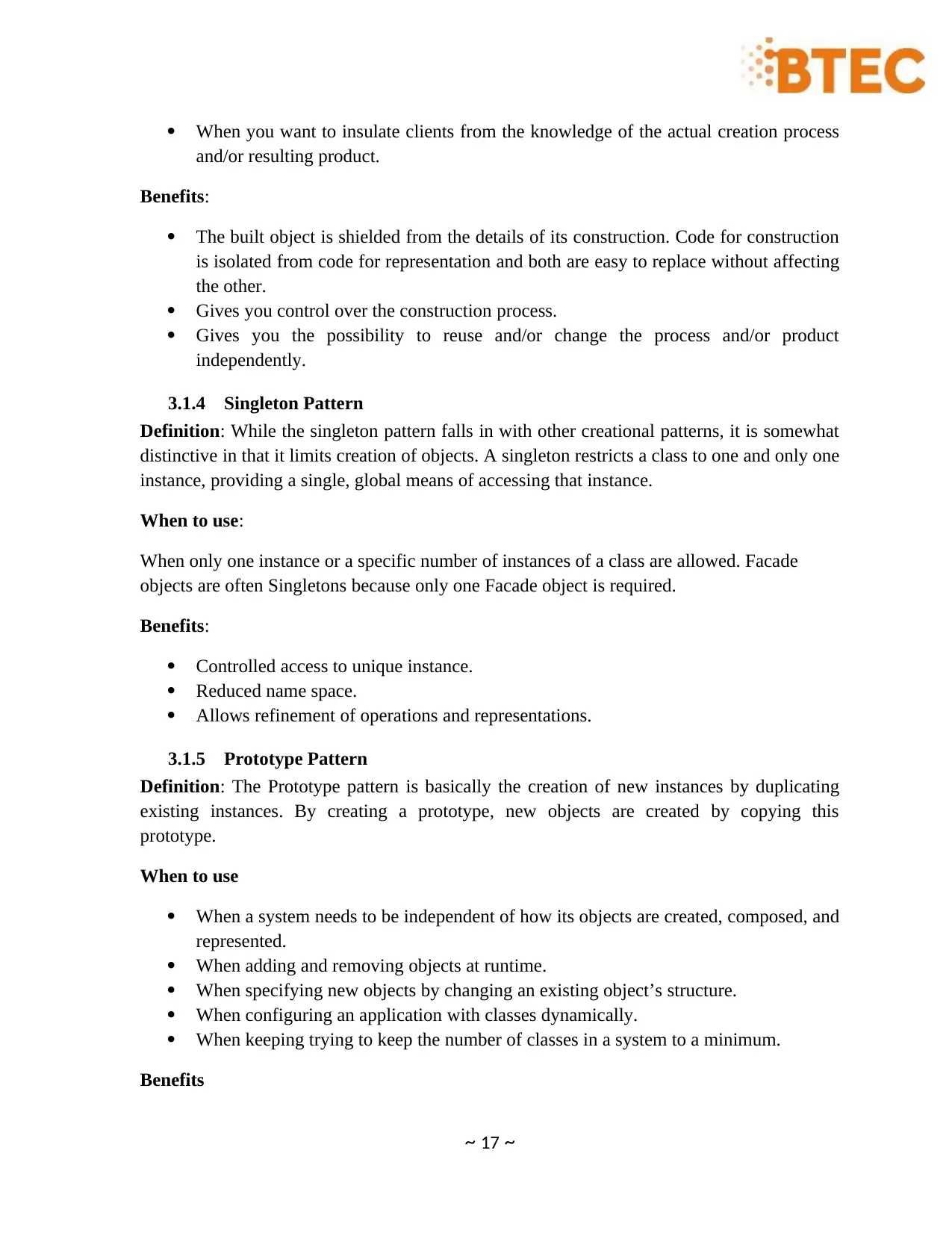
When you want to insulate clients from the knowledge of the actual creation process
and/or resulting product.
Benefits:
The built object is shielded from the details of its construction. Code for construction
is isolated from code for representation and both are easy to replace without affecting
the other.
Gives you control over the construction process.
Gives you the possibility to reuse and/or change the process and/or product
independently.
3.1.4 Singleton Pattern
Definition: While the singleton pattern falls in with other creational patterns, it is somewhat
distinctive in that it limits creation of objects. A singleton restricts a class to one and only one
instance, providing a single, global means of accessing that instance.
When to use:
When only one instance or a specific number of instances of a class are allowed. Facade
objects are often Singletons because only one Facade object is required.
Benefits:
Controlled access to unique instance.
Reduced name space.
Allows refinement of operations and representations.
3.1.5 Prototype Pattern
Definition: The Prototype pattern is basically the creation of new instances by duplicating
existing instances. By creating a prototype, new objects are created by copying this
prototype.
When to use
When a system needs to be independent of how its objects are created, composed, and
represented.
When adding and removing objects at runtime.
When specifying new objects by changing an existing object’s structure.
When configuring an application with classes dynamically.
When keeping trying to keep the number of classes in a system to a minimum.
Benefits
~ 17 ~
and/or resulting product.
Benefits:
The built object is shielded from the details of its construction. Code for construction
is isolated from code for representation and both are easy to replace without affecting
the other.
Gives you control over the construction process.
Gives you the possibility to reuse and/or change the process and/or product
independently.
3.1.4 Singleton Pattern
Definition: While the singleton pattern falls in with other creational patterns, it is somewhat
distinctive in that it limits creation of objects. A singleton restricts a class to one and only one
instance, providing a single, global means of accessing that instance.
When to use:
When only one instance or a specific number of instances of a class are allowed. Facade
objects are often Singletons because only one Facade object is required.
Benefits:
Controlled access to unique instance.
Reduced name space.
Allows refinement of operations and representations.
3.1.5 Prototype Pattern
Definition: The Prototype pattern is basically the creation of new instances by duplicating
existing instances. By creating a prototype, new objects are created by copying this
prototype.
When to use
When a system needs to be independent of how its objects are created, composed, and
represented.
When adding and removing objects at runtime.
When specifying new objects by changing an existing object’s structure.
When configuring an application with classes dynamically.
When keeping trying to keep the number of classes in a system to a minimum.
Benefits
~ 17 ~
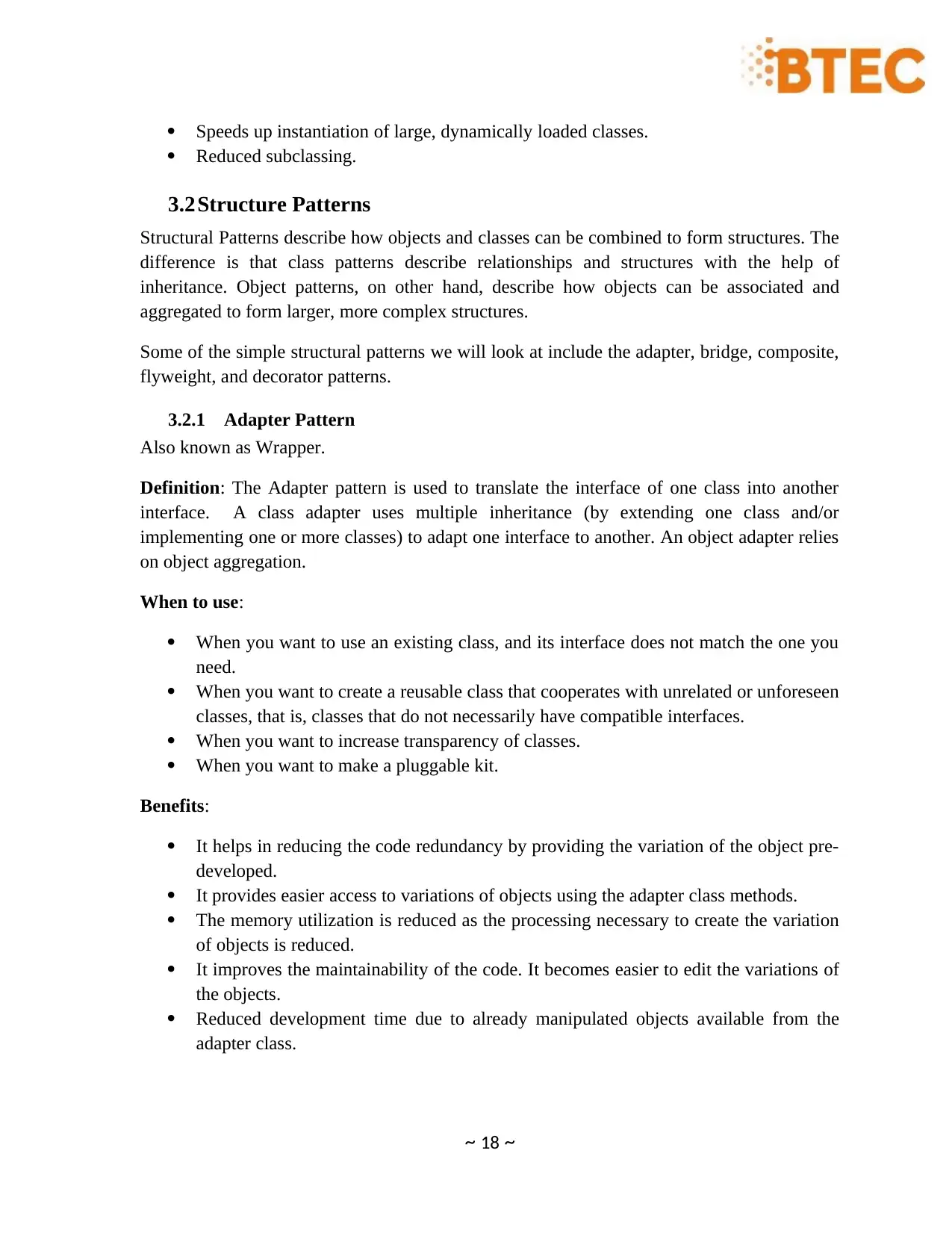
Speeds up instantiation of large, dynamically loaded classes.
Reduced subclassing.
3.2Structure Patterns
Structural Patterns describe how objects and classes can be combined to form structures. The
difference is that class patterns describe relationships and structures with the help of
inheritance. Object patterns, on other hand, describe how objects can be associated and
aggregated to form larger, more complex structures.
Some of the simple structural patterns we will look at include the adapter, bridge, composite,
flyweight, and decorator patterns.
3.2.1 Adapter Pattern
Also known as Wrapper.
Definition: The Adapter pattern is used to translate the interface of one class into another
interface. A class adapter uses multiple inheritance (by extending one class and/or
implementing one or more classes) to adapt one interface to another. An object adapter relies
on object aggregation.
When to use:
When you want to use an existing class, and its interface does not match the one you
need.
When you want to create a reusable class that cooperates with unrelated or unforeseen
classes, that is, classes that do not necessarily have compatible interfaces.
When you want to increase transparency of classes.
When you want to make a pluggable kit.
Benefits:
It helps in reducing the code redundancy by providing the variation of the object pre-
developed.
It provides easier access to variations of objects using the adapter class methods.
The memory utilization is reduced as the processing necessary to create the variation
of objects is reduced.
It improves the maintainability of the code. It becomes easier to edit the variations of
the objects.
Reduced development time due to already manipulated objects available from the
adapter class.
~ 18 ~
Reduced subclassing.
3.2Structure Patterns
Structural Patterns describe how objects and classes can be combined to form structures. The
difference is that class patterns describe relationships and structures with the help of
inheritance. Object patterns, on other hand, describe how objects can be associated and
aggregated to form larger, more complex structures.
Some of the simple structural patterns we will look at include the adapter, bridge, composite,
flyweight, and decorator patterns.
3.2.1 Adapter Pattern
Also known as Wrapper.
Definition: The Adapter pattern is used to translate the interface of one class into another
interface. A class adapter uses multiple inheritance (by extending one class and/or
implementing one or more classes) to adapt one interface to another. An object adapter relies
on object aggregation.
When to use:
When you want to use an existing class, and its interface does not match the one you
need.
When you want to create a reusable class that cooperates with unrelated or unforeseen
classes, that is, classes that do not necessarily have compatible interfaces.
When you want to increase transparency of classes.
When you want to make a pluggable kit.
Benefits:
It helps in reducing the code redundancy by providing the variation of the object pre-
developed.
It provides easier access to variations of objects using the adapter class methods.
The memory utilization is reduced as the processing necessary to create the variation
of objects is reduced.
It improves the maintainability of the code. It becomes easier to edit the variations of
the objects.
Reduced development time due to already manipulated objects available from the
adapter class.
~ 18 ~
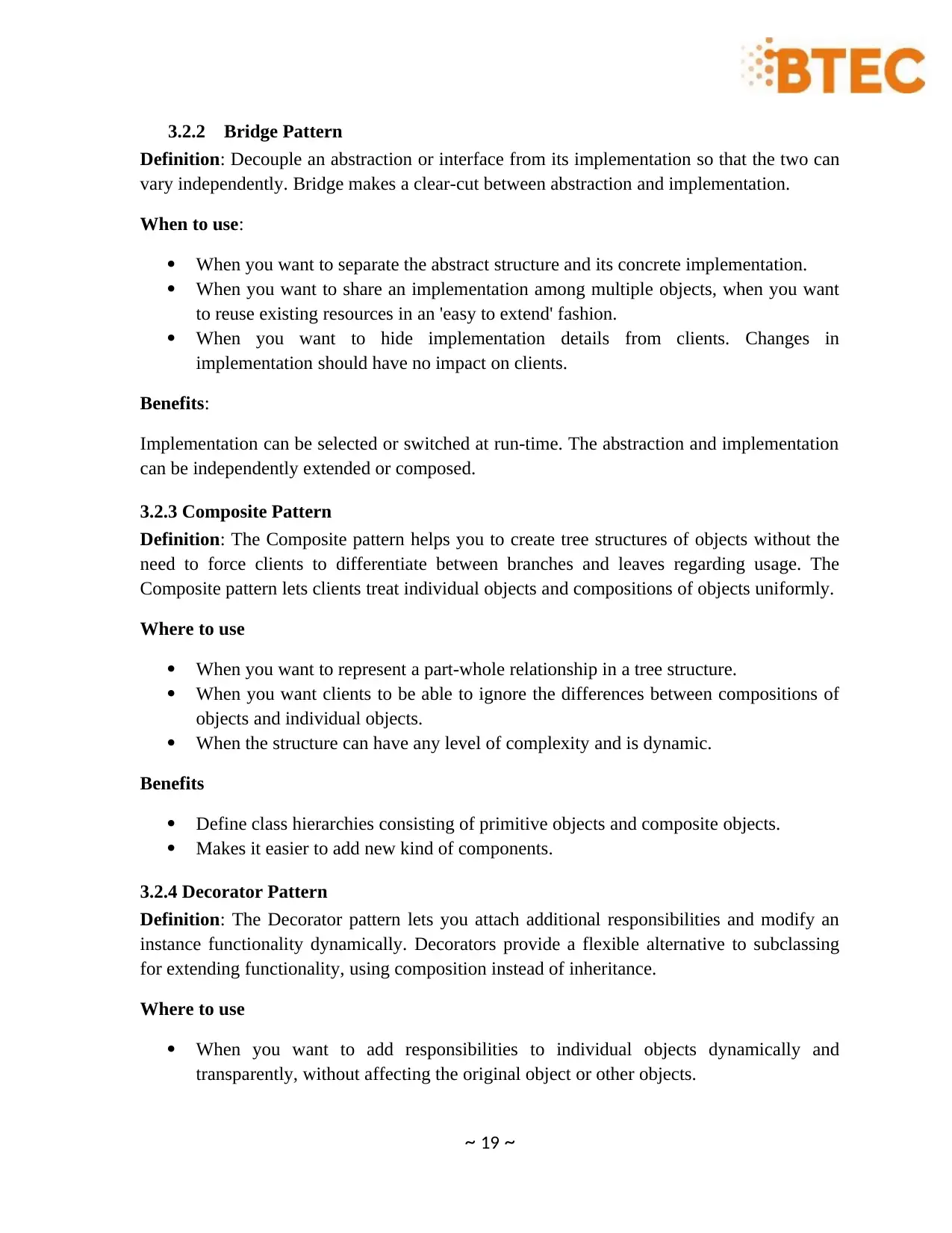
3.2.2 Bridge Pattern
Definition: Decouple an abstraction or interface from its implementation so that the two can
vary independently. Bridge makes a clear-cut between abstraction and implementation.
When to use:
When you want to separate the abstract structure and its concrete implementation.
When you want to share an implementation among multiple objects, when you want
to reuse existing resources in an 'easy to extend' fashion.
When you want to hide implementation details from clients. Changes in
implementation should have no impact on clients.
Benefits:
Implementation can be selected or switched at run-time. The abstraction and implementation
can be independently extended or composed.
3.2.3 Composite Pattern
Definition: The Composite pattern helps you to create tree structures of objects without the
need to force clients to differentiate between branches and leaves regarding usage. The
Composite pattern lets clients treat individual objects and compositions of objects uniformly.
Where to use
When you want to represent a part-whole relationship in a tree structure.
When you want clients to be able to ignore the differences between compositions of
objects and individual objects.
When the structure can have any level of complexity and is dynamic.
Benefits
Define class hierarchies consisting of primitive objects and composite objects.
Makes it easier to add new kind of components.
3.2.4 Decorator Pattern
Definition: The Decorator pattern lets you attach additional responsibilities and modify an
instance functionality dynamically. Decorators provide a flexible alternative to subclassing
for extending functionality, using composition instead of inheritance.
Where to use
When you want to add responsibilities to individual objects dynamically and
transparently, without affecting the original object or other objects.
~ 19 ~
Definition: Decouple an abstraction or interface from its implementation so that the two can
vary independently. Bridge makes a clear-cut between abstraction and implementation.
When to use:
When you want to separate the abstract structure and its concrete implementation.
When you want to share an implementation among multiple objects, when you want
to reuse existing resources in an 'easy to extend' fashion.
When you want to hide implementation details from clients. Changes in
implementation should have no impact on clients.
Benefits:
Implementation can be selected or switched at run-time. The abstraction and implementation
can be independently extended or composed.
3.2.3 Composite Pattern
Definition: The Composite pattern helps you to create tree structures of objects without the
need to force clients to differentiate between branches and leaves regarding usage. The
Composite pattern lets clients treat individual objects and compositions of objects uniformly.
Where to use
When you want to represent a part-whole relationship in a tree structure.
When you want clients to be able to ignore the differences between compositions of
objects and individual objects.
When the structure can have any level of complexity and is dynamic.
Benefits
Define class hierarchies consisting of primitive objects and composite objects.
Makes it easier to add new kind of components.
3.2.4 Decorator Pattern
Definition: The Decorator pattern lets you attach additional responsibilities and modify an
instance functionality dynamically. Decorators provide a flexible alternative to subclassing
for extending functionality, using composition instead of inheritance.
Where to use
When you want to add responsibilities to individual objects dynamically and
transparently, without affecting the original object or other objects.
~ 19 ~
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
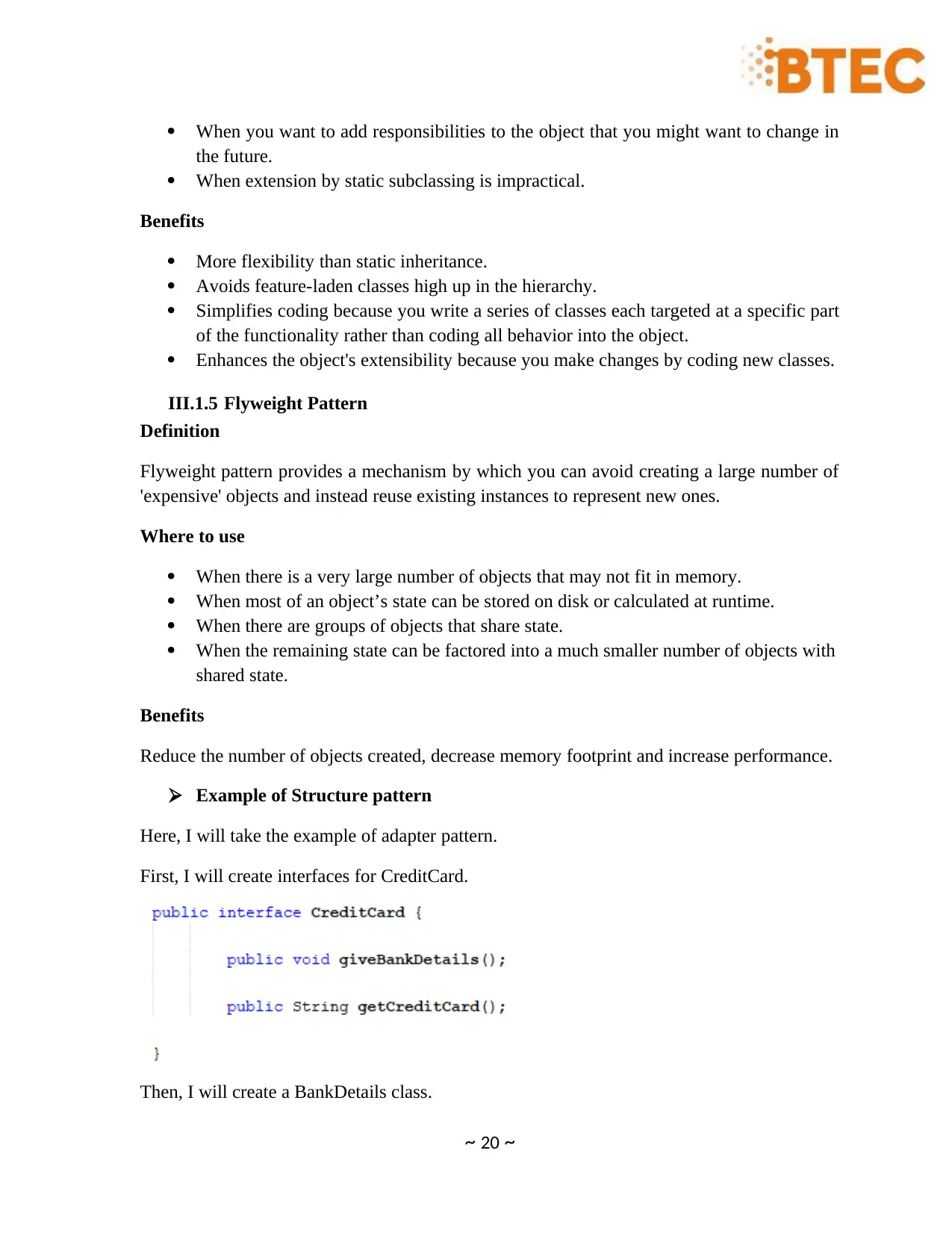
When you want to add responsibilities to the object that you might want to change in
the future.
When extension by static subclassing is impractical.
Benefits
More flexibility than static inheritance.
Avoids feature-laden classes high up in the hierarchy.
Simplifies coding because you write a series of classes each targeted at a specific part
of the functionality rather than coding all behavior into the object.
Enhances the object's extensibility because you make changes by coding new classes.
III.1.5 Flyweight Pattern
Definition
Flyweight pattern provides a mechanism by which you can avoid creating a large number of
'expensive' objects and instead reuse existing instances to represent new ones.
Where to use
When there is a very large number of objects that may not fit in memory.
When most of an object’s state can be stored on disk or calculated at runtime.
When there are groups of objects that share state.
When the remaining state can be factored into a much smaller number of objects with
shared state.
Benefits
Reduce the number of objects created, decrease memory footprint and increase performance.
Example of Structure pattern
Here, I will take the example of adapter pattern.
First, I will create interfaces for CreditCard.
Then, I will create a BankDetails class.
~ 20 ~
the future.
When extension by static subclassing is impractical.
Benefits
More flexibility than static inheritance.
Avoids feature-laden classes high up in the hierarchy.
Simplifies coding because you write a series of classes each targeted at a specific part
of the functionality rather than coding all behavior into the object.
Enhances the object's extensibility because you make changes by coding new classes.
III.1.5 Flyweight Pattern
Definition
Flyweight pattern provides a mechanism by which you can avoid creating a large number of
'expensive' objects and instead reuse existing instances to represent new ones.
Where to use
When there is a very large number of objects that may not fit in memory.
When most of an object’s state can be stored on disk or calculated at runtime.
When there are groups of objects that share state.
When the remaining state can be factored into a much smaller number of objects with
shared state.
Benefits
Reduce the number of objects created, decrease memory footprint and increase performance.
Example of Structure pattern
Here, I will take the example of adapter pattern.
First, I will create interfaces for CreditCard.
Then, I will create a BankDetails class.
~ 20 ~
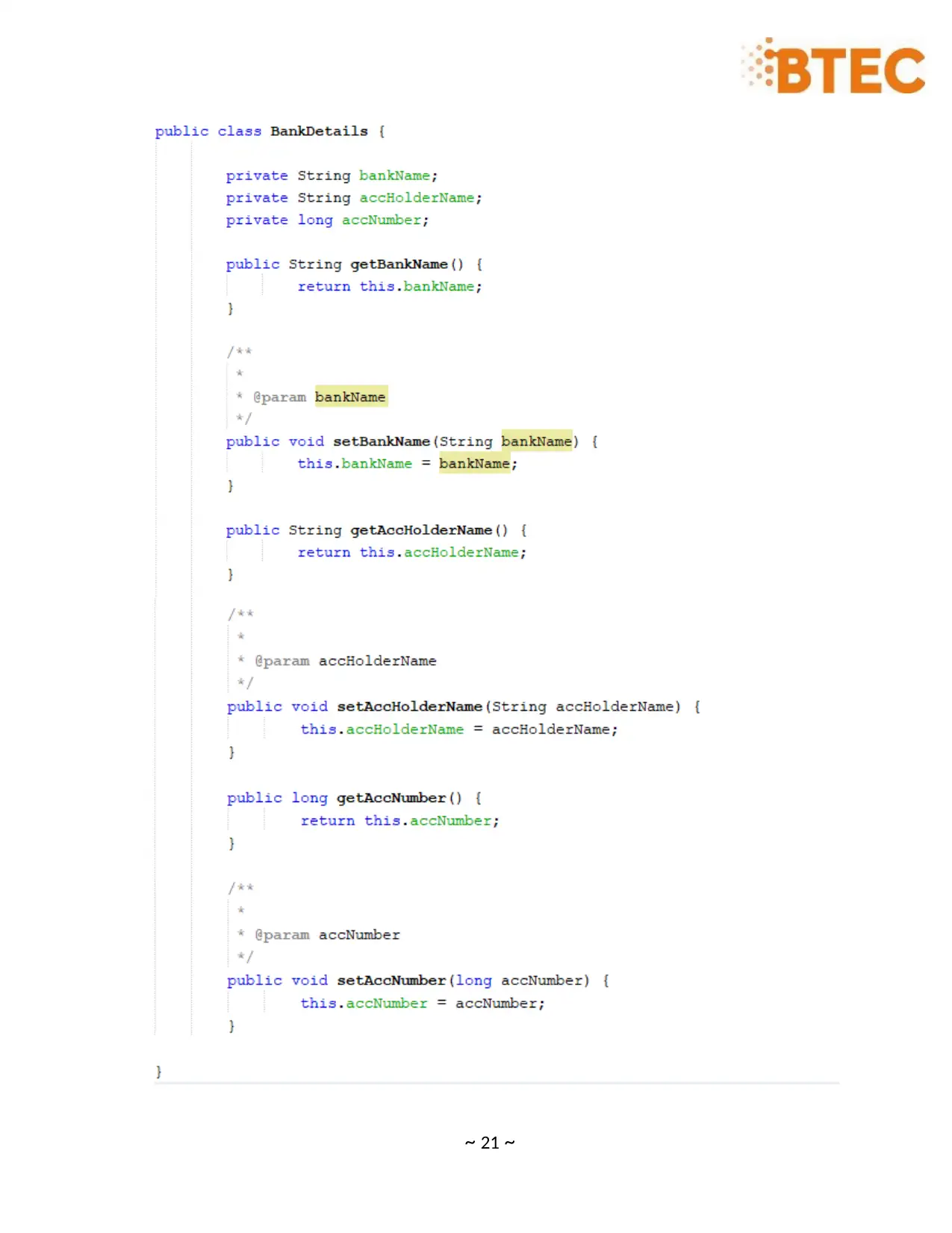
~ 21 ~
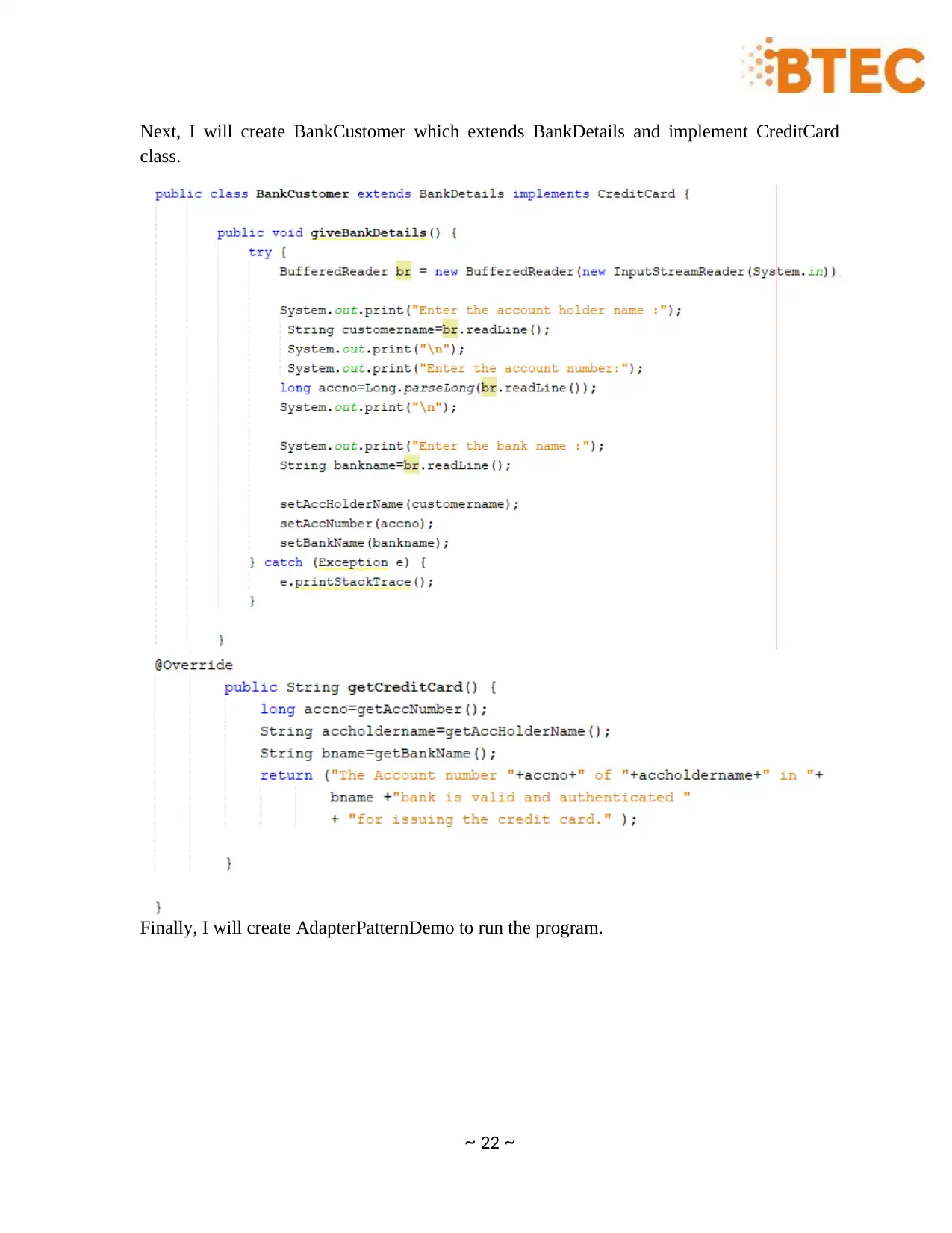
Next, I will create BankCustomer which extends BankDetails and implement CreditCard
class.
Finally, I will create AdapterPatternDemo to run the program.
~ 22 ~
class.
Finally, I will create AdapterPatternDemo to run the program.
~ 22 ~
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
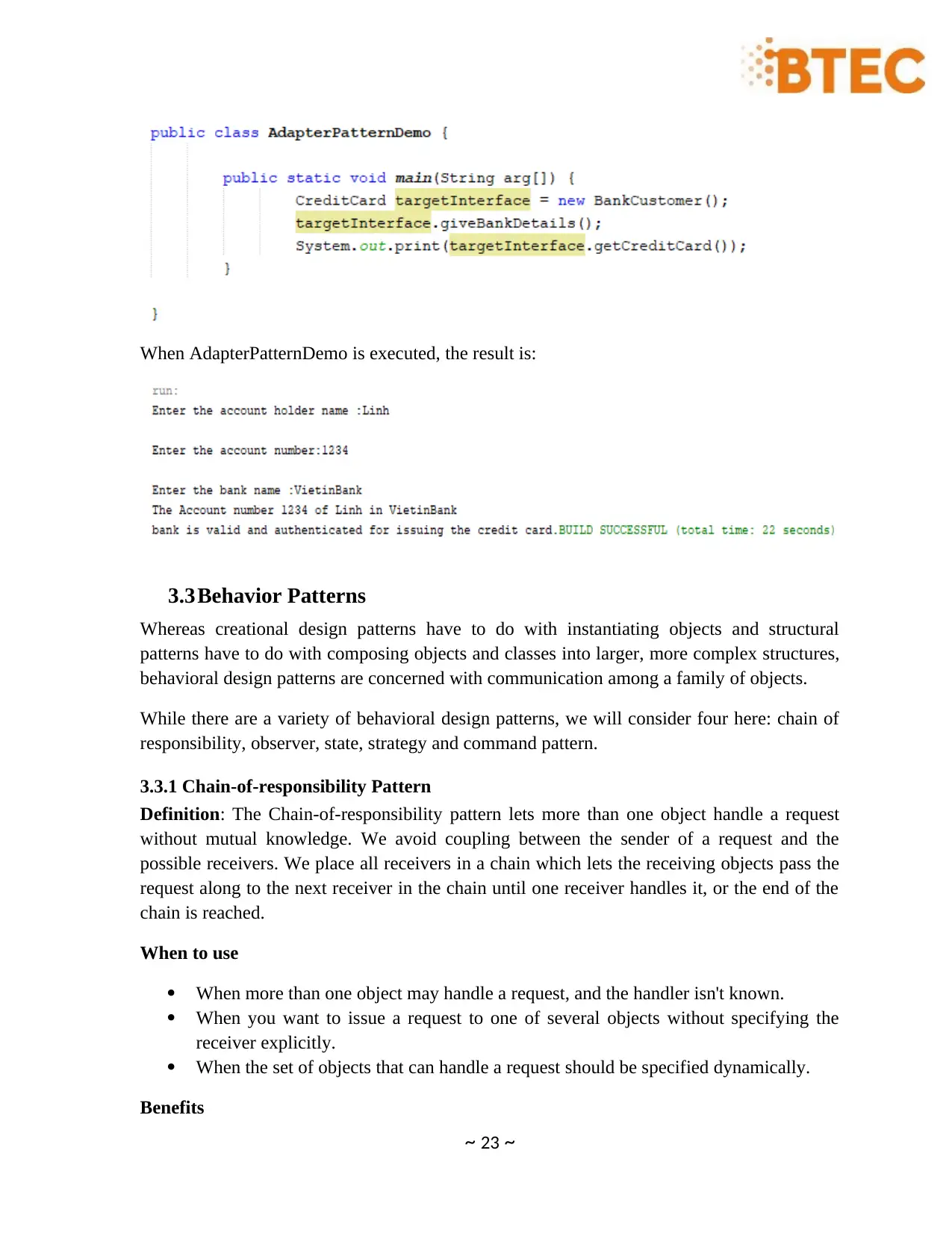
When AdapterPatternDemo is executed, the result is:
3.3Behavior Patterns
Whereas creational design patterns have to do with instantiating objects and structural
patterns have to do with composing objects and classes into larger, more complex structures,
behavioral design patterns are concerned with communication among a family of objects.
While there are a variety of behavioral design patterns, we will consider four here: chain of
responsibility, observer, state, strategy and command pattern.
3.3.1 Chain-of-responsibility Pattern
Definition: The Chain-of-responsibility pattern lets more than one object handle a request
without mutual knowledge. We avoid coupling between the sender of a request and the
possible receivers. We place all receivers in a chain which lets the receiving objects pass the
request along to the next receiver in the chain until one receiver handles it, or the end of the
chain is reached.
When to use
When more than one object may handle a request, and the handler isn't known.
When you want to issue a request to one of several objects without specifying the
receiver explicitly.
When the set of objects that can handle a request should be specified dynamically.
Benefits
~ 23 ~
3.3Behavior Patterns
Whereas creational design patterns have to do with instantiating objects and structural
patterns have to do with composing objects and classes into larger, more complex structures,
behavioral design patterns are concerned with communication among a family of objects.
While there are a variety of behavioral design patterns, we will consider four here: chain of
responsibility, observer, state, strategy and command pattern.
3.3.1 Chain-of-responsibility Pattern
Definition: The Chain-of-responsibility pattern lets more than one object handle a request
without mutual knowledge. We avoid coupling between the sender of a request and the
possible receivers. We place all receivers in a chain which lets the receiving objects pass the
request along to the next receiver in the chain until one receiver handles it, or the end of the
chain is reached.
When to use
When more than one object may handle a request, and the handler isn't known.
When you want to issue a request to one of several objects without specifying the
receiver explicitly.
When the set of objects that can handle a request should be specified dynamically.
Benefits
~ 23 ~
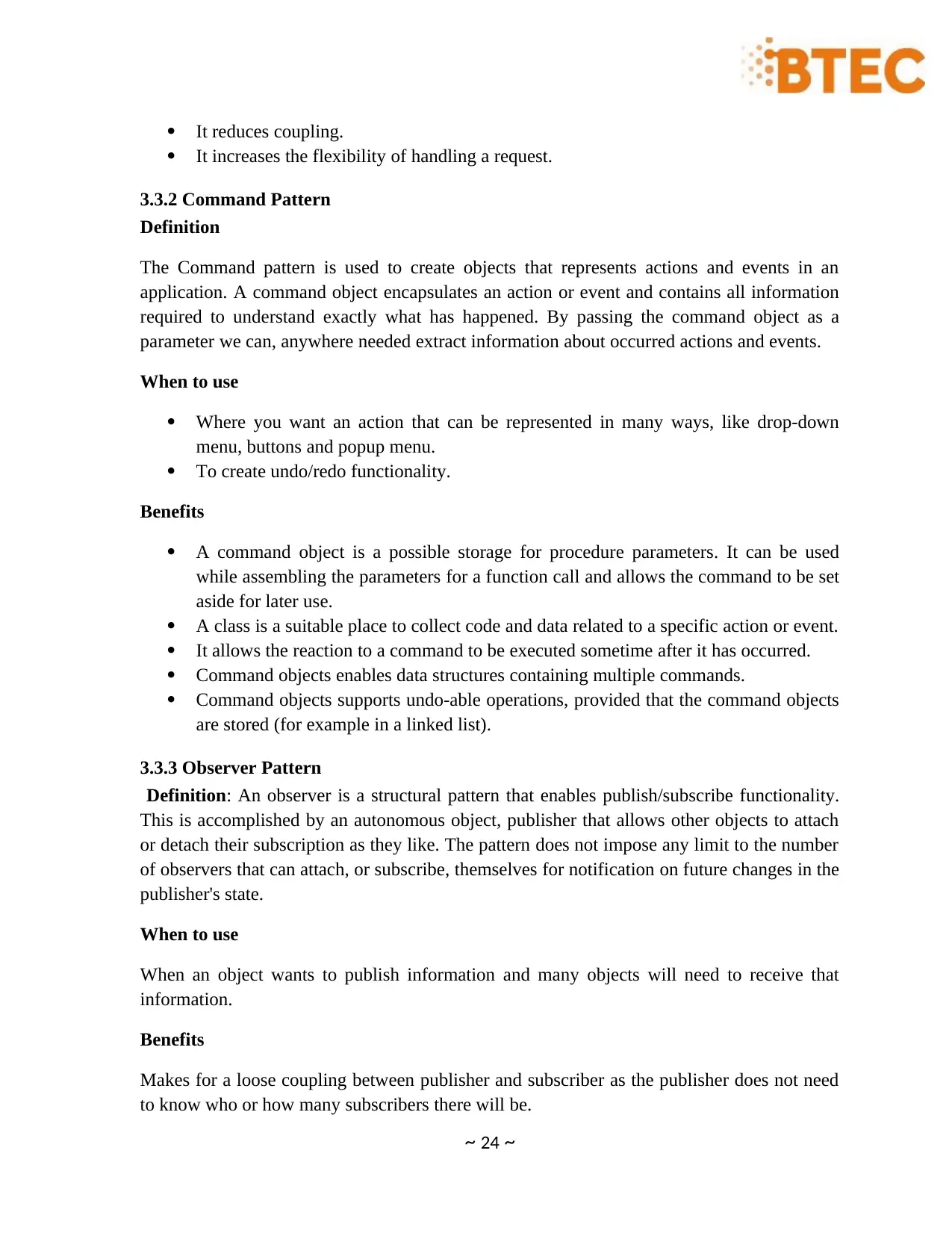
It reduces coupling.
It increases the flexibility of handling a request.
3.3.2 Command Pattern
Definition
The Command pattern is used to create objects that represents actions and events in an
application. A command object encapsulates an action or event and contains all information
required to understand exactly what has happened. By passing the command object as a
parameter we can, anywhere needed extract information about occurred actions and events.
When to use
Where you want an action that can be represented in many ways, like drop-down
menu, buttons and popup menu.
To create undo/redo functionality.
Benefits
A command object is a possible storage for procedure parameters. It can be used
while assembling the parameters for a function call and allows the command to be set
aside for later use.
A class is a suitable place to collect code and data related to a specific action or event.
It allows the reaction to a command to be executed sometime after it has occurred.
Command objects enables data structures containing multiple commands.
Command objects supports undo-able operations, provided that the command objects
are stored (for example in a linked list).
3.3.3 Observer Pattern
Definition: An observer is a structural pattern that enables publish/subscribe functionality.
This is accomplished by an autonomous object, publisher that allows other objects to attach
or detach their subscription as they like. The pattern does not impose any limit to the number
of observers that can attach, or subscribe, themselves for notification on future changes in the
publisher's state.
When to use
When an object wants to publish information and many objects will need to receive that
information.
Benefits
Makes for a loose coupling between publisher and subscriber as the publisher does not need
to know who or how many subscribers there will be.
~ 24 ~
It increases the flexibility of handling a request.
3.3.2 Command Pattern
Definition
The Command pattern is used to create objects that represents actions and events in an
application. A command object encapsulates an action or event and contains all information
required to understand exactly what has happened. By passing the command object as a
parameter we can, anywhere needed extract information about occurred actions and events.
When to use
Where you want an action that can be represented in many ways, like drop-down
menu, buttons and popup menu.
To create undo/redo functionality.
Benefits
A command object is a possible storage for procedure parameters. It can be used
while assembling the parameters for a function call and allows the command to be set
aside for later use.
A class is a suitable place to collect code and data related to a specific action or event.
It allows the reaction to a command to be executed sometime after it has occurred.
Command objects enables data structures containing multiple commands.
Command objects supports undo-able operations, provided that the command objects
are stored (for example in a linked list).
3.3.3 Observer Pattern
Definition: An observer is a structural pattern that enables publish/subscribe functionality.
This is accomplished by an autonomous object, publisher that allows other objects to attach
or detach their subscription as they like. The pattern does not impose any limit to the number
of observers that can attach, or subscribe, themselves for notification on future changes in the
publisher's state.
When to use
When an object wants to publish information and many objects will need to receive that
information.
Benefits
Makes for a loose coupling between publisher and subscriber as the publisher does not need
to know who or how many subscribers there will be.
~ 24 ~
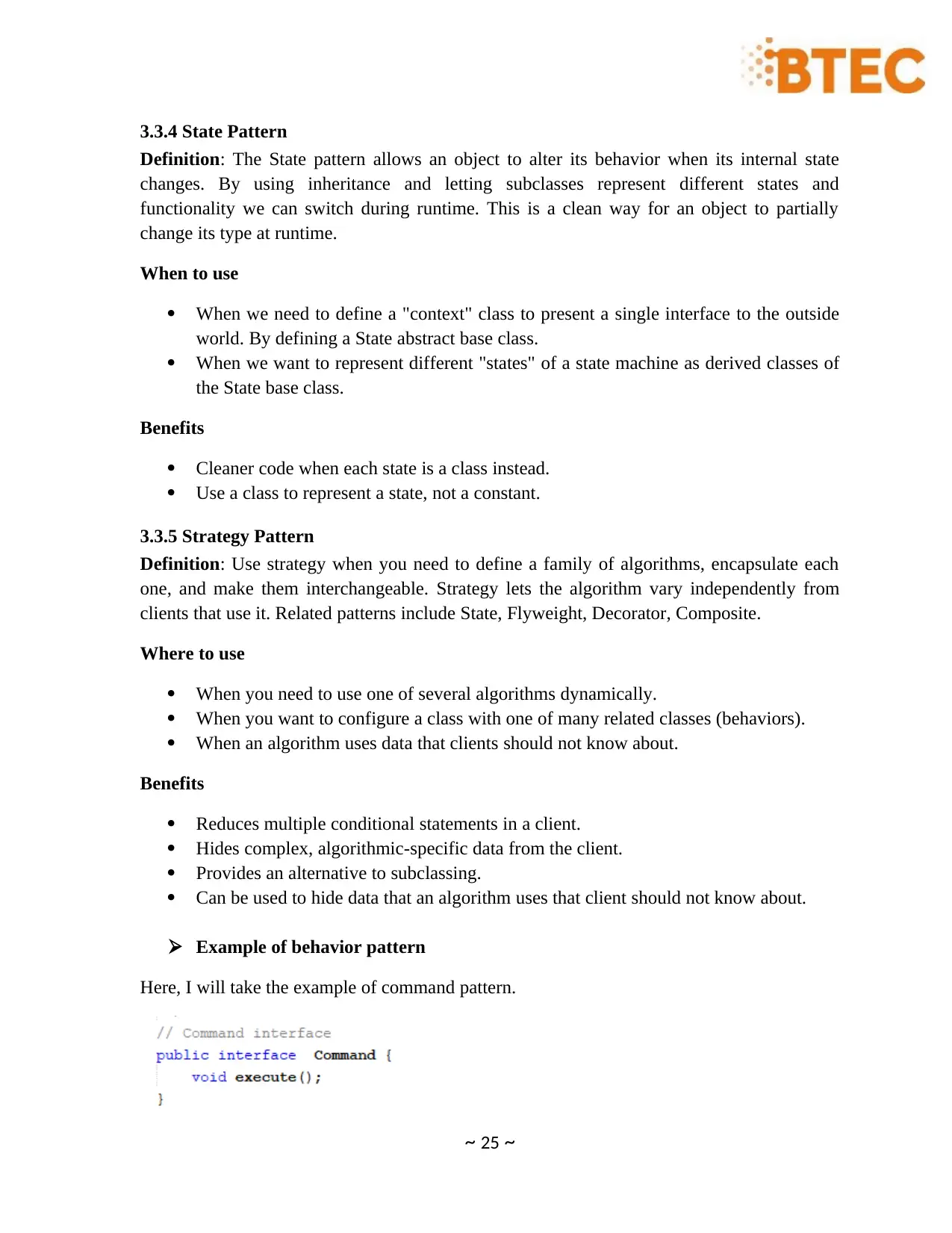
3.3.4 State Pattern
Definition: The State pattern allows an object to alter its behavior when its internal state
changes. By using inheritance and letting subclasses represent different states and
functionality we can switch during runtime. This is a clean way for an object to partially
change its type at runtime.
When to use
When we need to define a "context" class to present a single interface to the outside
world. By defining a State abstract base class.
When we want to represent different "states" of a state machine as derived classes of
the State base class.
Benefits
Cleaner code when each state is a class instead.
Use a class to represent a state, not a constant.
3.3.5 Strategy Pattern
Definition: Use strategy when you need to define a family of algorithms, encapsulate each
one, and make them interchangeable. Strategy lets the algorithm vary independently from
clients that use it. Related patterns include State, Flyweight, Decorator, Composite.
Where to use
When you need to use one of several algorithms dynamically.
When you want to configure a class with one of many related classes (behaviors).
When an algorithm uses data that clients should not know about.
Benefits
Reduces multiple conditional statements in a client.
Hides complex, algorithmic-specific data from the client.
Provides an alternative to subclassing.
Can be used to hide data that an algorithm uses that client should not know about.
Example of behavior pattern
Here, I will take the example of command pattern.
~ 25 ~
Definition: The State pattern allows an object to alter its behavior when its internal state
changes. By using inheritance and letting subclasses represent different states and
functionality we can switch during runtime. This is a clean way for an object to partially
change its type at runtime.
When to use
When we need to define a "context" class to present a single interface to the outside
world. By defining a State abstract base class.
When we want to represent different "states" of a state machine as derived classes of
the State base class.
Benefits
Cleaner code when each state is a class instead.
Use a class to represent a state, not a constant.
3.3.5 Strategy Pattern
Definition: Use strategy when you need to define a family of algorithms, encapsulate each
one, and make them interchangeable. Strategy lets the algorithm vary independently from
clients that use it. Related patterns include State, Flyweight, Decorator, Composite.
Where to use
When you need to use one of several algorithms dynamically.
When you want to configure a class with one of many related classes (behaviors).
When an algorithm uses data that clients should not know about.
Benefits
Reduces multiple conditional statements in a client.
Hides complex, algorithmic-specific data from the client.
Provides an alternative to subclassing.
Can be used to hide data that an algorithm uses that client should not know about.
Example of behavior pattern
Here, I will take the example of command pattern.
~ 25 ~
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
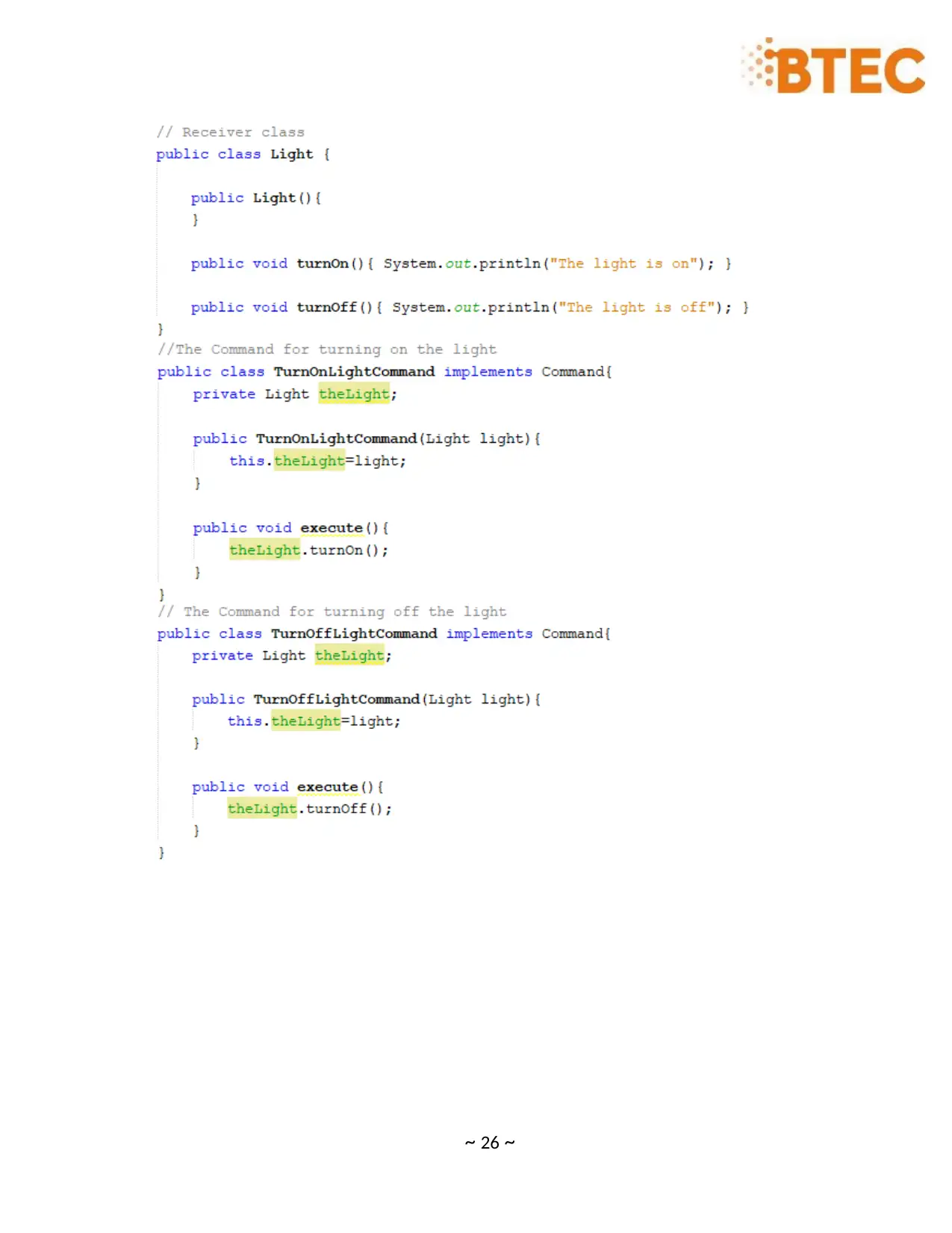
~ 26 ~
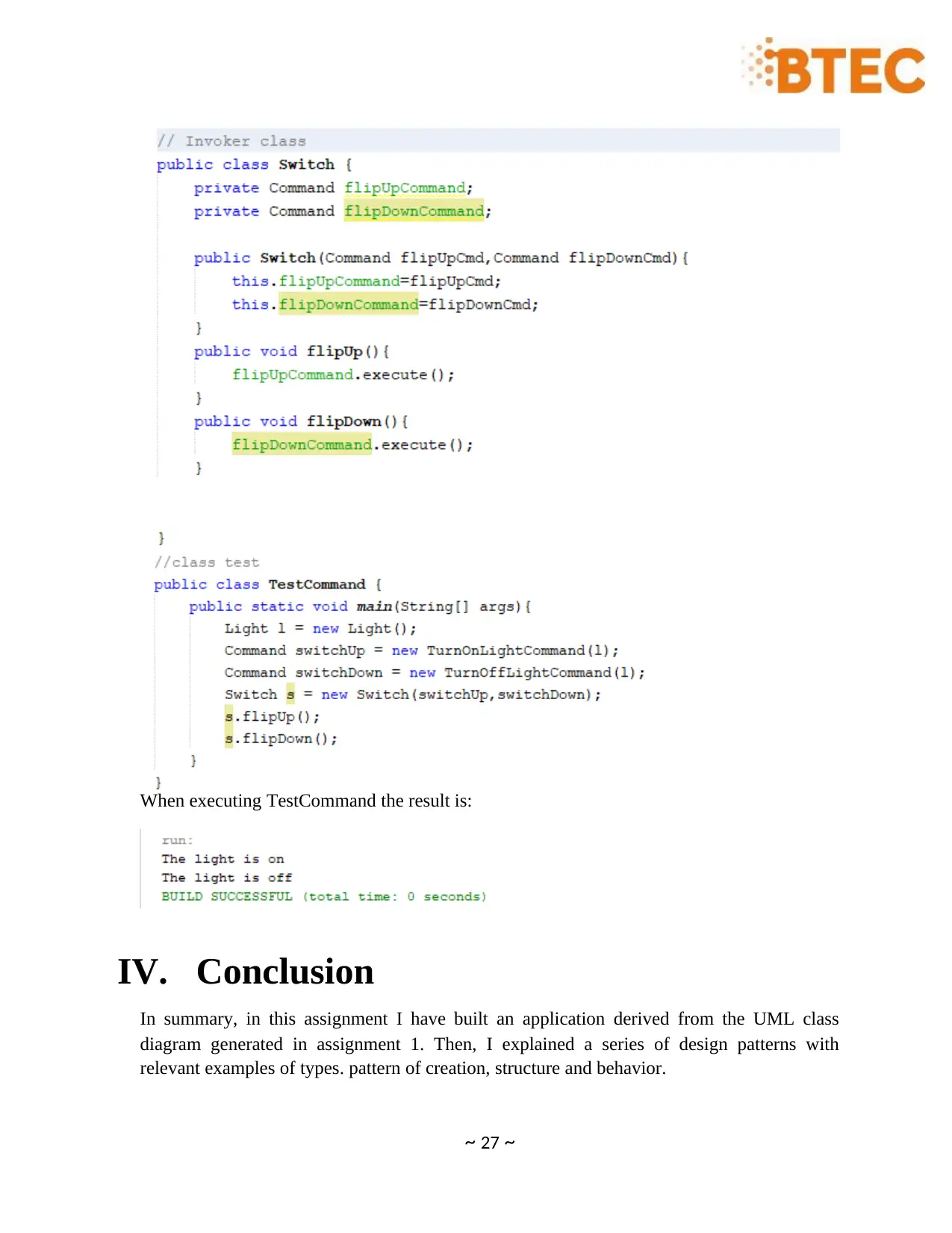
When executing TestCommand the result is:
IV. Conclusion
In summary, in this assignment I have built an application derived from the UML class
diagram generated in assignment 1. Then, I explained a series of design patterns with
relevant examples of types. pattern of creation, structure and behavior.
~ 27 ~
IV. Conclusion
In summary, in this assignment I have built an application derived from the UML class
diagram generated in assignment 1. Then, I explained a series of design patterns with
relevant examples of types. pattern of creation, structure and behavior.
~ 27 ~
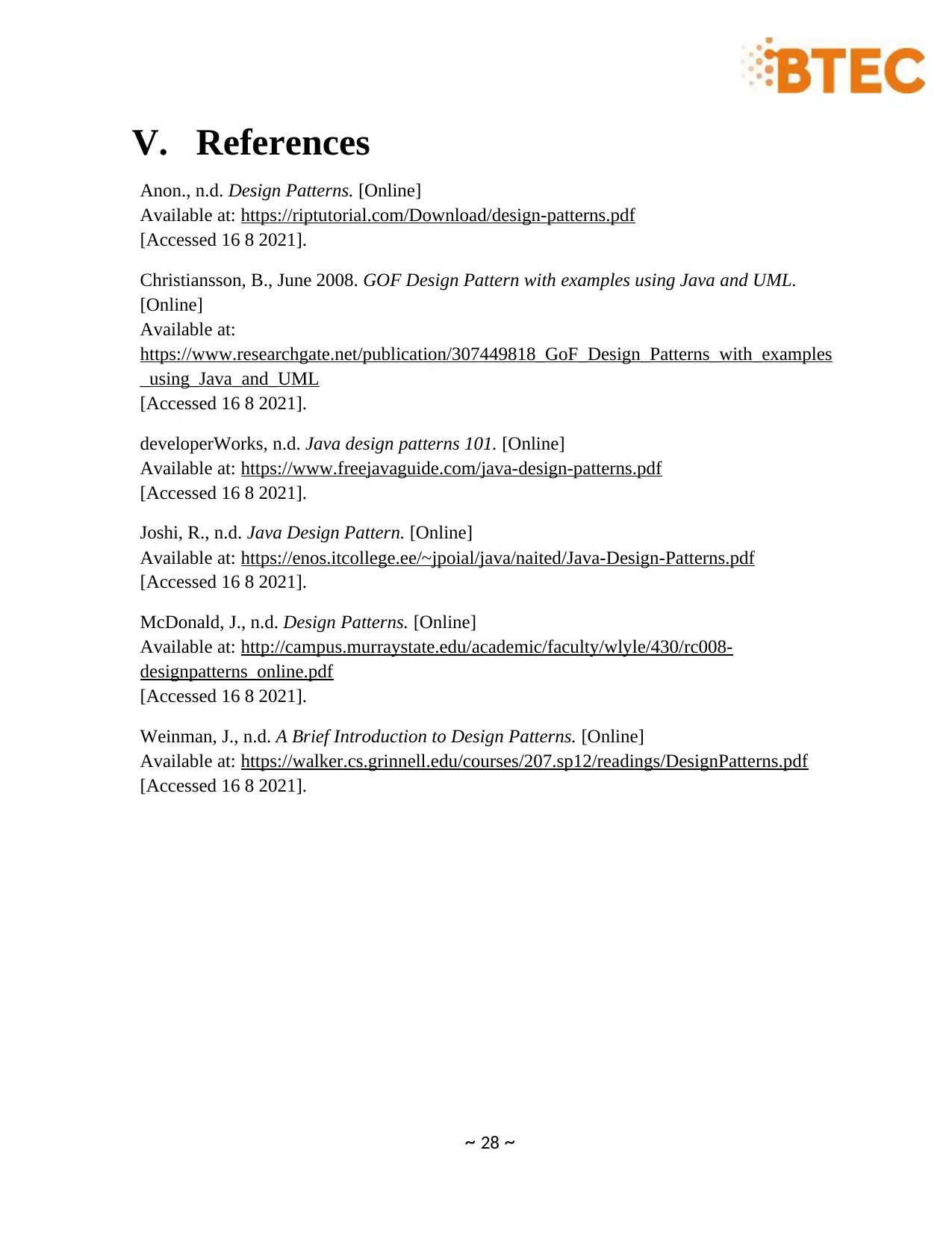
V. References
Anon., n.d. Design Patterns. [Online]
Available at: https://riptutorial.com/Download/design-patterns.pdf
[Accessed 16 8 2021].
Christiansson, B., June 2008. GOF Design Pattern with examples using Java and UML.
[Online]
Available at:
https://www.researchgate.net/publication/307449818_GoF_Design_Patterns_with_examples
_using_Java_and_UML
[Accessed 16 8 2021].
developerWorks, n.d. Java design patterns 101. [Online]
Available at: https://www.freejavaguide.com/java-design-patterns.pdf
[Accessed 16 8 2021].
Joshi, R., n.d. Java Design Pattern. [Online]
Available at: https://enos.itcollege.ee/~jpoial/java/naited/Java-Design-Patterns.pdf
[Accessed 16 8 2021].
McDonald, J., n.d. Design Patterns. [Online]
Available at: http://campus.murraystate.edu/academic/faculty/wlyle/430/rc008-
designpatterns_online.pdf
[Accessed 16 8 2021].
Weinman, J., n.d. A Brief Introduction to Design Patterns. [Online]
Available at: https://walker.cs.grinnell.edu/courses/207.sp12/readings/DesignPatterns.pdf
[Accessed 16 8 2021].
~ 28 ~
Anon., n.d. Design Patterns. [Online]
Available at: https://riptutorial.com/Download/design-patterns.pdf
[Accessed 16 8 2021].
Christiansson, B., June 2008. GOF Design Pattern with examples using Java and UML.
[Online]
Available at:
https://www.researchgate.net/publication/307449818_GoF_Design_Patterns_with_examples
_using_Java_and_UML
[Accessed 16 8 2021].
developerWorks, n.d. Java design patterns 101. [Online]
Available at: https://www.freejavaguide.com/java-design-patterns.pdf
[Accessed 16 8 2021].
Joshi, R., n.d. Java Design Pattern. [Online]
Available at: https://enos.itcollege.ee/~jpoial/java/naited/Java-Design-Patterns.pdf
[Accessed 16 8 2021].
McDonald, J., n.d. Design Patterns. [Online]
Available at: http://campus.murraystate.edu/academic/faculty/wlyle/430/rc008-
designpatterns_online.pdf
[Accessed 16 8 2021].
Weinman, J., n.d. A Brief Introduction to Design Patterns. [Online]
Available at: https://walker.cs.grinnell.edu/courses/207.sp12/readings/DesignPatterns.pdf
[Accessed 16 8 2021].
~ 28 ~
1 out of 28
Related Documents

Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.