Algorithms, Paradigms, and IDEs: A Comprehensive Examination
VerifiedAdded on 2023/02/07
|8
|1804
|83
Homework Assignment
AI Summary
This assignment delves into the definition of algorithms, outlining the application-building process, which includes problem analysis, design, coding, documentation, compilation, testing, debugging, and maintenance. It then explores procedural, object-oriented, and event-driven programming paradigms, detailing their characteristics and relationships. The assignment also covers the Integrated Development Environment (IDE), discussing its benefits and features such as text editors, debuggers, compilers, and code completion. The document also provides examples and definitions to clarify the concepts. This assignment is designed to give a solid foundation of the core concepts of software development.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
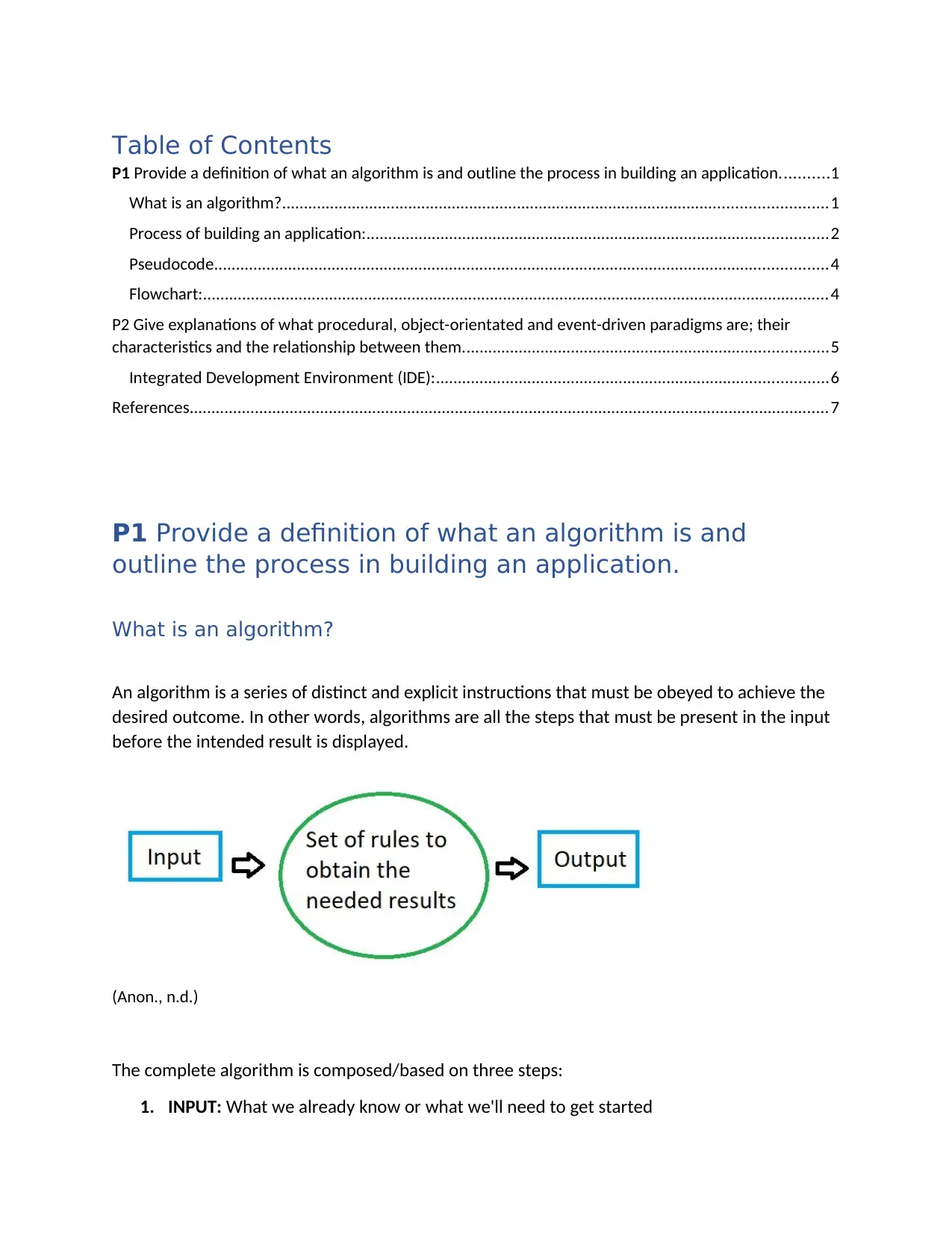
Table of Contents
P1 Provide a definition of what an algorithm is and outline the process in building an application...........1
What is an algorithm?.............................................................................................................................1
Process of building an application:..........................................................................................................2
Pseudocode.............................................................................................................................................4
Flowchart:................................................................................................................................................4
P2 Give explanations of what procedural, object-orientated and event-driven paradigms are; their
characteristics and the relationship between them....................................................................................5
Integrated Development Environment (IDE):..........................................................................................6
References...................................................................................................................................................7
P1 Provide a definition of what an algorithm is and
outline the process in building an application.
What is an algorithm?
An algorithm is a series of distinct and explicit instructions that must be obeyed to achieve the
desired outcome. In other words, algorithms are all the steps that must be present in the input
before the intended result is displayed.
(Anon., n.d.)
The complete algorithm is composed/based on three steps:
1. INPUT: What we already know or what we'll need to get started
P1 Provide a definition of what an algorithm is and outline the process in building an application...........1
What is an algorithm?.............................................................................................................................1
Process of building an application:..........................................................................................................2
Pseudocode.............................................................................................................................................4
Flowchart:................................................................................................................................................4
P2 Give explanations of what procedural, object-orientated and event-driven paradigms are; their
characteristics and the relationship between them....................................................................................5
Integrated Development Environment (IDE):..........................................................................................6
References...................................................................................................................................................7
P1 Provide a definition of what an algorithm is and
outline the process in building an application.
What is an algorithm?
An algorithm is a series of distinct and explicit instructions that must be obeyed to achieve the
desired outcome. In other words, algorithms are all the steps that must be present in the input
before the intended result is displayed.
(Anon., n.d.)
The complete algorithm is composed/based on three steps:
1. INPUT: What we already know or what we'll need to get started
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
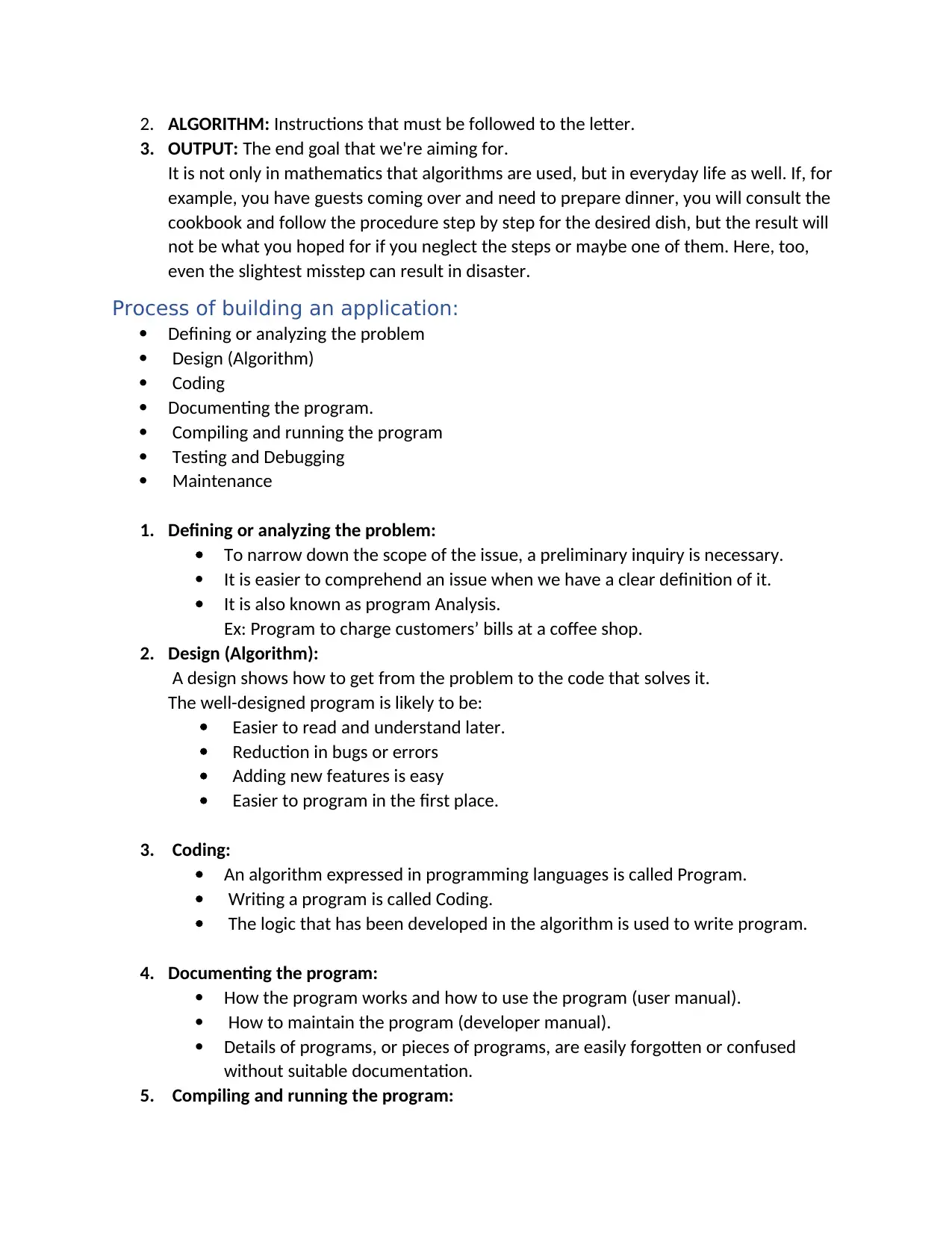
2. ALGORITHM: Instructions that must be followed to the letter.
3. OUTPUT: The end goal that we're aiming for.
It is not only in mathematics that algorithms are used, but in everyday life as well. If, for
example, you have guests coming over and need to prepare dinner, you will consult the
cookbook and follow the procedure step by step for the desired dish, but the result will
not be what you hoped for if you neglect the steps or maybe one of them. Here, too,
even the slightest misstep can result in disaster.
Process of building an application:
Defining or analyzing the problem
Design (Algorithm)
Coding
Documenting the program.
Compiling and running the program
Testing and Debugging
Maintenance
1. Defining or analyzing the problem:
To narrow down the scope of the issue, a preliminary inquiry is necessary.
It is easier to comprehend an issue when we have a clear definition of it.
It is also known as program Analysis.
Ex: Program to charge customers’ bills at a coffee shop.
2. Design (Algorithm):
A design shows how to get from the problem to the code that solves it.
The well-designed program is likely to be:
Easier to read and understand later.
Reduction in bugs or errors
Adding new features is easy
Easier to program in the first place.
3. Coding:
An algorithm expressed in programming languages is called Program.
Writing a program is called Coding.
The logic that has been developed in the algorithm is used to write program.
4. Documenting the program:
How the program works and how to use the program (user manual).
How to maintain the program (developer manual).
Details of programs, or pieces of programs, are easily forgotten or confused
without suitable documentation.
5. Compiling and running the program:
3. OUTPUT: The end goal that we're aiming for.
It is not only in mathematics that algorithms are used, but in everyday life as well. If, for
example, you have guests coming over and need to prepare dinner, you will consult the
cookbook and follow the procedure step by step for the desired dish, but the result will
not be what you hoped for if you neglect the steps or maybe one of them. Here, too,
even the slightest misstep can result in disaster.
Process of building an application:
Defining or analyzing the problem
Design (Algorithm)
Coding
Documenting the program.
Compiling and running the program
Testing and Debugging
Maintenance
1. Defining or analyzing the problem:
To narrow down the scope of the issue, a preliminary inquiry is necessary.
It is easier to comprehend an issue when we have a clear definition of it.
It is also known as program Analysis.
Ex: Program to charge customers’ bills at a coffee shop.
2. Design (Algorithm):
A design shows how to get from the problem to the code that solves it.
The well-designed program is likely to be:
Easier to read and understand later.
Reduction in bugs or errors
Adding new features is easy
Easier to program in the first place.
3. Coding:
An algorithm expressed in programming languages is called Program.
Writing a program is called Coding.
The logic that has been developed in the algorithm is used to write program.
4. Documenting the program:
How the program works and how to use the program (user manual).
How to maintain the program (developer manual).
Details of programs, or pieces of programs, are easily forgotten or confused
without suitable documentation.
5. Compiling and running the program:
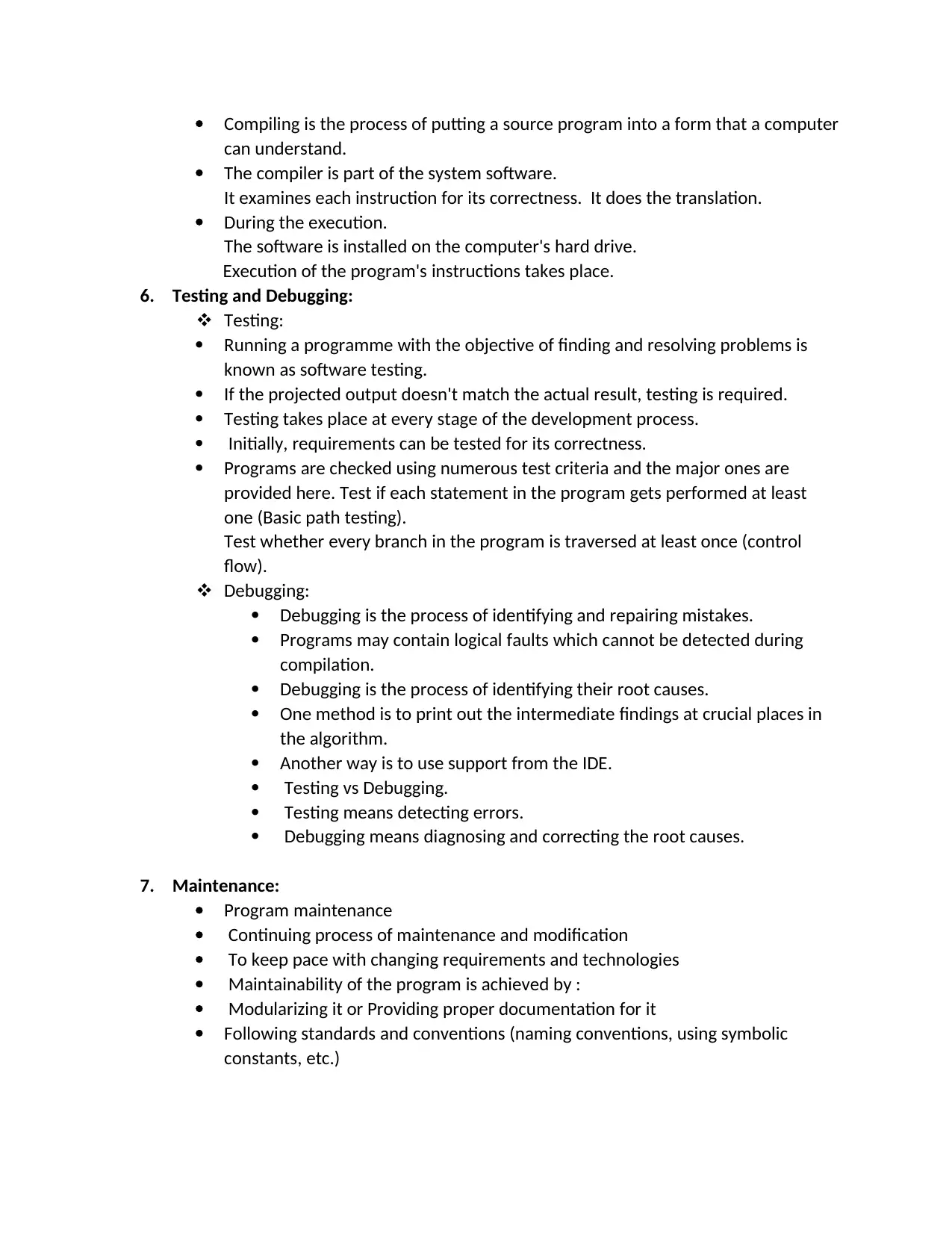
Compiling is the process of putting a source program into a form that a computer
can understand.
The compiler is part of the system software.
It examines each instruction for its correctness. It does the translation.
During the execution.
The software is installed on the computer's hard drive.
Execution of the program's instructions takes place.
6. Testing and Debugging:
Testing:
Running a programme with the objective of finding and resolving problems is
known as software testing.
If the projected output doesn't match the actual result, testing is required.
Testing takes place at every stage of the development process.
Initially, requirements can be tested for its correctness.
Programs are checked using numerous test criteria and the major ones are
provided here. Test if each statement in the program gets performed at least
one (Basic path testing).
Test whether every branch in the program is traversed at least once (control
flow).
Debugging:
Debugging is the process of identifying and repairing mistakes.
Programs may contain logical faults which cannot be detected during
compilation.
Debugging is the process of identifying their root causes.
One method is to print out the intermediate findings at crucial places in
the algorithm.
Another way is to use support from the IDE.
Testing vs Debugging.
Testing means detecting errors.
Debugging means diagnosing and correcting the root causes.
7. Maintenance:
Program maintenance
Continuing process of maintenance and modification
To keep pace with changing requirements and technologies
Maintainability of the program is achieved by :
Modularizing it or Providing proper documentation for it
Following standards and conventions (naming conventions, using symbolic
constants, etc.)
can understand.
The compiler is part of the system software.
It examines each instruction for its correctness. It does the translation.
During the execution.
The software is installed on the computer's hard drive.
Execution of the program's instructions takes place.
6. Testing and Debugging:
Testing:
Running a programme with the objective of finding and resolving problems is
known as software testing.
If the projected output doesn't match the actual result, testing is required.
Testing takes place at every stage of the development process.
Initially, requirements can be tested for its correctness.
Programs are checked using numerous test criteria and the major ones are
provided here. Test if each statement in the program gets performed at least
one (Basic path testing).
Test whether every branch in the program is traversed at least once (control
flow).
Debugging:
Debugging is the process of identifying and repairing mistakes.
Programs may contain logical faults which cannot be detected during
compilation.
Debugging is the process of identifying their root causes.
One method is to print out the intermediate findings at crucial places in
the algorithm.
Another way is to use support from the IDE.
Testing vs Debugging.
Testing means detecting errors.
Debugging means diagnosing and correcting the root causes.
7. Maintenance:
Program maintenance
Continuing process of maintenance and modification
To keep pace with changing requirements and technologies
Maintainability of the program is achieved by :
Modularizing it or Providing proper documentation for it
Following standards and conventions (naming conventions, using symbolic
constants, etc.)
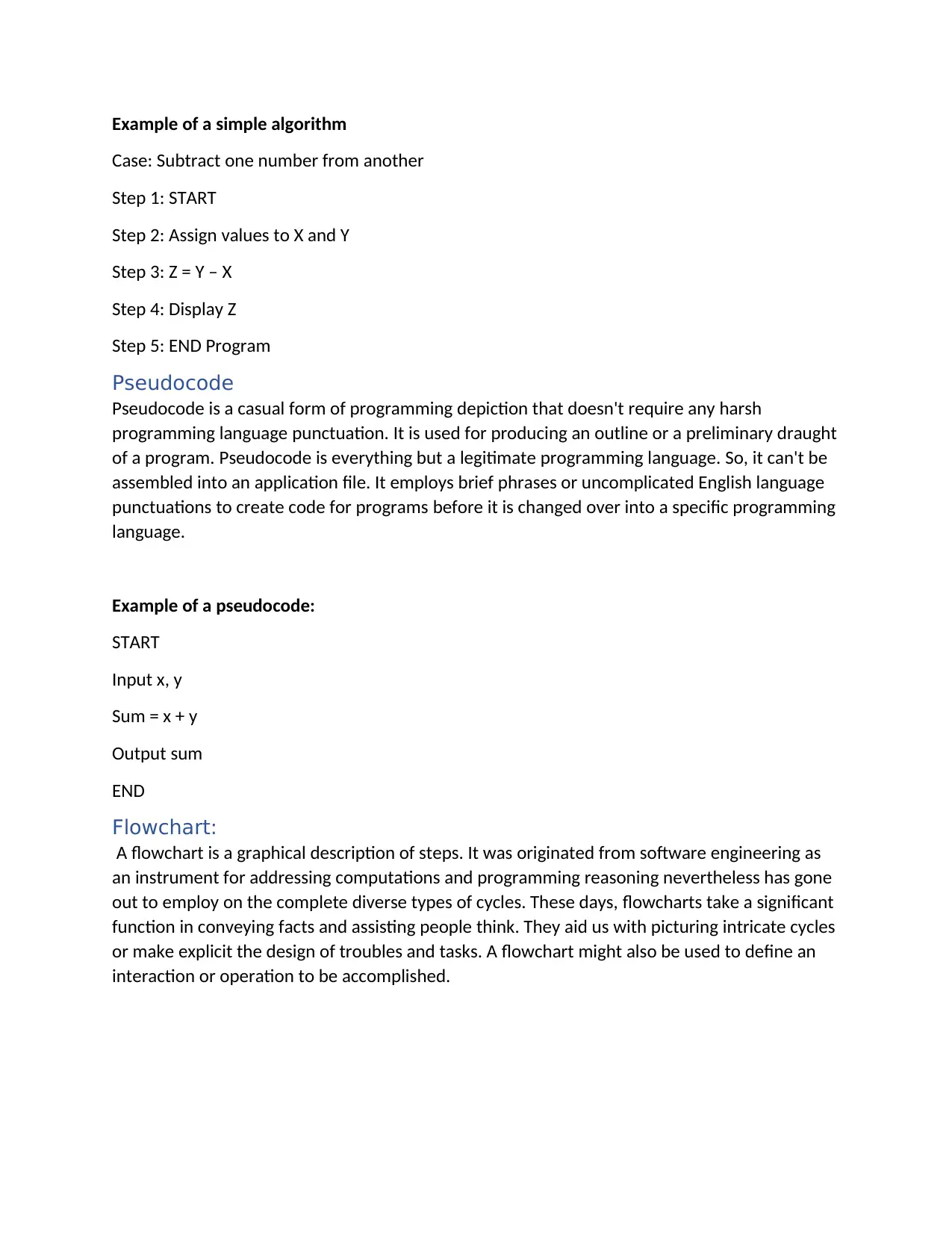
Example of a simple algorithm
Case: Subtract one number from another
Step 1: START
Step 2: Assign values to X and Y
Step 3: Z = Y – X
Step 4: Display Z
Step 5: END Program
Pseudocode
Pseudocode is a casual form of programming depiction that doesn't require any harsh
programming language punctuation. It is used for producing an outline or a preliminary draught
of a program. Pseudocode is everything but a legitimate programming language. So, it can't be
assembled into an application file. It employs brief phrases or uncomplicated English language
punctuations to create code for programs before it is changed over into a specific programming
language.
Example of a pseudocode:
START
Input x, y
Sum = x + y
Output sum
END
Flowchart:
A flowchart is a graphical description of steps. It was originated from software engineering as
an instrument for addressing computations and programming reasoning nevertheless has gone
out to employ on the complete diverse types of cycles. These days, flowcharts take a significant
function in conveying facts and assisting people think. They aid us with picturing intricate cycles
or make explicit the design of troubles and tasks. A flowchart might also be used to define an
interaction or operation to be accomplished.
Case: Subtract one number from another
Step 1: START
Step 2: Assign values to X and Y
Step 3: Z = Y – X
Step 4: Display Z
Step 5: END Program
Pseudocode
Pseudocode is a casual form of programming depiction that doesn't require any harsh
programming language punctuation. It is used for producing an outline or a preliminary draught
of a program. Pseudocode is everything but a legitimate programming language. So, it can't be
assembled into an application file. It employs brief phrases or uncomplicated English language
punctuations to create code for programs before it is changed over into a specific programming
language.
Example of a pseudocode:
START
Input x, y
Sum = x + y
Output sum
END
Flowchart:
A flowchart is a graphical description of steps. It was originated from software engineering as
an instrument for addressing computations and programming reasoning nevertheless has gone
out to employ on the complete diverse types of cycles. These days, flowcharts take a significant
function in conveying facts and assisting people think. They aid us with picturing intricate cycles
or make explicit the design of troubles and tasks. A flowchart might also be used to define an
interaction or operation to be accomplished.
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
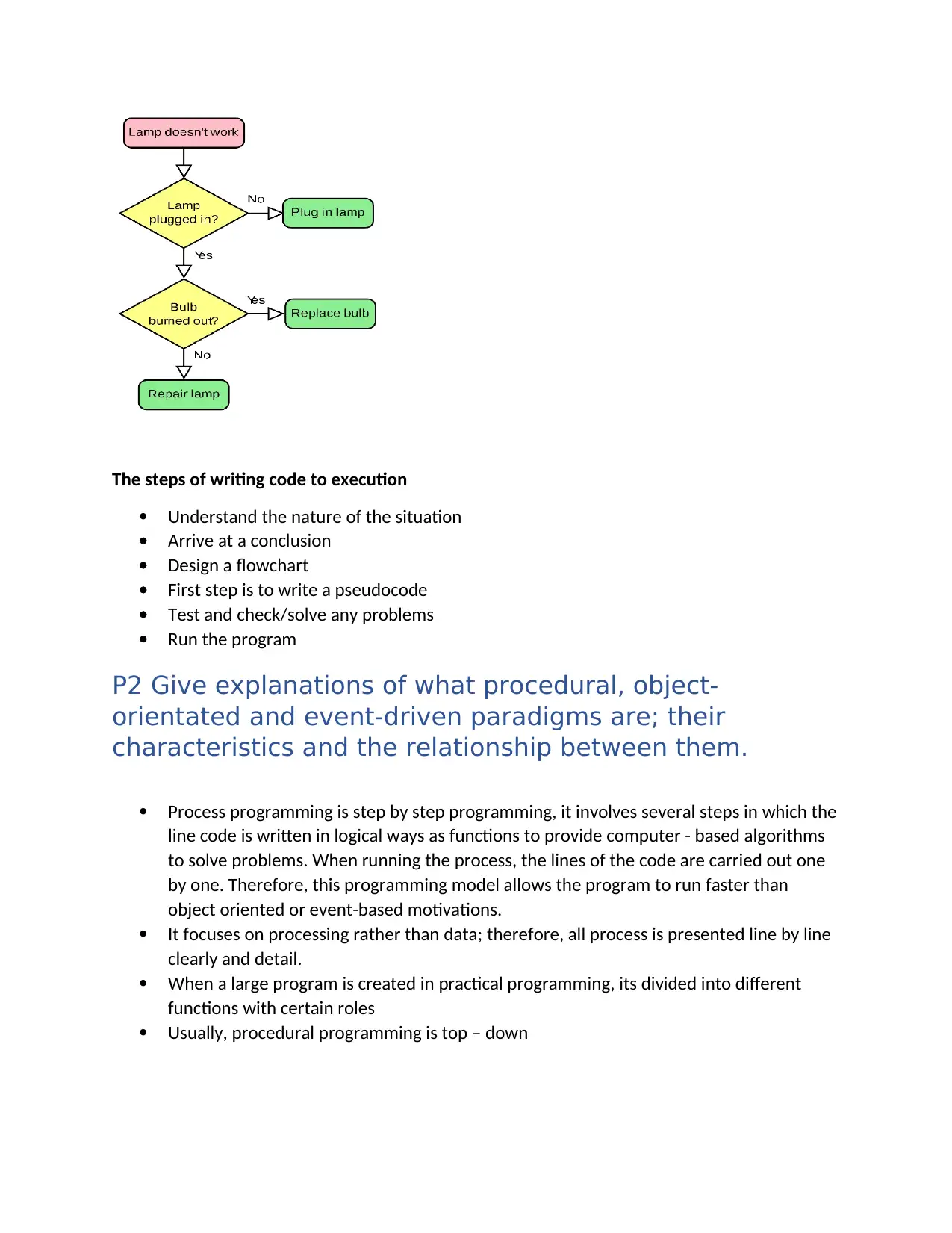
The steps of writing code to execution
Understand the nature of the situation
Arrive at a conclusion
Design a flowchart
First step is to write a pseudocode
Test and check/solve any problems
Run the program
P2 Give explanations of what procedural, object-
orientated and event-driven paradigms are; their
characteristics and the relationship between them.
Process programming is step by step programming, it involves several steps in which the
line code is written in logical ways as functions to provide computer - based algorithms
to solve problems. When running the process, the lines of the code are carried out one
by one. Therefore, this programming model allows the program to run faster than
object oriented or event-based motivations.
It focuses on processing rather than data; therefore, all process is presented line by line
clearly and detail.
When a large program is created in practical programming, its divided into different
functions with certain roles
Usually, procedural programming is top – down
Understand the nature of the situation
Arrive at a conclusion
Design a flowchart
First step is to write a pseudocode
Test and check/solve any problems
Run the program
P2 Give explanations of what procedural, object-
orientated and event-driven paradigms are; their
characteristics and the relationship between them.
Process programming is step by step programming, it involves several steps in which the
line code is written in logical ways as functions to provide computer - based algorithms
to solve problems. When running the process, the lines of the code are carried out one
by one. Therefore, this programming model allows the program to run faster than
object oriented or event-based motivations.
It focuses on processing rather than data; therefore, all process is presented line by line
clearly and detail.
When a large program is created in practical programming, its divided into different
functions with certain roles
Usually, procedural programming is top – down
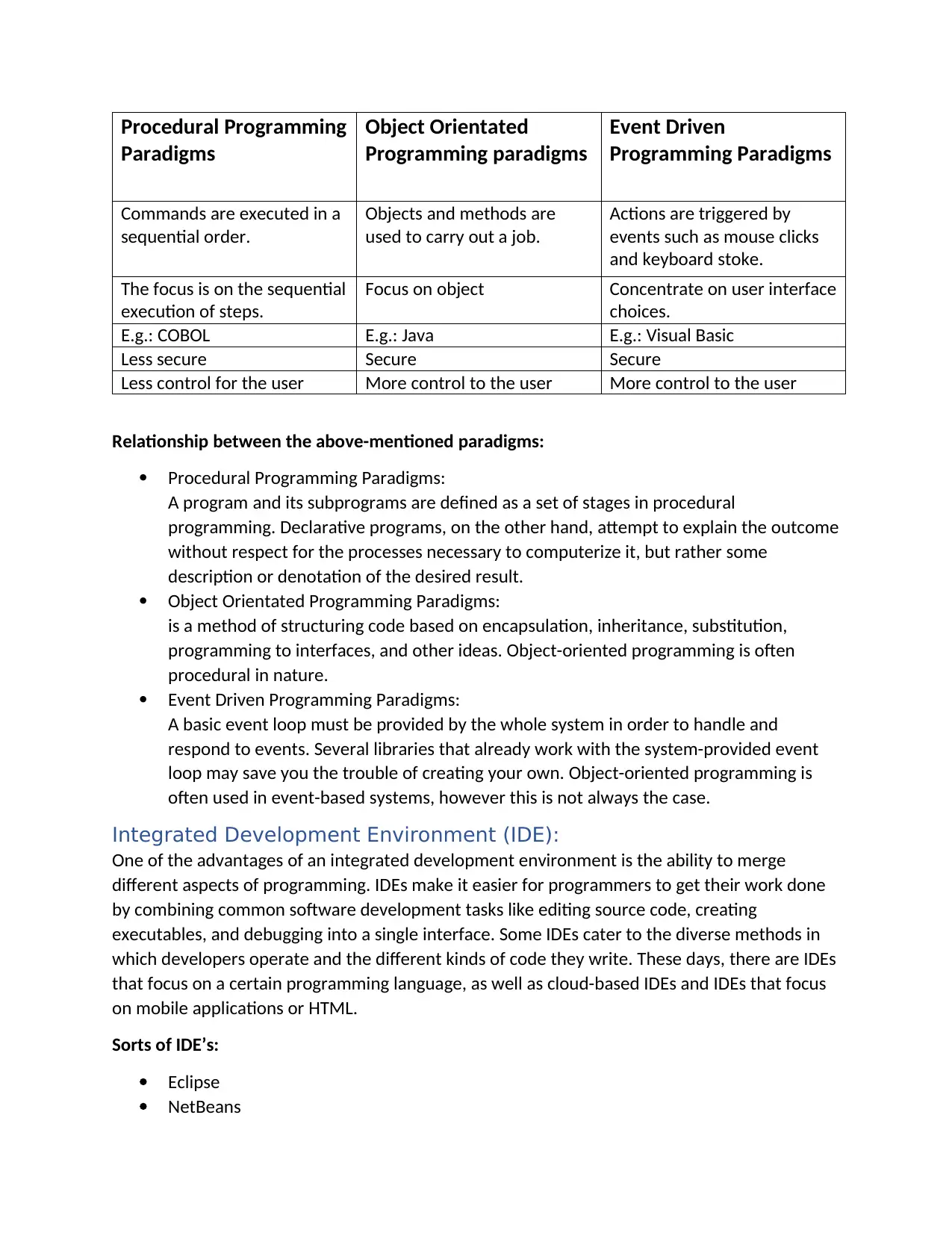
Procedural Programming
Paradigms
Object Orientated
Programming paradigms
Event Driven
Programming Paradigms
Commands are executed in a
sequential order.
Objects and methods are
used to carry out a job.
Actions are triggered by
events such as mouse clicks
and keyboard stoke.
The focus is on the sequential
execution of steps.
Focus on object Concentrate on user interface
choices.
E.g.: COBOL E.g.: Java E.g.: Visual Basic
Less secure Secure Secure
Less control for the user More control to the user More control to the user
Relationship between the above-mentioned paradigms:
Procedural Programming Paradigms:
A program and its subprograms are defined as a set of stages in procedural
programming. Declarative programs, on the other hand, attempt to explain the outcome
without respect for the processes necessary to computerize it, but rather some
description or denotation of the desired result.
Object Orientated Programming Paradigms:
is a method of structuring code based on encapsulation, inheritance, substitution,
programming to interfaces, and other ideas. Object-oriented programming is often
procedural in nature.
Event Driven Programming Paradigms:
A basic event loop must be provided by the whole system in order to handle and
respond to events. Several libraries that already work with the system-provided event
loop may save you the trouble of creating your own. Object-oriented programming is
often used in event-based systems, however this is not always the case.
Integrated Development Environment (IDE):
One of the advantages of an integrated development environment is the ability to merge
different aspects of programming. IDEs make it easier for programmers to get their work done
by combining common software development tasks like editing source code, creating
executables, and debugging into a single interface. Some IDEs cater to the diverse methods in
which developers operate and the different kinds of code they write. These days, there are IDEs
that focus on a certain programming language, as well as cloud-based IDEs and IDEs that focus
on mobile applications or HTML.
Sorts of IDE’s:
Eclipse
NetBeans
Paradigms
Object Orientated
Programming paradigms
Event Driven
Programming Paradigms
Commands are executed in a
sequential order.
Objects and methods are
used to carry out a job.
Actions are triggered by
events such as mouse clicks
and keyboard stoke.
The focus is on the sequential
execution of steps.
Focus on object Concentrate on user interface
choices.
E.g.: COBOL E.g.: Java E.g.: Visual Basic
Less secure Secure Secure
Less control for the user More control to the user More control to the user
Relationship between the above-mentioned paradigms:
Procedural Programming Paradigms:
A program and its subprograms are defined as a set of stages in procedural
programming. Declarative programs, on the other hand, attempt to explain the outcome
without respect for the processes necessary to computerize it, but rather some
description or denotation of the desired result.
Object Orientated Programming Paradigms:
is a method of structuring code based on encapsulation, inheritance, substitution,
programming to interfaces, and other ideas. Object-oriented programming is often
procedural in nature.
Event Driven Programming Paradigms:
A basic event loop must be provided by the whole system in order to handle and
respond to events. Several libraries that already work with the system-provided event
loop may save you the trouble of creating your own. Object-oriented programming is
often used in event-based systems, however this is not always the case.
Integrated Development Environment (IDE):
One of the advantages of an integrated development environment is the ability to merge
different aspects of programming. IDEs make it easier for programmers to get their work done
by combining common software development tasks like editing source code, creating
executables, and debugging into a single interface. Some IDEs cater to the diverse methods in
which developers operate and the different kinds of code they write. These days, there are IDEs
that focus on a certain programming language, as well as cloud-based IDEs and IDEs that focus
on mobile applications or HTML.
Sorts of IDE’s:
Eclipse
NetBeans
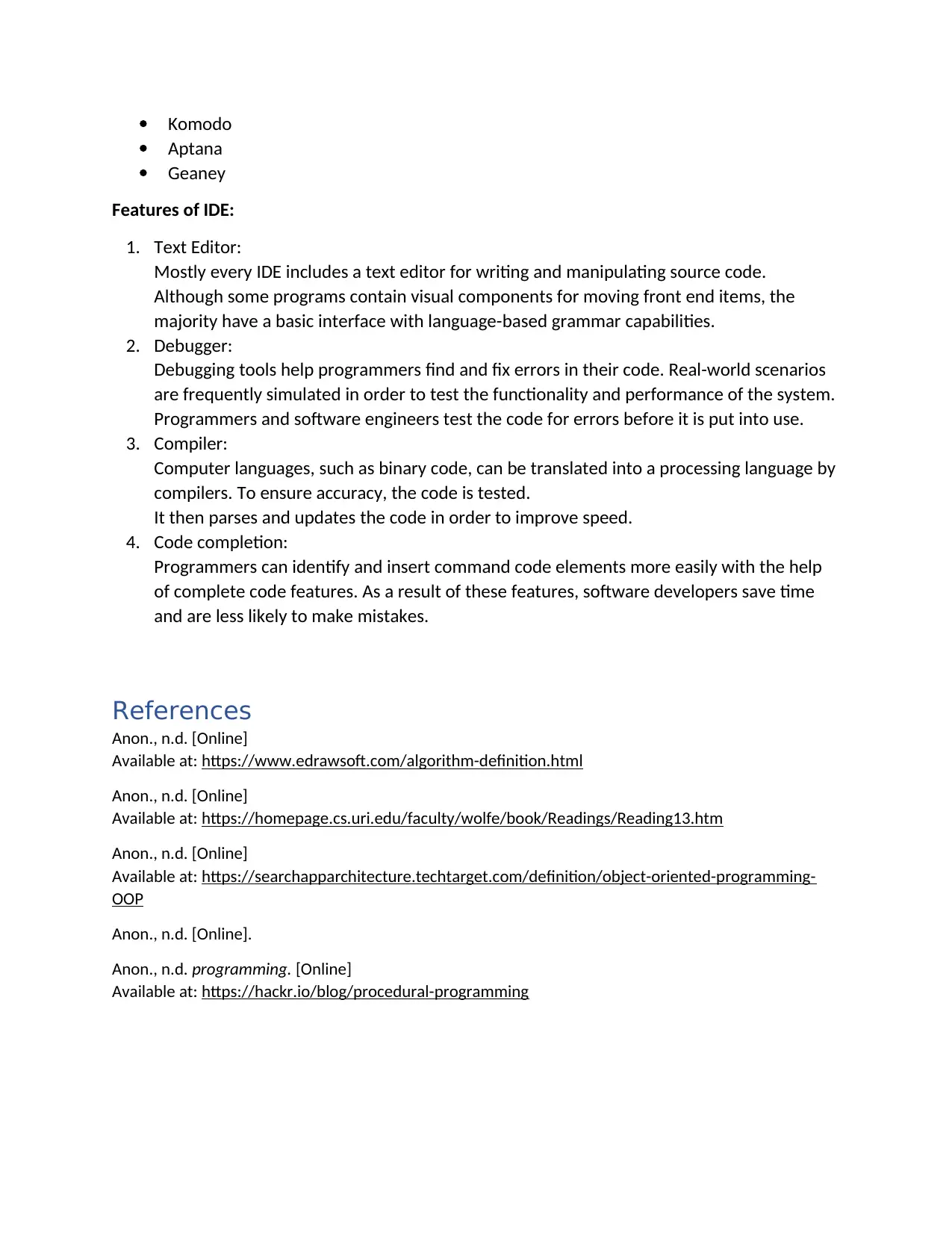
Komodo
Aptana
Geaney
Features of IDE:
1. Text Editor:
Mostly every IDE includes a text editor for writing and manipulating source code.
Although some programs contain visual components for moving front end items, the
majority have a basic interface with language-based grammar capabilities.
2. Debugger:
Debugging tools help programmers find and fix errors in their code. Real-world scenarios
are frequently simulated in order to test the functionality and performance of the system.
Programmers and software engineers test the code for errors before it is put into use.
3. Compiler:
Computer languages, such as binary code, can be translated into a processing language by
compilers. To ensure accuracy, the code is tested.
It then parses and updates the code in order to improve speed.
4. Code completion:
Programmers can identify and insert command code elements more easily with the help
of complete code features. As a result of these features, software developers save time
and are less likely to make mistakes.
References
Anon., n.d. [Online]
Available at: https://www.edrawsoft.com/algorithm-definition.html
Anon., n.d. [Online]
Available at: https://homepage.cs.uri.edu/faculty/wolfe/book/Readings/Reading13.htm
Anon., n.d. [Online]
Available at: https://searchapparchitecture.techtarget.com/definition/object-oriented-programming-
OOP
Anon., n.d. [Online].
Anon., n.d. programming. [Online]
Available at: https://hackr.io/blog/procedural-programming
Aptana
Geaney
Features of IDE:
1. Text Editor:
Mostly every IDE includes a text editor for writing and manipulating source code.
Although some programs contain visual components for moving front end items, the
majority have a basic interface with language-based grammar capabilities.
2. Debugger:
Debugging tools help programmers find and fix errors in their code. Real-world scenarios
are frequently simulated in order to test the functionality and performance of the system.
Programmers and software engineers test the code for errors before it is put into use.
3. Compiler:
Computer languages, such as binary code, can be translated into a processing language by
compilers. To ensure accuracy, the code is tested.
It then parses and updates the code in order to improve speed.
4. Code completion:
Programmers can identify and insert command code elements more easily with the help
of complete code features. As a result of these features, software developers save time
and are less likely to make mistakes.
References
Anon., n.d. [Online]
Available at: https://www.edrawsoft.com/algorithm-definition.html
Anon., n.d. [Online]
Available at: https://homepage.cs.uri.edu/faculty/wolfe/book/Readings/Reading13.htm
Anon., n.d. [Online]
Available at: https://searchapparchitecture.techtarget.com/definition/object-oriented-programming-
OOP
Anon., n.d. [Online].
Anon., n.d. programming. [Online]
Available at: https://hackr.io/blog/procedural-programming
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
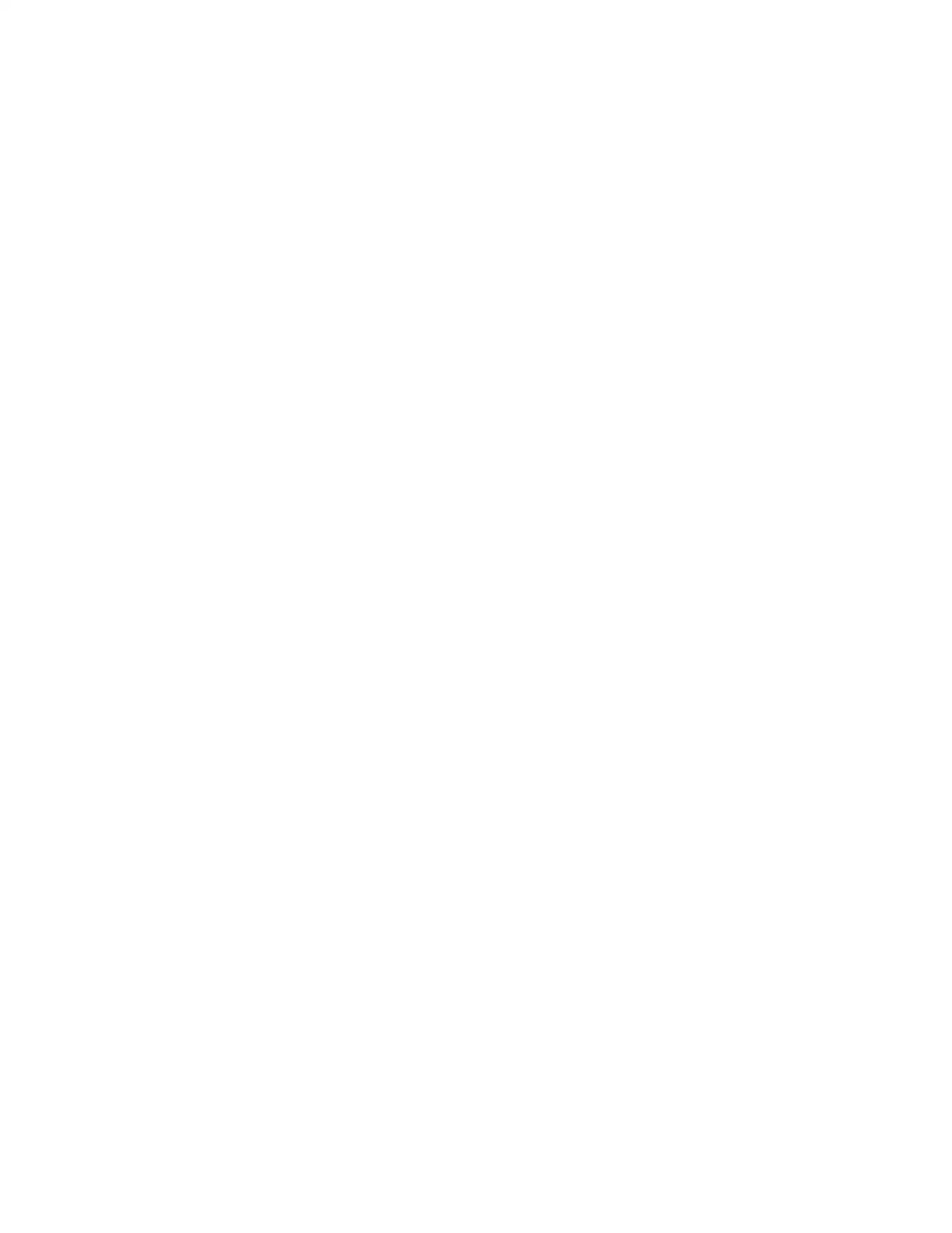
1 out of 8
Related Documents

Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.