Object-Oriented Programming Review: AP Computer Science A Units 1 & 2
VerifiedAdded on 2022/10/20

AP Computer Science A
Unit 1: Object Oriented Programming
Object-Oriented Programming: An approach to creating and using models of physical or
imagined objects
Compile: To translate a computer program from a high level language into another language
stats
Class: A user-definded blueprint of attributes and behaviors of an object
Object: An instance of a class
Attribute: A characteristic of an object
Behavior: An action that an object can perform
Each method is followed by parentheses()
Each method is written on its own line
Correct Syntax: art.paint(“blue”);
Incorrect Syntax: art.Paint
Software: A set of specific instructions that enables a computer to perform a task
Method: A named set of instructions to perform a task
Syntax: Rules for how code must be entered for a computer to understand
Case-Sensitive: Differentiating between lower and uppercase letters
Statement: Instructions that tell Java what to do\
Syntax Error: Mistakes in the code, such as typos and misspellings, that do not follow the syntax
rules of the programming language
Paraphrase This Document

An algorithm is a set of instructions to solve a problem or accomplish a task.
Bug: An error in the code
Boolean: A data type that can either be true or false
Conditional Statement: A statement that only runs under certain conditions
Algorithm: A set of instructions to solve a problem or accomplish a task
Debugging: Finding and fixing problems in an algorithm or program
Logic Error: When the code runs but does not do what was expected
Class & Object- ex: capeColor- blue, superPower- flying
Constructor- a block of code in a class that tells the computer how to create a new object
Constructor Signature- the first line of the constructor which includes the constructor name and
lists the attributes of the object that can be specified when the object is initialized
Initialize- create a new object with a specific name and a set of starting attributes
Actual Parameter- the value given to an attribute when initializing and object
Formal Parameter- the name of the attributes in the constructor signature

Syntax Error: Mistakes in the code, such as typos and misspelling, that do not follow the syntax
rules of the programming language
Method: A named set of instructions to perform a task
Object: an instance of class
Class: A user-defined blueprint from which objects are created
Debugging: Finding and fixing problems in an algorithm or program
Boolean: A data type that can be either true or false
Conditional Statement: A statement that only tuns under certain conditions
Compile: To translate human-readable source code into computer-executable machine code
Constructor: A block of code in the class that tells the computer how to create a new object
Initialize: Create a new object with a specific name and starting values for its attributes
Constructor Signature: The first line of the constructor which includes the constructor name and
lists the object’s attributes that can be specified when it’s initialized
Formal Parameter: The name of the attribute in the constructor signature
Actual Parameter: The value given to an attribute when initializing an object
Efficiency: Getting the best outcome with the least amount of waste
Always need two brackets at the end of the code, to define the class and object.
Subclass= truck, more specific
Super class= vehicle, broad
In Java, we can use ! to represent "not".
⊘ This is a preview!⊘
Do you want full access?
Subscribe today to unlock all pages.

Trusted by 1+ million students worldwide

!true reads "not true"
! reads as “not”.
Example: If it’s emily.(!canPaint), it means emily can’t paint
Inheritance: An object-oriented programming principle where a subclass inherits the instance
variables and methods of a superclass
Ex of an Inheritance: superclass- Cookie subclass- SugarCookie
Subclass: A class that inherits attributes and behaviors from a superclass
Superclass: A class from which subclasses can be created
Class Header: Consists of the keyword class and the name of the class
An edge case is a situation in a program that produces a different outcome and requires special
error handling.
Ex of edge case is, painter cannot move because of an obstacle
Paraphrase This Document

algorithm in English)
Example of the SprayPainter
sprayPaint (“blue”, “green”){
While (p.canMove);
p.turnLeft();
p.turnRight();
p.move();
p.paint(“blue”,”green”)
Test-Driven Development: A process that involves writing the test first, then writing the code to
make the test pass
Inheritance- ex. Painterplus inherits methods from Painter
String- it’s in quotes
Public= it is available to everyone
static= this method won’t be changed
void= this method does not return anything
main(...)= this method will execute code that does stuff when compiled and executed
{},[],()- requires open and close
{}= code blocks, code goes in here
()- method calls, parameter definitions, conditionals
Every line of code that does something requires a semi-color unless it’s in a conditional:
kitty.canMove();
If (kitty.canMove()){
}
Booleans- true or false
Negation- flips the value, !true= false, !canMove=can’t move

class
Conditionals - if/else/while
Inheritance implies a/an “is-a” relationship
EX: United Airlines is an Airline, Blue is a Color
Serialization- the processs of turning an object into a string
- JSON- used for serialization for JAVA
Deserialization- the process of turning a string into an object
- JSON- used for deserialization for JAVA
Variable is to be able to reuse data or to make it obvious what data it is or it has
- To store data
- To reuse data
- To give simple naming
- Represents variables of an object
A string can be a number, if it is in quotation marks
- A string is a value inside of quotation marks
A constructor sets the initial state of an object
Public- accessible outside of the class
Private- only inside of the class
Void- doesn’t return anything
Formal parameters- (int x, int y, string color)
Actual parameters- (0,0, “green”)
int: A data type that can store integers, or whole numbers
double: A data type that can store a decimal number
boolean: A data type that can be either true or false
⊘ This is a preview!⊘
Do you want full access?
Subscribe today to unlock all pages.

Trusted by 1+ million students worldwide

Variable: A container that stores a value in memory
Declaration: Giving a name and data type to a variable
Initialization: Giving a starting value to a variable using the assignment operator (=)
Data Type: The format of the data that can be stored in a variable
Primitive Type: A basic data type that is predefined by Java
Reference Type: A data type that contains a pointer to the memory location of an object
This., refers to it being used from a different class, used when they have the same name
Ex:
int i =10
String s = “10”
Private int chips;
Public cookie (int chips){
This.chips = chips;
}
Local variable can only be used inside of constructor, only where they are created
S in string is always capatilized in java
Char[] is the same as String
^
Character Array
System.out.println from java = console.log from javascript
Unit 2: Giving Objects State
Paraphrase This Document

- These represent the attributes, characteristics of an object
Intsance variables in the private class means they are only accessible from inside the class itself
Private Class, so that no one messes with the stuff inside of the class (keeps code clean and safe,
could introduce security vulnerabilities)
Data Encapsulation- (information hiding) an object-oriented programming concept where the
details of a class are hidden from the user
Different types of names for variables- double, int, and boolean
How to declare variables-
First put the type
Then put the value (if known)
Ex: double cakePrice;
double cakePrice = 1.25
Instance variables= variables that are used inside of a certain class
Ex.
this.cookie= cookie
this.color= color
this.flavor= flavor this. is used if you want to name the variable the same name

public: Indicates that an instance variable or method is accessible from outside of the class
private: Indicates that an instance variable or method is accessible only from within the class
Instance Variable: A variable defined in a class which represents an attribute of an object
Attribute: A characteristic of an object
Has-A Relationship: The relationship between an object and its instance variables
Data Encapsulation: An object-oriented programming concept where the details of a class are
hidden from the user; also referred to as information hiding
Variables are containers that allow us to hold something.
int= whole number
string= anything in “”
double= decimal numbers
Assignment: Using the assignment operator (=) to initialize or change the value stored in a
variable
Literal: A source code representation of a value, such as a number or text
int: A data type that can store integers, or whole numbers
double: A data type that can store a decimal number
String: A data type that contains a sequence of characters enclosed in double quotations (" ")
boolean: A data type that can be either true or false
concactenation= string + string
null: Indicates that the variable does not refer to any object
Truncate: rounding down (used when dividing integers) ex: 3.8 = 3 2.2= 2
⊘ This is a preview!⊘
Do you want full access?
Subscribe today to unlock all pages.

Trusted by 1+ million students worldwide
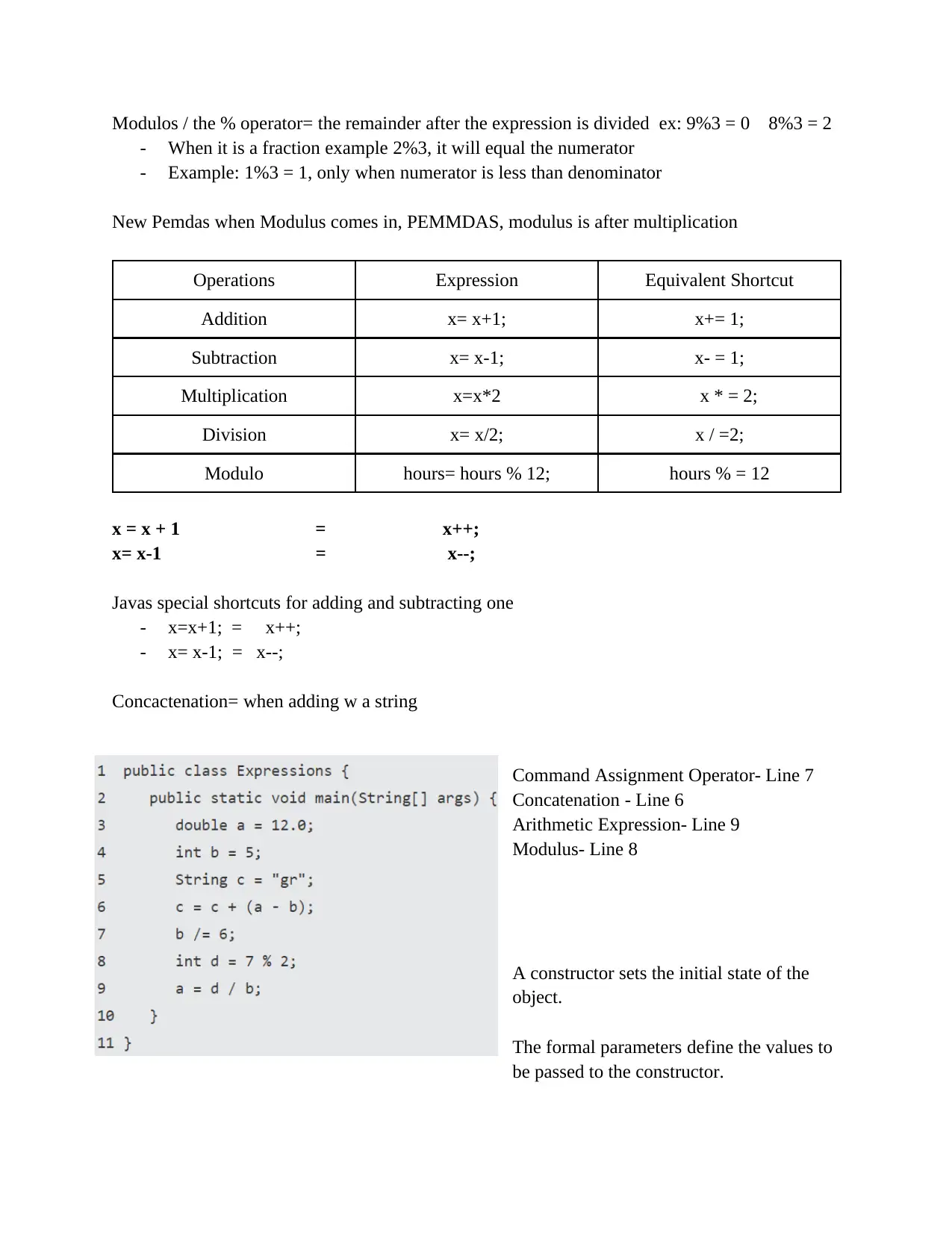
- When it is a fraction example 2%3, it will equal the numerator
- Example: 1%3 = 1, only when numerator is less than denominator
New Pemdas when Modulus comes in, PEMMDAS, modulus is after multiplication
Operations Expression Equivalent Shortcut
Addition x= x+1; x+= 1;
Subtraction x= x-1; x- = 1;
Multiplication x=x*2 x * = 2;
Division x= x/2; x / =2;
Modulo hours= hours % 12; hours % = 12
x = x + 1 = x++;
x= x-1 = x--;
Javas special shortcuts for adding and subtracting one
- x=x+1; = x++;
- x= x-1; = x--;
Concactenation= when adding w a string
Command Assignment Operator- Line 7
Concatenation - Line 6
Arithmetic Expression- Line 9
Modulus- Line 8
A constructor sets the initial state of the
object.
The formal parameters define the values to
be passed to the constructor.
Paraphrase This Document

Constructor signature- includes the constructor's name and lists the attributes that can be
specified when it is initialized.
Scope: Where a variable can be used
This keyword : refers to the current object in a method or a constructor
Inheritance: An object-oriented programming principle where a subclass inherits the instance
variables and methods of a superclass
super: A keyword used to refer to the superclass
DRY Principle: Stands for “Don’t Repeat Yourself”; a software development principle to reduce
repetition in code
Refactoring: Improving the readability, reusability, or structure of program code without altering
its functionality
Accessor Method- public method, that lets you get the value of each instance variable from
outside of the class
String- an object
Int & double- primatives (raw values)
Class and object are capitalized
Accessor Method: Gives the value stored in an instance variable
Method Signature: Consists of a name and parameter list
return Keyword: Exits a method and provides the value to where the method is called
Return Type: The type of the value to be given from the method
Return By Value: A copy of the value is given to where the method is called
Dot Operator: Used to call a method in a class
State: The attributes of an object that are represented by its instance variables

Accesibility categories in Java: private, public, protected
Public string toString(){
Return “Number of Calories:” + calories + “Name: “ + name + “Price “ + price;
}
^ Different from print info because it is specific
Escape sequences are character pairs with special meaning. They are used within Strings to
format output , EX: \n= new line \”= quotes inside of the string \\= a backslash in the code
Unit 3: Expanding Program Data
⊘ This is a preview!⊘
Do you want full access?
Subscribe today to unlock all pages.

Trusted by 1+ million students worldwide

One-Dimensional Array - a linear structure that stores one or more values of the same data type
Element- item stored in the array
Index values- numerical location in the array, beginning with 0 (First element is index 0)
Length: The size of the array that is set when it is created
Initializer List - Creates and initializes the elements in an array to a list of values given inside
curly braces { }
Format for Arrays : declaring and initializing
- String[]names= new String [5];
- String [] flavors = {“chocolate”, “vanilla”, “red velvet”, “strawberry”, “banana”
If you try to use an index that is out of the bounds of the array (trying to print out 5 when there is
only 4), ArrayIndexOutOfBoundsException
ArrayOutOfBoundsExecption: An index value that is either negative or greater than or equal to
the length of the array
Immutable- cannot be changed
Length-1 is the last item in an array, if called it prints out the last item
Example of a for loop:
For (int i = 0; i<5; i++){
System.out.println(i);
}
The order is initialization, condition, iteration
i starts out as zero, this code will run while i is less than 5, each time the code runs it adds 1, this
prints, 0,1,2,3,4
Loop control variable- the variable that is used in the loop (ex. i, x, y)
i++ = i+1
Paraphrase This Document

Other variables can be used, but i is most widely used
Outer Loop = vertical aspect (rows)
Inner Loop= horizontal (column)
It is easier to understand a for loop as, (x,y,z)
- X, is what it starts off as
- Y, is the condition that it runs under (will not run if condition is not applicable)
- Z, the update that happens each time the loop is run
x += y in Java is the same as x = x + y
for Loop: A loop that has a predetermined beginning, end, and increment or decrement
Traverse: To move through an array
Increment: To increase a value by another value
Decrement: To decrease a value by another value
Loop Control Variable: A variable declared and used within a loop to count iterations
through the loop
Infinite loop- loop that never stops running
If the Boolean expression evaluates to false initially, the loop body is not executed.
while Loop: Executes a block of code repeatedly as long as a condition is true
Polymorphism is the ability to perform an action in different ways.
Ex : getting a constructor to work, with the classes in dif places (has to do with
inheritance)
while loops are used when you want the loop to execute until a condition is met.
for loops are used when you know the number of times you want the loop to execute.
An enhanced for-loop is a simplified for loop to iterate through every element of an array
starting at index 0.

Enhanced for Loop Variable: A variable declared and used within an enhanced for loop
to store a copy of the element visited
A static variable is a variable defined in a class that stores a shared value for all
instances of the class.
- can be created by adding the static
- keyword before the variable during declaration.
The static keyword is used to specify that a variable or method belongs to a class
instead of an instance of a class.
When we assign a value to an instance variable, it is only changed for the object that it
belongs to.
If we change the value of a static variable for one object,
it changes it for all objects of the class.
A constant is a variable containing a value that cannot be changed.
final Keyword: The keyword used to specify that a value cannot be changed
An off-by-one error occurs when we try to access an index value that is greater than the
length of the array. (same as index out of bounds)
Algorithm: A set of instructions to solve a problem or accomplish a task
A nested loop is a loop that exists in the body of another loop.
- Outer loop represents vertical
- Inner loop represents the horizontal
(int)(Math.random()*range) + min moves the random number into a range starting from
a minimum number.
The range is the (max number - min number + 1).
⊘ This is a preview!⊘
Do you want full access?
Subscribe today to unlock all pages.

Trusted by 1+ million students worldwide

To Access a Row:
arrName[row]
To Access a Value:
arrName[row][column]
Return -1 means it’s not found/not there
Do % 10 to get the rightmost value
When you distribute ! the && turns into a ||
If they are all ors || or all ands &&, get ride of parentheses
!(!)(a&&b)||(c||!d) = (a&&b)
int[][] values = {{7, 4, 6}, {9, 2, 5}, {8, 3, 1}};
int theNumber = values[0][0];
int result = 0;
for (int row = 0; row < values.length; row++) {
for (int col = 0; col < values[0].length; col++) {
if (values[row][col] > theNumber) {
theNumber = values[row][col];
result = row;
}
}
}
System.out.println("Result = " + result);
Row with the greatest number is 1, because it has 9 . this code returns 1.
Natural Processing Language: The ability of a computer program to understand human
language
Documentation: Written descriptions of the purpose and functionality of code
Javadocs: The documentation tool for Java that generates an HTML document from
comments written inside /** */ and formatted using @ tags
Paraphrase This Document

files to be displayed on the World Wide Web
Precondition: A condition that must be true just before a block of code can execute
Postcondition: A condition that must be true just after a block of code executes
Reference types have capital letter objects, primitives are lowercase
Primitive stores just the value
Reference is where the variable has the memory location of the object
For every primitive type, java has a class
Wrapper classes: provide a way to use primitive data types ( int , boolean , etc..) as
objects

⊘ This is a preview!⊘
Do you want full access?
Subscribe today to unlock all pages.

Trusted by 1+ million students worldwide


Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
© 2024 | Zucol Services PVT LTD | All rights reserved.