Object-Oriented C++ Design for Genetic Algorithm Solver Project, BEng
VerifiedAdded on 2023/01/18
|8
|1573
|93
Project
AI Summary
This project details the implementation of a genetic algorithm solver using C++ and object-oriented design principles. The project focuses on analyzing data from two text files, implementing the algorithm to determine the best value, generation value, and fitness value. It incorporates concepts such as encapsulation, inheritance, and polymorphism within the object-oriented design. The project includes a UML class diagram to illustrate the relationships between different classes like Genetic Solver, Initialization, Best Fitness, Parameter, and Best Value. The algorithm analyzes various strategies like mutation and crossover to optimize the solver's performance. The analysis includes calculating values like generation count, average fitness, and standard deviation to determine the best fitness value. The project successfully demonstrates the application of the genetic algorithm solver for the given data variables.
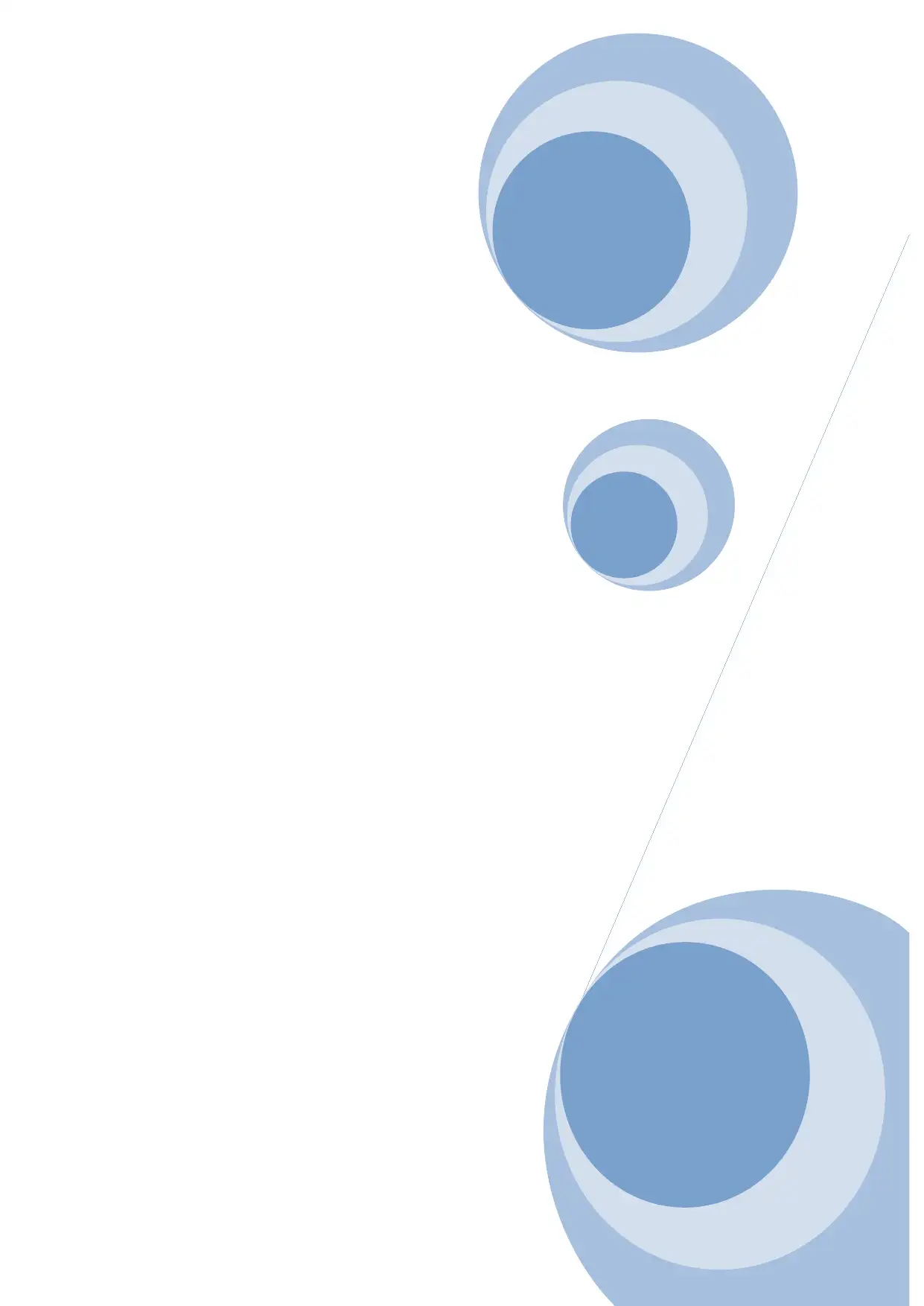
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
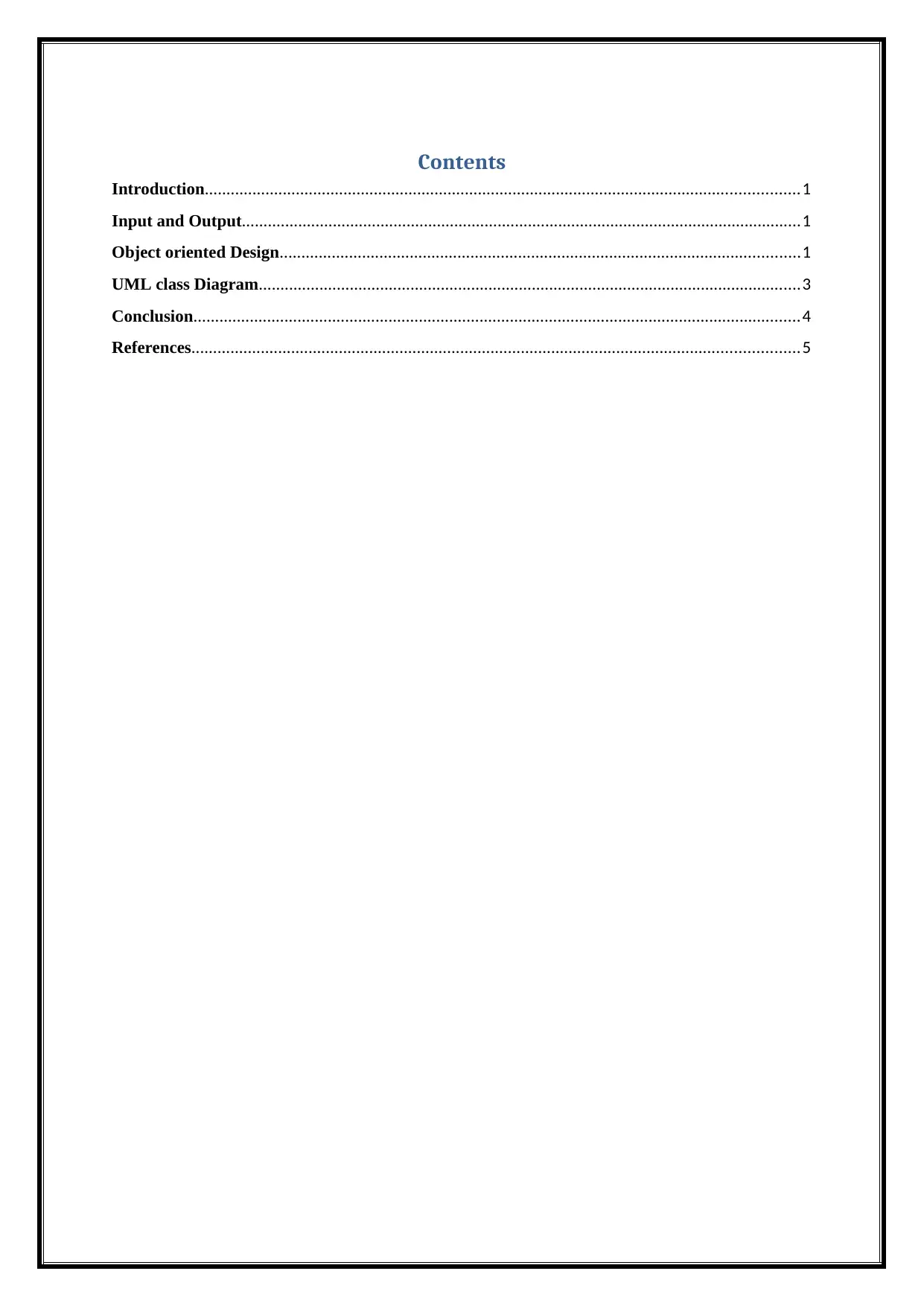
Contents
Introduction.........................................................................................................................................1
Input and Output.................................................................................................................................1
Object oriented Design........................................................................................................................1
UML class Diagram.............................................................................................................................3
Conclusion............................................................................................................................................4
References............................................................................................................................................5
Introduction.........................................................................................................................................1
Input and Output.................................................................................................................................1
Object oriented Design........................................................................................................................1
UML class Diagram.............................................................................................................................3
Conclusion............................................................................................................................................4
References............................................................................................................................................5
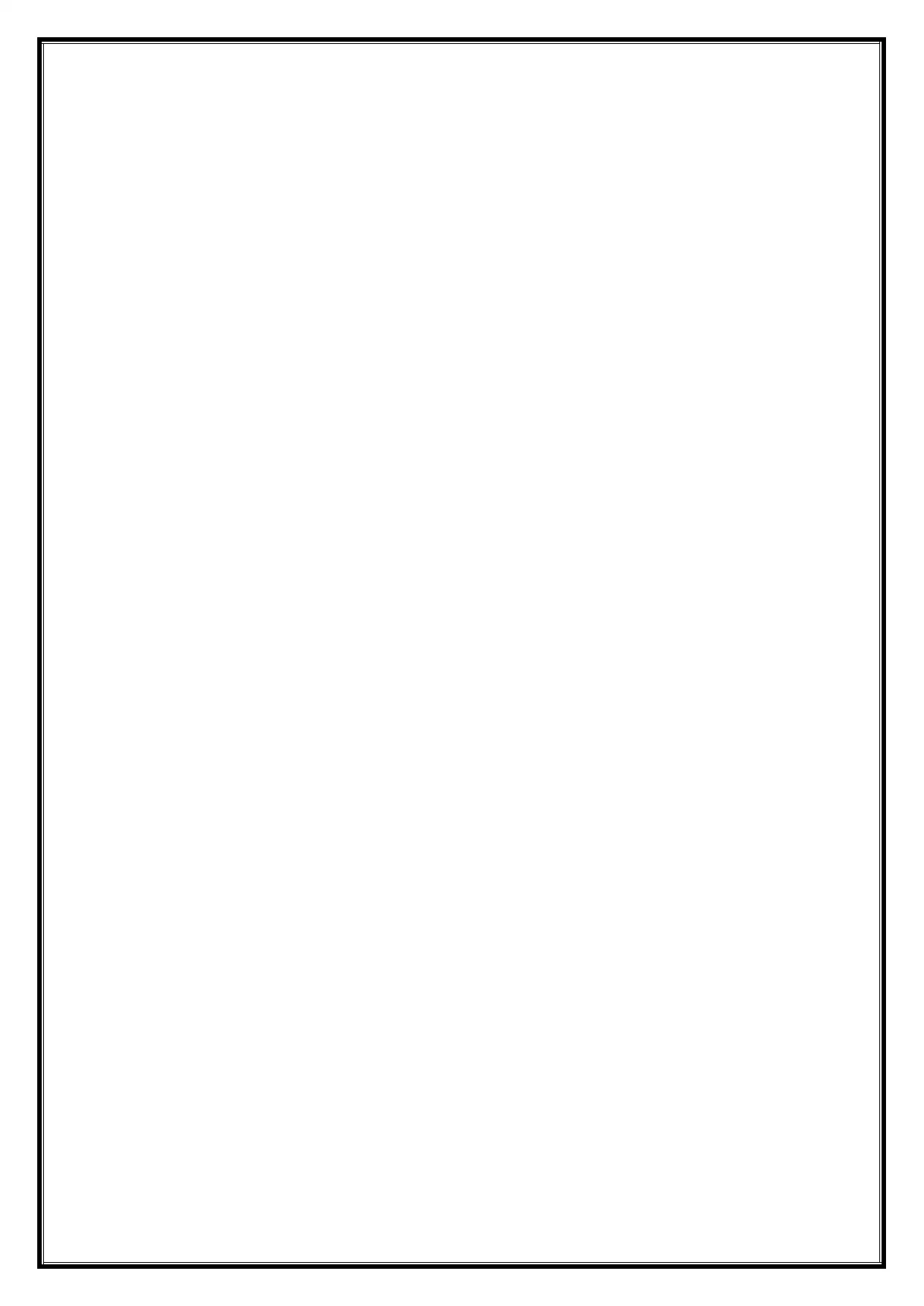
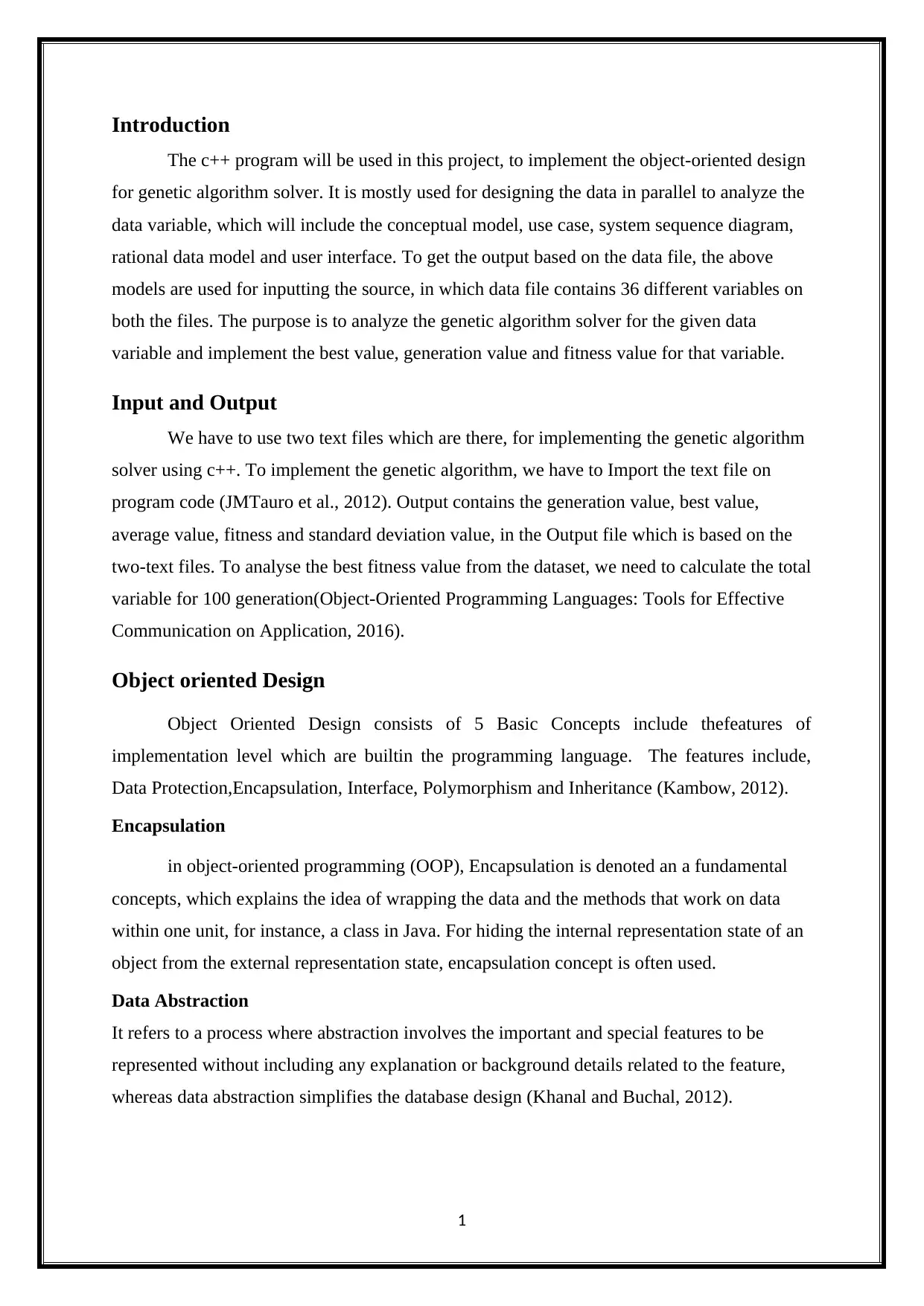
Introduction
The c++ program will be used in this project, to implement the object-oriented design
for genetic algorithm solver. It is mostly used for designing the data in parallel to analyze the
data variable, which will include the conceptual model, use case, system sequence diagram,
rational data model and user interface. To get the output based on the data file, the above
models are used for inputting the source, in which data file contains 36 different variables on
both the files. The purpose is to analyze the genetic algorithm solver for the given data
variable and implement the best value, generation value and fitness value for that variable.
Input and Output
We have to use two text files which are there, for implementing the genetic algorithm
solver using c++. To implement the genetic algorithm, we have to Import the text file on
program code (JMTauro et al., 2012). Output contains the generation value, best value,
average value, fitness and standard deviation value, in the Output file which is based on the
two-text files. To analyse the best fitness value from the dataset, we need to calculate the total
variable for 100 generation(Object-Oriented Programming Languages: Tools for Effective
Communication on Application, 2016).
Object oriented Design
Object Oriented Design consists of 5 Basic Concepts include thefeatures of
implementation level which are builtin the programming language. The features include,
Data Protection,Encapsulation, Interface, Polymorphism and Inheritance (Kambow, 2012).
Encapsulation
in object-oriented programming (OOP), Encapsulation is denoted an a fundamental
concepts, which explains the idea of wrapping the data and the methods that work on data
within one unit, for instance, a class in Java. For hiding the internal representation state of an
object from the external representation state, encapsulation concept is often used.
Data Abstraction
It refers to a process where abstraction involves the important and special features to be
represented without including any explanation or background details related to the feature,
whereas data abstraction simplifies the database design (Khanal and Buchal, 2012).
1
The c++ program will be used in this project, to implement the object-oriented design
for genetic algorithm solver. It is mostly used for designing the data in parallel to analyze the
data variable, which will include the conceptual model, use case, system sequence diagram,
rational data model and user interface. To get the output based on the data file, the above
models are used for inputting the source, in which data file contains 36 different variables on
both the files. The purpose is to analyze the genetic algorithm solver for the given data
variable and implement the best value, generation value and fitness value for that variable.
Input and Output
We have to use two text files which are there, for implementing the genetic algorithm
solver using c++. To implement the genetic algorithm, we have to Import the text file on
program code (JMTauro et al., 2012). Output contains the generation value, best value,
average value, fitness and standard deviation value, in the Output file which is based on the
two-text files. To analyse the best fitness value from the dataset, we need to calculate the total
variable for 100 generation(Object-Oriented Programming Languages: Tools for Effective
Communication on Application, 2016).
Object oriented Design
Object Oriented Design consists of 5 Basic Concepts include thefeatures of
implementation level which are builtin the programming language. The features include,
Data Protection,Encapsulation, Interface, Polymorphism and Inheritance (Kambow, 2012).
Encapsulation
in object-oriented programming (OOP), Encapsulation is denoted an a fundamental
concepts, which explains the idea of wrapping the data and the methods that work on data
within one unit, for instance, a class in Java. For hiding the internal representation state of an
object from the external representation state, encapsulation concept is often used.
Data Abstraction
It refers to a process where abstraction involves the important and special features to be
represented without including any explanation or background details related to the feature,
whereas data abstraction simplifies the database design (Khanal and Buchal, 2012).
1
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
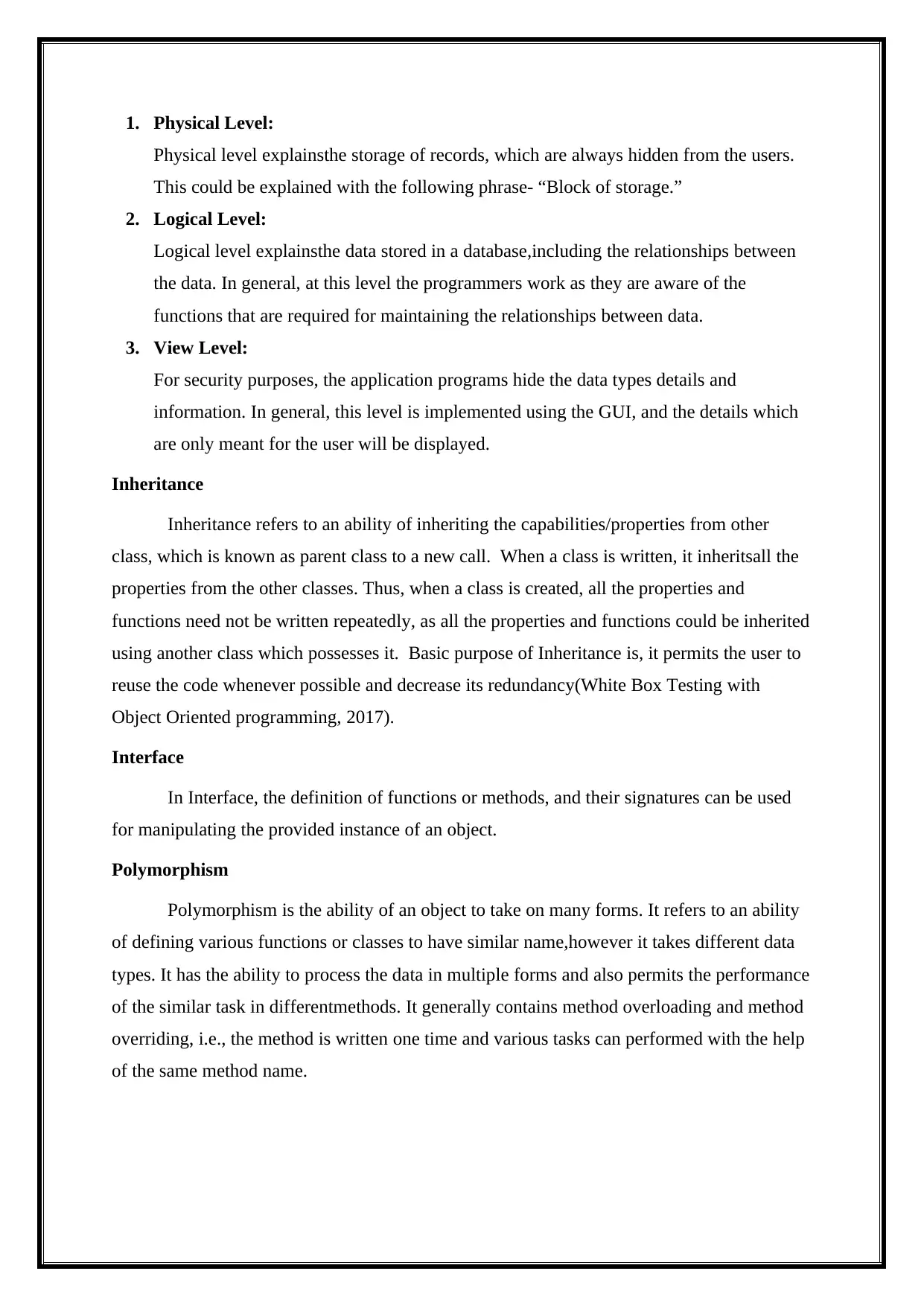
1. Physical Level:
Physical level explainsthe storage of records, which are always hidden from the users.
This could be explained with the following phrase- “Block of storage.”
2. Logical Level:
Logical level explainsthe data stored in a database,including the relationships between
the data. In general, at this level the programmers work as they are aware of the
functions that are required for maintaining the relationships between data.
3. View Level:
For security purposes, the application programs hide the data types details and
information. In general, this level is implemented using the GUI, and the details which
are only meant for the user will be displayed.
Inheritance
Inheritance refers to an ability of inheriting the capabilities/properties from other
class, which is known as parent class to a new call. When a class is written, it inheritsall the
properties from the other classes. Thus, when a class is created, all the properties and
functions need not be written repeatedly, as all the properties and functions could be inherited
using another class which possesses it. Basic purpose of Inheritance is, it permits the user to
reuse the code whenever possible and decrease its redundancy(White Box Testing with
Object Oriented programming, 2017).
Interface
In Interface, the definition of functions or methods, and their signatures can be used
for manipulating the provided instance of an object.
Polymorphism
Polymorphism is the ability of an object to take on many forms. It refers to an ability
of defining various functions or classes to have similar name,however it takes different data
types. It has the ability to process the data in multiple forms and also permits the performance
of the similar task in differentmethods. It generally contains method overloading and method
overriding, i.e., the method is written one time and various tasks can performed with the help
of the same method name.
Physical level explainsthe storage of records, which are always hidden from the users.
This could be explained with the following phrase- “Block of storage.”
2. Logical Level:
Logical level explainsthe data stored in a database,including the relationships between
the data. In general, at this level the programmers work as they are aware of the
functions that are required for maintaining the relationships between data.
3. View Level:
For security purposes, the application programs hide the data types details and
information. In general, this level is implemented using the GUI, and the details which
are only meant for the user will be displayed.
Inheritance
Inheritance refers to an ability of inheriting the capabilities/properties from other
class, which is known as parent class to a new call. When a class is written, it inheritsall the
properties from the other classes. Thus, when a class is created, all the properties and
functions need not be written repeatedly, as all the properties and functions could be inherited
using another class which possesses it. Basic purpose of Inheritance is, it permits the user to
reuse the code whenever possible and decrease its redundancy(White Box Testing with
Object Oriented programming, 2017).
Interface
In Interface, the definition of functions or methods, and their signatures can be used
for manipulating the provided instance of an object.
Polymorphism
Polymorphism is the ability of an object to take on many forms. It refers to an ability
of defining various functions or classes to have similar name,however it takes different data
types. It has the ability to process the data in multiple forms and also permits the performance
of the similar task in differentmethods. It generally contains method overloading and method
overriding, i.e., the method is written one time and various tasks can performed with the help
of the same method name.
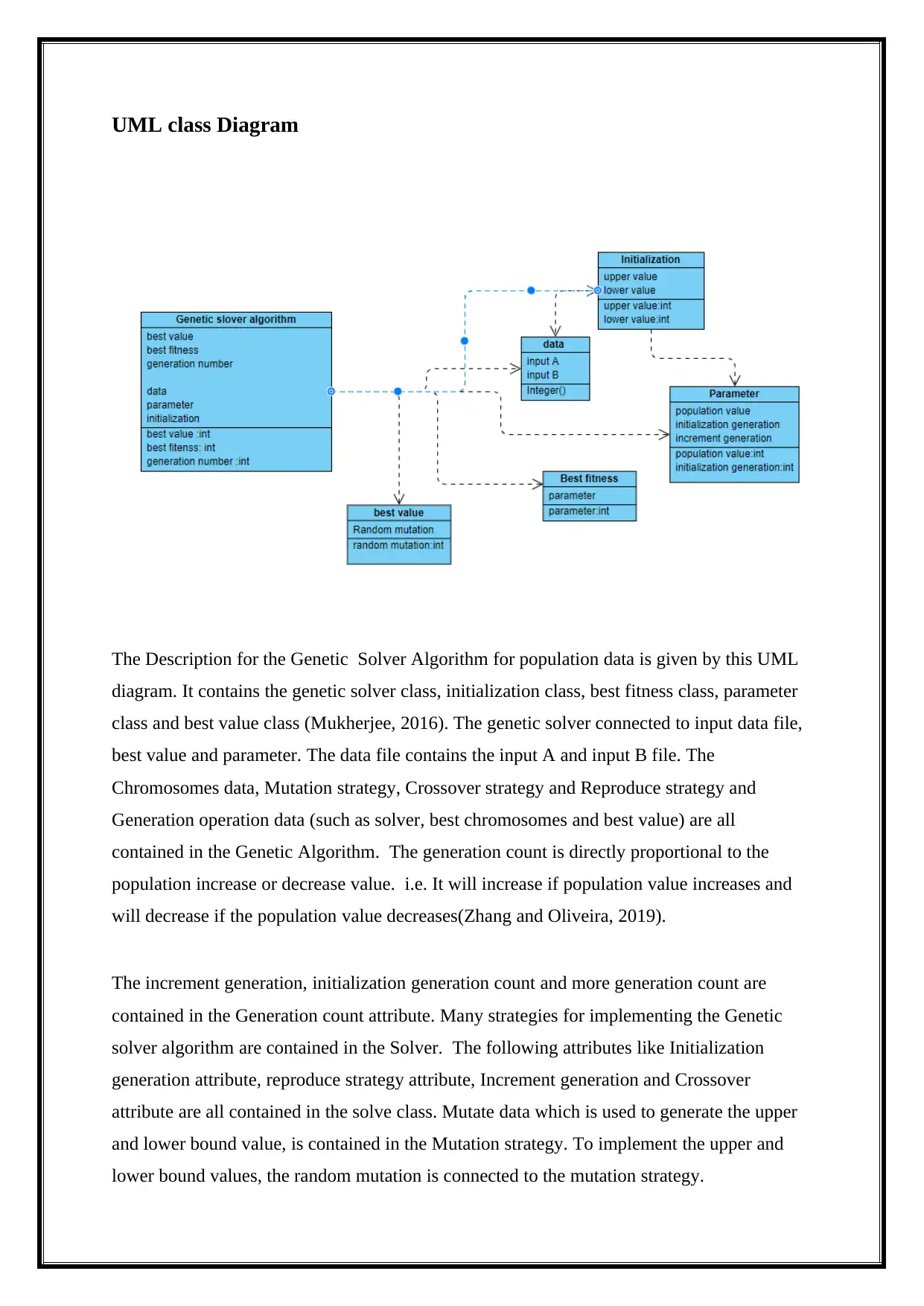
UML class Diagram
The Description for the Genetic Solver Algorithm for population data is given by this UML
diagram. It contains the genetic solver class, initialization class, best fitness class, parameter
class and best value class (Mukherjee, 2016). The genetic solver connected to input data file,
best value and parameter. The data file contains the input A and input B file. The
Chromosomes data, Mutation strategy, Crossover strategy and Reproduce strategy and
Generation operation data (such as solver, best chromosomes and best value) are all
contained in the Genetic Algorithm. The generation count is directly proportional to the
population increase or decrease value. i.e. It will increase if population value increases and
will decrease if the population value decreases(Zhang and Oliveira, 2019).
The increment generation, initialization generation count and more generation count are
contained in the Generation count attribute. Many strategies for implementing the Genetic
solver algorithm are contained in the Solver. The following attributes like Initialization
generation attribute, reproduce strategy attribute, Increment generation and Crossover
attribute are all contained in the solve class. Mutate data which is used to generate the upper
and lower bound value, is contained in the Mutation strategy. To implement the upper and
lower bound values, the random mutation is connected to the mutation strategy.
The Description for the Genetic Solver Algorithm for population data is given by this UML
diagram. It contains the genetic solver class, initialization class, best fitness class, parameter
class and best value class (Mukherjee, 2016). The genetic solver connected to input data file,
best value and parameter. The data file contains the input A and input B file. The
Chromosomes data, Mutation strategy, Crossover strategy and Reproduce strategy and
Generation operation data (such as solver, best chromosomes and best value) are all
contained in the Genetic Algorithm. The generation count is directly proportional to the
population increase or decrease value. i.e. It will increase if population value increases and
will decrease if the population value decreases(Zhang and Oliveira, 2019).
The increment generation, initialization generation count and more generation count are
contained in the Generation count attribute. Many strategies for implementing the Genetic
solver algorithm are contained in the Solver. The following attributes like Initialization
generation attribute, reproduce strategy attribute, Increment generation and Crossover
attribute are all contained in the solve class. Mutate data which is used to generate the upper
and lower bound value, is contained in the Mutation strategy. To implement the upper and
lower bound values, the random mutation is connected to the mutation strategy.
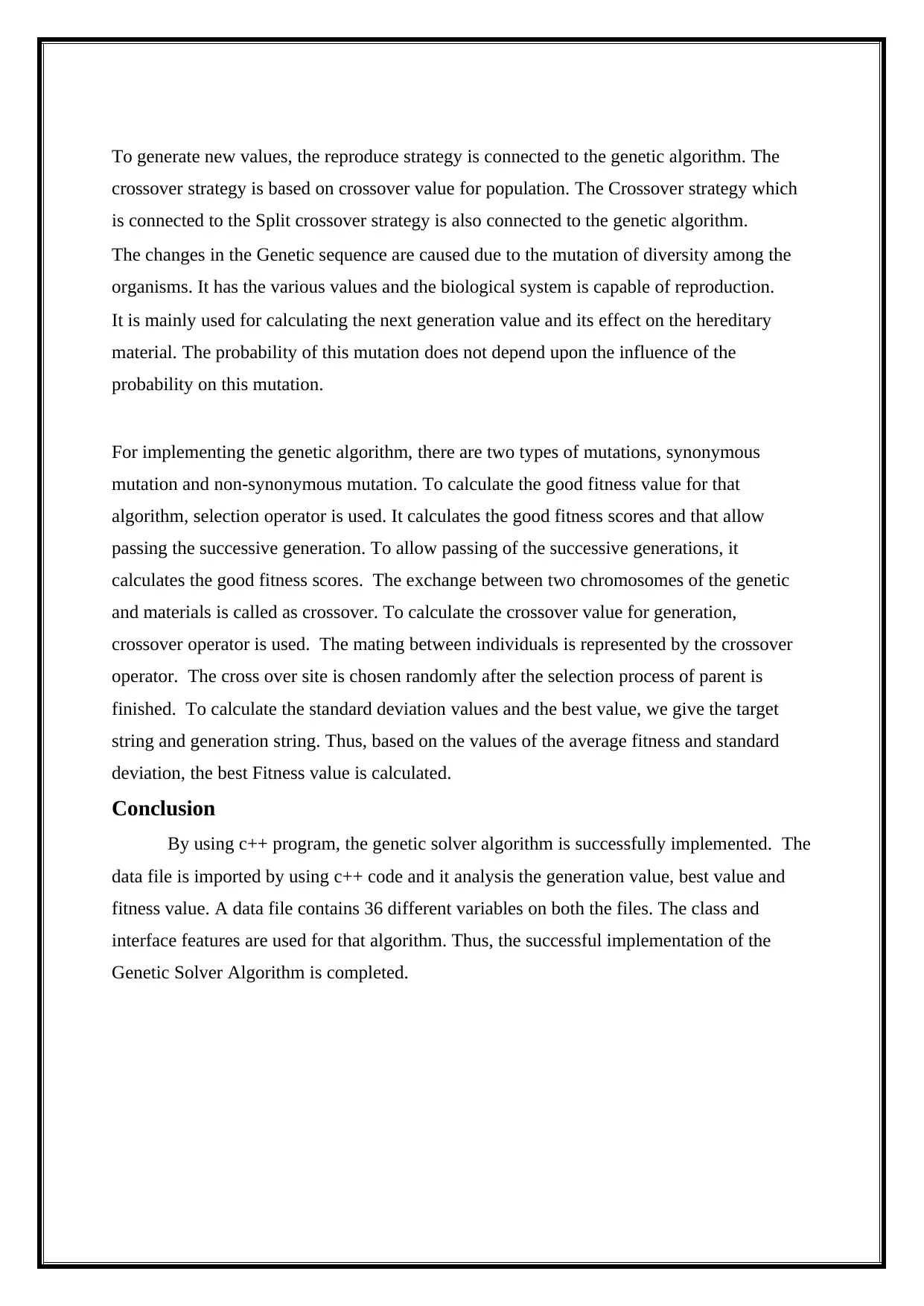
To generate new values, the reproduce strategy is connected to the genetic algorithm. The
crossover strategy is based on crossover value for population. The Crossover strategy which
is connected to the Split crossover strategy is also connected to the genetic algorithm.
The changes in the Genetic sequence are caused due to the mutation of diversity among the
organisms. It has the various values and the biological system is capable of reproduction.
It is mainly used for calculating the next generation value and its effect on the hereditary
material. The probability of this mutation does not depend upon the influence of the
probability on this mutation.
For implementing the genetic algorithm, there are two types of mutations, synonymous
mutation and non-synonymous mutation. To calculate the good fitness value for that
algorithm, selection operator is used. It calculates the good fitness scores and that allow
passing the successive generation. To allow passing of the successive generations, it
calculates the good fitness scores. The exchange between two chromosomes of the genetic
and materials is called as crossover. To calculate the crossover value for generation,
crossover operator is used. The mating between individuals is represented by the crossover
operator. The cross over site is chosen randomly after the selection process of parent is
finished. To calculate the standard deviation values and the best value, we give the target
string and generation string. Thus, based on the values of the average fitness and standard
deviation, the best Fitness value is calculated.
Conclusion
By using c++ program, the genetic solver algorithm is successfully implemented. The
data file is imported by using c++ code and it analysis the generation value, best value and
fitness value. A data file contains 36 different variables on both the files. The class and
interface features are used for that algorithm. Thus, the successful implementation of the
Genetic Solver Algorithm is completed.
crossover strategy is based on crossover value for population. The Crossover strategy which
is connected to the Split crossover strategy is also connected to the genetic algorithm.
The changes in the Genetic sequence are caused due to the mutation of diversity among the
organisms. It has the various values and the biological system is capable of reproduction.
It is mainly used for calculating the next generation value and its effect on the hereditary
material. The probability of this mutation does not depend upon the influence of the
probability on this mutation.
For implementing the genetic algorithm, there are two types of mutations, synonymous
mutation and non-synonymous mutation. To calculate the good fitness value for that
algorithm, selection operator is used. It calculates the good fitness scores and that allow
passing the successive generation. To allow passing of the successive generations, it
calculates the good fitness scores. The exchange between two chromosomes of the genetic
and materials is called as crossover. To calculate the crossover value for generation,
crossover operator is used. The mating between individuals is represented by the crossover
operator. The cross over site is chosen randomly after the selection process of parent is
finished. To calculate the standard deviation values and the best value, we give the target
string and generation string. Thus, based on the values of the average fitness and standard
deviation, the best Fitness value is calculated.
Conclusion
By using c++ program, the genetic solver algorithm is successfully implemented. The
data file is imported by using c++ code and it analysis the generation value, best value and
fitness value. A data file contains 36 different variables on both the files. The class and
interface features are used for that algorithm. Thus, the successful implementation of the
Genetic Solver Algorithm is completed.
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
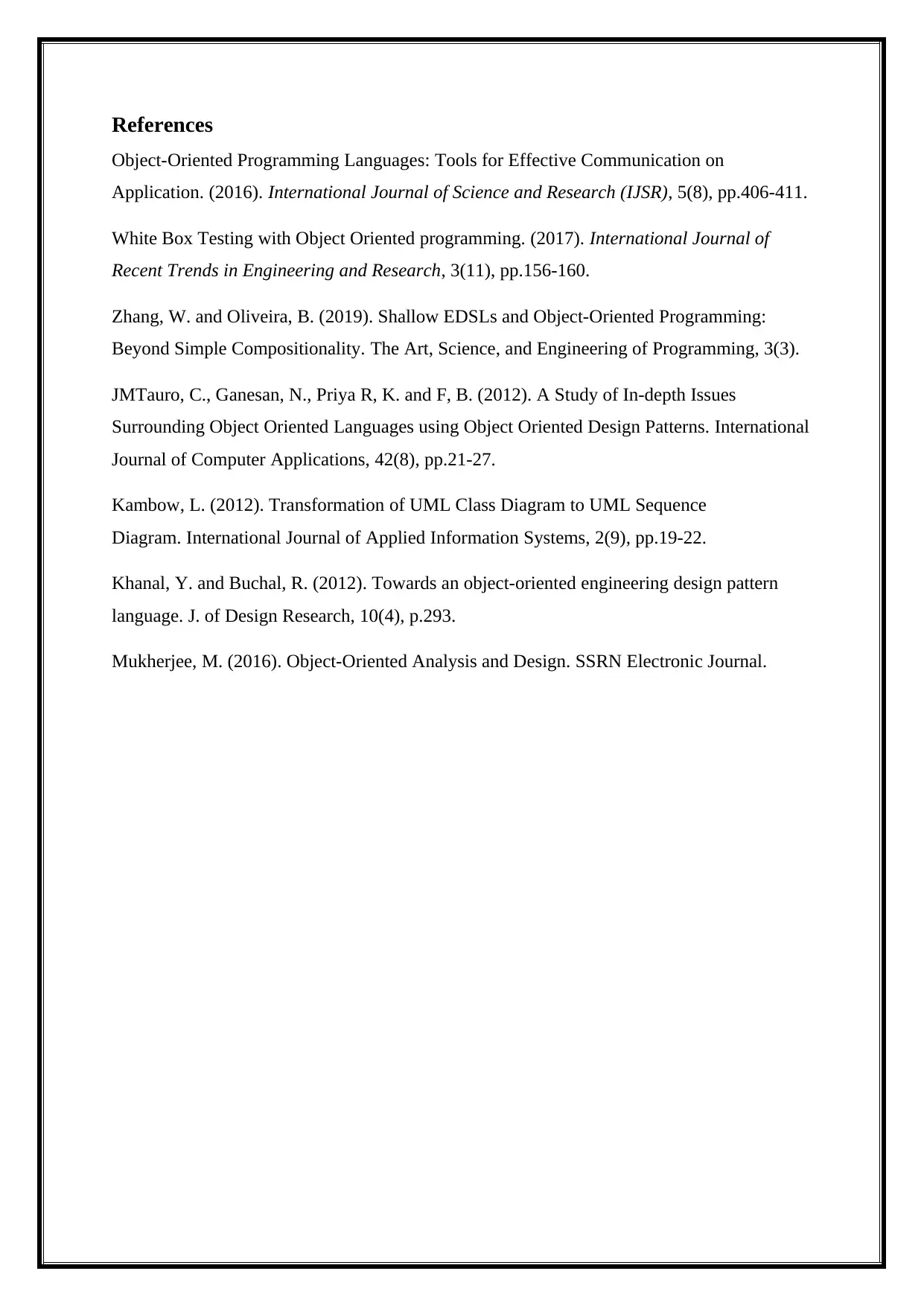
References
Object-Oriented Programming Languages: Tools for Effective Communication on
Application. (2016). International Journal of Science and Research (IJSR), 5(8), pp.406-411.
White Box Testing with Object Oriented programming. (2017). International Journal of
Recent Trends in Engineering and Research, 3(11), pp.156-160.
Zhang, W. and Oliveira, B. (2019). Shallow EDSLs and Object-Oriented Programming:
Beyond Simple Compositionality. The Art, Science, and Engineering of Programming, 3(3).
JMTauro, C., Ganesan, N., Priya R, K. and F, B. (2012). A Study of In-depth Issues
Surrounding Object Oriented Languages using Object Oriented Design Patterns. International
Journal of Computer Applications, 42(8), pp.21-27.
Kambow, L. (2012). Transformation of UML Class Diagram to UML Sequence
Diagram. International Journal of Applied Information Systems, 2(9), pp.19-22.
Khanal, Y. and Buchal, R. (2012). Towards an object-oriented engineering design pattern
language. J. of Design Research, 10(4), p.293.
Mukherjee, M. (2016). Object-Oriented Analysis and Design. SSRN Electronic Journal.
Object-Oriented Programming Languages: Tools for Effective Communication on
Application. (2016). International Journal of Science and Research (IJSR), 5(8), pp.406-411.
White Box Testing with Object Oriented programming. (2017). International Journal of
Recent Trends in Engineering and Research, 3(11), pp.156-160.
Zhang, W. and Oliveira, B. (2019). Shallow EDSLs and Object-Oriented Programming:
Beyond Simple Compositionality. The Art, Science, and Engineering of Programming, 3(3).
JMTauro, C., Ganesan, N., Priya R, K. and F, B. (2012). A Study of In-depth Issues
Surrounding Object Oriented Languages using Object Oriented Design Patterns. International
Journal of Computer Applications, 42(8), pp.21-27.
Kambow, L. (2012). Transformation of UML Class Diagram to UML Sequence
Diagram. International Journal of Applied Information Systems, 2(9), pp.19-22.
Khanal, Y. and Buchal, R. (2012). Towards an object-oriented engineering design pattern
language. J. of Design Research, 10(4), p.293.
Mukherjee, M. (2016). Object-Oriented Analysis and Design. SSRN Electronic Journal.
1 out of 8
Related Documents

Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.