C++ Programming: Week 03 Assignment Solutions for Beginners
VerifiedAdded on 2020/10/12
|11
|3656
|107
Homework Assignment
AI Summary
This document presents a comprehensive collection of C++ code solutions for a series of programming problems assigned in Week 03. The solutions cover a range of topics, including conditional statements (if/else), mathematical operations, and loop structures. Each problem is clearly defined with its corresponding C++ code, demonstrating various programming concepts such as input/output operations using `iostream`, mathematical functions from the `cmath` library, and the use of data types like `float`, `double`, and `int`. The solutions address problems involving comparisons of numerical values, calculating roots of quadratic equations, determining leap years, and calculating series sums. The code is well-commented, explaining the logic and purpose of each code segment. This resource serves as a valuable guide for students learning C++ programming, providing practical examples and insights into problem-solving techniques. The document showcases how to write basic programs to solve mathematical and logical problems using the C++ programming language.
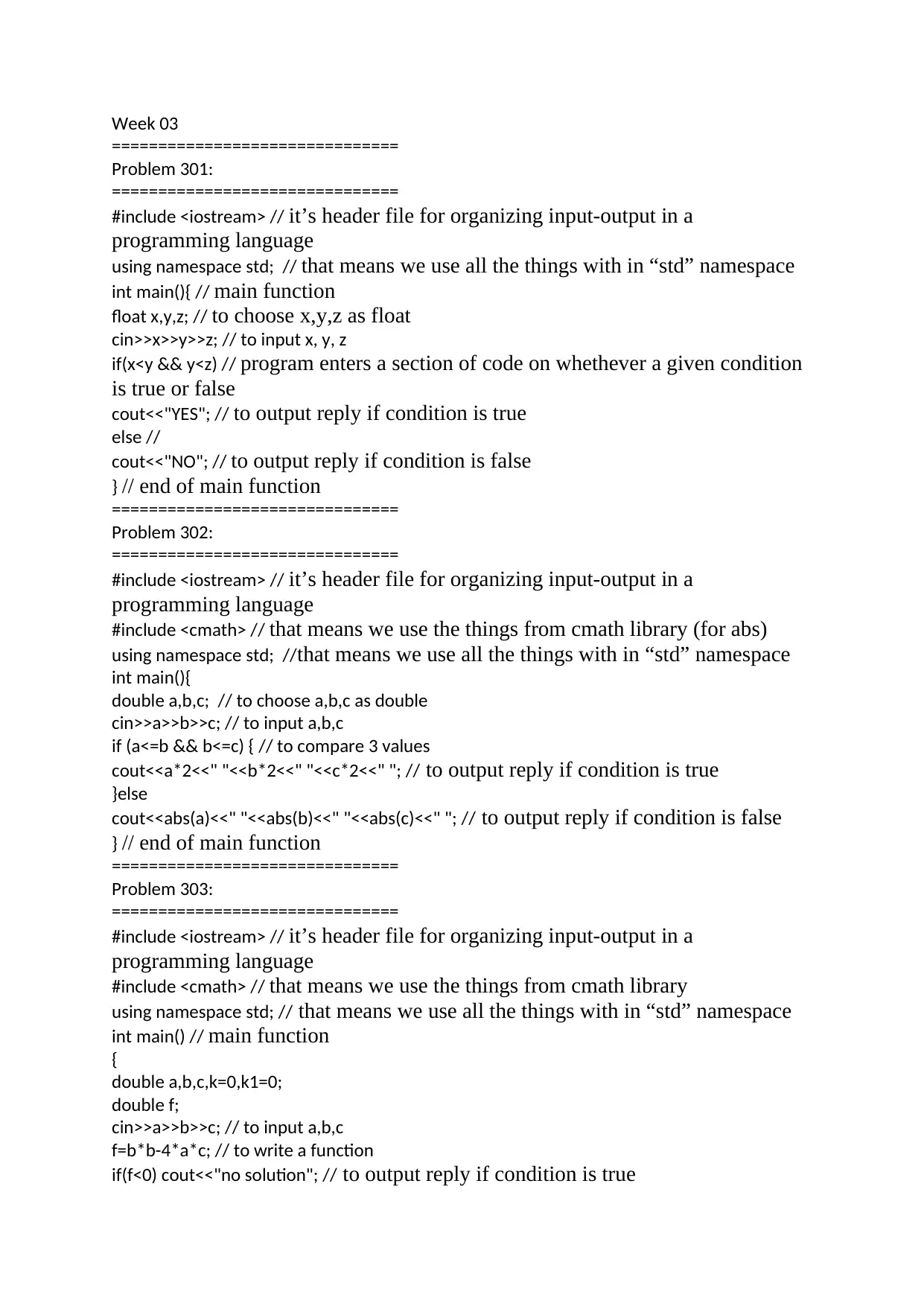
Week 03
===============================
Problem 301:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
using namespace std; // that means we use all the things with in “std” namespace
int main(){ // main function
float x,y,z; // to choose x,y,z as float
cin>>x>>y>>z; // to input x, y, z
if(x<y && y<z) // program enters a section of code on whethever a given condition
is true or false
cout<<"YES"; // to output reply if condition is true
else //
cout<<"NO"; // to output reply if condition is false
} // end of main function
===============================
Problem 302:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
#include <cmath> // that means we use the things from cmath library (for abs)
using namespace std; //that means we use all the things with in “std” namespace
int main(){
double a,b,c; // to choose a,b,c as double
cin>>a>>b>>c; // to input a,b,c
if (a<=b && b<=c) { // to compare 3 values
cout<<a*2<<" "<<b*2<<" "<<c*2<<" "; // to output reply if condition is true
}else
cout<<abs(a)<<" "<<abs(b)<<" "<<abs(c)<<" "; // to output reply if condition is false
} // end of main function
===============================
Problem 303:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
#include <cmath> // that means we use the things from cmath library
using namespace std; // that means we use all the things with in “std” namespace
int main() // main function
{
double a,b,c,k=0,k1=0;
double f;
cin>>a>>b>>c; // to input a,b,c
f=b*b-4*a*c; // to write a function
if(f<0) cout<<"no solution"; // to output reply if condition is true
===============================
Problem 301:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
using namespace std; // that means we use all the things with in “std” namespace
int main(){ // main function
float x,y,z; // to choose x,y,z as float
cin>>x>>y>>z; // to input x, y, z
if(x<y && y<z) // program enters a section of code on whethever a given condition
is true or false
cout<<"YES"; // to output reply if condition is true
else //
cout<<"NO"; // to output reply if condition is false
} // end of main function
===============================
Problem 302:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
#include <cmath> // that means we use the things from cmath library (for abs)
using namespace std; //that means we use all the things with in “std” namespace
int main(){
double a,b,c; // to choose a,b,c as double
cin>>a>>b>>c; // to input a,b,c
if (a<=b && b<=c) { // to compare 3 values
cout<<a*2<<" "<<b*2<<" "<<c*2<<" "; // to output reply if condition is true
}else
cout<<abs(a)<<" "<<abs(b)<<" "<<abs(c)<<" "; // to output reply if condition is false
} // end of main function
===============================
Problem 303:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
#include <cmath> // that means we use the things from cmath library
using namespace std; // that means we use all the things with in “std” namespace
int main() // main function
{
double a,b,c,k=0,k1=0;
double f;
cin>>a>>b>>c; // to input a,b,c
f=b*b-4*a*c; // to write a function
if(f<0) cout<<"no solution"; // to output reply if condition is true
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
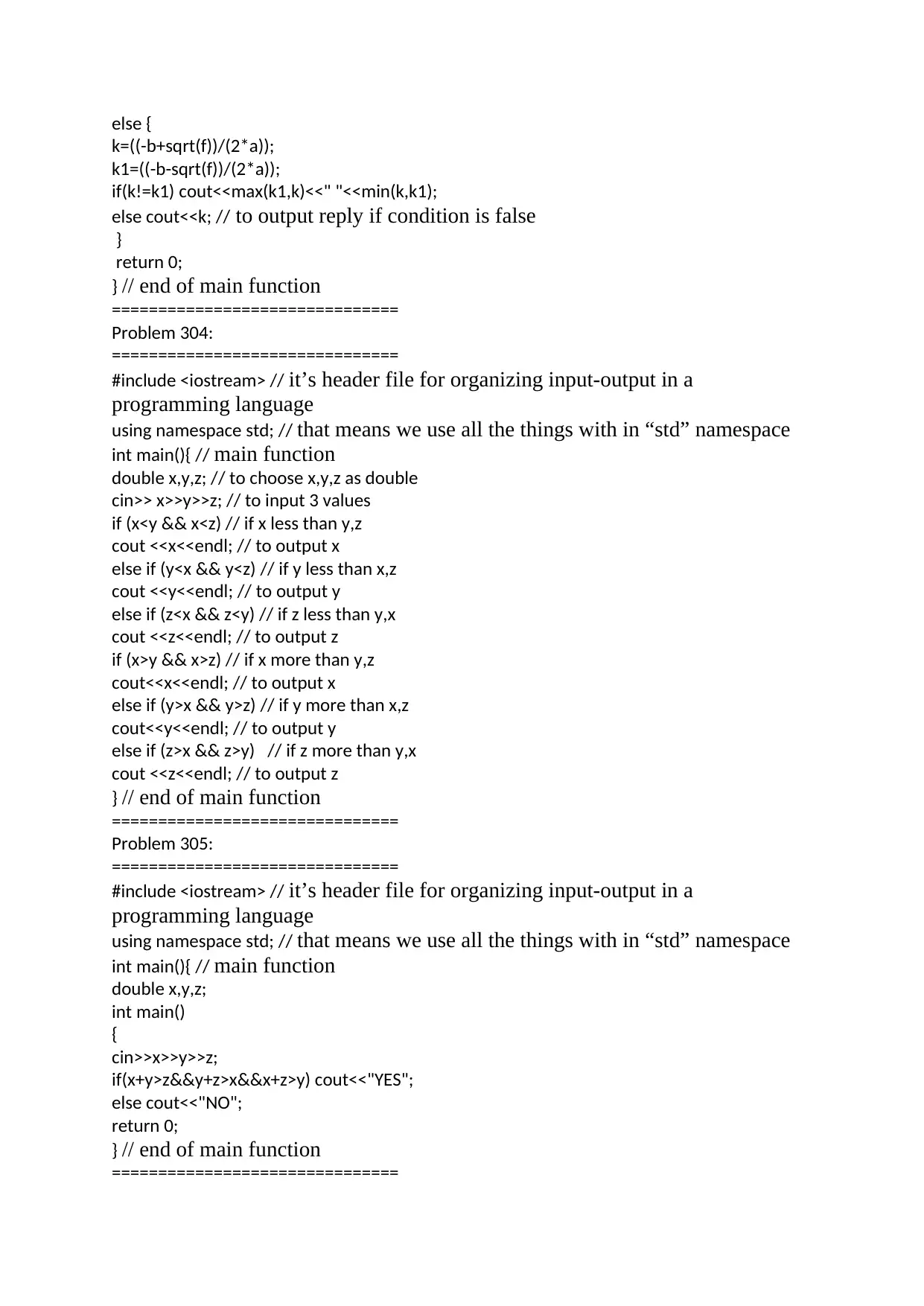
else {
k=((-b+sqrt(f))/(2*a));
k1=((-b-sqrt(f))/(2*a));
if(k!=k1) cout<<max(k1,k)<<" "<<min(k,k1);
else cout<<k; // to output reply if condition is false
}
return 0;
} // end of main function
===============================
Problem 304:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
using namespace std; // that means we use all the things with in “std” namespace
int main(){ // main function
double x,y,z; // to choose x,y,z as double
cin>> x>>y>>z; // to input 3 values
if (x<y && x<z) // if x less than y,z
cout <<x<<endl; // to output x
else if (y<x && y<z) // if y less than x,z
cout <<y<<endl; // to output y
else if (z<x && z<y) // if z less than y,x
cout <<z<<endl; // to output z
if (x>y && x>z) // if x more than y,z
cout<<x<<endl; // to output x
else if (y>x && y>z) // if y more than x,z
cout<<y<<endl; // to output y
else if (z>x && z>y) // if z more than y,x
cout <<z<<endl; // to output z
} // end of main function
===============================
Problem 305:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
using namespace std; // that means we use all the things with in “std” namespace
int main(){ // main function
double x,y,z;
int main()
{
cin>>x>>y>>z;
if(x+y>z&&y+z>x&&x+z>y) cout<<"YES";
else cout<<"NO";
return 0;
} // end of main function
===============================
k=((-b+sqrt(f))/(2*a));
k1=((-b-sqrt(f))/(2*a));
if(k!=k1) cout<<max(k1,k)<<" "<<min(k,k1);
else cout<<k; // to output reply if condition is false
}
return 0;
} // end of main function
===============================
Problem 304:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
using namespace std; // that means we use all the things with in “std” namespace
int main(){ // main function
double x,y,z; // to choose x,y,z as double
cin>> x>>y>>z; // to input 3 values
if (x<y && x<z) // if x less than y,z
cout <<x<<endl; // to output x
else if (y<x && y<z) // if y less than x,z
cout <<y<<endl; // to output y
else if (z<x && z<y) // if z less than y,x
cout <<z<<endl; // to output z
if (x>y && x>z) // if x more than y,z
cout<<x<<endl; // to output x
else if (y>x && y>z) // if y more than x,z
cout<<y<<endl; // to output y
else if (z>x && z>y) // if z more than y,x
cout <<z<<endl; // to output z
} // end of main function
===============================
Problem 305:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
using namespace std; // that means we use all the things with in “std” namespace
int main(){ // main function
double x,y,z;
int main()
{
cin>>x>>y>>z;
if(x+y>z&&y+z>x&&x+z>y) cout<<"YES";
else cout<<"NO";
return 0;
} // end of main function
===============================
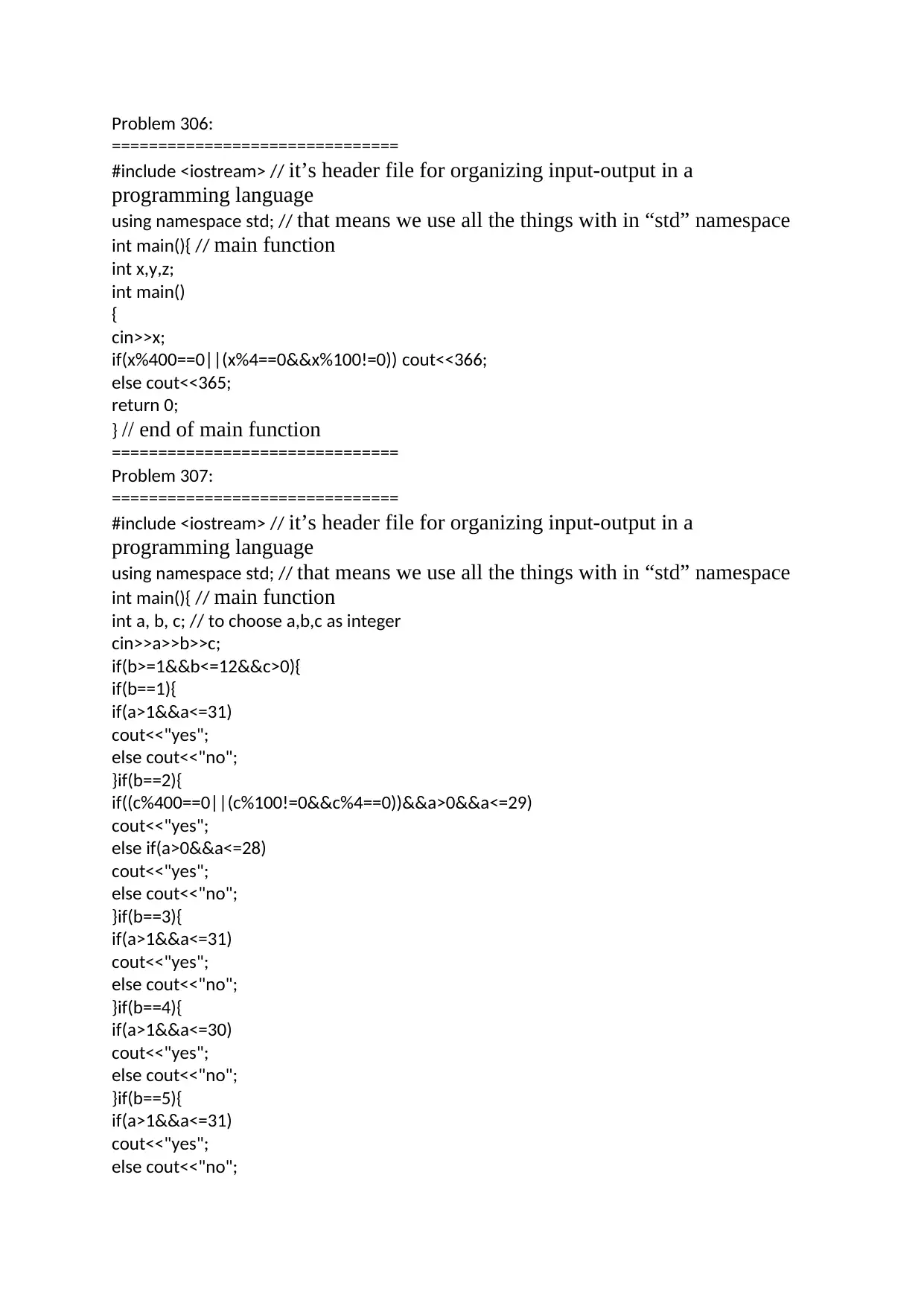
Problem 306:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
using namespace std; // that means we use all the things with in “std” namespace
int main(){ // main function
int x,y,z;
int main()
{
cin>>x;
if(x%400==0||(x%4==0&&x%100!=0)) cout<<366;
else cout<<365;
return 0;
} // end of main function
===============================
Problem 307:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
using namespace std; // that means we use all the things with in “std” namespace
int main(){ // main function
int a, b, c; // to choose a,b,c as integer
cin>>a>>b>>c;
if(b>=1&&b<=12&&c>0){
if(b==1){
if(a>1&&a<=31)
cout<<"yes";
else cout<<"no";
}if(b==2){
if((c%400==0||(c%100!=0&&c%4==0))&&a>0&&a<=29)
cout<<"yes";
else if(a>0&&a<=28)
cout<<"yes";
else cout<<"no";
}if(b==3){
if(a>1&&a<=31)
cout<<"yes";
else cout<<"no";
}if(b==4){
if(a>1&&a<=30)
cout<<"yes";
else cout<<"no";
}if(b==5){
if(a>1&&a<=31)
cout<<"yes";
else cout<<"no";
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
using namespace std; // that means we use all the things with in “std” namespace
int main(){ // main function
int x,y,z;
int main()
{
cin>>x;
if(x%400==0||(x%4==0&&x%100!=0)) cout<<366;
else cout<<365;
return 0;
} // end of main function
===============================
Problem 307:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
using namespace std; // that means we use all the things with in “std” namespace
int main(){ // main function
int a, b, c; // to choose a,b,c as integer
cin>>a>>b>>c;
if(b>=1&&b<=12&&c>0){
if(b==1){
if(a>1&&a<=31)
cout<<"yes";
else cout<<"no";
}if(b==2){
if((c%400==0||(c%100!=0&&c%4==0))&&a>0&&a<=29)
cout<<"yes";
else if(a>0&&a<=28)
cout<<"yes";
else cout<<"no";
}if(b==3){
if(a>1&&a<=31)
cout<<"yes";
else cout<<"no";
}if(b==4){
if(a>1&&a<=30)
cout<<"yes";
else cout<<"no";
}if(b==5){
if(a>1&&a<=31)
cout<<"yes";
else cout<<"no";
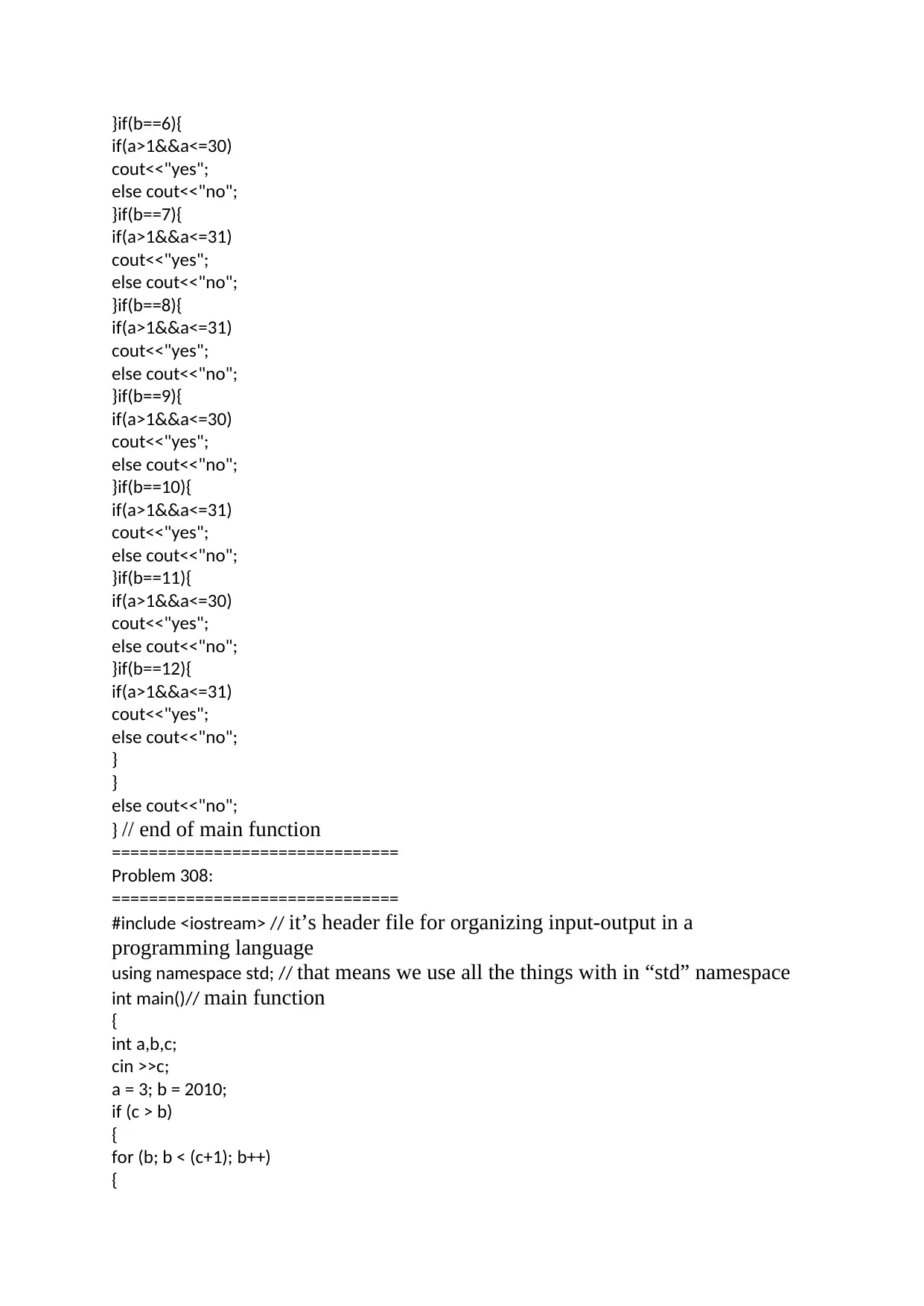
}if(b==6){
if(a>1&&a<=30)
cout<<"yes";
else cout<<"no";
}if(b==7){
if(a>1&&a<=31)
cout<<"yes";
else cout<<"no";
}if(b==8){
if(a>1&&a<=31)
cout<<"yes";
else cout<<"no";
}if(b==9){
if(a>1&&a<=30)
cout<<"yes";
else cout<<"no";
}if(b==10){
if(a>1&&a<=31)
cout<<"yes";
else cout<<"no";
}if(b==11){
if(a>1&&a<=30)
cout<<"yes";
else cout<<"no";
}if(b==12){
if(a>1&&a<=31)
cout<<"yes";
else cout<<"no";
}
}
else cout<<"no";
} // end of main function
===============================
Problem 308:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
using namespace std; // that means we use all the things with in “std” namespace
int main()// main function
{
int a,b,c;
cin >>c;
a = 3; b = 2010;
if (c > b)
{
for (b; b < (c+1); b++)
{
if(a>1&&a<=30)
cout<<"yes";
else cout<<"no";
}if(b==7){
if(a>1&&a<=31)
cout<<"yes";
else cout<<"no";
}if(b==8){
if(a>1&&a<=31)
cout<<"yes";
else cout<<"no";
}if(b==9){
if(a>1&&a<=30)
cout<<"yes";
else cout<<"no";
}if(b==10){
if(a>1&&a<=31)
cout<<"yes";
else cout<<"no";
}if(b==11){
if(a>1&&a<=30)
cout<<"yes";
else cout<<"no";
}if(b==12){
if(a>1&&a<=31)
cout<<"yes";
else cout<<"no";
}
}
else cout<<"no";
} // end of main function
===============================
Problem 308:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
using namespace std; // that means we use all the things with in “std” namespace
int main()// main function
{
int a,b,c;
cin >>c;
a = 3; b = 2010;
if (c > b)
{
for (b; b < (c+1); b++)
{
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
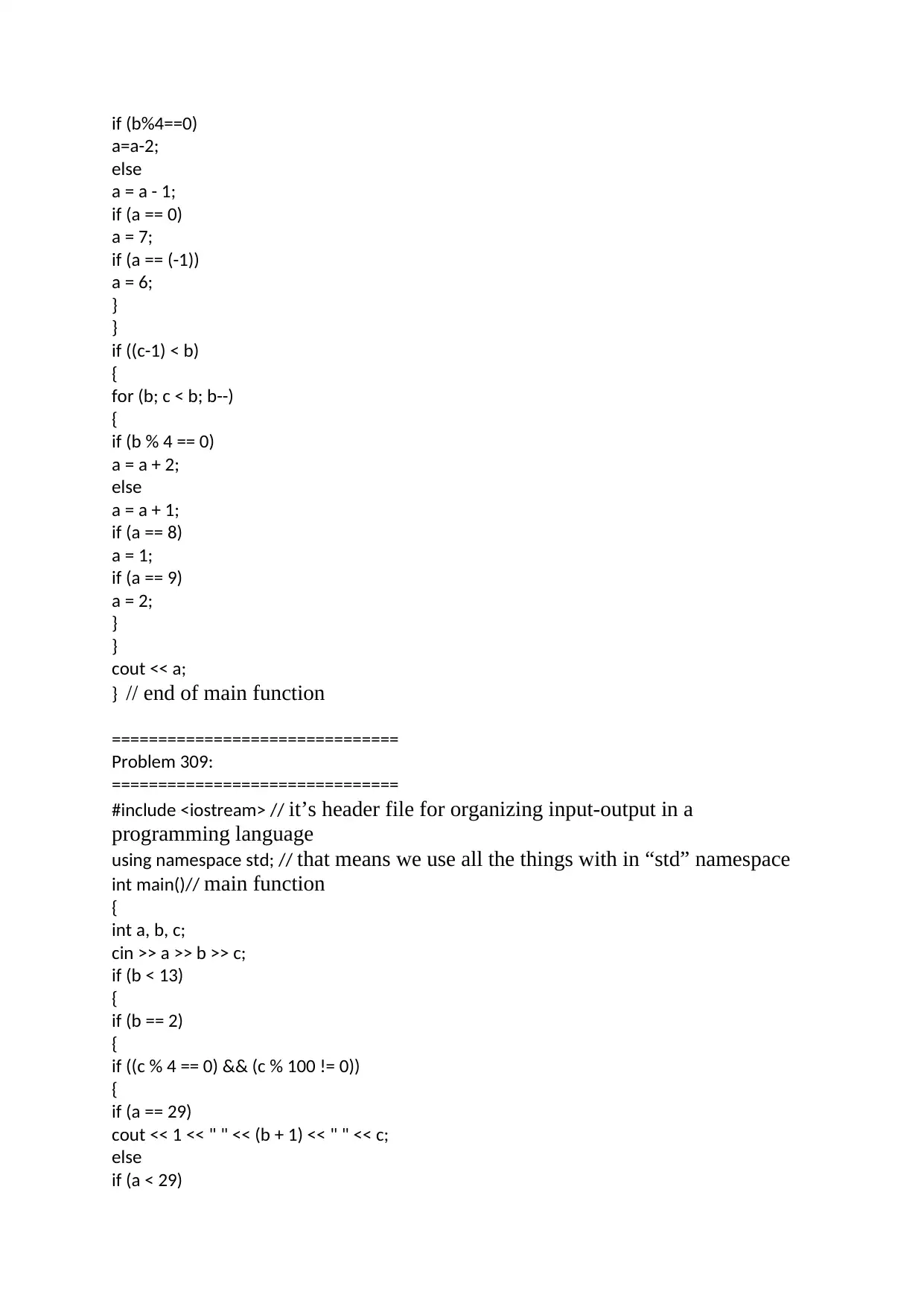
if (b%4==0)
a=a-2;
else
a = a - 1;
if (a == 0)
a = 7;
if (a == (-1))
a = 6;
}
}
if ((c-1) < b)
{
for (b; c < b; b--)
{
if (b % 4 == 0)
a = a + 2;
else
a = a + 1;
if (a == 8)
a = 1;
if (a == 9)
a = 2;
}
}
cout << a;
} // end of main function
===============================
Problem 309:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
using namespace std; // that means we use all the things with in “std” namespace
int main()// main function
{
int a, b, c;
cin >> a >> b >> c;
if (b < 13)
{
if (b == 2)
{
if ((c % 4 == 0) && (c % 100 != 0))
{
if (a == 29)
cout << 1 << " " << (b + 1) << " " << c;
else
if (a < 29)
a=a-2;
else
a = a - 1;
if (a == 0)
a = 7;
if (a == (-1))
a = 6;
}
}
if ((c-1) < b)
{
for (b; c < b; b--)
{
if (b % 4 == 0)
a = a + 2;
else
a = a + 1;
if (a == 8)
a = 1;
if (a == 9)
a = 2;
}
}
cout << a;
} // end of main function
===============================
Problem 309:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
using namespace std; // that means we use all the things with in “std” namespace
int main()// main function
{
int a, b, c;
cin >> a >> b >> c;
if (b < 13)
{
if (b == 2)
{
if ((c % 4 == 0) && (c % 100 != 0))
{
if (a == 29)
cout << 1 << " " << (b + 1) << " " << c;
else
if (a < 29)
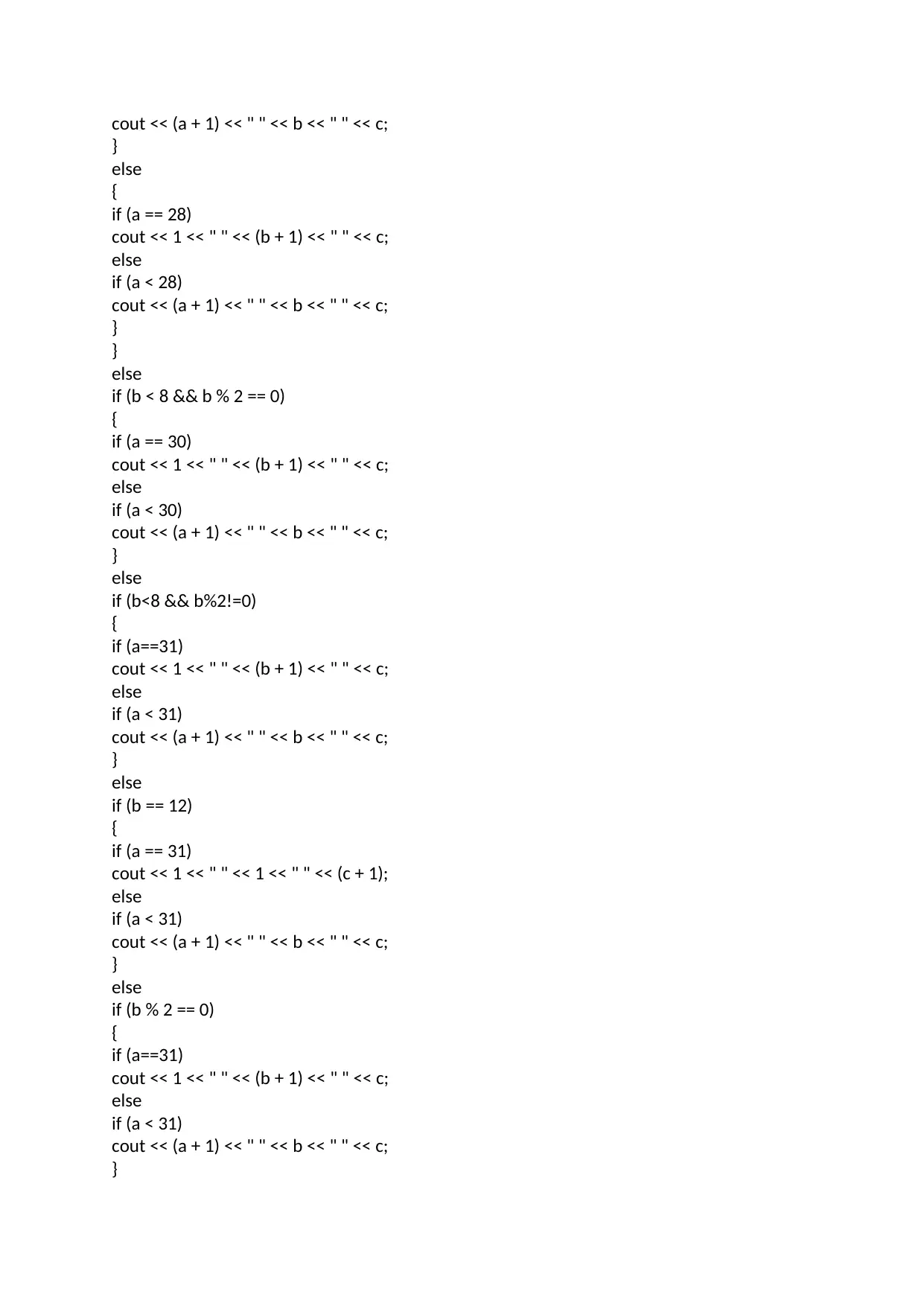
cout << (a + 1) << " " << b << " " << c;
}
else
{
if (a == 28)
cout << 1 << " " << (b + 1) << " " << c;
else
if (a < 28)
cout << (a + 1) << " " << b << " " << c;
}
}
else
if (b < 8 && b % 2 == 0)
{
if (a == 30)
cout << 1 << " " << (b + 1) << " " << c;
else
if (a < 30)
cout << (a + 1) << " " << b << " " << c;
}
else
if (b<8 && b%2!=0)
{
if (a==31)
cout << 1 << " " << (b + 1) << " " << c;
else
if (a < 31)
cout << (a + 1) << " " << b << " " << c;
}
else
if (b == 12)
{
if (a == 31)
cout << 1 << " " << 1 << " " << (c + 1);
else
if (a < 31)
cout << (a + 1) << " " << b << " " << c;
}
else
if (b % 2 == 0)
{
if (a==31)
cout << 1 << " " << (b + 1) << " " << c;
else
if (a < 31)
cout << (a + 1) << " " << b << " " << c;
}
}
else
{
if (a == 28)
cout << 1 << " " << (b + 1) << " " << c;
else
if (a < 28)
cout << (a + 1) << " " << b << " " << c;
}
}
else
if (b < 8 && b % 2 == 0)
{
if (a == 30)
cout << 1 << " " << (b + 1) << " " << c;
else
if (a < 30)
cout << (a + 1) << " " << b << " " << c;
}
else
if (b<8 && b%2!=0)
{
if (a==31)
cout << 1 << " " << (b + 1) << " " << c;
else
if (a < 31)
cout << (a + 1) << " " << b << " " << c;
}
else
if (b == 12)
{
if (a == 31)
cout << 1 << " " << 1 << " " << (c + 1);
else
if (a < 31)
cout << (a + 1) << " " << b << " " << c;
}
else
if (b % 2 == 0)
{
if (a==31)
cout << 1 << " " << (b + 1) << " " << c;
else
if (a < 31)
cout << (a + 1) << " " << b << " " << c;
}
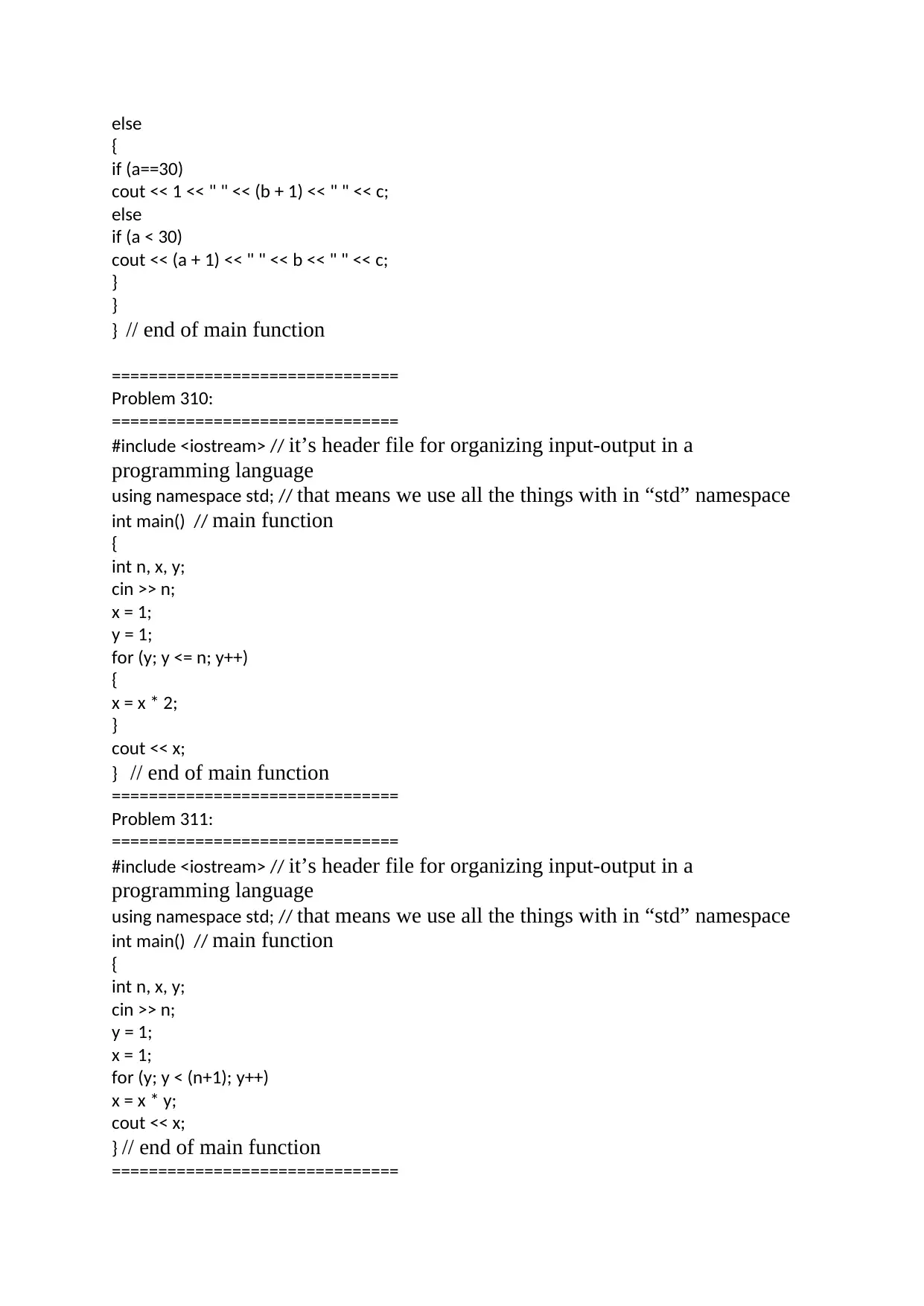
else
{
if (a==30)
cout << 1 << " " << (b + 1) << " " << c;
else
if (a < 30)
cout << (a + 1) << " " << b << " " << c;
}
}
} // end of main function
===============================
Problem 310:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
using namespace std; // that means we use all the things with in “std” namespace
int main() // main function
{
int n, x, y;
cin >> n;
x = 1;
y = 1;
for (y; y <= n; y++)
{
x = x * 2;
}
cout << x;
} // end of main function
===============================
Problem 311:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
using namespace std; // that means we use all the things with in “std” namespace
int main() // main function
{
int n, x, y;
cin >> n;
y = 1;
x = 1;
for (y; y < (n+1); y++)
x = x * y;
cout << x;
} // end of main function
===============================
{
if (a==30)
cout << 1 << " " << (b + 1) << " " << c;
else
if (a < 30)
cout << (a + 1) << " " << b << " " << c;
}
}
} // end of main function
===============================
Problem 310:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
using namespace std; // that means we use all the things with in “std” namespace
int main() // main function
{
int n, x, y;
cin >> n;
x = 1;
y = 1;
for (y; y <= n; y++)
{
x = x * 2;
}
cout << x;
} // end of main function
===============================
Problem 311:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
using namespace std; // that means we use all the things with in “std” namespace
int main() // main function
{
int n, x, y;
cin >> n;
y = 1;
x = 1;
for (y; y < (n+1); y++)
x = x * y;
cout << x;
} // end of main function
===============================
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
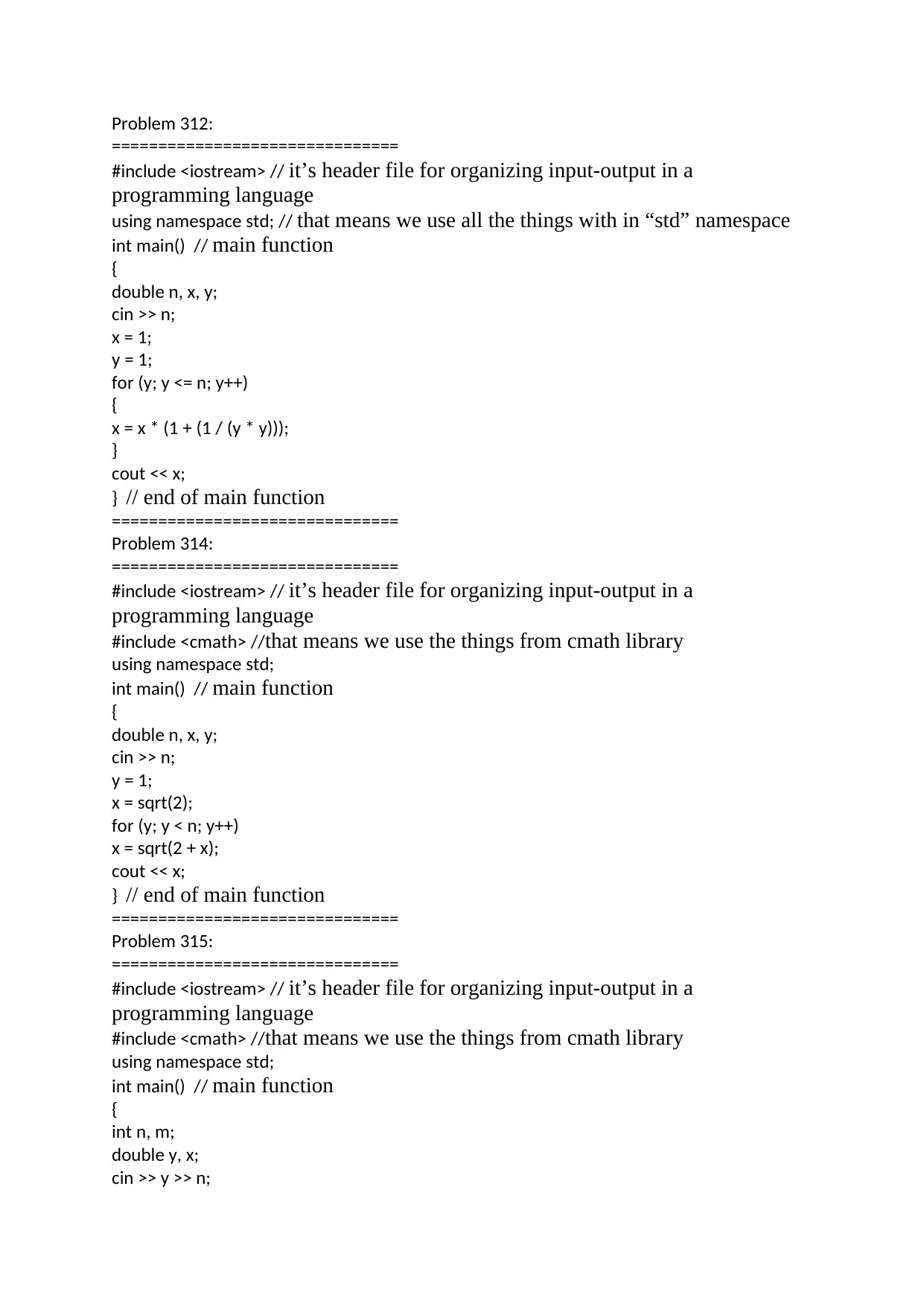
Problem 312:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
using namespace std; // that means we use all the things with in “std” namespace
int main() // main function
{
double n, x, y;
cin >> n;
x = 1;
y = 1;
for (y; y <= n; y++)
{
x = x * (1 + (1 / (y * y)));
}
cout << x;
} // end of main function
===============================
Problem 314:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
#include <cmath> //that means we use the things from cmath library
using namespace std;
int main() // main function
{
double n, x, y;
cin >> n;
y = 1;
x = sqrt(2);
for (y; y < n; y++)
x = sqrt(2 + x);
cout << x;
} // end of main function
===============================
Problem 315:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
#include <cmath> //that means we use the things from cmath library
using namespace std;
int main() // main function
{
int n, m;
double y, x;
cin >> y >> n;
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
using namespace std; // that means we use all the things with in “std” namespace
int main() // main function
{
double n, x, y;
cin >> n;
x = 1;
y = 1;
for (y; y <= n; y++)
{
x = x * (1 + (1 / (y * y)));
}
cout << x;
} // end of main function
===============================
Problem 314:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
#include <cmath> //that means we use the things from cmath library
using namespace std;
int main() // main function
{
double n, x, y;
cin >> n;
y = 1;
x = sqrt(2);
for (y; y < n; y++)
x = sqrt(2 + x);
cout << x;
} // end of main function
===============================
Problem 315:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
#include <cmath> //that means we use the things from cmath library
using namespace std;
int main() // main function
{
int n, m;
double y, x;
cin >> y >> n;
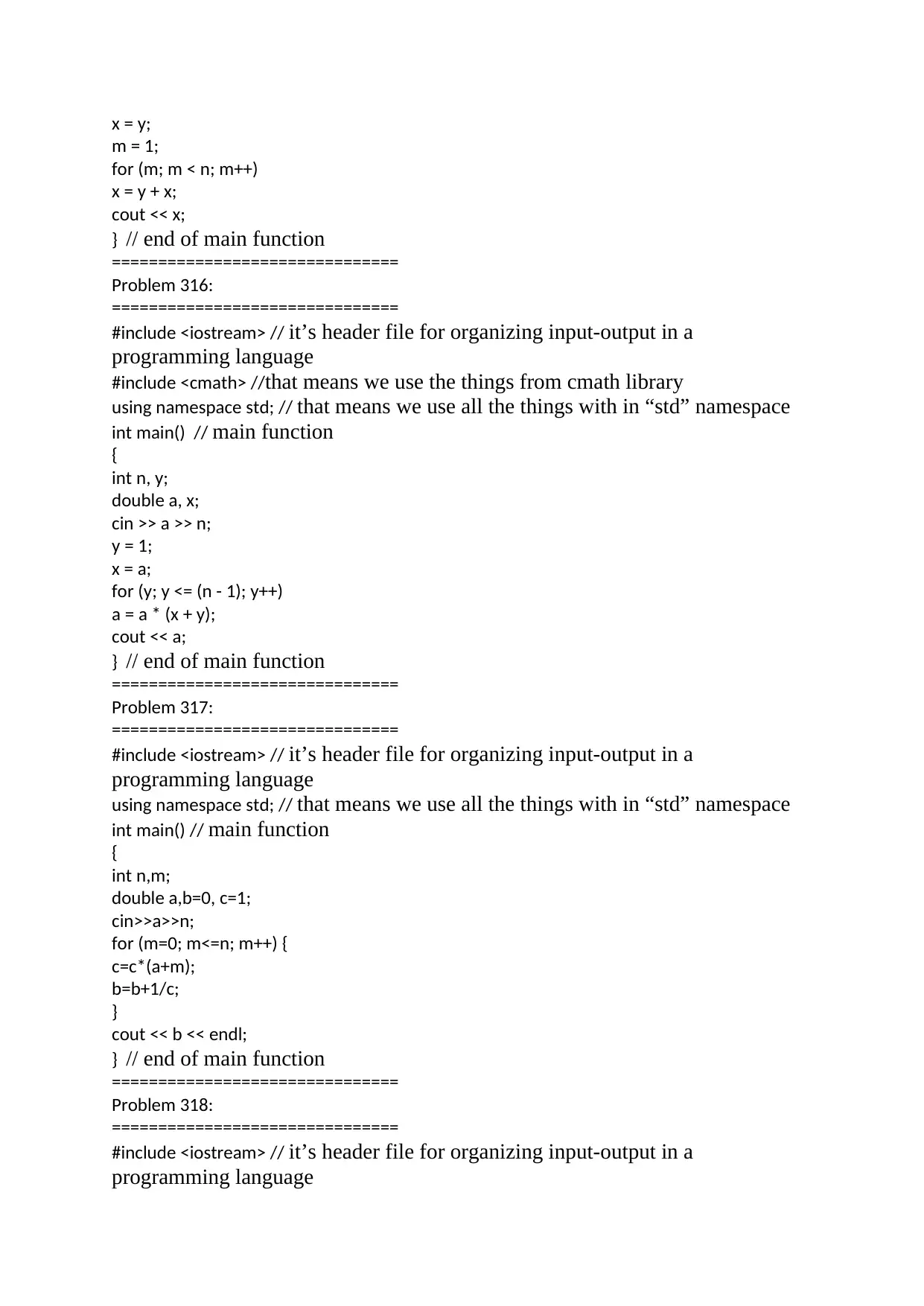
x = y;
m = 1;
for (m; m < n; m++)
x = y + x;
cout << x;
} // end of main function
===============================
Problem 316:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
#include <cmath> //that means we use the things from cmath library
using namespace std; // that means we use all the things with in “std” namespace
int main() // main function
{
int n, y;
double a, x;
cin >> a >> n;
y = 1;
x = a;
for (y; y <= (n - 1); y++)
a = a * (x + y);
cout << a;
} // end of main function
===============================
Problem 317:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
using namespace std; // that means we use all the things with in “std” namespace
int main() // main function
{
int n,m;
double a,b=0, c=1;
cin>>a>>n;
for (m=0; m<=n; m++) {
c=c*(a+m);
b=b+1/c;
}
cout << b << endl;
} // end of main function
===============================
Problem 318:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
m = 1;
for (m; m < n; m++)
x = y + x;
cout << x;
} // end of main function
===============================
Problem 316:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
#include <cmath> //that means we use the things from cmath library
using namespace std; // that means we use all the things with in “std” namespace
int main() // main function
{
int n, y;
double a, x;
cin >> a >> n;
y = 1;
x = a;
for (y; y <= (n - 1); y++)
a = a * (x + y);
cout << a;
} // end of main function
===============================
Problem 317:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
using namespace std; // that means we use all the things with in “std” namespace
int main() // main function
{
int n,m;
double a,b=0, c=1;
cin>>a>>n;
for (m=0; m<=n; m++) {
c=c*(a+m);
b=b+1/c;
}
cout << b << endl;
} // end of main function
===============================
Problem 318:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
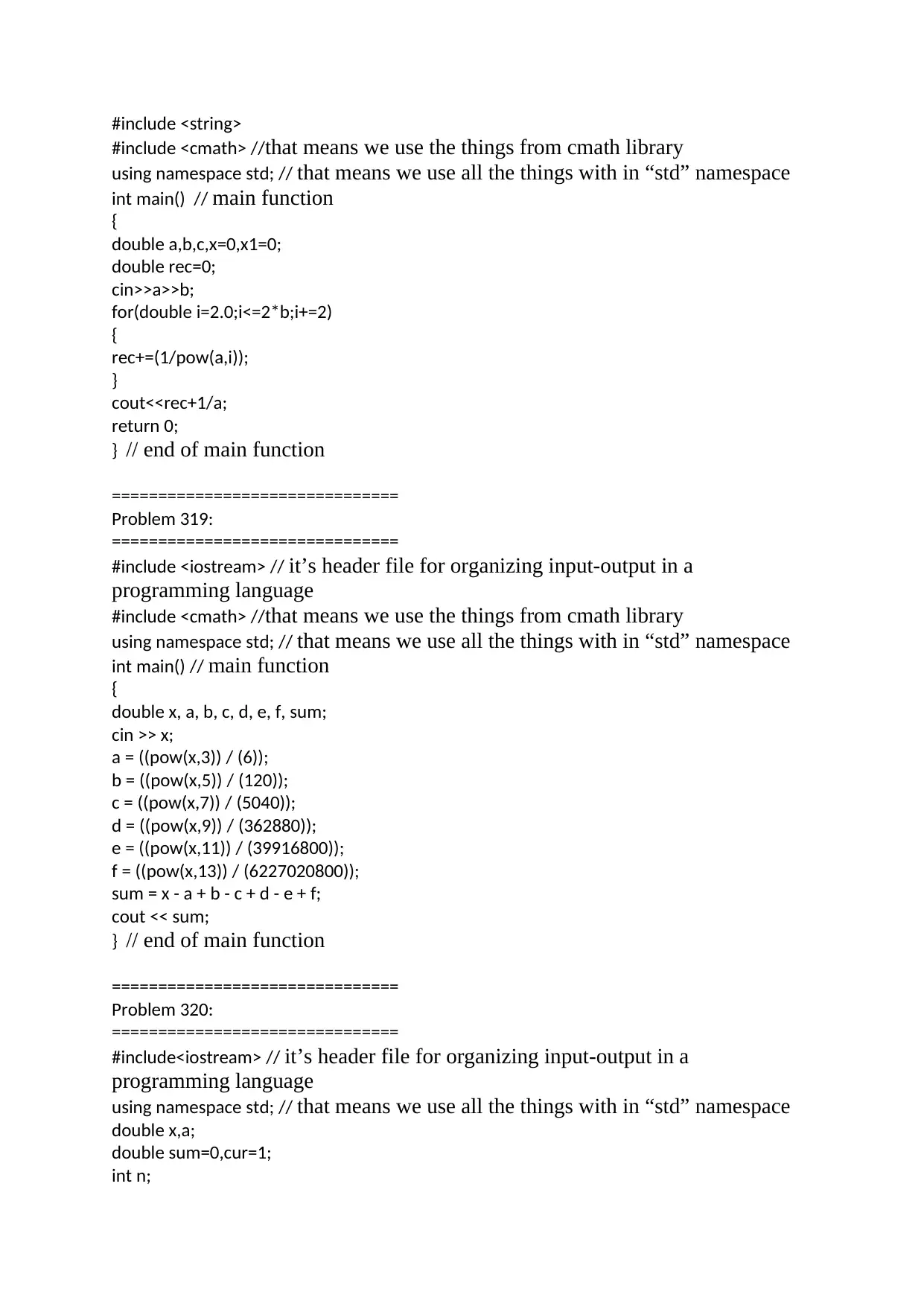
#include <string>
#include <cmath> //that means we use the things from cmath library
using namespace std; // that means we use all the things with in “std” namespace
int main() // main function
{
double a,b,c,x=0,x1=0;
double rec=0;
cin>>a>>b;
for(double i=2.0;i<=2*b;i+=2)
{
rec+=(1/pow(a,i));
}
cout<<rec+1/a;
return 0;
} // end of main function
===============================
Problem 319:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
#include <cmath> //that means we use the things from cmath library
using namespace std; // that means we use all the things with in “std” namespace
int main() // main function
{
double x, a, b, c, d, e, f, sum;
cin >> x;
a = ((pow(x,3)) / (6));
b = ((pow(x,5)) / (120));
c = ((pow(x,7)) / (5040));
d = ((pow(x,9)) / (362880));
e = ((pow(x,11)) / (39916800));
f = ((pow(x,13)) / (6227020800));
sum = x - a + b - c + d - e + f;
cout << sum;
} // end of main function
===============================
Problem 320:
===============================
#include<iostream> // it’s header file for organizing input-output in a
programming language
using namespace std; // that means we use all the things with in “std” namespace
double x,a;
double sum=0,cur=1;
int n;
#include <cmath> //that means we use the things from cmath library
using namespace std; // that means we use all the things with in “std” namespace
int main() // main function
{
double a,b,c,x=0,x1=0;
double rec=0;
cin>>a>>b;
for(double i=2.0;i<=2*b;i+=2)
{
rec+=(1/pow(a,i));
}
cout<<rec+1/a;
return 0;
} // end of main function
===============================
Problem 319:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
#include <cmath> //that means we use the things from cmath library
using namespace std; // that means we use all the things with in “std” namespace
int main() // main function
{
double x, a, b, c, d, e, f, sum;
cin >> x;
a = ((pow(x,3)) / (6));
b = ((pow(x,5)) / (120));
c = ((pow(x,7)) / (5040));
d = ((pow(x,9)) / (362880));
e = ((pow(x,11)) / (39916800));
f = ((pow(x,13)) / (6227020800));
sum = x - a + b - c + d - e + f;
cout << sum;
} // end of main function
===============================
Problem 320:
===============================
#include<iostream> // it’s header file for organizing input-output in a
programming language
using namespace std; // that means we use all the things with in “std” namespace
double x,a;
double sum=0,cur=1;
int n;
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
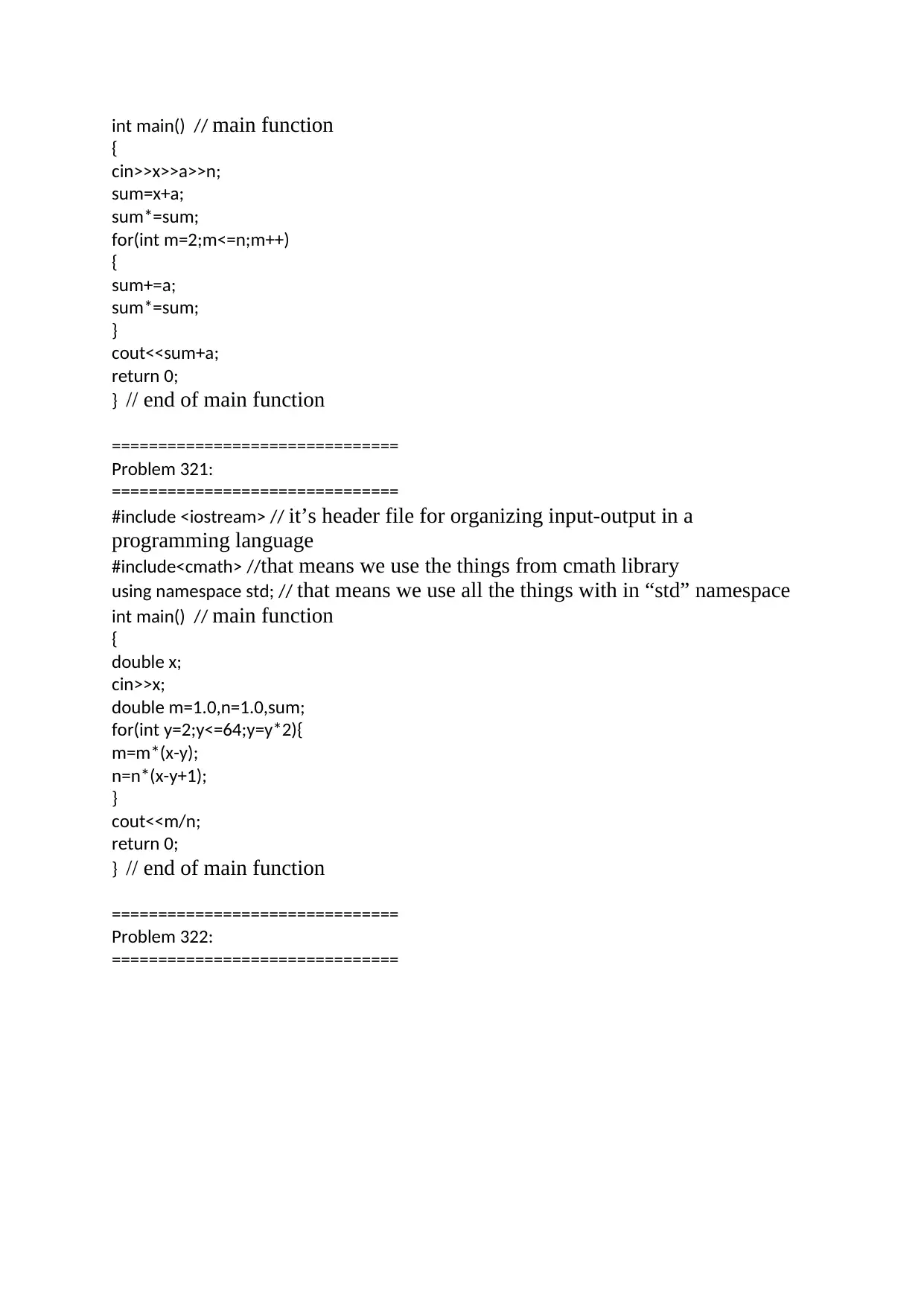
int main() // main function
{
cin>>x>>a>>n;
sum=x+a;
sum*=sum;
for(int m=2;m<=n;m++)
{
sum+=a;
sum*=sum;
}
cout<<sum+a;
return 0;
} // end of main function
===============================
Problem 321:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
#include<cmath> //that means we use the things from cmath library
using namespace std; // that means we use all the things with in “std” namespace
int main() // main function
{
double x;
cin>>x;
double m=1.0,n=1.0,sum;
for(int y=2;y<=64;y=y*2){
m=m*(x-y);
n=n*(x-y+1);
}
cout<<m/n;
return 0;
} // end of main function
===============================
Problem 322:
===============================
{
cin>>x>>a>>n;
sum=x+a;
sum*=sum;
for(int m=2;m<=n;m++)
{
sum+=a;
sum*=sum;
}
cout<<sum+a;
return 0;
} // end of main function
===============================
Problem 321:
===============================
#include <iostream> // it’s header file for organizing input-output in a
programming language
#include<cmath> //that means we use the things from cmath library
using namespace std; // that means we use all the things with in “std” namespace
int main() // main function
{
double x;
cin>>x;
double m=1.0,n=1.0,sum;
for(int y=2;y<=64;y=y*2){
m=m*(x-y);
n=n*(x-y+1);
}
cout<<m/n;
return 0;
} // end of main function
===============================
Problem 322:
===============================
1 out of 11
Related Documents

Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.