BTEC Level 5 HND Computing Unit 20: Design Patterns Report Analysis
VerifiedAdded on 2022/02/17
|26
|4542
|15
Report
AI Summary
This report is a comprehensive analysis of design patterns, focusing on their application in advanced programming. It begins with an introduction to design patterns, their importance, and the three main categories: creational, structural, and behavioral. The report then delves into the practical application of the Builder pattern through a case study of a fast-food ordering system, including UML class diagrams and code implementation in C#. The report further explores and compares other design patterns, such as Factory Method and Prototype, evaluating their suitability against the chosen scenario and justifying the selection of the Builder pattern. The document includes detailed diagrams, code snippets, and a comparative table to illustrate the implementation and outcomes. This report is a valuable resource for students studying design patterns and software development, providing insights into their practical application and comparative analysis.
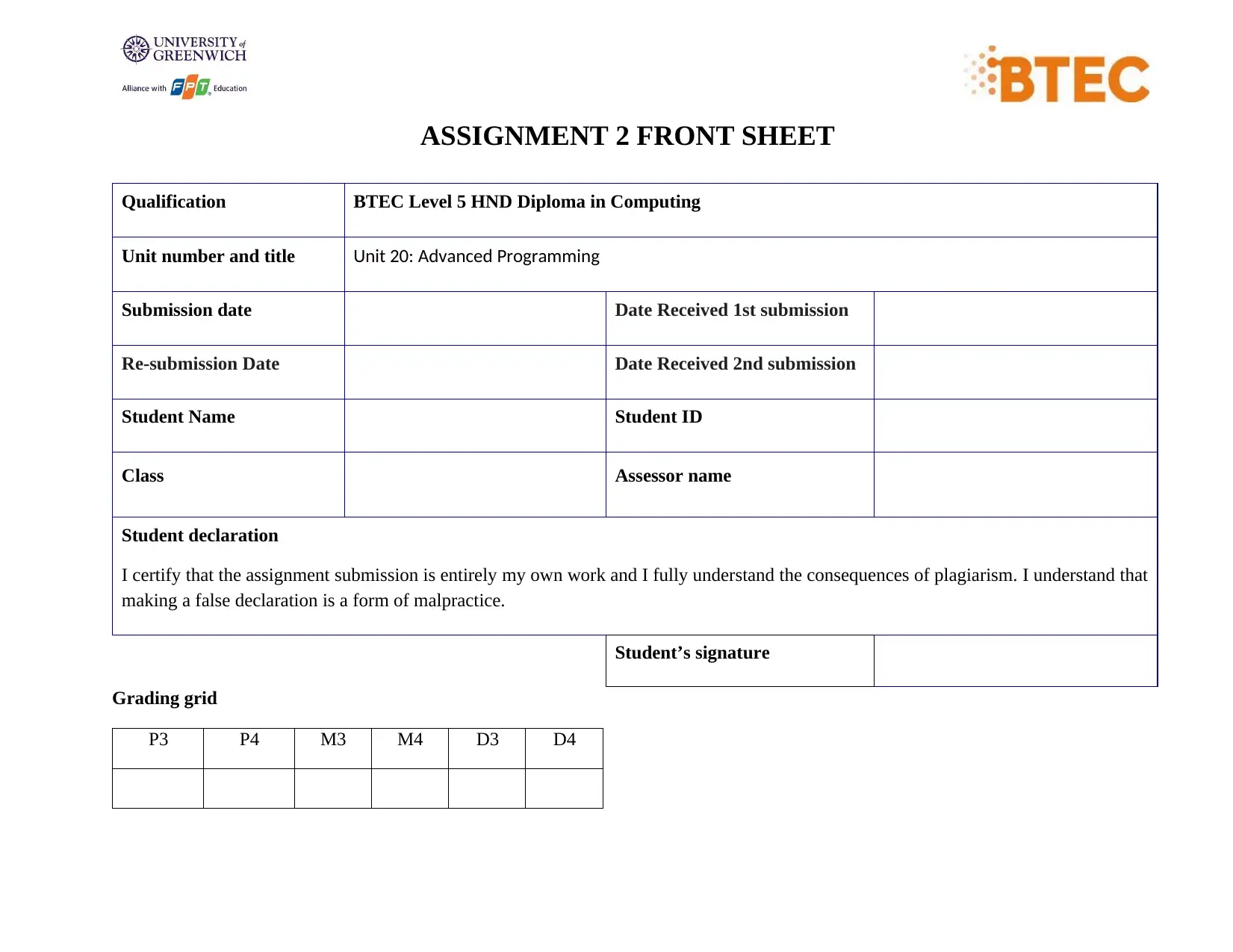
ASSIGNMENT 2 FRONT SHEET
Qualification BTEC Level 5 HND Diploma in Computing
Unit number and title Unit 20: Advanced Programming
Submission date Date Received 1st submission
Re-submission Date Date Received 2nd submission
Student Name Student ID
Class Assessor name
Student declaration
I certify that the assignment submission is entirely my own work and I fully understand the consequences of plagiarism. I understand that
making a false declaration is a form of malpractice.
Student’s signature
Grading grid
P3 P4 M3 M4 D3 D4
Qualification BTEC Level 5 HND Diploma in Computing
Unit number and title Unit 20: Advanced Programming
Submission date Date Received 1st submission
Re-submission Date Date Received 2nd submission
Student Name Student ID
Class Assessor name
Student declaration
I certify that the assignment submission is entirely my own work and I fully understand the consequences of plagiarism. I understand that
making a false declaration is a form of malpractice.
Student’s signature
Grading grid
P3 P4 M3 M4 D3 D4
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
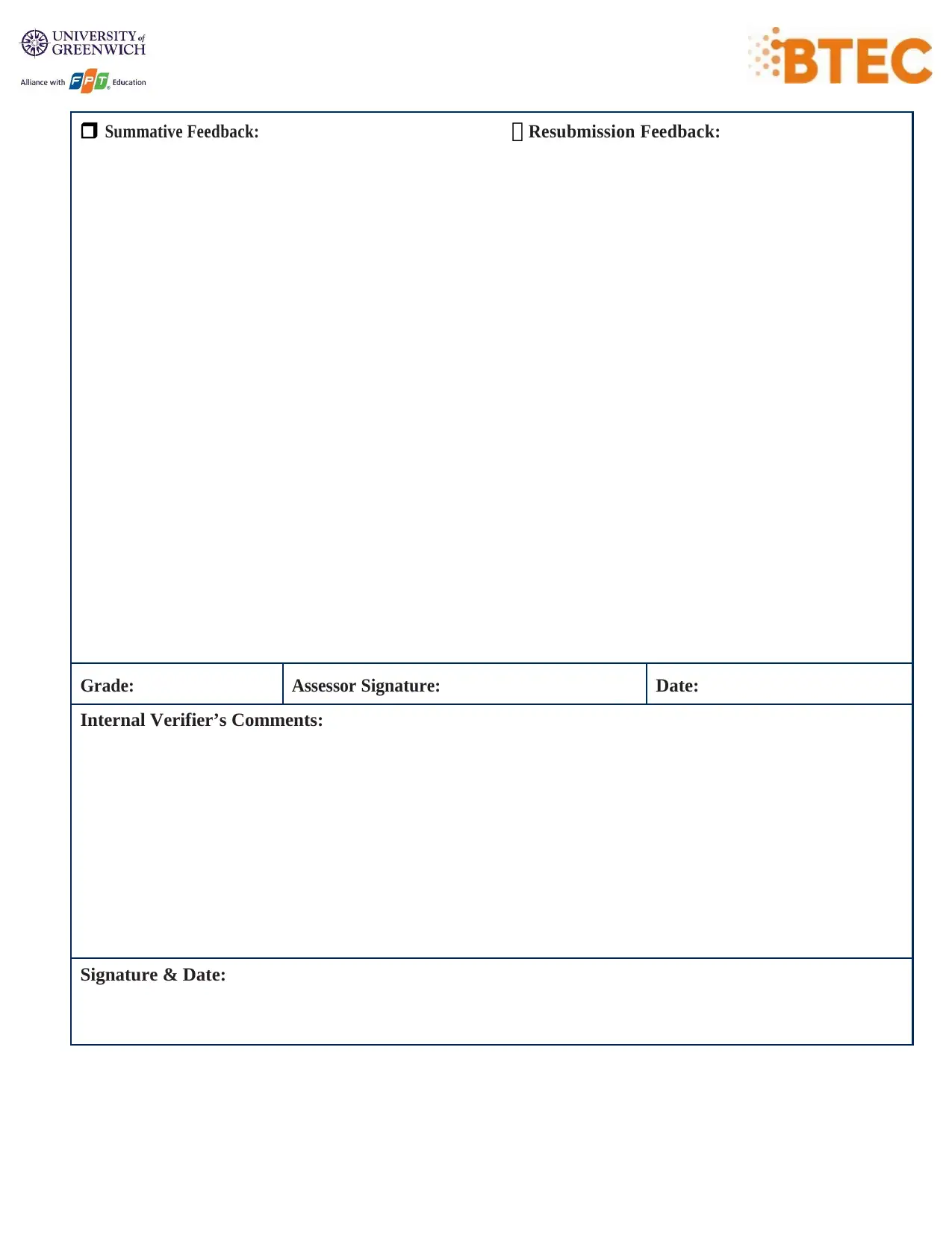
Summative Feedback: Resubmission Feedback:
Grade: Assessor Signature: Date:
Internal Verifier’s Comments:
Signature & Date:
Grade: Assessor Signature: Date:
Internal Verifier’s Comments:
Signature & Date:
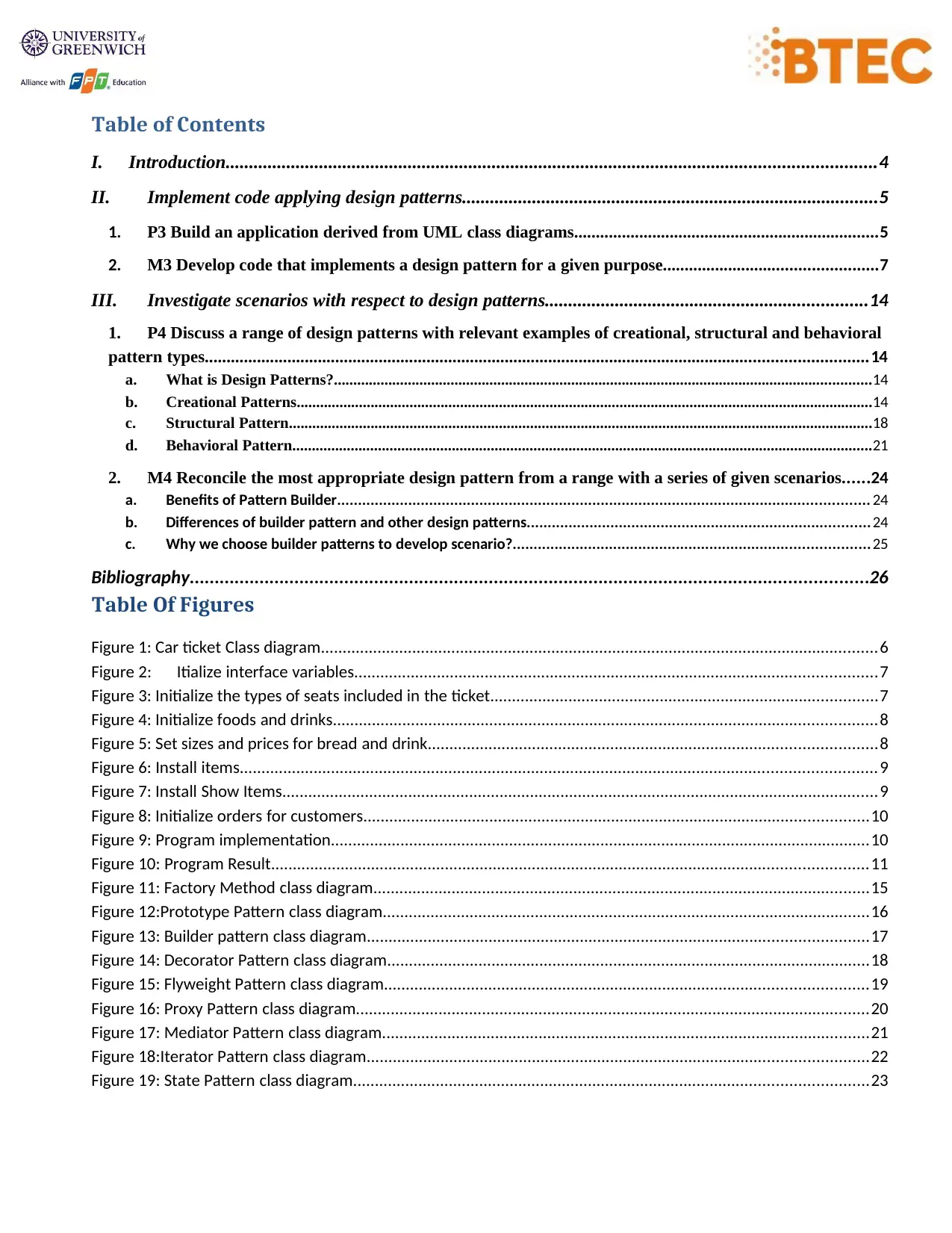
Table of Contents
I. Introduction...........................................................................................................................................4
II. Implement code applying design patterns.........................................................................................5
1. P3 Build an application derived from UML class diagrams......................................................................5
2. M3 Develop code that implements a design pattern for a given purpose.................................................7
III. Investigate scenarios with respect to design patterns.....................................................................14
1. P4 Discuss a range of design patterns with relevant examples of creational, structural and behavioral
pattern types........................................................................................................................................................14
a. What is Design Patterns?..........................................................................................................................................14
b. Creational Patterns....................................................................................................................................................14
c. Structural Pattern......................................................................................................................................................18
d. Behavioral Pattern.....................................................................................................................................................21
2. M4 Reconcile the most appropriate design pattern from a range with a series of given scenarios......24
a. Benefits of Pattern Builder............................................................................................................................... 24
b. Differences of builder pattern and other design patterns..................................................................................24
c. Why we choose builder patterns to develop scenario?.....................................................................................25
Bibliography........................................................................................................................................26
Table Of Figures
Figure 1: Car ticket Class diagram................................................................................................................................6
Figure 2: Itialize interface variables........................................................................................................................7
Figure 3: Initialize the types of seats included in the ticket.........................................................................................7
Figure 4: Initialize foods and drinks.............................................................................................................................8
Figure 5: Set sizes and prices for bread and drink.......................................................................................................8
Figure 6: Install items..................................................................................................................................................9
Figure 7: Install Show Items.........................................................................................................................................9
Figure 8: Initialize orders for customers....................................................................................................................10
Figure 9: Program implementation............................................................................................................................10
Figure 10: Program Result.........................................................................................................................................11
Figure 11: Factory Method class diagram..................................................................................................................15
Figure 12:Prototype Pattern class diagram................................................................................................................16
Figure 13: Builder pattern class diagram...................................................................................................................17
Figure 14: Decorator Pattern class diagram...............................................................................................................18
Figure 15: Flyweight Pattern class diagram...............................................................................................................19
Figure 16: Proxy Pattern class diagram......................................................................................................................20
Figure 17: Mediator Pattern class diagram................................................................................................................21
Figure 18:Iterator Pattern class diagram...................................................................................................................22
Figure 19: State Pattern class diagram......................................................................................................................23
I. Introduction...........................................................................................................................................4
II. Implement code applying design patterns.........................................................................................5
1. P3 Build an application derived from UML class diagrams......................................................................5
2. M3 Develop code that implements a design pattern for a given purpose.................................................7
III. Investigate scenarios with respect to design patterns.....................................................................14
1. P4 Discuss a range of design patterns with relevant examples of creational, structural and behavioral
pattern types........................................................................................................................................................14
a. What is Design Patterns?..........................................................................................................................................14
b. Creational Patterns....................................................................................................................................................14
c. Structural Pattern......................................................................................................................................................18
d. Behavioral Pattern.....................................................................................................................................................21
2. M4 Reconcile the most appropriate design pattern from a range with a series of given scenarios......24
a. Benefits of Pattern Builder............................................................................................................................... 24
b. Differences of builder pattern and other design patterns..................................................................................24
c. Why we choose builder patterns to develop scenario?.....................................................................................25
Bibliography........................................................................................................................................26
Table Of Figures
Figure 1: Car ticket Class diagram................................................................................................................................6
Figure 2: Itialize interface variables........................................................................................................................7
Figure 3: Initialize the types of seats included in the ticket.........................................................................................7
Figure 4: Initialize foods and drinks.............................................................................................................................8
Figure 5: Set sizes and prices for bread and drink.......................................................................................................8
Figure 6: Install items..................................................................................................................................................9
Figure 7: Install Show Items.........................................................................................................................................9
Figure 8: Initialize orders for customers....................................................................................................................10
Figure 9: Program implementation............................................................................................................................10
Figure 10: Program Result.........................................................................................................................................11
Figure 11: Factory Method class diagram..................................................................................................................15
Figure 12:Prototype Pattern class diagram................................................................................................................16
Figure 13: Builder pattern class diagram...................................................................................................................17
Figure 14: Decorator Pattern class diagram...............................................................................................................18
Figure 15: Flyweight Pattern class diagram...............................................................................................................19
Figure 16: Proxy Pattern class diagram......................................................................................................................20
Figure 17: Mediator Pattern class diagram................................................................................................................21
Figure 18:Iterator Pattern class diagram...................................................................................................................22
Figure 19: State Pattern class diagram......................................................................................................................23
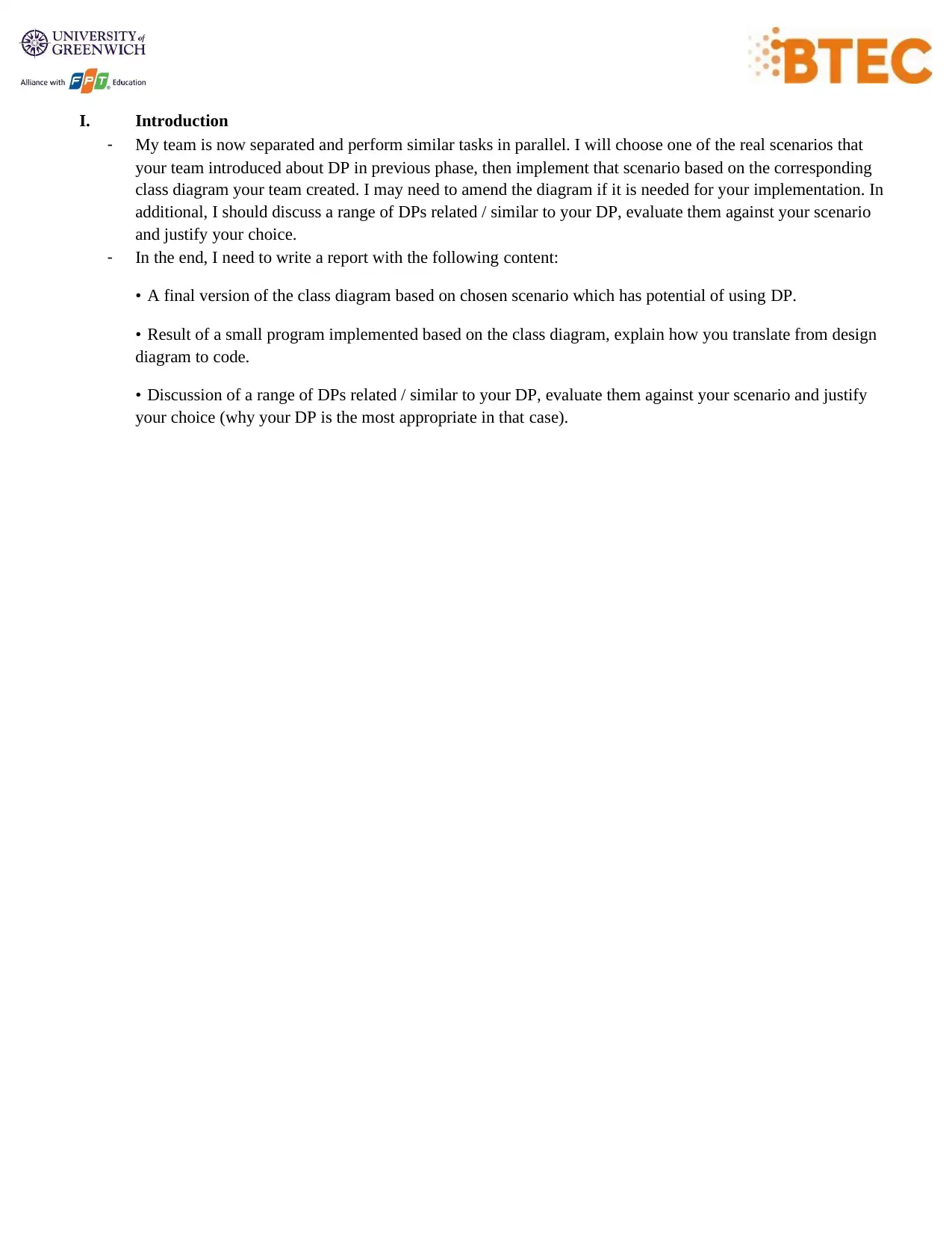
I. Introduction
- My team is now separated and perform similar tasks in parallel. I will choose one of the real scenarios that
your team introduced about DP in previous phase, then implement that scenario based on the corresponding
class diagram your team created. I may need to amend the diagram if it is needed for your implementation. In
additional, I should discuss a range of DPs related / similar to your DP, evaluate them against your scenario
and justify your choice.
- In the end, I need to write a report with the following content:
• A final version of the class diagram based on chosen scenario which has potential of using DP.
• Result of a small program implemented based on the class diagram, explain how you translate from design
diagram to code.
• Discussion of a range of DPs related / similar to your DP, evaluate them against your scenario and justify
your choice (why your DP is the most appropriate in that case).
- My team is now separated and perform similar tasks in parallel. I will choose one of the real scenarios that
your team introduced about DP in previous phase, then implement that scenario based on the corresponding
class diagram your team created. I may need to amend the diagram if it is needed for your implementation. In
additional, I should discuss a range of DPs related / similar to your DP, evaluate them against your scenario
and justify your choice.
- In the end, I need to write a report with the following content:
• A final version of the class diagram based on chosen scenario which has potential of using DP.
• Result of a small program implemented based on the class diagram, explain how you translate from design
diagram to code.
• Discussion of a range of DPs related / similar to your DP, evaluate them against your scenario and justify
your choice (why your DP is the most appropriate in that case).
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
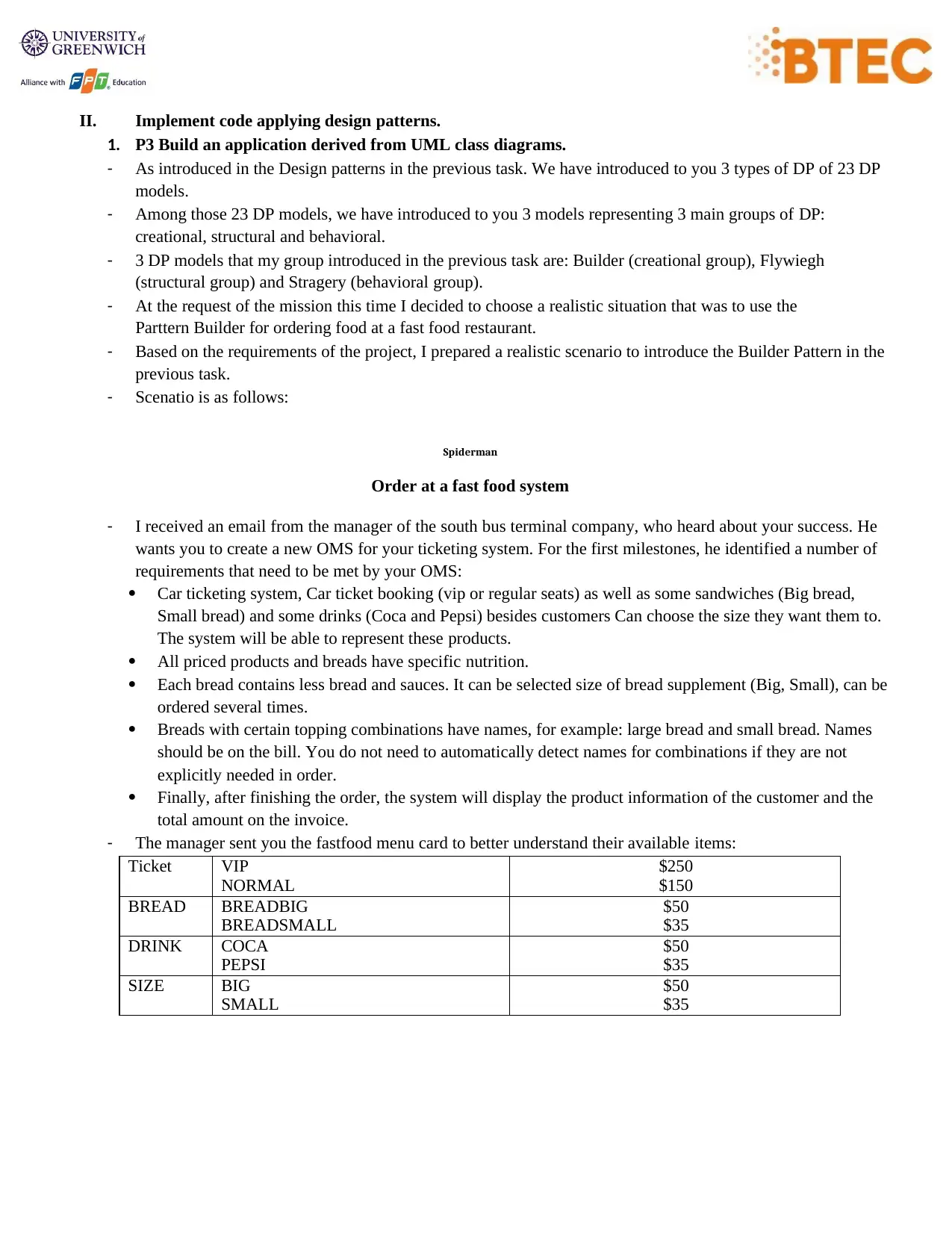
II. Implement code applying design patterns.
1. P3 Build an application derived from UML class diagrams.
- As introduced in the Design patterns in the previous task. We have introduced to you 3 types of DP of 23 DP
models.
- Among those 23 DP models, we have introduced to you 3 models representing 3 main groups of DP:
creational, structural and behavioral.
- 3 DP models that my group introduced in the previous task are: Builder (creational group), Flywiegh
(structural group) and Stragery (behavioral group).
- At the request of the mission this time I decided to choose a realistic situation that was to use the
Parttern Builder for ordering food at a fast food restaurant.
- Based on the requirements of the project, I prepared a realistic scenario to introduce the Builder Pattern in the
previous task.
- Scenatio is as follows:
Spiderman
Order at a fast food system
- I received an email from the manager of the south bus terminal company, who heard about your success. He
wants you to create a new OMS for your ticketing system. For the first milestones, he identified a number of
requirements that need to be met by your OMS:
Car ticketing system, Car ticket booking (vip or regular seats) as well as some sandwiches (Big bread,
Small bread) and some drinks (Coca and Pepsi) besides customers Can choose the size they want them to.
The system will be able to represent these products.
All priced products and breads have specific nutrition.
Each bread contains less bread and sauces. It can be selected size of bread supplement (Big, Small), can be
ordered several times.
Breads with certain topping combinations have names, for example: large bread and small bread. Names
should be on the bill. You do not need to automatically detect names for combinations if they are not
explicitly needed in order.
Finally, after finishing the order, the system will display the product information of the customer and the
total amount on the invoice.
- The manager sent you the fastfood menu card to better understand their available items:
Ticket VIP
NORMAL
$250
$150
BREAD BREADBIG
BREADSMALL
$50
$35
DRINK COCA
PEPSI
$50
$35
SIZE BIG
SMALL
$50
$35
1. P3 Build an application derived from UML class diagrams.
- As introduced in the Design patterns in the previous task. We have introduced to you 3 types of DP of 23 DP
models.
- Among those 23 DP models, we have introduced to you 3 models representing 3 main groups of DP:
creational, structural and behavioral.
- 3 DP models that my group introduced in the previous task are: Builder (creational group), Flywiegh
(structural group) and Stragery (behavioral group).
- At the request of the mission this time I decided to choose a realistic situation that was to use the
Parttern Builder for ordering food at a fast food restaurant.
- Based on the requirements of the project, I prepared a realistic scenario to introduce the Builder Pattern in the
previous task.
- Scenatio is as follows:
Spiderman
Order at a fast food system
- I received an email from the manager of the south bus terminal company, who heard about your success. He
wants you to create a new OMS for your ticketing system. For the first milestones, he identified a number of
requirements that need to be met by your OMS:
Car ticketing system, Car ticket booking (vip or regular seats) as well as some sandwiches (Big bread,
Small bread) and some drinks (Coca and Pepsi) besides customers Can choose the size they want them to.
The system will be able to represent these products.
All priced products and breads have specific nutrition.
Each bread contains less bread and sauces. It can be selected size of bread supplement (Big, Small), can be
ordered several times.
Breads with certain topping combinations have names, for example: large bread and small bread. Names
should be on the bill. You do not need to automatically detect names for combinations if they are not
explicitly needed in order.
Finally, after finishing the order, the system will display the product information of the customer and the
total amount on the invoice.
- The manager sent you the fastfood menu card to better understand their available items:
Ticket VIP
NORMAL
$250
$150
BREAD BREADBIG
BREADSMALL
$50
$35
DRINK COCA
PEPSI
$50
$35
SIZE BIG
SMALL
$50
$35
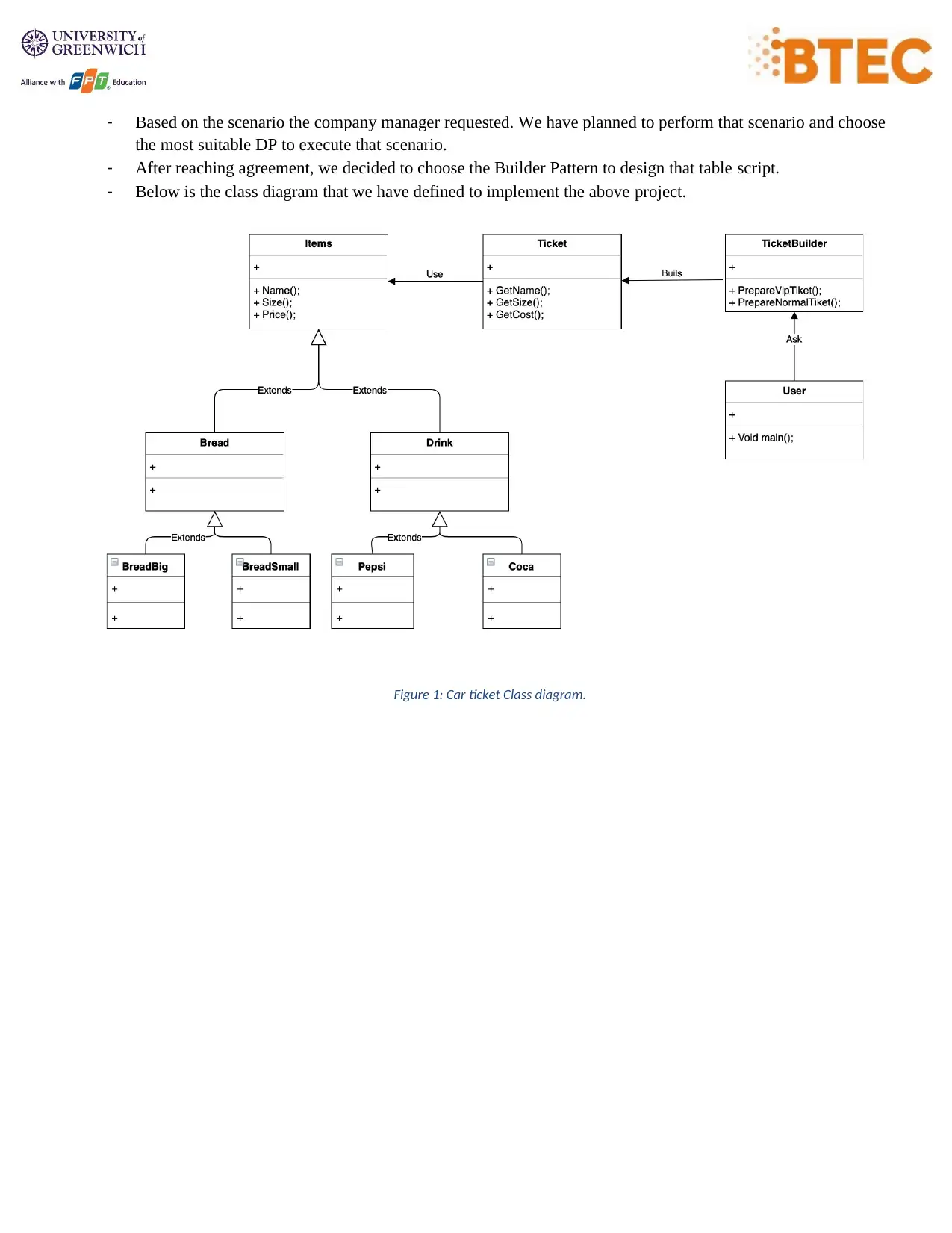
- Based on the scenario the company manager requested. We have planned to perform that scenario and choose
the most suitable DP to execute that scenario.
- After reaching agreement, we decided to choose the Builder Pattern to design that table script.
- Below is the class diagram that we have defined to implement the above project.
Figure 1: Car ticket Class diagram.
the most suitable DP to execute that scenario.
- After reaching agreement, we decided to choose the Builder Pattern to design that table script.
- Below is the class diagram that we have defined to implement the above project.
Figure 1: Car ticket Class diagram.
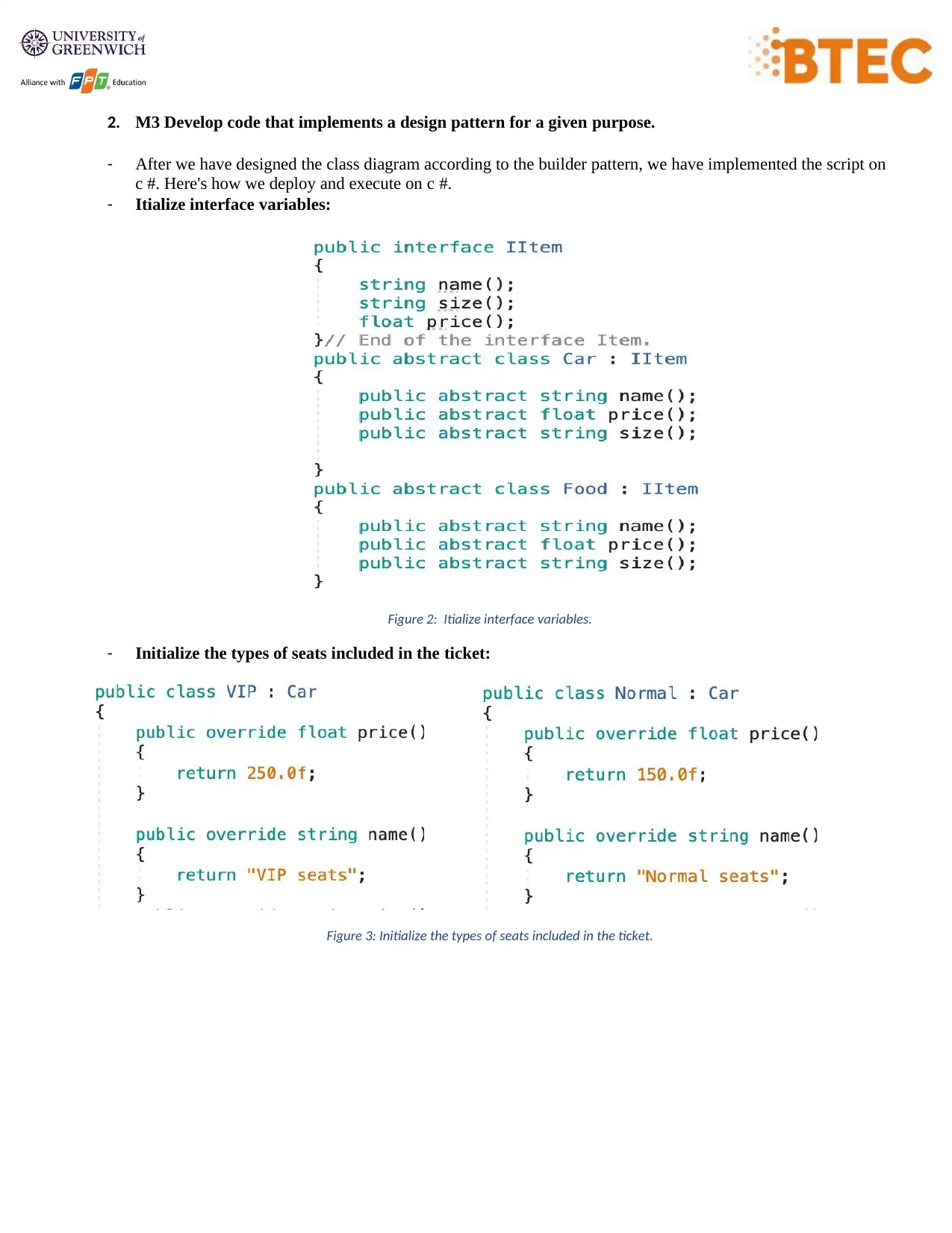
2. M3 Develop code that implements a design pattern for a given purpose.
- After we have designed the class diagram according to the builder pattern, we have implemented the script on
c #. Here's how we deploy and execute on c #.
- Itialize interface variables:
Figure 2: Itialize interface variables.
- Initialize the types of seats included in the ticket:
Figure 3: Initialize the types of seats included in the ticket.
- After we have designed the class diagram according to the builder pattern, we have implemented the script on
c #. Here's how we deploy and execute on c #.
- Itialize interface variables:
Figure 2: Itialize interface variables.
- Initialize the types of seats included in the ticket:
Figure 3: Initialize the types of seats included in the ticket.
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
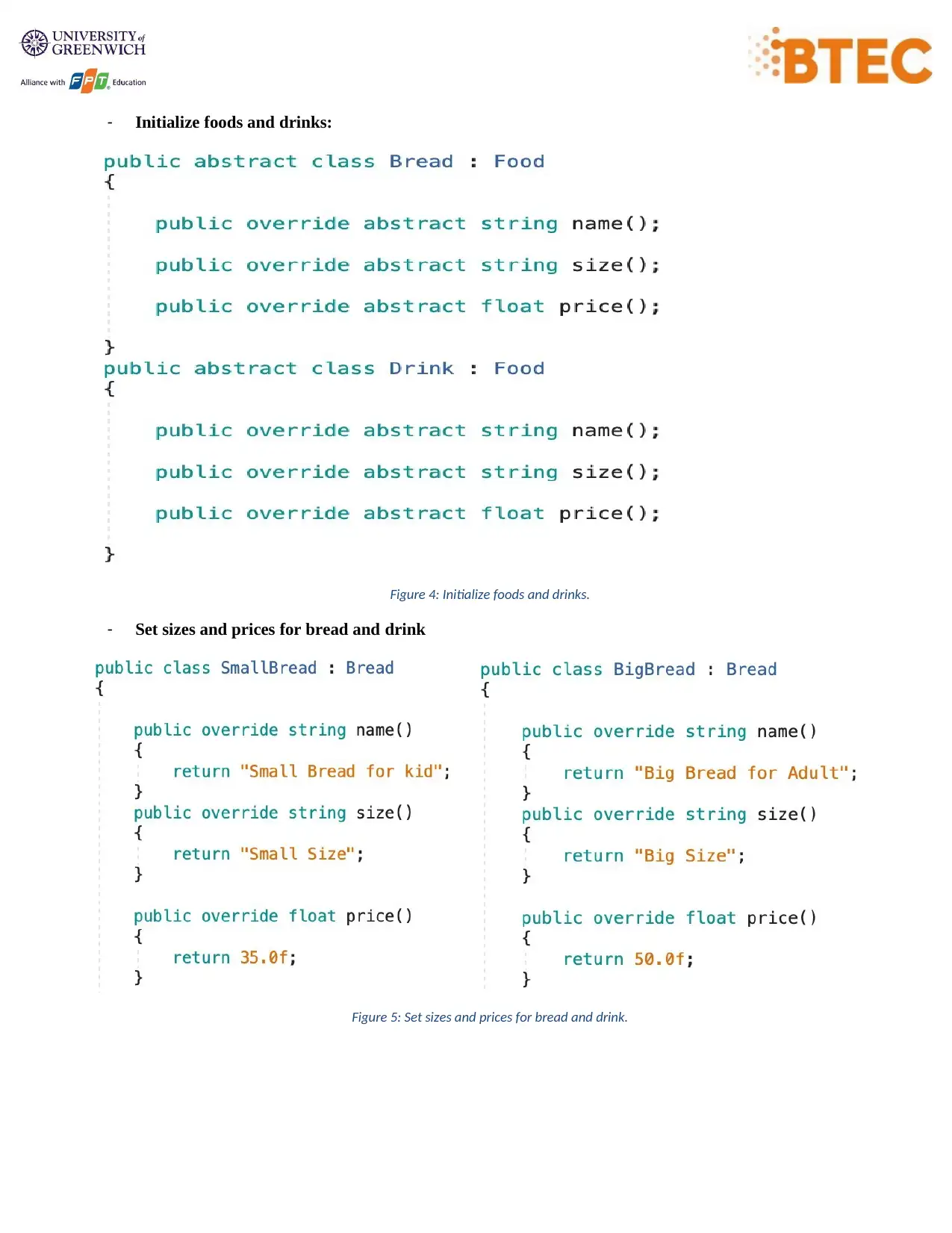
- Initialize foods and drinks:
Figure 4: Initialize foods and drinks.
- Set sizes and prices for bread and drink
Figure 5: Set sizes and prices for bread and drink.
Figure 4: Initialize foods and drinks.
- Set sizes and prices for bread and drink
Figure 5: Set sizes and prices for bread and drink.
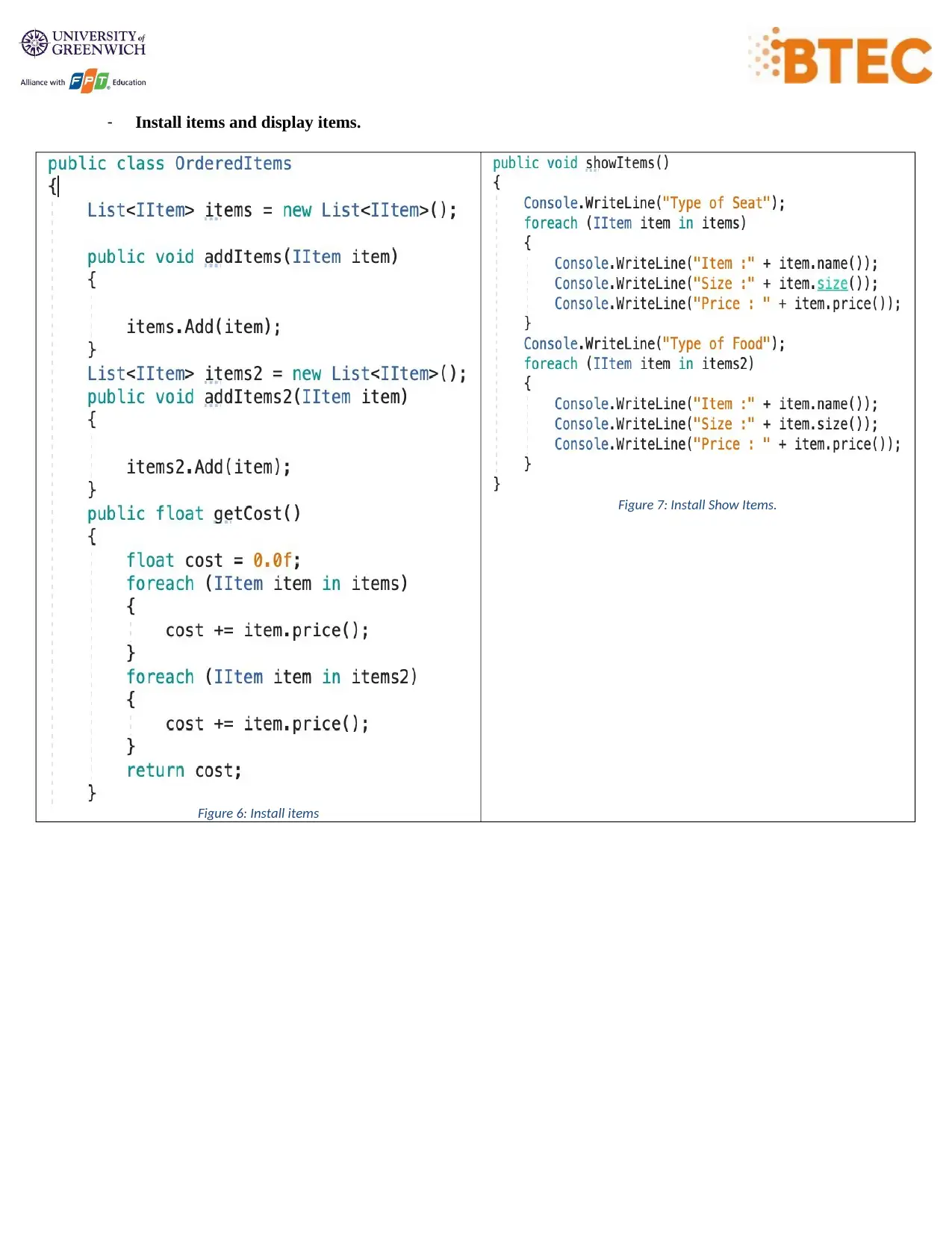
- Install items and display items.
Figure 7: Install Show Items.
Figure 6: Install items
Figure 7: Install Show Items.
Figure 6: Install items
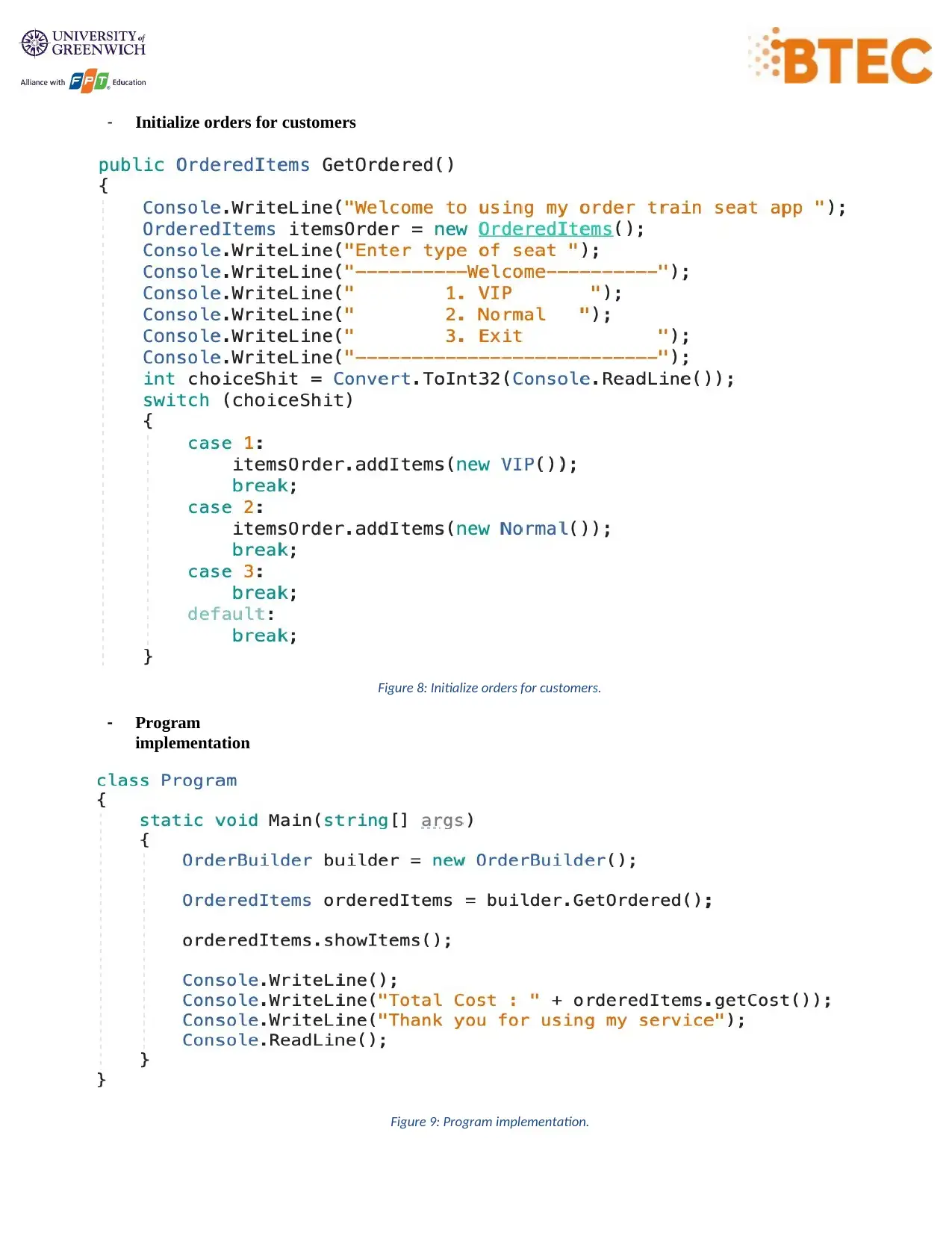
- Initialize orders for customers
- Program
implementation
Figure 8: Initialize orders for customers.
Figure 9: Program implementation.
- Program
implementation
Figure 8: Initialize orders for customers.
Figure 9: Program implementation.
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
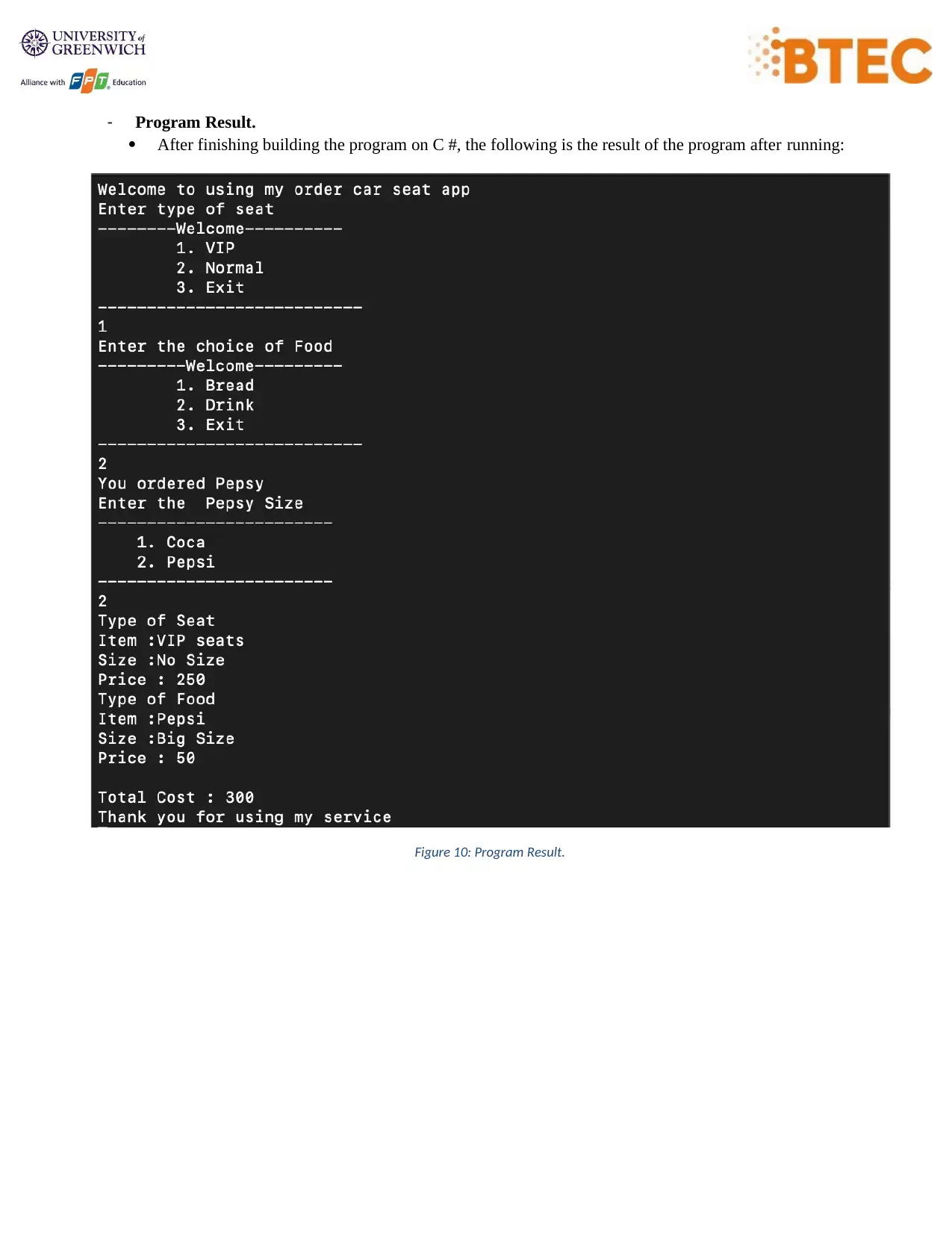
- Program Result.
After finishing building the program on C #, the following is the result of the program after running:
Figure 10: Program Result.
After finishing building the program on C #, the following is the result of the program after running:
Figure 10: Program Result.
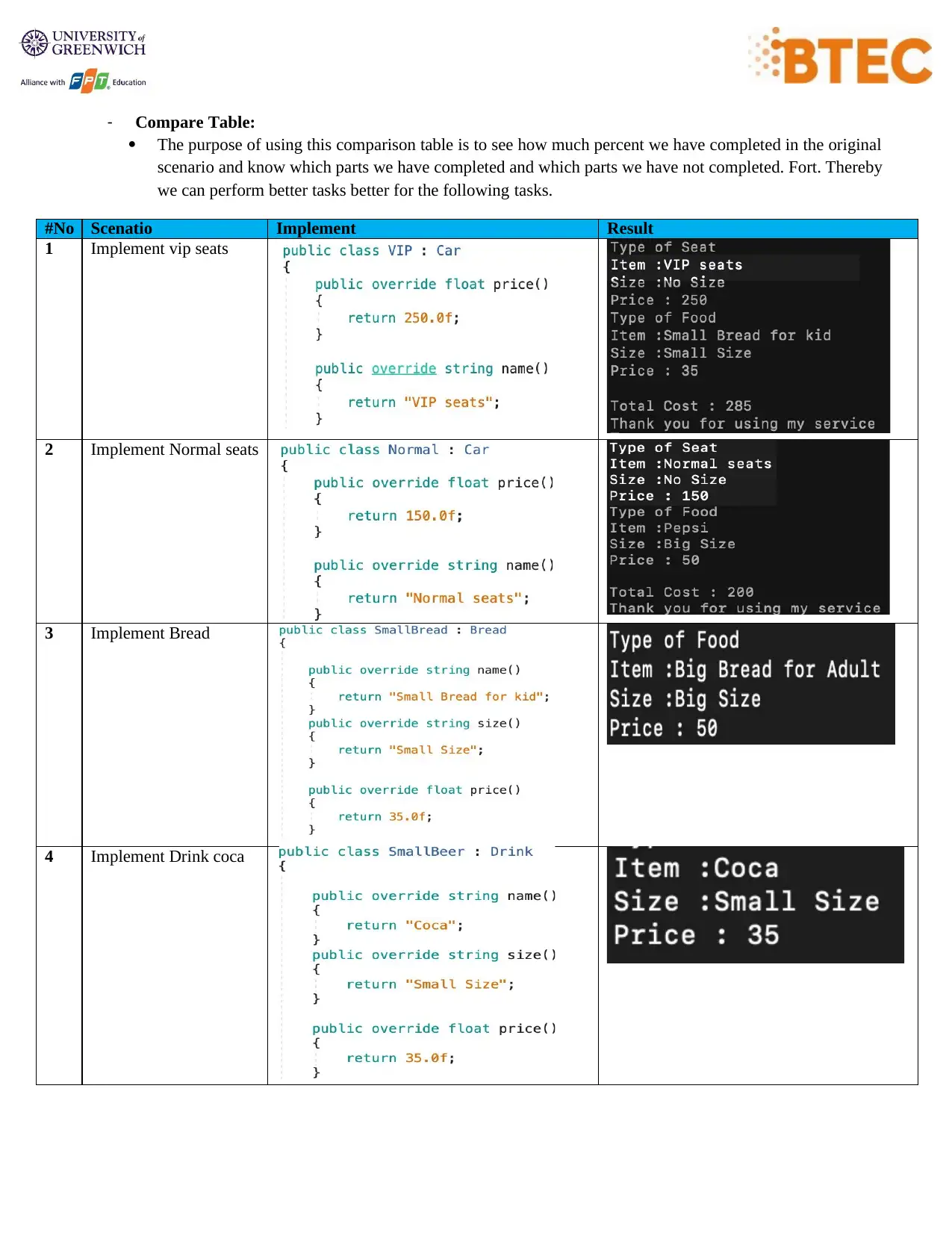
- Compare Table:
The purpose of using this comparison table is to see how much percent we have completed in the original
scenario and know which parts we have completed and which parts we have not completed. Fort. Thereby
we can perform better tasks better for the following tasks.
#No Scenatio Implement Result
1 Implement vip seats
2 Implement Normal seats
3 Implement Bread
4 Implement Drink coca
The purpose of using this comparison table is to see how much percent we have completed in the original
scenario and know which parts we have completed and which parts we have not completed. Fort. Thereby
we can perform better tasks better for the following tasks.
#No Scenatio Implement Result
1 Implement vip seats
2 Implement Normal seats
3 Implement Bread
4 Implement Drink coca
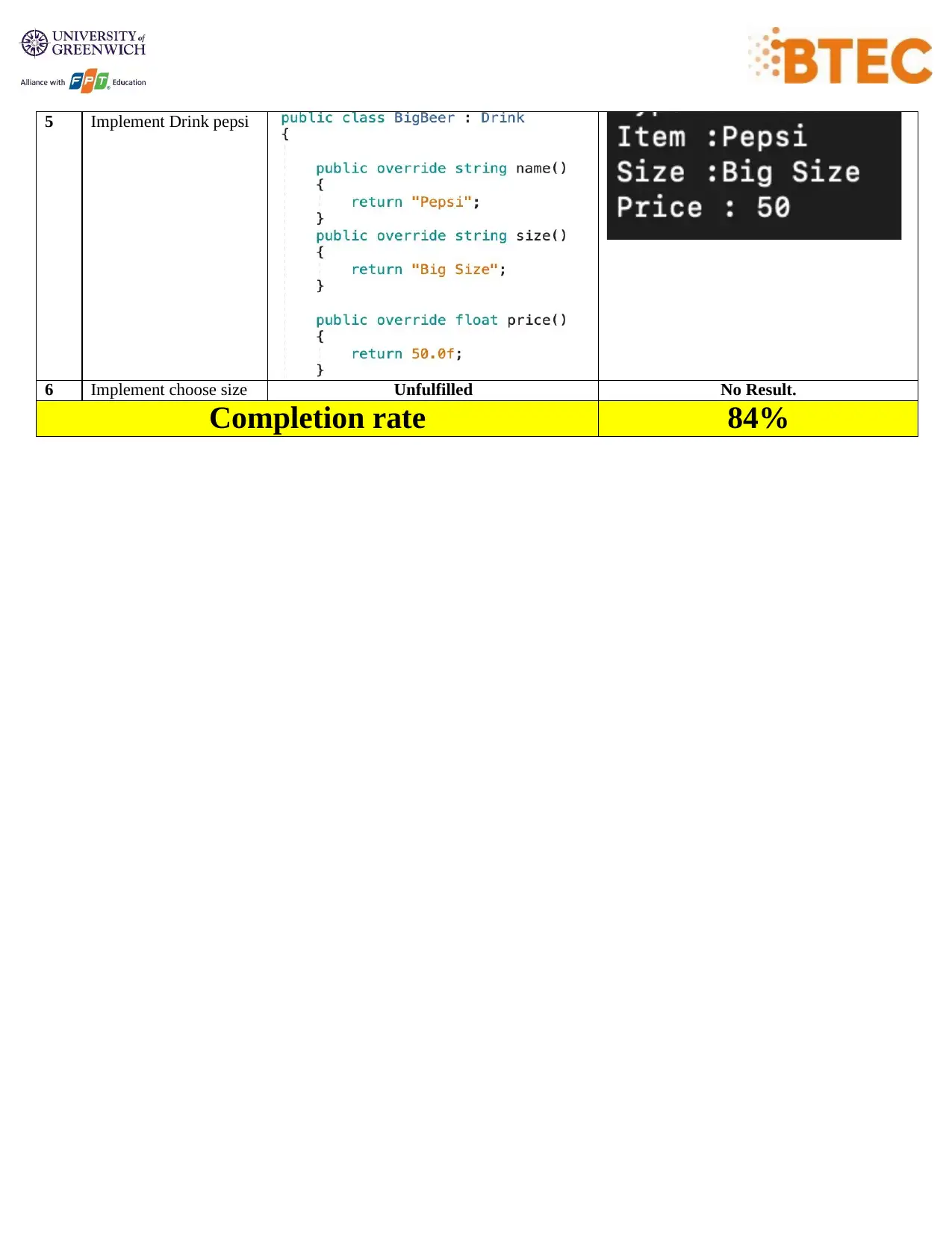
5 Implement Drink pepsi
6 Implement choose size Unfulfilled No Result.
Completion rate 84%
6 Implement choose size Unfulfilled No Result.
Completion rate 84%
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
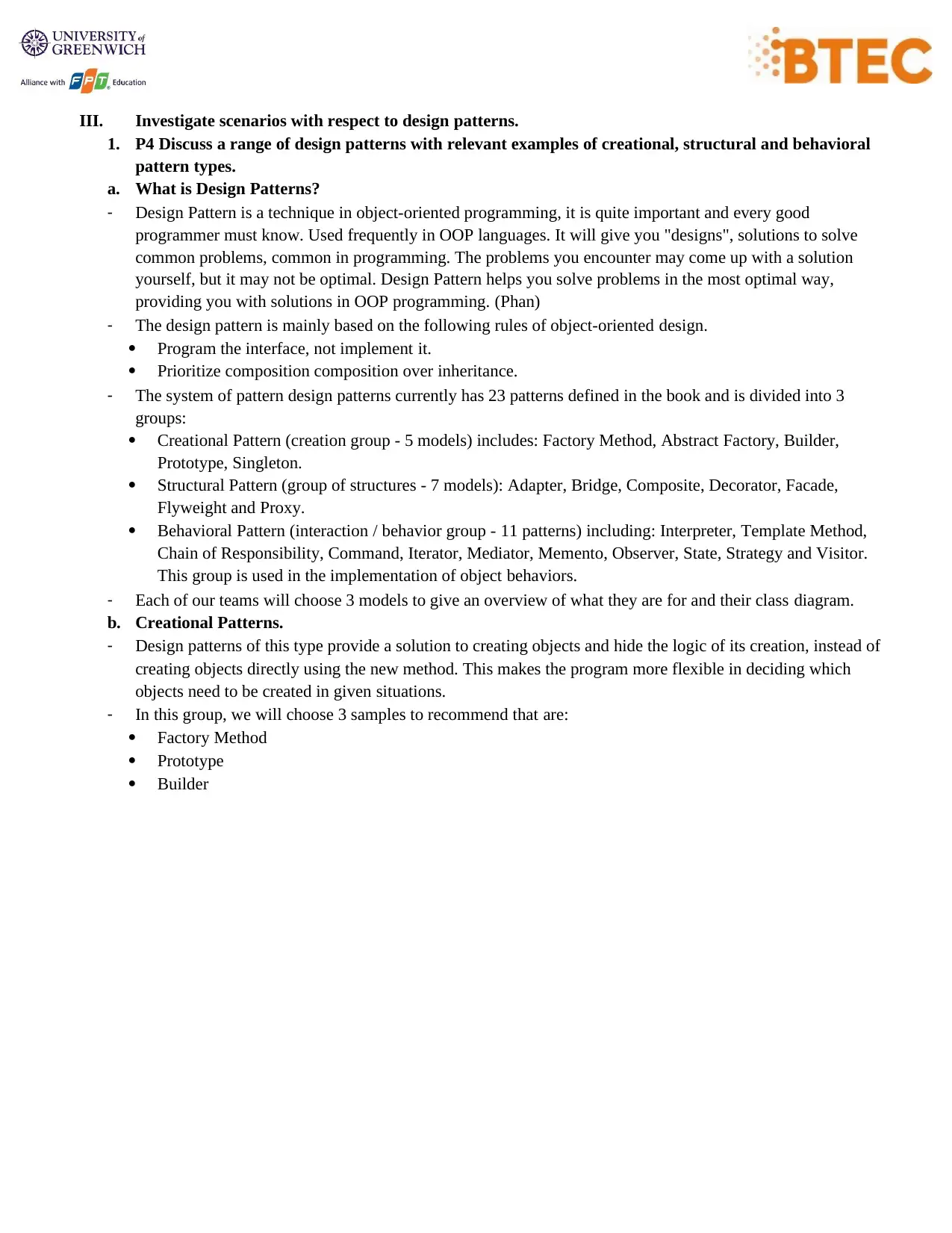
III. Investigate scenarios with respect to design patterns.
1. P4 Discuss a range of design patterns with relevant examples of creational, structural and behavioral
pattern types.
a. What is Design Patterns?
- Design Pattern is a technique in object-oriented programming, it is quite important and every good
programmer must know. Used frequently in OOP languages. It will give you "designs", solutions to solve
common problems, common in programming. The problems you encounter may come up with a solution
yourself, but it may not be optimal. Design Pattern helps you solve problems in the most optimal way,
providing you with solutions in OOP programming. (Phan)
- The design pattern is mainly based on the following rules of object-oriented design.
Program the interface, not implement it.
Prioritize composition composition over inheritance.
- The system of pattern design patterns currently has 23 patterns defined in the book and is divided into 3
groups:
Creational Pattern (creation group - 5 models) includes: Factory Method, Abstract Factory, Builder,
Prototype, Singleton.
Structural Pattern (group of structures - 7 models): Adapter, Bridge, Composite, Decorator, Facade,
Flyweight and Proxy.
Behavioral Pattern (interaction / behavior group - 11 patterns) including: Interpreter, Template Method,
Chain of Responsibility, Command, Iterator, Mediator, Memento, Observer, State, Strategy and Visitor.
This group is used in the implementation of object behaviors.
- Each of our teams will choose 3 models to give an overview of what they are for and their class diagram.
b. Creational Patterns.
- Design patterns of this type provide a solution to creating objects and hide the logic of its creation, instead of
creating objects directly using the new method. This makes the program more flexible in deciding which
objects need to be created in given situations.
- In this group, we will choose 3 samples to recommend that are:
Factory Method
Prototype
Builder
1. P4 Discuss a range of design patterns with relevant examples of creational, structural and behavioral
pattern types.
a. What is Design Patterns?
- Design Pattern is a technique in object-oriented programming, it is quite important and every good
programmer must know. Used frequently in OOP languages. It will give you "designs", solutions to solve
common problems, common in programming. The problems you encounter may come up with a solution
yourself, but it may not be optimal. Design Pattern helps you solve problems in the most optimal way,
providing you with solutions in OOP programming. (Phan)
- The design pattern is mainly based on the following rules of object-oriented design.
Program the interface, not implement it.
Prioritize composition composition over inheritance.
- The system of pattern design patterns currently has 23 patterns defined in the book and is divided into 3
groups:
Creational Pattern (creation group - 5 models) includes: Factory Method, Abstract Factory, Builder,
Prototype, Singleton.
Structural Pattern (group of structures - 7 models): Adapter, Bridge, Composite, Decorator, Facade,
Flyweight and Proxy.
Behavioral Pattern (interaction / behavior group - 11 patterns) including: Interpreter, Template Method,
Chain of Responsibility, Command, Iterator, Mediator, Memento, Observer, State, Strategy and Visitor.
This group is used in the implementation of object behaviors.
- Each of our teams will choose 3 models to give an overview of what they are for and their class diagram.
b. Creational Patterns.
- Design patterns of this type provide a solution to creating objects and hide the logic of its creation, instead of
creating objects directly using the new method. This makes the program more flexible in deciding which
objects need to be created in given situations.
- In this group, we will choose 3 samples to recommend that are:
Factory Method
Prototype
Builder
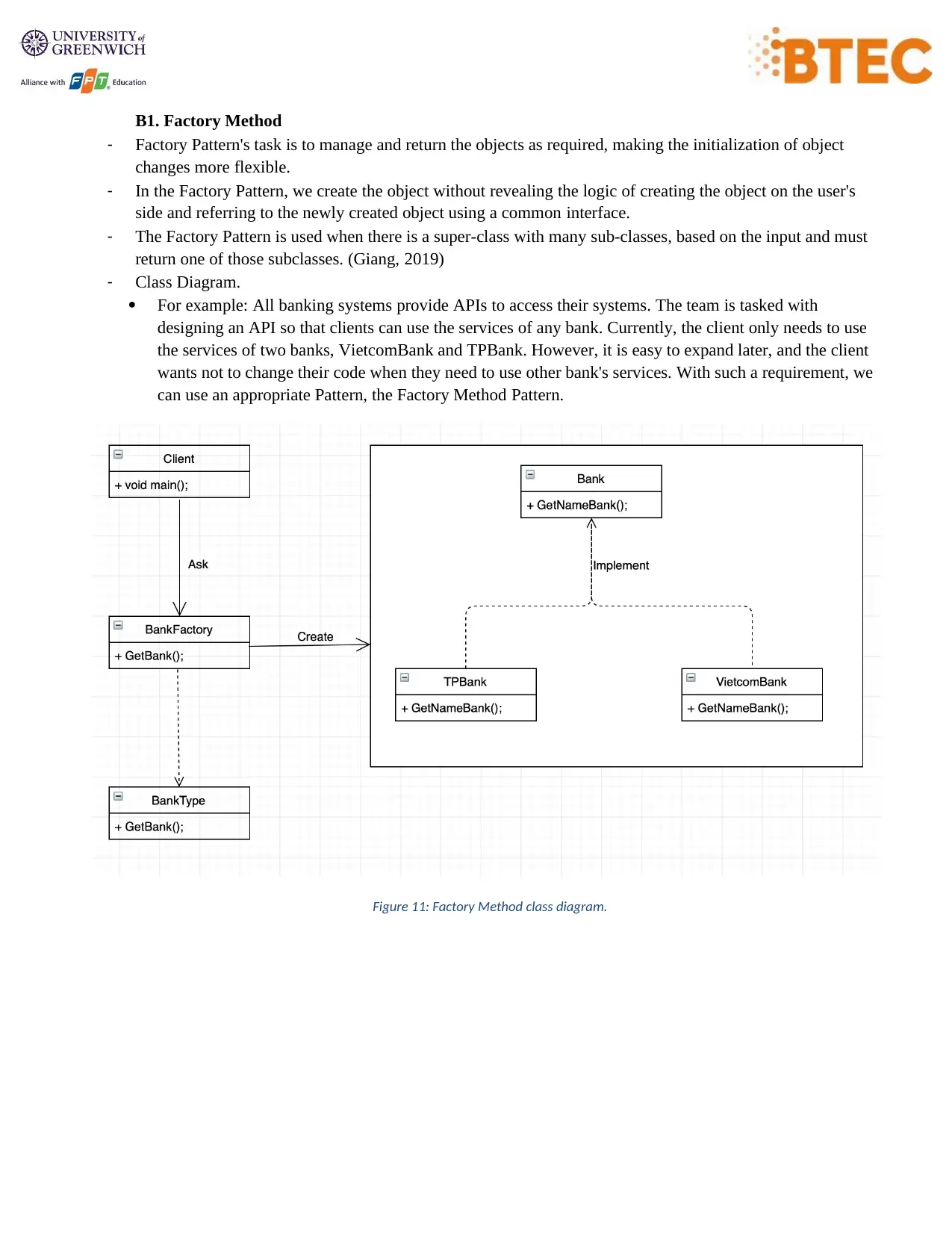
B1. Factory Method
- Factory Pattern's task is to manage and return the objects as required, making the initialization of object
changes more flexible.
- In the Factory Pattern, we create the object without revealing the logic of creating the object on the user's
side and referring to the newly created object using a common interface.
- The Factory Pattern is used when there is a super-class with many sub-classes, based on the input and must
return one of those subclasses. (Giang, 2019)
- Class Diagram.
For example: All banking systems provide APIs to access their systems. The team is tasked with
designing an API so that clients can use the services of any bank. Currently, the client only needs to use
the services of two banks, VietcomBank and TPBank. However, it is easy to expand later, and the client
wants not to change their code when they need to use other bank's services. With such a requirement, we
can use an appropriate Pattern, the Factory Method Pattern.
Figure 11: Factory Method class diagram.
- Factory Pattern's task is to manage and return the objects as required, making the initialization of object
changes more flexible.
- In the Factory Pattern, we create the object without revealing the logic of creating the object on the user's
side and referring to the newly created object using a common interface.
- The Factory Pattern is used when there is a super-class with many sub-classes, based on the input and must
return one of those subclasses. (Giang, 2019)
- Class Diagram.
For example: All banking systems provide APIs to access their systems. The team is tasked with
designing an API so that clients can use the services of any bank. Currently, the client only needs to use
the services of two banks, VietcomBank and TPBank. However, it is easy to expand later, and the client
wants not to change their code when they need to use other bank's services. With such a requirement, we
can use an appropriate Pattern, the Factory Method Pattern.
Figure 11: Factory Method class diagram.
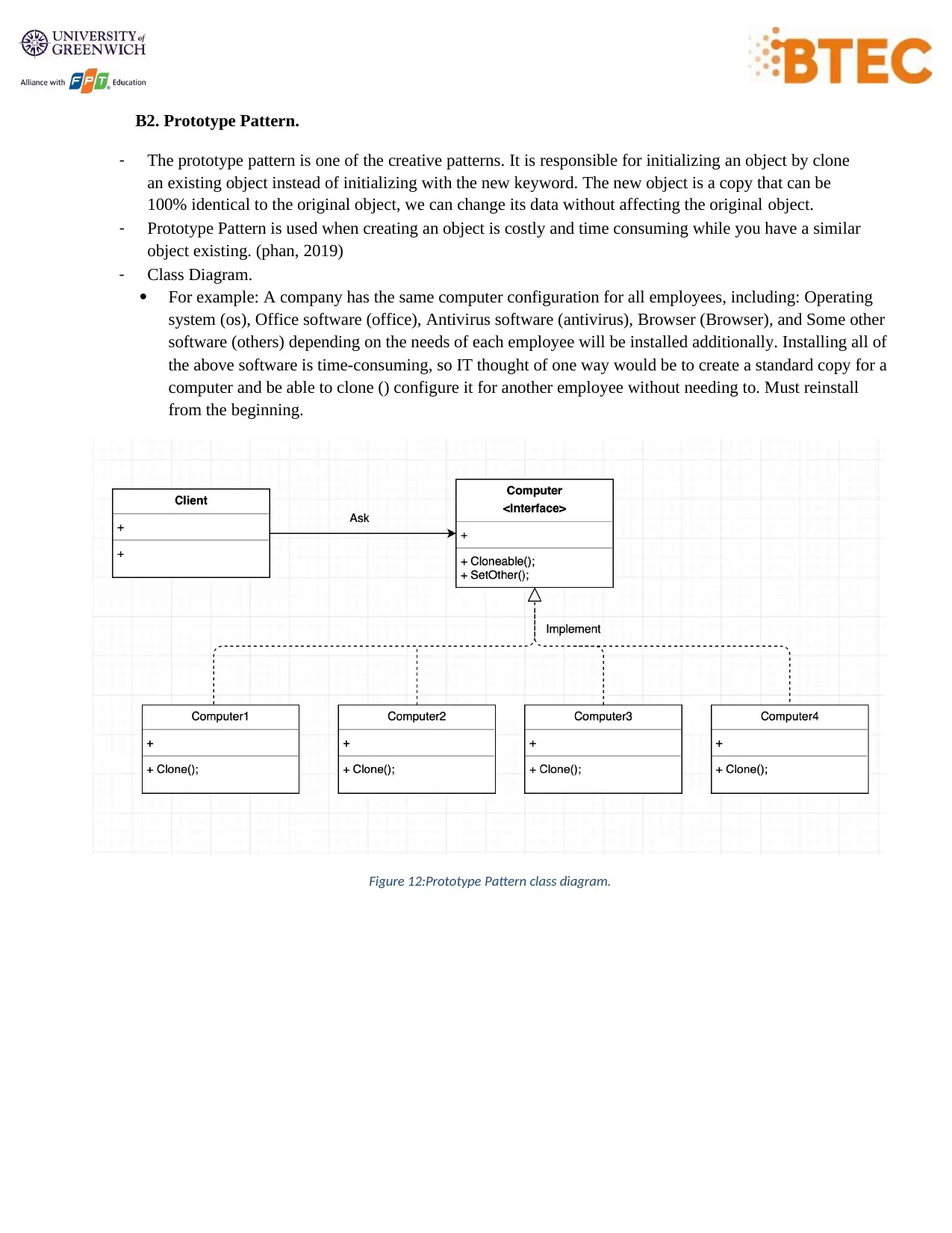
B2. Prototype Pattern.
- The prototype pattern is one of the creative patterns. It is responsible for initializing an object by clone
an existing object instead of initializing with the new keyword. The new object is a copy that can be
100% identical to the original object, we can change its data without affecting the original object.
- Prototype Pattern is used when creating an object is costly and time consuming while you have a similar
object existing. (phan, 2019)
- Class Diagram.
For example: A company has the same computer configuration for all employees, including: Operating
system (os), Office software (office), Antivirus software (antivirus), Browser (Browser), and Some other
software (others) depending on the needs of each employee will be installed additionally. Installing all of
the above software is time-consuming, so IT thought of one way would be to create a standard copy for a
computer and be able to clone () configure it for another employee without needing to. Must reinstall
from the beginning.
Figure 12:Prototype Pattern class diagram.
- The prototype pattern is one of the creative patterns. It is responsible for initializing an object by clone
an existing object instead of initializing with the new keyword. The new object is a copy that can be
100% identical to the original object, we can change its data without affecting the original object.
- Prototype Pattern is used when creating an object is costly and time consuming while you have a similar
object existing. (phan, 2019)
- Class Diagram.
For example: A company has the same computer configuration for all employees, including: Operating
system (os), Office software (office), Antivirus software (antivirus), Browser (Browser), and Some other
software (others) depending on the needs of each employee will be installed additionally. Installing all of
the above software is time-consuming, so IT thought of one way would be to create a standard copy for a
computer and be able to clone () configure it for another employee without needing to. Must reinstall
from the beginning.
Figure 12:Prototype Pattern class diagram.
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
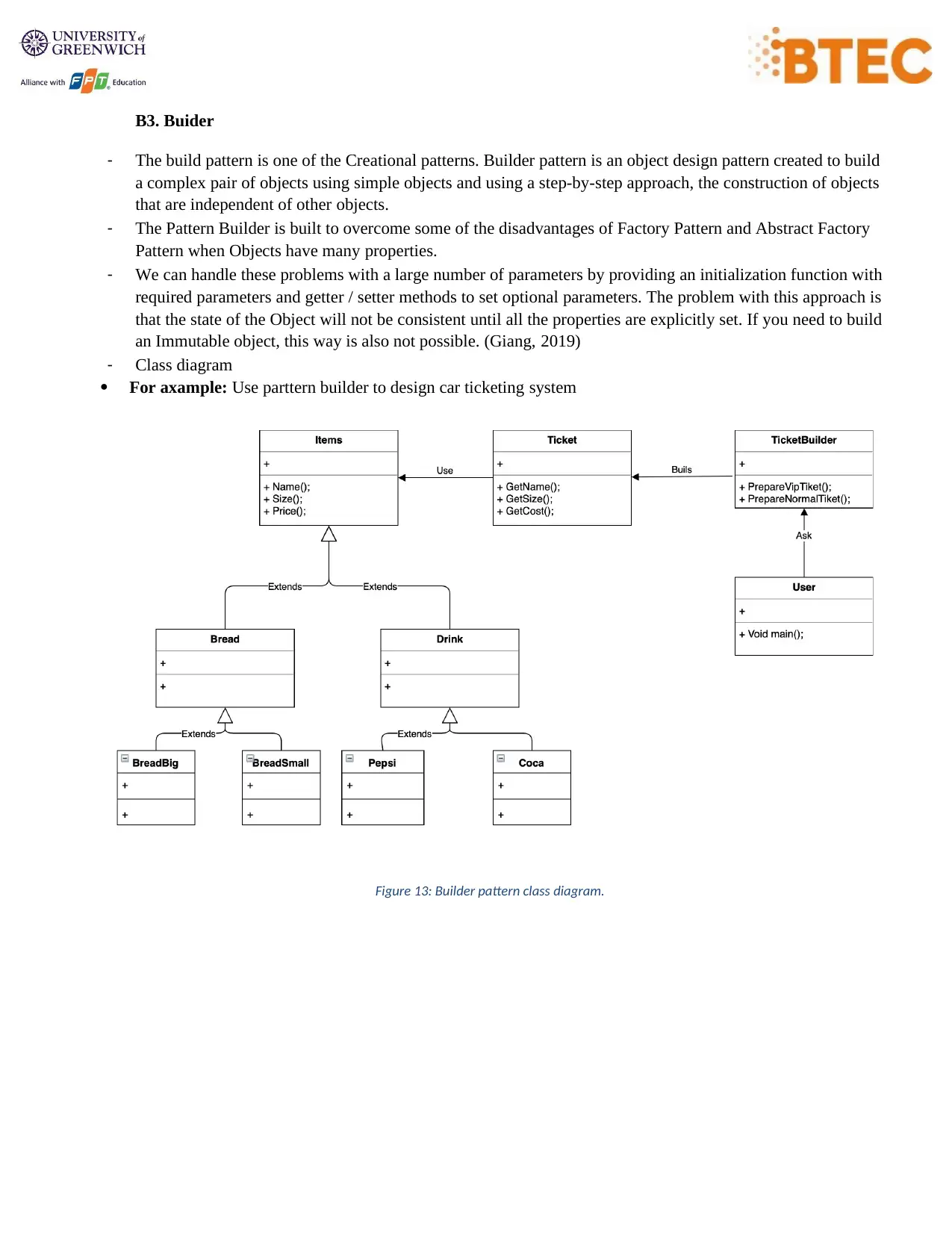
B3. Buider
- The build pattern is one of the Creational patterns. Builder pattern is an object design pattern created to build
a complex pair of objects using simple objects and using a step-by-step approach, the construction of objects
that are independent of other objects.
- The Pattern Builder is built to overcome some of the disadvantages of Factory Pattern and Abstract Factory
Pattern when Objects have many properties.
- We can handle these problems with a large number of parameters by providing an initialization function with
required parameters and getter / setter methods to set optional parameters. The problem with this approach is
that the state of the Object will not be consistent until all the properties are explicitly set. If you need to build
an Immutable object, this way is also not possible. (Giang, 2019)
- Class diagram
For axample: Use parttern builder to design car ticketing system
Figure 13: Builder pattern class diagram.
- The build pattern is one of the Creational patterns. Builder pattern is an object design pattern created to build
a complex pair of objects using simple objects and using a step-by-step approach, the construction of objects
that are independent of other objects.
- The Pattern Builder is built to overcome some of the disadvantages of Factory Pattern and Abstract Factory
Pattern when Objects have many properties.
- We can handle these problems with a large number of parameters by providing an initialization function with
required parameters and getter / setter methods to set optional parameters. The problem with this approach is
that the state of the Object will not be consistent until all the properties are explicitly set. If you need to build
an Immutable object, this way is also not possible. (Giang, 2019)
- Class diagram
For axample: Use parttern builder to design car ticketing system
Figure 13: Builder pattern class diagram.
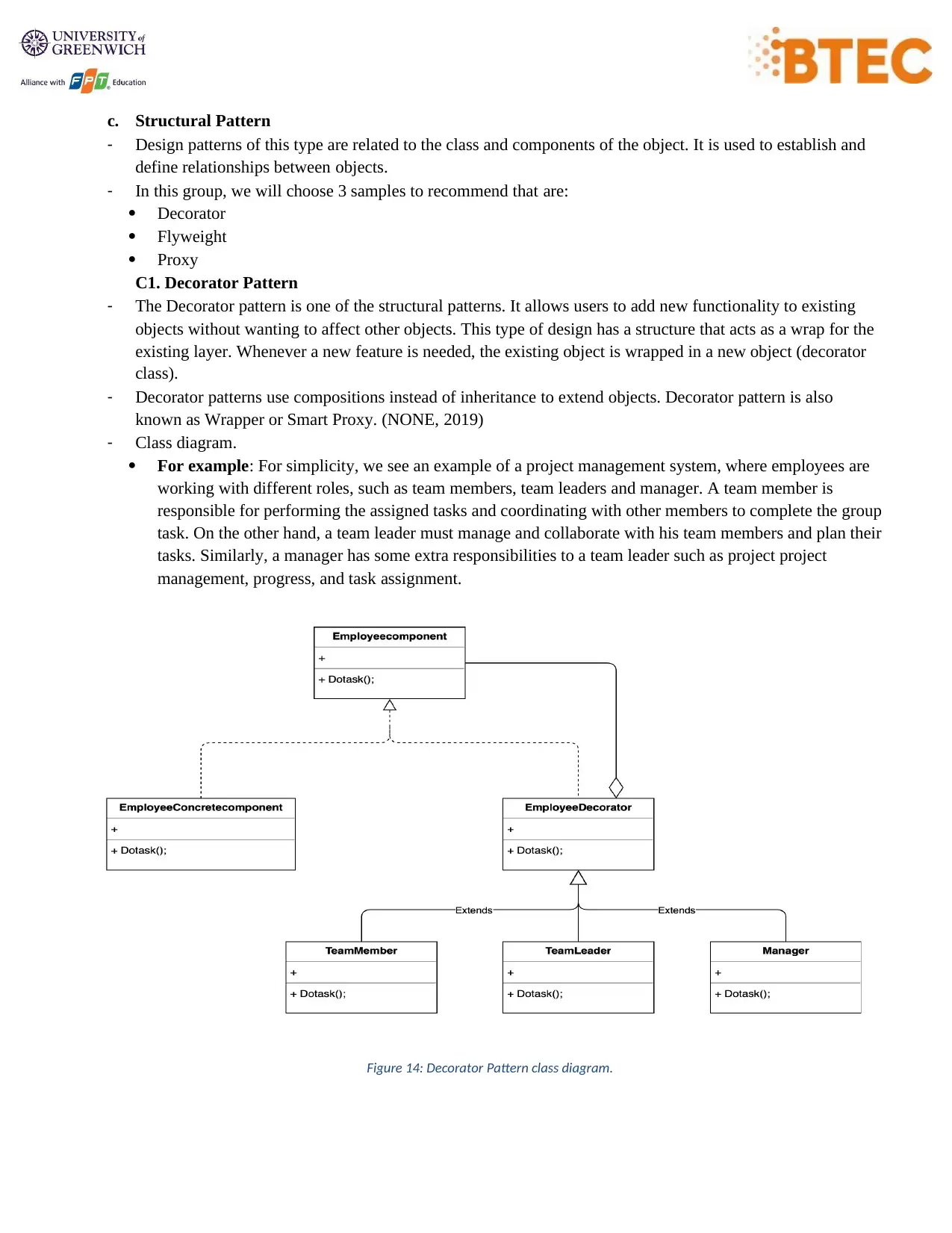
c. Structural Pattern
- Design patterns of this type are related to the class and components of the object. It is used to establish and
define relationships between objects.
- In this group, we will choose 3 samples to recommend that are:
Decorator
Flyweight
Proxy
C1. Decorator Pattern
- The Decorator pattern is one of the structural patterns. It allows users to add new functionality to existing
objects without wanting to affect other objects. This type of design has a structure that acts as a wrap for the
existing layer. Whenever a new feature is needed, the existing object is wrapped in a new object (decorator
class).
- Decorator patterns use compositions instead of inheritance to extend objects. Decorator pattern is also
known as Wrapper or Smart Proxy. (NONE, 2019)
- Class diagram.
For example: For simplicity, we see an example of a project management system, where employees are
working with different roles, such as team members, team leaders and manager. A team member is
responsible for performing the assigned tasks and coordinating with other members to complete the group
task. On the other hand, a team leader must manage and collaborate with his team members and plan their
tasks. Similarly, a manager has some extra responsibilities to a team leader such as project project
management, progress, and task assignment.
Figure 14: Decorator Pattern class diagram.
- Design patterns of this type are related to the class and components of the object. It is used to establish and
define relationships between objects.
- In this group, we will choose 3 samples to recommend that are:
Decorator
Flyweight
Proxy
C1. Decorator Pattern
- The Decorator pattern is one of the structural patterns. It allows users to add new functionality to existing
objects without wanting to affect other objects. This type of design has a structure that acts as a wrap for the
existing layer. Whenever a new feature is needed, the existing object is wrapped in a new object (decorator
class).
- Decorator patterns use compositions instead of inheritance to extend objects. Decorator pattern is also
known as Wrapper or Smart Proxy. (NONE, 2019)
- Class diagram.
For example: For simplicity, we see an example of a project management system, where employees are
working with different roles, such as team members, team leaders and manager. A team member is
responsible for performing the assigned tasks and coordinating with other members to complete the group
task. On the other hand, a team leader must manage and collaborate with his team members and plan their
tasks. Similarly, a manager has some extra responsibilities to a team leader such as project project
management, progress, and task assignment.
Figure 14: Decorator Pattern class diagram.
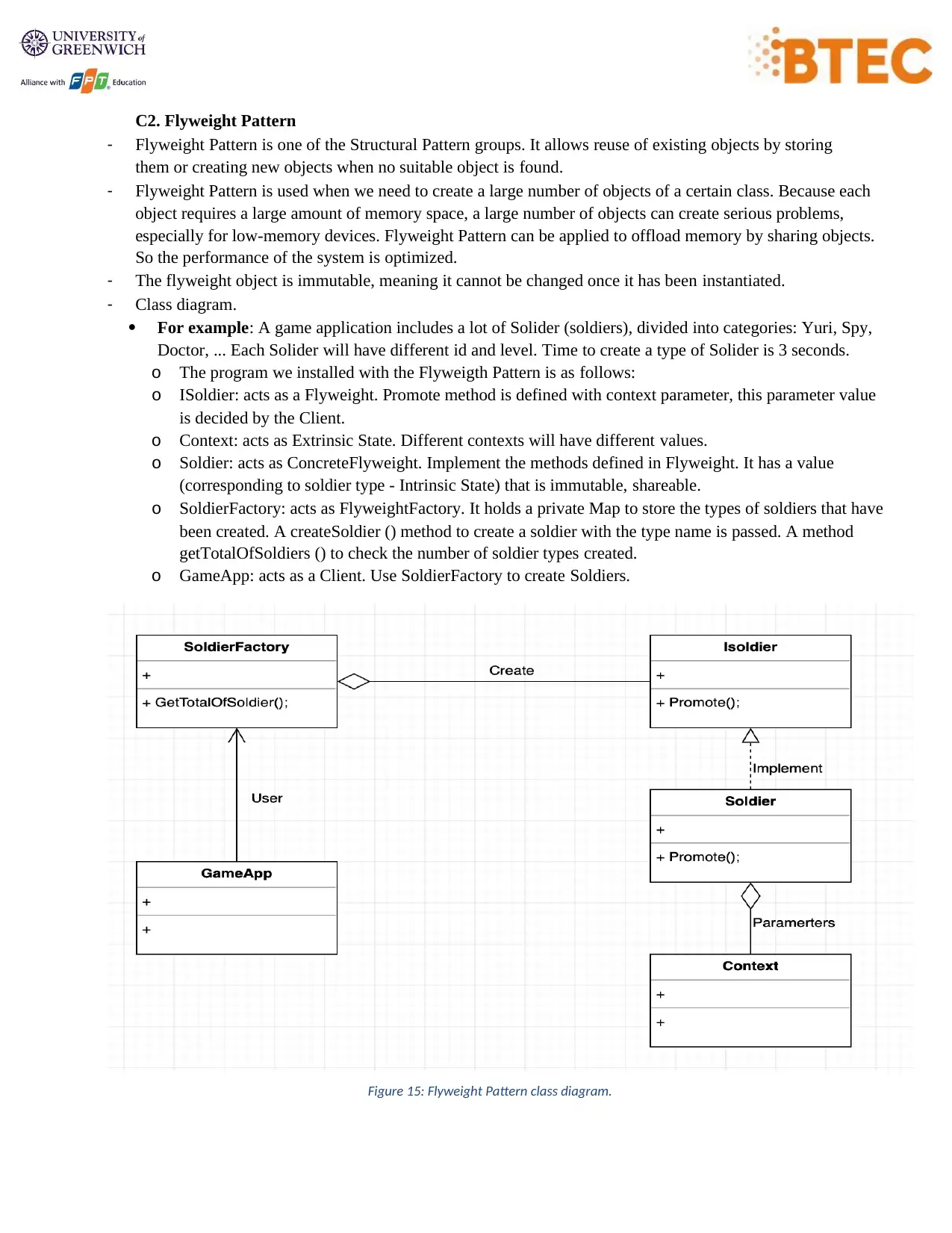
C2. Flyweight Pattern
- Flyweight Pattern is one of the Structural Pattern groups. It allows reuse of existing objects by storing
them or creating new objects when no suitable object is found.
- Flyweight Pattern is used when we need to create a large number of objects of a certain class. Because each
object requires a large amount of memory space, a large number of objects can create serious problems,
especially for low-memory devices. Flyweight Pattern can be applied to offload memory by sharing objects.
So the performance of the system is optimized.
- The flyweight object is immutable, meaning it cannot be changed once it has been instantiated.
- Class diagram.
For example: A game application includes a lot of Solider (soldiers), divided into categories: Yuri, Spy,
Doctor, ... Each Solider will have different id and level. Time to create a type of Solider is 3 seconds.
o The program we installed with the Flyweigth Pattern is as follows:
o ISoldier: acts as a Flyweight. Promote method is defined with context parameter, this parameter value
is decided by the Client.
o Context: acts as Extrinsic State. Different contexts will have different values.
o Soldier: acts as ConcreteFlyweight. Implement the methods defined in Flyweight. It has a value
(corresponding to soldier type - Intrinsic State) that is immutable, shareable.
o SoldierFactory: acts as FlyweightFactory. It holds a private Map to store the types of soldiers that have
been created. A createSoldier () method to create a soldier with the type name is passed. A method
getTotalOfSoldiers () to check the number of soldier types created.
o GameApp: acts as a Client. Use SoldierFactory to create Soldiers.
Figure 15: Flyweight Pattern class diagram.
- Flyweight Pattern is one of the Structural Pattern groups. It allows reuse of existing objects by storing
them or creating new objects when no suitable object is found.
- Flyweight Pattern is used when we need to create a large number of objects of a certain class. Because each
object requires a large amount of memory space, a large number of objects can create serious problems,
especially for low-memory devices. Flyweight Pattern can be applied to offload memory by sharing objects.
So the performance of the system is optimized.
- The flyweight object is immutable, meaning it cannot be changed once it has been instantiated.
- Class diagram.
For example: A game application includes a lot of Solider (soldiers), divided into categories: Yuri, Spy,
Doctor, ... Each Solider will have different id and level. Time to create a type of Solider is 3 seconds.
o The program we installed with the Flyweigth Pattern is as follows:
o ISoldier: acts as a Flyweight. Promote method is defined with context parameter, this parameter value
is decided by the Client.
o Context: acts as Extrinsic State. Different contexts will have different values.
o Soldier: acts as ConcreteFlyweight. Implement the methods defined in Flyweight. It has a value
(corresponding to soldier type - Intrinsic State) that is immutable, shareable.
o SoldierFactory: acts as FlyweightFactory. It holds a private Map to store the types of soldiers that have
been created. A createSoldier () method to create a soldier with the type name is passed. A method
getTotalOfSoldiers () to check the number of soldier types created.
o GameApp: acts as a Client. Use SoldierFactory to create Soldiers.
Figure 15: Flyweight Pattern class diagram.
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
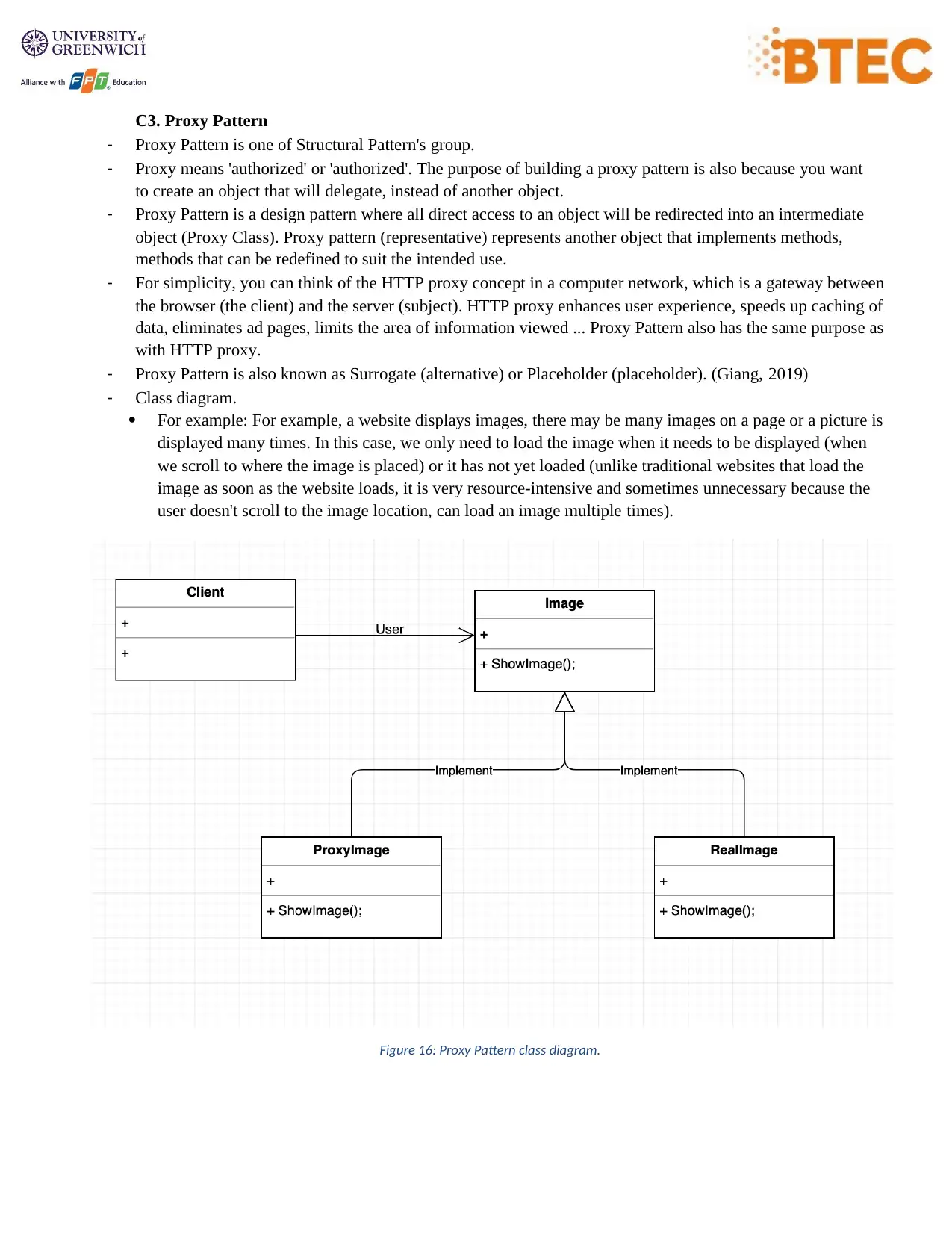
C3. Proxy Pattern
- Proxy Pattern is one of Structural Pattern's group.
- Proxy means 'authorized' or 'authorized'. The purpose of building a proxy pattern is also because you want
to create an object that will delegate, instead of another object.
- Proxy Pattern is a design pattern where all direct access to an object will be redirected into an intermediate
object (Proxy Class). Proxy pattern (representative) represents another object that implements methods,
methods that can be redefined to suit the intended use.
- For simplicity, you can think of the HTTP proxy concept in a computer network, which is a gateway between
the browser (the client) and the server (subject). HTTP proxy enhances user experience, speeds up caching of
data, eliminates ad pages, limits the area of information viewed ... Proxy Pattern also has the same purpose as
with HTTP proxy.
- Proxy Pattern is also known as Surrogate (alternative) or Placeholder (placeholder). (Giang, 2019)
- Class diagram.
For example: For example, a website displays images, there may be many images on a page or a picture is
displayed many times. In this case, we only need to load the image when it needs to be displayed (when
we scroll to where the image is placed) or it has not yet loaded (unlike traditional websites that load the
image as soon as the website loads, it is very resource-intensive and sometimes unnecessary because the
user doesn't scroll to the image location, can load an image multiple times).
Figure 16: Proxy Pattern class diagram.
- Proxy Pattern is one of Structural Pattern's group.
- Proxy means 'authorized' or 'authorized'. The purpose of building a proxy pattern is also because you want
to create an object that will delegate, instead of another object.
- Proxy Pattern is a design pattern where all direct access to an object will be redirected into an intermediate
object (Proxy Class). Proxy pattern (representative) represents another object that implements methods,
methods that can be redefined to suit the intended use.
- For simplicity, you can think of the HTTP proxy concept in a computer network, which is a gateway between
the browser (the client) and the server (subject). HTTP proxy enhances user experience, speeds up caching of
data, eliminates ad pages, limits the area of information viewed ... Proxy Pattern also has the same purpose as
with HTTP proxy.
- Proxy Pattern is also known as Surrogate (alternative) or Placeholder (placeholder). (Giang, 2019)
- Class diagram.
For example: For example, a website displays images, there may be many images on a page or a picture is
displayed many times. In this case, we only need to load the image when it needs to be displayed (when
we scroll to where the image is placed) or it has not yet loaded (unlike traditional websites that load the
image as soon as the website loads, it is very resource-intensive and sometimes unnecessary because the
user doesn't scroll to the image location, can load an image multiple times).
Figure 16: Proxy Pattern class diagram.
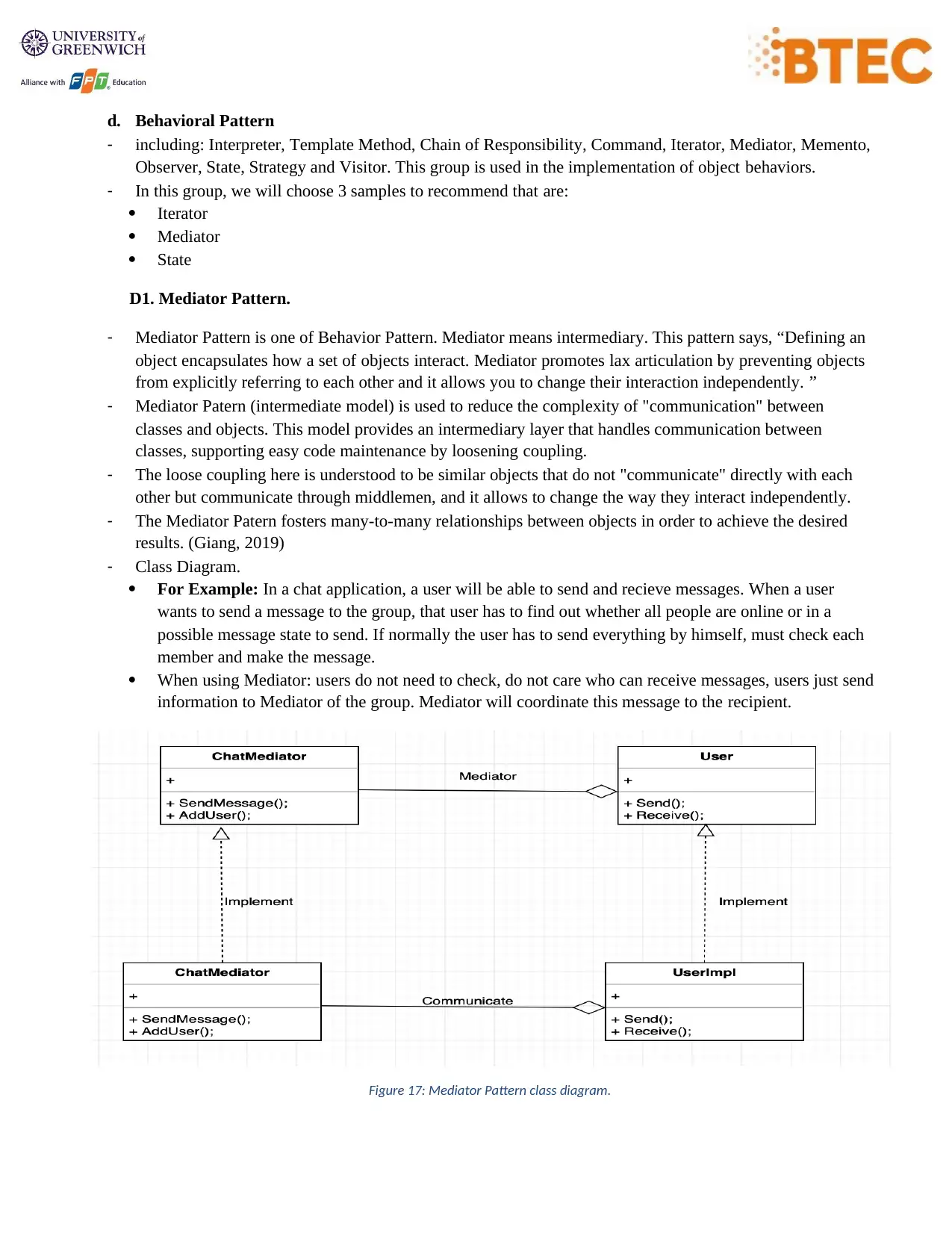
d. Behavioral Pattern
- including: Interpreter, Template Method, Chain of Responsibility, Command, Iterator, Mediator, Memento,
Observer, State, Strategy and Visitor. This group is used in the implementation of object behaviors.
- In this group, we will choose 3 samples to recommend that are:
Iterator
Mediator
State
D1. Mediator Pattern.
- Mediator Pattern is one of Behavior Pattern. Mediator means intermediary. This pattern says, “Defining an
object encapsulates how a set of objects interact. Mediator promotes lax articulation by preventing objects
from explicitly referring to each other and it allows you to change their interaction independently. ”
- Mediator Patern (intermediate model) is used to reduce the complexity of "communication" between
classes and objects. This model provides an intermediary layer that handles communication between
classes, supporting easy code maintenance by loosening coupling.
- The loose coupling here is understood to be similar objects that do not "communicate" directly with each
other but communicate through middlemen, and it allows to change the way they interact independently.
- The Mediator Patern fosters many-to-many relationships between objects in order to achieve the desired
results. (Giang, 2019)
- Class Diagram.
For Example: In a chat application, a user will be able to send and recieve messages. When a user
wants to send a message to the group, that user has to find out whether all people are online or in a
possible message state to send. If normally the user has to send everything by himself, must check each
member and make the message.
When using Mediator: users do not need to check, do not care who can receive messages, users just send
information to Mediator of the group. Mediator will coordinate this message to the recipient.
Figure 17: Mediator Pattern class diagram.
- including: Interpreter, Template Method, Chain of Responsibility, Command, Iterator, Mediator, Memento,
Observer, State, Strategy and Visitor. This group is used in the implementation of object behaviors.
- In this group, we will choose 3 samples to recommend that are:
Iterator
Mediator
State
D1. Mediator Pattern.
- Mediator Pattern is one of Behavior Pattern. Mediator means intermediary. This pattern says, “Defining an
object encapsulates how a set of objects interact. Mediator promotes lax articulation by preventing objects
from explicitly referring to each other and it allows you to change their interaction independently. ”
- Mediator Patern (intermediate model) is used to reduce the complexity of "communication" between
classes and objects. This model provides an intermediary layer that handles communication between
classes, supporting easy code maintenance by loosening coupling.
- The loose coupling here is understood to be similar objects that do not "communicate" directly with each
other but communicate through middlemen, and it allows to change the way they interact independently.
- The Mediator Patern fosters many-to-many relationships between objects in order to achieve the desired
results. (Giang, 2019)
- Class Diagram.
For Example: In a chat application, a user will be able to send and recieve messages. When a user
wants to send a message to the group, that user has to find out whether all people are online or in a
possible message state to send. If normally the user has to send everything by himself, must check each
member and make the message.
When using Mediator: users do not need to check, do not care who can receive messages, users just send
information to Mediator of the group. Mediator will coordinate this message to the recipient.
Figure 17: Mediator Pattern class diagram.
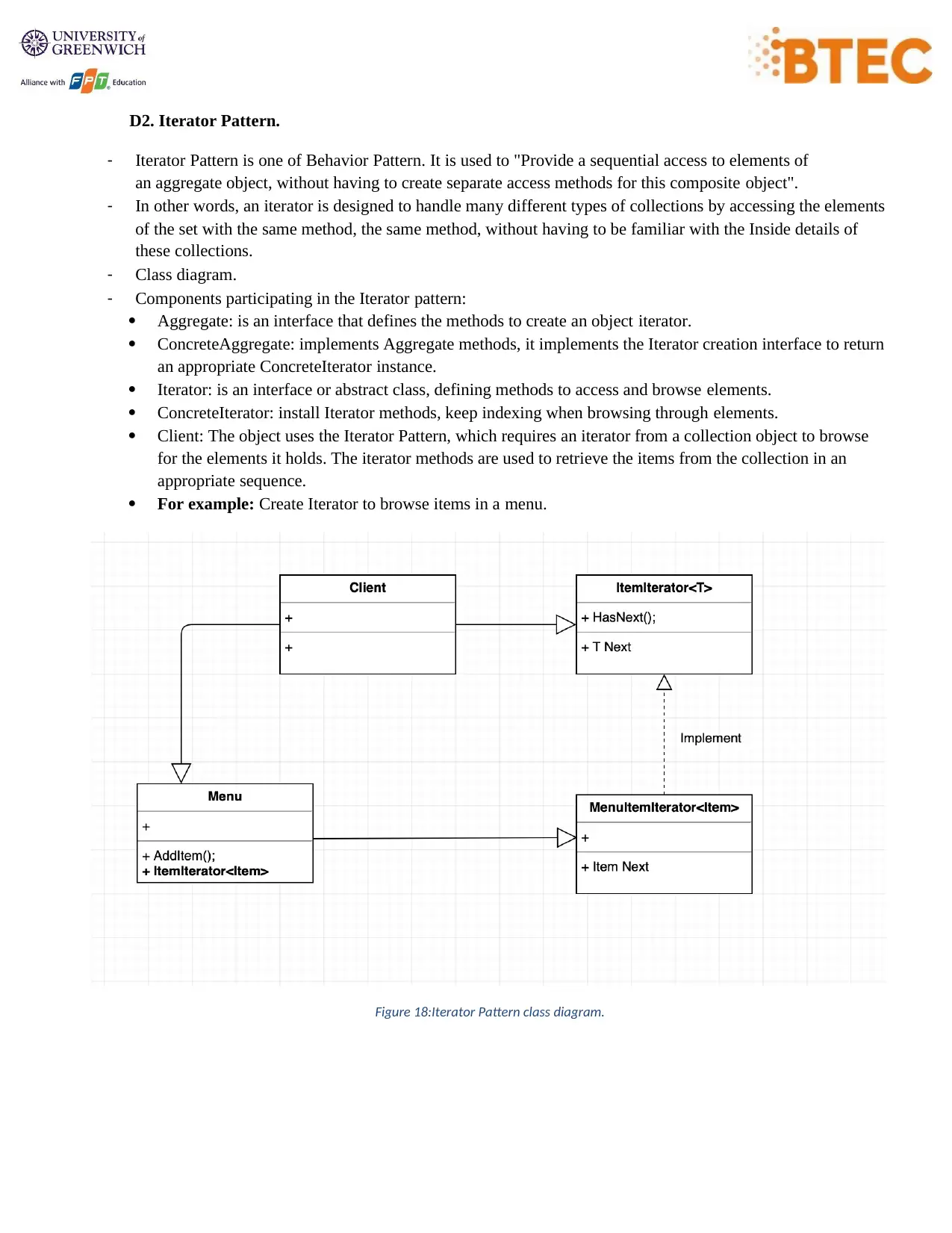
D2. Iterator Pattern.
- Iterator Pattern is one of Behavior Pattern. It is used to "Provide a sequential access to elements of
an aggregate object, without having to create separate access methods for this composite object".
- In other words, an iterator is designed to handle many different types of collections by accessing the elements
of the set with the same method, the same method, without having to be familiar with the Inside details of
these collections.
- Class diagram.
- Components participating in the Iterator pattern:
Aggregate: is an interface that defines the methods to create an object iterator.
ConcreteAggregate: implements Aggregate methods, it implements the Iterator creation interface to return
an appropriate ConcreteIterator instance.
Iterator: is an interface or abstract class, defining methods to access and browse elements.
ConcreteIterator: install Iterator methods, keep indexing when browsing through elements.
Client: The object uses the Iterator Pattern, which requires an iterator from a collection object to browse
for the elements it holds. The iterator methods are used to retrieve the items from the collection in an
appropriate sequence.
For example: Create Iterator to browse items in a menu.
Figure 18:Iterator Pattern class diagram.
- Iterator Pattern is one of Behavior Pattern. It is used to "Provide a sequential access to elements of
an aggregate object, without having to create separate access methods for this composite object".
- In other words, an iterator is designed to handle many different types of collections by accessing the elements
of the set with the same method, the same method, without having to be familiar with the Inside details of
these collections.
- Class diagram.
- Components participating in the Iterator pattern:
Aggregate: is an interface that defines the methods to create an object iterator.
ConcreteAggregate: implements Aggregate methods, it implements the Iterator creation interface to return
an appropriate ConcreteIterator instance.
Iterator: is an interface or abstract class, defining methods to access and browse elements.
ConcreteIterator: install Iterator methods, keep indexing when browsing through elements.
Client: The object uses the Iterator Pattern, which requires an iterator from a collection object to browse
for the elements it holds. The iterator methods are used to retrieve the items from the collection in an
appropriate sequence.
For example: Create Iterator to browse items in a menu.
Figure 18:Iterator Pattern class diagram.
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
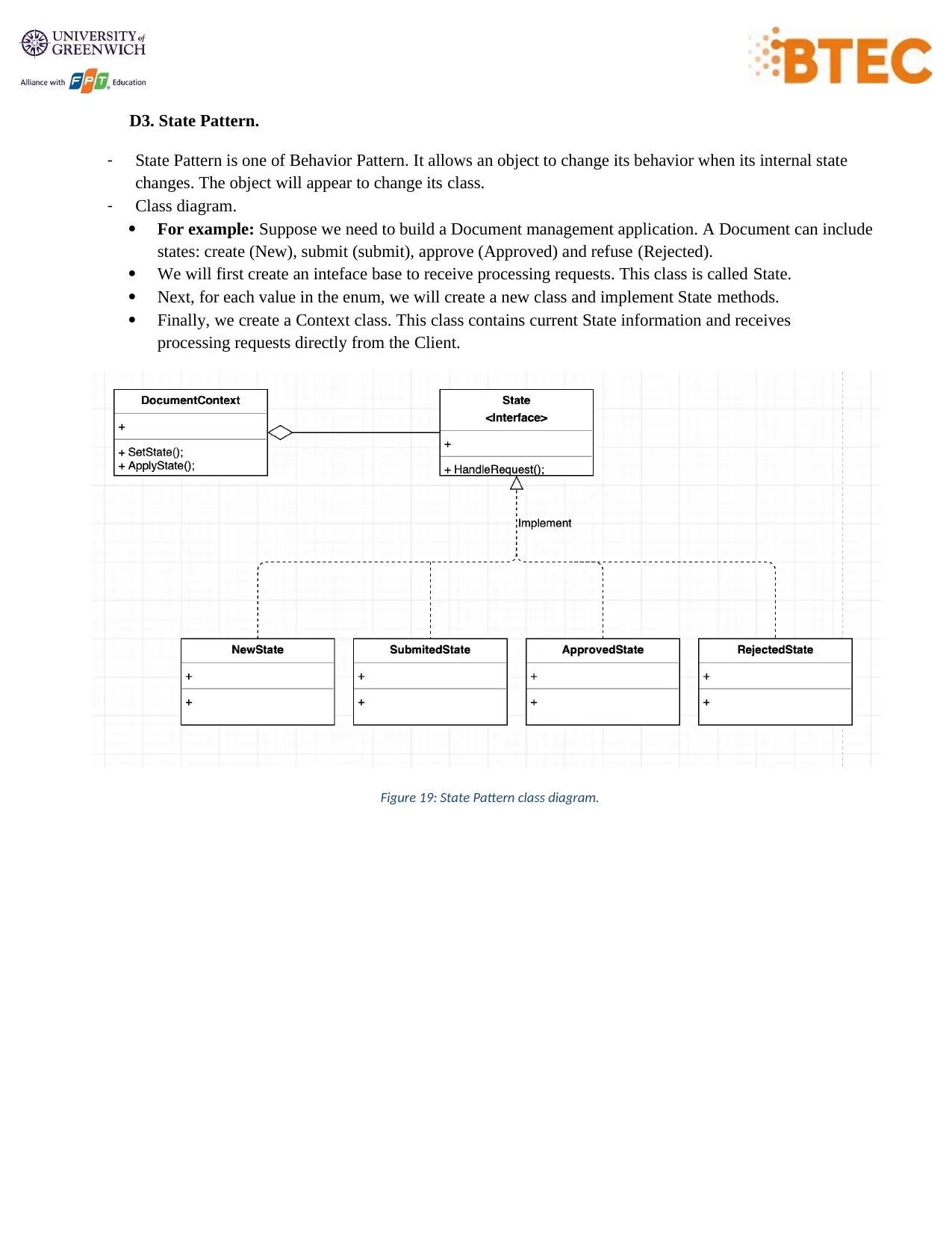
D3. State Pattern.
- State Pattern is one of Behavior Pattern. It allows an object to change its behavior when its internal state
changes. The object will appear to change its class.
- Class diagram.
For example: Suppose we need to build a Document management application. A Document can include
states: create (New), submit (submit), approve (Approved) and refuse (Rejected).
We will first create an inteface base to receive processing requests. This class is called State.
Next, for each value in the enum, we will create a new class and implement State methods.
Finally, we create a Context class. This class contains current State information and receives
processing requests directly from the Client.
Figure 19: State Pattern class diagram.
- State Pattern is one of Behavior Pattern. It allows an object to change its behavior when its internal state
changes. The object will appear to change its class.
- Class diagram.
For example: Suppose we need to build a Document management application. A Document can include
states: create (New), submit (submit), approve (Approved) and refuse (Rejected).
We will first create an inteface base to receive processing requests. This class is called State.
Next, for each value in the enum, we will create a new class and implement State methods.
Finally, we create a Context class. This class contains current State information and receives
processing requests directly from the Client.
Figure 19: State Pattern class diagram.
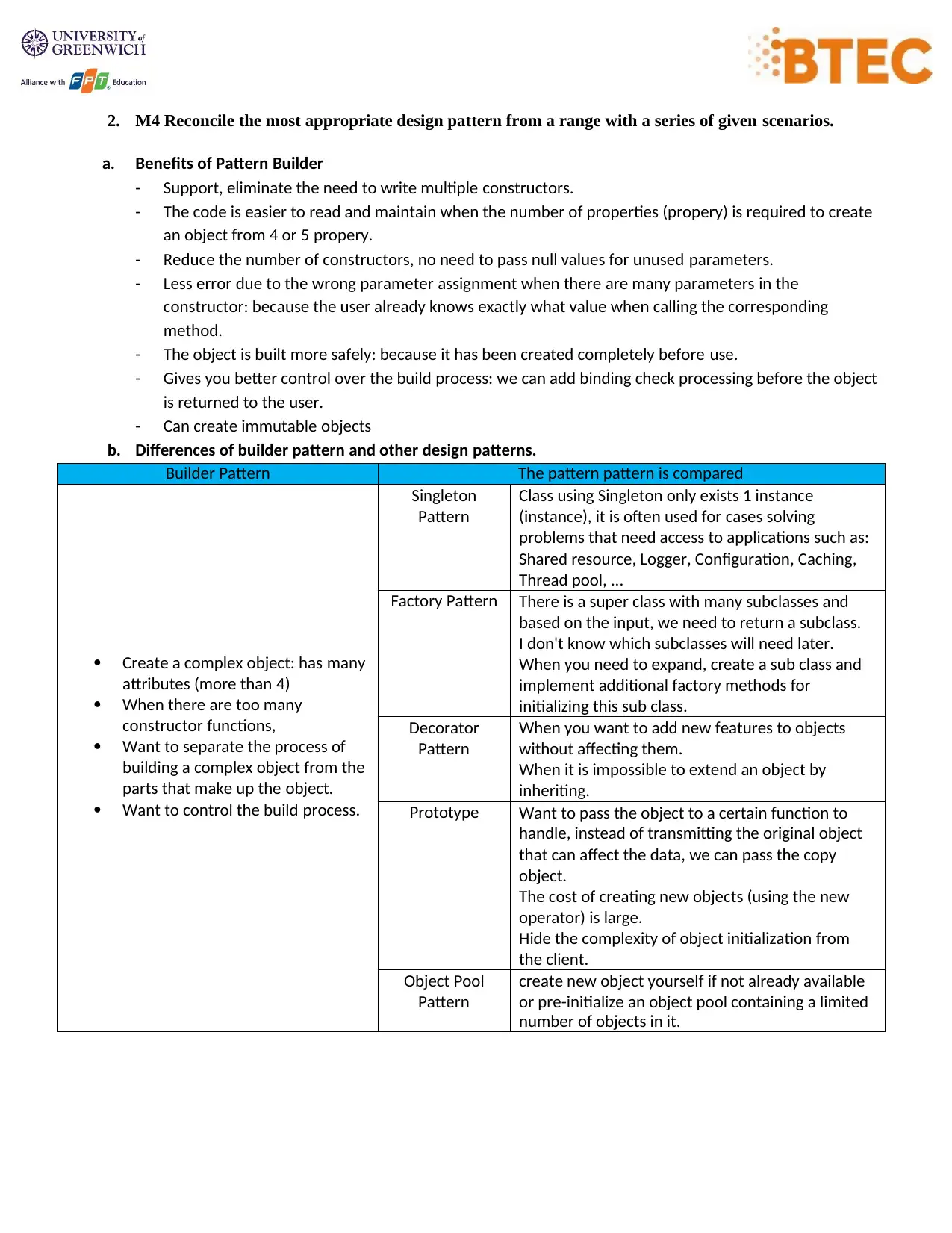
2. M4 Reconcile the most appropriate design pattern from a range with a series of given scenarios.
a. Benefits of Pattern Builder
- Support, eliminate the need to write multiple constructors.
- The code is easier to read and maintain when the number of properties (propery) is required to create
an object from 4 or 5 propery.
- Reduce the number of constructors, no need to pass null values for unused parameters.
- Less error due to the wrong parameter assignment when there are many parameters in the
constructor: because the user already knows exactly what value when calling the corresponding
method.
- The object is built more safely: because it has been created completely before use.
- Gives you better control over the build process: we can add binding check processing before the object
is returned to the user.
- Can create immutable objects
b. Differences of builder pattern and other design patterns.
Builder Pattern The pattern pattern is compared
Create a complex object: has many
attributes (more than 4)
When there are too many
constructor functions,
Want to separate the process of
building a complex object from the
parts that make up the object.
Want to control the build process.
Singleton
Pattern
Class using Singleton only exists 1 instance
(instance), it is often used for cases solving
problems that need access to applications such as:
Shared resource, Logger, Configuration, Caching,
Thread pool, ...
Factory Pattern There is a super class with many subclasses and
based on the input, we need to return a subclass.
I don't know which subclasses will need later.
When you need to expand, create a sub class and
implement additional factory methods for
initializing this sub class.
Decorator
Pattern
When you want to add new features to objects
without affecting them.
When it is impossible to extend an object by
inheriting.
Prototype Want to pass the object to a certain function to
handle, instead of transmitting the original object
that can affect the data, we can pass the copy
object.
The cost of creating new objects (using the new
operator) is large.
Hide the complexity of object initialization from
the client.
Object Pool
Pattern
create new object yourself if not already available
or pre-initialize an object pool containing a limited
number of objects in it.
a. Benefits of Pattern Builder
- Support, eliminate the need to write multiple constructors.
- The code is easier to read and maintain when the number of properties (propery) is required to create
an object from 4 or 5 propery.
- Reduce the number of constructors, no need to pass null values for unused parameters.
- Less error due to the wrong parameter assignment when there are many parameters in the
constructor: because the user already knows exactly what value when calling the corresponding
method.
- The object is built more safely: because it has been created completely before use.
- Gives you better control over the build process: we can add binding check processing before the object
is returned to the user.
- Can create immutable objects
b. Differences of builder pattern and other design patterns.
Builder Pattern The pattern pattern is compared
Create a complex object: has many
attributes (more than 4)
When there are too many
constructor functions,
Want to separate the process of
building a complex object from the
parts that make up the object.
Want to control the build process.
Singleton
Pattern
Class using Singleton only exists 1 instance
(instance), it is often used for cases solving
problems that need access to applications such as:
Shared resource, Logger, Configuration, Caching,
Thread pool, ...
Factory Pattern There is a super class with many subclasses and
based on the input, we need to return a subclass.
I don't know which subclasses will need later.
When you need to expand, create a sub class and
implement additional factory methods for
initializing this sub class.
Decorator
Pattern
When you want to add new features to objects
without affecting them.
When it is impossible to extend an object by
inheriting.
Prototype Want to pass the object to a certain function to
handle, instead of transmitting the original object
that can affect the data, we can pass the copy
object.
The cost of creating new objects (using the new
operator) is large.
Hide the complexity of object initialization from
the client.
Object Pool
Pattern
create new object yourself if not already available
or pre-initialize an object pool containing a limited
number of objects in it.
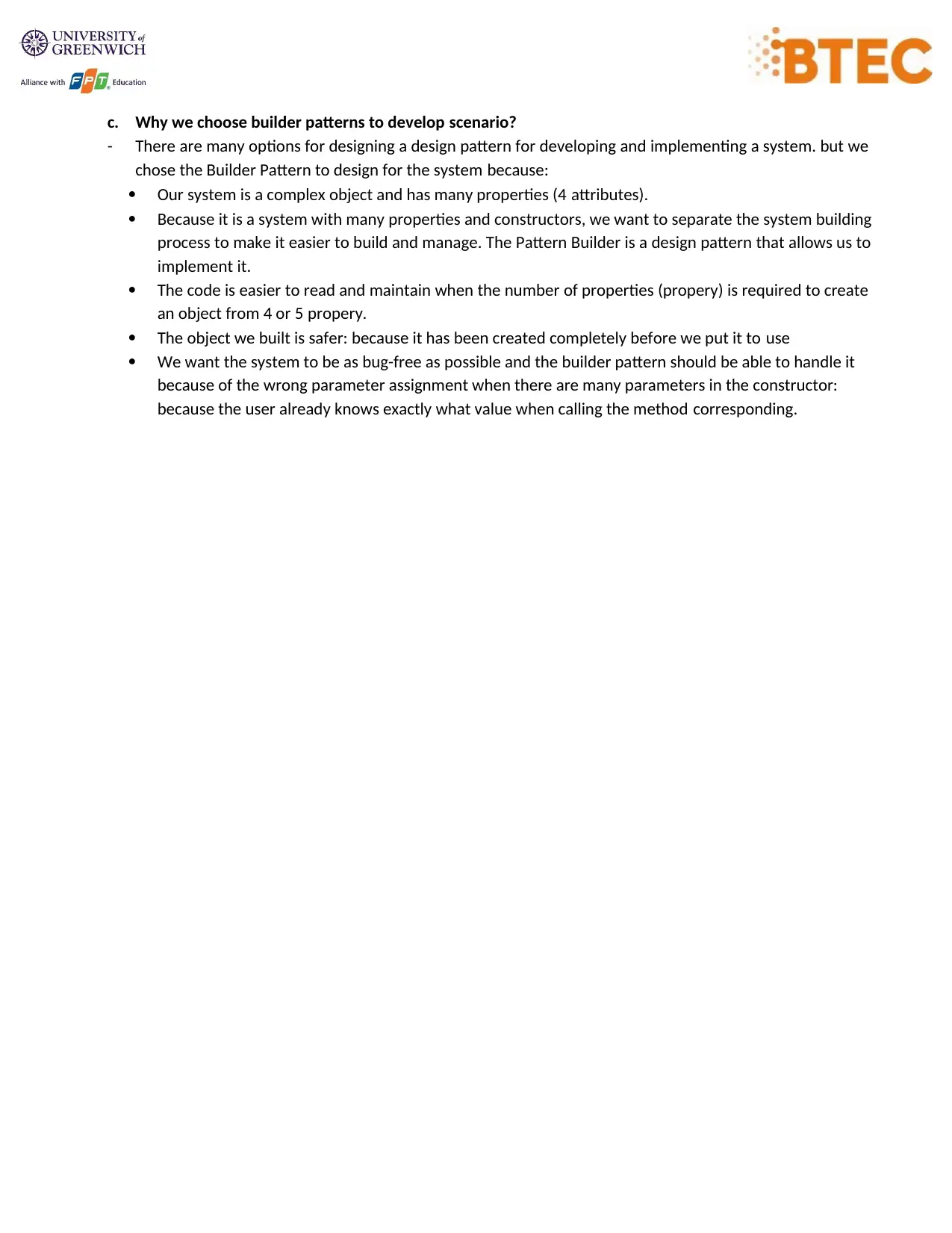
c. Why we choose builder patterns to develop scenario?
- There are many options for designing a design pattern for developing and implementing a system. but we
chose the Builder Pattern to design for the system because:
Our system is a complex object and has many properties (4 attributes).
Because it is a system with many properties and constructors, we want to separate the system building
process to make it easier to build and manage. The Pattern Builder is a design pattern that allows us to
implement it.
The code is easier to read and maintain when the number of properties (propery) is required to create
an object from 4 or 5 propery.
The object we built is safer: because it has been created completely before we put it to use
We want the system to be as bug-free as possible and the builder pattern should be able to handle it
because of the wrong parameter assignment when there are many parameters in the constructor:
because the user already knows exactly what value when calling the method corresponding.
- There are many options for designing a design pattern for developing and implementing a system. but we
chose the Builder Pattern to design for the system because:
Our system is a complex object and has many properties (4 attributes).
Because it is a system with many properties and constructors, we want to separate the system building
process to make it easier to build and manage. The Pattern Builder is a design pattern that allows us to
implement it.
The code is easier to read and maintain when the number of properties (propery) is required to create
an object from 4 or 5 propery.
The object we built is safer: because it has been created completely before we put it to use
We want the system to be as bug-free as possible and the builder pattern should be able to handle it
because of the wrong parameter assignment when there are many parameters in the constructor:
because the user already knows exactly what value when calling the method corresponding.
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
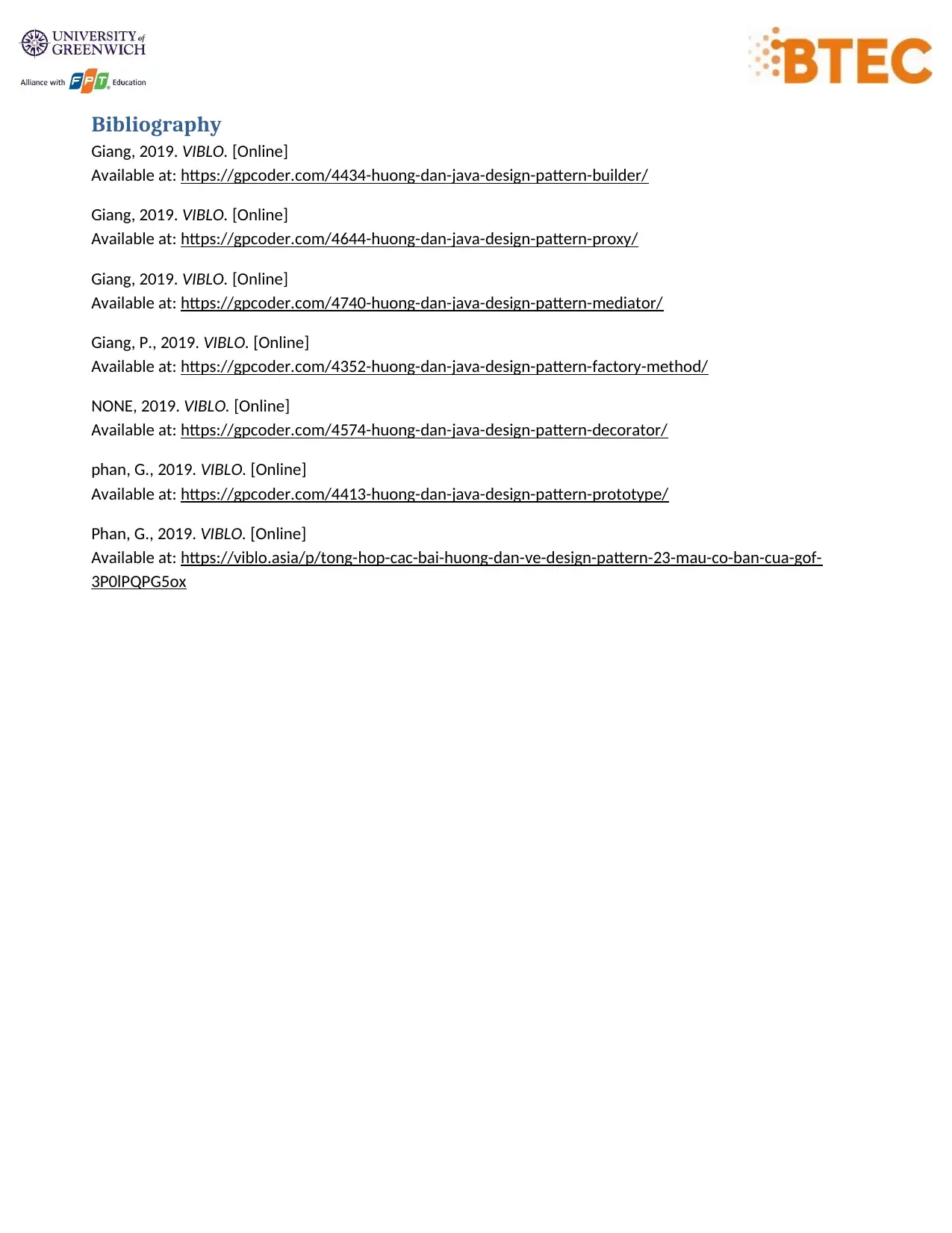
Bibliography
Giang, 2019. VIBLO. [Online]
Available at: https://gpcoder.com/4434-huong-dan-java-design-pattern-builder/
Giang, 2019. VIBLO. [Online]
Available at: https://gpcoder.com/4644-huong-dan-java-design-pattern-proxy/
Giang, 2019. VIBLO. [Online]
Available at: https://gpcoder.com/4740-huong-dan-java-design-pattern-mediator/
Giang, P., 2019. VIBLO. [Online]
Available at: https://gpcoder.com/4352-huong-dan-java-design-pattern-factory-method/
NONE, 2019. VIBLO. [Online]
Available at: https://gpcoder.com/4574-huong-dan-java-design-pattern-decorator/
phan, G., 2019. VIBLO. [Online]
Available at: https://gpcoder.com/4413-huong-dan-java-design-pattern-prototype/
Phan, G., 2019. VIBLO. [Online]
Available at: https://viblo.asia/p/tong-hop-cac-bai-huong-dan-ve-design-pattern-23-mau-co-ban-cua-gof-
3P0lPQPG5ox
Giang, 2019. VIBLO. [Online]
Available at: https://gpcoder.com/4434-huong-dan-java-design-pattern-builder/
Giang, 2019. VIBLO. [Online]
Available at: https://gpcoder.com/4644-huong-dan-java-design-pattern-proxy/
Giang, 2019. VIBLO. [Online]
Available at: https://gpcoder.com/4740-huong-dan-java-design-pattern-mediator/
Giang, P., 2019. VIBLO. [Online]
Available at: https://gpcoder.com/4352-huong-dan-java-design-pattern-factory-method/
NONE, 2019. VIBLO. [Online]
Available at: https://gpcoder.com/4574-huong-dan-java-design-pattern-decorator/
phan, G., 2019. VIBLO. [Online]
Available at: https://gpcoder.com/4413-huong-dan-java-design-pattern-prototype/
Phan, G., 2019. VIBLO. [Online]
Available at: https://viblo.asia/p/tong-hop-cac-bai-huong-dan-ve-design-pattern-23-mau-co-ban-cua-gof-
3P0lPQPG5ox
1 out of 26
Related Documents

Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.