ENEX 20001 Assignment 2: Solutions for C Programming Tasks, Flowcharts
VerifiedAdded on 2022/10/06
|19
|2541
|16
Homework Assignment
AI Summary
This document presents solutions for ENEX 20001 Assignment 2, focusing on C programming tasks for hardware applications. The solutions include code snippets, flowcharts, and detailed explanations of the algorithms used. The assignment covers several key areas, including password validation using a keypad, distance measurement with an ultrasonic sensor, and control logic for water level management. The programs are designed to work on a CQU PIC Development board using MPLABX IDE with the MCC18 C compiler. The document also includes a solution for calculating the binomial coefficient, demonstrating the application of recursive functions and input validation. The solutions are presented with clear instructions, comments, and screenshots to aid understanding. Students can use this resource to study and understand the requirements of the assignment.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
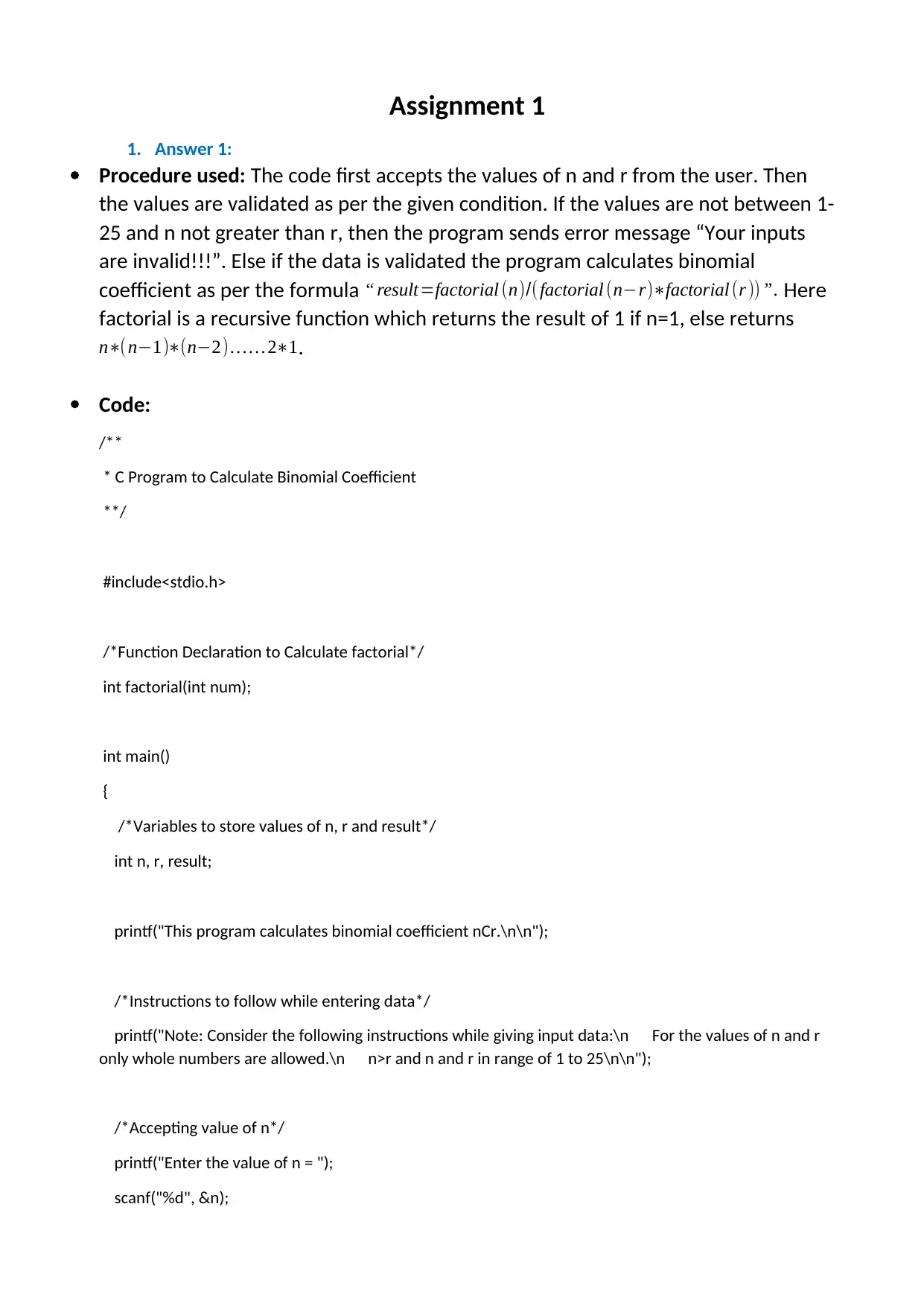
Assignment 1
1. Answer 1:
Procedure used: The code first accepts the values of n and r from the user. Then
the values are validated as per the given condition. If the values are not between 1-
25 and n not greater than r, then the program sends error message “Your inputs
are invalid!!!”. Else if the data is validated the program calculates binomial
coefficient as per the formula “ result=factorial (n)/(factorial (n−r)∗factorial (r ))”. Here
factorial is a recursive function which returns the result of 1 if n=1, else returns
n∗(n−1)∗(n−2) … …2∗1.
Code:
/**
* C Program to Calculate Binomial Coefficient
**/
#include<stdio.h>
/*Function Declaration to Calculate factorial*/
int factorial(int num);
int main()
{
/*Variables to store values of n, r and result*/
int n, r, result;
printf("This program calculates binomial coefficient nCr.\n\n");
/*Instructions to follow while entering data*/
printf("Note: Consider the following instructions while giving input data:\n For the values of n and r
only whole numbers are allowed.\n n>r and n and r in range of 1 to 25\n\n");
/*Accepting value of n*/
printf("Enter the value of n = ");
scanf("%d", &n);
1. Answer 1:
Procedure used: The code first accepts the values of n and r from the user. Then
the values are validated as per the given condition. If the values are not between 1-
25 and n not greater than r, then the program sends error message “Your inputs
are invalid!!!”. Else if the data is validated the program calculates binomial
coefficient as per the formula “ result=factorial (n)/(factorial (n−r)∗factorial (r ))”. Here
factorial is a recursive function which returns the result of 1 if n=1, else returns
n∗(n−1)∗(n−2) … …2∗1.
Code:
/**
* C Program to Calculate Binomial Coefficient
**/
#include<stdio.h>
/*Function Declaration to Calculate factorial*/
int factorial(int num);
int main()
{
/*Variables to store values of n, r and result*/
int n, r, result;
printf("This program calculates binomial coefficient nCr.\n\n");
/*Instructions to follow while entering data*/
printf("Note: Consider the following instructions while giving input data:\n For the values of n and r
only whole numbers are allowed.\n n>r and n and r in range of 1 to 25\n\n");
/*Accepting value of n*/
printf("Enter the value of n = ");
scanf("%d", &n);
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
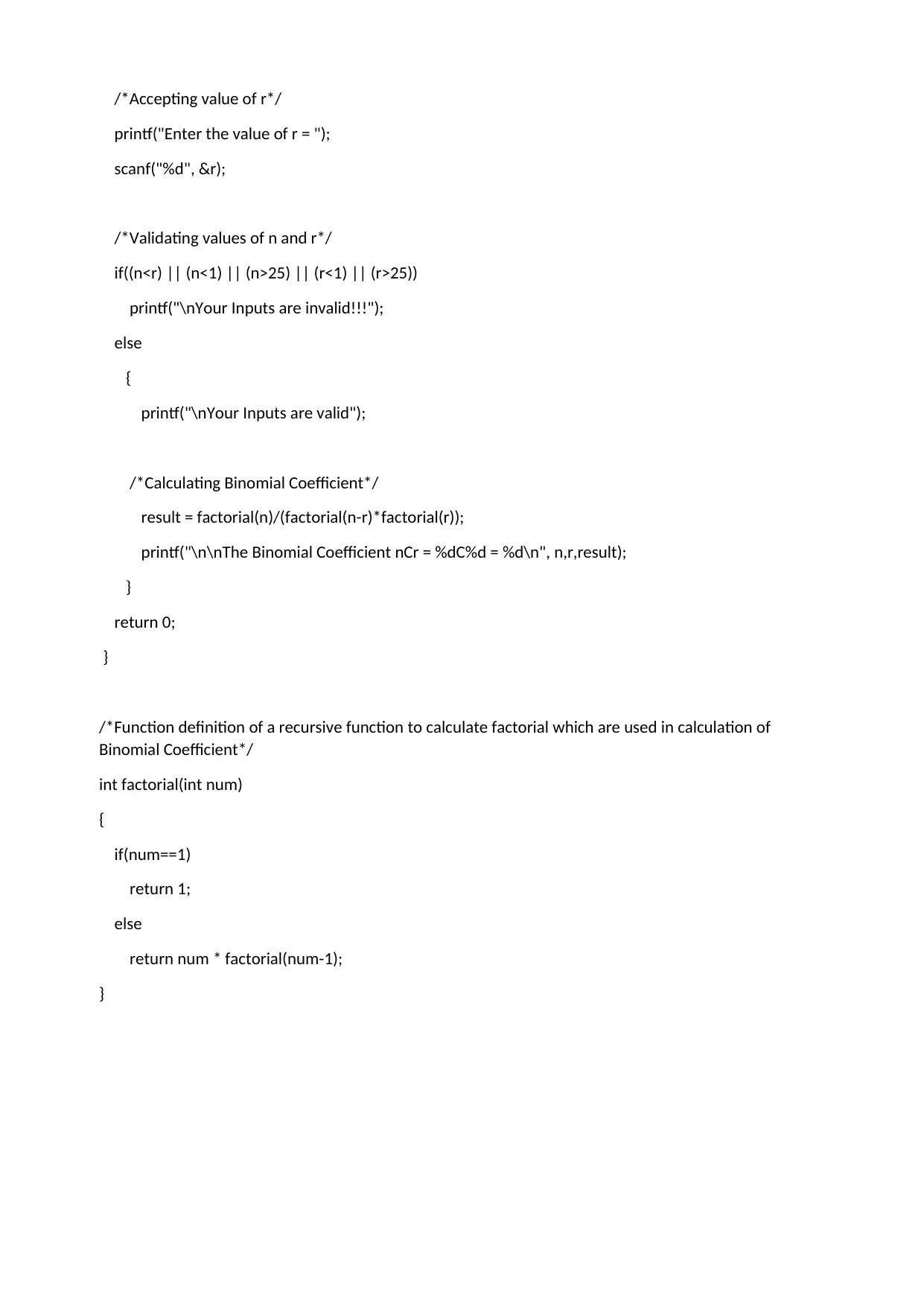
/*Accepting value of r*/
printf("Enter the value of r = ");
scanf("%d", &r);
/*Validating values of n and r*/
if((n<r) || (n<1) || (n>25) || (r<1) || (r>25))
printf("\nYour Inputs are invalid!!!");
else
{
printf("\nYour Inputs are valid");
/*Calculating Binomial Coefficient*/
result = factorial(n)/(factorial(n-r)*factorial(r));
printf("\n\nThe Binomial Coefficient nCr = %dC%d = %d\n", n,r,result);
}
return 0;
}
/*Function definition of a recursive function to calculate factorial which are used in calculation of
Binomial Coefficient*/
int factorial(int num)
{
if(num==1)
return 1;
else
return num * factorial(num-1);
}
printf("Enter the value of r = ");
scanf("%d", &r);
/*Validating values of n and r*/
if((n<r) || (n<1) || (n>25) || (r<1) || (r>25))
printf("\nYour Inputs are invalid!!!");
else
{
printf("\nYour Inputs are valid");
/*Calculating Binomial Coefficient*/
result = factorial(n)/(factorial(n-r)*factorial(r));
printf("\n\nThe Binomial Coefficient nCr = %dC%d = %d\n", n,r,result);
}
return 0;
}
/*Function definition of a recursive function to calculate factorial which are used in calculation of
Binomial Coefficient*/
int factorial(int num)
{
if(num==1)
return 1;
else
return num * factorial(num-1);
}
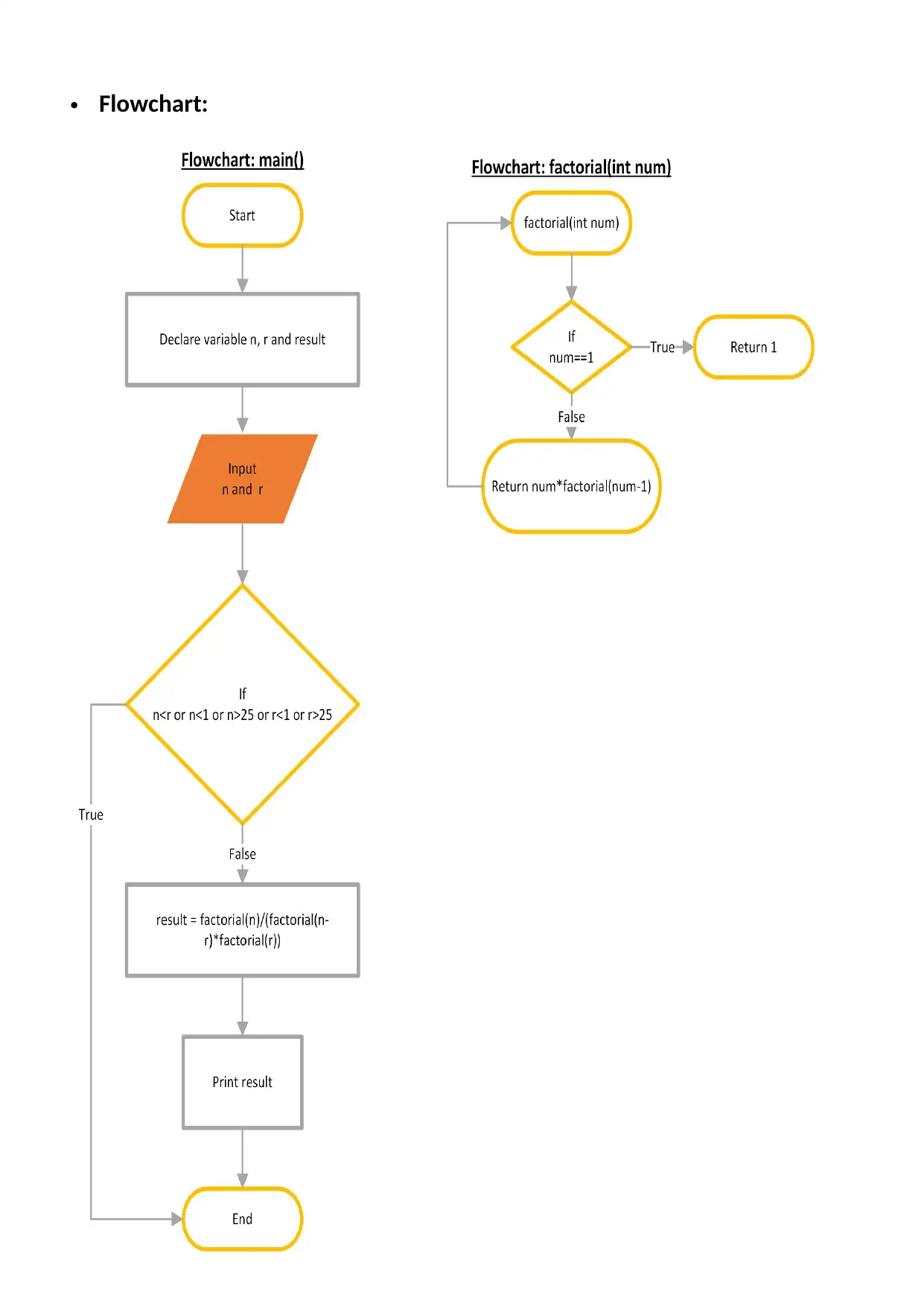
Flowchart:
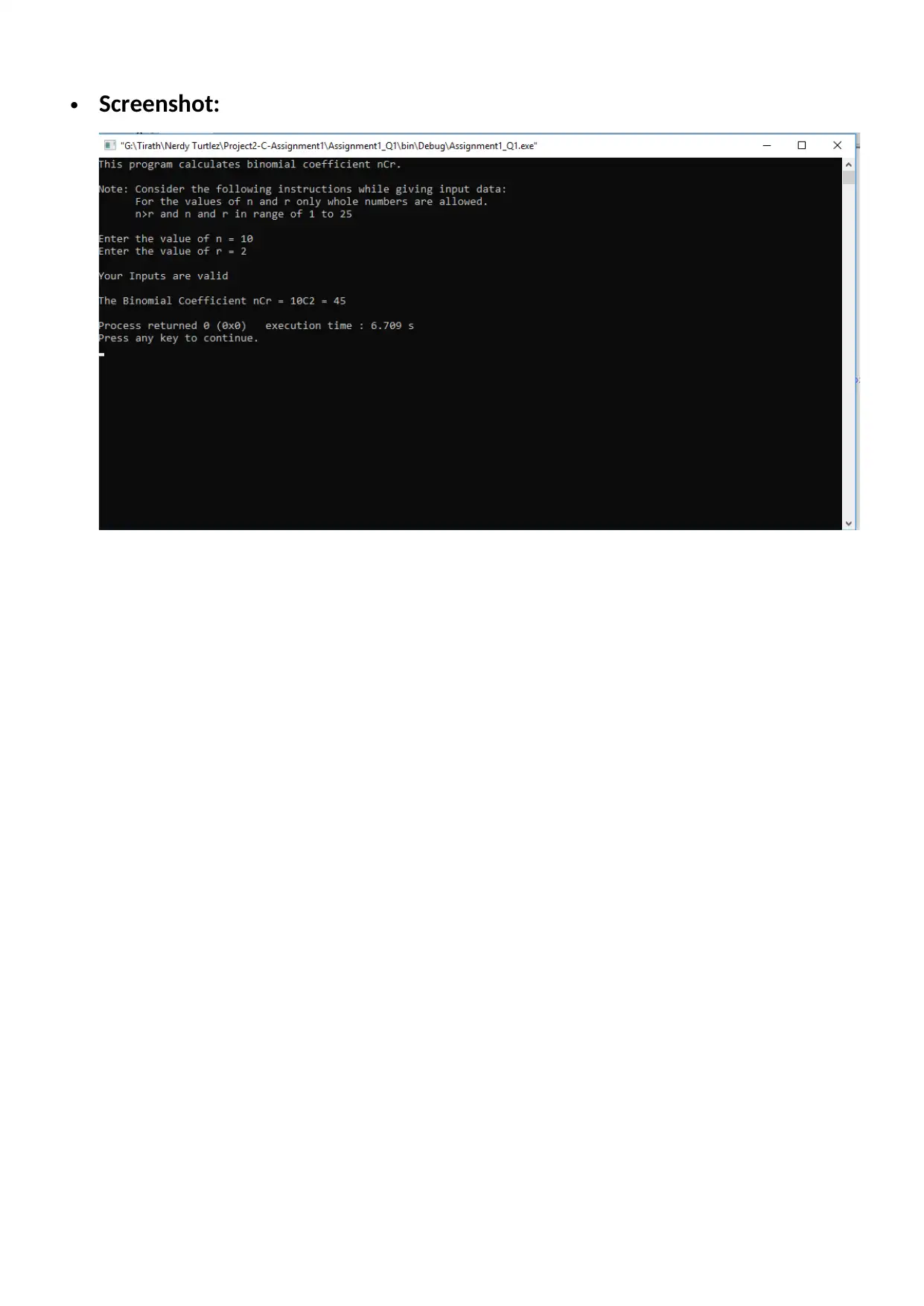
Screenshot:
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
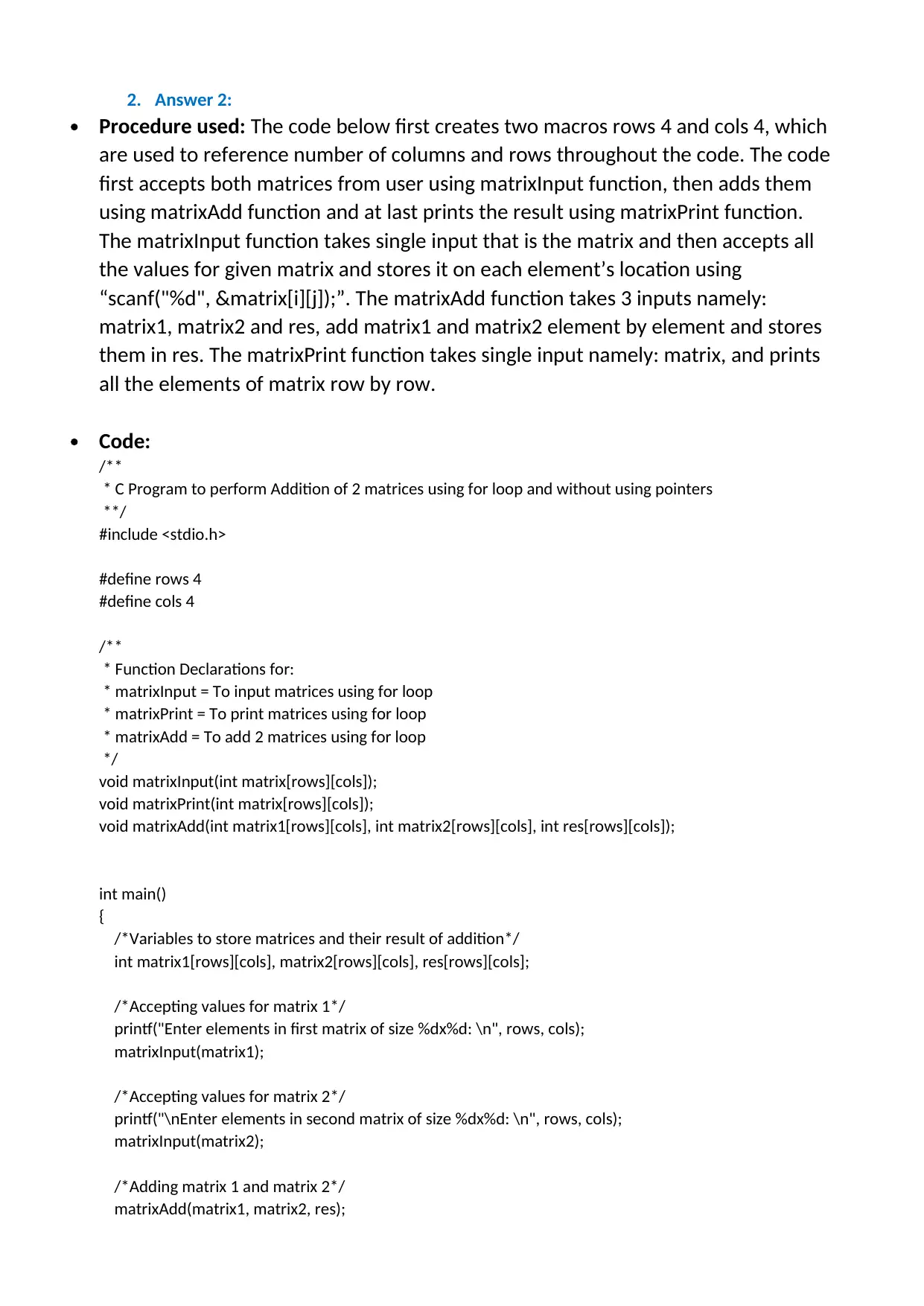
2. Answer 2:
Procedure used: The code below first creates two macros rows 4 and cols 4, which
are used to reference number of columns and rows throughout the code. The code
first accepts both matrices from user using matrixInput function, then adds them
using matrixAdd function and at last prints the result using matrixPrint function.
The matrixInput function takes single input that is the matrix and then accepts all
the values for given matrix and stores it on each element’s location using
“scanf("%d", &matrix[i][j]);”. The matrixAdd function takes 3 inputs namely:
matrix1, matrix2 and res, add matrix1 and matrix2 element by element and stores
them in res. The matrixPrint function takes single input namely: matrix, and prints
all the elements of matrix row by row.
Code:
/**
* C Program to perform Addition of 2 matrices using for loop and without using pointers
**/
#include <stdio.h>
#define rows 4
#define cols 4
/**
* Function Declarations for:
* matrixInput = To input matrices using for loop
* matrixPrint = To print matrices using for loop
* matrixAdd = To add 2 matrices using for loop
*/
void matrixInput(int matrix[rows][cols]);
void matrixPrint(int matrix[rows][cols]);
void matrixAdd(int matrix1[rows][cols], int matrix2[rows][cols], int res[rows][cols]);
int main()
{
/*Variables to store matrices and their result of addition*/
int matrix1[rows][cols], matrix2[rows][cols], res[rows][cols];
/*Accepting values for matrix 1*/
printf("Enter elements in first matrix of size %dx%d: \n", rows, cols);
matrixInput(matrix1);
/*Accepting values for matrix 2*/
printf("\nEnter elements in second matrix of size %dx%d: \n", rows, cols);
matrixInput(matrix2);
/*Adding matrix 1 and matrix 2*/
matrixAdd(matrix1, matrix2, res);
Procedure used: The code below first creates two macros rows 4 and cols 4, which
are used to reference number of columns and rows throughout the code. The code
first accepts both matrices from user using matrixInput function, then adds them
using matrixAdd function and at last prints the result using matrixPrint function.
The matrixInput function takes single input that is the matrix and then accepts all
the values for given matrix and stores it on each element’s location using
“scanf("%d", &matrix[i][j]);”. The matrixAdd function takes 3 inputs namely:
matrix1, matrix2 and res, add matrix1 and matrix2 element by element and stores
them in res. The matrixPrint function takes single input namely: matrix, and prints
all the elements of matrix row by row.
Code:
/**
* C Program to perform Addition of 2 matrices using for loop and without using pointers
**/
#include <stdio.h>
#define rows 4
#define cols 4
/**
* Function Declarations for:
* matrixInput = To input matrices using for loop
* matrixPrint = To print matrices using for loop
* matrixAdd = To add 2 matrices using for loop
*/
void matrixInput(int matrix[rows][cols]);
void matrixPrint(int matrix[rows][cols]);
void matrixAdd(int matrix1[rows][cols], int matrix2[rows][cols], int res[rows][cols]);
int main()
{
/*Variables to store matrices and their result of addition*/
int matrix1[rows][cols], matrix2[rows][cols], res[rows][cols];
/*Accepting values for matrix 1*/
printf("Enter elements in first matrix of size %dx%d: \n", rows, cols);
matrixInput(matrix1);
/*Accepting values for matrix 2*/
printf("\nEnter elements in second matrix of size %dx%d: \n", rows, cols);
matrixInput(matrix2);
/*Adding matrix 1 and matrix 2*/
matrixAdd(matrix1, matrix2, res);
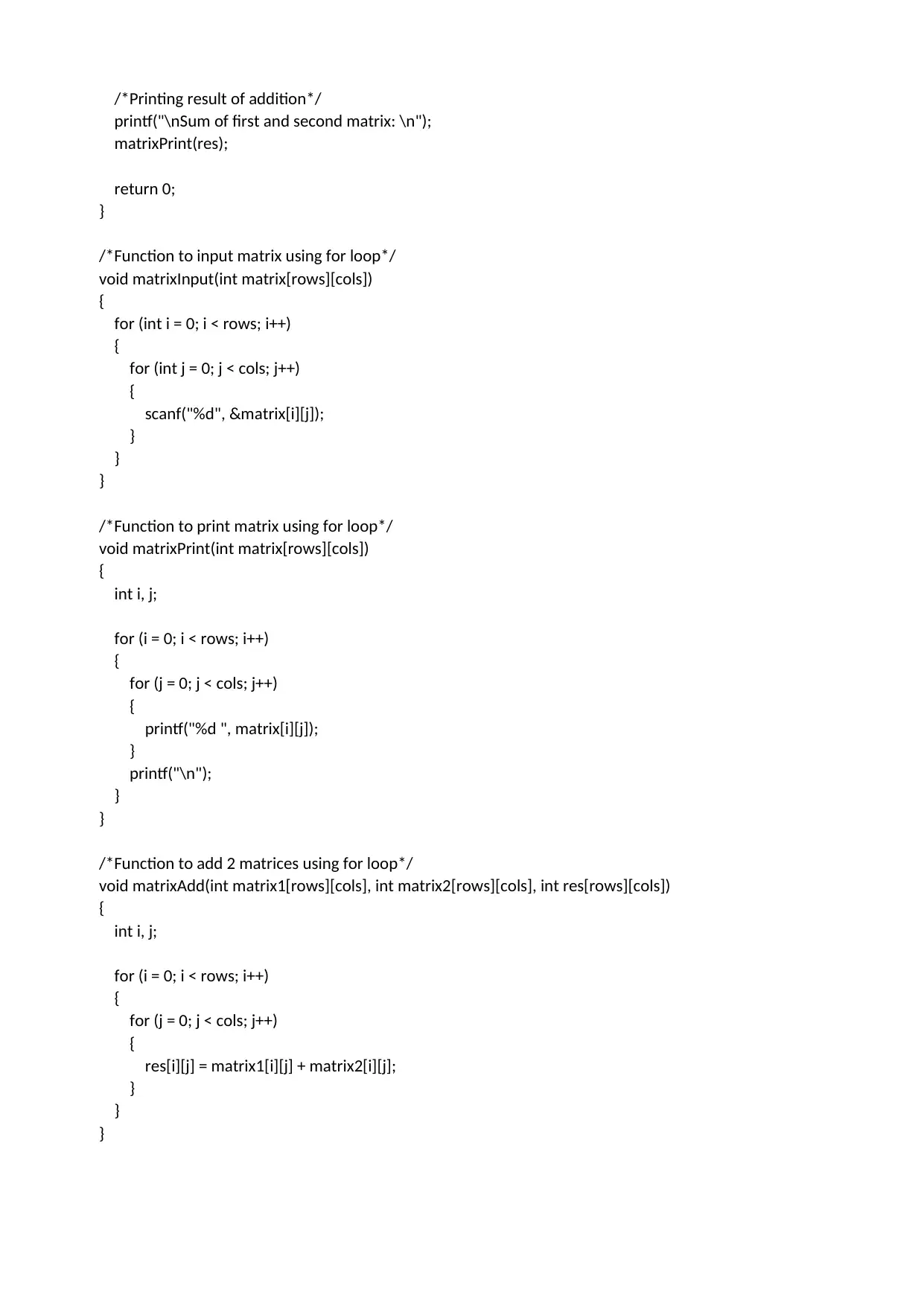
/*Printing result of addition*/
printf("\nSum of first and second matrix: \n");
matrixPrint(res);
return 0;
}
/*Function to input matrix using for loop*/
void matrixInput(int matrix[rows][cols])
{
for (int i = 0; i < rows; i++)
{
for (int j = 0; j < cols; j++)
{
scanf("%d", &matrix[i][j]);
}
}
}
/*Function to print matrix using for loop*/
void matrixPrint(int matrix[rows][cols])
{
int i, j;
for (i = 0; i < rows; i++)
{
for (j = 0; j < cols; j++)
{
printf("%d ", matrix[i][j]);
}
printf("\n");
}
}
/*Function to add 2 matrices using for loop*/
void matrixAdd(int matrix1[rows][cols], int matrix2[rows][cols], int res[rows][cols])
{
int i, j;
for (i = 0; i < rows; i++)
{
for (j = 0; j < cols; j++)
{
res[i][j] = matrix1[i][j] + matrix2[i][j];
}
}
}
printf("\nSum of first and second matrix: \n");
matrixPrint(res);
return 0;
}
/*Function to input matrix using for loop*/
void matrixInput(int matrix[rows][cols])
{
for (int i = 0; i < rows; i++)
{
for (int j = 0; j < cols; j++)
{
scanf("%d", &matrix[i][j]);
}
}
}
/*Function to print matrix using for loop*/
void matrixPrint(int matrix[rows][cols])
{
int i, j;
for (i = 0; i < rows; i++)
{
for (j = 0; j < cols; j++)
{
printf("%d ", matrix[i][j]);
}
printf("\n");
}
}
/*Function to add 2 matrices using for loop*/
void matrixAdd(int matrix1[rows][cols], int matrix2[rows][cols], int res[rows][cols])
{
int i, j;
for (i = 0; i < rows; i++)
{
for (j = 0; j < cols; j++)
{
res[i][j] = matrix1[i][j] + matrix2[i][j];
}
}
}
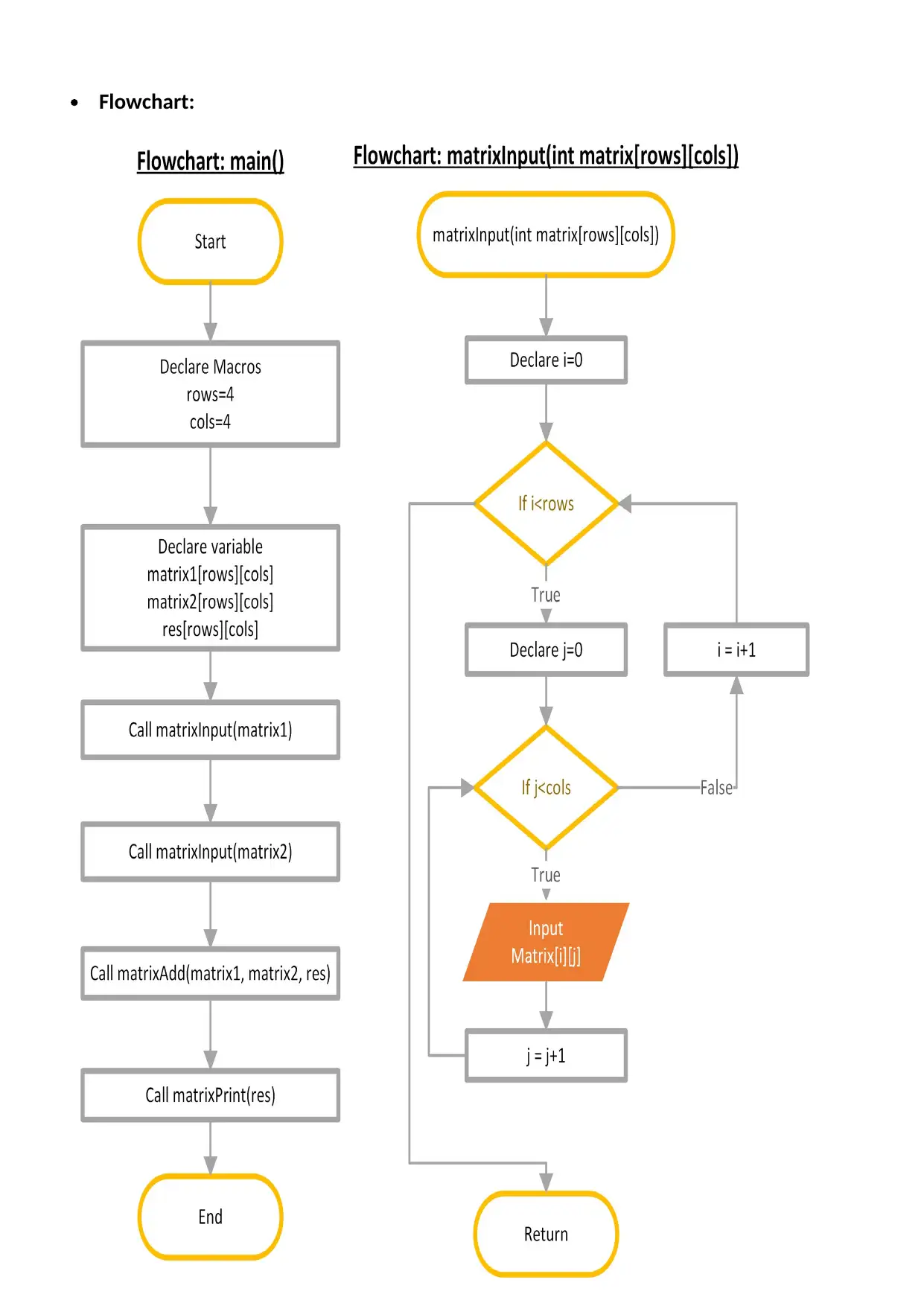
Flowchart:
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
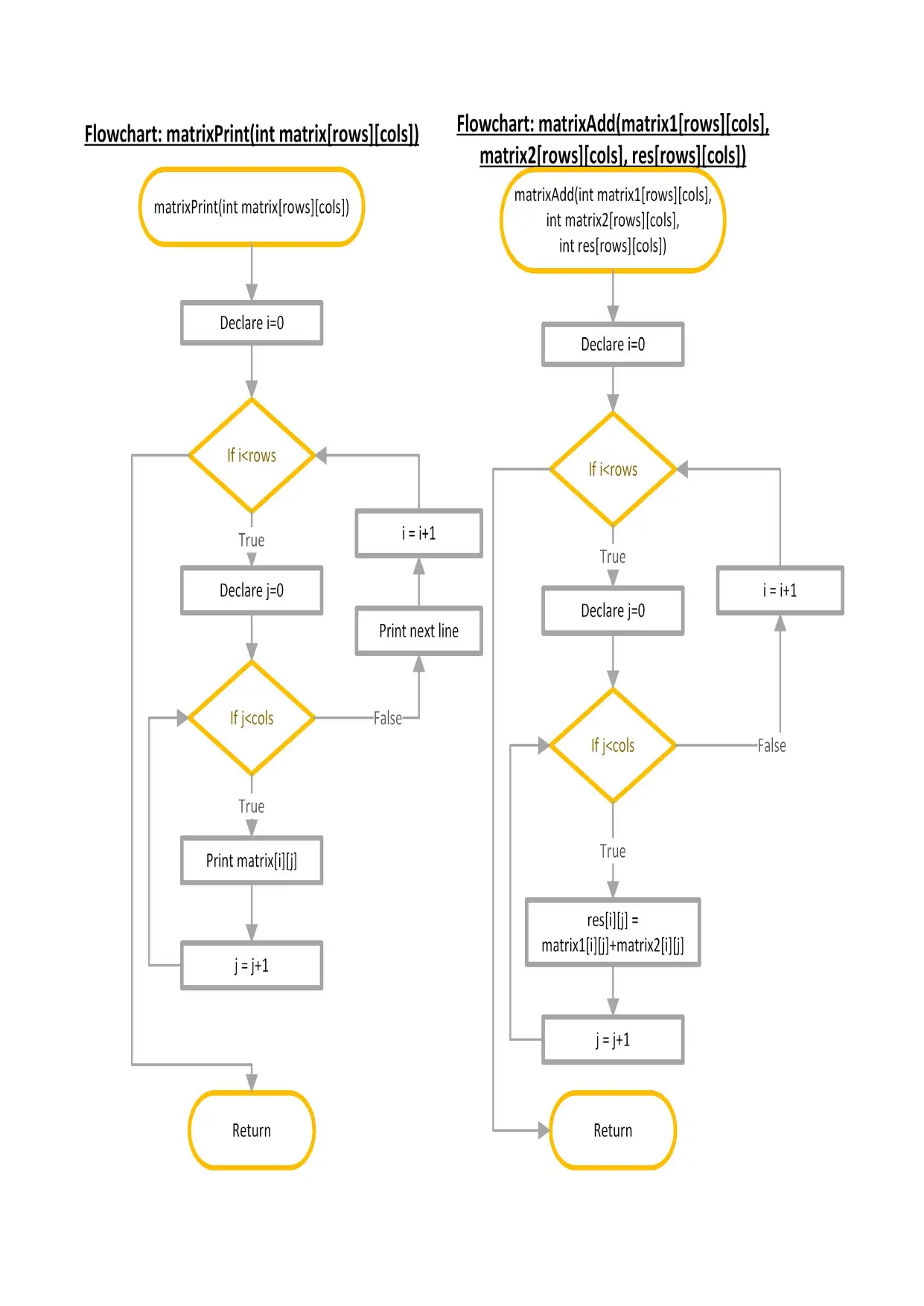
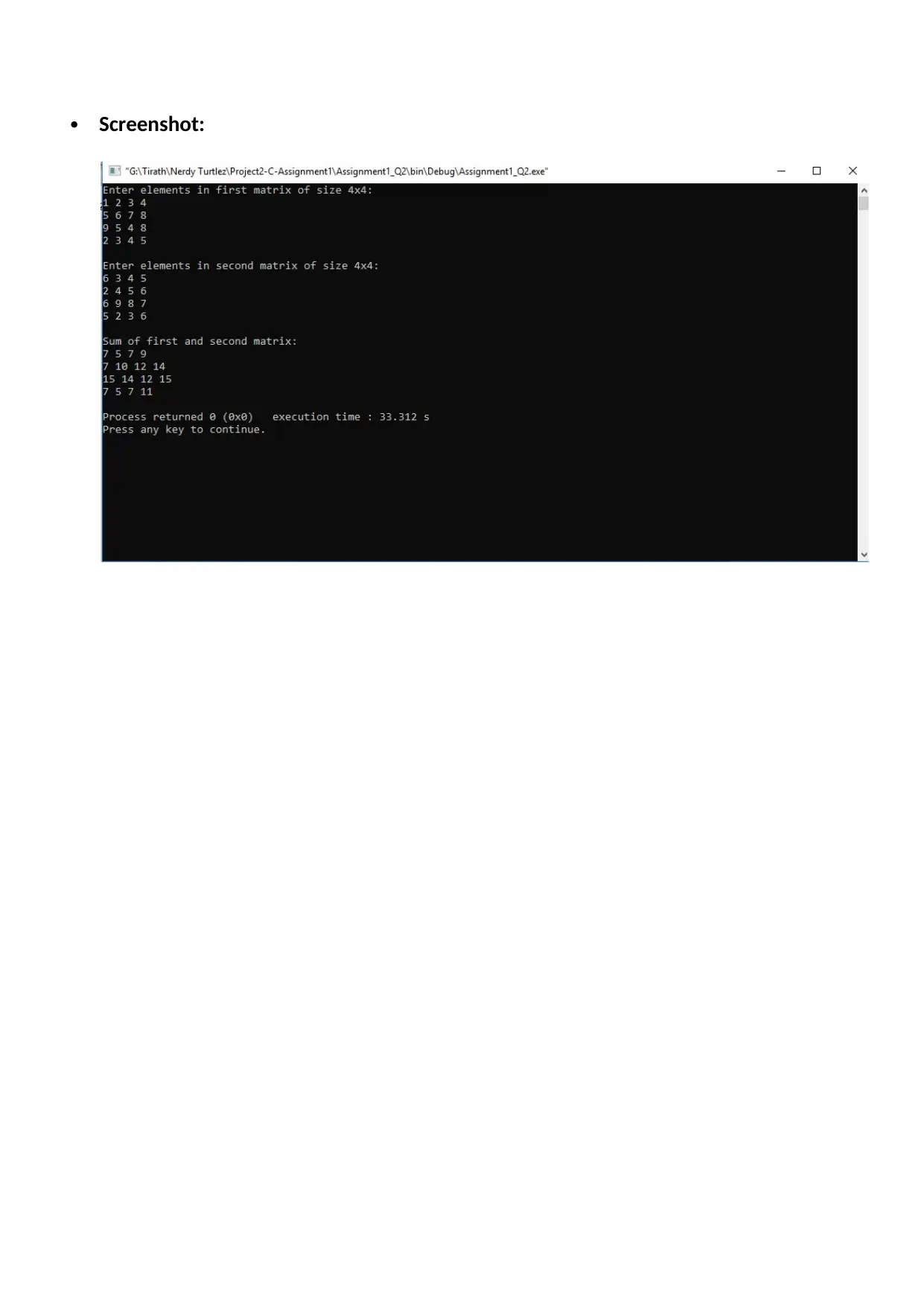
Screenshot:
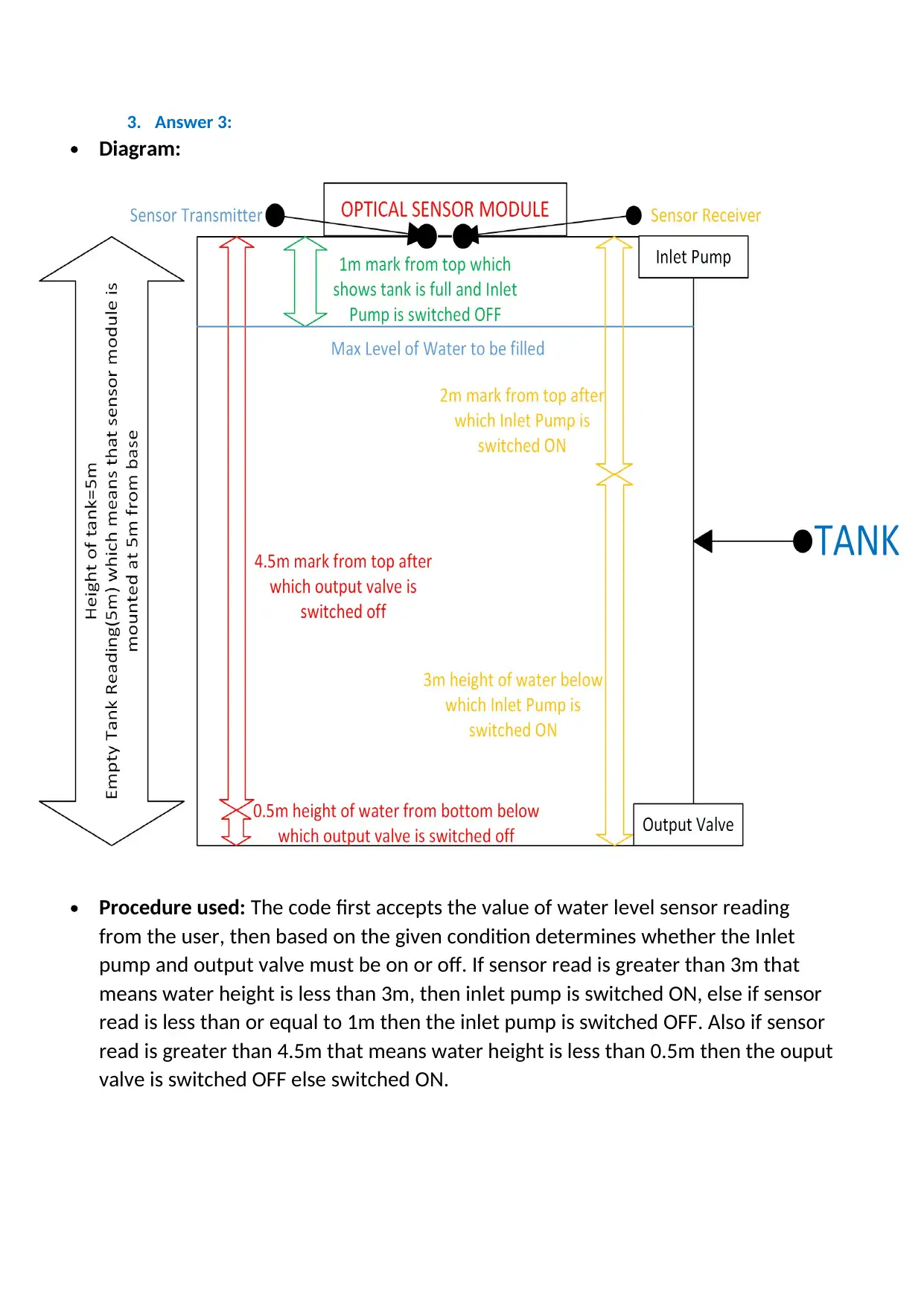
3. Answer 3:
Diagram:
Procedure used: The code first accepts the value of water level sensor reading
from the user, then based on the given condition determines whether the Inlet
pump and output valve must be on or off. If sensor read is greater than 3m that
means water height is less than 3m, then inlet pump is switched ON, else if sensor
read is less than or equal to 1m then the inlet pump is switched OFF. Also if sensor
read is greater than 4.5m that means water height is less than 0.5m then the ouput
valve is switched OFF else switched ON.
Diagram:
Procedure used: The code first accepts the value of water level sensor reading
from the user, then based on the given condition determines whether the Inlet
pump and output valve must be on or off. If sensor read is greater than 3m that
means water height is less than 3m, then inlet pump is switched ON, else if sensor
read is less than or equal to 1m then the inlet pump is switched OFF. Also if sensor
read is greater than 4.5m that means water height is less than 0.5m then the ouput
valve is switched OFF else switched ON.
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
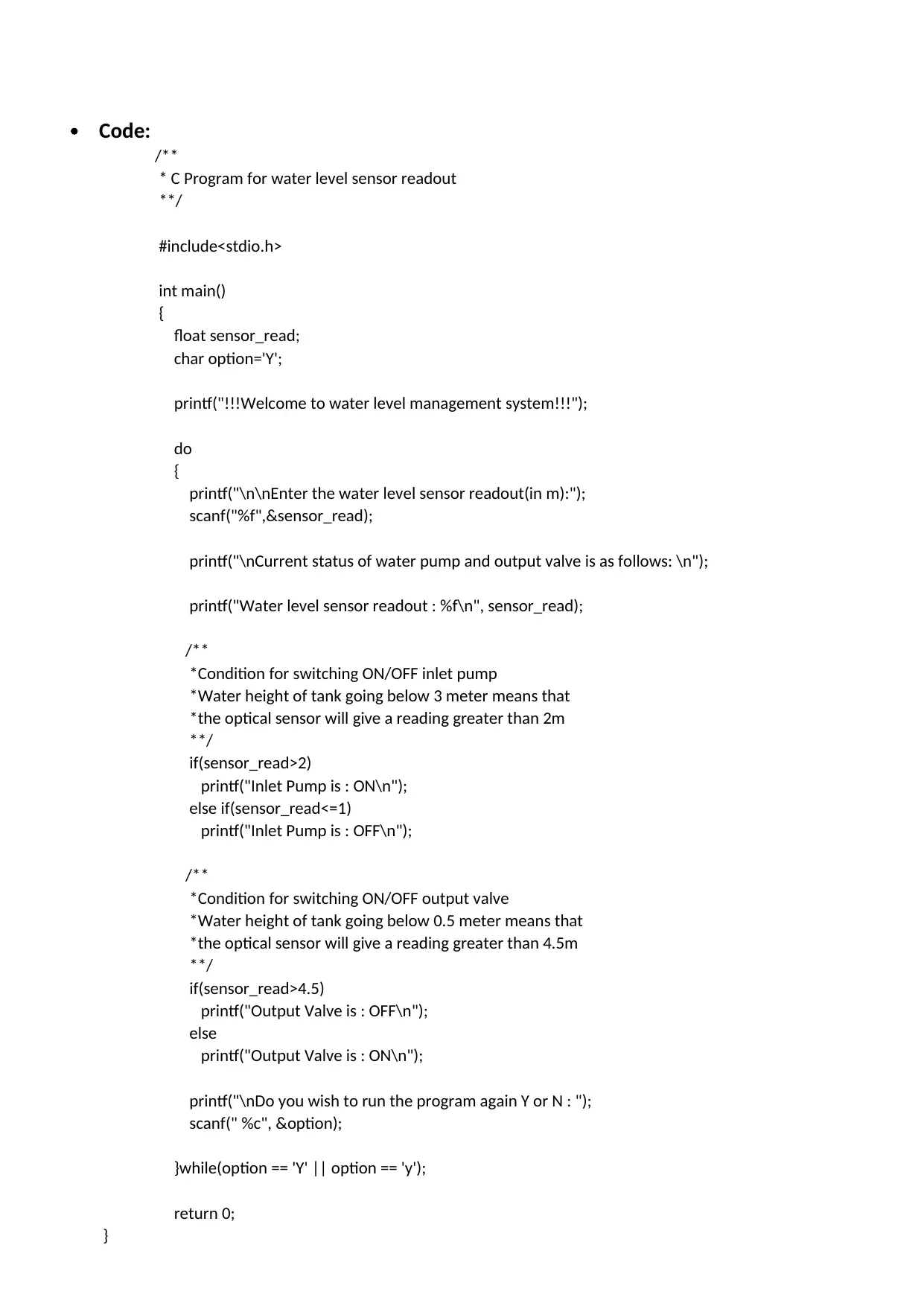
Code:
/**
* C Program for water level sensor readout
**/
#include<stdio.h>
int main()
{
float sensor_read;
char option='Y';
printf("!!!Welcome to water level management system!!!");
do
{
printf("\n\nEnter the water level sensor readout(in m):");
scanf("%f",&sensor_read);
printf("\nCurrent status of water pump and output valve is as follows: \n");
printf("Water level sensor readout : %f\n", sensor_read);
/**
*Condition for switching ON/OFF inlet pump
*Water height of tank going below 3 meter means that
*the optical sensor will give a reading greater than 2m
**/
if(sensor_read>2)
printf("Inlet Pump is : ON\n");
else if(sensor_read<=1)
printf("Inlet Pump is : OFF\n");
/**
*Condition for switching ON/OFF output valve
*Water height of tank going below 0.5 meter means that
*the optical sensor will give a reading greater than 4.5m
**/
if(sensor_read>4.5)
printf("Output Valve is : OFF\n");
else
printf("Output Valve is : ON\n");
printf("\nDo you wish to run the program again Y or N : ");
scanf(" %c", &option);
}while(option == 'Y' || option == 'y');
return 0;
}
/**
* C Program for water level sensor readout
**/
#include<stdio.h>
int main()
{
float sensor_read;
char option='Y';
printf("!!!Welcome to water level management system!!!");
do
{
printf("\n\nEnter the water level sensor readout(in m):");
scanf("%f",&sensor_read);
printf("\nCurrent status of water pump and output valve is as follows: \n");
printf("Water level sensor readout : %f\n", sensor_read);
/**
*Condition for switching ON/OFF inlet pump
*Water height of tank going below 3 meter means that
*the optical sensor will give a reading greater than 2m
**/
if(sensor_read>2)
printf("Inlet Pump is : ON\n");
else if(sensor_read<=1)
printf("Inlet Pump is : OFF\n");
/**
*Condition for switching ON/OFF output valve
*Water height of tank going below 0.5 meter means that
*the optical sensor will give a reading greater than 4.5m
**/
if(sensor_read>4.5)
printf("Output Valve is : OFF\n");
else
printf("Output Valve is : ON\n");
printf("\nDo you wish to run the program again Y or N : ");
scanf(" %c", &option);
}while(option == 'Y' || option == 'y');
return 0;
}
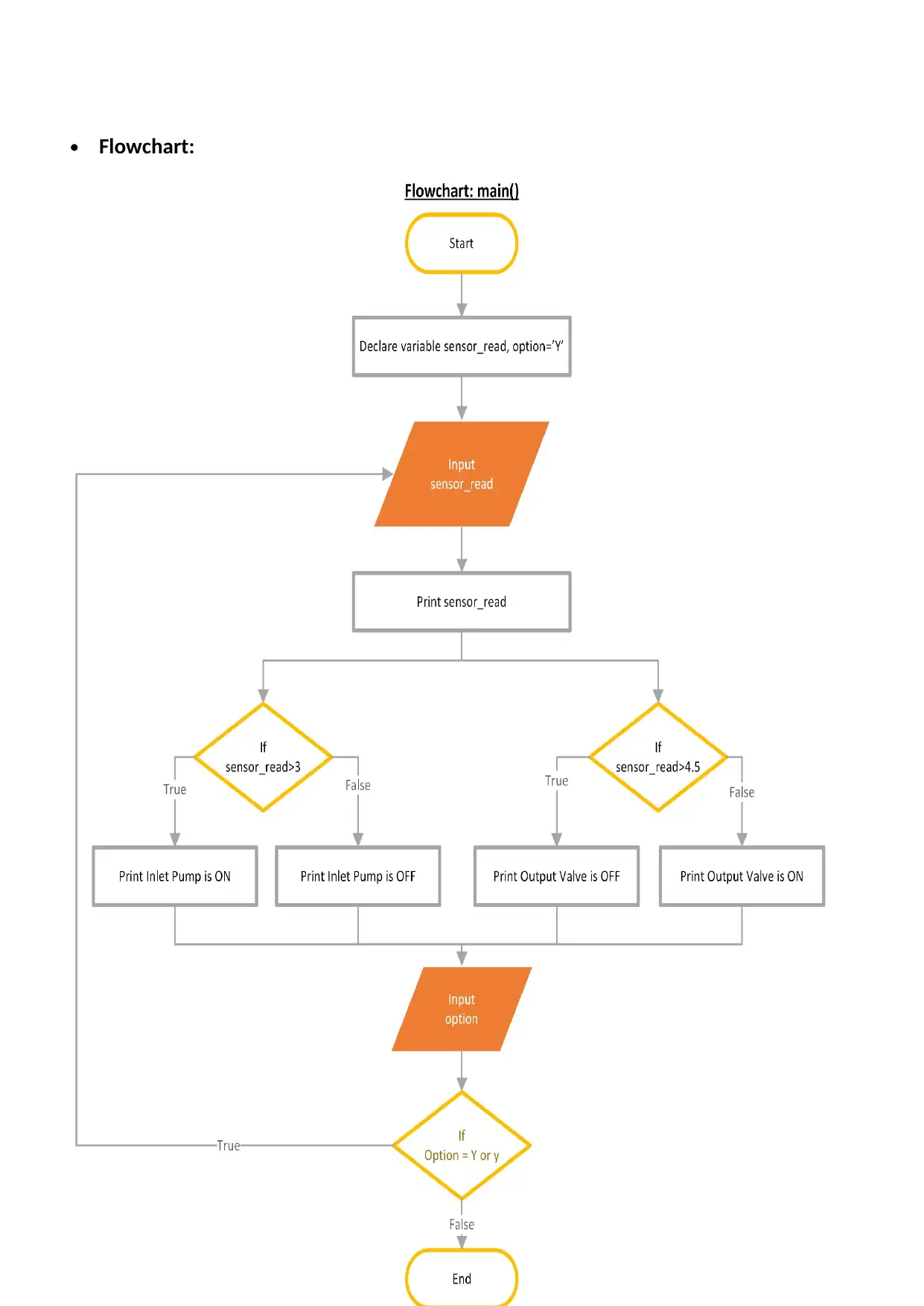
Flowchart:
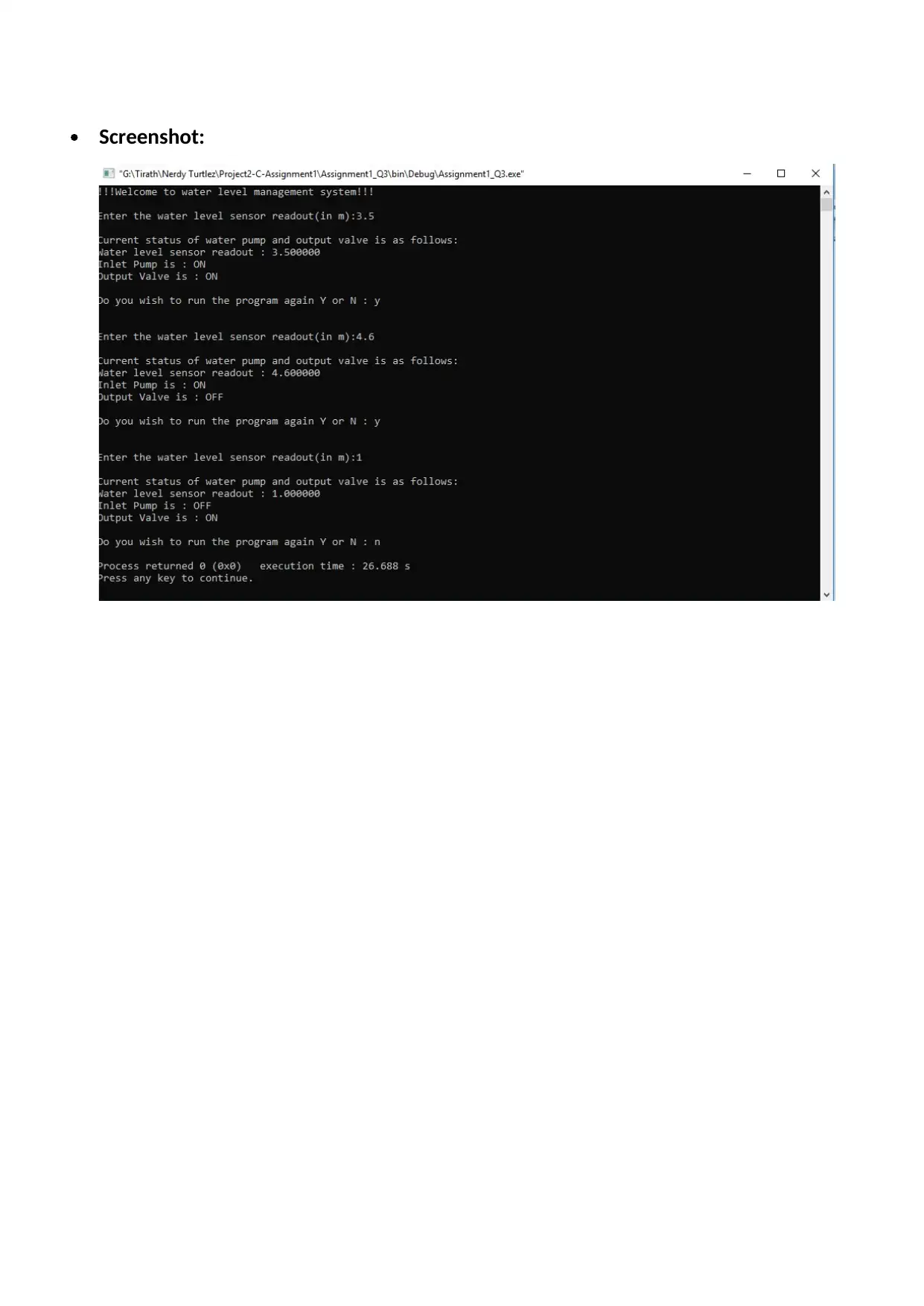
Screenshot:
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
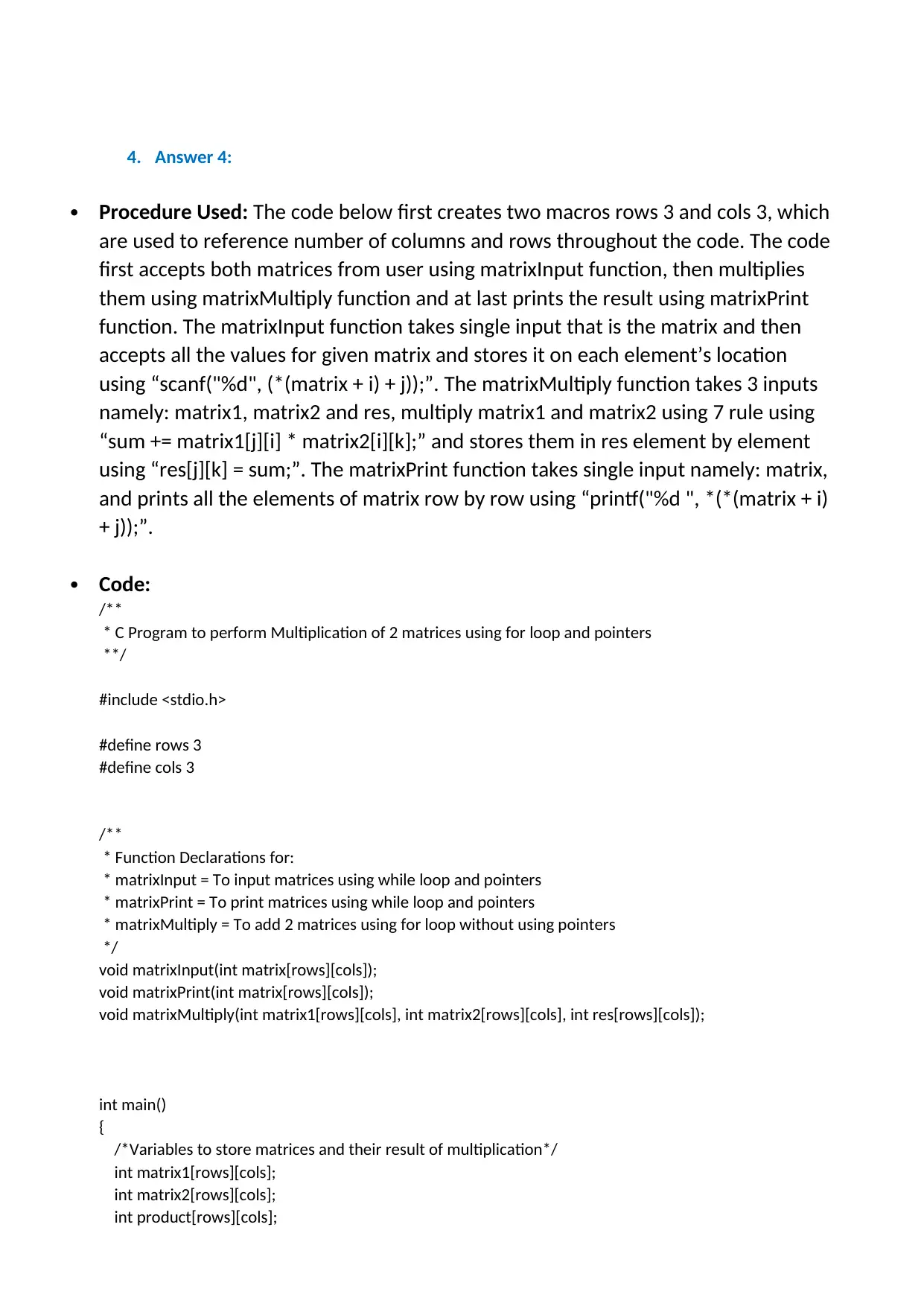
4. Answer 4:
Procedure Used: The code below first creates two macros rows 3 and cols 3, which
are used to reference number of columns and rows throughout the code. The code
first accepts both matrices from user using matrixInput function, then multiplies
them using matrixMultiply function and at last prints the result using matrixPrint
function. The matrixInput function takes single input that is the matrix and then
accepts all the values for given matrix and stores it on each element’s location
using “scanf("%d", (*(matrix + i) + j));”. The matrixMultiply function takes 3 inputs
namely: matrix1, matrix2 and res, multiply matrix1 and matrix2 using 7 rule using
“sum += matrix1[j][i] * matrix2[i][k];” and stores them in res element by element
using “res[j][k] = sum;”. The matrixPrint function takes single input namely: matrix,
and prints all the elements of matrix row by row using “printf("%d ", *(*(matrix + i)
+ j));”.
Code:
/**
* C Program to perform Multiplication of 2 matrices using for loop and pointers
**/
#include <stdio.h>
#define rows 3
#define cols 3
/**
* Function Declarations for:
* matrixInput = To input matrices using while loop and pointers
* matrixPrint = To print matrices using while loop and pointers
* matrixMultiply = To add 2 matrices using for loop without using pointers
*/
void matrixInput(int matrix[rows][cols]);
void matrixPrint(int matrix[rows][cols]);
void matrixMultiply(int matrix1[rows][cols], int matrix2[rows][cols], int res[rows][cols]);
int main()
{
/*Variables to store matrices and their result of multiplication*/
int matrix1[rows][cols];
int matrix2[rows][cols];
int product[rows][cols];
Procedure Used: The code below first creates two macros rows 3 and cols 3, which
are used to reference number of columns and rows throughout the code. The code
first accepts both matrices from user using matrixInput function, then multiplies
them using matrixMultiply function and at last prints the result using matrixPrint
function. The matrixInput function takes single input that is the matrix and then
accepts all the values for given matrix and stores it on each element’s location
using “scanf("%d", (*(matrix + i) + j));”. The matrixMultiply function takes 3 inputs
namely: matrix1, matrix2 and res, multiply matrix1 and matrix2 using 7 rule using
“sum += matrix1[j][i] * matrix2[i][k];” and stores them in res element by element
using “res[j][k] = sum;”. The matrixPrint function takes single input namely: matrix,
and prints all the elements of matrix row by row using “printf("%d ", *(*(matrix + i)
+ j));”.
Code:
/**
* C Program to perform Multiplication of 2 matrices using for loop and pointers
**/
#include <stdio.h>
#define rows 3
#define cols 3
/**
* Function Declarations for:
* matrixInput = To input matrices using while loop and pointers
* matrixPrint = To print matrices using while loop and pointers
* matrixMultiply = To add 2 matrices using for loop without using pointers
*/
void matrixInput(int matrix[rows][cols]);
void matrixPrint(int matrix[rows][cols]);
void matrixMultiply(int matrix1[rows][cols], int matrix2[rows][cols], int res[rows][cols]);
int main()
{
/*Variables to store matrices and their result of multiplication*/
int matrix1[rows][cols];
int matrix2[rows][cols];
int product[rows][cols];
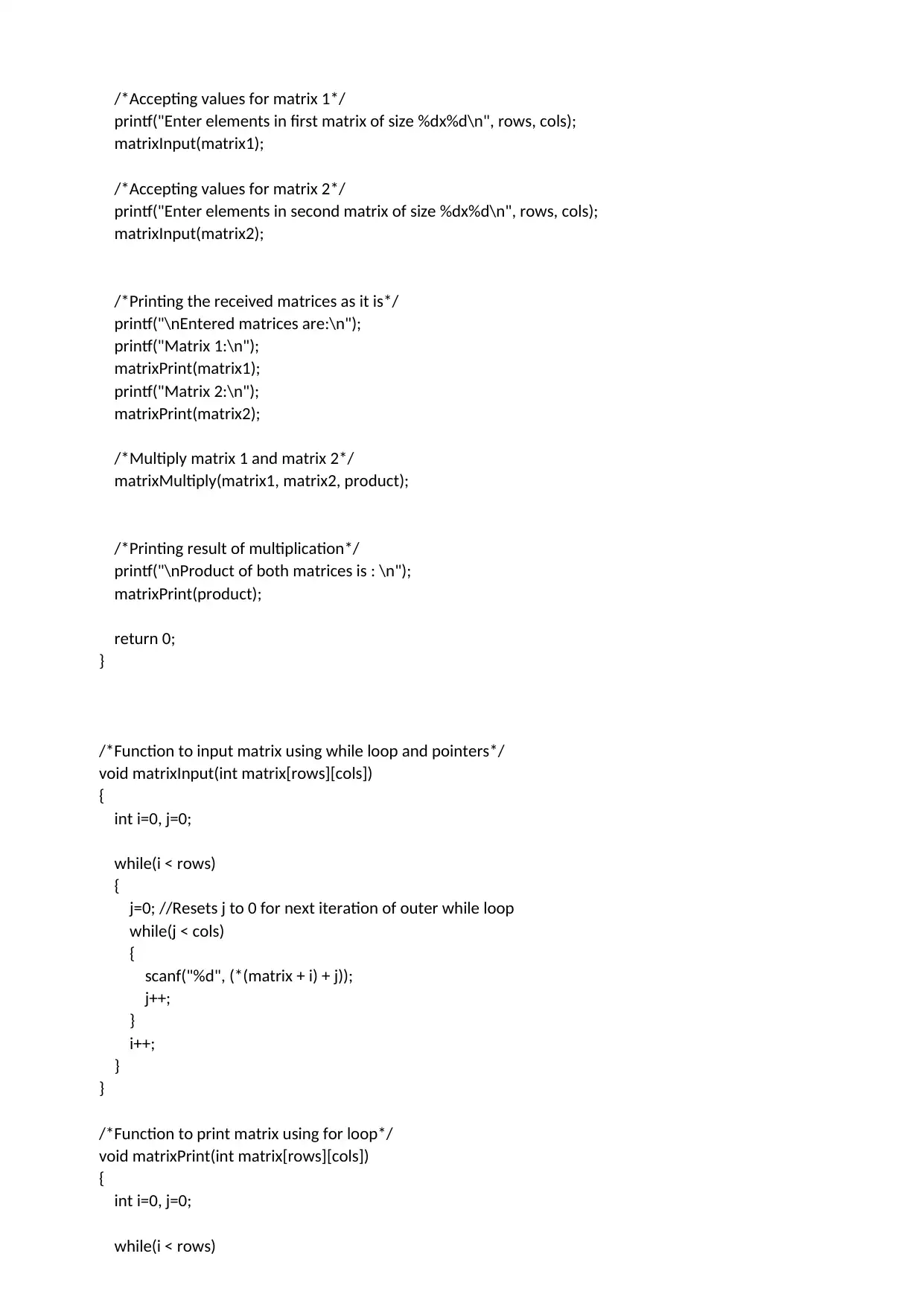
/*Accepting values for matrix 1*/
printf("Enter elements in first matrix of size %dx%d\n", rows, cols);
matrixInput(matrix1);
/*Accepting values for matrix 2*/
printf("Enter elements in second matrix of size %dx%d\n", rows, cols);
matrixInput(matrix2);
/*Printing the received matrices as it is*/
printf("\nEntered matrices are:\n");
printf("Matrix 1:\n");
matrixPrint(matrix1);
printf("Matrix 2:\n");
matrixPrint(matrix2);
/*Multiply matrix 1 and matrix 2*/
matrixMultiply(matrix1, matrix2, product);
/*Printing result of multiplication*/
printf("\nProduct of both matrices is : \n");
matrixPrint(product);
return 0;
}
/*Function to input matrix using while loop and pointers*/
void matrixInput(int matrix[rows][cols])
{
int i=0, j=0;
while(i < rows)
{
j=0; //Resets j to 0 for next iteration of outer while loop
while(j < cols)
{
scanf("%d", (*(matrix + i) + j));
j++;
}
i++;
}
}
/*Function to print matrix using for loop*/
void matrixPrint(int matrix[rows][cols])
{
int i=0, j=0;
while(i < rows)
printf("Enter elements in first matrix of size %dx%d\n", rows, cols);
matrixInput(matrix1);
/*Accepting values for matrix 2*/
printf("Enter elements in second matrix of size %dx%d\n", rows, cols);
matrixInput(matrix2);
/*Printing the received matrices as it is*/
printf("\nEntered matrices are:\n");
printf("Matrix 1:\n");
matrixPrint(matrix1);
printf("Matrix 2:\n");
matrixPrint(matrix2);
/*Multiply matrix 1 and matrix 2*/
matrixMultiply(matrix1, matrix2, product);
/*Printing result of multiplication*/
printf("\nProduct of both matrices is : \n");
matrixPrint(product);
return 0;
}
/*Function to input matrix using while loop and pointers*/
void matrixInput(int matrix[rows][cols])
{
int i=0, j=0;
while(i < rows)
{
j=0; //Resets j to 0 for next iteration of outer while loop
while(j < cols)
{
scanf("%d", (*(matrix + i) + j));
j++;
}
i++;
}
}
/*Function to print matrix using for loop*/
void matrixPrint(int matrix[rows][cols])
{
int i=0, j=0;
while(i < rows)
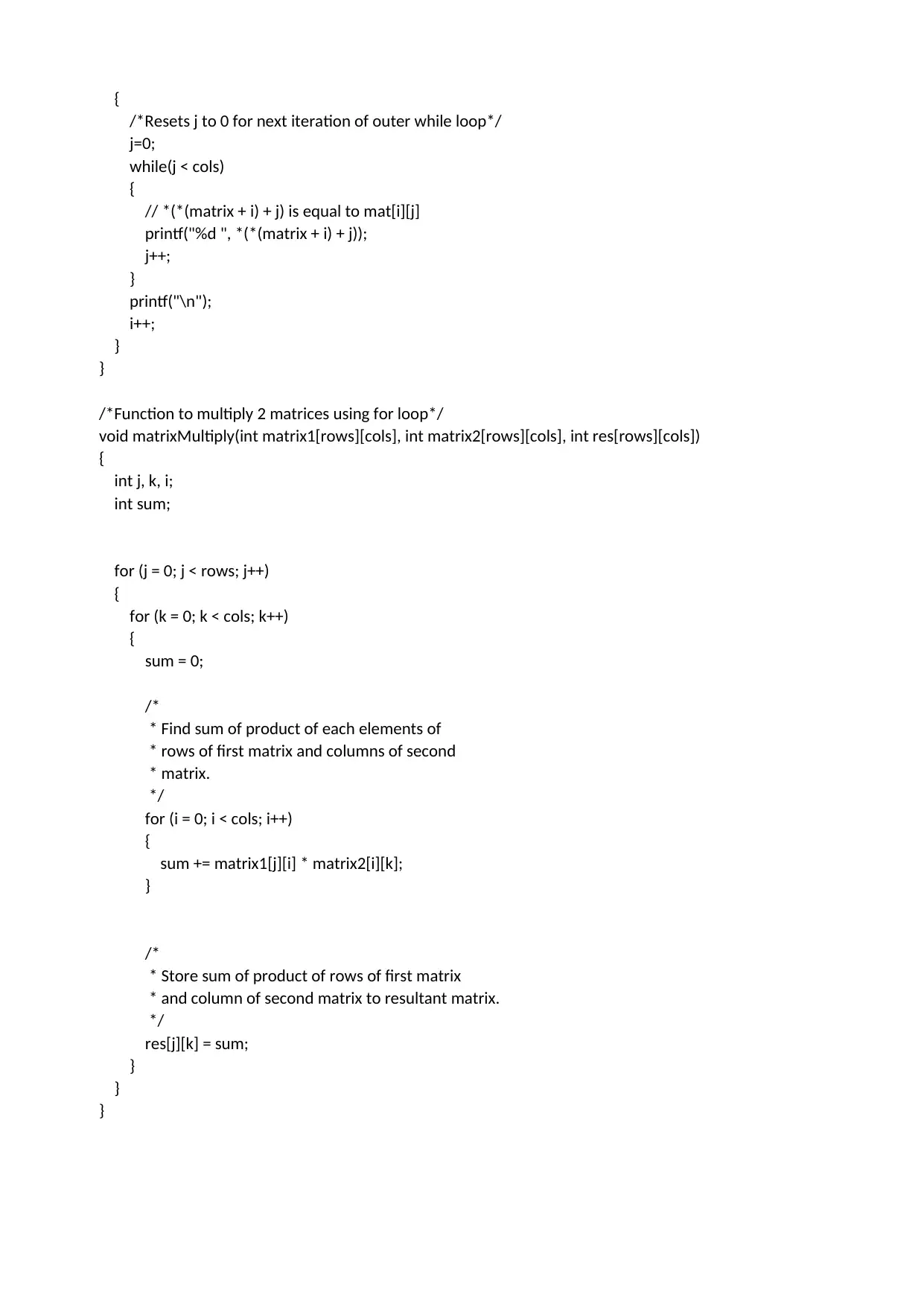
{
/*Resets j to 0 for next iteration of outer while loop*/
j=0;
while(j < cols)
{
// *(*(matrix + i) + j) is equal to mat[i][j]
printf("%d ", *(*(matrix + i) + j));
j++;
}
printf("\n");
i++;
}
}
/*Function to multiply 2 matrices using for loop*/
void matrixMultiply(int matrix1[rows][cols], int matrix2[rows][cols], int res[rows][cols])
{
int j, k, i;
int sum;
for (j = 0; j < rows; j++)
{
for (k = 0; k < cols; k++)
{
sum = 0;
/*
* Find sum of product of each elements of
* rows of first matrix and columns of second
* matrix.
*/
for (i = 0; i < cols; i++)
{
sum += matrix1[j][i] * matrix2[i][k];
}
/*
* Store sum of product of rows of first matrix
* and column of second matrix to resultant matrix.
*/
res[j][k] = sum;
}
}
}
/*Resets j to 0 for next iteration of outer while loop*/
j=0;
while(j < cols)
{
// *(*(matrix + i) + j) is equal to mat[i][j]
printf("%d ", *(*(matrix + i) + j));
j++;
}
printf("\n");
i++;
}
}
/*Function to multiply 2 matrices using for loop*/
void matrixMultiply(int matrix1[rows][cols], int matrix2[rows][cols], int res[rows][cols])
{
int j, k, i;
int sum;
for (j = 0; j < rows; j++)
{
for (k = 0; k < cols; k++)
{
sum = 0;
/*
* Find sum of product of each elements of
* rows of first matrix and columns of second
* matrix.
*/
for (i = 0; i < cols; i++)
{
sum += matrix1[j][i] * matrix2[i][k];
}
/*
* Store sum of product of rows of first matrix
* and column of second matrix to resultant matrix.
*/
res[j][k] = sum;
}
}
}
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
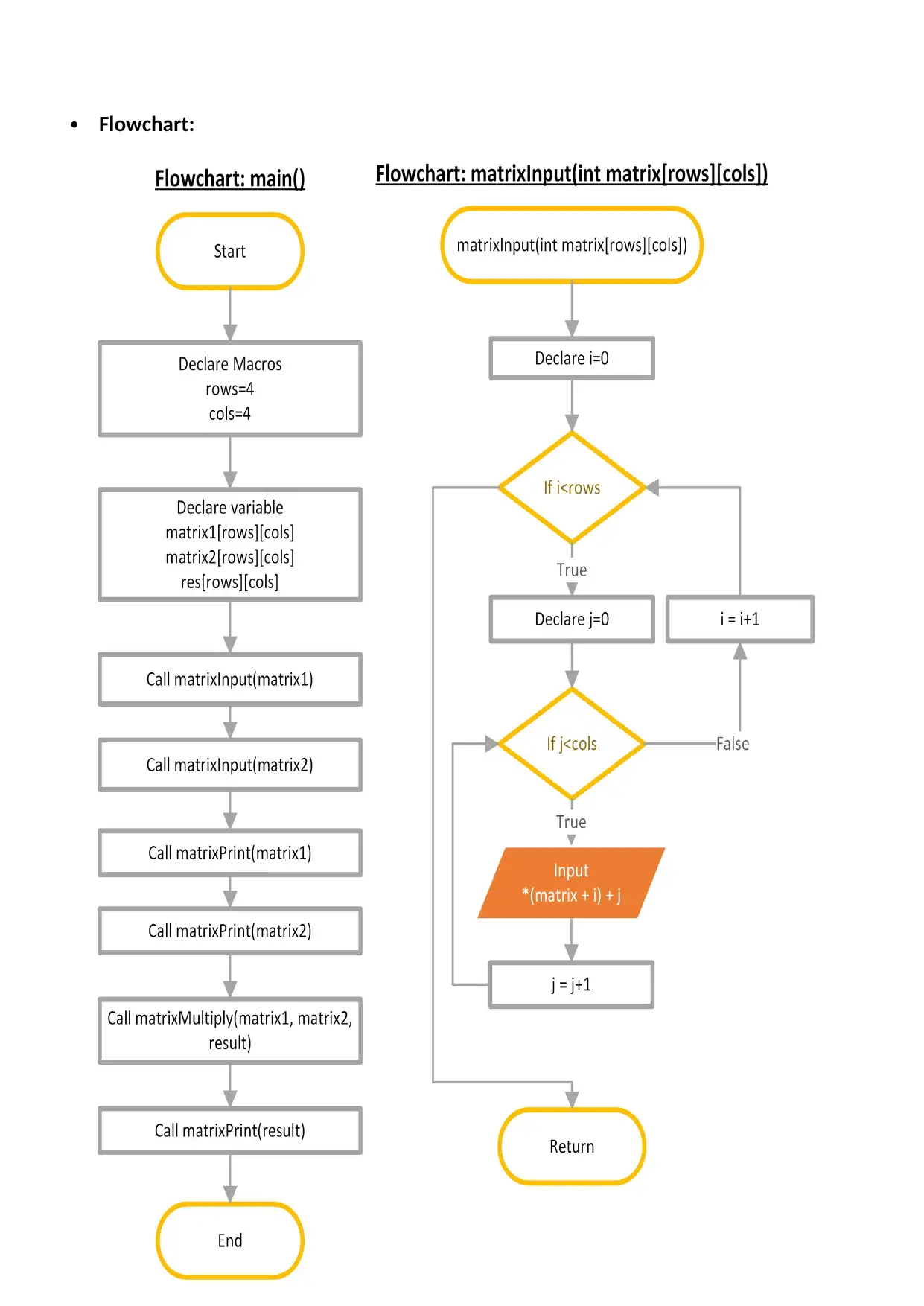
Flowchart:

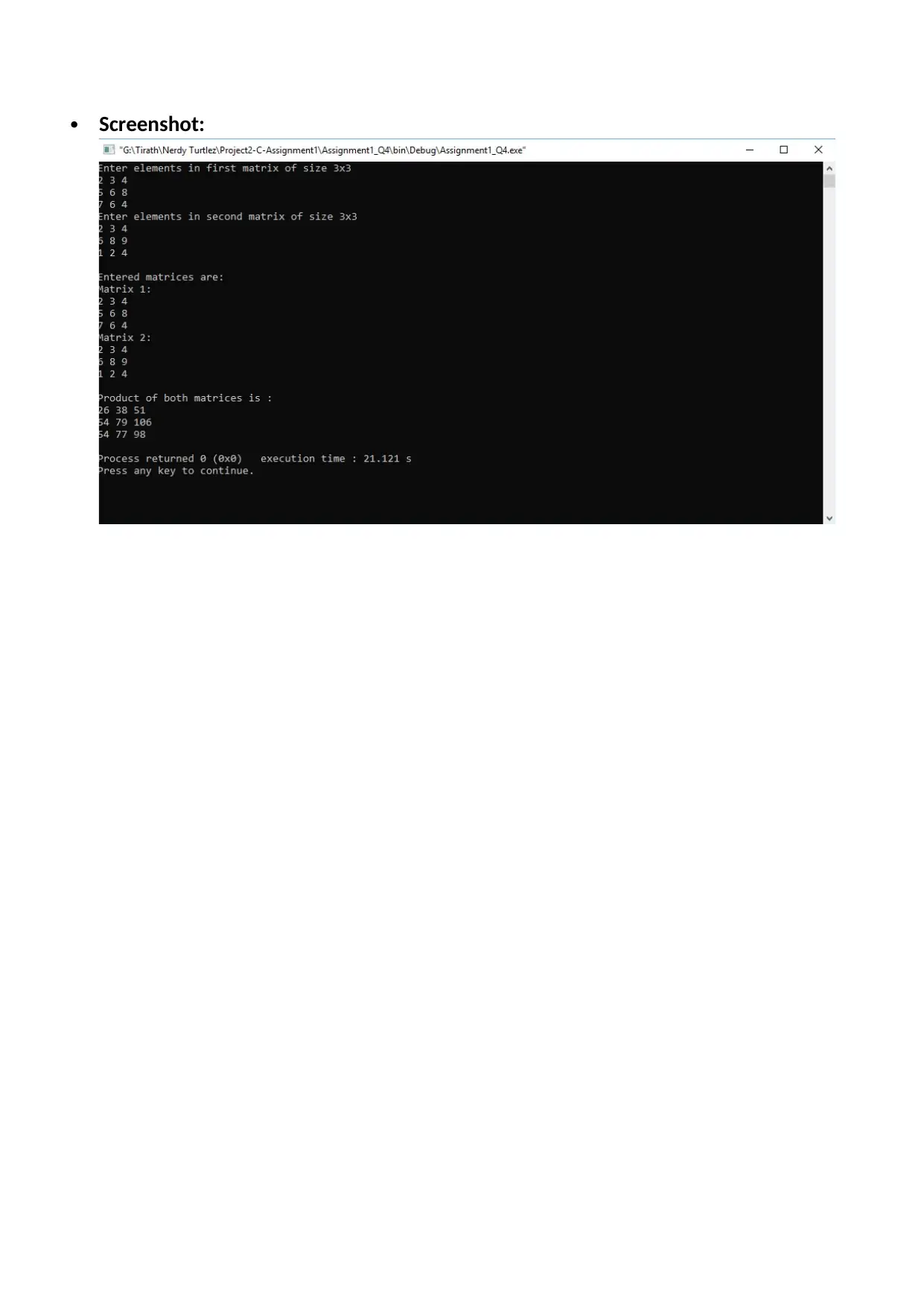
Screenshot:
1 out of 19

Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.