University of West London, CP30681E ISD Log Book Assignment
VerifiedAdded on 2021/04/21
|38
|6072
|250
Homework Assignment
AI Summary
This document presents a student's log book from an Introduction to Software Development (ISD) module, likely at the University of West London. The log book covers the first four weeks of the course, detailing practical sessions and assignments. Week 1 introduces code repositories, software layers, and basic computer concepts. Week 2 focuses on algorithms, program structure, Python basics, and the IDLE shell. Week 3 delves into Python fundamentals, variable types, data type conversion, and online code repositories. Week 4 explores Python keywords, operators, data type conversions, and error handling. The assignments include writing algorithms, Python programs, and identifying and correcting errors in code. The log book also covers topics such as variable naming conventions and format specifiers. The provided solutions demonstrate the application of concepts learned in each week.

INTRODUCTION TO SOFTWARE DEVELOPMENT
MODULE CODE: CP30681E
LOG BOOK
STUDENT ID: XXXXXXXXXXX
SCHOOL OF COMPUTING AND ENGINEERING
UNIVERSITY OF WEST LONDON
MODULE CODE: CP30681E
LOG BOOK
STUDENT ID: XXXXXXXXXXX
SCHOOL OF COMPUTING AND ENGINEERING
UNIVERSITY OF WEST LONDON
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
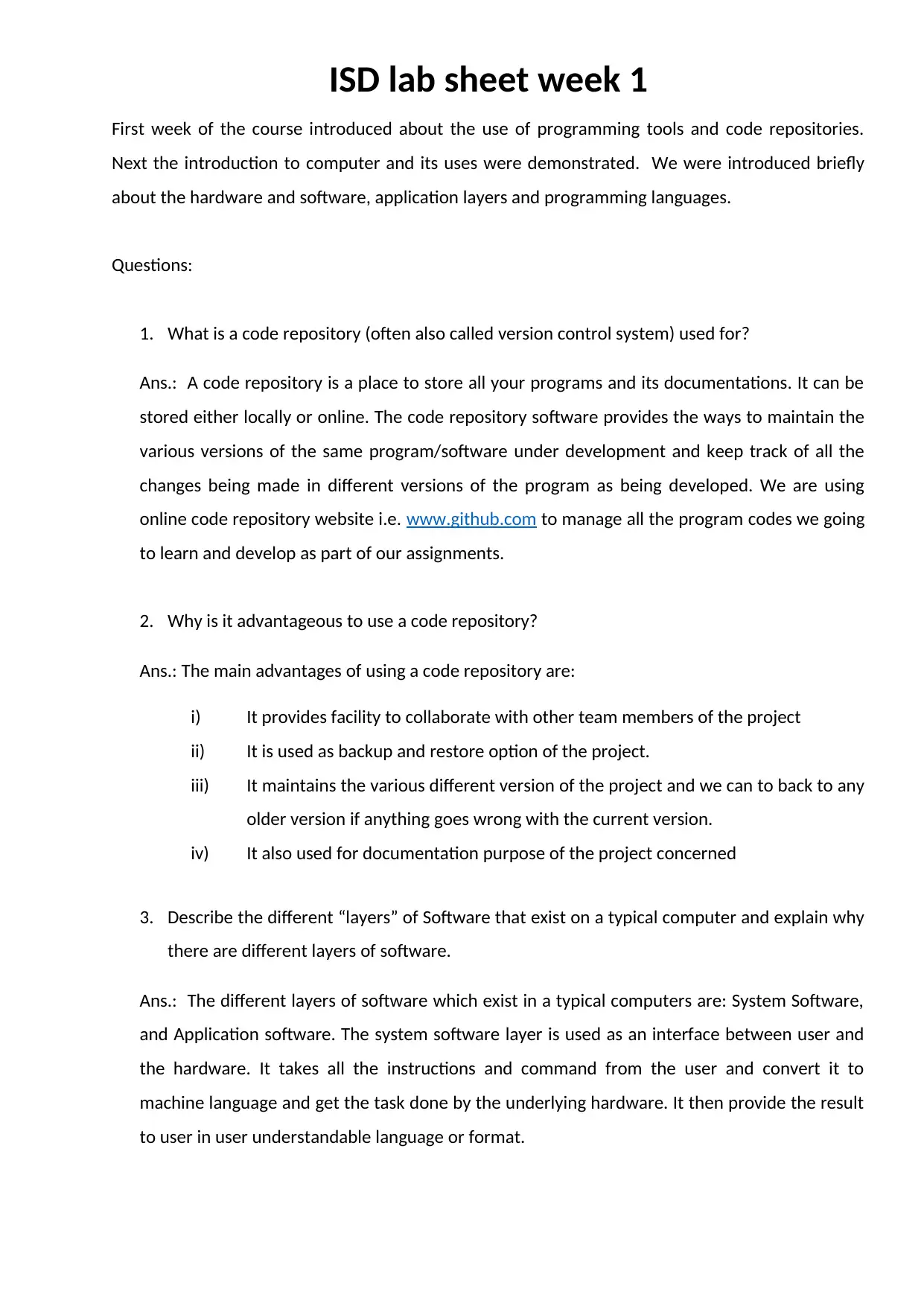
ISD lab sheet week 1
First week of the course introduced about the use of programming tools and code repositories.
Next the introduction to computer and its uses were demonstrated. We were introduced briefly
about the hardware and software, application layers and programming languages.
Questions:
1. What is a code repository (often also called version control system) used for?
Ans.: A code repository is a place to store all your programs and its documentations. It can be
stored either locally or online. The code repository software provides the ways to maintain the
various versions of the same program/software under development and keep track of all the
changes being made in different versions of the program as being developed. We are using
online code repository website i.e. www.github.com to manage all the program codes we going
to learn and develop as part of our assignments.
2. Why is it advantageous to use a code repository?
Ans.: The main advantages of using a code repository are:
i) It provides facility to collaborate with other team members of the project
ii) It is used as backup and restore option of the project.
iii) It maintains the various different version of the project and we can to back to any
older version if anything goes wrong with the current version.
iv) It also used for documentation purpose of the project concerned
3. Describe the different “layers” of Software that exist on a typical computer and explain why
there are different layers of software.
Ans.: The different layers of software which exist in a typical computers are: System Software,
and Application software. The system software layer is used as an interface between user and
the hardware. It takes all the instructions and command from the user and convert it to
machine language and get the task done by the underlying hardware. It then provide the result
to user in user understandable language or format.
First week of the course introduced about the use of programming tools and code repositories.
Next the introduction to computer and its uses were demonstrated. We were introduced briefly
about the hardware and software, application layers and programming languages.
Questions:
1. What is a code repository (often also called version control system) used for?
Ans.: A code repository is a place to store all your programs and its documentations. It can be
stored either locally or online. The code repository software provides the ways to maintain the
various versions of the same program/software under development and keep track of all the
changes being made in different versions of the program as being developed. We are using
online code repository website i.e. www.github.com to manage all the program codes we going
to learn and develop as part of our assignments.
2. Why is it advantageous to use a code repository?
Ans.: The main advantages of using a code repository are:
i) It provides facility to collaborate with other team members of the project
ii) It is used as backup and restore option of the project.
iii) It maintains the various different version of the project and we can to back to any
older version if anything goes wrong with the current version.
iv) It also used for documentation purpose of the project concerned
3. Describe the different “layers” of Software that exist on a typical computer and explain why
there are different layers of software.
Ans.: The different layers of software which exist in a typical computers are: System Software,
and Application software. The system software layer is used as an interface between user and
the hardware. It takes all the instructions and command from the user and convert it to
machine language and get the task done by the underlying hardware. It then provide the result
to user in user understandable language or format.
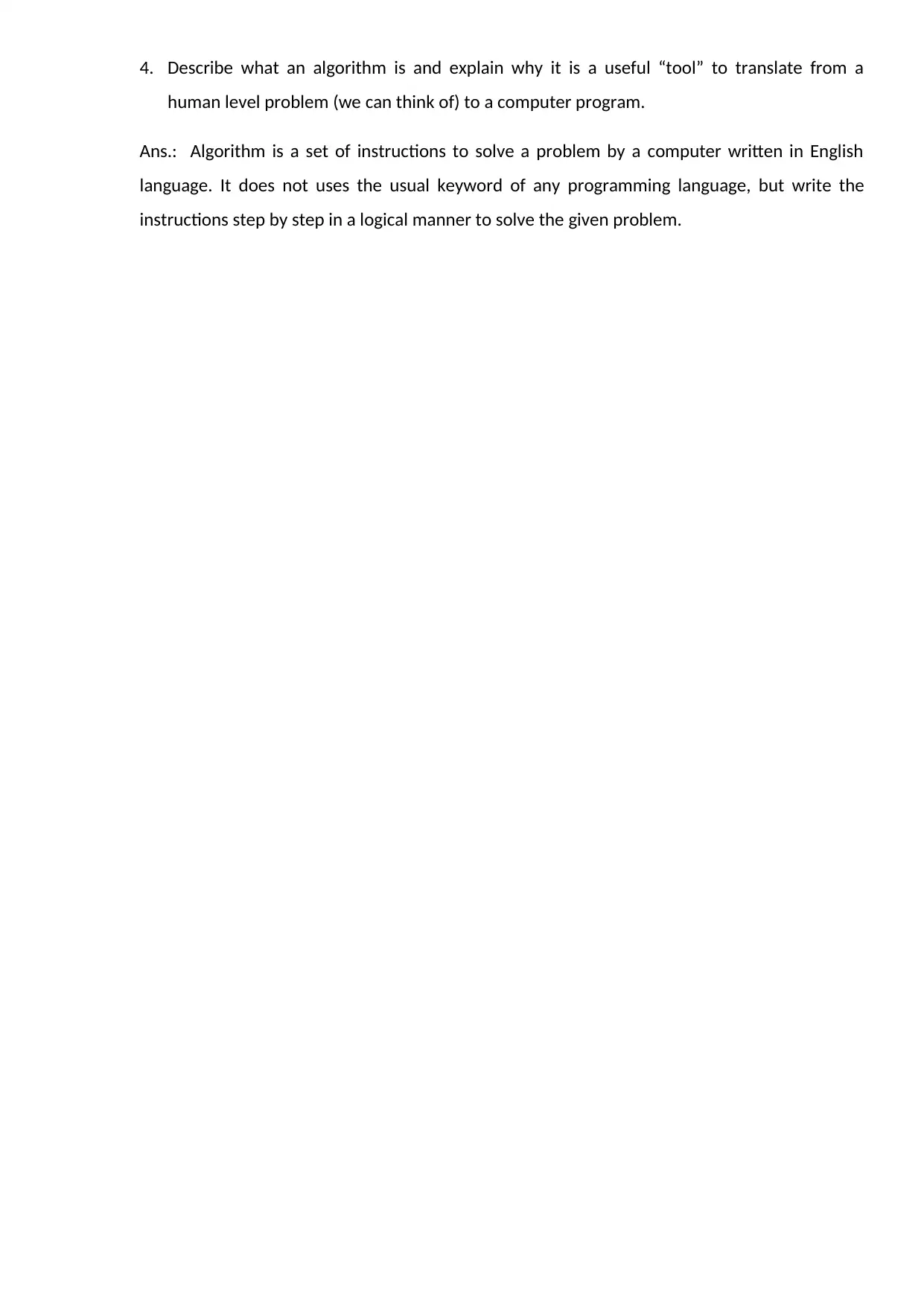
4. Describe what an algorithm is and explain why it is a useful “tool” to translate from a
human level problem (we can think of) to a computer program.
Ans.: Algorithm is a set of instructions to solve a problem by a computer written in English
language. It does not uses the usual keyword of any programming language, but write the
instructions step by step in a logical manner to solve the given problem.
human level problem (we can think of) to a computer program.
Ans.: Algorithm is a set of instructions to solve a problem by a computer written in English
language. It does not uses the usual keyword of any programming language, but write the
instructions step by step in a logical manner to solve the given problem.
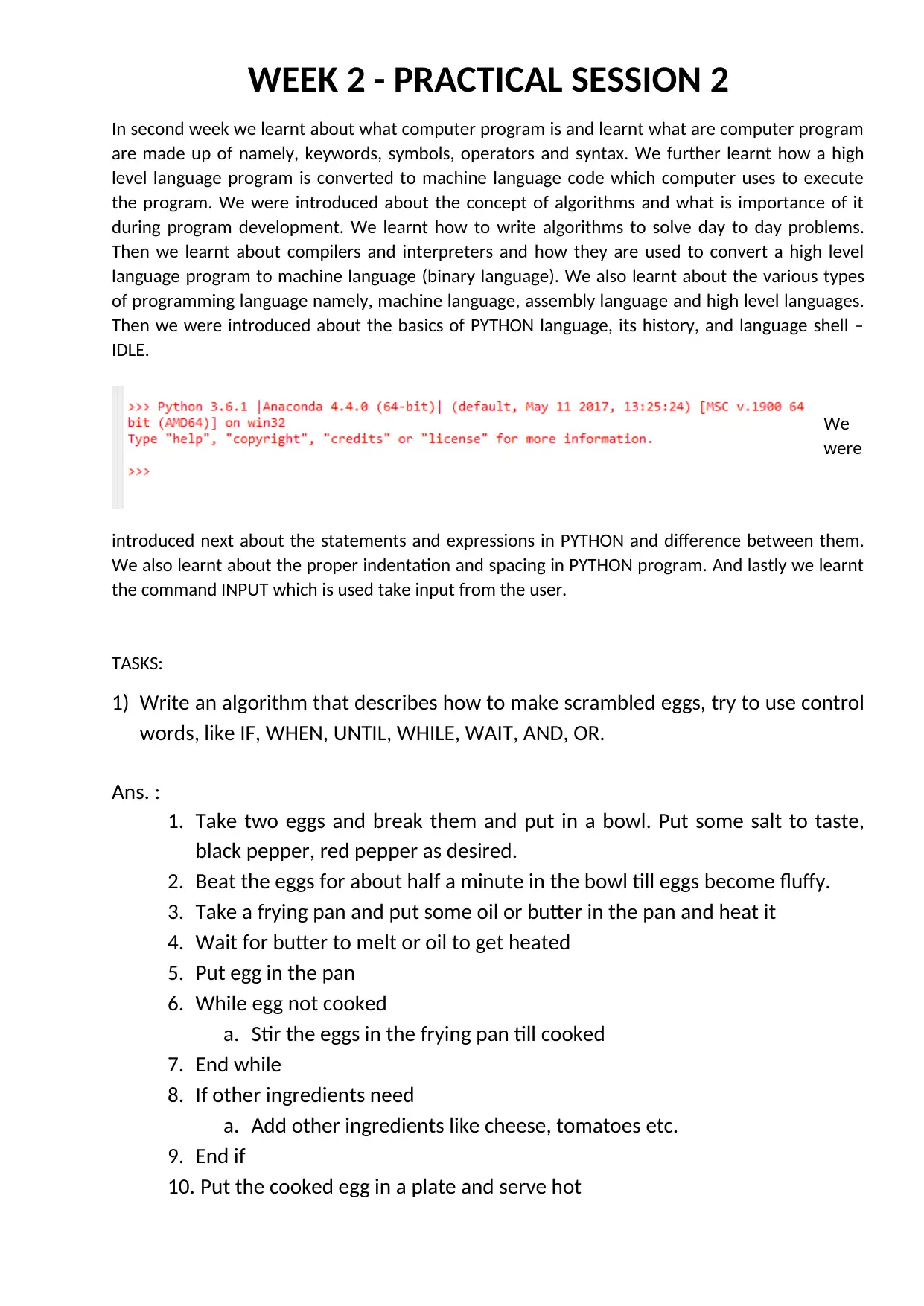
WEEK 2 - PRACTICAL SESSION 2
In second week we learnt about what computer program is and learnt what are computer program
are made up of namely, keywords, symbols, operators and syntax. We further learnt how a high
level language program is converted to machine language code which computer uses to execute
the program. We were introduced about the concept of algorithms and what is importance of it
during program development. We learnt how to write algorithms to solve day to day problems.
Then we learnt about compilers and interpreters and how they are used to convert a high level
language program to machine language (binary language). We also learnt about the various types
of programming language namely, machine language, assembly language and high level languages.
Then we were introduced about the basics of PYTHON language, its history, and language shell –
IDLE.
We
were
introduced next about the statements and expressions in PYTHON and difference between them.
We also learnt about the proper indentation and spacing in PYTHON program. And lastly we learnt
the command INPUT which is used take input from the user.
TASKS:
1) Write an algorithm that describes how to make scrambled eggs, try to use control
words, like IF, WHEN, UNTIL, WHILE, WAIT, AND, OR.
Ans. :
1. Take two eggs and break them and put in a bowl. Put some salt to taste,
black pepper, red pepper as desired.
2. Beat the eggs for about half a minute in the bowl till eggs become fluffy.
3. Take a frying pan and put some oil or butter in the pan and heat it
4. Wait for butter to melt or oil to get heated
5. Put egg in the pan
6. While egg not cooked
a. Stir the eggs in the frying pan till cooked
7. End while
8. If other ingredients need
a. Add other ingredients like cheese, tomatoes etc.
9. End if
10. Put the cooked egg in a plate and serve hot
In second week we learnt about what computer program is and learnt what are computer program
are made up of namely, keywords, symbols, operators and syntax. We further learnt how a high
level language program is converted to machine language code which computer uses to execute
the program. We were introduced about the concept of algorithms and what is importance of it
during program development. We learnt how to write algorithms to solve day to day problems.
Then we learnt about compilers and interpreters and how they are used to convert a high level
language program to machine language (binary language). We also learnt about the various types
of programming language namely, machine language, assembly language and high level languages.
Then we were introduced about the basics of PYTHON language, its history, and language shell –
IDLE.
We
were
introduced next about the statements and expressions in PYTHON and difference between them.
We also learnt about the proper indentation and spacing in PYTHON program. And lastly we learnt
the command INPUT which is used take input from the user.
TASKS:
1) Write an algorithm that describes how to make scrambled eggs, try to use control
words, like IF, WHEN, UNTIL, WHILE, WAIT, AND, OR.
Ans. :
1. Take two eggs and break them and put in a bowl. Put some salt to taste,
black pepper, red pepper as desired.
2. Beat the eggs for about half a minute in the bowl till eggs become fluffy.
3. Take a frying pan and put some oil or butter in the pan and heat it
4. Wait for butter to melt or oil to get heated
5. Put egg in the pan
6. While egg not cooked
a. Stir the eggs in the frying pan till cooked
7. End while
8. If other ingredients need
a. Add other ingredients like cheese, tomatoes etc.
9. End if
10. Put the cooked egg in a plate and serve hot
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
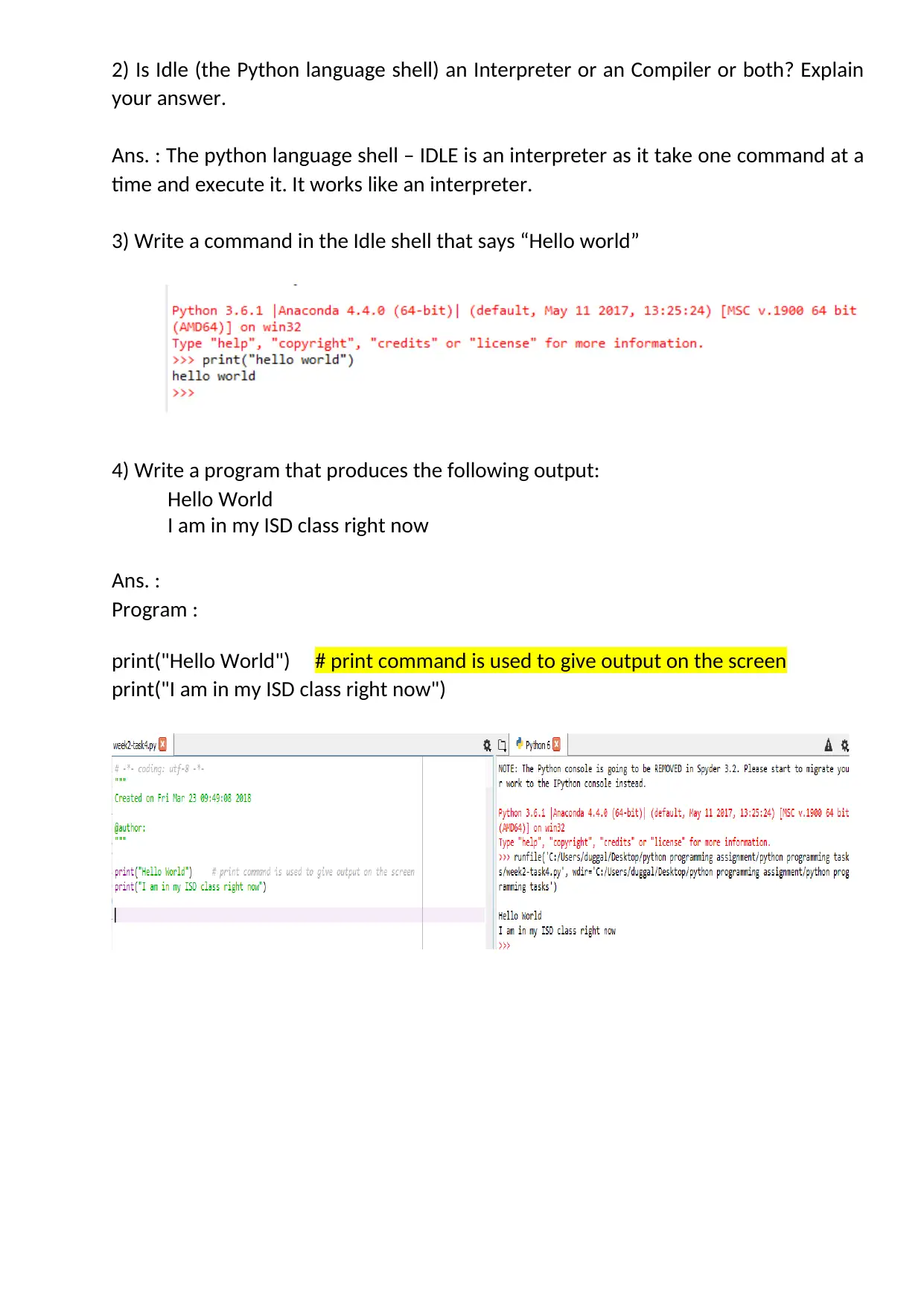
2) Is Idle (the Python language shell) an Interpreter or an Compiler or both? Explain
your answer.
Ans. : The python language shell – IDLE is an interpreter as it take one command at a
time and execute it. It works like an interpreter.
3) Write a command in the Idle shell that says “Hello world”
4) Write a program that produces the following output:
Hello World
I am in my ISD class right now
Ans. :
Program :
print("Hello World") # print command is used to give output on the screen
print("I am in my ISD class right now")
your answer.
Ans. : The python language shell – IDLE is an interpreter as it take one command at a
time and execute it. It works like an interpreter.
3) Write a command in the Idle shell that says “Hello world”
4) Write a program that produces the following output:
Hello World
I am in my ISD class right now
Ans. :
Program :
print("Hello World") # print command is used to give output on the screen
print("I am in my ISD class right now")
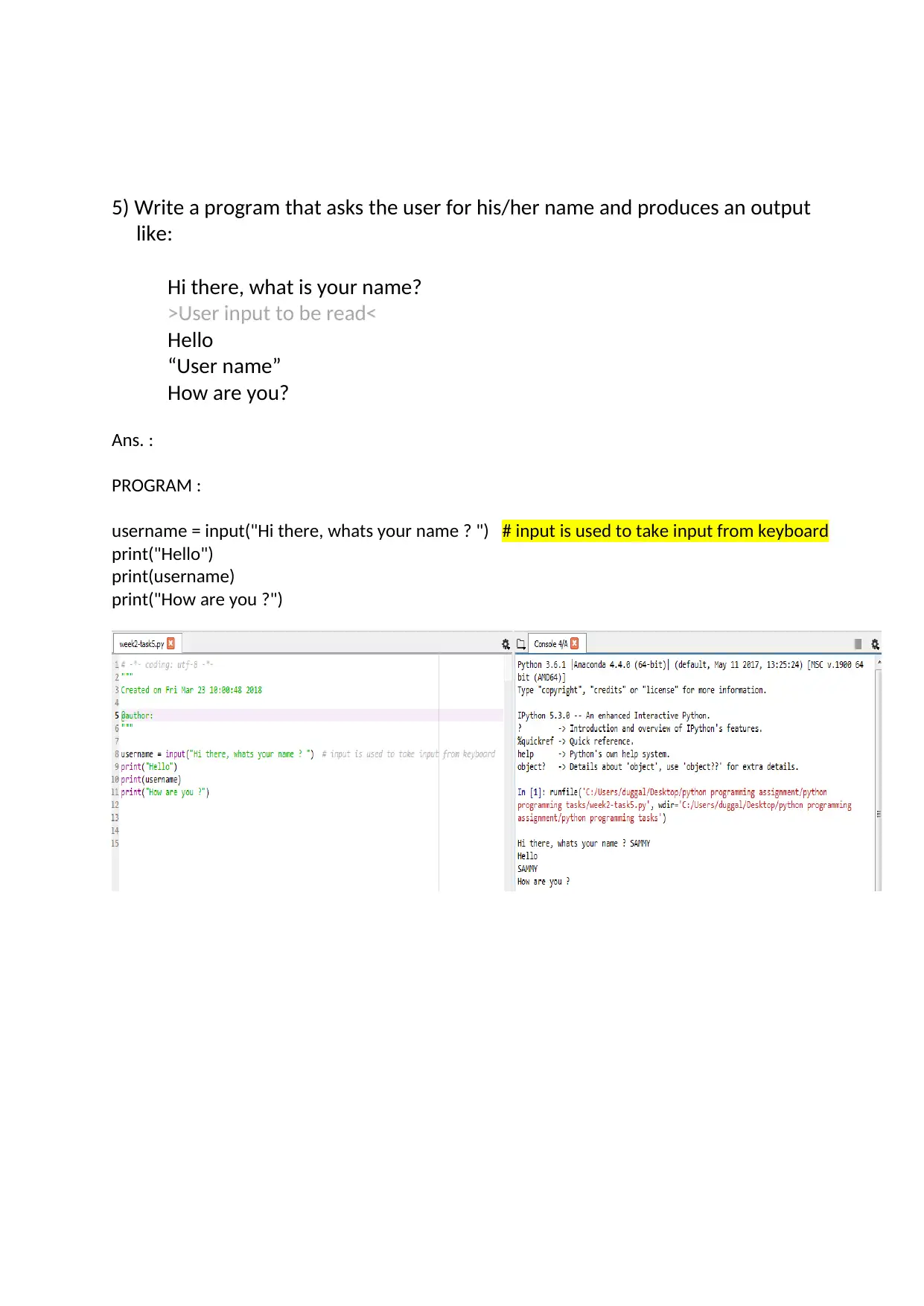
5) Write a program that asks the user for his/her name and produces an output
like:
Hi there, what is your name?
>User input to be read<
Hello
“User name”
How are you?
Ans. :
PROGRAM :
username = input("Hi there, whats your name ? ") # input is used to take input from keyboard
print("Hello")
print(username)
print("How are you ?")
like:
Hi there, what is your name?
>User input to be read<
Hello
“User name”
How are you?
Ans. :
PROGRAM :
username = input("Hi there, whats your name ? ") # input is used to take input from keyboard
print("Hello")
print(username)
print("How are you ?")
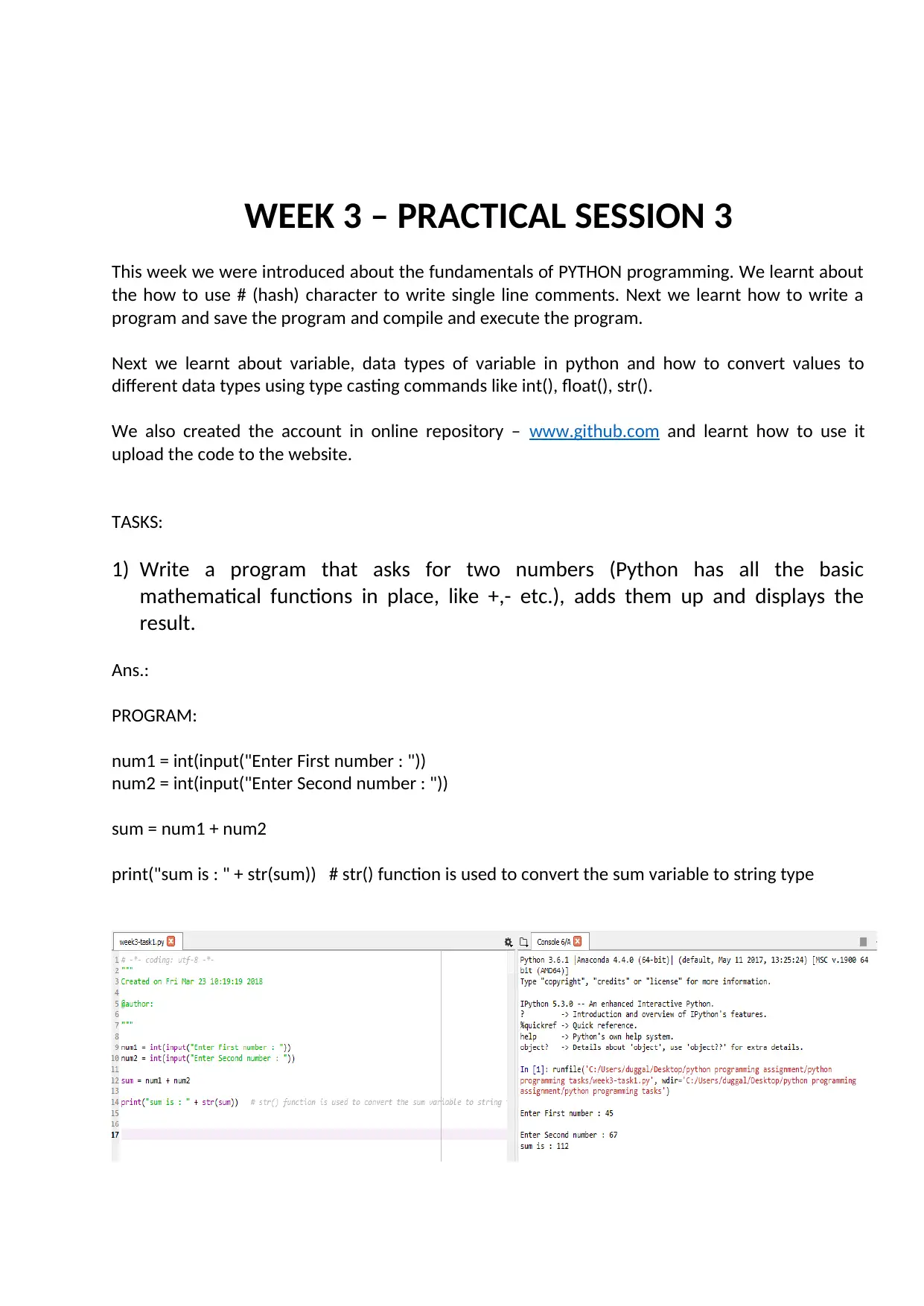
WEEK 3 – PRACTICAL SESSION 3
This week we were introduced about the fundamentals of PYTHON programming. We learnt about
the how to use # (hash) character to write single line comments. Next we learnt how to write a
program and save the program and compile and execute the program.
Next we learnt about variable, data types of variable in python and how to convert values to
different data types using type casting commands like int(), float(), str().
We also created the account in online repository – www.github.com and learnt how to use it
upload the code to the website.
TASKS:
1) Write a program that asks for two numbers (Python has all the basic
mathematical functions in place, like +,- etc.), adds them up and displays the
result.
Ans.:
PROGRAM:
num1 = int(input("Enter First number : "))
num2 = int(input("Enter Second number : "))
sum = num1 + num2
print("sum is : " + str(sum)) # str() function is used to convert the sum variable to string type
This week we were introduced about the fundamentals of PYTHON programming. We learnt about
the how to use # (hash) character to write single line comments. Next we learnt how to write a
program and save the program and compile and execute the program.
Next we learnt about variable, data types of variable in python and how to convert values to
different data types using type casting commands like int(), float(), str().
We also created the account in online repository – www.github.com and learnt how to use it
upload the code to the website.
TASKS:
1) Write a program that asks for two numbers (Python has all the basic
mathematical functions in place, like +,- etc.), adds them up and displays the
result.
Ans.:
PROGRAM:
num1 = int(input("Enter First number : "))
num2 = int(input("Enter Second number : "))
sum = num1 + num2
print("sum is : " + str(sum)) # str() function is used to convert the sum variable to string type
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
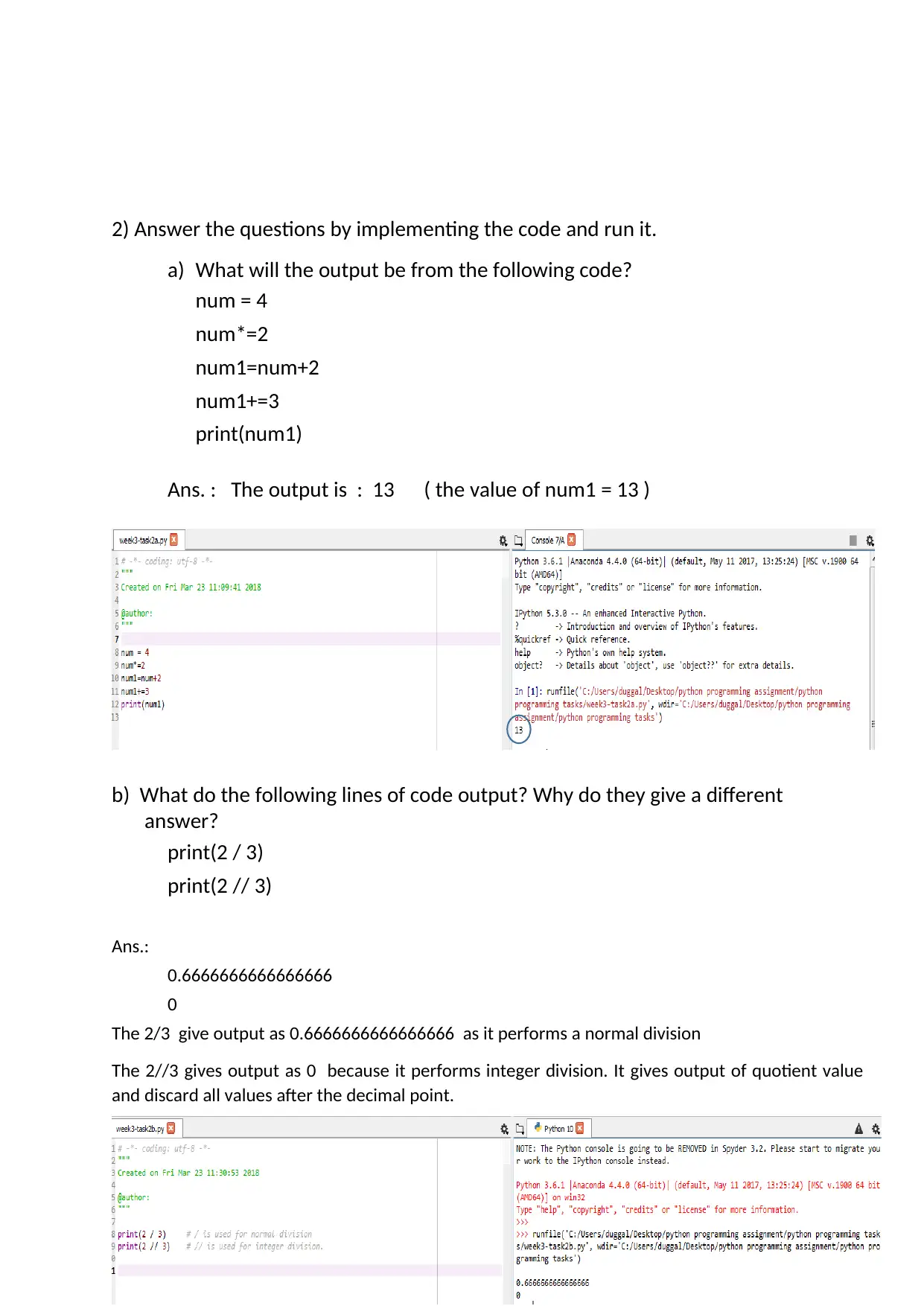
2) Answer the questions by implementing the code and run it.
a) What will the output be from the following code?
num = 4
num*=2
num1=num+2
num1+=3
print(num1)
Ans. : The output is : 13 ( the value of num1 = 13 )
b) What do the following lines of code output? Why do they give a different
answer?
print(2 / 3)
print(2 // 3)
Ans.:
0.6666666666666666
0
The 2/3 give output as 0.6666666666666666 as it performs a normal division
The 2//3 gives output as 0 because it performs integer division. It gives output of quotient value
and discard all values after the decimal point.
a) What will the output be from the following code?
num = 4
num*=2
num1=num+2
num1+=3
print(num1)
Ans. : The output is : 13 ( the value of num1 = 13 )
b) What do the following lines of code output? Why do they give a different
answer?
print(2 / 3)
print(2 // 3)
Ans.:
0.6666666666666666
0
The 2/3 give output as 0.6666666666666666 as it performs a normal division
The 2//3 gives output as 0 because it performs integer division. It gives output of quotient value
and discard all values after the decimal point.
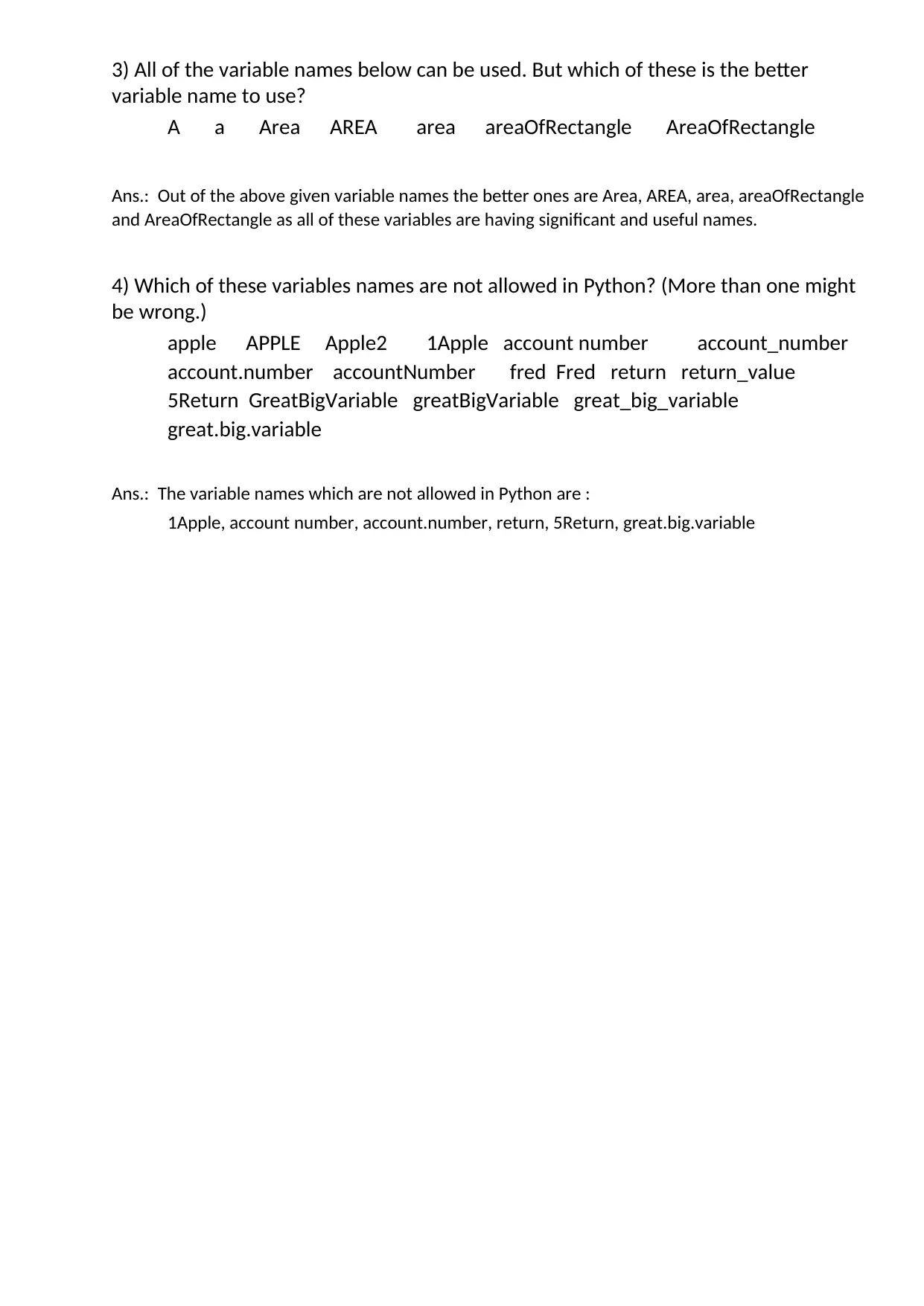
3) All of the variable names below can be used. But which of these is the better
variable name to use?
A a Area AREA area areaOfRectangle AreaOfRectangle
Ans.: Out of the above given variable names the better ones are Area, AREA, area, areaOfRectangle
and AreaOfRectangle as all of these variables are having significant and useful names.
4) Which of these variables names are not allowed in Python? (More than one might
be wrong.)
apple APPLE Apple2 1Apple account number account_number
account.number accountNumber fred Fred return return_value
5Return GreatBigVariable greatBigVariable great_big_variable
great.big.variable
Ans.: The variable names which are not allowed in Python are :
1Apple, account number, account.number, return, 5Return, great.big.variable
variable name to use?
A a Area AREA area areaOfRectangle AreaOfRectangle
Ans.: Out of the above given variable names the better ones are Area, AREA, area, areaOfRectangle
and AreaOfRectangle as all of these variables are having significant and useful names.
4) Which of these variables names are not allowed in Python? (More than one might
be wrong.)
apple APPLE Apple2 1Apple account number account_number
account.number accountNumber fred Fred return return_value
5Return GreatBigVariable greatBigVariable great_big_variable
great.big.variable
Ans.: The variable names which are not allowed in Python are :
1Apple, account number, account.number, return, 5Return, great.big.variable
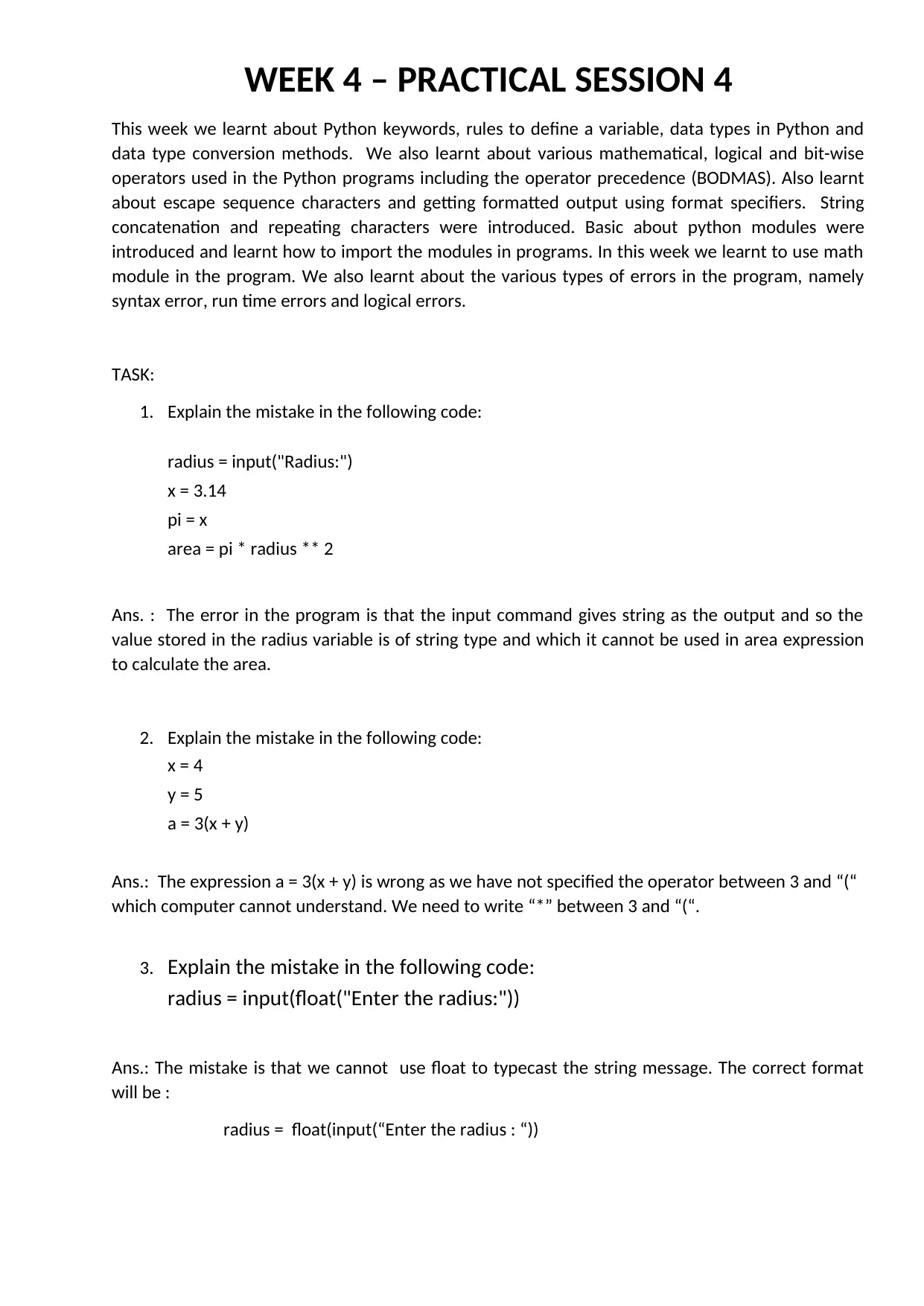
WEEK 4 – PRACTICAL SESSION 4
This week we learnt about Python keywords, rules to define a variable, data types in Python and
data type conversion methods. We also learnt about various mathematical, logical and bit-wise
operators used in the Python programs including the operator precedence (BODMAS). Also learnt
about escape sequence characters and getting formatted output using format specifiers. String
concatenation and repeating characters were introduced. Basic about python modules were
introduced and learnt how to import the modules in programs. In this week we learnt to use math
module in the program. We also learnt about the various types of errors in the program, namely
syntax error, run time errors and logical errors.
TASK:
1. Explain the mistake in the following code:
radius = input("Radius:")
x = 3.14
pi = x
area = pi * radius ** 2
Ans. : The error in the program is that the input command gives string as the output and so the
value stored in the radius variable is of string type and which it cannot be used in area expression
to calculate the area.
2. Explain the mistake in the following code:
x = 4
y = 5
a = 3(x + y)
Ans.: The expression a = 3(x + y) is wrong as we have not specified the operator between 3 and “(“
which computer cannot understand. We need to write “*” between 3 and “(“.
3. Explain the mistake in the following code:
radius = input(float("Enter the radius:"))
Ans.: The mistake is that we cannot use float to typecast the string message. The correct format
will be :
radius = float(input(“Enter the radius : “))
This week we learnt about Python keywords, rules to define a variable, data types in Python and
data type conversion methods. We also learnt about various mathematical, logical and bit-wise
operators used in the Python programs including the operator precedence (BODMAS). Also learnt
about escape sequence characters and getting formatted output using format specifiers. String
concatenation and repeating characters were introduced. Basic about python modules were
introduced and learnt how to import the modules in programs. In this week we learnt to use math
module in the program. We also learnt about the various types of errors in the program, namely
syntax error, run time errors and logical errors.
TASK:
1. Explain the mistake in the following code:
radius = input("Radius:")
x = 3.14
pi = x
area = pi * radius ** 2
Ans. : The error in the program is that the input command gives string as the output and so the
value stored in the radius variable is of string type and which it cannot be used in area expression
to calculate the area.
2. Explain the mistake in the following code:
x = 4
y = 5
a = 3(x + y)
Ans.: The expression a = 3(x + y) is wrong as we have not specified the operator between 3 and “(“
which computer cannot understand. We need to write “*” between 3 and “(“.
3. Explain the mistake in the following code:
radius = input(float("Enter the radius:"))
Ans.: The mistake is that we cannot use float to typecast the string message. The correct format
will be :
radius = float(input(“Enter the radius : “))
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
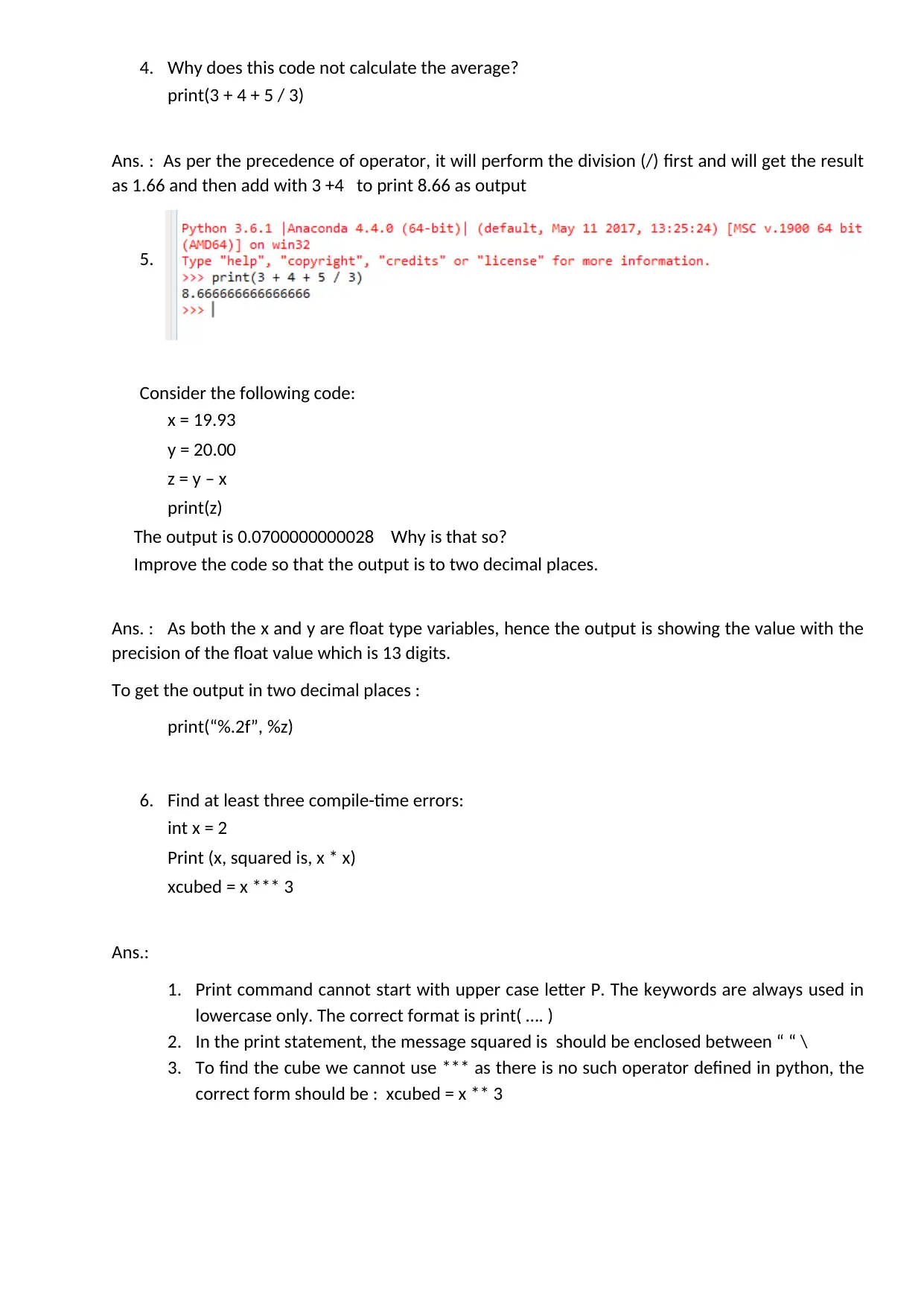
4. Why does this code not calculate the average?
print(3 + 4 + 5 / 3)
Ans. : As per the precedence of operator, it will perform the division (/) first and will get the result
as 1.66 and then add with 3 +4 to print 8.66 as output
5.
Consider the following code:
x = 19.93
y = 20.00
z = y – x
print(z)
The output is 0.0700000000028 Why is that so?
Improve the code so that the output is to two decimal places.
Ans. : As both the x and y are float type variables, hence the output is showing the value with the
precision of the float value which is 13 digits.
To get the output in two decimal places :
print(“%.2f”, %z)
6. Find at least three compile-time errors:
int x = 2
Print (x, squared is, x * x)
xcubed = x *** 3
Ans.:
1. Print command cannot start with upper case letter P. The keywords are always used in
lowercase only. The correct format is print( …. )
2. In the print statement, the message squared is should be enclosed between “ “ \
3. To find the cube we cannot use *** as there is no such operator defined in python, the
correct form should be : xcubed = x ** 3
print(3 + 4 + 5 / 3)
Ans. : As per the precedence of operator, it will perform the division (/) first and will get the result
as 1.66 and then add with 3 +4 to print 8.66 as output
5.
Consider the following code:
x = 19.93
y = 20.00
z = y – x
print(z)
The output is 0.0700000000028 Why is that so?
Improve the code so that the output is to two decimal places.
Ans. : As both the x and y are float type variables, hence the output is showing the value with the
precision of the float value which is 13 digits.
To get the output in two decimal places :
print(“%.2f”, %z)
6. Find at least three compile-time errors:
int x = 2
Print (x, squared is, x * x)
xcubed = x *** 3
Ans.:
1. Print command cannot start with upper case letter P. The keywords are always used in
lowercase only. The correct format is print( …. )
2. In the print statement, the message squared is should be enclosed between “ “ \
3. To find the cube we cannot use *** as there is no such operator defined in python, the
correct form should be : xcubed = x ** 3
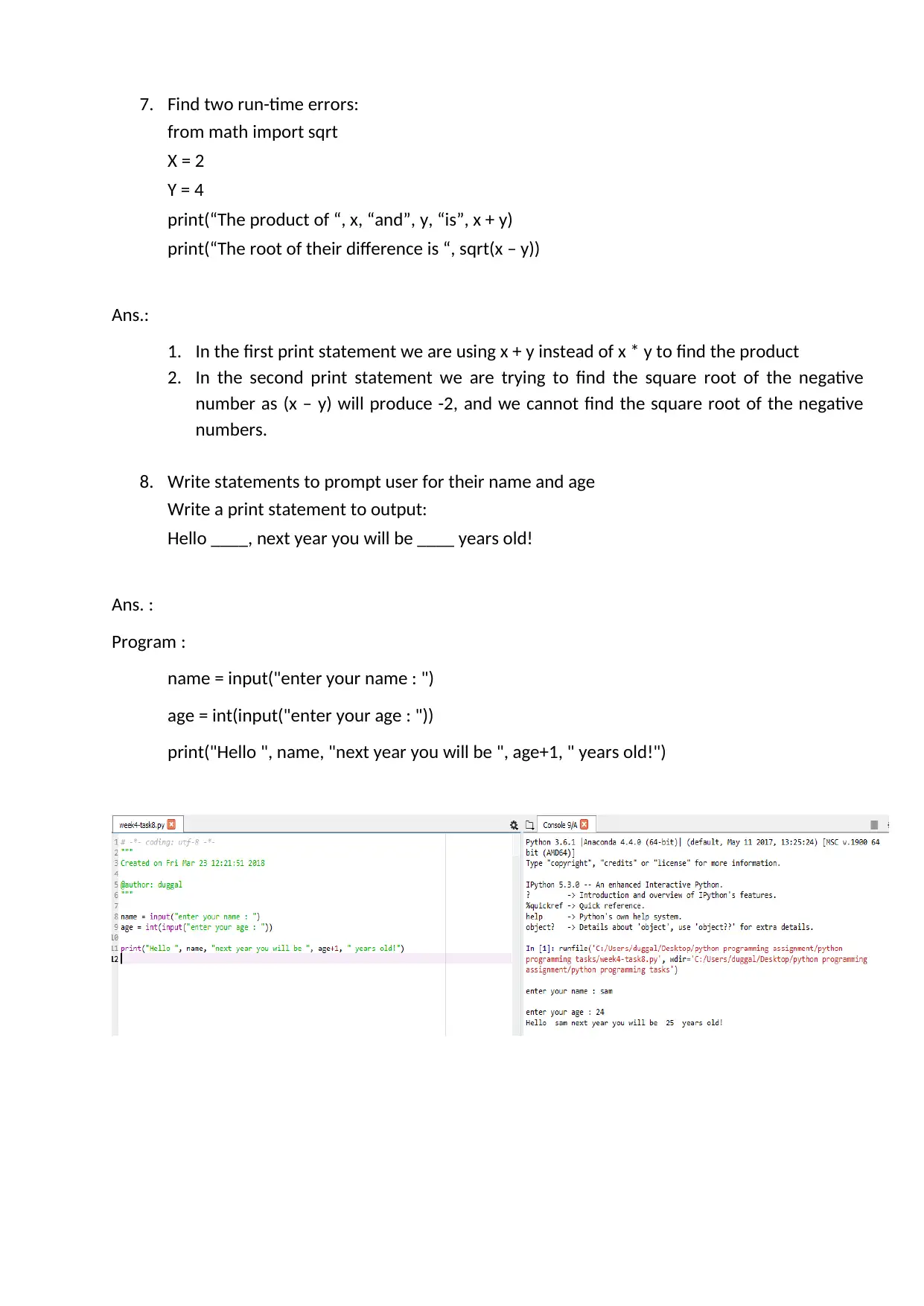
7. Find two run-time errors:
from math import sqrt
X = 2
Y = 4
print(“The product of “, x, “and”, y, “is”, x + y)
print(“The root of their difference is “, sqrt(x – y))
Ans.:
1. In the first print statement we are using x + y instead of x * y to find the product
2. In the second print statement we are trying to find the square root of the negative
number as (x – y) will produce -2, and we cannot find the square root of the negative
numbers.
8. Write statements to prompt user for their name and age
Write a print statement to output:
Hello ____, next year you will be ____ years old!
Ans. :
Program :
name = input("enter your name : ")
age = int(input("enter your age : "))
print("Hello ", name, "next year you will be ", age+1, " years old!")
from math import sqrt
X = 2
Y = 4
print(“The product of “, x, “and”, y, “is”, x + y)
print(“The root of their difference is “, sqrt(x – y))
Ans.:
1. In the first print statement we are using x + y instead of x * y to find the product
2. In the second print statement we are trying to find the square root of the negative
number as (x – y) will produce -2, and we cannot find the square root of the negative
numbers.
8. Write statements to prompt user for their name and age
Write a print statement to output:
Hello ____, next year you will be ____ years old!
Ans. :
Program :
name = input("enter your name : ")
age = int(input("enter your age : "))
print("Hello ", name, "next year you will be ", age+1, " years old!")
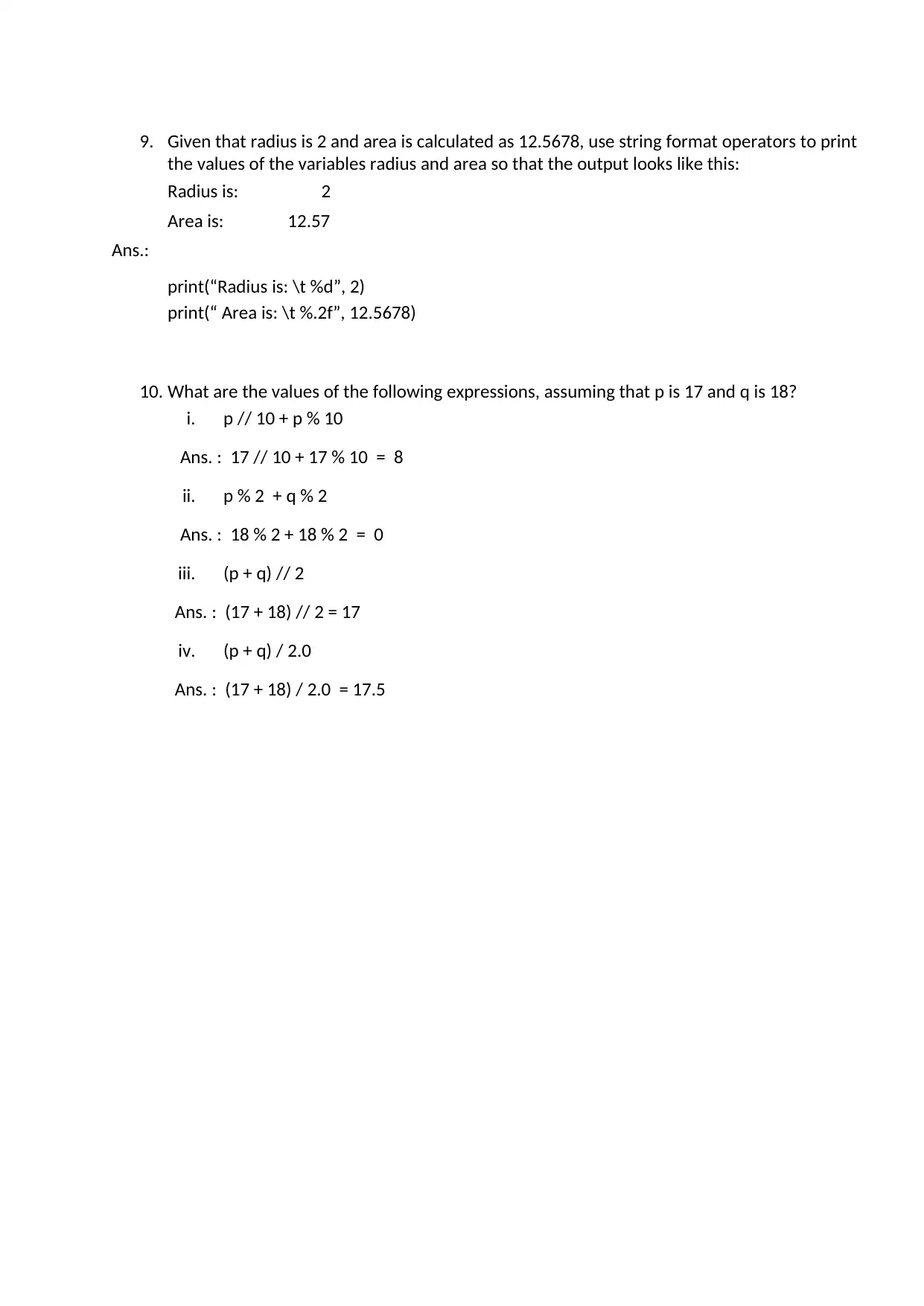
9. Given that radius is 2 and area is calculated as 12.5678, use string format operators to print
the values of the variables radius and area so that the output looks like this:
Radius is: 2
Area is: 12.57
Ans.:
print(“Radius is: \t %d”, 2)
print(“ Area is: \t %.2f”, 12.5678)
10. What are the values of the following expressions, assuming that p is 17 and q is 18?
i. p // 10 + p % 10
Ans. : 17 // 10 + 17 % 10 = 8
ii. p % 2 + q % 2
Ans. : 18 % 2 + 18 % 2 = 0
iii. (p + q) // 2
Ans. : (17 + 18) // 2 = 17
iv. (p + q) / 2.0
Ans. : (17 + 18) / 2.0 = 17.5
the values of the variables radius and area so that the output looks like this:
Radius is: 2
Area is: 12.57
Ans.:
print(“Radius is: \t %d”, 2)
print(“ Area is: \t %.2f”, 12.5678)
10. What are the values of the following expressions, assuming that p is 17 and q is 18?
i. p // 10 + p % 10
Ans. : 17 // 10 + 17 % 10 = 8
ii. p % 2 + q % 2
Ans. : 18 % 2 + 18 % 2 = 0
iii. (p + q) // 2
Ans. : (17 + 18) // 2 = 17
iv. (p + q) / 2.0
Ans. : (17 + 18) / 2.0 = 17.5
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
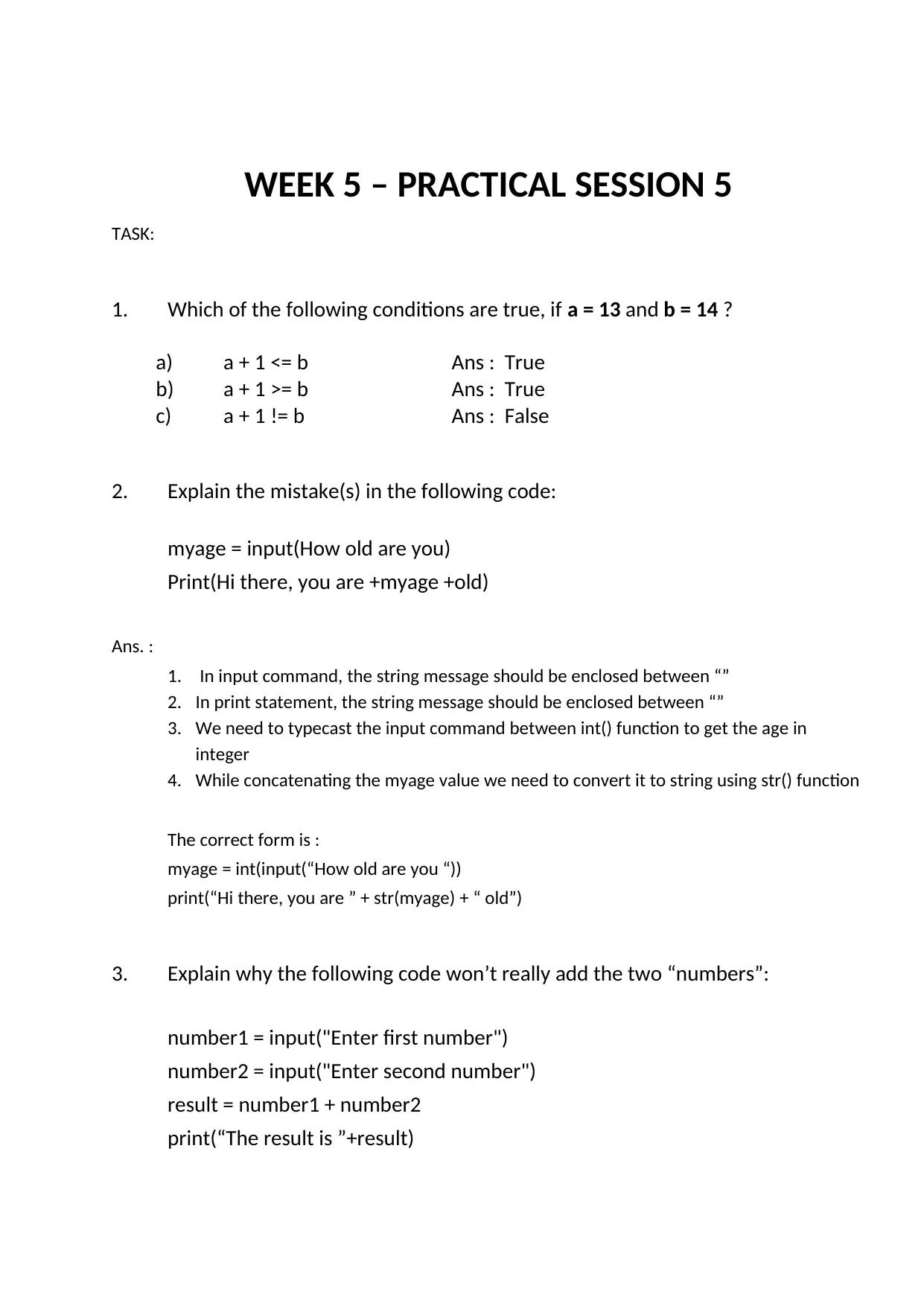
WEEK 5 – PRACTICAL SESSION 5
TASK:
1. Which of the following conditions are true, if a = 13 and b = 14 ?
a) a + 1 <= b Ans : True
b) a + 1 >= b Ans : True
c) a + 1 != b Ans : False
2. Explain the mistake(s) in the following code:
myage = input(How old are you)
Print(Hi there, you are +myage +old)
Ans. :
1. In input command, the string message should be enclosed between “”
2. In print statement, the string message should be enclosed between “”
3. We need to typecast the input command between int() function to get the age in
integer
4. While concatenating the myage value we need to convert it to string using str() function
The correct form is :
myage = int(input(“How old are you “))
print(“Hi there, you are ” + str(myage) + “ old”)
3. Explain why the following code won’t really add the two “numbers”:
number1 = input("Enter first number")
number2 = input("Enter second number")
result = number1 + number2
print(“The result is ”+result)
TASK:
1. Which of the following conditions are true, if a = 13 and b = 14 ?
a) a + 1 <= b Ans : True
b) a + 1 >= b Ans : True
c) a + 1 != b Ans : False
2. Explain the mistake(s) in the following code:
myage = input(How old are you)
Print(Hi there, you are +myage +old)
Ans. :
1. In input command, the string message should be enclosed between “”
2. In print statement, the string message should be enclosed between “”
3. We need to typecast the input command between int() function to get the age in
integer
4. While concatenating the myage value we need to convert it to string using str() function
The correct form is :
myage = int(input(“How old are you “))
print(“Hi there, you are ” + str(myage) + “ old”)
3. Explain why the following code won’t really add the two “numbers”:
number1 = input("Enter first number")
number2 = input("Enter second number")
result = number1 + number2
print(“The result is ”+result)
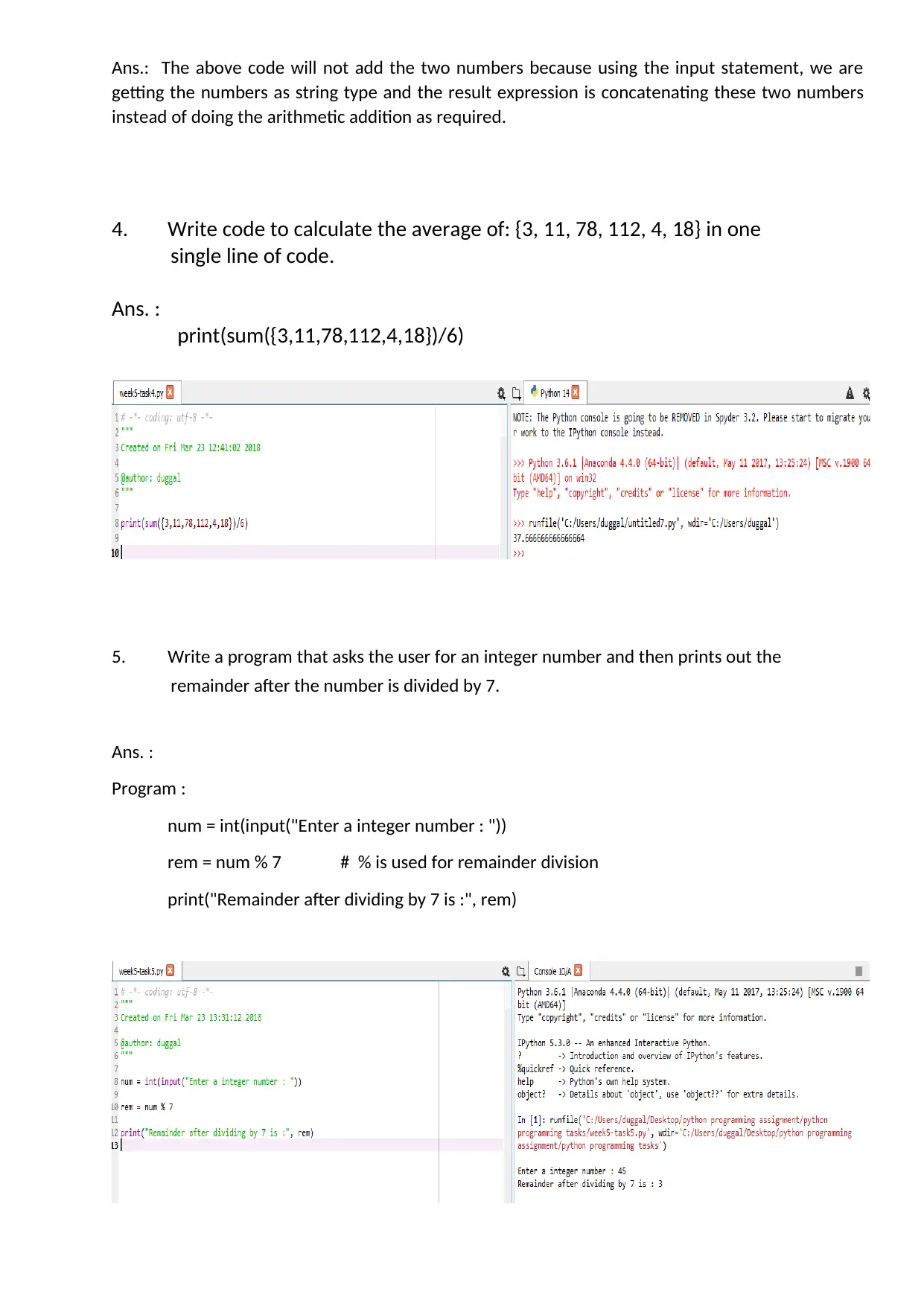
Ans.: The above code will not add the two numbers because using the input statement, we are
getting the numbers as string type and the result expression is concatenating these two numbers
instead of doing the arithmetic addition as required.
4. Write code to calculate the average of: {3, 11, 78, 112, 4, 18} in one
single line of code.
Ans. :
print(sum({3,11,78,112,4,18})/6)
5. Write a program that asks the user for an integer number and then prints out the
remainder after the number is divided by 7.
Ans. :
Program :
num = int(input("Enter a integer number : "))
rem = num % 7 # % is used for remainder division
print("Remainder after dividing by 7 is :", rem)
getting the numbers as string type and the result expression is concatenating these two numbers
instead of doing the arithmetic addition as required.
4. Write code to calculate the average of: {3, 11, 78, 112, 4, 18} in one
single line of code.
Ans. :
print(sum({3,11,78,112,4,18})/6)
5. Write a program that asks the user for an integer number and then prints out the
remainder after the number is divided by 7.
Ans. :
Program :
num = int(input("Enter a integer number : "))
rem = num % 7 # % is used for remainder division
print("Remainder after dividing by 7 is :", rem)
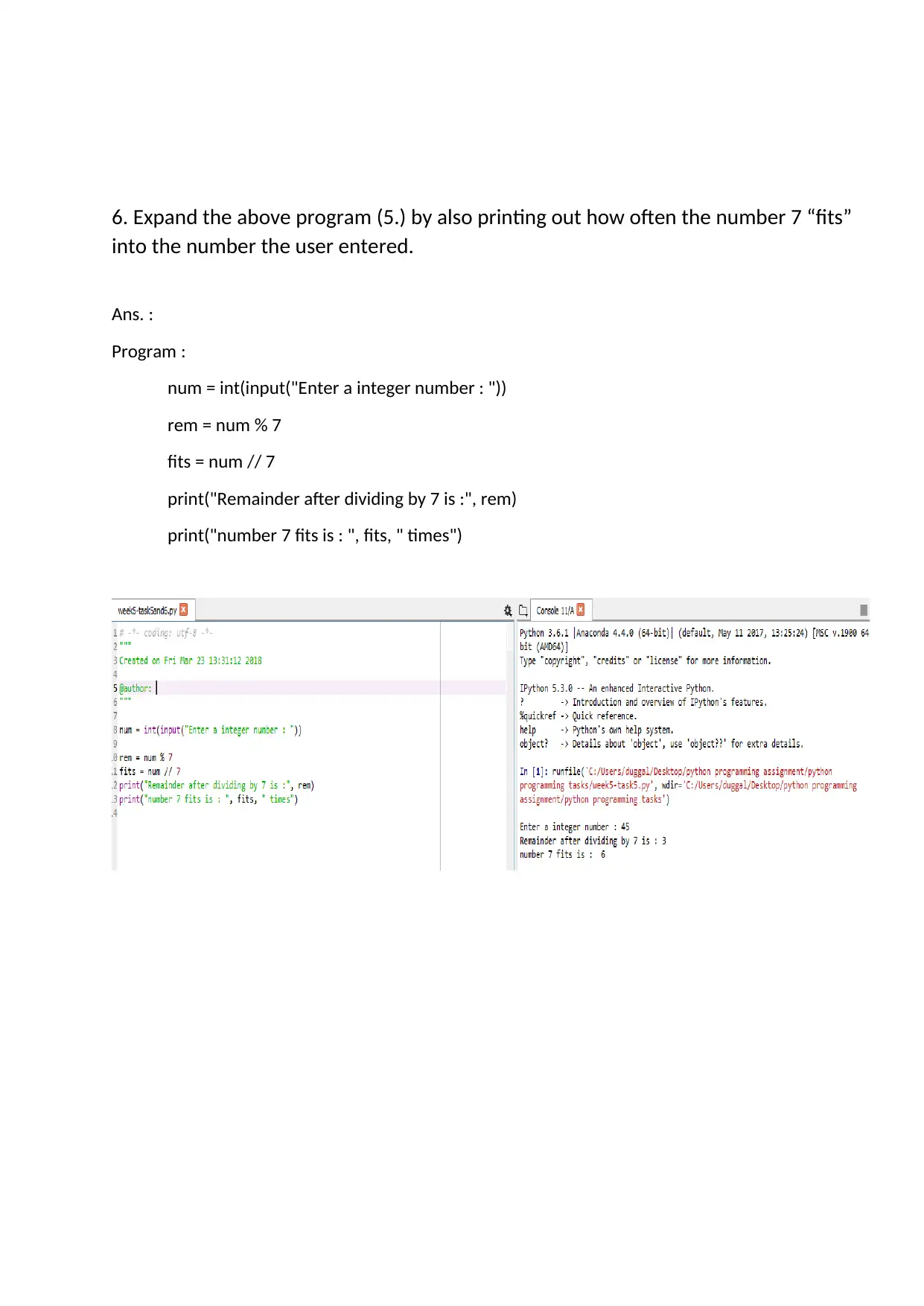
6. Expand the above program (5.) by also printing out how often the number 7 “fits”
into the number the user entered.
Ans. :
Program :
num = int(input("Enter a integer number : "))
rem = num % 7
fits = num // 7
print("Remainder after dividing by 7 is :", rem)
print("number 7 fits is : ", fits, " times")
into the number the user entered.
Ans. :
Program :
num = int(input("Enter a integer number : "))
rem = num % 7
fits = num // 7
print("Remainder after dividing by 7 is :", rem)
print("number 7 fits is : ", fits, " times")
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
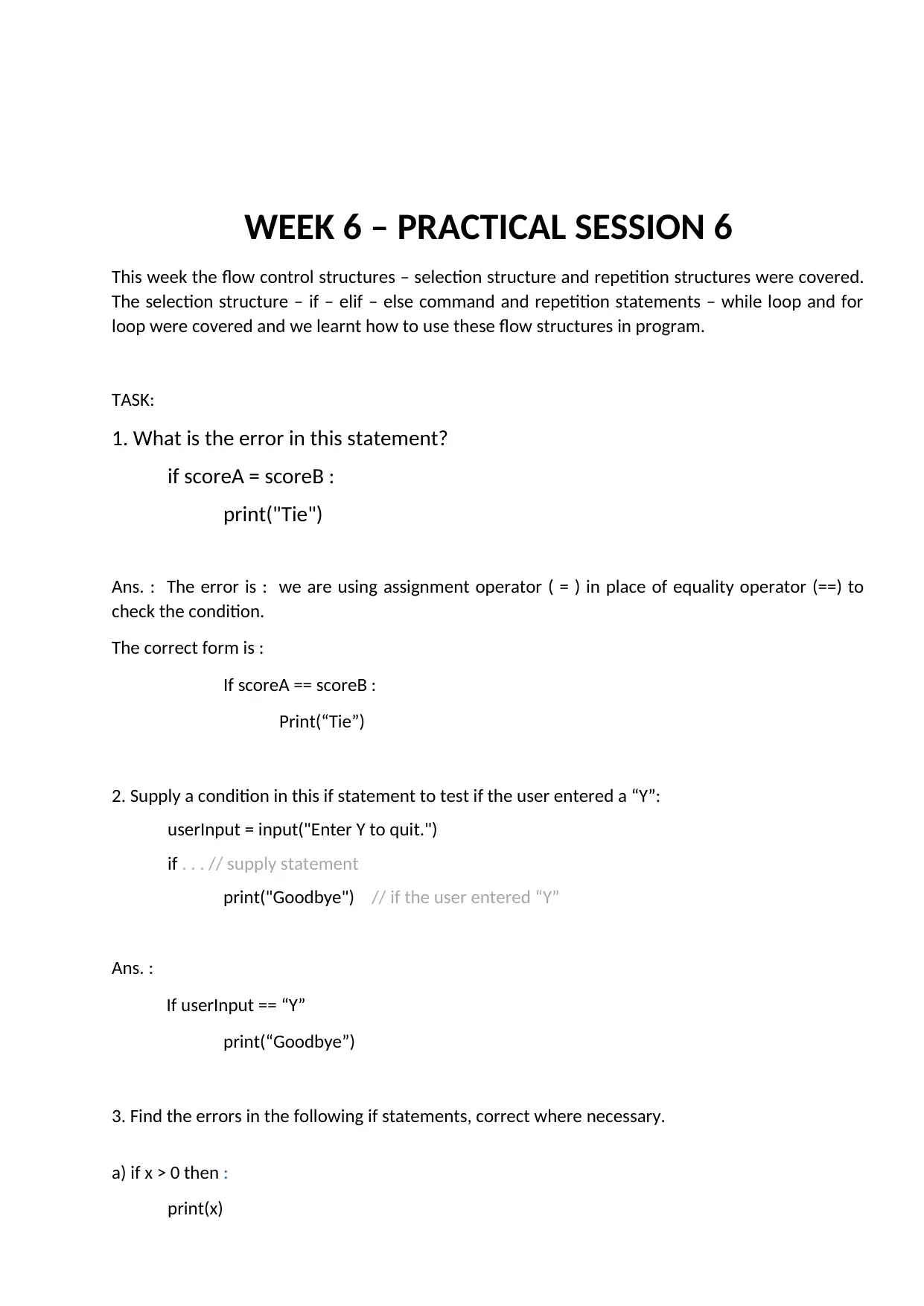
WEEK 6 – PRACTICAL SESSION 6
This week the flow control structures – selection structure and repetition structures were covered.
The selection structure – if – elif – else command and repetition statements – while loop and for
loop were covered and we learnt how to use these flow structures in program.
TASK:
1. What is the error in this statement?
if scoreA = scoreB :
print("Tie")
Ans. : The error is : we are using assignment operator ( = ) in place of equality operator (==) to
check the condition.
The correct form is :
If scoreA == scoreB :
Print(“Tie”)
2. Supply a condition in this if statement to test if the user entered a “Y”:
userInput = input("Enter Y to quit.")
if . . . // supply statement
print("Goodbye") // if the user entered “Y”
Ans. :
If userInput == “Y”
print(“Goodbye”)
3. Find the errors in the following if statements, correct where necessary.
a) if x > 0 then :
print(x)
This week the flow control structures – selection structure and repetition structures were covered.
The selection structure – if – elif – else command and repetition statements – while loop and for
loop were covered and we learnt how to use these flow structures in program.
TASK:
1. What is the error in this statement?
if scoreA = scoreB :
print("Tie")
Ans. : The error is : we are using assignment operator ( = ) in place of equality operator (==) to
check the condition.
The correct form is :
If scoreA == scoreB :
Print(“Tie”)
2. Supply a condition in this if statement to test if the user entered a “Y”:
userInput = input("Enter Y to quit.")
if . . . // supply statement
print("Goodbye") // if the user entered “Y”
Ans. :
If userInput == “Y”
print(“Goodbye”)
3. Find the errors in the following if statements, correct where necessary.
a) if x > 0 then :
print(x)
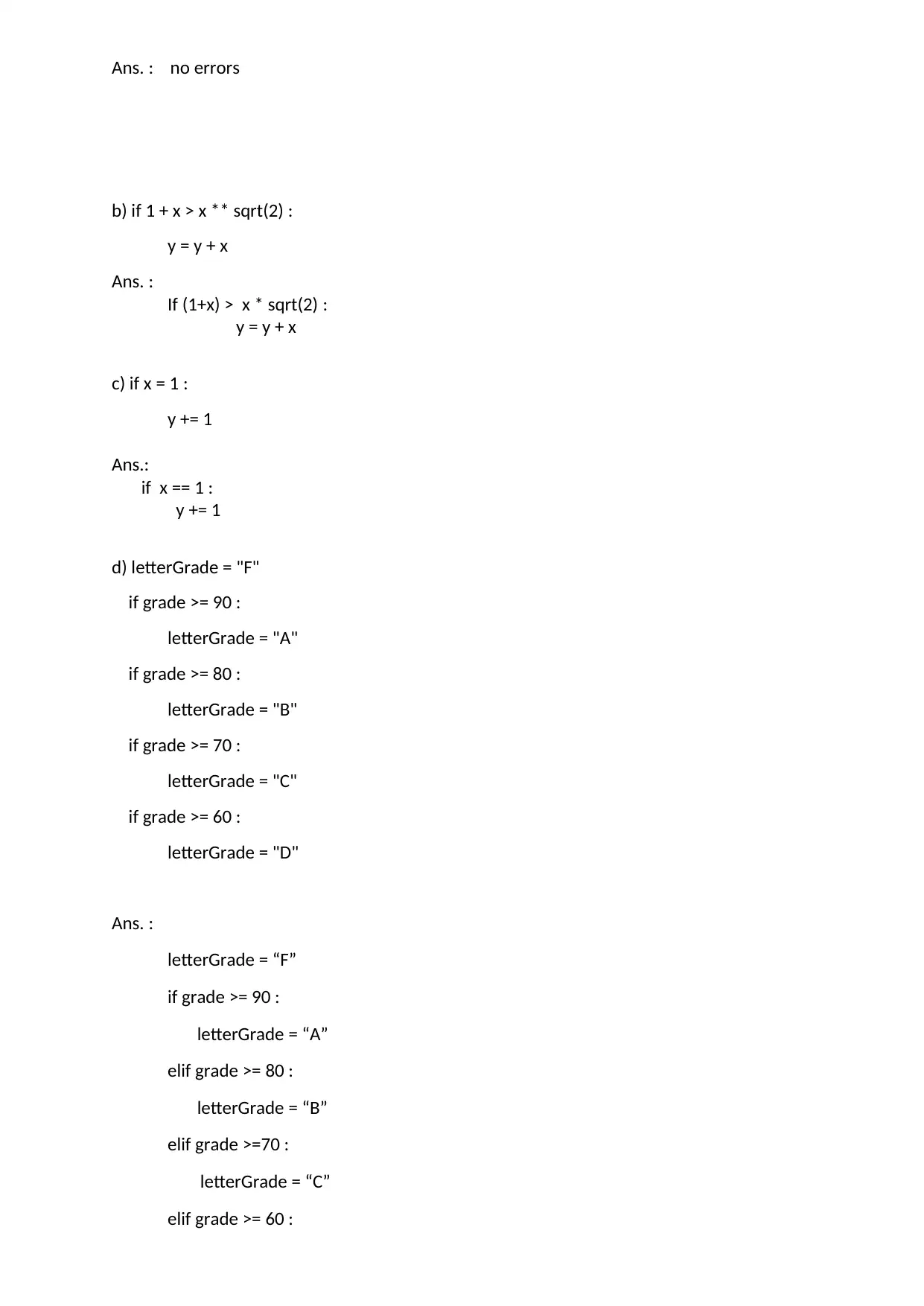
Ans. : no errors
b) if 1 + x > x ** sqrt(2) :
y = y + x
Ans. :
If (1+x) > x * sqrt(2) :
y = y + x
c) if x = 1 :
y += 1
Ans.:
if x == 1 :
y += 1
d) letterGrade = "F"
if grade >= 90 :
letterGrade = "A"
if grade >= 80 :
letterGrade = "B"
if grade >= 70 :
letterGrade = "C"
if grade >= 60 :
letterGrade = "D"
Ans. :
letterGrade = “F”
if grade >= 90 :
letterGrade = “A”
elif grade >= 80 :
letterGrade = “B”
elif grade >=70 :
letterGrade = “C”
elif grade >= 60 :
b) if 1 + x > x ** sqrt(2) :
y = y + x
Ans. :
If (1+x) > x * sqrt(2) :
y = y + x
c) if x = 1 :
y += 1
Ans.:
if x == 1 :
y += 1
d) letterGrade = "F"
if grade >= 90 :
letterGrade = "A"
if grade >= 80 :
letterGrade = "B"
if grade >= 70 :
letterGrade = "C"
if grade >= 60 :
letterGrade = "D"
Ans. :
letterGrade = “F”
if grade >= 90 :
letterGrade = “A”
elif grade >= 80 :
letterGrade = “B”
elif grade >=70 :
letterGrade = “C”
elif grade >= 60 :
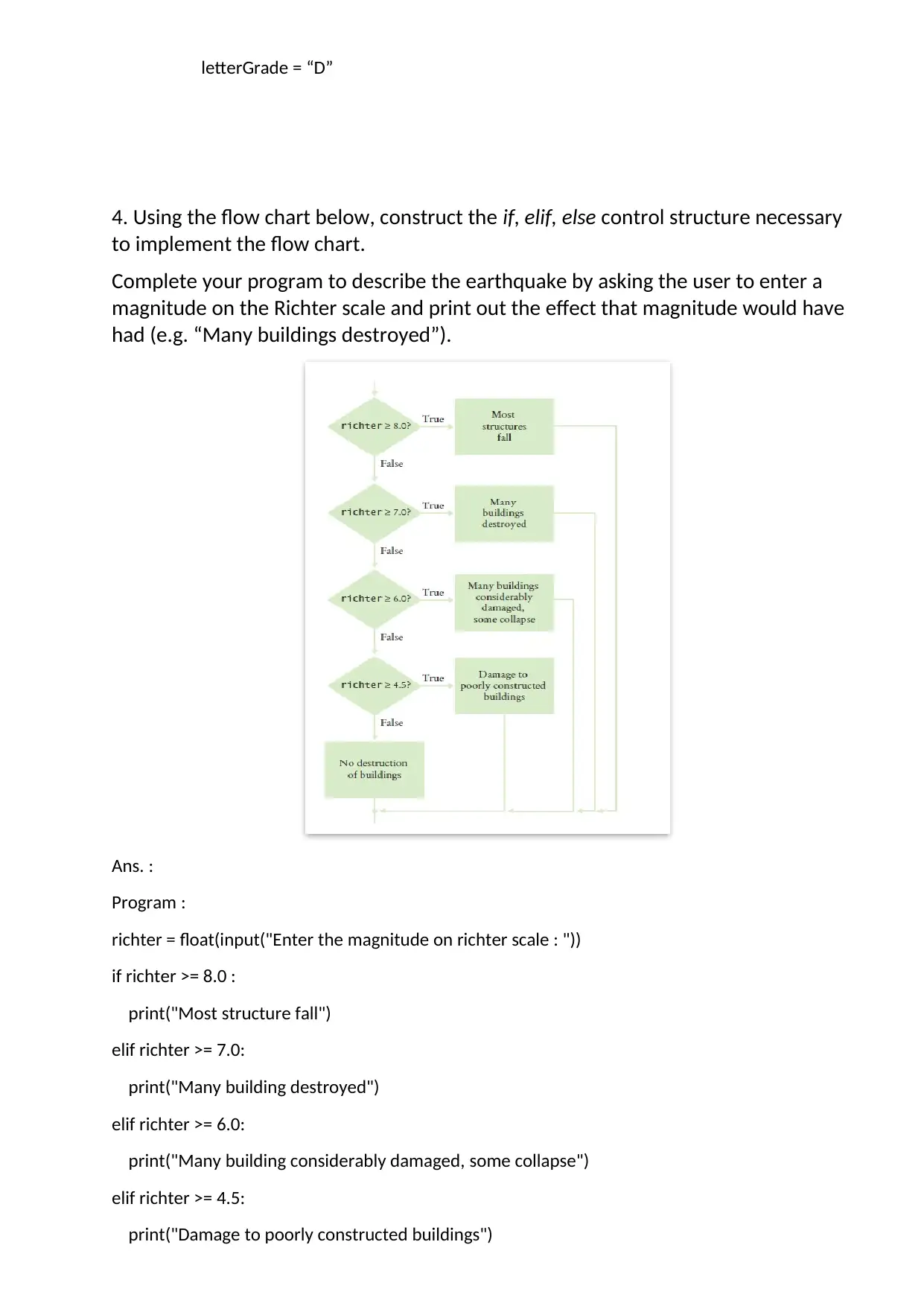
letterGrade = “D”
4. Using the flow chart below, construct the if, elif, else control structure necessary
to implement the flow chart.
Complete your program to describe the earthquake by asking the user to enter a
magnitude on the Richter scale and print out the effect that magnitude would have
had (e.g. “Many buildings destroyed”).
Ans. :
Program :
richter = float(input("Enter the magnitude on richter scale : "))
if richter >= 8.0 :
print("Most structure fall")
elif richter >= 7.0:
print("Many building destroyed")
elif richter >= 6.0:
print("Many building considerably damaged, some collapse")
elif richter >= 4.5:
print("Damage to poorly constructed buildings")
4. Using the flow chart below, construct the if, elif, else control structure necessary
to implement the flow chart.
Complete your program to describe the earthquake by asking the user to enter a
magnitude on the Richter scale and print out the effect that magnitude would have
had (e.g. “Many buildings destroyed”).
Ans. :
Program :
richter = float(input("Enter the magnitude on richter scale : "))
if richter >= 8.0 :
print("Most structure fall")
elif richter >= 7.0:
print("Many building destroyed")
elif richter >= 6.0:
print("Many building considerably damaged, some collapse")
elif richter >= 4.5:
print("Damage to poorly constructed buildings")
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
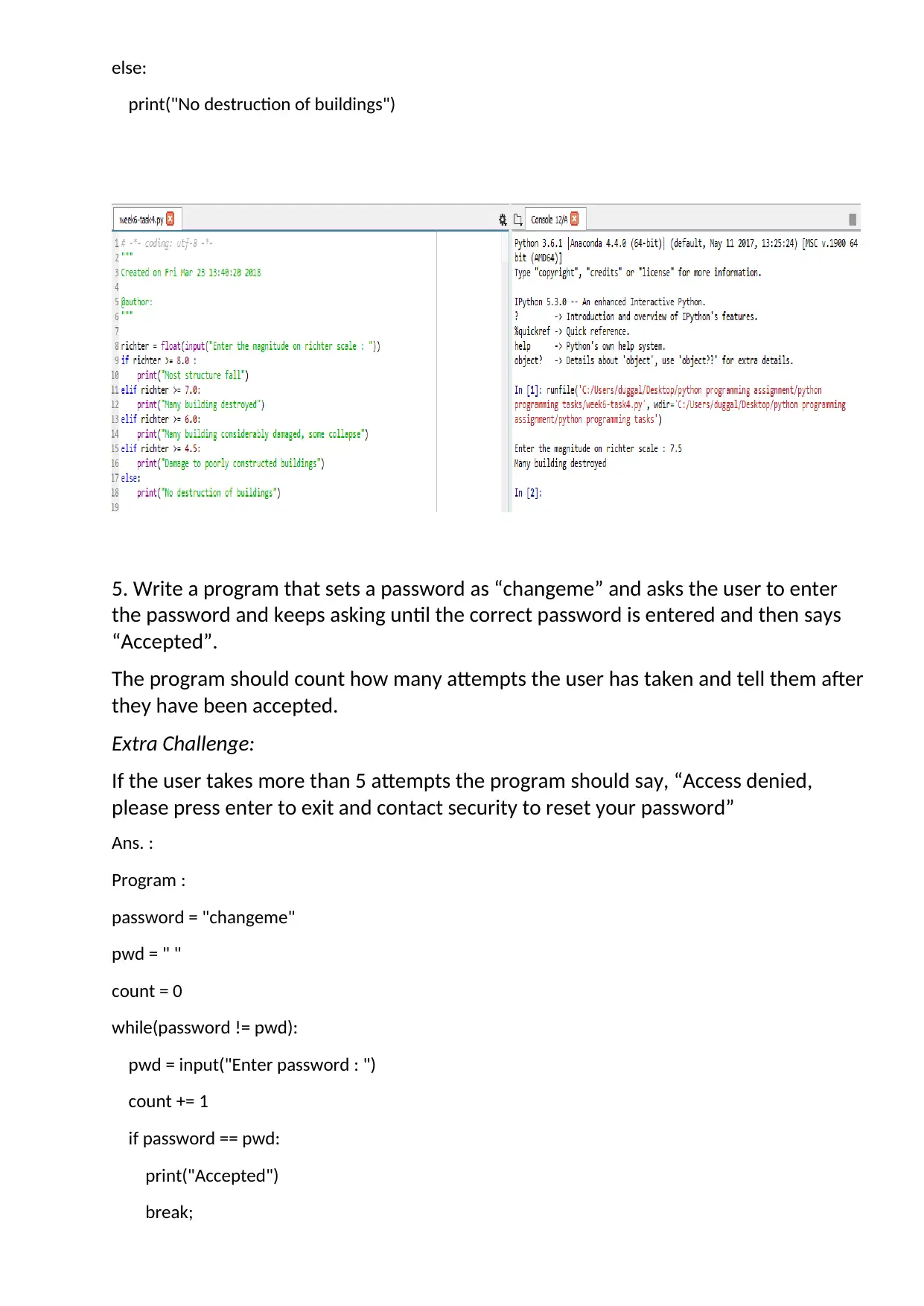
else:
print("No destruction of buildings")
5. Write a program that sets a password as “changeme” and asks the user to enter
the password and keeps asking until the correct password is entered and then says
“Accepted”.
The program should count how many attempts the user has taken and tell them after
they have been accepted.
Extra Challenge:
If the user takes more than 5 attempts the program should say, “Access denied,
please press enter to exit and contact security to reset your password”
Ans. :
Program :
password = "changeme"
pwd = " "
count = 0
while(password != pwd):
pwd = input("Enter password : ")
count += 1
if password == pwd:
print("Accepted")
break;
print("No destruction of buildings")
5. Write a program that sets a password as “changeme” and asks the user to enter
the password and keeps asking until the correct password is entered and then says
“Accepted”.
The program should count how many attempts the user has taken and tell them after
they have been accepted.
Extra Challenge:
If the user takes more than 5 attempts the program should say, “Access denied,
please press enter to exit and contact security to reset your password”
Ans. :
Program :
password = "changeme"
pwd = " "
count = 0
while(password != pwd):
pwd = input("Enter password : ")
count += 1
if password == pwd:
print("Accepted")
break;
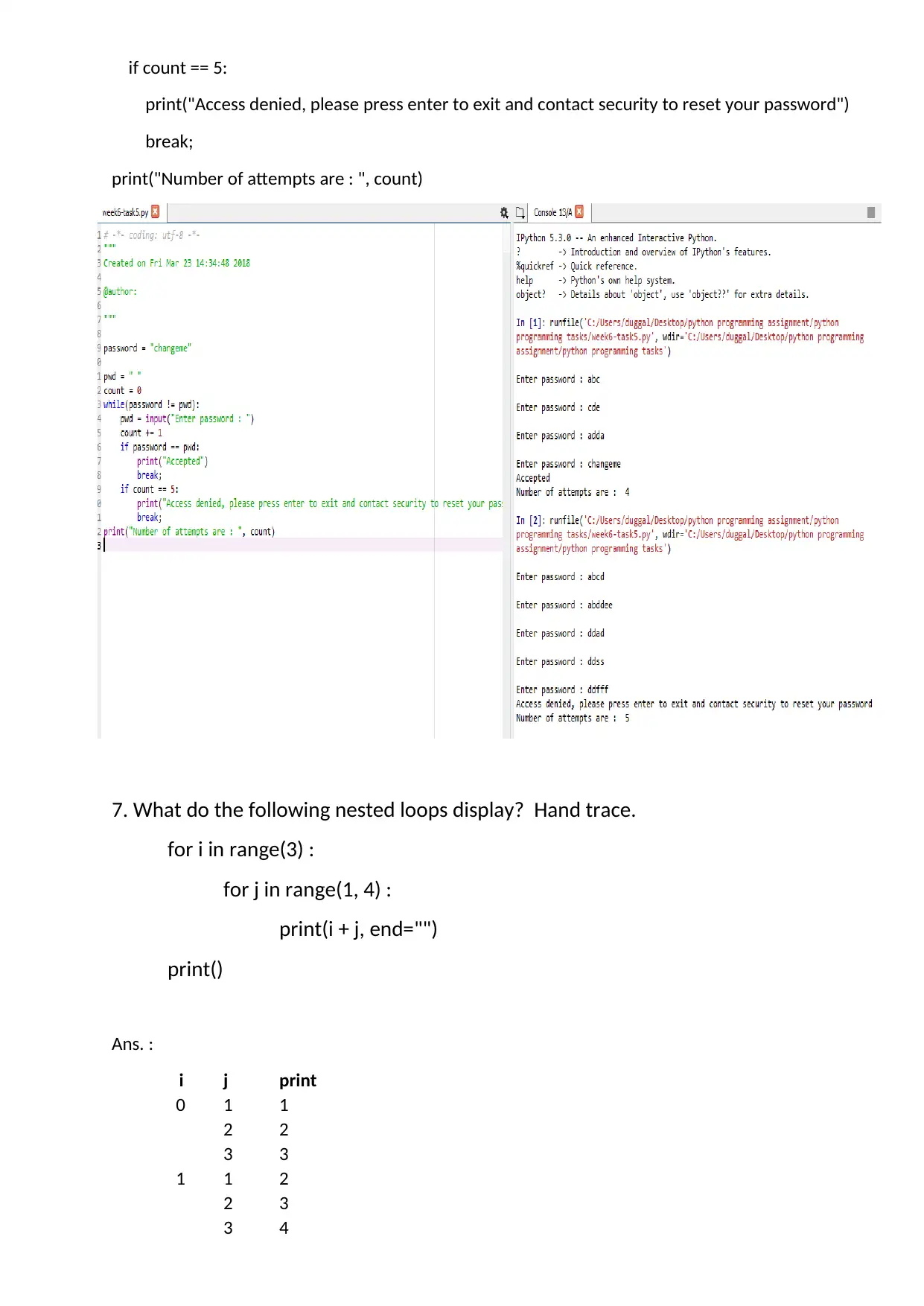
if count == 5:
print("Access denied, please press enter to exit and contact security to reset your password")
break;
print("Number of attempts are : ", count)
7. What do the following nested loops display? Hand trace.
for i in range(3) :
for j in range(1, 4) :
print(i + j, end="")
print()
Ans. :
i j print
0 1 1
2 2
3 3
1 1 2
2 3
3 4
print("Access denied, please press enter to exit and contact security to reset your password")
break;
print("Number of attempts are : ", count)
7. What do the following nested loops display? Hand trace.
for i in range(3) :
for j in range(1, 4) :
print(i + j, end="")
print()
Ans. :
i j print
0 1 1
2 2
3 3
1 1 2
2 3
3 4
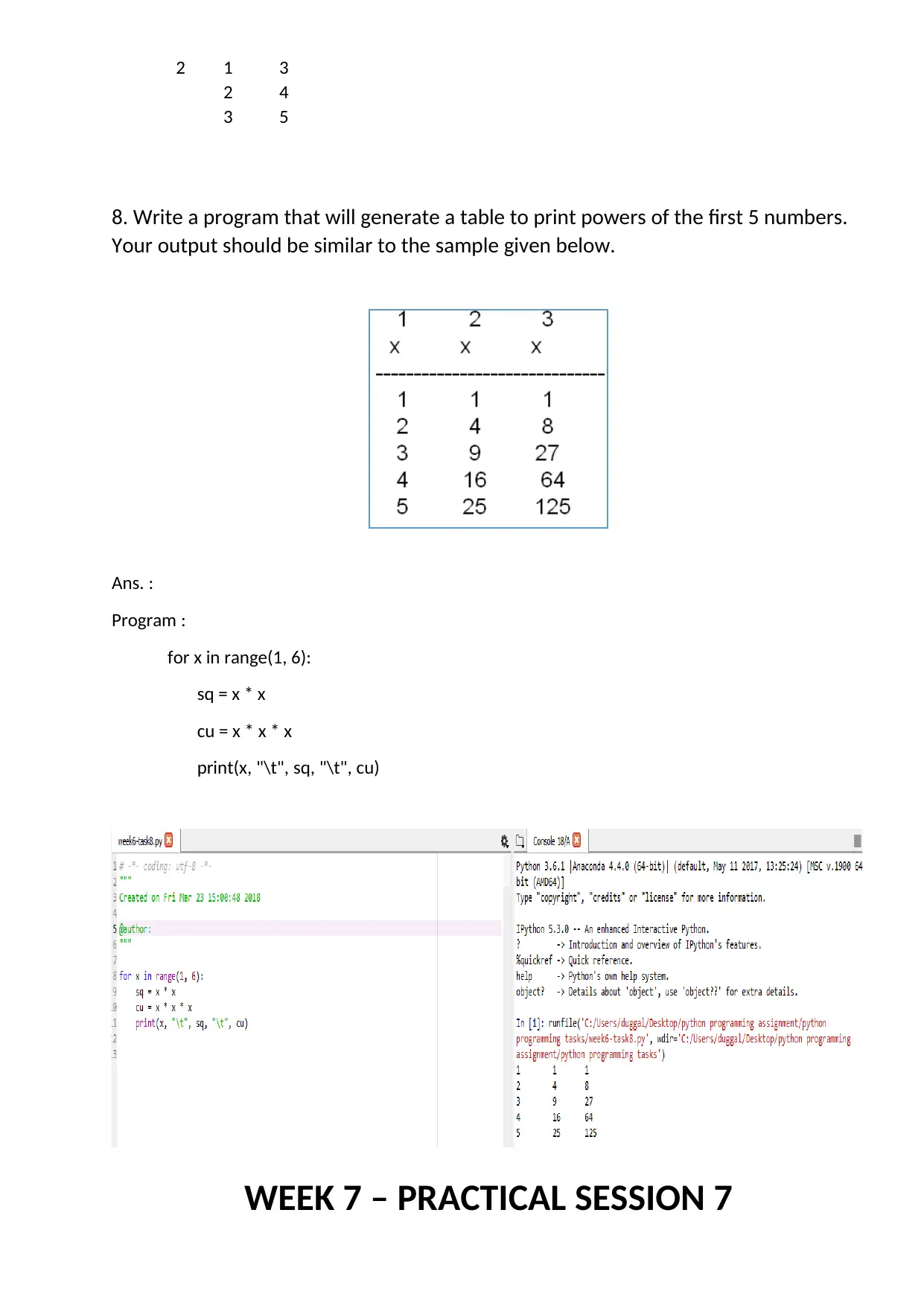
2 1 3
2 4
3 5
8. Write a program that will generate a table to print powers of the first 5 numbers.
Your output should be similar to the sample given below.
Ans. :
Program :
for x in range(1, 6):
sq = x * x
cu = x * x * x
print(x, "\t", sq, "\t", cu)
WEEK 7 – PRACTICAL SESSION 7
2 4
3 5
8. Write a program that will generate a table to print powers of the first 5 numbers.
Your output should be similar to the sample given below.
Ans. :
Program :
for x in range(1, 6):
sq = x * x
cu = x * x * x
print(x, "\t", sq, "\t", cu)
WEEK 7 – PRACTICAL SESSION 7
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
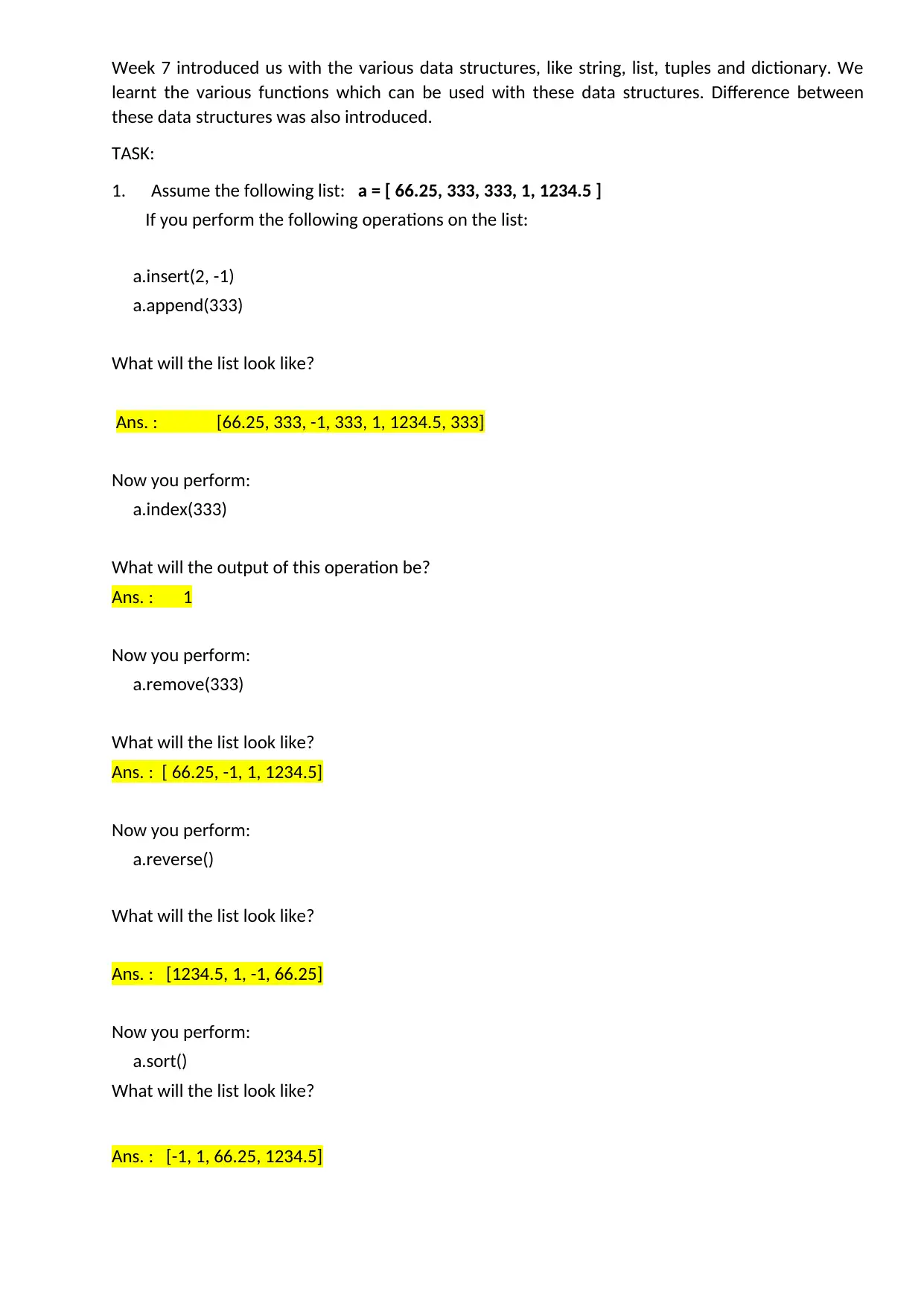
Week 7 introduced us with the various data structures, like string, list, tuples and dictionary. We
learnt the various functions which can be used with these data structures. Difference between
these data structures was also introduced.
TASK:
1. Assume the following list: a = [ 66.25, 333, 333, 1, 1234.5 ]
If you perform the following operations on the list:
a.insert(2, -1)
a.append(333)
What will the list look like?
Ans. : [66.25, 333, -1, 333, 1, 1234.5, 333]
Now you perform:
a.index(333)
What will the output of this operation be?
Ans. : 1
Now you perform:
a.remove(333)
What will the list look like?
Ans. : [ 66.25, -1, 1, 1234.5]
Now you perform:
a.reverse()
What will the list look like?
Ans. : [1234.5, 1, -1, 66.25]
Now you perform:
a.sort()
What will the list look like?
Ans. : [-1, 1, 66.25, 1234.5]
learnt the various functions which can be used with these data structures. Difference between
these data structures was also introduced.
TASK:
1. Assume the following list: a = [ 66.25, 333, 333, 1, 1234.5 ]
If you perform the following operations on the list:
a.insert(2, -1)
a.append(333)
What will the list look like?
Ans. : [66.25, 333, -1, 333, 1, 1234.5, 333]
Now you perform:
a.index(333)
What will the output of this operation be?
Ans. : 1
Now you perform:
a.remove(333)
What will the list look like?
Ans. : [ 66.25, -1, 1, 1234.5]
Now you perform:
a.reverse()
What will the list look like?
Ans. : [1234.5, 1, -1, 66.25]
Now you perform:
a.sort()
What will the list look like?
Ans. : [-1, 1, 66.25, 1234.5]
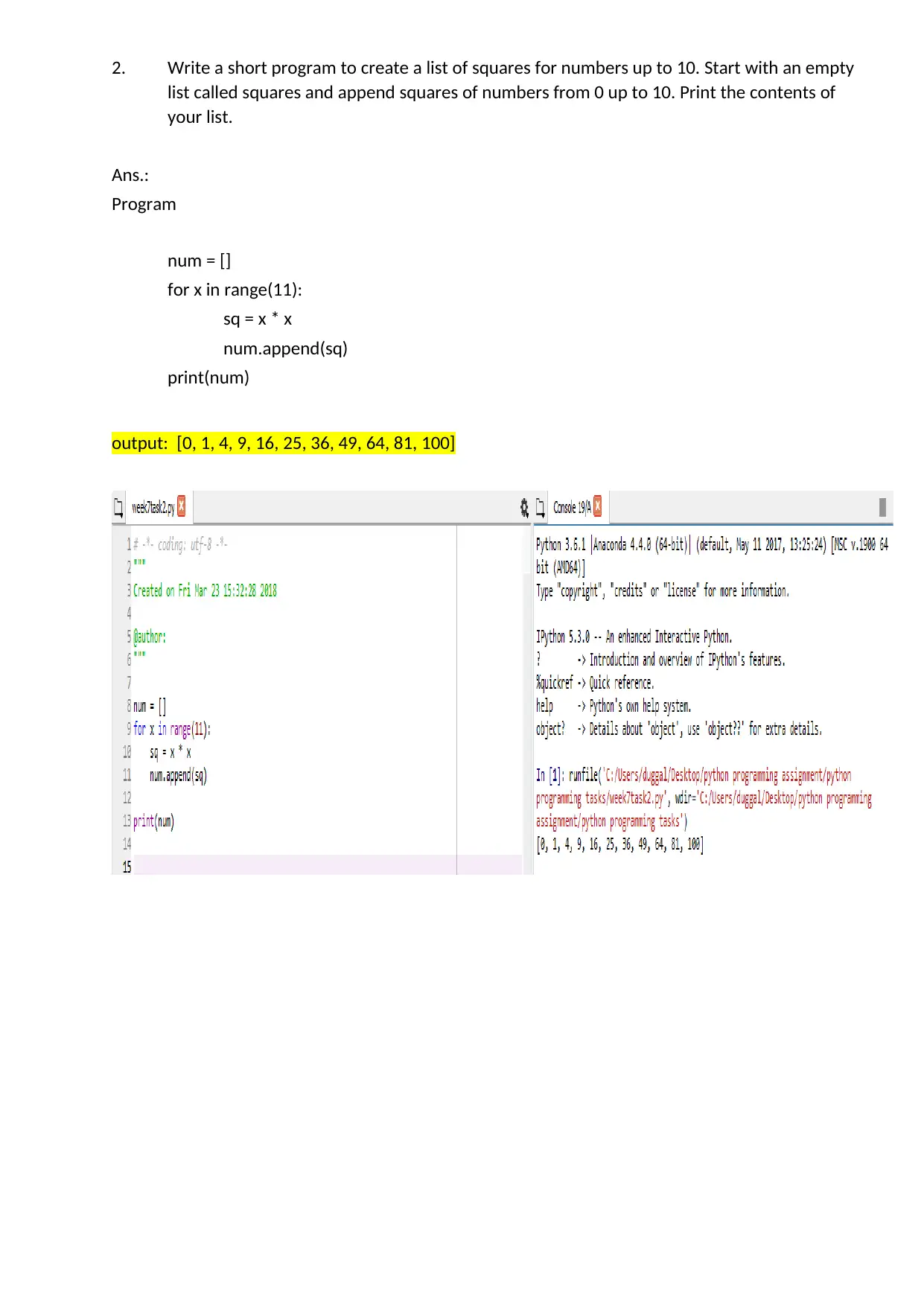
2. Write a short program to create a list of squares for numbers up to 10. Start with an empty
list called squares and append squares of numbers from 0 up to 10. Print the contents of
your list.
Ans.:
Program
num = []
for x in range(11):
sq = x * x
num.append(sq)
print(num)
output: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
list called squares and append squares of numbers from 0 up to 10. Print the contents of
your list.
Ans.:
Program
num = []
for x in range(11):
sq = x * x
num.append(sq)
print(num)
output: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
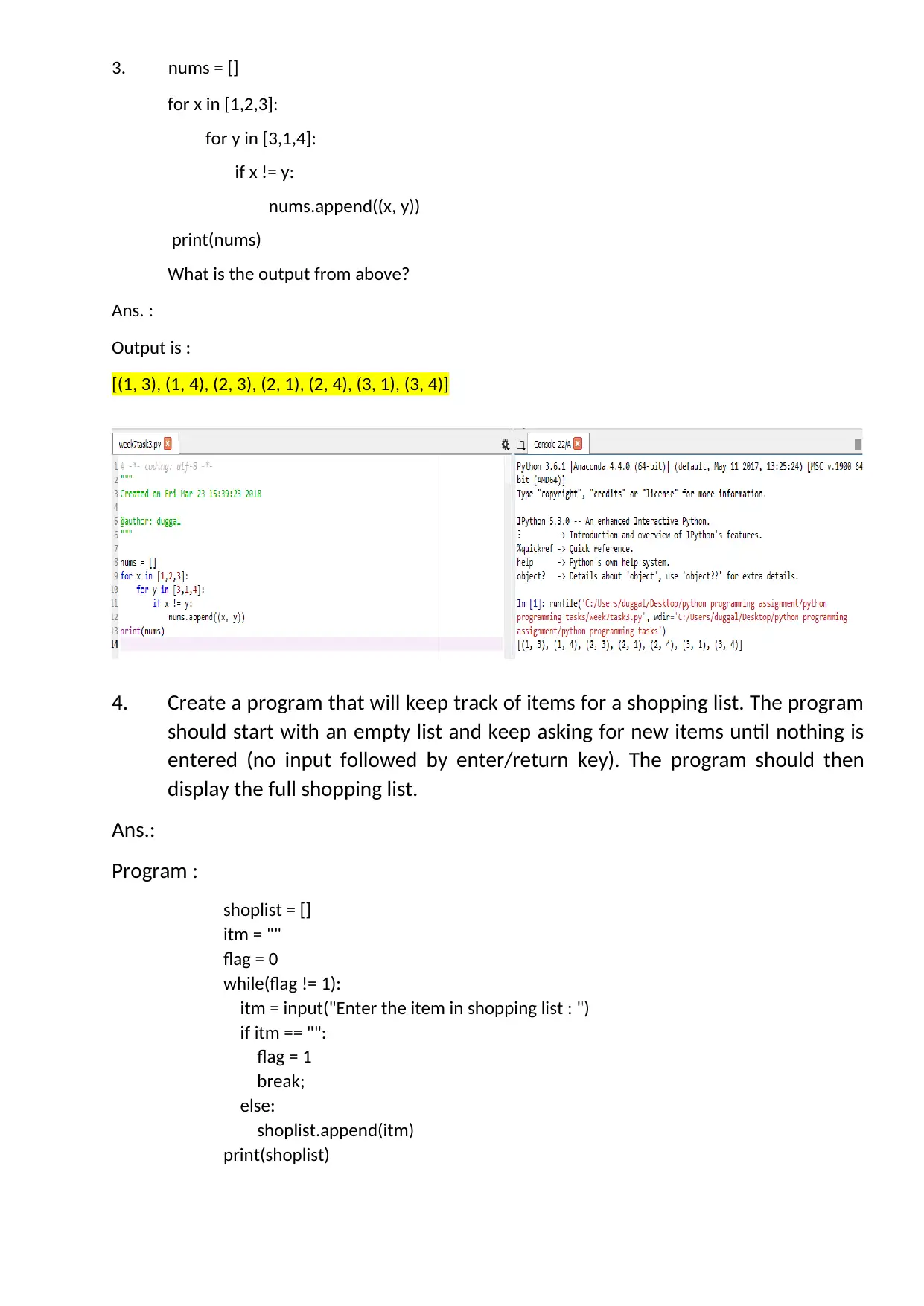
3. nums = []
for x in [1,2,3]:
for y in [3,1,4]:
if x != y:
nums.append((x, y))
print(nums)
What is the output from above?
Ans. :
Output is :
[(1, 3), (1, 4), (2, 3), (2, 1), (2, 4), (3, 1), (3, 4)]
4. Create a program that will keep track of items for a shopping list. The program
should start with an empty list and keep asking for new items until nothing is
entered (no input followed by enter/return key). The program should then
display the full shopping list.
Ans.:
Program :
shoplist = []
itm = ""
flag = 0
while(flag != 1):
itm = input("Enter the item in shopping list : ")
if itm == "":
flag = 1
break;
else:
shoplist.append(itm)
print(shoplist)
for x in [1,2,3]:
for y in [3,1,4]:
if x != y:
nums.append((x, y))
print(nums)
What is the output from above?
Ans. :
Output is :
[(1, 3), (1, 4), (2, 3), (2, 1), (2, 4), (3, 1), (3, 4)]
4. Create a program that will keep track of items for a shopping list. The program
should start with an empty list and keep asking for new items until nothing is
entered (no input followed by enter/return key). The program should then
display the full shopping list.
Ans.:
Program :
shoplist = []
itm = ""
flag = 0
while(flag != 1):
itm = input("Enter the item in shopping list : ")
if itm == "":
flag = 1
break;
else:
shoplist.append(itm)
print(shoplist)
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
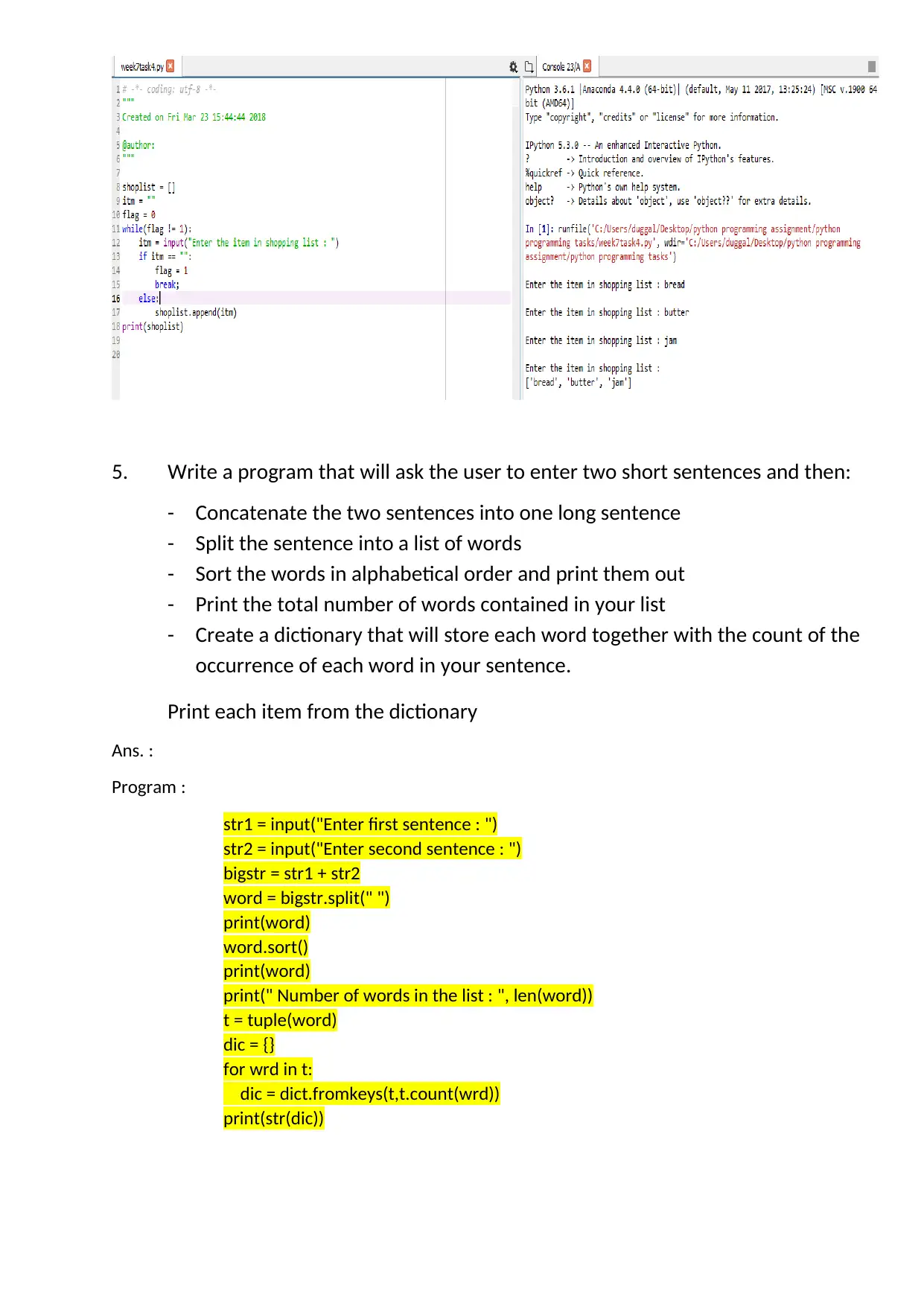
5. Write a program that will ask the user to enter two short sentences and then:
- Concatenate the two sentences into one long sentence
- Split the sentence into a list of words
- Sort the words in alphabetical order and print them out
- Print the total number of words contained in your list
- Create a dictionary that will store each word together with the count of the
occurrence of each word in your sentence.
Print each item from the dictionary
Ans. :
Program :
str1 = input("Enter first sentence : ")
str2 = input("Enter second sentence : ")
bigstr = str1 + str2
word = bigstr.split(" ")
print(word)
word.sort()
print(word)
print(" Number of words in the list : ", len(word))
t = tuple(word)
dic = {}
for wrd in t:
dic = dict.fromkeys(t,t.count(wrd))
print(str(dic))
- Concatenate the two sentences into one long sentence
- Split the sentence into a list of words
- Sort the words in alphabetical order and print them out
- Print the total number of words contained in your list
- Create a dictionary that will store each word together with the count of the
occurrence of each word in your sentence.
Print each item from the dictionary
Ans. :
Program :
str1 = input("Enter first sentence : ")
str2 = input("Enter second sentence : ")
bigstr = str1 + str2
word = bigstr.split(" ")
print(word)
word.sort()
print(word)
print(" Number of words in the list : ", len(word))
t = tuple(word)
dic = {}
for wrd in t:
dic = dict.fromkeys(t,t.count(wrd))
print(str(dic))
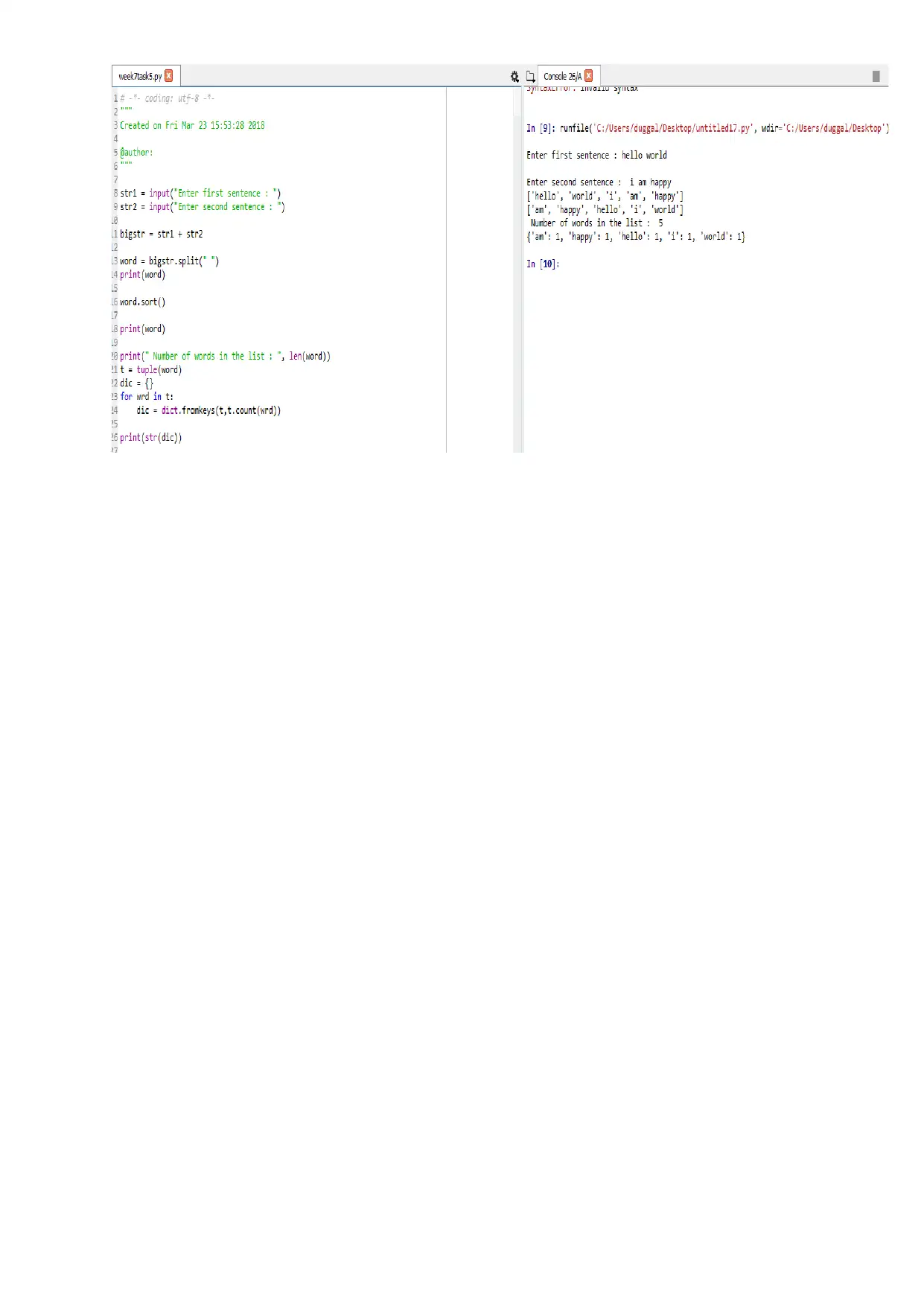
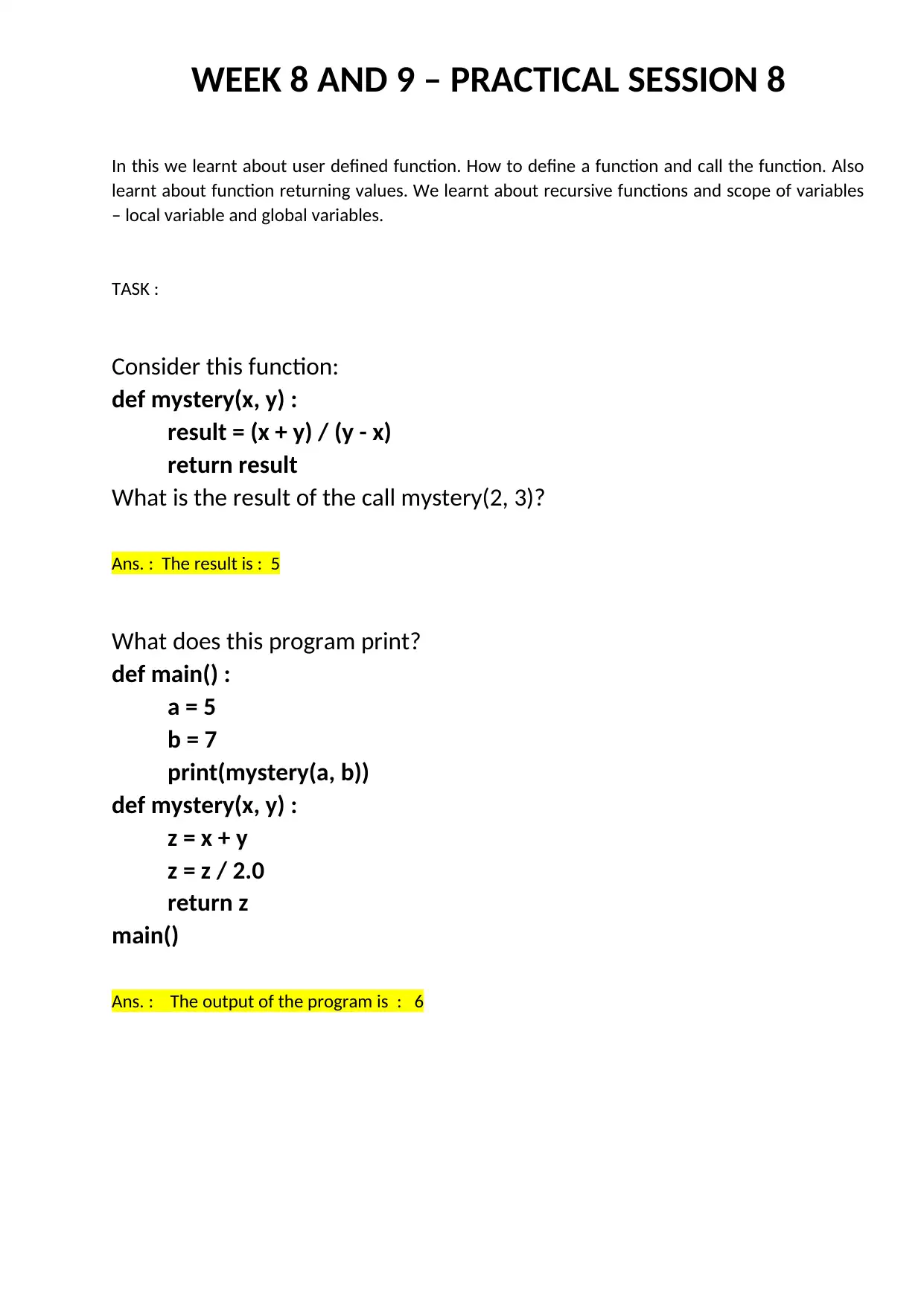
WEEK 8 AND 9 – PRACTICAL SESSION 8
In this we learnt about user defined function. How to define a function and call the function. Also
learnt about function returning values. We learnt about recursive functions and scope of variables
– local variable and global variables.
TASK :
Consider this function:
def mystery(x, y) :
result = (x + y) / (y - x)
return result
What is the result of the call mystery(2, 3)?
Ans. : The result is : 5
What does this program print?
def main() :
a = 5
b = 7
print(mystery(a, b))
def mystery(x, y) :
z = x + y
z = z / 2.0
return z
main()
Ans. : The output of the program is : 6
In this we learnt about user defined function. How to define a function and call the function. Also
learnt about function returning values. We learnt about recursive functions and scope of variables
– local variable and global variables.
TASK :
Consider this function:
def mystery(x, y) :
result = (x + y) / (y - x)
return result
What is the result of the call mystery(2, 3)?
Ans. : The result is : 5
What does this program print?
def main() :
a = 5
b = 7
print(mystery(a, b))
def mystery(x, y) :
z = x + y
z = z / 2.0
return z
main()
Ans. : The output of the program is : 6
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
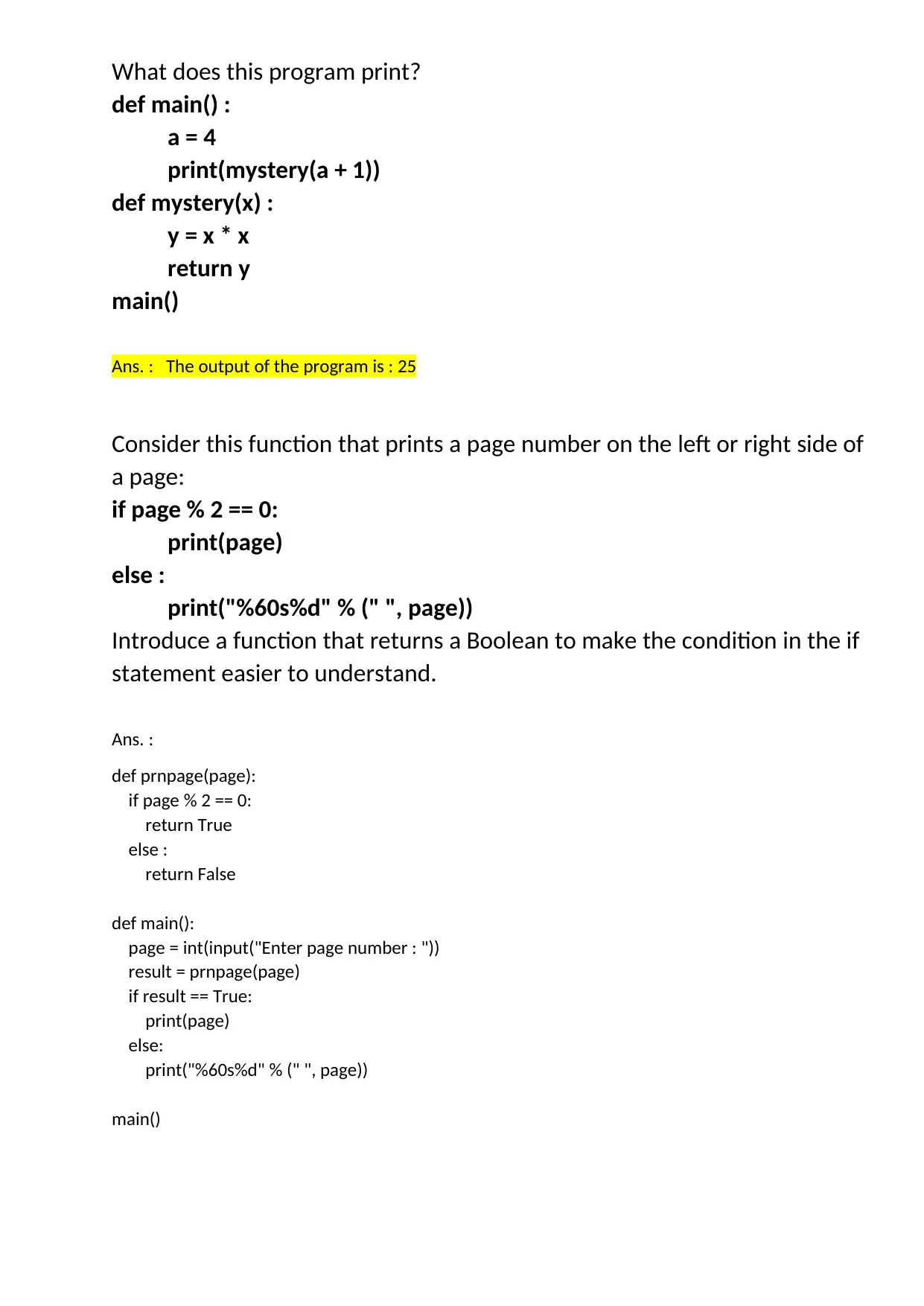
What does this program print?
def main() :
a = 4
print(mystery(a + 1))
def mystery(x) :
y = x * x
return y
main()
Ans. : The output of the program is : 25
Consider this function that prints a page number on the left or right side of
a page:
if page % 2 == 0:
print(page)
else :
print("%60s%d" % (" ", page))
Introduce a function that returns a Boolean to make the condition in the if
statement easier to understand.
Ans. :
def prnpage(page):
if page % 2 == 0:
return True
else :
return False
def main():
page = int(input("Enter page number : "))
result = prnpage(page)
if result == True:
print(page)
else:
print("%60s%d" % (" ", page))
main()
def main() :
a = 4
print(mystery(a + 1))
def mystery(x) :
y = x * x
return y
main()
Ans. : The output of the program is : 25
Consider this function that prints a page number on the left or right side of
a page:
if page % 2 == 0:
print(page)
else :
print("%60s%d" % (" ", page))
Introduce a function that returns a Boolean to make the condition in the if
statement easier to understand.
Ans. :
def prnpage(page):
if page % 2 == 0:
return True
else :
return False
def main():
page = int(input("Enter page number : "))
result = prnpage(page)
if result == True:
print(page)
else:
print("%60s%d" % (" ", page))
main()
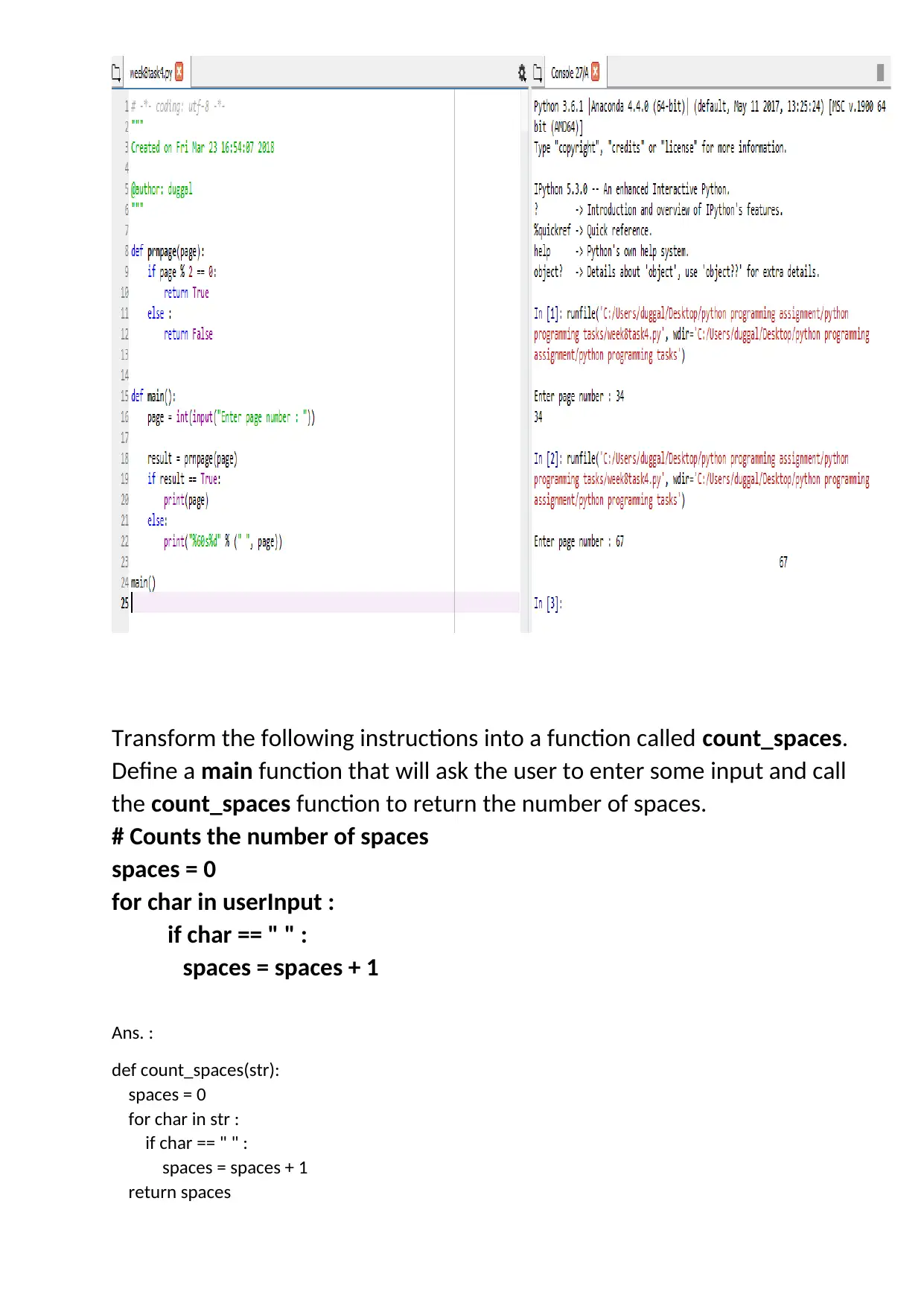
Transform the following instructions into a function called count_spaces.
Define a main function that will ask the user to enter some input and call
the count_spaces function to return the number of spaces.
# Counts the number of spaces
spaces = 0
for char in userInput :
if char == " " :
spaces = spaces + 1
Ans. :
def count_spaces(str):
spaces = 0
for char in str :
if char == " " :
spaces = spaces + 1
return spaces
Define a main function that will ask the user to enter some input and call
the count_spaces function to return the number of spaces.
# Counts the number of spaces
spaces = 0
for char in userInput :
if char == " " :
spaces = spaces + 1
Ans. :
def count_spaces(str):
spaces = 0
for char in str :
if char == " " :
spaces = spaces + 1
return spaces
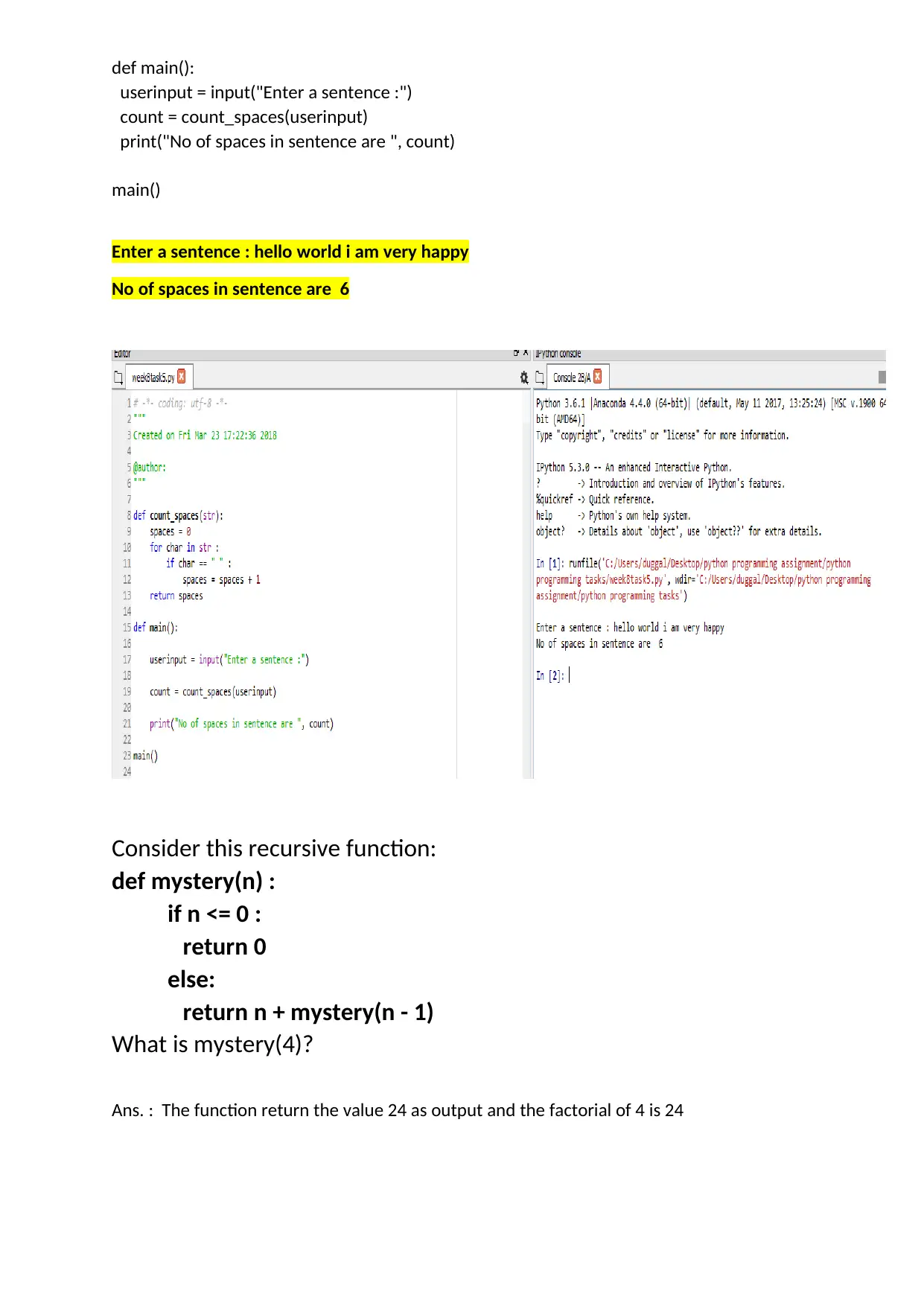
def main():
userinput = input("Enter a sentence :")
count = count_spaces(userinput)
print("No of spaces in sentence are ", count)
main()
Enter a sentence : hello world i am very happy
No of spaces in sentence are 6
Consider this recursive function:
def mystery(n) :
if n <= 0 :
return 0
else:
return n + mystery(n - 1)
What is mystery(4)?
Ans. : The function return the value 24 as output and the factorial of 4 is 24
userinput = input("Enter a sentence :")
count = count_spaces(userinput)
print("No of spaces in sentence are ", count)
main()
Enter a sentence : hello world i am very happy
No of spaces in sentence are 6
Consider this recursive function:
def mystery(n) :
if n <= 0 :
return 0
else:
return n + mystery(n - 1)
What is mystery(4)?
Ans. : The function return the value 24 as output and the factorial of 4 is 24
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
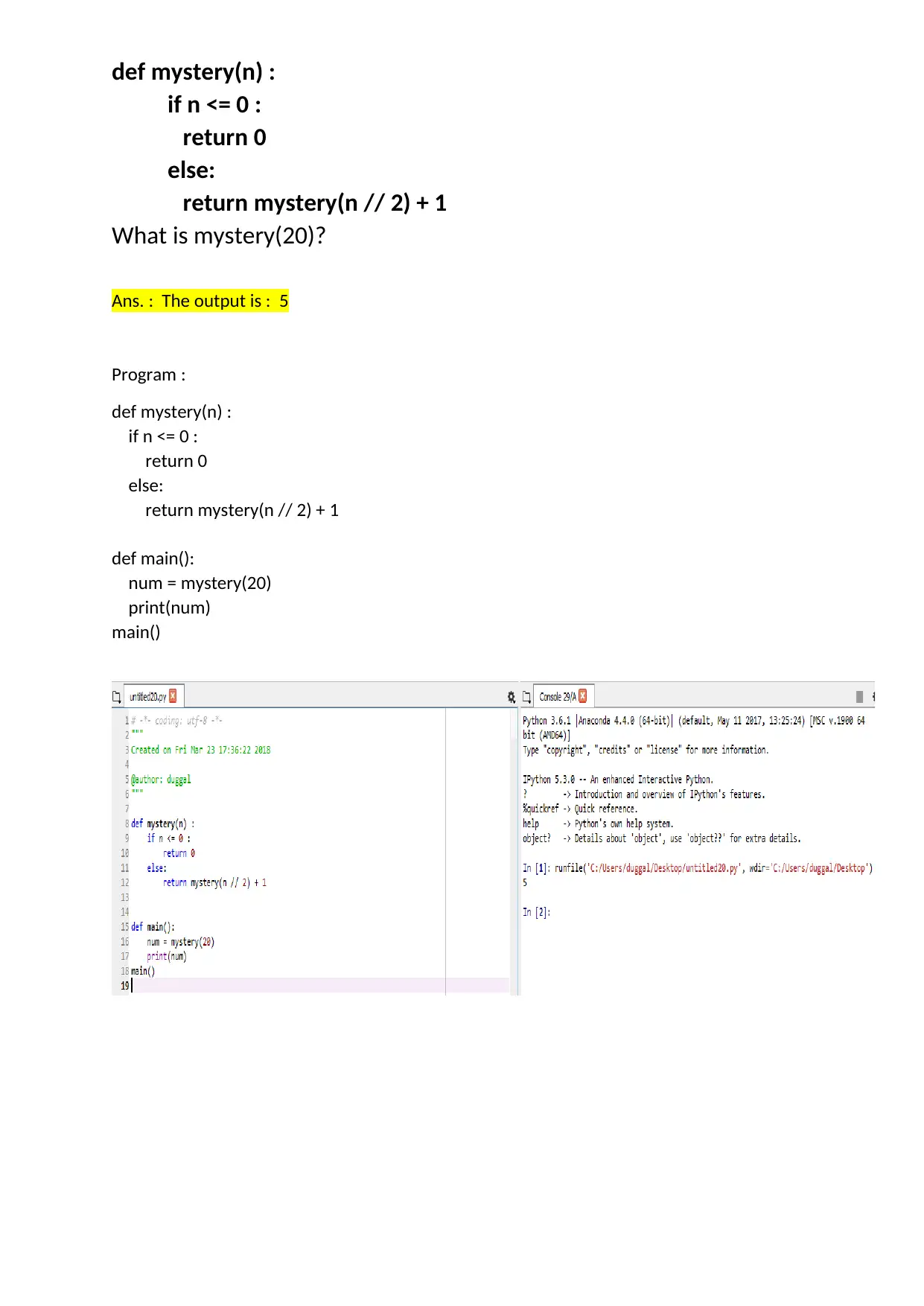
def mystery(n) :
if n <= 0 :
return 0
else:
return mystery(n // 2) + 1
What is mystery(20)?
Ans. : The output is : 5
Program :
def mystery(n) :
if n <= 0 :
return 0
else:
return mystery(n // 2) + 1
def main():
num = mystery(20)
print(num)
main()
if n <= 0 :
return 0
else:
return mystery(n // 2) + 1
What is mystery(20)?
Ans. : The output is : 5
Program :
def mystery(n) :
if n <= 0 :
return 0
else:
return mystery(n // 2) + 1
def main():
num = mystery(20)
print(num)
main()
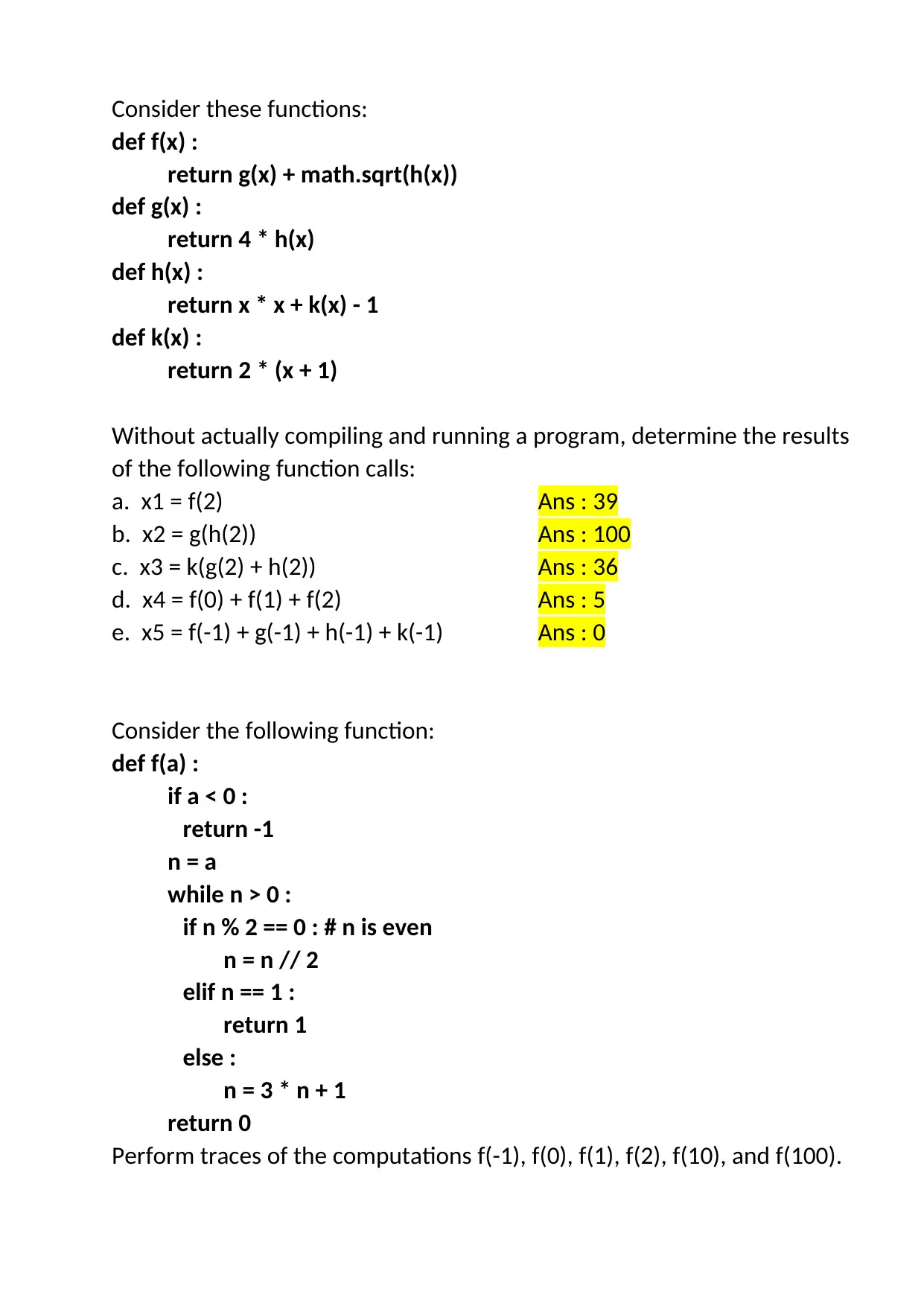
Consider these functions:
def f(x) :
return g(x) + math.sqrt(h(x))
def g(x) :
return 4 * h(x)
def h(x) :
return x * x + k(x) - 1
def k(x) :
return 2 * (x + 1)
Without actually compiling and running a program, determine the results
of the following function calls:
a. x1 = f(2) Ans : 39
b. x2 = g(h(2)) Ans : 100
c. x3 = k(g(2) + h(2)) Ans : 36
d. x4 = f(0) + f(1) + f(2) Ans : 5
e. x5 = f(-1) + g(-1) + h(-1) + k(-1) Ans : 0
Consider the following function:
def f(a) :
if a < 0 :
return -1
n = a
while n > 0 :
if n % 2 == 0 : # n is even
n = n // 2
elif n == 1 :
return 1
else :
n = 3 * n + 1
return 0
Perform traces of the computations f(-1), f(0), f(1), f(2), f(10), and f(100).
def f(x) :
return g(x) + math.sqrt(h(x))
def g(x) :
return 4 * h(x)
def h(x) :
return x * x + k(x) - 1
def k(x) :
return 2 * (x + 1)
Without actually compiling and running a program, determine the results
of the following function calls:
a. x1 = f(2) Ans : 39
b. x2 = g(h(2)) Ans : 100
c. x3 = k(g(2) + h(2)) Ans : 36
d. x4 = f(0) + f(1) + f(2) Ans : 5
e. x5 = f(-1) + g(-1) + h(-1) + k(-1) Ans : 0
Consider the following function:
def f(a) :
if a < 0 :
return -1
n = a
while n > 0 :
if n % 2 == 0 : # n is even
n = n // 2
elif n == 1 :
return 1
else :
n = 3 * n + 1
return 0
Perform traces of the computations f(-1), f(0), f(1), f(2), f(10), and f(100).
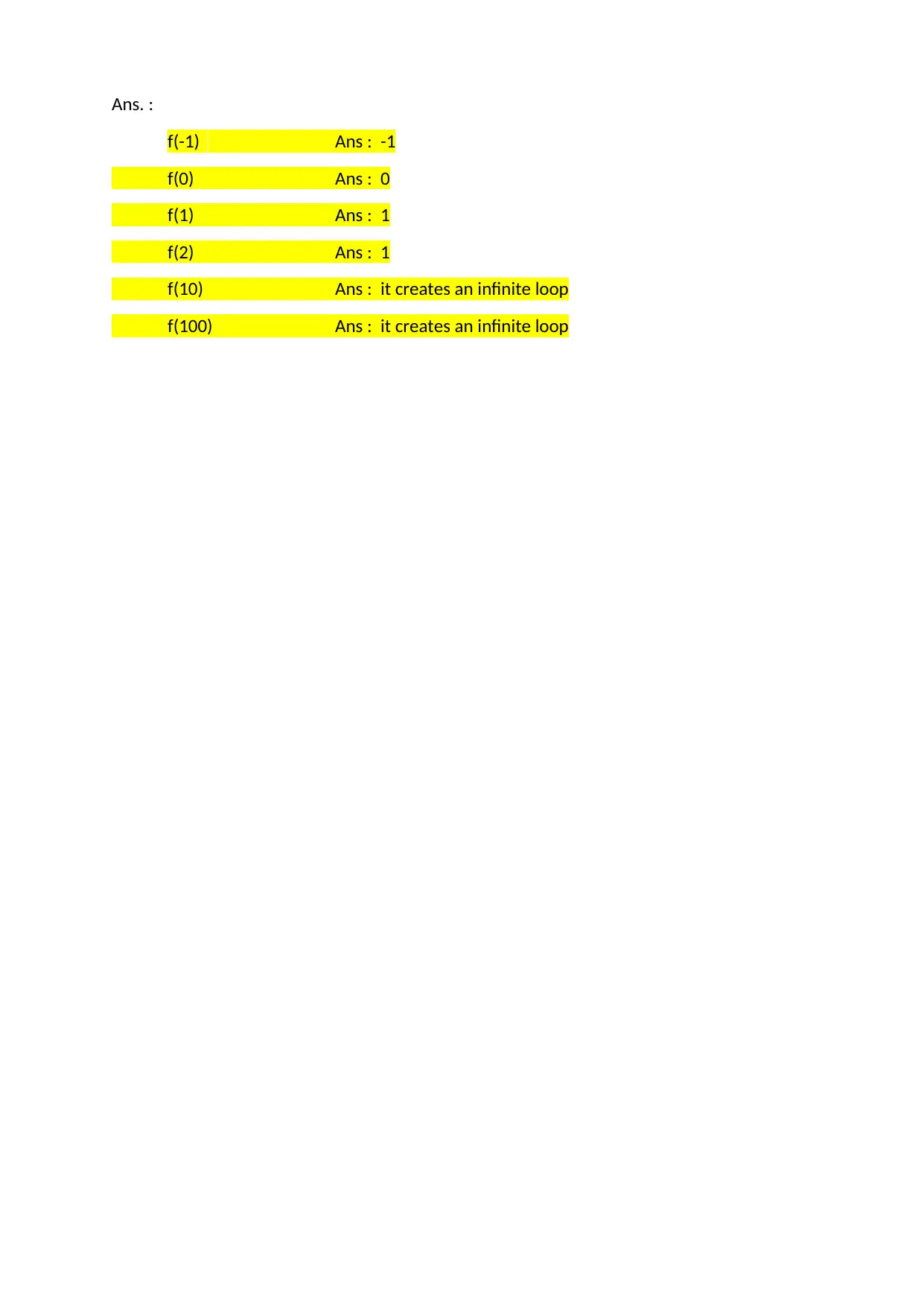
Ans. :
f(-1) Ans : -1
f(0) Ans : 0
f(1) Ans : 1
f(2) Ans : 1
f(10) Ans : it creates an infinite loop
f(100) Ans : it creates an infinite loop
f(-1) Ans : -1
f(0) Ans : 0
f(1) Ans : 1
f(2) Ans : 1
f(10) Ans : it creates an infinite loop
f(100) Ans : it creates an infinite loop
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
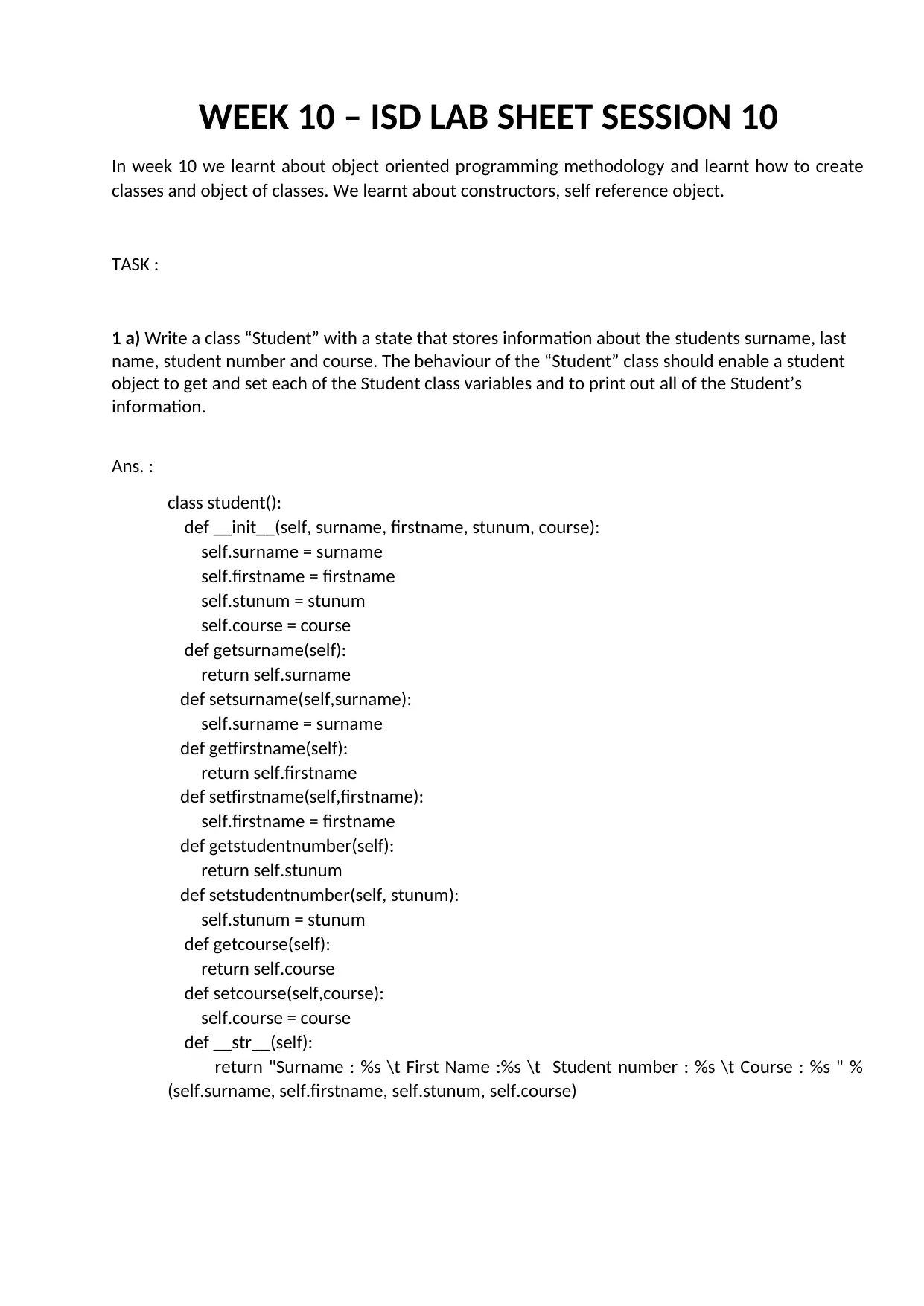
WEEK 10 – ISD LAB SHEET SESSION 10
In week 10 we learnt about object oriented programming methodology and learnt how to create
classes and object of classes. We learnt about constructors, self reference object.
TASK :
1 a) Write a class “Student” with a state that stores information about the students surname, last
name, student number and course. The behaviour of the “Student” class should enable a student
object to get and set each of the Student class variables and to print out all of the Student’s
information.
Ans. :
class student():
def __init__(self, surname, firstname, stunum, course):
self.surname = surname
self.firstname = firstname
self.stunum = stunum
self.course = course
def getsurname(self):
return self.surname
def setsurname(self,surname):
self.surname = surname
def getfirstname(self):
return self.firstname
def setfirstname(self,firstname):
self.firstname = firstname
def getstudentnumber(self):
return self.stunum
def setstudentnumber(self, stunum):
self.stunum = stunum
def getcourse(self):
return self.course
def setcourse(self,course):
self.course = course
def __str__(self):
return "Surname : %s \t First Name :%s \t Student number : %s \t Course : %s " %
(self.surname, self.firstname, self.stunum, self.course)
In week 10 we learnt about object oriented programming methodology and learnt how to create
classes and object of classes. We learnt about constructors, self reference object.
TASK :
1 a) Write a class “Student” with a state that stores information about the students surname, last
name, student number and course. The behaviour of the “Student” class should enable a student
object to get and set each of the Student class variables and to print out all of the Student’s
information.
Ans. :
class student():
def __init__(self, surname, firstname, stunum, course):
self.surname = surname
self.firstname = firstname
self.stunum = stunum
self.course = course
def getsurname(self):
return self.surname
def setsurname(self,surname):
self.surname = surname
def getfirstname(self):
return self.firstname
def setfirstname(self,firstname):
self.firstname = firstname
def getstudentnumber(self):
return self.stunum
def setstudentnumber(self, stunum):
self.stunum = stunum
def getcourse(self):
return self.course
def setcourse(self,course):
self.course = course
def __str__(self):
return "Surname : %s \t First Name :%s \t Student number : %s \t Course : %s " %
(self.surname, self.firstname, self.stunum, self.course)
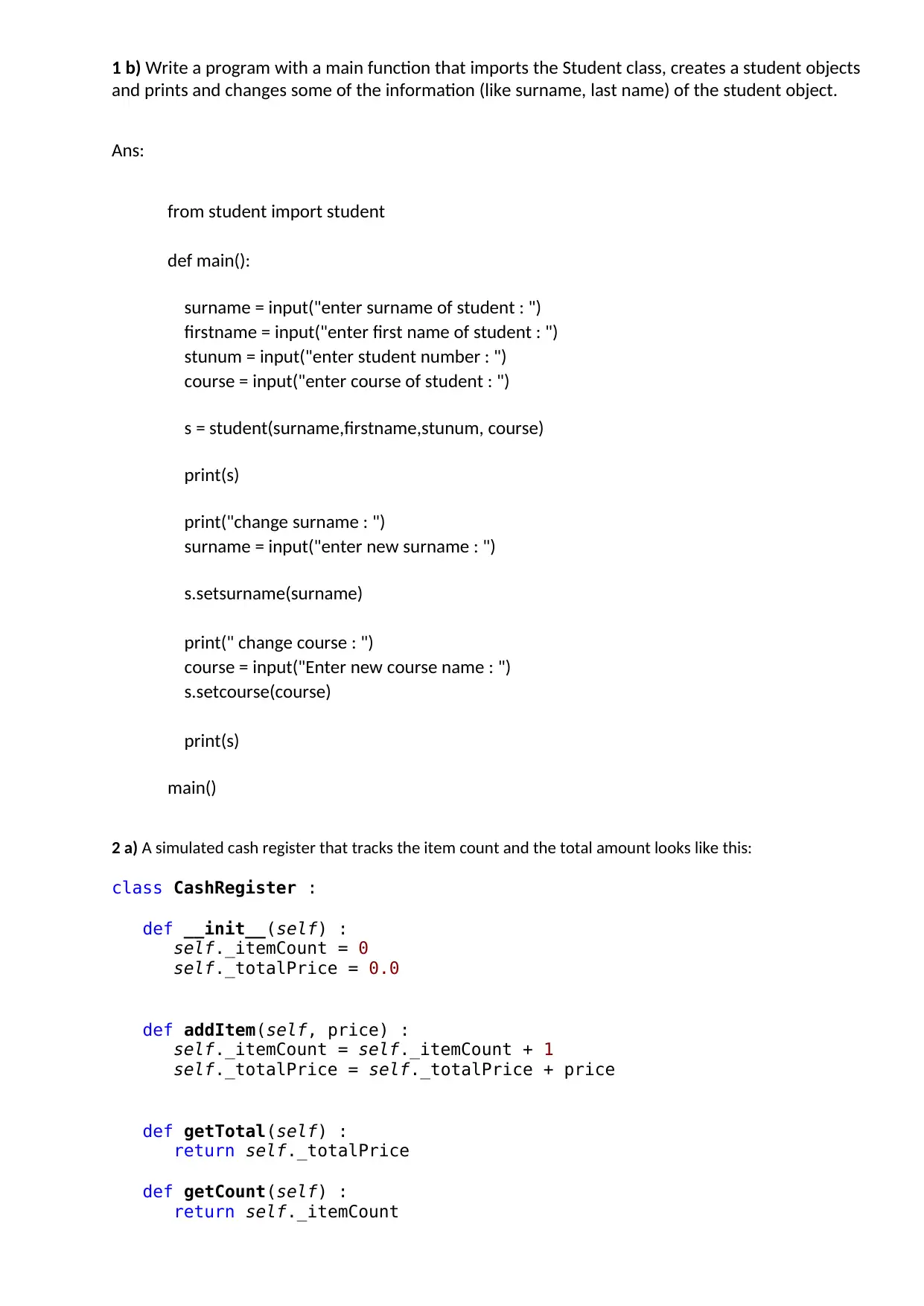
1 b) Write a program with a main function that imports the Student class, creates a student objects
and prints and changes some of the information (like surname, last name) of the student object.
Ans:
from student import student
def main():
surname = input("enter surname of student : ")
firstname = input("enter first name of student : ")
stunum = input("enter student number : ")
course = input("enter course of student : ")
s = student(surname,firstname,stunum, course)
print(s)
print("change surname : ")
surname = input("enter new surname : ")
s.setsurname(surname)
print(" change course : ")
course = input("Enter new course name : ")
s.setcourse(course)
print(s)
main()
2 a) A simulated cash register that tracks the item count and the total amount looks like this: due
class CashRegister :
def __init__(self) :
self._itemCount = 0
self._totalPrice = 0.0
def addItem(self, price) :
self._itemCount = self._itemCount + 1
self._totalPrice = self._totalPrice + price
def getTotal(self) :
return self._totalPrice
def getCount(self) :
return self._itemCount
and prints and changes some of the information (like surname, last name) of the student object.
Ans:
from student import student
def main():
surname = input("enter surname of student : ")
firstname = input("enter first name of student : ")
stunum = input("enter student number : ")
course = input("enter course of student : ")
s = student(surname,firstname,stunum, course)
print(s)
print("change surname : ")
surname = input("enter new surname : ")
s.setsurname(surname)
print(" change course : ")
course = input("Enter new course name : ")
s.setcourse(course)
print(s)
main()
2 a) A simulated cash register that tracks the item count and the total amount looks like this: due
class CashRegister :
def __init__(self) :
self._itemCount = 0
self._totalPrice = 0.0
def addItem(self, price) :
self._itemCount = self._itemCount + 1
self._totalPrice = self._totalPrice + price
def getTotal(self) :
return self._totalPrice
def getCount(self) :
return self._itemCount
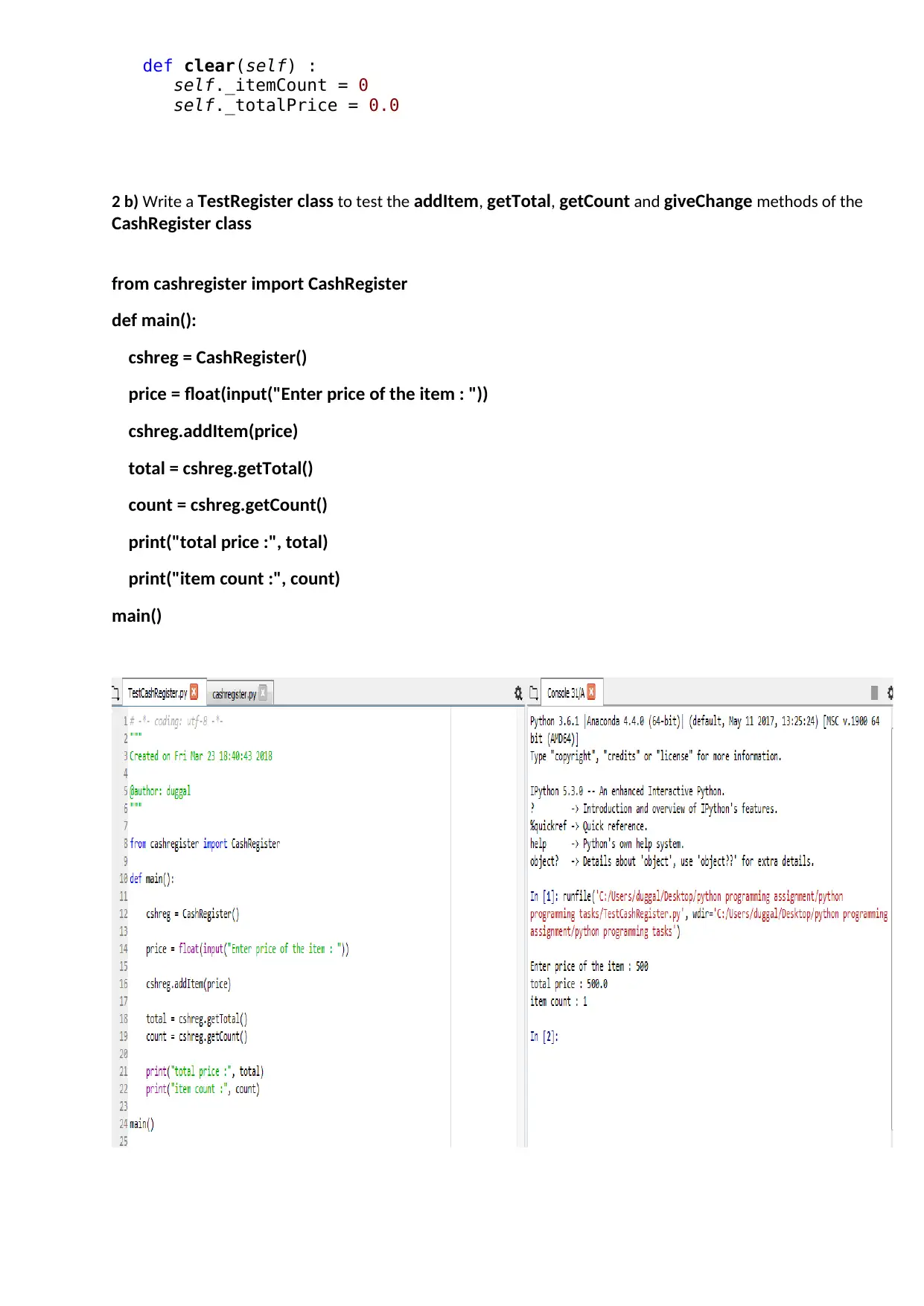
def clear(self) :
self._itemCount = 0
self._totalPrice = 0.0
2 b) Write a TestRegister class to test the addItem, getTotal, getCount and giveChange methods of the
CashRegister class
from cashregister import CashRegister
def main():
cshreg = CashRegister()
price = float(input("Enter price of the item : "))
cshreg.addItem(price)
total = cshreg.getTotal()
count = cshreg.getCount()
print("total price :", total)
print("item count :", count)
main()
self._itemCount = 0
self._totalPrice = 0.0
2 b) Write a TestRegister class to test the addItem, getTotal, getCount and giveChange methods of the
CashRegister class
from cashregister import CashRegister
def main():
cshreg = CashRegister()
price = float(input("Enter price of the item : "))
cshreg.addItem(price)
total = cshreg.getTotal()
count = cshreg.getCount()
print("total price :", total)
print("item count :", count)
main()
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
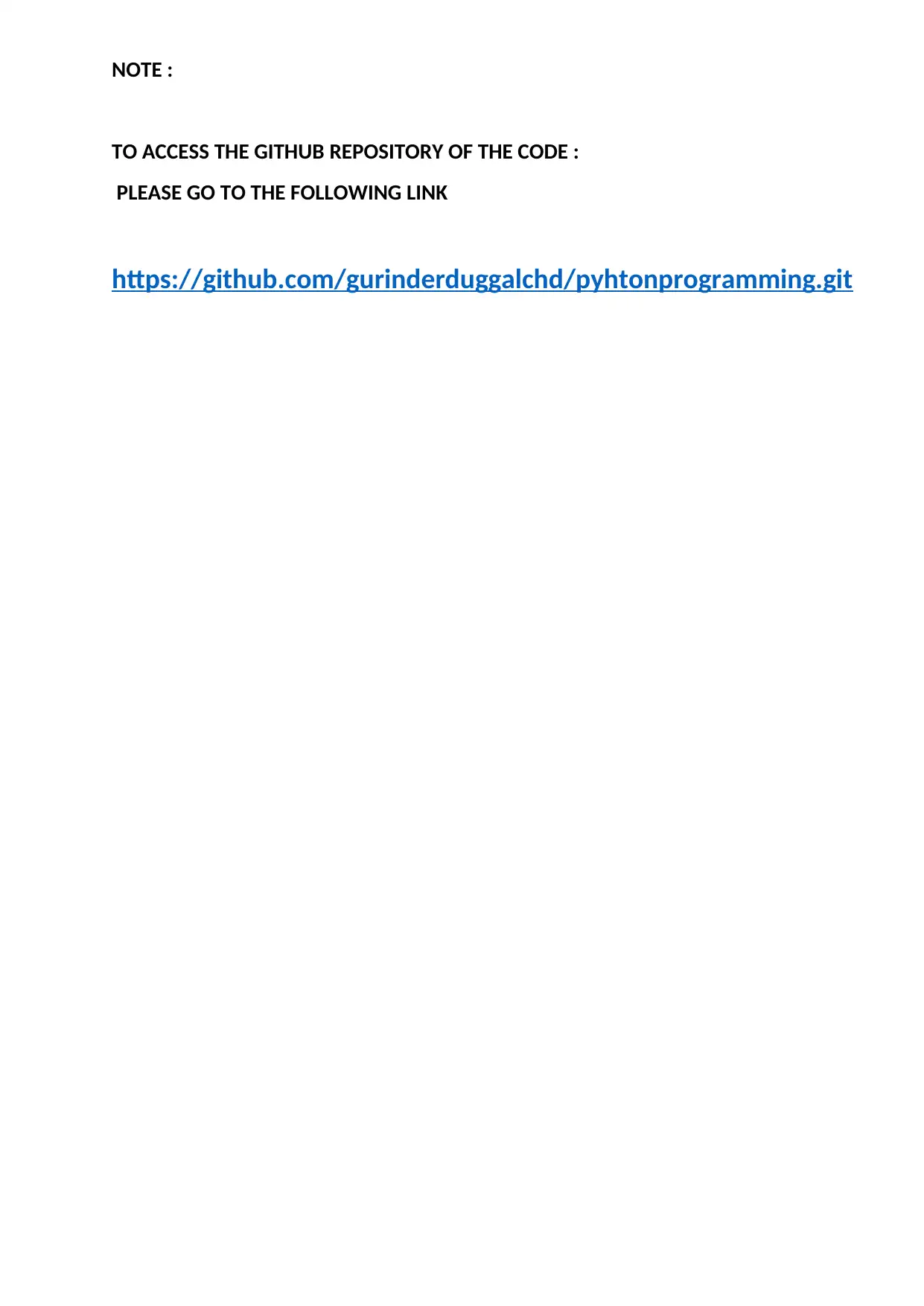
NOTE :
TO ACCESS THE GITHUB REPOSITORY OF THE CODE :
PLEASE GO TO THE FOLLOWING LINK
https://github.com/gurinderduggalchd/pyhtonprogramming.git
TO ACCESS THE GITHUB REPOSITORY OF THE CODE :
PLEASE GO TO THE FOLLOWING LINK
https://github.com/gurinderduggalchd/pyhtonprogramming.git
1 out of 38
Related Documents

Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.