Installation and Implementation of Java Client-Server Socket Program
VerifiedAdded on 2023/06/10
|17
|1542
|79
Practical Assignment
AI Summary
This assignment provides a detailed guide to Java client-server socket programming. It begins with instructions on installing the necessary software, including the Java Development Kit (JDK) and NetBeans IDE. The assignment then walks through the process of creating a client-server chat progr...
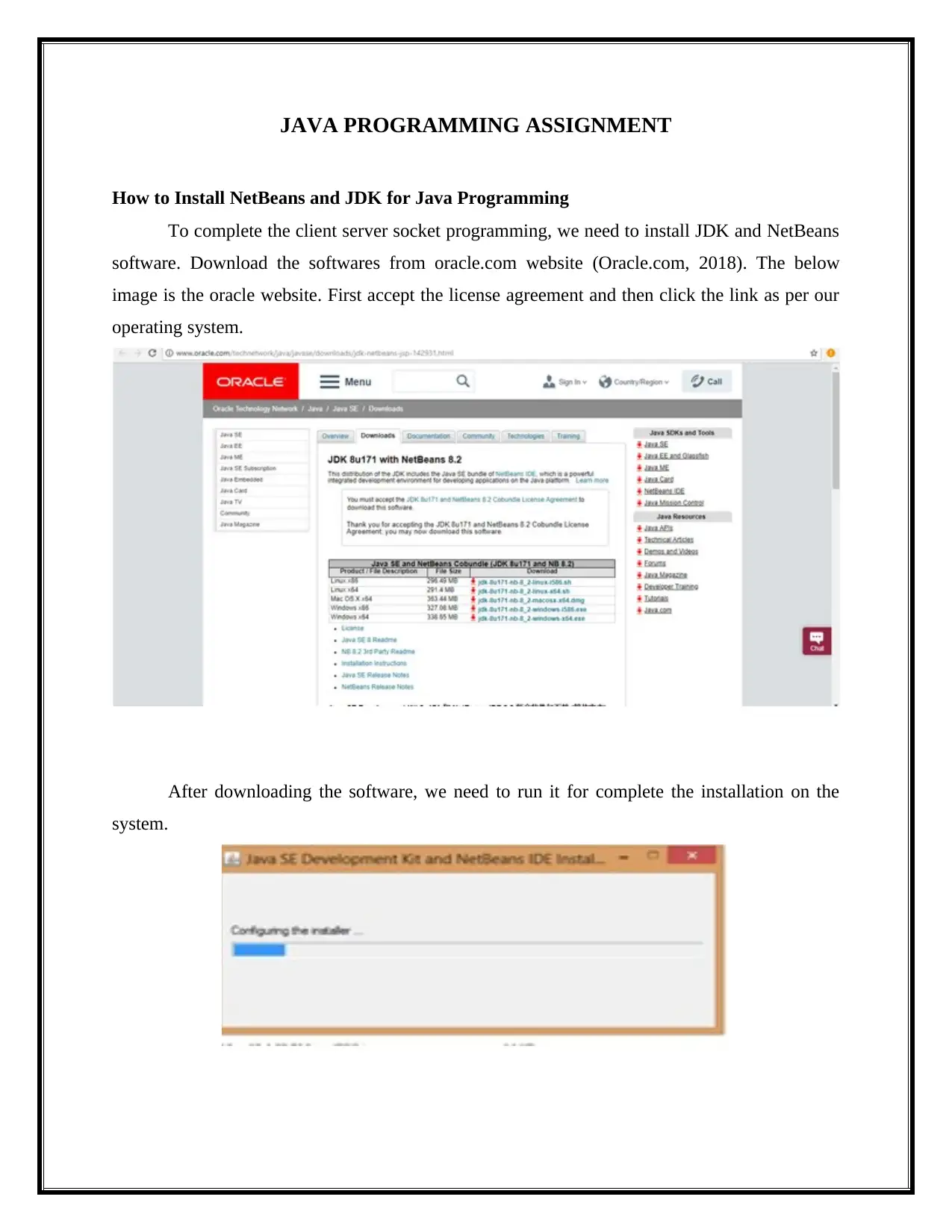
JAVA PROGRAMMING ASSIGNMENT
How to Install NetBeans and JDK for Java Programming
To complete the client server socket programming, we need to install JDK and NetBeans
software. Download the softwares from oracle.com website (Oracle.com, 2018). The below
image is the oracle website. First accept the license agreement and then click the link as per our
operating system.
After downloading the software, we need to run it for complete the installation on the
system.
How to Install NetBeans and JDK for Java Programming
To complete the client server socket programming, we need to install JDK and NetBeans
software. Download the softwares from oracle.com website (Oracle.com, 2018). The below
image is the oracle website. First accept the license agreement and then click the link as per our
operating system.
After downloading the software, we need to run it for complete the installation on the
system.
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
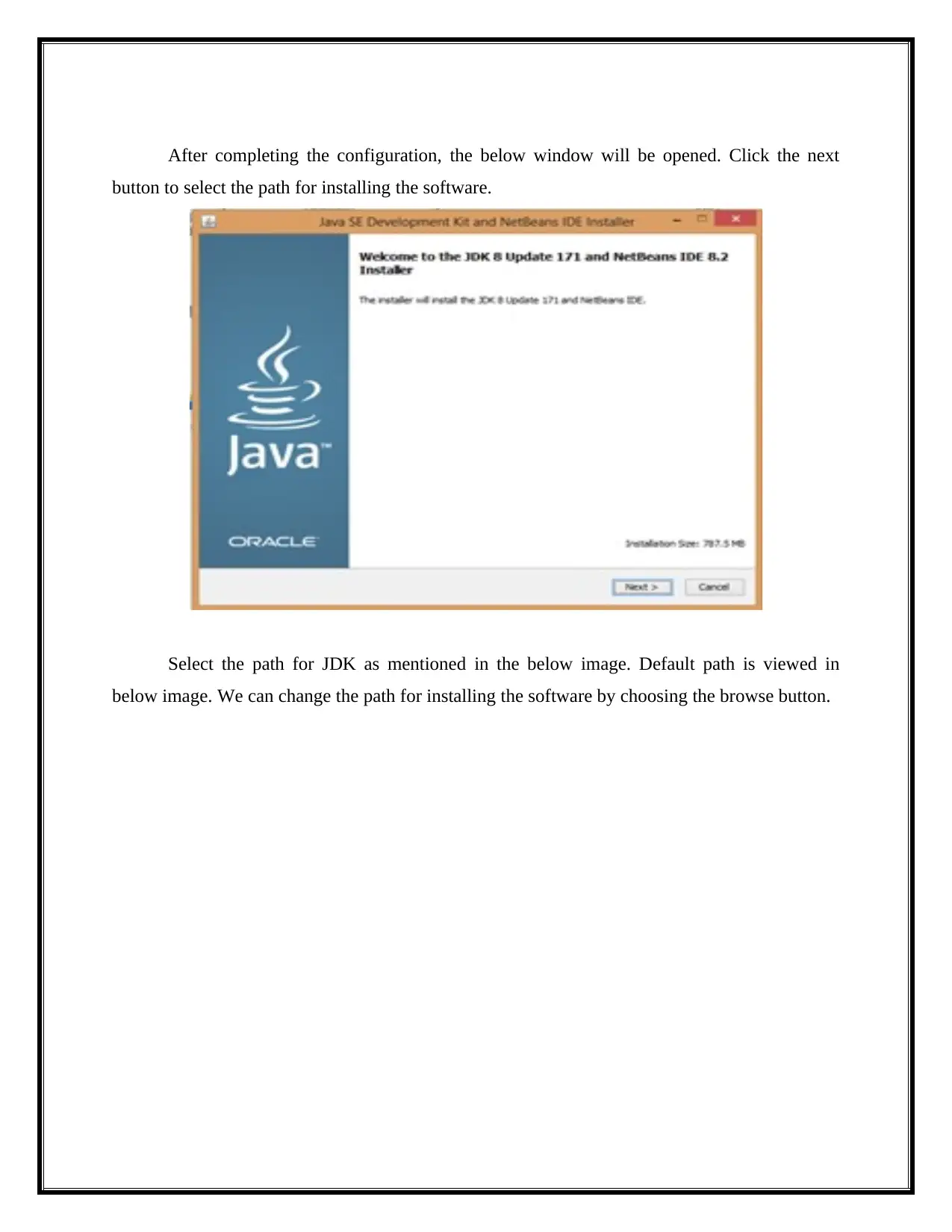
After completing the configuration, the below window will be opened. Click the next
button to select the path for installing the software.
Select the path for JDK as mentioned in the below image. Default path is viewed in
below image. We can change the path for installing the software by choosing the browse button.
button to select the path for installing the software.
Select the path for JDK as mentioned in the below image. Default path is viewed in
below image. We can change the path for installing the software by choosing the browse button.
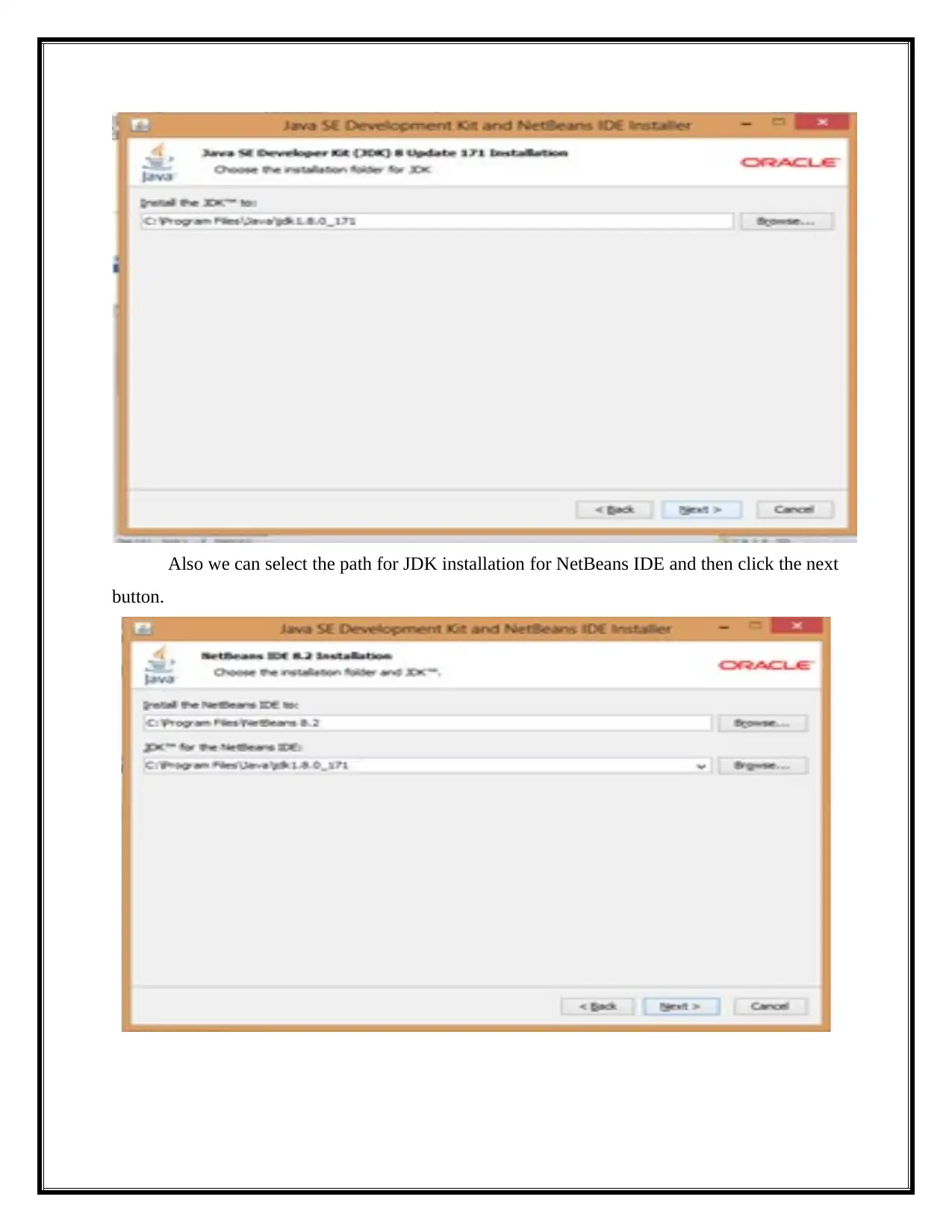
Also we can select the path for JDK installation for NetBeans IDE and then click the next
button.
button.
⊘ This is a preview!⊘
Do you want full access?
Subscribe today to unlock all pages.

Trusted by 1+ million students worldwide
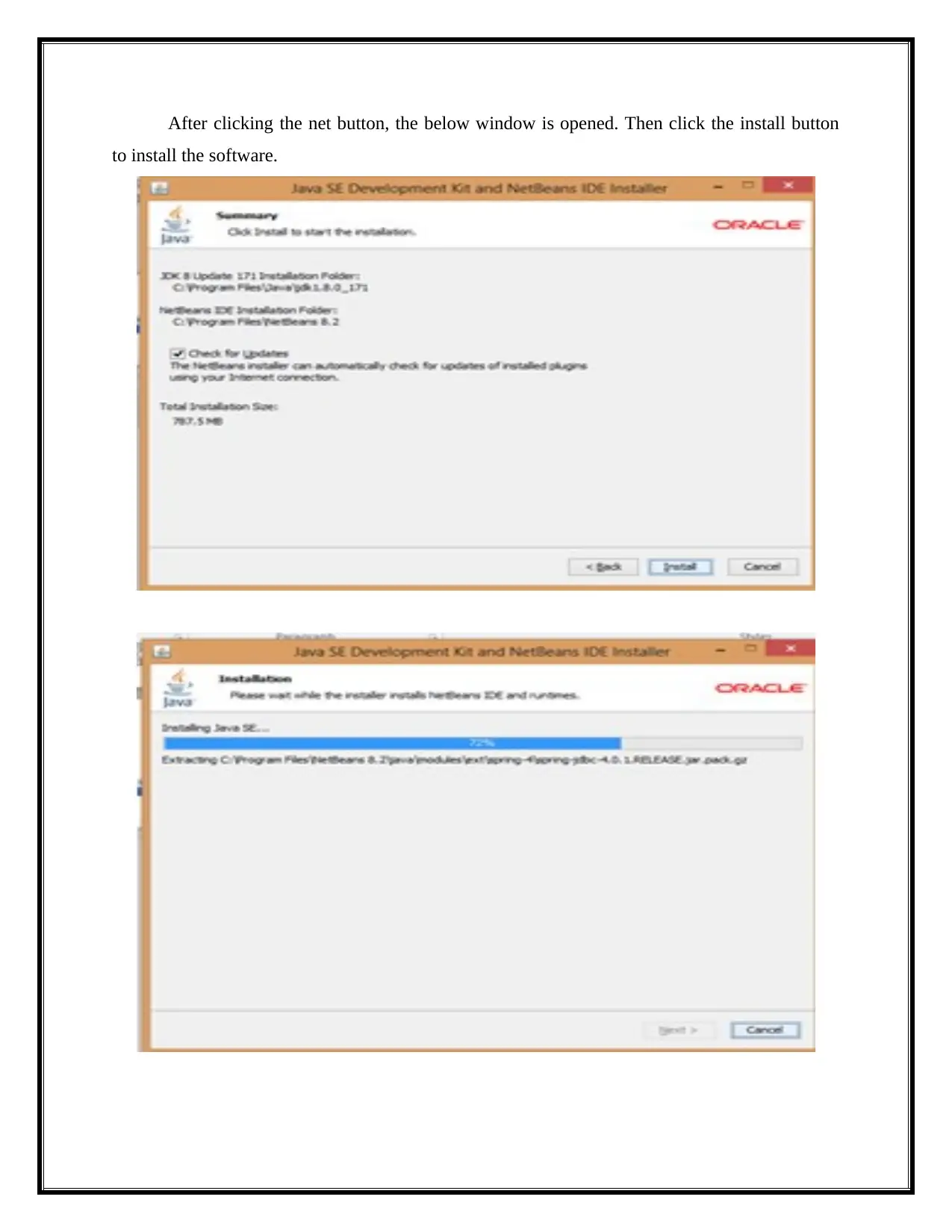
After clicking the net button, the below window is opened. Then click the install button
to install the software.
to install the software.
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
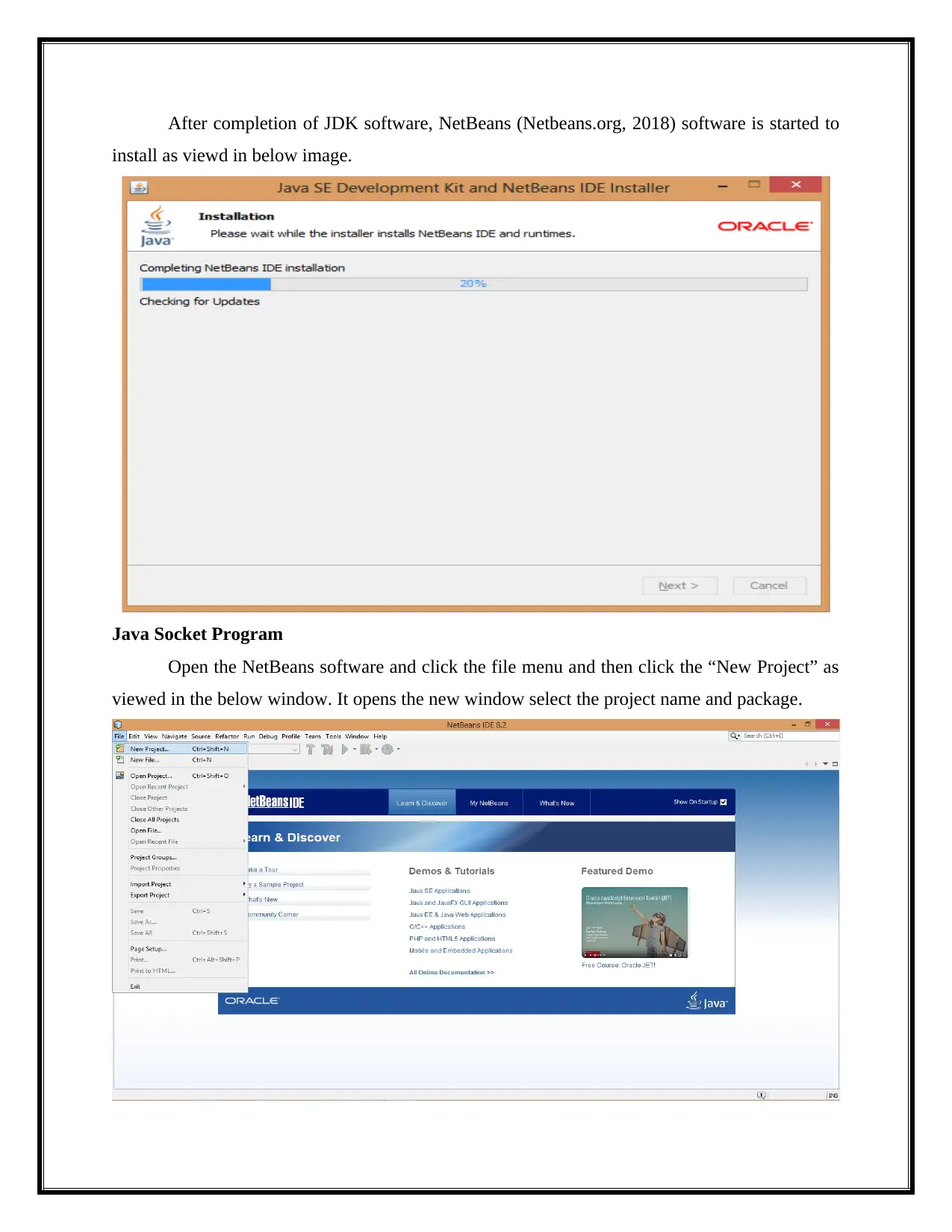
After completion of JDK software, NetBeans (Netbeans.org, 2018) software is started to
install as viewd in below image.
Java Socket Program
Open the NetBeans software and click the file menu and then click the “New Project” as
viewed in the below window. It opens the new window select the project name and package.
install as viewd in below image.
Java Socket Program
Open the NetBeans software and click the file menu and then click the “New Project” as
viewed in the below window. It opens the new window select the project name and package.
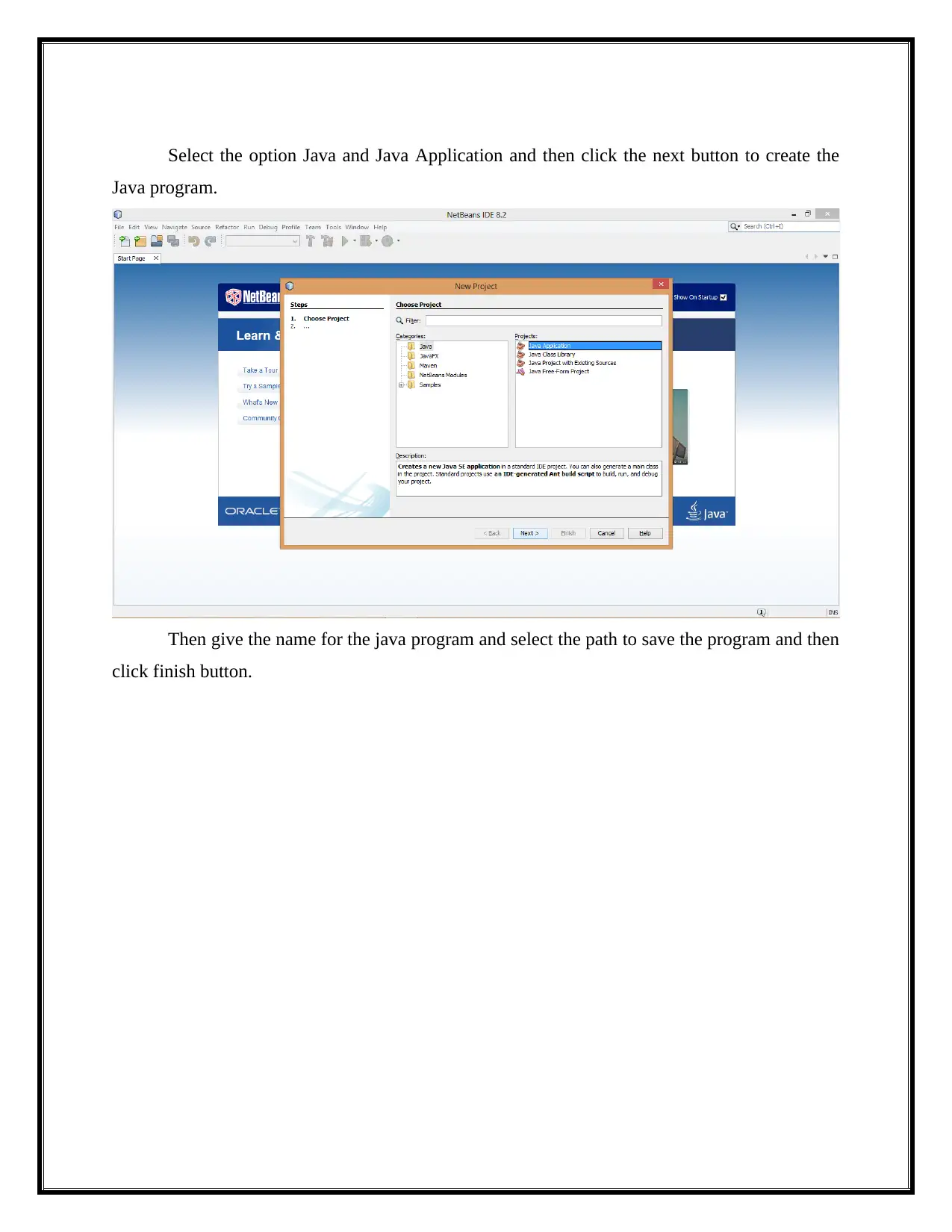
Select the option Java and Java Application and then click the next button to create the
Java program.
Then give the name for the java program and select the path to save the program and then
click finish button.
Java program.
Then give the name for the java program and select the path to save the program and then
click finish button.
⊘ This is a preview!⊘
Do you want full access?
Subscribe today to unlock all pages.

Trusted by 1+ million students worldwide
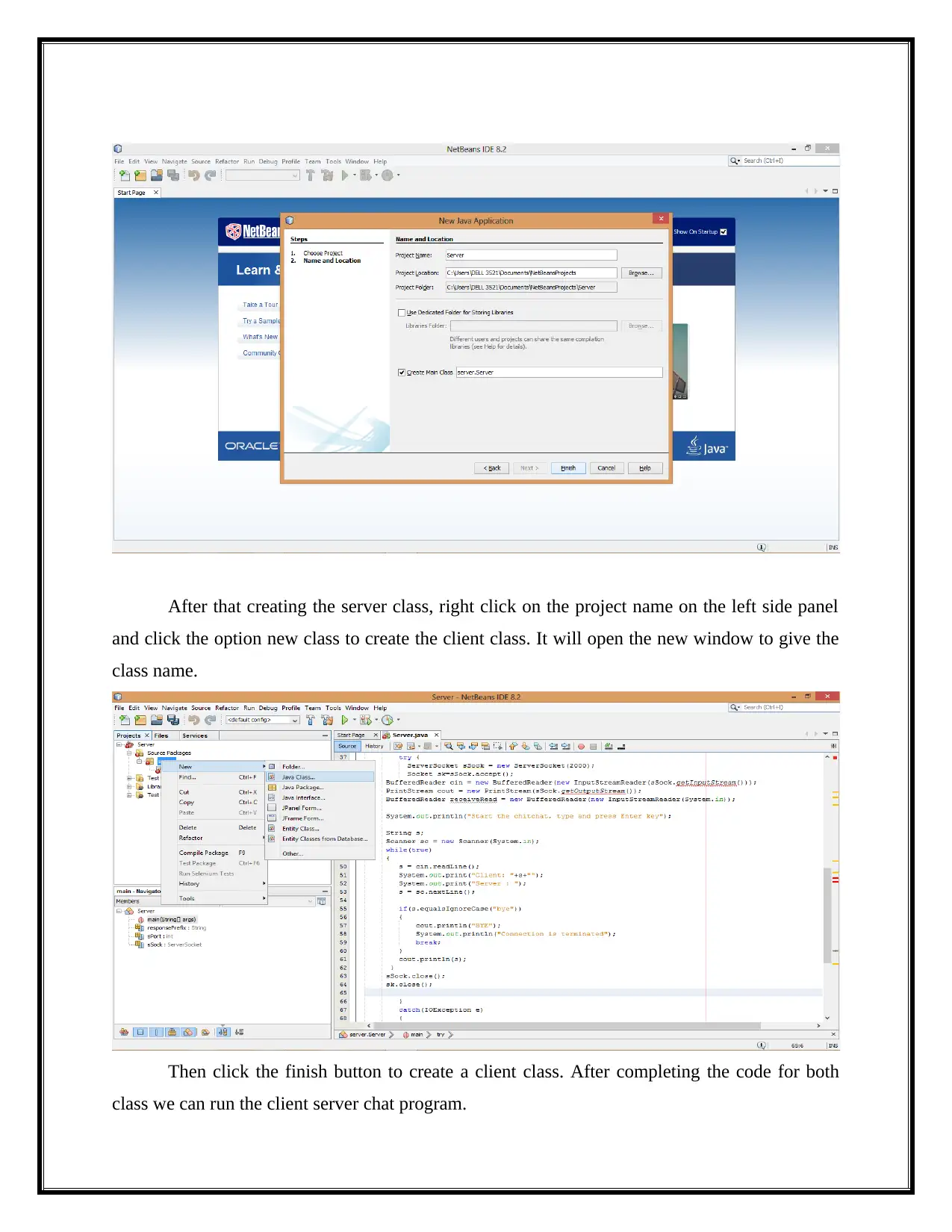
After that creating the server class, right click on the project name on the left side panel
and click the option new class to create the client class. It will open the new window to give the
class name.
Then click the finish button to create a client class. After completing the code for both
class we can run the client server chat program.
and click the option new class to create the client class. It will open the new window to give the
class name.
Then click the finish button to create a client class. After completing the code for both
class we can run the client server chat program.
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
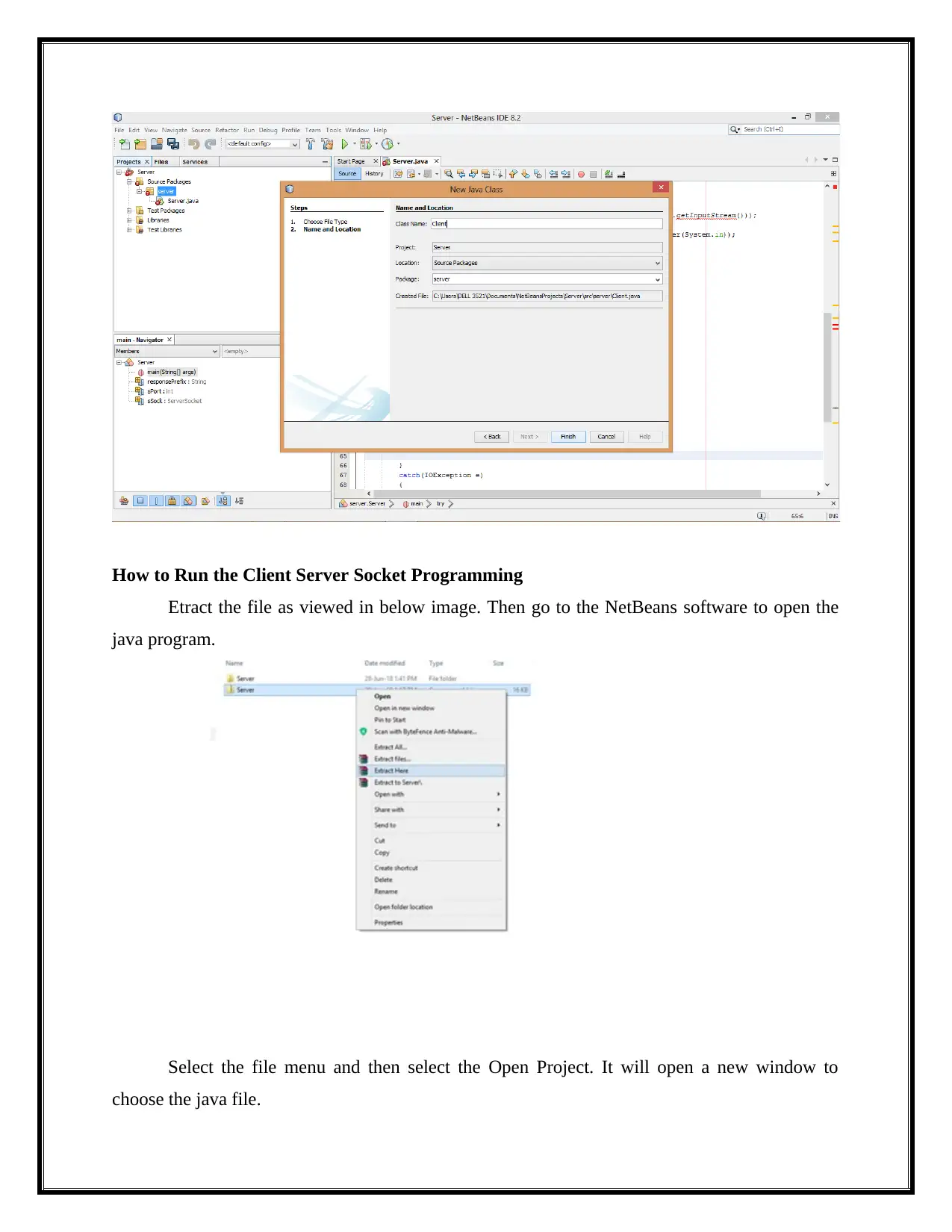
How to Run the Client Server Socket Programming
Etract the file as viewed in below image. Then go to the NetBeans software to open the
java program.
Select the file menu and then select the Open Project. It will open a new window to
choose the java file.
Etract the file as viewed in below image. Then go to the NetBeans software to open the
java program.
Select the file menu and then select the Open Project. It will open a new window to
choose the java file.
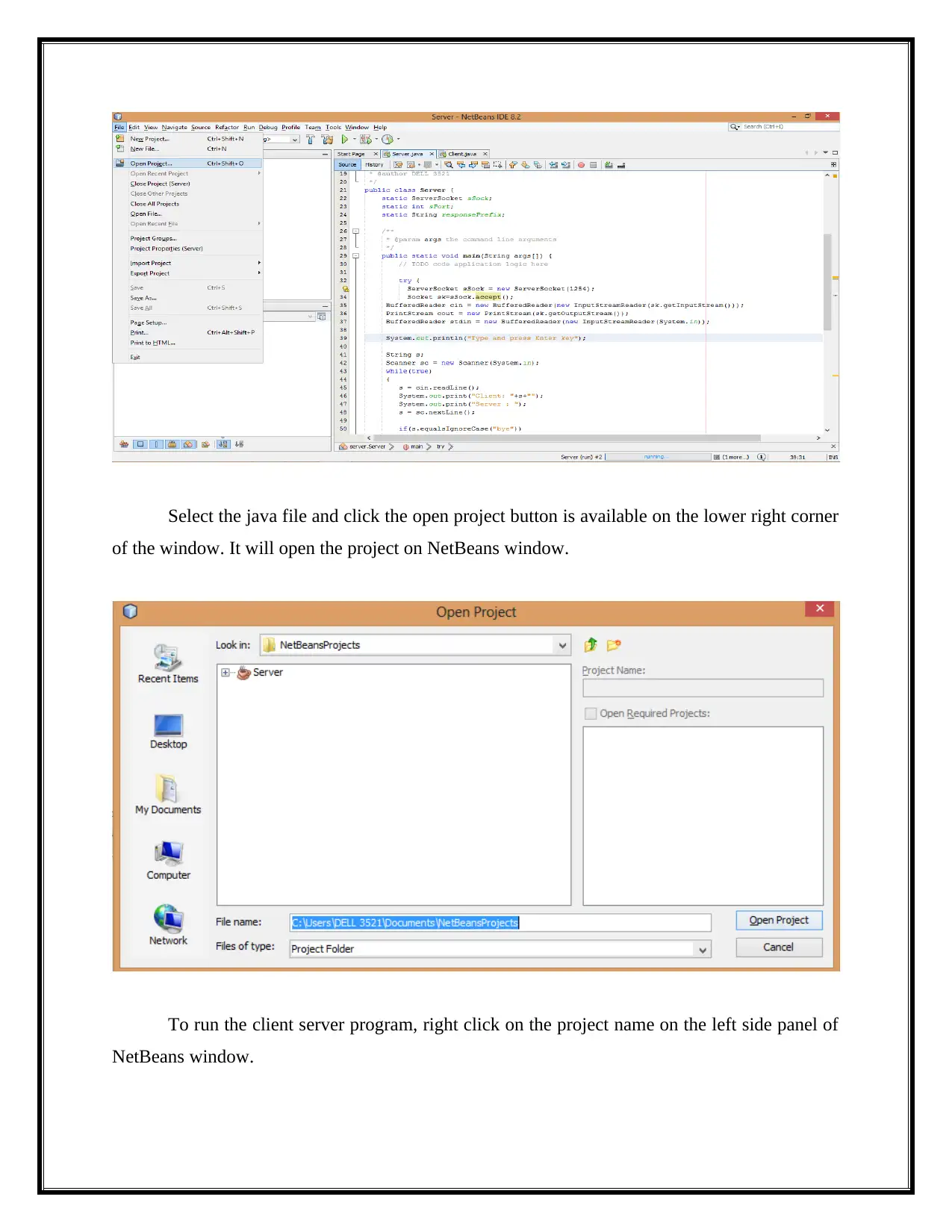
Select the java file and click the open project button is available on the lower right corner
of the window. It will open the project on NetBeans window.
To run the client server program, right click on the project name on the left side panel of
NetBeans window.
of the window. It will open the project on NetBeans window.
To run the client server program, right click on the project name on the left side panel of
NetBeans window.
⊘ This is a preview!⊘
Do you want full access?
Subscribe today to unlock all pages.

Trusted by 1+ million students worldwide
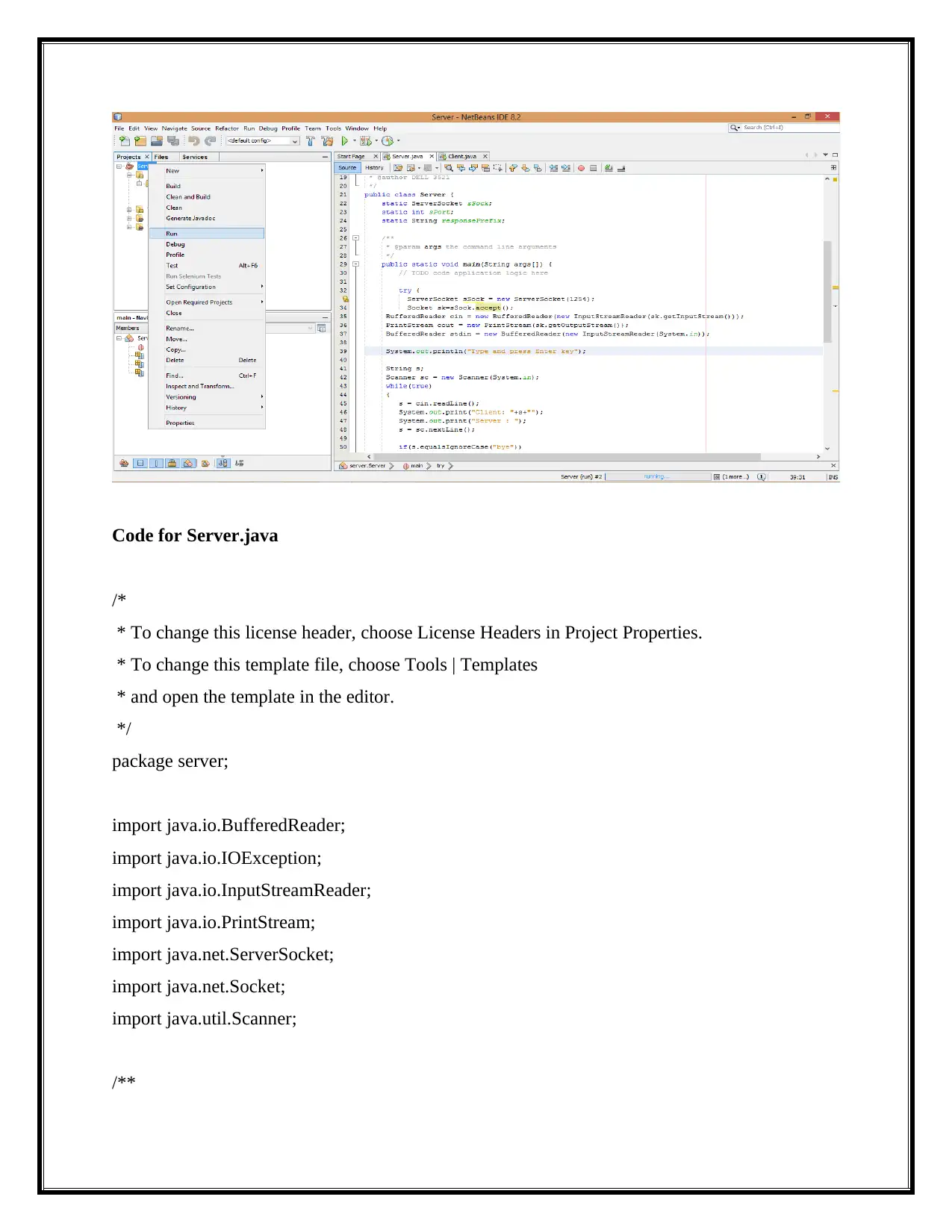
Code for Server.java
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package server;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.PrintStream;
import java.net.ServerSocket;
import java.net.Socket;
import java.util.Scanner;
/**
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package server;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.PrintStream;
import java.net.ServerSocket;
import java.net.Socket;
import java.util.Scanner;
/**
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
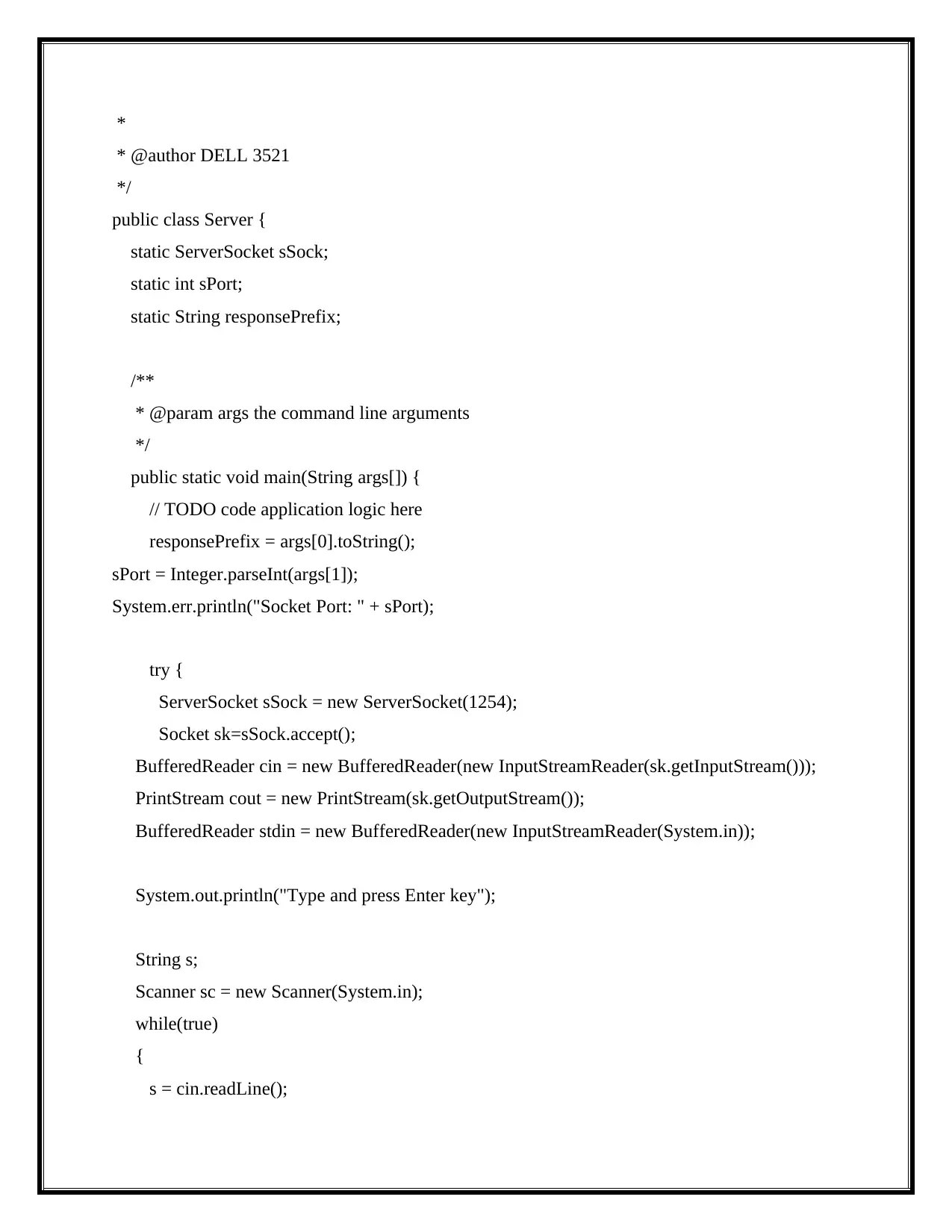
*
* @author DELL 3521
*/
public class Server {
static ServerSocket sSock;
static int sPort;
static String responsePrefix;
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
// TODO code application logic here
responsePrefix = args[0].toString();
sPort = Integer.parseInt(args[1]);
System.err.println("Socket Port: " + sPort);
try {
ServerSocket sSock = new ServerSocket(1254);
Socket sk=sSock.accept();
BufferedReader cin = new BufferedReader(new InputStreamReader(sk.getInputStream()));
PrintStream cout = new PrintStream(sk.getOutputStream());
BufferedReader stdin = new BufferedReader(new InputStreamReader(System.in));
System.out.println("Type and press Enter key");
String s;
Scanner sc = new Scanner(System.in);
while(true)
{
s = cin.readLine();
* @author DELL 3521
*/
public class Server {
static ServerSocket sSock;
static int sPort;
static String responsePrefix;
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
// TODO code application logic here
responsePrefix = args[0].toString();
sPort = Integer.parseInt(args[1]);
System.err.println("Socket Port: " + sPort);
try {
ServerSocket sSock = new ServerSocket(1254);
Socket sk=sSock.accept();
BufferedReader cin = new BufferedReader(new InputStreamReader(sk.getInputStream()));
PrintStream cout = new PrintStream(sk.getOutputStream());
BufferedReader stdin = new BufferedReader(new InputStreamReader(System.in));
System.out.println("Type and press Enter key");
String s;
Scanner sc = new Scanner(System.in);
while(true)
{
s = cin.readLine();
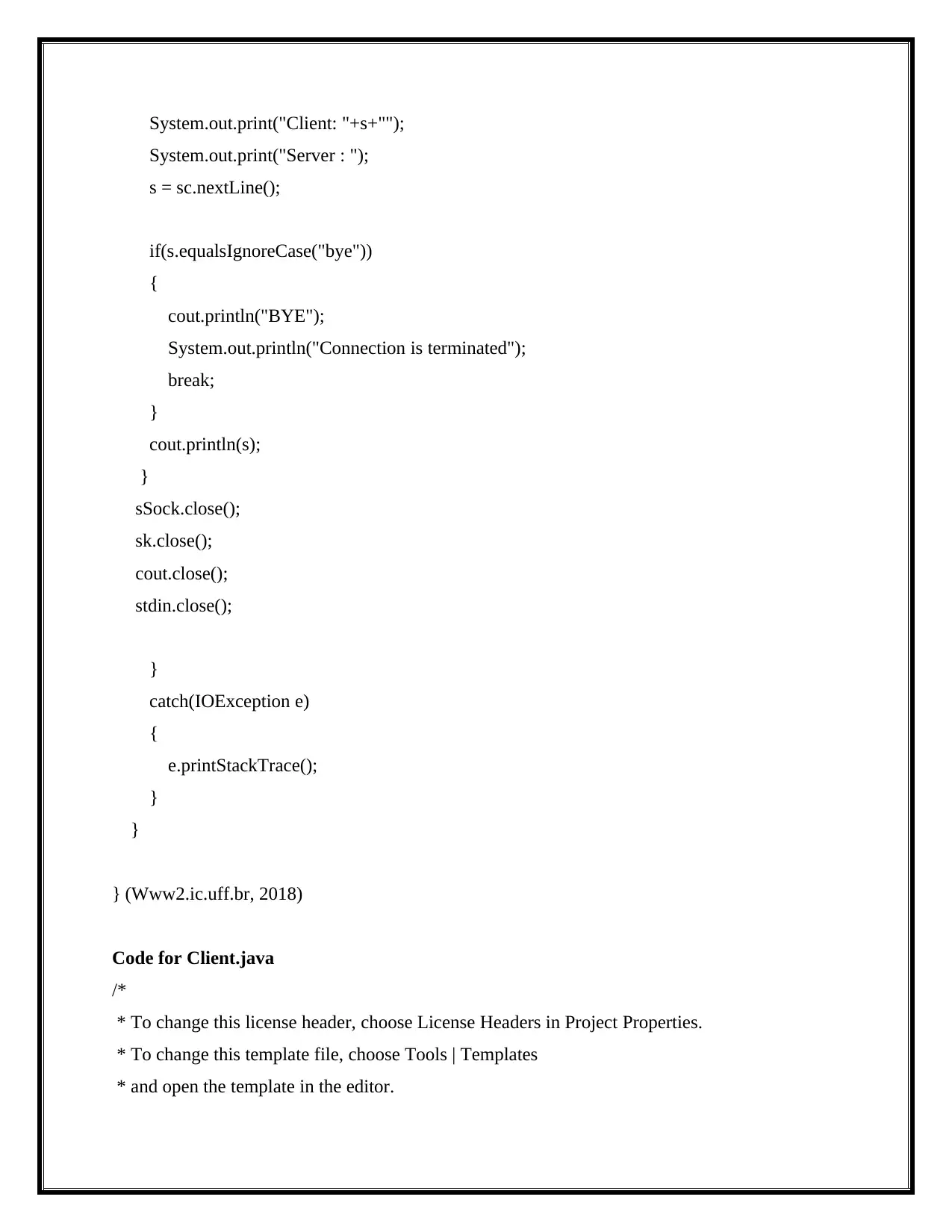
System.out.print("Client: "+s+"");
System.out.print("Server : ");
s = sc.nextLine();
if(s.equalsIgnoreCase("bye"))
{
cout.println("BYE");
System.out.println("Connection is terminated");
break;
}
cout.println(s);
}
sSock.close();
sk.close();
cout.close();
stdin.close();
}
catch(IOException e)
{
e.printStackTrace();
}
}
} (Www2.ic.uff.br, 2018)
Code for Client.java
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
System.out.print("Server : ");
s = sc.nextLine();
if(s.equalsIgnoreCase("bye"))
{
cout.println("BYE");
System.out.println("Connection is terminated");
break;
}
cout.println(s);
}
sSock.close();
sk.close();
cout.close();
stdin.close();
}
catch(IOException e)
{
e.printStackTrace();
}
}
} (Www2.ic.uff.br, 2018)
Code for Client.java
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
⊘ This is a preview!⊘
Do you want full access?
Subscribe today to unlock all pages.

Trusted by 1+ million students worldwide
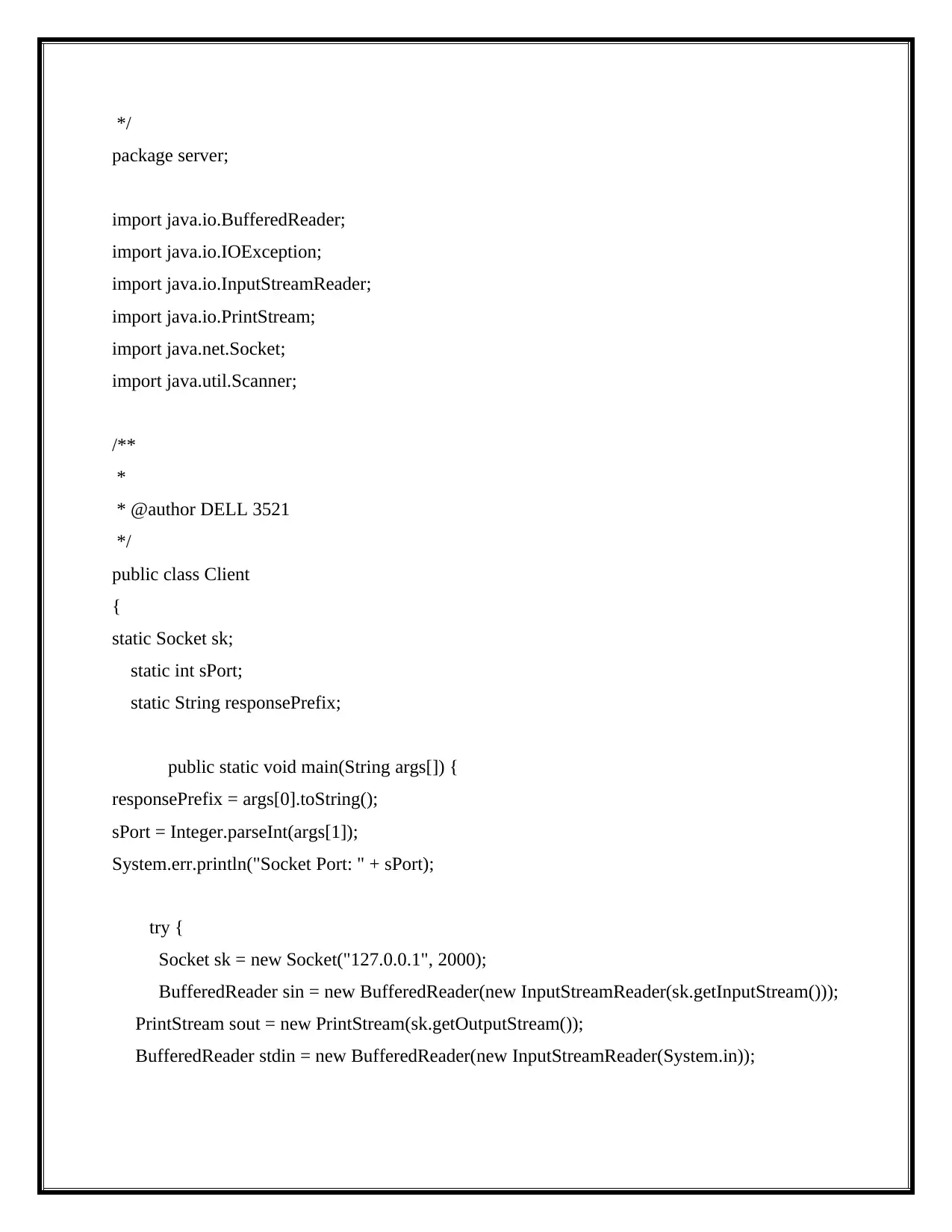
*/
package server;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.PrintStream;
import java.net.Socket;
import java.util.Scanner;
/**
*
* @author DELL 3521
*/
public class Client
{
static Socket sk;
static int sPort;
static String responsePrefix;
public static void main(String args[]) {
responsePrefix = args[0].toString();
sPort = Integer.parseInt(args[1]);
System.err.println("Socket Port: " + sPort);
try {
Socket sk = new Socket("127.0.0.1", 2000);
BufferedReader sin = new BufferedReader(new InputStreamReader(sk.getInputStream()));
PrintStream sout = new PrintStream(sk.getOutputStream());
BufferedReader stdin = new BufferedReader(new InputStreamReader(System.in));
package server;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.PrintStream;
import java.net.Socket;
import java.util.Scanner;
/**
*
* @author DELL 3521
*/
public class Client
{
static Socket sk;
static int sPort;
static String responsePrefix;
public static void main(String args[]) {
responsePrefix = args[0].toString();
sPort = Integer.parseInt(args[1]);
System.err.println("Socket Port: " + sPort);
try {
Socket sk = new Socket("127.0.0.1", 2000);
BufferedReader sin = new BufferedReader(new InputStreamReader(sk.getInputStream()));
PrintStream sout = new PrintStream(sk.getOutputStream());
BufferedReader stdin = new BufferedReader(new InputStreamReader(System.in));
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
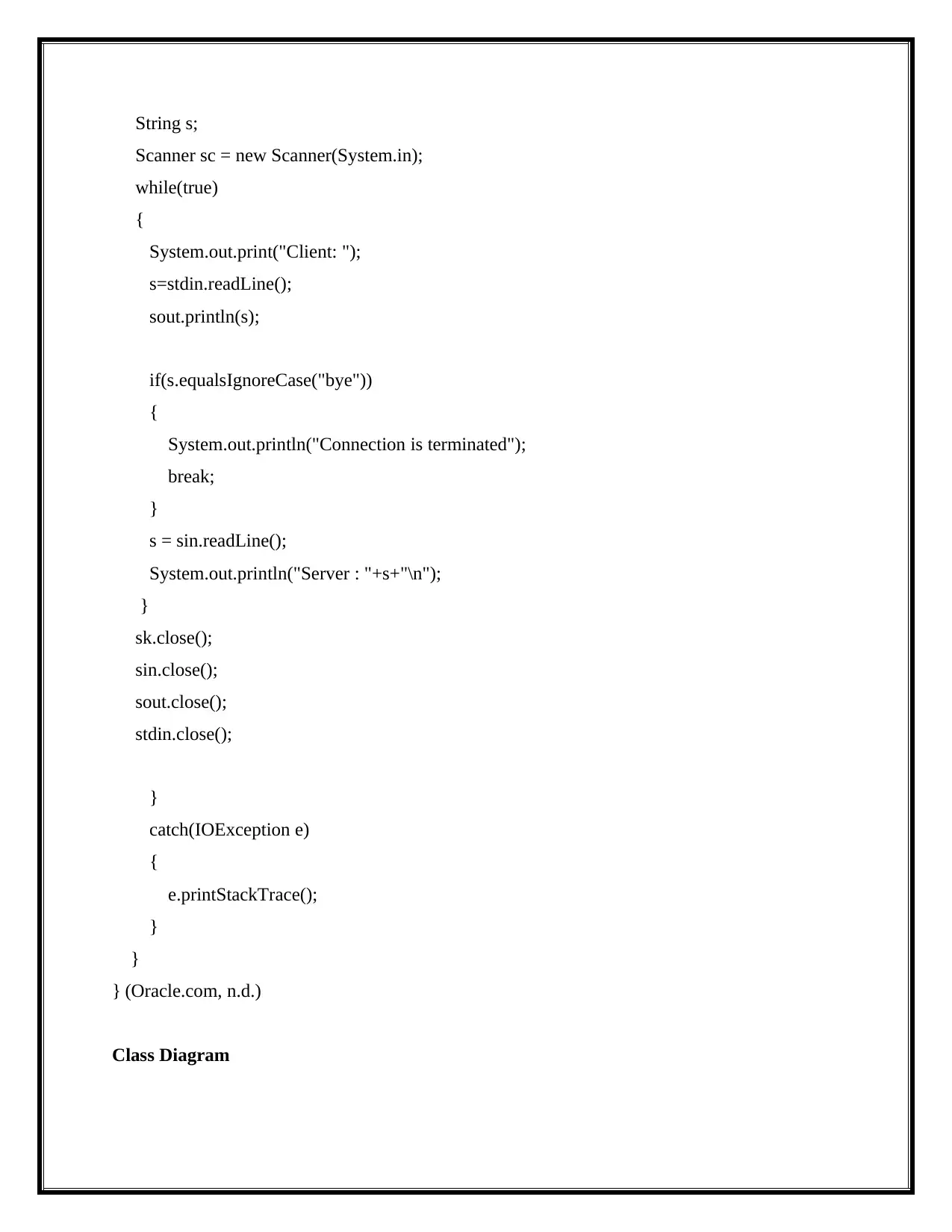
String s;
Scanner sc = new Scanner(System.in);
while(true)
{
System.out.print("Client: ");
s=stdin.readLine();
sout.println(s);
if(s.equalsIgnoreCase("bye"))
{
System.out.println("Connection is terminated");
break;
}
s = sin.readLine();
System.out.println("Server : "+s+"\n");
}
sk.close();
sin.close();
sout.close();
stdin.close();
}
catch(IOException e)
{
e.printStackTrace();
}
}
} (Oracle.com, n.d.)
Class Diagram
Scanner sc = new Scanner(System.in);
while(true)
{
System.out.print("Client: ");
s=stdin.readLine();
sout.println(s);
if(s.equalsIgnoreCase("bye"))
{
System.out.println("Connection is terminated");
break;
}
s = sin.readLine();
System.out.println("Server : "+s+"\n");
}
sk.close();
sin.close();
sout.close();
stdin.close();
}
catch(IOException e)
{
e.printStackTrace();
}
}
} (Oracle.com, n.d.)
Class Diagram
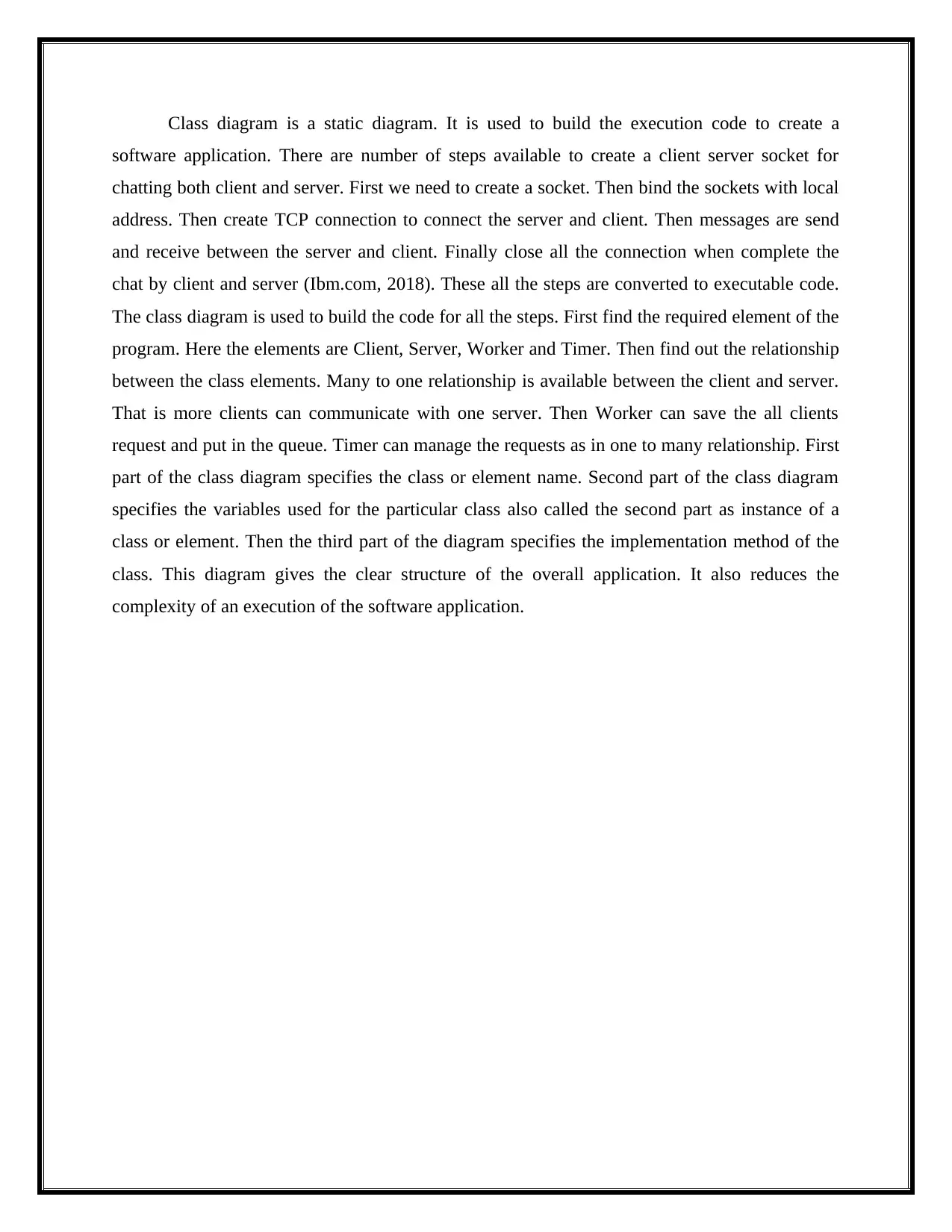
Class diagram is a static diagram. It is used to build the execution code to create a
software application. There are number of steps available to create a client server socket for
chatting both client and server. First we need to create a socket. Then bind the sockets with local
address. Then create TCP connection to connect the server and client. Then messages are send
and receive between the server and client. Finally close all the connection when complete the
chat by client and server (Ibm.com, 2018). These all the steps are converted to executable code.
The class diagram is used to build the code for all the steps. First find the required element of the
program. Here the elements are Client, Server, Worker and Timer. Then find out the relationship
between the class elements. Many to one relationship is available between the client and server.
That is more clients can communicate with one server. Then Worker can save the all clients
request and put in the queue. Timer can manage the requests as in one to many relationship. First
part of the class diagram specifies the class or element name. Second part of the class diagram
specifies the variables used for the particular class also called the second part as instance of a
class or element. Then the third part of the diagram specifies the implementation method of the
class. This diagram gives the clear structure of the overall application. It also reduces the
complexity of an execution of the software application.
software application. There are number of steps available to create a client server socket for
chatting both client and server. First we need to create a socket. Then bind the sockets with local
address. Then create TCP connection to connect the server and client. Then messages are send
and receive between the server and client. Finally close all the connection when complete the
chat by client and server (Ibm.com, 2018). These all the steps are converted to executable code.
The class diagram is used to build the code for all the steps. First find the required element of the
program. Here the elements are Client, Server, Worker and Timer. Then find out the relationship
between the class elements. Many to one relationship is available between the client and server.
That is more clients can communicate with one server. Then Worker can save the all clients
request and put in the queue. Timer can manage the requests as in one to many relationship. First
part of the class diagram specifies the class or element name. Second part of the class diagram
specifies the variables used for the particular class also called the second part as instance of a
class or element. Then the third part of the diagram specifies the implementation method of the
class. This diagram gives the clear structure of the overall application. It also reduces the
complexity of an execution of the software application.
⊘ This is a preview!⊘
Do you want full access?
Subscribe today to unlock all pages.

Trusted by 1+ million students worldwide
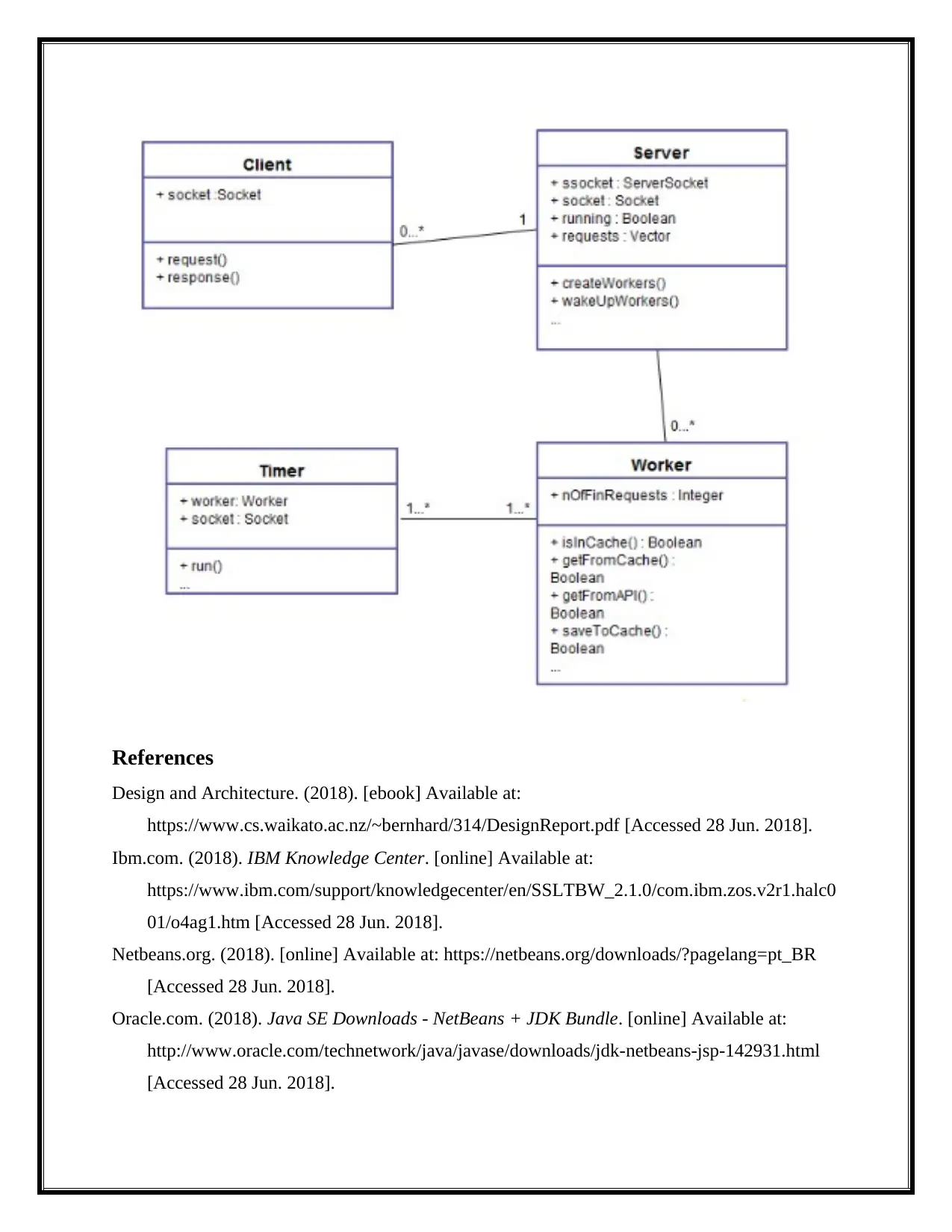
References
Design and Architecture. (2018). [ebook] Available at:
https://www.cs.waikato.ac.nz/~bernhard/314/DesignReport.pdf [Accessed 28 Jun. 2018].
Ibm.com. (2018). IBM Knowledge Center. [online] Available at:
https://www.ibm.com/support/knowledgecenter/en/SSLTBW_2.1.0/com.ibm.zos.v2r1.halc0
01/o4ag1.htm [Accessed 28 Jun. 2018].
Netbeans.org. (2018). [online] Available at: https://netbeans.org/downloads/?pagelang=pt_BR
[Accessed 28 Jun. 2018].
Oracle.com. (2018). Java SE Downloads - NetBeans + JDK Bundle. [online] Available at:
http://www.oracle.com/technetwork/java/javase/downloads/jdk-netbeans-jsp-142931.html
[Accessed 28 Jun. 2018].
Design and Architecture. (2018). [ebook] Available at:
https://www.cs.waikato.ac.nz/~bernhard/314/DesignReport.pdf [Accessed 28 Jun. 2018].
Ibm.com. (2018). IBM Knowledge Center. [online] Available at:
https://www.ibm.com/support/knowledgecenter/en/SSLTBW_2.1.0/com.ibm.zos.v2r1.halc0
01/o4ag1.htm [Accessed 28 Jun. 2018].
Netbeans.org. (2018). [online] Available at: https://netbeans.org/downloads/?pagelang=pt_BR
[Accessed 28 Jun. 2018].
Oracle.com. (2018). Java SE Downloads - NetBeans + JDK Bundle. [online] Available at:
http://www.oracle.com/technetwork/java/javase/downloads/jdk-netbeans-jsp-142931.html
[Accessed 28 Jun. 2018].
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
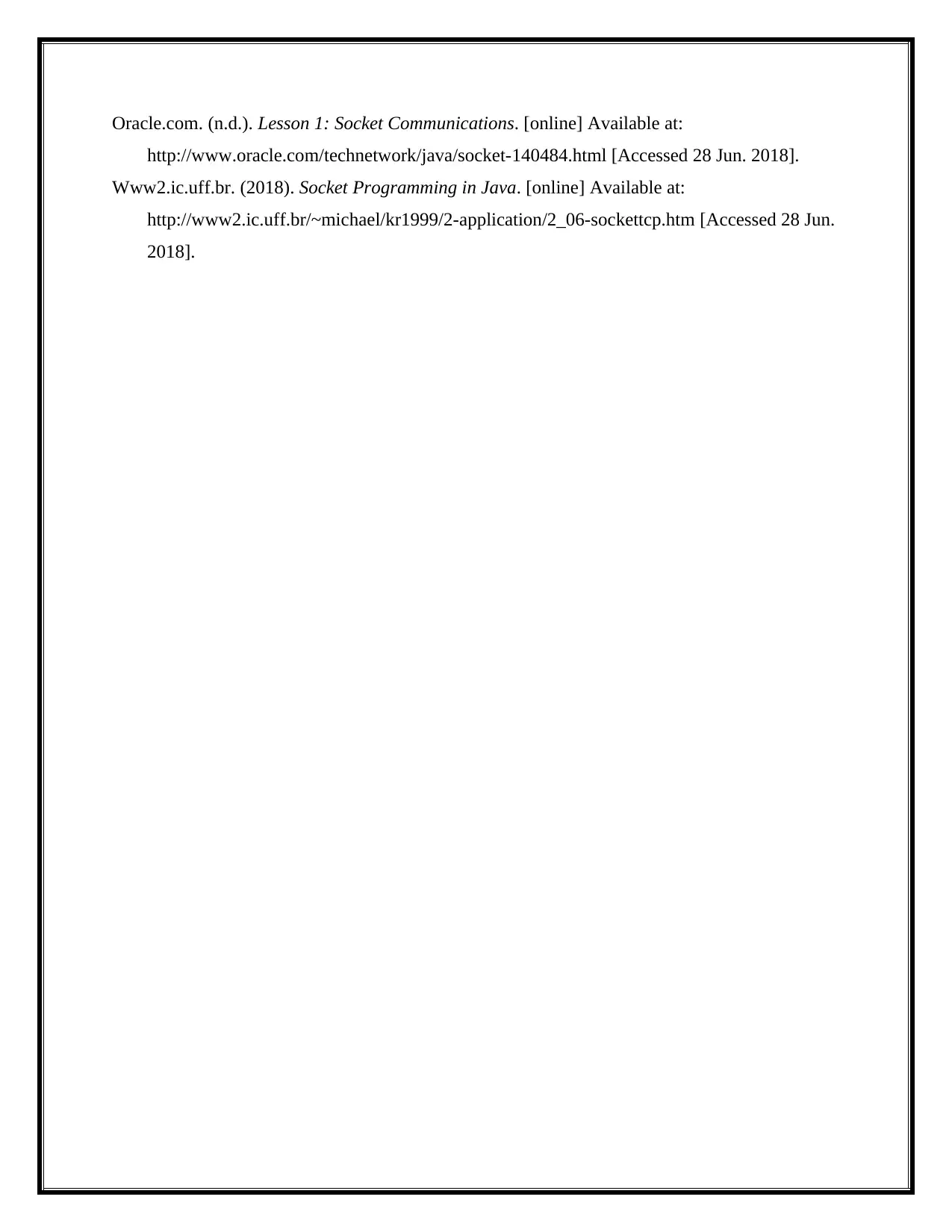
Oracle.com. (n.d.). Lesson 1: Socket Communications. [online] Available at:
http://www.oracle.com/technetwork/java/socket-140484.html [Accessed 28 Jun. 2018].
Www2.ic.uff.br. (2018). Socket Programming in Java. [online] Available at:
http://www2.ic.uff.br/~michael/kr1999/2-application/2_06-sockettcp.htm [Accessed 28 Jun.
2018].
http://www.oracle.com/technetwork/java/socket-140484.html [Accessed 28 Jun. 2018].
Www2.ic.uff.br. (2018). Socket Programming in Java. [online] Available at:
http://www2.ic.uff.br/~michael/kr1999/2-application/2_06-sockettcp.htm [Accessed 28 Jun.
2018].
1 out of 17
Related Documents

Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.