Report: Java Project on File System Simulation and Image Processing
VerifiedAdded on 2021/12/15
|7
|2002
|167
Report
AI Summary
This report presents a Java project that simulates a file system, encompassing various functionalities. The project is structured into several segments, including displaying a file's content, showing the file table, presenting the free space bitmap, displaying a disk block, copying files between the simulation and the real system, deleting files, and performing image processing operations. The code utilizes several Java packages such as java.io.* for input/output operations, java.util.* for utility functions, java.awt.image.BufferedImage and javax.imageio.ImageIO for image handling. The report explains the code for each function, including file display, file table display, disk space details, disk block display, file copying between simulated and real environments, file deletion, and image processing. Each function's code snippet is followed by a brief discussion of its purpose. The project concludes with a system exit command and includes references to relevant research papers on image processing and Java programming.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
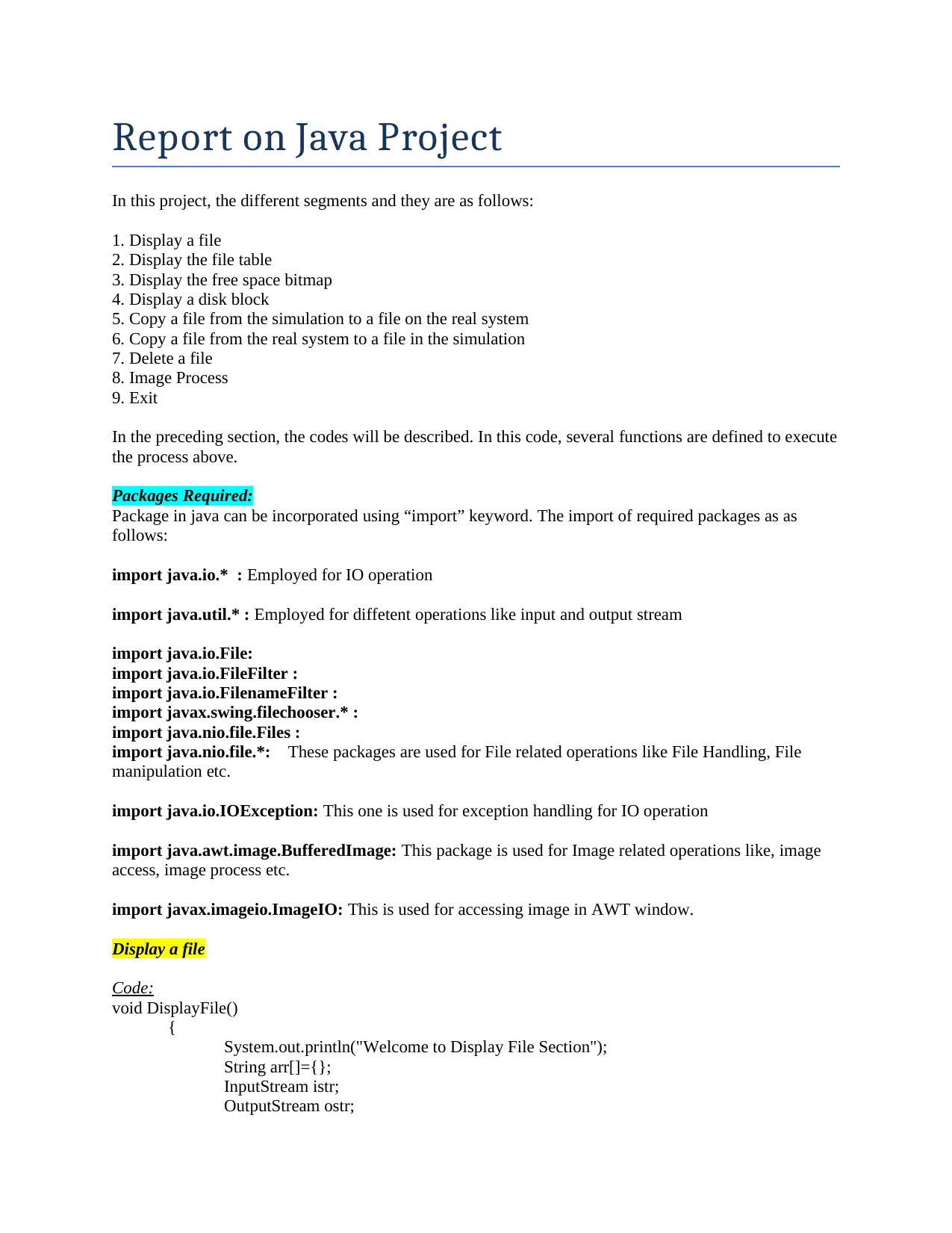
Report on Java Project
In this project, the different segments and they are as follows:
1. Display a file
2. Display the file table
3. Display the free space bitmap
4. Display a disk block
5. Copy a file from the simulation to a file on the real system
6. Copy a file from the real system to a file in the simulation
7. Delete a file
8. Image Process
9. Exit
In the preceding section, the codes will be described. In this code, several functions are defined to execute
the process above.
Packages Required:
Package in java can be incorporated using “import” keyword. The import of required packages as as
follows:
import java.io.* : Employed for IO operation
import java.util.* : Employed for diffetent operations like input and output stream
import java.io.File:
import java.io.FileFilter :
import java.io.FilenameFilter :
import javax.swing.filechooser.* :
import java.nio.file.Files :
import java.nio.file.*: These packages are used for File related operations like File Handling, File
manipulation etc.
import java.io.IOException: This one is used for exception handling for IO operation
import java.awt.image.BufferedImage: This package is used for Image related operations like, image
access, image process etc.
import javax.imageio.ImageIO: This is used for accessing image in AWT window.
Display a file
Code:
void DisplayFile()
{
System.out.println("Welcome to Display File Section");
String arr[]={};
InputStream istr;
OutputStream ostr;
In this project, the different segments and they are as follows:
1. Display a file
2. Display the file table
3. Display the free space bitmap
4. Display a disk block
5. Copy a file from the simulation to a file on the real system
6. Copy a file from the real system to a file in the simulation
7. Delete a file
8. Image Process
9. Exit
In the preceding section, the codes will be described. In this code, several functions are defined to execute
the process above.
Packages Required:
Package in java can be incorporated using “import” keyword. The import of required packages as as
follows:
import java.io.* : Employed for IO operation
import java.util.* : Employed for diffetent operations like input and output stream
import java.io.File:
import java.io.FileFilter :
import java.io.FilenameFilter :
import javax.swing.filechooser.* :
import java.nio.file.Files :
import java.nio.file.*: These packages are used for File related operations like File Handling, File
manipulation etc.
import java.io.IOException: This one is used for exception handling for IO operation
import java.awt.image.BufferedImage: This package is used for Image related operations like, image
access, image process etc.
import javax.imageio.ImageIO: This is used for accessing image in AWT window.
Display a file
Code:
void DisplayFile()
{
System.out.println("Welcome to Display File Section");
String arr[]={};
InputStream istr;
OutputStream ostr;
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
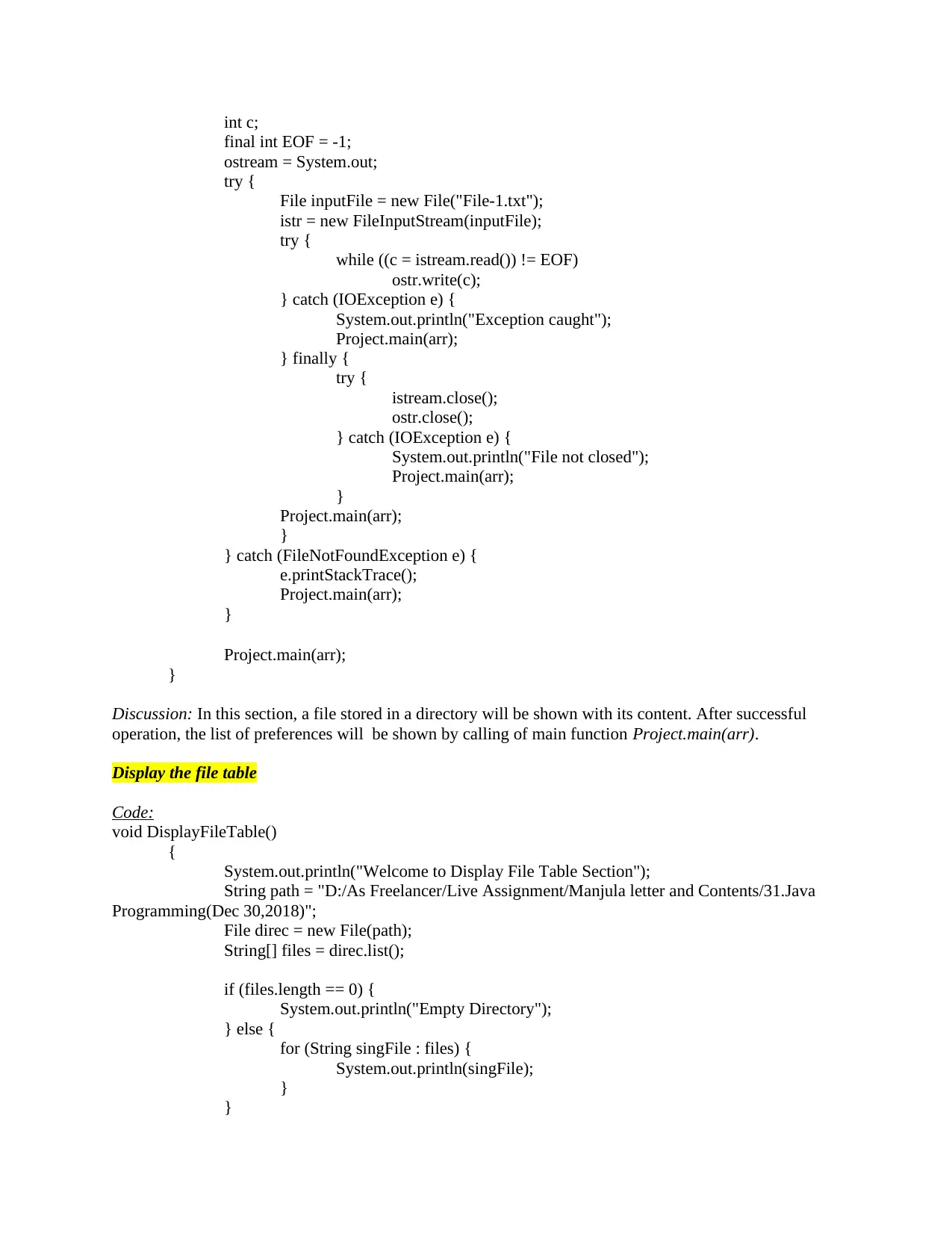
int c;
final int EOF = -1;
ostream = System.out;
try {
File inputFile = new File("File-1.txt");
istr = new FileInputStream(inputFile);
try {
while ((c = istream.read()) != EOF)
ostr.write(c);
} catch (IOException e) {
System.out.println("Exception caught");
Project.main(arr);
} finally {
try {
istream.close();
ostr.close();
} catch (IOException e) {
System.out.println("File not closed");
Project.main(arr);
}
Project.main(arr);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
Project.main(arr);
}
Project.main(arr);
}
Discussion: In this section, a file stored in a directory will be shown with its content. After successful
operation, the list of preferences will be shown by calling of main function Project.main(arr).
Display the file table
Code:
void DisplayFileTable()
{
System.out.println("Welcome to Display File Table Section");
String path = "D:/As Freelancer/Live Assignment/Manjula letter and Contents/31.Java
Programming(Dec 30,2018)";
File direc = new File(path);
String[] files = direc.list();
if (files.length == 0) {
System.out.println("Empty Directory");
} else {
for (String singFile : files) {
System.out.println(singFile);
}
}
final int EOF = -1;
ostream = System.out;
try {
File inputFile = new File("File-1.txt");
istr = new FileInputStream(inputFile);
try {
while ((c = istream.read()) != EOF)
ostr.write(c);
} catch (IOException e) {
System.out.println("Exception caught");
Project.main(arr);
} finally {
try {
istream.close();
ostr.close();
} catch (IOException e) {
System.out.println("File not closed");
Project.main(arr);
}
Project.main(arr);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
Project.main(arr);
}
Project.main(arr);
}
Discussion: In this section, a file stored in a directory will be shown with its content. After successful
operation, the list of preferences will be shown by calling of main function Project.main(arr).
Display the file table
Code:
void DisplayFileTable()
{
System.out.println("Welcome to Display File Table Section");
String path = "D:/As Freelancer/Live Assignment/Manjula letter and Contents/31.Java
Programming(Dec 30,2018)";
File direc = new File(path);
String[] files = direc.list();
if (files.length == 0) {
System.out.println("Empty Directory");
} else {
for (String singFile : files) {
System.out.println(singFile);
}
}
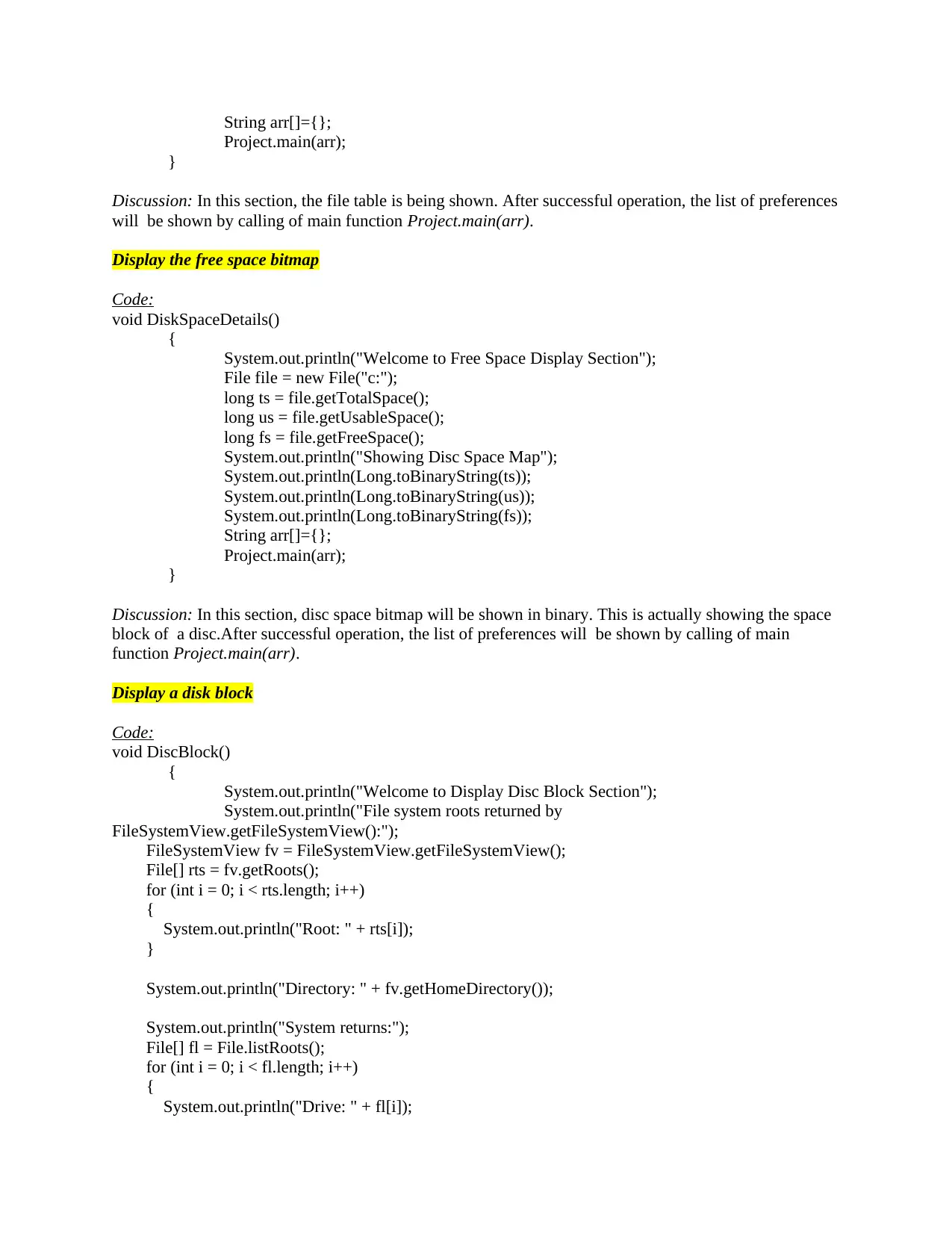
String arr[]={};
Project.main(arr);
}
Discussion: In this section, the file table is being shown. After successful operation, the list of preferences
will be shown by calling of main function Project.main(arr).
Display the free space bitmap
Code:
void DiskSpaceDetails()
{
System.out.println("Welcome to Free Space Display Section");
File file = new File("c:");
long ts = file.getTotalSpace();
long us = file.getUsableSpace();
long fs = file.getFreeSpace();
System.out.println("Showing Disc Space Map");
System.out.println(Long.toBinaryString(ts));
System.out.println(Long.toBinaryString(us));
System.out.println(Long.toBinaryString(fs));
String arr[]={};
Project.main(arr);
}
Discussion: In this section, disc space bitmap will be shown in binary. This is actually showing the space
block of a disc.After successful operation, the list of preferences will be shown by calling of main
function Project.main(arr).
Display a disk block
Code:
void DiscBlock()
{
System.out.println("Welcome to Display Disc Block Section");
System.out.println("File system roots returned by
FileSystemView.getFileSystemView():");
FileSystemView fv = FileSystemView.getFileSystemView();
File[] rts = fv.getRoots();
for (int i = 0; i < rts.length; i++)
{
System.out.println("Root: " + rts[i]);
}
System.out.println("Directory: " + fv.getHomeDirectory());
System.out.println("System returns:");
File[] fl = File.listRoots();
for (int i = 0; i < fl.length; i++)
{
System.out.println("Drive: " + fl[i]);
Project.main(arr);
}
Discussion: In this section, the file table is being shown. After successful operation, the list of preferences
will be shown by calling of main function Project.main(arr).
Display the free space bitmap
Code:
void DiskSpaceDetails()
{
System.out.println("Welcome to Free Space Display Section");
File file = new File("c:");
long ts = file.getTotalSpace();
long us = file.getUsableSpace();
long fs = file.getFreeSpace();
System.out.println("Showing Disc Space Map");
System.out.println(Long.toBinaryString(ts));
System.out.println(Long.toBinaryString(us));
System.out.println(Long.toBinaryString(fs));
String arr[]={};
Project.main(arr);
}
Discussion: In this section, disc space bitmap will be shown in binary. This is actually showing the space
block of a disc.After successful operation, the list of preferences will be shown by calling of main
function Project.main(arr).
Display a disk block
Code:
void DiscBlock()
{
System.out.println("Welcome to Display Disc Block Section");
System.out.println("File system roots returned by
FileSystemView.getFileSystemView():");
FileSystemView fv = FileSystemView.getFileSystemView();
File[] rts = fv.getRoots();
for (int i = 0; i < rts.length; i++)
{
System.out.println("Root: " + rts[i]);
}
System.out.println("Directory: " + fv.getHomeDirectory());
System.out.println("System returns:");
File[] fl = File.listRoots();
for (int i = 0; i < fl.length; i++)
{
System.out.println("Drive: " + fl[i]);
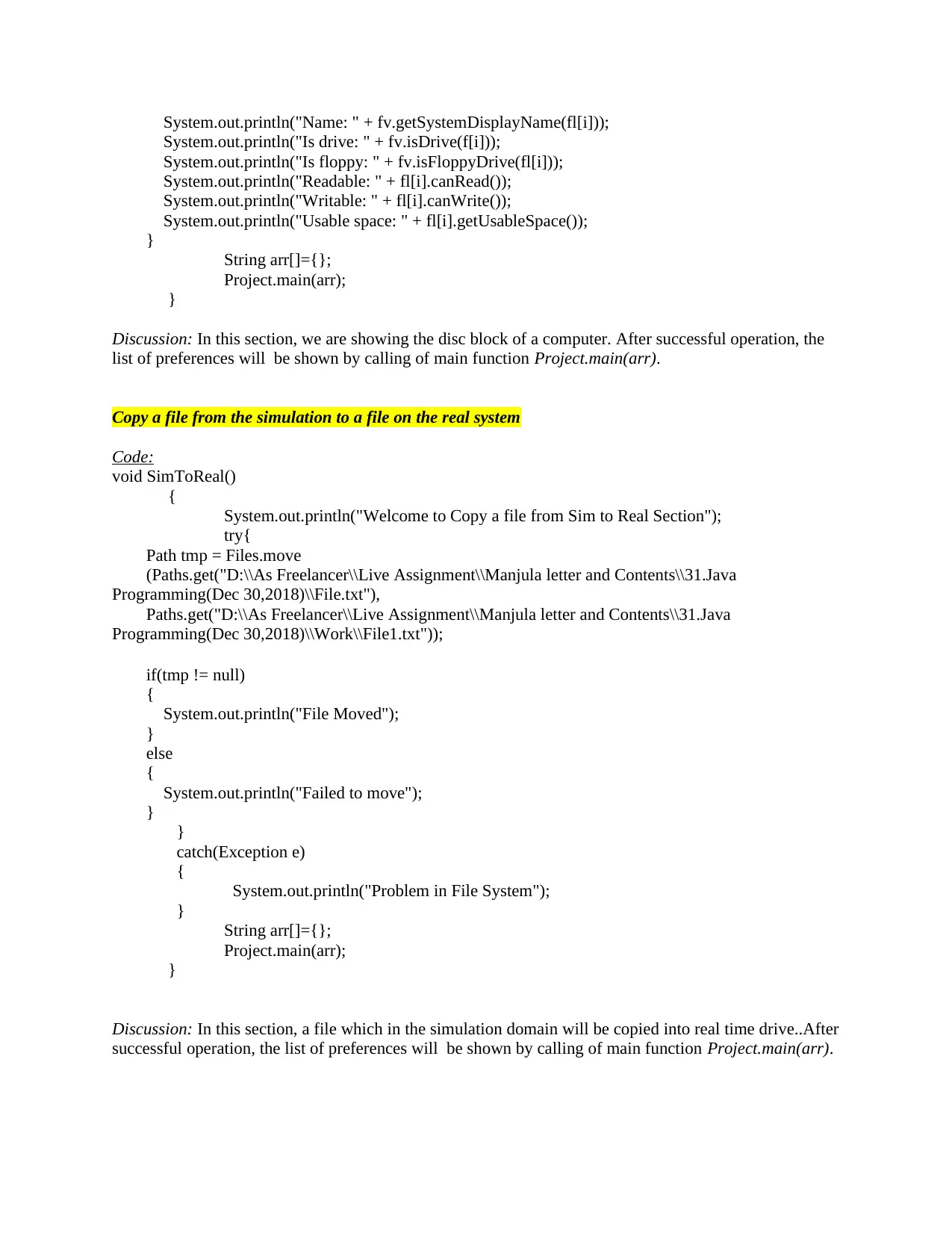
System.out.println("Name: " + fv.getSystemDisplayName(fl[i]));
System.out.println("Is drive: " + fv.isDrive(f[i]));
System.out.println("Is floppy: " + fv.isFloppyDrive(fl[i]));
System.out.println("Readable: " + fl[i].canRead());
System.out.println("Writable: " + fl[i].canWrite());
System.out.println("Usable space: " + fl[i].getUsableSpace());
}
String arr[]={};
Project.main(arr);
}
Discussion: In this section, we are showing the disc block of a computer. After successful operation, the
list of preferences will be shown by calling of main function Project.main(arr).
Copy a file from the simulation to a file on the real system
Code:
void SimToReal()
{
System.out.println("Welcome to Copy a file from Sim to Real Section");
try{
Path tmp = Files.move
(Paths.get("D:\\As Freelancer\\Live Assignment\\Manjula letter and Contents\\31.Java
Programming(Dec 30,2018)\\File.txt"),
Paths.get("D:\\As Freelancer\\Live Assignment\\Manjula letter and Contents\\31.Java
Programming(Dec 30,2018)\\Work\\File1.txt"));
if(tmp != null)
{
System.out.println("File Moved");
}
else
{
System.out.println("Failed to move");
}
}
catch(Exception e)
{
System.out.println("Problem in File System");
}
String arr[]={};
Project.main(arr);
}
Discussion: In this section, a file which in the simulation domain will be copied into real time drive..After
successful operation, the list of preferences will be shown by calling of main function Project.main(arr).
System.out.println("Is drive: " + fv.isDrive(f[i]));
System.out.println("Is floppy: " + fv.isFloppyDrive(fl[i]));
System.out.println("Readable: " + fl[i].canRead());
System.out.println("Writable: " + fl[i].canWrite());
System.out.println("Usable space: " + fl[i].getUsableSpace());
}
String arr[]={};
Project.main(arr);
}
Discussion: In this section, we are showing the disc block of a computer. After successful operation, the
list of preferences will be shown by calling of main function Project.main(arr).
Copy a file from the simulation to a file on the real system
Code:
void SimToReal()
{
System.out.println("Welcome to Copy a file from Sim to Real Section");
try{
Path tmp = Files.move
(Paths.get("D:\\As Freelancer\\Live Assignment\\Manjula letter and Contents\\31.Java
Programming(Dec 30,2018)\\File.txt"),
Paths.get("D:\\As Freelancer\\Live Assignment\\Manjula letter and Contents\\31.Java
Programming(Dec 30,2018)\\Work\\File1.txt"));
if(tmp != null)
{
System.out.println("File Moved");
}
else
{
System.out.println("Failed to move");
}
}
catch(Exception e)
{
System.out.println("Problem in File System");
}
String arr[]={};
Project.main(arr);
}
Discussion: In this section, a file which in the simulation domain will be copied into real time drive..After
successful operation, the list of preferences will be shown by calling of main function Project.main(arr).
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
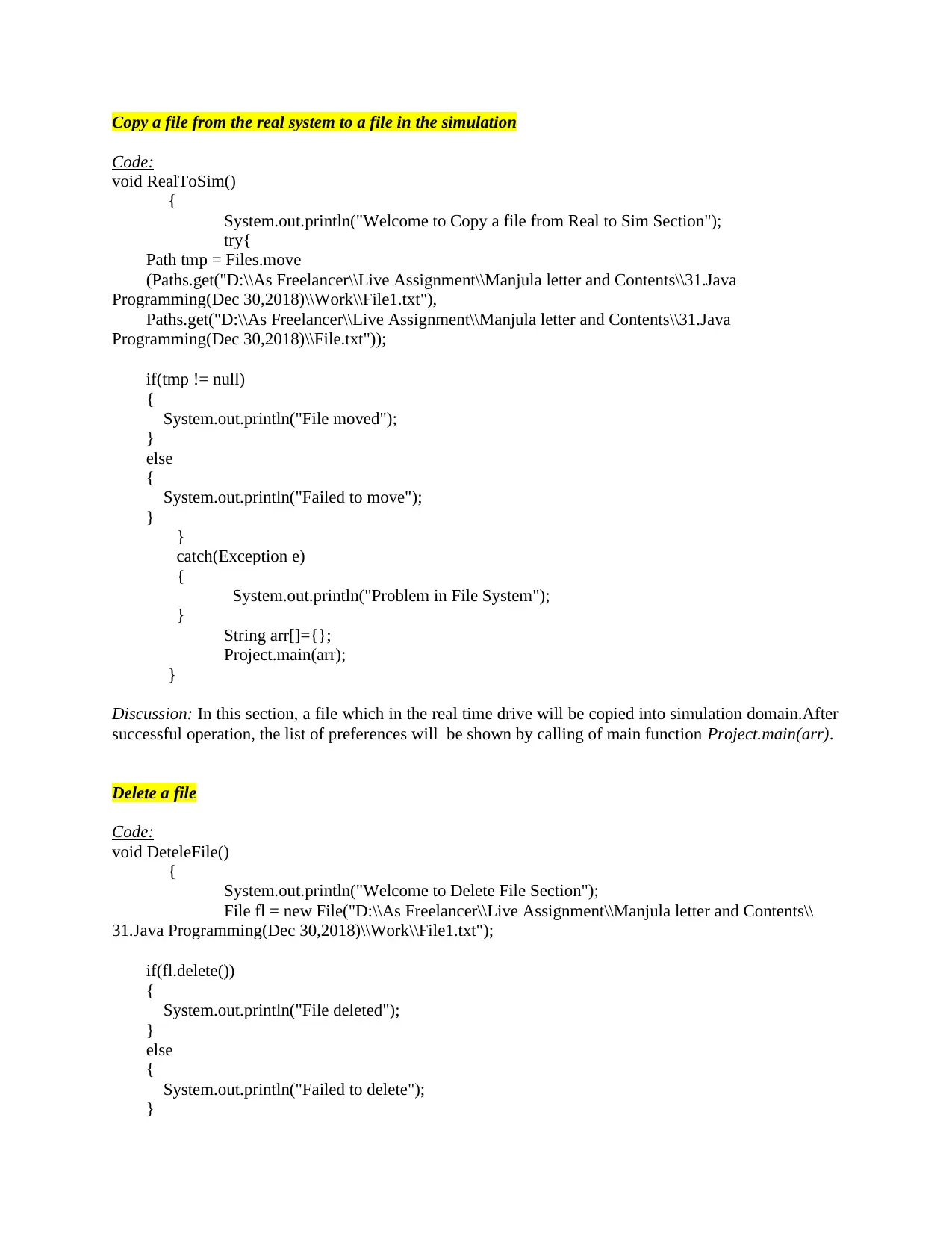
Copy a file from the real system to a file in the simulation
Code:
void RealToSim()
{
System.out.println("Welcome to Copy a file from Real to Sim Section");
try{
Path tmp = Files.move
(Paths.get("D:\\As Freelancer\\Live Assignment\\Manjula letter and Contents\\31.Java
Programming(Dec 30,2018)\\Work\\File1.txt"),
Paths.get("D:\\As Freelancer\\Live Assignment\\Manjula letter and Contents\\31.Java
Programming(Dec 30,2018)\\File.txt"));
if(tmp != null)
{
System.out.println("File moved");
}
else
{
System.out.println("Failed to move");
}
}
catch(Exception e)
{
System.out.println("Problem in File System");
}
String arr[]={};
Project.main(arr);
}
Discussion: In this section, a file which in the real time drive will be copied into simulation domain.After
successful operation, the list of preferences will be shown by calling of main function Project.main(arr).
Delete a file
Code:
void DeteleFile()
{
System.out.println("Welcome to Delete File Section");
File fl = new File("D:\\As Freelancer\\Live Assignment\\Manjula letter and Contents\\
31.Java Programming(Dec 30,2018)\\Work\\File1.txt");
if(fl.delete())
{
System.out.println("File deleted");
}
else
{
System.out.println("Failed to delete");
}
Code:
void RealToSim()
{
System.out.println("Welcome to Copy a file from Real to Sim Section");
try{
Path tmp = Files.move
(Paths.get("D:\\As Freelancer\\Live Assignment\\Manjula letter and Contents\\31.Java
Programming(Dec 30,2018)\\Work\\File1.txt"),
Paths.get("D:\\As Freelancer\\Live Assignment\\Manjula letter and Contents\\31.Java
Programming(Dec 30,2018)\\File.txt"));
if(tmp != null)
{
System.out.println("File moved");
}
else
{
System.out.println("Failed to move");
}
}
catch(Exception e)
{
System.out.println("Problem in File System");
}
String arr[]={};
Project.main(arr);
}
Discussion: In this section, a file which in the real time drive will be copied into simulation domain.After
successful operation, the list of preferences will be shown by calling of main function Project.main(arr).
Delete a file
Code:
void DeteleFile()
{
System.out.println("Welcome to Delete File Section");
File fl = new File("D:\\As Freelancer\\Live Assignment\\Manjula letter and Contents\\
31.Java Programming(Dec 30,2018)\\Work\\File1.txt");
if(fl.delete())
{
System.out.println("File deleted");
}
else
{
System.out.println("Failed to delete");
}
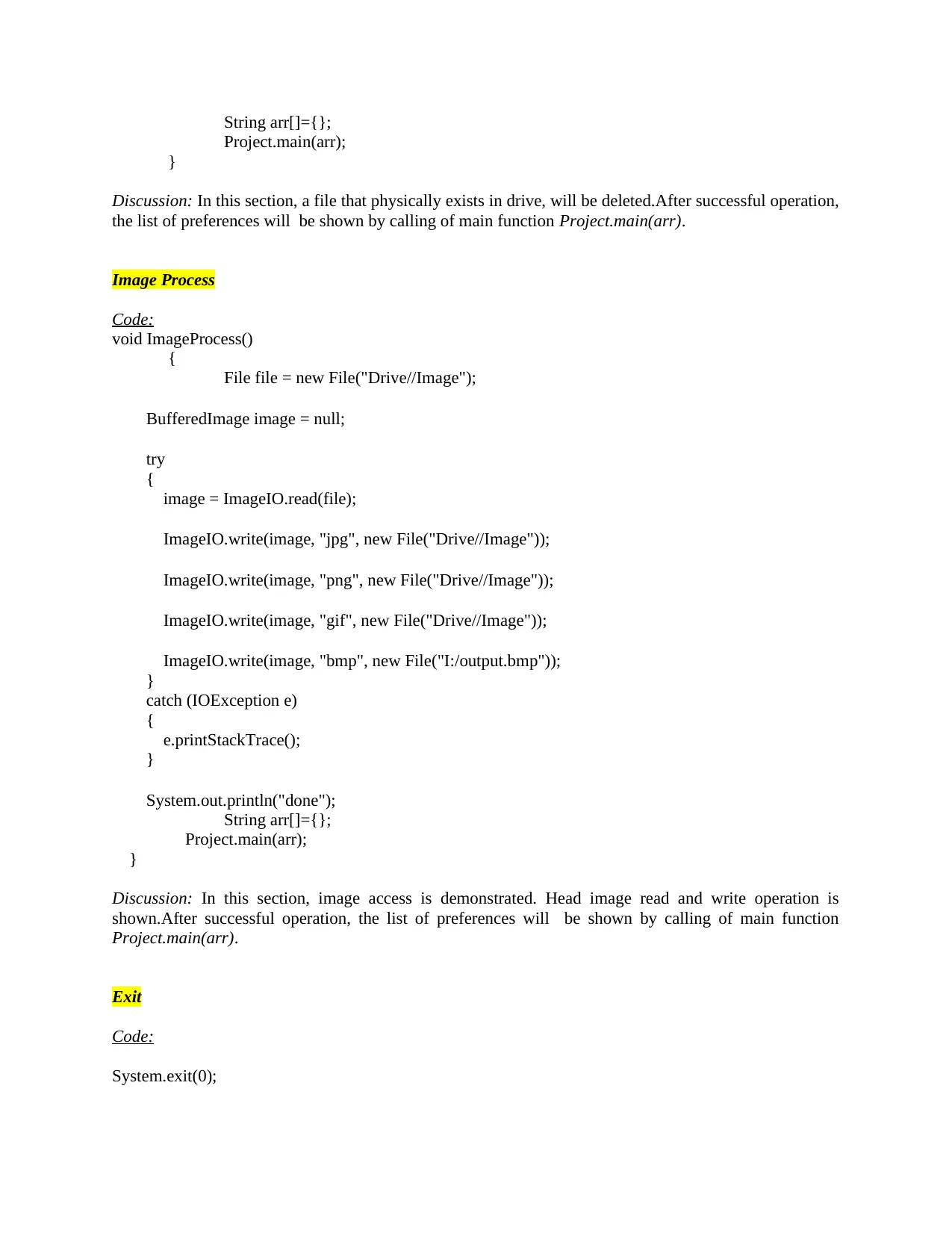
String arr[]={};
Project.main(arr);
}
Discussion: In this section, a file that physically exists in drive, will be deleted.After successful operation,
the list of preferences will be shown by calling of main function Project.main(arr).
Image Process
Code:
void ImageProcess()
{
File file = new File("Drive//Image");
BufferedImage image = null;
try
{
image = ImageIO.read(file);
ImageIO.write(image, "jpg", new File("Drive//Image"));
ImageIO.write(image, "png", new File("Drive//Image"));
ImageIO.write(image, "gif", new File("Drive//Image"));
ImageIO.write(image, "bmp", new File("I:/output.bmp"));
}
catch (IOException e)
{
e.printStackTrace();
}
System.out.println("done");
String arr[]={};
Project.main(arr);
}
Discussion: In this section, image access is demonstrated. Head image read and write operation is
shown.After successful operation, the list of preferences will be shown by calling of main function
Project.main(arr).
Exit
Code:
System.exit(0);
Project.main(arr);
}
Discussion: In this section, a file that physically exists in drive, will be deleted.After successful operation,
the list of preferences will be shown by calling of main function Project.main(arr).
Image Process
Code:
void ImageProcess()
{
File file = new File("Drive//Image");
BufferedImage image = null;
try
{
image = ImageIO.read(file);
ImageIO.write(image, "jpg", new File("Drive//Image"));
ImageIO.write(image, "png", new File("Drive//Image"));
ImageIO.write(image, "gif", new File("Drive//Image"));
ImageIO.write(image, "bmp", new File("I:/output.bmp"));
}
catch (IOException e)
{
e.printStackTrace();
}
System.out.println("done");
String arr[]={};
Project.main(arr);
}
Discussion: In this section, image access is demonstrated. Head image read and write operation is
shown.After successful operation, the list of preferences will be shown by calling of main function
Project.main(arr).
Exit
Code:
System.exit(0);
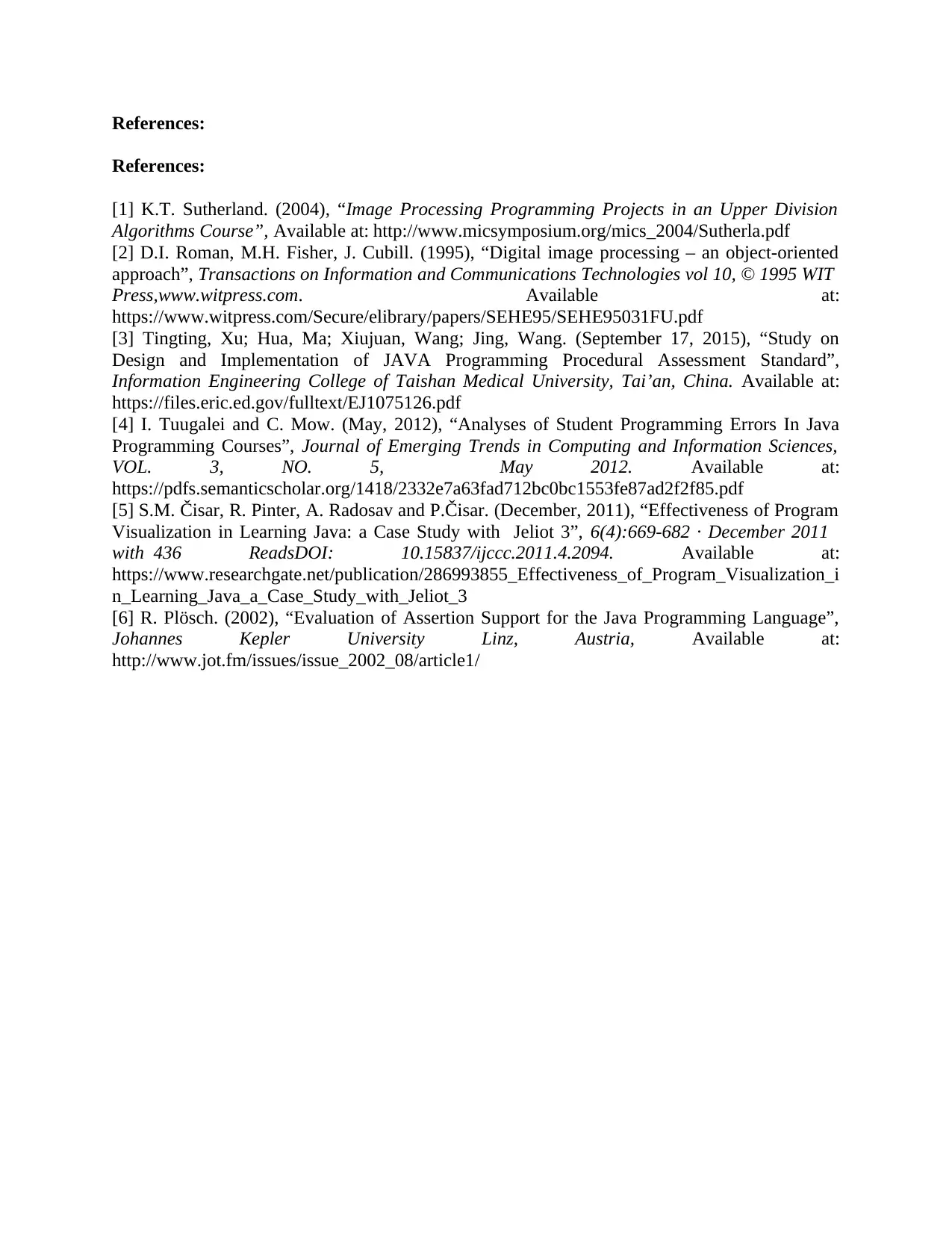
References:
References:
[1] K.T. Sutherland. (2004), “Image Processing Programming Projects in an Upper Division
Algorithms Course”, Available at: http://www.micsymposium.org/mics_2004/Sutherla.pdf
[2] D.I. Roman, M.H. Fisher, J. Cubill. (1995), “Digital image processing – an object-oriented
approach”, Transactions on Information and Communications Technologies vol 10, © 1995 WIT
Press,www.witpress.com. Available at:
https://www.witpress.com/Secure/elibrary/papers/SEHE95/SEHE95031FU.pdf
[3] Tingting, Xu; Hua, Ma; Xiujuan, Wang; Jing, Wang. (September 17, 2015), “Study on
Design and Implementation of JAVA Programming Procedural Assessment Standard”,
Information Engineering College of Taishan Medical University, Tai’an, China. Available at:
https://files.eric.ed.gov/fulltext/EJ1075126.pdf
[4] I. Tuugalei and C. Mow. (May, 2012), “Analyses of Student Programming Errors In Java
Programming Courses”, Journal of Emerging Trends in Computing and Information Sciences,
VOL. 3, NO. 5, May 2012. Available at:
https://pdfs.semanticscholar.org/1418/2332e7a63fad712bc0bc1553fe87ad2f2f85.pdf
[5] S.M. Čisar, R. Pinter, A. Radosav and P.Čisar. (December, 2011), “Effectiveness of Program
Visualization in Learning Java: a Case Study with Jeliot 3”, 6(4):669-682 · December 2011
with 436 ReadsDOI: 10.15837/ijccc.2011.4.2094. Available at:
https://www.researchgate.net/publication/286993855_Effectiveness_of_Program_Visualization_i
n_Learning_Java_a_Case_Study_with_Jeliot_3
[6] R. Plösch. (2002), “Evaluation of Assertion Support for the Java Programming Language”,
Johannes Kepler University Linz, Austria, Available at:
http://www.jot.fm/issues/issue_2002_08/article1/
References:
[1] K.T. Sutherland. (2004), “Image Processing Programming Projects in an Upper Division
Algorithms Course”, Available at: http://www.micsymposium.org/mics_2004/Sutherla.pdf
[2] D.I. Roman, M.H. Fisher, J. Cubill. (1995), “Digital image processing – an object-oriented
approach”, Transactions on Information and Communications Technologies vol 10, © 1995 WIT
Press,www.witpress.com. Available at:
https://www.witpress.com/Secure/elibrary/papers/SEHE95/SEHE95031FU.pdf
[3] Tingting, Xu; Hua, Ma; Xiujuan, Wang; Jing, Wang. (September 17, 2015), “Study on
Design and Implementation of JAVA Programming Procedural Assessment Standard”,
Information Engineering College of Taishan Medical University, Tai’an, China. Available at:
https://files.eric.ed.gov/fulltext/EJ1075126.pdf
[4] I. Tuugalei and C. Mow. (May, 2012), “Analyses of Student Programming Errors In Java
Programming Courses”, Journal of Emerging Trends in Computing and Information Sciences,
VOL. 3, NO. 5, May 2012. Available at:
https://pdfs.semanticscholar.org/1418/2332e7a63fad712bc0bc1553fe87ad2f2f85.pdf
[5] S.M. Čisar, R. Pinter, A. Radosav and P.Čisar. (December, 2011), “Effectiveness of Program
Visualization in Learning Java: a Case Study with Jeliot 3”, 6(4):669-682 · December 2011
with 436 ReadsDOI: 10.15837/ijccc.2011.4.2094. Available at:
https://www.researchgate.net/publication/286993855_Effectiveness_of_Program_Visualization_i
n_Learning_Java_a_Case_Study_with_Jeliot_3
[6] R. Plösch. (2002), “Evaluation of Assertion Support for the Java Programming Language”,
Johannes Kepler University Linz, Austria, Available at:
http://www.jot.fm/issues/issue_2002_08/article1/
1 out of 7

Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.