Java Assignment: Class Design, Implementation, and Testing of Gadgets
VerifiedAdded on 2019/09/20
|16
|1551
|57
Practical Assignment
AI Summary
This Java assignment focuses on the implementation of a Gadget class and its subclasses, Mobile and MP3. The solution includes the creation of these classes with appropriate attributes and methods, such as `addCallingCredits`, `phoneCall`, `downloadMusic`, and `deleteMusic`. The code demonstrates inheritance and object-oriented principles. Test cases are provided to validate the functionality of each class, including scenarios for adding calling credits, making phone calls, downloading and deleting music. A class diagram visually represents the class structure and relationships. The `TestClass` demonstrates how to create and interact with objects of each class, showcasing the expected behavior through various test scenarios. This assignment provides a comprehensive understanding of Java programming, class design, and testing methodologies.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
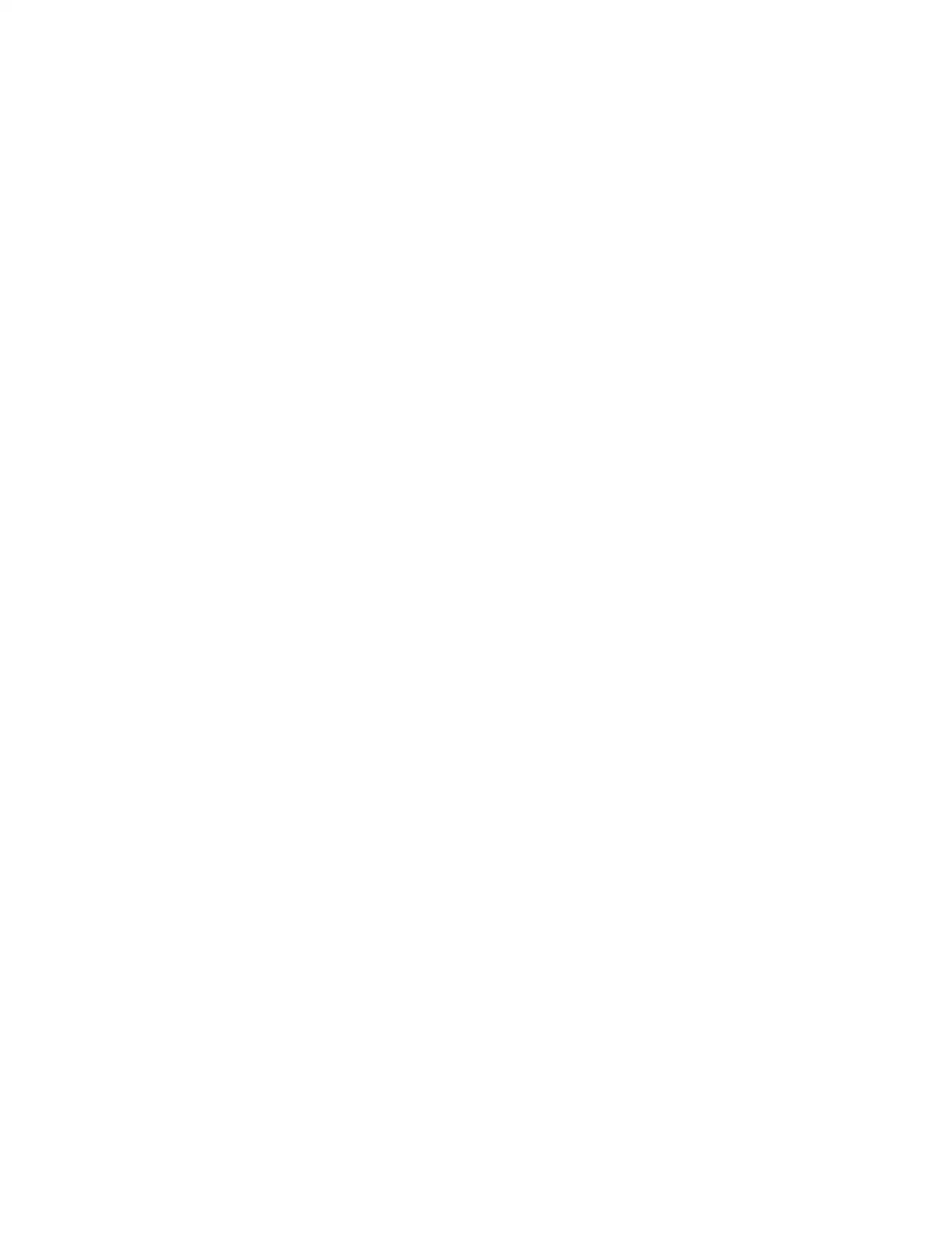
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
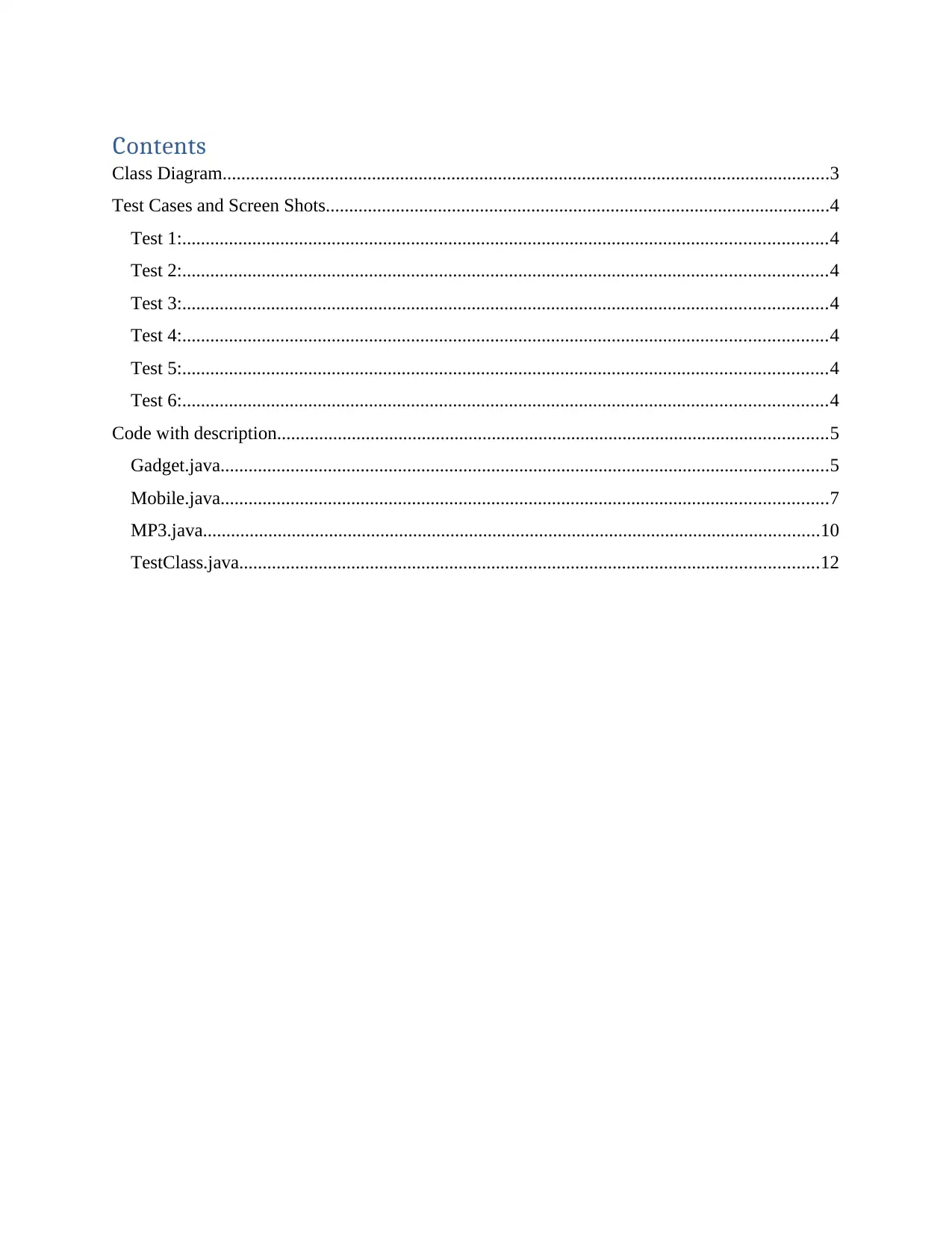
Contents
Class Diagram..................................................................................................................................3
Test Cases and Screen Shots............................................................................................................4
Test 1:..........................................................................................................................................4
Test 2:..........................................................................................................................................4
Test 3:..........................................................................................................................................4
Test 4:..........................................................................................................................................4
Test 5:..........................................................................................................................................4
Test 6:..........................................................................................................................................4
Code with description......................................................................................................................5
Gadget.java..................................................................................................................................5
Mobile.java..................................................................................................................................7
MP3.java....................................................................................................................................10
TestClass.java............................................................................................................................12
Class Diagram..................................................................................................................................3
Test Cases and Screen Shots............................................................................................................4
Test 1:..........................................................................................................................................4
Test 2:..........................................................................................................................................4
Test 3:..........................................................................................................................................4
Test 4:..........................................................................................................................................4
Test 5:..........................................................................................................................................4
Test 6:..........................................................................................................................................4
Code with description......................................................................................................................5
Gadget.java..................................................................................................................................5
Mobile.java..................................................................................................................................7
MP3.java....................................................................................................................................10
TestClass.java............................................................................................................................12
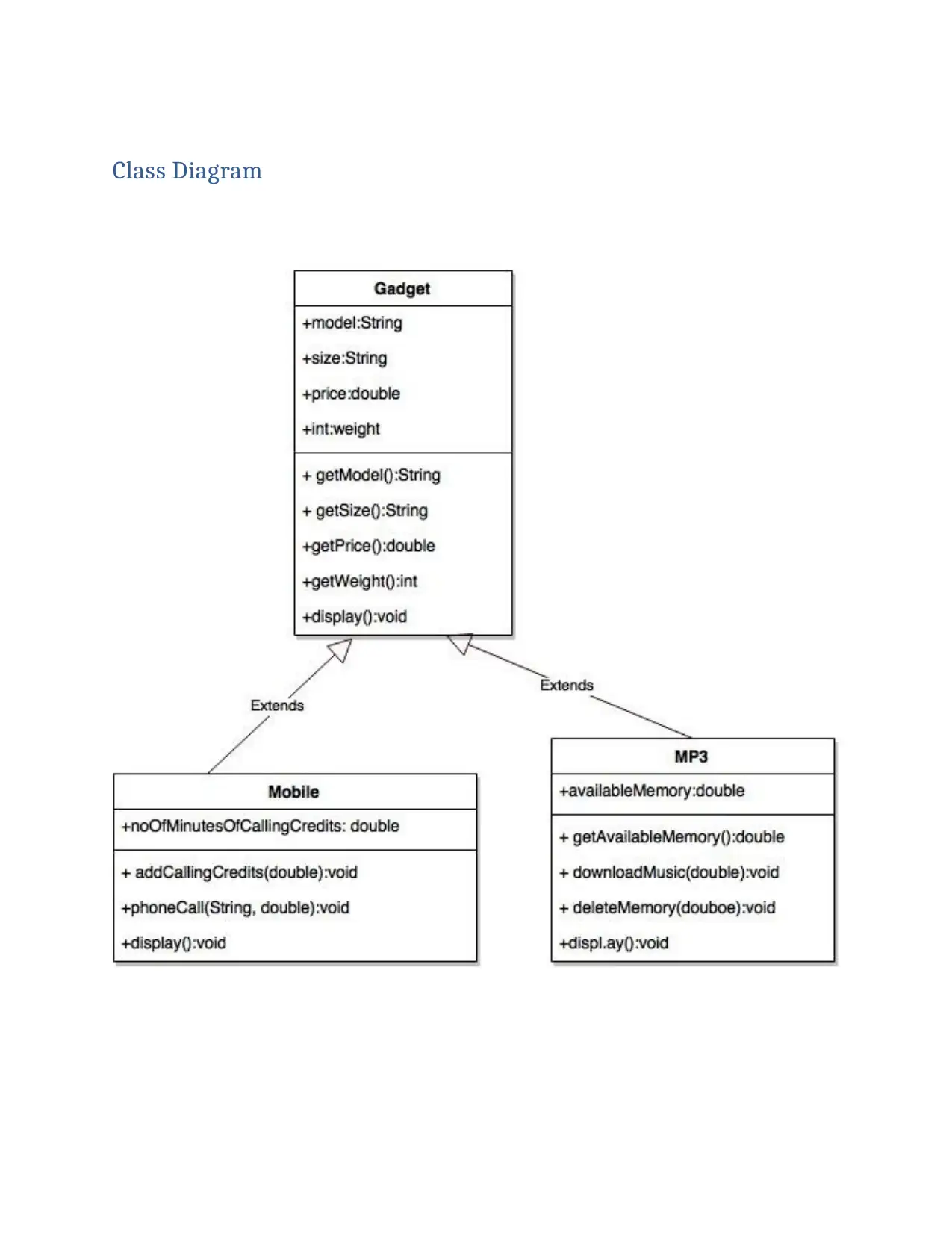
Class Diagram
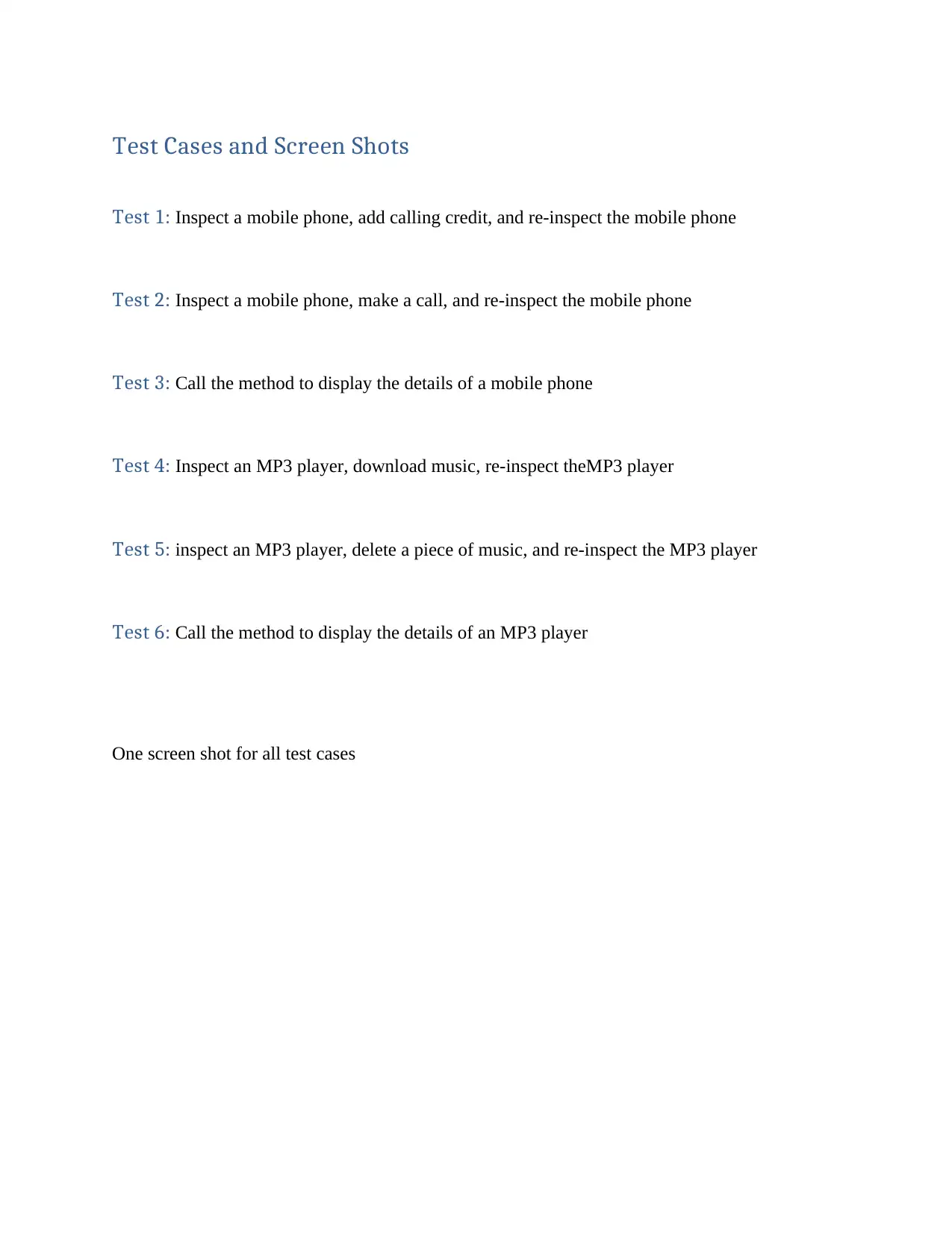
Test Cases and Screen Shots
Test 1: Inspect a mobile phone, add calling credit, and re-inspect the mobile phone
Test 2: Inspect a mobile phone, make a call, and re-inspect the mobile phone
Test 3: Call the method to display the details of a mobile phone
Test 4: Inspect an MP3 player, download music, re-inspect theMP3 player
Test 5: inspect an MP3 player, delete a piece of music, and re-inspect the MP3 player
Test 6: Call the method to display the details of an MP3 player
One screen shot for all test cases
Test 1: Inspect a mobile phone, add calling credit, and re-inspect the mobile phone
Test 2: Inspect a mobile phone, make a call, and re-inspect the mobile phone
Test 3: Call the method to display the details of a mobile phone
Test 4: Inspect an MP3 player, download music, re-inspect theMP3 player
Test 5: inspect an MP3 player, delete a piece of music, and re-inspect the MP3 player
Test 6: Call the method to display the details of an MP3 player
One screen shot for all test cases
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
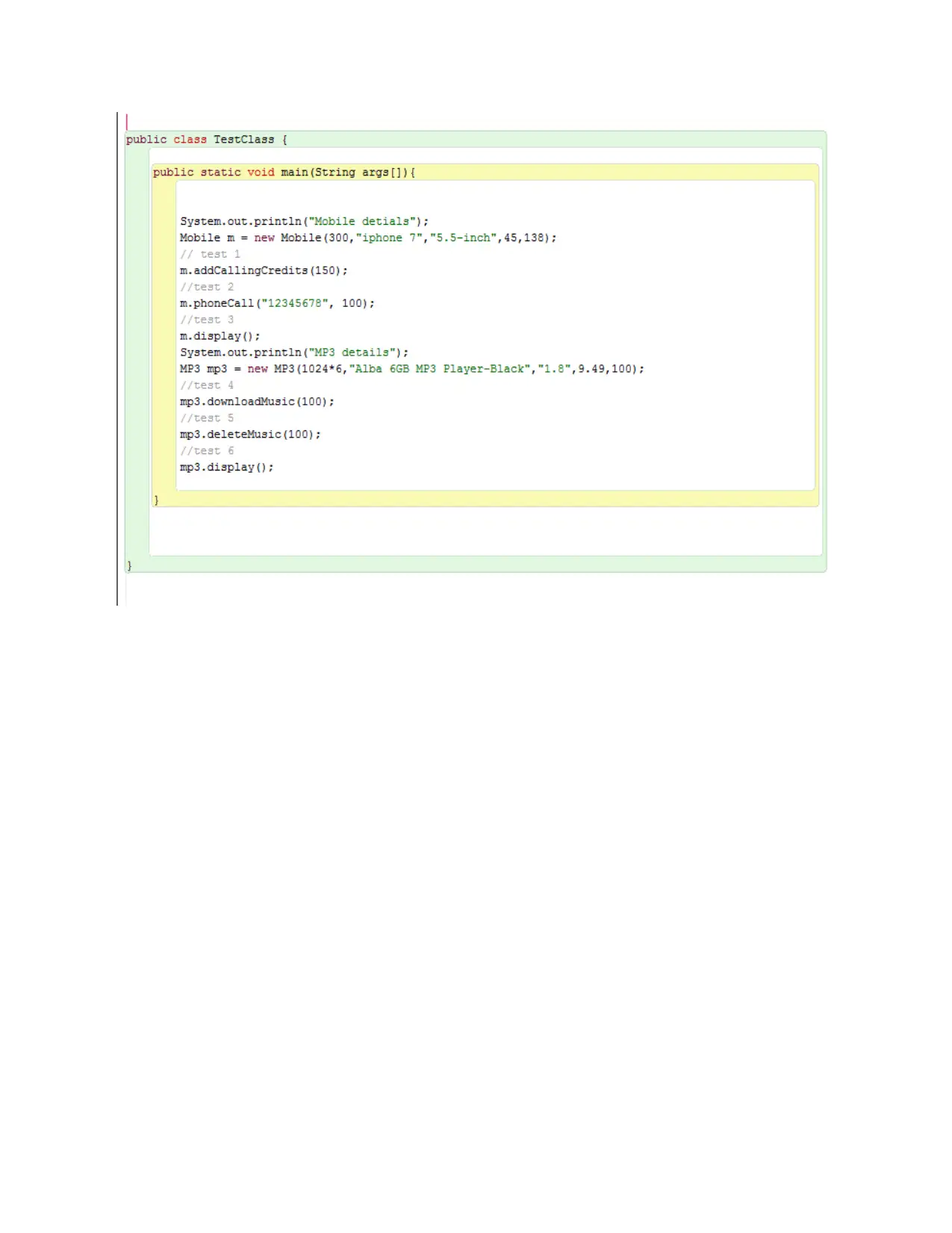
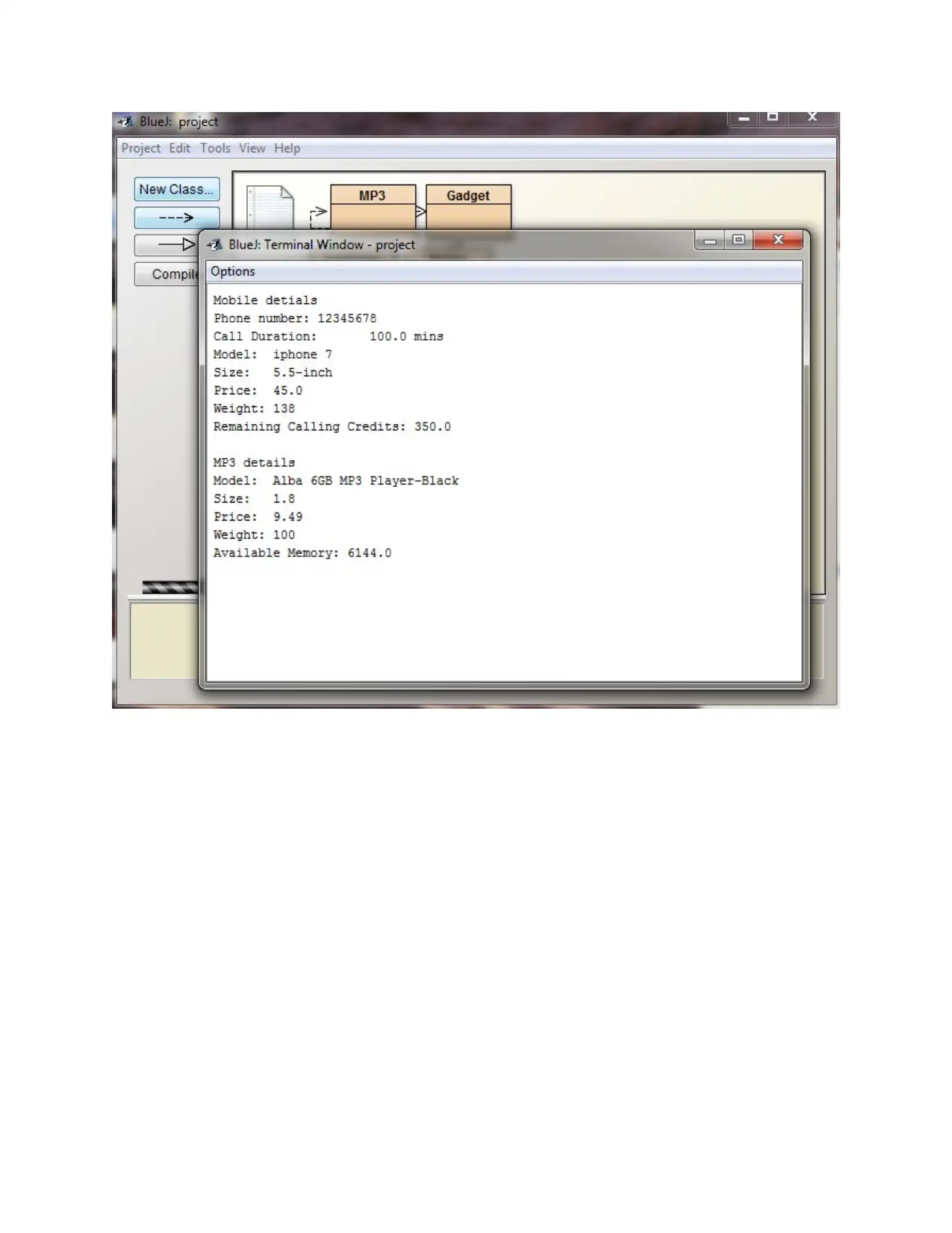
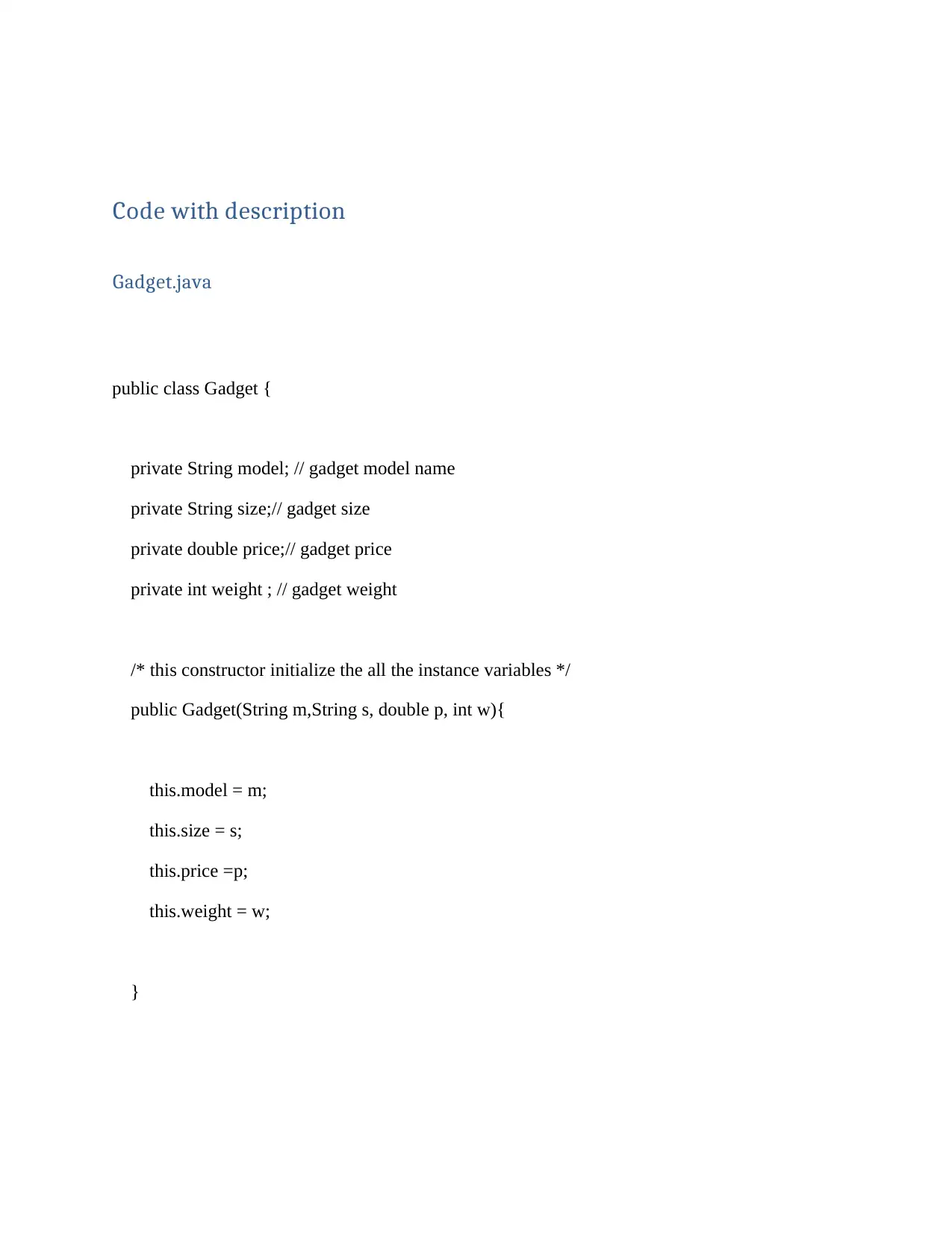
Code with description
Gadget.java
public class Gadget {
private String model; // gadget model name
private String size;// gadget size
private double price;// gadget price
private int weight ; // gadget weight
/* this constructor initialize the all the instance variables */
public Gadget(String m,String s, double p, int w){
this.model = m;
this.size = s;
this.price =p;
this.weight = w;
}
Gadget.java
public class Gadget {
private String model; // gadget model name
private String size;// gadget size
private double price;// gadget price
private int weight ; // gadget weight
/* this constructor initialize the all the instance variables */
public Gadget(String m,String s, double p, int w){
this.model = m;
this.size = s;
this.price =p;
this.weight = w;
}
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
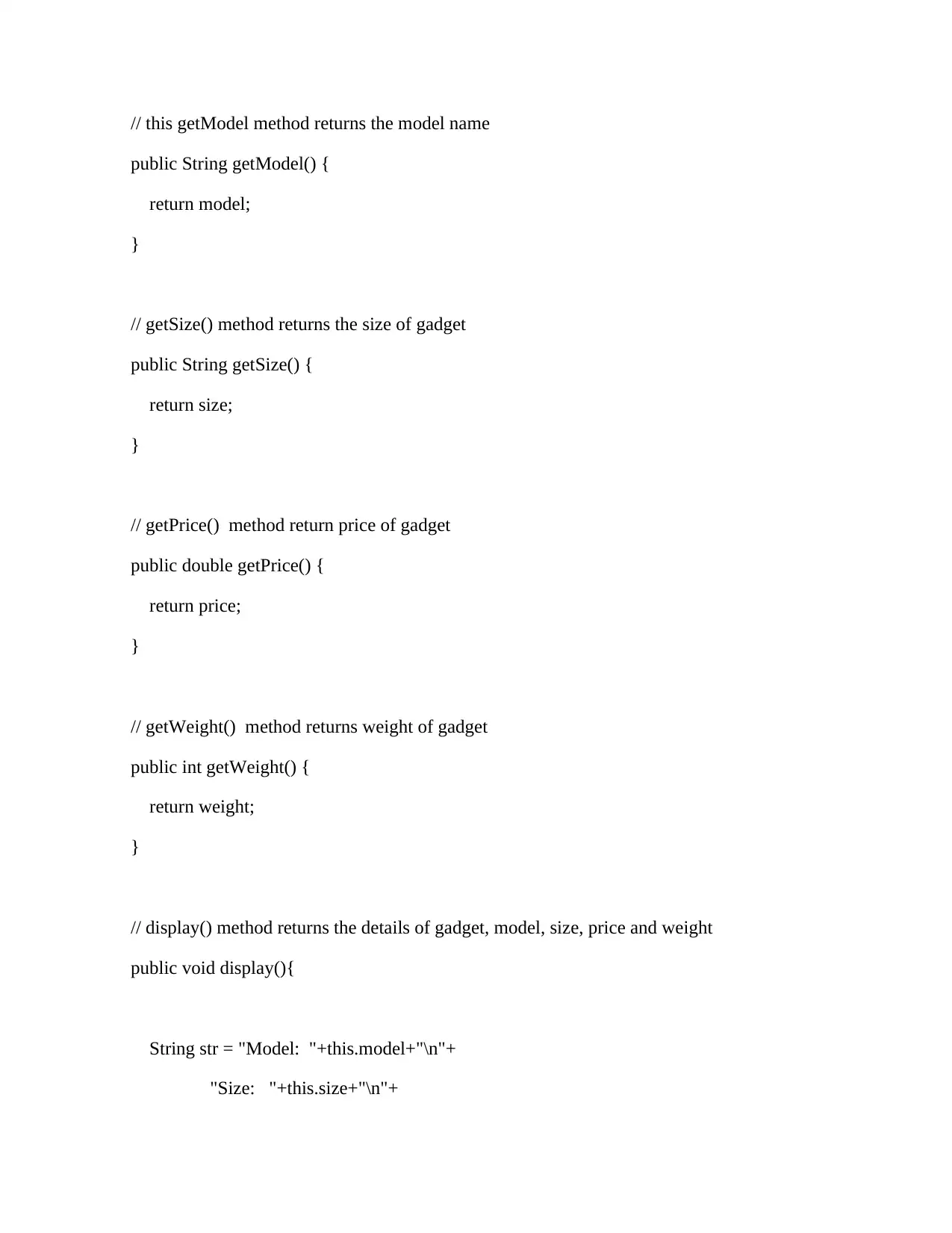
// this getModel method returns the model name
public String getModel() {
return model;
}
// getSize() method returns the size of gadget
public String getSize() {
return size;
}
// getPrice() method return price of gadget
public double getPrice() {
return price;
}
// getWeight() method returns weight of gadget
public int getWeight() {
return weight;
}
// display() method returns the details of gadget, model, size, price and weight
public void display(){
String str = "Model: "+this.model+"\n"+
"Size: "+this.size+"\n"+
public String getModel() {
return model;
}
// getSize() method returns the size of gadget
public String getSize() {
return size;
}
// getPrice() method return price of gadget
public double getPrice() {
return price;
}
// getWeight() method returns weight of gadget
public int getWeight() {
return weight;
}
// display() method returns the details of gadget, model, size, price and weight
public void display(){
String str = "Model: "+this.model+"\n"+
"Size: "+this.size+"\n"+
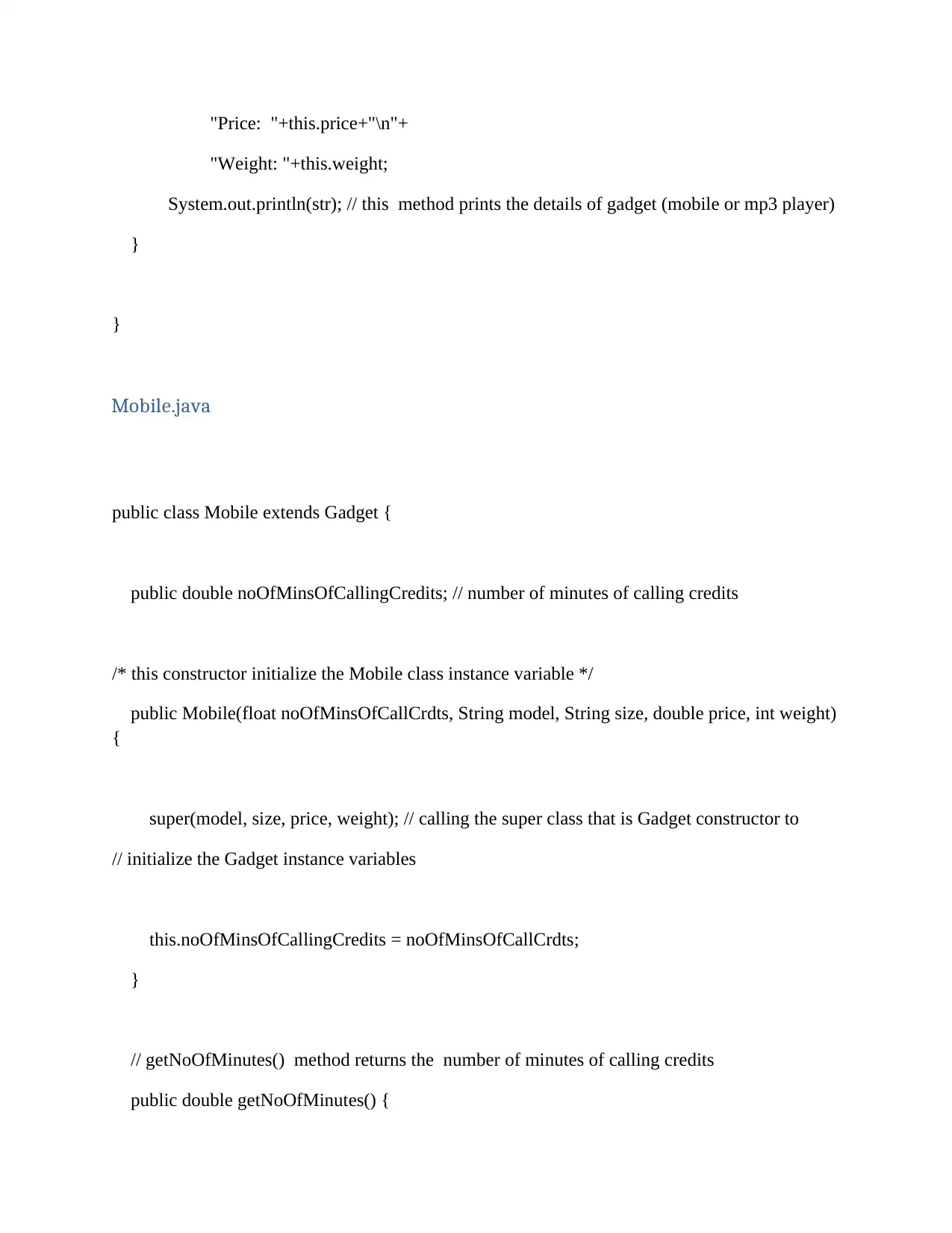
"Price: "+this.price+"\n"+
"Weight: "+this.weight;
System.out.println(str); // this method prints the details of gadget (mobile or mp3 player)
}
}
Mobile.java
public class Mobile extends Gadget {
public double noOfMinsOfCallingCredits; // number of minutes of calling credits
/* this constructor initialize the Mobile class instance variable */
public Mobile(float noOfMinsOfCallCrdts, String model, String size, double price, int weight)
{
super(model, size, price, weight); // calling the super class that is Gadget constructor to
// initialize the Gadget instance variables
this.noOfMinsOfCallingCredits = noOfMinsOfCallCrdts;
}
// getNoOfMinutes() method returns the number of minutes of calling credits
public double getNoOfMinutes() {
"Weight: "+this.weight;
System.out.println(str); // this method prints the details of gadget (mobile or mp3 player)
}
}
Mobile.java
public class Mobile extends Gadget {
public double noOfMinsOfCallingCredits; // number of minutes of calling credits
/* this constructor initialize the Mobile class instance variable */
public Mobile(float noOfMinsOfCallCrdts, String model, String size, double price, int weight)
{
super(model, size, price, weight); // calling the super class that is Gadget constructor to
// initialize the Gadget instance variables
this.noOfMinsOfCallingCredits = noOfMinsOfCallCrdts;
}
// getNoOfMinutes() method returns the number of minutes of calling credits
public double getNoOfMinutes() {
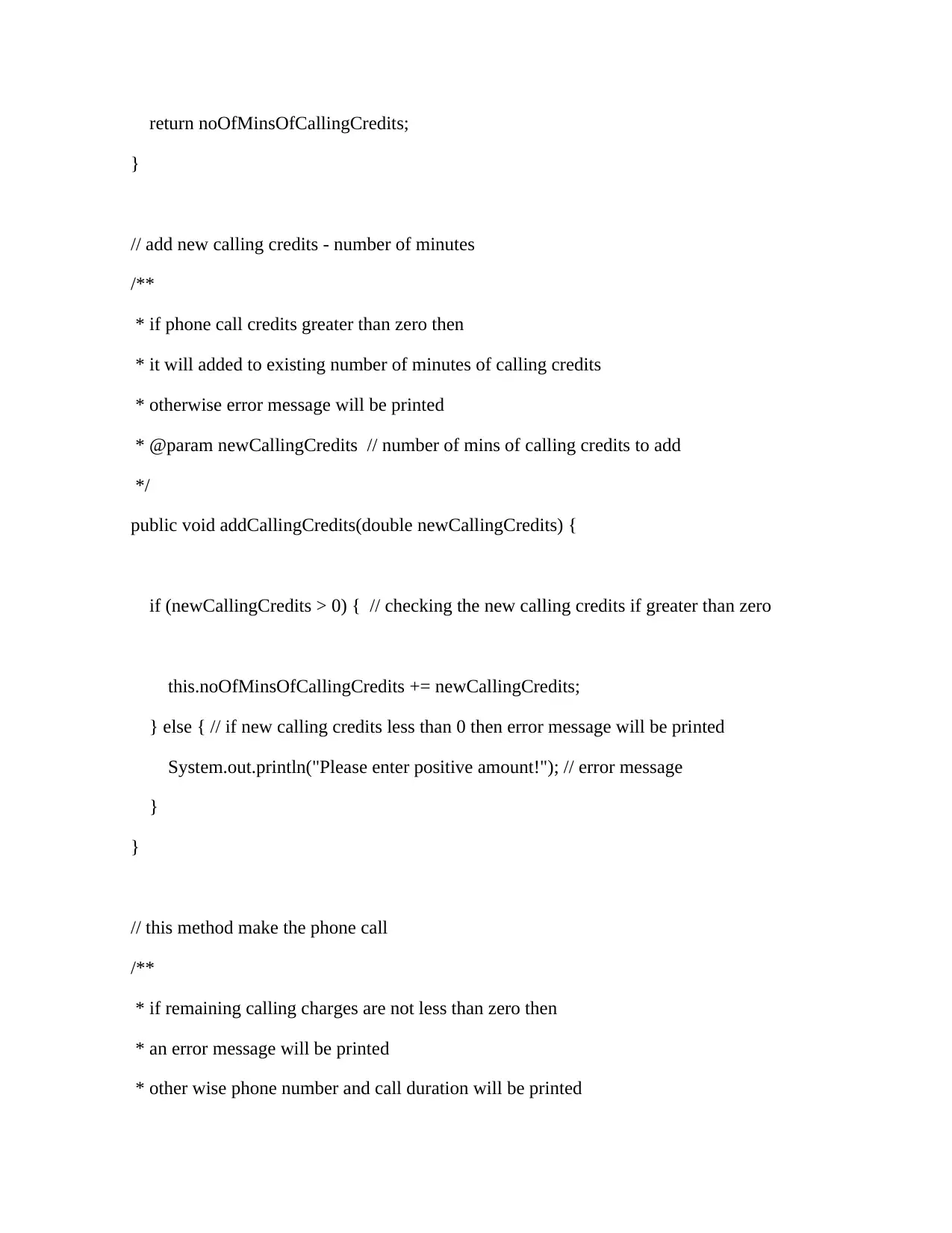
return noOfMinsOfCallingCredits;
}
// add new calling credits - number of minutes
/**
* if phone call credits greater than zero then
* it will added to existing number of minutes of calling credits
* otherwise error message will be printed
* @param newCallingCredits // number of mins of calling credits to add
*/
public void addCallingCredits(double newCallingCredits) {
if (newCallingCredits > 0) { // checking the new calling credits if greater than zero
this.noOfMinsOfCallingCredits += newCallingCredits;
} else { // if new calling credits less than 0 then error message will be printed
System.out.println("Please enter positive amount!"); // error message
}
}
// this method make the phone call
/**
* if remaining calling charges are not less than zero then
* an error message will be printed
* other wise phone number and call duration will be printed
}
// add new calling credits - number of minutes
/**
* if phone call credits greater than zero then
* it will added to existing number of minutes of calling credits
* otherwise error message will be printed
* @param newCallingCredits // number of mins of calling credits to add
*/
public void addCallingCredits(double newCallingCredits) {
if (newCallingCredits > 0) { // checking the new calling credits if greater than zero
this.noOfMinsOfCallingCredits += newCallingCredits;
} else { // if new calling credits less than 0 then error message will be printed
System.out.println("Please enter positive amount!"); // error message
}
}
// this method make the phone call
/**
* if remaining calling charges are not less than zero then
* an error message will be printed
* other wise phone number and call duration will be printed
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
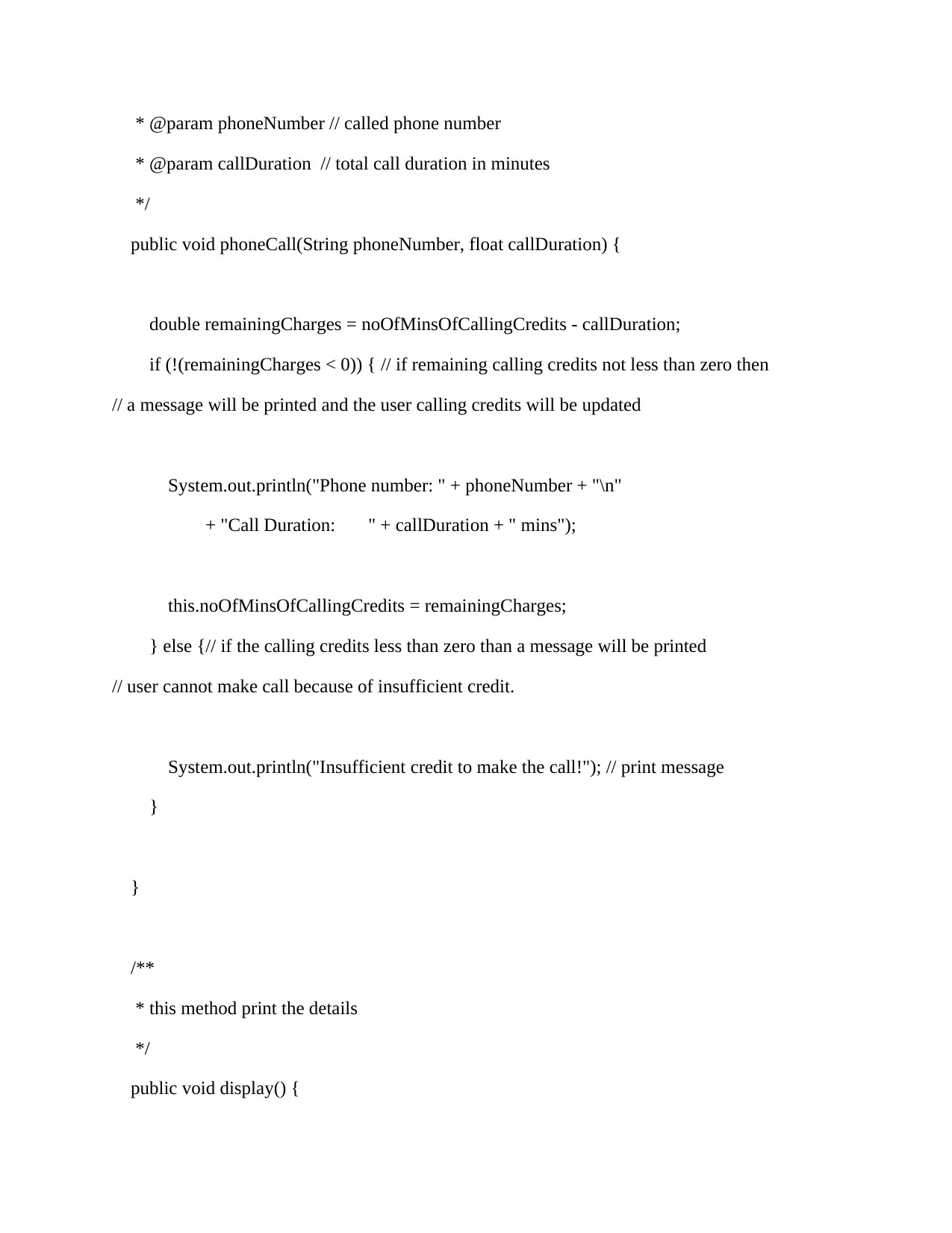
* @param phoneNumber // called phone number
* @param callDuration // total call duration in minutes
*/
public void phoneCall(String phoneNumber, float callDuration) {
double remainingCharges = noOfMinsOfCallingCredits - callDuration;
if (!(remainingCharges < 0)) { // if remaining calling credits not less than zero then
// a message will be printed and the user calling credits will be updated
System.out.println("Phone number: " + phoneNumber + "\n"
+ "Call Duration: " + callDuration + " mins");
this.noOfMinsOfCallingCredits = remainingCharges;
} else {// if the calling credits less than zero than a message will be printed
// user cannot make call because of insufficient credit.
System.out.println("Insufficient credit to make the call!"); // print message
}
}
/**
* this method print the details
*/
public void display() {
* @param callDuration // total call duration in minutes
*/
public void phoneCall(String phoneNumber, float callDuration) {
double remainingCharges = noOfMinsOfCallingCredits - callDuration;
if (!(remainingCharges < 0)) { // if remaining calling credits not less than zero then
// a message will be printed and the user calling credits will be updated
System.out.println("Phone number: " + phoneNumber + "\n"
+ "Call Duration: " + callDuration + " mins");
this.noOfMinsOfCallingCredits = remainingCharges;
} else {// if the calling credits less than zero than a message will be printed
// user cannot make call because of insufficient credit.
System.out.println("Insufficient credit to make the call!"); // print message
}
}
/**
* this method print the details
*/
public void display() {
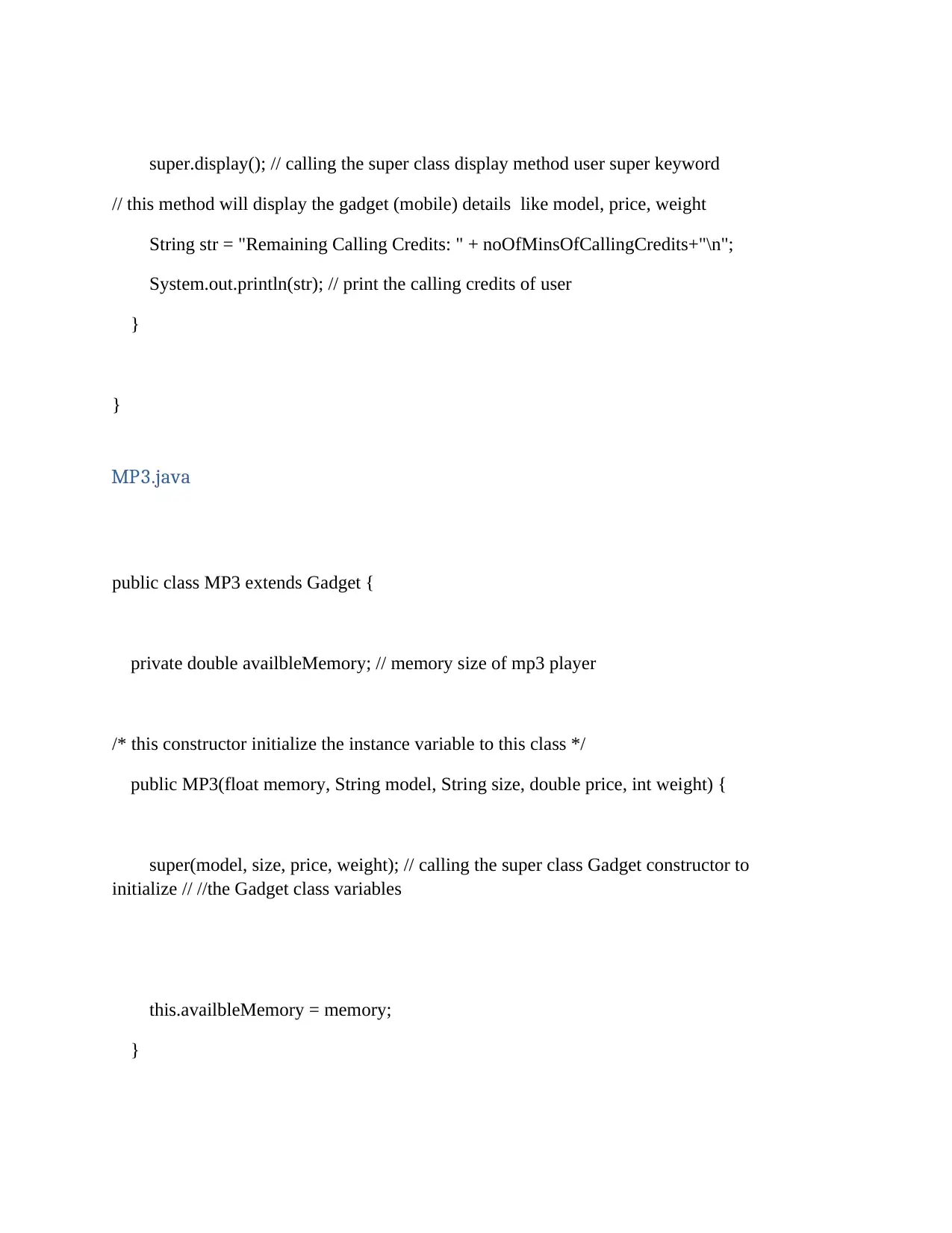
super.display(); // calling the super class display method user super keyword
// this method will display the gadget (mobile) details like model, price, weight
String str = "Remaining Calling Credits: " + noOfMinsOfCallingCredits+"\n";
System.out.println(str); // print the calling credits of user
}
}
MP3.java
public class MP3 extends Gadget {
private double availbleMemory; // memory size of mp3 player
/* this constructor initialize the instance variable to this class */
public MP3(float memory, String model, String size, double price, int weight) {
super(model, size, price, weight); // calling the super class Gadget constructor to
initialize // //the Gadget class variables
this.availbleMemory = memory;
}
// this method will display the gadget (mobile) details like model, price, weight
String str = "Remaining Calling Credits: " + noOfMinsOfCallingCredits+"\n";
System.out.println(str); // print the calling credits of user
}
}
MP3.java
public class MP3 extends Gadget {
private double availbleMemory; // memory size of mp3 player
/* this constructor initialize the instance variable to this class */
public MP3(float memory, String model, String size, double price, int weight) {
super(model, size, price, weight); // calling the super class Gadget constructor to
initialize // //the Gadget class variables
this.availbleMemory = memory;
}
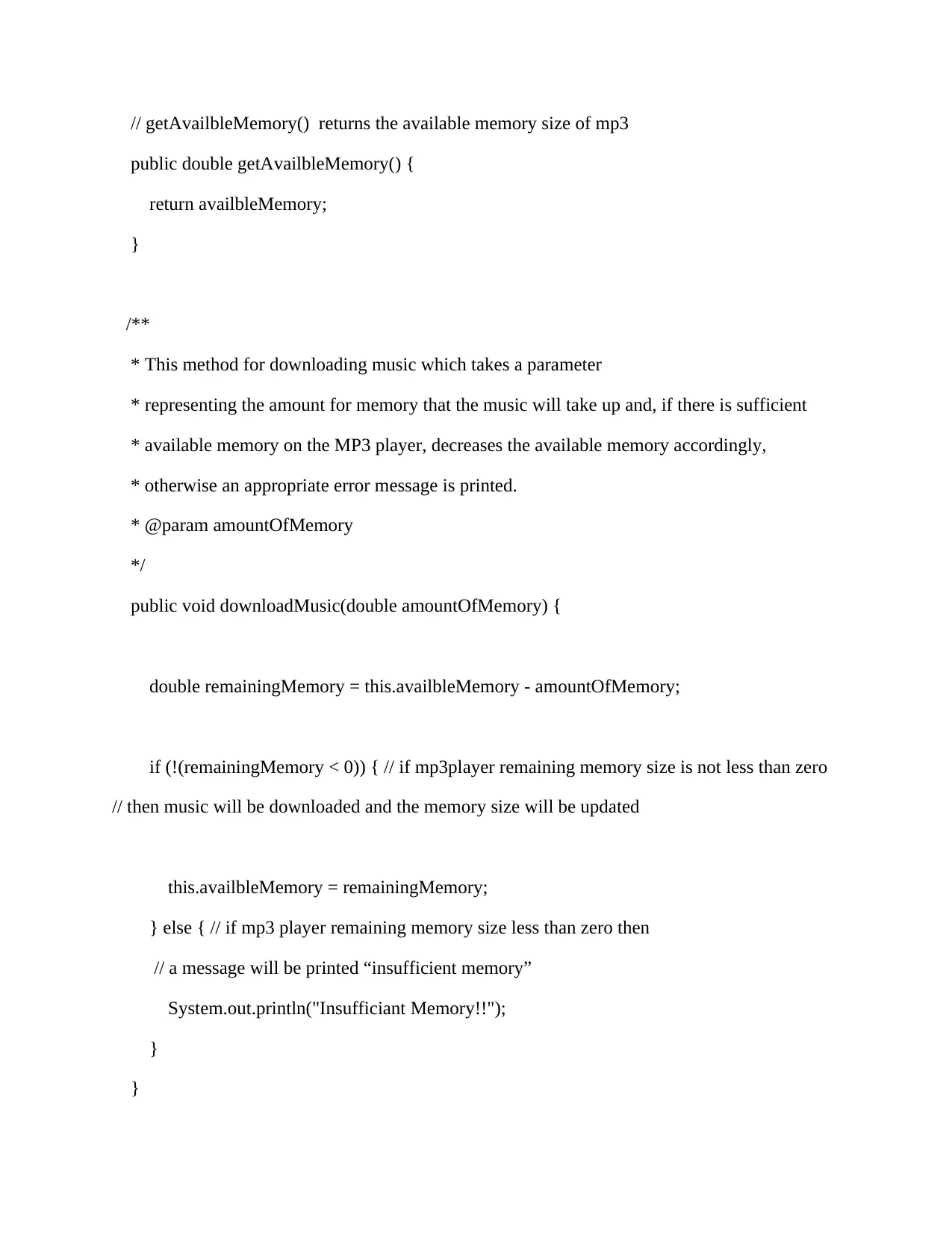
// getAvailbleMemory() returns the available memory size of mp3
public double getAvailbleMemory() {
return availbleMemory;
}
/**
* This method for downloading music which takes a parameter
* representing the amount for memory that the music will take up and, if there is sufficient
* available memory on the MP3 player, decreases the available memory accordingly,
* otherwise an appropriate error message is printed.
* @param amountOfMemory
*/
public void downloadMusic(double amountOfMemory) {
double remainingMemory = this.availbleMemory - amountOfMemory;
if (!(remainingMemory < 0)) { // if mp3player remaining memory size is not less than zero
// then music will be downloaded and the memory size will be updated
this.availbleMemory = remainingMemory;
} else { // if mp3 player remaining memory size less than zero then
// a message will be printed “insufficient memory”
System.out.println("Insufficiant Memory!!");
}
}
public double getAvailbleMemory() {
return availbleMemory;
}
/**
* This method for downloading music which takes a parameter
* representing the amount for memory that the music will take up and, if there is sufficient
* available memory on the MP3 player, decreases the available memory accordingly,
* otherwise an appropriate error message is printed.
* @param amountOfMemory
*/
public void downloadMusic(double amountOfMemory) {
double remainingMemory = this.availbleMemory - amountOfMemory;
if (!(remainingMemory < 0)) { // if mp3player remaining memory size is not less than zero
// then music will be downloaded and the memory size will be updated
this.availbleMemory = remainingMemory;
} else { // if mp3 player remaining memory size less than zero then
// a message will be printed “insufficient memory”
System.out.println("Insufficiant Memory!!");
}
}
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
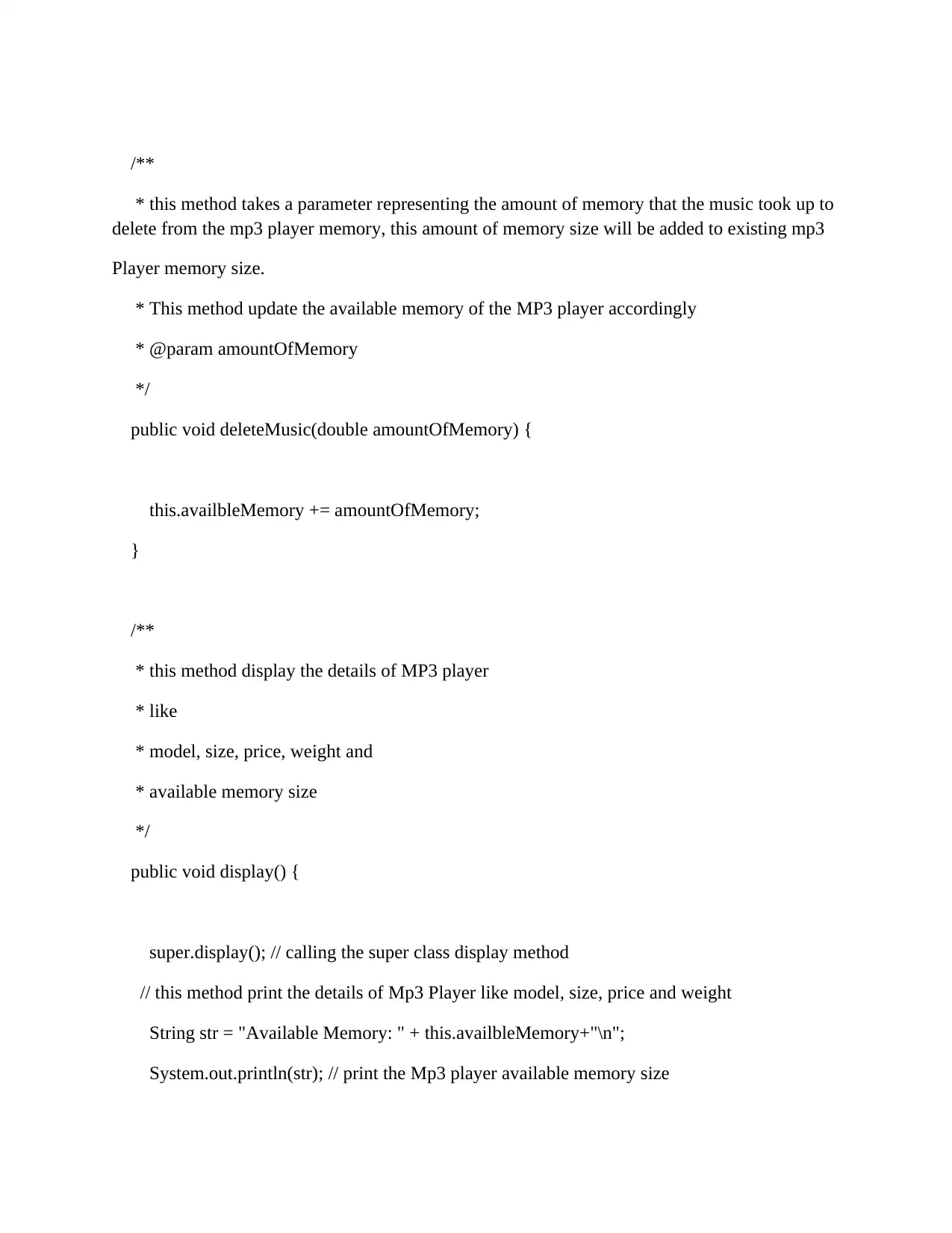
/**
* this method takes a parameter representing the amount of memory that the music took up to
delete from the mp3 player memory, this amount of memory size will be added to existing mp3
Player memory size.
* This method update the available memory of the MP3 player accordingly
* @param amountOfMemory
*/
public void deleteMusic(double amountOfMemory) {
this.availbleMemory += amountOfMemory;
}
/**
* this method display the details of MP3 player
* like
* model, size, price, weight and
* available memory size
*/
public void display() {
super.display(); // calling the super class display method
// this method print the details of Mp3 Player like model, size, price and weight
String str = "Available Memory: " + this.availbleMemory+"\n";
System.out.println(str); // print the Mp3 player available memory size
* this method takes a parameter representing the amount of memory that the music took up to
delete from the mp3 player memory, this amount of memory size will be added to existing mp3
Player memory size.
* This method update the available memory of the MP3 player accordingly
* @param amountOfMemory
*/
public void deleteMusic(double amountOfMemory) {
this.availbleMemory += amountOfMemory;
}
/**
* this method display the details of MP3 player
* like
* model, size, price, weight and
* available memory size
*/
public void display() {
super.display(); // calling the super class display method
// this method print the details of Mp3 Player like model, size, price and weight
String str = "Available Memory: " + this.availbleMemory+"\n";
System.out.println(str); // print the Mp3 player available memory size
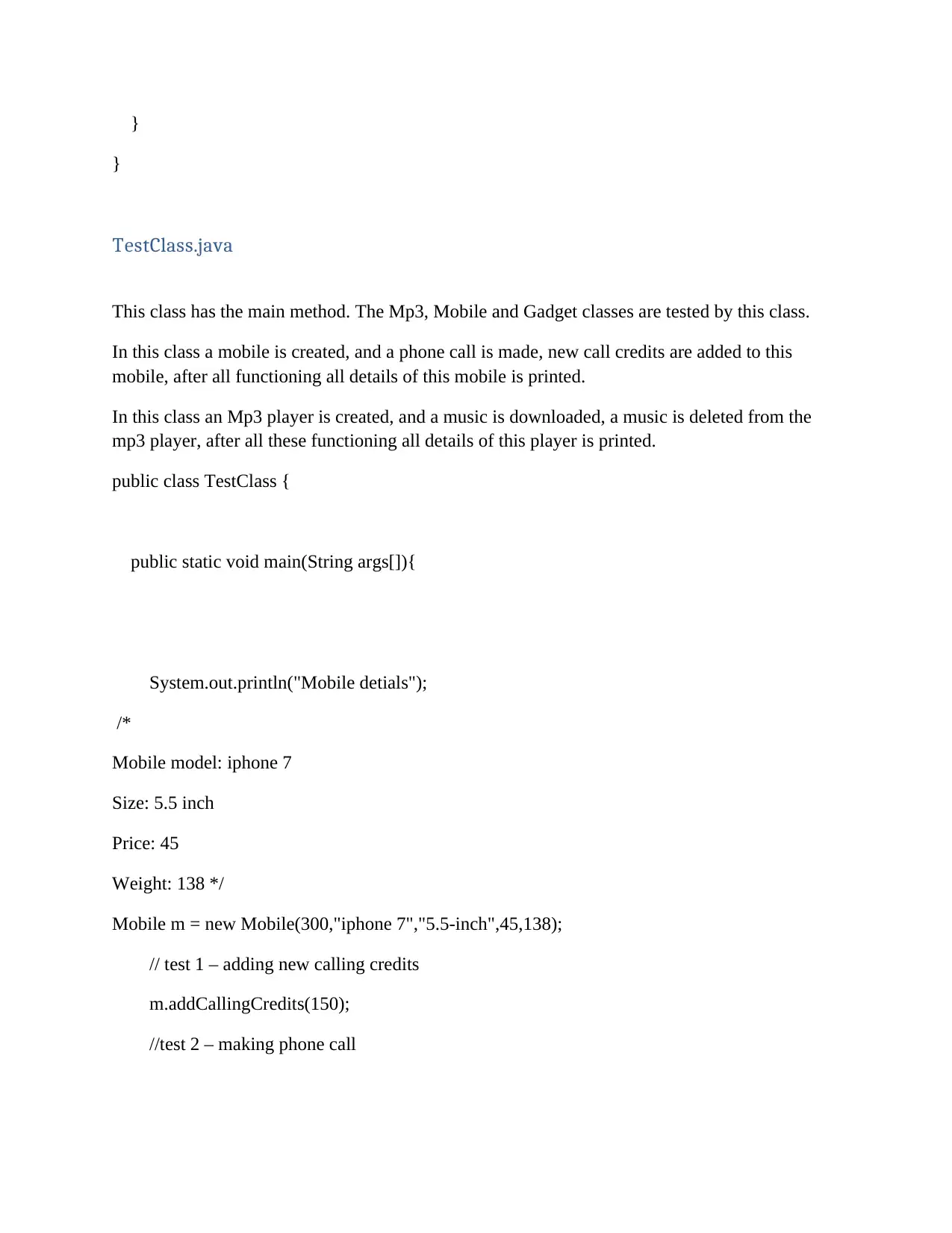
}
}
TestClass.java
This class has the main method. The Mp3, Mobile and Gadget classes are tested by this class.
In this class a mobile is created, and a phone call is made, new call credits are added to this
mobile, after all functioning all details of this mobile is printed.
In this class an Mp3 player is created, and a music is downloaded, a music is deleted from the
mp3 player, after all these functioning all details of this player is printed.
public class TestClass {
public static void main(String args[]){
System.out.println("Mobile detials");
/*
Mobile model: iphone 7
Size: 5.5 inch
Price: 45
Weight: 138 */
Mobile m = new Mobile(300,"iphone 7","5.5-inch",45,138);
// test 1 – adding new calling credits
m.addCallingCredits(150);
//test 2 – making phone call
}
TestClass.java
This class has the main method. The Mp3, Mobile and Gadget classes are tested by this class.
In this class a mobile is created, and a phone call is made, new call credits are added to this
mobile, after all functioning all details of this mobile is printed.
In this class an Mp3 player is created, and a music is downloaded, a music is deleted from the
mp3 player, after all these functioning all details of this player is printed.
public class TestClass {
public static void main(String args[]){
System.out.println("Mobile detials");
/*
Mobile model: iphone 7
Size: 5.5 inch
Price: 45
Weight: 138 */
Mobile m = new Mobile(300,"iphone 7","5.5-inch",45,138);
// test 1 – adding new calling credits
m.addCallingCredits(150);
//test 2 – making phone call
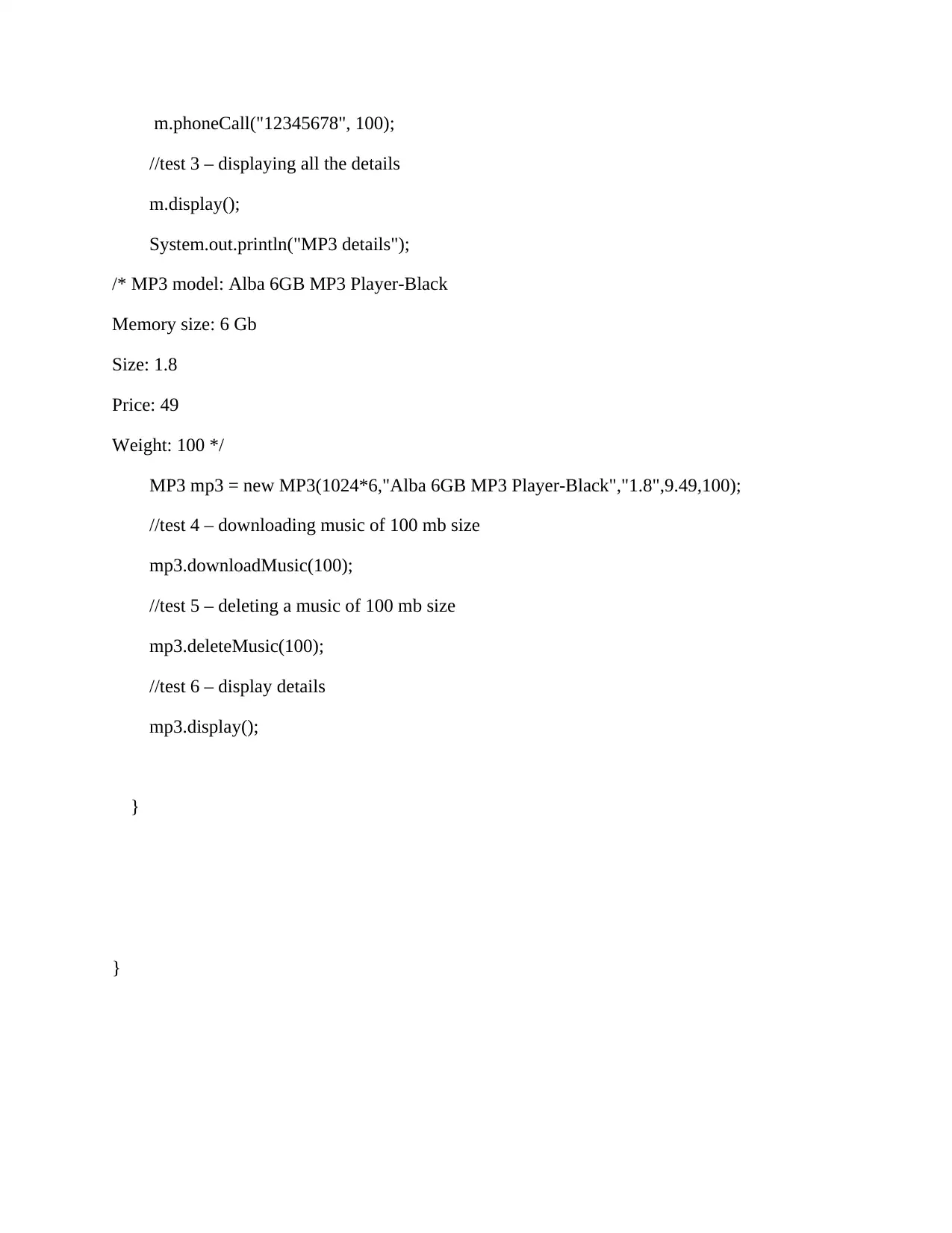
m.phoneCall("12345678", 100);
//test 3 – displaying all the details
m.display();
System.out.println("MP3 details");
/* MP3 model: Alba 6GB MP3 Player-Black
Memory size: 6 Gb
Size: 1.8
Price: 49
Weight: 100 */
MP3 mp3 = new MP3(1024*6,"Alba 6GB MP3 Player-Black","1.8",9.49,100);
//test 4 – downloading music of 100 mb size
mp3.downloadMusic(100);
//test 5 – deleting a music of 100 mb size
mp3.deleteMusic(100);
//test 6 – display details
mp3.display();
}
}
//test 3 – displaying all the details
m.display();
System.out.println("MP3 details");
/* MP3 model: Alba 6GB MP3 Player-Black
Memory size: 6 Gb
Size: 1.8
Price: 49
Weight: 100 */
MP3 mp3 = new MP3(1024*6,"Alba 6GB MP3 Player-Black","1.8",9.49,100);
//test 4 – downloading music of 100 mb size
mp3.downloadMusic(100);
//test 5 – deleting a music of 100 mb size
mp3.deleteMusic(100);
//test 6 – display details
mp3.display();
}
}
1 out of 16
Related Documents

Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.