Java Project: Analyzing the NYC Flights Dataset from CSV
VerifiedAdded on  2023/06/10
|26
|1439
|140
Practical Assignment
AI Summary
This assignment provides a Java-based solution for analyzing the NYC flights dataset, which is converted into a CSV format for easier processing. The code includes steps for importing the CSV file, determining the dataset's dimensions, sorting the data into tables, counting columns, and counting flights originating from EWR, JFK, and LGA. It also demonstrates how to view portions of the dataset, calculate mean, median, maximum, and minimum values. The solution includes Java code snippets for reading the CSV file, creating a flight scheduler, and reallocating flights based on certain criteria. The assignment makes use of data structures like HashMaps and HashSets to manage airports and flights. The provided code also includes screenshots that show the implementation of the java codes. Desklib offers this solution along with other solved assignments and past papers to aid students in their studies.
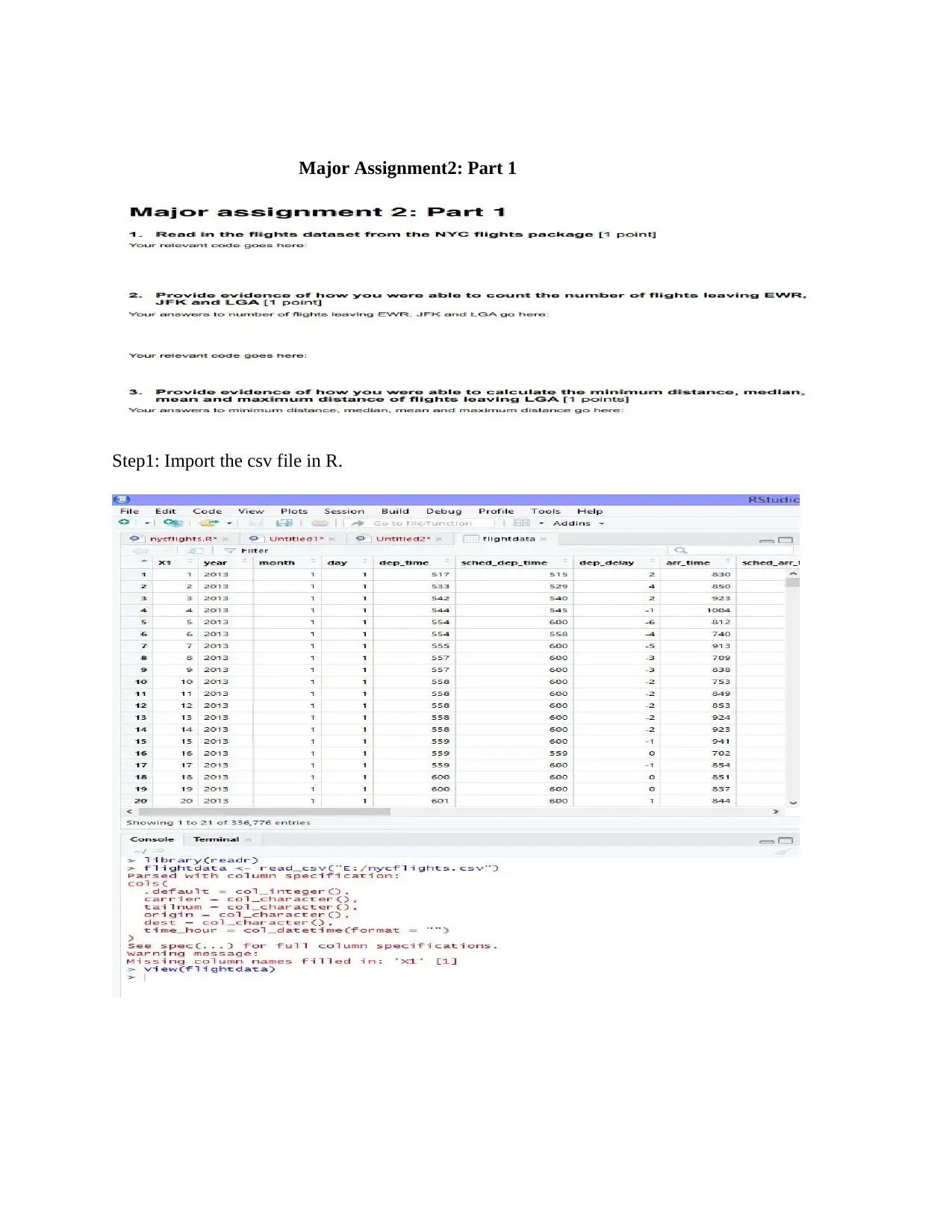
Major Assignment2: Part 1
Step1: Import the csv file in R.
Step1: Import the csv file in R.
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
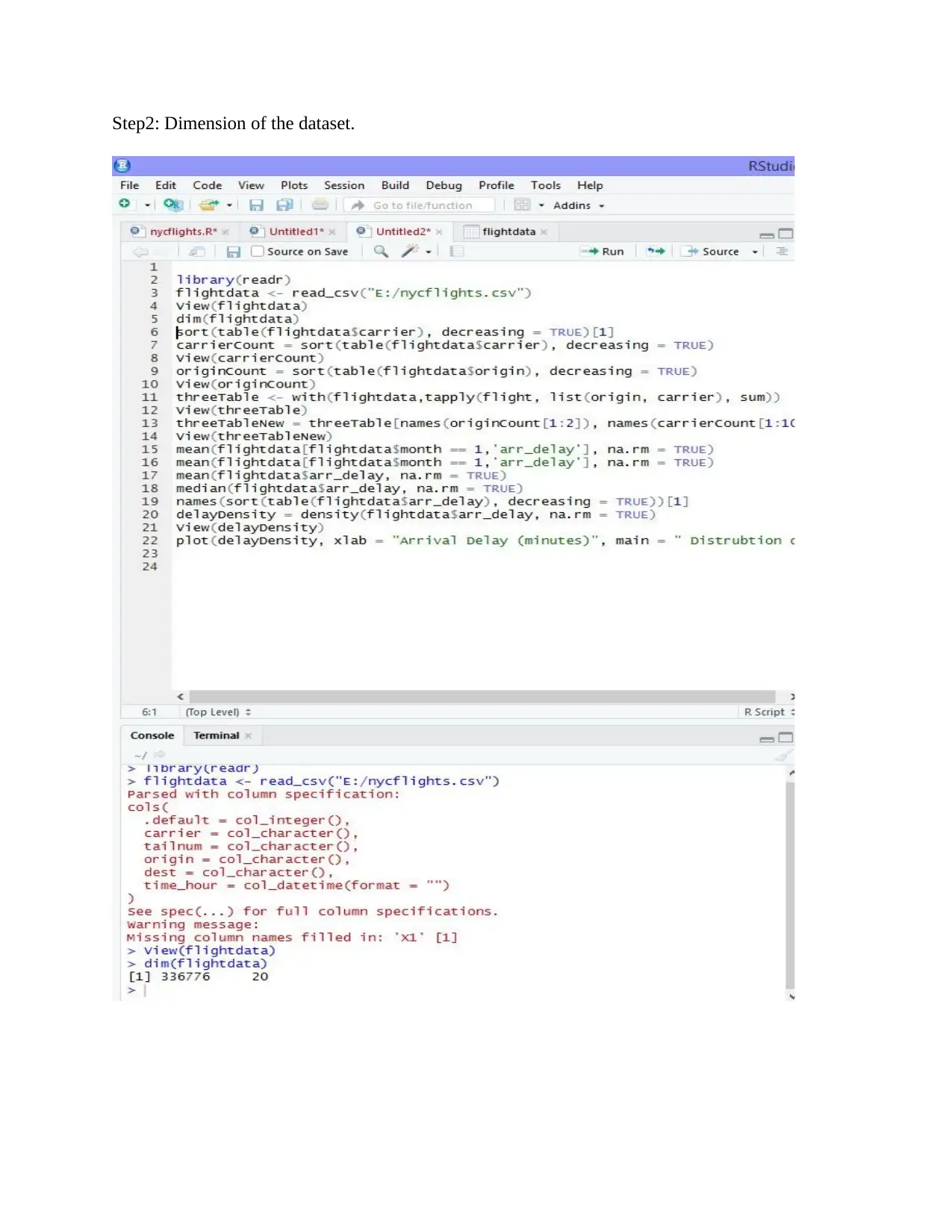
Step2: Dimension of the dataset.
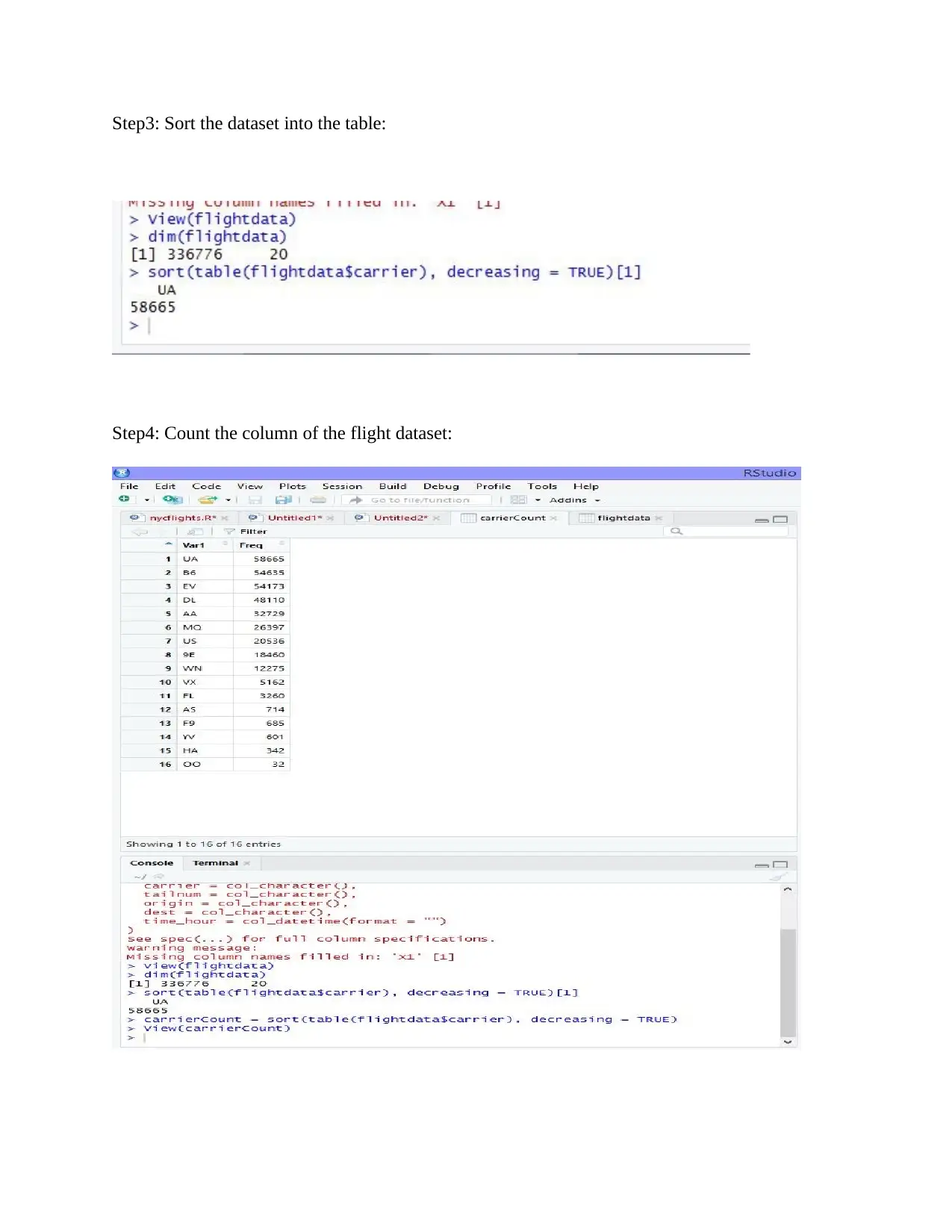
Step3: Sort the dataset into the table:
Step4: Count the column of the flight dataset:
Step4: Count the column of the flight dataset:
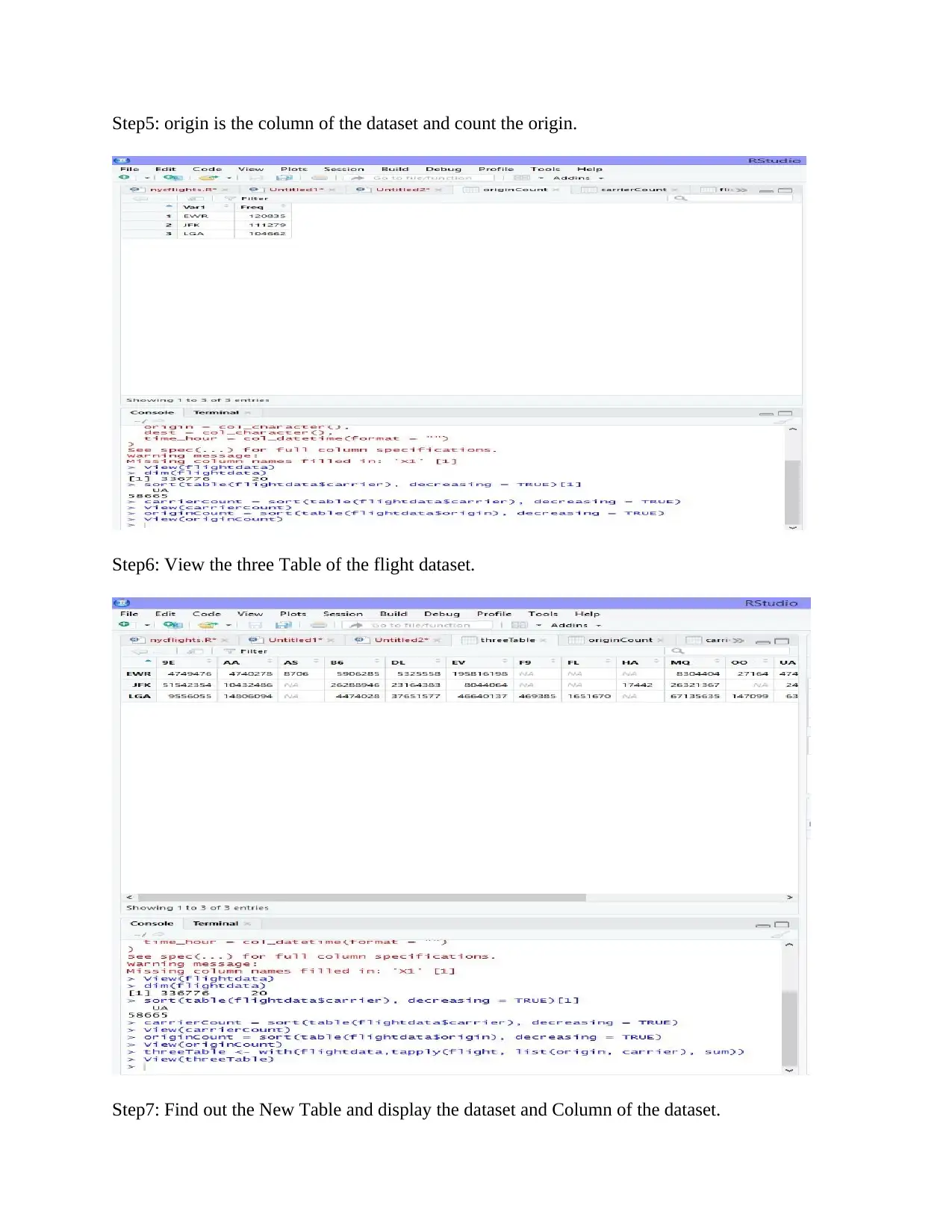
Step5: origin is the column of the dataset and count the origin.
Step6: View the three Table of the flight dataset.
Step7: Find out the New Table and display the dataset and Column of the dataset.
Step6: View the three Table of the flight dataset.
Step7: Find out the New Table and display the dataset and Column of the dataset.
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
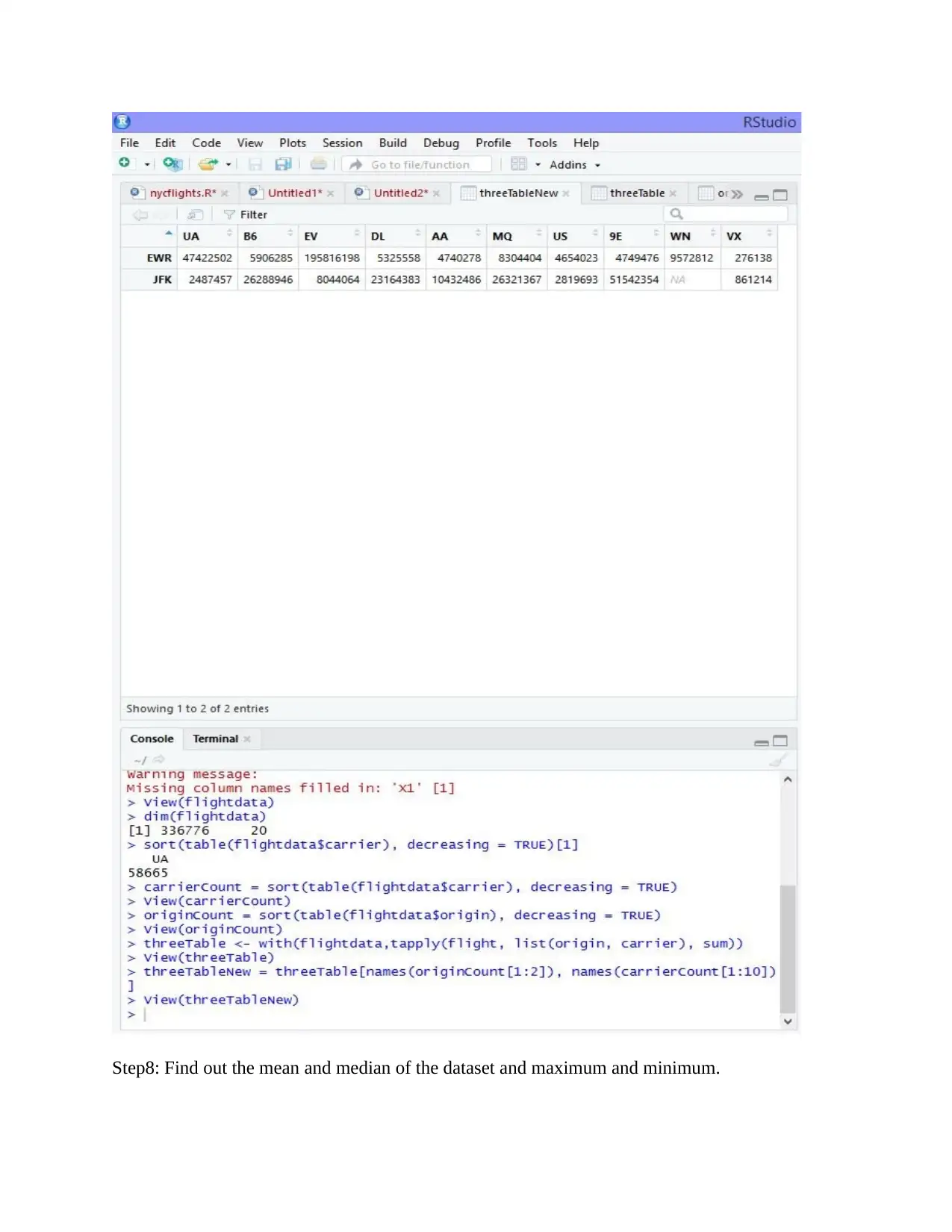
Step8: Find out the mean and median of the dataset and maximum and minimum.
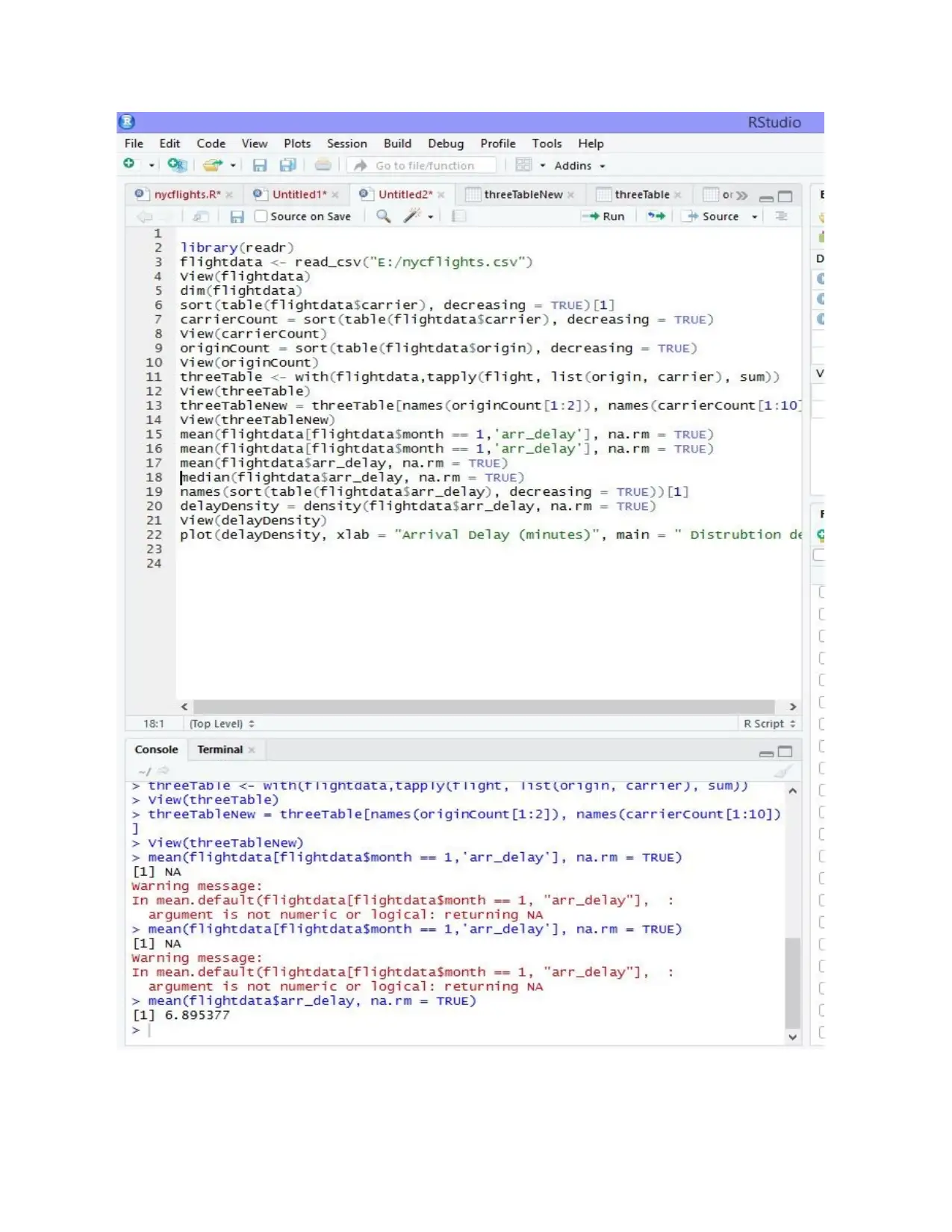
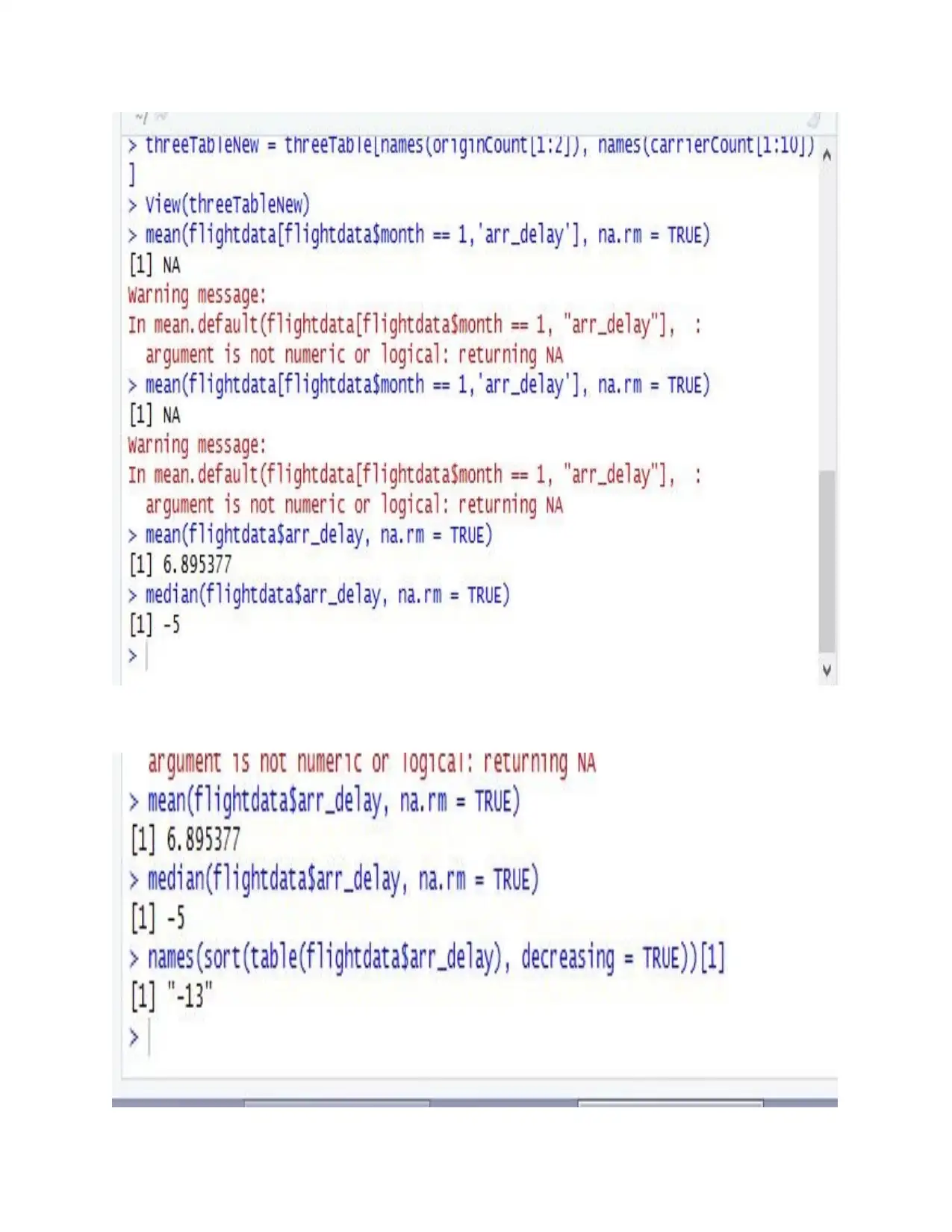
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
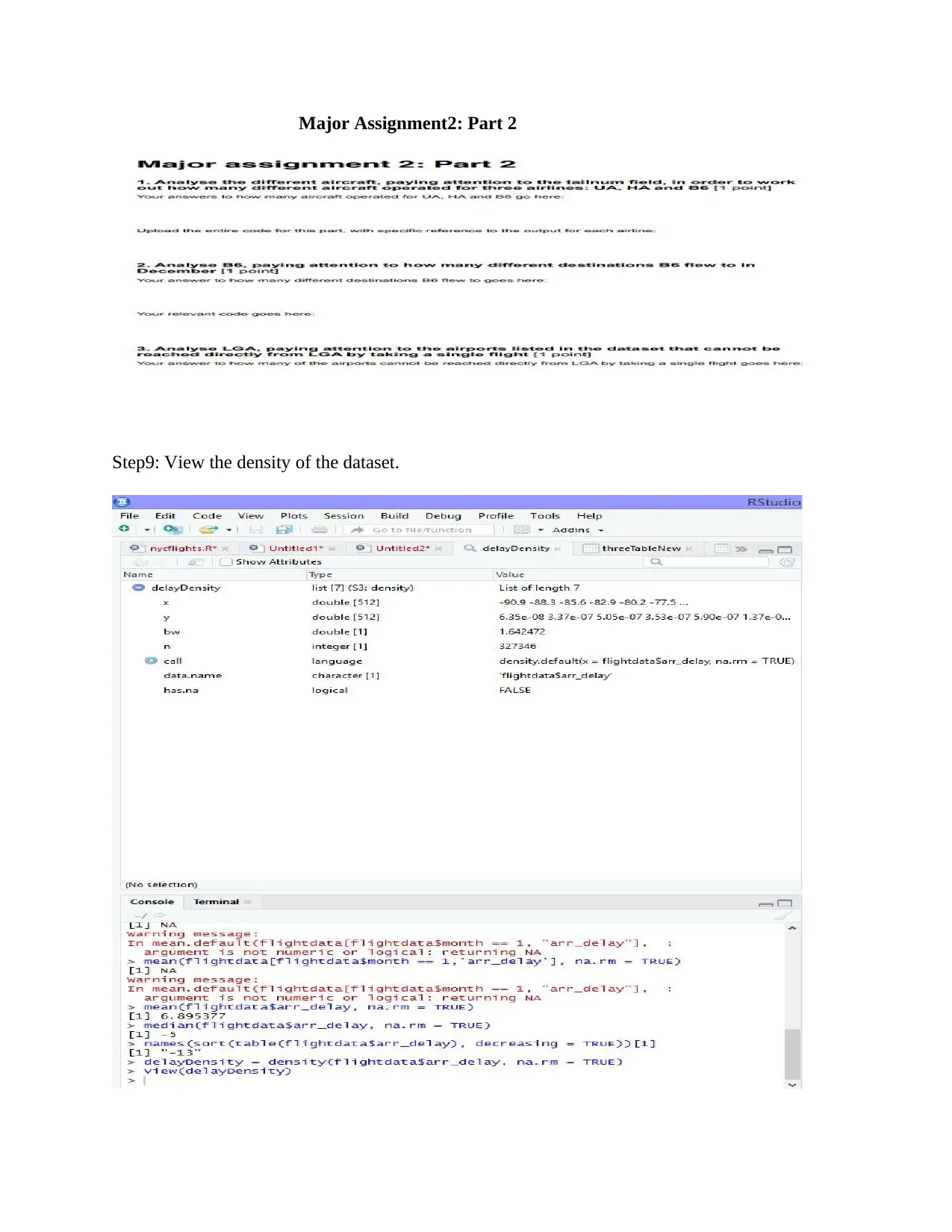
Major Assignment2: Part 2
Step9: View the density of the dataset.
Step9: View the density of the dataset.
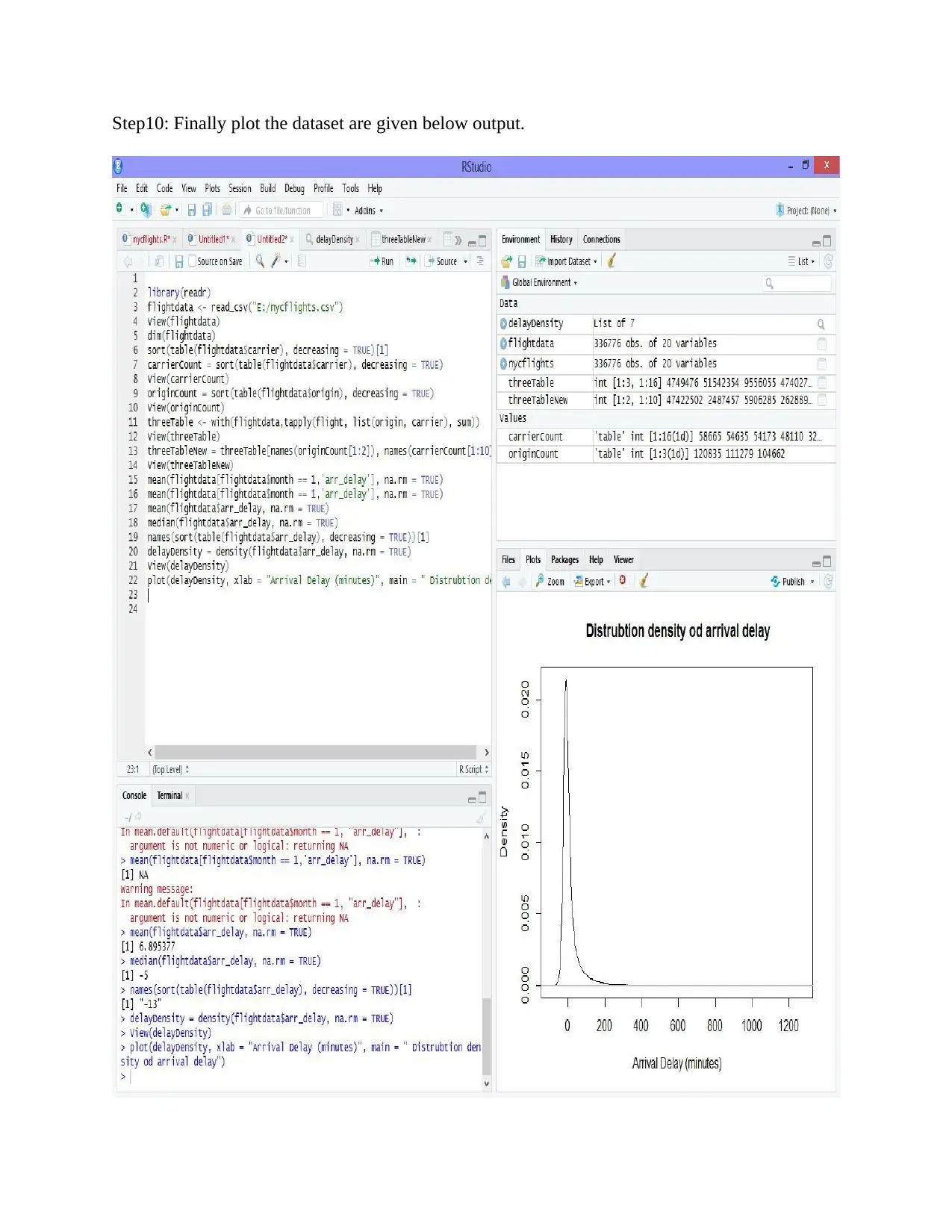
Step10: Finally plot the dataset are given below output.
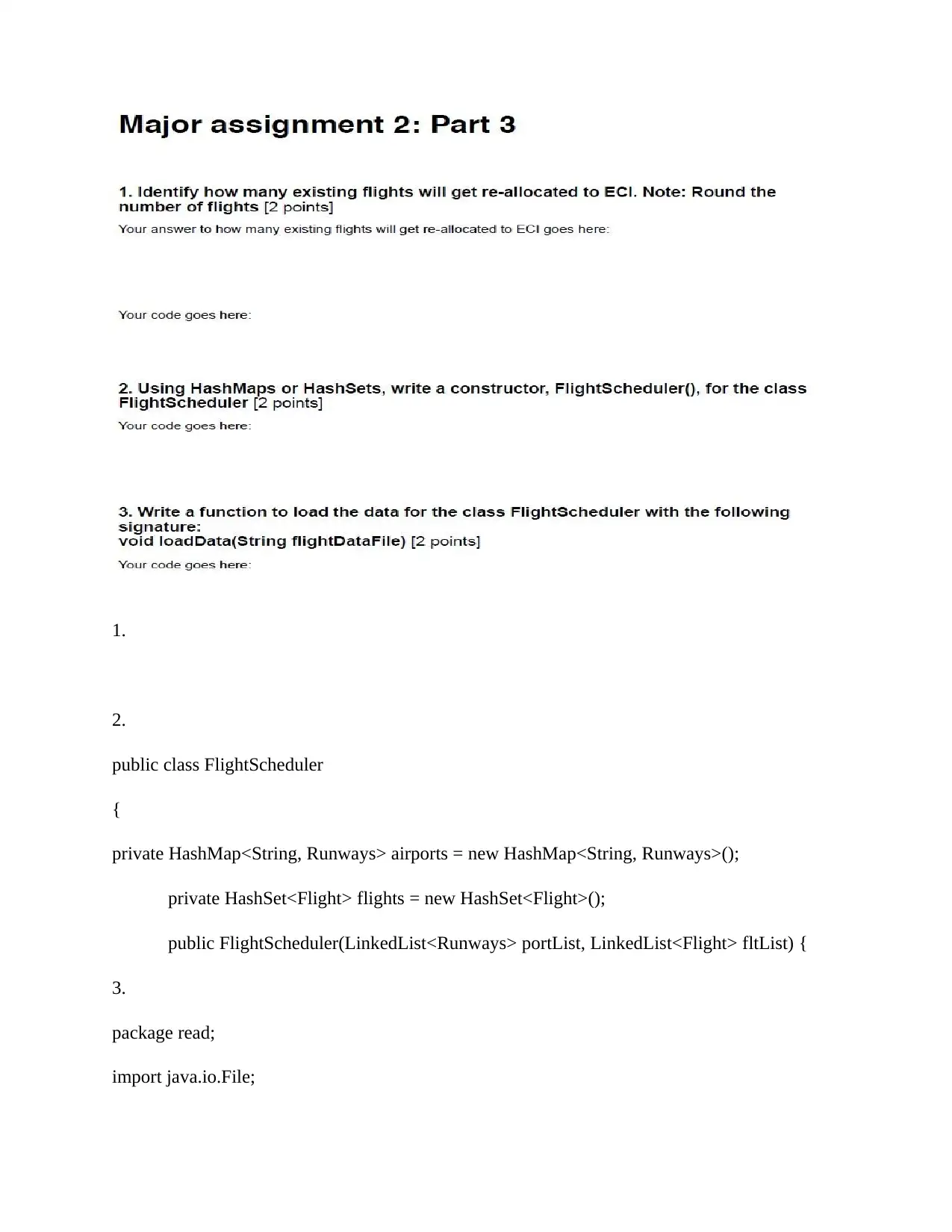
1.
2.
public class FlightScheduler
{
private HashMap<String, Runways> airports = new HashMap<String, Runways>();
private HashSet<Flight> flights = new HashSet<Flight>();
public FlightScheduler(LinkedList<Runways> portList, LinkedList<Flight> fltList) {
3.
package read;
import java.io.File;
2.
public class FlightScheduler
{
private HashMap<String, Runways> airports = new HashMap<String, Runways>();
private HashSet<Flight> flights = new HashSet<Flight>();
public FlightScheduler(LinkedList<Runways> portList, LinkedList<Flight> fltList) {
3.
package read;
import java.io.File;
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
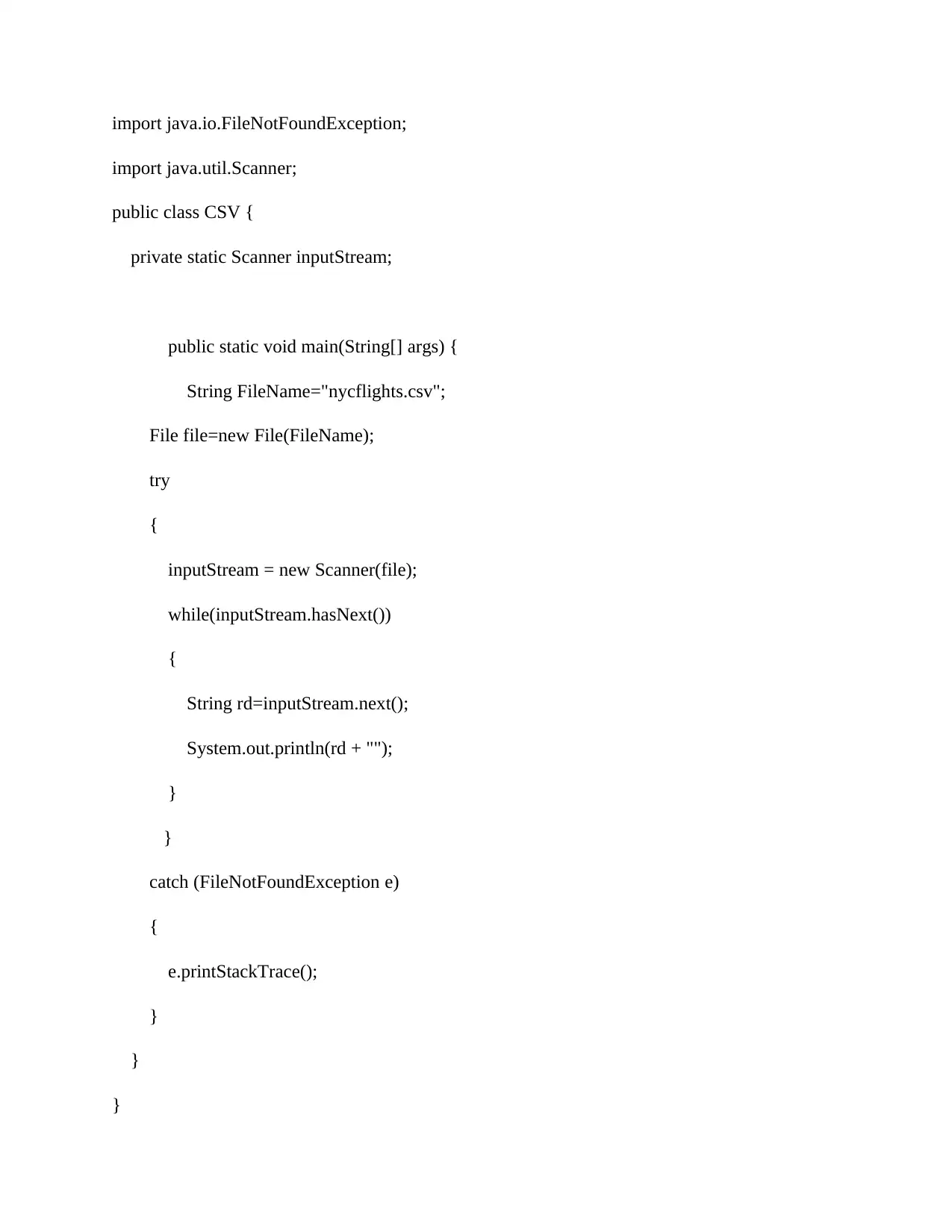
import java.io.FileNotFoundException;
import java.util.Scanner;
public class CSV {
private static Scanner inputStream;
public static void main(String[] args) {
String FileName="nycflights.csv";
File file=new File(FileName);
try
{
inputStream = new Scanner(file);
while(inputStream.hasNext())
{
String rd=inputStream.next();
System.out.println(rd + "");
}
}
catch (FileNotFoundException e)
{
e.printStackTrace();
}
}
}
import java.util.Scanner;
public class CSV {
private static Scanner inputStream;
public static void main(String[] args) {
String FileName="nycflights.csv";
File file=new File(FileName);
try
{
inputStream = new Scanner(file);
while(inputStream.hasNext())
{
String rd=inputStream.next();
System.out.println(rd + "");
}
}
catch (FileNotFoundException e)
{
e.printStackTrace();
}
}
}
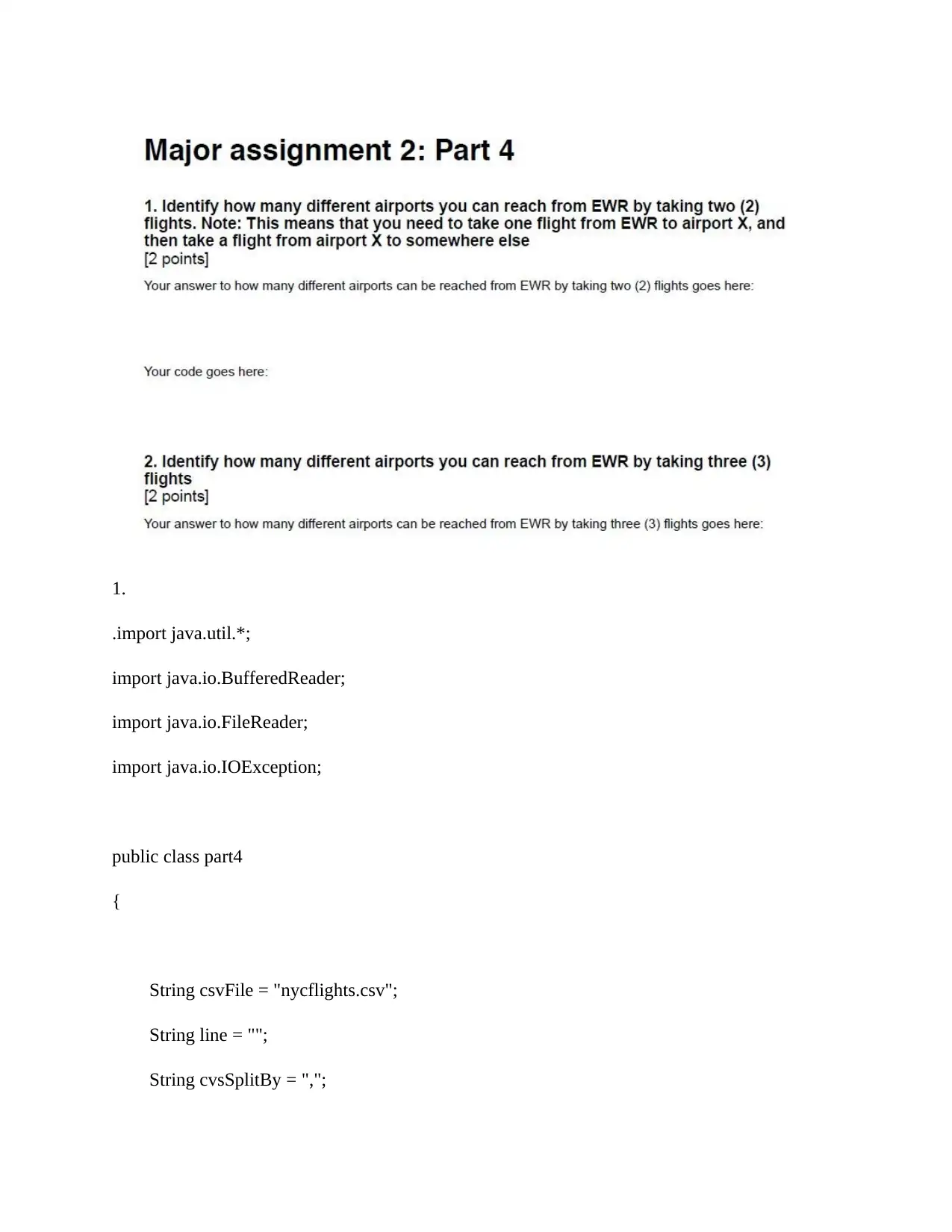
1.
.import java.util.*;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class part4
{
String csvFile = "nycflights.csv";
String line = "";
String cvsSplitBy = ",";
.import java.util.*;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class part4
{
String csvFile = "nycflights.csv";
String line = "";
String cvsSplitBy = ",";
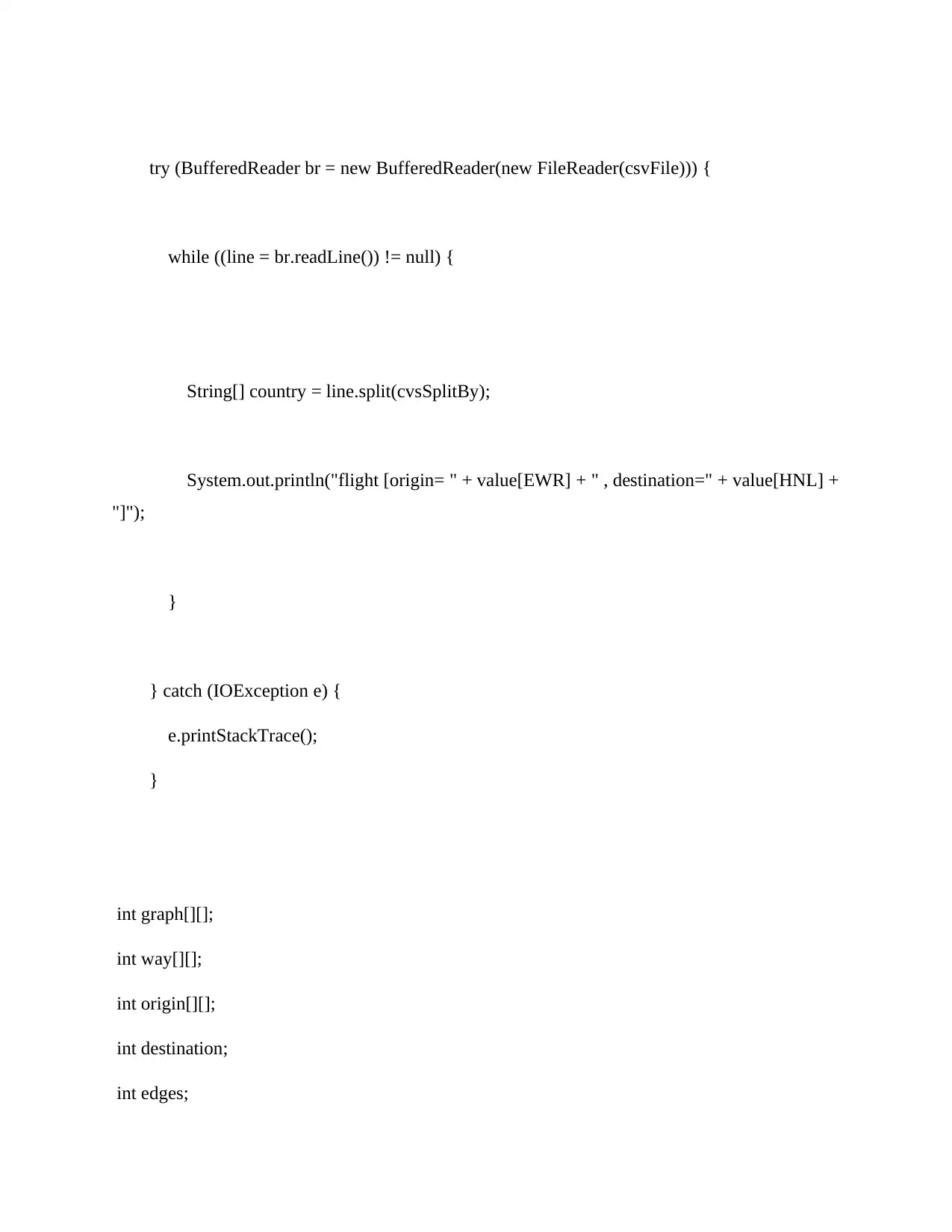
try (BufferedReader br = new BufferedReader(new FileReader(csvFile))) {
while ((line = br.readLine()) != null) {
String[] country = line.split(cvsSplitBy);
System.out.println("flight [origin= " + value[EWR] + " , destination=" + value[HNL] +
"]");
}
} catch (IOException e) {
e.printStackTrace();
}
int graph[][];
int way[][];
int origin[][];
int destination;
int edges;
while ((line = br.readLine()) != null) {
String[] country = line.split(cvsSplitBy);
System.out.println("flight [origin= " + value[EWR] + " , destination=" + value[HNL] +
"]");
}
} catch (IOException e) {
e.printStackTrace();
}
int graph[][];
int way[][];
int origin[][];
int destination;
int edges;
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
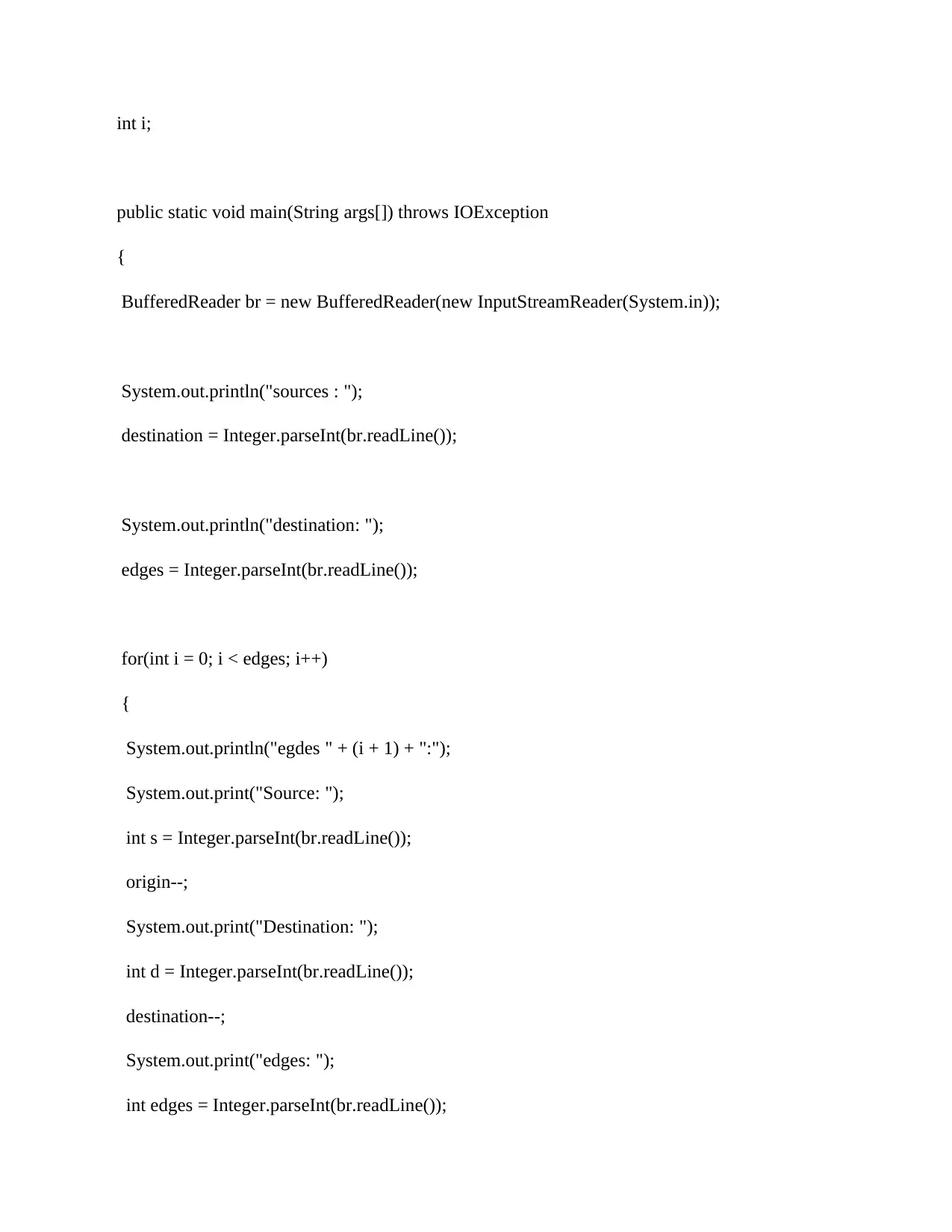
int i;
public static void main(String args[]) throws IOException
{
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
System.out.println("sources : ");
destination = Integer.parseInt(br.readLine());
System.out.println("destination: ");
edges = Integer.parseInt(br.readLine());
for(int i = 0; i < edges; i++)
{
System.out.println("egdes " + (i + 1) + ":");
System.out.print("Source: ");
int s = Integer.parseInt(br.readLine());
origin--;
System.out.print("Destination: ");
int d = Integer.parseInt(br.readLine());
destination--;
System.out.print("edges: ");
int edges = Integer.parseInt(br.readLine());
public static void main(String args[]) throws IOException
{
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
System.out.println("sources : ");
destination = Integer.parseInt(br.readLine());
System.out.println("destination: ");
edges = Integer.parseInt(br.readLine());
for(int i = 0; i < edges; i++)
{
System.out.println("egdes " + (i + 1) + ":");
System.out.print("Source: ");
int s = Integer.parseInt(br.readLine());
origin--;
System.out.print("Destination: ");
int d = Integer.parseInt(br.readLine());
destination--;
System.out.print("edges: ");
int edges = Integer.parseInt(br.readLine());
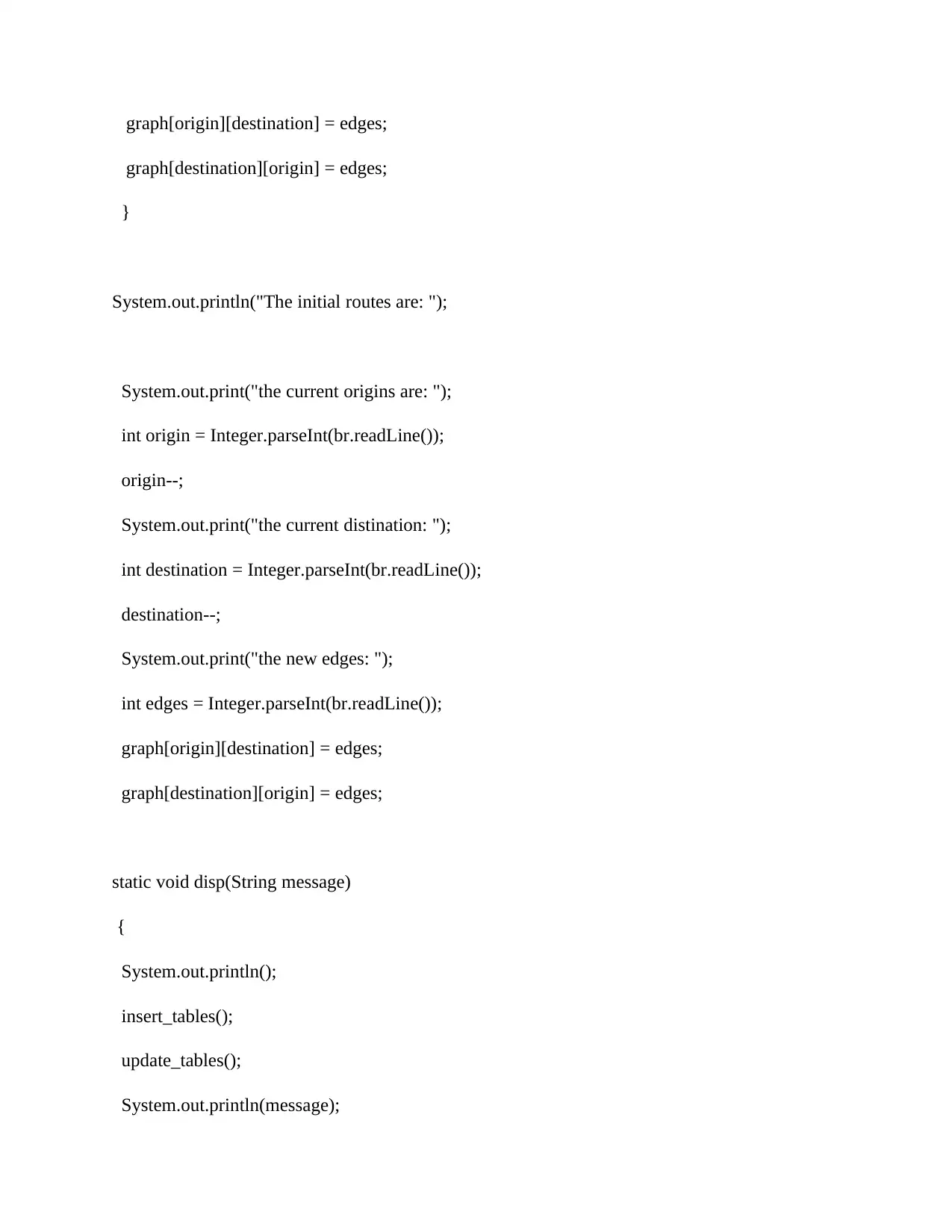
graph[origin][destination] = edges;
graph[destination][origin] = edges;
}
System.out.println("The initial routes are: ");
System.out.print("the current origins are: ");
int origin = Integer.parseInt(br.readLine());
origin--;
System.out.print("the current distination: ");
int destination = Integer.parseInt(br.readLine());
destination--;
System.out.print("the new edges: ");
int edges = Integer.parseInt(br.readLine());
graph[origin][destination] = edges;
graph[destination][origin] = edges;
static void disp(String message)
{
System.out.println();
insert_tables();
update_tables();
System.out.println(message);
graph[destination][origin] = edges;
}
System.out.println("The initial routes are: ");
System.out.print("the current origins are: ");
int origin = Integer.parseInt(br.readLine());
origin--;
System.out.print("the current distination: ");
int destination = Integer.parseInt(br.readLine());
destination--;
System.out.print("the new edges: ");
int edges = Integer.parseInt(br.readLine());
graph[origin][destination] = edges;
graph[destination][origin] = edges;
static void disp(String message)
{
System.out.println();
insert_tables();
update_tables();
System.out.println(message);
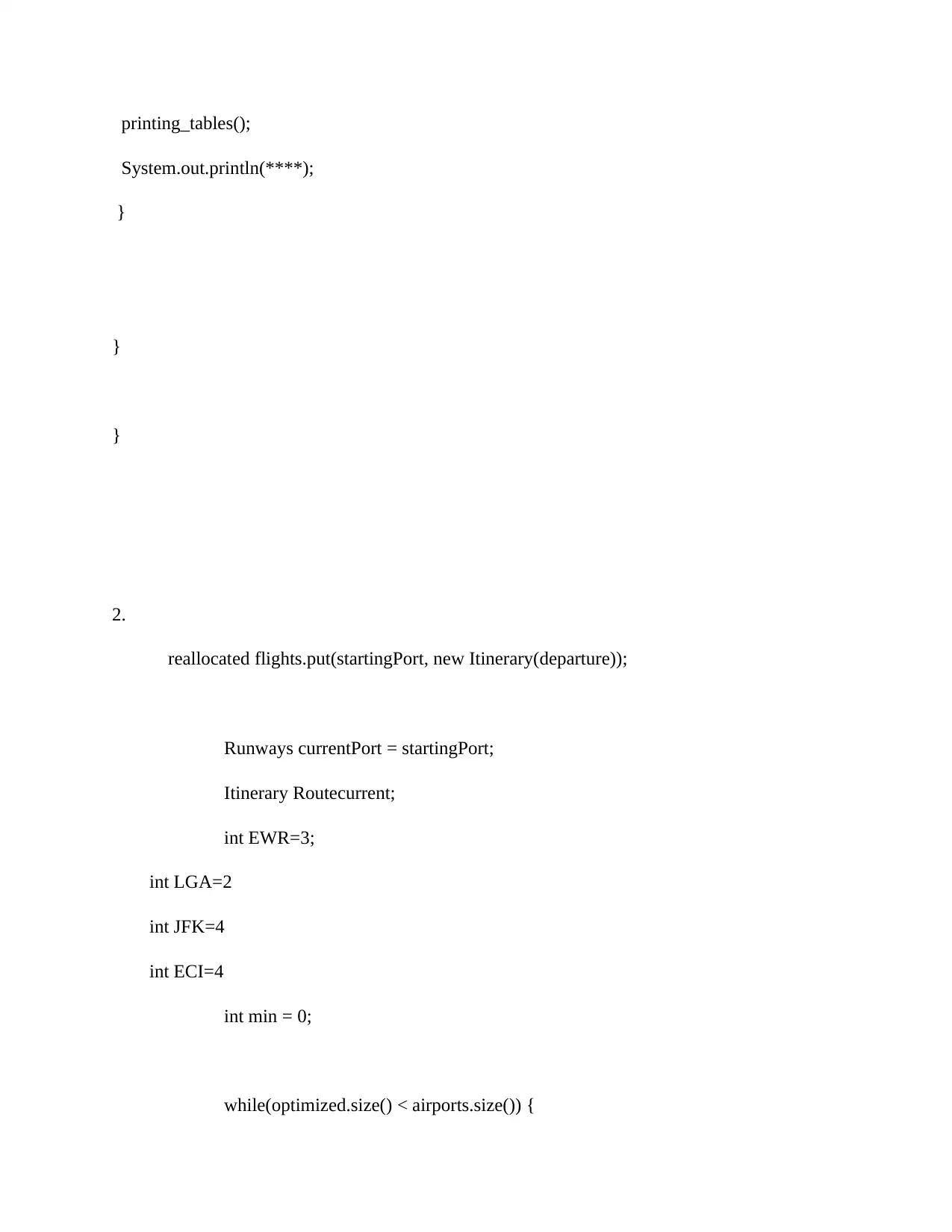
printing_tables();
System.out.println(****);
}
}
}
2.
reallocated flights.put(startingPort, new Itinerary(departure));
Runways currentPort = startingPort;
Itinerary Routecurrent;
int EWR=3;
int LGA=2
int JFK=4
int ECI=4
int min = 0;
while(optimized.size() < airports.size()) {
System.out.println(****);
}
}
}
2.
reallocated flights.put(startingPort, new Itinerary(departure));
Runways currentPort = startingPort;
Itinerary Routecurrent;
int EWR=3;
int LGA=2
int JFK=4
int ECI=4
int min = 0;
while(optimized.size() < airports.size()) {
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
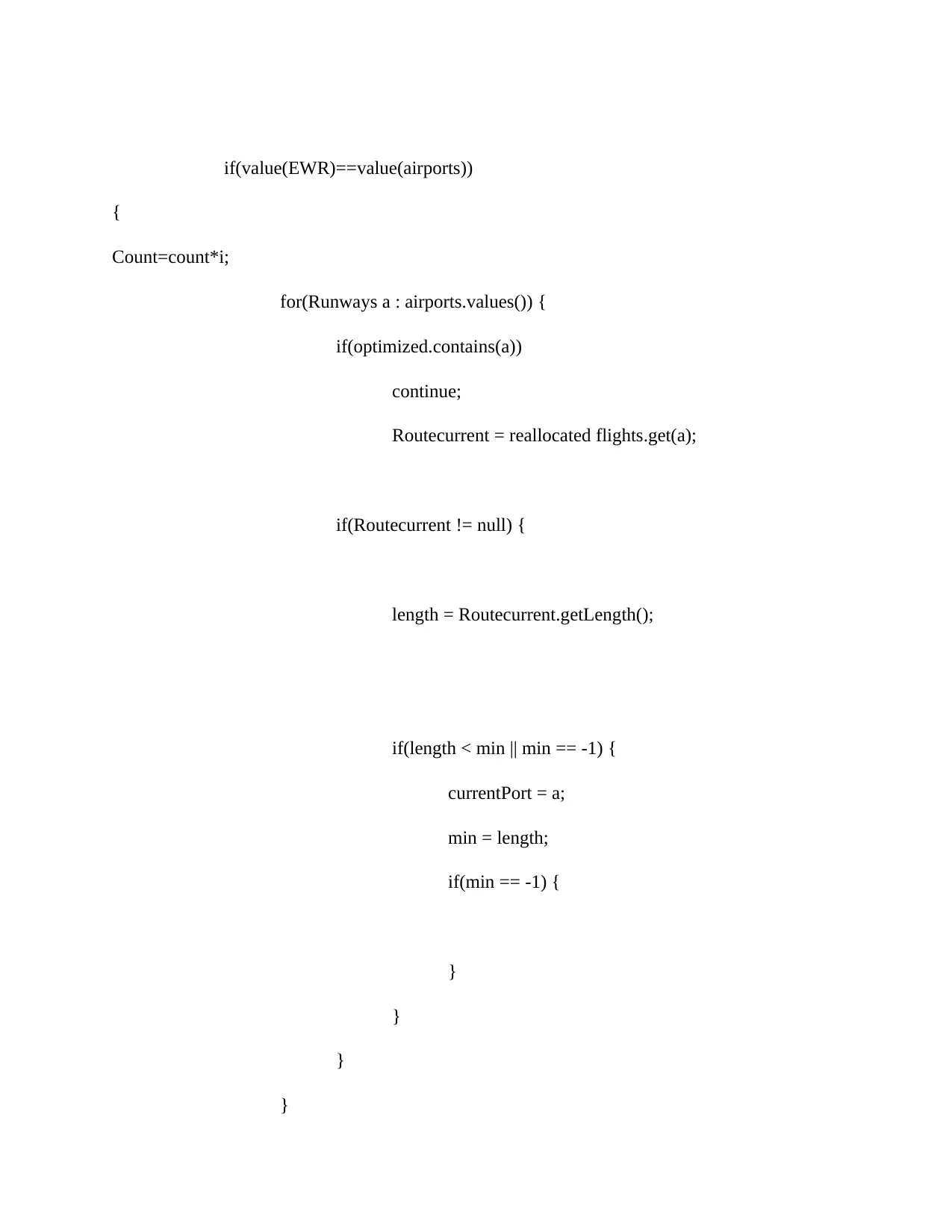
if(value(EWR)==value(airports))
{
Count=count*i;
for(Runways a : airports.values()) {
if(optimized.contains(a))
continue;
Routecurrent = reallocated flights.get(a);
if(Routecurrent != null) {
length = Routecurrent.getLength();
if(length < min || min == -1) {
currentPort = a;
min = length;
if(min == -1) {
}
}
}
}
{
Count=count*i;
for(Runways a : airports.values()) {
if(optimized.contains(a))
continue;
Routecurrent = reallocated flights.get(a);
if(Routecurrent != null) {
length = Routecurrent.getLength();
if(length < min || min == -1) {
currentPort = a;
min = length;
if(min == -1) {
}
}
}
}
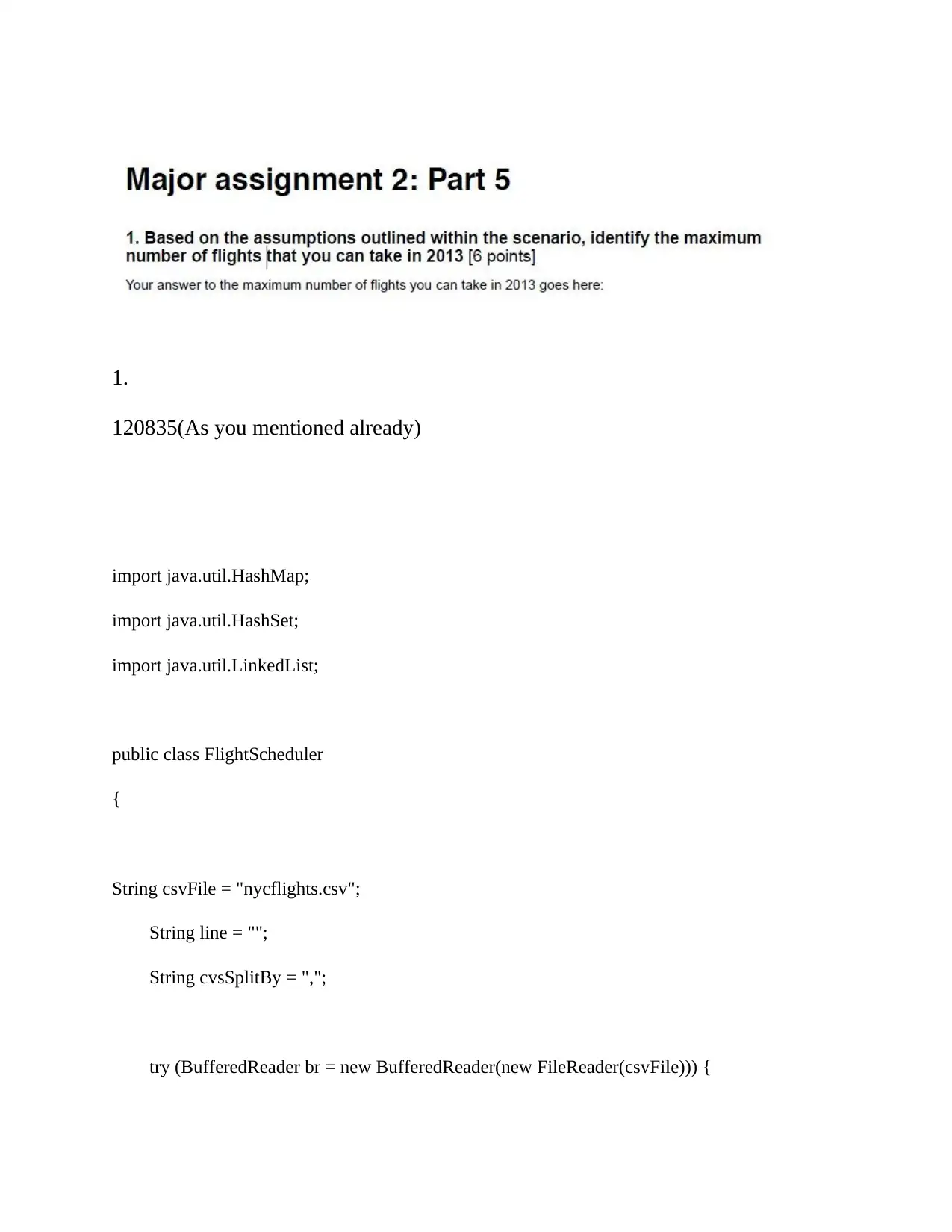
1.
120835(As you mentioned already)
import java.util.HashMap;
import java.util.HashSet;
import java.util.LinkedList;
public class FlightScheduler
{
String csvFile = "nycflights.csv";
String line = "";
String cvsSplitBy = ",";
try (BufferedReader br = new BufferedReader(new FileReader(csvFile))) {
120835(As you mentioned already)
import java.util.HashMap;
import java.util.HashSet;
import java.util.LinkedList;
public class FlightScheduler
{
String csvFile = "nycflights.csv";
String line = "";
String cvsSplitBy = ",";
try (BufferedReader br = new BufferedReader(new FileReader(csvFile))) {
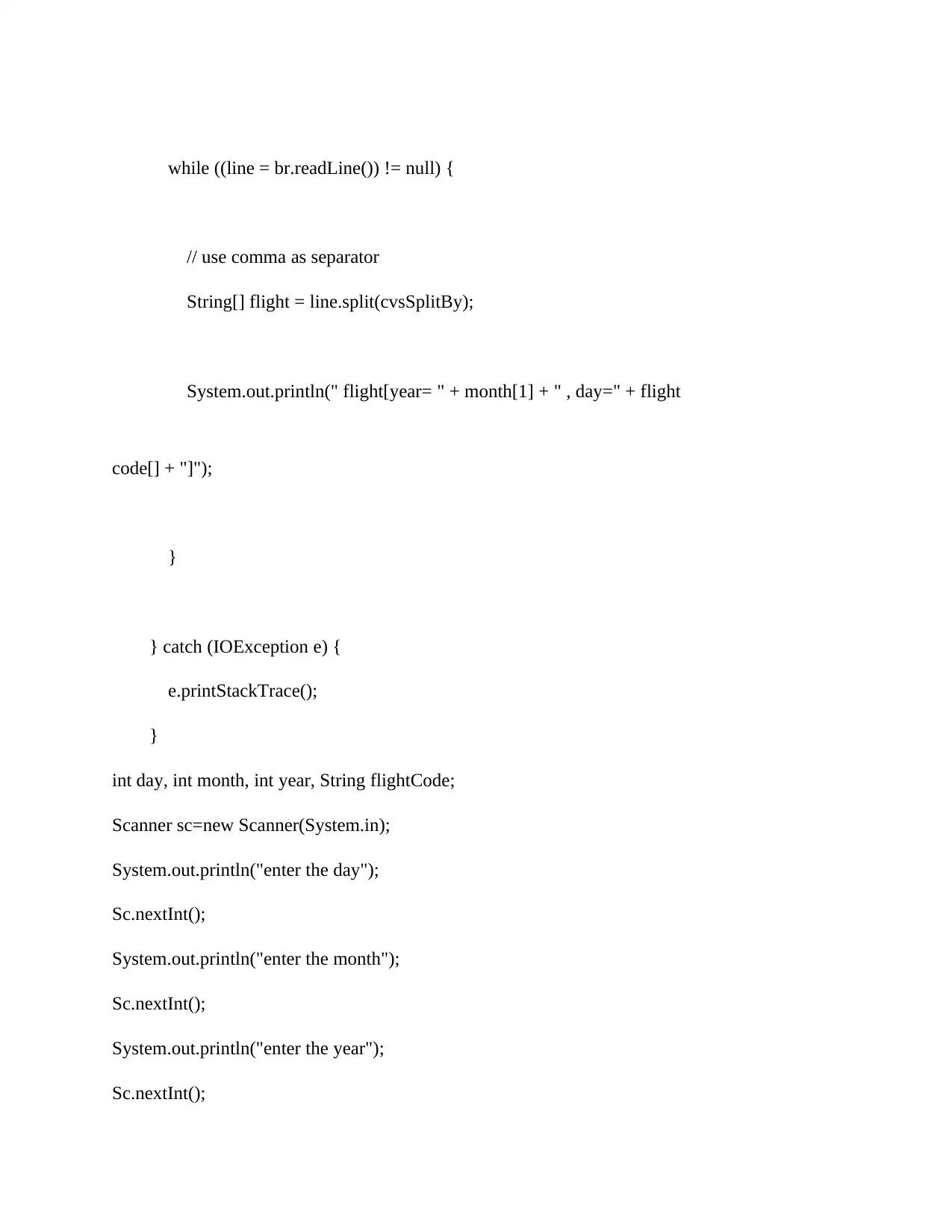
while ((line = br.readLine()) != null) {
// use comma as separator
String[] flight = line.split(cvsSplitBy);
System.out.println(" flight[year= " + month[1] + " , day=" + flight
code[] + "]");
}
} catch (IOException e) {
e.printStackTrace();
}
int day, int month, int year, String flightCode;
Scanner sc=new Scanner(System.in);
System.out.println("enter the day");
Sc.nextInt();
System.out.println("enter the month");
Sc.nextInt();
System.out.println("enter the year");
Sc.nextInt();
// use comma as separator
String[] flight = line.split(cvsSplitBy);
System.out.println(" flight[year= " + month[1] + " , day=" + flight
code[] + "]");
}
} catch (IOException e) {
e.printStackTrace();
}
int day, int month, int year, String flightCode;
Scanner sc=new Scanner(System.in);
System.out.println("enter the day");
Sc.nextInt();
System.out.println("enter the month");
Sc.nextInt();
System.out.println("enter the year");
Sc.nextInt();
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
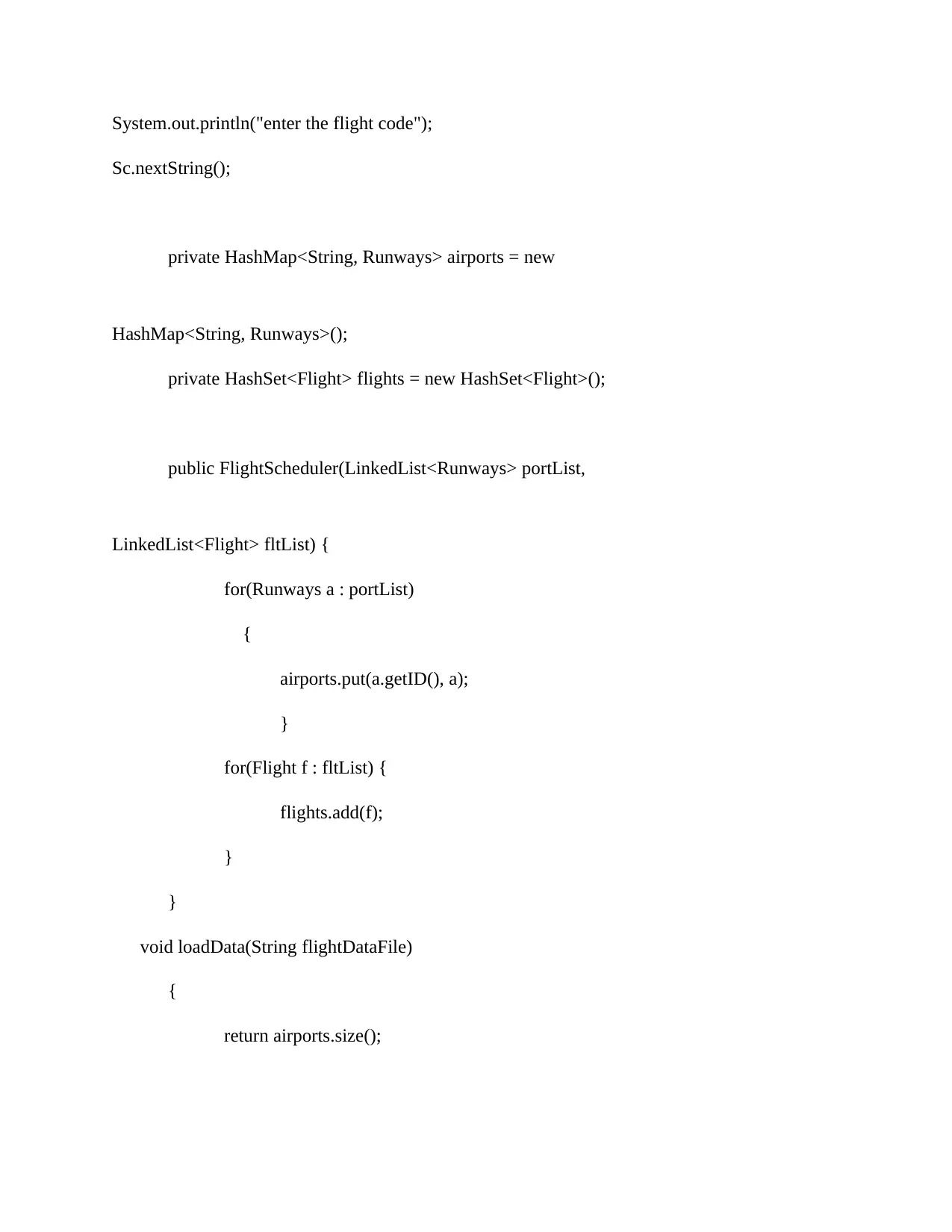
System.out.println("enter the flight code");
Sc.nextString();
private HashMap<String, Runways> airports = new
HashMap<String, Runways>();
private HashSet<Flight> flights = new HashSet<Flight>();
public FlightScheduler(LinkedList<Runways> portList,
LinkedList<Flight> fltList) {
for(Runways a : portList)
{
airports.put(a.getID(), a);
}
for(Flight f : fltList) {
flights.add(f);
}
}
void loadData(String flightDataFile)
{
return airports.size();
Sc.nextString();
private HashMap<String, Runways> airports = new
HashMap<String, Runways>();
private HashSet<Flight> flights = new HashSet<Flight>();
public FlightScheduler(LinkedList<Runways> portList,
LinkedList<Flight> fltList) {
for(Runways a : portList)
{
airports.put(a.getID(), a);
}
for(Flight f : fltList) {
flights.add(f);
}
}
void loadData(String flightDataFile)
{
return airports.size();
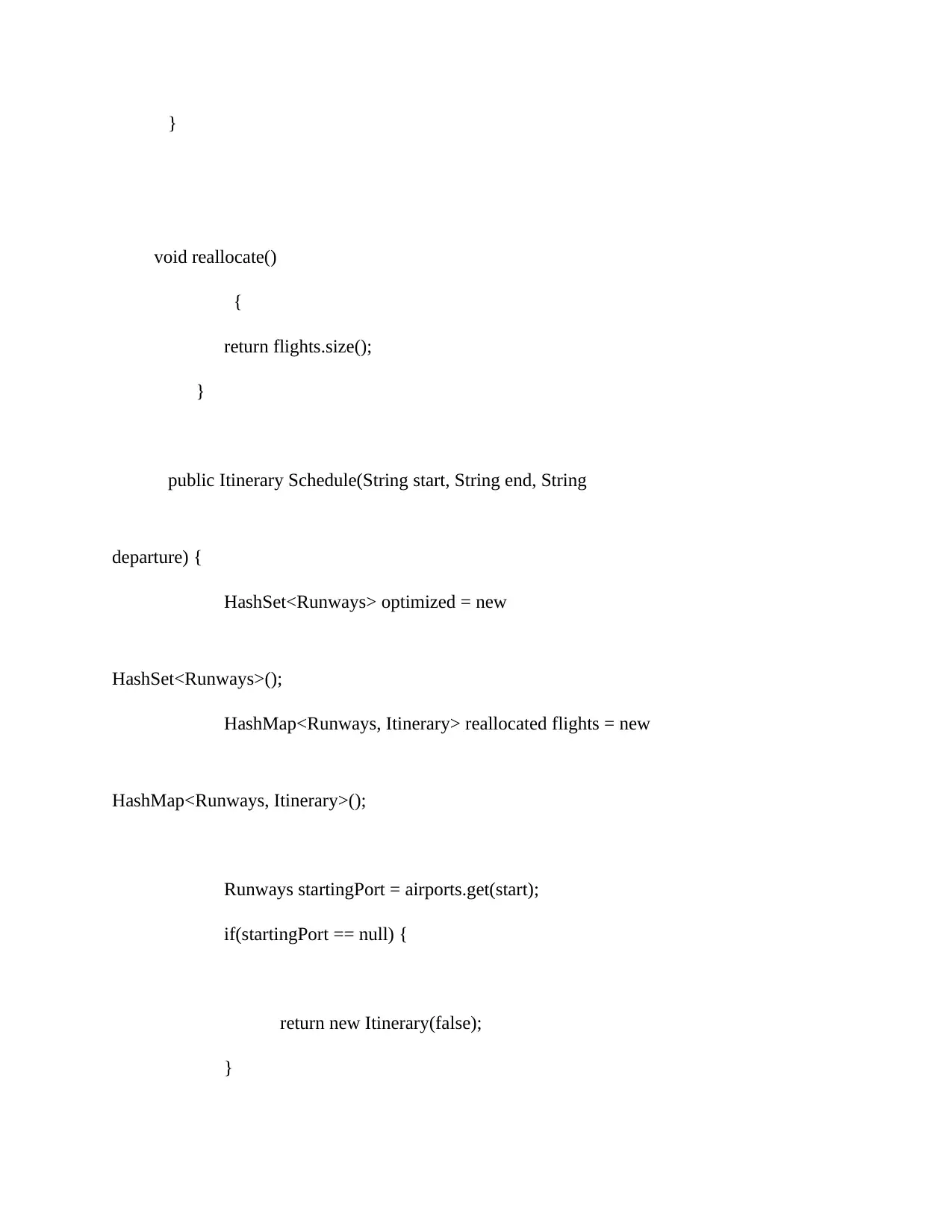
}
void reallocate()
{
return flights.size();
}
public Itinerary Schedule(String start, String end, String
departure) {
HashSet<Runways> optimized = new
HashSet<Runways>();
HashMap<Runways, Itinerary> reallocated flights = new
HashMap<Runways, Itinerary>();
Runways startingPort = airports.get(start);
if(startingPort == null) {
return new Itinerary(false);
}
void reallocate()
{
return flights.size();
}
public Itinerary Schedule(String start, String end, String
departure) {
HashSet<Runways> optimized = new
HashSet<Runways>();
HashMap<Runways, Itinerary> reallocated flights = new
HashMap<Runways, Itinerary>();
Runways startingPort = airports.get(start);
if(startingPort == null) {
return new Itinerary(false);
}
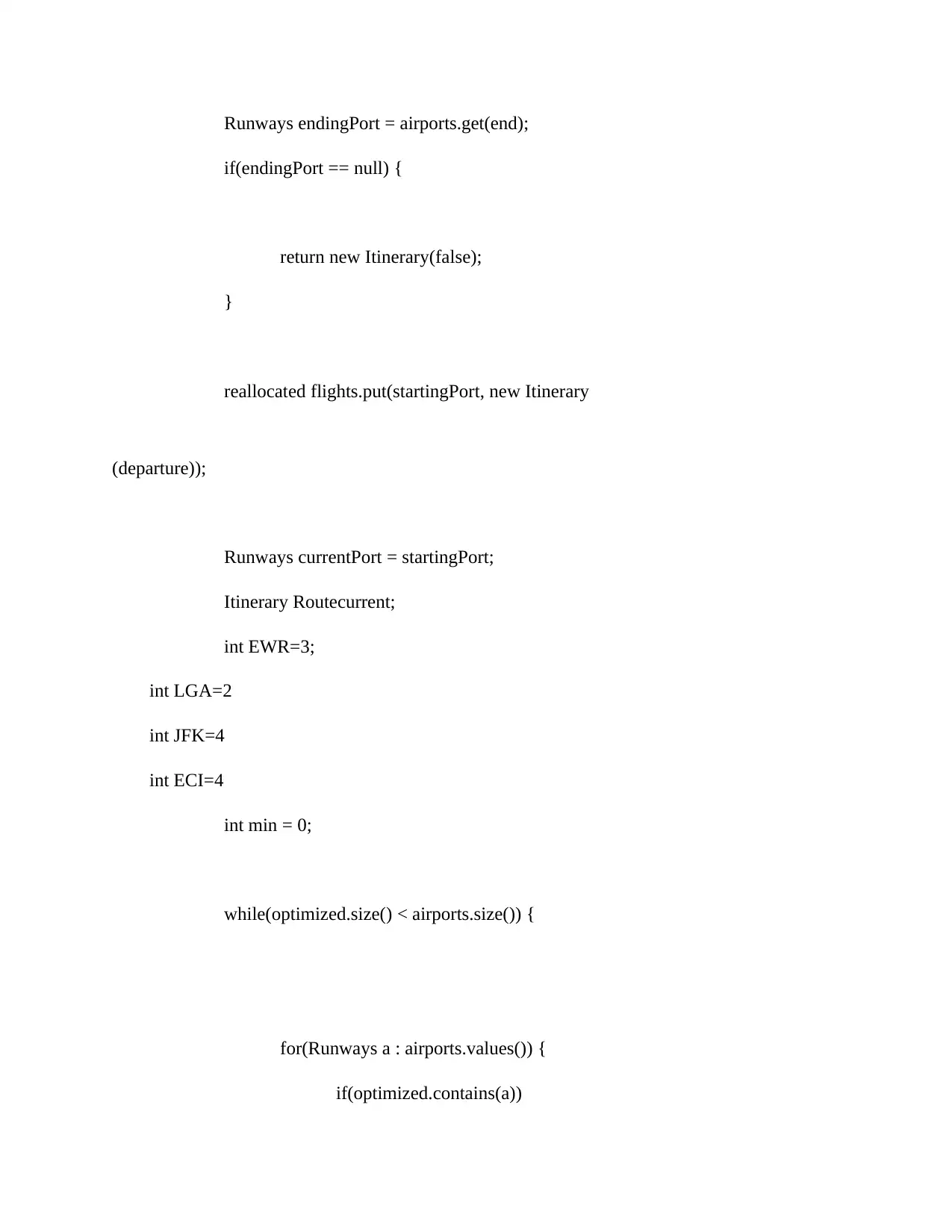
Runways endingPort = airports.get(end);
if(endingPort == null) {
return new Itinerary(false);
}
reallocated flights.put(startingPort, new Itinerary
(departure));
Runways currentPort = startingPort;
Itinerary Routecurrent;
int EWR=3;
int LGA=2
int JFK=4
int ECI=4
int min = 0;
while(optimized.size() < airports.size()) {
for(Runways a : airports.values()) {
if(optimized.contains(a))
if(endingPort == null) {
return new Itinerary(false);
}
reallocated flights.put(startingPort, new Itinerary
(departure));
Runways currentPort = startingPort;
Itinerary Routecurrent;
int EWR=3;
int LGA=2
int JFK=4
int ECI=4
int min = 0;
while(optimized.size() < airports.size()) {
for(Runways a : airports.values()) {
if(optimized.contains(a))
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
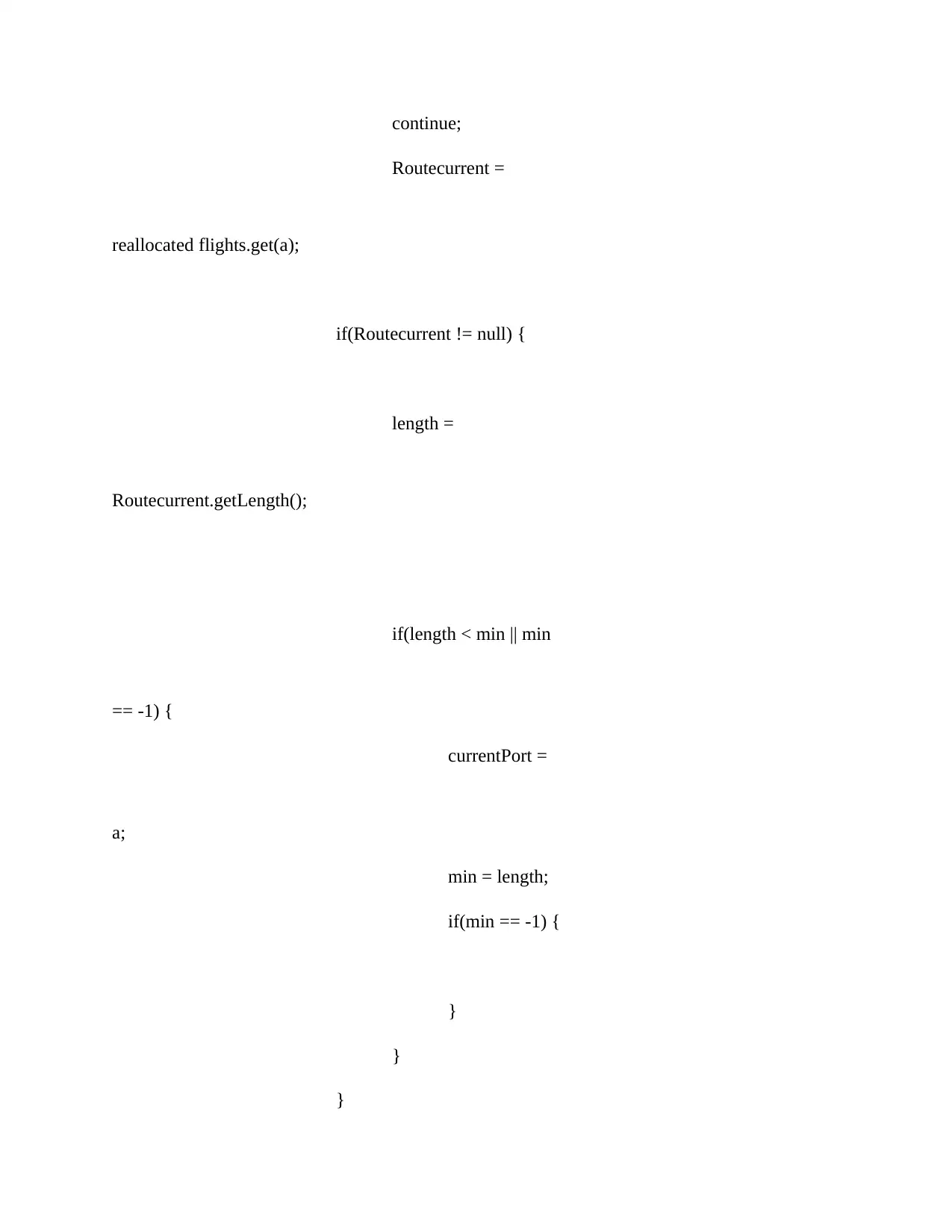
continue;
Routecurrent =
reallocated flights.get(a);
if(Routecurrent != null) {
length =
Routecurrent.getLength();
if(length < min || min
== -1) {
currentPort =
a;
min = length;
if(min == -1) {
}
}
}
Routecurrent =
reallocated flights.get(a);
if(Routecurrent != null) {
length =
Routecurrent.getLength();
if(length < min || min
== -1) {
currentPort =
a;
min = length;
if(min == -1) {
}
}
}
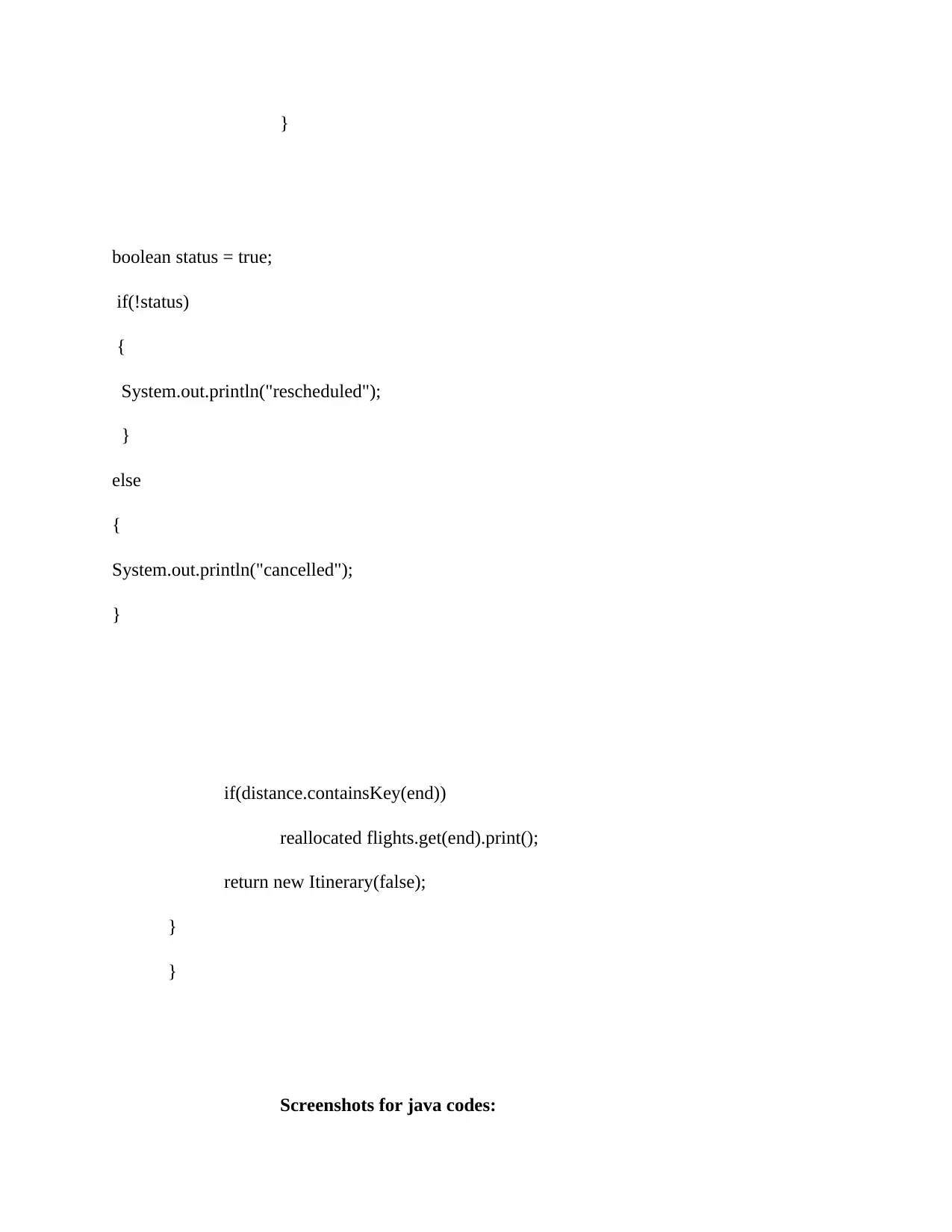
}
boolean status = true;
if(!status)
{
System.out.println("rescheduled");
}
else
{
System.out.println("cancelled");
}
if(distance.containsKey(end))
reallocated flights.get(end).print();
return new Itinerary(false);
}
}
Screenshots for java codes:
boolean status = true;
if(!status)
{
System.out.println("rescheduled");
}
else
{
System.out.println("cancelled");
}
if(distance.containsKey(end))
reallocated flights.get(end).print();
return new Itinerary(false);
}
}
Screenshots for java codes:
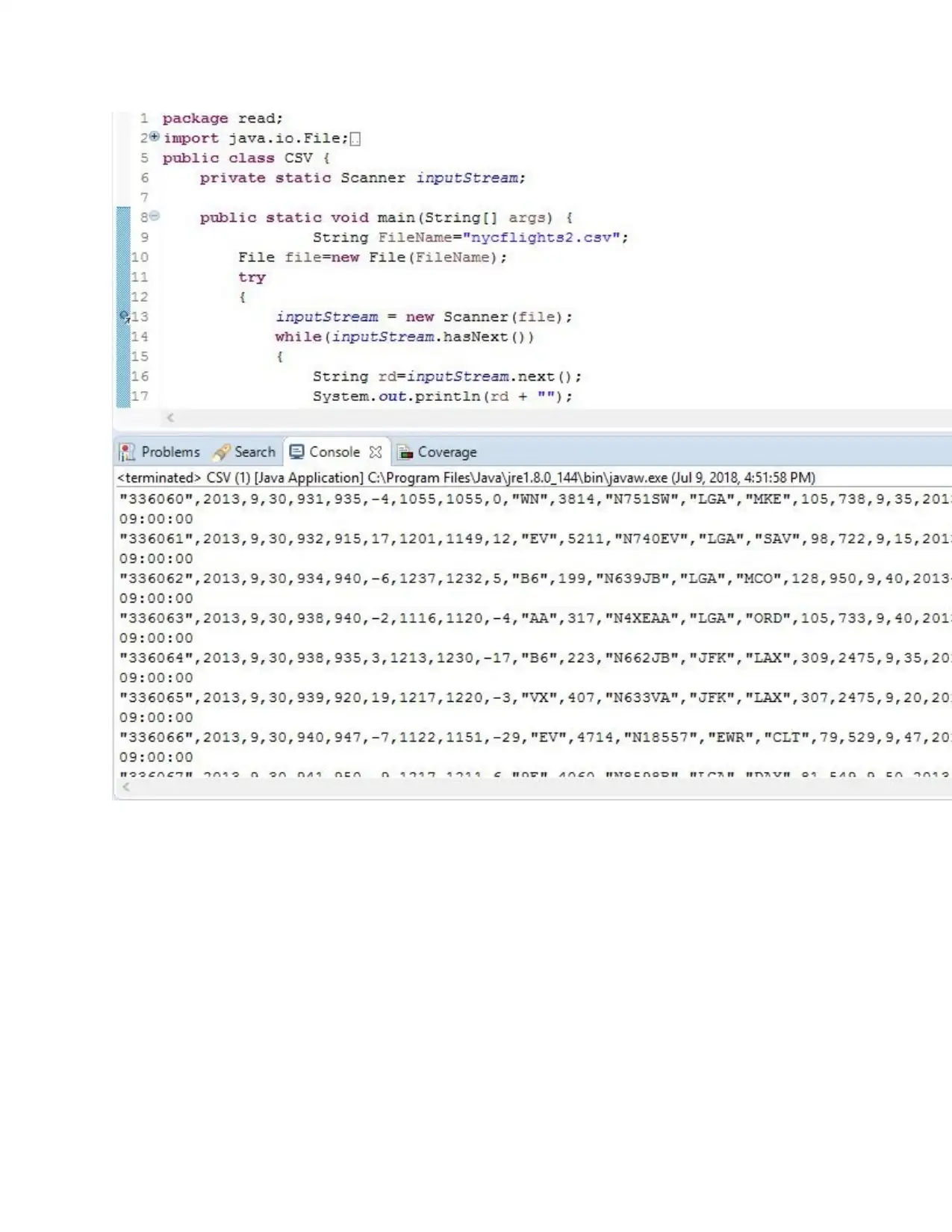
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
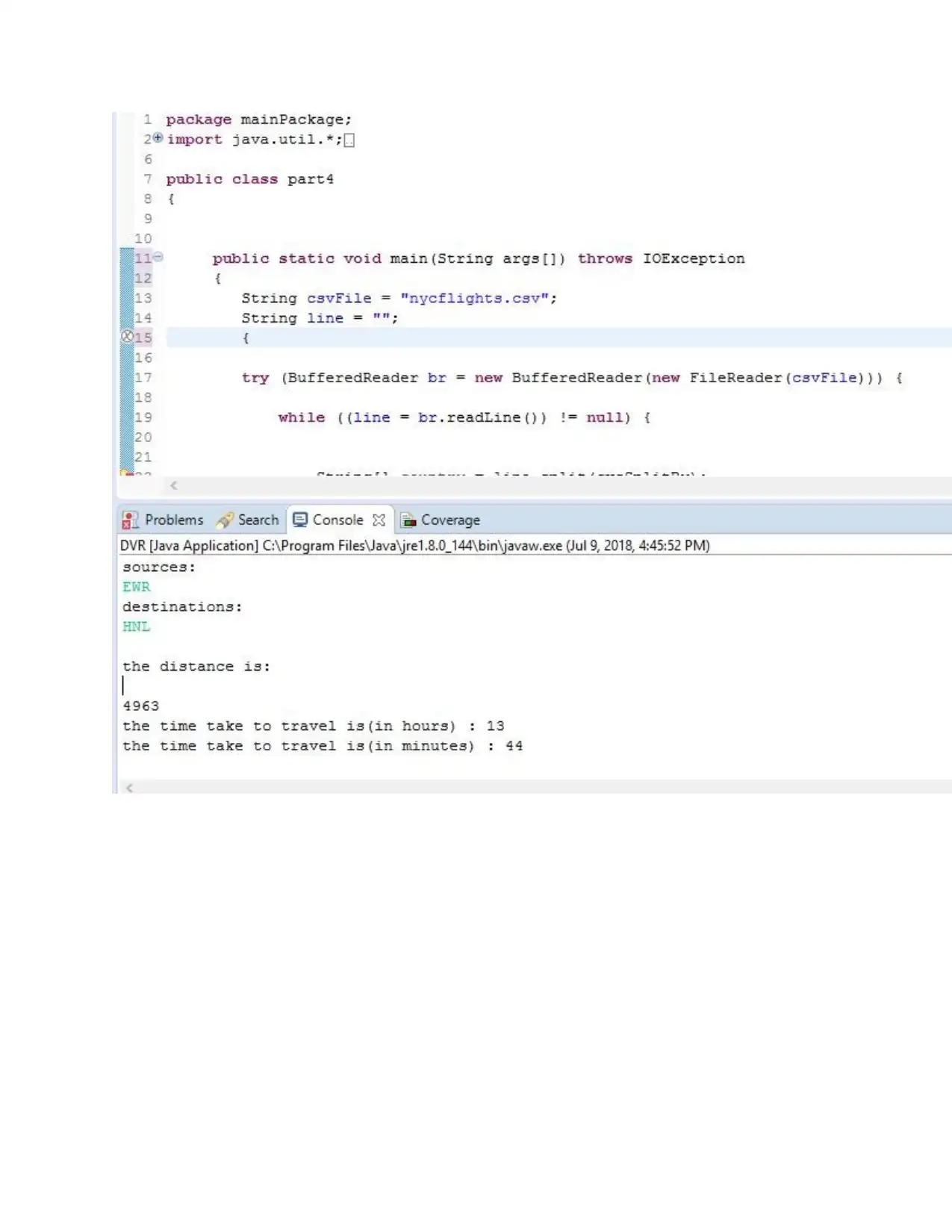
1 out of 26

Your All-in-One AI-Powered Toolkit for Academic Success.
 +13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024  |  Zucol Services PVT LTD  |  All rights reserved.