JavaFX Project: Course Management System
VerifiedAdded on  2019/10/16
|22
|3494
|297
Project
AI Summary
This document outlines a JavaFX project assignment, requiring students to develop an application using JavaFX, event handling, file I/O (sequential or random), and ArrayLists. Students can choose their project topic, but it must include functionalities like adding, editing, deleting, and searching records. The document provides two detailed examples: a course management system and a quiz game. The course management system example includes file structures, UML diagrams, class descriptions, and detailed functionality explanations, covering aspects like startup, file handling, adding, editing, saving, deleting, and searching records. The quiz game example provides a basic structure for a different type of application. The project is worth 15% of the final grade and can be done individually or in groups of two.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
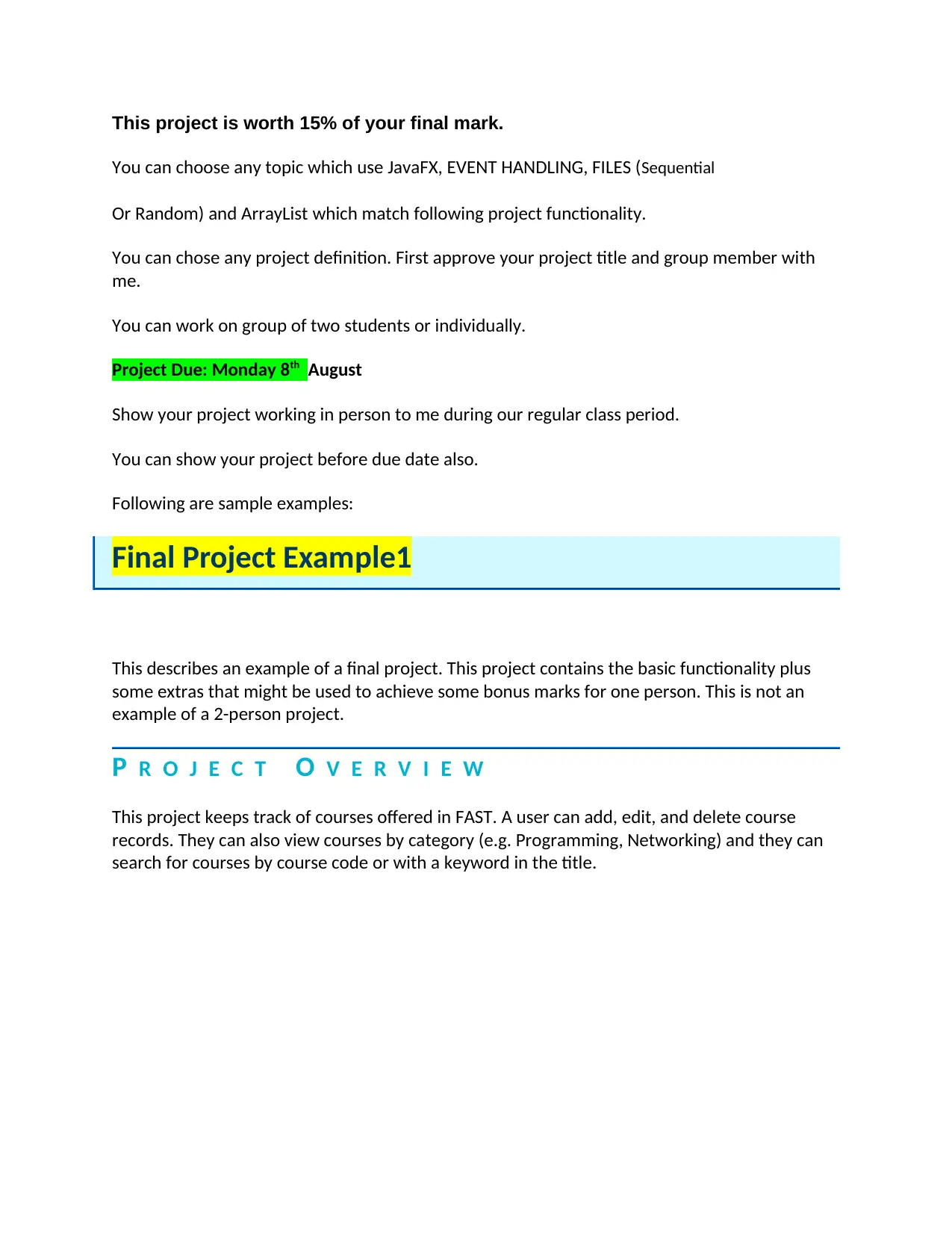
This project is worth 15% of your final mark.
You can choose any topic which use JavaFX, EVENT HANDLING, FILES (Sequential
Or Random) and ArrayList which match following project functionality.
You can chose any project definition. First approve your project title and group member with
me.
You can work on group of two students or individually.
Project Due: Monday 8th August
Show your project working in person to me during our regular class period.
You can show your project before due date also.
Following are sample examples:
Final Project Example1
This describes an example of a final project. This project contains the basic functionality plus
some extras that might be used to achieve some bonus marks for one person. This is not an
example of a 2-person project.
P R O J E C T O V E R V I E W
This project keeps track of courses offered in FAST. A user can add, edit, and delete course
records. They can also view courses by category (e.g. Programming, Networking) and they can
search for courses by course code or with a keyword in the title.
You can choose any topic which use JavaFX, EVENT HANDLING, FILES (Sequential
Or Random) and ArrayList which match following project functionality.
You can chose any project definition. First approve your project title and group member with
me.
You can work on group of two students or individually.
Project Due: Monday 8th August
Show your project working in person to me during our regular class period.
You can show your project before due date also.
Following are sample examples:
Final Project Example1
This describes an example of a final project. This project contains the basic functionality plus
some extras that might be used to achieve some bonus marks for one person. This is not an
example of a 2-person project.
P R O J E C T O V E R V I E W
This project keeps track of courses offered in FAST. A user can add, edit, and delete course
records. They can also view courses by category (e.g. Programming, Networking) and they can
search for courses by course code or with a keyword in the title.
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
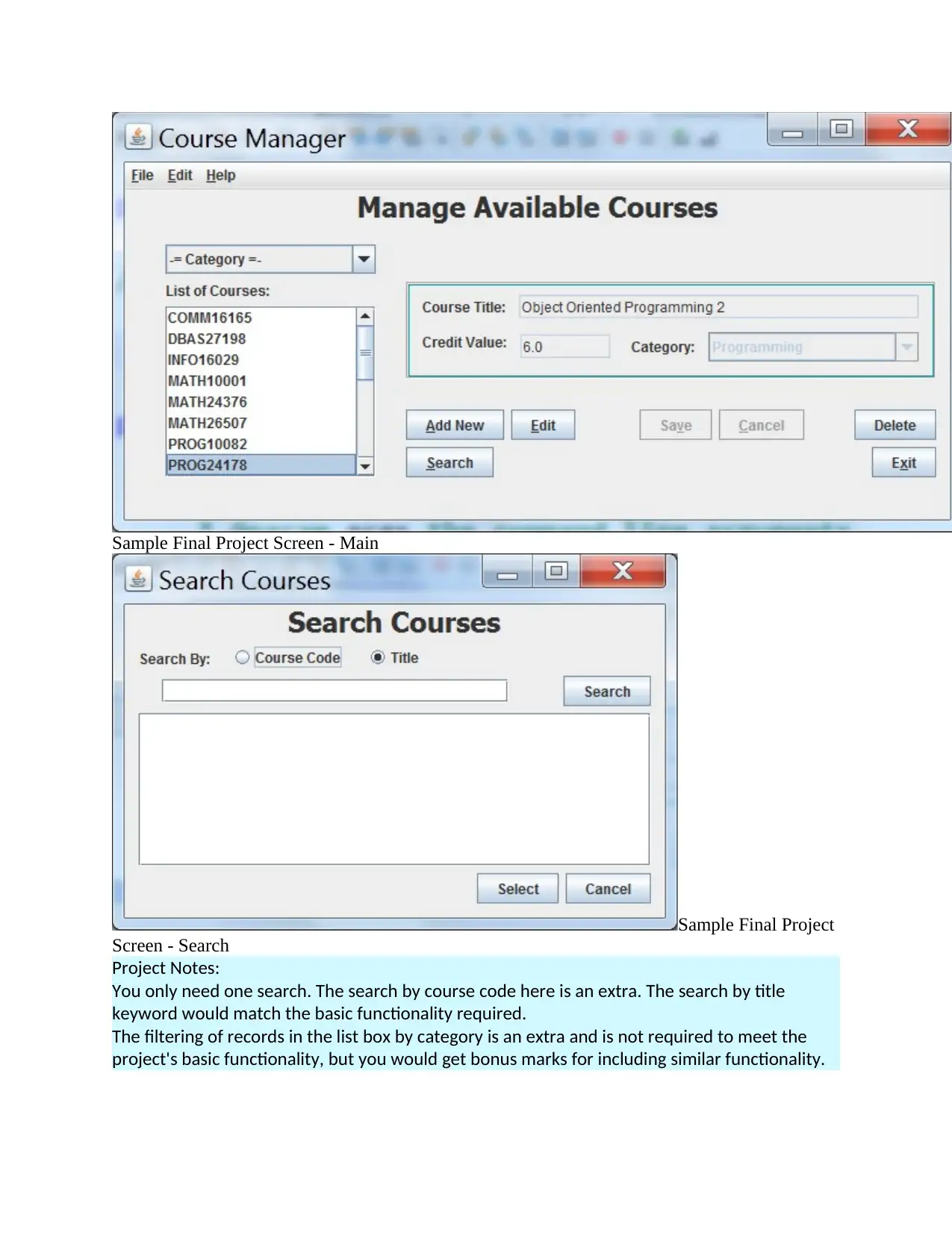
Sample Final Project Screen - Main
Sample Final Project
Screen - Search
Project Notes:
You only need one search. The search by course code here is an extra. The search by title
keyword would match the basic functionality required.
The filtering of records in the list box by category is an extra and is not required to meet the
project's basic functionality, but you would get bonus marks for including similar functionality.
Sample Final Project
Screen - Search
Project Notes:
You only need one search. The search by course code here is an extra. The search by title
keyword would match the basic functionality required.
The filtering of records in the list box by category is an extra and is not required to meet the
project's basic functionality, but you would get bonus marks for including similar functionality.
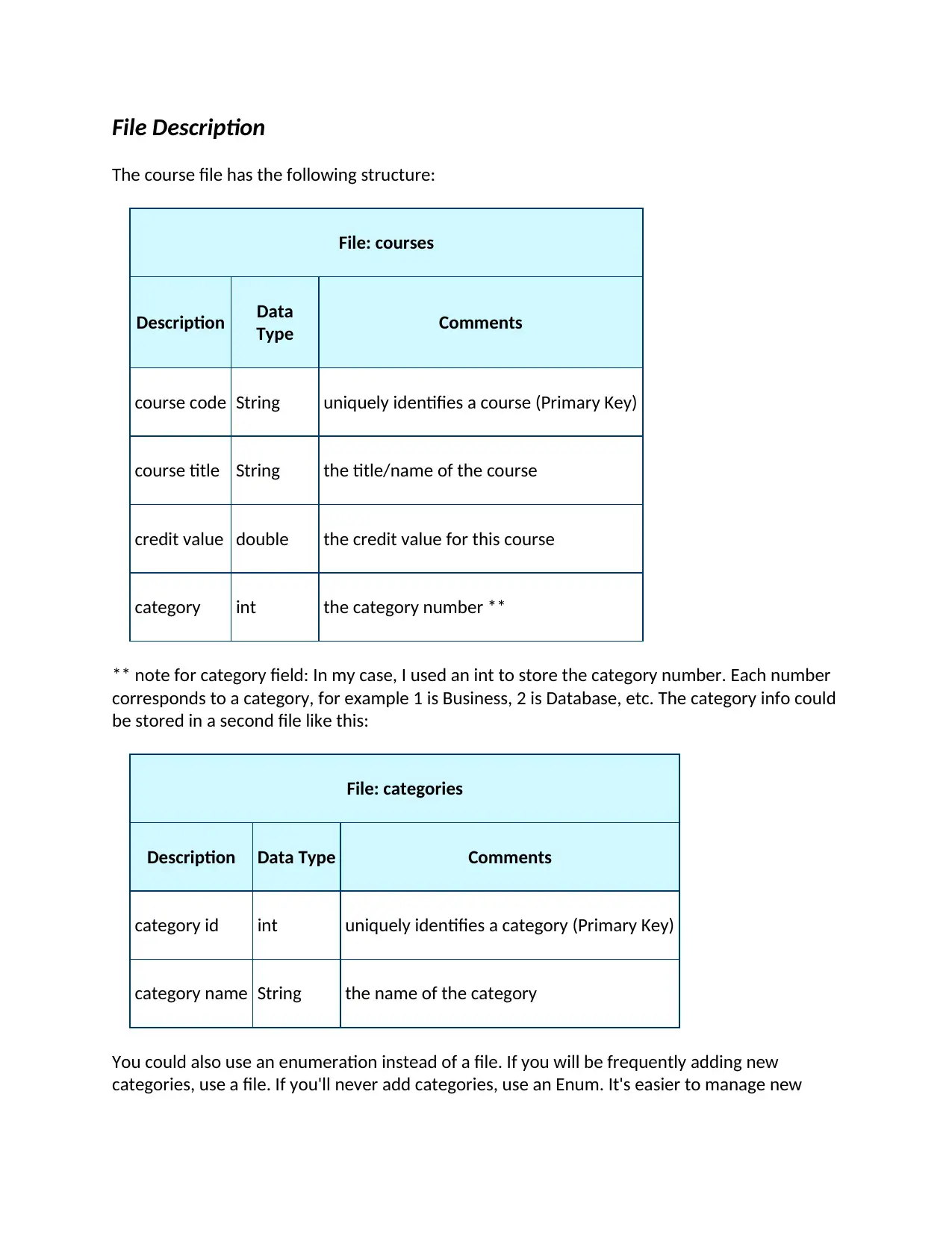
File Description
The course file has the following structure:
File: courses
Description Data
Type Comments
course code String uniquely identifies a course (Primary Key)
course title String the title/name of the course
credit value double the credit value for this course
category int the category number **
** note for category field: In my case, I used an int to store the category number. Each number
corresponds to a category, for example 1 is Business, 2 is Database, etc. The category info could
be stored in a second file like this:
File: categories
Description Data Type Comments
category id int uniquely identifies a category (Primary Key)
category name String the name of the category
You could also use an enumeration instead of a file. If you will be frequently adding new
categories, use a file. If you'll never add categories, use an Enum. It's easier to manage new
The course file has the following structure:
File: courses
Description Data
Type Comments
course code String uniquely identifies a course (Primary Key)
course title String the title/name of the course
credit value double the credit value for this course
category int the category number **
** note for category field: In my case, I used an int to store the category number. Each number
corresponds to a category, for example 1 is Business, 2 is Database, etc. The category info could
be stored in a second file like this:
File: categories
Description Data Type Comments
category id int uniquely identifies a category (Primary Key)
category name String the name of the category
You could also use an enumeration instead of a file. If you will be frequently adding new
categories, use a file. If you'll never add categories, use an Enum. It's easier to manage new
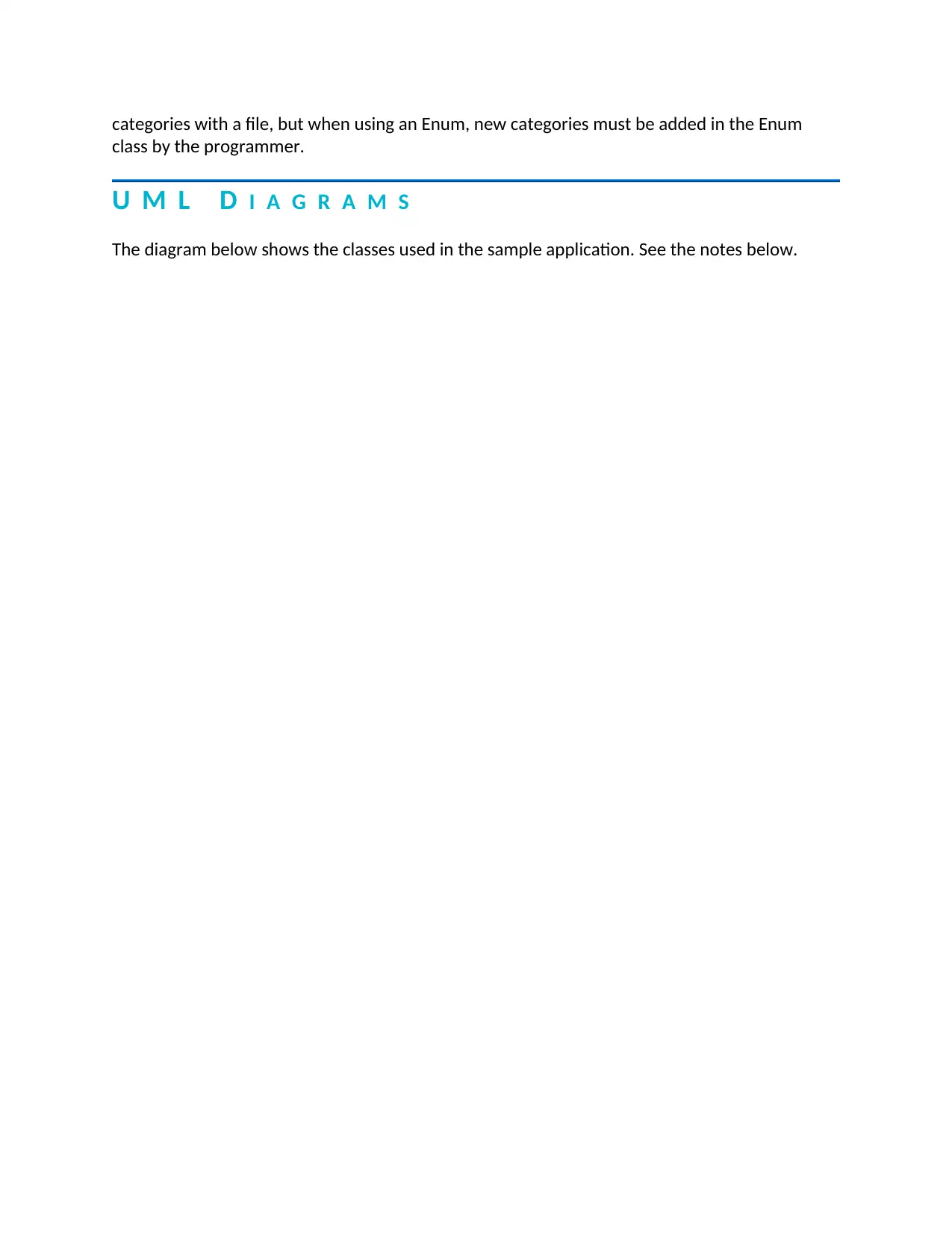
categories with a file, but when using an Enum, new categories must be added in the Enum
class by the programmer.
U M L D I A G R A M S
The diagram below shows the classes used in the sample application. See the notes below.
class by the programmer.
U M L D I A G R A M S
The diagram below shows the classes used in the sample application. See the notes below.
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
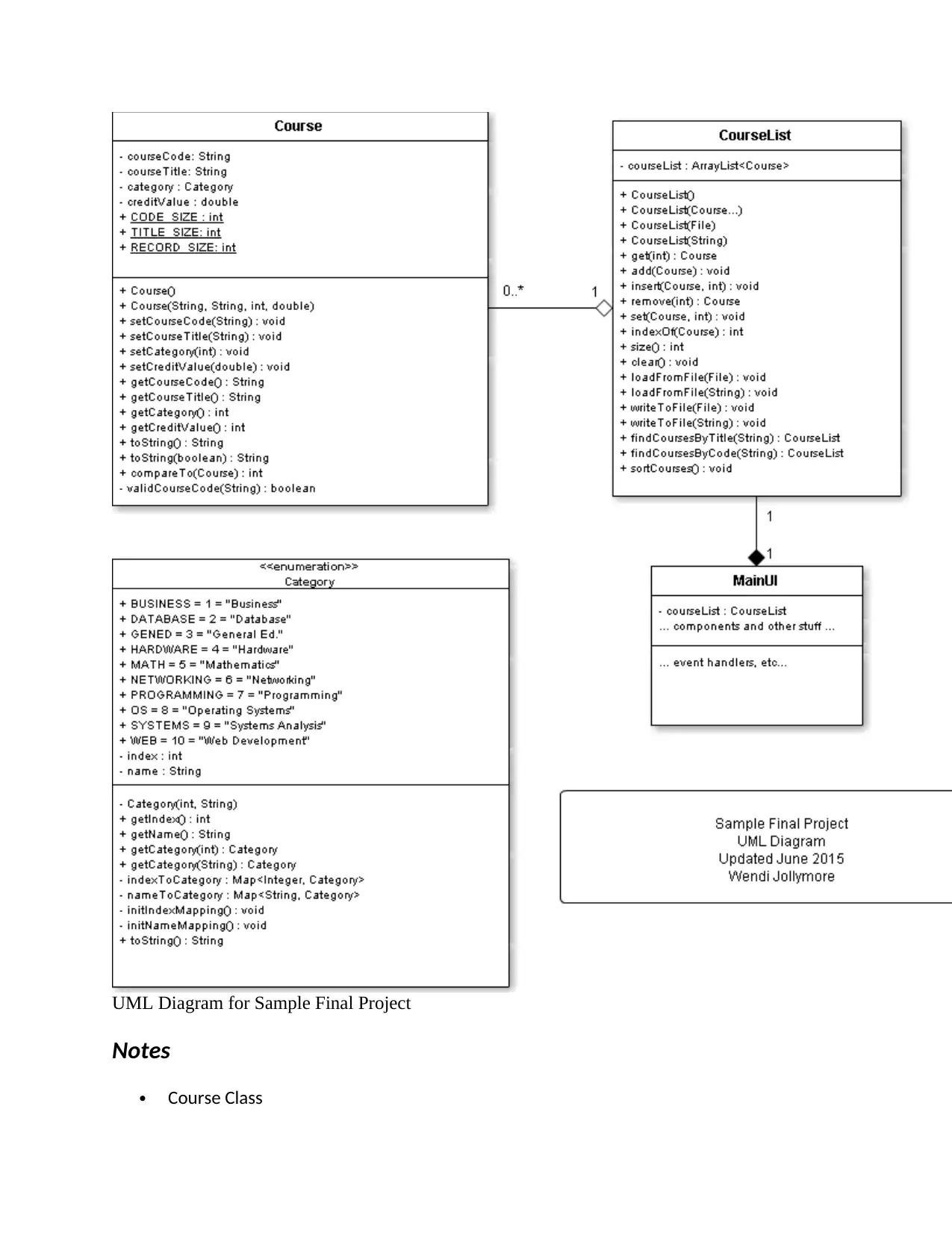
UML Diagram for Sample Final Project
Notes
ď‚· Course Class
Notes
ď‚· Course Class
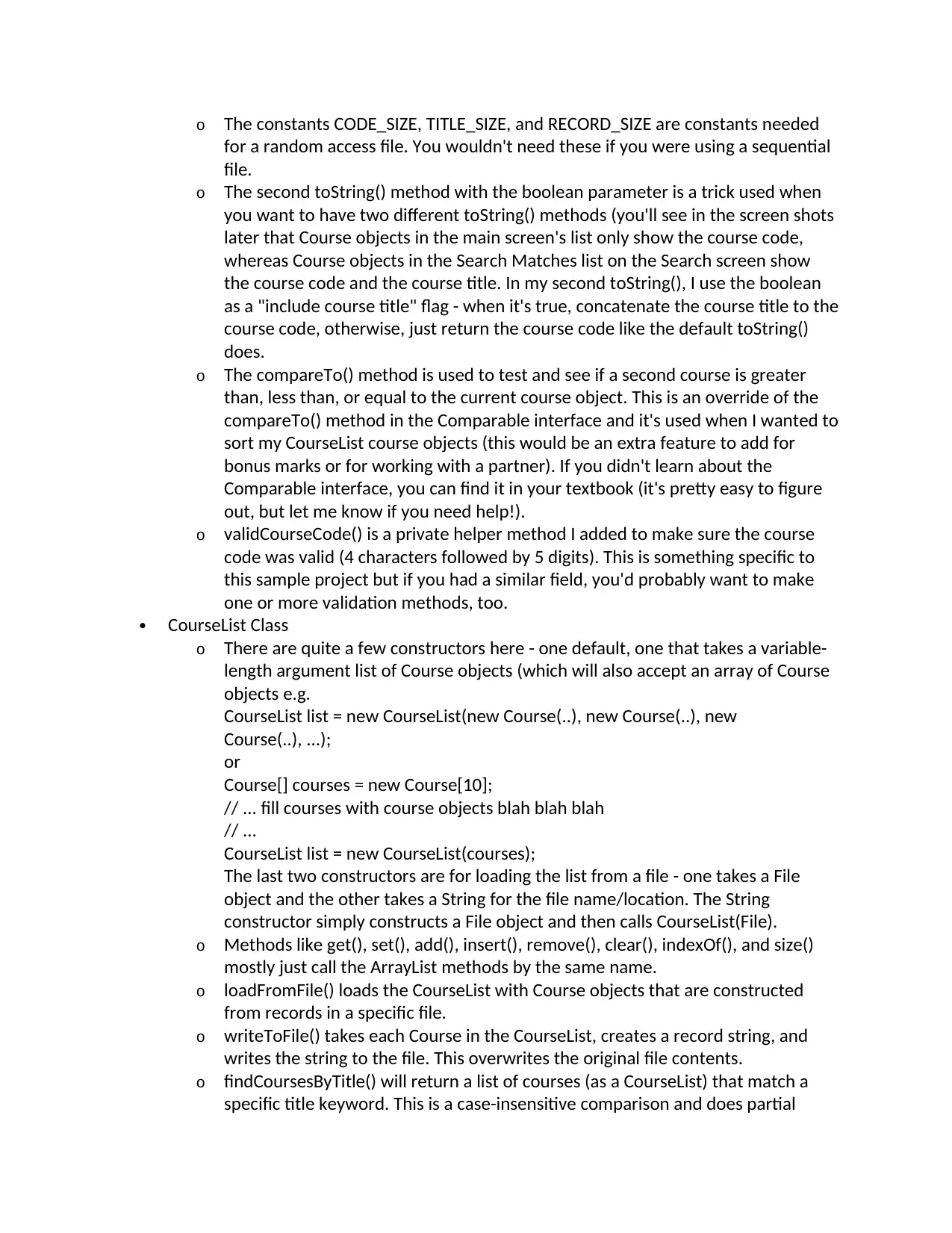
o The constants CODE_SIZE, TITLE_SIZE, and RECORD_SIZE are constants needed
for a random access file. You wouldn't need these if you were using a sequential
file.
o The second toString() method with the boolean parameter is a trick used when
you want to have two different toString() methods (you'll see in the screen shots
later that Course objects in the main screen's list only show the course code,
whereas Course objects in the Search Matches list on the Search screen show
the course code and the course title. In my second toString(), I use the boolean
as a "include course title" flag - when it's true, concatenate the course title to the
course code, otherwise, just return the course code like the default toString()
does.
o The compareTo() method is used to test and see if a second course is greater
than, less than, or equal to the current course object. This is an override of the
compareTo() method in the Comparable interface and it's used when I wanted to
sort my CourseList course objects (this would be an extra feature to add for
bonus marks or for working with a partner). If you didn't learn about the
Comparable interface, you can find it in your textbook (it's pretty easy to figure
out, but let me know if you need help!).
o validCourseCode() is a private helper method I added to make sure the course
code was valid (4 characters followed by 5 digits). This is something specific to
this sample project but if you had a similar field, you'd probably want to make
one or more validation methods, too.
ď‚· CourseList Class
o There are quite a few constructors here - one default, one that takes a variable-
length argument list of Course objects (which will also accept an array of Course
objects e.g.
CourseList list = new CourseList(new Course(..), new Course(..), new
Course(..), ...);
or
Course[] courses = new Course[10];
// ... fill courses with course objects blah blah blah
// ...
CourseList list = new CourseList(courses);
The last two constructors are for loading the list from a file - one takes a File
object and the other takes a String for the file name/location. The String
constructor simply constructs a File object and then calls CourseList(File).
o Methods like get(), set(), add(), insert(), remove(), clear(), indexOf(), and size()
mostly just call the ArrayList methods by the same name.
o loadFromFile() loads the CourseList with Course objects that are constructed
from records in a specific file.
o writeToFile() takes each Course in the CourseList, creates a record string, and
writes the string to the file. This overwrites the original file contents.
o findCoursesByTitle() will return a list of courses (as a CourseList) that match a
specific title keyword. This is a case-insensitive comparison and does partial
for a random access file. You wouldn't need these if you were using a sequential
file.
o The second toString() method with the boolean parameter is a trick used when
you want to have two different toString() methods (you'll see in the screen shots
later that Course objects in the main screen's list only show the course code,
whereas Course objects in the Search Matches list on the Search screen show
the course code and the course title. In my second toString(), I use the boolean
as a "include course title" flag - when it's true, concatenate the course title to the
course code, otherwise, just return the course code like the default toString()
does.
o The compareTo() method is used to test and see if a second course is greater
than, less than, or equal to the current course object. This is an override of the
compareTo() method in the Comparable interface and it's used when I wanted to
sort my CourseList course objects (this would be an extra feature to add for
bonus marks or for working with a partner). If you didn't learn about the
Comparable interface, you can find it in your textbook (it's pretty easy to figure
out, but let me know if you need help!).
o validCourseCode() is a private helper method I added to make sure the course
code was valid (4 characters followed by 5 digits). This is something specific to
this sample project but if you had a similar field, you'd probably want to make
one or more validation methods, too.
ď‚· CourseList Class
o There are quite a few constructors here - one default, one that takes a variable-
length argument list of Course objects (which will also accept an array of Course
objects e.g.
CourseList list = new CourseList(new Course(..), new Course(..), new
Course(..), ...);
or
Course[] courses = new Course[10];
// ... fill courses with course objects blah blah blah
// ...
CourseList list = new CourseList(courses);
The last two constructors are for loading the list from a file - one takes a File
object and the other takes a String for the file name/location. The String
constructor simply constructs a File object and then calls CourseList(File).
o Methods like get(), set(), add(), insert(), remove(), clear(), indexOf(), and size()
mostly just call the ArrayList methods by the same name.
o loadFromFile() loads the CourseList with Course objects that are constructed
from records in a specific file.
o writeToFile() takes each Course in the CourseList, creates a record string, and
writes the string to the file. This overwrites the original file contents.
o findCoursesByTitle() will return a list of courses (as a CourseList) that match a
specific title keyword. This is a case-insensitive comparison and does partial
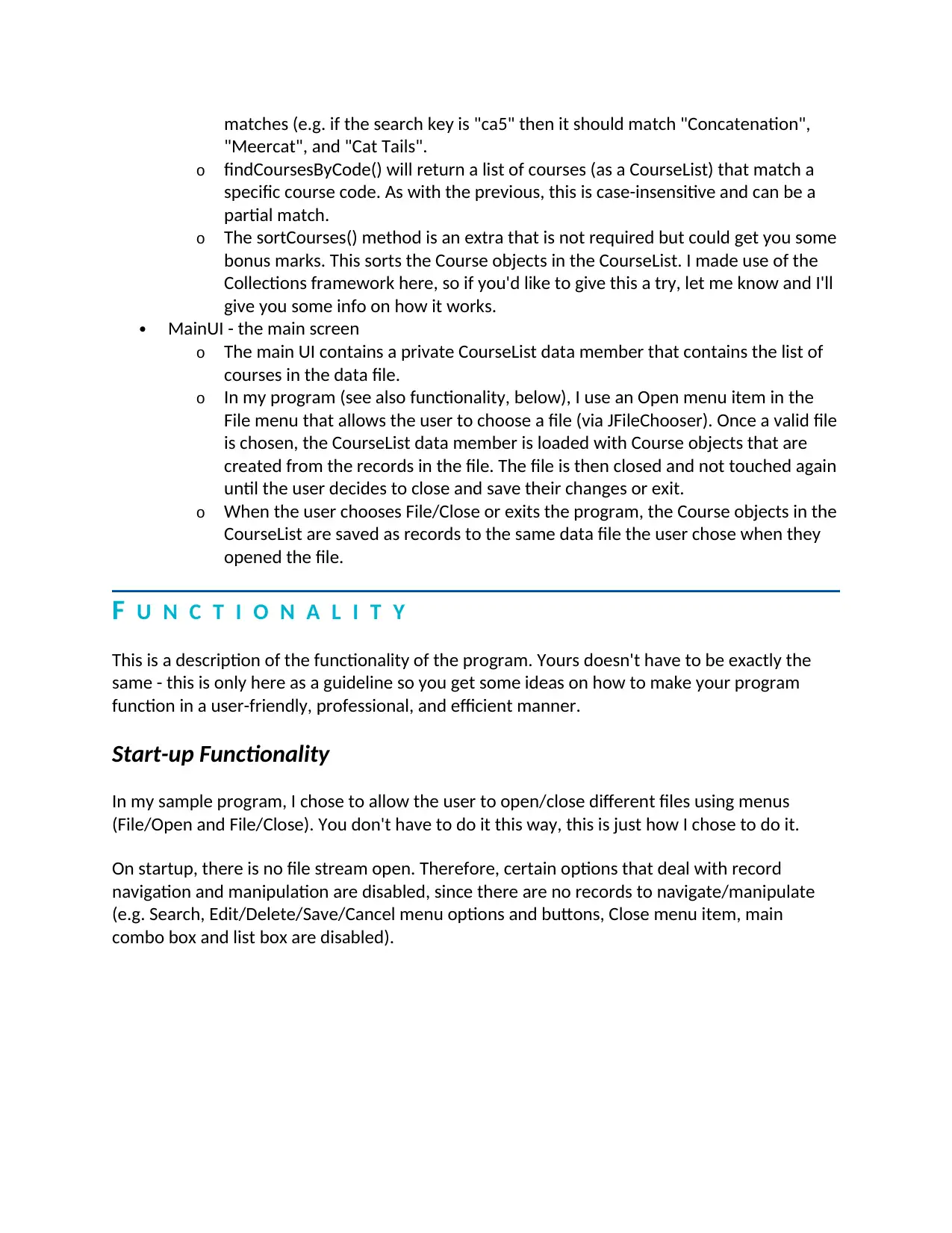
matches (e.g. if the search key is "ca5" then it should match "Concatenation",
"Meercat", and "Cat Tails".
o findCoursesByCode() will return a list of courses (as a CourseList) that match a
specific course code. As with the previous, this is case-insensitive and can be a
partial match.
o The sortCourses() method is an extra that is not required but could get you some
bonus marks. This sorts the Course objects in the CourseList. I made use of the
Collections framework here, so if you'd like to give this a try, let me know and I'll
give you some info on how it works.
ď‚· MainUI - the main screen
o The main UI contains a private CourseList data member that contains the list of
courses in the data file.
o In my program (see also functionality, below), I use an Open menu item in the
File menu that allows the user to choose a file (via JFileChooser). Once a valid file
is chosen, the CourseList data member is loaded with Course objects that are
created from the records in the file. The file is then closed and not touched again
until the user decides to close and save their changes or exit.
o When the user chooses File/Close or exits the program, the Course objects in the
CourseList are saved as records to the same data file the user chose when they
opened the file.
F U N C T I O N A L I T Y
This is a description of the functionality of the program. Yours doesn't have to be exactly the
same - this is only here as a guideline so you get some ideas on how to make your program
function in a user-friendly, professional, and efficient manner.
Start-up Functionality
In my sample program, I chose to allow the user to open/close different files using menus
(File/Open and File/Close). You don't have to do it this way, this is just how I chose to do it.
On startup, there is no file stream open. Therefore, certain options that deal with record
navigation and manipulation are disabled, since there are no records to navigate/manipulate
(e.g. Search, Edit/Delete/Save/Cancel menu options and buttons, Close menu item, main
combo box and list box are disabled).
"Meercat", and "Cat Tails".
o findCoursesByCode() will return a list of courses (as a CourseList) that match a
specific course code. As with the previous, this is case-insensitive and can be a
partial match.
o The sortCourses() method is an extra that is not required but could get you some
bonus marks. This sorts the Course objects in the CourseList. I made use of the
Collections framework here, so if you'd like to give this a try, let me know and I'll
give you some info on how it works.
ď‚· MainUI - the main screen
o The main UI contains a private CourseList data member that contains the list of
courses in the data file.
o In my program (see also functionality, below), I use an Open menu item in the
File menu that allows the user to choose a file (via JFileChooser). Once a valid file
is chosen, the CourseList data member is loaded with Course objects that are
created from the records in the file. The file is then closed and not touched again
until the user decides to close and save their changes or exit.
o When the user chooses File/Close or exits the program, the Course objects in the
CourseList are saved as records to the same data file the user chose when they
opened the file.
F U N C T I O N A L I T Y
This is a description of the functionality of the program. Yours doesn't have to be exactly the
same - this is only here as a guideline so you get some ideas on how to make your program
function in a user-friendly, professional, and efficient manner.
Start-up Functionality
In my sample program, I chose to allow the user to open/close different files using menus
(File/Open and File/Close). You don't have to do it this way, this is just how I chose to do it.
On startup, there is no file stream open. Therefore, certain options that deal with record
navigation and manipulation are disabled, since there are no records to navigate/manipulate
(e.g. Search, Edit/Delete/Save/Cancel menu options and buttons, Close menu item, main
combo box and list box are disabled).
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
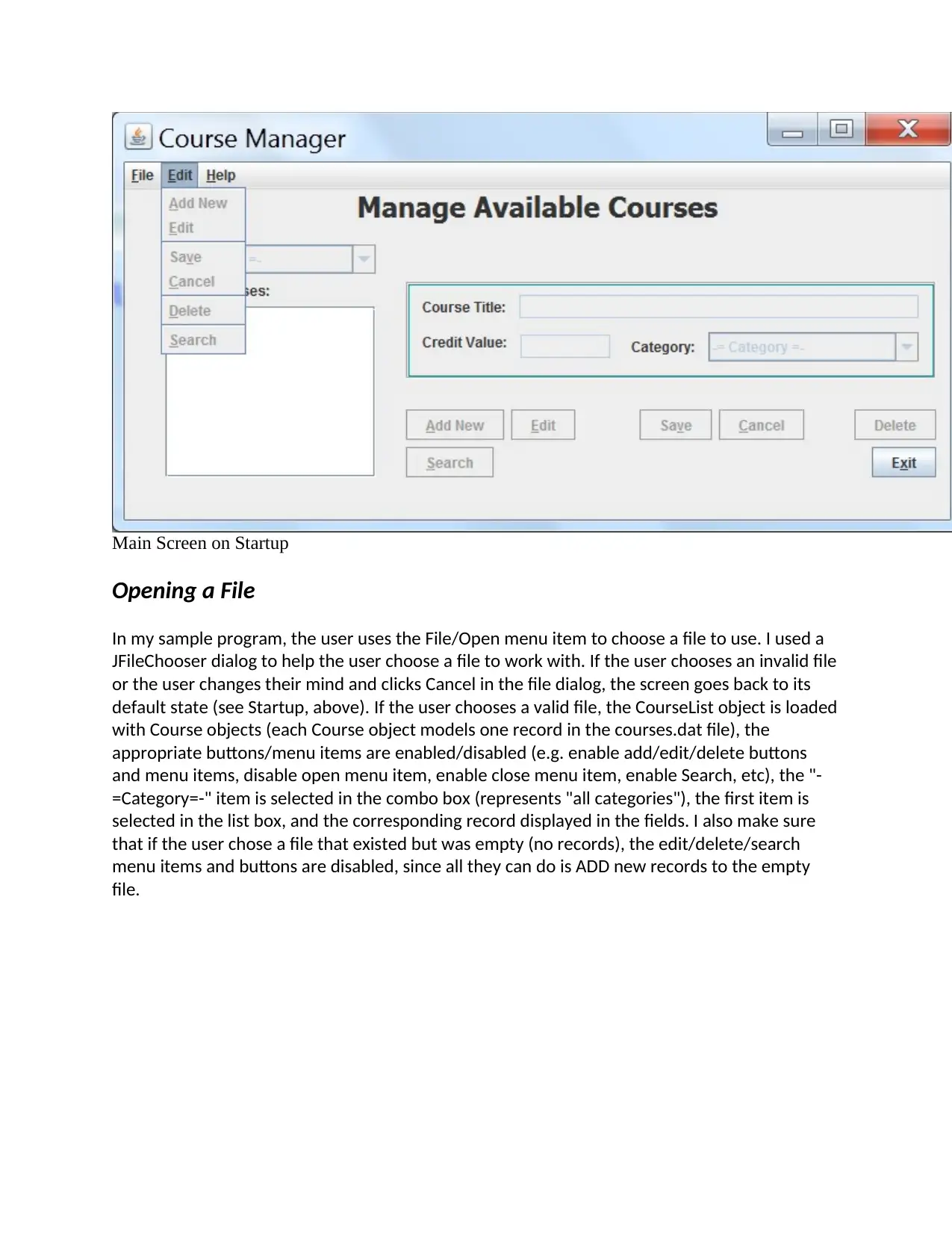
Main Screen on Startup
Opening a File
In my sample program, the user uses the File/Open menu item to choose a file to use. I used a
JFileChooser dialog to help the user choose a file to work with. If the user chooses an invalid file
or the user changes their mind and clicks Cancel in the file dialog, the screen goes back to its
default state (see Startup, above). If the user chooses a valid file, the CourseList object is loaded
with Course objects (each Course object models one record in the courses.dat file), the
appropriate buttons/menu items are enabled/disabled (e.g. enable add/edit/delete buttons
and menu items, disable open menu item, enable close menu item, enable Search, etc), the "-
=Category=-" item is selected in the combo box (represents "all categories"), the first item is
selected in the list box, and the corresponding record displayed in the fields. I also make sure
that if the user chose a file that existed but was empty (no records), the edit/delete/search
menu items and buttons are disabled, since all they can do is ADD new records to the empty
file.
Opening a File
In my sample program, the user uses the File/Open menu item to choose a file to use. I used a
JFileChooser dialog to help the user choose a file to work with. If the user chooses an invalid file
or the user changes their mind and clicks Cancel in the file dialog, the screen goes back to its
default state (see Startup, above). If the user chooses a valid file, the CourseList object is loaded
with Course objects (each Course object models one record in the courses.dat file), the
appropriate buttons/menu items are enabled/disabled (e.g. enable add/edit/delete buttons
and menu items, disable open menu item, enable close menu item, enable Search, etc), the "-
=Category=-" item is selected in the combo box (represents "all categories"), the first item is
selected in the list box, and the corresponding record displayed in the fields. I also make sure
that if the user chose a file that existed but was empty (no records), the edit/delete/search
menu items and buttons are disabled, since all they can do is ADD new records to the empty
file.
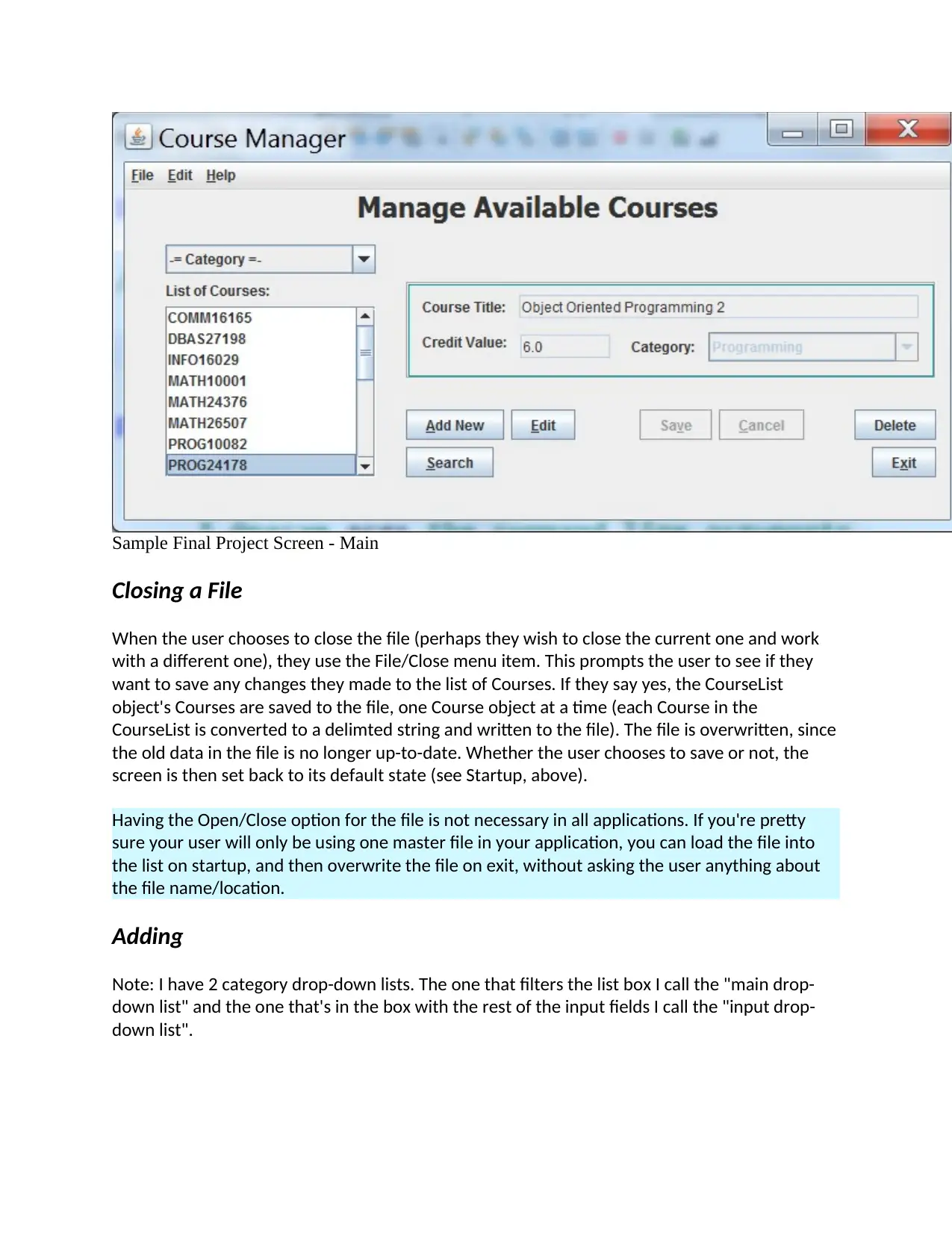
Sample Final Project Screen - Main
Closing a File
When the user chooses to close the file (perhaps they wish to close the current one and work
with a different one), they use the File/Close menu item. This prompts the user to see if they
want to save any changes they made to the list of Courses. If they say yes, the CourseList
object's Courses are saved to the file, one Course object at a time (each Course in the
CourseList is converted to a delimted string and written to the file). The file is overwritten, since
the old data in the file is no longer up-to-date. Whether the user chooses to save or not, the
screen is then set back to its default state (see Startup, above).
Having the Open/Close option for the file is not necessary in all applications. If you're pretty
sure your user will only be using one master file in your application, you can load the file into
the list on startup, and then overwrite the file on exit, without asking the user anything about
the file name/location.
Adding
Note: I have 2 category drop-down lists. The one that filters the list box I call the "main drop-
down list" and the one that's in the box with the rest of the input fields I call the "input drop-
down list".
Closing a File
When the user chooses to close the file (perhaps they wish to close the current one and work
with a different one), they use the File/Close menu item. This prompts the user to see if they
want to save any changes they made to the list of Courses. If they say yes, the CourseList
object's Courses are saved to the file, one Course object at a time (each Course in the
CourseList is converted to a delimted string and written to the file). The file is overwritten, since
the old data in the file is no longer up-to-date. Whether the user chooses to save or not, the
screen is then set back to its default state (see Startup, above).
Having the Open/Close option for the file is not necessary in all applications. If you're pretty
sure your user will only be using one master file in your application, you can load the file into
the list on startup, and then overwrite the file on exit, without asking the user anything about
the file name/location.
Adding
Note: I have 2 category drop-down lists. The one that filters the list box I call the "main drop-
down list" and the one that's in the box with the rest of the input fields I call the "input drop-
down list".
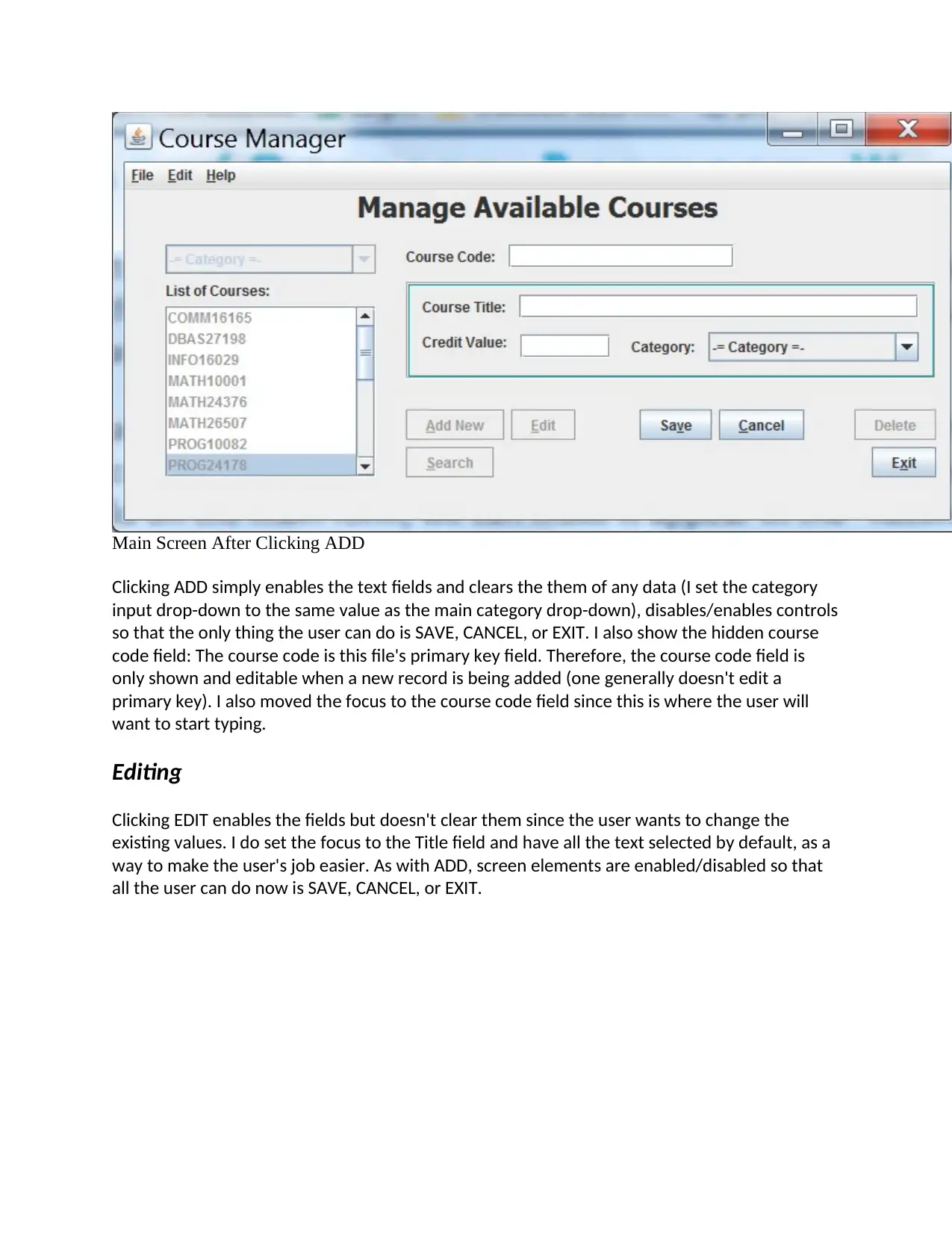
Main Screen After Clicking ADD
Clicking ADD simply enables the text fields and clears the them of any data (I set the category
input drop-down to the same value as the main category drop-down), disables/enables controls
so that the only thing the user can do is SAVE, CANCEL, or EXIT. I also show the hidden course
code field: The course code is this file's primary key field. Therefore, the course code field is
only shown and editable when a new record is being added (one generally doesn't edit a
primary key). I also moved the focus to the course code field since this is where the user will
want to start typing.
Editing
Clicking EDIT enables the fields but doesn't clear them since the user wants to change the
existing values. I do set the focus to the Title field and have all the text selected by default, as a
way to make the user's job easier. As with ADD, screen elements are enabled/disabled so that
all the user can do now is SAVE, CANCEL, or EXIT.
Clicking ADD simply enables the text fields and clears the them of any data (I set the category
input drop-down to the same value as the main category drop-down), disables/enables controls
so that the only thing the user can do is SAVE, CANCEL, or EXIT. I also show the hidden course
code field: The course code is this file's primary key field. Therefore, the course code field is
only shown and editable when a new record is being added (one generally doesn't edit a
primary key). I also moved the focus to the course code field since this is where the user will
want to start typing.
Editing
Clicking EDIT enables the fields but doesn't clear them since the user wants to change the
existing values. I do set the focus to the Title field and have all the text selected by default, as a
way to make the user's job easier. As with ADD, screen elements are enabled/disabled so that
all the user can do now is SAVE, CANCEL, or EXIT.
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
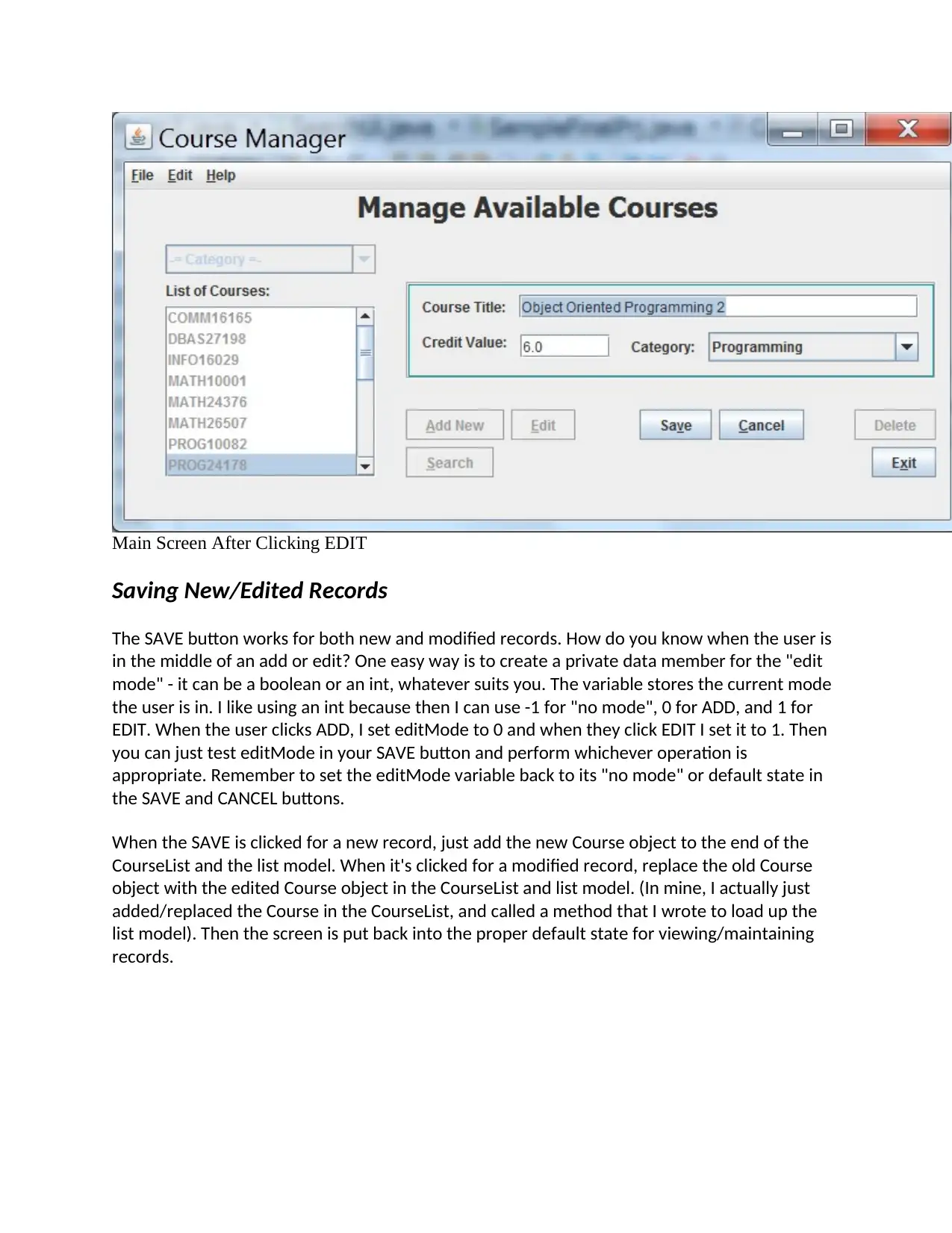
Main Screen After Clicking EDIT
Saving New/Edited Records
The SAVE button works for both new and modified records. How do you know when the user is
in the middle of an add or edit? One easy way is to create a private data member for the "edit
mode" - it can be a boolean or an int, whatever suits you. The variable stores the current mode
the user is in. I like using an int because then I can use -1 for "no mode", 0 for ADD, and 1 for
EDIT. When the user clicks ADD, I set editMode to 0 and when they click EDIT I set it to 1. Then
you can just test editMode in your SAVE button and perform whichever operation is
appropriate. Remember to set the editMode variable back to its "no mode" or default state in
the SAVE and CANCEL buttons.
When the SAVE is clicked for a new record, just add the new Course object to the end of the
CourseList and the list model. When it's clicked for a modified record, replace the old Course
object with the edited Course object in the CourseList and list model. (In mine, I actually just
added/replaced the Course in the CourseList, and called a method that I wrote to load up the
list model). Then the screen is put back into the proper default state for viewing/maintaining
records.
Saving New/Edited Records
The SAVE button works for both new and modified records. How do you know when the user is
in the middle of an add or edit? One easy way is to create a private data member for the "edit
mode" - it can be a boolean or an int, whatever suits you. The variable stores the current mode
the user is in. I like using an int because then I can use -1 for "no mode", 0 for ADD, and 1 for
EDIT. When the user clicks ADD, I set editMode to 0 and when they click EDIT I set it to 1. Then
you can just test editMode in your SAVE button and perform whichever operation is
appropriate. Remember to set the editMode variable back to its "no mode" or default state in
the SAVE and CANCEL buttons.
When the SAVE is clicked for a new record, just add the new Course object to the end of the
CourseList and the list model. When it's clicked for a modified record, replace the old Course
object with the edited Course object in the CourseList and list model. (In mine, I actually just
added/replaced the Course in the CourseList, and called a method that I wrote to load up the
list model). Then the screen is put back into the proper default state for viewing/maintaining
records.
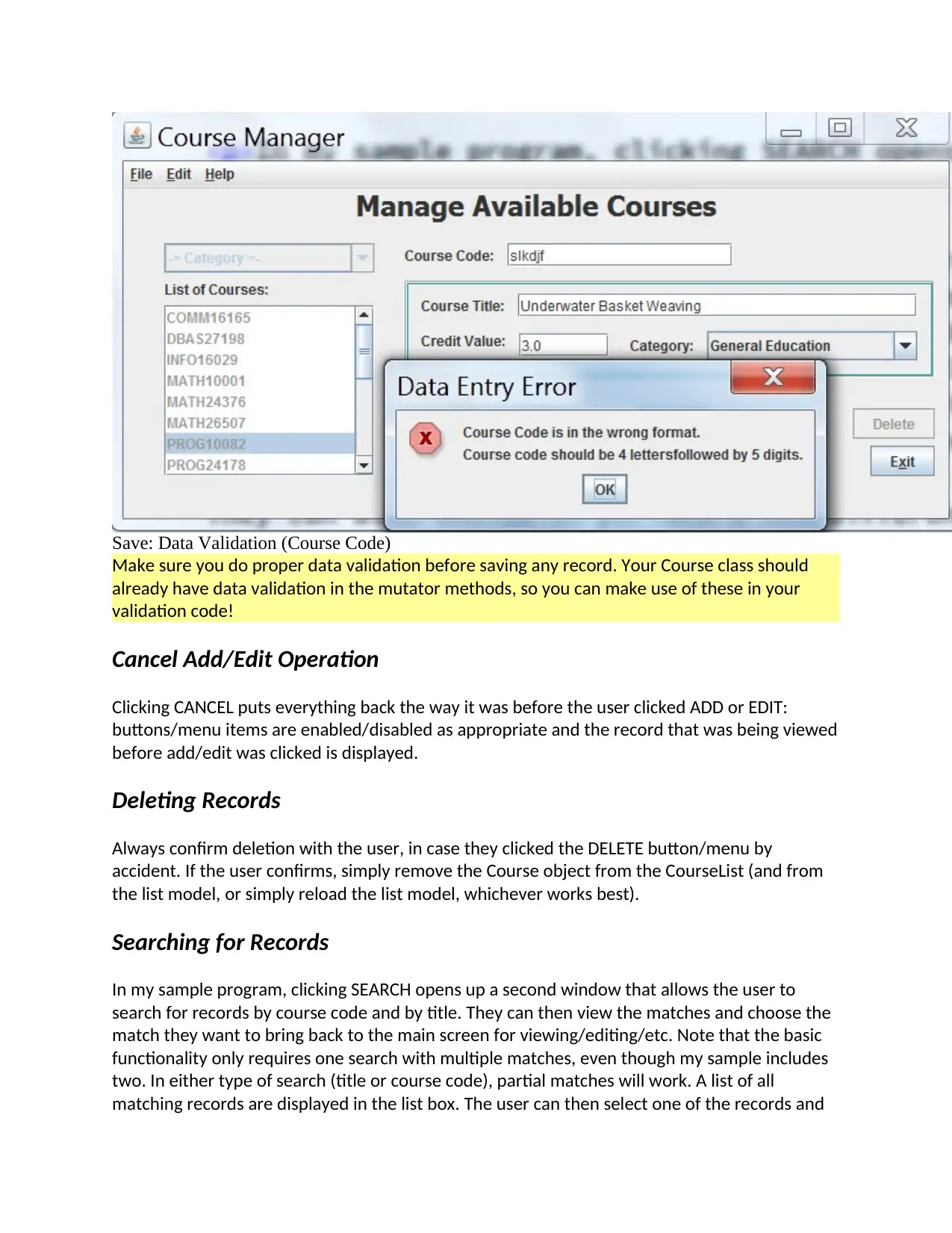
Save: Data Validation (Course Code)
Make sure you do proper data validation before saving any record. Your Course class should
already have data validation in the mutator methods, so you can make use of these in your
validation code!
Cancel Add/Edit Operation
Clicking CANCEL puts everything back the way it was before the user clicked ADD or EDIT:
buttons/menu items are enabled/disabled as appropriate and the record that was being viewed
before add/edit was clicked is displayed.
Deleting Records
Always confirm deletion with the user, in case they clicked the DELETE button/menu by
accident. If the user confirms, simply remove the Course object from the CourseList (and from
the list model, or simply reload the list model, whichever works best).
Searching for Records
In my sample program, clicking SEARCH opens up a second window that allows the user to
search for records by course code and by title. They can then view the matches and choose the
match they want to bring back to the main screen for viewing/editing/etc. Note that the basic
functionality only requires one search with multiple matches, even though my sample includes
two. In either type of search (title or course code), partial matches will work. A list of all
matching records are displayed in the list box. The user can then select one of the records and
Make sure you do proper data validation before saving any record. Your Course class should
already have data validation in the mutator methods, so you can make use of these in your
validation code!
Cancel Add/Edit Operation
Clicking CANCEL puts everything back the way it was before the user clicked ADD or EDIT:
buttons/menu items are enabled/disabled as appropriate and the record that was being viewed
before add/edit was clicked is displayed.
Deleting Records
Always confirm deletion with the user, in case they clicked the DELETE button/menu by
accident. If the user confirms, simply remove the Course object from the CourseList (and from
the list model, or simply reload the list model, whichever works best).
Searching for Records
In my sample program, clicking SEARCH opens up a second window that allows the user to
search for records by course code and by title. They can then view the matches and choose the
match they want to bring back to the main screen for viewing/editing/etc. Note that the basic
functionality only requires one search with multiple matches, even though my sample includes
two. In either type of search (title or course code), partial matches will work. A list of all
matching records are displayed in the list box. The user can then select one of the records and
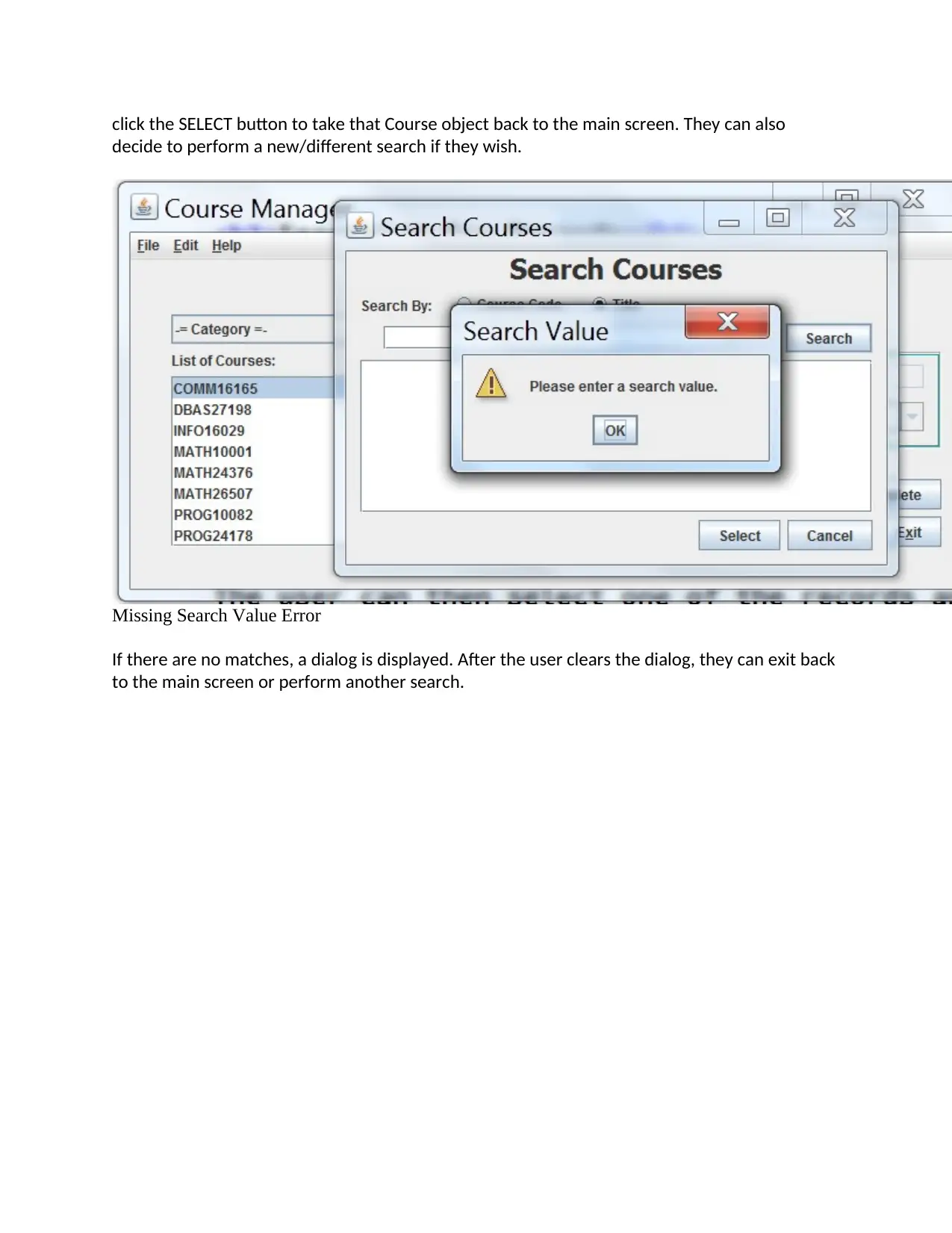
click the SELECT button to take that Course object back to the main screen. They can also
decide to perform a new/different search if they wish.
Missing Search Value Error
If there are no matches, a dialog is displayed. After the user clears the dialog, they can exit back
to the main screen or perform another search.
decide to perform a new/different search if they wish.
Missing Search Value Error
If there are no matches, a dialog is displayed. After the user clears the dialog, they can exit back
to the main screen or perform another search.
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
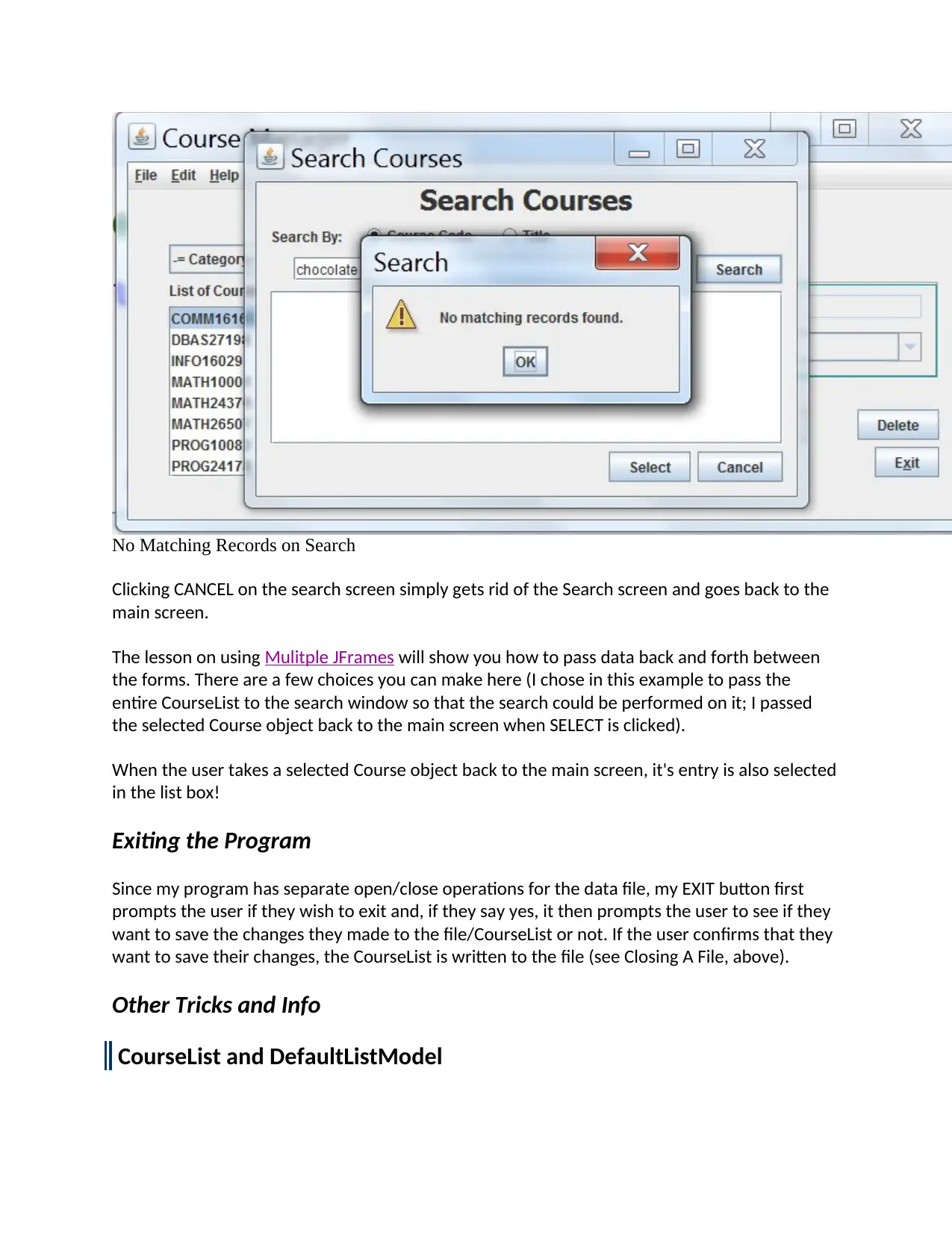
No Matching Records on Search
Clicking CANCEL on the search screen simply gets rid of the Search screen and goes back to the
main screen.
The lesson on using Mulitple JFrames will show you how to pass data back and forth between
the forms. There are a few choices you can make here (I chose in this example to pass the
entire CourseList to the search window so that the search could be performed on it; I passed
the selected Course object back to the main screen when SELECT is clicked).
When the user takes a selected Course object back to the main screen, it's entry is also selected
in the list box!
Exiting the Program
Since my program has separate open/close operations for the data file, my EXIT button first
prompts the user if they wish to exit and, if they say yes, it then prompts the user to see if they
want to save the changes they made to the file/CourseList or not. If the user confirms that they
want to save their changes, the CourseList is written to the file (see Closing A File, above).
Other Tricks and Info
CourseList and DefaultListModel
Clicking CANCEL on the search screen simply gets rid of the Search screen and goes back to the
main screen.
The lesson on using Mulitple JFrames will show you how to pass data back and forth between
the forms. There are a few choices you can make here (I chose in this example to pass the
entire CourseList to the search window so that the search could be performed on it; I passed
the selected Course object back to the main screen when SELECT is clicked).
When the user takes a selected Course object back to the main screen, it's entry is also selected
in the list box!
Exiting the Program
Since my program has separate open/close operations for the data file, my EXIT button first
prompts the user if they wish to exit and, if they say yes, it then prompts the user to see if they
want to save the changes they made to the file/CourseList or not. If the user confirms that they
want to save their changes, the CourseList is written to the file (see Closing A File, above).
Other Tricks and Info
CourseList and DefaultListModel
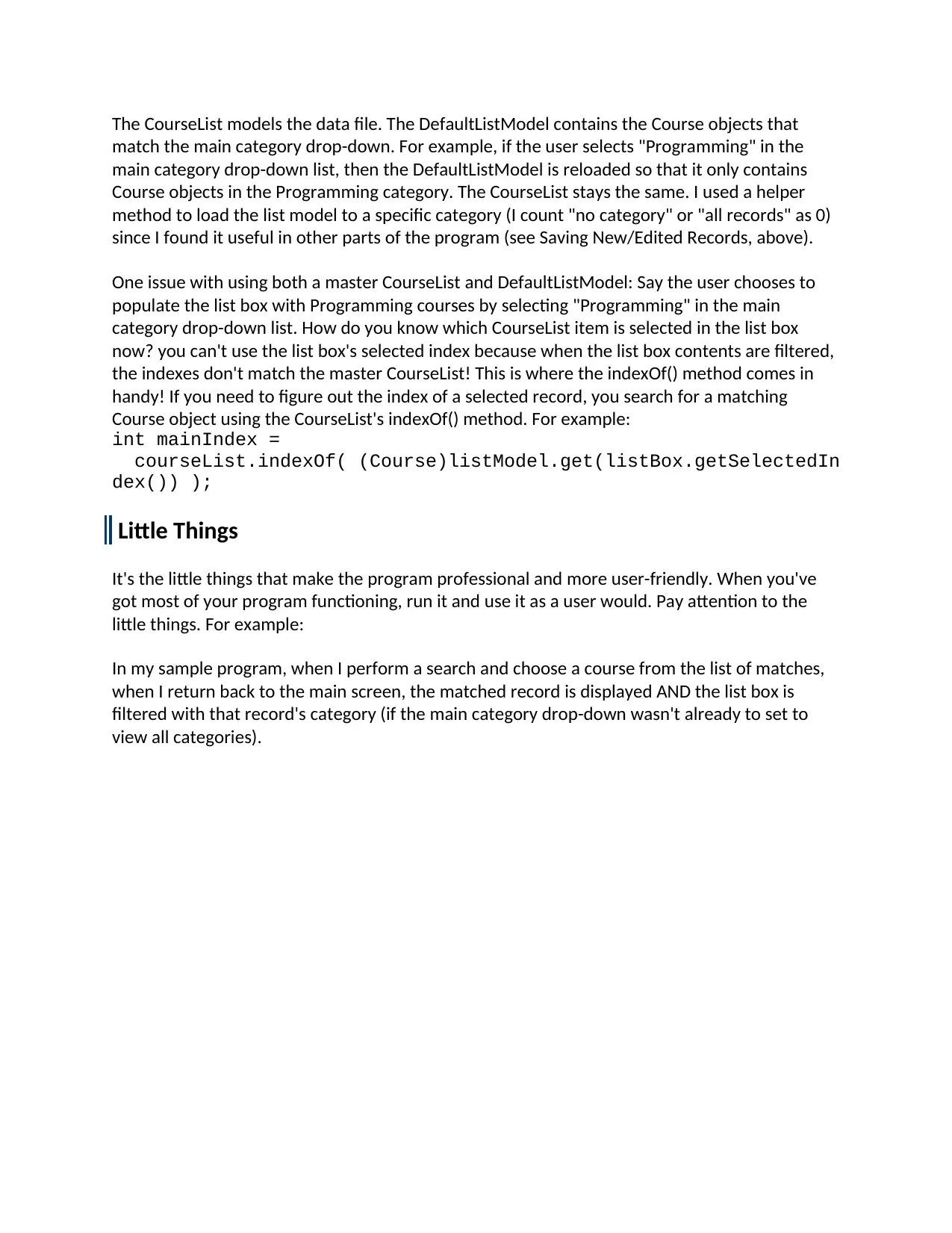
The CourseList models the data file. The DefaultListModel contains the Course objects that
match the main category drop-down. For example, if the user selects "Programming" in the
main category drop-down list, then the DefaultListModel is reloaded so that it only contains
Course objects in the Programming category. The CourseList stays the same. I used a helper
method to load the list model to a specific category (I count "no category" or "all records" as 0)
since I found it useful in other parts of the program (see Saving New/Edited Records, above).
One issue with using both a master CourseList and DefaultListModel: Say the user chooses to
populate the list box with Programming courses by selecting "Programming" in the main
category drop-down list. How do you know which CourseList item is selected in the list box
now? you can't use the list box's selected index because when the list box contents are filtered,
the indexes don't match the master CourseList! This is where the indexOf() method comes in
handy! If you need to figure out the index of a selected record, you search for a matching
Course object using the CourseList's indexOf() method. For example:
int mainIndex =
courseList.indexOf( (Course)listModel.get(listBox.getSelectedIn
dex()) );
Little Things
It's the little things that make the program professional and more user-friendly. When you've
got most of your program functioning, run it and use it as a user would. Pay attention to the
little things. For example:
In my sample program, when I perform a search and choose a course from the list of matches,
when I return back to the main screen, the matched record is displayed AND the list box is
filtered with that record's category (if the main category drop-down wasn't already to set to
view all categories).
match the main category drop-down. For example, if the user selects "Programming" in the
main category drop-down list, then the DefaultListModel is reloaded so that it only contains
Course objects in the Programming category. The CourseList stays the same. I used a helper
method to load the list model to a specific category (I count "no category" or "all records" as 0)
since I found it useful in other parts of the program (see Saving New/Edited Records, above).
One issue with using both a master CourseList and DefaultListModel: Say the user chooses to
populate the list box with Programming courses by selecting "Programming" in the main
category drop-down list. How do you know which CourseList item is selected in the list box
now? you can't use the list box's selected index because when the list box contents are filtered,
the indexes don't match the master CourseList! This is where the indexOf() method comes in
handy! If you need to figure out the index of a selected record, you search for a matching
Course object using the CourseList's indexOf() method. For example:
int mainIndex =
courseList.indexOf( (Course)listModel.get(listBox.getSelectedIn
dex()) );
Little Things
It's the little things that make the program professional and more user-friendly. When you've
got most of your program functioning, run it and use it as a user would. Pay attention to the
little things. For example:
In my sample program, when I perform a search and choose a course from the list of matches,
when I return back to the main screen, the matched record is displayed AND the list box is
filtered with that record's category (if the main category drop-down wasn't already to set to
view all categories).
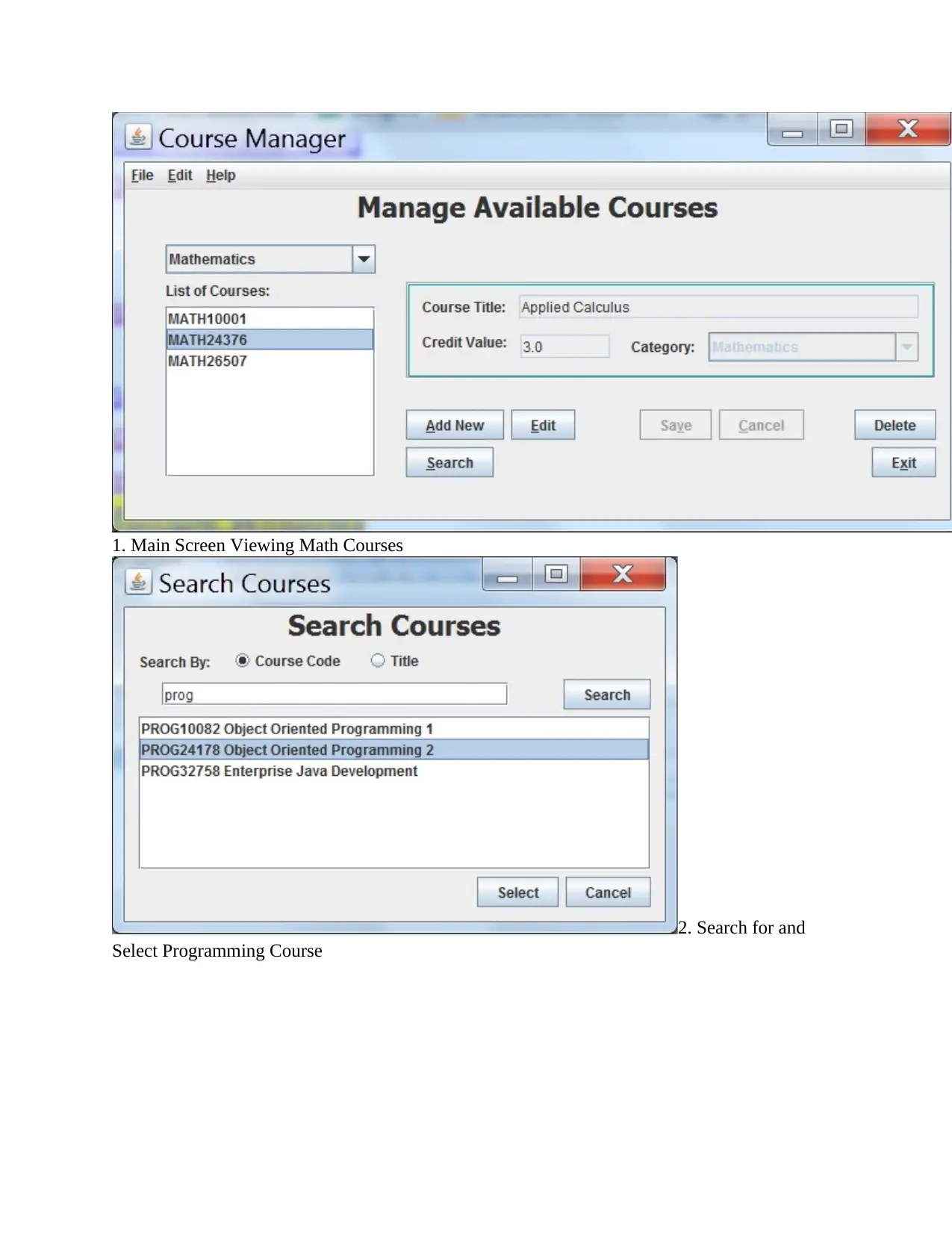
1. Main Screen Viewing Math Courses
2. Search for and
Select Programming Course
2. Search for and
Select Programming Course
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
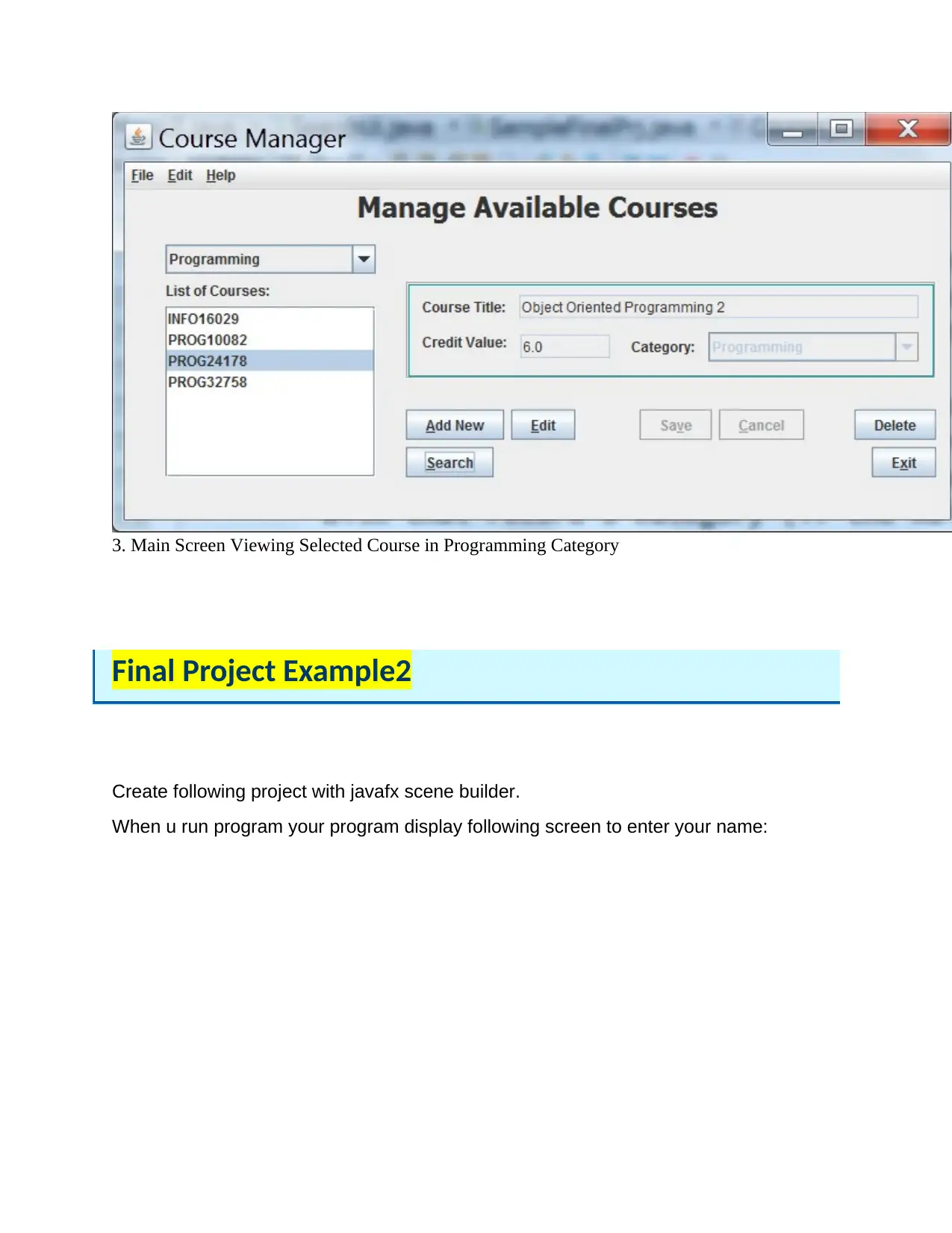
3. Main Screen Viewing Selected Course in Programming Category
Final Project Example2
Create following project with javafx scene builder.
When u run program your program display following screen to enter your name:
Final Project Example2
Create following project with javafx scene builder.
When u run program your program display following screen to enter your name:
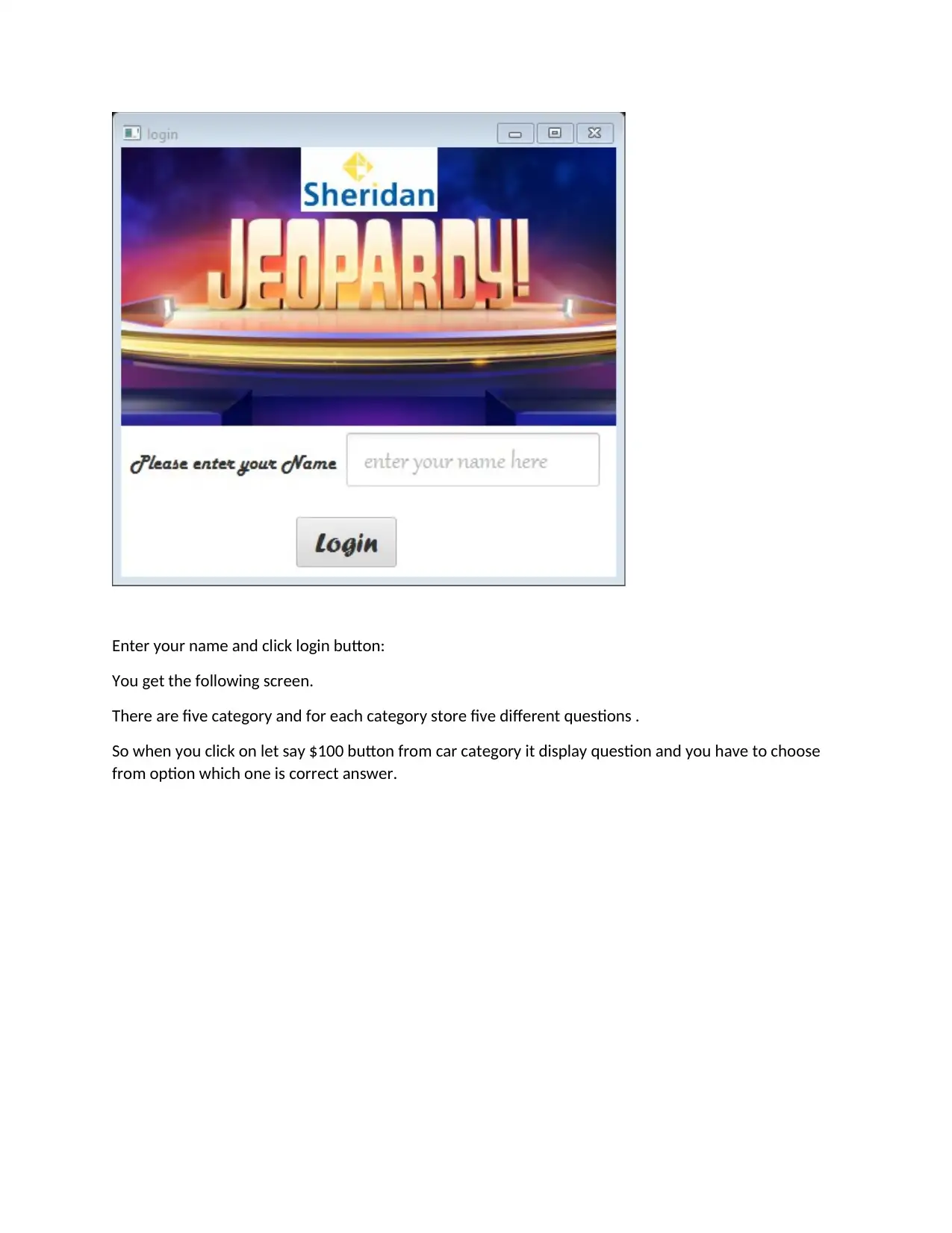
Enter your name and click login button:
You get the following screen.
There are five category and for each category store five different questions .
So when you click on let say $100 button from car category it display question and you have to choose
from option which one is correct answer.
You get the following screen.
There are five category and for each category store five different questions .
So when you click on let say $100 button from car category it display question and you have to choose
from option which one is correct answer.
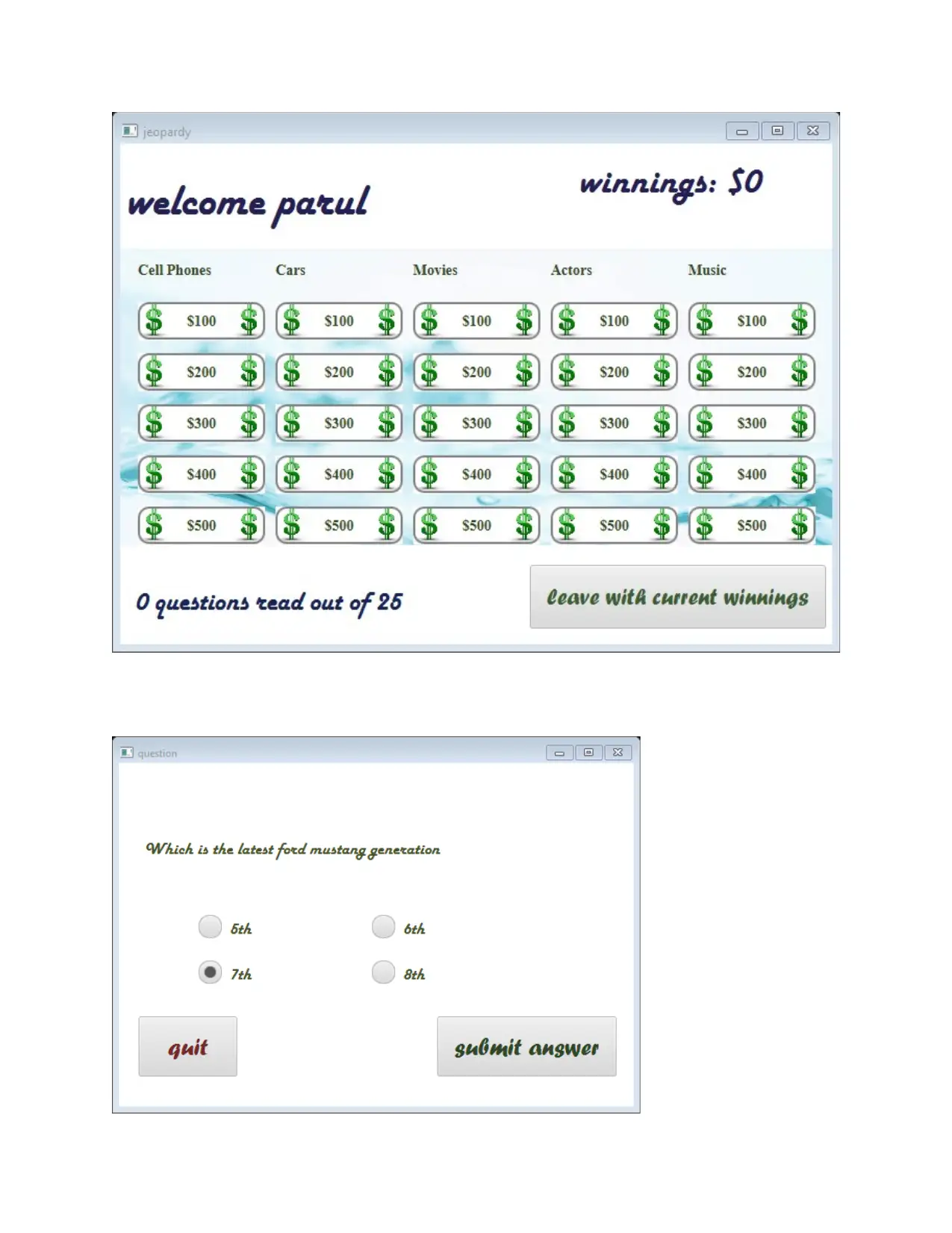
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
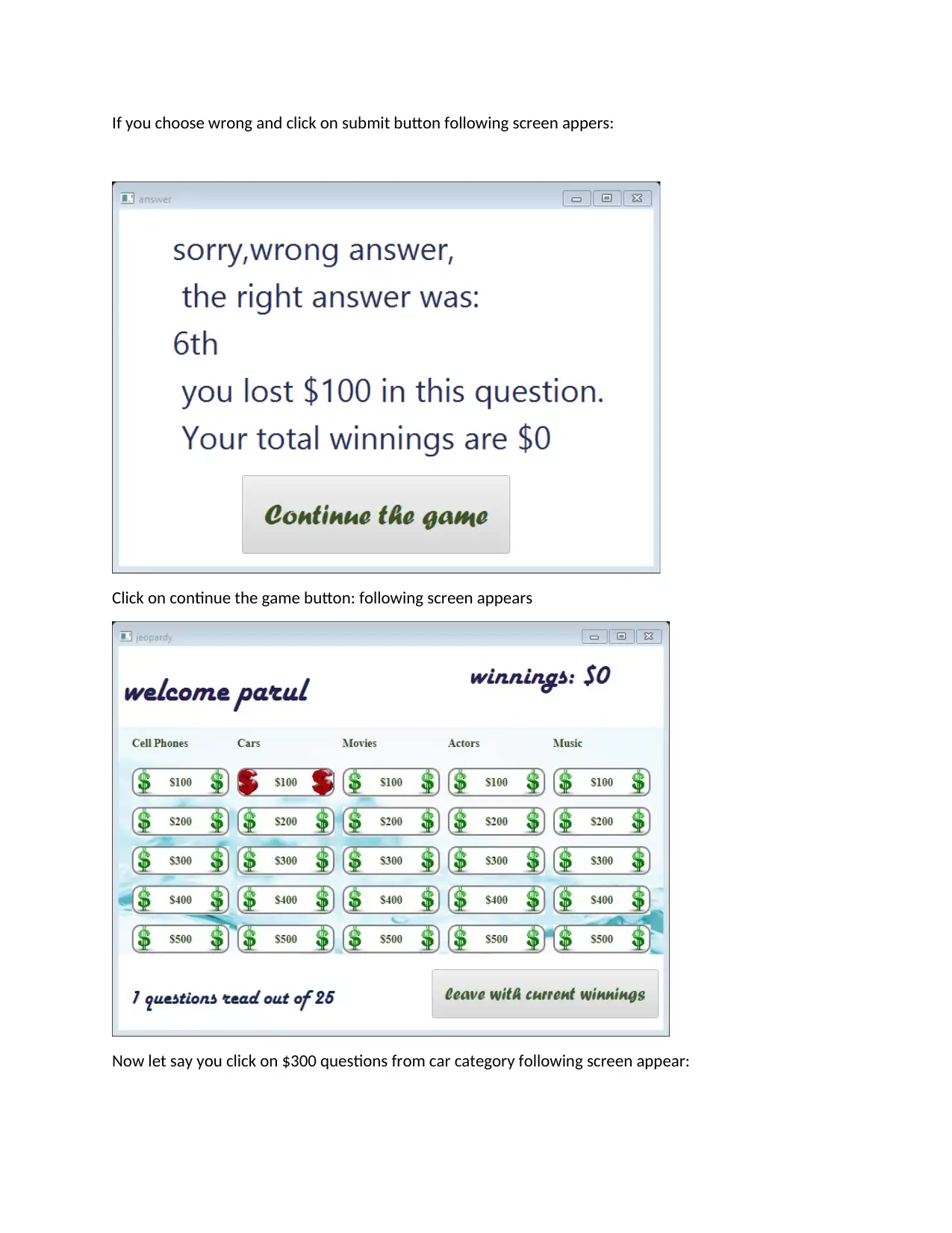
If you choose wrong and click on submit button following screen appers:
Click on continue the game button: following screen appears
Now let say you click on $300 questions from car category following screen appear:
Click on continue the game button: following screen appears
Now let say you click on $300 questions from car category following screen appear:
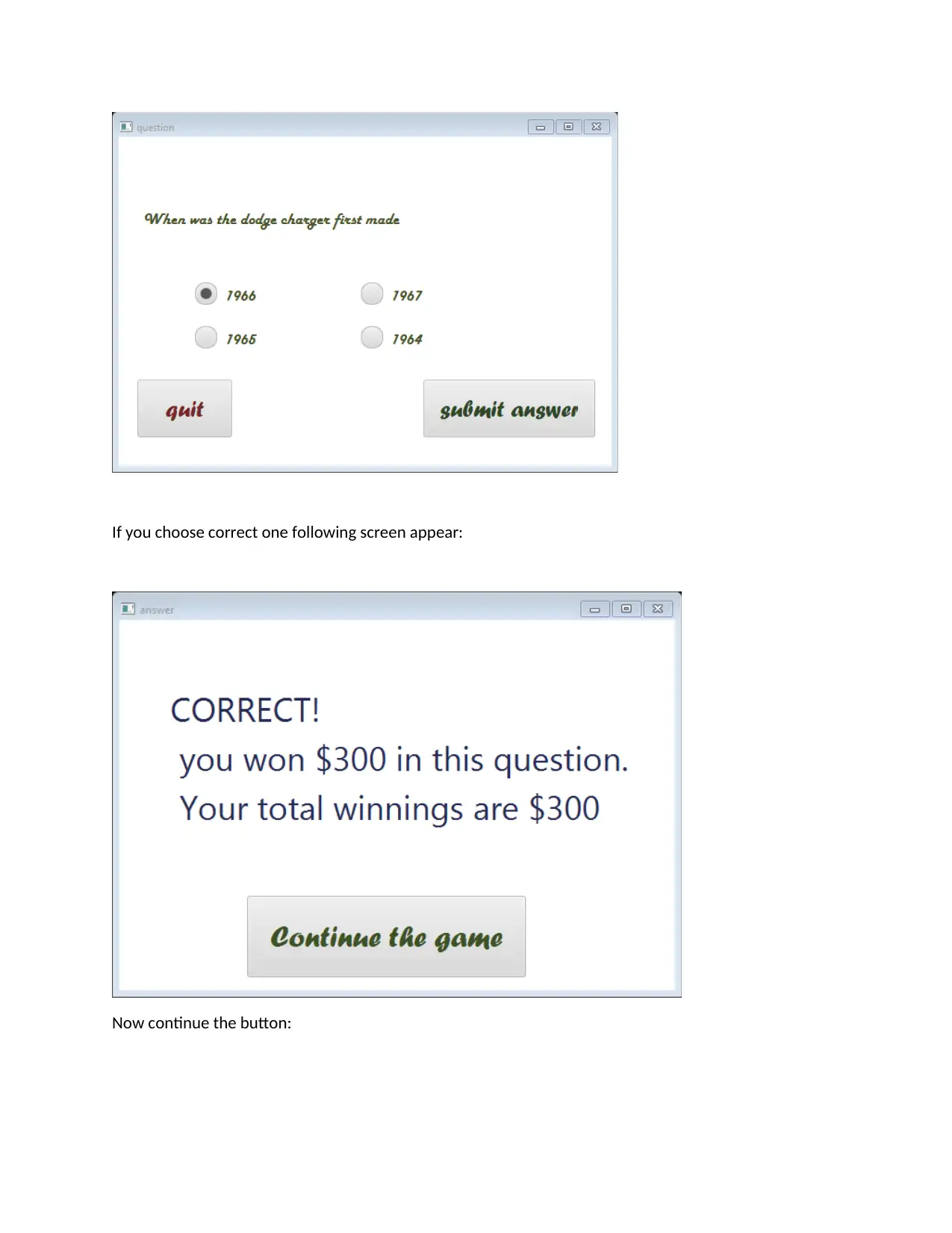
If you choose correct one following screen appear:
Now continue the button:
Now continue the button:
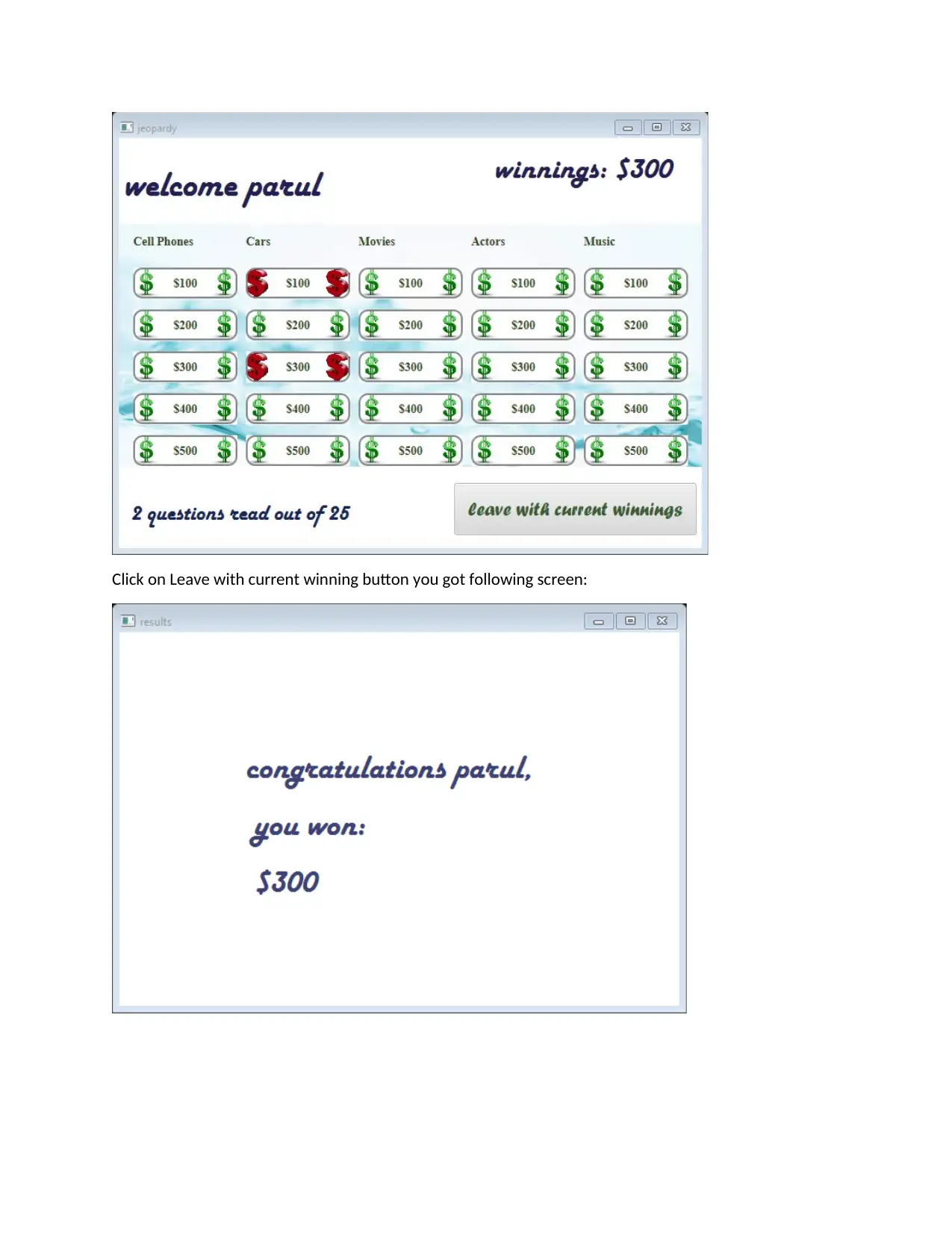
Click on Leave with current winning button you got following screen:
1 out of 22

Your All-in-One AI-Powered Toolkit for Academic Success.
 +13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024  |  Zucol Services PVT LTD  |  All rights reserved.