JHC System Design: Detailed Analysis of Appointment Reminder Subsystem
VerifiedAdded on 2020/05/16
|12
|2024
|77
Project
AI Summary
This project report details the design of the JHC Appointment Reminder subsystem, a crucial component of James Healthcare Centre's IT infrastructure. The system is designed using an object-oriented approach and implemented in Java. It focuses on storing patient appointment details, including name, contact information, appointment date and time, and provider's name. The primary function of the system is to identify and display appointments scheduled within the next two days, enabling administrators to send reminders. The report includes detailed descriptions of the system's architecture, including the use of classes, objects, and methods like setDetails() and upcomingApps(). It also covers data structures, variable descriptions, and the storage mechanism. The project considers future improvements, such as database integration and SMS reminder functionality. The report includes testing and output details, and it concludes with a bibliography of relevant sources. This project highlights the practical application of object-oriented programming principles in designing a healthcare information system.
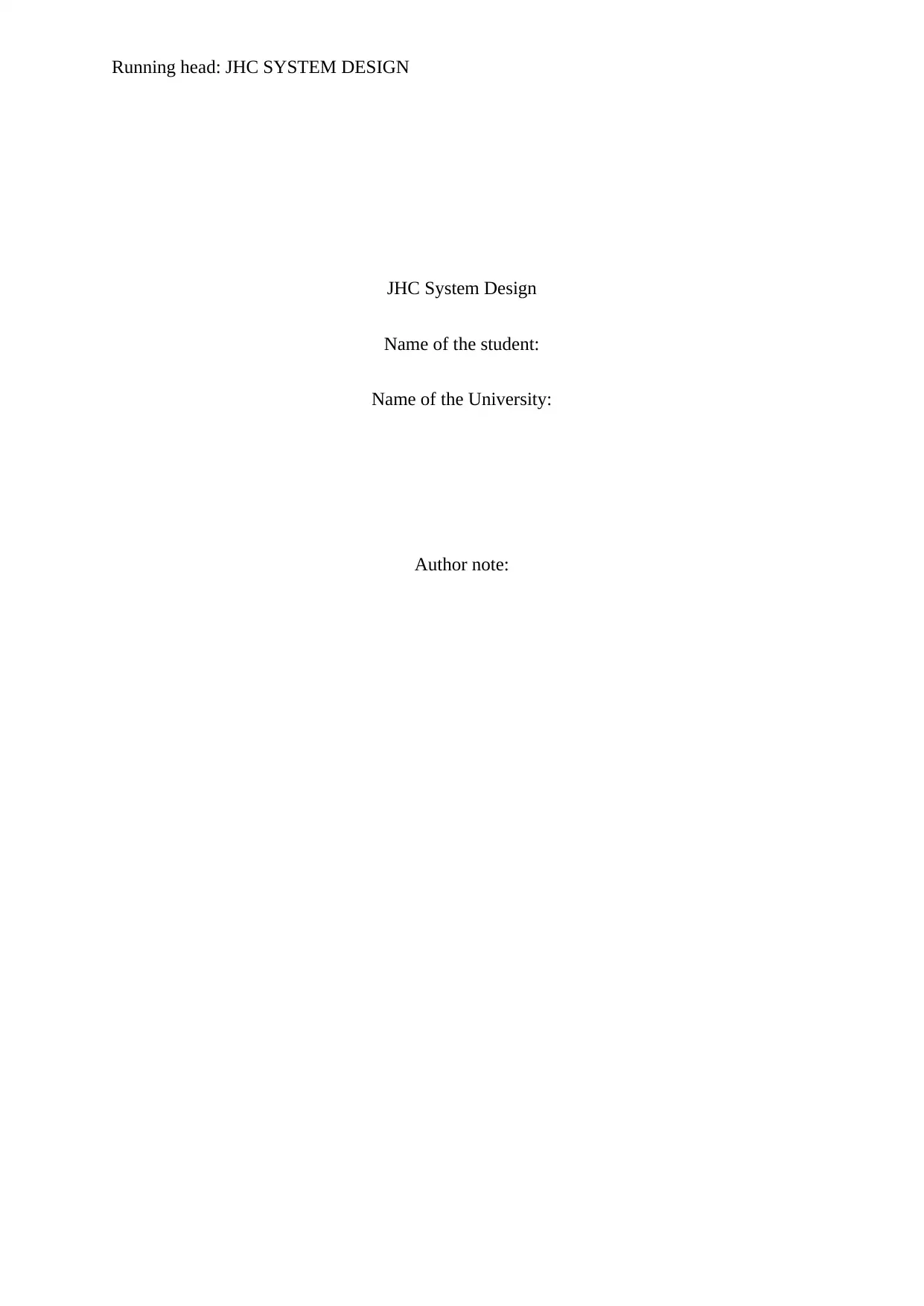
Running head: JHC SYSTEM DESIGN
JHC System Design
Name of the student:
Name of the University:
Author note:
JHC System Design
Name of the student:
Name of the University:
Author note:
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
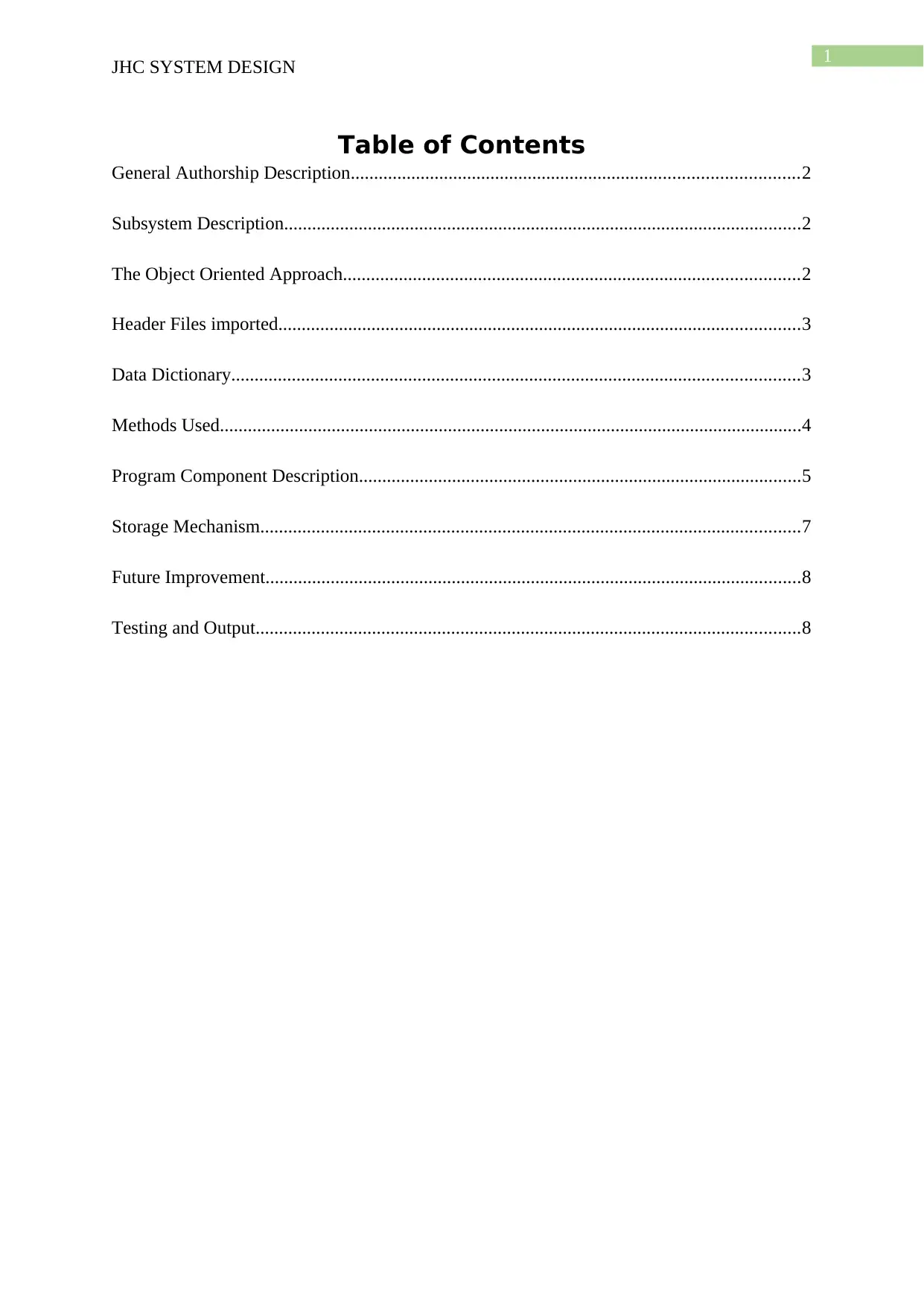
1
JHC SYSTEM DESIGN
Table of Contents
General Authorship Description................................................................................................2
Subsystem Description...............................................................................................................2
The Object Oriented Approach..................................................................................................2
Header Files imported................................................................................................................3
Data Dictionary..........................................................................................................................3
Methods Used.............................................................................................................................4
Program Component Description...............................................................................................5
Storage Mechanism....................................................................................................................7
Future Improvement...................................................................................................................8
Testing and Output.....................................................................................................................8
JHC SYSTEM DESIGN
Table of Contents
General Authorship Description................................................................................................2
Subsystem Description...............................................................................................................2
The Object Oriented Approach..................................................................................................2
Header Files imported................................................................................................................3
Data Dictionary..........................................................................................................................3
Methods Used.............................................................................................................................4
Program Component Description...............................................................................................5
Storage Mechanism....................................................................................................................7
Future Improvement...................................................................................................................8
Testing and Output.....................................................................................................................8
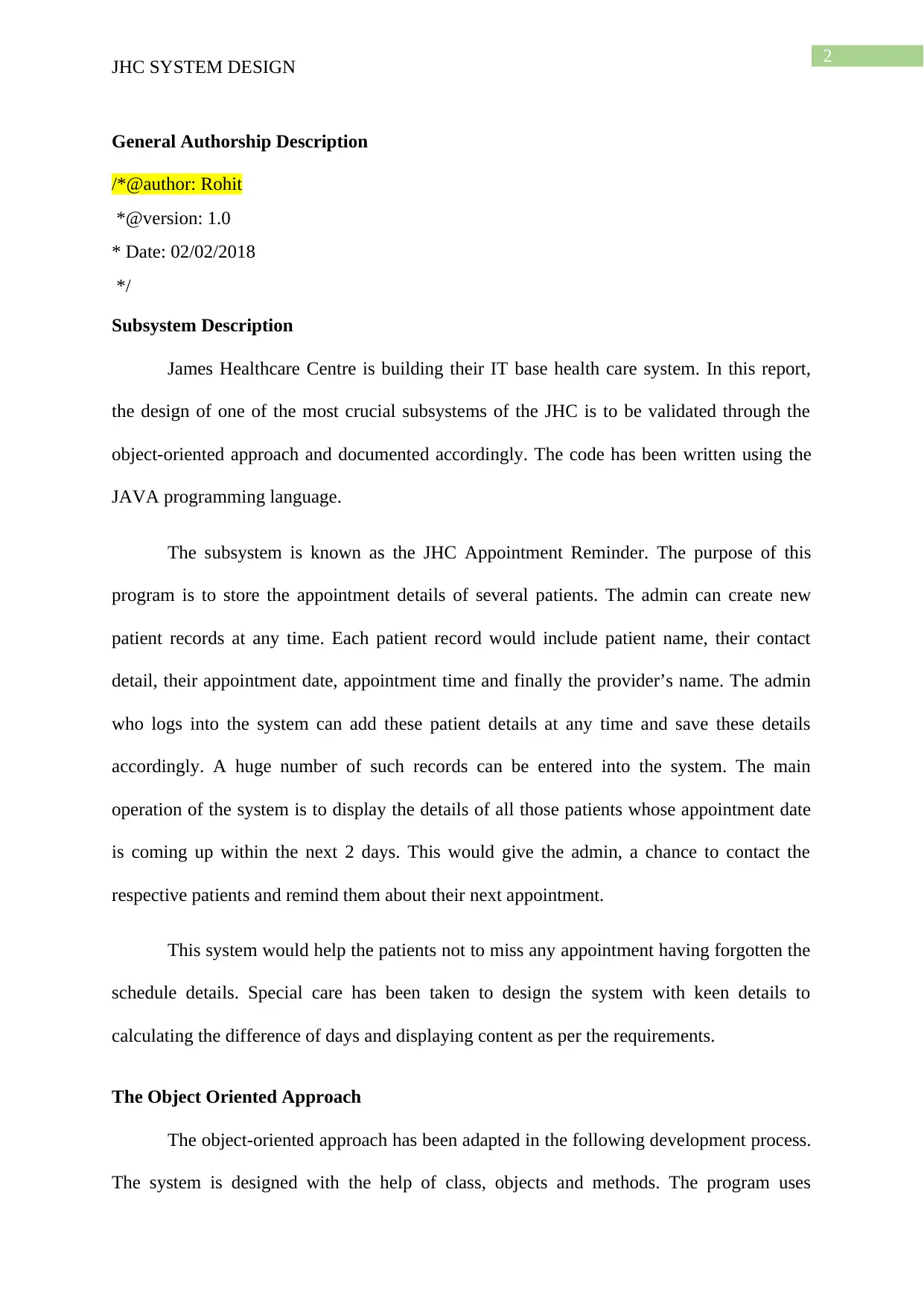
2
JHC SYSTEM DESIGN
General Authorship Description
/*@author: Rohit
*@version: 1.0
* Date: 02/02/2018
*/
Subsystem Description
James Healthcare Centre is building their IT base health care system. In this report,
the design of one of the most crucial subsystems of the JHC is to be validated through the
object-oriented approach and documented accordingly. The code has been written using the
JAVA programming language.
The subsystem is known as the JHC Appointment Reminder. The purpose of this
program is to store the appointment details of several patients. The admin can create new
patient records at any time. Each patient record would include patient name, their contact
detail, their appointment date, appointment time and finally the provider’s name. The admin
who logs into the system can add these patient details at any time and save these details
accordingly. A huge number of such records can be entered into the system. The main
operation of the system is to display the details of all those patients whose appointment date
is coming up within the next 2 days. This would give the admin, a chance to contact the
respective patients and remind them about their next appointment.
This system would help the patients not to miss any appointment having forgotten the
schedule details. Special care has been taken to design the system with keen details to
calculating the difference of days and displaying content as per the requirements.
The Object Oriented Approach
The object-oriented approach has been adapted in the following development process.
The system is designed with the help of class, objects and methods. The program uses
JHC SYSTEM DESIGN
General Authorship Description
/*@author: Rohit
*@version: 1.0
* Date: 02/02/2018
*/
Subsystem Description
James Healthcare Centre is building their IT base health care system. In this report,
the design of one of the most crucial subsystems of the JHC is to be validated through the
object-oriented approach and documented accordingly. The code has been written using the
JAVA programming language.
The subsystem is known as the JHC Appointment Reminder. The purpose of this
program is to store the appointment details of several patients. The admin can create new
patient records at any time. Each patient record would include patient name, their contact
detail, their appointment date, appointment time and finally the provider’s name. The admin
who logs into the system can add these patient details at any time and save these details
accordingly. A huge number of such records can be entered into the system. The main
operation of the system is to display the details of all those patients whose appointment date
is coming up within the next 2 days. This would give the admin, a chance to contact the
respective patients and remind them about their next appointment.
This system would help the patients not to miss any appointment having forgotten the
schedule details. Special care has been taken to design the system with keen details to
calculating the difference of days and displaying content as per the requirements.
The Object Oriented Approach
The object-oriented approach has been adapted in the following development process.
The system is designed with the help of class, objects and methods. The program uses
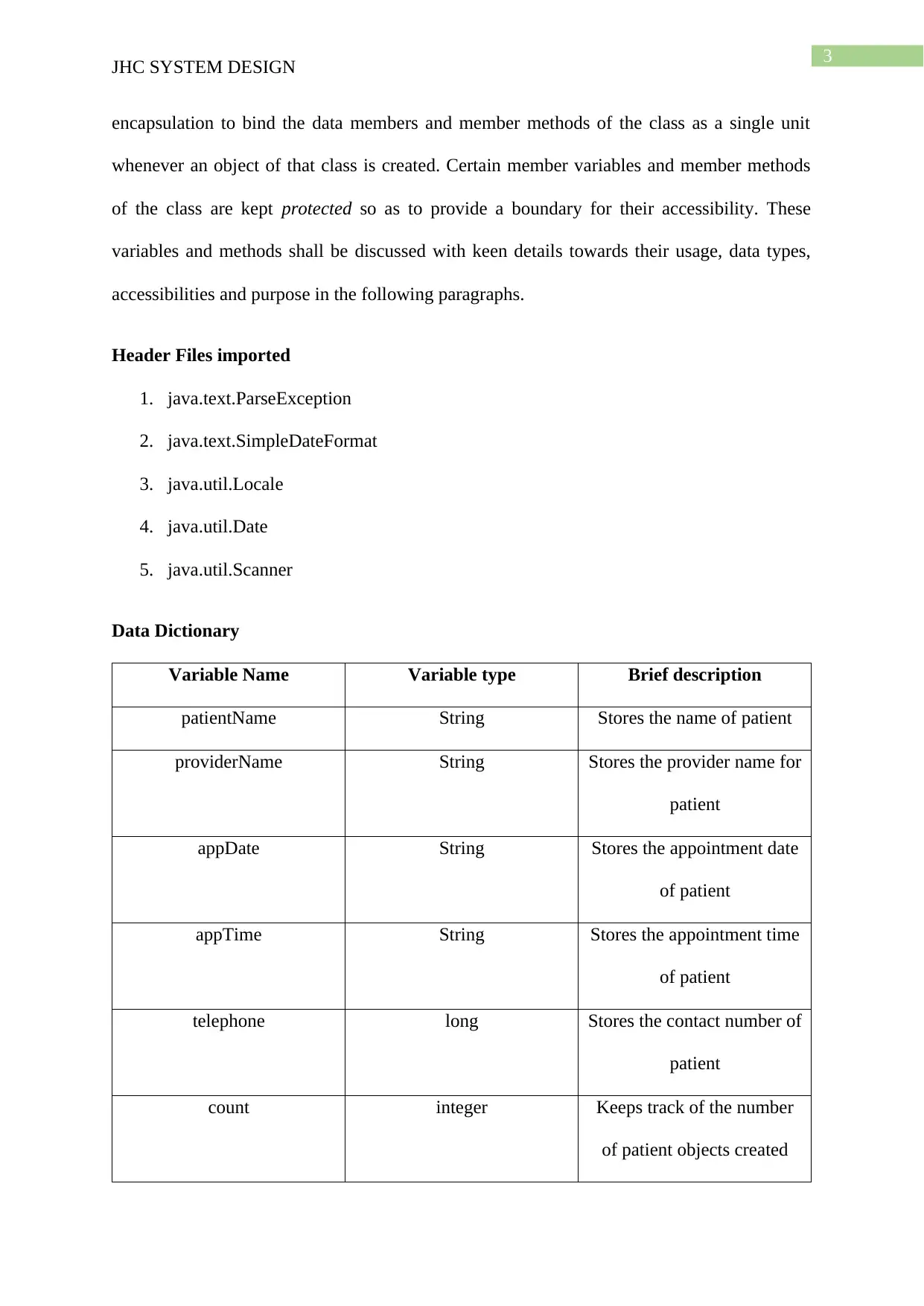
3
JHC SYSTEM DESIGN
encapsulation to bind the data members and member methods of the class as a single unit
whenever an object of that class is created. Certain member variables and member methods
of the class are kept protected so as to provide a boundary for their accessibility. These
variables and methods shall be discussed with keen details towards their usage, data types,
accessibilities and purpose in the following paragraphs.
Header Files imported
1. java.text.ParseException
2. java.text.SimpleDateFormat
3. java.util.Locale
4. java.util.Date
5. java.util.Scanner
Data Dictionary
Variable Name Variable type Brief description
patientName String Stores the name of patient
providerName String Stores the provider name for
patient
appDate String Stores the appointment date
of patient
appTime String Stores the appointment time
of patient
telephone long Stores the contact number of
patient
count integer Keeps track of the number
of patient objects created
JHC SYSTEM DESIGN
encapsulation to bind the data members and member methods of the class as a single unit
whenever an object of that class is created. Certain member variables and member methods
of the class are kept protected so as to provide a boundary for their accessibility. These
variables and methods shall be discussed with keen details towards their usage, data types,
accessibilities and purpose in the following paragraphs.
Header Files imported
1. java.text.ParseException
2. java.text.SimpleDateFormat
3. java.util.Locale
4. java.util.Date
5. java.util.Scanner
Data Dictionary
Variable Name Variable type Brief description
patientName String Stores the name of patient
providerName String Stores the provider name for
patient
appDate String Stores the appointment date
of patient
appTime String Stores the appointment time
of patient
telephone long Stores the contact number of
patient
count integer Keeps track of the number
of patient objects created
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
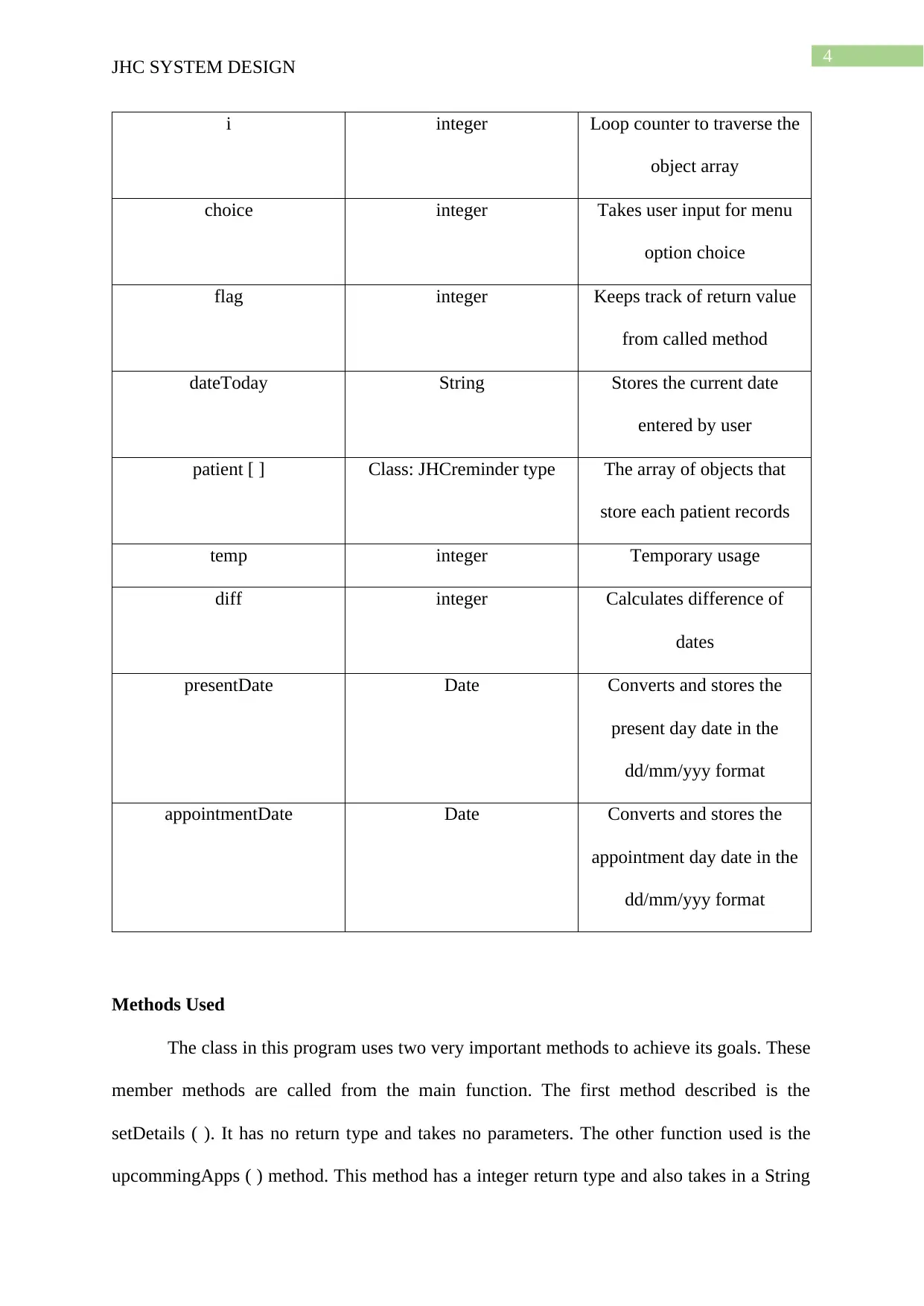
4
JHC SYSTEM DESIGN
i integer Loop counter to traverse the
object array
choice integer Takes user input for menu
option choice
flag integer Keeps track of return value
from called method
dateToday String Stores the current date
entered by user
patient [ ] Class: JHCreminder type The array of objects that
store each patient records
temp integer Temporary usage
diff integer Calculates difference of
dates
presentDate Date Converts and stores the
present day date in the
dd/mm/yyy format
appointmentDate Date Converts and stores the
appointment day date in the
dd/mm/yyy format
Methods Used
The class in this program uses two very important methods to achieve its goals. These
member methods are called from the main function. The first method described is the
setDetails ( ). It has no return type and takes no parameters. The other function used is the
upcommingApps ( ) method. This method has a integer return type and also takes in a String
JHC SYSTEM DESIGN
i integer Loop counter to traverse the
object array
choice integer Takes user input for menu
option choice
flag integer Keeps track of return value
from called method
dateToday String Stores the current date
entered by user
patient [ ] Class: JHCreminder type The array of objects that
store each patient records
temp integer Temporary usage
diff integer Calculates difference of
dates
presentDate Date Converts and stores the
present day date in the
dd/mm/yyy format
appointmentDate Date Converts and stores the
appointment day date in the
dd/mm/yyy format
Methods Used
The class in this program uses two very important methods to achieve its goals. These
member methods are called from the main function. The first method described is the
setDetails ( ). It has no return type and takes no parameters. The other function used is the
upcommingApps ( ) method. This method has a integer return type and also takes in a String
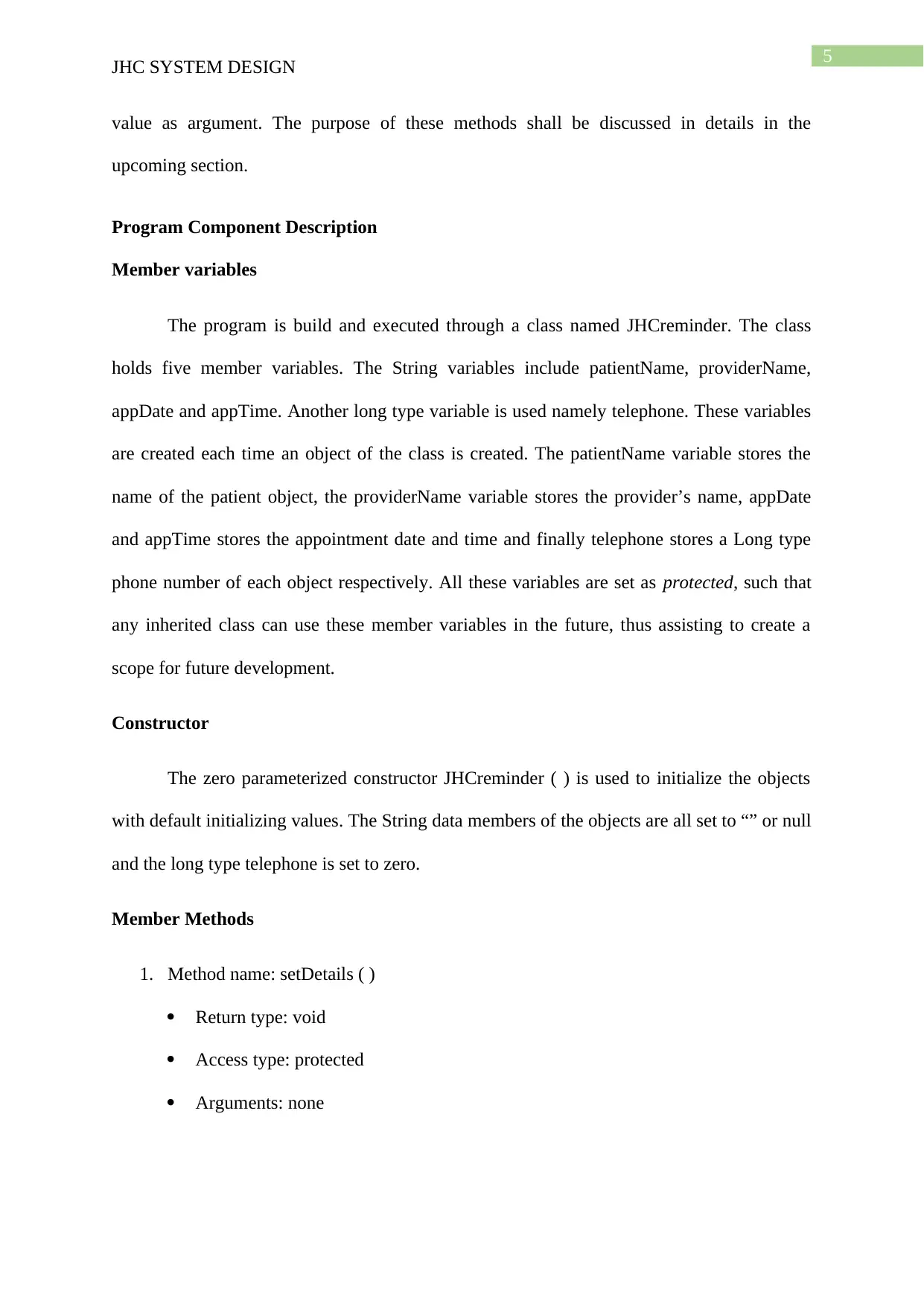
5
JHC SYSTEM DESIGN
value as argument. The purpose of these methods shall be discussed in details in the
upcoming section.
Program Component Description
Member variables
The program is build and executed through a class named JHCreminder. The class
holds five member variables. The String variables include patientName, providerName,
appDate and appTime. Another long type variable is used namely telephone. These variables
are created each time an object of the class is created. The patientName variable stores the
name of the patient object, the providerName variable stores the provider’s name, appDate
and appTime stores the appointment date and time and finally telephone stores a Long type
phone number of each object respectively. All these variables are set as protected, such that
any inherited class can use these member variables in the future, thus assisting to create a
scope for future development.
Constructor
The zero parameterized constructor JHCreminder ( ) is used to initialize the objects
with default initializing values. The String data members of the objects are all set to “” or null
and the long type telephone is set to zero.
Member Methods
1. Method name: setDetails ( )
Return type: void
Access type: protected
Arguments: none
JHC SYSTEM DESIGN
value as argument. The purpose of these methods shall be discussed in details in the
upcoming section.
Program Component Description
Member variables
The program is build and executed through a class named JHCreminder. The class
holds five member variables. The String variables include patientName, providerName,
appDate and appTime. Another long type variable is used namely telephone. These variables
are created each time an object of the class is created. The patientName variable stores the
name of the patient object, the providerName variable stores the provider’s name, appDate
and appTime stores the appointment date and time and finally telephone stores a Long type
phone number of each object respectively. All these variables are set as protected, such that
any inherited class can use these member variables in the future, thus assisting to create a
scope for future development.
Constructor
The zero parameterized constructor JHCreminder ( ) is used to initialize the objects
with default initializing values. The String data members of the objects are all set to “” or null
and the long type telephone is set to zero.
Member Methods
1. Method name: setDetails ( )
Return type: void
Access type: protected
Arguments: none
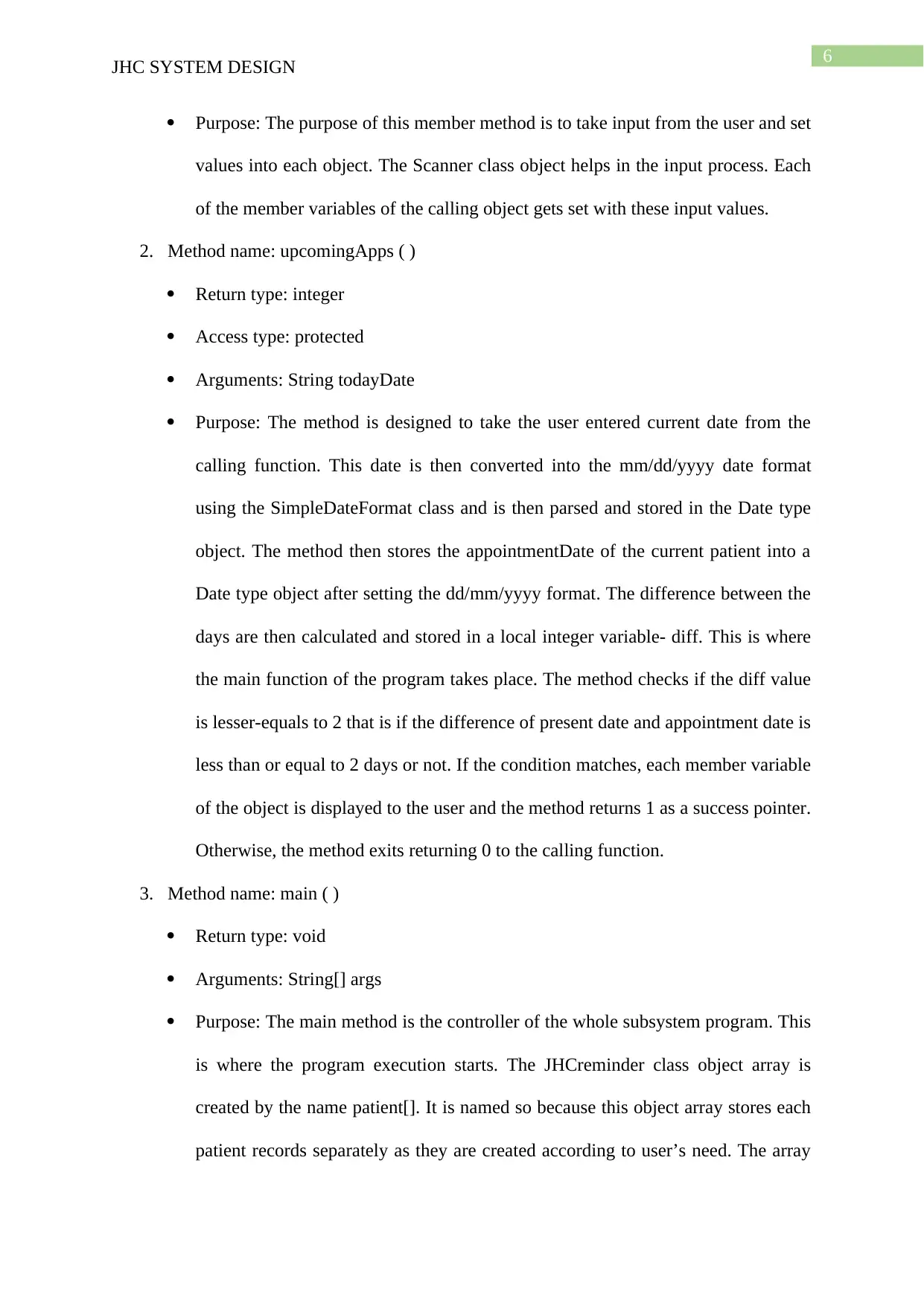
6
JHC SYSTEM DESIGN
Purpose: The purpose of this member method is to take input from the user and set
values into each object. The Scanner class object helps in the input process. Each
of the member variables of the calling object gets set with these input values.
2. Method name: upcomingApps ( )
Return type: integer
Access type: protected
Arguments: String todayDate
Purpose: The method is designed to take the user entered current date from the
calling function. This date is then converted into the mm/dd/yyyy date format
using the SimpleDateFormat class and is then parsed and stored in the Date type
object. The method then stores the appointmentDate of the current patient into a
Date type object after setting the dd/mm/yyyy format. The difference between the
days are then calculated and stored in a local integer variable- diff. This is where
the main function of the program takes place. The method checks if the diff value
is lesser-equals to 2 that is if the difference of present date and appointment date is
less than or equal to 2 days or not. If the condition matches, each member variable
of the object is displayed to the user and the method returns 1 as a success pointer.
Otherwise, the method exits returning 0 to the calling function.
3. Method name: main ( )
Return type: void
Arguments: String[] args
Purpose: The main method is the controller of the whole subsystem program. This
is where the program execution starts. The JHCreminder class object array is
created by the name patient[]. It is named so because this object array stores each
patient records separately as they are created according to user’s need. The array
JHC SYSTEM DESIGN
Purpose: The purpose of this member method is to take input from the user and set
values into each object. The Scanner class object helps in the input process. Each
of the member variables of the calling object gets set with these input values.
2. Method name: upcomingApps ( )
Return type: integer
Access type: protected
Arguments: String todayDate
Purpose: The method is designed to take the user entered current date from the
calling function. This date is then converted into the mm/dd/yyyy date format
using the SimpleDateFormat class and is then parsed and stored in the Date type
object. The method then stores the appointmentDate of the current patient into a
Date type object after setting the dd/mm/yyyy format. The difference between the
days are then calculated and stored in a local integer variable- diff. This is where
the main function of the program takes place. The method checks if the diff value
is lesser-equals to 2 that is if the difference of present date and appointment date is
less than or equal to 2 days or not. If the condition matches, each member variable
of the object is displayed to the user and the method returns 1 as a success pointer.
Otherwise, the method exits returning 0 to the calling function.
3. Method name: main ( )
Return type: void
Arguments: String[] args
Purpose: The main method is the controller of the whole subsystem program. This
is where the program execution starts. The JHCreminder class object array is
created by the name patient[]. It is named so because this object array stores each
patient records separately as they are created according to user’s need. The array
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
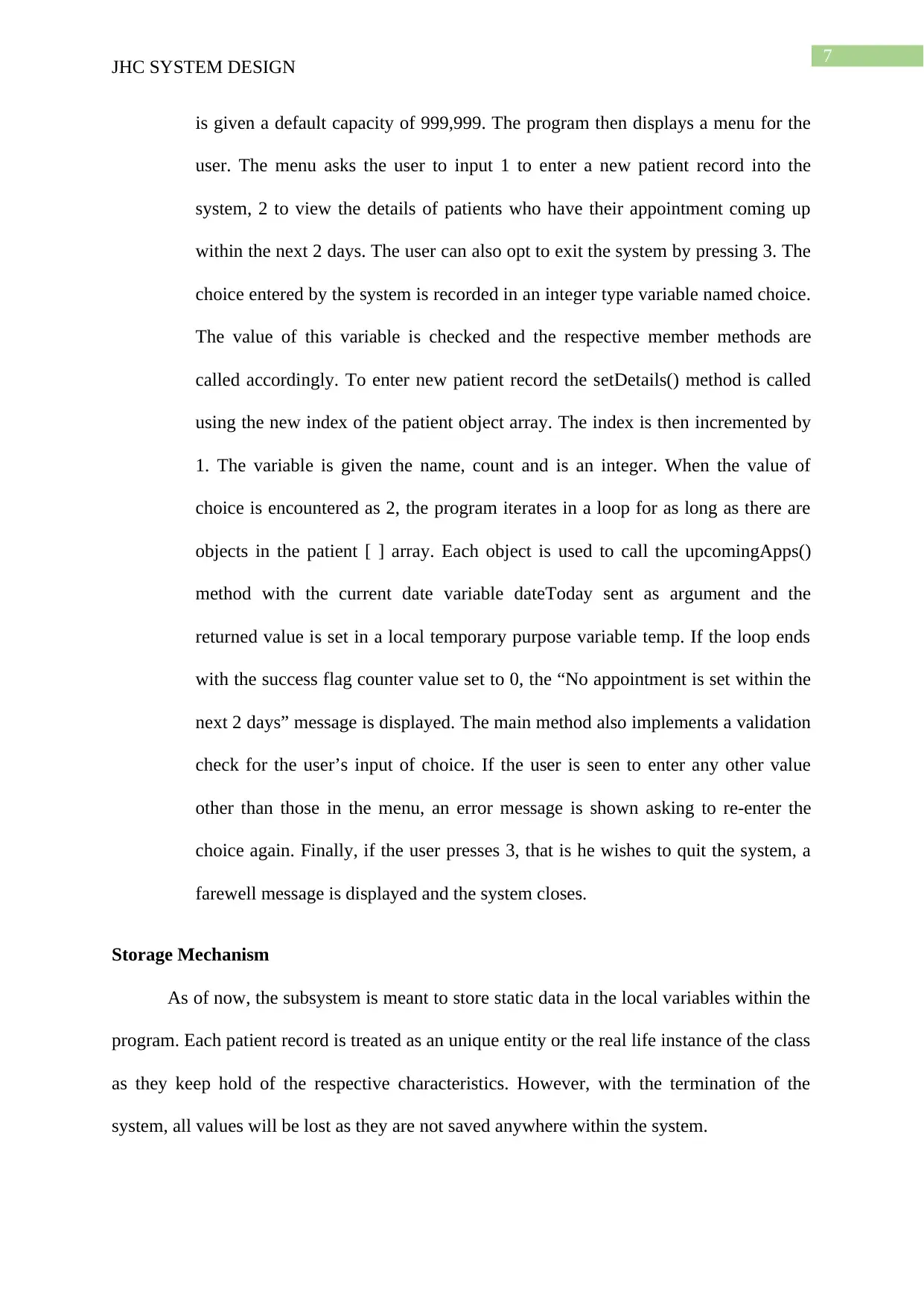
7
JHC SYSTEM DESIGN
is given a default capacity of 999,999. The program then displays a menu for the
user. The menu asks the user to input 1 to enter a new patient record into the
system, 2 to view the details of patients who have their appointment coming up
within the next 2 days. The user can also opt to exit the system by pressing 3. The
choice entered by the system is recorded in an integer type variable named choice.
The value of this variable is checked and the respective member methods are
called accordingly. To enter new patient record the setDetails() method is called
using the new index of the patient object array. The index is then incremented by
1. The variable is given the name, count and is an integer. When the value of
choice is encountered as 2, the program iterates in a loop for as long as there are
objects in the patient [ ] array. Each object is used to call the upcomingApps()
method with the current date variable dateToday sent as argument and the
returned value is set in a local temporary purpose variable temp. If the loop ends
with the success flag counter value set to 0, the “No appointment is set within the
next 2 days” message is displayed. The main method also implements a validation
check for the user’s input of choice. If the user is seen to enter any other value
other than those in the menu, an error message is shown asking to re-enter the
choice again. Finally, if the user presses 3, that is he wishes to quit the system, a
farewell message is displayed and the system closes.
Storage Mechanism
As of now, the subsystem is meant to store static data in the local variables within the
program. Each patient record is treated as an unique entity or the real life instance of the class
as they keep hold of the respective characteristics. However, with the termination of the
system, all values will be lost as they are not saved anywhere within the system.
JHC SYSTEM DESIGN
is given a default capacity of 999,999. The program then displays a menu for the
user. The menu asks the user to input 1 to enter a new patient record into the
system, 2 to view the details of patients who have their appointment coming up
within the next 2 days. The user can also opt to exit the system by pressing 3. The
choice entered by the system is recorded in an integer type variable named choice.
The value of this variable is checked and the respective member methods are
called accordingly. To enter new patient record the setDetails() method is called
using the new index of the patient object array. The index is then incremented by
1. The variable is given the name, count and is an integer. When the value of
choice is encountered as 2, the program iterates in a loop for as long as there are
objects in the patient [ ] array. Each object is used to call the upcomingApps()
method with the current date variable dateToday sent as argument and the
returned value is set in a local temporary purpose variable temp. If the loop ends
with the success flag counter value set to 0, the “No appointment is set within the
next 2 days” message is displayed. The main method also implements a validation
check for the user’s input of choice. If the user is seen to enter any other value
other than those in the menu, an error message is shown asking to re-enter the
choice again. Finally, if the user presses 3, that is he wishes to quit the system, a
farewell message is displayed and the system closes.
Storage Mechanism
As of now, the subsystem is meant to store static data in the local variables within the
program. Each patient record is treated as an unique entity or the real life instance of the class
as they keep hold of the respective characteristics. However, with the termination of the
system, all values will be lost as they are not saved anywhere within the system.
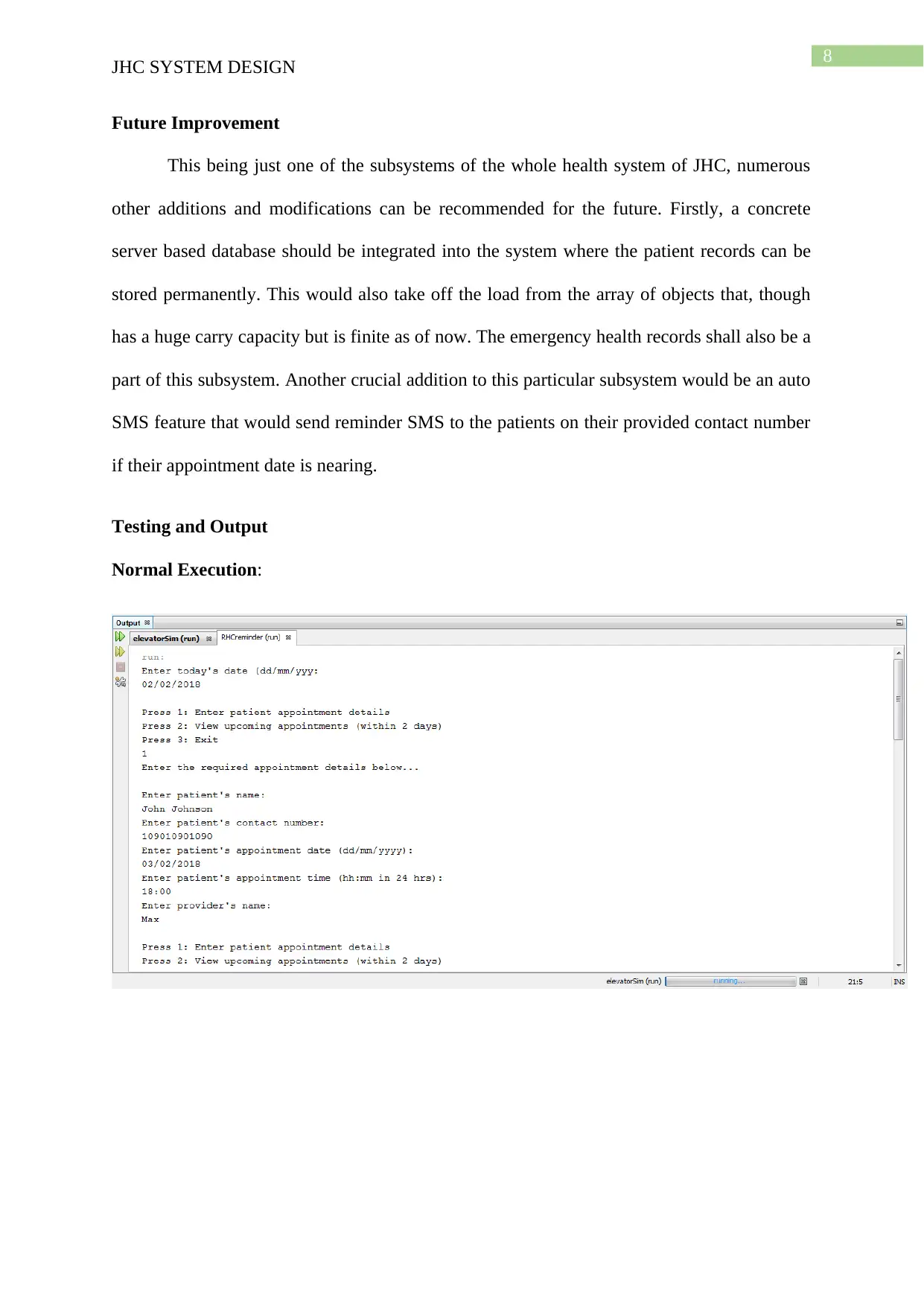
8
JHC SYSTEM DESIGN
Future Improvement
This being just one of the subsystems of the whole health system of JHC, numerous
other additions and modifications can be recommended for the future. Firstly, a concrete
server based database should be integrated into the system where the patient records can be
stored permanently. This would also take off the load from the array of objects that, though
has a huge carry capacity but is finite as of now. The emergency health records shall also be a
part of this subsystem. Another crucial addition to this particular subsystem would be an auto
SMS feature that would send reminder SMS to the patients on their provided contact number
if their appointment date is nearing.
Testing and Output
Normal Execution:
JHC SYSTEM DESIGN
Future Improvement
This being just one of the subsystems of the whole health system of JHC, numerous
other additions and modifications can be recommended for the future. Firstly, a concrete
server based database should be integrated into the system where the patient records can be
stored permanently. This would also take off the load from the array of objects that, though
has a huge carry capacity but is finite as of now. The emergency health records shall also be a
part of this subsystem. Another crucial addition to this particular subsystem would be an auto
SMS feature that would send reminder SMS to the patients on their provided contact number
if their appointment date is nearing.
Testing and Output
Normal Execution:
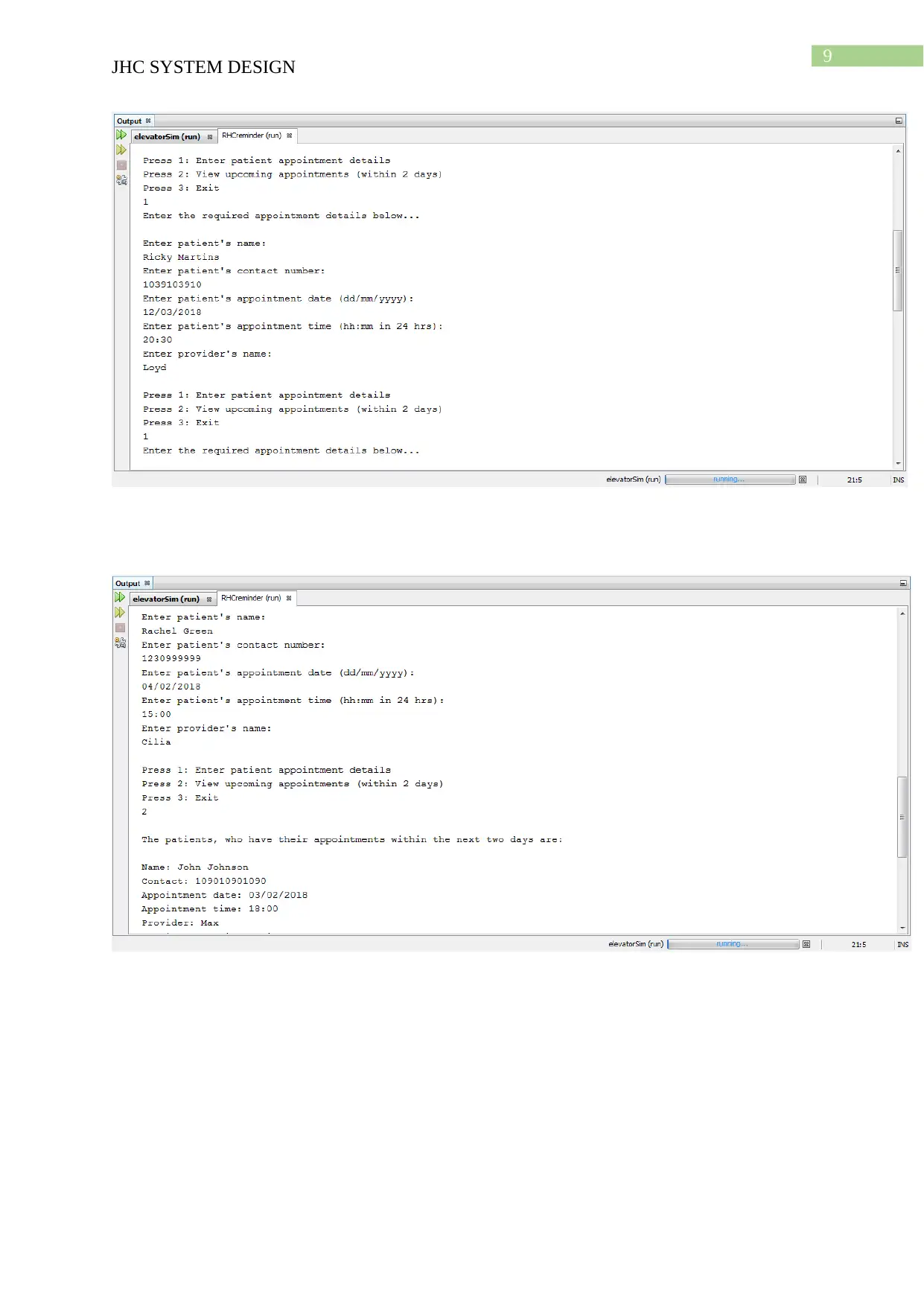
9
JHC SYSTEM DESIGN
JHC SYSTEM DESIGN
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
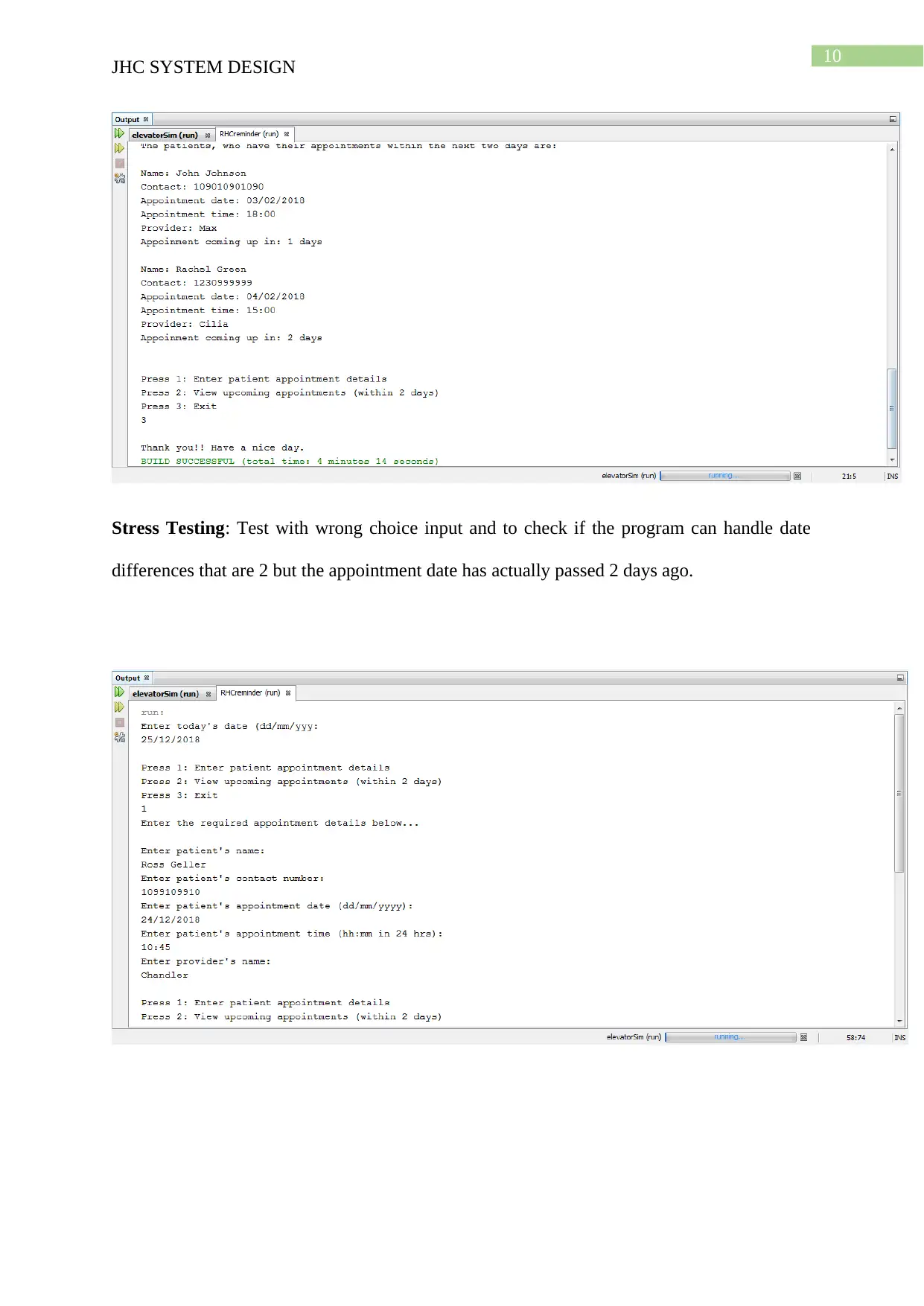
10
JHC SYSTEM DESIGN
Stress Testing: Test with wrong choice input and to check if the program can handle date
differences that are 2 but the appointment date has actually passed 2 days ago.
JHC SYSTEM DESIGN
Stress Testing: Test with wrong choice input and to check if the program can handle date
differences that are 2 but the appointment date has actually passed 2 days ago.
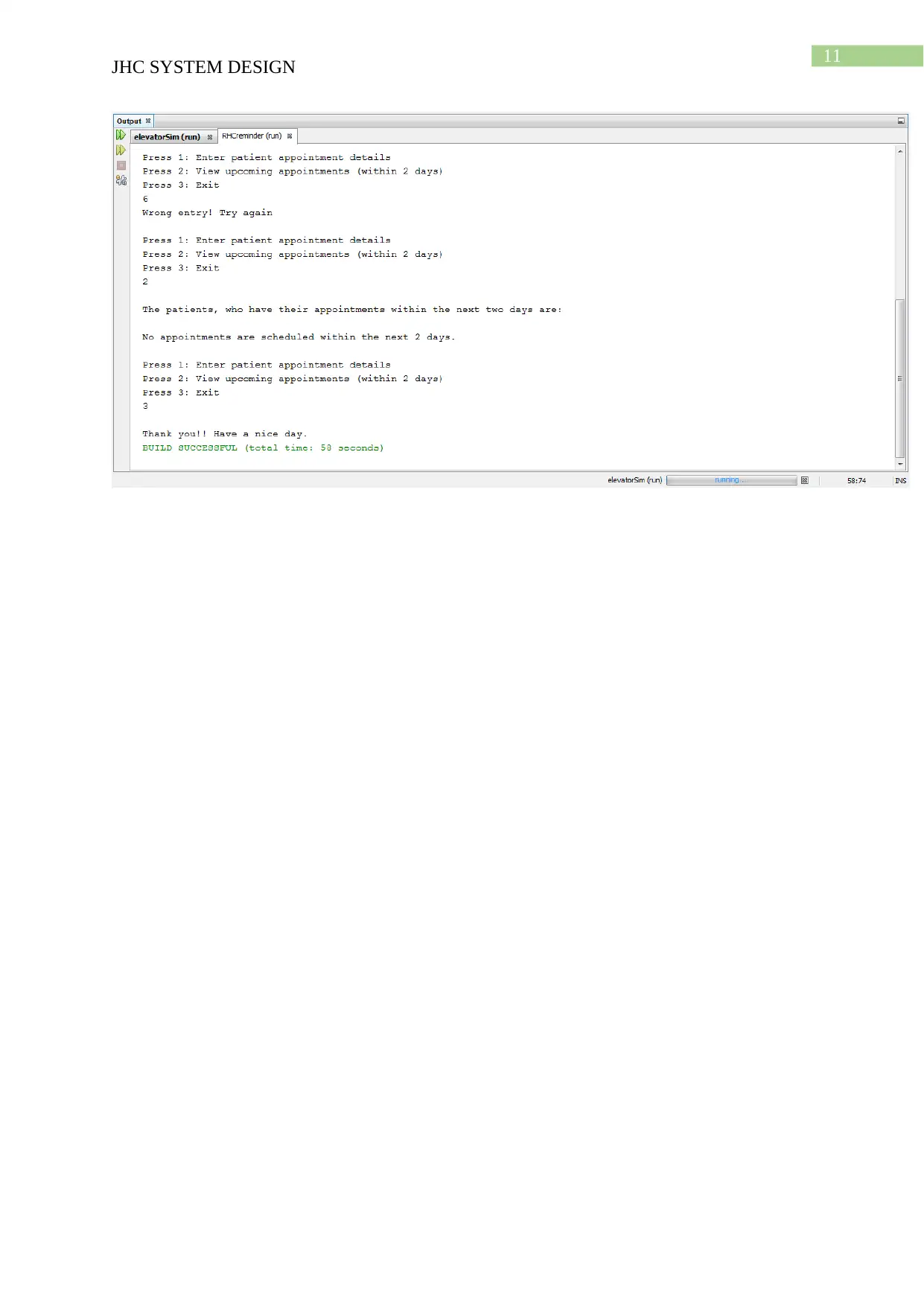
11
JHC SYSTEM DESIGN
JHC SYSTEM DESIGN
1 out of 12
Related Documents

Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.