CS 6233: Operating System Design Report - Kernel Changes
VerifiedAdded on 2021/12/22
|10
|1302
|58
Report
AI Summary
This report details a student's exploration of the Linux kernel, specifically focusing on modifying the kernel to understand process management. The assignment involves using the fork() system call to create parent and child processes, demonstrating an understanding of how processes are created and managed within the operating system. The report outlines the steps taken to download, modify, and recompile the kernel, including the use of `wget`, `tar`, and `make menuconfig`. The student created a program to verify the changes, illustrating the division of processes and the implications of process termination. The report also demonstrates the understanding of the kernel layout, including the directory structure and the purpose of different directories like Arch, Include, Init, Mm, Drivers, Ipc, Modules, Fs, Kernel, Net, Lib and Script. The conclusion highlights the use of fork() system call for distinguishing the process and the unpredictable nature of the output due to the CPU scheduler.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
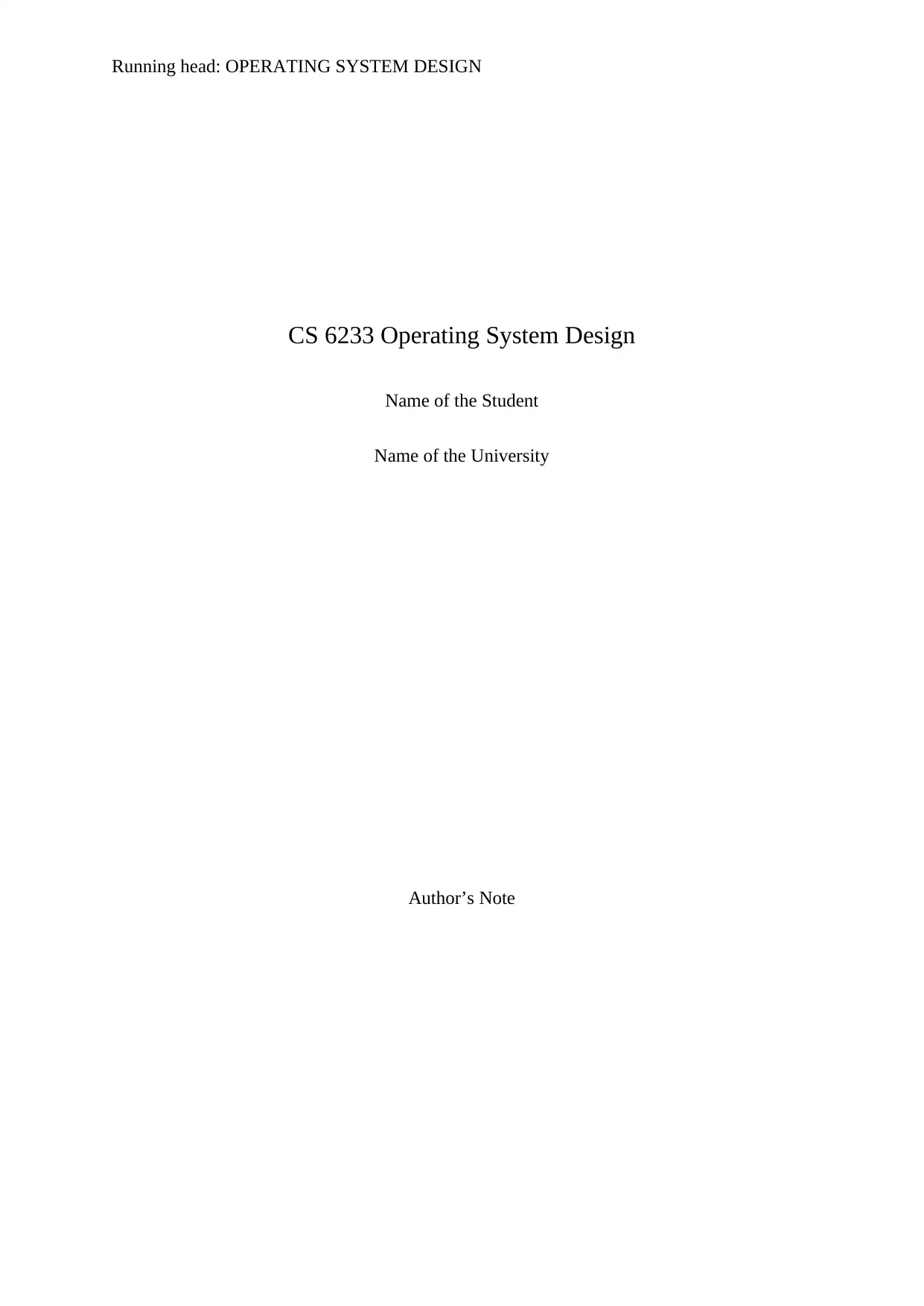
Running head: OPERATING SYSTEM DESIGN
CS 6233 Operating System Design
Name of the Student
Name of the University
Author’s Note
CS 6233 Operating System Design
Name of the Student
Name of the University
Author’s Note
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
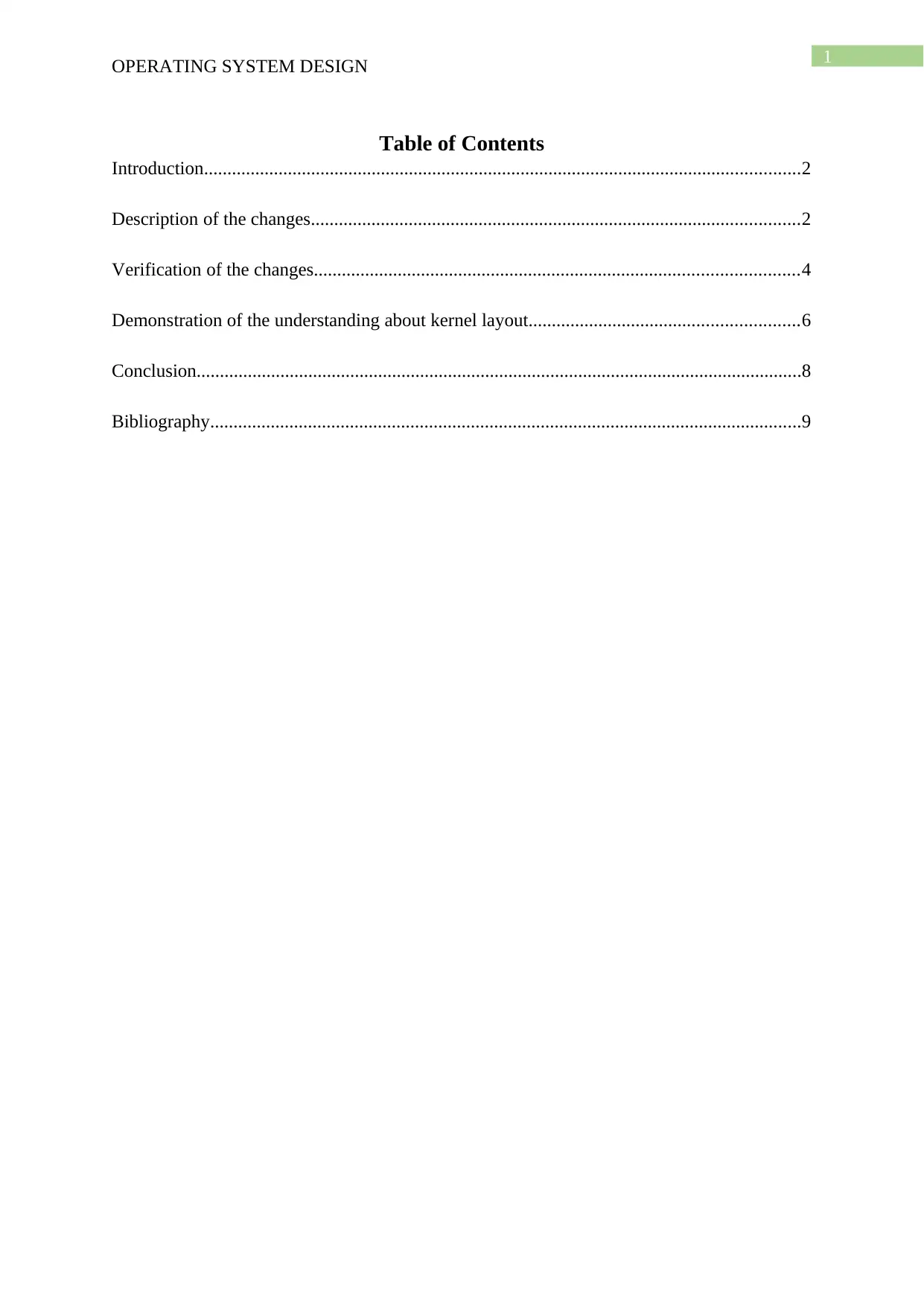
1
OPERATING SYSTEM DESIGN
Table of Contents
Introduction................................................................................................................................2
Description of the changes.........................................................................................................2
Verification of the changes........................................................................................................4
Demonstration of the understanding about kernel layout..........................................................6
Conclusion..................................................................................................................................8
Bibliography...............................................................................................................................9
OPERATING SYSTEM DESIGN
Table of Contents
Introduction................................................................................................................................2
Description of the changes.........................................................................................................2
Verification of the changes........................................................................................................4
Demonstration of the understanding about kernel layout..........................................................6
Conclusion..................................................................................................................................8
Bibliography...............................................................................................................................9
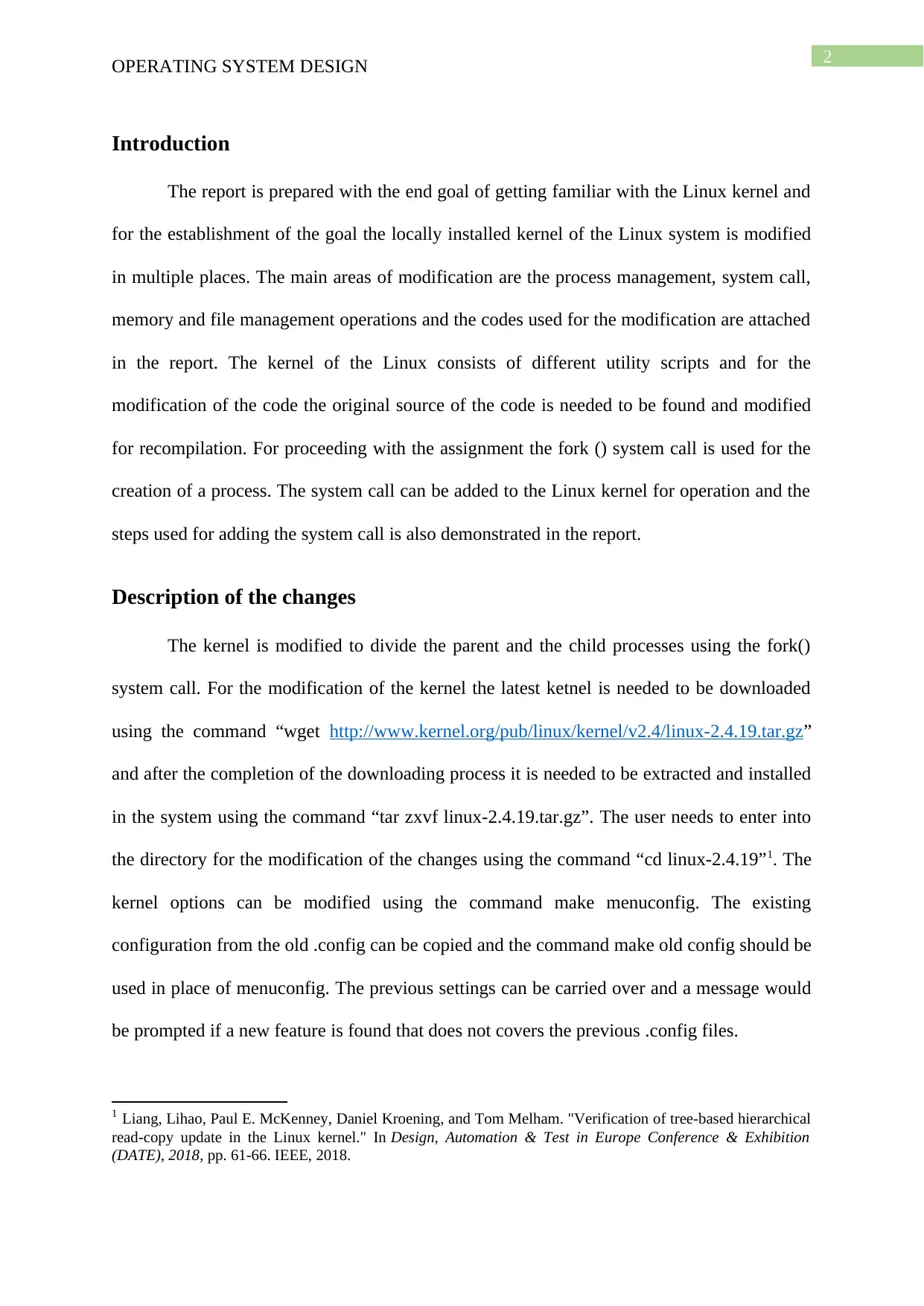
2
OPERATING SYSTEM DESIGN
Introduction
The report is prepared with the end goal of getting familiar with the Linux kernel and
for the establishment of the goal the locally installed kernel of the Linux system is modified
in multiple places. The main areas of modification are the process management, system call,
memory and file management operations and the codes used for the modification are attached
in the report. The kernel of the Linux consists of different utility scripts and for the
modification of the code the original source of the code is needed to be found and modified
for recompilation. For proceeding with the assignment the fork () system call is used for the
creation of a process. The system call can be added to the Linux kernel for operation and the
steps used for adding the system call is also demonstrated in the report.
Description of the changes
The kernel is modified to divide the parent and the child processes using the fork()
system call. For the modification of the kernel the latest ketnel is needed to be downloaded
using the command “wget http://www.kernel.org/pub/linux/kernel/v2.4/linux-2.4.19.tar.gz”
and after the completion of the downloading process it is needed to be extracted and installed
in the system using the command “tar zxvf linux-2.4.19.tar.gz”. The user needs to enter into
the directory for the modification of the changes using the command “cd linux-2.4.19”1. The
kernel options can be modified using the command make menuconfig. The existing
configuration from the old .config can be copied and the command make old config should be
used in place of menuconfig. The previous settings can be carried over and a message would
be prompted if a new feature is found that does not covers the previous .config files.
1 Liang, Lihao, Paul E. McKenney, Daniel Kroening, and Tom Melham. "Verification of tree-based hierarchical
read-copy update in the Linux kernel." In Design, Automation & Test in Europe Conference & Exhibition
(DATE), 2018, pp. 61-66. IEEE, 2018.
OPERATING SYSTEM DESIGN
Introduction
The report is prepared with the end goal of getting familiar with the Linux kernel and
for the establishment of the goal the locally installed kernel of the Linux system is modified
in multiple places. The main areas of modification are the process management, system call,
memory and file management operations and the codes used for the modification are attached
in the report. The kernel of the Linux consists of different utility scripts and for the
modification of the code the original source of the code is needed to be found and modified
for recompilation. For proceeding with the assignment the fork () system call is used for the
creation of a process. The system call can be added to the Linux kernel for operation and the
steps used for adding the system call is also demonstrated in the report.
Description of the changes
The kernel is modified to divide the parent and the child processes using the fork()
system call. For the modification of the kernel the latest ketnel is needed to be downloaded
using the command “wget http://www.kernel.org/pub/linux/kernel/v2.4/linux-2.4.19.tar.gz”
and after the completion of the downloading process it is needed to be extracted and installed
in the system using the command “tar zxvf linux-2.4.19.tar.gz”. The user needs to enter into
the directory for the modification of the changes using the command “cd linux-2.4.19”1. The
kernel options can be modified using the command make menuconfig. The existing
configuration from the old .config can be copied and the command make old config should be
used in place of menuconfig. The previous settings can be carried over and a message would
be prompted if a new feature is found that does not covers the previous .config files.
1 Liang, Lihao, Paul E. McKenney, Daniel Kroening, and Tom Melham. "Verification of tree-based hierarchical
read-copy update in the Linux kernel." In Design, Automation & Test in Europe Conference & Exhibition
(DATE), 2018, pp. 61-66. IEEE, 2018.
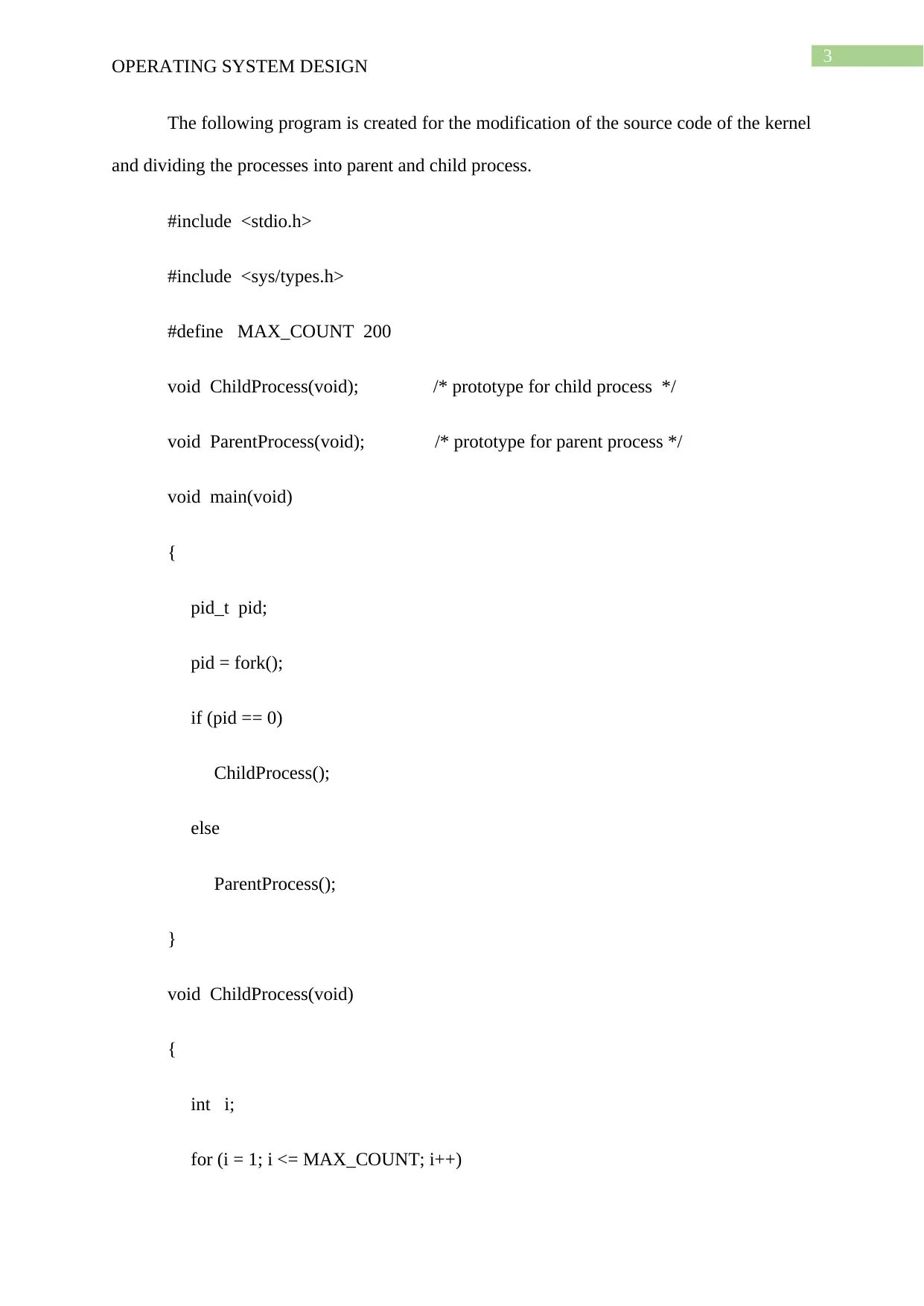
3
OPERATING SYSTEM DESIGN
The following program is created for the modification of the source code of the kernel
and dividing the processes into parent and child process.
#include <stdio.h>
#include <sys/types.h>
#define MAX_COUNT 200
void ChildProcess(void); /* prototype for child process */
void ParentProcess(void); /* prototype for parent process */
void main(void)
{
pid_t pid;
pid = fork();
if (pid == 0)
ChildProcess();
else
ParentProcess();
}
void ChildProcess(void)
{
int i;
for (i = 1; i <= MAX_COUNT; i++)
OPERATING SYSTEM DESIGN
The following program is created for the modification of the source code of the kernel
and dividing the processes into parent and child process.
#include <stdio.h>
#include <sys/types.h>
#define MAX_COUNT 200
void ChildProcess(void); /* prototype for child process */
void ParentProcess(void); /* prototype for parent process */
void main(void)
{
pid_t pid;
pid = fork();
if (pid == 0)
ChildProcess();
else
ParentProcess();
}
void ChildProcess(void)
{
int i;
for (i = 1; i <= MAX_COUNT; i++)
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
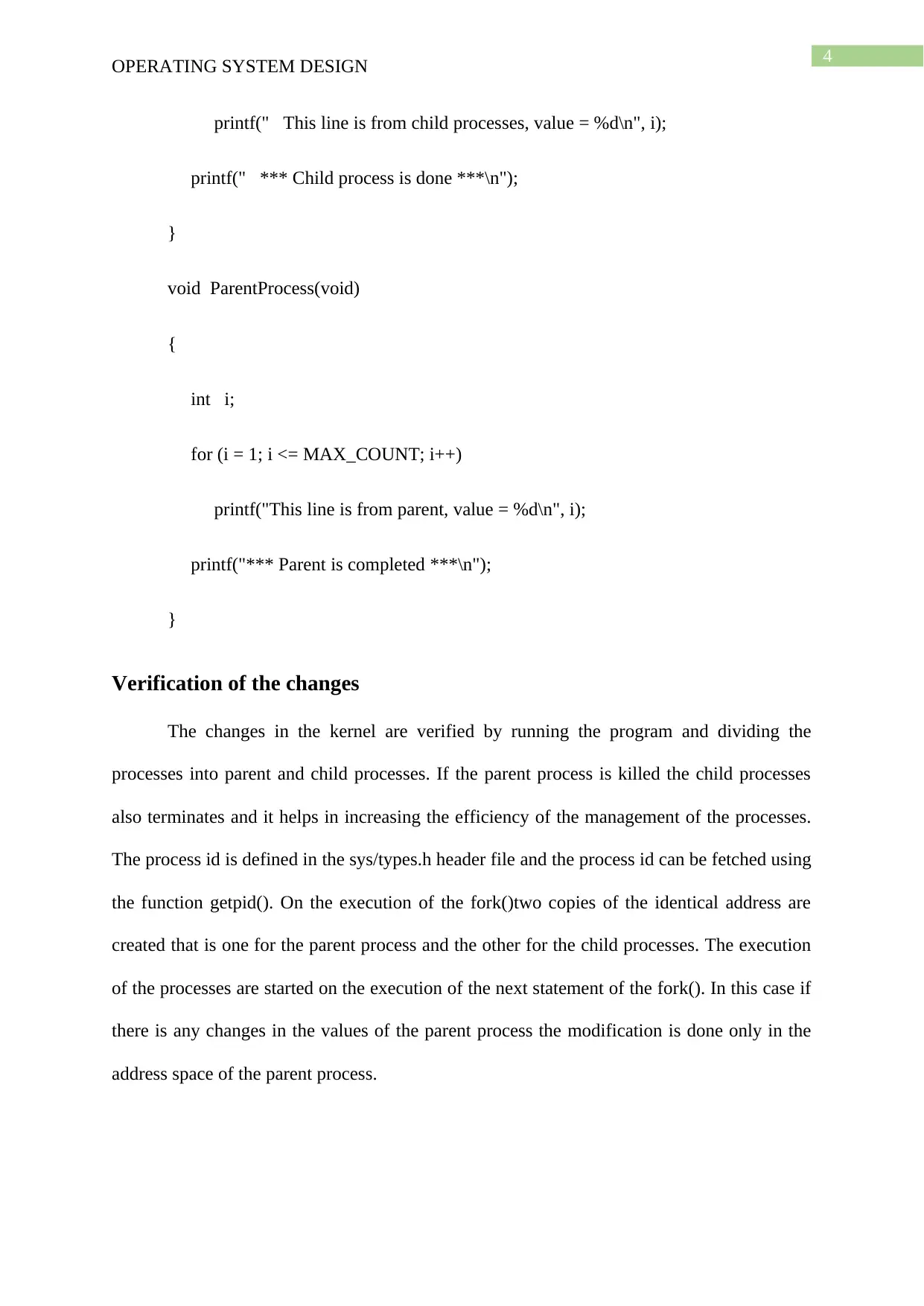
4
OPERATING SYSTEM DESIGN
printf(" This line is from child processes, value = %d\n", i);
printf(" *** Child process is done ***\n");
}
void ParentProcess(void)
{
int i;
for (i = 1; i <= MAX_COUNT; i++)
printf("This line is from parent, value = %d\n", i);
printf("*** Parent is completed ***\n");
}
Verification of the changes
The changes in the kernel are verified by running the program and dividing the
processes into parent and child processes. If the parent process is killed the child processes
also terminates and it helps in increasing the efficiency of the management of the processes.
The process id is defined in the sys/types.h header file and the process id can be fetched using
the function getpid(). On the execution of the fork()two copies of the identical address are
created that is one for the parent process and the other for the child processes. The execution
of the processes are started on the execution of the next statement of the fork(). In this case if
there is any changes in the values of the parent process the modification is done only in the
address space of the parent process.
OPERATING SYSTEM DESIGN
printf(" This line is from child processes, value = %d\n", i);
printf(" *** Child process is done ***\n");
}
void ParentProcess(void)
{
int i;
for (i = 1; i <= MAX_COUNT; i++)
printf("This line is from parent, value = %d\n", i);
printf("*** Parent is completed ***\n");
}
Verification of the changes
The changes in the kernel are verified by running the program and dividing the
processes into parent and child processes. If the parent process is killed the child processes
also terminates and it helps in increasing the efficiency of the management of the processes.
The process id is defined in the sys/types.h header file and the process id can be fetched using
the function getpid(). On the execution of the fork()two copies of the identical address are
created that is one for the parent process and the other for the child processes. The execution
of the processes are started on the execution of the next statement of the fork(). In this case if
there is any changes in the values of the parent process the modification is done only in the
address space of the parent process.
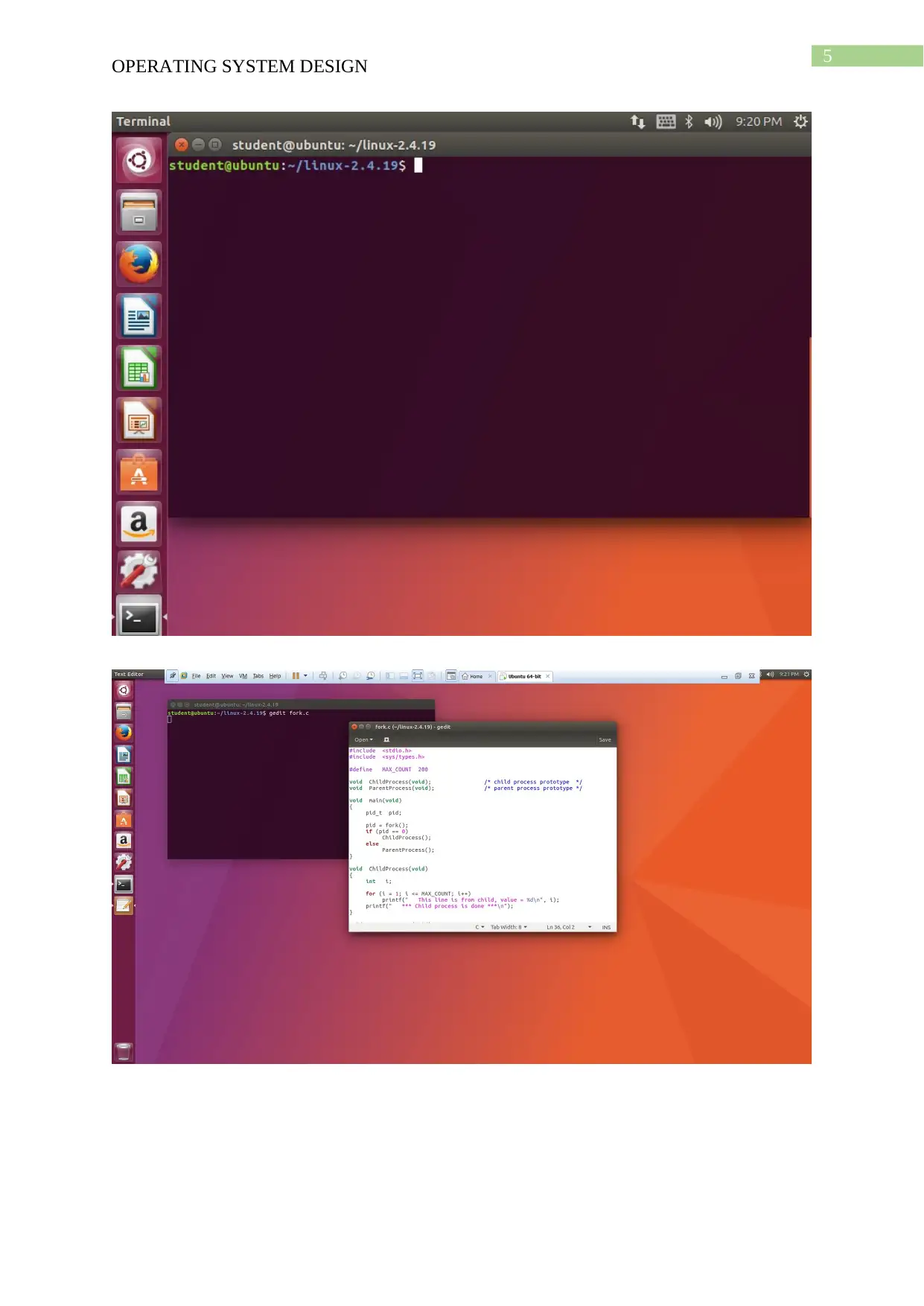
5
OPERATING SYSTEM DESIGN
OPERATING SYSTEM DESIGN
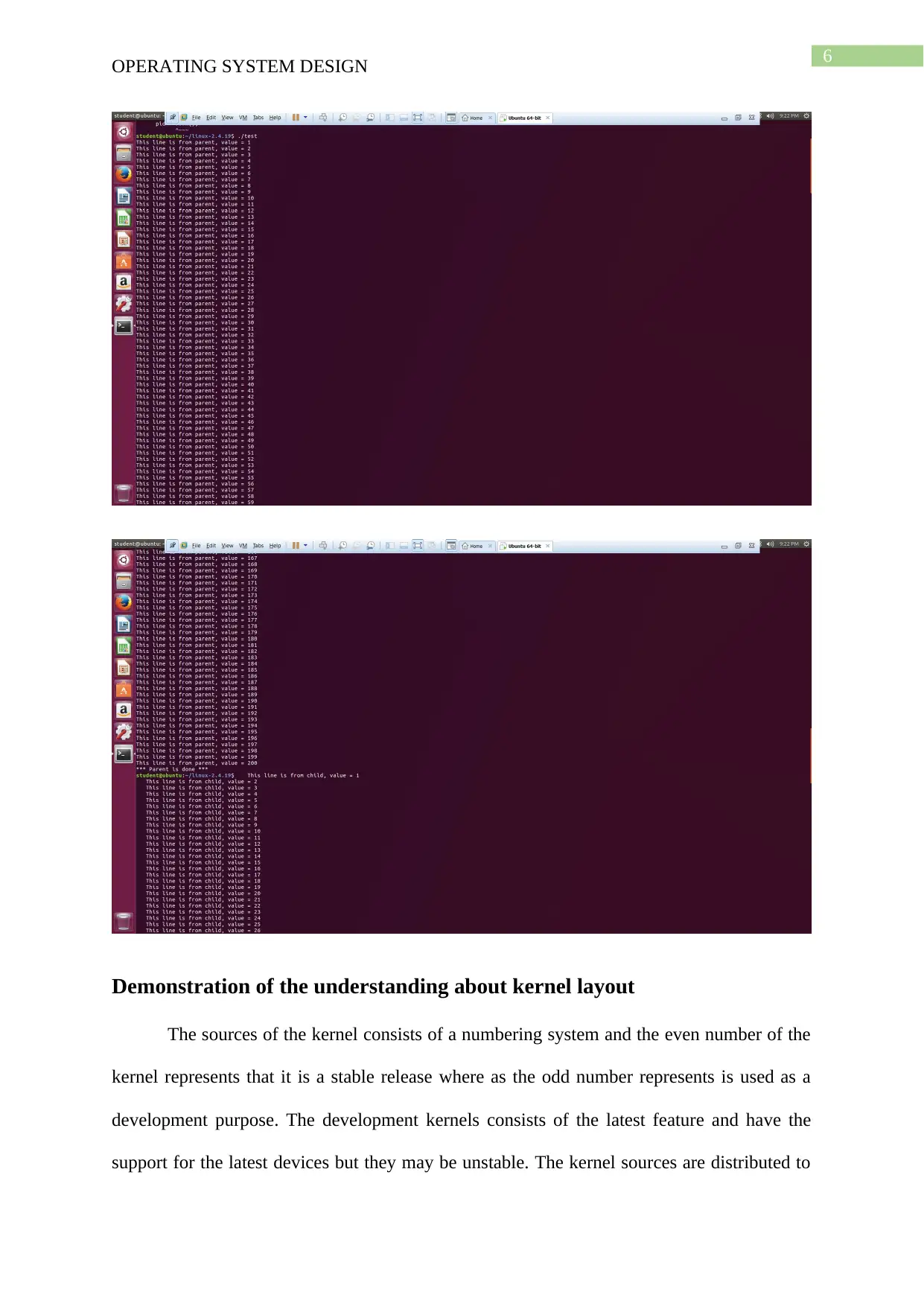
6
OPERATING SYSTEM DESIGN
Demonstration of the understanding about kernel layout
The sources of the kernel consists of a numbering system and the even number of the
kernel represents that it is a stable release where as the odd number represents is used as a
development purpose. The development kernels consists of the latest feature and have the
support for the latest devices but they may be unstable. The kernel sources are distributed to
OPERATING SYSTEM DESIGN
Demonstration of the understanding about kernel layout
The sources of the kernel consists of a numbering system and the even number of the
kernel represents that it is a stable release where as the odd number represents is used as a
development purpose. The development kernels consists of the latest feature and have the
support for the latest devices but they may be unstable. The kernel sources are distributed to
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
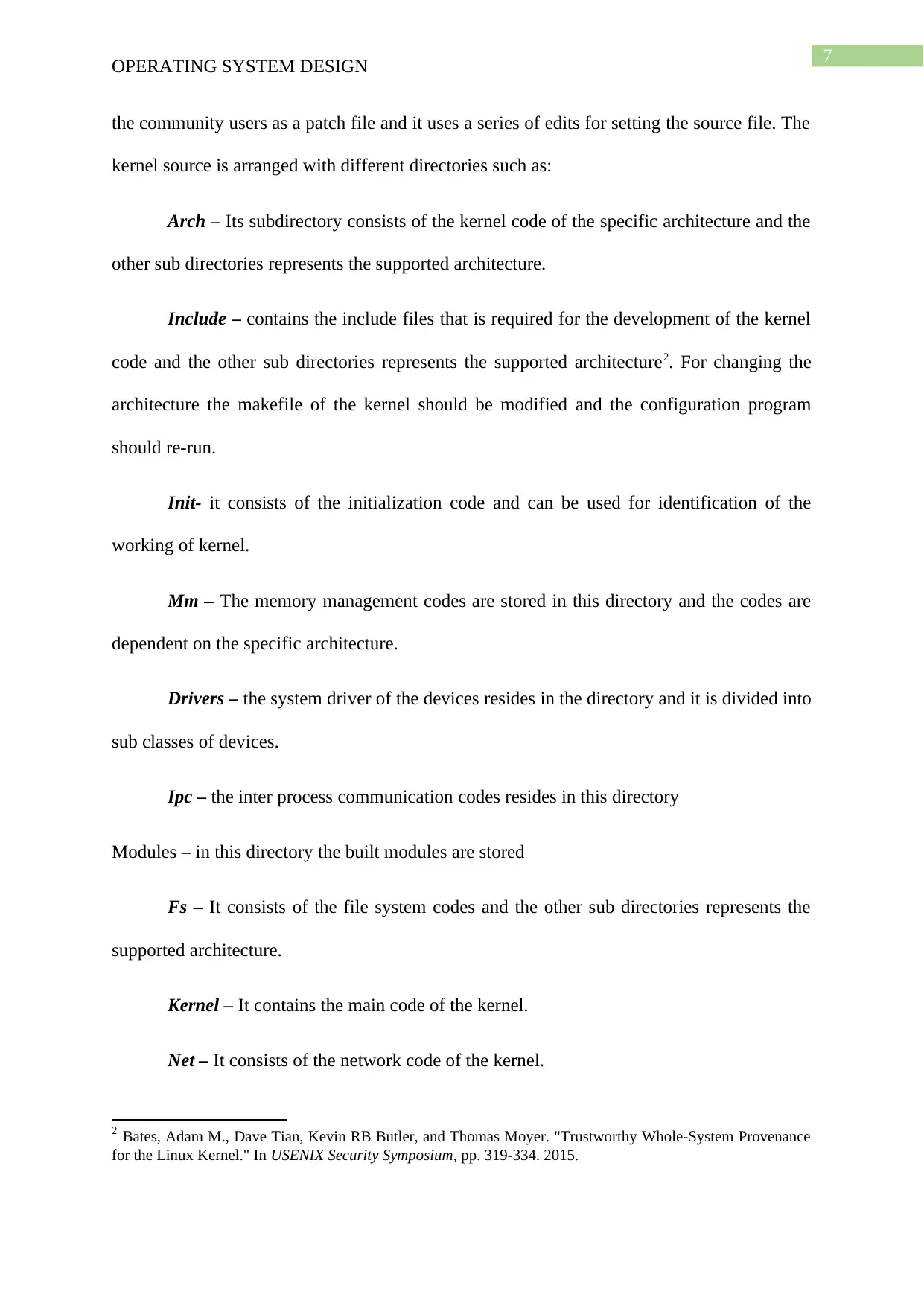
7
OPERATING SYSTEM DESIGN
the community users as a patch file and it uses a series of edits for setting the source file. The
kernel source is arranged with different directories such as:
Arch – Its subdirectory consists of the kernel code of the specific architecture and the
other sub directories represents the supported architecture.
Include – contains the include files that is required for the development of the kernel
code and the other sub directories represents the supported architecture2. For changing the
architecture the makefile of the kernel should be modified and the configuration program
should re-run.
Init- it consists of the initialization code and can be used for identification of the
working of kernel.
Mm – The memory management codes are stored in this directory and the codes are
dependent on the specific architecture.
Drivers – the system driver of the devices resides in the directory and it is divided into
sub classes of devices.
Ipc – the inter process communication codes resides in this directory
Modules – in this directory the built modules are stored
Fs – It consists of the file system codes and the other sub directories represents the
supported architecture.
Kernel – It contains the main code of the kernel.
Net – It consists of the network code of the kernel.
2 Bates, Adam M., Dave Tian, Kevin RB Butler, and Thomas Moyer. "Trustworthy Whole-System Provenance
for the Linux Kernel." In USENIX Security Symposium, pp. 319-334. 2015.
OPERATING SYSTEM DESIGN
the community users as a patch file and it uses a series of edits for setting the source file. The
kernel source is arranged with different directories such as:
Arch – Its subdirectory consists of the kernel code of the specific architecture and the
other sub directories represents the supported architecture.
Include – contains the include files that is required for the development of the kernel
code and the other sub directories represents the supported architecture2. For changing the
architecture the makefile of the kernel should be modified and the configuration program
should re-run.
Init- it consists of the initialization code and can be used for identification of the
working of kernel.
Mm – The memory management codes are stored in this directory and the codes are
dependent on the specific architecture.
Drivers – the system driver of the devices resides in the directory and it is divided into
sub classes of devices.
Ipc – the inter process communication codes resides in this directory
Modules – in this directory the built modules are stored
Fs – It consists of the file system codes and the other sub directories represents the
supported architecture.
Kernel – It contains the main code of the kernel.
Net – It consists of the network code of the kernel.
2 Bates, Adam M., Dave Tian, Kevin RB Butler, and Thomas Moyer. "Trustworthy Whole-System Provenance
for the Linux Kernel." In USENIX Security Symposium, pp. 319-334. 2015.
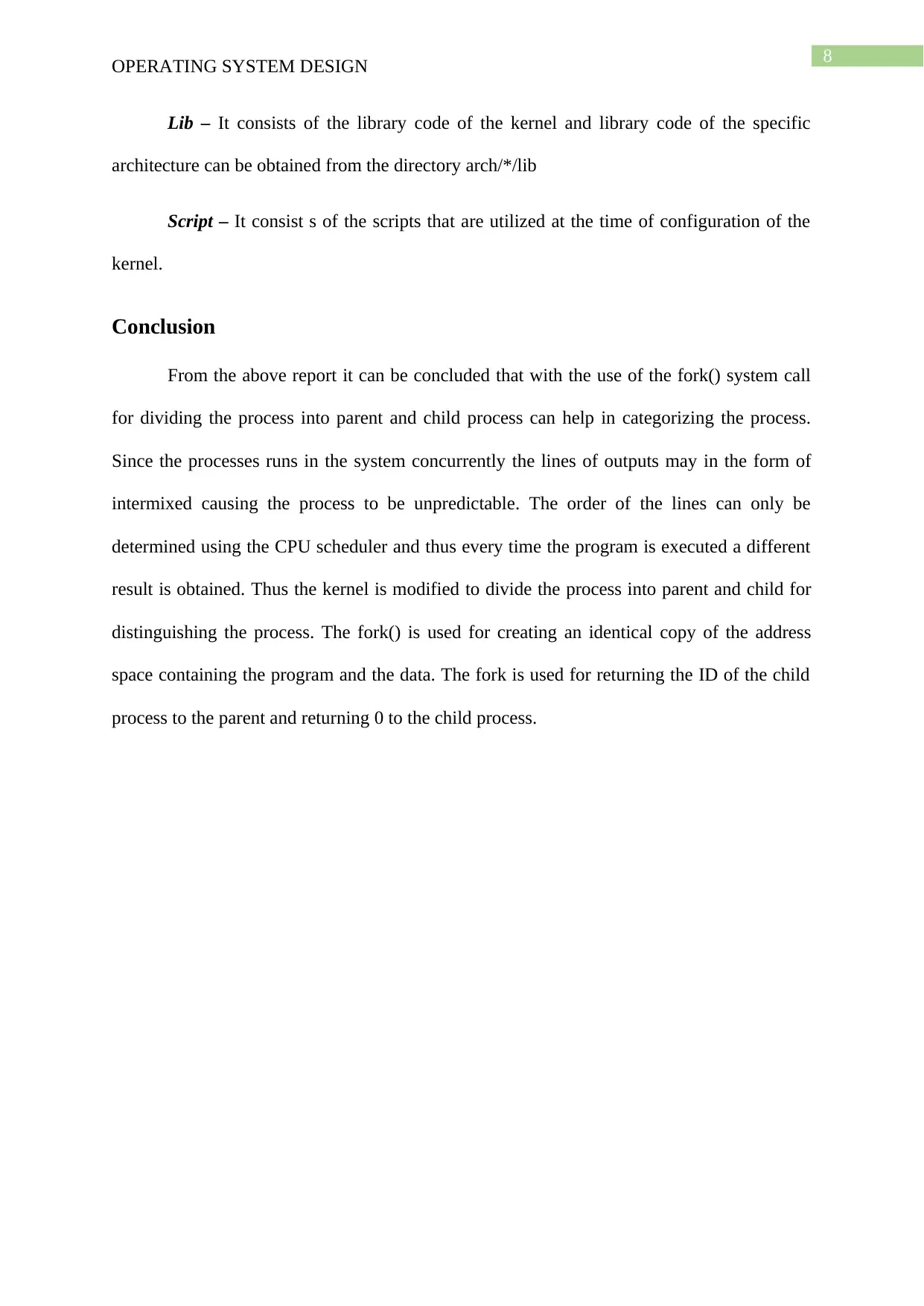
8
OPERATING SYSTEM DESIGN
Lib – It consists of the library code of the kernel and library code of the specific
architecture can be obtained from the directory arch/*/lib
Script – It consist s of the scripts that are utilized at the time of configuration of the
kernel.
Conclusion
From the above report it can be concluded that with the use of the fork() system call
for dividing the process into parent and child process can help in categorizing the process.
Since the processes runs in the system concurrently the lines of outputs may in the form of
intermixed causing the process to be unpredictable. The order of the lines can only be
determined using the CPU scheduler and thus every time the program is executed a different
result is obtained. Thus the kernel is modified to divide the process into parent and child for
distinguishing the process. The fork() is used for creating an identical copy of the address
space containing the program and the data. The fork is used for returning the ID of the child
process to the parent and returning 0 to the child process.
OPERATING SYSTEM DESIGN
Lib – It consists of the library code of the kernel and library code of the specific
architecture can be obtained from the directory arch/*/lib
Script – It consist s of the scripts that are utilized at the time of configuration of the
kernel.
Conclusion
From the above report it can be concluded that with the use of the fork() system call
for dividing the process into parent and child process can help in categorizing the process.
Since the processes runs in the system concurrently the lines of outputs may in the form of
intermixed causing the process to be unpredictable. The order of the lines can only be
determined using the CPU scheduler and thus every time the program is executed a different
result is obtained. Thus the kernel is modified to divide the process into parent and child for
distinguishing the process. The fork() is used for creating an identical copy of the address
space containing the program and the data. The fork is used for returning the ID of the child
process to the parent and returning 0 to the child process.
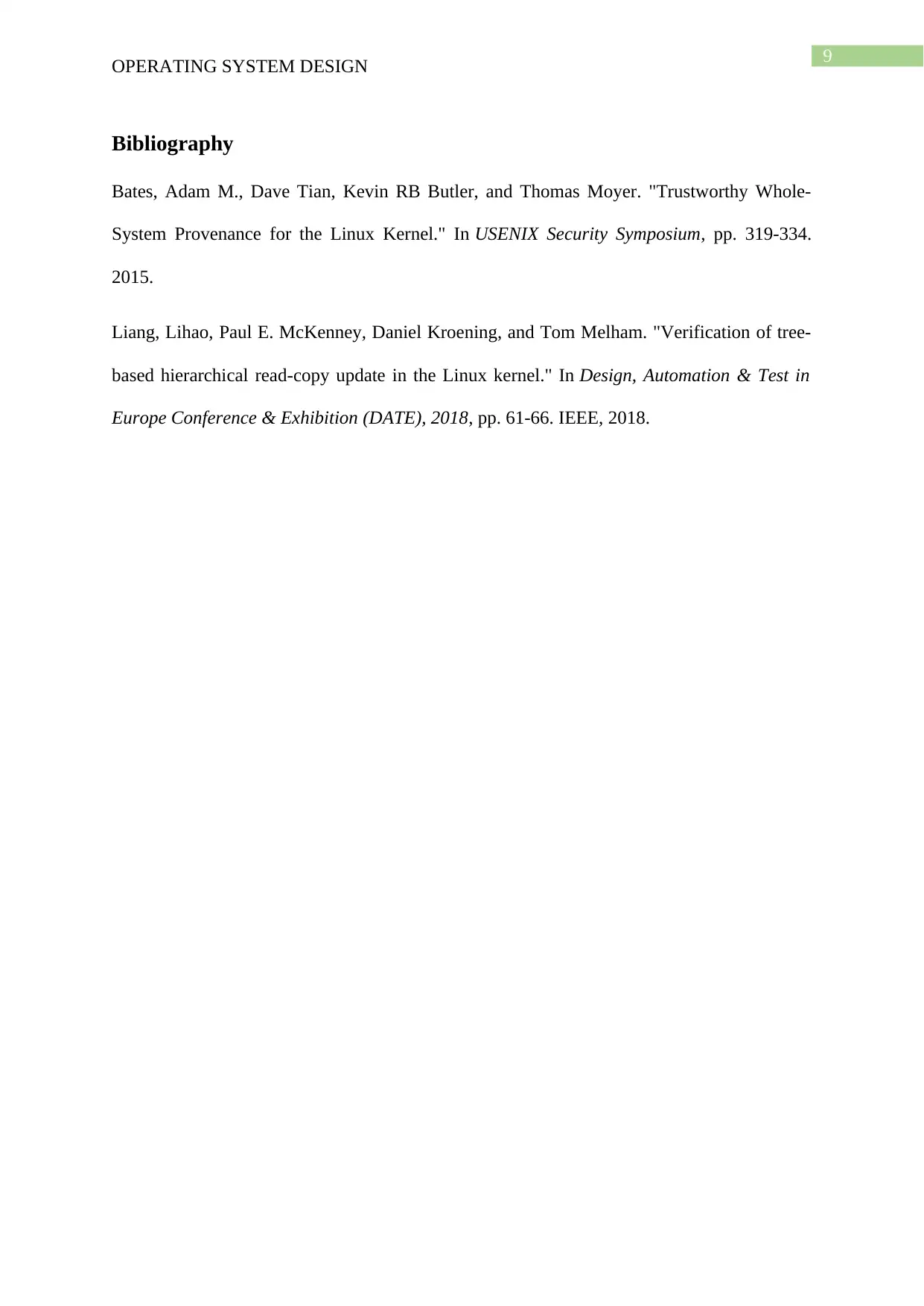
9
OPERATING SYSTEM DESIGN
Bibliography
Bates, Adam M., Dave Tian, Kevin RB Butler, and Thomas Moyer. "Trustworthy Whole-
System Provenance for the Linux Kernel." In USENIX Security Symposium, pp. 319-334.
2015.
Liang, Lihao, Paul E. McKenney, Daniel Kroening, and Tom Melham. "Verification of tree-
based hierarchical read-copy update in the Linux kernel." In Design, Automation & Test in
Europe Conference & Exhibition (DATE), 2018, pp. 61-66. IEEE, 2018.
OPERATING SYSTEM DESIGN
Bibliography
Bates, Adam M., Dave Tian, Kevin RB Butler, and Thomas Moyer. "Trustworthy Whole-
System Provenance for the Linux Kernel." In USENIX Security Symposium, pp. 319-334.
2015.
Liang, Lihao, Paul E. McKenney, Daniel Kroening, and Tom Melham. "Verification of tree-
based hierarchical read-copy update in the Linux kernel." In Design, Automation & Test in
Europe Conference & Exhibition (DATE), 2018, pp. 61-66. IEEE, 2018.
1 out of 10

Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.